CSS 342 DATA S TR U CTURES ALGO
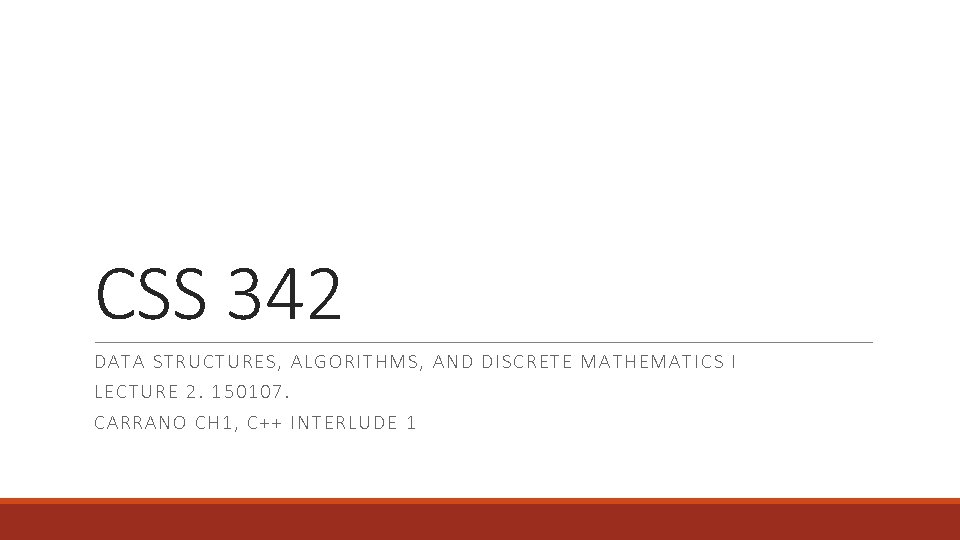
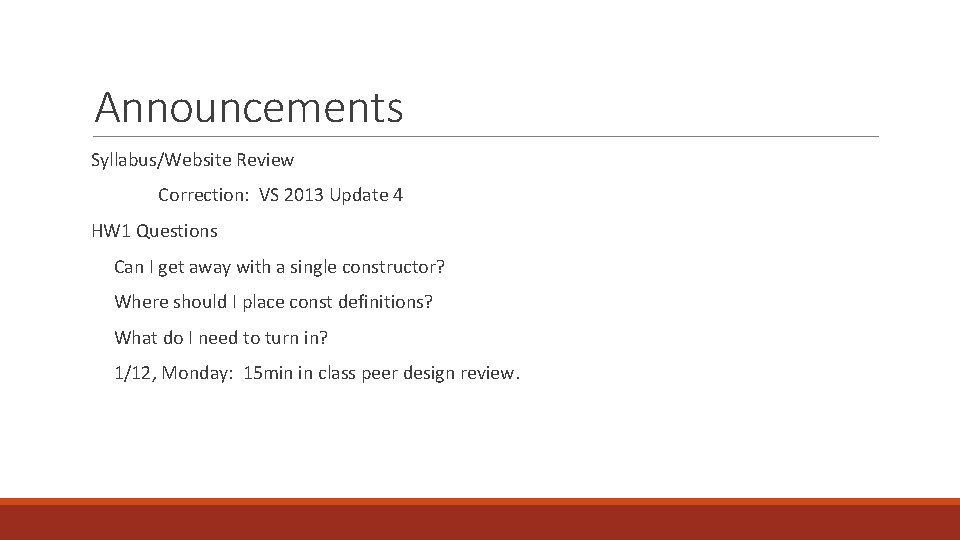
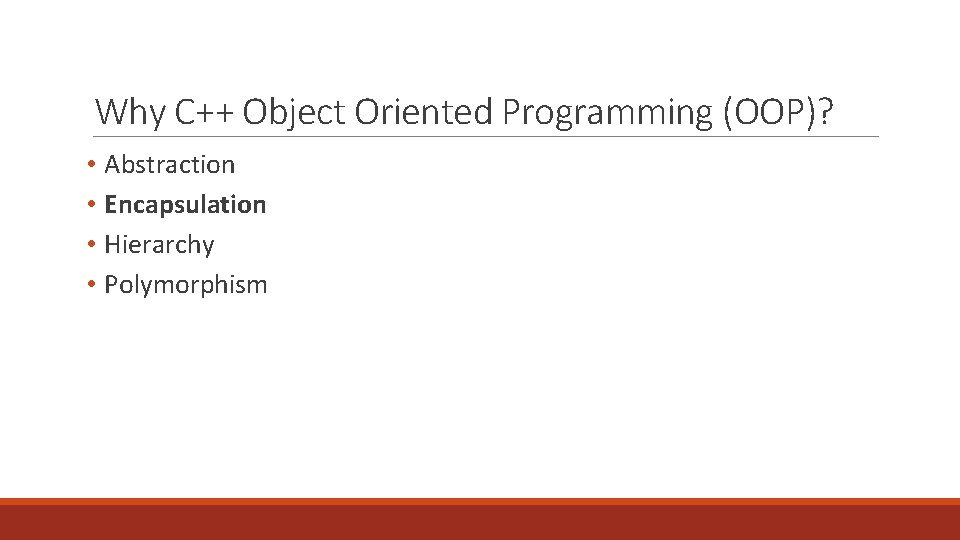
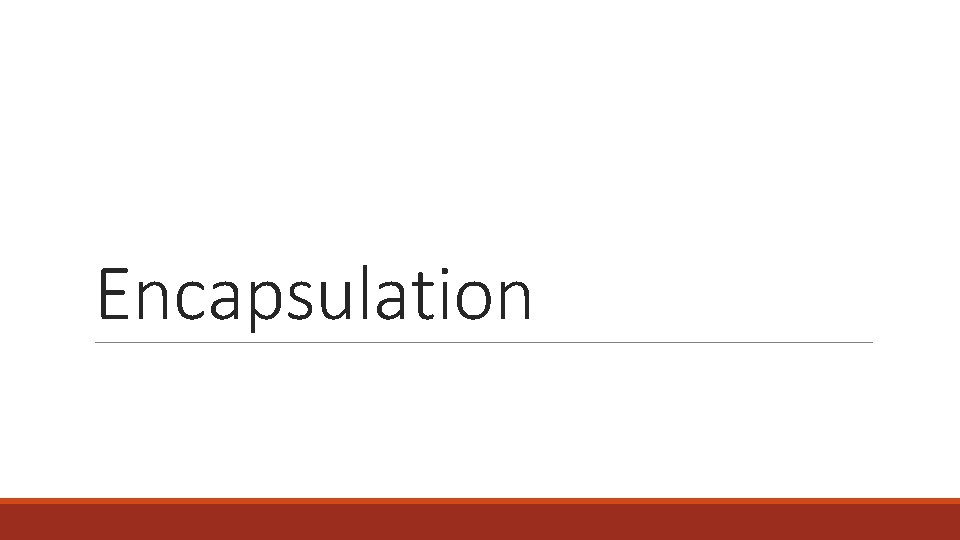
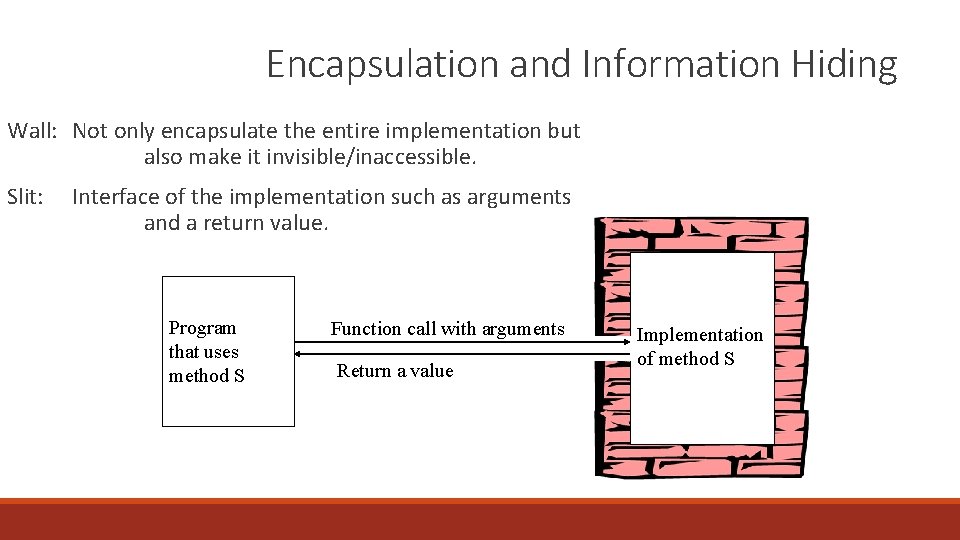
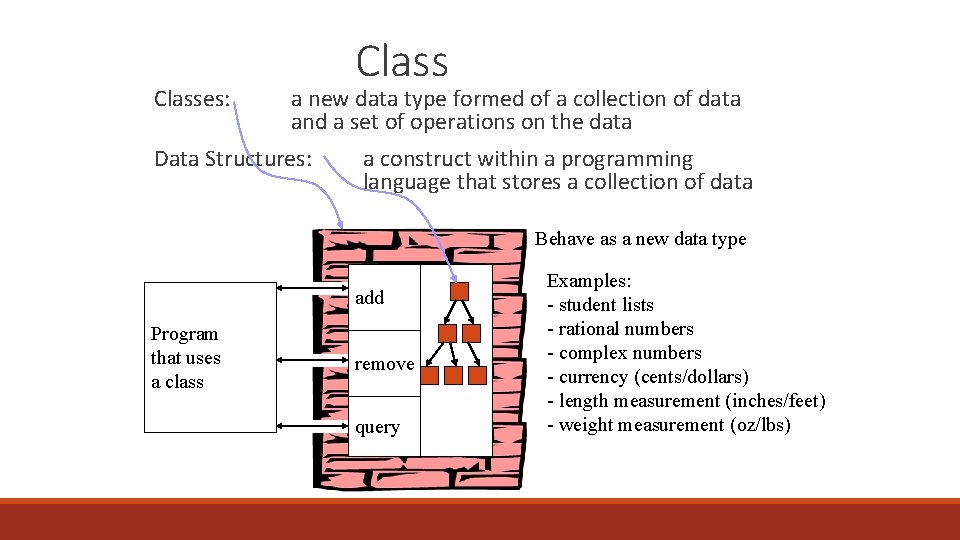
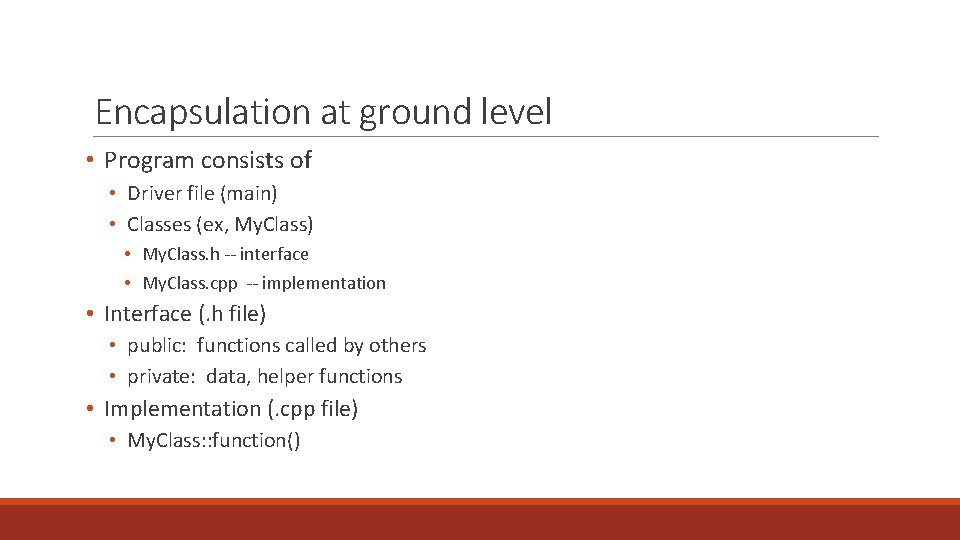
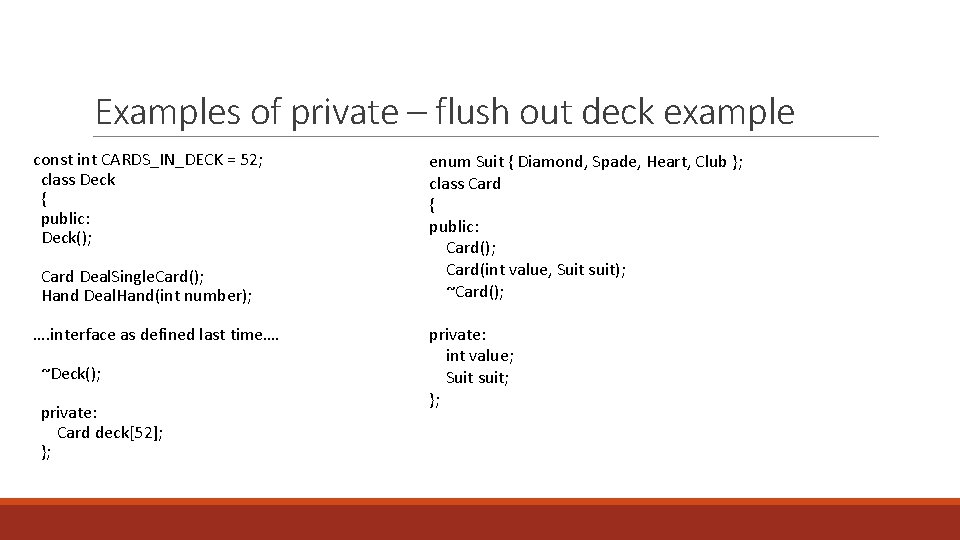
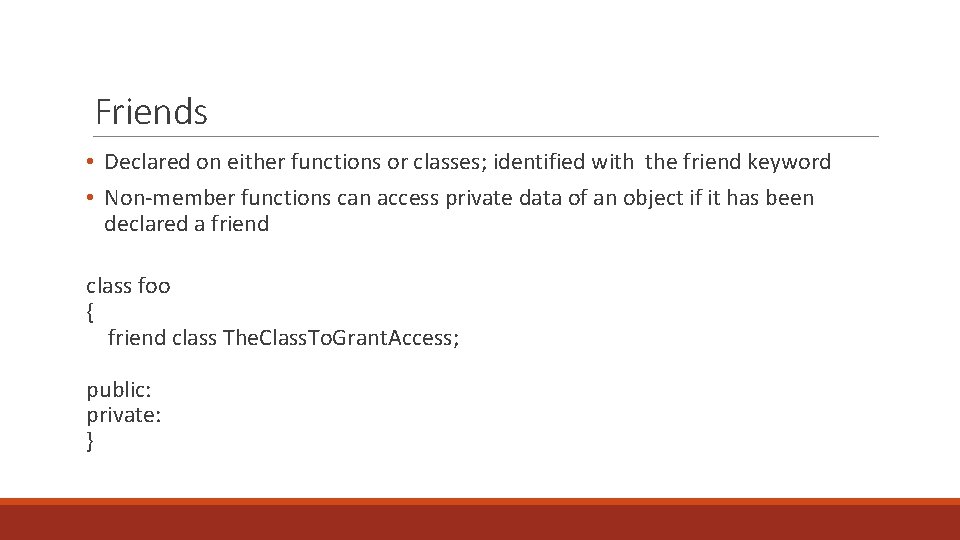
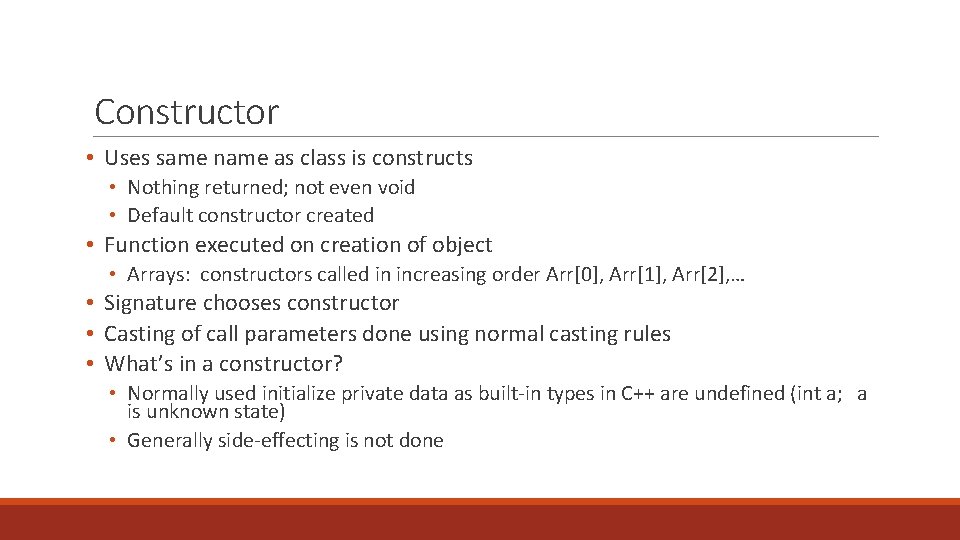
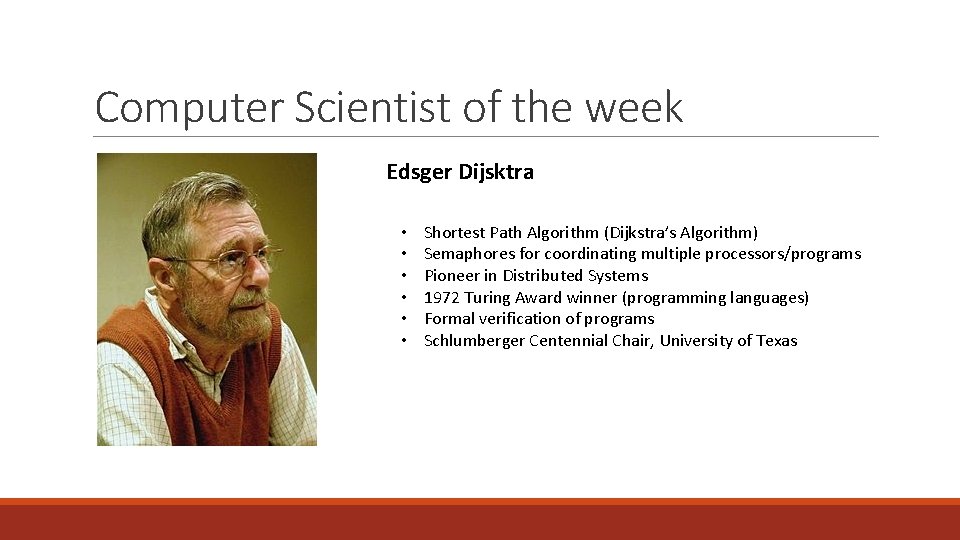
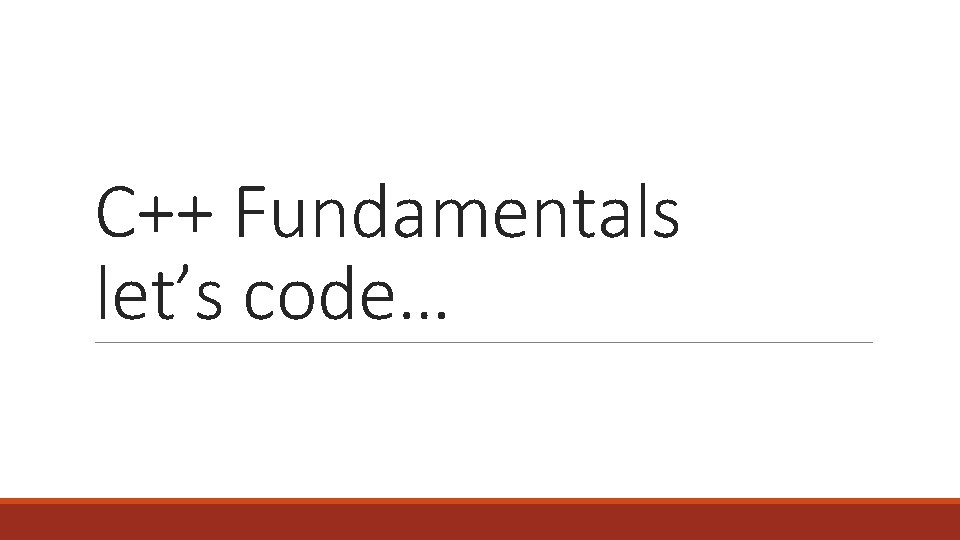
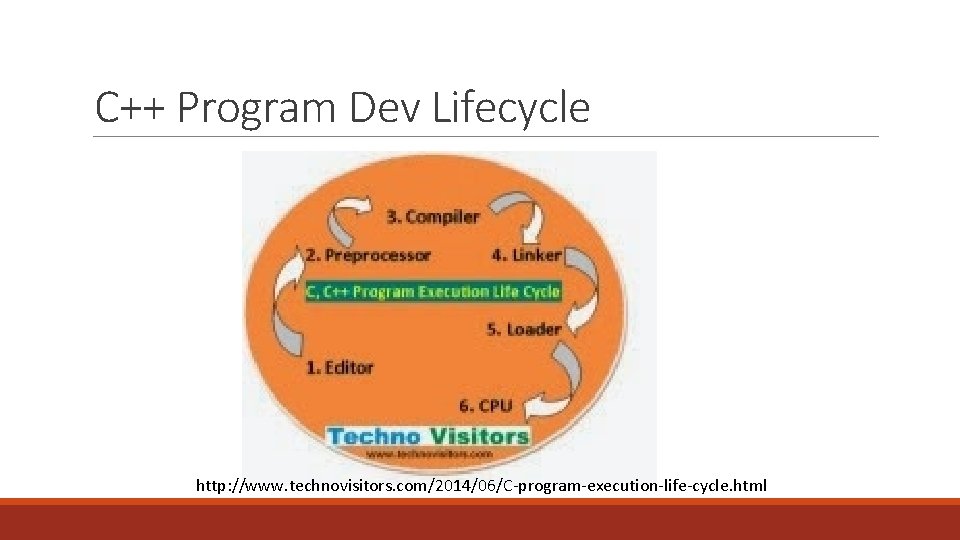
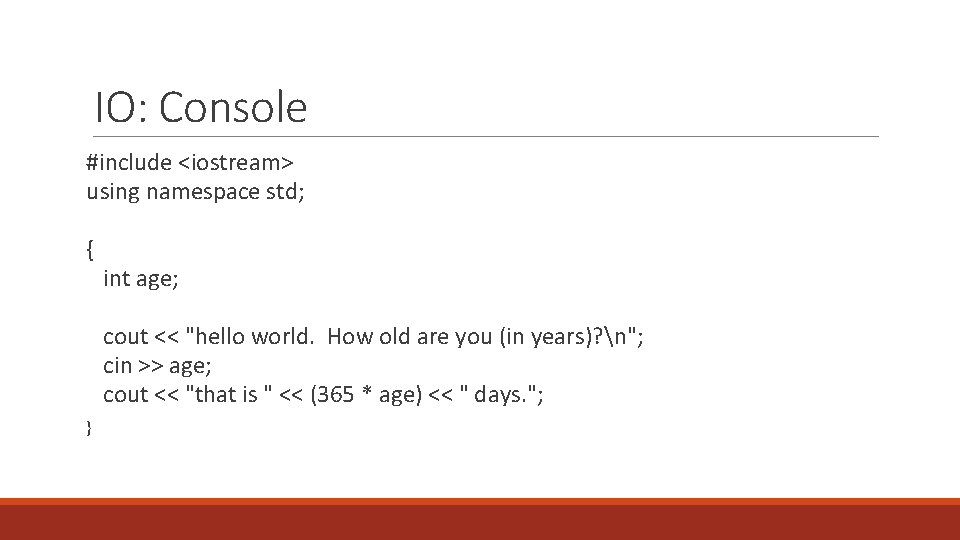
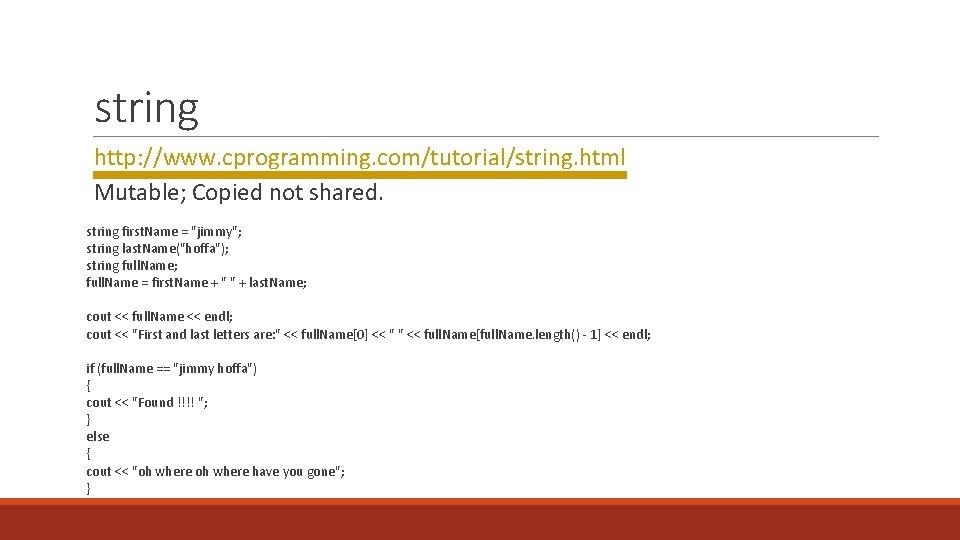
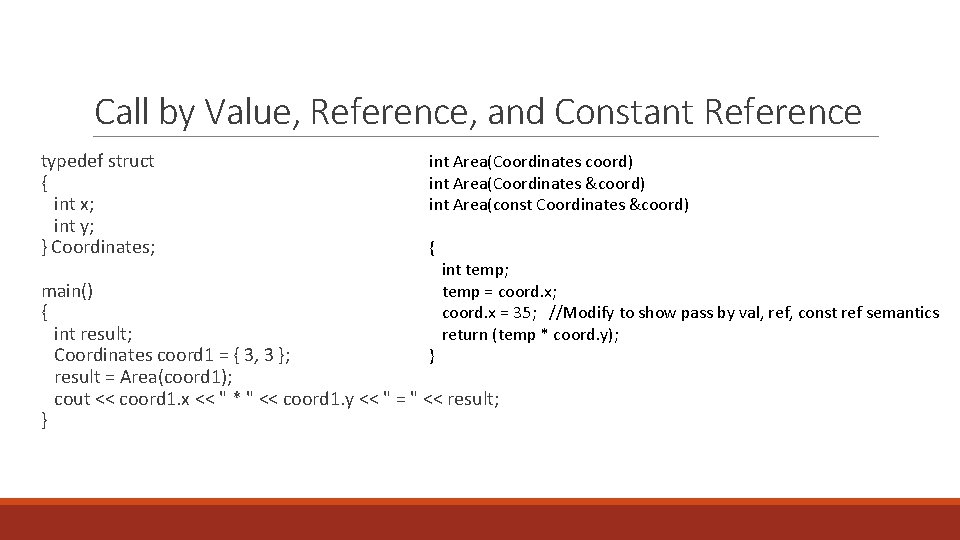
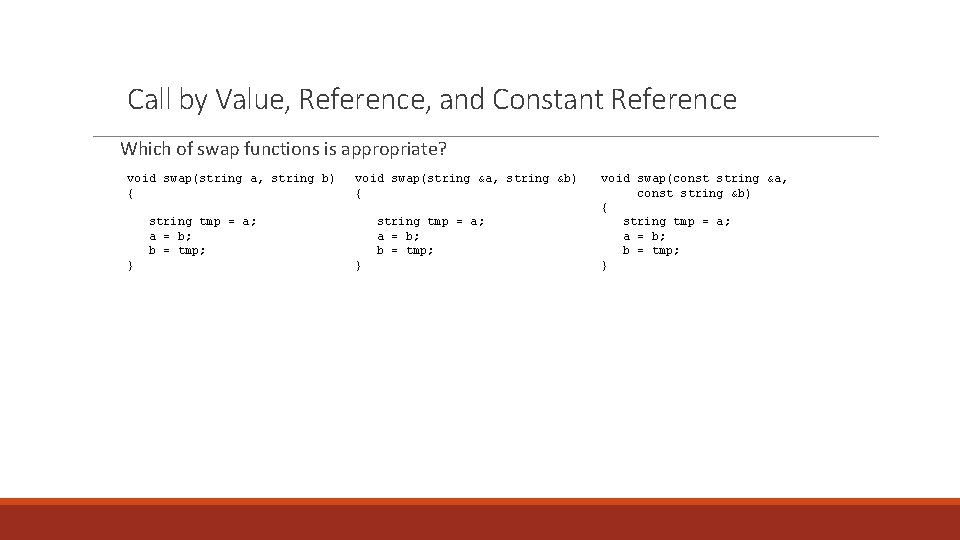
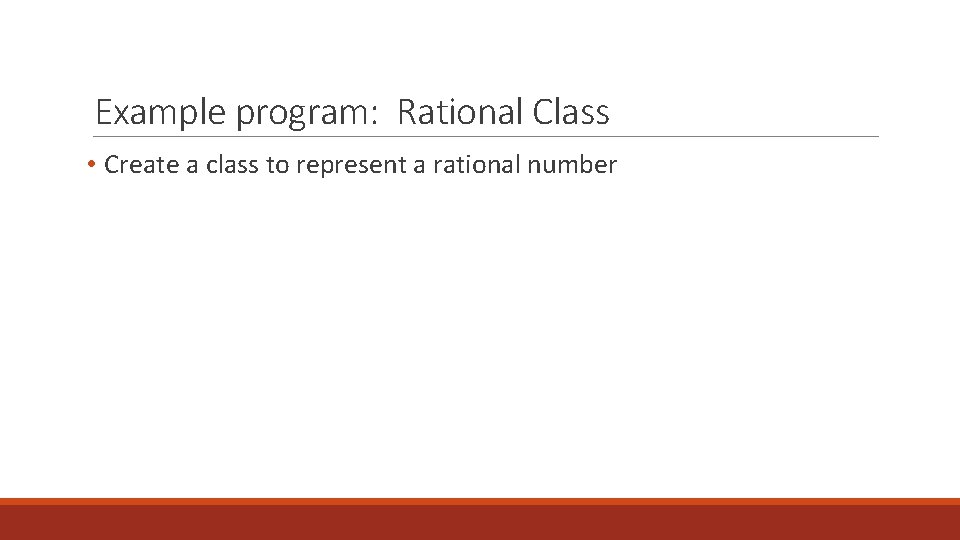
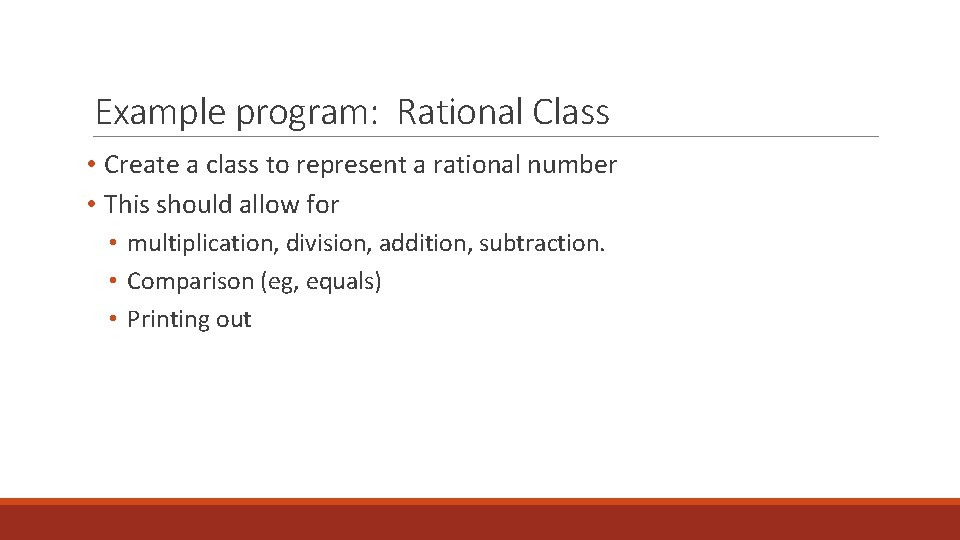
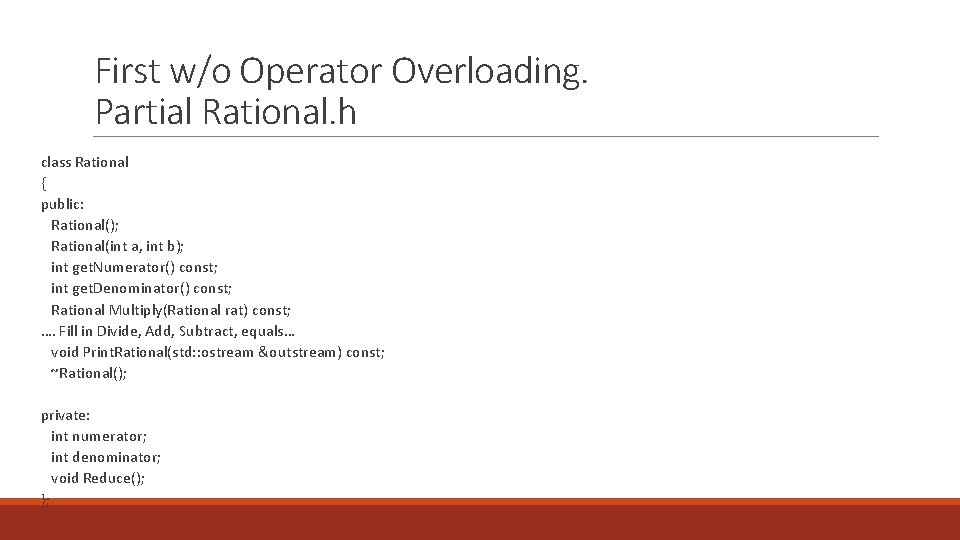
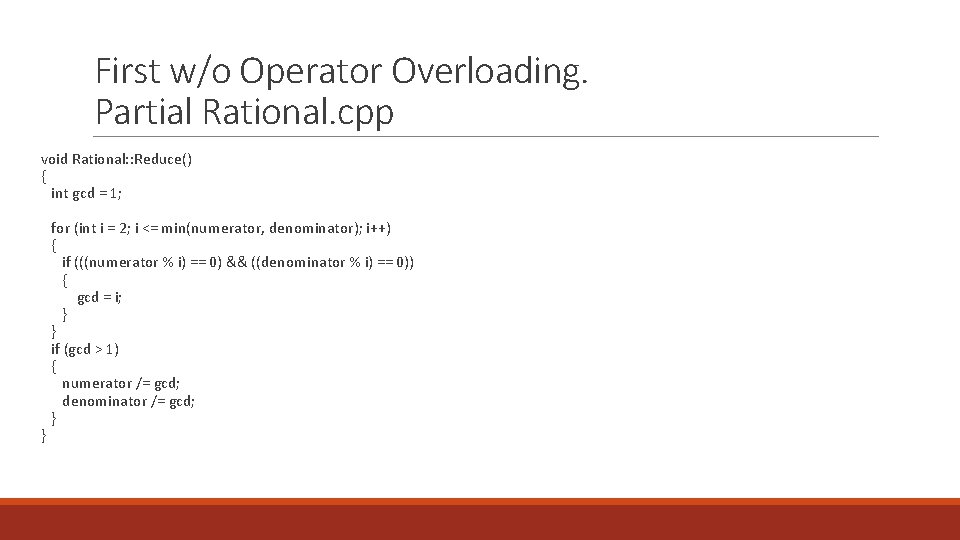
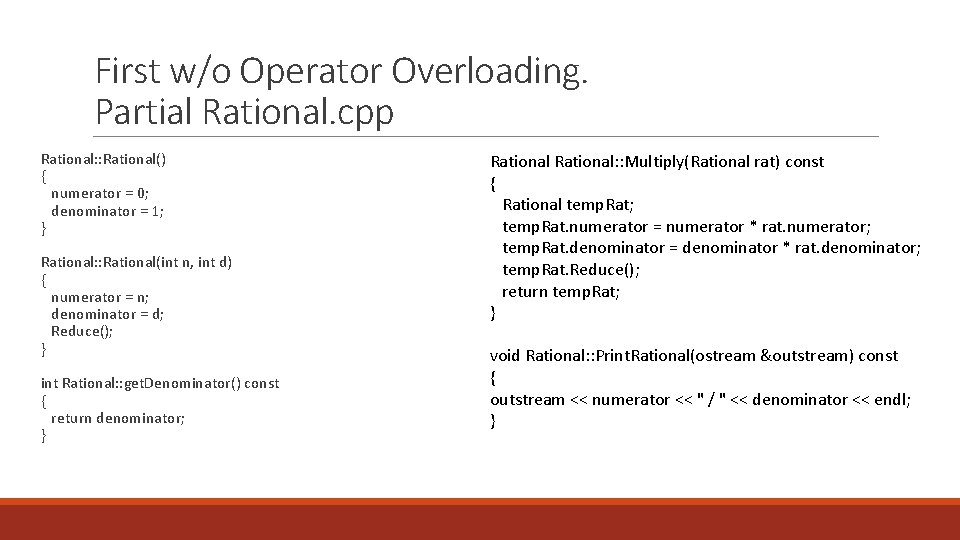
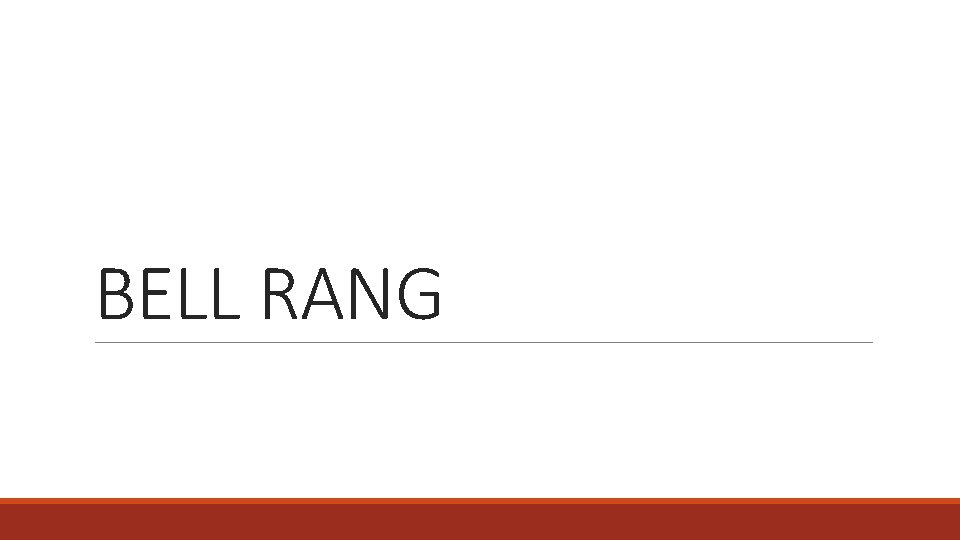
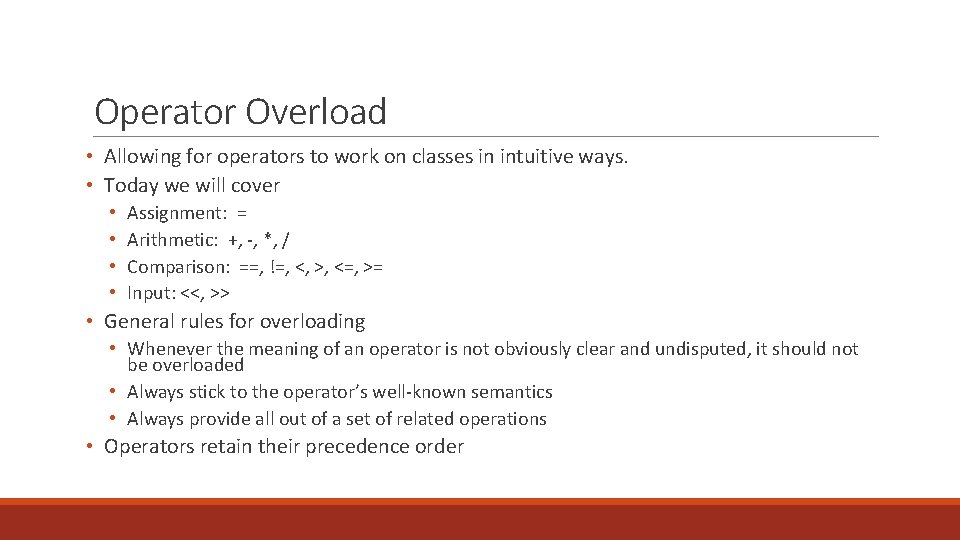
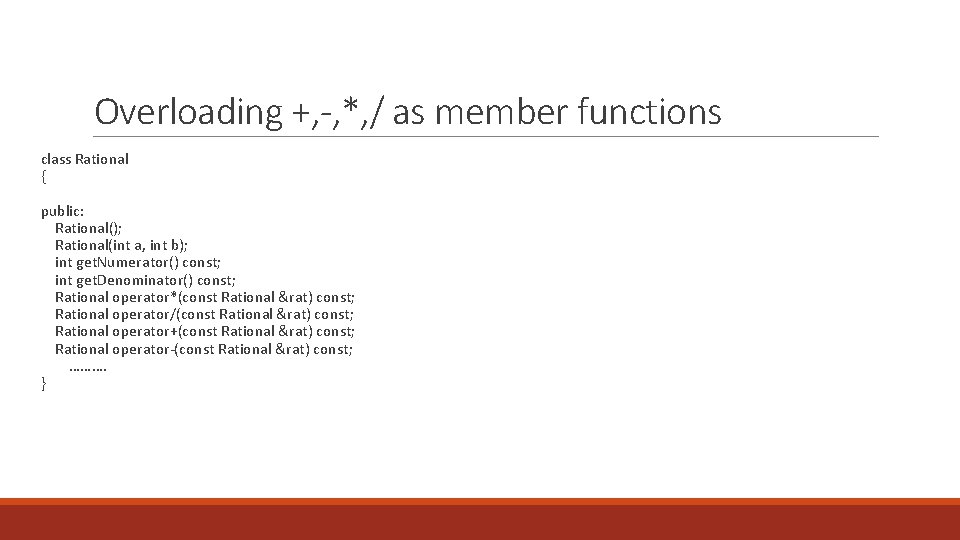
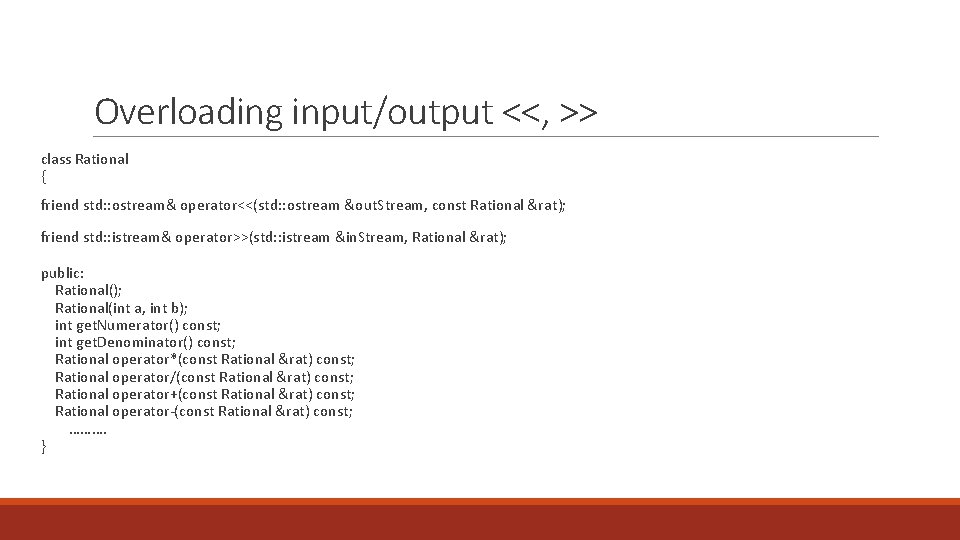
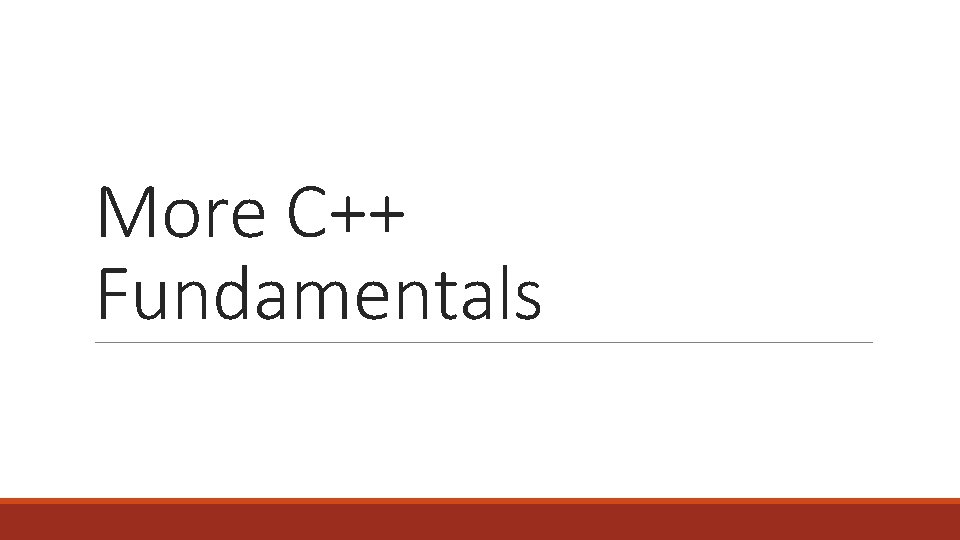
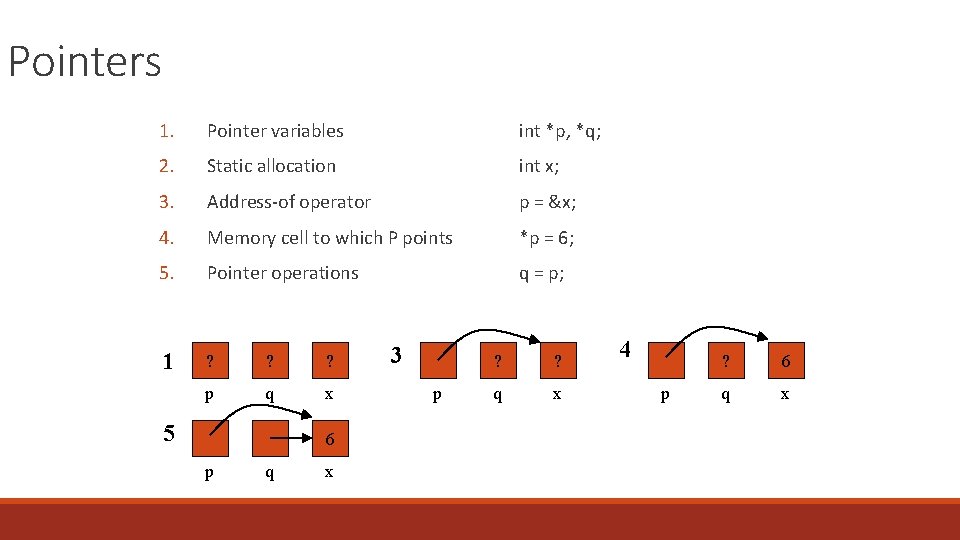
- Slides: 28
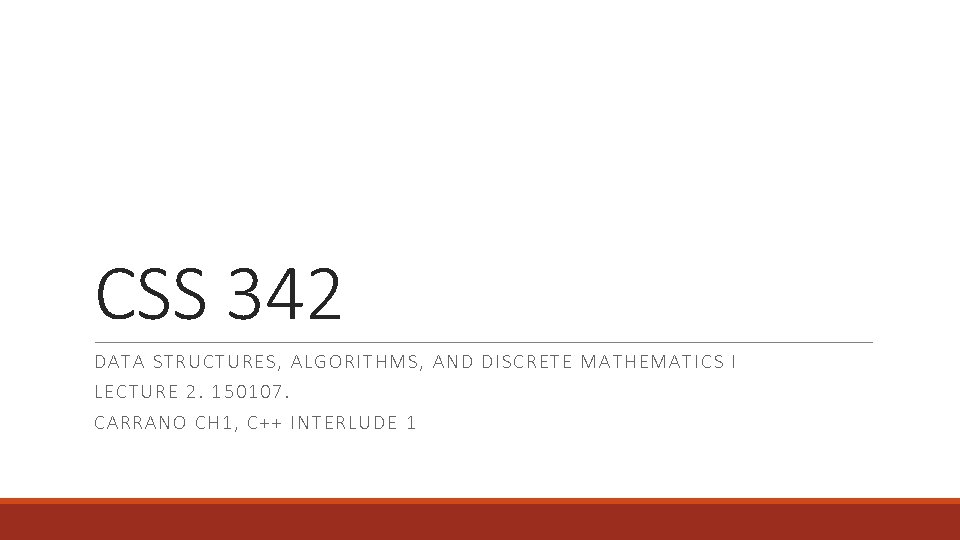
CSS 342 DATA S TR U CTURES, ALGO RITHMS , AND DISCRETE MATHEMATICS I LECTU RE 2. 150107. CARR AN O C H 1, C++ INTER LUDE 1
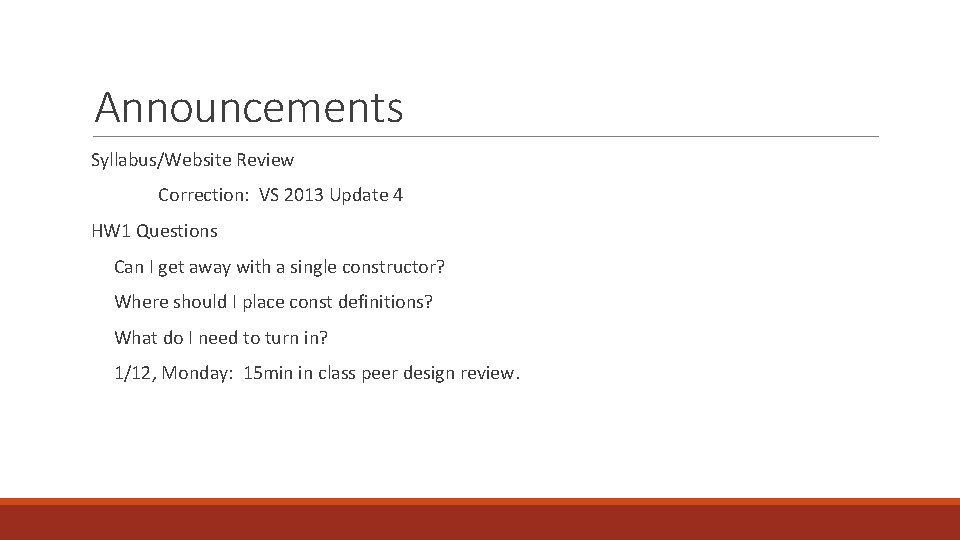
Announcements Syllabus/Website Review Correction: VS 2013 Update 4 HW 1 Questions Can I get away with a single constructor? Where should I place const definitions? What do I need to turn in? 1/12, Monday: 15 min in class peer design review.
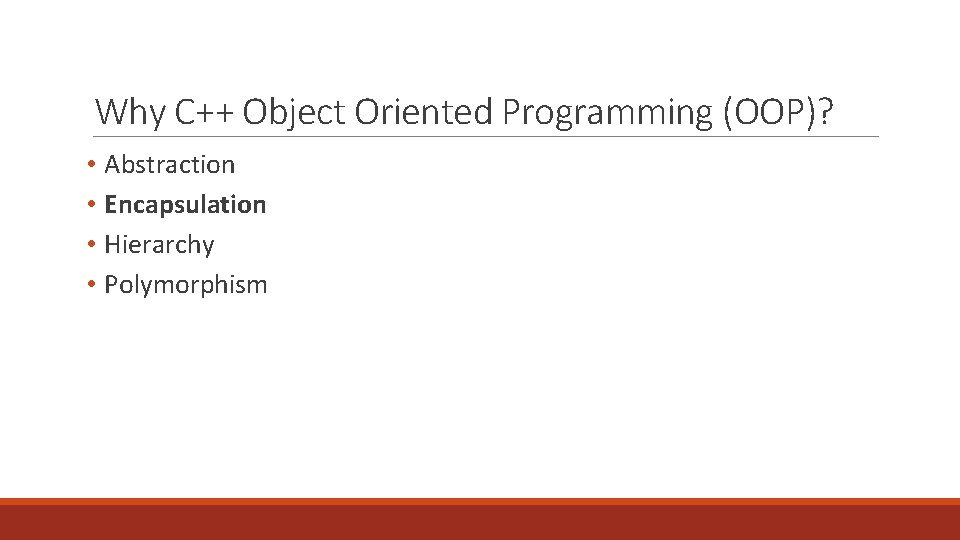
Why C++ Object Oriented Programming (OOP)? • Abstraction • Encapsulation • Hierarchy • Polymorphism
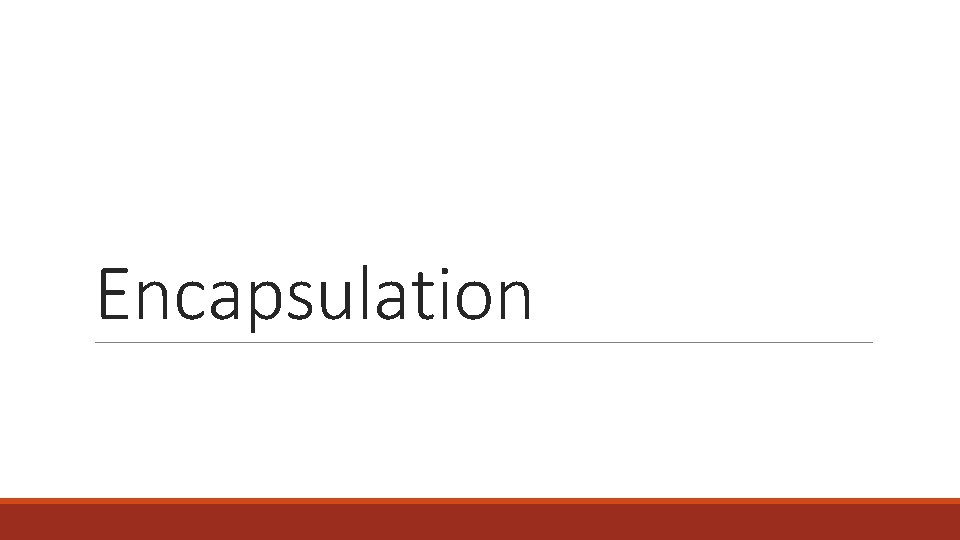
Encapsulation
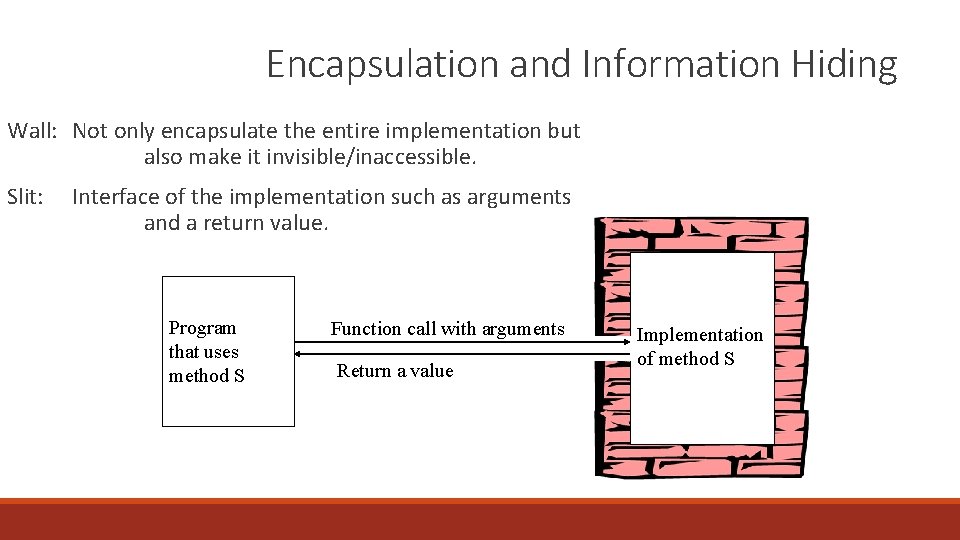
Encapsulation and Information Hiding Wall: Not only encapsulate the entire implementation but also make it invisible/inaccessible. Slit: Interface of the implementation such as arguments and a return value. Program that uses method S Function call with arguments Return a value Implementation of method S
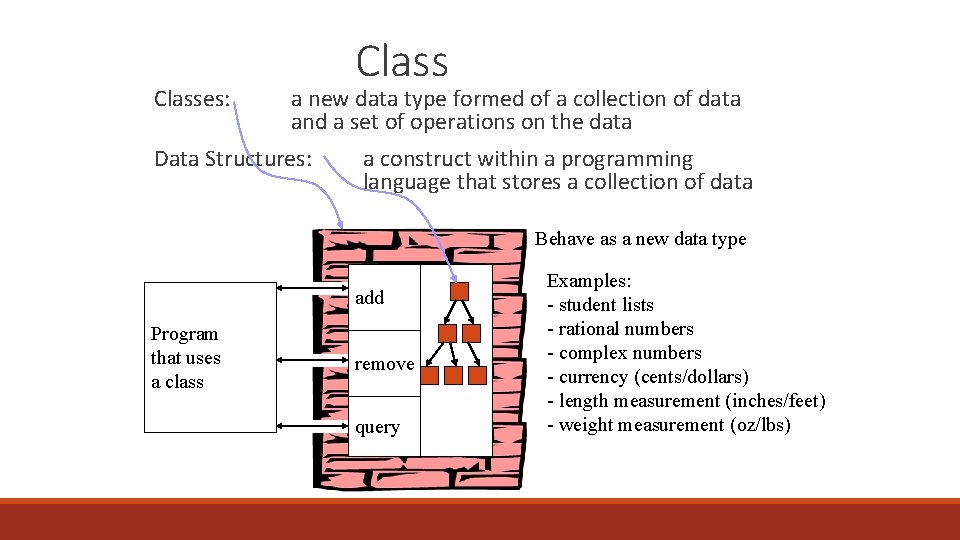
Classes: Class a new data type formed of a collection of data and a set of operations on the data Data Structures: a construct within a programming language that stores a collection of data Behave as a new data type add Program that uses a class remove query Examples: - student lists - rational numbers - complex numbers - currency (cents/dollars) - length measurement (inches/feet) - weight measurement (oz/lbs)
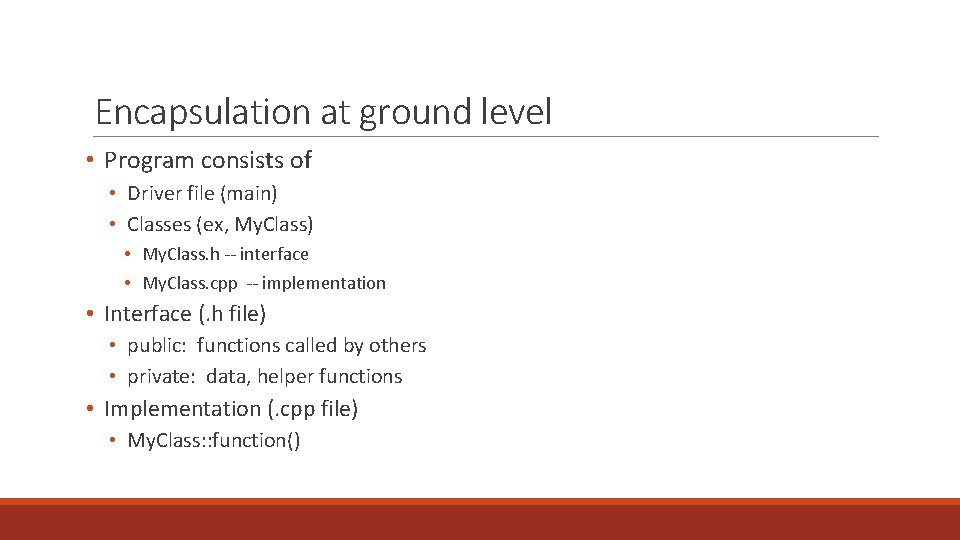
Encapsulation at ground level • Program consists of • Driver file (main) • Classes (ex, My. Class) • My. Class. h -- interface • My. Class. cpp -- implementation • Interface (. h file) • public: functions called by others • private: data, helper functions • Implementation (. cpp file) • My. Class: : function()
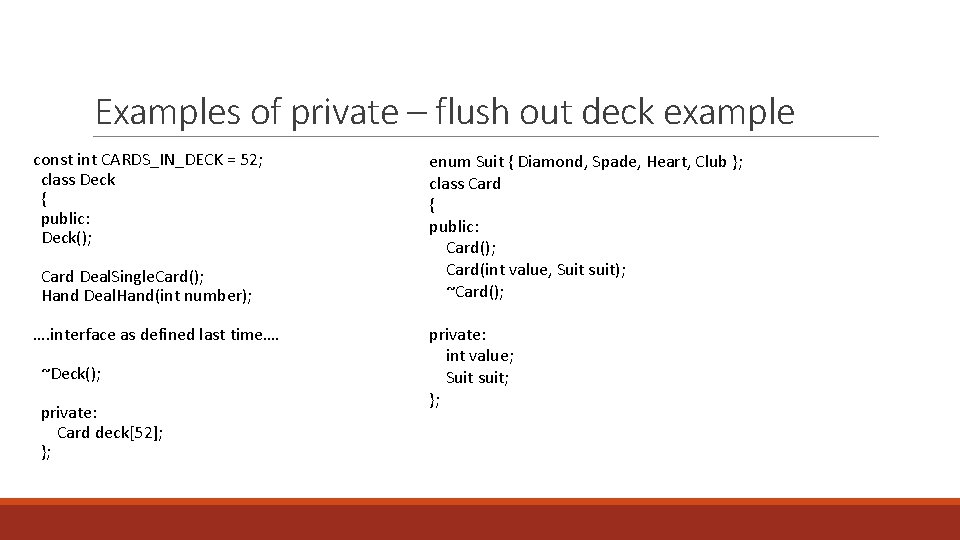
Examples of private – flush out deck example const int CARDS_IN_DECK = 52; class Deck { public: Deck(); Card Deal. Single. Card(); Hand Deal. Hand(int number); …. interface as defined last time…. ~Deck(); private: Card deck[52]; }; enum Suit { Diamond, Spade, Heart, Club }; class Card { public: Card(); Card(int value, Suit suit); ~Card(); private: int value; Suit suit; };
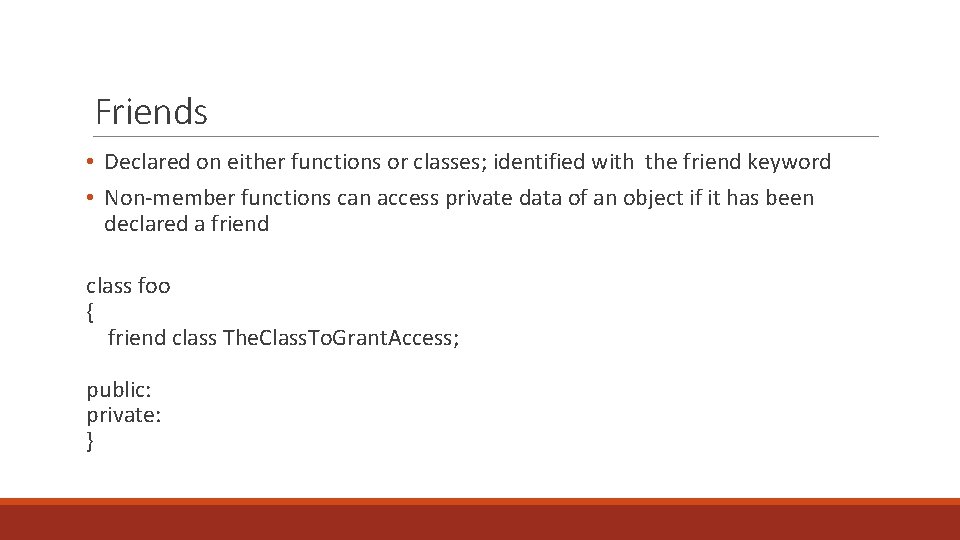
Friends • Declared on either functions or classes; identified with the friend keyword • Non-member functions can access private data of an object if it has been declared a friend class foo { friend class The. Class. To. Grant. Access; public: private: }
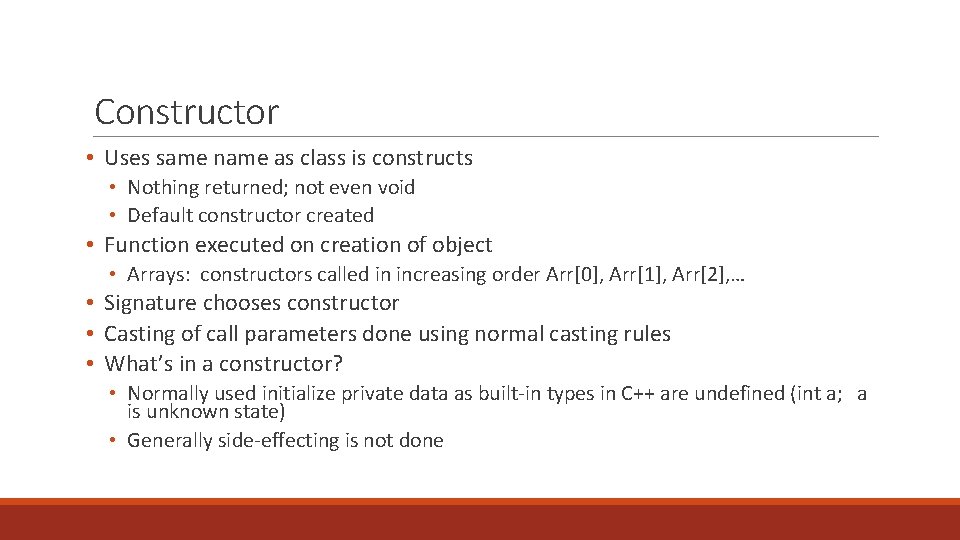
Constructor • Uses same name as class is constructs • Nothing returned; not even void • Default constructor created • Function executed on creation of object • Arrays: constructors called in increasing order Arr[0], Arr[1], Arr[2], … • Signature chooses constructor • Casting of call parameters done using normal casting rules • What’s in a constructor? • Normally used initialize private data as built-in types in C++ are undefined (int a; a is unknown state) • Generally side-effecting is not done
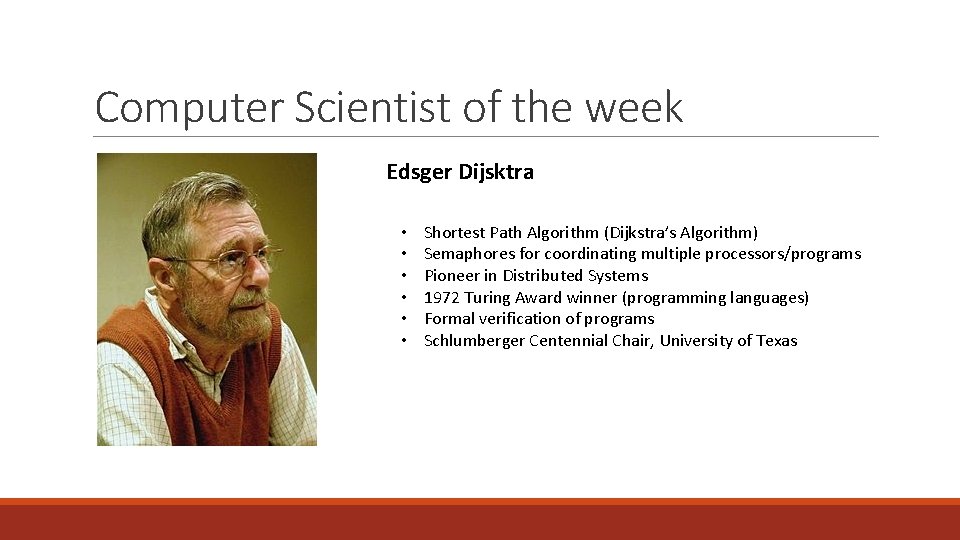
Computer Scientist of the week Edsger Dijsktra • • • Shortest Path Algorithm (Dijkstra’s Algorithm) Semaphores for coordinating multiple processors/programs Pioneer in Distributed Systems 1972 Turing Award winner (programming languages) Formal verification of programs Schlumberger Centennial Chair, University of Texas
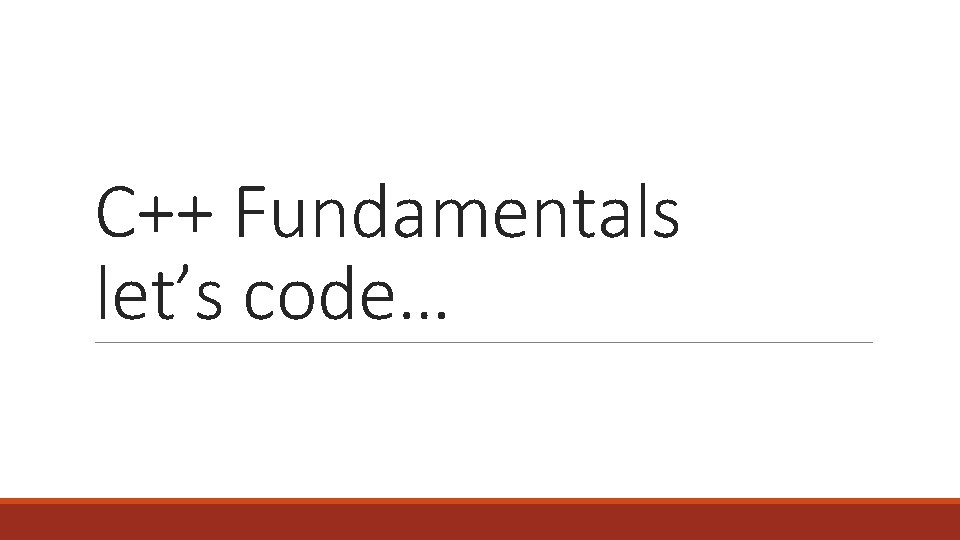
C++ Fundamentals let’s code…
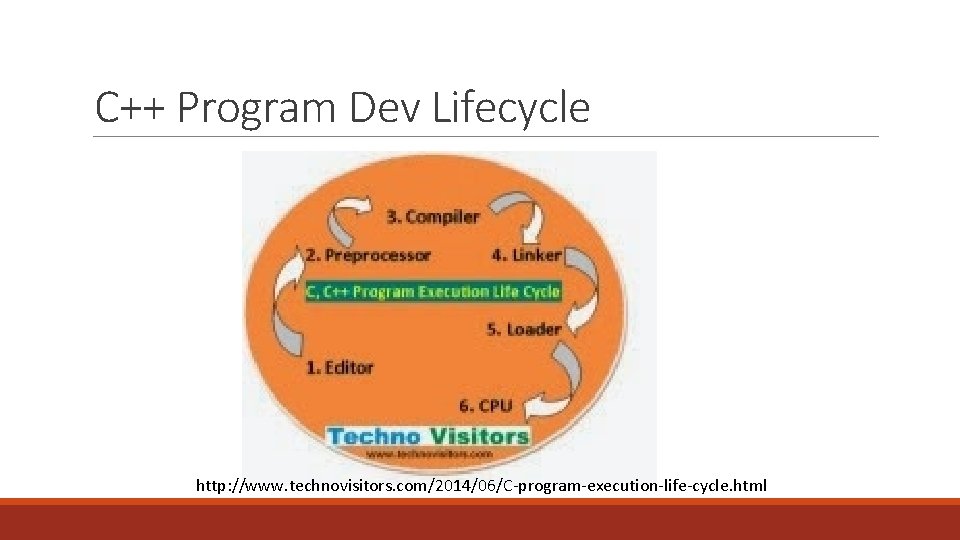
C++ Program Dev Lifecycle http: //www. technovisitors. com/2014/06/C-program-execution-life-cycle. html
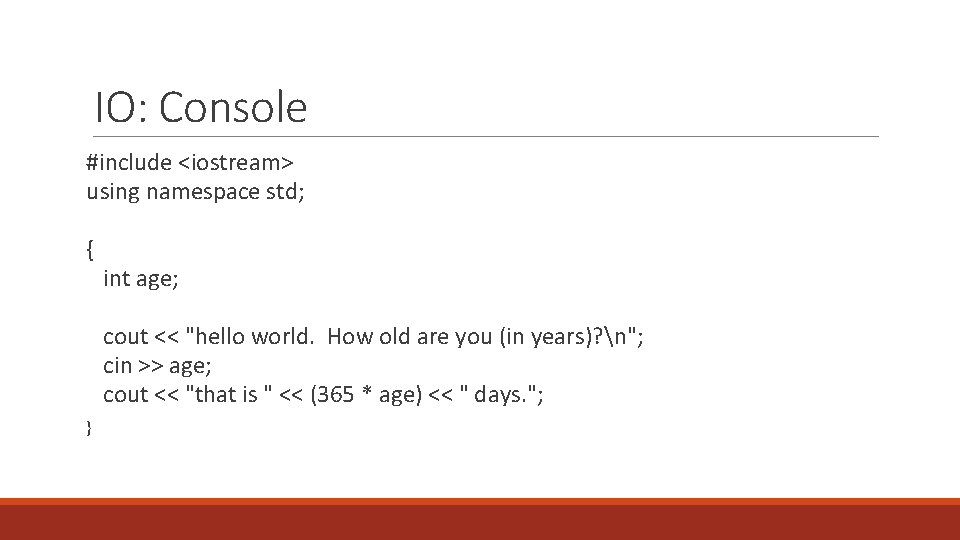
IO: Console #include <iostream> using namespace std; { int age; cout << "hello world. How old are you (in years)? n"; cin >> age; cout << "that is " << (365 * age) << " days. "; }
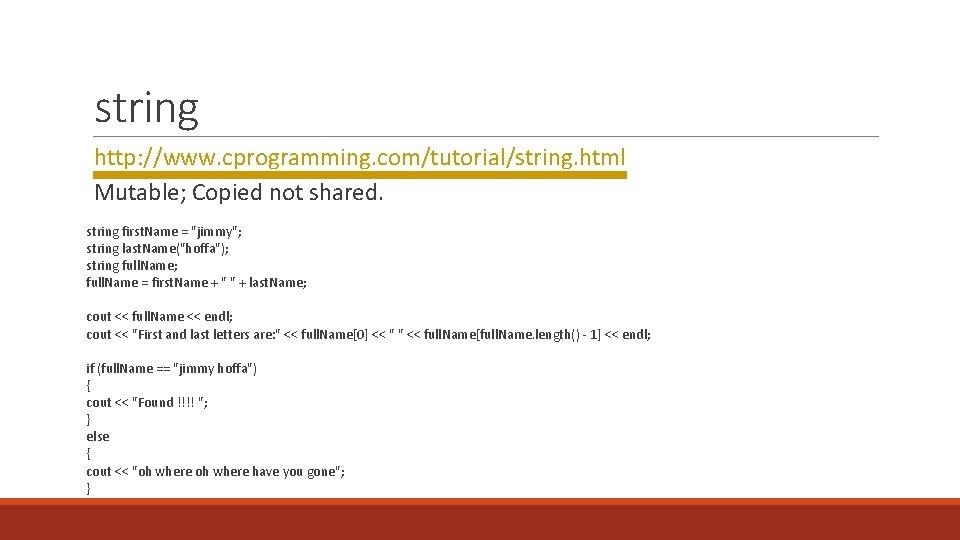
string http: //www. cprogramming. com/tutorial/string. html Mutable; Copied not shared. string first. Name = "jimmy"; string last. Name("hoffa"); string full. Name; full. Name = first. Name + " " + last. Name; cout << full. Name << endl; cout << "First and last letters are: " << full. Name[0] << " " << full. Name[full. Name. length() - 1] << endl; if (full. Name == "jimmy hoffa") { cout << "Found !!!! "; } else { cout << "oh where have you gone"; }
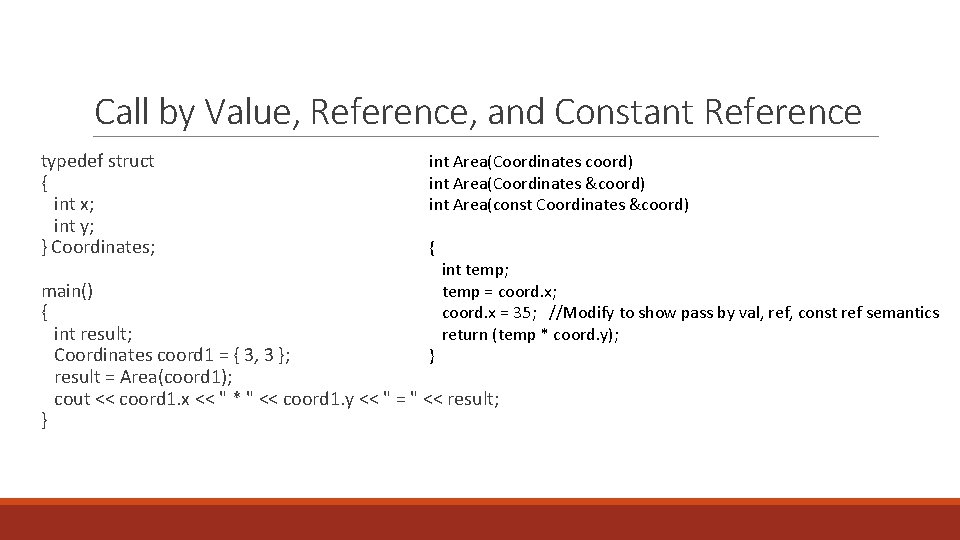
Call by Value, Reference, and Constant Reference typedef struct { int x; int y; } Coordinates; int Area(Coordinates coord) int Area(Coordinates &coord) int Area(const Coordinates &coord) { int temp; temp = coord. x; coord. x = 35; //Modify to show pass by val, ref, const ref semantics return (temp * coord. y); main() { int result; Coordinates coord 1 = { 3, 3 }; } result = Area(coord 1); cout << coord 1. x << " * " << coord 1. y << " = " << result; }
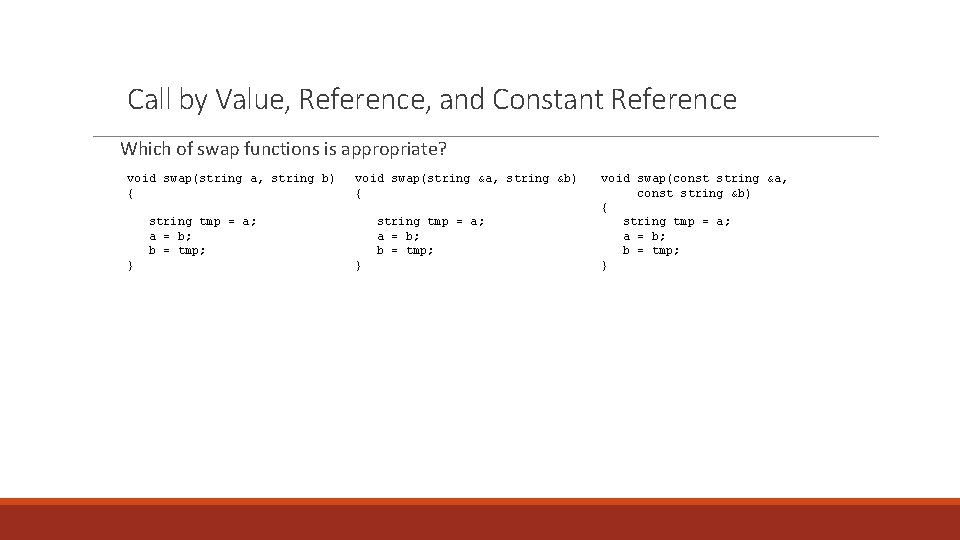
Call by Value, Reference, and Constant Reference Which of swap functions is appropriate? void swap(string a, string b) { void swap(string &a, string &b) { string tmp = a; a = b; b = tmp; } void swap(const string &a, const string &b) { string tmp = a; a = b; b = tmp; }
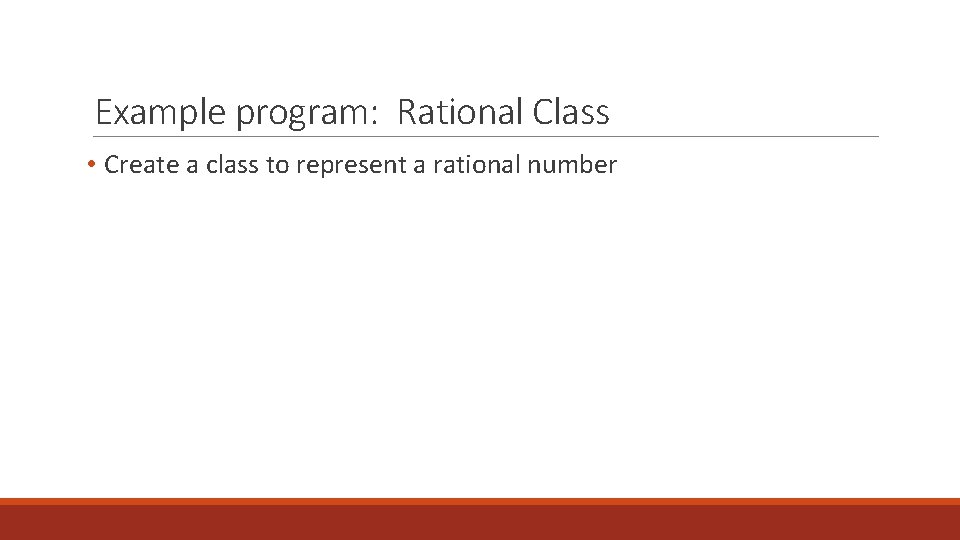
Example program: Rational Class • Create a class to represent a rational number
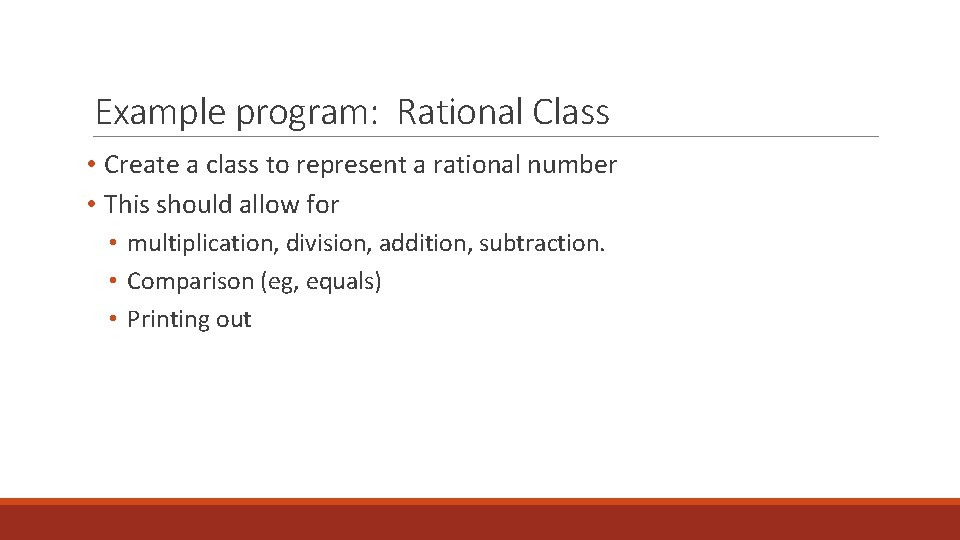
Example program: Rational Class • Create a class to represent a rational number • This should allow for • multiplication, division, addition, subtraction. • Comparison (eg, equals) • Printing out
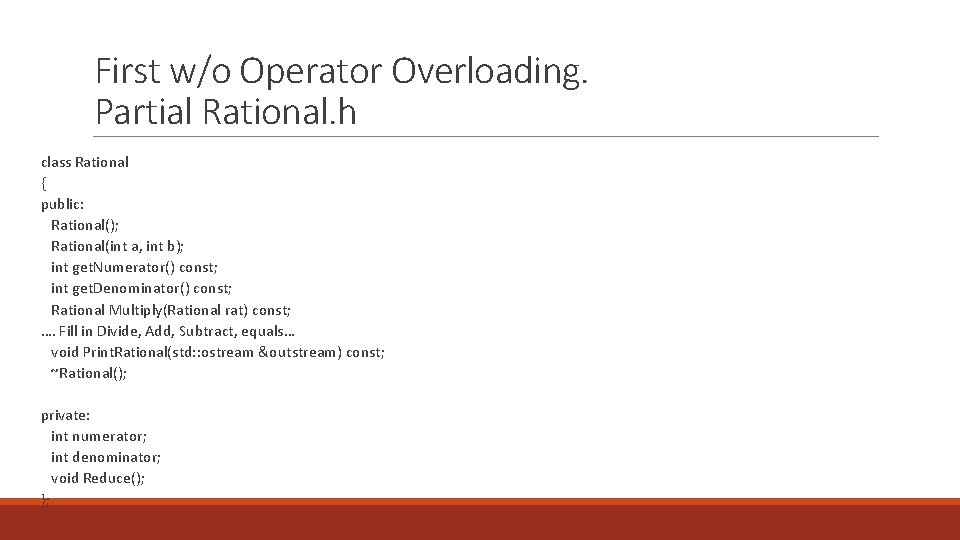
First w/o Operator Overloading. Partial Rational. h class Rational { public: Rational(); Rational(int a, int b); int get. Numerator() const; int get. Denominator() const; Rational Multiply(Rational rat) const; …. Fill in Divide, Add, Subtract, equals… void Print. Rational(std: : ostream &outstream) const; ~Rational(); private: int numerator; int denominator; void Reduce(); };
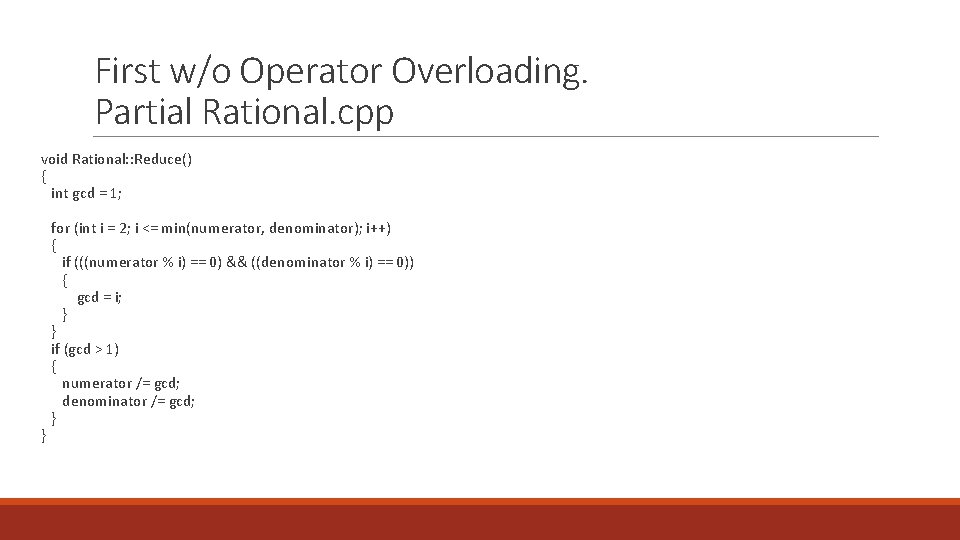
First w/o Operator Overloading. Partial Rational. cpp void Rational: : Reduce() { int gcd = 1; } for (int i = 2; i <= min(numerator, denominator); i++) { if (((numerator % i) == 0) && ((denominator % i) == 0)) { gcd = i; } } if (gcd > 1) { numerator /= gcd; denominator /= gcd; }
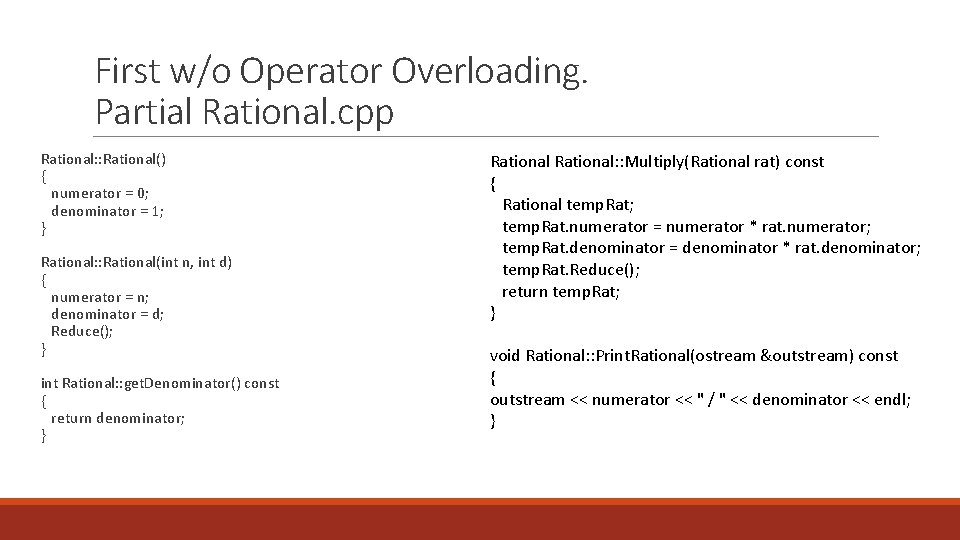
First w/o Operator Overloading. Partial Rational. cpp Rational: : Rational() { numerator = 0; denominator = 1; } Rational: : Rational(int n, int d) { numerator = n; denominator = d; Reduce(); } int Rational: : get. Denominator() const { return denominator; } Rational: : Multiply(Rational rat) const { Rational temp. Rat; temp. Rat. numerator = numerator * rat. numerator; temp. Rat. denominator = denominator * rat. denominator; temp. Rat. Reduce(); return temp. Rat; } void Rational: : Print. Rational(ostream &outstream) const { outstream << numerator << " / " << denominator << endl; }
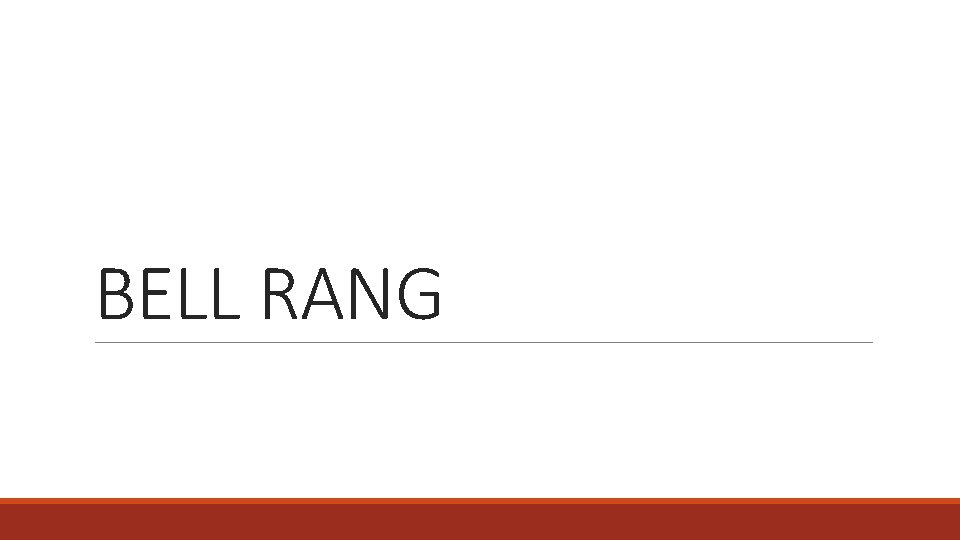
BELL RANG
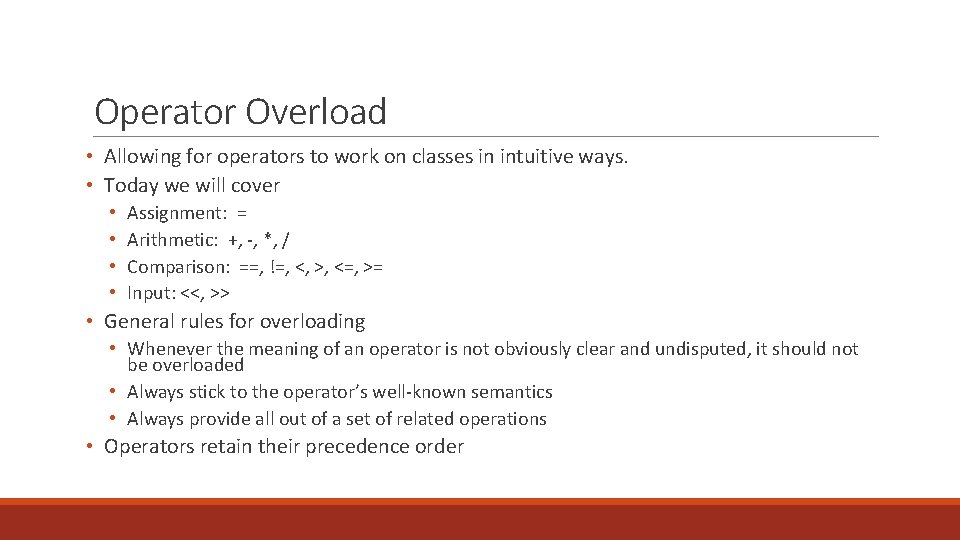
Operator Overload • Allowing for operators to work on classes in intuitive ways. • Today we will cover • • Assignment: = Arithmetic: +, -, *, / Comparison: ==, !=, <, >, <=, >= Input: <<, >> • General rules for overloading • Whenever the meaning of an operator is not obviously clear and undisputed, it should not be overloaded • Always stick to the operator’s well-known semantics • Always provide all out of a set of related operations • Operators retain their precedence order
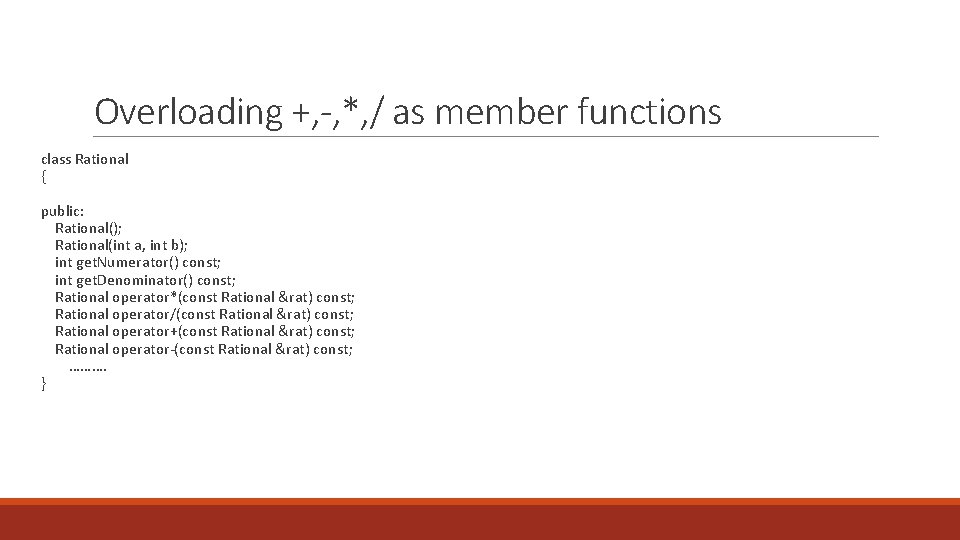
Overloading +, -, *, / as member functions class Rational { public: Rational(); Rational(int a, int b); int get. Numerator() const; int get. Denominator() const; Rational operator*(const Rational &rat) const; Rational operator/(const Rational &rat) const; Rational operator+(const Rational &rat) const; Rational operator-(const Rational &rat) const; ………. }
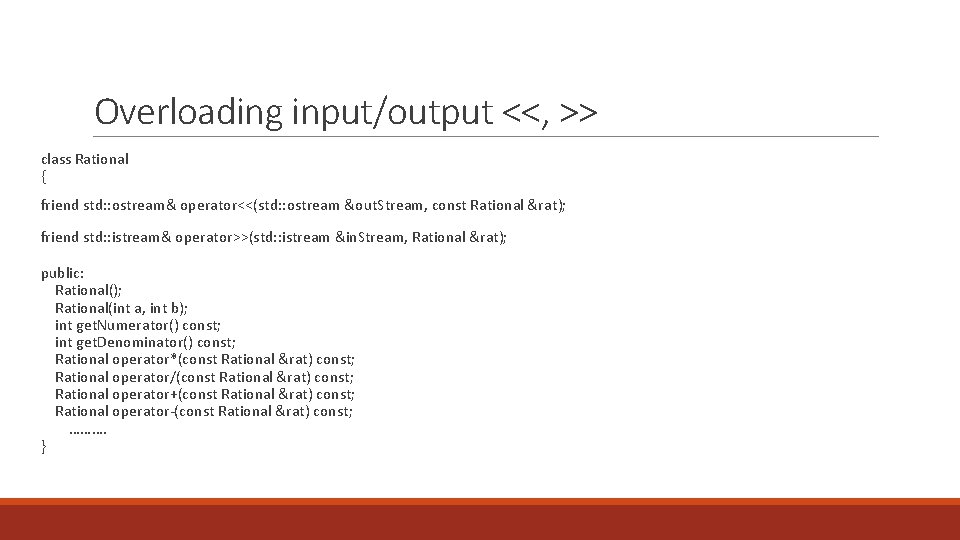
Overloading input/output <<, >> class Rational { friend std: : ostream& operator<<(std: : ostream &out. Stream, const Rational &rat); friend std: : istream& operator>>(std: : istream &in. Stream, Rational &rat); public: Rational(); Rational(int a, int b); int get. Numerator() const; int get. Denominator() const; Rational operator*(const Rational &rat) const; Rational operator/(const Rational &rat) const; Rational operator+(const Rational &rat) const; Rational operator-(const Rational &rat) const; ………. }
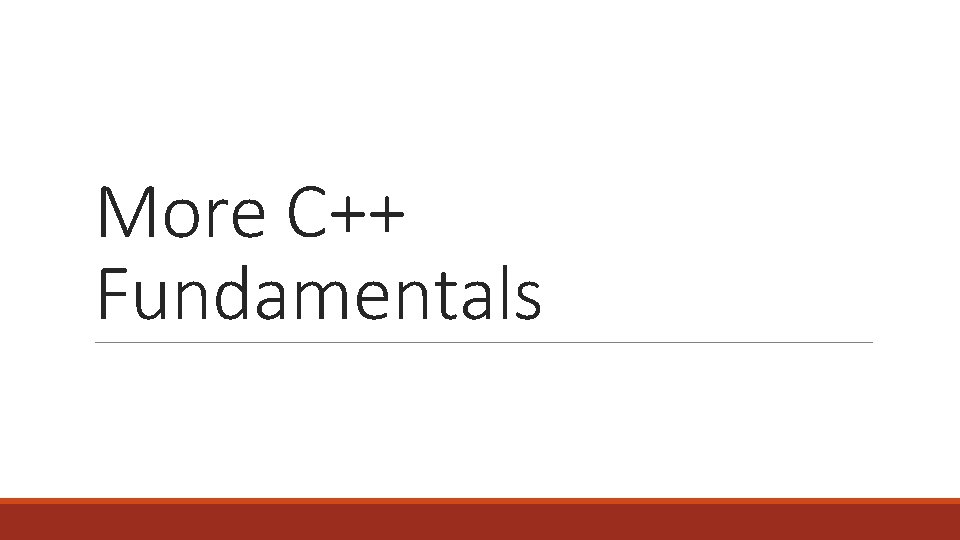
More C++ Fundamentals
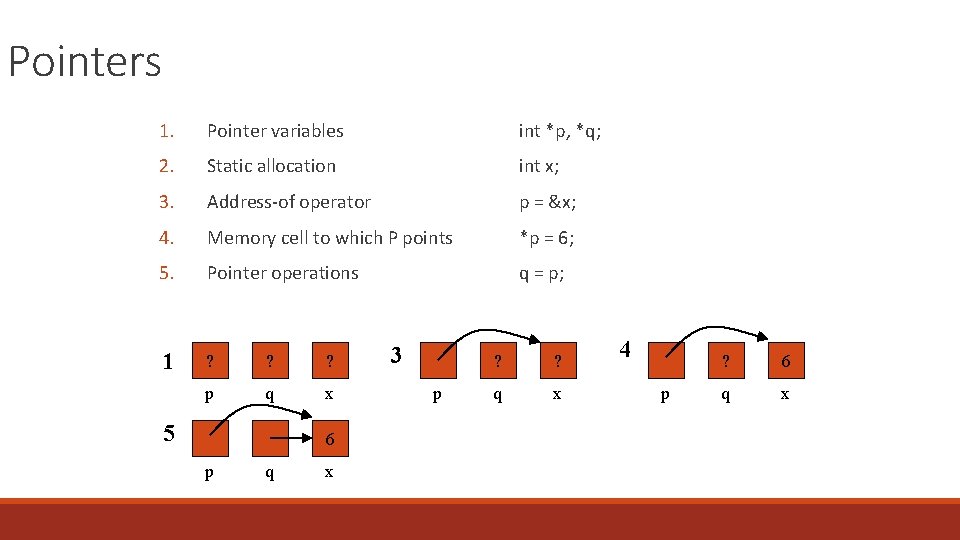
Pointers 1. Pointer variables int *p, *q; 2. Static allocation int x; 3. Address-of operator p = &x; 4. Memory cell to which P points *p = 6; 5. Pointer operations q = p; 1 ? ? ? p q x 5 6 p q x 3 p ? ? q x 4 p ? 6 q x