CS 240 Data Structures Monday July 30 th
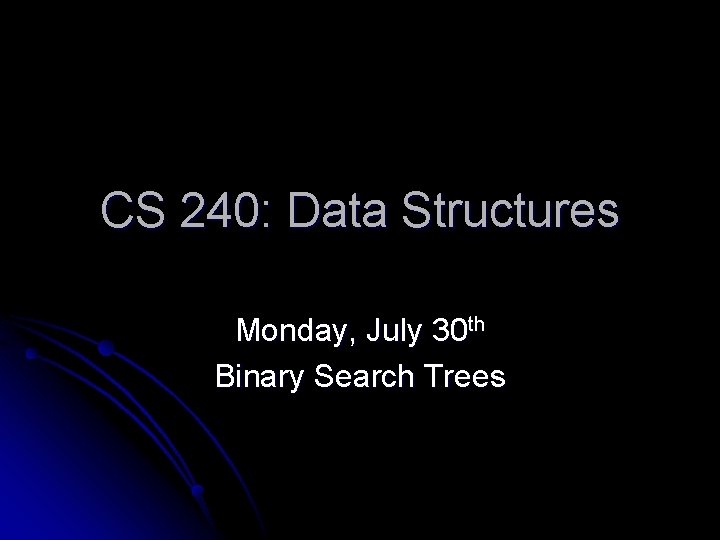
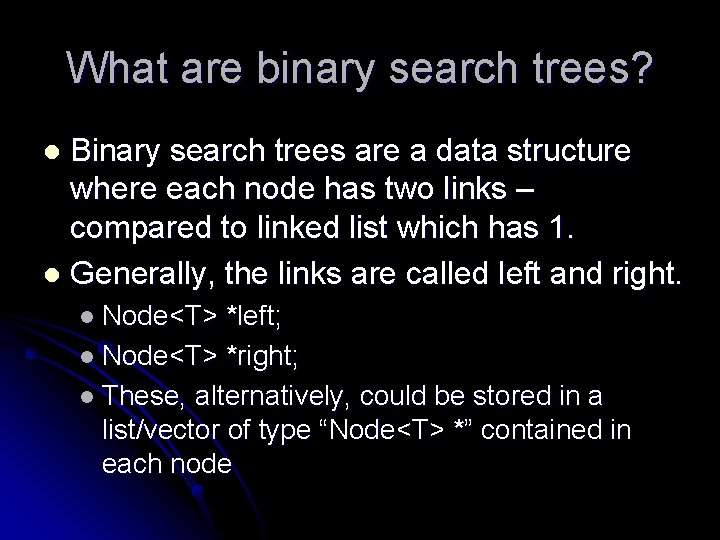
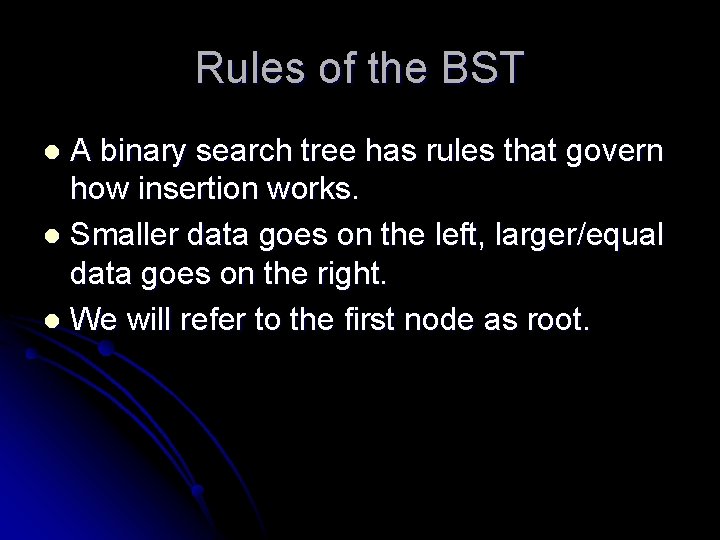
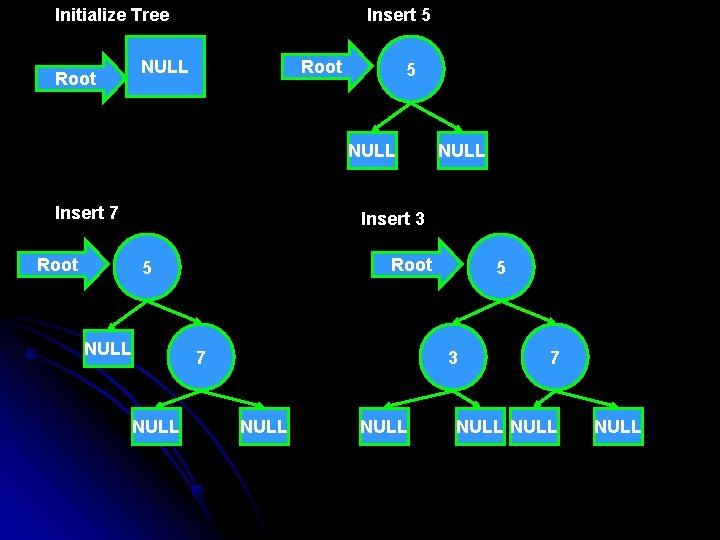
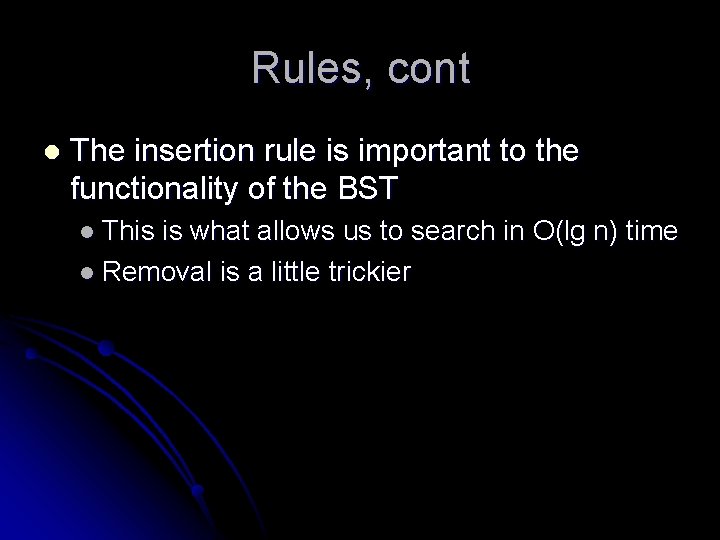
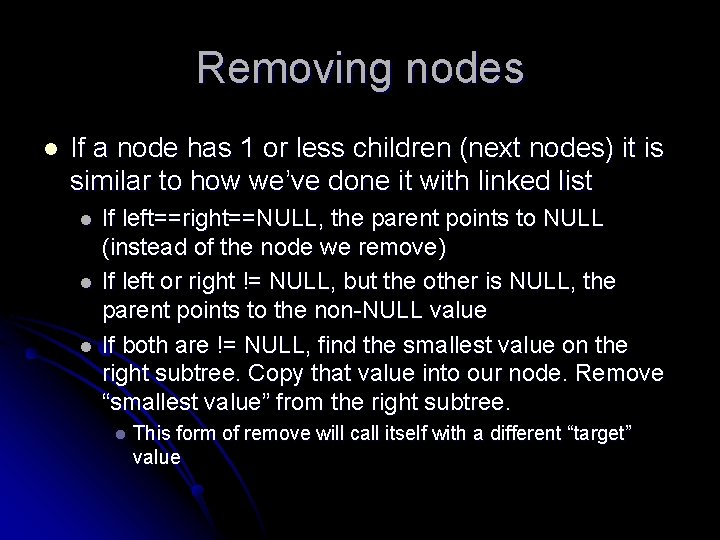
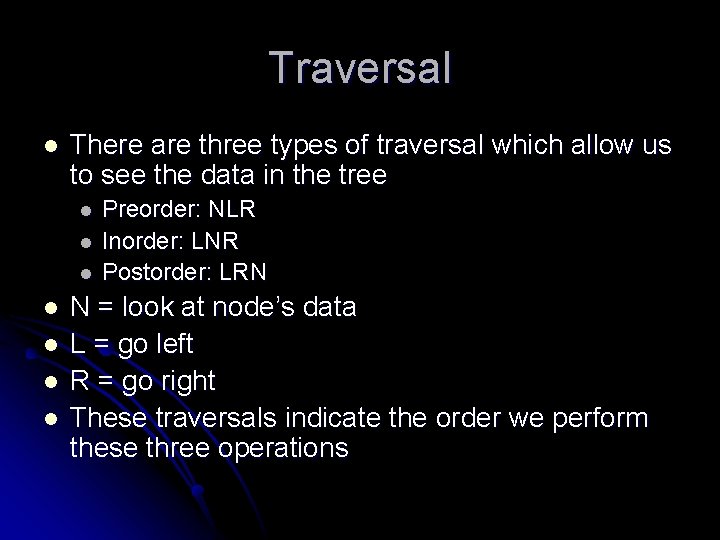
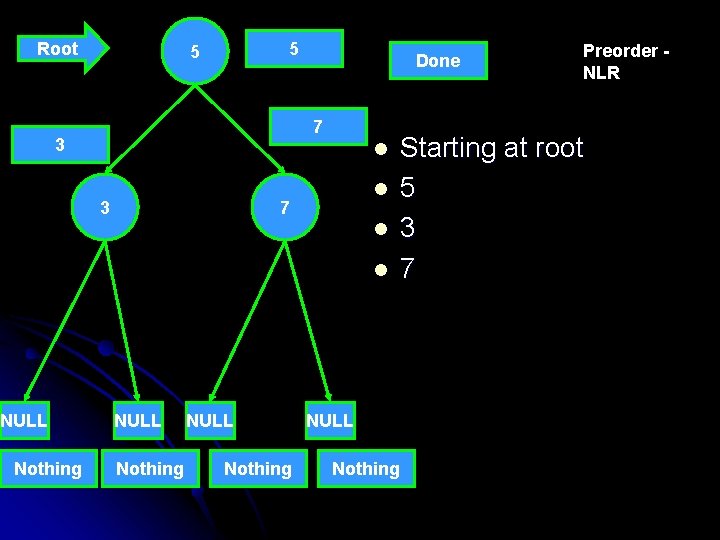
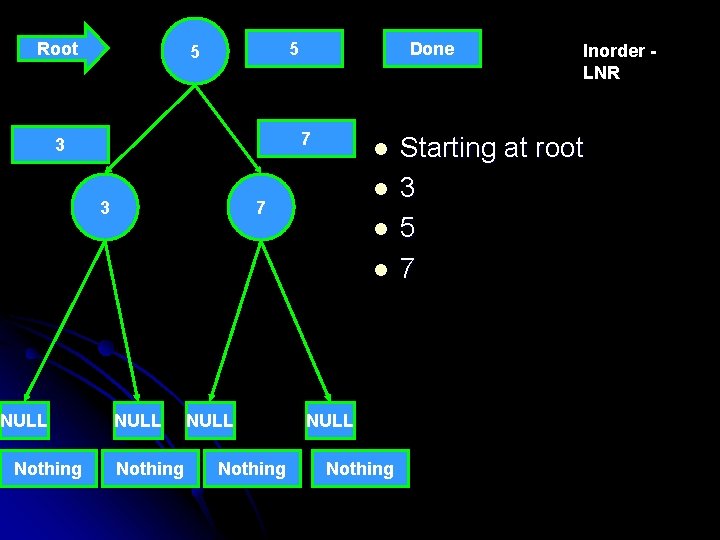
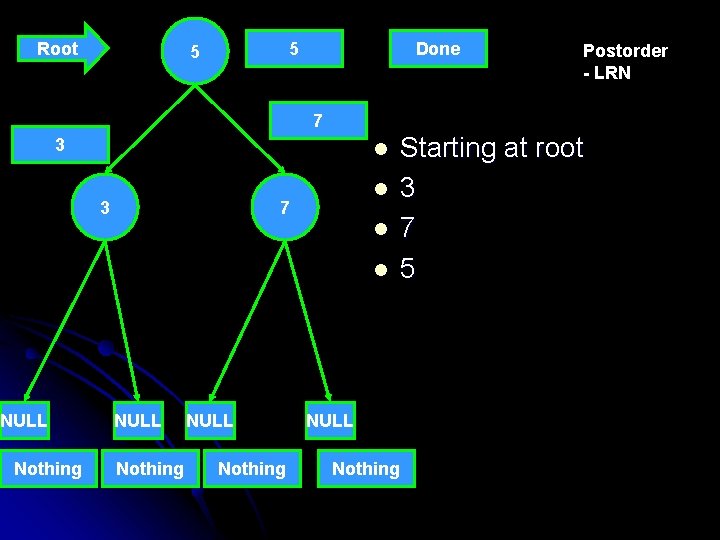
- Slides: 10
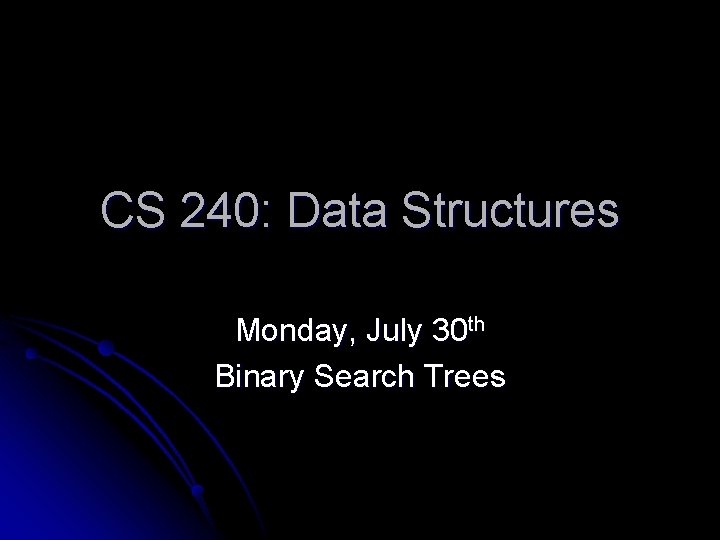
CS 240: Data Structures Monday, July 30 th Binary Search Trees
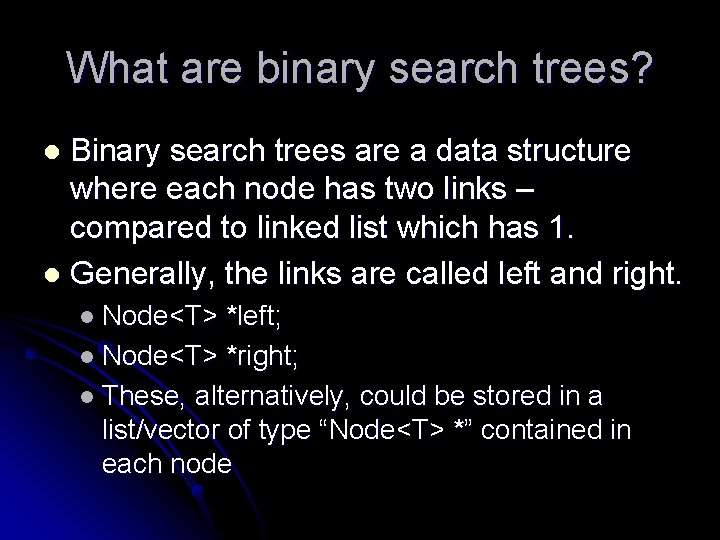
What are binary search trees? Binary search trees are a data structure where each node has two links – compared to linked list which has 1. l Generally, the links are called left and right. l l Node<T> *left; l Node<T> *right; l These, alternatively, could be stored in a list/vector of type “Node<T> *” contained in each node
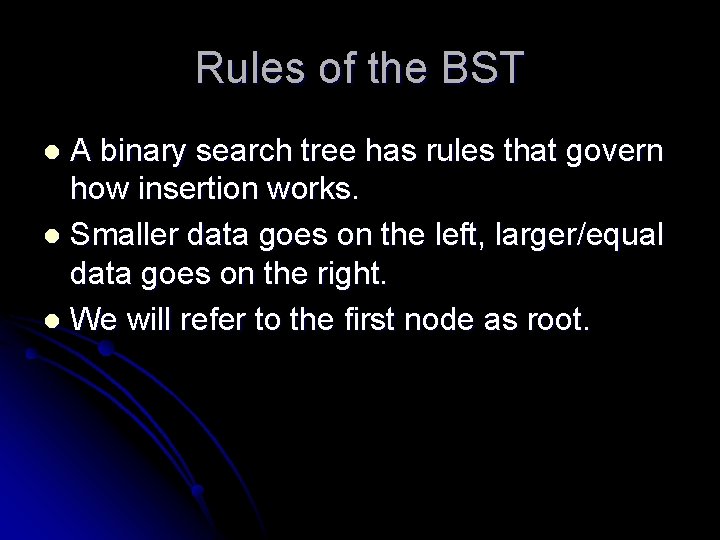
Rules of the BST A binary search tree has rules that govern how insertion works. l Smaller data goes on the left, larger/equal data goes on the right. l We will refer to the first node as root. l
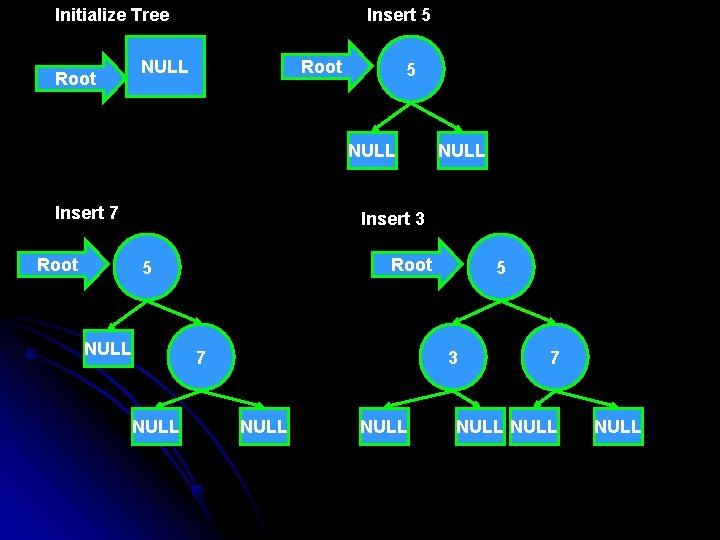
Initialize Tree Root Insert 5 Root NULL 5 NULL Insert 7 Root NULL Insert 3 Root 5 NULL 7 NULL 5 3 NULL 7 NULL
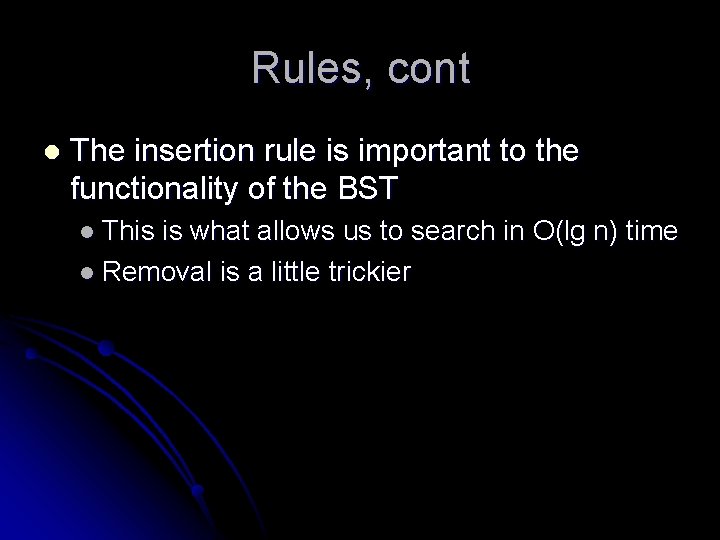
Rules, cont l The insertion rule is important to the functionality of the BST l This is what allows us to search in O(lg n) time l Removal is a little trickier
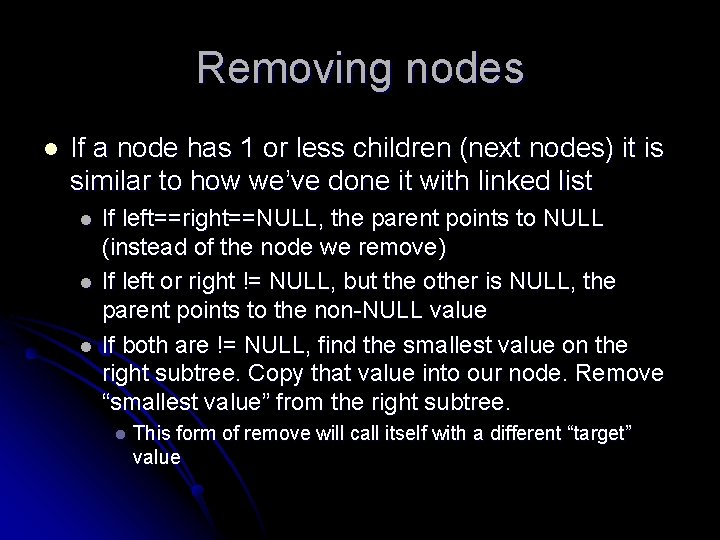
Removing nodes l If a node has 1 or less children (next nodes) it is similar to how we’ve done it with linked list l l l If left==right==NULL, the parent points to NULL (instead of the node we remove) If left or right != NULL, but the other is NULL, the parent points to the non-NULL value If both are != NULL, find the smallest value on the right subtree. Copy that value into our node. Remove “smallest value” from the right subtree. l This form of remove will call itself with a different “target” value
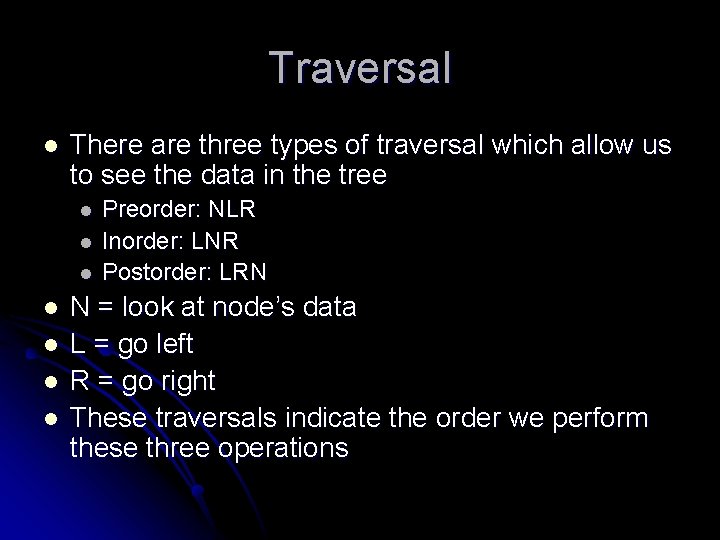
Traversal l There are three types of traversal which allow us to see the data in the tree l l l l Preorder: NLR Inorder: LNR Postorder: LRN N = look at node’s data L = go left R = go right These traversals indicate the order we perform these three operations
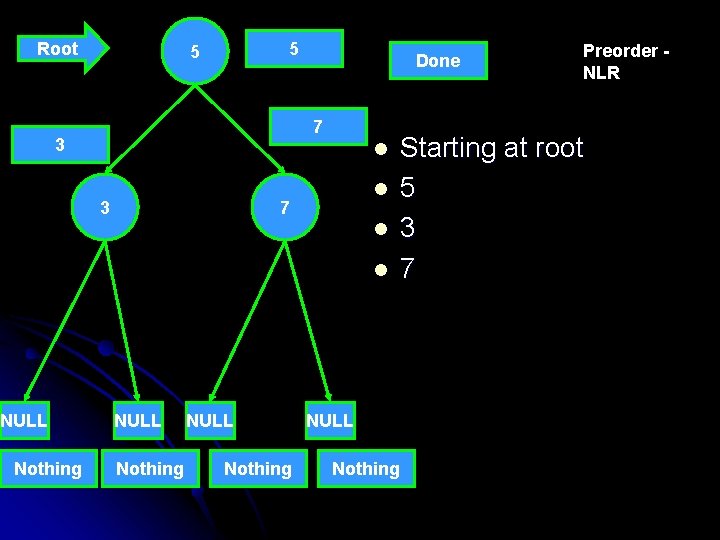
Root NLR: R: 5 555 5 Done NLR: R: 7 777 NLR: R: 3 333 l 7 l l NULL Nothing Preorder NLR Starting at root 5 3 7
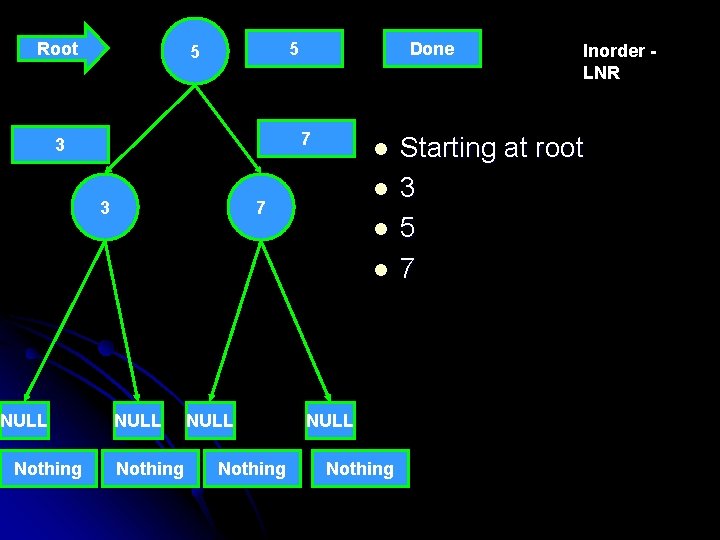
Root LNR: R: 5 555 5 Done LNR: R: 7 777 LNR: R: 3 333 3 l l 7 l l NULL Nothing Inorder LNR Starting at root 3 5 7
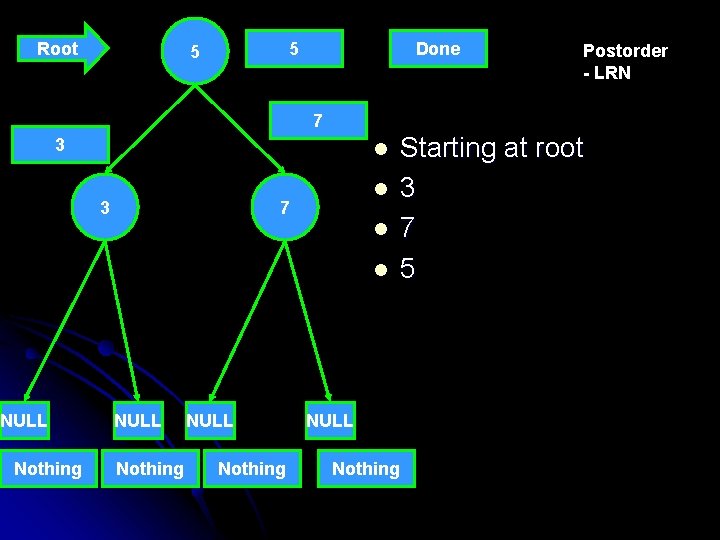
Root LRN: N: 5 555 5 Done Postorder - LRN: RN: N: 7 777 LRN: N: 3 333 l 7 l l NULL Nothing Starting at root 3 7 5