CONTROL STRUCTURES If else Else if While Do
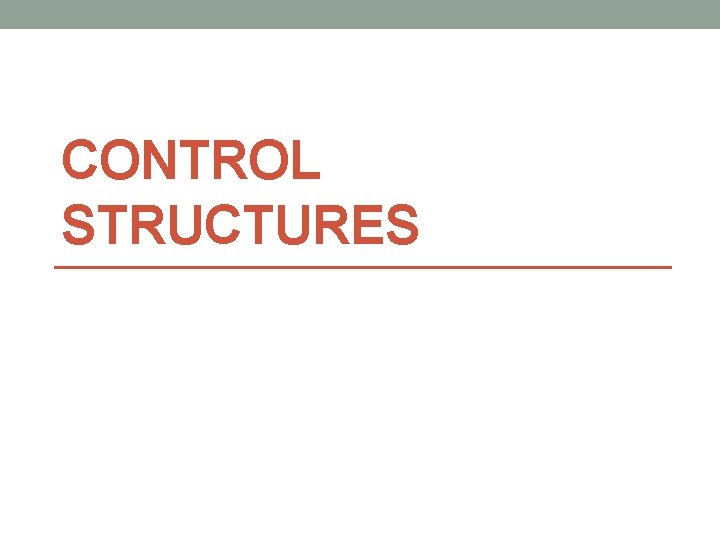
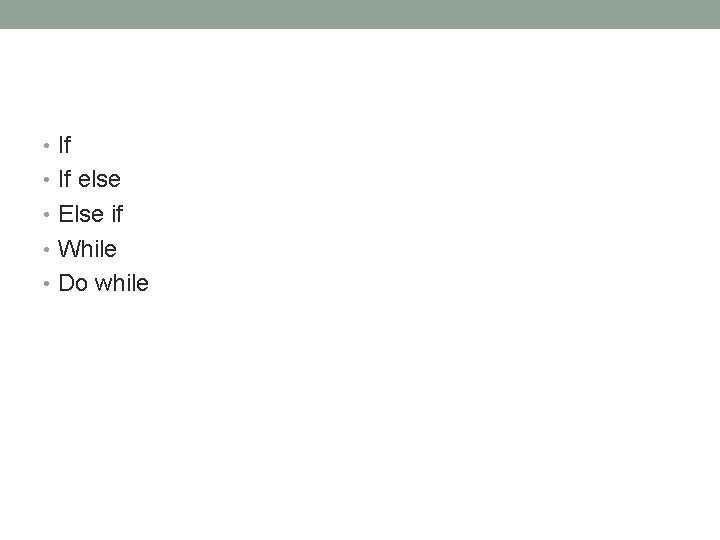
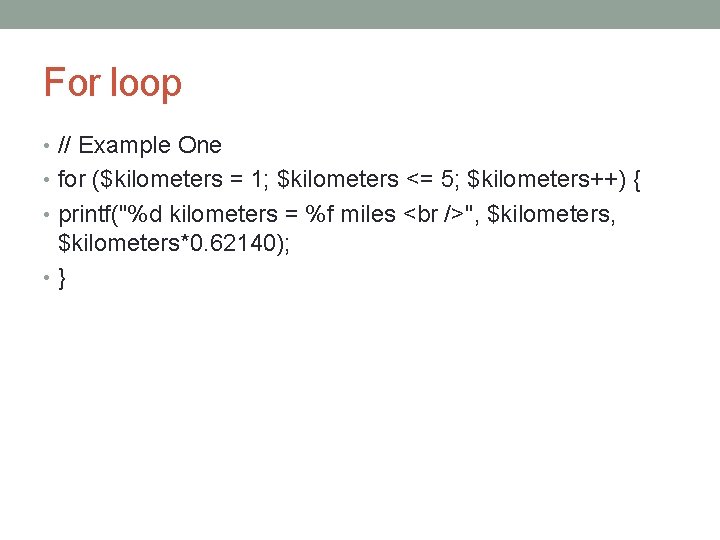
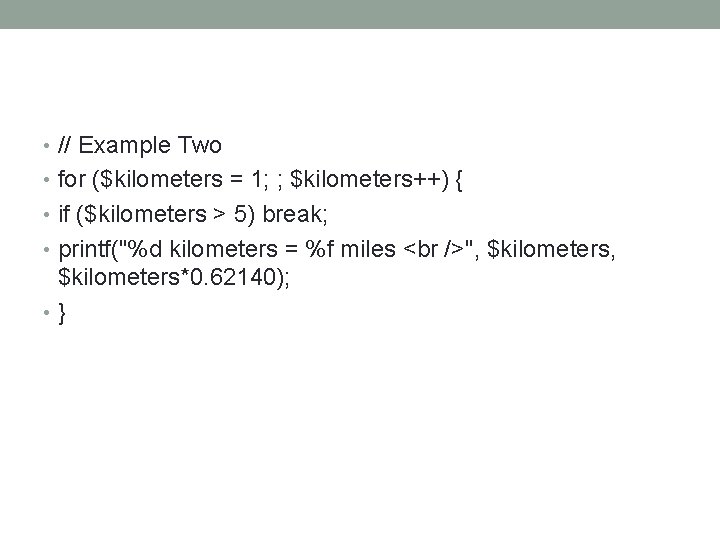
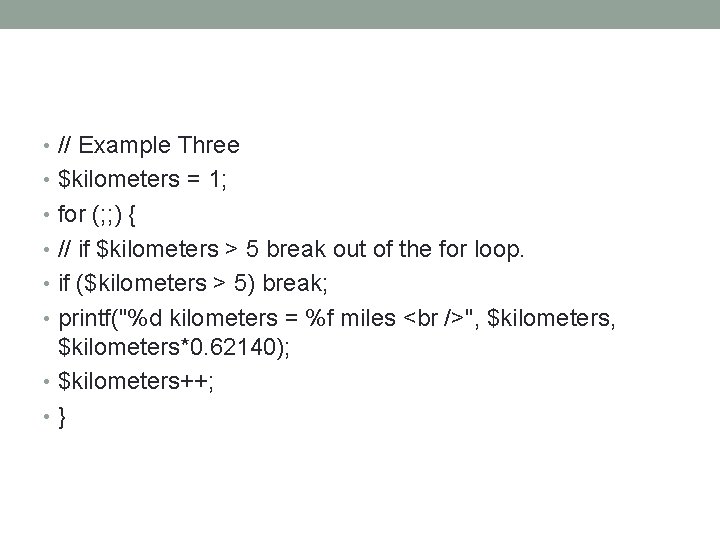
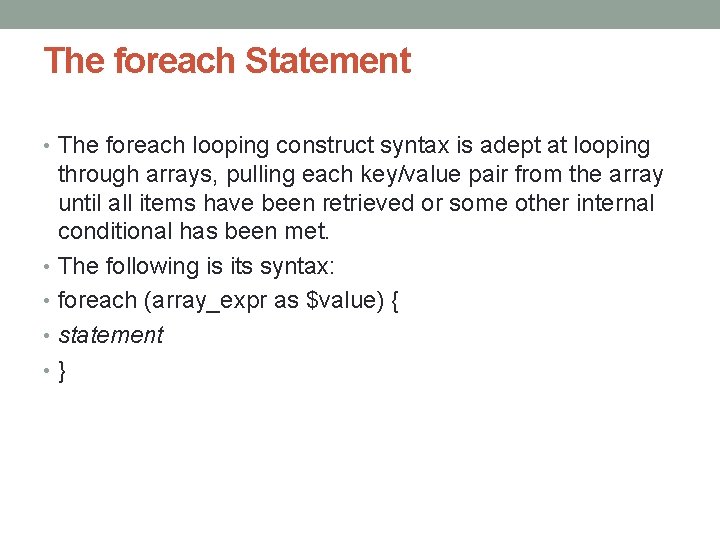
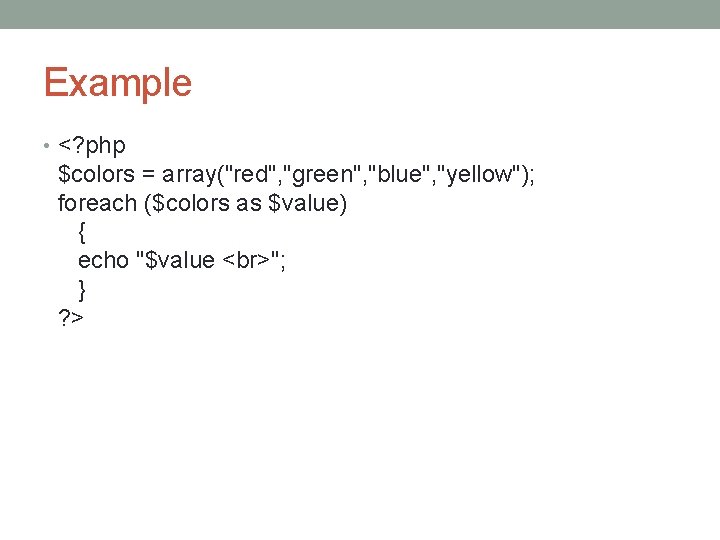
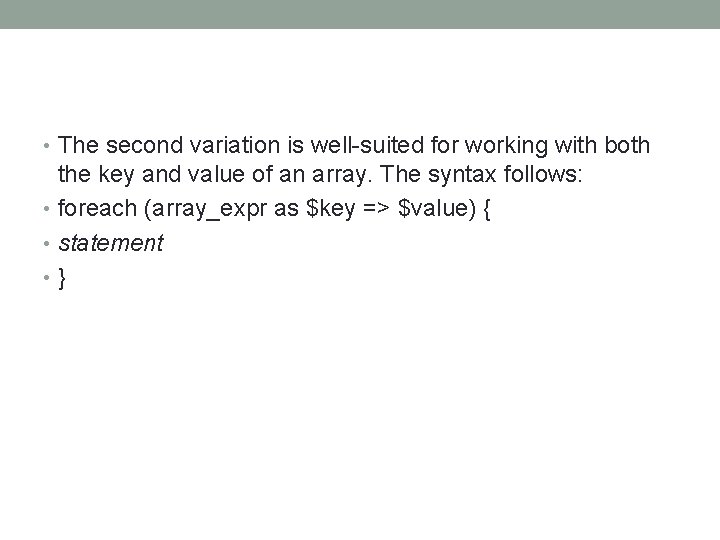
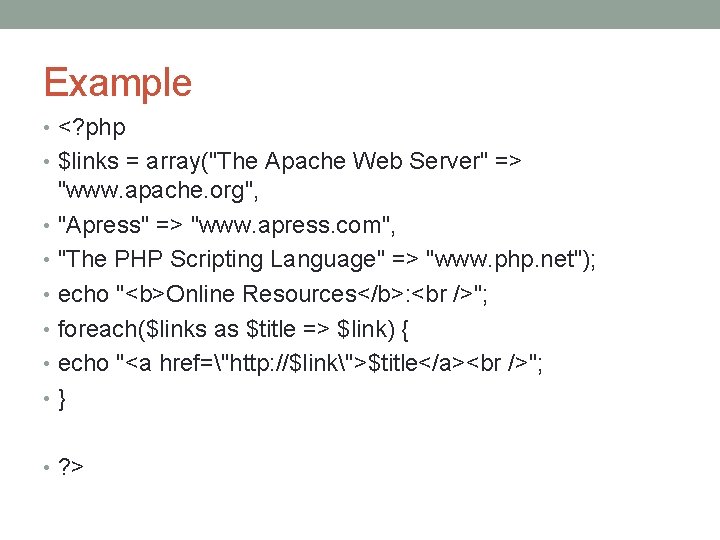
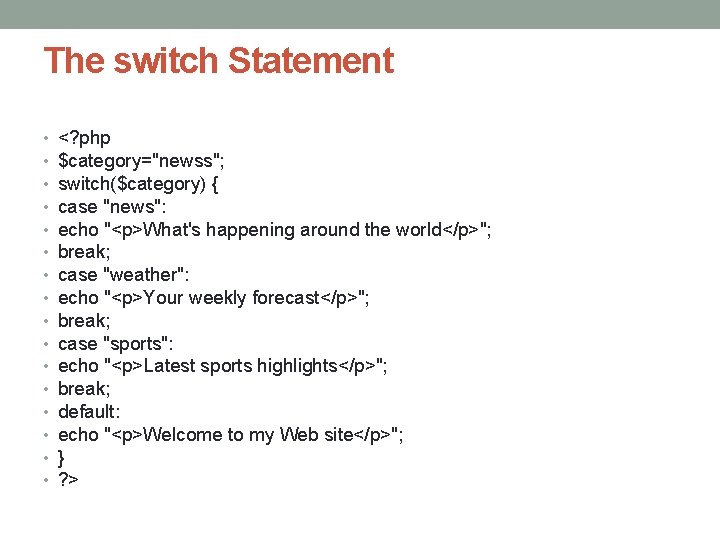
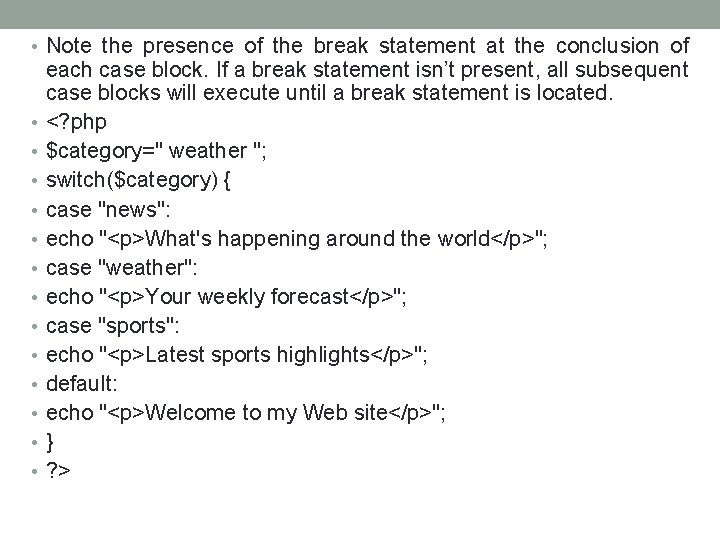
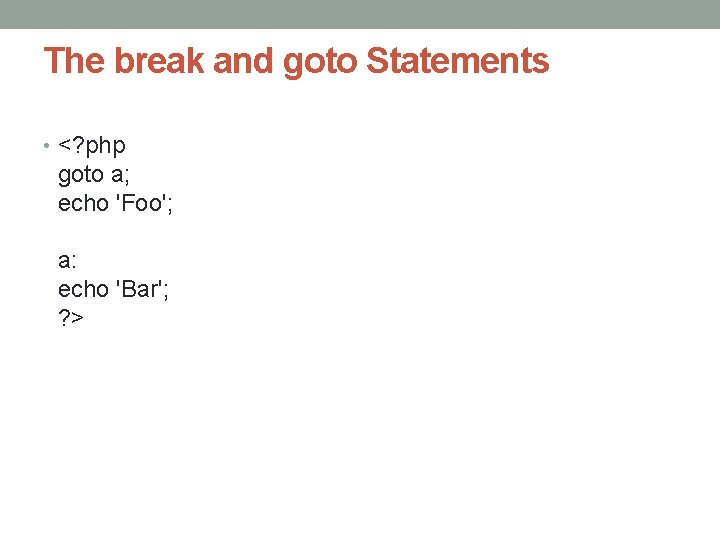
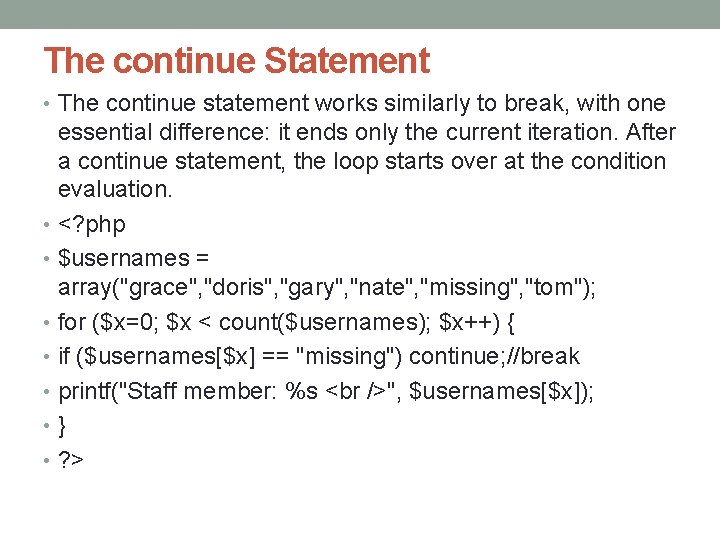
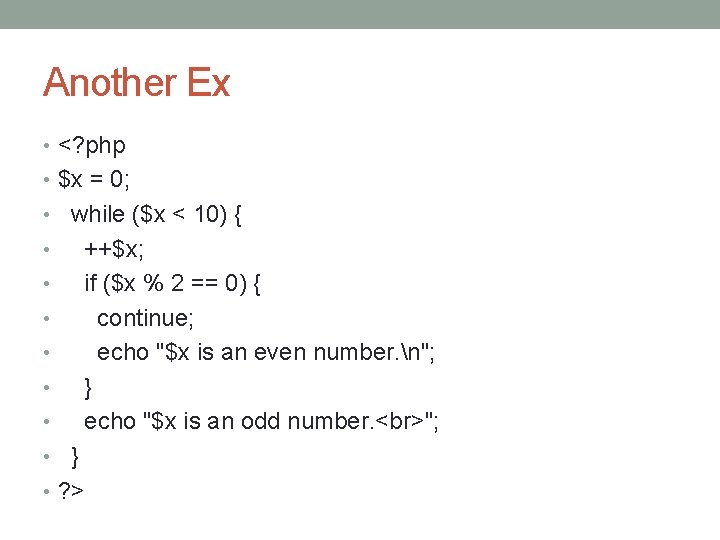
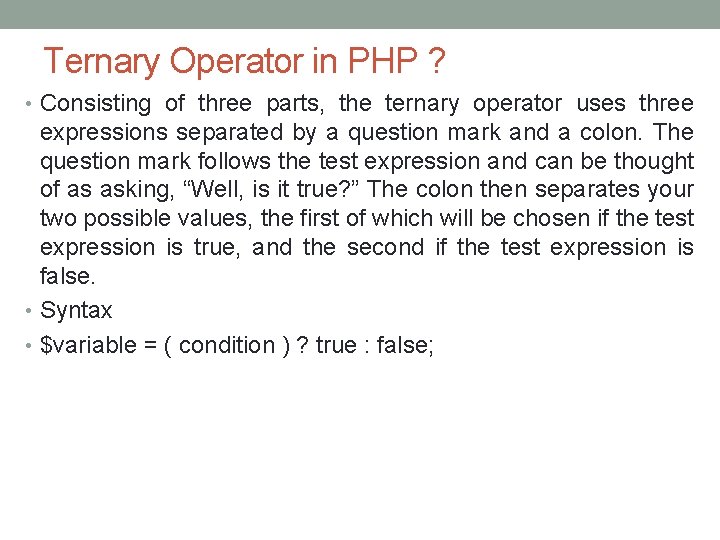
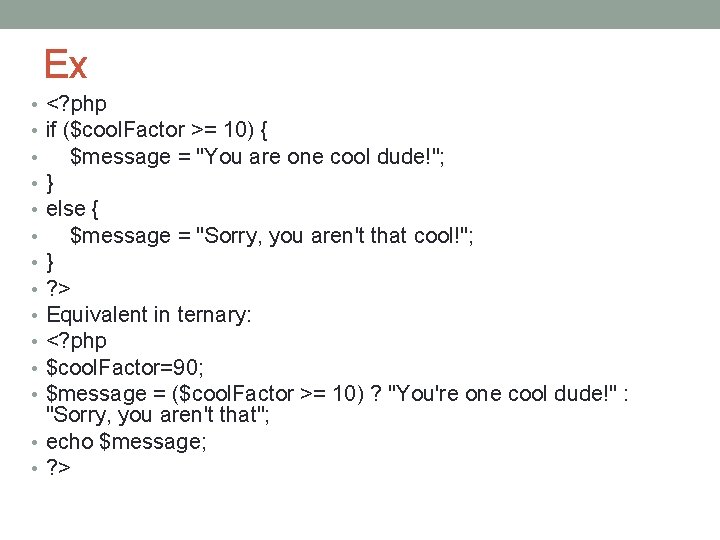
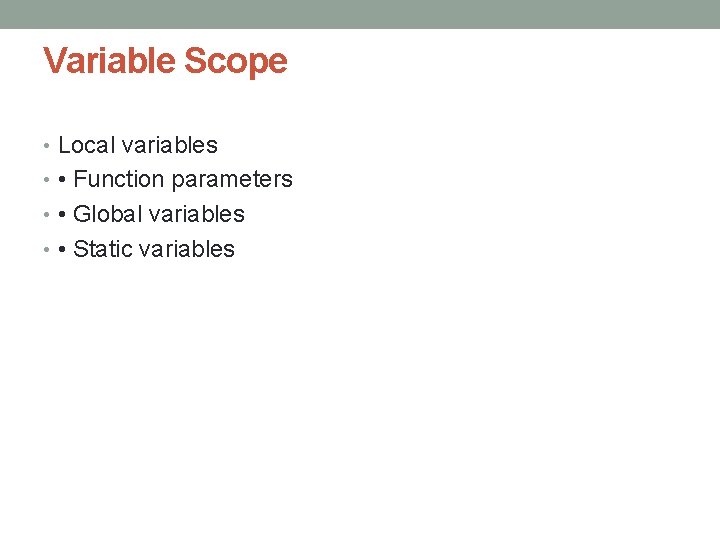
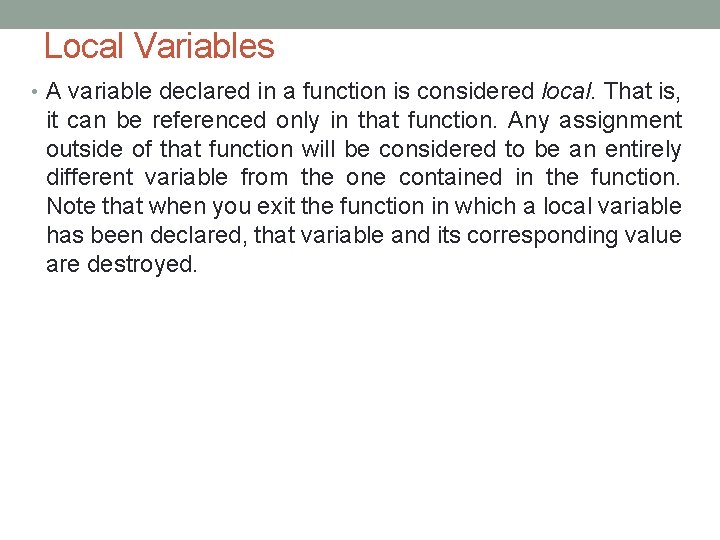
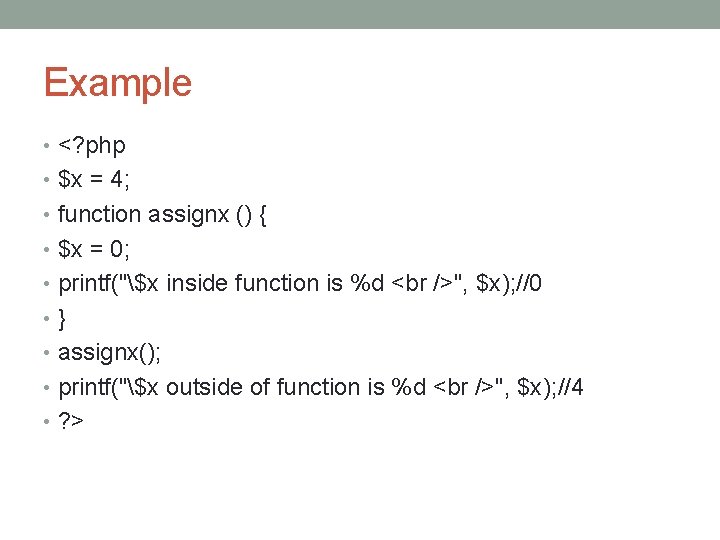
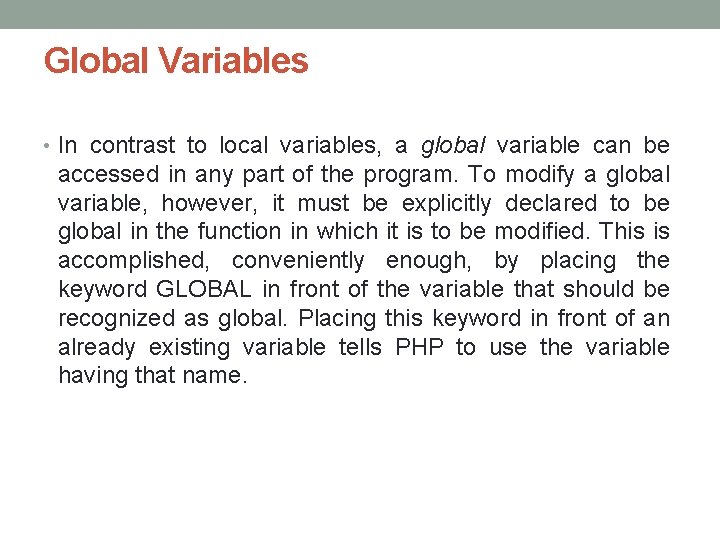
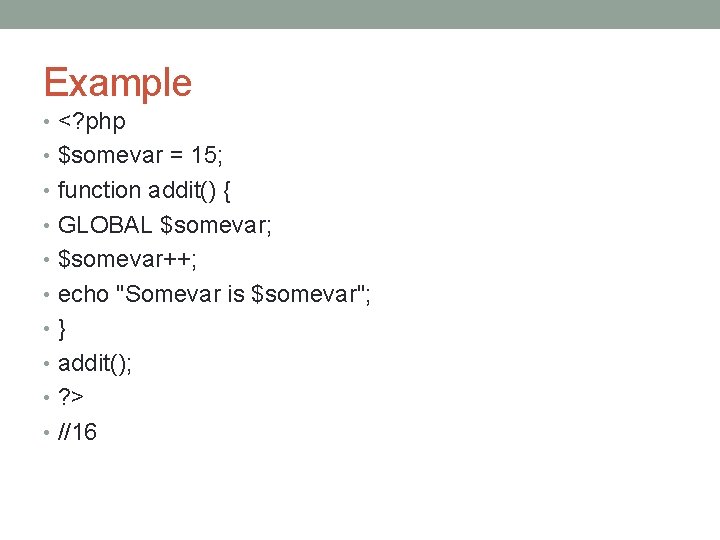
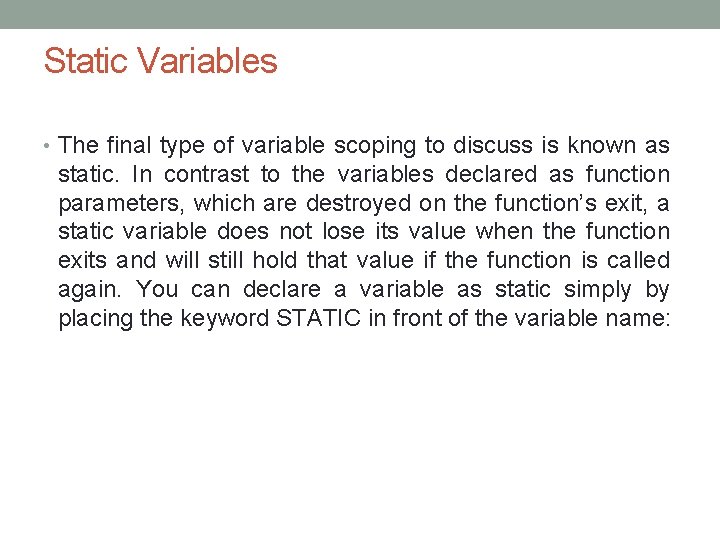
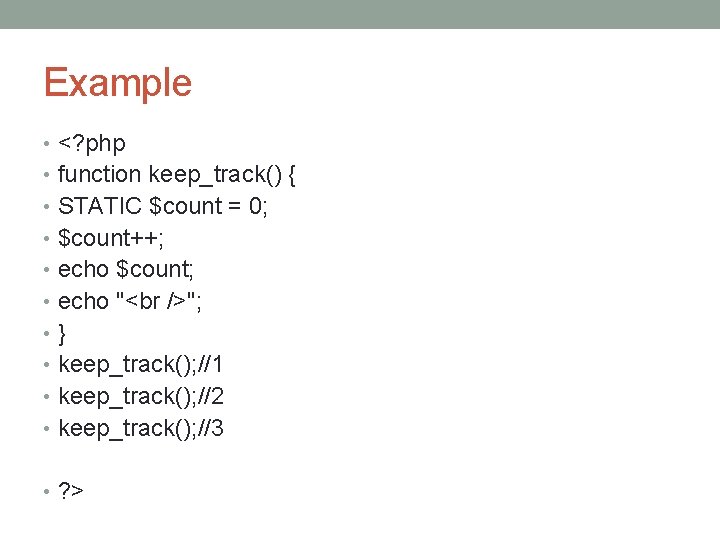
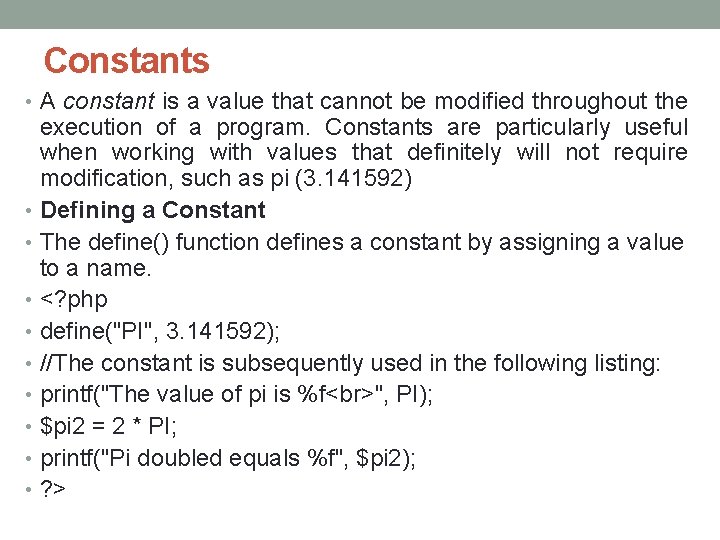
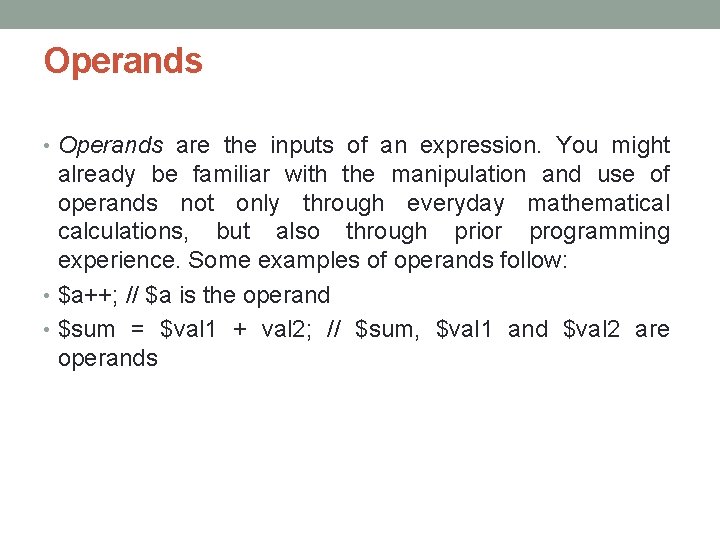
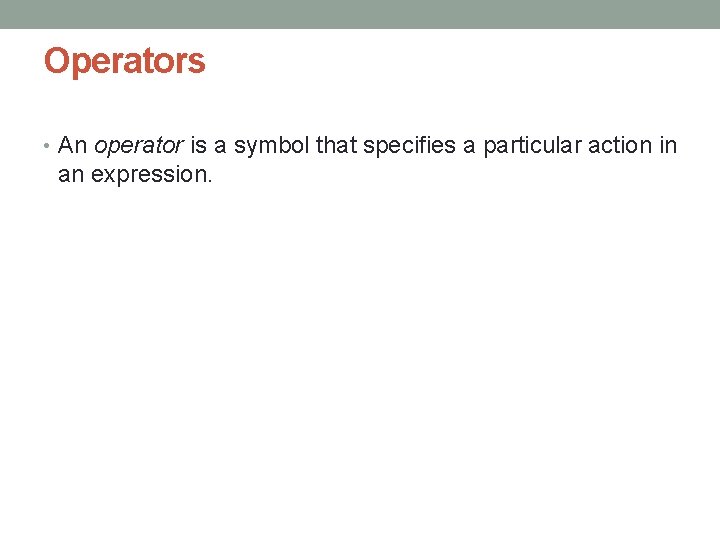
- Slides: 26
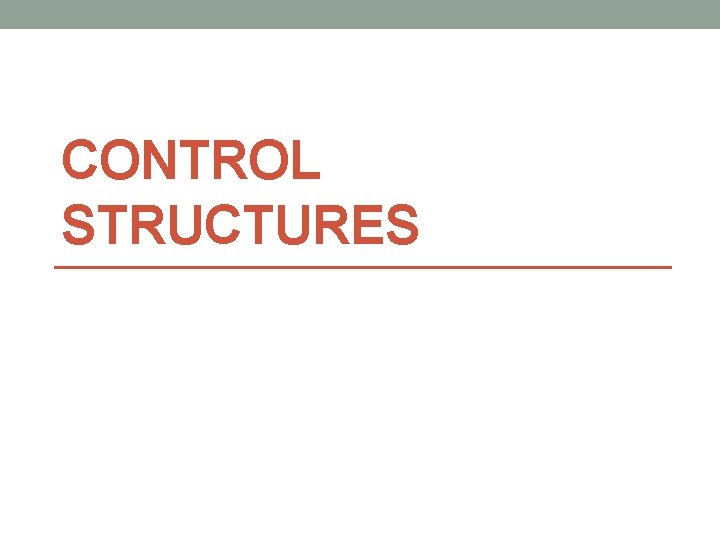
CONTROL STRUCTURES
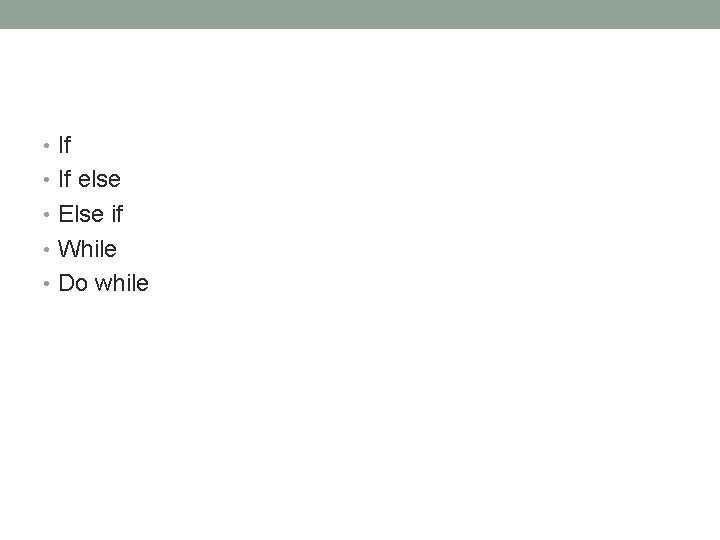
• If else • Else if • While • Do while
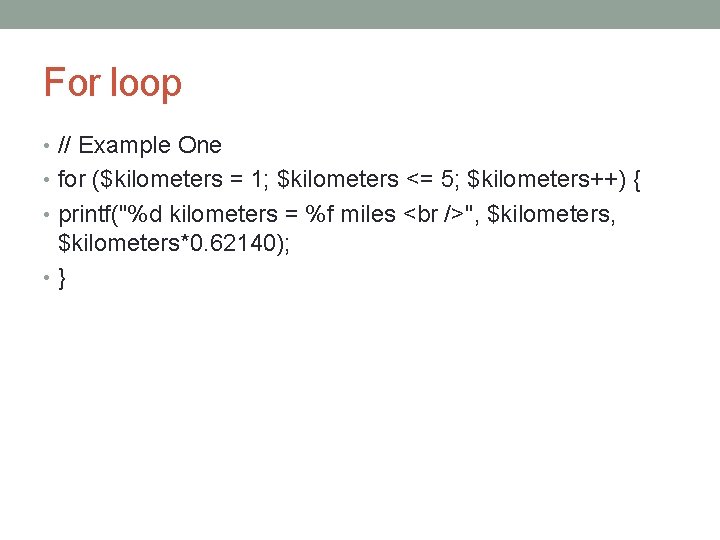
For loop • // Example One • for ($kilometers = 1; $kilometers <= 5; $kilometers++) { • printf("%d kilometers = %f miles ", $kilometers, $kilometers*0. 62140); • }
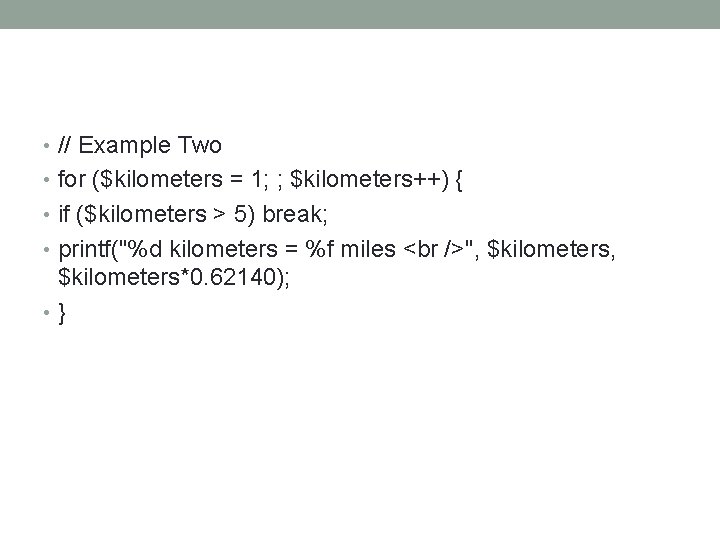
• // Example Two • for ($kilometers = 1; ; $kilometers++) { • if ($kilometers > 5) break; • printf("%d kilometers = %f miles ", $kilometers, $kilometers*0. 62140); • }
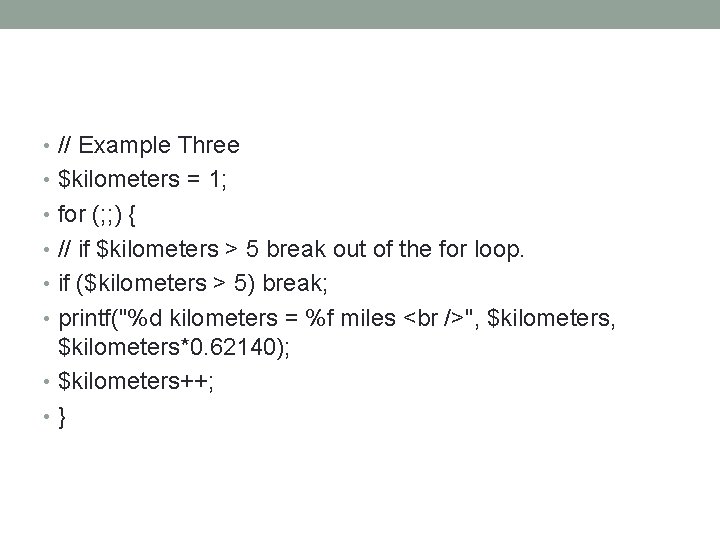
• // Example Three • $kilometers = 1; • for (; ; ) { • // if $kilometers > 5 break out of the for loop. • if ($kilometers > 5) break; • printf("%d kilometers = %f miles ", $kilometers, $kilometers*0. 62140); • $kilometers++; • }
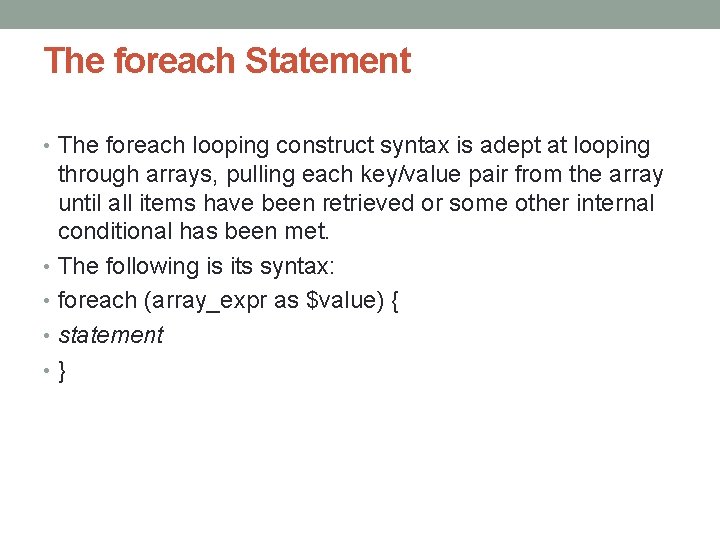
The foreach Statement • The foreach looping construct syntax is adept at looping through arrays, pulling each key/value pair from the array until all items have been retrieved or some other internal conditional has been met. • The following is its syntax: • foreach (array_expr as $value) { • statement • }
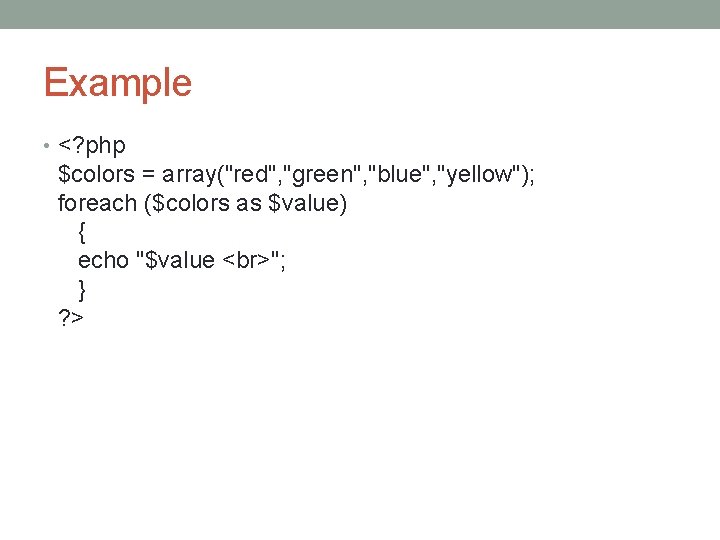
Example • <? php $colors = array("red", "green", "blue", "yellow"); foreach ($colors as $value) { echo "$value "; } ? >
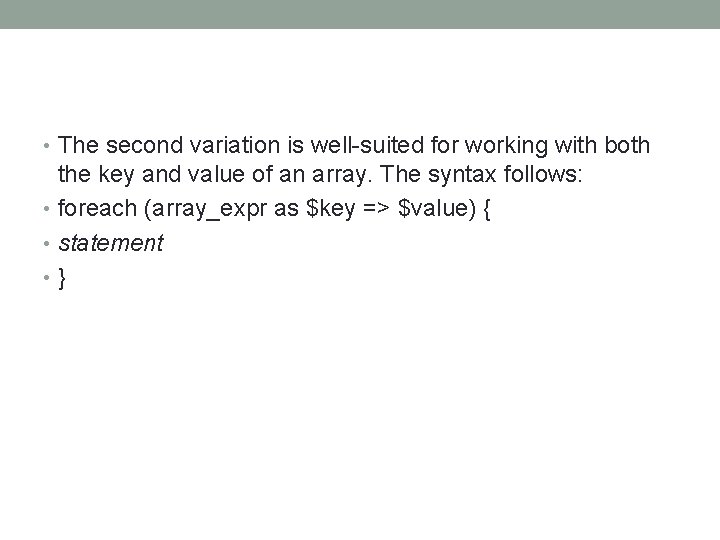
• The second variation is well-suited for working with both the key and value of an array. The syntax follows: • foreach (array_expr as $key => $value) { • statement • }
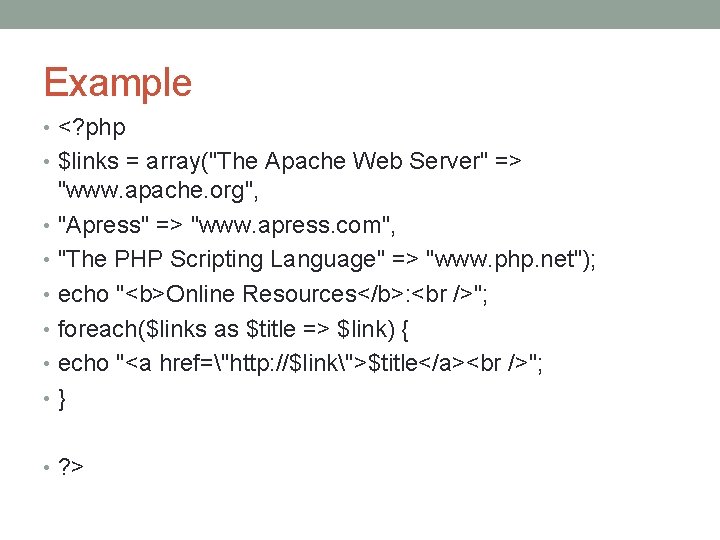
Example • <? php • $links = array("The Apache Web Server" => "www. apache. org", • "Apress" => "www. apress. com", • "The PHP Scripting Language" => "www. php. net"); • echo "<b>Online Resources</b>: "; • foreach($links as $title => $link) { • echo "<a href="http: //$link">$title</a> "; • } • ? >
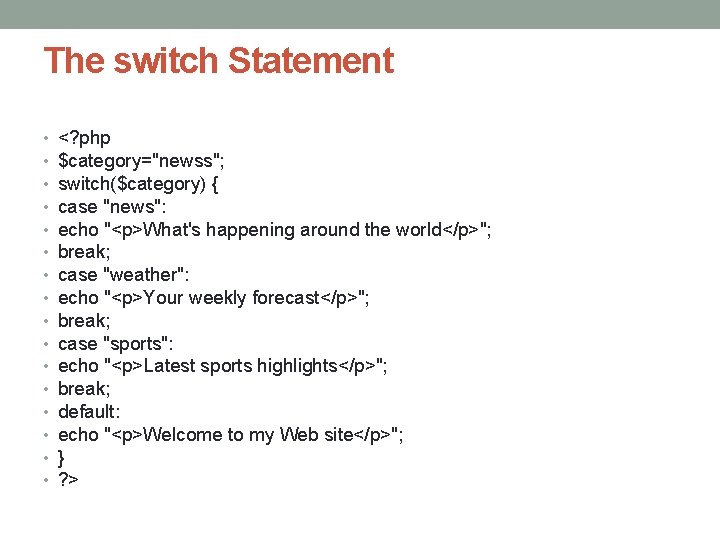
The switch Statement • • • • <? php $category="newss"; switch($category) { case "news": echo "<p>What's happening around the world</p>"; break; case "weather": echo "<p>Your weekly forecast</p>"; break; case "sports": echo "<p>Latest sports highlights</p>"; break; default: echo "<p>Welcome to my Web site</p>"; } ? >
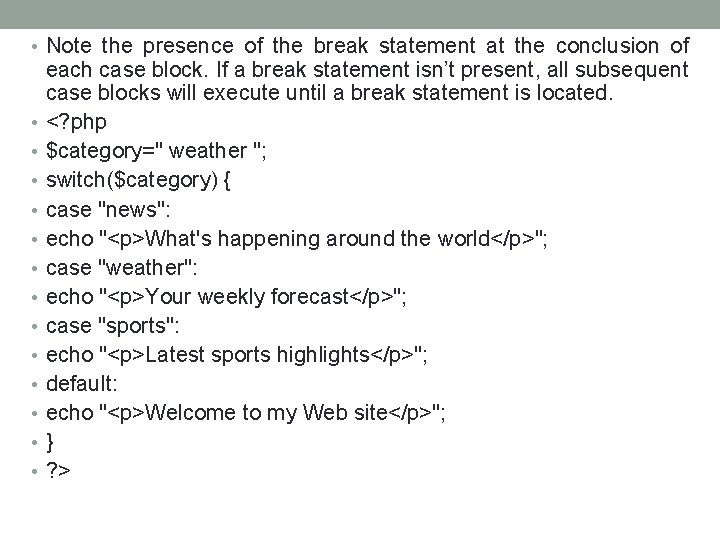
• Note the presence of the break statement at the conclusion of • • • • each case block. If a break statement isn’t present, all subsequent case blocks will execute until a break statement is located. <? php $category=" weather "; switch($category) { case "news": echo "<p>What's happening around the world</p>"; case "weather": echo "<p>Your weekly forecast</p>"; case "sports": echo "<p>Latest sports highlights</p>"; default: echo "<p>Welcome to my Web site</p>"; } ? >
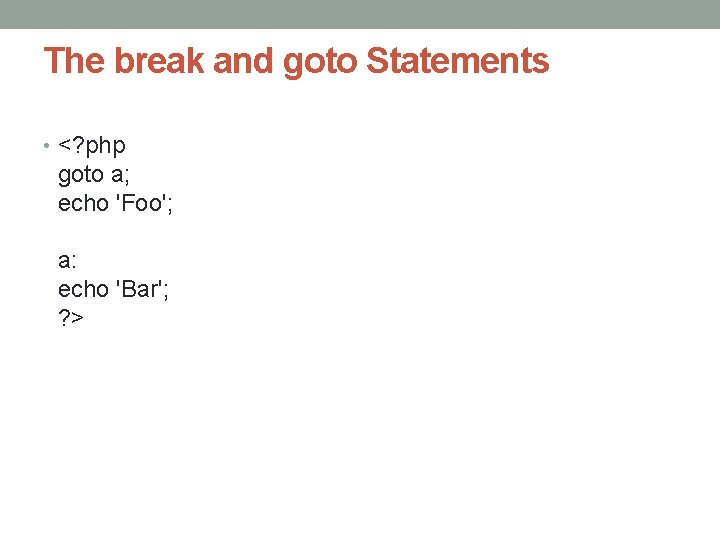
The break and goto Statements • <? php goto a; echo 'Foo'; a: echo 'Bar'; ? >
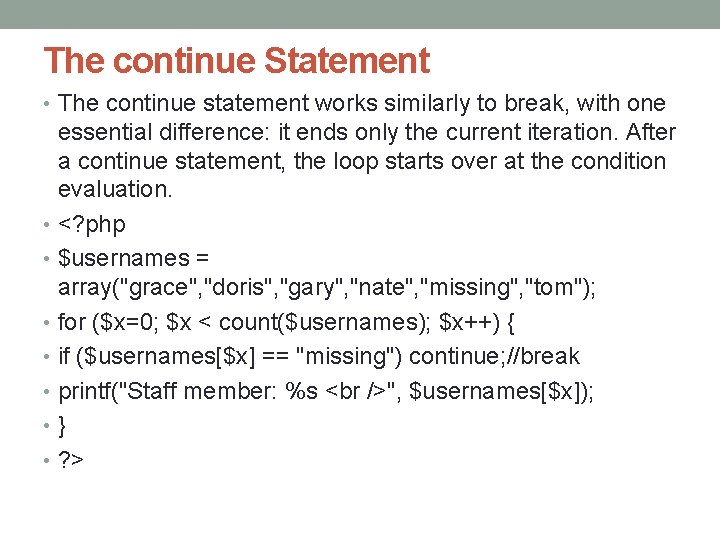
The continue Statement • The continue statement works similarly to break, with one essential difference: it ends only the current iteration. After a continue statement, the loop starts over at the condition evaluation. • <? php • $usernames = array("grace", "doris", "gary", "nate", "missing", "tom"); • for ($x=0; $x < count($usernames); $x++) { • if ($usernames[$x] == "missing") continue; //break • printf("Staff member: %s ", $usernames[$x]); • } • ? >
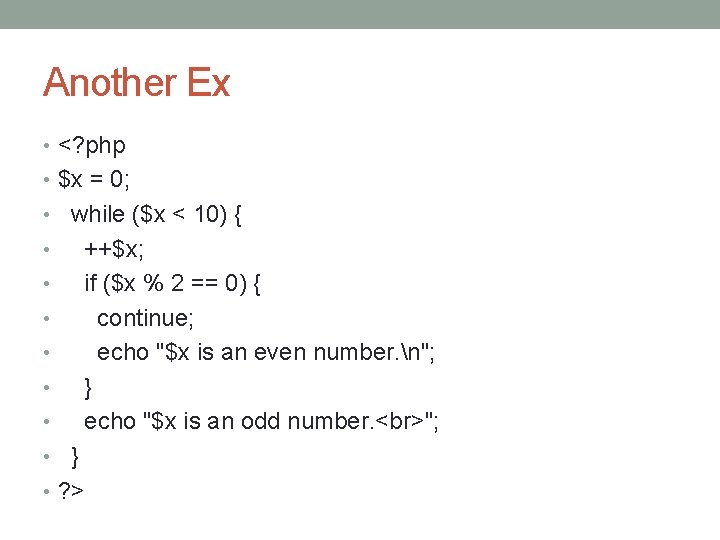
Another Ex • <? php • $x = 0; • while ($x < 10) { • • • ++$x; if ($x % 2 == 0) { continue; echo "$x is an even number. n"; } echo "$x is an odd number. "; • } • ? >
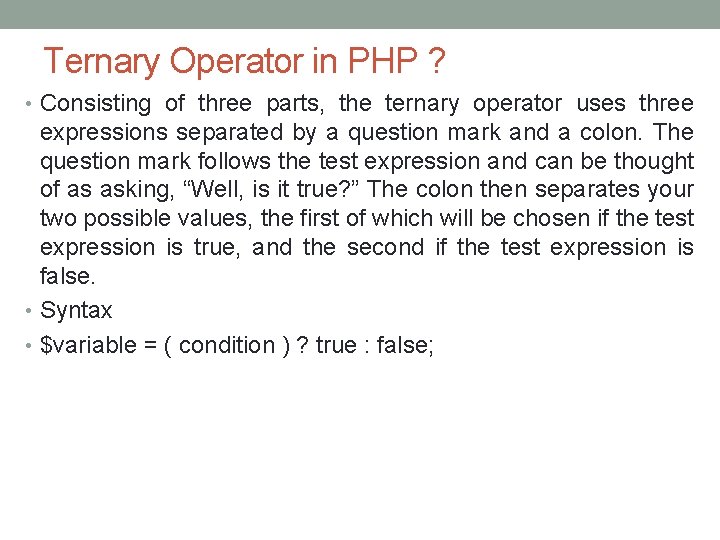
Ternary Operator in PHP ? • Consisting of three parts, the ternary operator uses three expressions separated by a question mark and a colon. The question mark follows the test expression and can be thought of as asking, “Well, is it true? ” The colon then separates your two possible values, the first of which will be chosen if the test expression is true, and the second if the test expression is false. • Syntax • $variable = ( condition ) ? true : false;
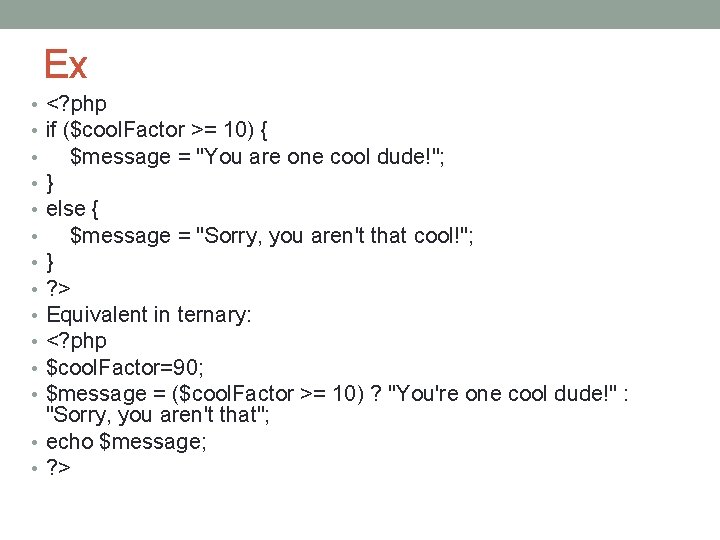
Ex <? php if ($cool. Factor >= 10) { $message = "You are one cool dude!"; } else { $message = "Sorry, you aren't that cool!"; } ? > Equivalent in ternary: <? php $cool. Factor=90; $message = ($cool. Factor >= 10) ? "You're one cool dude!" : "Sorry, you aren't that"; • echo $message; • ? > • • •
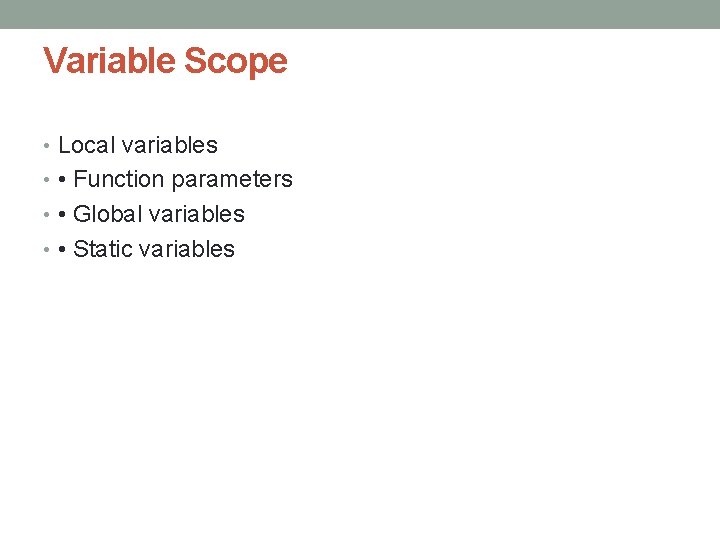
Variable Scope • Local variables • • Function parameters • • Global variables • • Static variables
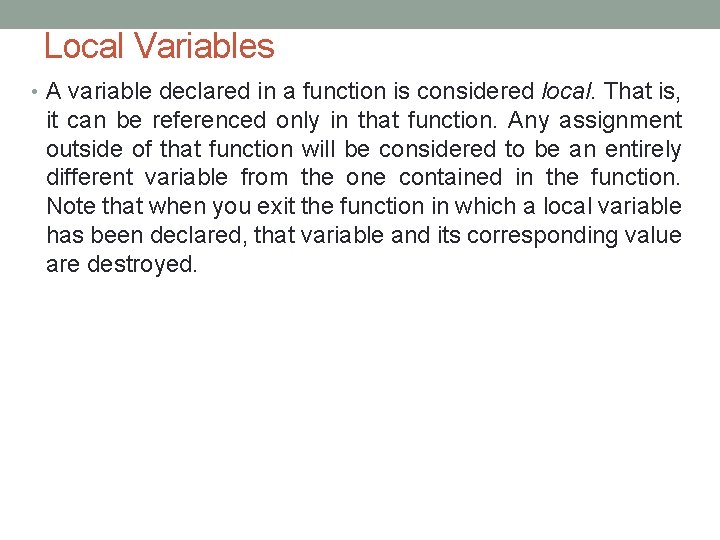
Local Variables • A variable declared in a function is considered local. That is, it can be referenced only in that function. Any assignment outside of that function will be considered to be an entirely different variable from the one contained in the function. Note that when you exit the function in which a local variable has been declared, that variable and its corresponding value are destroyed.
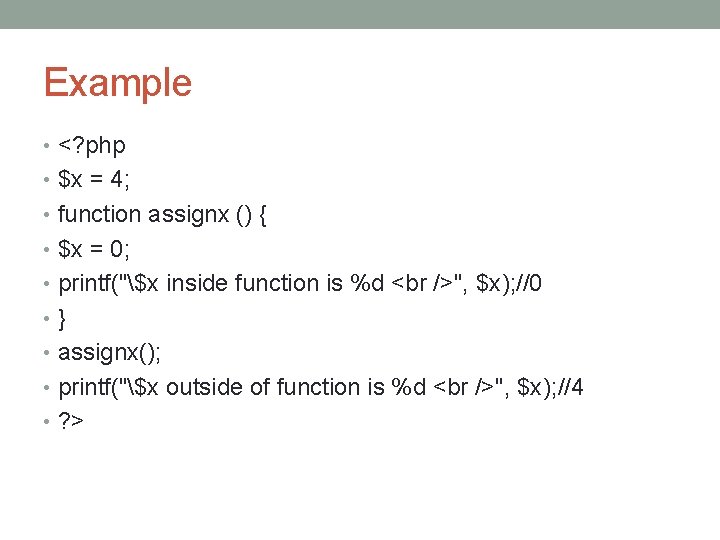
Example • <? php • $x = 4; • function assignx () { • $x = 0; • printf("$x inside function is %d ", $x); //0 • } • assignx(); • printf("$x outside of function is %d ", $x); //4 • ? >
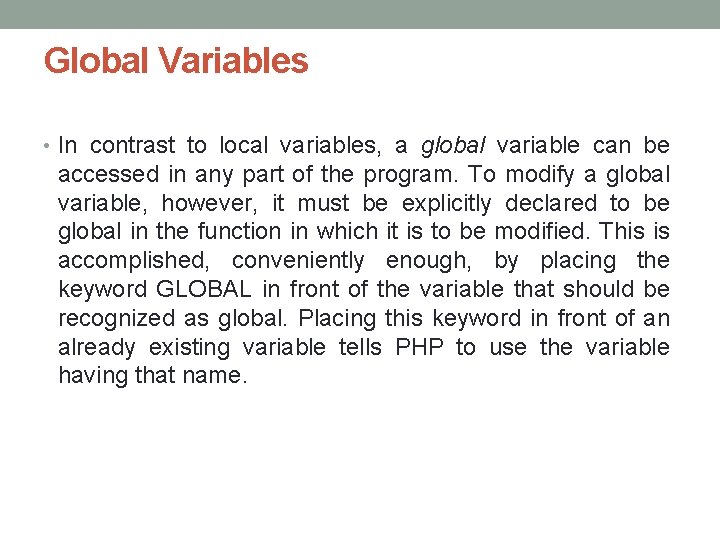
Global Variables • In contrast to local variables, a global variable can be accessed in any part of the program. To modify a global variable, however, it must be explicitly declared to be global in the function in which it is to be modified. This is accomplished, conveniently enough, by placing the keyword GLOBAL in front of the variable that should be recognized as global. Placing this keyword in front of an already existing variable tells PHP to use the variable having that name.
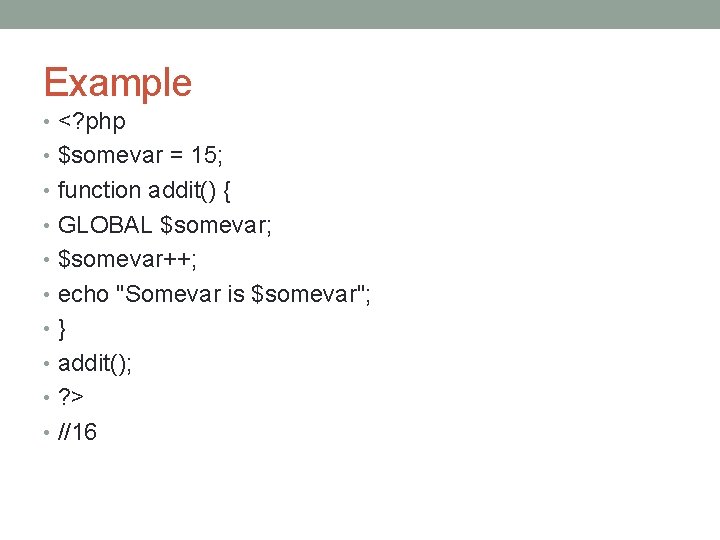
Example • <? php • $somevar = 15; • function addit() { • GLOBAL $somevar; • $somevar++; • echo "Somevar is $somevar"; • } • addit(); • ? > • //16
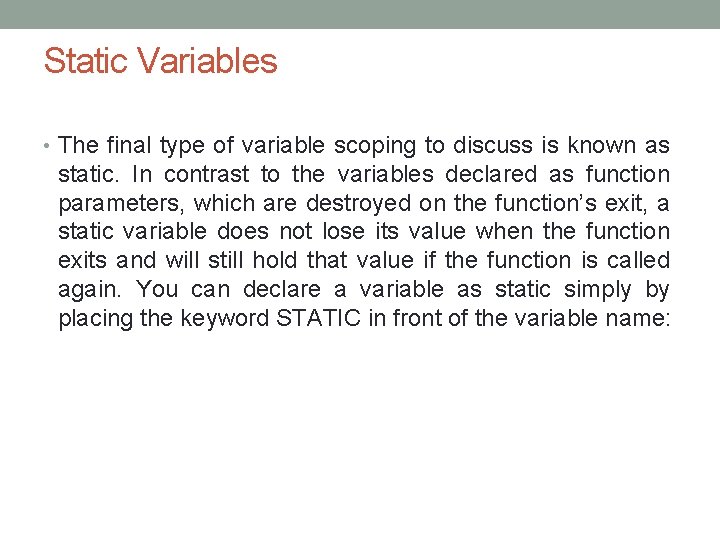
Static Variables • The final type of variable scoping to discuss is known as static. In contrast to the variables declared as function parameters, which are destroyed on the function’s exit, a static variable does not lose its value when the function exits and will still hold that value if the function is called again. You can declare a variable as static simply by placing the keyword STATIC in front of the variable name:
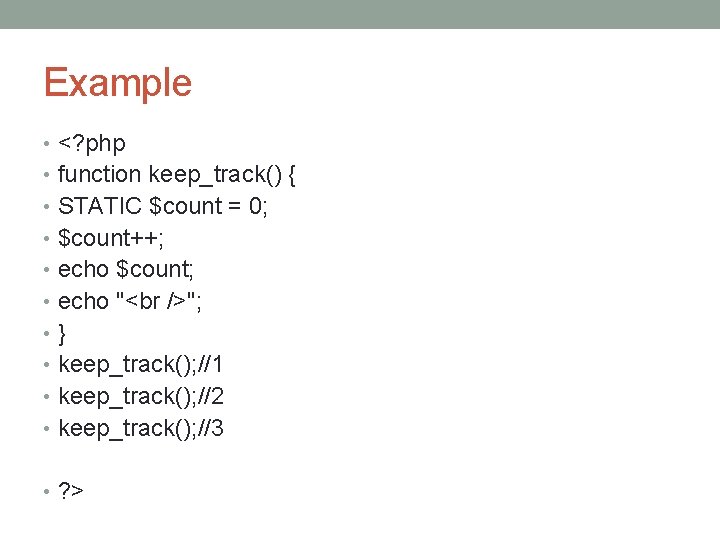
Example • <? php • function keep_track() { • STATIC $count = 0; • $count++; • echo $count; • echo " "; • } • keep_track(); //1 • keep_track(); //2 • keep_track(); //3 • ? >
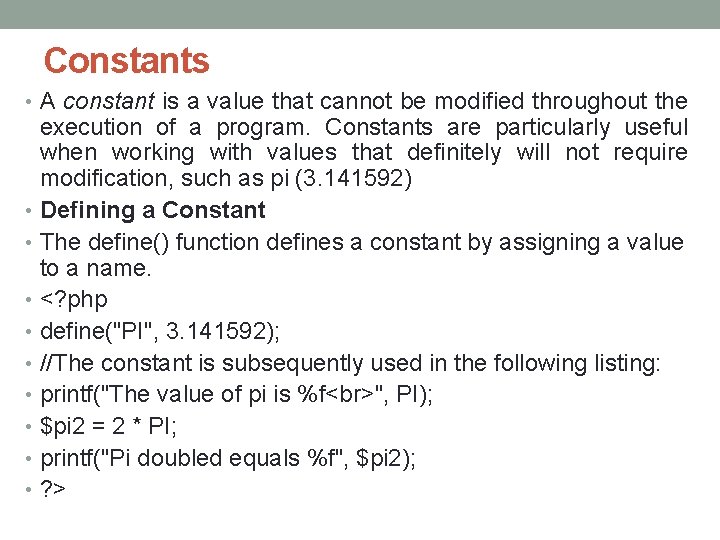
Constants • A constant is a value that cannot be modified throughout the execution of a program. Constants are particularly useful when working with values that definitely will not require modification, such as pi (3. 141592) • Defining a Constant • The define() function defines a constant by assigning a value to a name. • <? php • define("PI", 3. 141592); • //The constant is subsequently used in the following listing: • printf("The value of pi is %f ", PI); • $pi 2 = 2 * PI; • printf("Pi doubled equals %f", $pi 2); • ? >
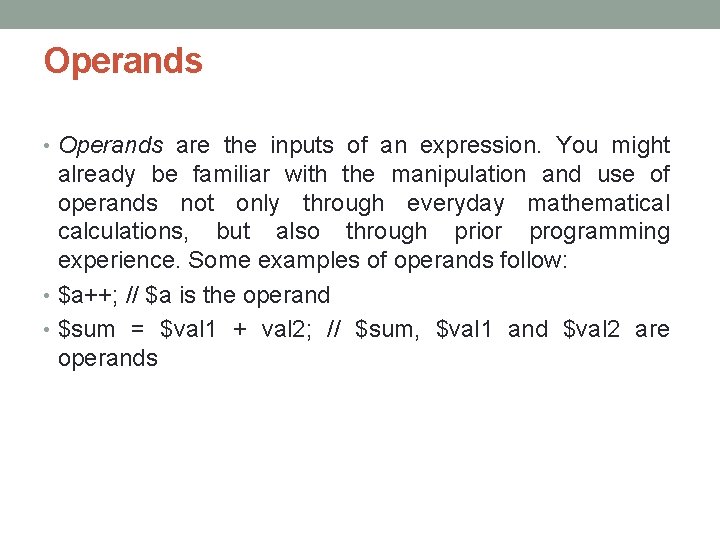
Operands • Operands are the inputs of an expression. You might already be familiar with the manipulation and use of operands not only through everyday mathematical calculations, but also through prior programming experience. Some examples of operands follow: • $a++; // $a is the operand • $sum = $val 1 + val 2; // $sum, $val 1 and $val 2 are operands
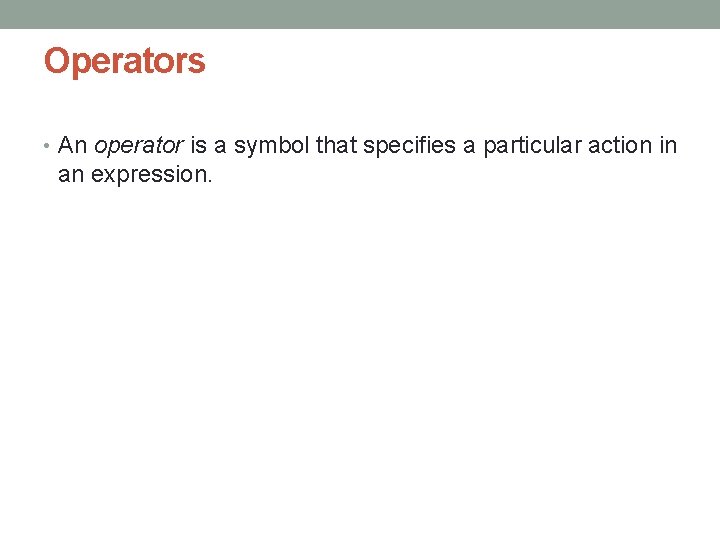
Operators • An operator is a symbol that specifies a particular action in an expression.
While loops and if-else structures
Dug in behind enemy lines
Pernyataan do while
Homologous structures
What can you do to control your emotions while driving
Hardware and control structures
Statement level control structures
Control structures in php
Control structures in c
Flag controlled loop c++
Types of control structures
Loop instruction in 8086
Statement level control structures
Control structures in php
Iteration control structures
If a do…while structure is used:
Iteration control structures
Entity life history
Pseudocode outline
Visual basic control structures
Primary control vs secondary control
Product control
Fluids mechanics
Stock control e flow control
Control volume vs control surface
Positive control vs negative control gene expression
What is variable in research