C IF ELSE IF ELSE STATEMENT If statements
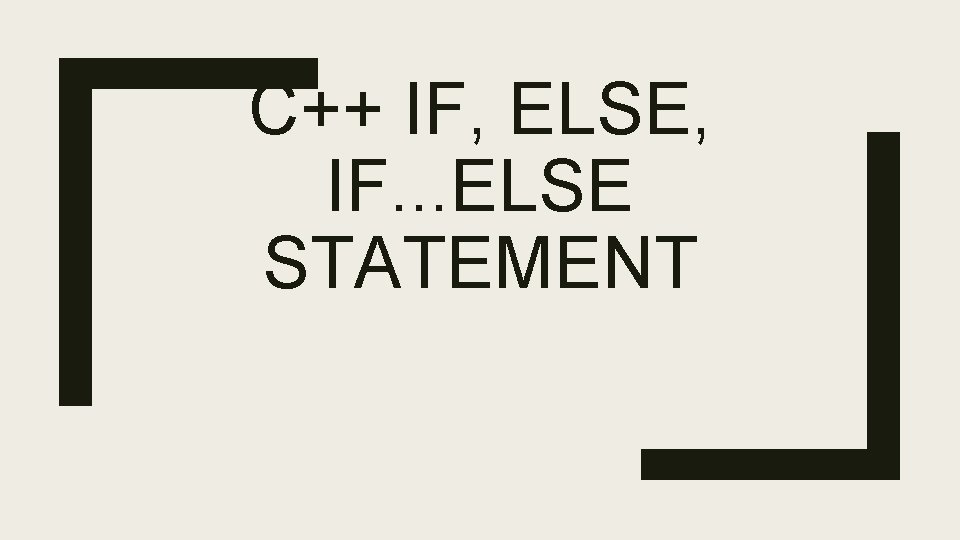
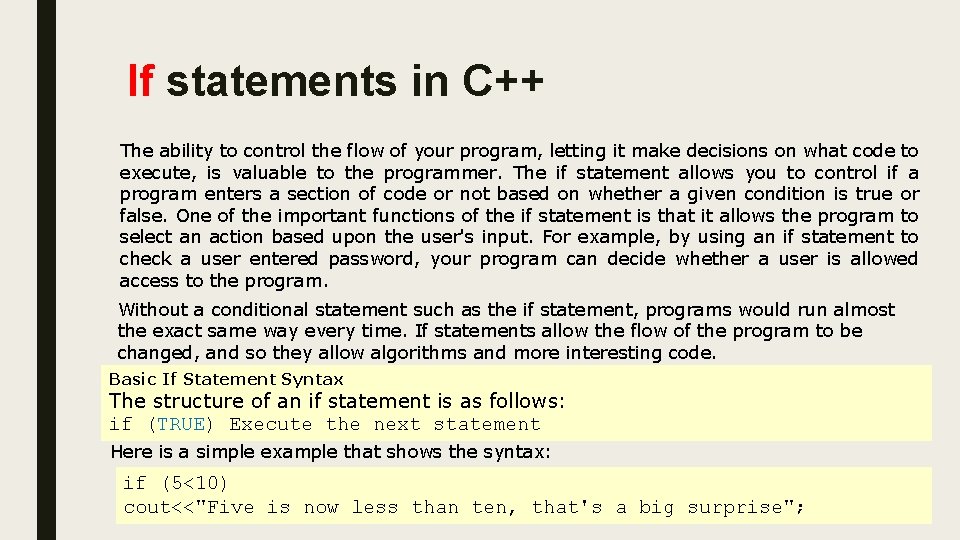
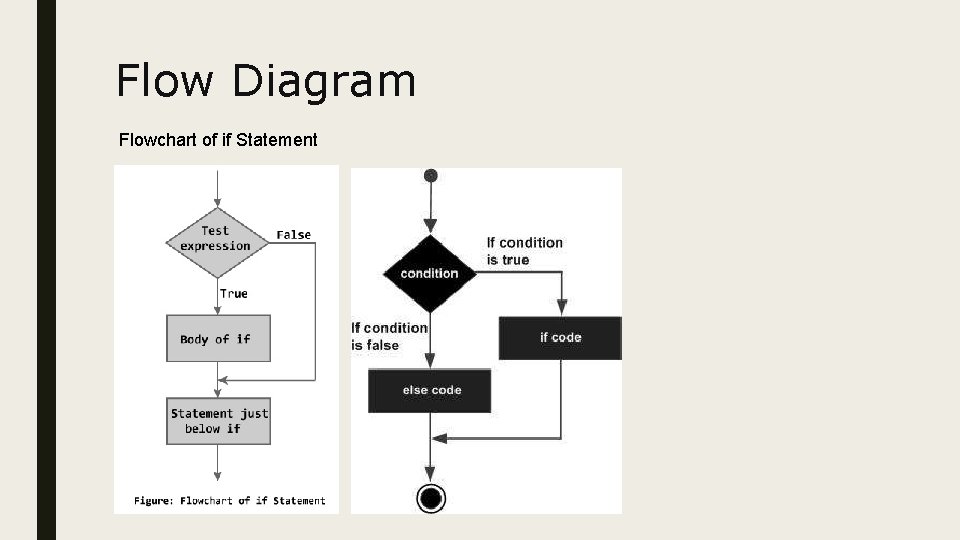
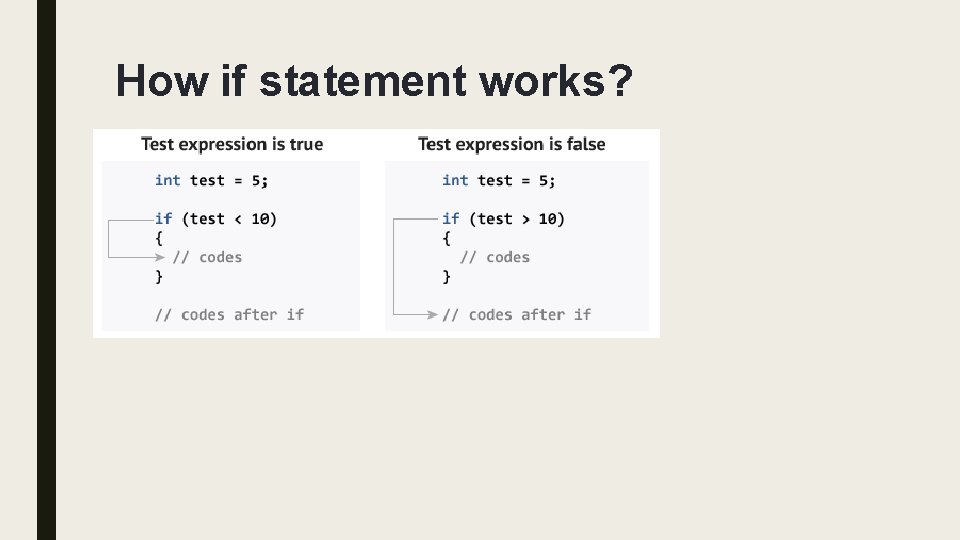
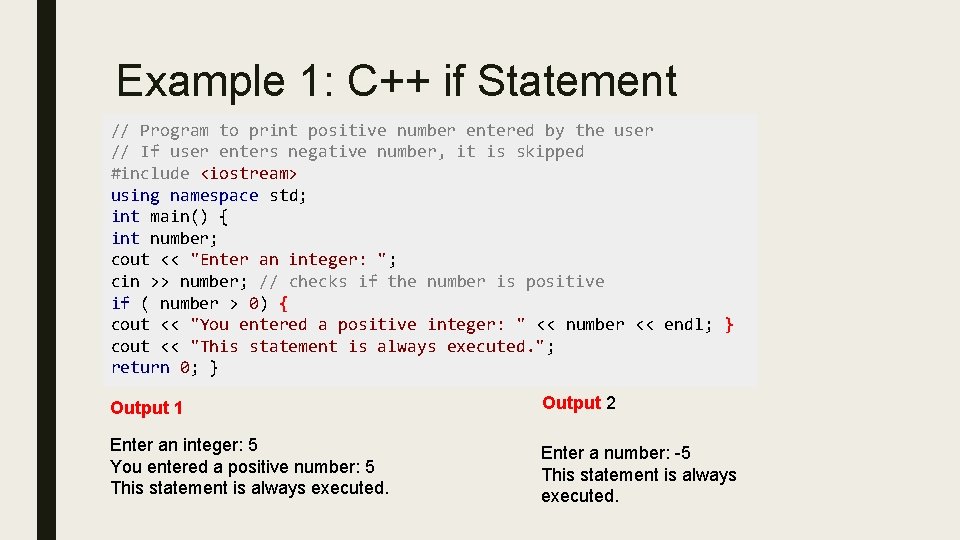
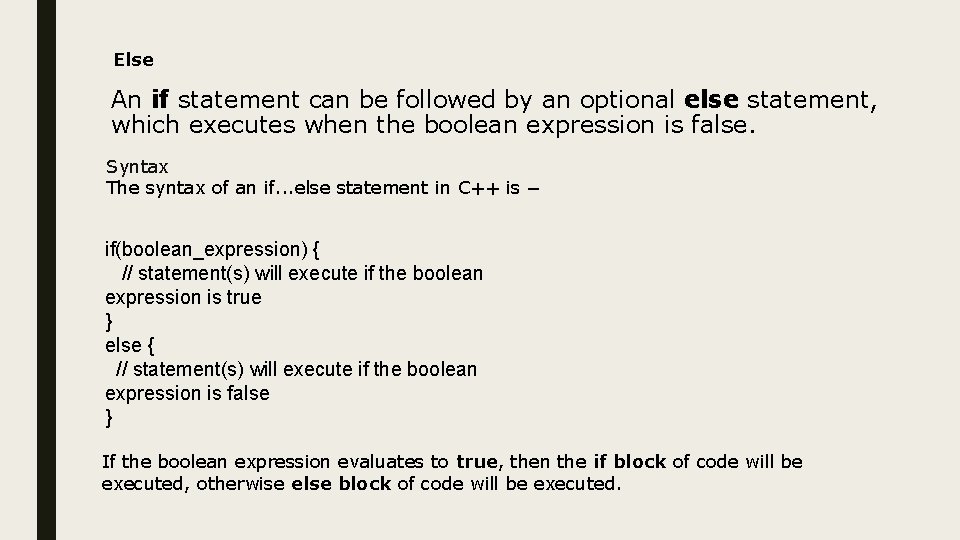
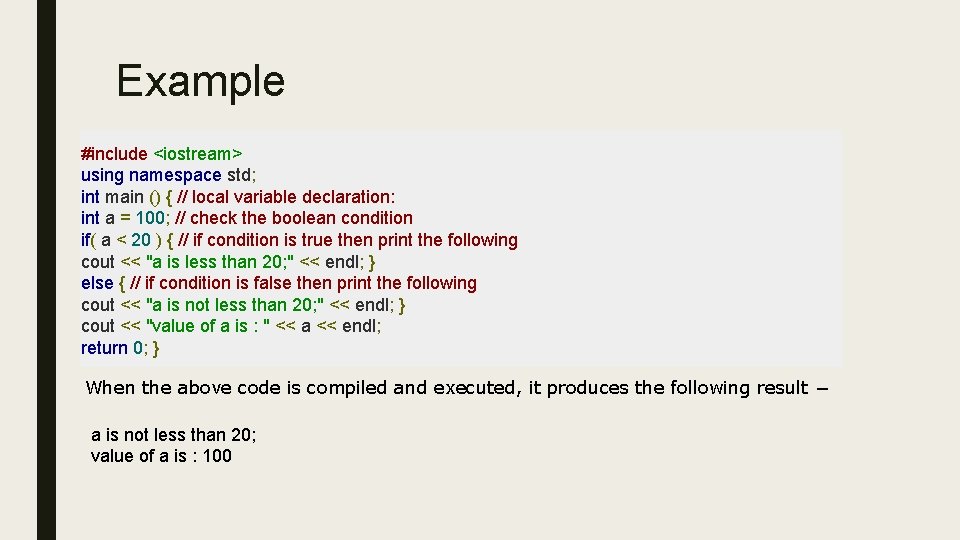
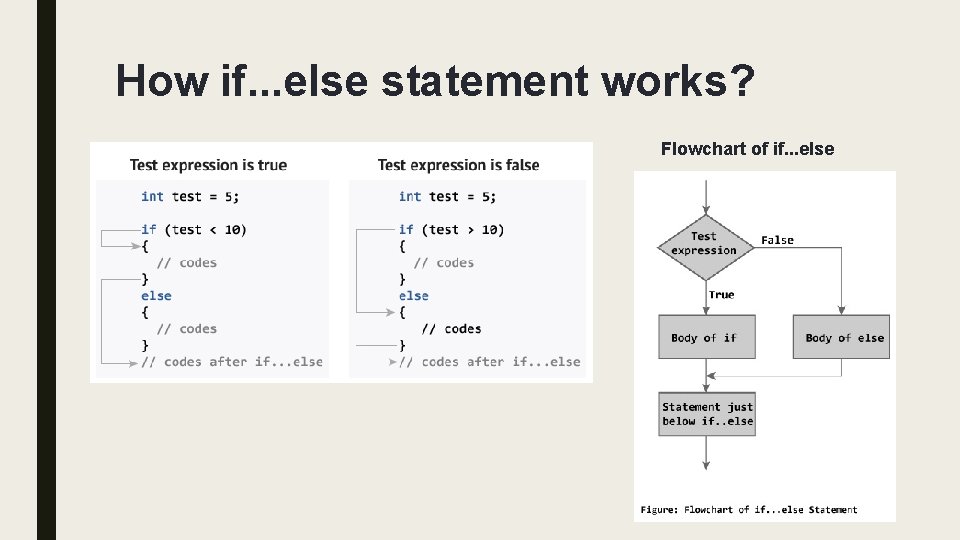
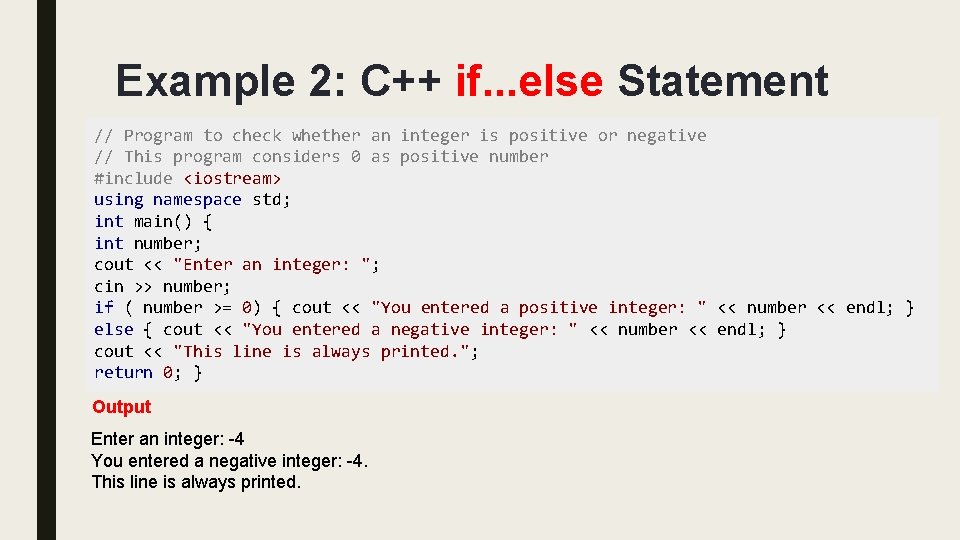
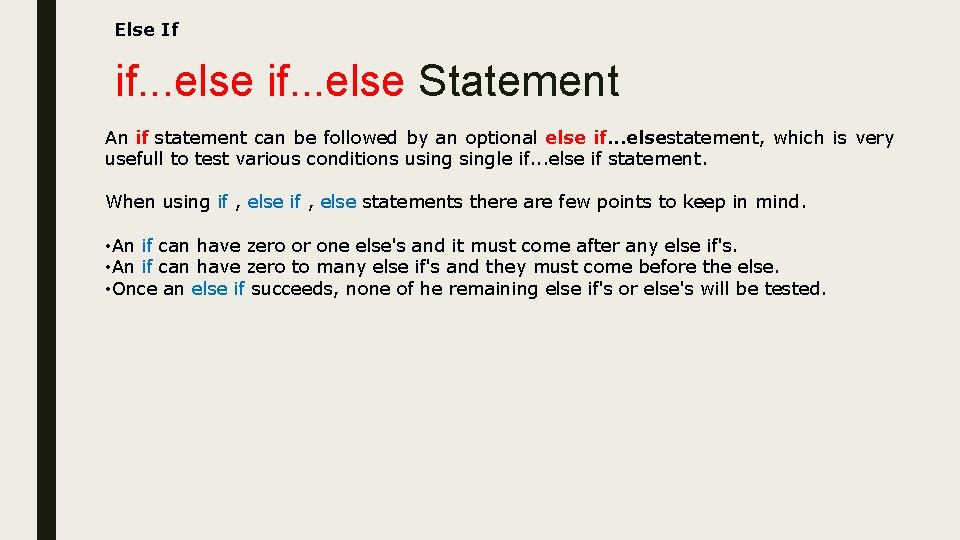
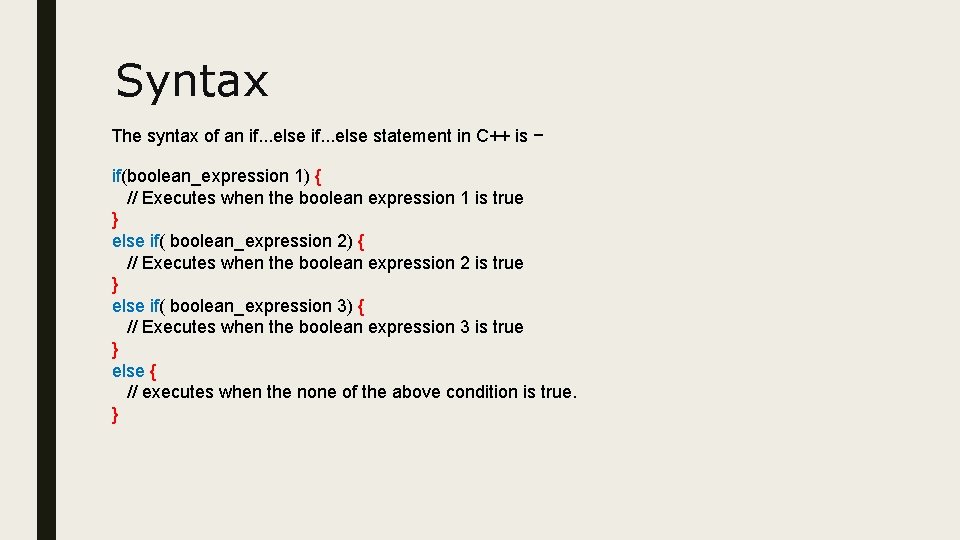
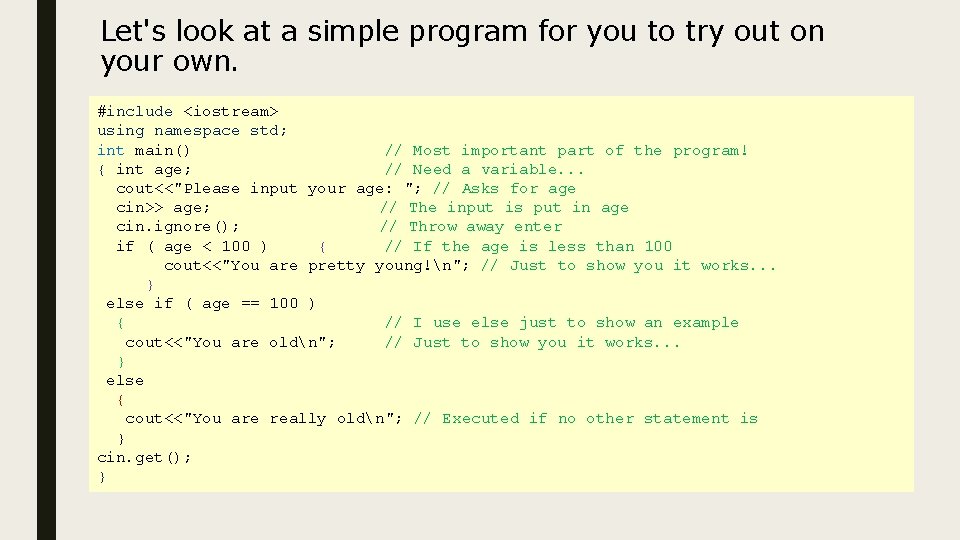
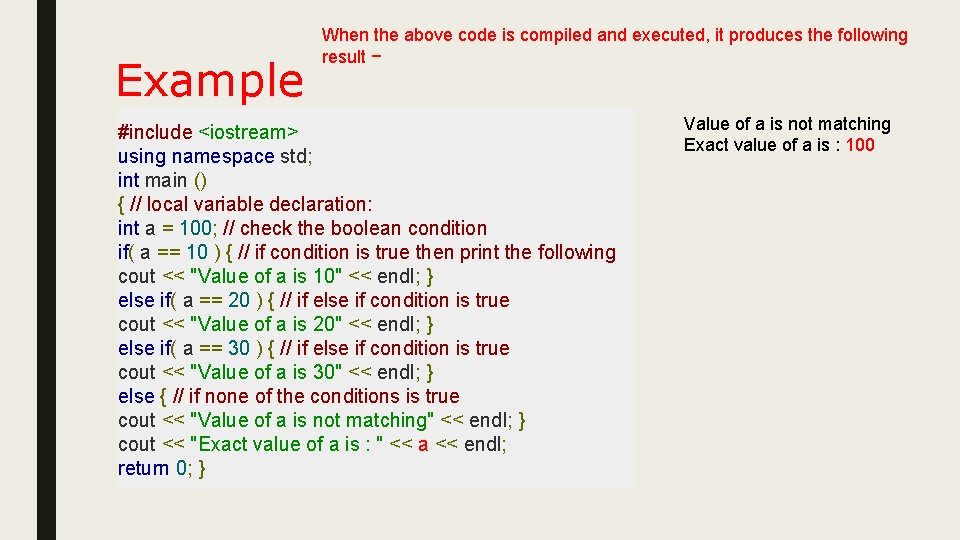
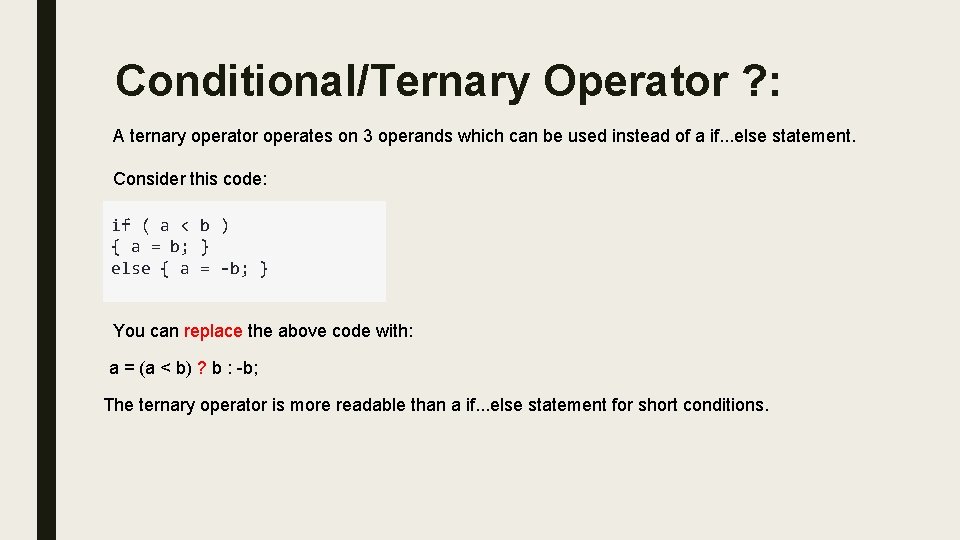
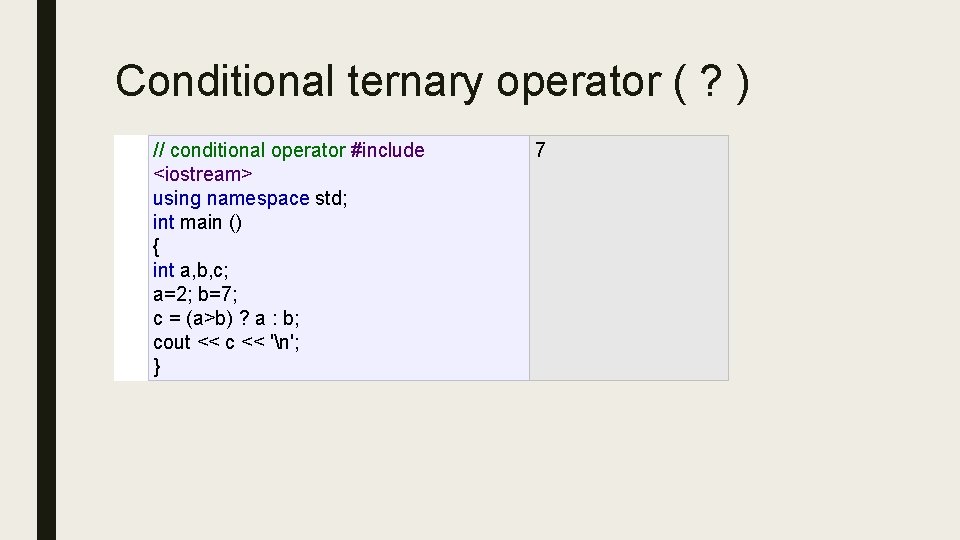
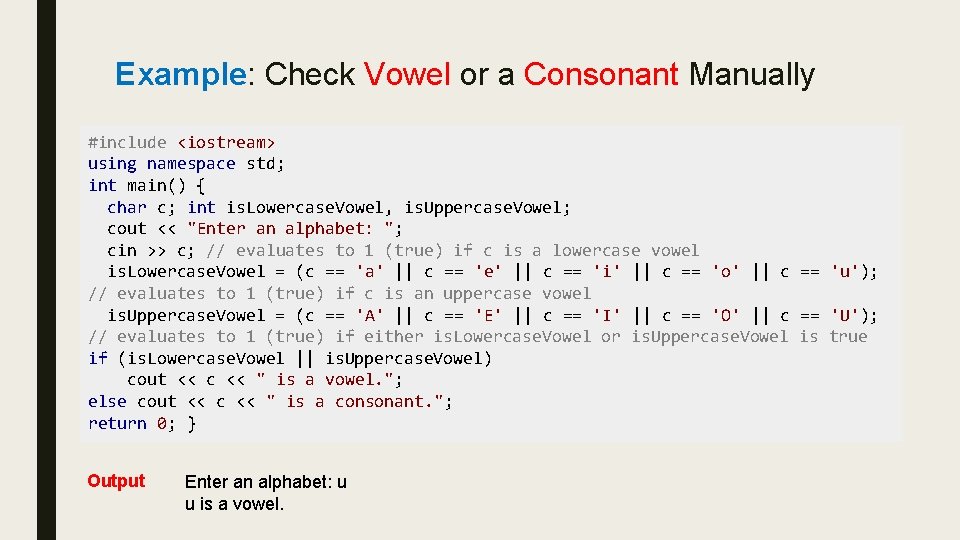
- Slides: 16
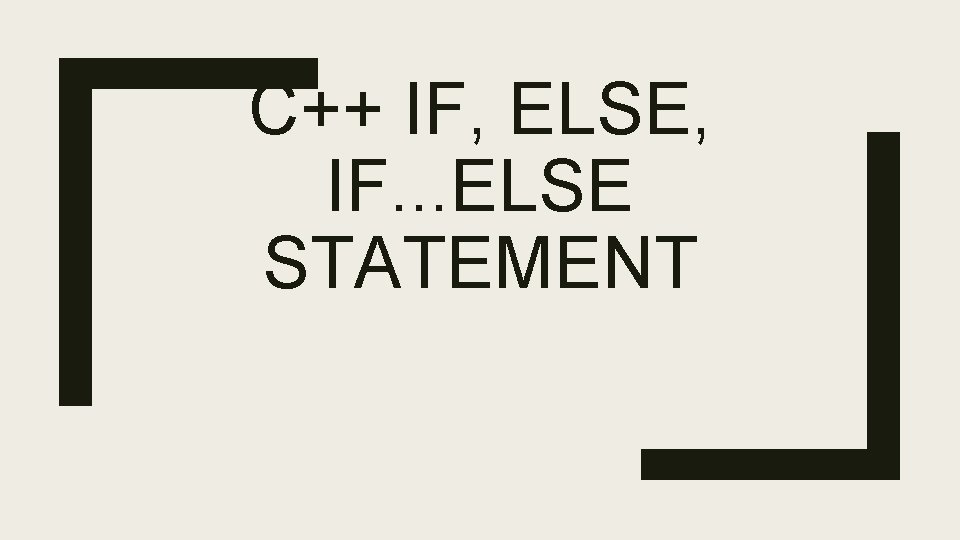
C++ IF, ELSE, IF. . . ELSE STATEMENT
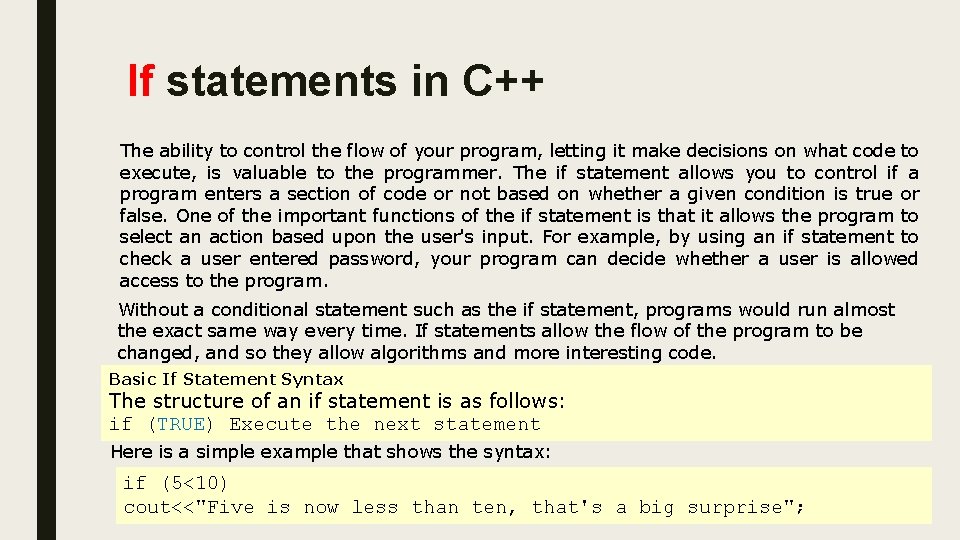
If statements in C++ The ability to control the flow of your program, letting it make decisions on what code to execute, is valuable to the programmer. The if statement allows you to control if a program enters a section of code or not based on whether a given condition is true or false. One of the important functions of the if statement is that it allows the program to select an action based upon the user's input. For example, by using an if statement to check a user entered password, your program can decide whether a user is allowed access to the program. Without a conditional statement such as the if statement, programs would run almost the exact same way every time. If statements allow the flow of the program to be changed, and so they allow algorithms and more interesting code. Basic If Statement Syntax The structure of an if statement is as follows: if (TRUE) Execute the next statement Here is a simple example that shows the syntax: if (5<10) cout<<"Five is now less than ten, that's a big surprise";
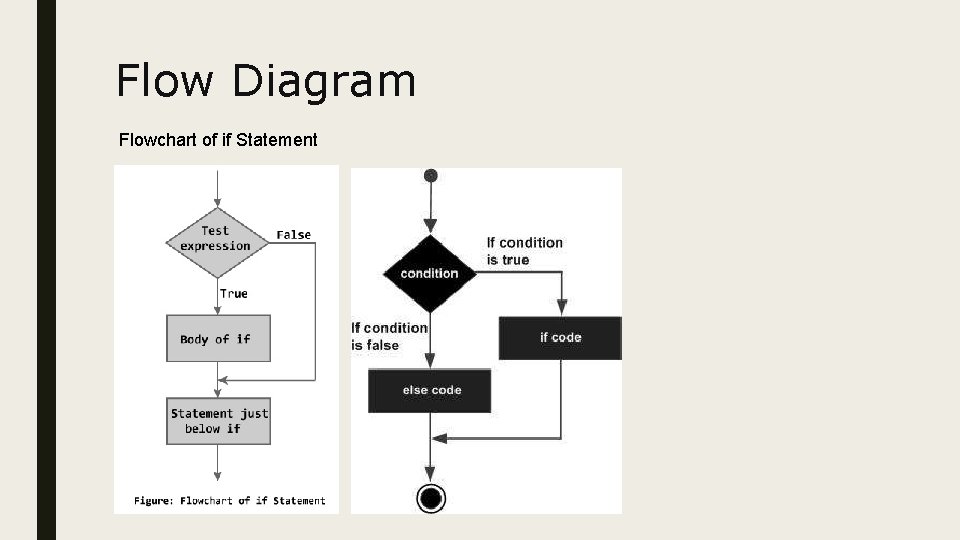
Flow Diagram Flowchart of if Statement
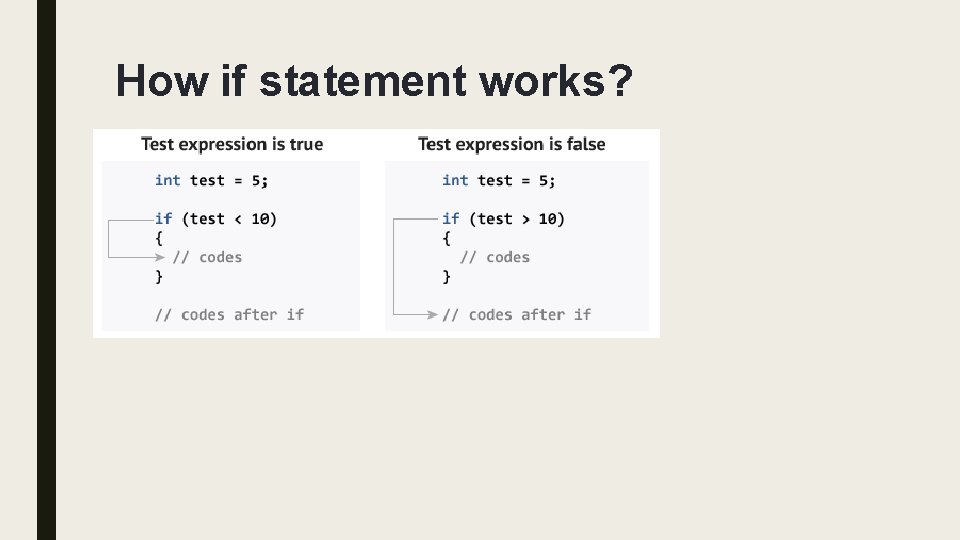
How if statement works?
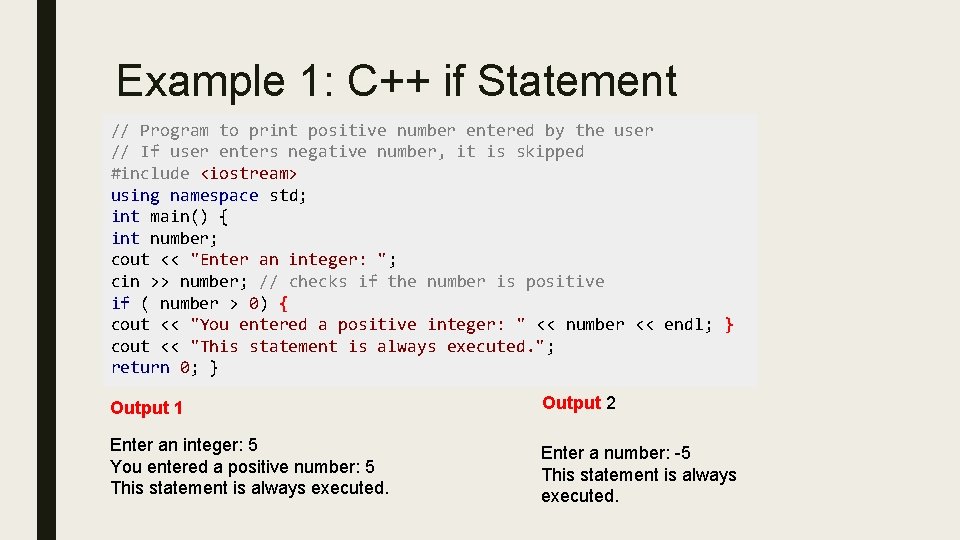
Example 1: C++ if Statement // Program to print positive number entered by the user // If user enters negative number, it is skipped #include <iostream> using namespace std; int main() { int number; cout << "Enter an integer: "; cin >> number; // checks if the number is positive if ( number > 0) { cout << "You entered a positive integer: " << number << endl; } cout << "This statement is always executed. "; return 0; } Output 1 Output 2 Enter an integer: 5 You entered a positive number: 5 This statement is always executed. Enter a number: -5 This statement is always executed.
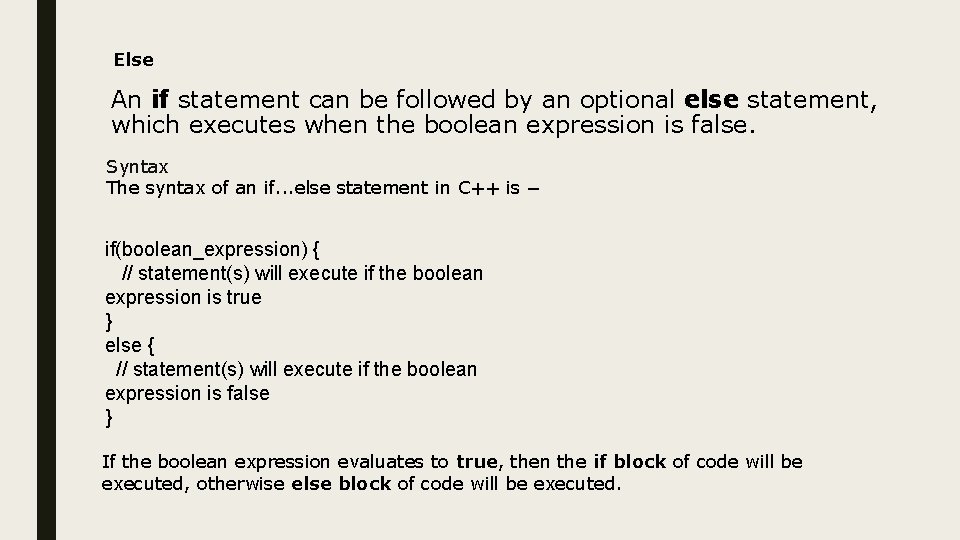
Else An if statement can be followed by an optional else statement, which executes when the boolean expression is false. Syntax The syntax of an if. . . else statement in C++ is − if(boolean_expression) { // statement(s) will execute if the boolean expression is true } else { // statement(s) will execute if the boolean expression is false } If the boolean expression evaluates to true, then the if block of code will be executed, otherwise else block of code will be executed.
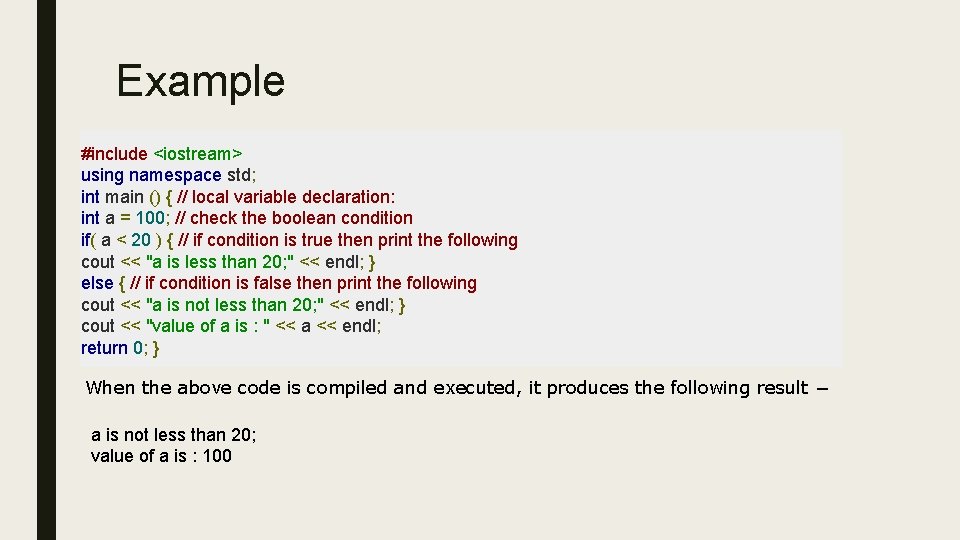
Example #include <iostream> using namespace std; int main () { // local variable declaration: int a = 100; // check the boolean condition if( a < 20 ) { // if condition is true then print the following cout << "a is less than 20; " << endl; } else { // if condition is false then print the following cout << "a is not less than 20; " << endl; } cout << "value of a is : " << a << endl; return 0; } When the above code is compiled and executed, it produces the following result − a is not less than 20; value of a is : 100
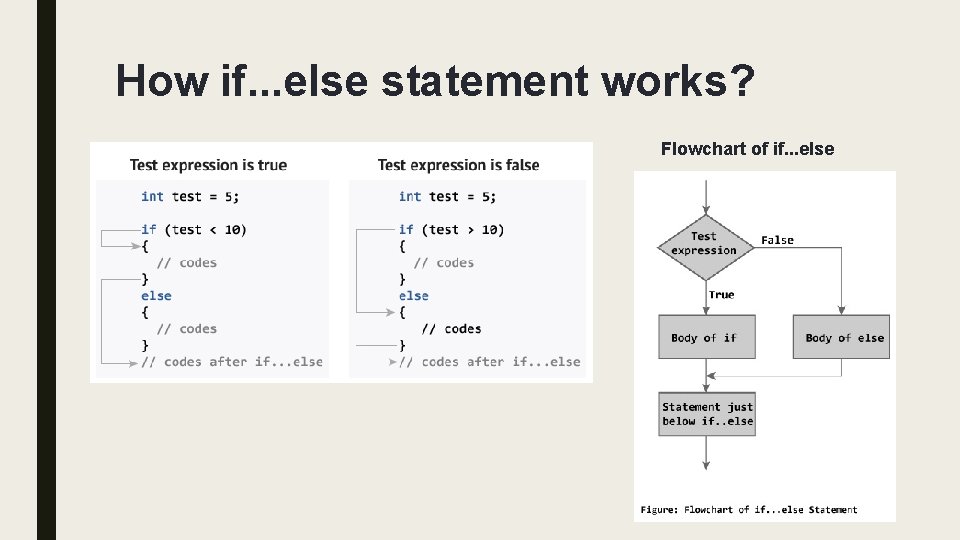
How if. . . else statement works? Flowchart of if. . . else
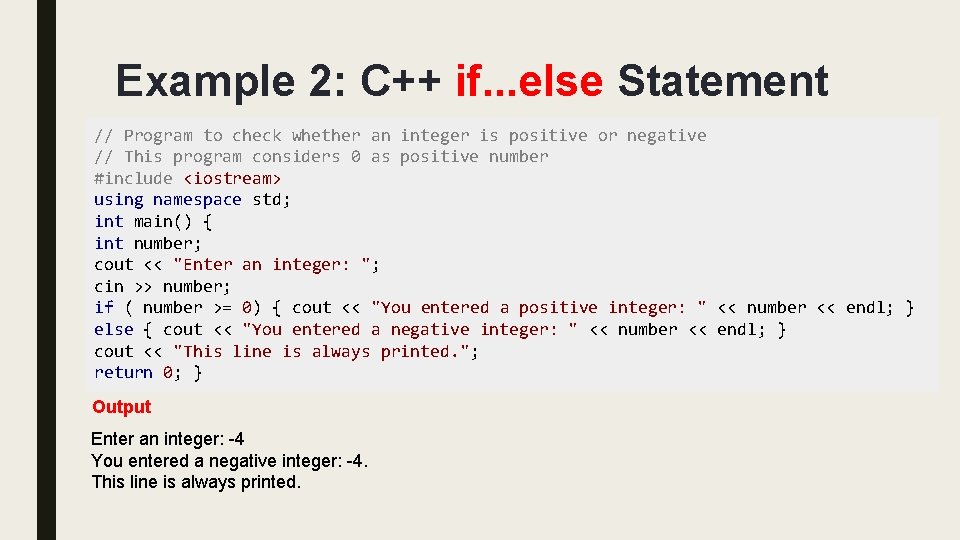
Example 2: C++ if. . . else Statement // Program to check whether an integer is positive or negative // This program considers 0 as positive number #include <iostream> using namespace std; int main() { int number; cout << "Enter an integer: "; cin >> number; if ( number >= 0) { cout << "You entered a positive integer: " << number << endl; } else { cout << "You entered a negative integer: " << number << endl; } cout << "This line is always printed. "; return 0; } Output Enter an integer: -4 You entered a negative integer: -4. This line is always printed.
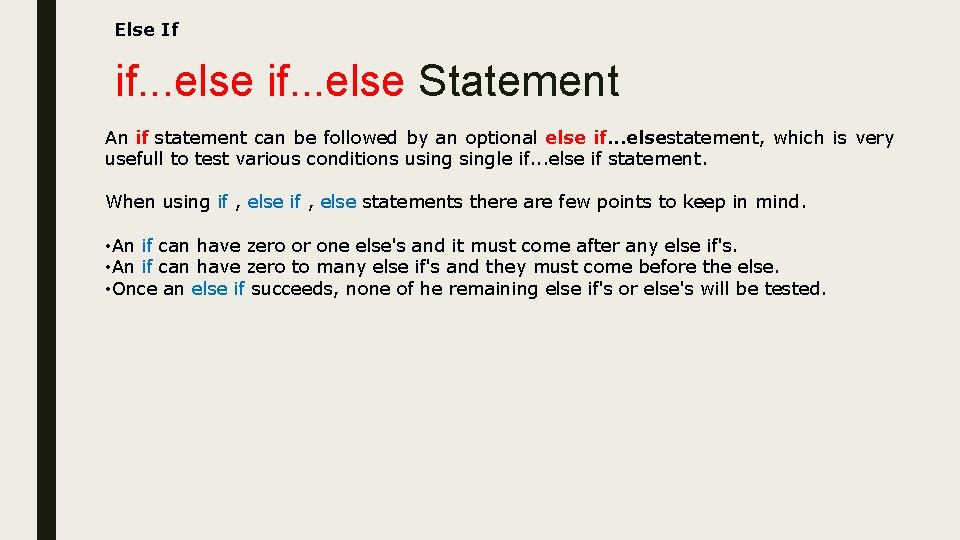
Else If if. . . else Statement An if statement can be followed by an optional else if. . . elsestatement, which is very usefull to test various conditions usingle if. . . else if statement. When using if , else statements there are few points to keep in mind. • An if can have zero or one else's and it must come after any else if's. • An if can have zero to many else if's and they must come before the else. • Once an else if succeeds, none of he remaining else if's or else's will be tested.
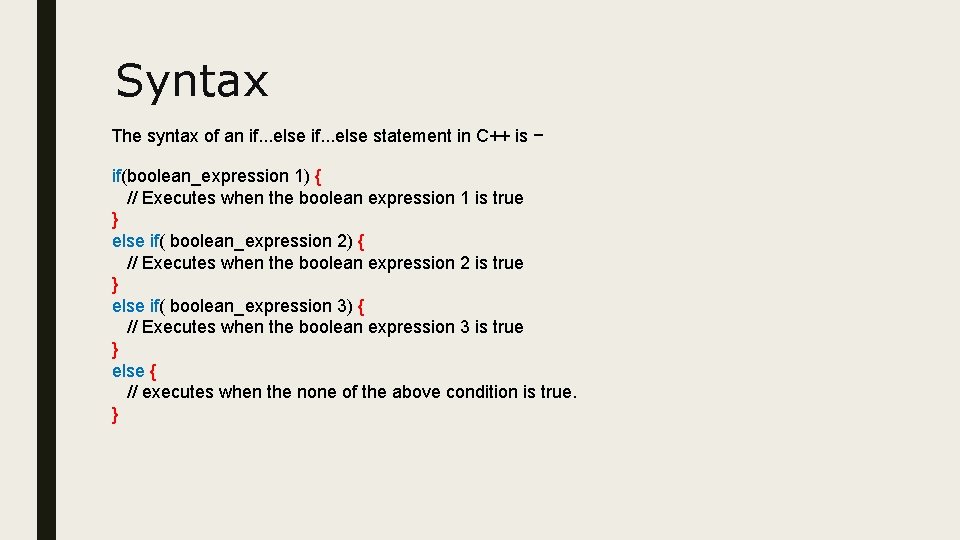
Syntax The syntax of an if. . . else statement in C++ is − if(boolean_expression 1) { // Executes when the boolean expression 1 is true } else if( boolean_expression 2) { // Executes when the boolean expression 2 is true } else if( boolean_expression 3) { // Executes when the boolean expression 3 is true } else { // executes when the none of the above condition is true. }
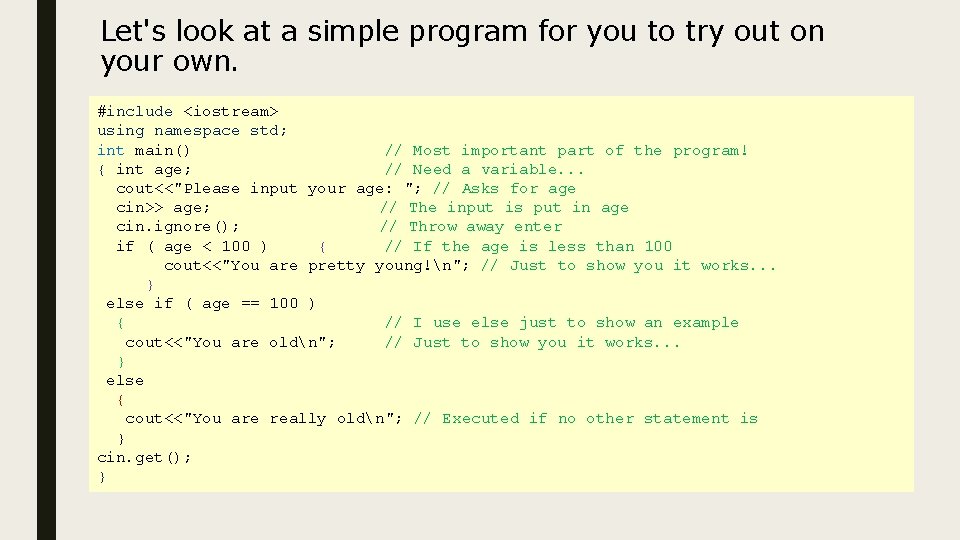
Let's look at a simple program for you to try out on your own. #include <iostream> using namespace std; int main() // Most important part of the program! { int age; // Need a variable. . . cout<<"Please input your age: "; // Asks for age cin>> age; // The input is put in age cin. ignore(); // Throw away enter if ( age < 100 ) { // If the age is less than 100 cout<<"You are pretty young!n"; // Just to show you it works. . . } else if ( age == 100 ) { // I use else just to show an example cout<<"You are oldn"; // Just to show you it works. . . } else { cout<<"You are really oldn"; // Executed if no other statement is } cin. get(); }
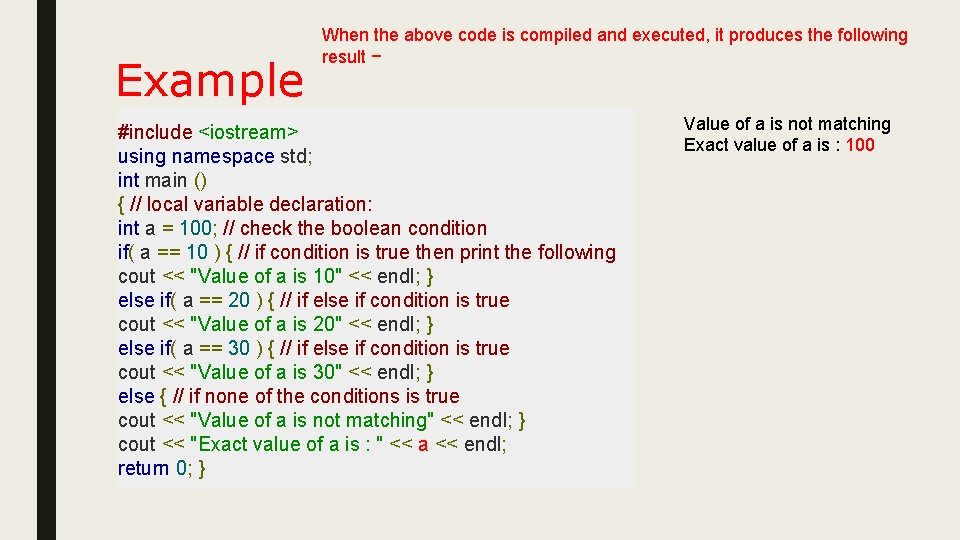
Example When the above code is compiled and executed, it produces the following result − #include <iostream> using namespace std; int main () { // local variable declaration: int a = 100; // check the boolean condition if( a == 10 ) { // if condition is true then print the following cout << "Value of a is 10" << endl; } else if( a == 20 ) { // if else if condition is true cout << "Value of a is 20" << endl; } else if( a == 30 ) { // if else if condition is true cout << "Value of a is 30" << endl; } else { // if none of the conditions is true cout << "Value of a is not matching" << endl; } cout << "Exact value of a is : " << a << endl; return 0; } Value of a is not matching Exact value of a is : 100
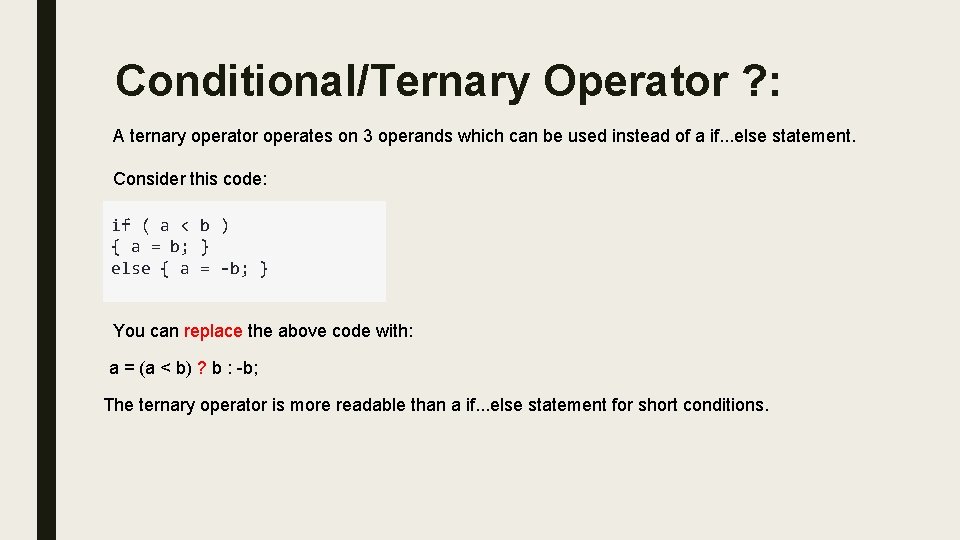
Conditional/Ternary Operator ? : A ternary operator operates on 3 operands which can be used instead of a if. . . else statement. Consider this code: if ( a < b ) { a = b; } else { a = -b; } You can replace the above code with: a = (a < b) ? b : -b; The ternary operator is more readable than a if. . . else statement for short conditions.
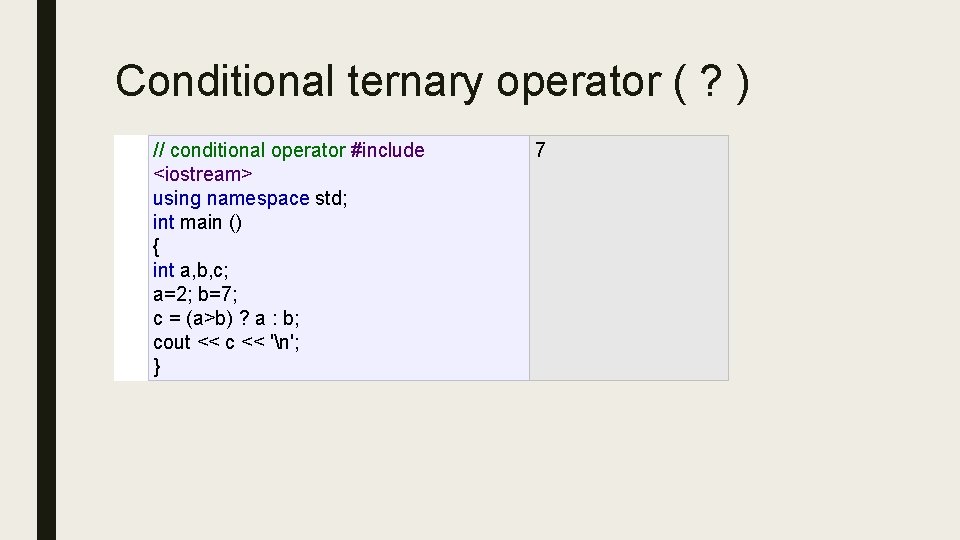
Conditional ternary operator ( ? ) // conditional operator #include <iostream> using namespace std; int main () { int a, b, c; a=2; b=7; c = (a>b) ? a : b; cout << c << 'n'; } 7
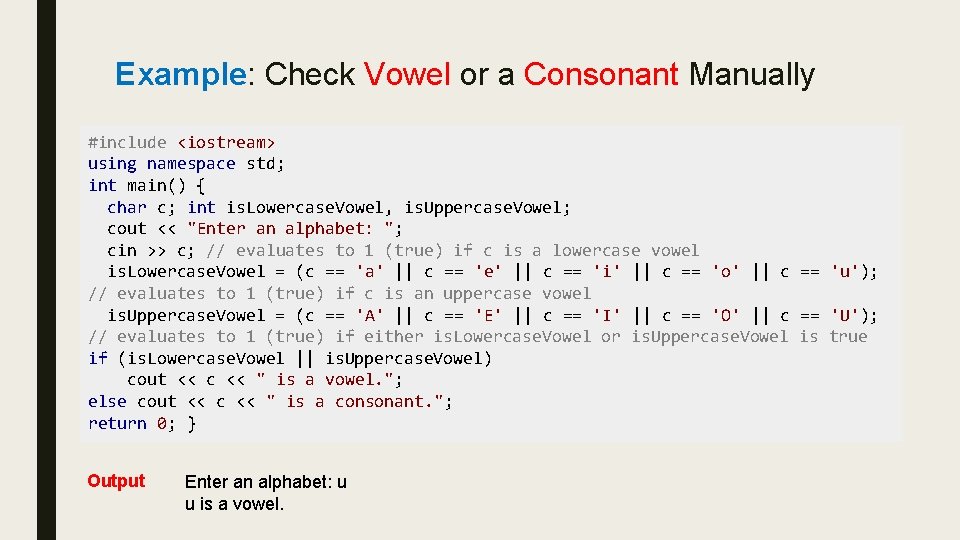
Example: Check Vowel or a Consonant Manually #include <iostream> using namespace std; int main() { char c; int is. Lowercase. Vowel, is. Uppercase. Vowel; cout << "Enter an alphabet: "; cin >> c; // evaluates to 1 (true) if c is a lowercase vowel is. Lowercase. Vowel = (c == 'a' || c == 'e' || c == 'i' || c == 'o' || c == 'u'); // evaluates to 1 (true) if c is an uppercase vowel is. Uppercase. Vowel = (c == 'A' || c == 'E' || c == 'I' || c == 'O' || c == 'U'); // evaluates to 1 (true) if either is. Lowercase. Vowel or is. Uppercase. Vowel is true if (is. Lowercase. Vowel || is. Uppercase. Vowel) cout << c << " is a vowel. "; else cout << c << " is a consonant. "; return 0; } Output Enter an alphabet: u u is a vowel.
His bloody life in my bloody hands
Are switch statements faster than if/else
Flowchart else if
What is nested if else statement in c
Grammar vs syntax vs semantics
If in assembly 8086
Else statement
If else statement robotc
Flowchart for if else statement
Mips assembly if statement
Loop instruction in 8086 with example
Ibm cognos if then else statement examples
Rise and shine algorithm
Asymptotic analysis
What is nested if else statement in c
Assembly jumps
Picoblaze instruction set