CONST keyword this pointer OPERATOR OVERLOADING CS 104
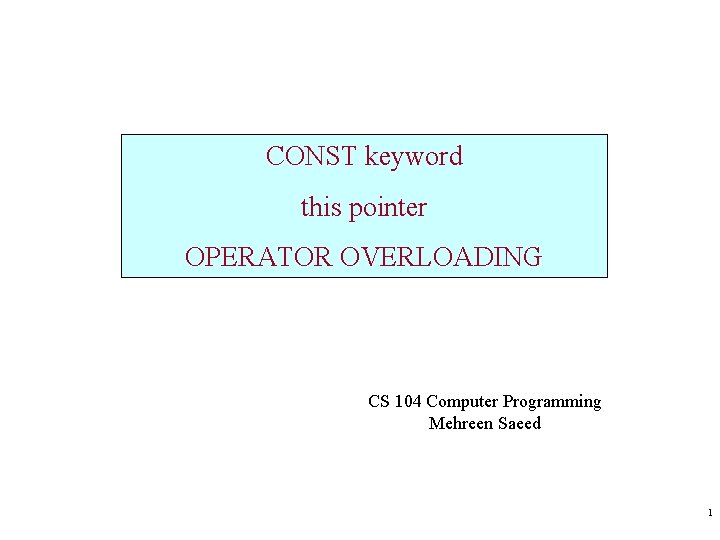
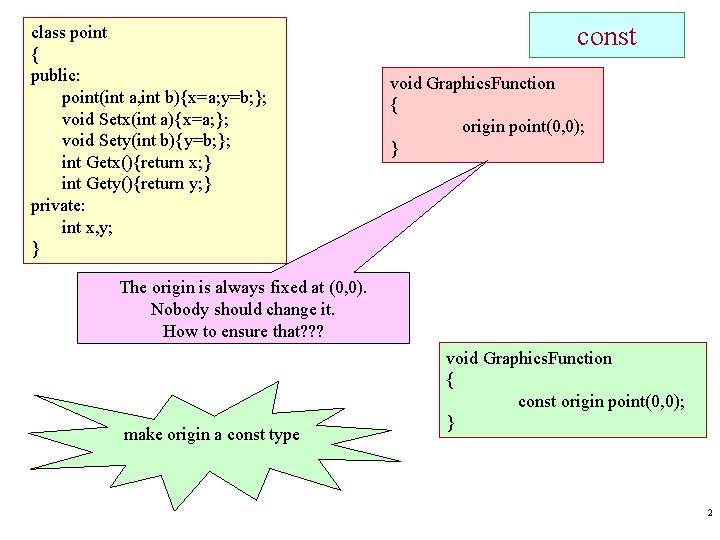
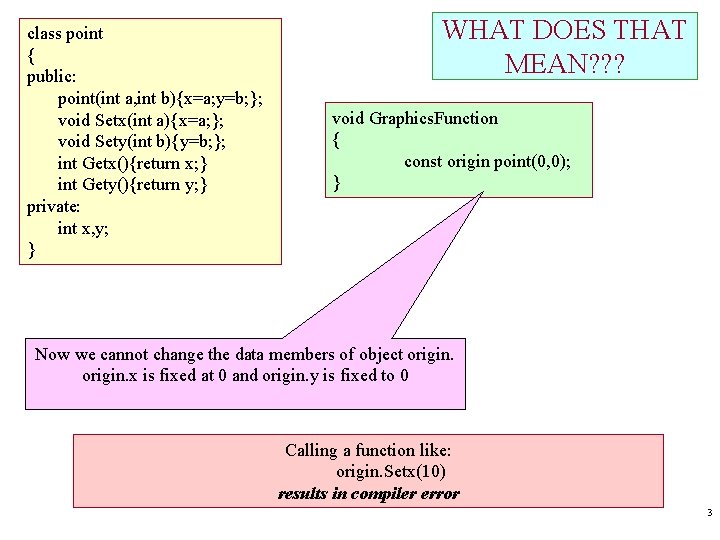
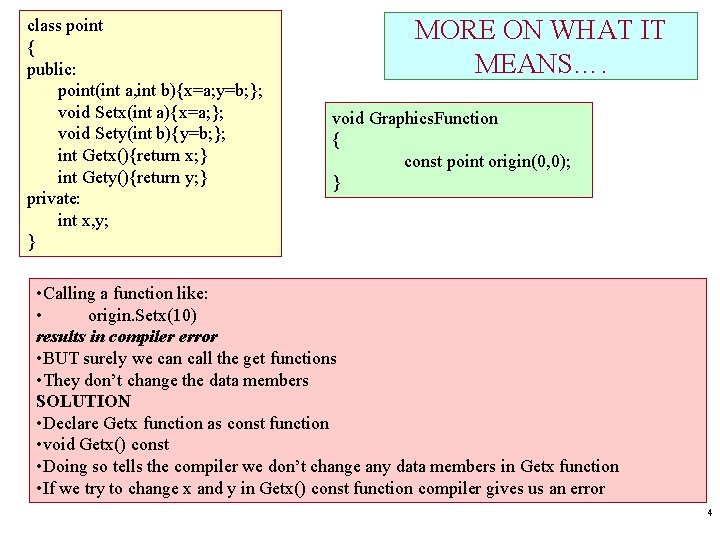
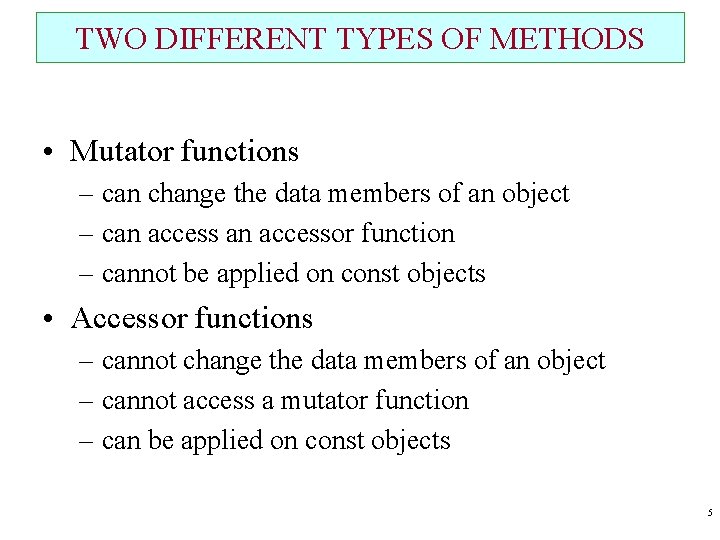
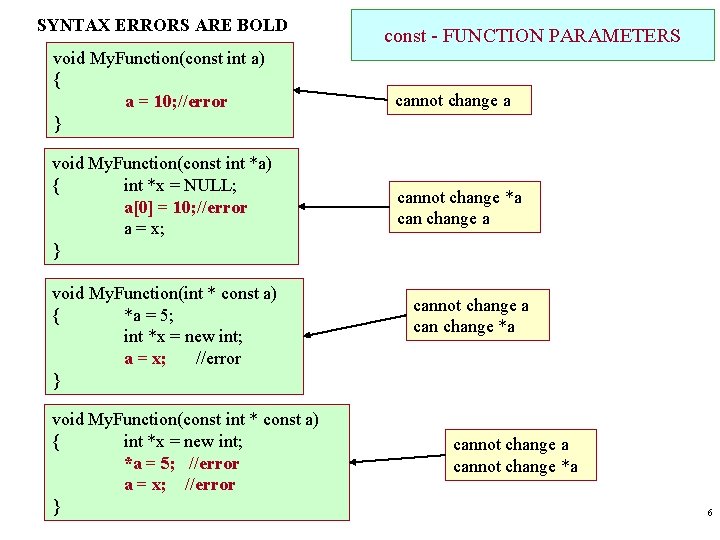
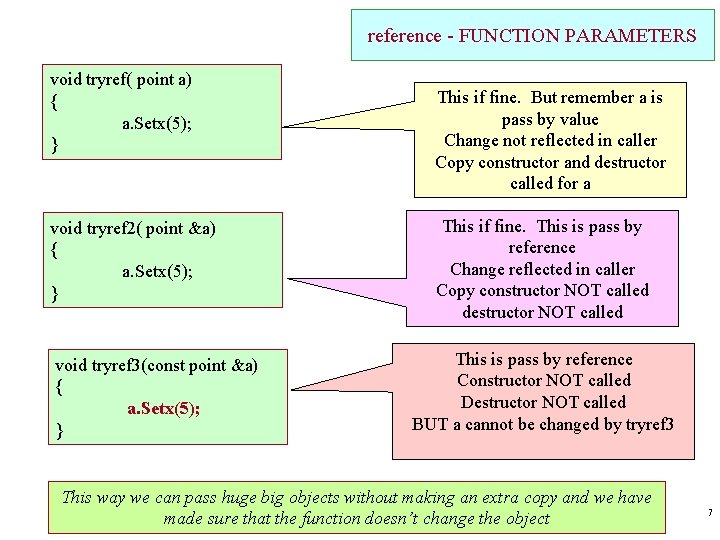
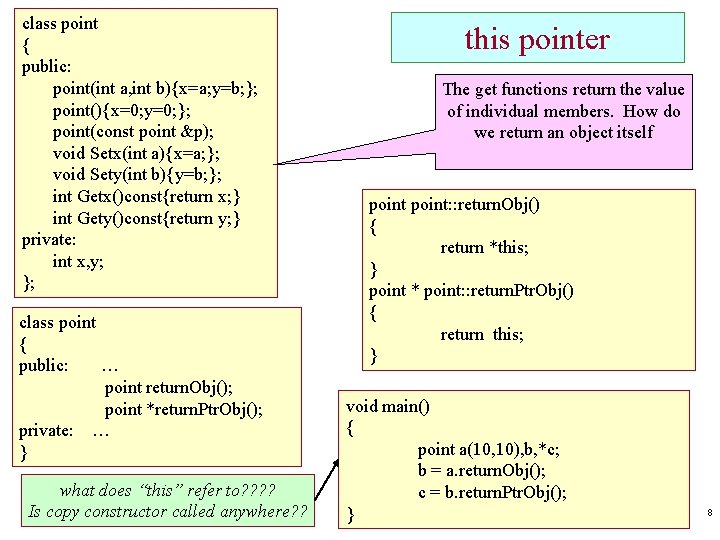
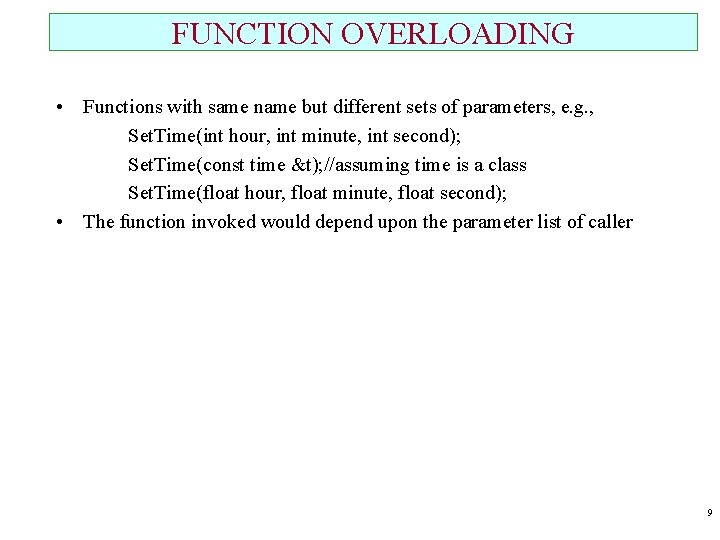
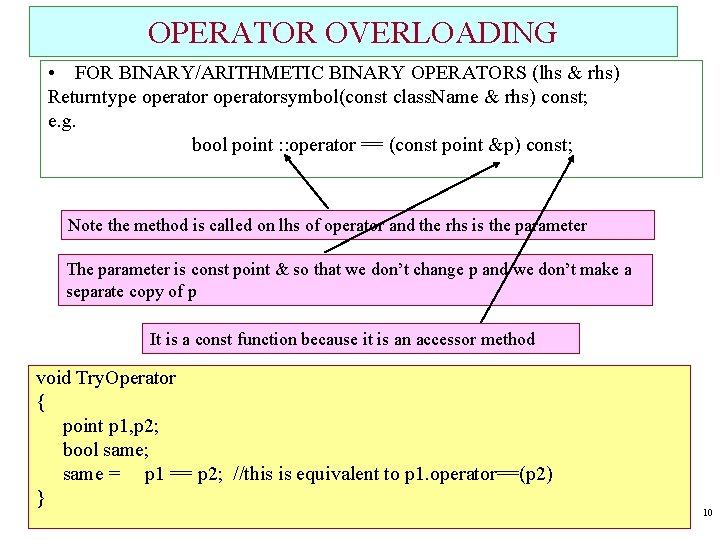
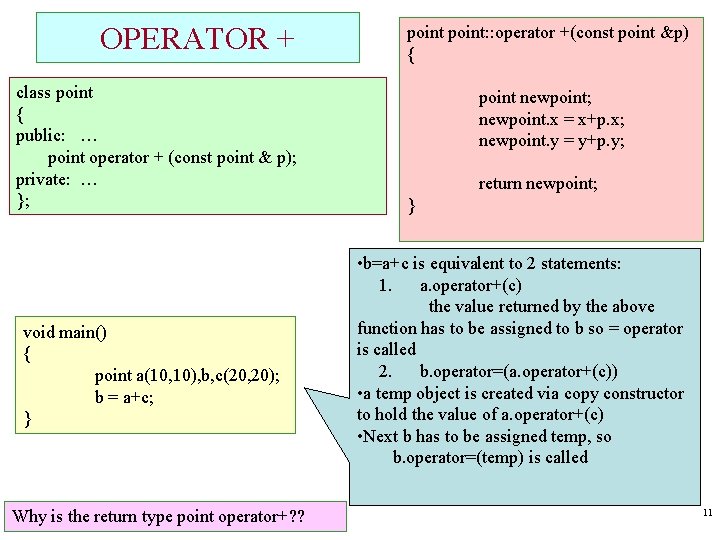
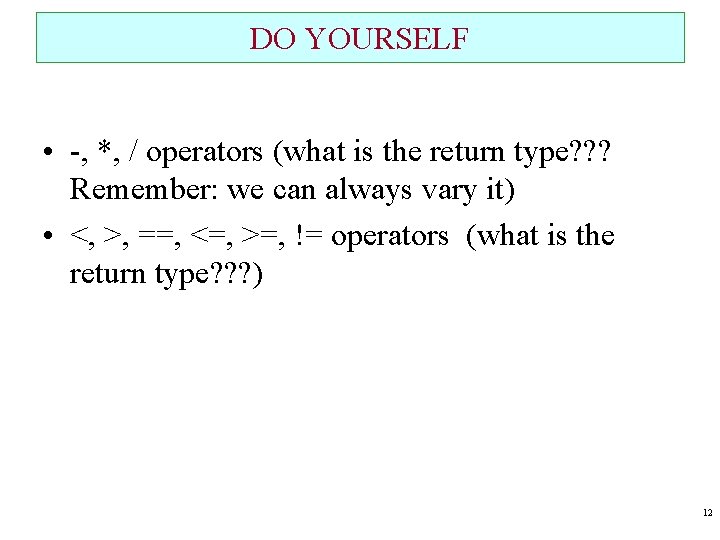
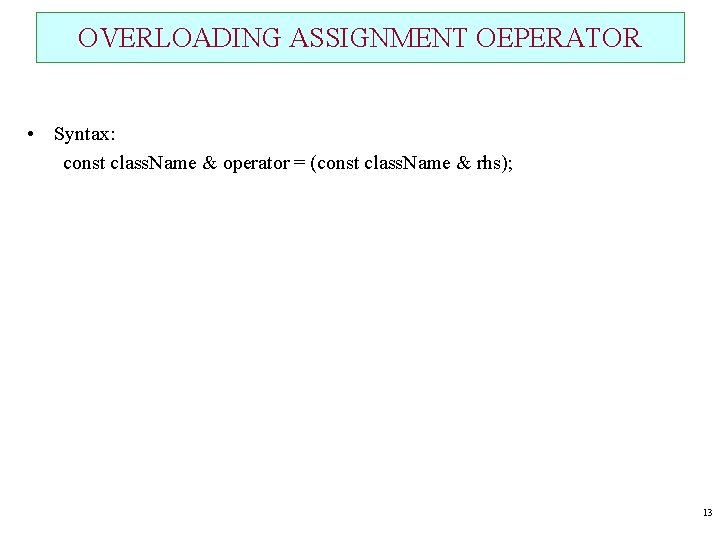
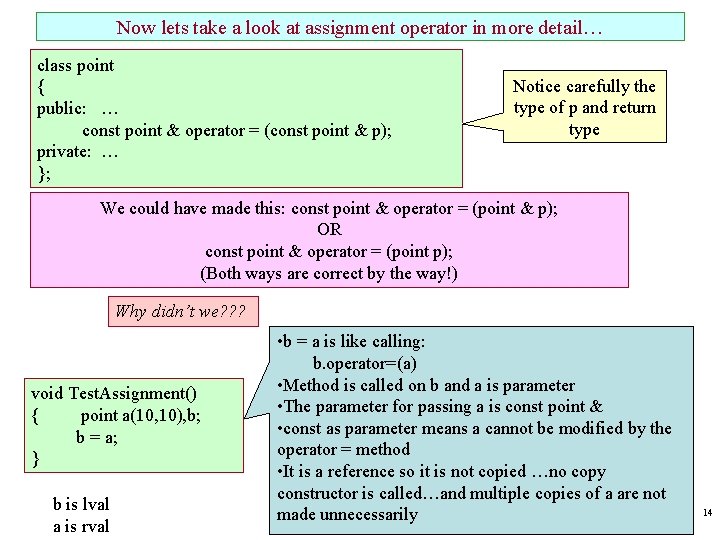
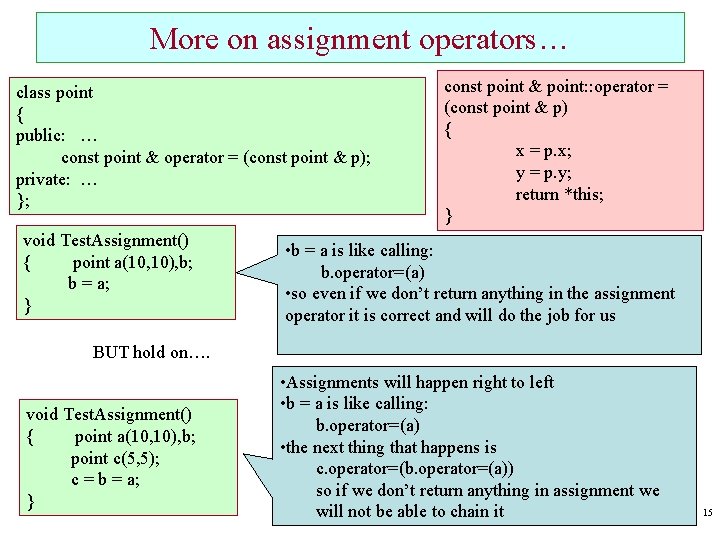
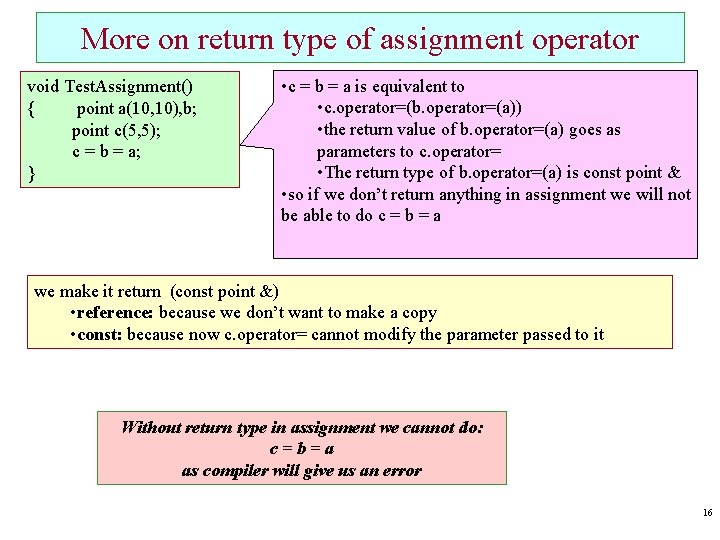
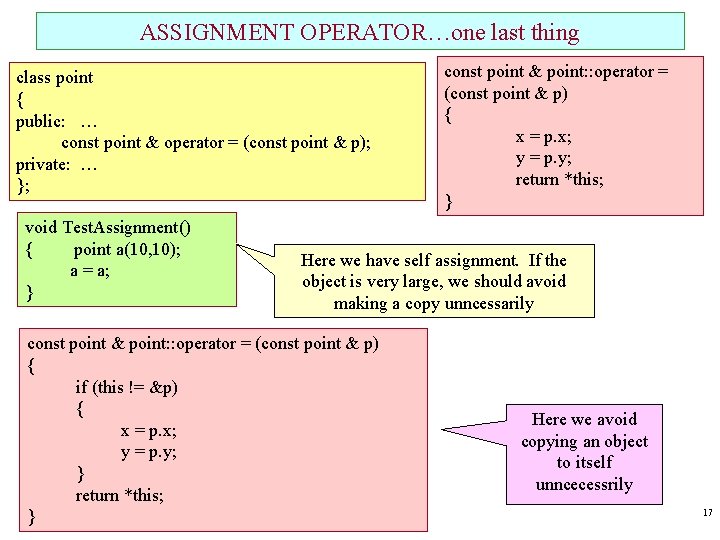
- Slides: 17
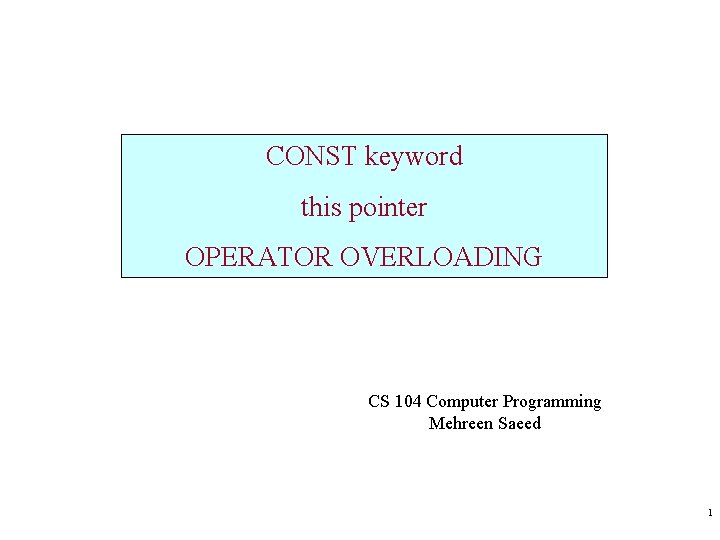
CONST keyword this pointer OPERATOR OVERLOADING CS 104 Computer Programming Mehreen Saeed 1
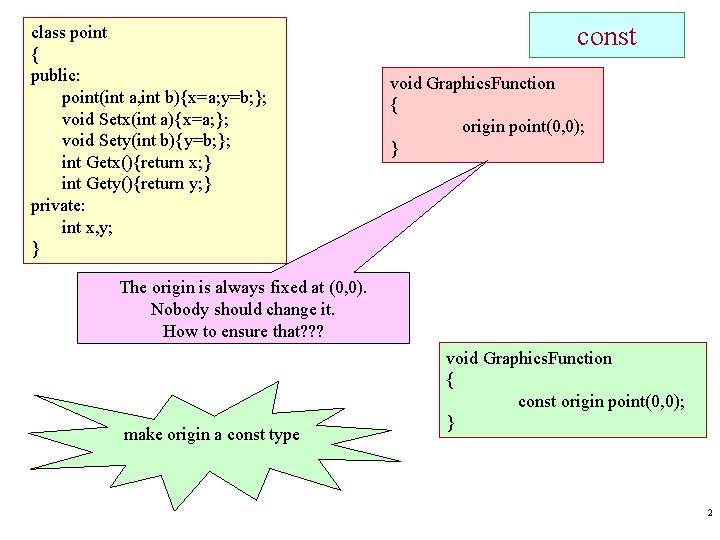
class point { public: point(int a, int b){x=a; y=b; }; void Setx(int a){x=a; }; void Sety(int b){y=b; }; int Getx(){return x; } int Gety(){return y; } private: int x, y; } const void Graphics. Function { origin point(0, 0); } The origin is always fixed at (0, 0). Nobody should change it. How to ensure that? ? ? make origin a const type void Graphics. Function { const origin point(0, 0); } 2
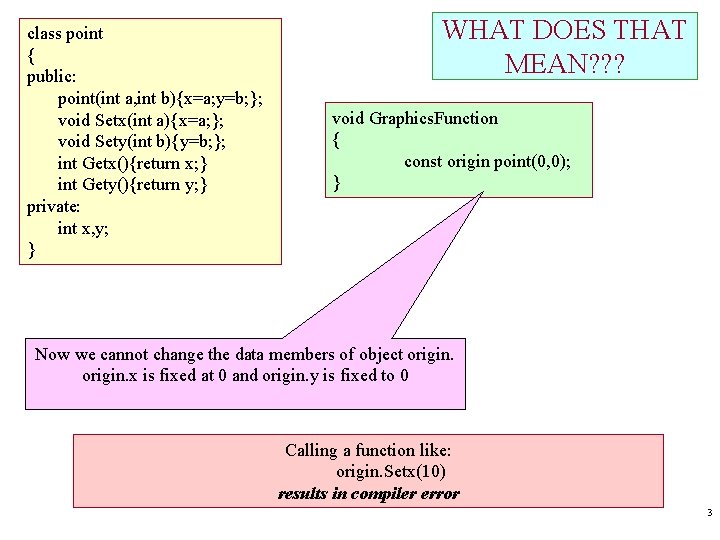
class point { public: point(int a, int b){x=a; y=b; }; void Setx(int a){x=a; }; void Sety(int b){y=b; }; int Getx(){return x; } int Gety(){return y; } private: int x, y; } WHAT DOES THAT MEAN? ? ? void Graphics. Function { const origin point(0, 0); } Now we cannot change the data members of object origin. x is fixed at 0 and origin. y is fixed to 0 Calling a function like: origin. Setx(10) results in compiler error 3
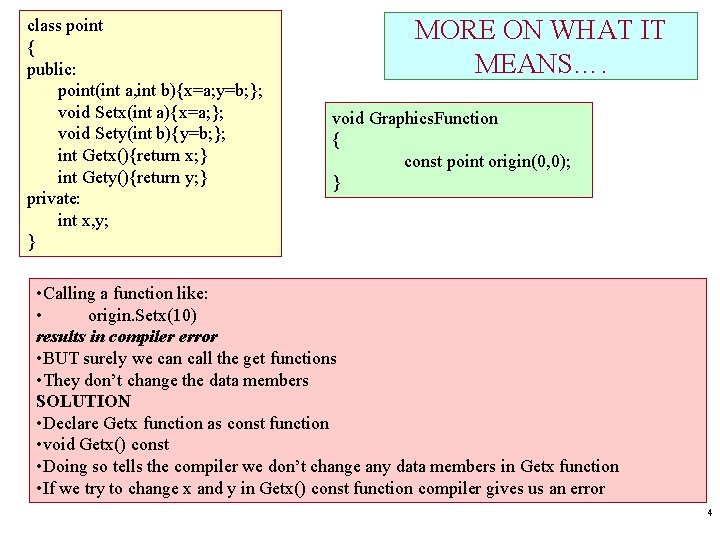
class point { public: point(int a, int b){x=a; y=b; }; void Setx(int a){x=a; }; void Sety(int b){y=b; }; int Getx(){return x; } int Gety(){return y; } private: int x, y; } MORE ON WHAT IT MEANS…. void Graphics. Function { const point origin(0, 0); } • Calling a function like: • origin. Setx(10) results in compiler error • BUT surely we can call the get functions • They don’t change the data members SOLUTION • Declare Getx function as const function • void Getx() const • Doing so tells the compiler we don’t change any data members in Getx function • If we try to change x and y in Getx() const function compiler gives us an error 4
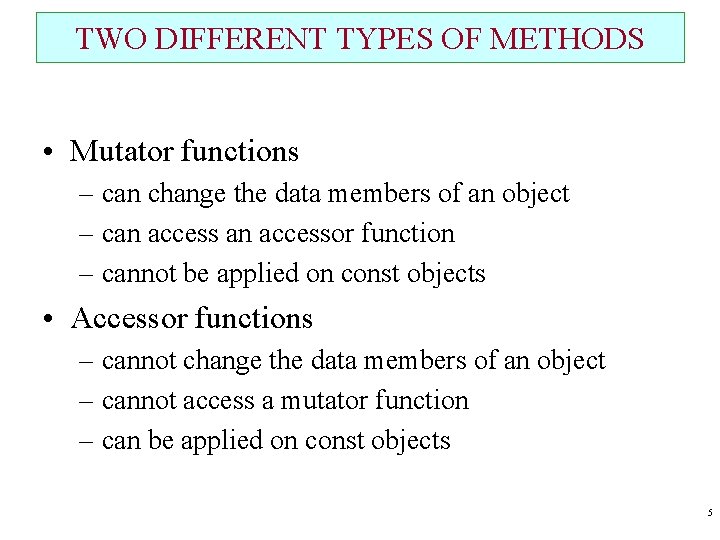
TWO DIFFERENT TYPES OF METHODS • Mutator functions – can change the data members of an object – can accessor function – cannot be applied on const objects • Accessor functions – cannot change the data members of an object – cannot access a mutator function – can be applied on const objects 5
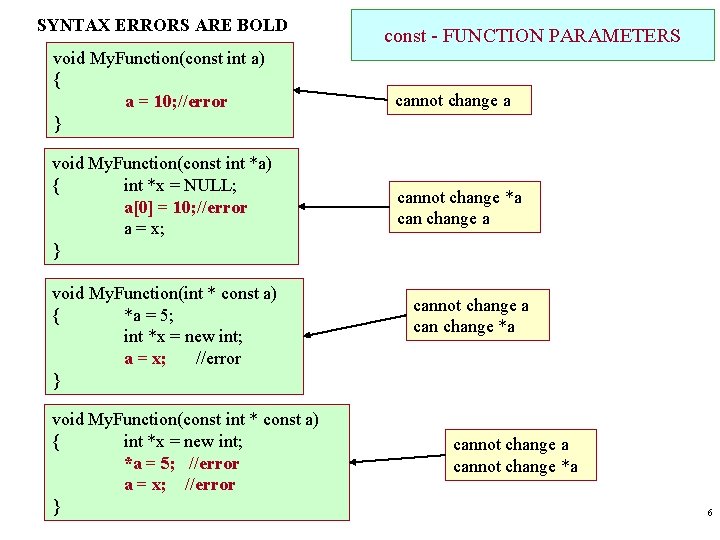
SYNTAX ERRORS ARE BOLD void My. Function(const int a) { a = 10; //error } void My. Function(const int *a) { int *x = NULL; a[0] = 10; //error a = x; } void My. Function(int * const a) { *a = 5; int *x = new int; a = x; //error } void My. Function(const int * const a) { int *x = new int; *a = 5; //error a = x; //error } const - FUNCTION PARAMETERS cannot change a cannot change *a can change a cannot change a can change *a cannot change *a 6
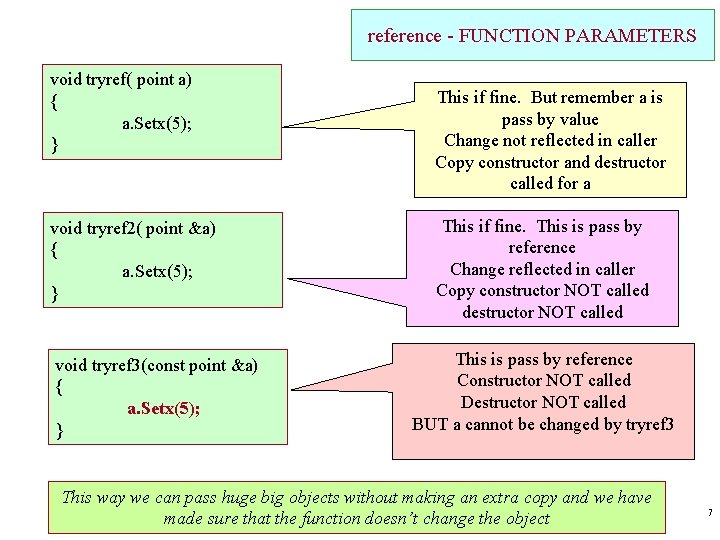
reference - FUNCTION PARAMETERS void tryref( point a) { a. Setx(5); } void tryref 2( point &a) { a. Setx(5); } void tryref 3(const point &a) { a. Setx(5); } This if fine. But remember a is pass by value Change not reflected in caller Copy constructor and destructor called for a This if fine. This is pass by reference Change reflected in caller Copy constructor NOT called destructor NOT called This is pass by reference Constructor NOT called Destructor NOT called BUT a cannot be changed by tryref 3 This way we can pass huge big objects without making an extra copy and we have made sure that the function doesn’t change the object 7
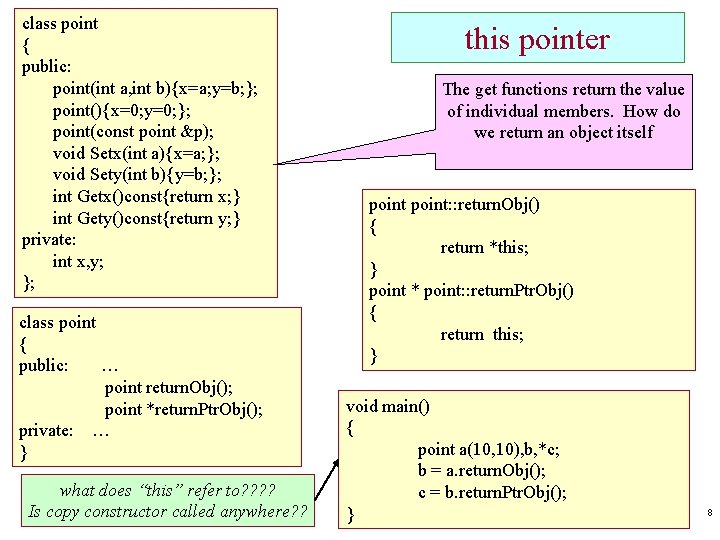
class point { public: point(int a, int b){x=a; y=b; }; point(){x=0; y=0; }; point(const point &p); void Setx(int a){x=a; }; void Sety(int b){y=b; }; int Getx()const{return x; } int Gety()const{return y; } private: int x, y; }; class point { public: … point return. Obj(); point *return. Ptr. Obj(); private: … } what does “this” refer to? ? Is copy constructor called anywhere? ? this pointer The get functions return the value of individual members. How do we return an object itself point: : return. Obj() { return *this; } point * point: : return. Ptr. Obj() { return this; } void main() { point a(10, 10), b, *c; b = a. return. Obj(); c = b. return. Ptr. Obj(); } 8
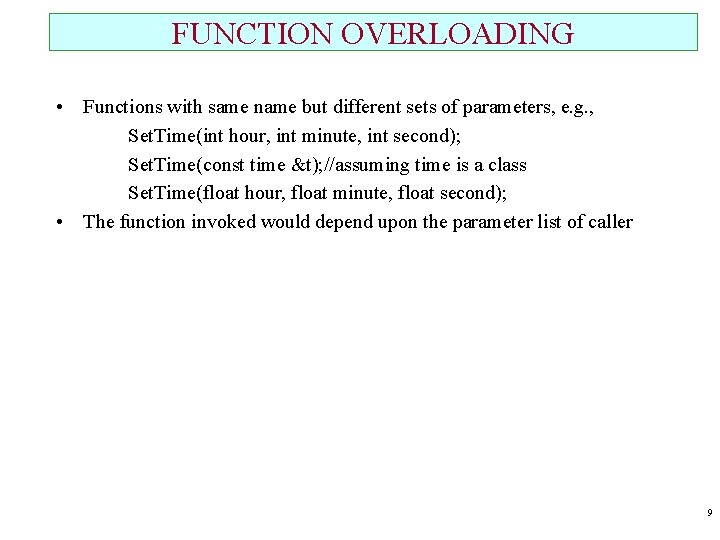
FUNCTION OVERLOADING • Functions with same name but different sets of parameters, e. g. , Set. Time(int hour, int minute, int second); Set. Time(const time &t); //assuming time is a class Set. Time(float hour, float minute, float second); • The function invoked would depend upon the parameter list of caller 9
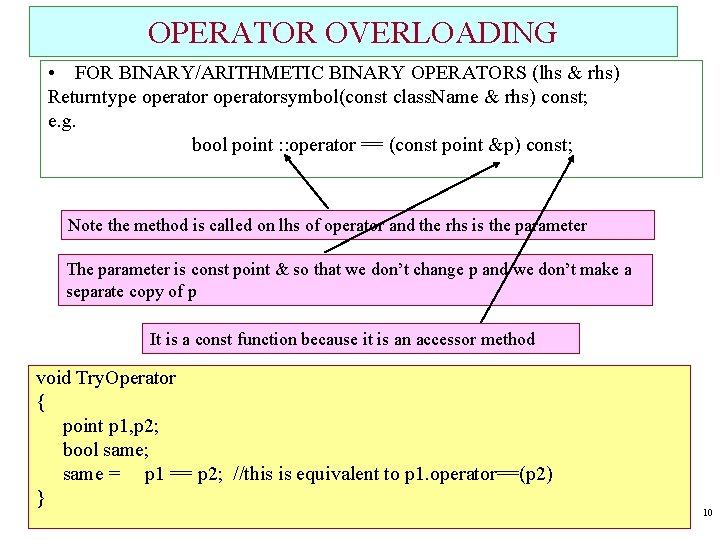
OPERATOR OVERLOADING • FOR BINARY/ARITHMETIC BINARY OPERATORS (lhs & rhs) Returntype operatorsymbol(const class. Name & rhs) const; e. g. bool point : : operator == (const point &p) const; Note the method is called on lhs of operator and the rhs is the parameter The parameter is const point & so that we don’t change p and we don’t make a separate copy of p It is a const function because it is an accessor method void Try. Operator { point p 1, p 2; bool same; same = p 1 == p 2; //this is equivalent to p 1. operator==(p 2) } 10
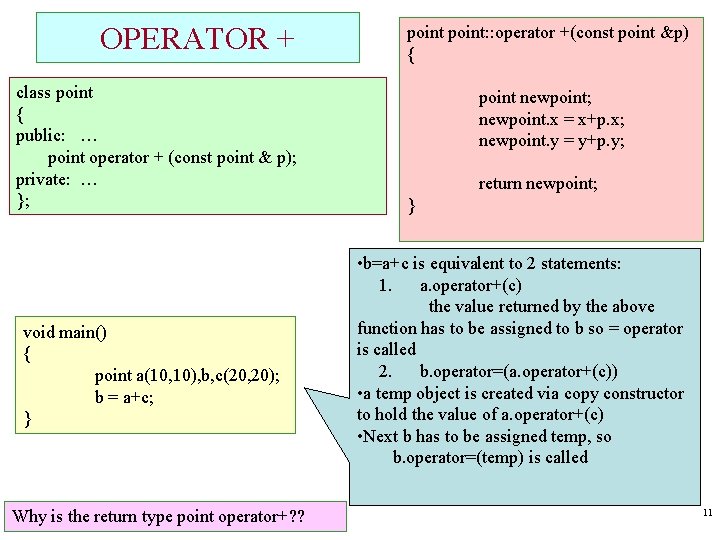
OPERATOR + class point { public: … point operator + (const point & p); private: … }; void main() { point a(10, 10), b, c(20, 20); b = a+c; } Why is the return type point operator+? ? point: : operator +(const point &p) { point newpoint; newpoint. x = x+p. x; newpoint. y = y+p. y; return newpoint; } • b=a+c is equivalent to 2 statements: 1. a. operator+(c) the value returned by the above function has to be assigned to b so = operator is called 2. b. operator=(a. operator+(c)) • a temp object is created via copy constructor to hold the value of a. operator+(c) • Next b has to be assigned temp, so b. operator=(temp) is called 11
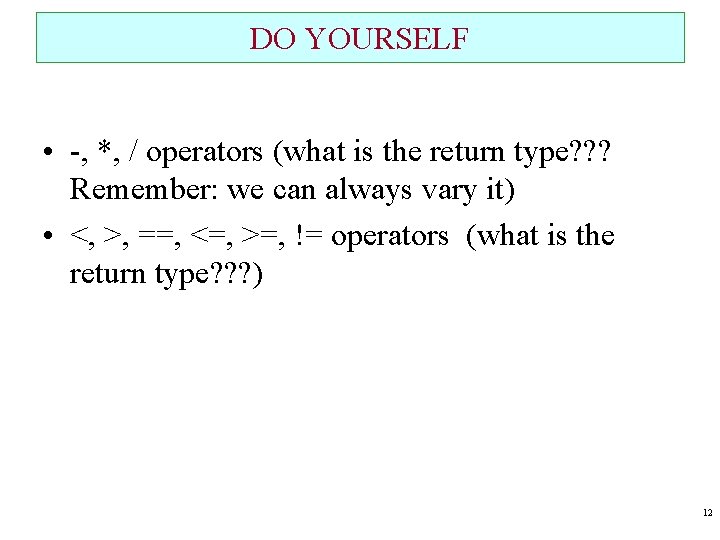
DO YOURSELF • -, *, / operators (what is the return type? ? ? Remember: we can always vary it) • <, >, ==, <=, >=, != operators (what is the return type? ? ? ) 12
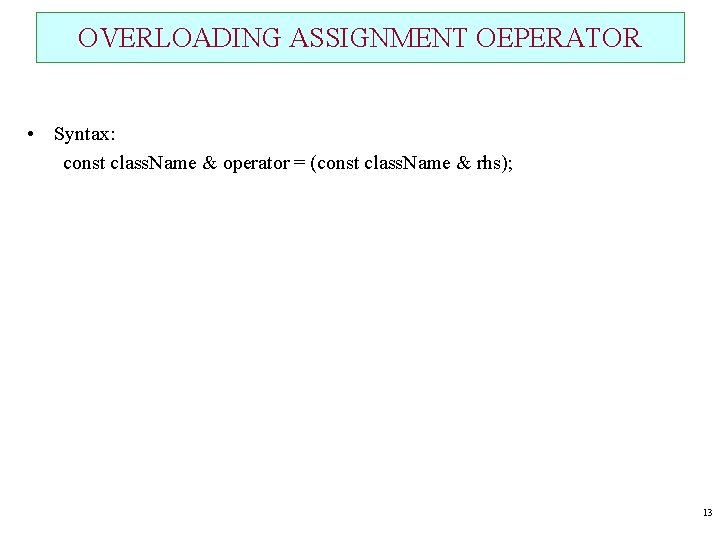
OVERLOADING ASSIGNMENT OEPERATOR • Syntax: const class. Name & operator = (const class. Name & rhs); 13
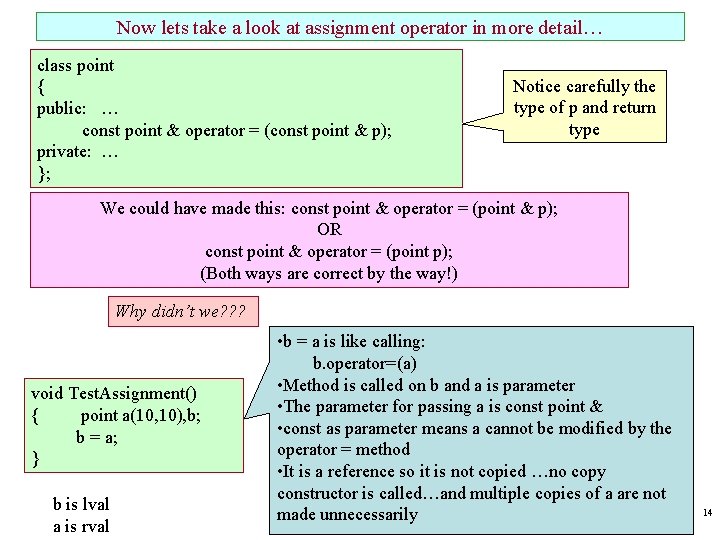
Now lets take a look at assignment operator in more detail… class point { public: … const point & operator = (const point & p); private: … }; Notice carefully the type of p and return type We could have made this: const point & operator = (point & p); OR const point & operator = (point p); (Both ways are correct by the way!) Why didn’t we? ? ? void Test. Assignment() { point a(10, 10), b; b = a; } b is lval a is rval • b = a is like calling: b. operator=(a) • Method is called on b and a is parameter • The parameter for passing a is const point & • const as parameter means a cannot be modified by the operator = method • It is a reference so it is not copied …no copy constructor is called…and multiple copies of a are not made unnecessarily 14
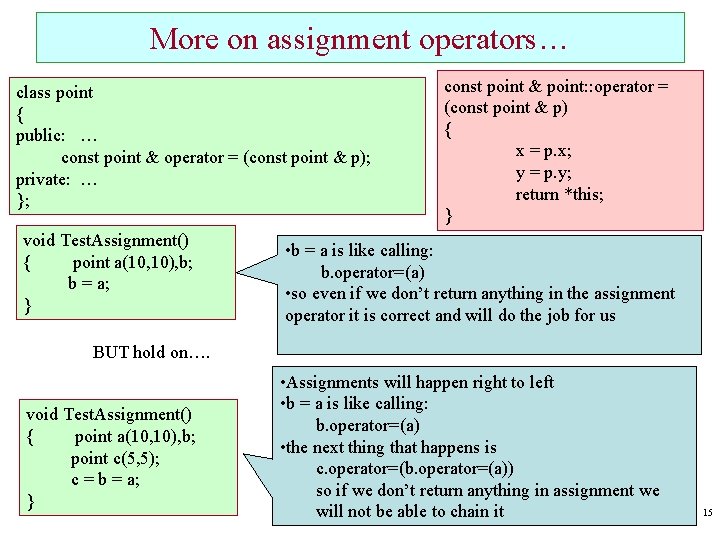
More on assignment operators… class point { public: … const point & operator = (const point & p); private: … }; void Test. Assignment() { point a(10, 10), b; b = a; } const point & point: : operator = (const point & p) { x = p. x; y = p. y; return *this; } • b = a is like calling: b. operator=(a) • so even if we don’t return anything in the assignment operator it is correct and will do the job for us BUT hold on…. void Test. Assignment() { point a(10, 10), b; point c(5, 5); c = b = a; } • Assignments will happen right to left • b = a is like calling: b. operator=(a) • the next thing that happens is c. operator=(b. operator=(a)) so if we don’t return anything in assignment we will not be able to chain it 15
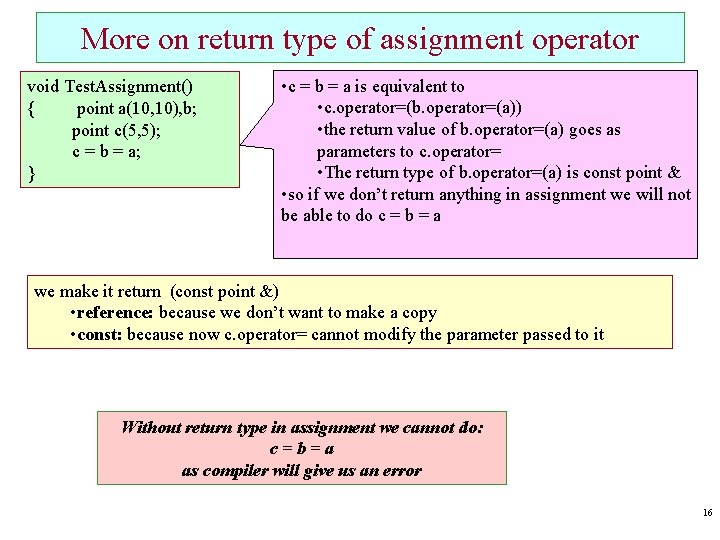
More on return type of assignment operator void Test. Assignment() { point a(10, 10), b; point c(5, 5); c = b = a; } • c = b = a is equivalent to • c. operator=(b. operator=(a)) • the return value of b. operator=(a) goes as parameters to c. operator= • The return type of b. operator=(a) is const point & • so if we don’t return anything in assignment we will not be able to do c = b = a we make it return (const point &) • reference: because we don’t want to make a copy • const: because now c. operator= cannot modify the parameter passed to it Without return type in assignment we cannot do: c=b=a as compiler will give us an error 16
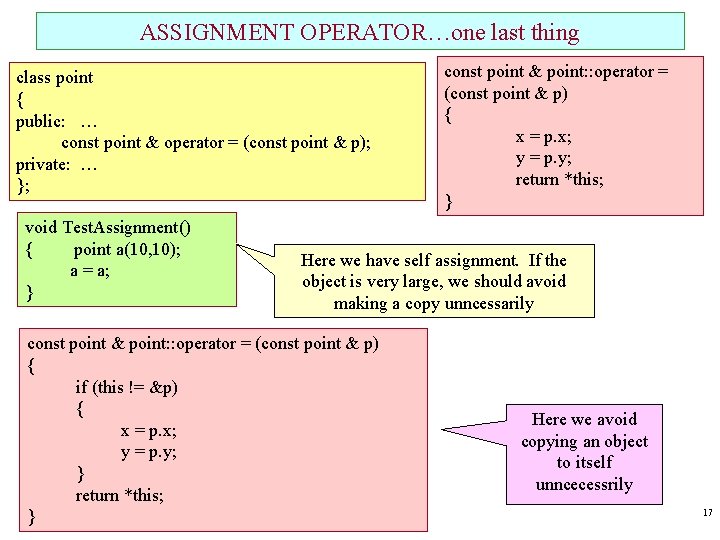
ASSIGNMENT OPERATOR…one last thing class point { public: … const point & operator = (const point & p); private: … }; void Test. Assignment() { point a(10, 10); a = a; } const point & point: : operator = (const point & p) { x = p. x; y = p. y; return *this; } Here we have self assignment. If the object is very large, we should avoid making a copy unncessarily const point & point: : operator = (const point & p) { if (this != &p) { x = p. x; y = p. y; } return *this; } Here we avoid copying an object to itself unncecessrily 17
Const char * vs char * const
Char name 20
V char
Const char *s=""
Bracket operator overloading c++
Example of operator overloading in c++
C++ operator overloading template
Unary operator overloading
Pitfalls of operator overloading in c++
New and delete operators can be overloaded
Cps235
Binary operator overloading using friend function
Unary operator overloading
Compound assignment operators in c with example
Pointer expressions
9 pointers
Pointer pointer
Constant to pointer in c