Console Write Line class Program static void Mainstring
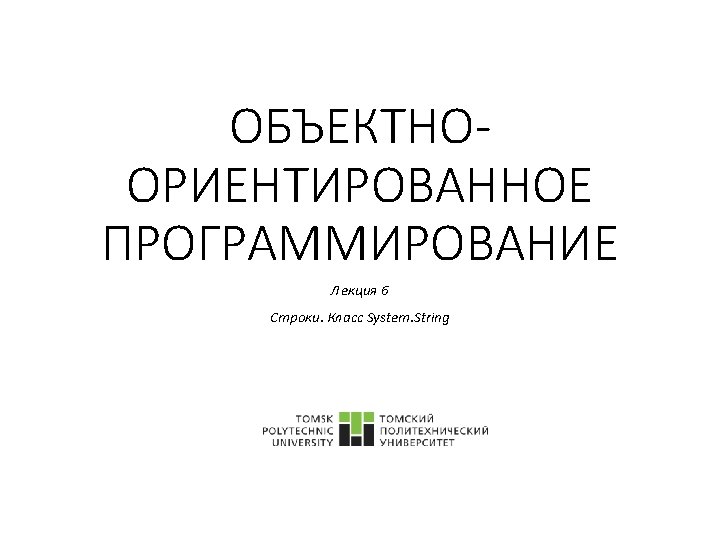
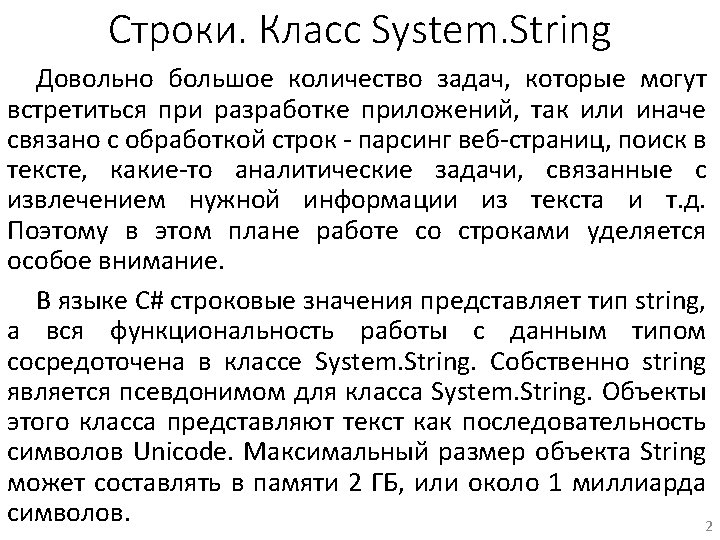
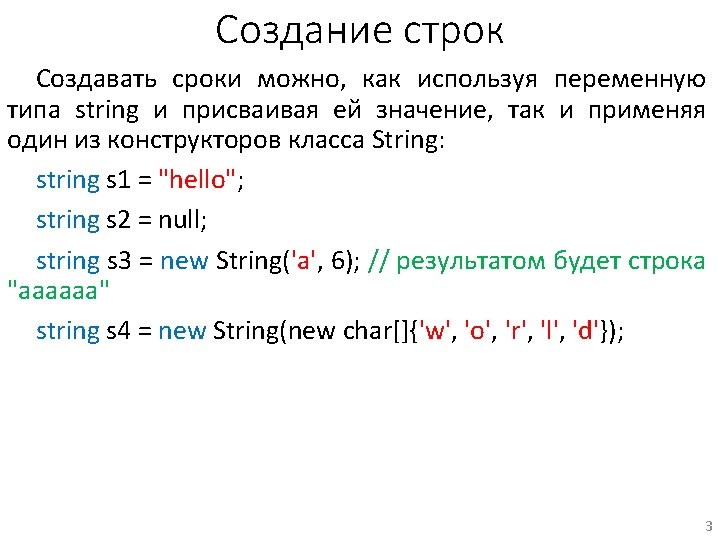
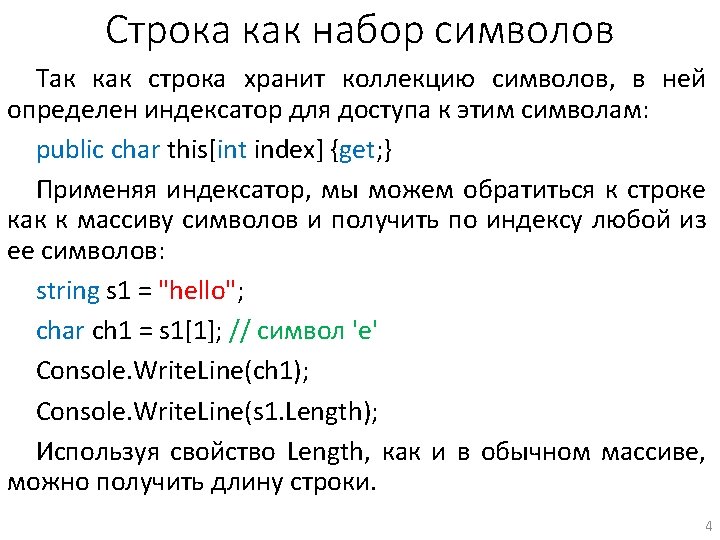
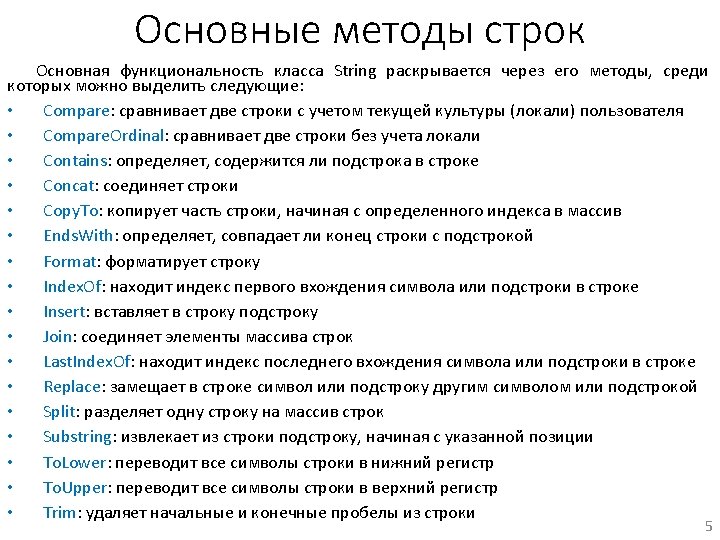
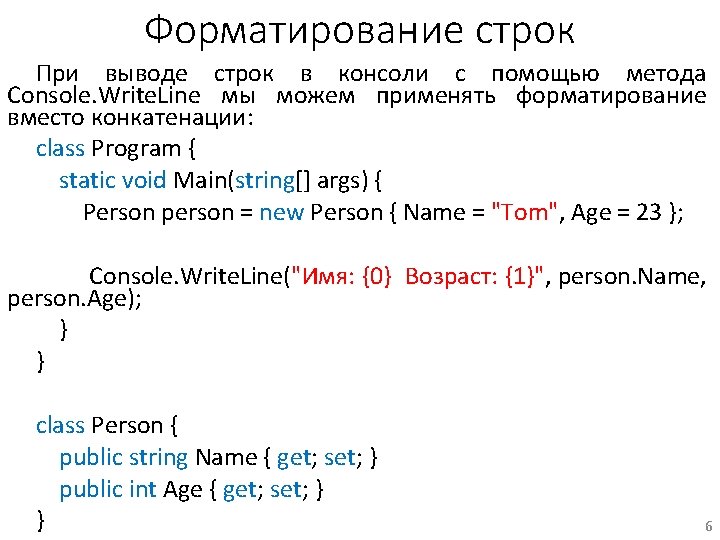
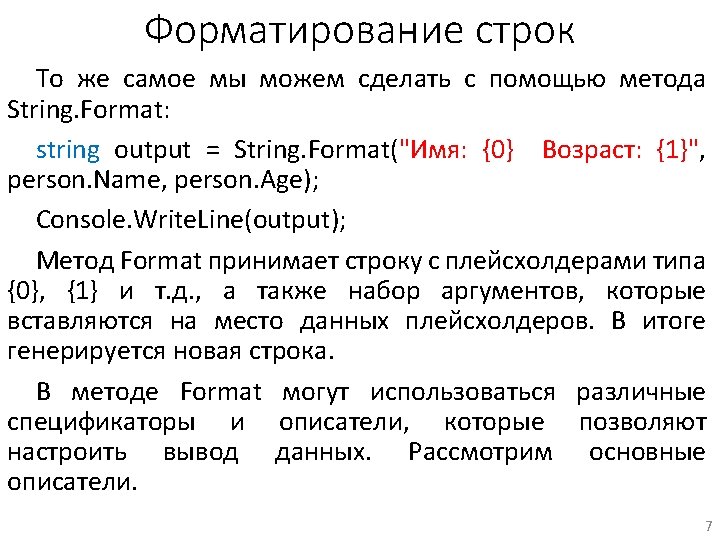
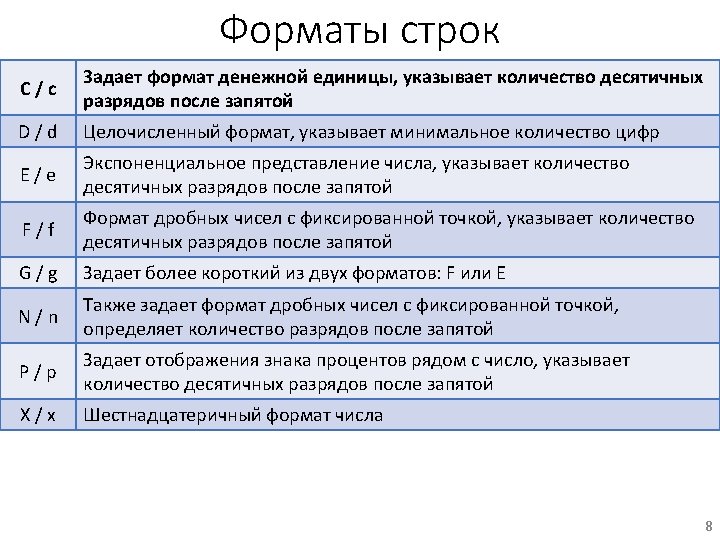
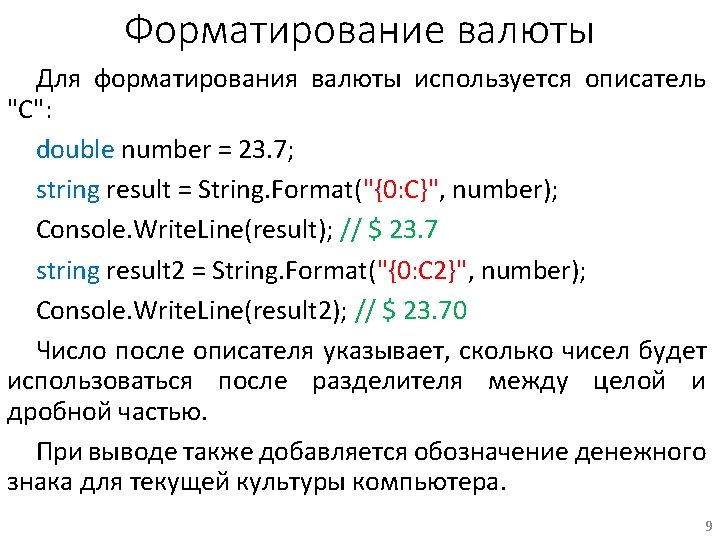
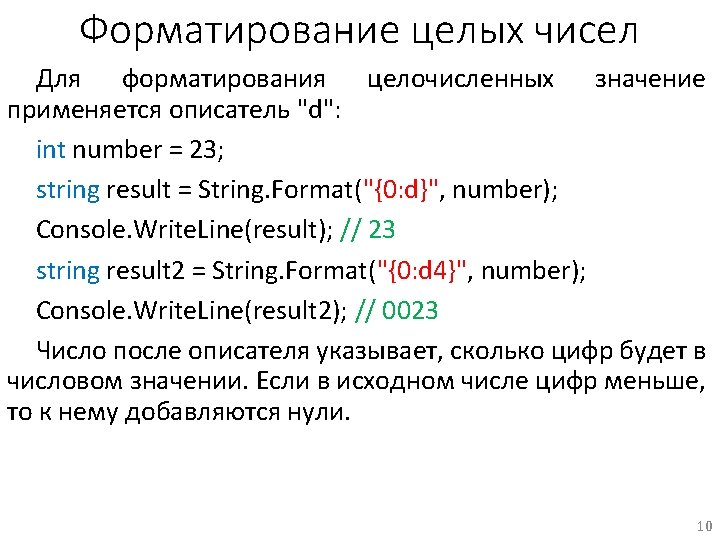
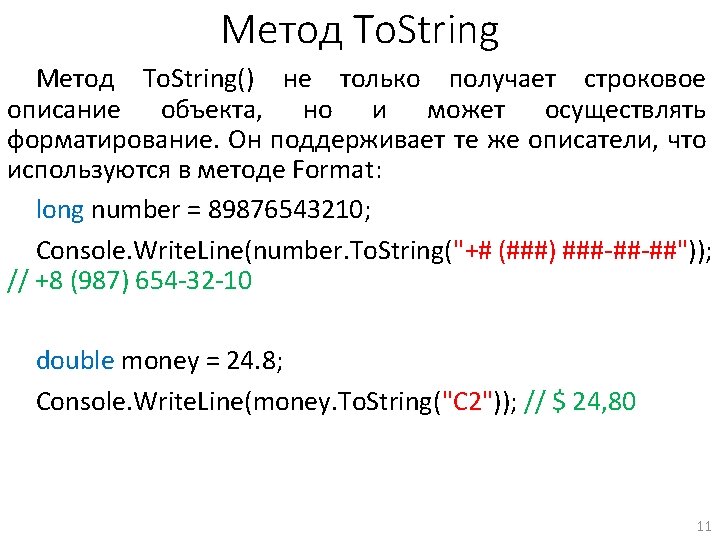
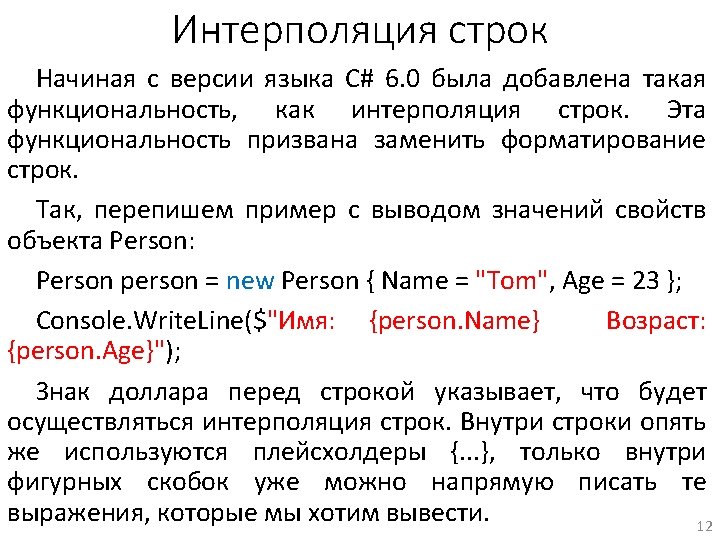
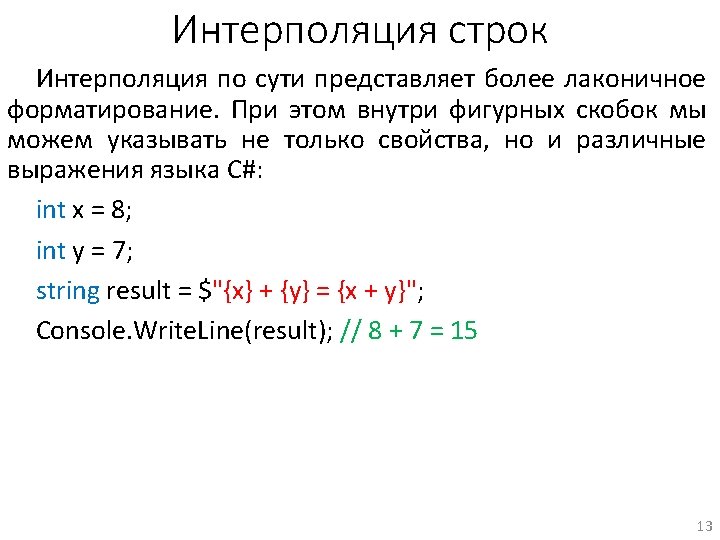
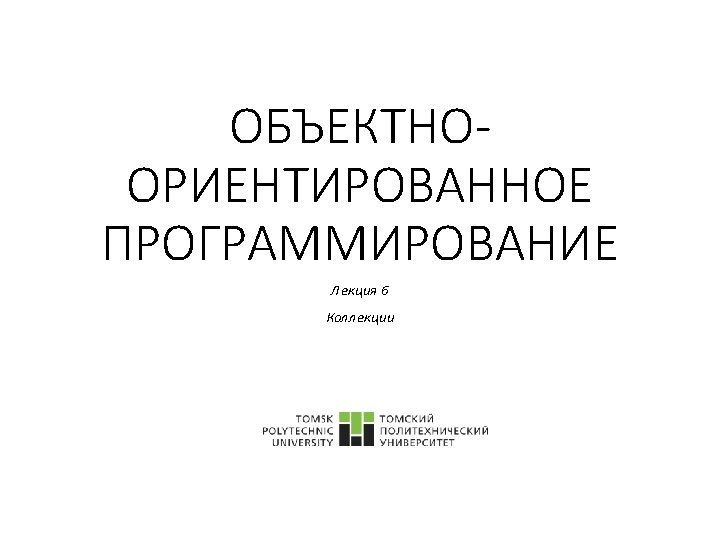
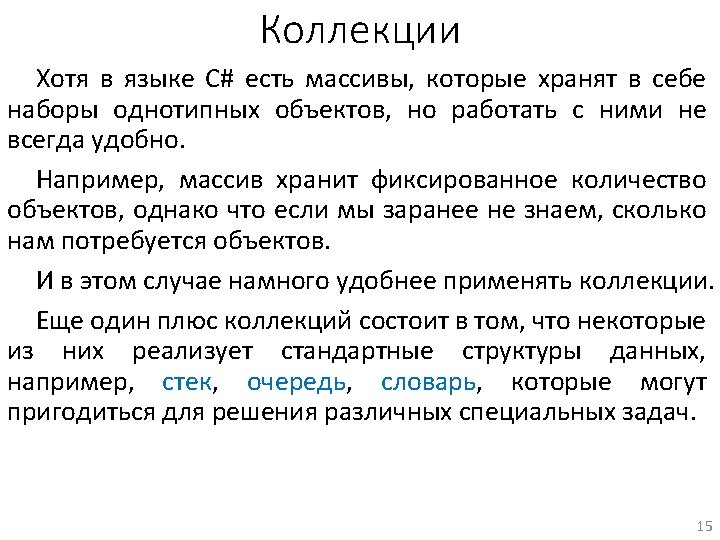
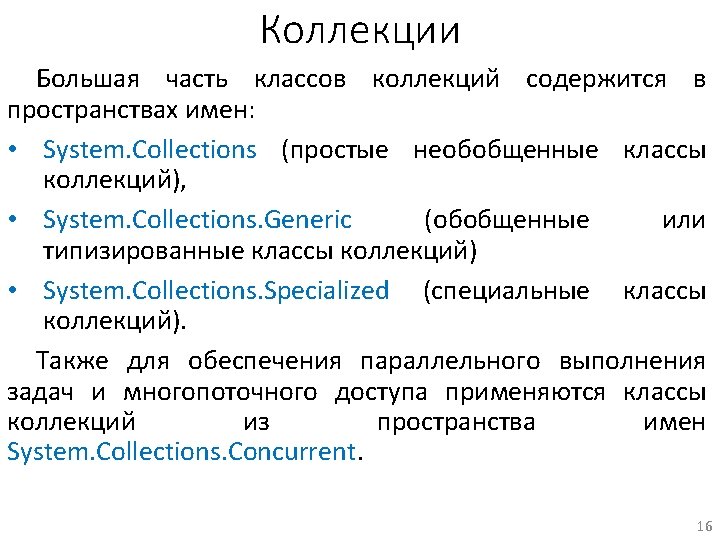
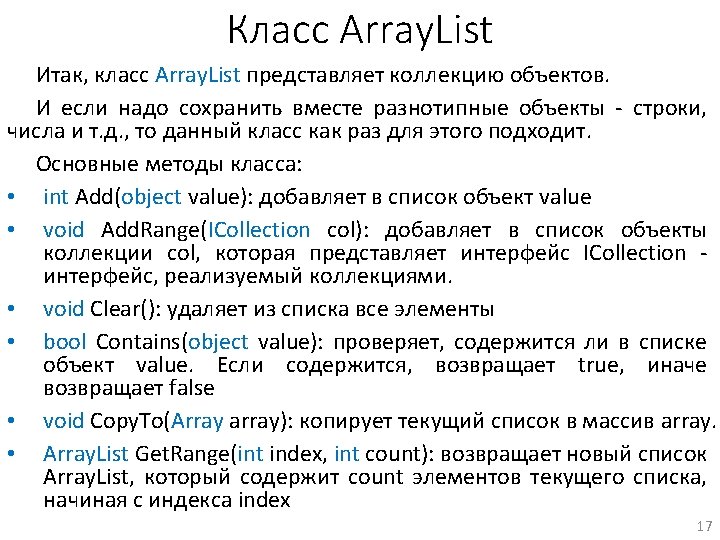
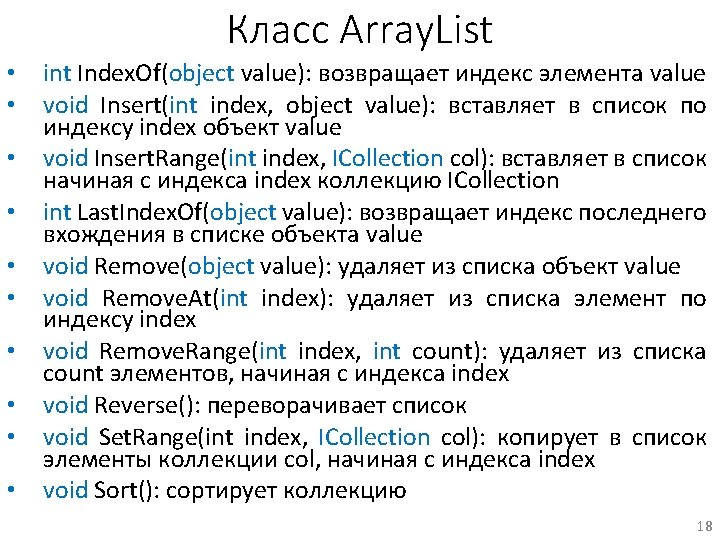
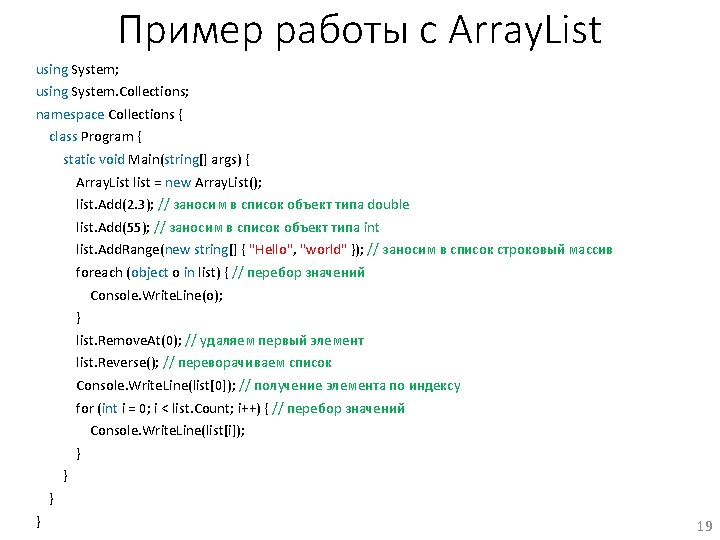
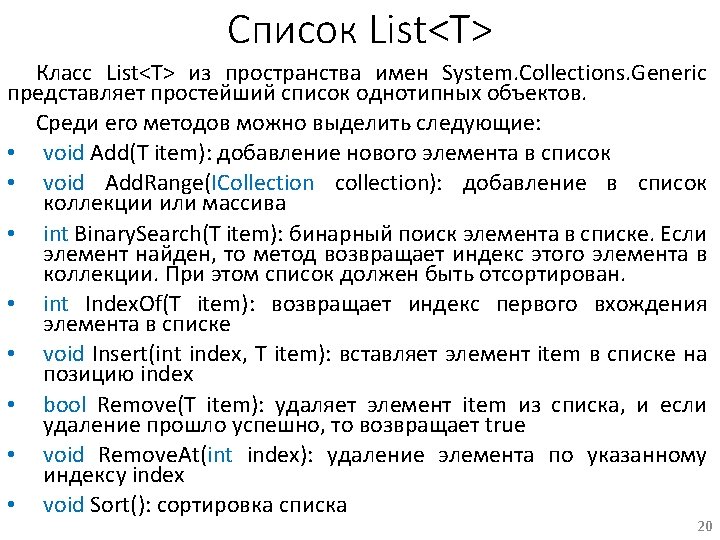
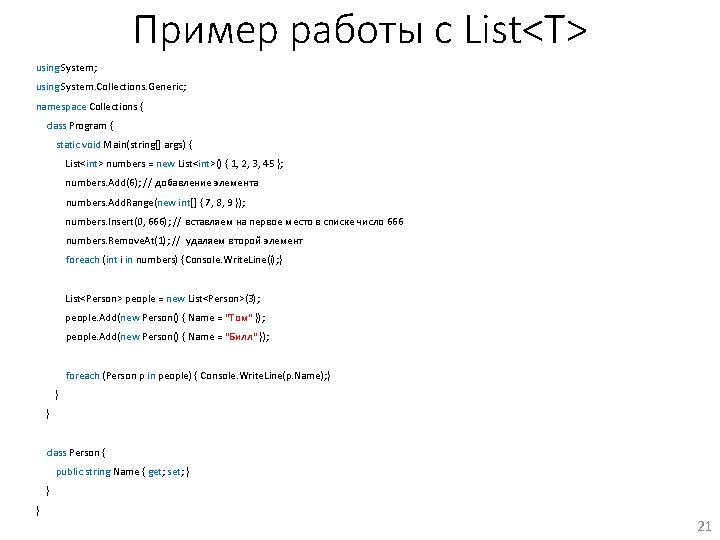
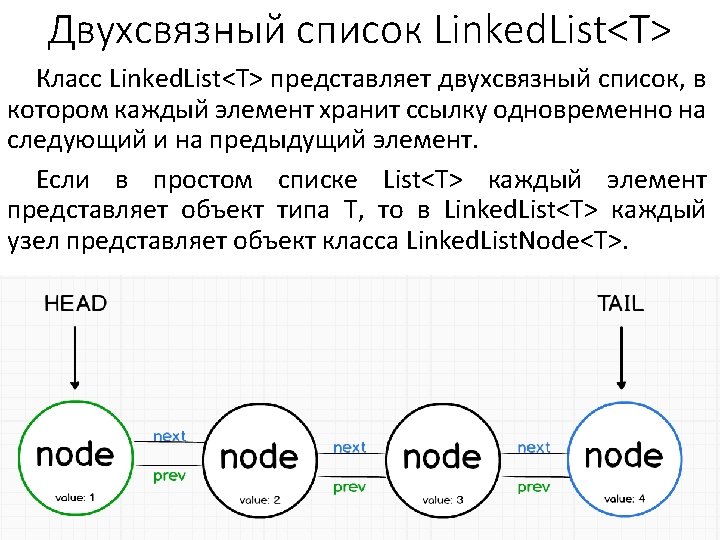
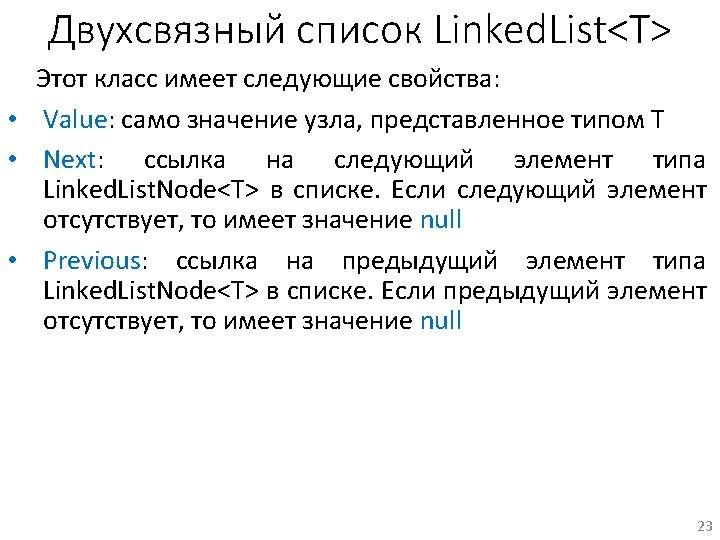
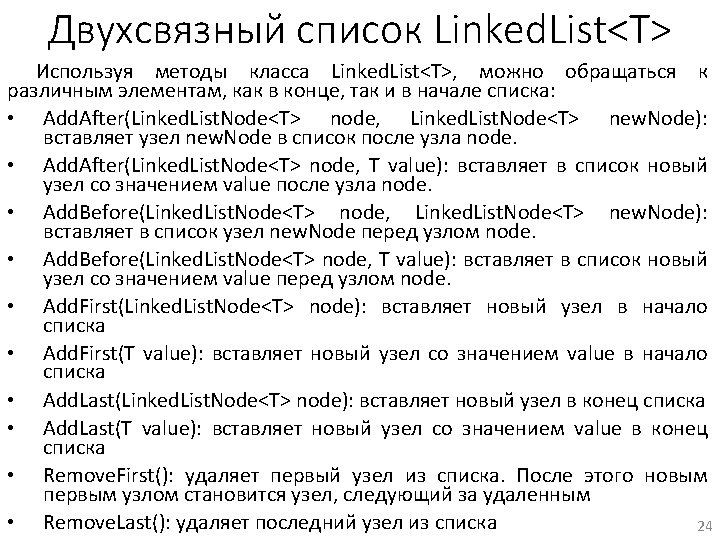
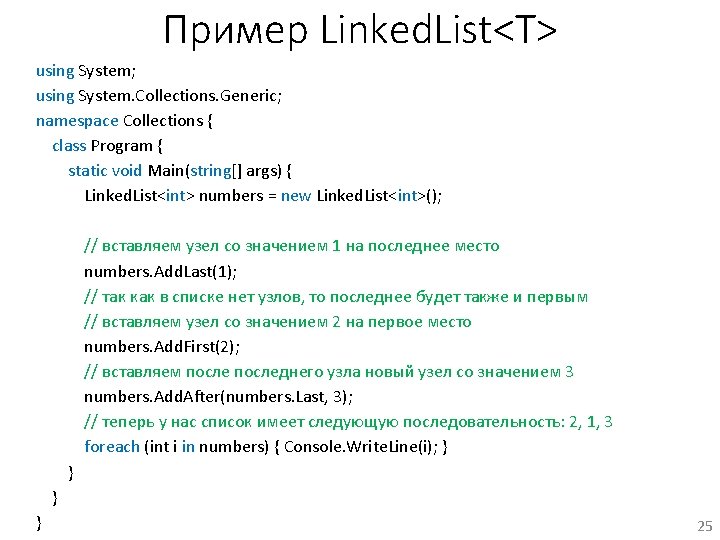
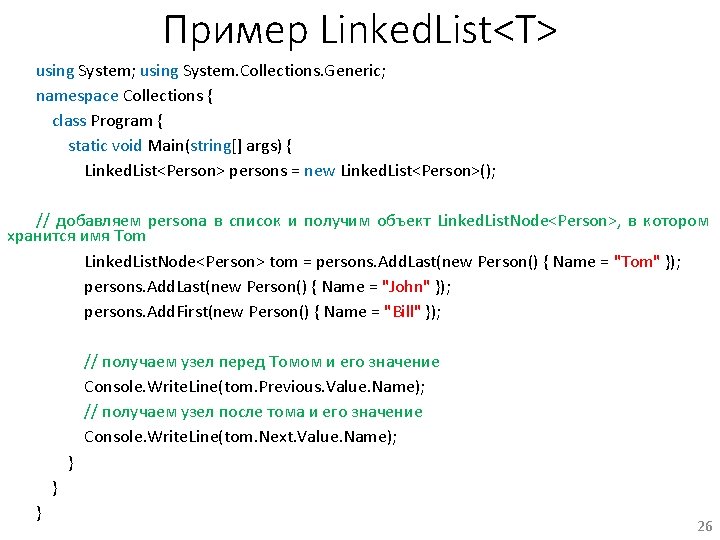
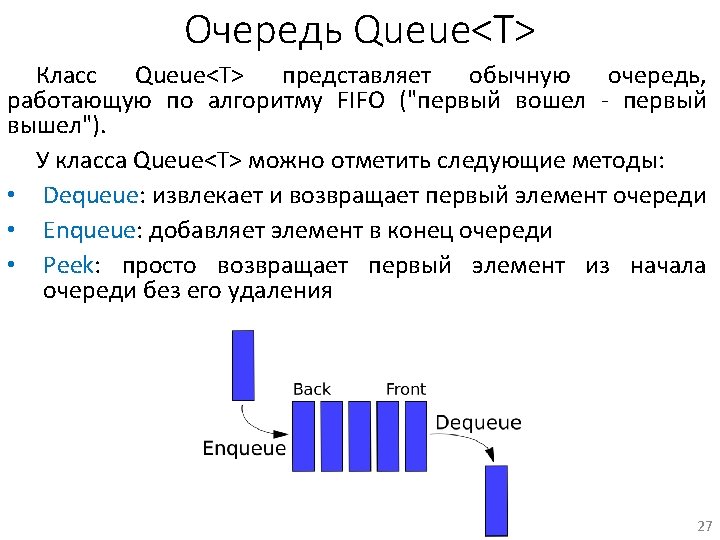
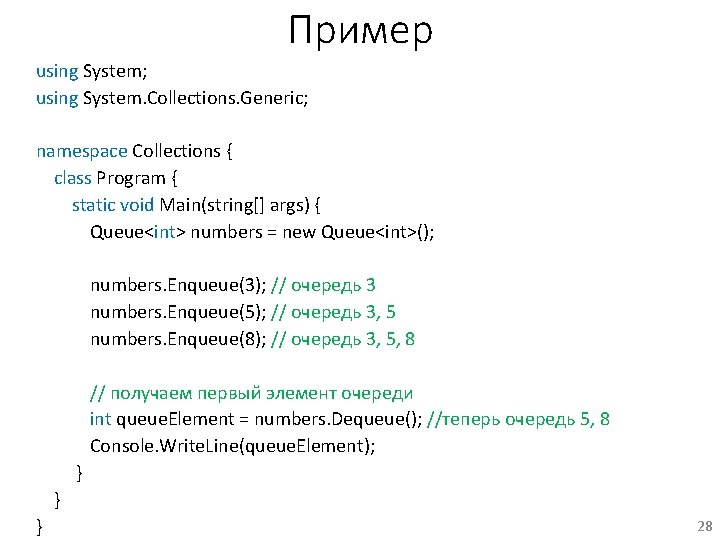
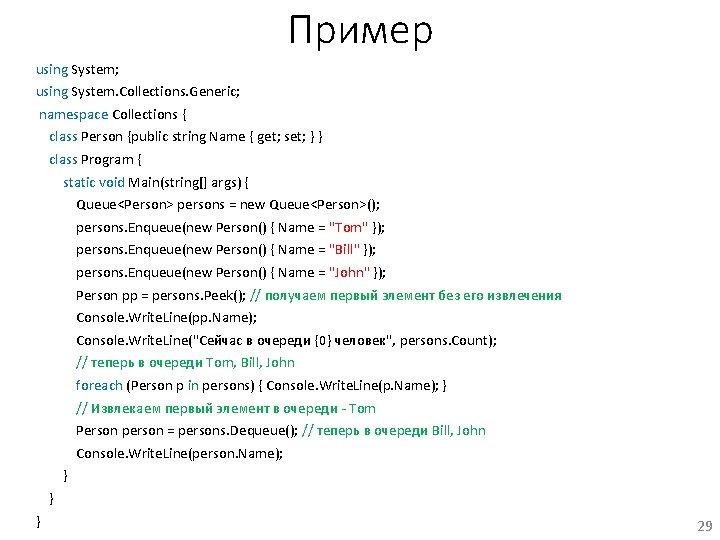
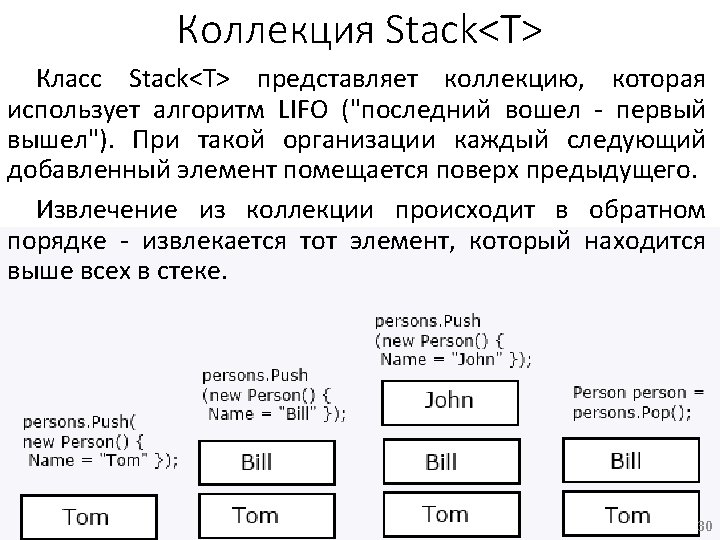
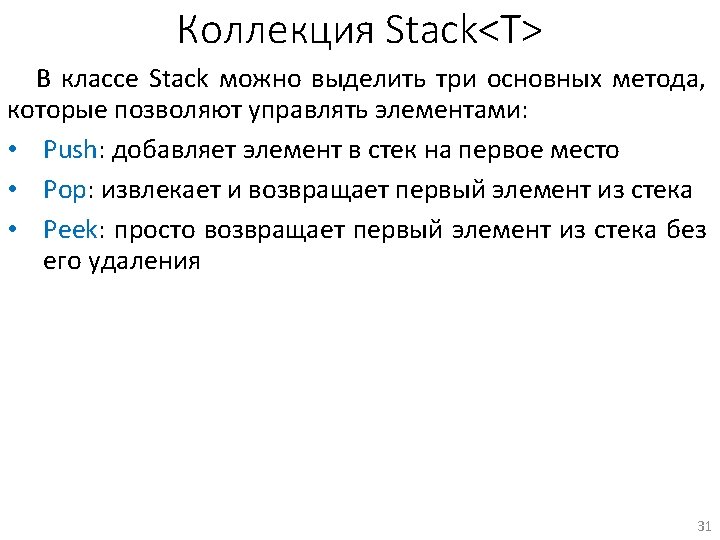
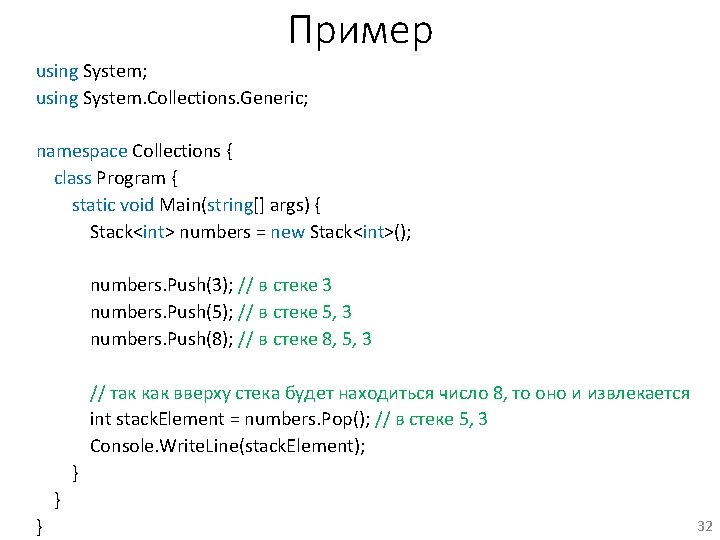
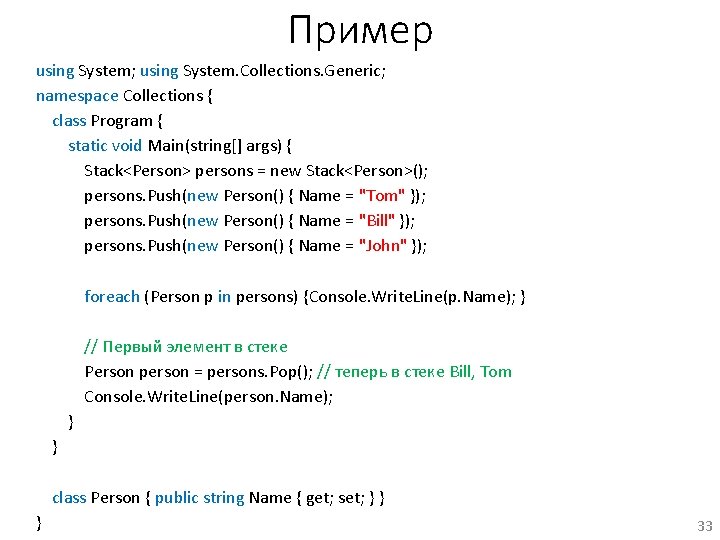
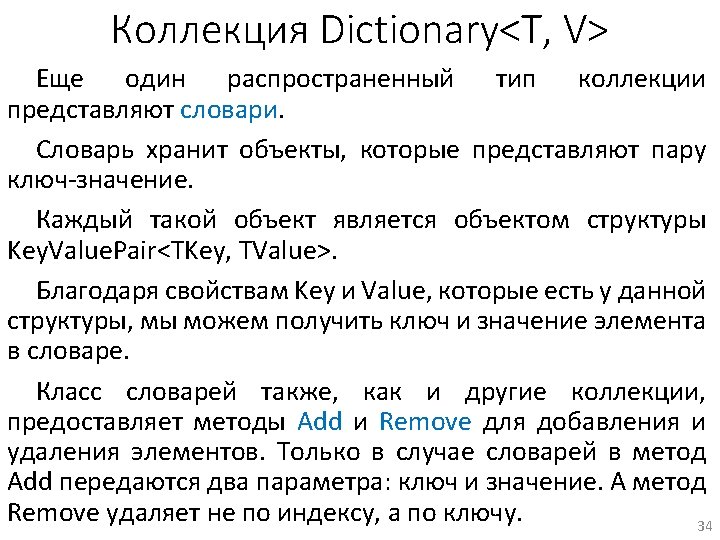
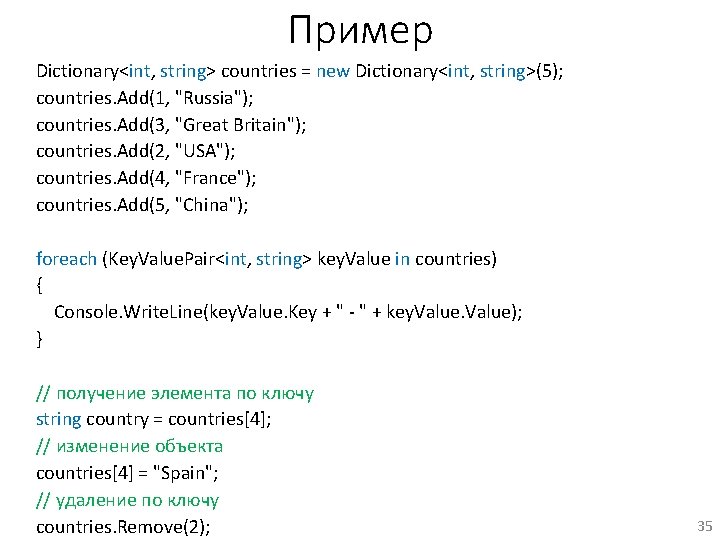
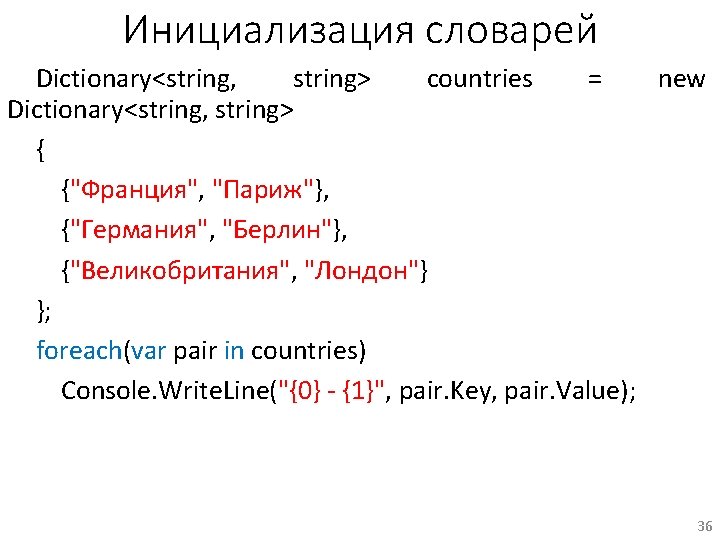
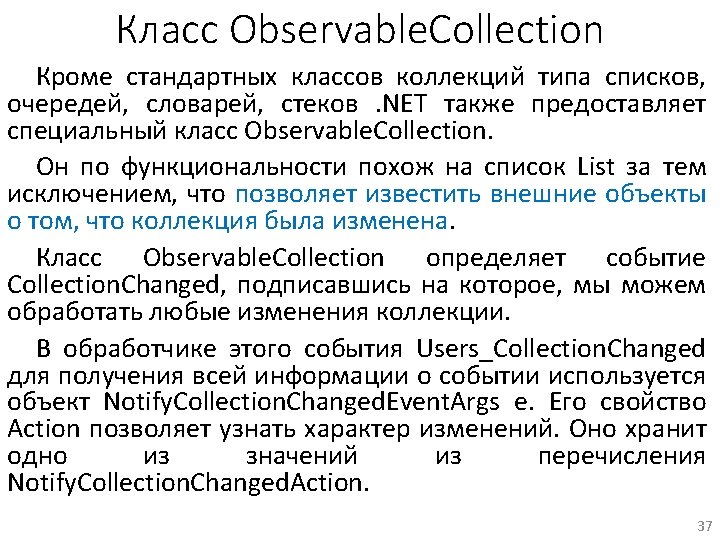
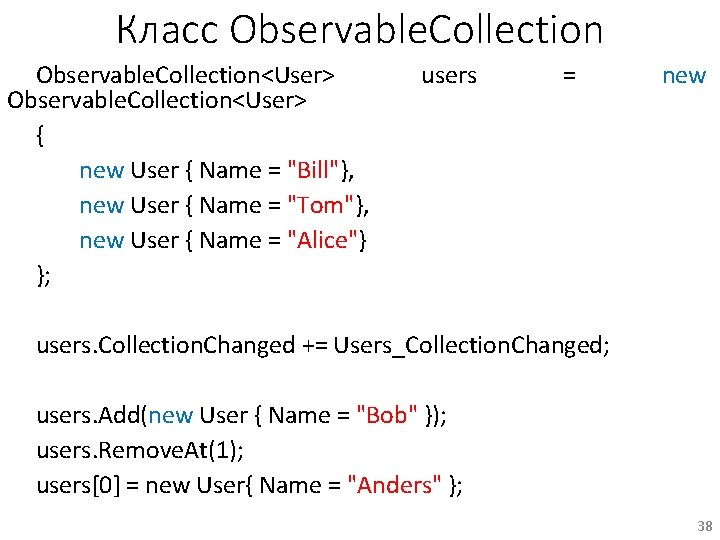
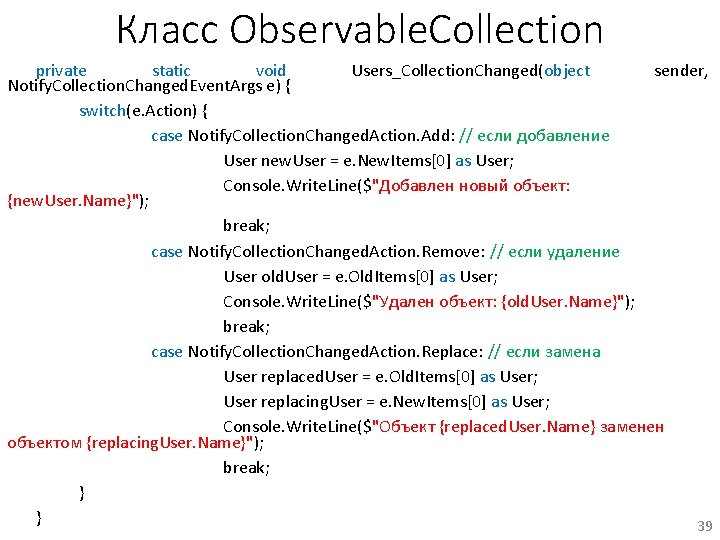
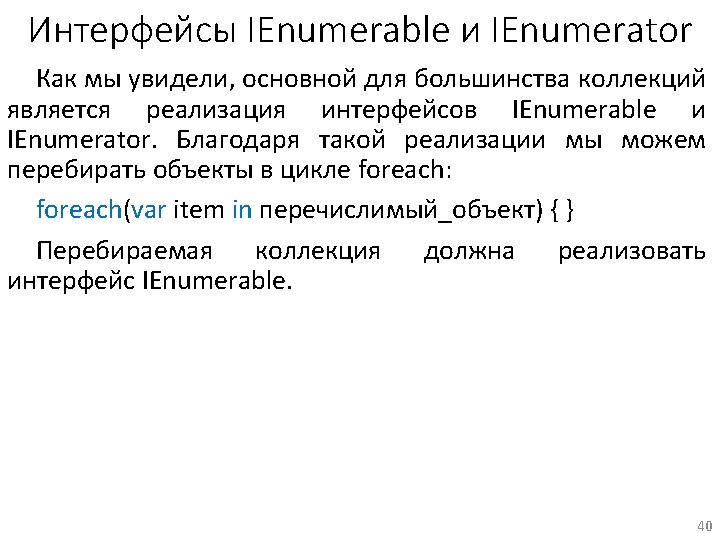
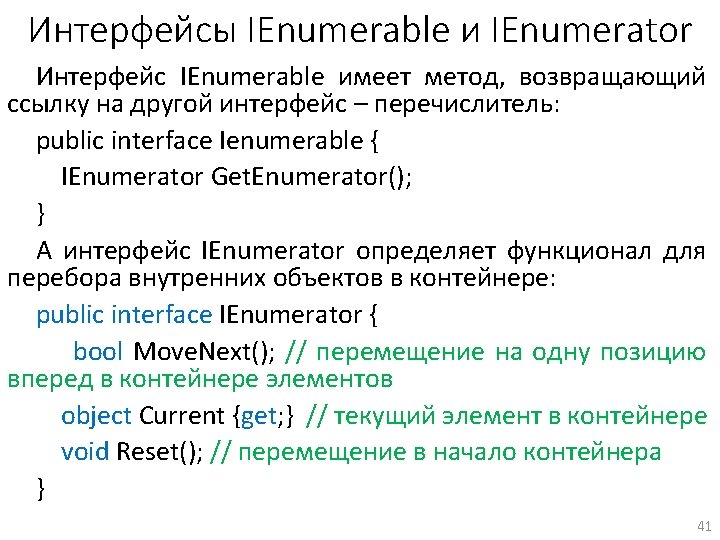
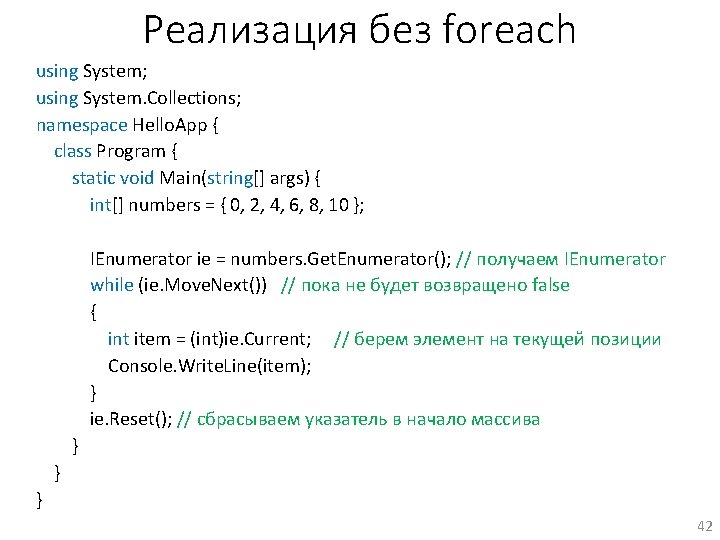
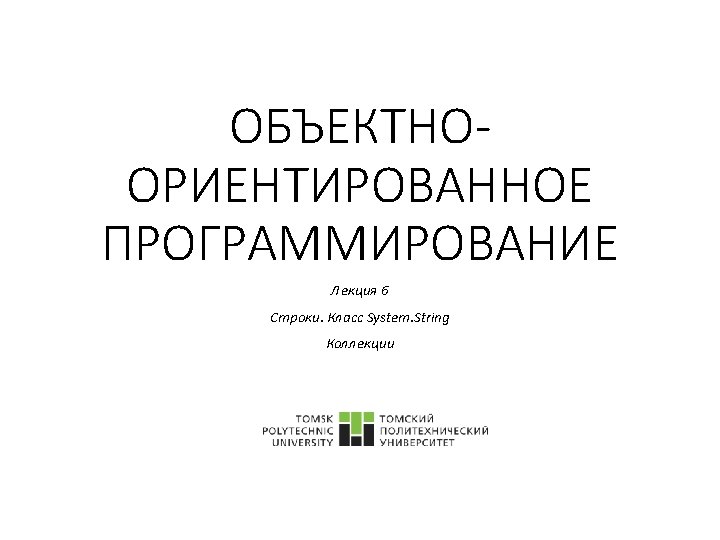
- Slides: 43
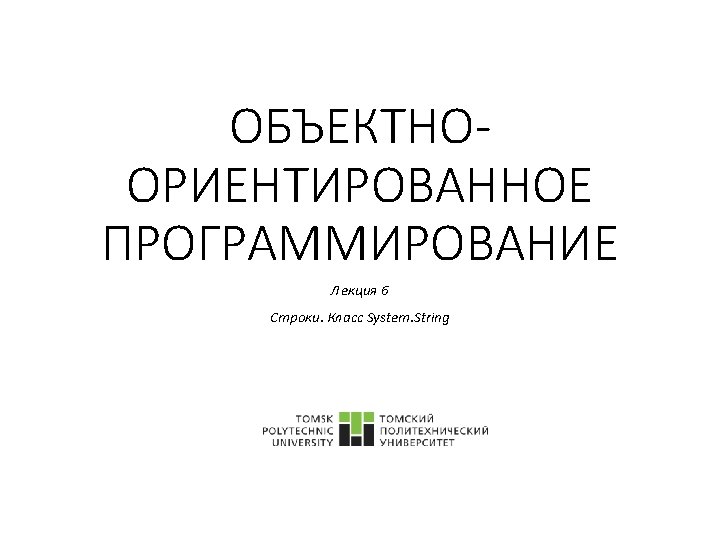
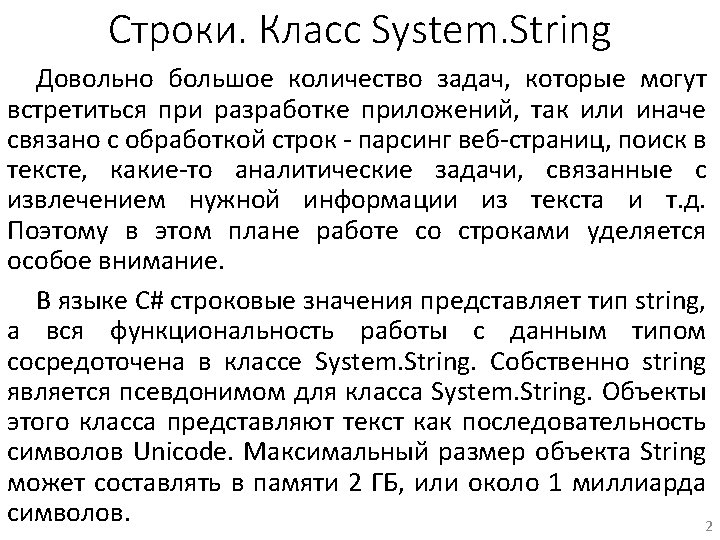
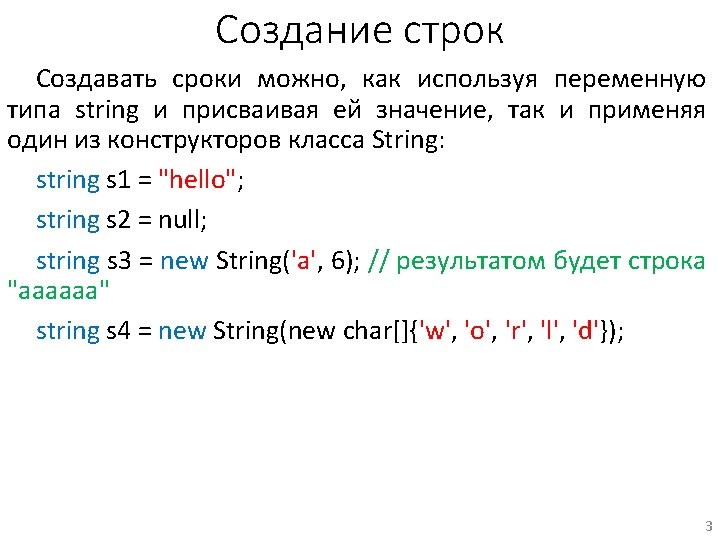
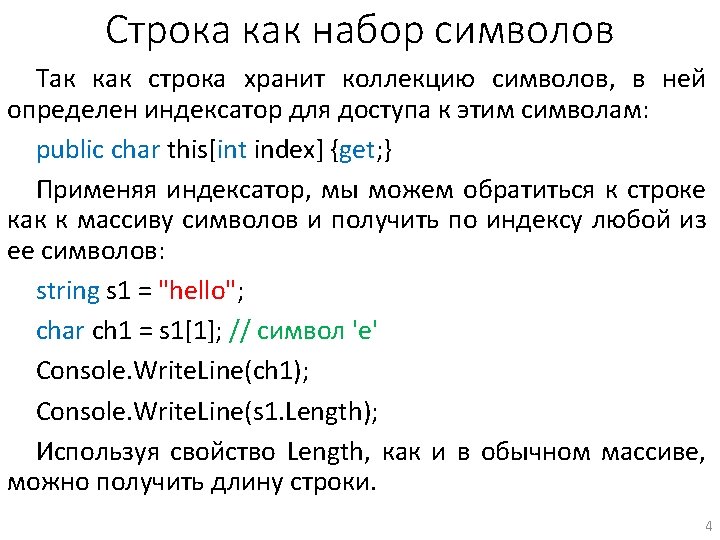
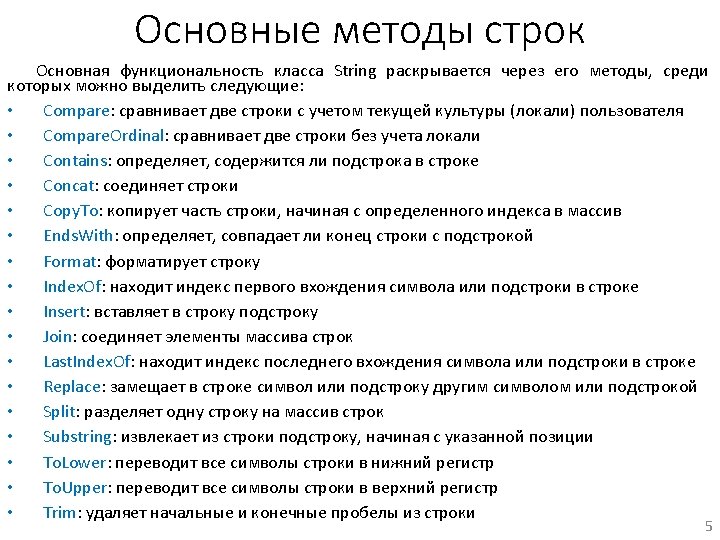
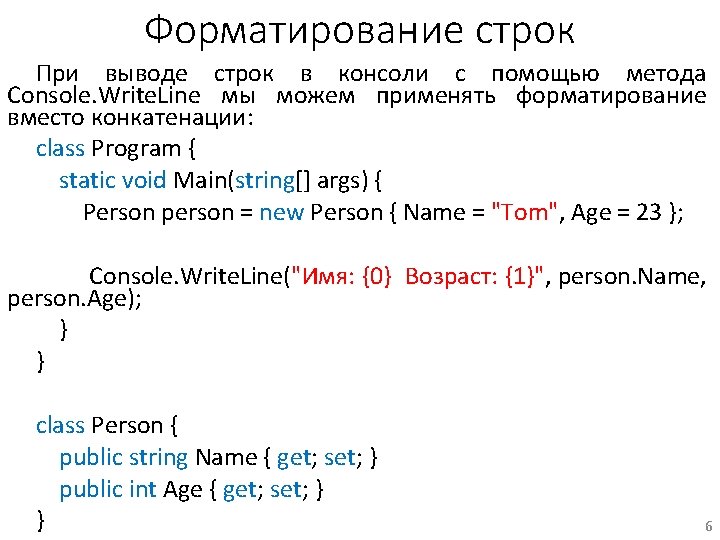
Форматирование строк При выводе строк в консоли с помощью метода Console. Write. Line мы можем применять форматирование вместо конкатенации: class Program { static void Main(string[] args) { Person person = new Person { Name = "Tom", Age = 23 }; Console. Write. Line("Имя: {0} Возраст: {1}", person. Name, person. Age); } } class Person { public string Name { get; set; } public int Age { get; set; } } 6
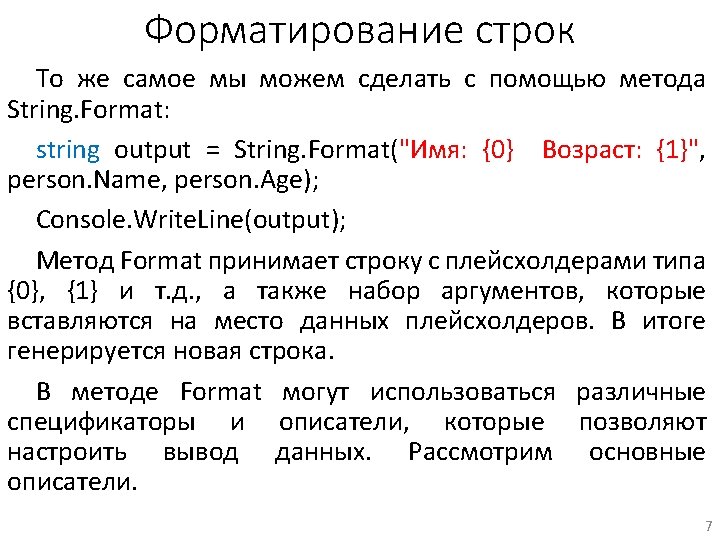
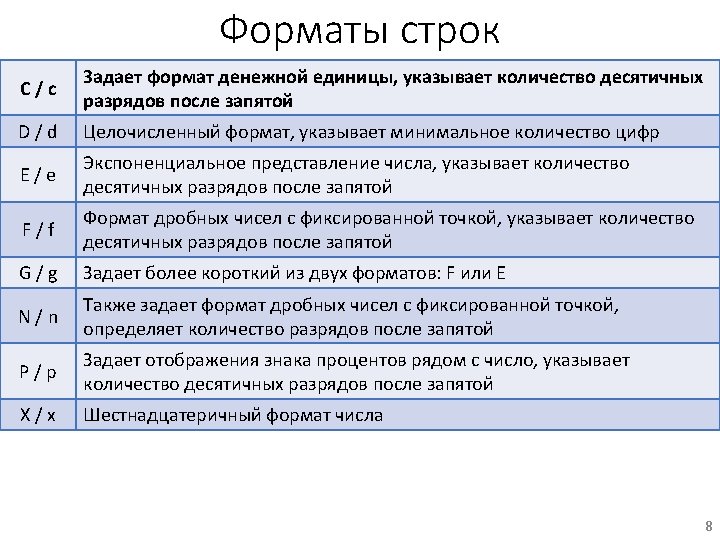
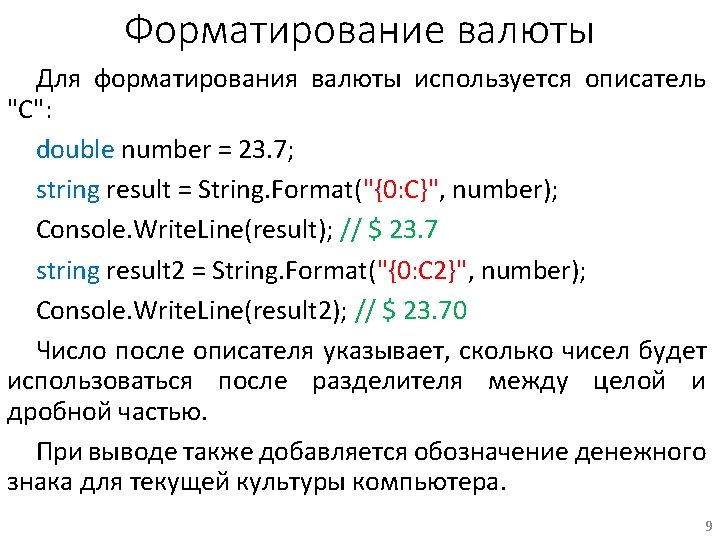
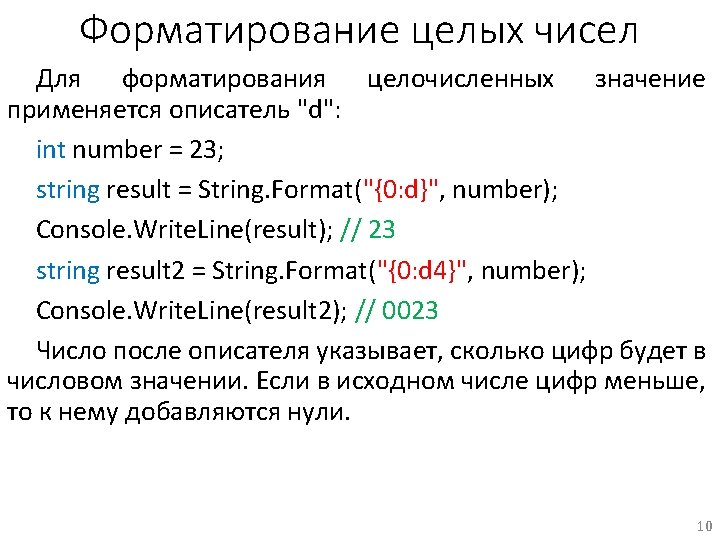
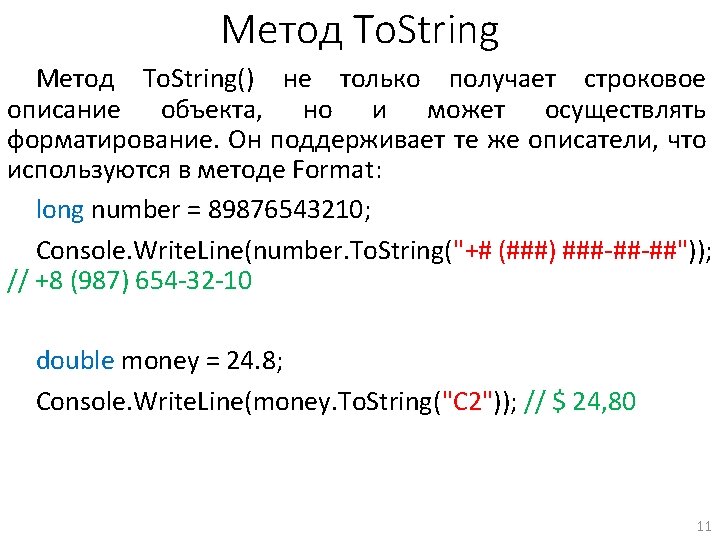
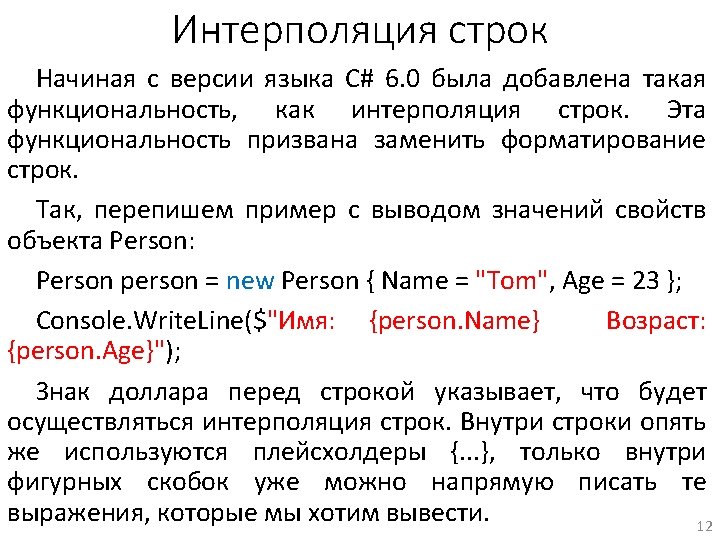
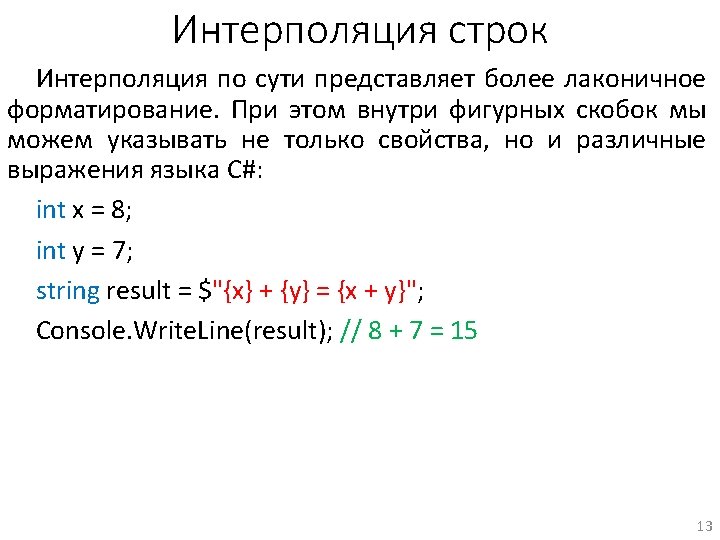
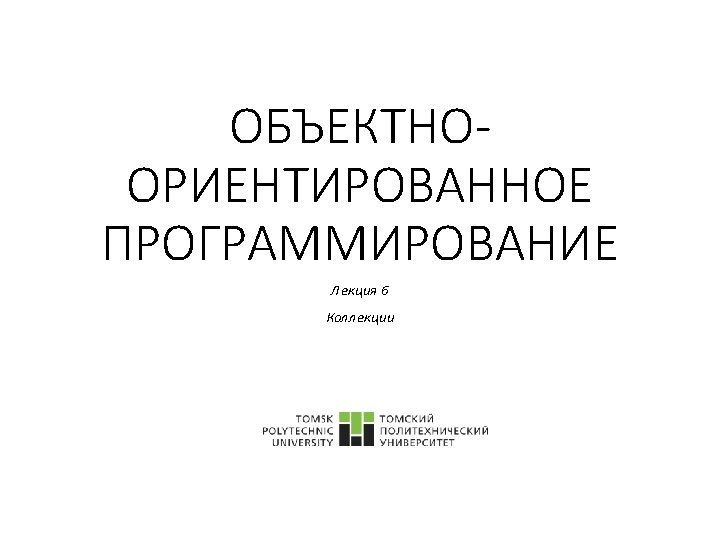
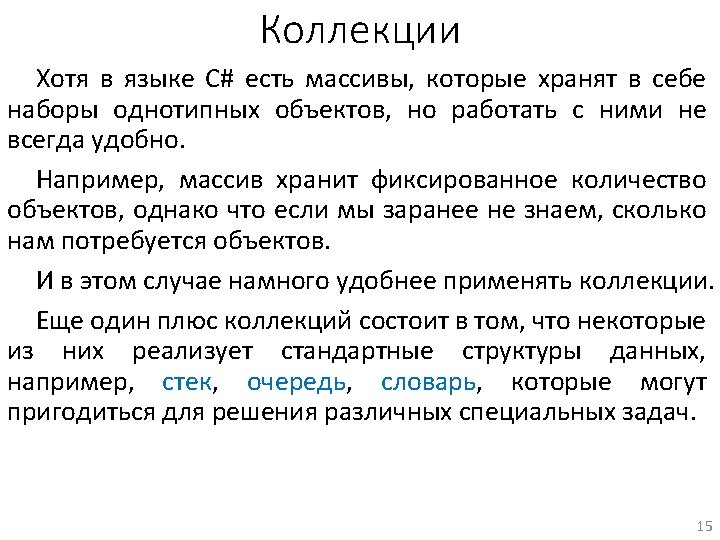
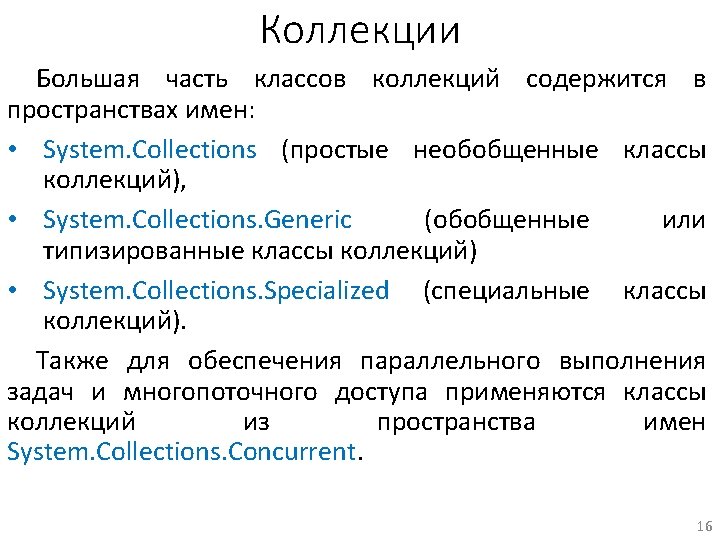
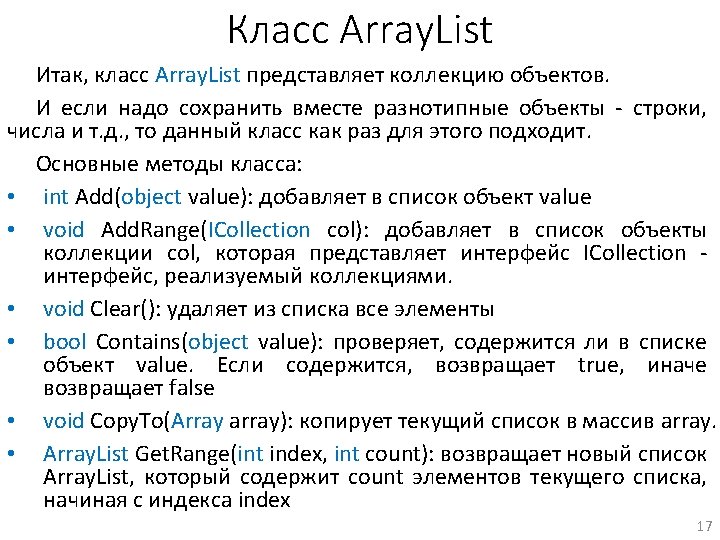
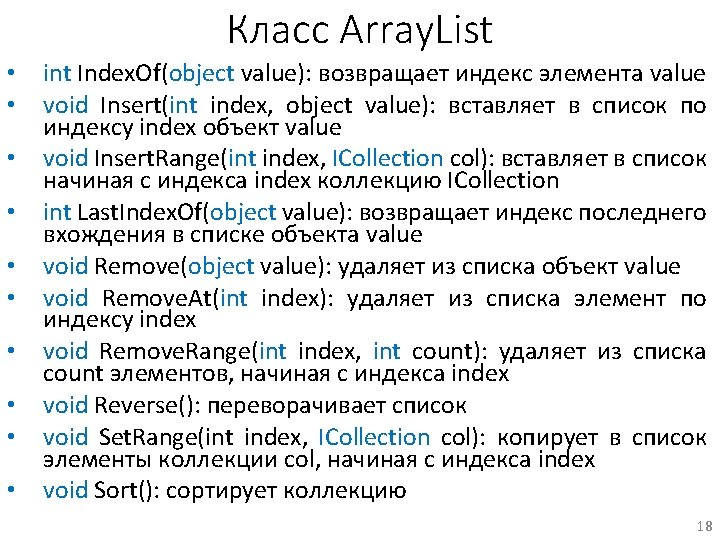
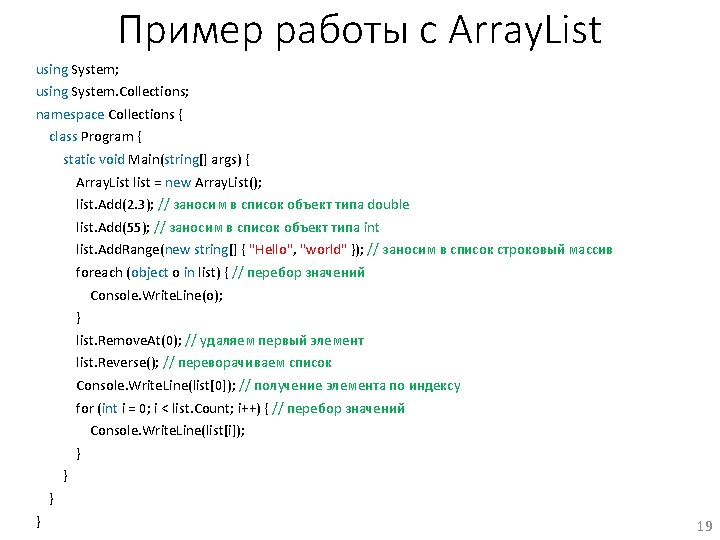
Пример работы с Array. List using System; using System. Collections; namespace Collections { class Program { static void Main(string[] args) { Array. List list = new Array. List(); list. Add(2. 3); // заносим в список объект типа double list. Add(55); // заносим в список объект типа int list. Add. Range(new string[] { "Hello", "world" }); // заносим в список строковый массив foreach (object o in list) { // перебор значений Console. Write. Line(o); } list. Remove. At(0); // удаляем первый элемент list. Reverse(); // переворачиваем список Console. Write. Line(list[0]); // получение элемента по индексу for (int i = 0; i < list. Count; i++) { // перебор значений Console. Write. Line(list[i]); } } 19
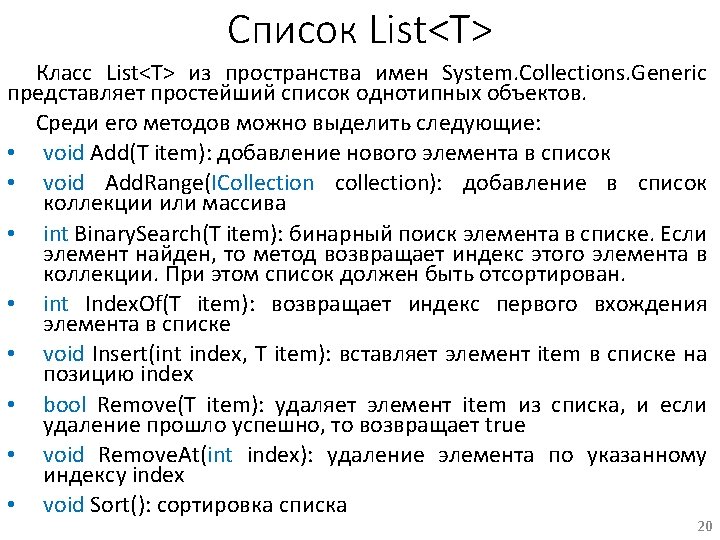
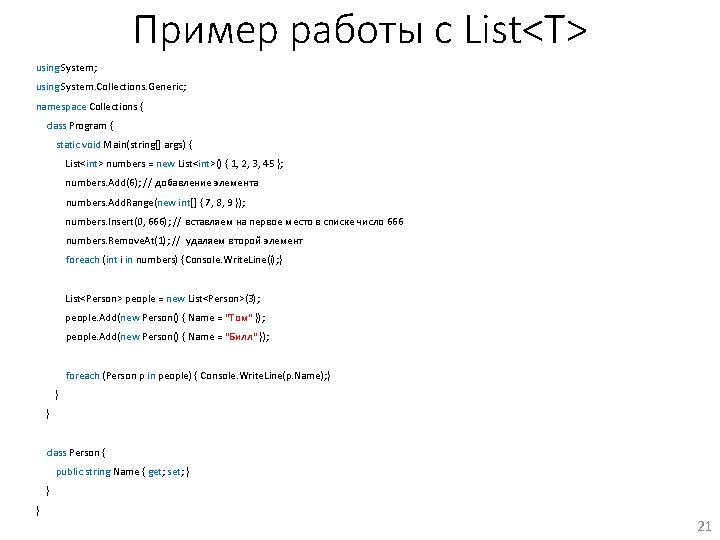
Пример работы с List<T> using System; using System. Collections. Generic; namespace Collections { class Program { static void Main(string[] args) { List<int> numbers = new List<int>() { 1, 2, 3, 45 }; numbers. Add(6); // добавление элемента numbers. Add. Range(new int[] { 7, 8, 9 }); numbers. Insert(0, 666); // вставляем на первое место в списке число 666 numbers. Remove. At(1); // удаляем второй элемент foreach (int i in numbers) {Console. Write. Line(i); } List<Person> people = new List<Person>(3); people. Add(new Person() { Name = "Том" }); people. Add(new Person() { Name = "Билл" }); foreach (Person p in people) { Console. Write. Line(p. Name); } } } class Person { public string Name { get; set; } } } 21
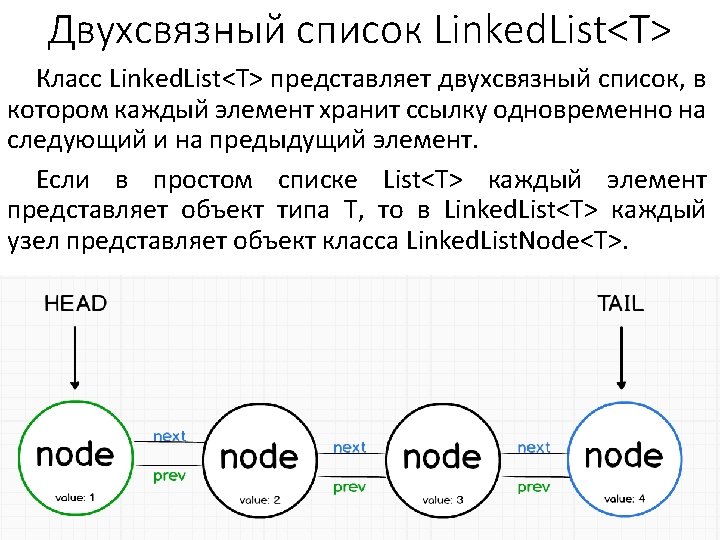
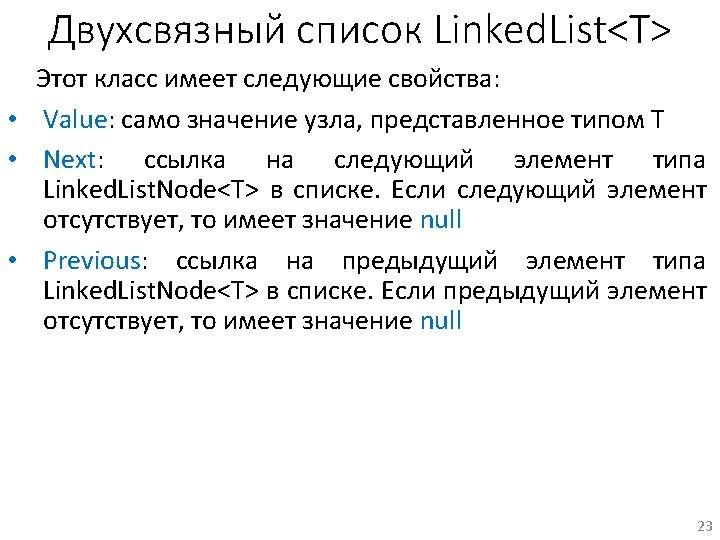
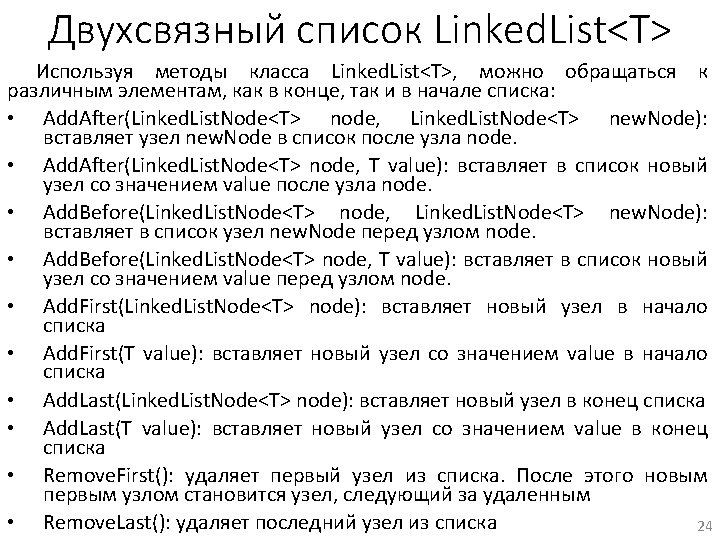
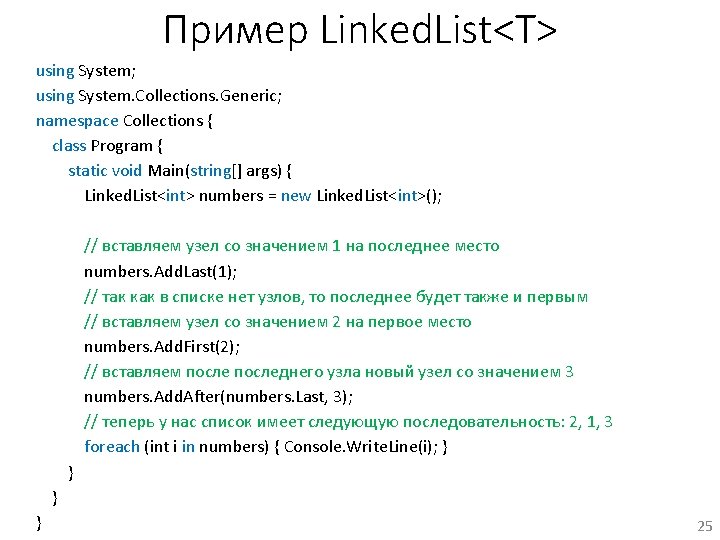
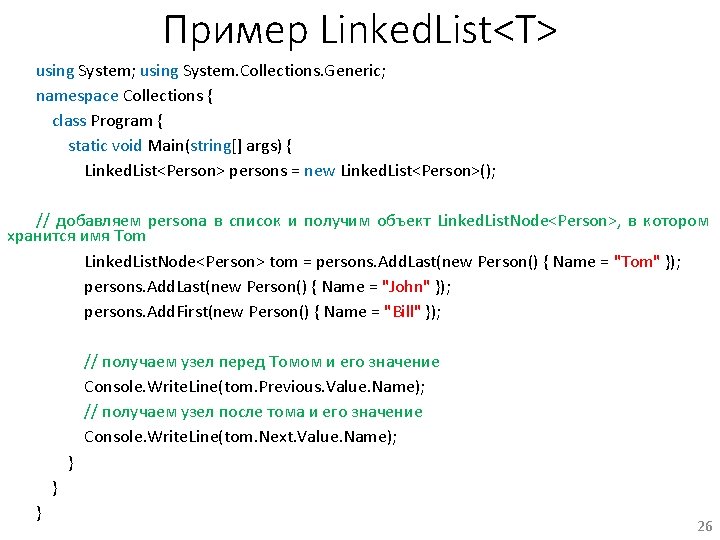
Пример Linked. List<T> using System; using System. Collections. Generic; namespace Collections { class Program { static void Main(string[] args) { Linked. List<Person> persons = new Linked. List<Person>(); // добавляем persona в список и получим объект Linked. List. Node<Person>, в котором хранится имя Tom Linked. List. Node<Person> tom = persons. Add. Last(new Person() { Name = "Tom" }); persons. Add. Last(new Person() { Name = "John" }); persons. Add. First(new Person() { Name = "Bill" }); // получаем узел перед Томом и его значение Console. Write. Line(tom. Previous. Value. Name); // получаем узел после тома и его значение Console. Write. Line(tom. Next. Value. Name); } } } 26
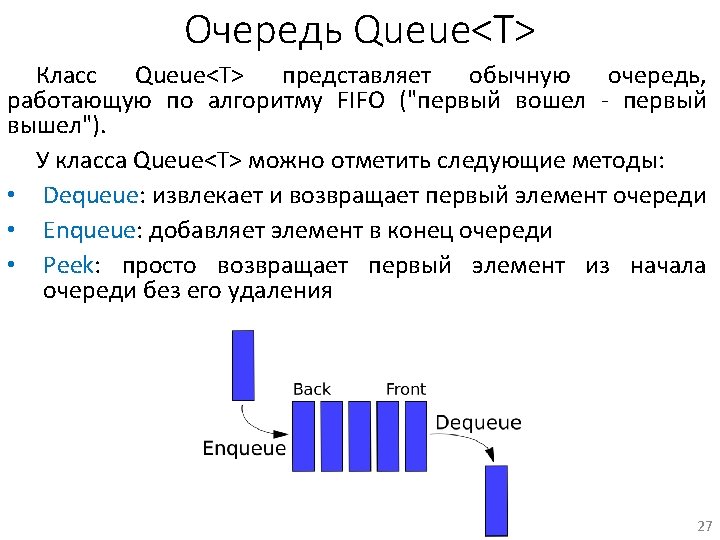
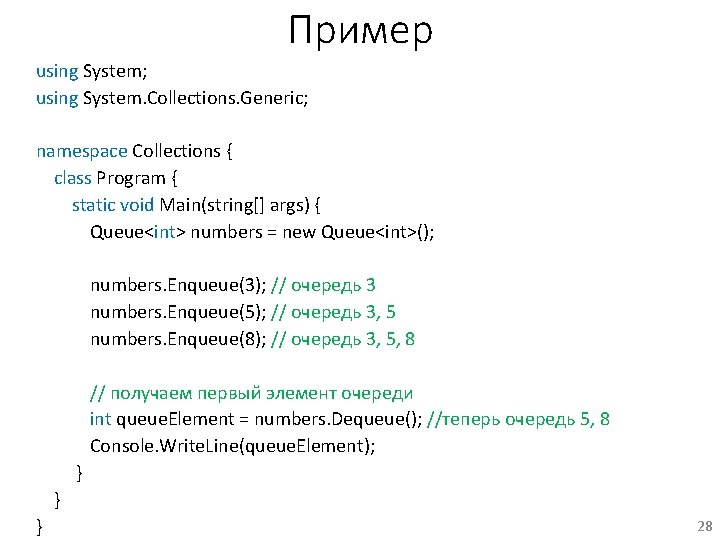
Пример using System; using System. Collections. Generic; namespace Collections { class Program { static void Main(string[] args) { Queue<int> numbers = new Queue<int>(); numbers. Enqueue(3); // очередь 3 numbers. Enqueue(5); // очередь 3, 5 numbers. Enqueue(8); // очередь 3, 5, 8 // получаем первый элемент очереди int queue. Element = numbers. Dequeue(); //теперь очередь 5, 8 Console. Write. Line(queue. Element); } } } 28
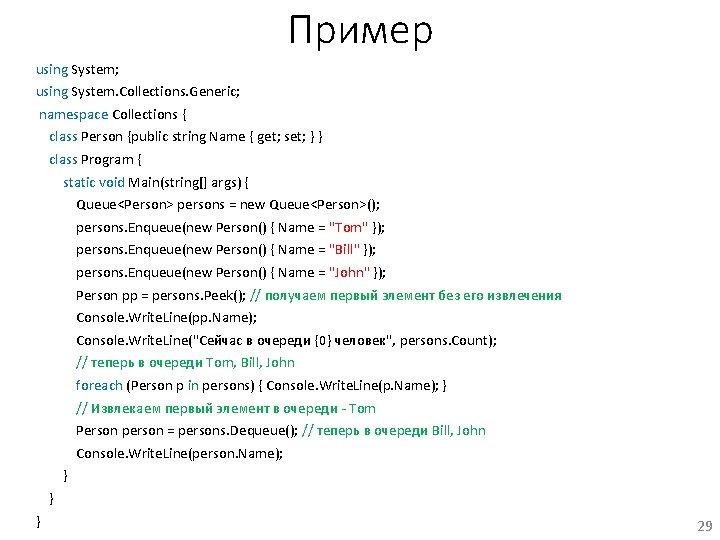
Пример using System; using System. Collections. Generic; namespace Collections { class Person {public string Name { get; set; } } class Program { static void Main(string[] args) { Queue<Person> persons = new Queue<Person>(); persons. Enqueue(new Person() { Name = "Tom" }); persons. Enqueue(new Person() { Name = "Bill" }); persons. Enqueue(new Person() { Name = "John" }); Person pp = persons. Peek(); // получаем первый элемент без его извлечения Console. Write. Line(pp. Name); Console. Write. Line("Сейчас в очереди {0} человек", persons. Count); // теперь в очереди Tom, Bill, John foreach (Person p in persons) { Console. Write. Line(p. Name); } // Извлекаем первый элемент в очереди - Tom Person person = persons. Dequeue(); // теперь в очереди Bill, John Console. Write. Line(person. Name); } } } 29
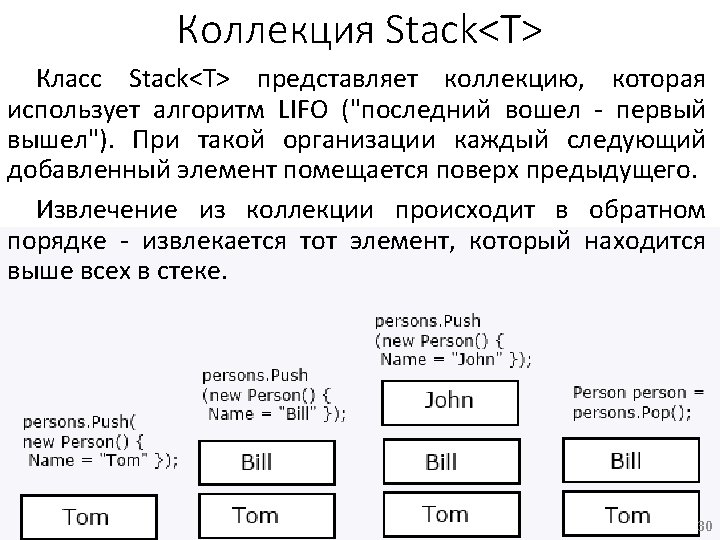
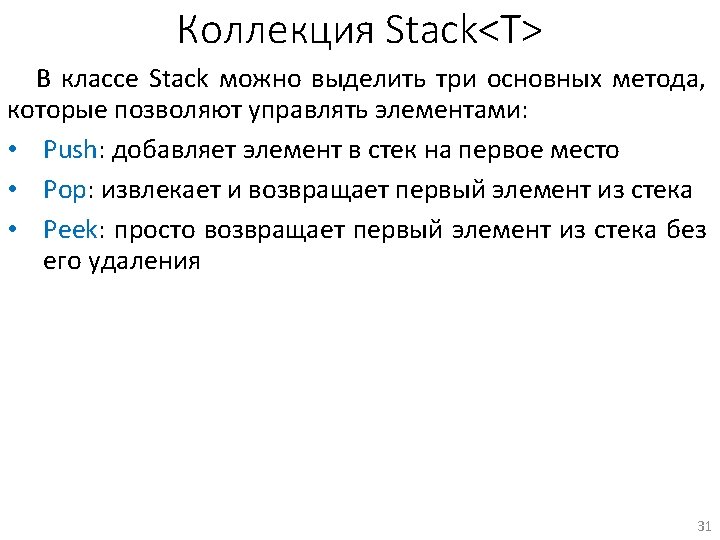
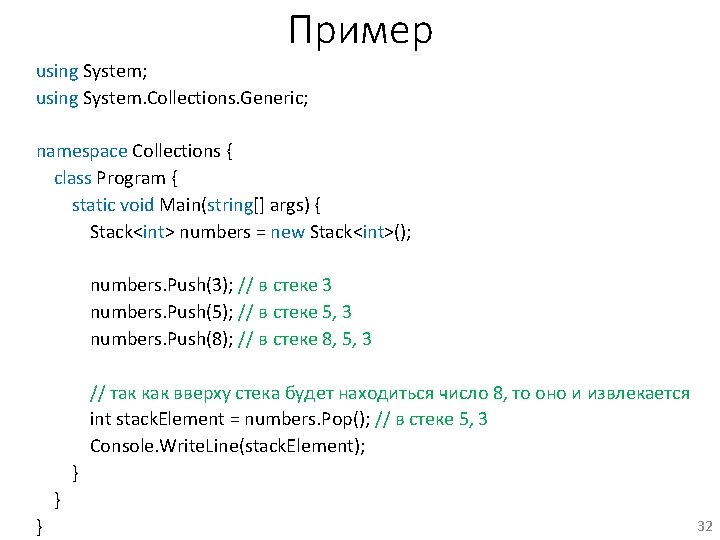
Пример using System; using System. Collections. Generic; namespace Collections { class Program { static void Main(string[] args) { Stack<int> numbers = new Stack<int>(); numbers. Push(3); // в стеке 3 numbers. Push(5); // в стеке 5, 3 numbers. Push(8); // в стеке 8, 5, 3 // так как вверху стека будет находиться число 8, то оно и извлекается int stack. Element = numbers. Pop(); // в стеке 5, 3 Console. Write. Line(stack. Element); } } } 32
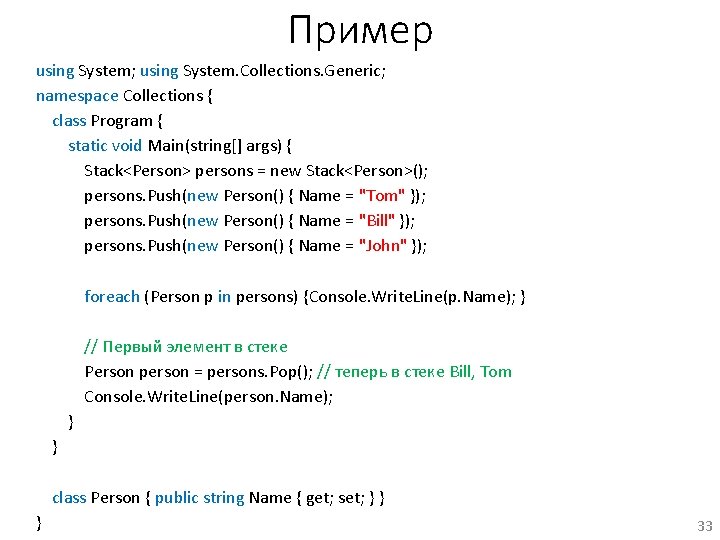
Пример using System; using System. Collections. Generic; namespace Collections { class Program { static void Main(string[] args) { Stack<Person> persons = new Stack<Person>(); persons. Push(new Person() { Name = "Tom" }); persons. Push(new Person() { Name = "Bill" }); persons. Push(new Person() { Name = "John" }); foreach (Person p in persons) {Console. Write. Line(p. Name); } // Первый элемент в стеке Person person = persons. Pop(); // теперь в стеке Bill, Tom Console. Write. Line(person. Name); } } class Person { public string Name { get; set; } } } 33
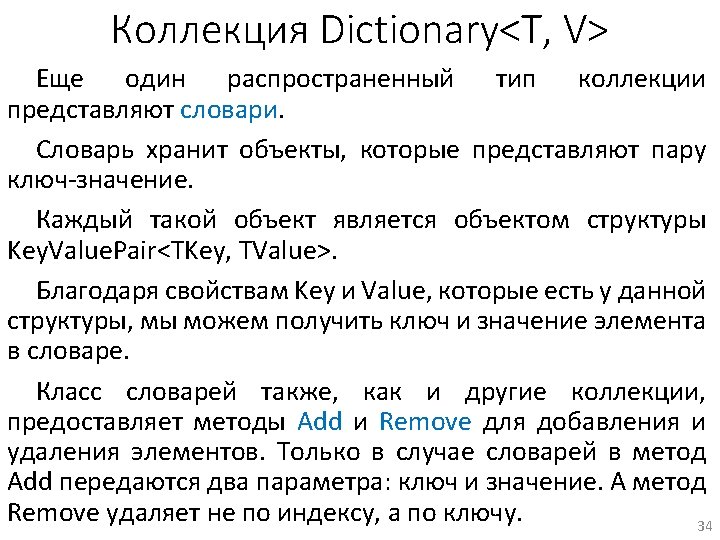
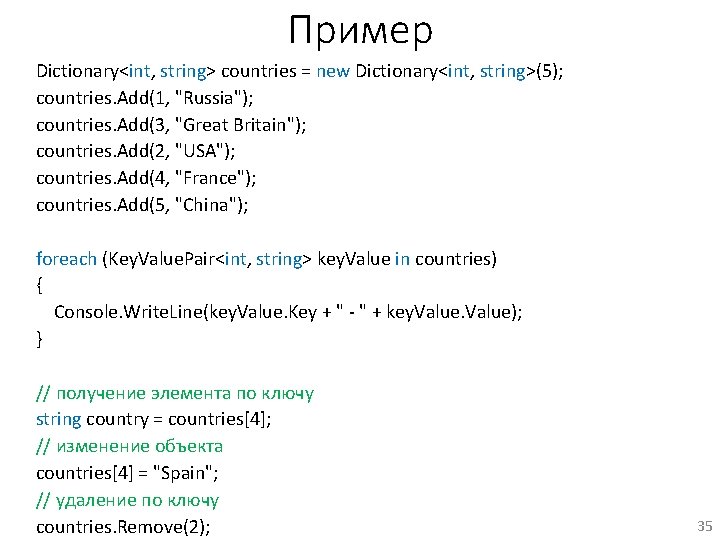
Пример Dictionary<int, string> countries = new Dictionary<int, string>(5); countries. Add(1, "Russia"); countries. Add(3, "Great Britain"); countries. Add(2, "USA"); countries. Add(4, "France"); countries. Add(5, "China"); foreach (Key. Value. Pair<int, string> key. Value in countries) { Console. Write. Line(key. Value. Key + " - " + key. Value); } // получение элемента по ключу string country = countries[4]; // изменение объекта countries[4] = "Spain"; // удаление по ключу countries. Remove(2); 35
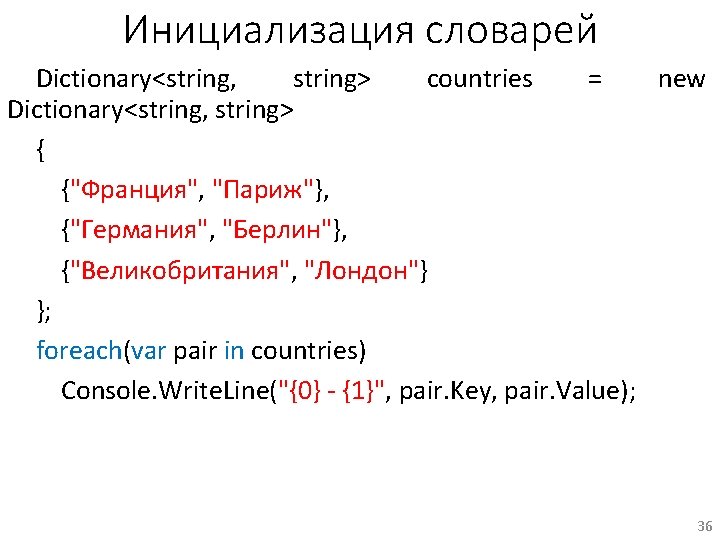
Инициализация словарей Dictionary<string, string> countries = new Dictionary<string, string> { {"Франция", "Париж"}, {"Германия", "Берлин"}, {"Великобритания", "Лондон"} }; foreach(var pair in countries) Console. Write. Line("{0} - {1}", pair. Key, pair. Value); 36
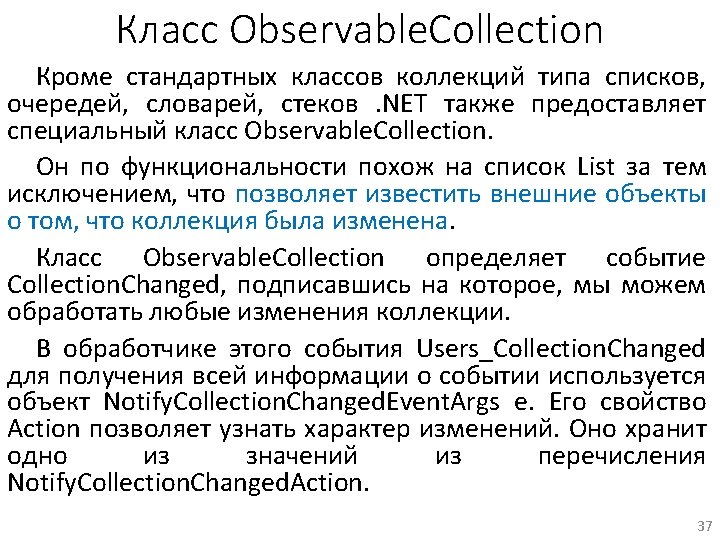
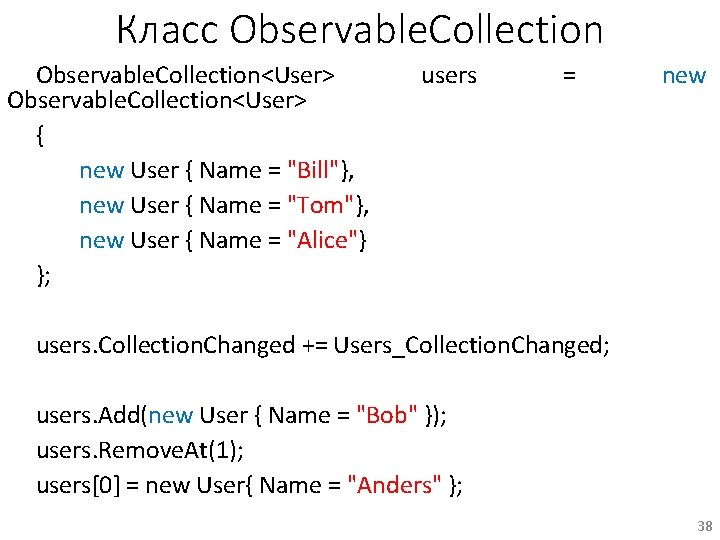
Класс Observable. Collection<User> { new User { Name = "Bill"}, new User { Name = "Tom"}, new User { Name = "Alice"} }; users = new users. Collection. Changed += Users_Collection. Changed; users. Add(new User { Name = "Bob" }); users. Remove. At(1); users[0] = new User{ Name = "Anders" }; 38
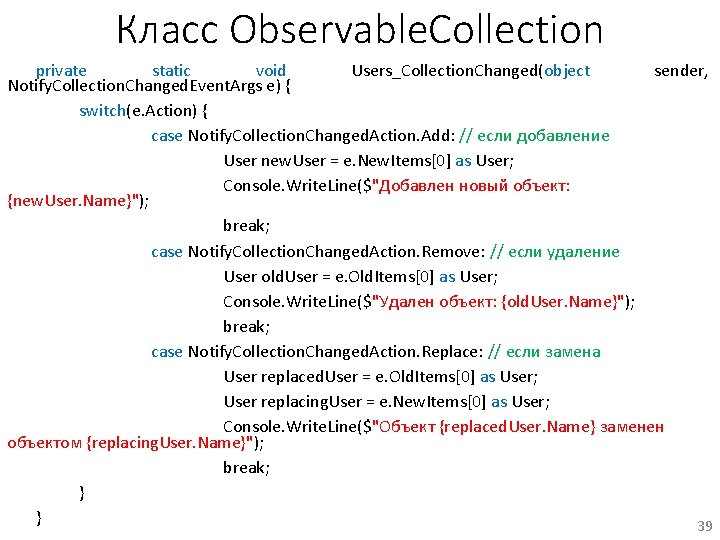
Класс Observable. Collection private static void Users_Collection. Changed(object sender, Notify. Collection. Changed. Event. Args e) { switch(e. Action) { case Notify. Collection. Changed. Action. Add: // если добавление User new. User = e. New. Items[0] as User; Console. Write. Line($"Добавлен новый объект: {new. User. Name}"); break; case Notify. Collection. Changed. Action. Remove: // если удаление User old. User = e. Old. Items[0] as User; Console. Write. Line($"Удален объект: {old. User. Name}"); break; case Notify. Collection. Changed. Action. Replace: // если замена User replaced. User = e. Old. Items[0] as User; User replacing. User = e. New. Items[0] as User; Console. Write. Line($"Объект {replaced. User. Name} заменен объектом {replacing. User. Name}"); break; } } 39
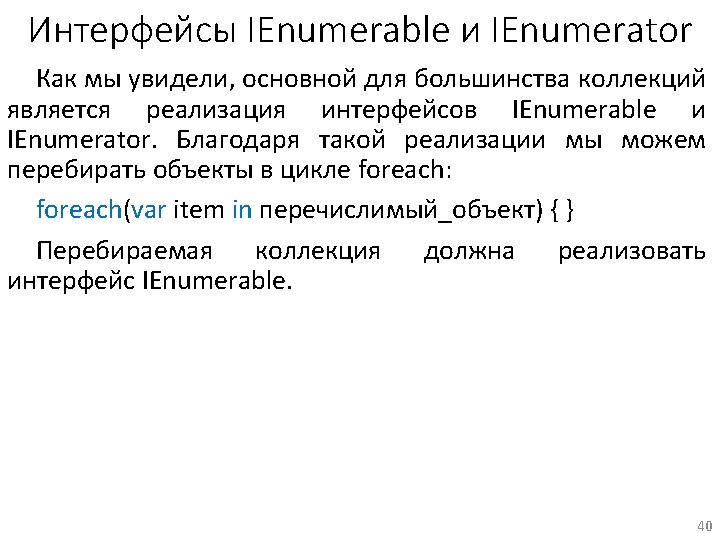
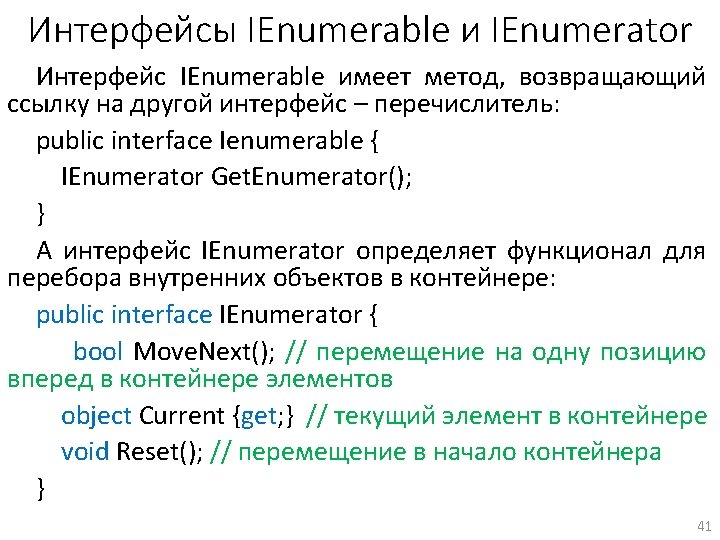
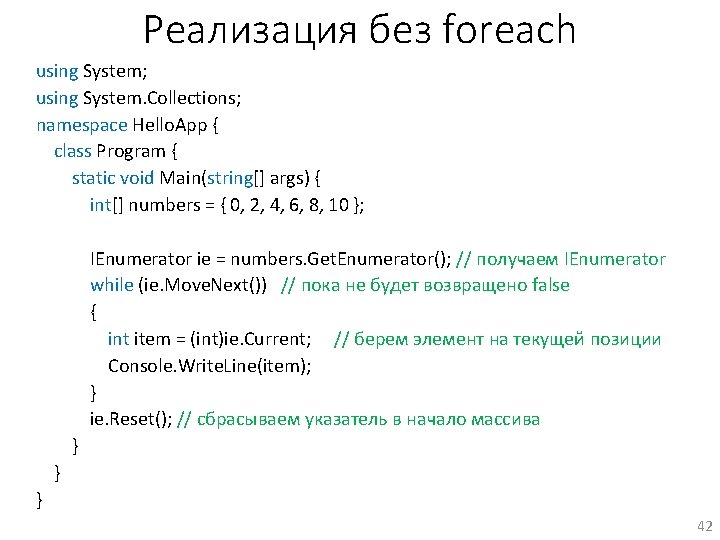
Реализация без foreach using System; using System. Collections; namespace Hello. App { class Program { static void Main(string[] args) { int[] numbers = { 0, 2, 4, 6, 8, 10 }; IEnumerator ie = numbers. Get. Enumerator(); // получаем IEnumerator while (ie. Move. Next()) // пока не будет возвращено false { int item = (int)ie. Current; // берем элемент на текущей позиции Console. Write. Line(item); } ie. Reset(); // сбрасываем указатель в начало массива } } } 42
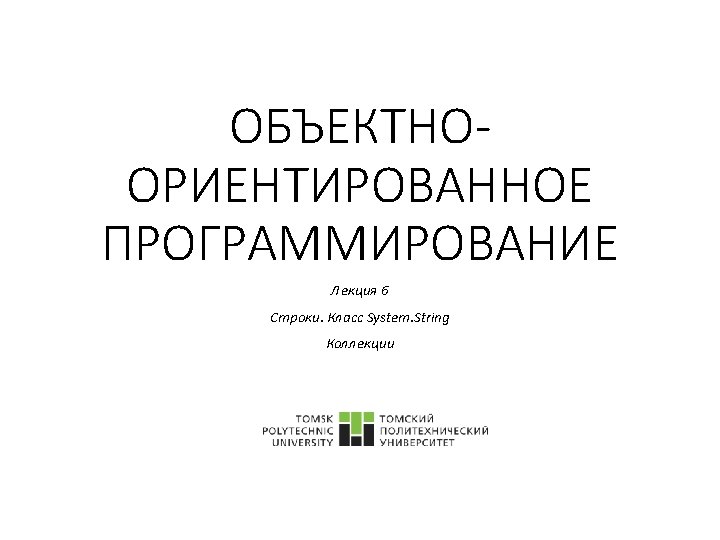
Class operators public static void main
Public static void main
Class tester public static void main
Public class x public static void main
Public class x
Public class x public static void main
Public static void main(string[] args){
Public static void main (string args) is a
Public static void main int args
Mainstring
Public static void main(string args)
Mainstring
Void setup(void)
Void main(void)
Void main(void)
Consolewrite
Static class loading and dynamic class loading
Analysis class diagram example
Public static void main string args
Exitint
Public static void
A____block enclose the code that could throw an exception.
Linked list diagram in java
Tonight i can write by pablo neruda summary
Classification of surveying
Class a void foo() throws exception
Class a void foo() throws exception
Public abstract class fish private void swim
Are all the methods in the collections class static?
Uml class diagram static
Uml static method
Public class welcome
Class math static int x
Snmpc management console
Eat sleep console scoring sheet
Cisco arc console
Quinta generazione console
Vts console
Nuuo main console
Smartdock av control console
Geneos tutorial
Igel ums firmware update
Getch vs getchar
Application virtualization client management console