CMSC 341 Linked Lists Stacks and Queues 832007
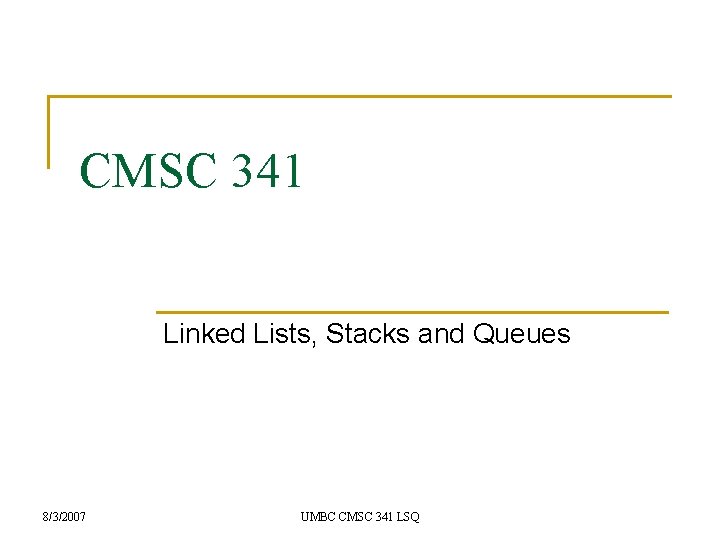
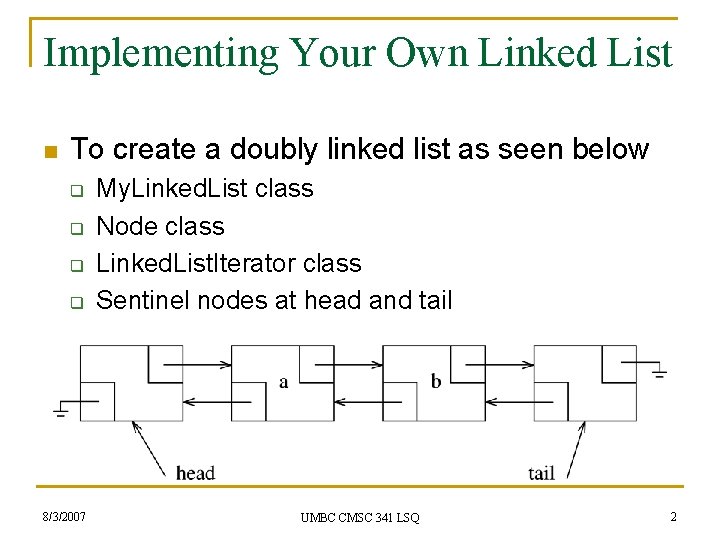
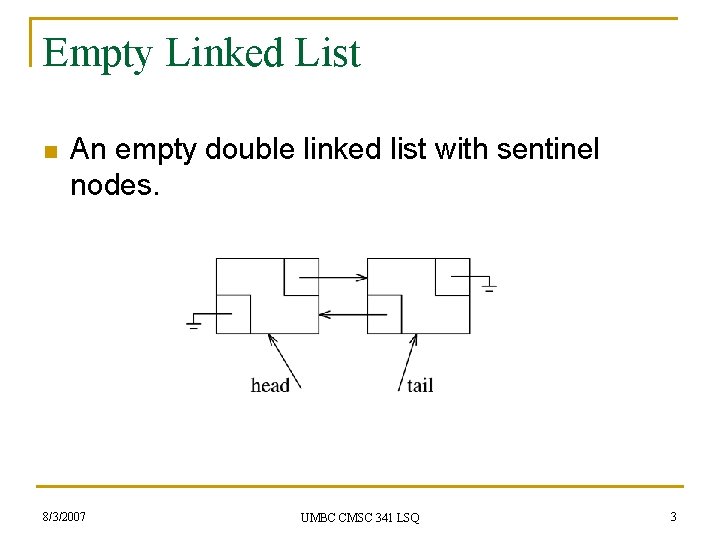
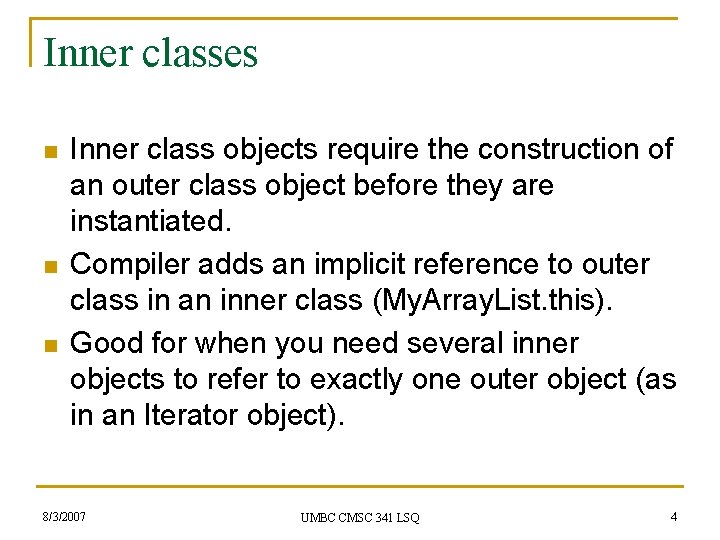
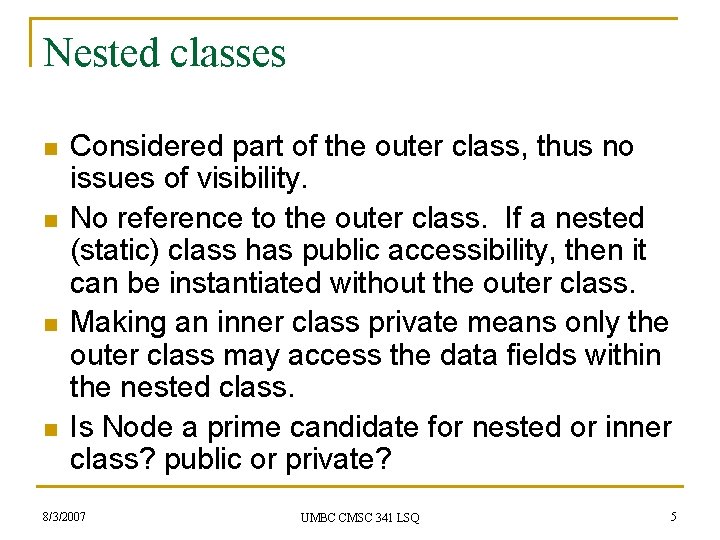
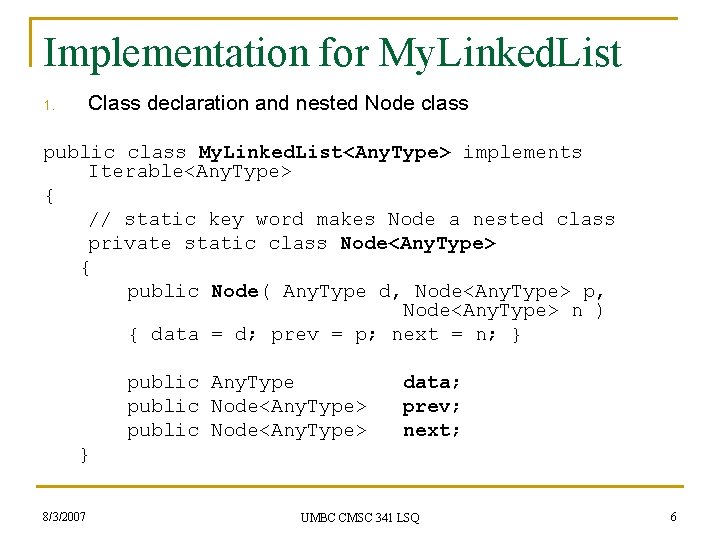
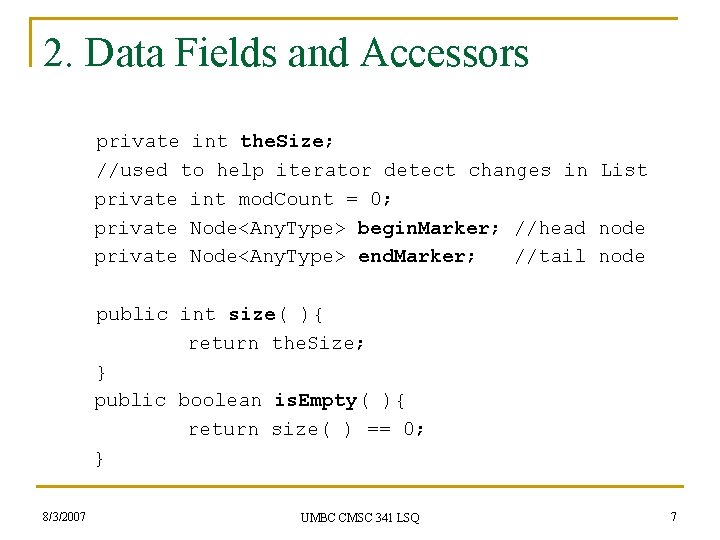
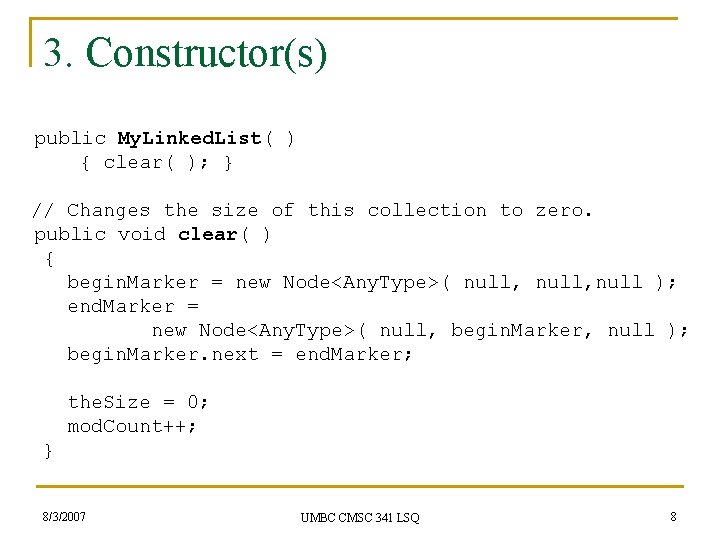
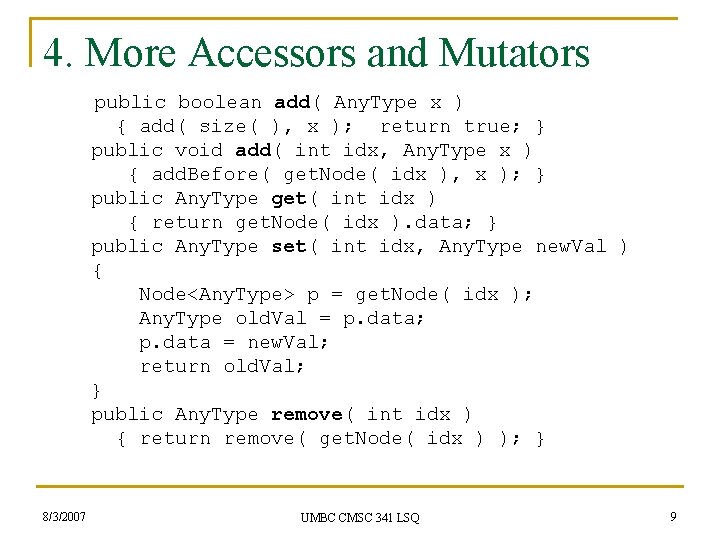
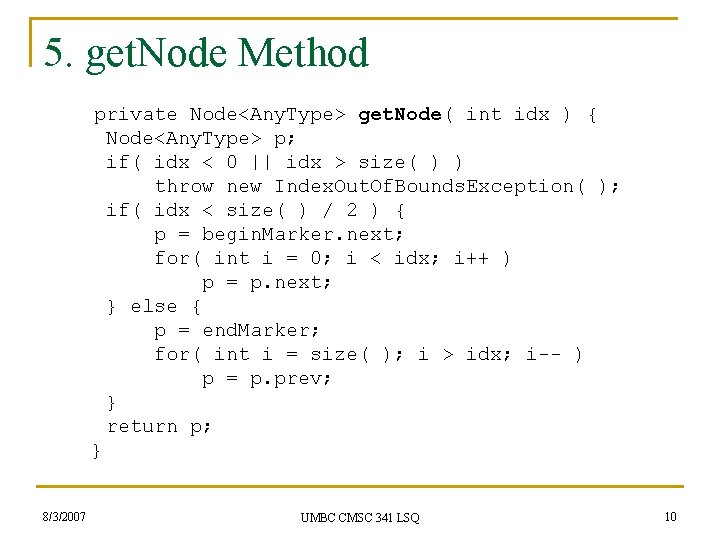
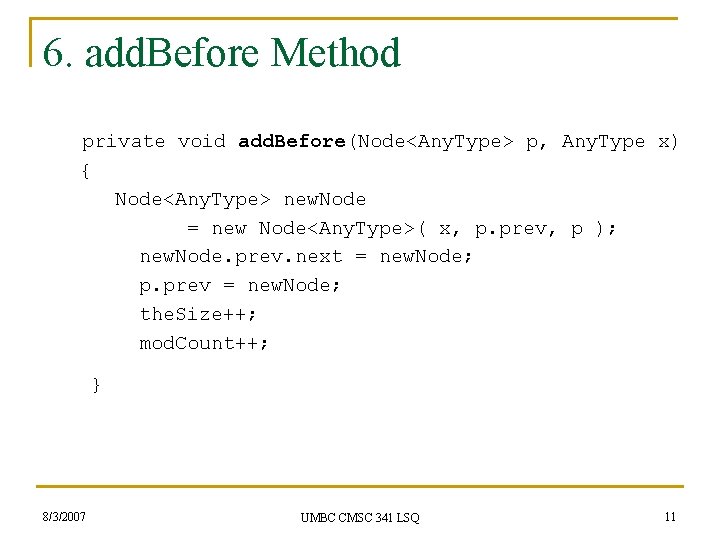
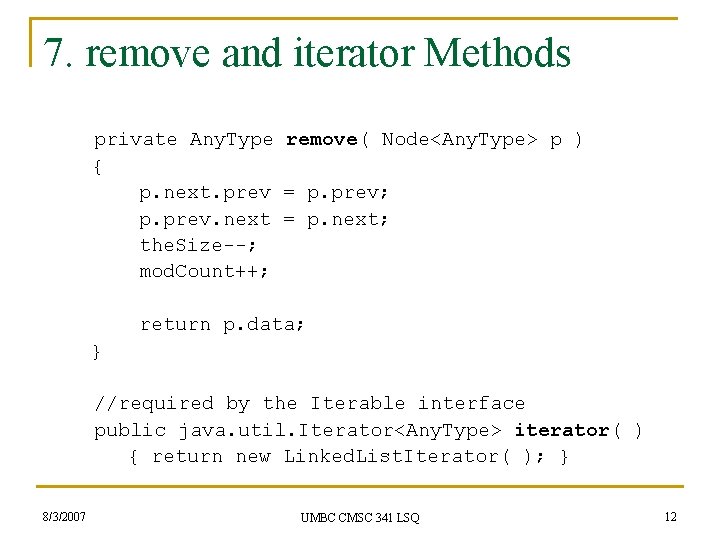
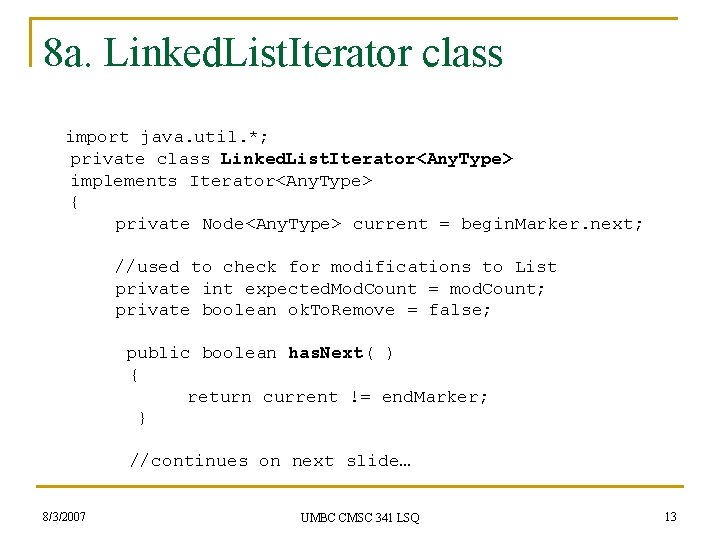
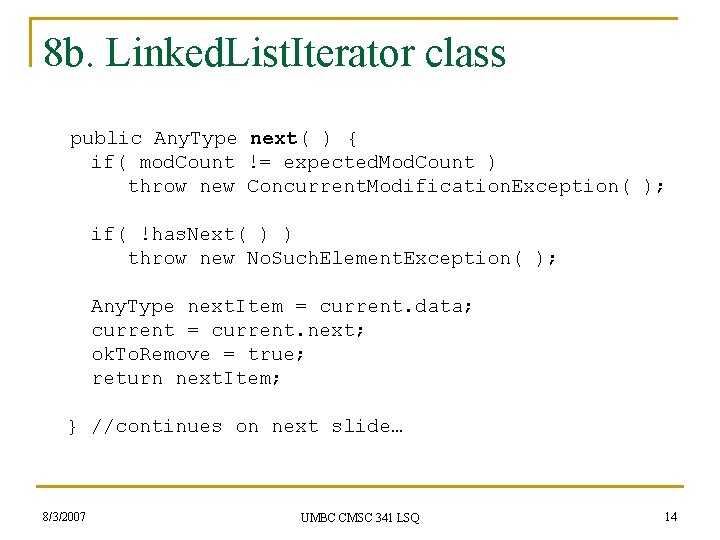
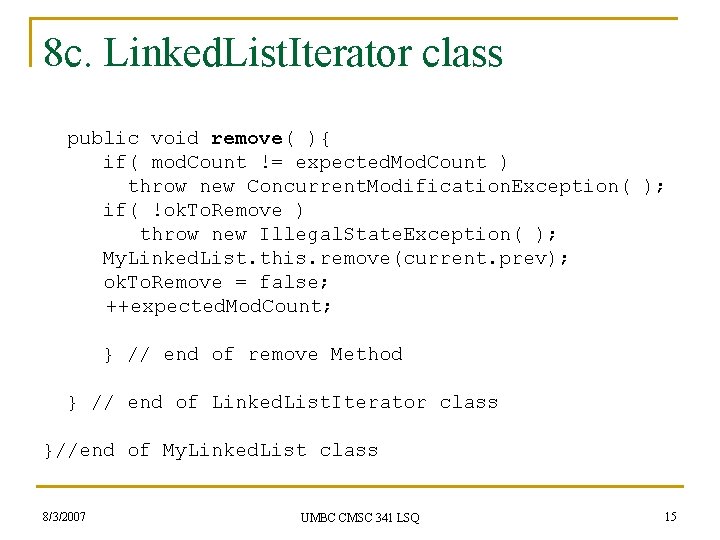
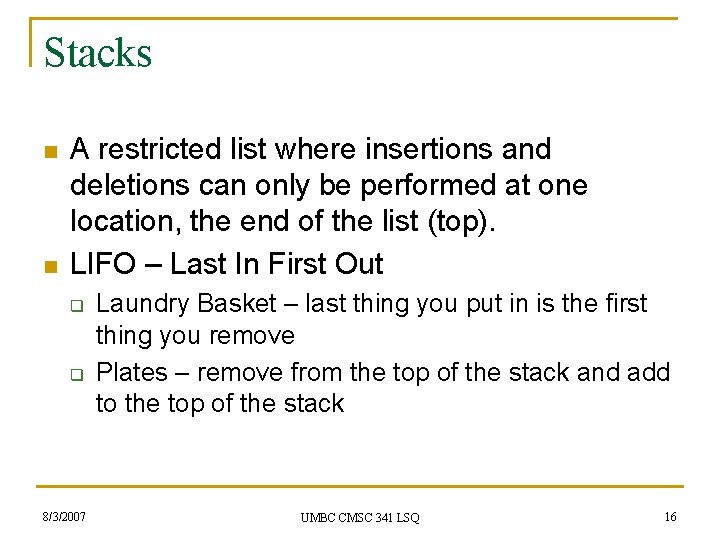
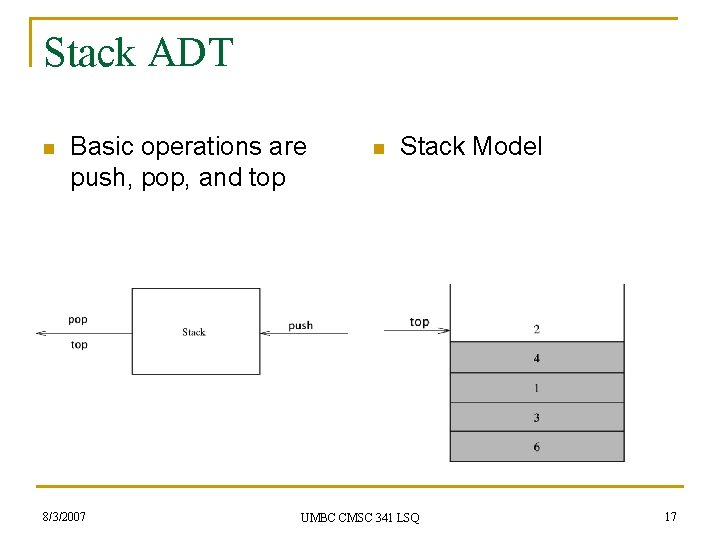
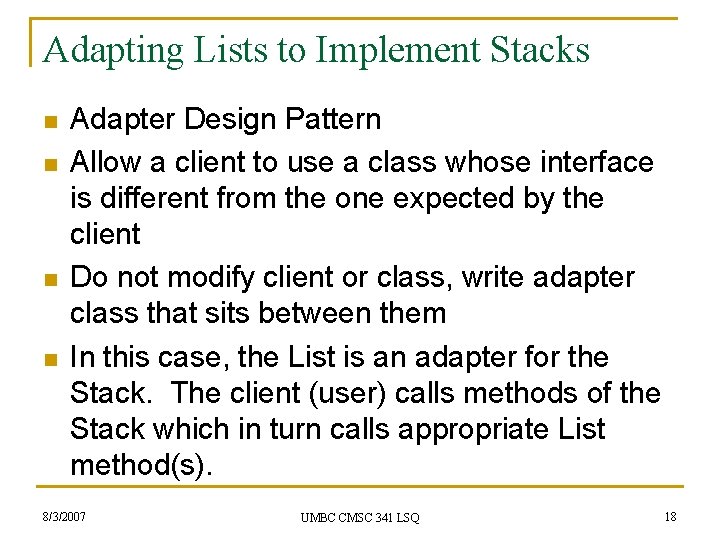
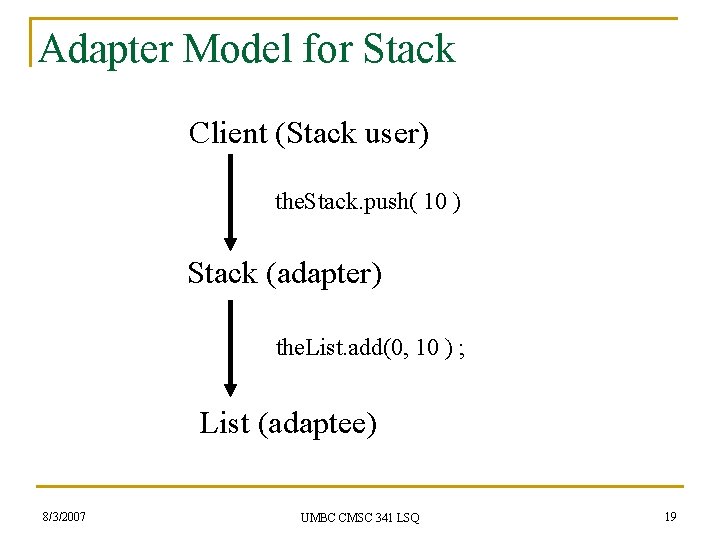
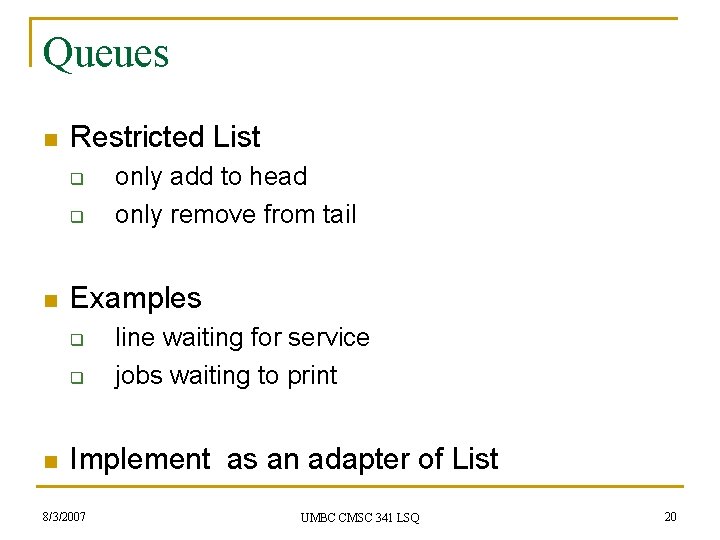
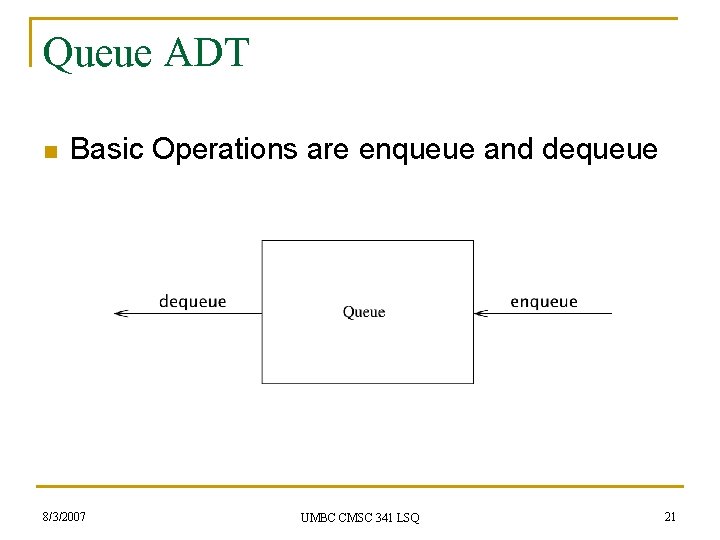
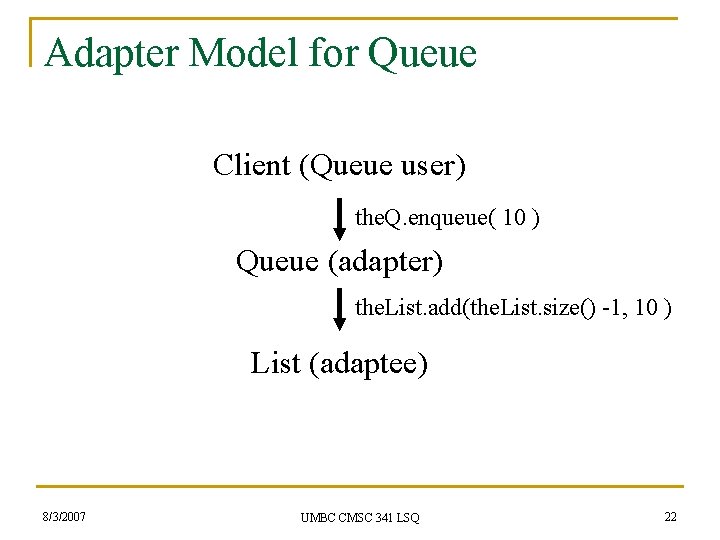
- Slides: 22
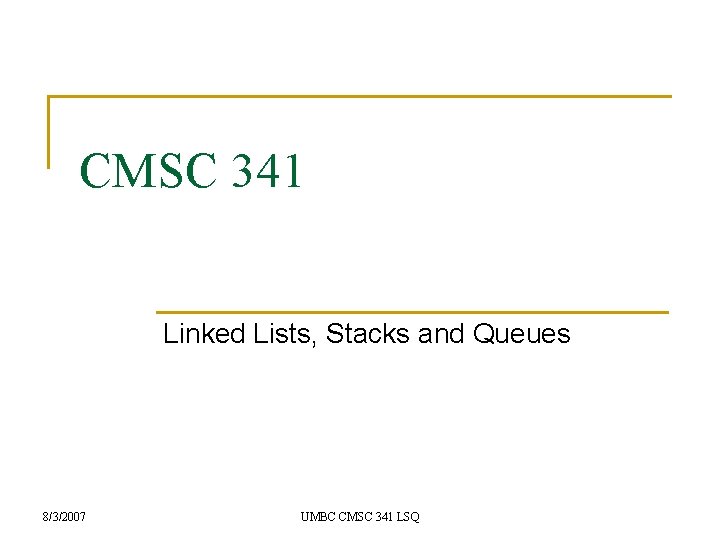
CMSC 341 Linked Lists, Stacks and Queues 8/3/2007 UMBC CMSC 341 LSQ
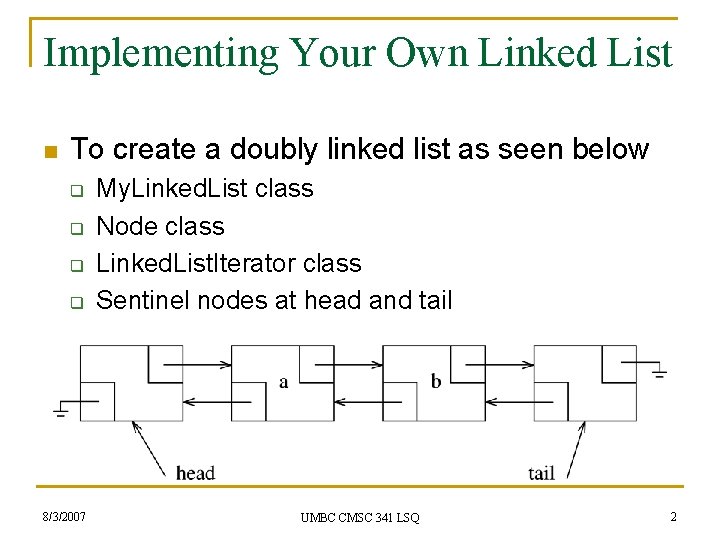
Implementing Your Own Linked List n To create a doubly linked list as seen below q q 8/3/2007 My. Linked. List class Node class Linked. List. Iterator class Sentinel nodes at head and tail UMBC CMSC 341 LSQ 2
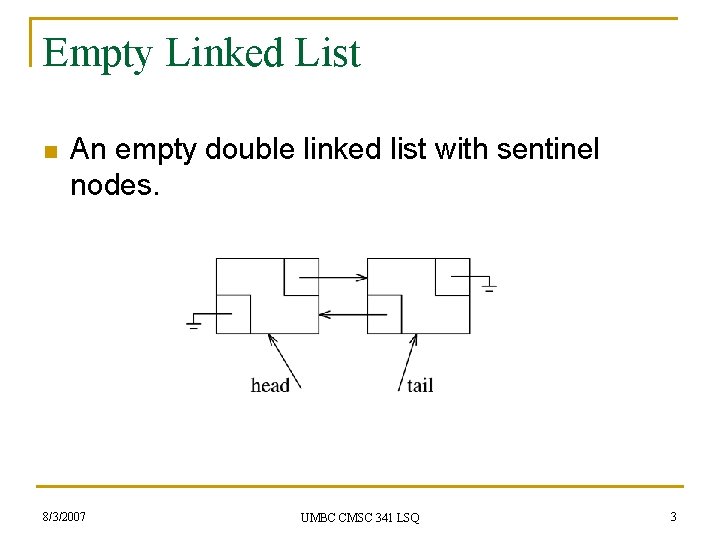
Empty Linked List n An empty double linked list with sentinel nodes. 8/3/2007 UMBC CMSC 341 LSQ 3
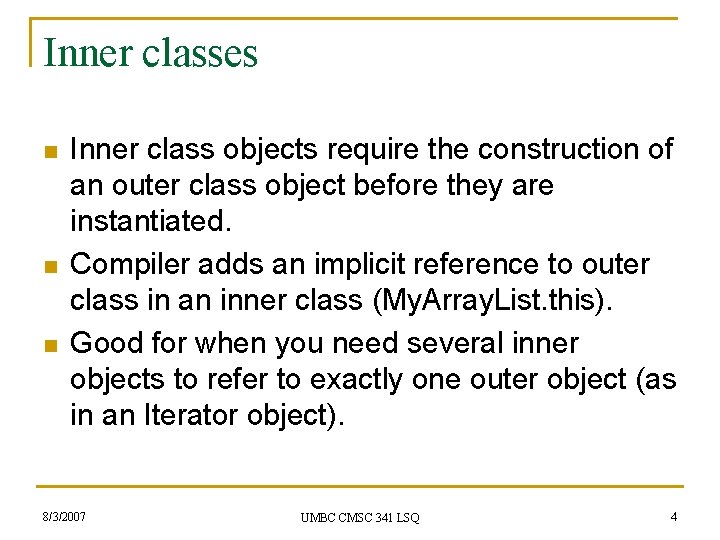
Inner classes n n n Inner class objects require the construction of an outer class object before they are instantiated. Compiler adds an implicit reference to outer class in an inner class (My. Array. List. this). Good for when you need several inner objects to refer to exactly one outer object (as in an Iterator object). 8/3/2007 UMBC CMSC 341 LSQ 4
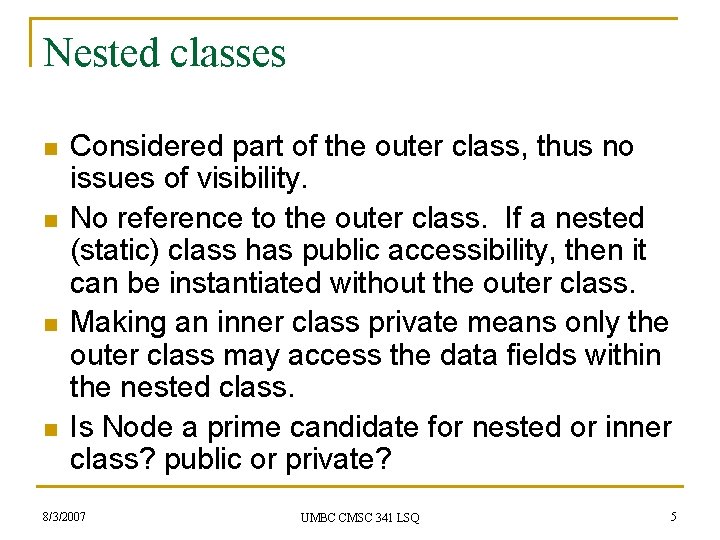
Nested classes n n Considered part of the outer class, thus no issues of visibility. No reference to the outer class. If a nested (static) class has public accessibility, then it can be instantiated without the outer class. Making an inner class private means only the outer class may access the data fields within the nested class. Is Node a prime candidate for nested or inner class? public or private? 8/3/2007 UMBC CMSC 341 LSQ 5
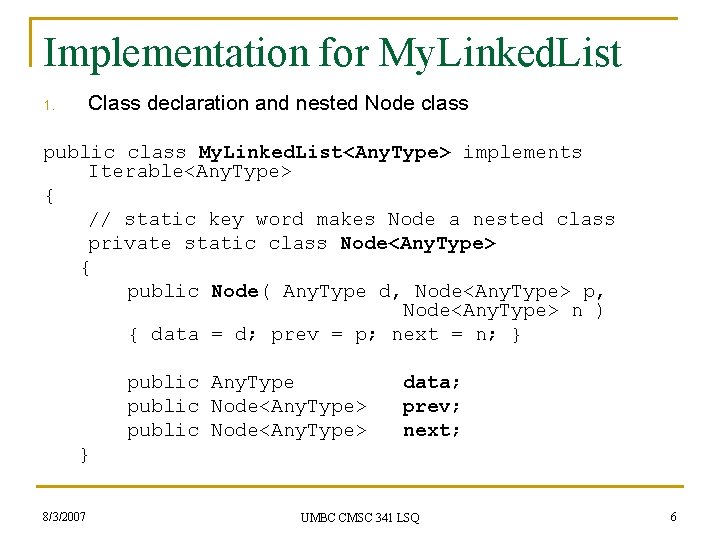
Implementation for My. Linked. List Class declaration and nested Node class 1. public class My. Linked. List<Any. Type> implements Iterable<Any. Type> { // static key word makes Node a nested class private static class Node<Any. Type> { public Node( Any. Type d, Node<Any. Type> p, Node<Any. Type> n ) { data = d; prev = p; next = n; } public Any. Type public Node<Any. Type> data; prev; next; } 8/3/2007 UMBC CMSC 341 LSQ 6
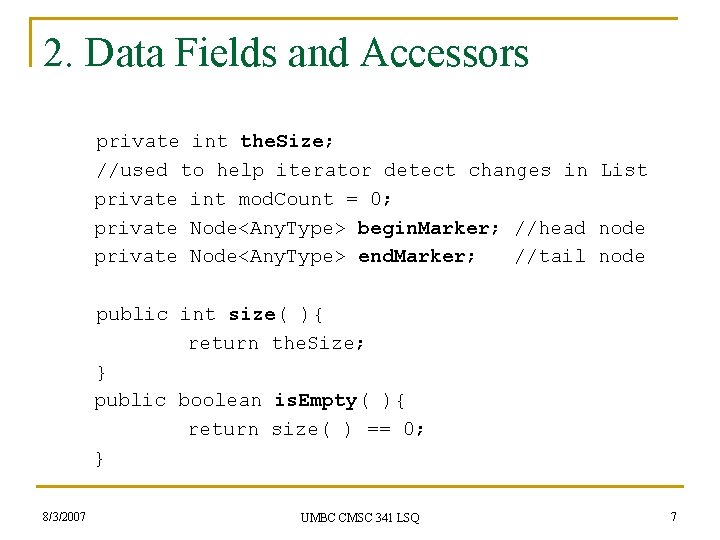
2. Data Fields and Accessors private int the. Size; //used to help iterator detect changes in List private int mod. Count = 0; private Node<Any. Type> begin. Marker; //head node private Node<Any. Type> end. Marker; //tail node public int size( ){ return the. Size; } public boolean is. Empty( ){ return size( ) == 0; } 8/3/2007 UMBC CMSC 341 LSQ 7
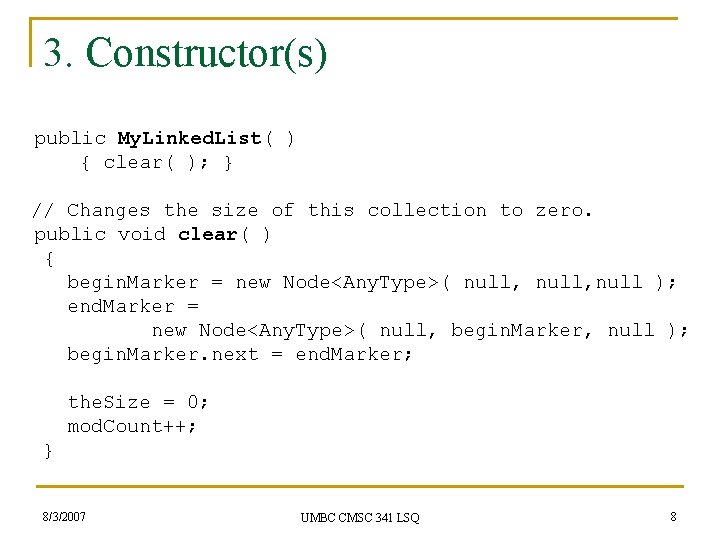
3. Constructor(s) public My. Linked. List( ) { clear( ); } // Changes the size of this collection to zero. public void clear( ) { begin. Marker = new Node<Any. Type>( null, null ); end. Marker = new Node<Any. Type>( null, begin. Marker, null ); begin. Marker. next = end. Marker; the. Size = 0; mod. Count++; } 8/3/2007 UMBC CMSC 341 LSQ 8
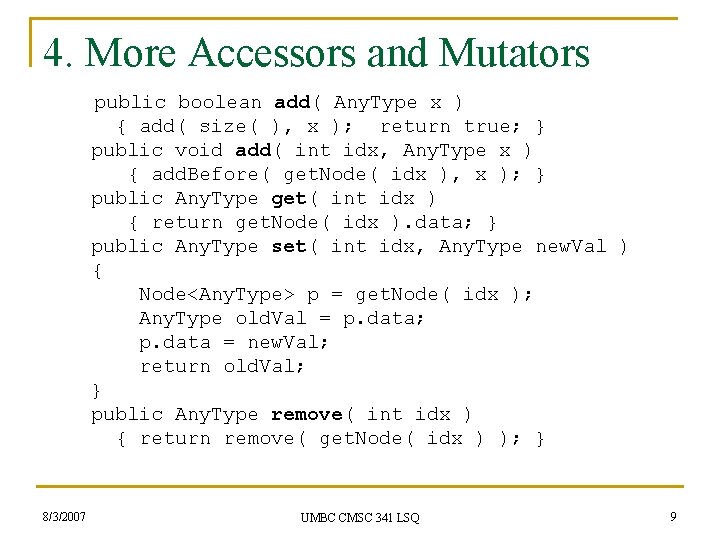
4. More Accessors and Mutators public boolean add( Any. Type x ) { add( size( ), x ); return true; } public void add( int idx, Any. Type x ) { add. Before( get. Node( idx ), x ); } public Any. Type get( int idx ) { return get. Node( idx ). data; } public Any. Type set( int idx, Any. Type new. Val ) { Node<Any. Type> p = get. Node( idx ); Any. Type old. Val = p. data; p. data = new. Val; return old. Val; } public Any. Type remove( int idx ) { return remove( get. Node( idx ) ); } 8/3/2007 UMBC CMSC 341 LSQ 9
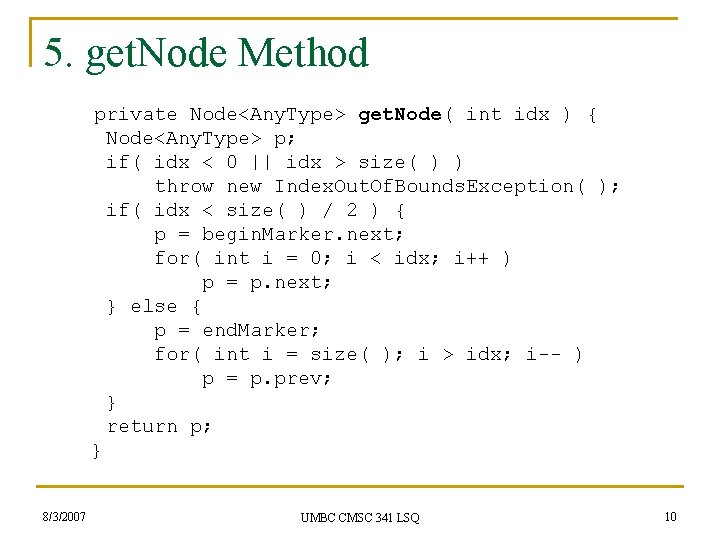
5. get. Node Method private Node<Any. Type> get. Node( int idx ) { Node<Any. Type> p; if( idx < 0 || idx > size( ) ) throw new Index. Out. Of. Bounds. Exception( ); if( idx < size( ) / 2 ) { p = begin. Marker. next; for( int i = 0; i < idx; i++ ) p = p. next; } else { p = end. Marker; for( int i = size( ); i > idx; i-- ) p = p. prev; } return p; } 8/3/2007 UMBC CMSC 341 LSQ 10
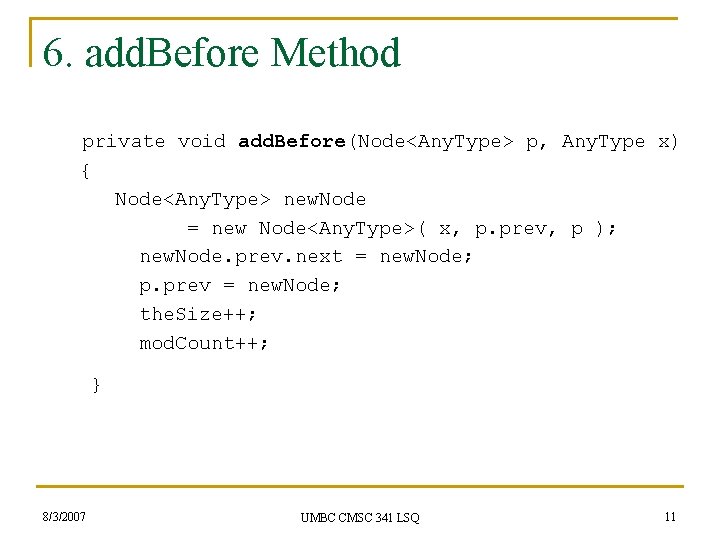
6. add. Before Method private void add. Before(Node<Any. Type> p, Any. Type x) { Node<Any. Type> new. Node = new Node<Any. Type>( x, p. prev, p ); new. Node. prev. next = new. Node; p. prev = new. Node; the. Size++; mod. Count++; } 8/3/2007 UMBC CMSC 341 LSQ 11
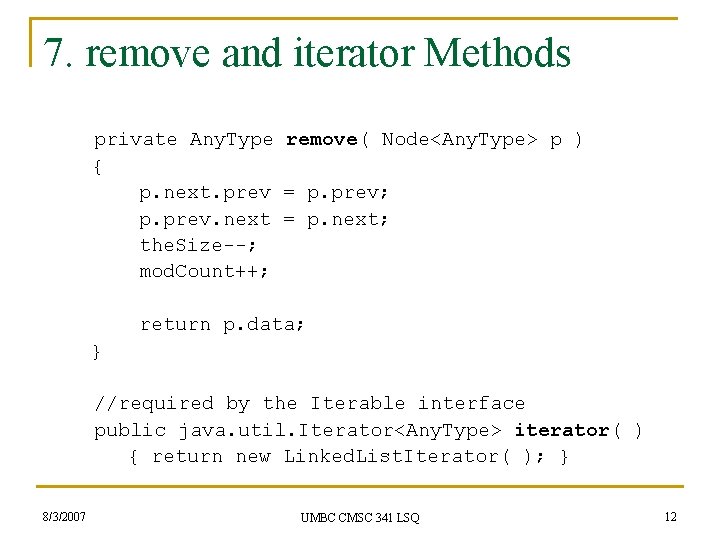
7. remove and iterator Methods private Any. Type remove( Node<Any. Type> p ) { p. next. prev = p. prev; p. prev. next = p. next; the. Size--; mod. Count++; return p. data; } //required by the Iterable interface public java. util. Iterator<Any. Type> iterator( ) { return new Linked. List. Iterator( ); } 8/3/2007 UMBC CMSC 341 LSQ 12
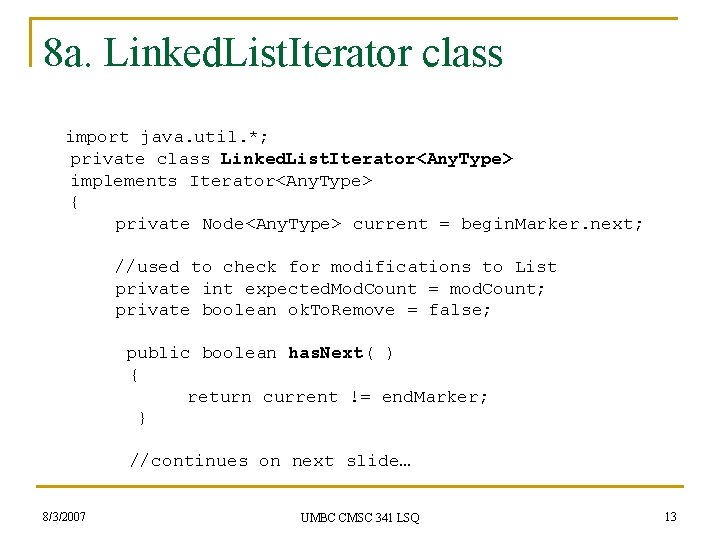
8 a. Linked. List. Iterator class import java. util. *; private class Linked. List. Iterator<Any. Type> implements Iterator<Any. Type> { private Node<Any. Type> current = begin. Marker. next; //used to check for modifications to List private int expected. Mod. Count = mod. Count; private boolean ok. To. Remove = false; public boolean has. Next( ) { return current != end. Marker; } //continues on next slide… 8/3/2007 UMBC CMSC 341 LSQ 13
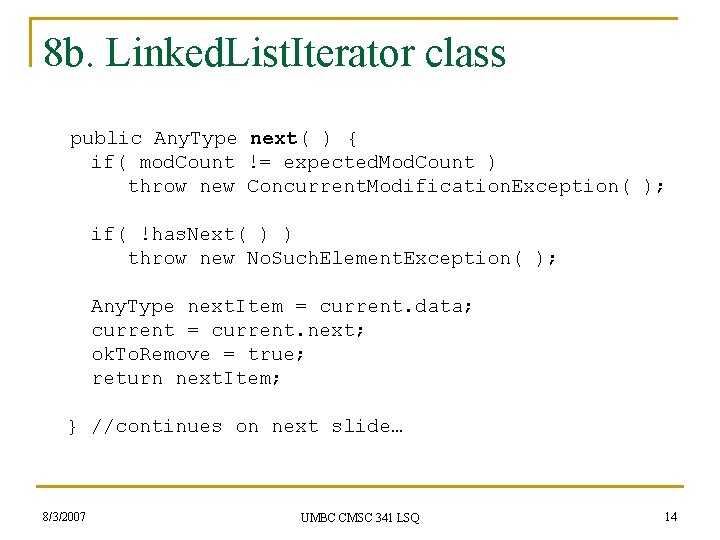
8 b. Linked. List. Iterator class public Any. Type next( ) { if( mod. Count != expected. Mod. Count ) throw new Concurrent. Modification. Exception( ); if( !has. Next( ) ) throw new No. Such. Element. Exception( ); Any. Type next. Item = current. data; current = current. next; ok. To. Remove = true; return next. Item; } //continues on next slide… 8/3/2007 UMBC CMSC 341 LSQ 14
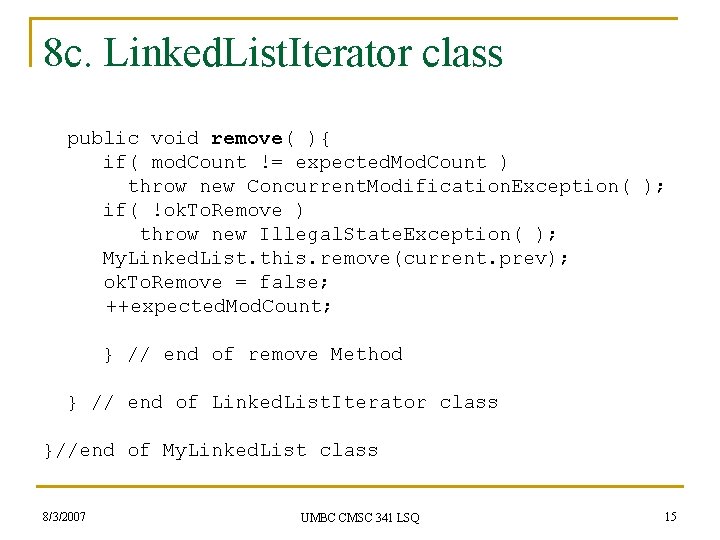
8 c. Linked. List. Iterator class public void remove( ){ if( mod. Count != expected. Mod. Count ) throw new Concurrent. Modification. Exception( ); if( !ok. To. Remove ) throw new Illegal. State. Exception( ); My. Linked. List. this. remove(current. prev); ok. To. Remove = false; ++expected. Mod. Count; } // end of remove Method } // end of Linked. List. Iterator class }//end of My. Linked. List class 8/3/2007 UMBC CMSC 341 LSQ 15
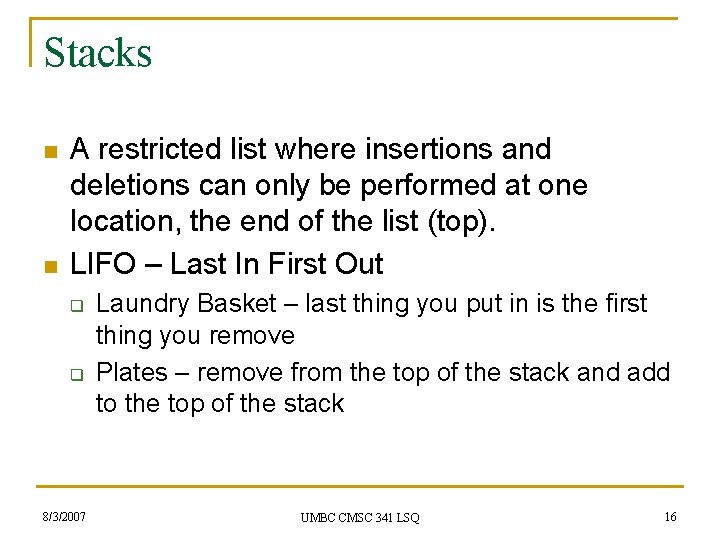
Stacks n n A restricted list where insertions and deletions can only be performed at one location, the end of the list (top). LIFO – Last In First Out q q 8/3/2007 Laundry Basket – last thing you put in is the first thing you remove Plates – remove from the top of the stack and add to the top of the stack UMBC CMSC 341 LSQ 16
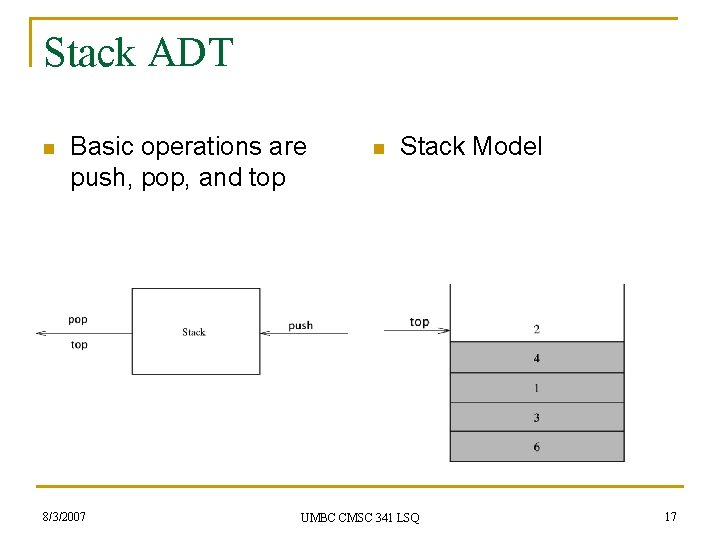
Stack ADT n Basic operations are push, pop, and top 8/3/2007 n Stack Model UMBC CMSC 341 LSQ 17
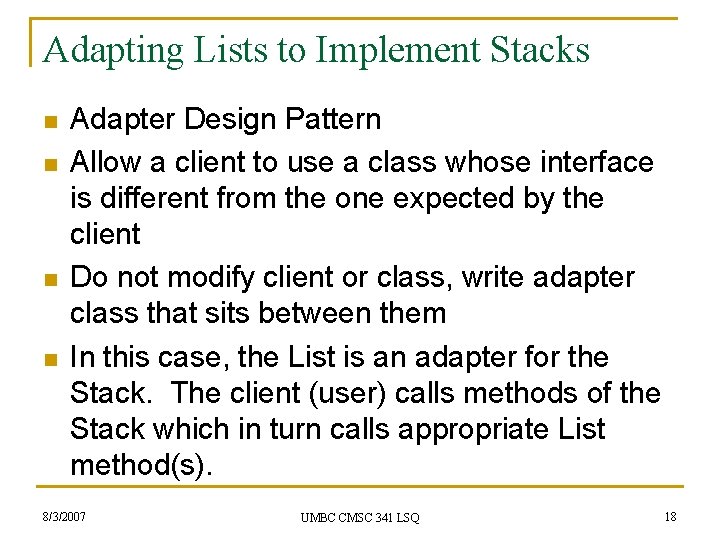
Adapting Lists to Implement Stacks n n Adapter Design Pattern Allow a client to use a class whose interface is different from the one expected by the client Do not modify client or class, write adapter class that sits between them In this case, the List is an adapter for the Stack. The client (user) calls methods of the Stack which in turn calls appropriate List method(s). 8/3/2007 UMBC CMSC 341 LSQ 18
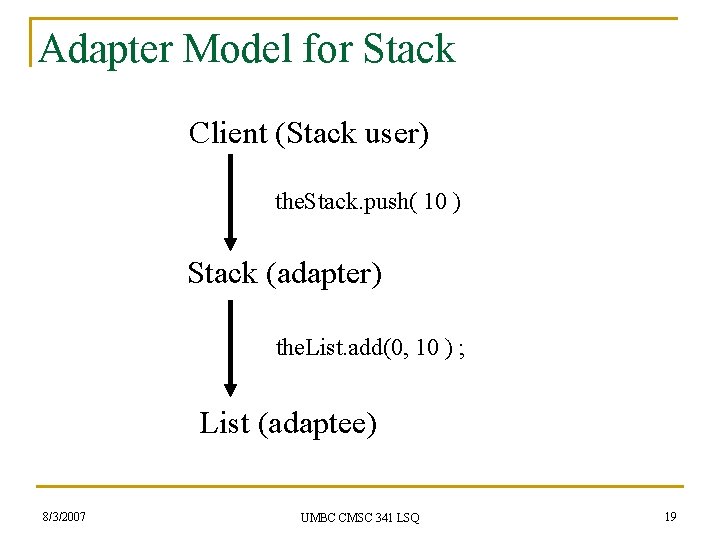
Adapter Model for Stack Client (Stack user) the. Stack. push( 10 ) Stack (adapter) the. List. add(0, 10 ) ; List (adaptee) 8/3/2007 UMBC CMSC 341 LSQ 19
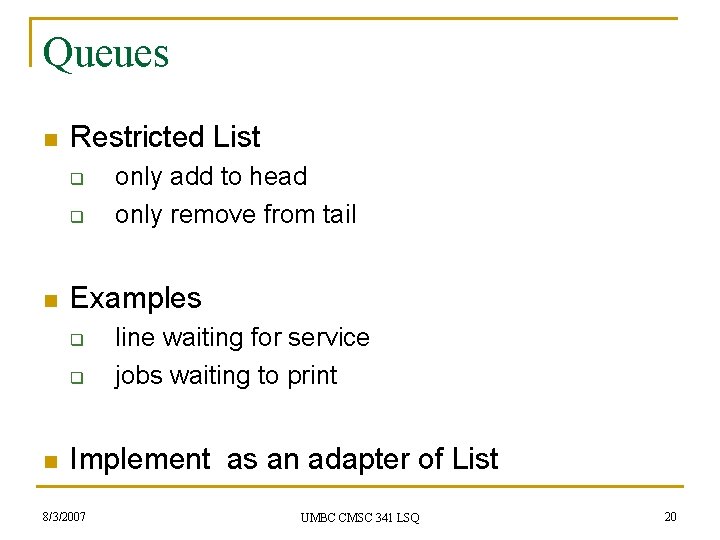
Queues n Restricted List q q n Examples q q n only add to head only remove from tail line waiting for service jobs waiting to print Implement as an adapter of List 8/3/2007 UMBC CMSC 341 LSQ 20
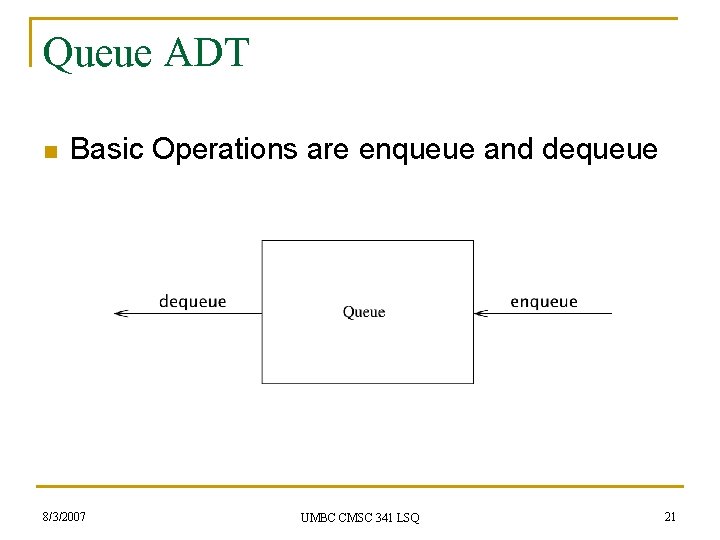
Queue ADT n Basic Operations are enqueue and dequeue 8/3/2007 UMBC CMSC 341 LSQ 21
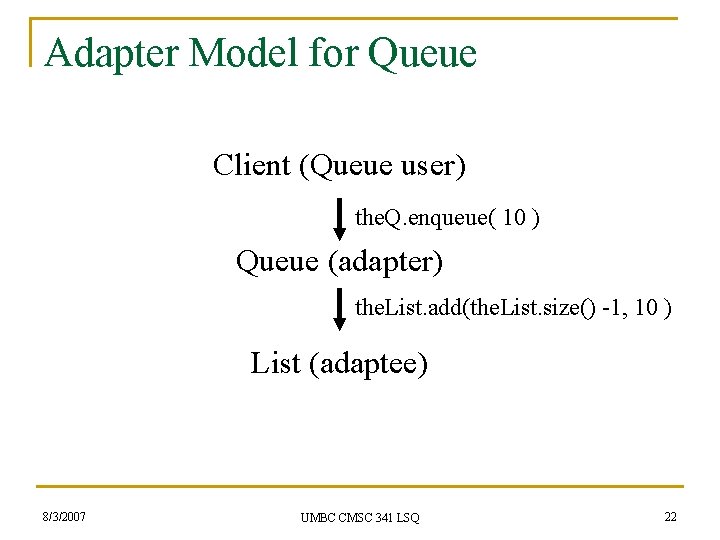
Adapter Model for Queue Client (Queue user) the. Q. enqueue( 10 ) Queue (adapter) the. List. add(the. List. size() -1, 10 ) List (adaptee) 8/3/2007 UMBC CMSC 341 LSQ 22
Python stack and queue
Java stacks and queues
Java stack exercises
Java stacks and queues
Cmsc 341
Umbc cmsc 341
Cmsc 341
Umbc cmsc 341
Cmsc 341
Cmsc 341
Cmsc 341
Cmsc 341
Cmsc 341 umbc
Cmsc 341
Cmsc 341
Singly linked list vs doubly linked list
Singly vs doubly linked list
Perbedaan single linked list dan double linked list
What is this
Priority queues: quiz
Representation of queues
Message queues in unix
Priority queue adt