CHAPTER 1 INTRODUCTION C Programming CS 241 Course
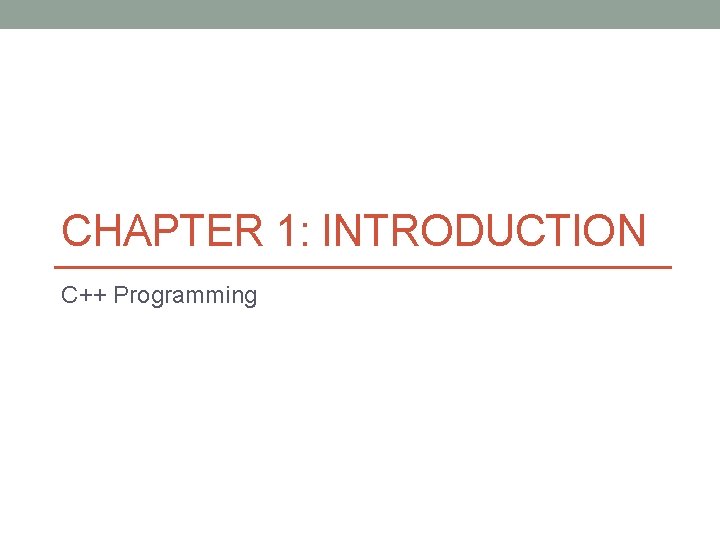
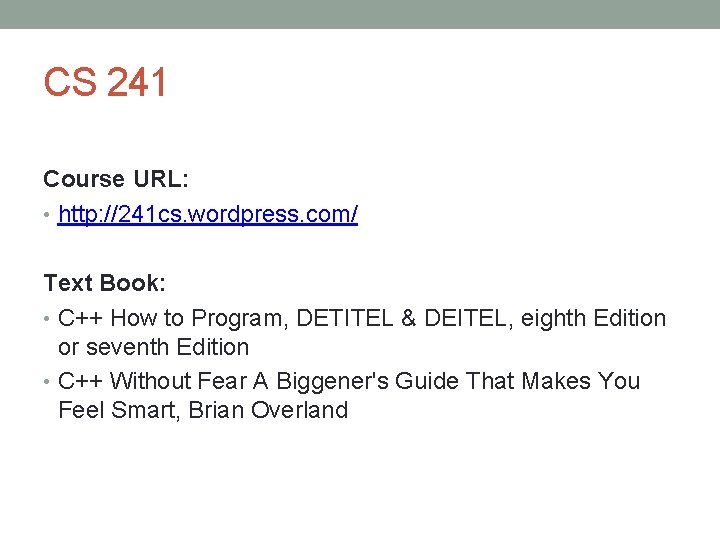
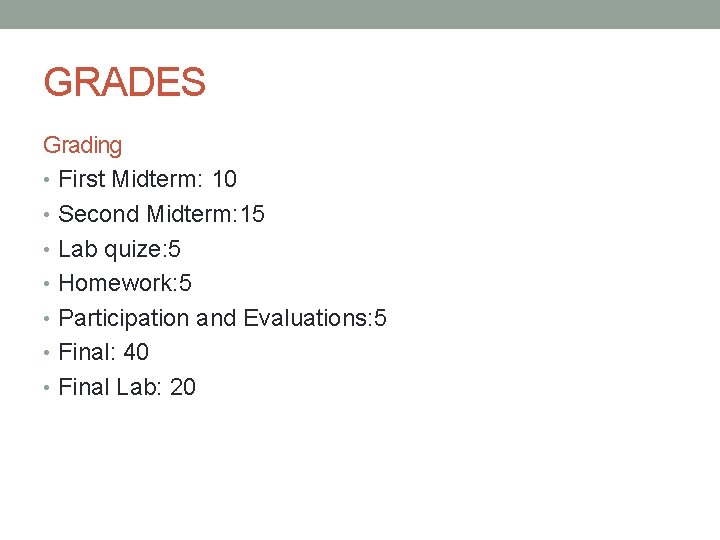
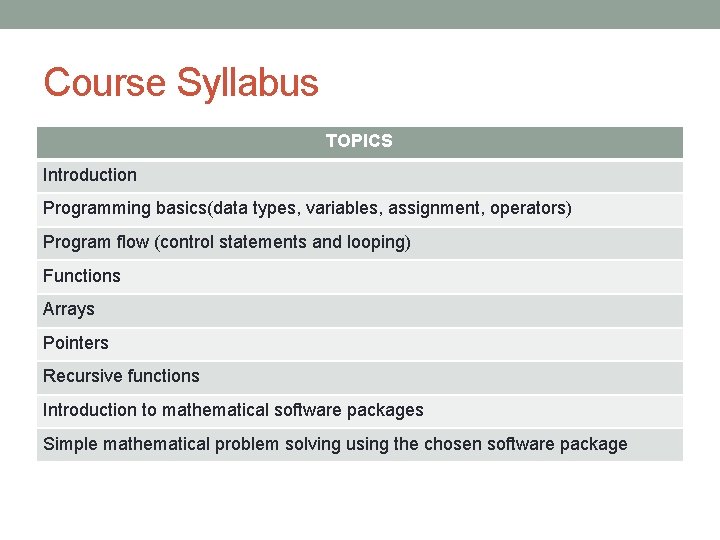
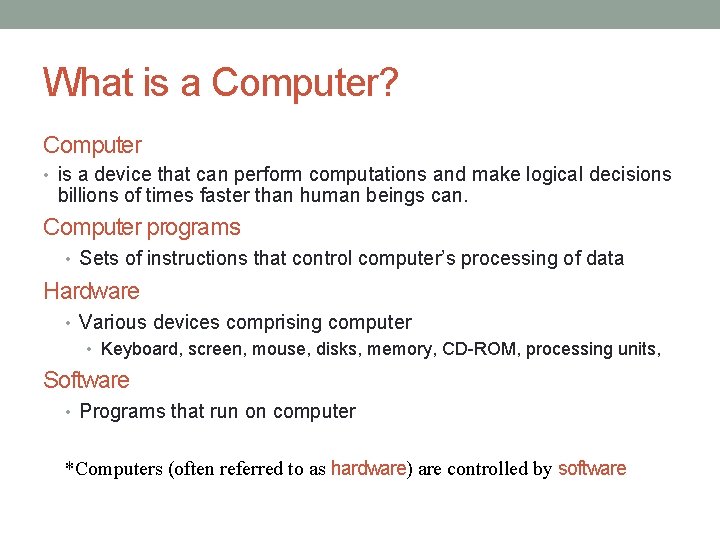
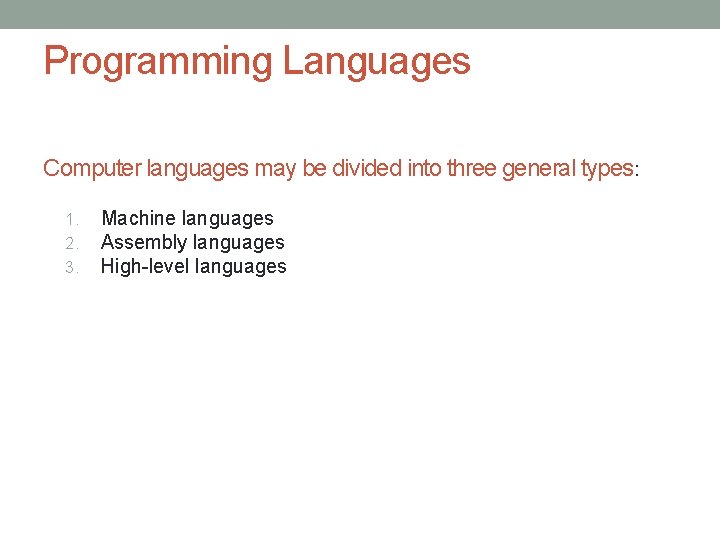
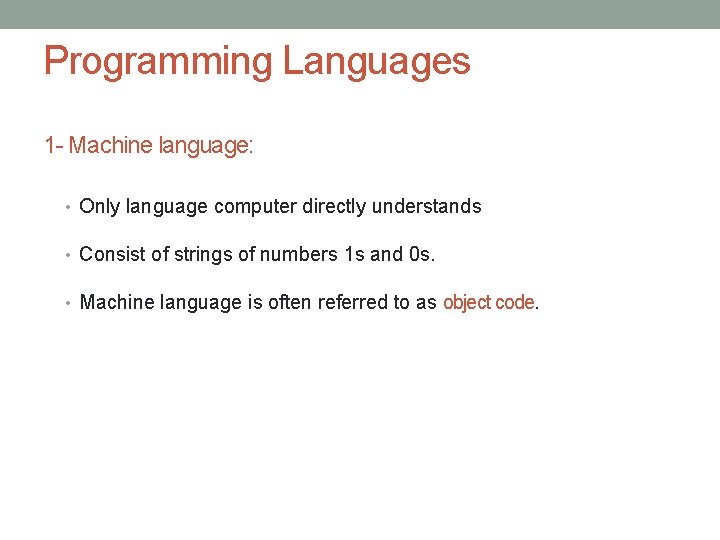
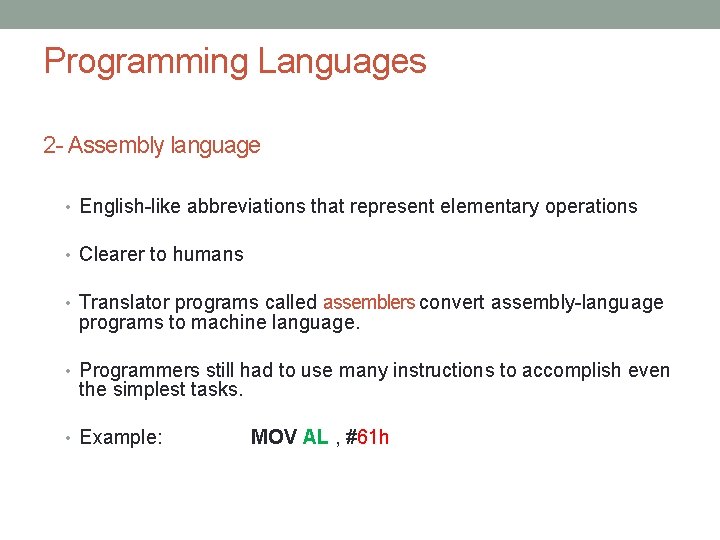
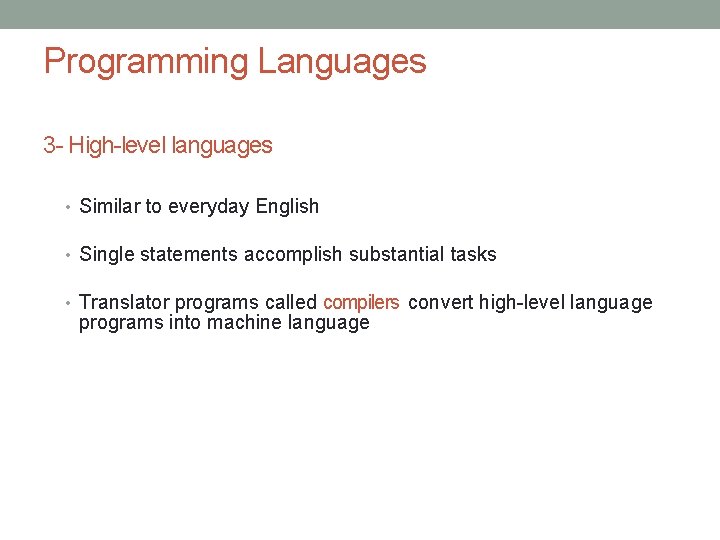
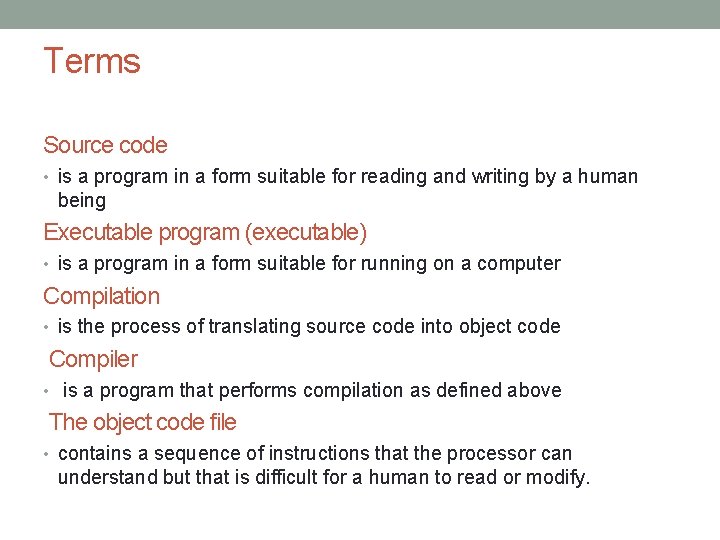
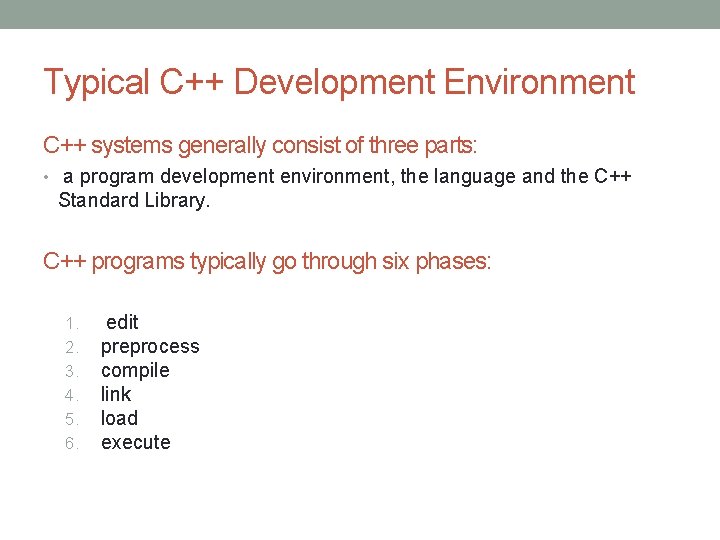
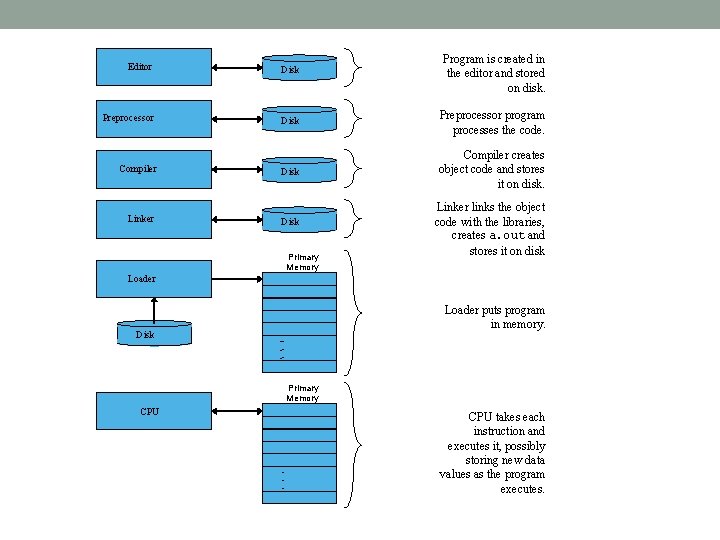
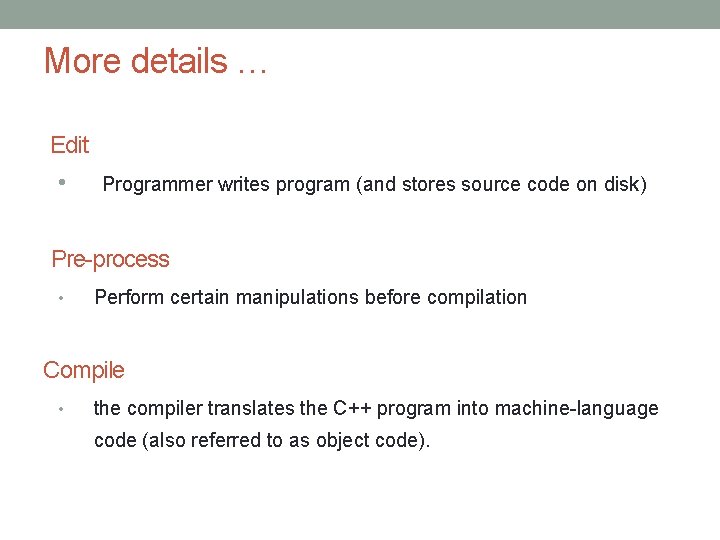
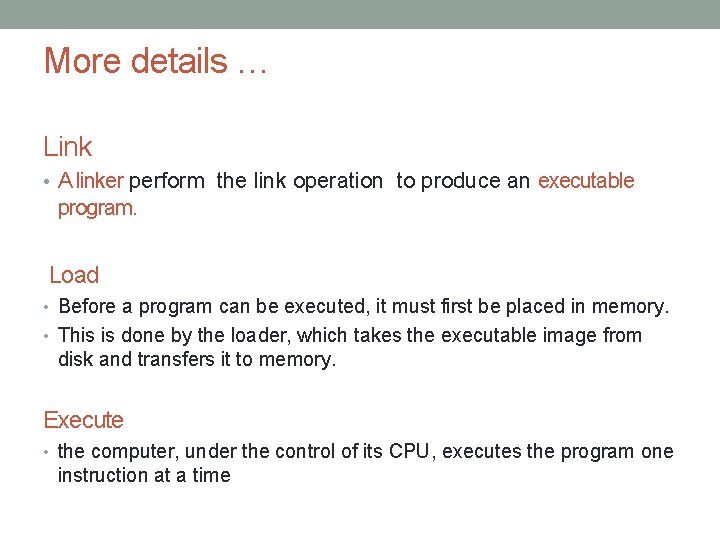
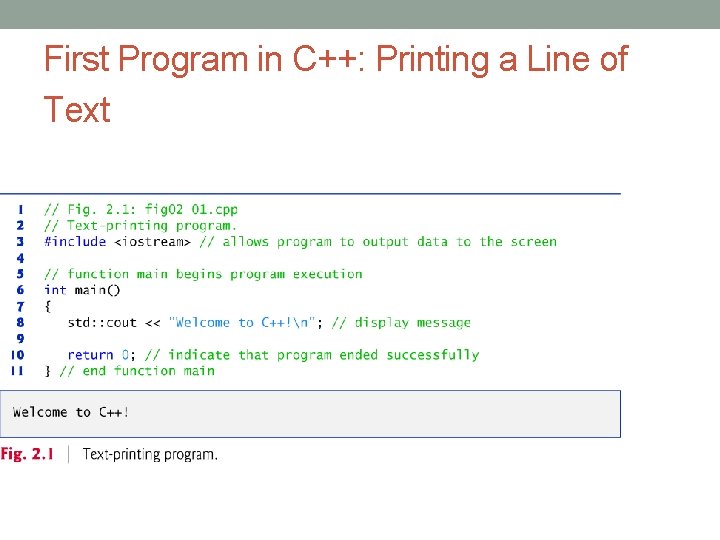
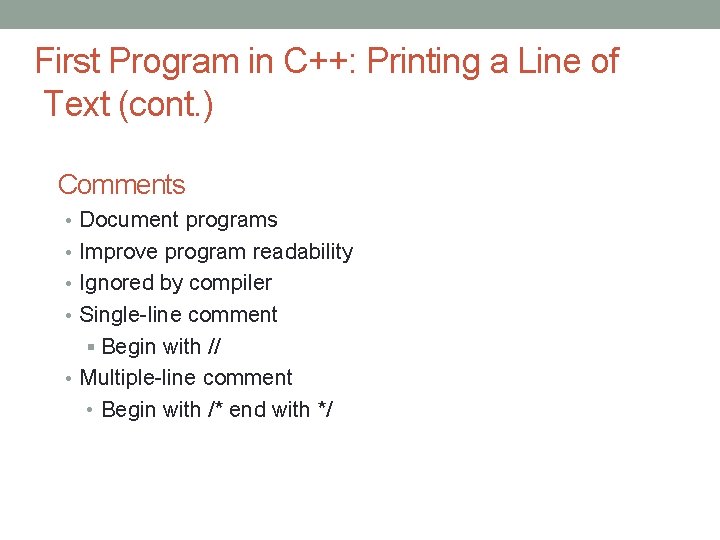
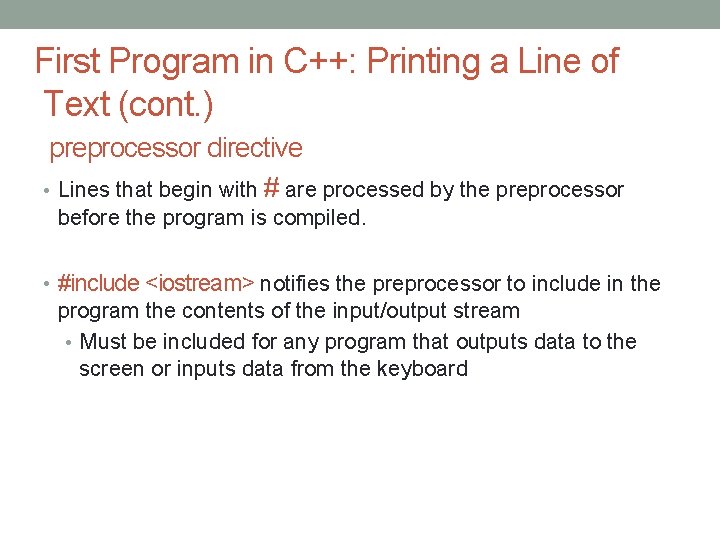
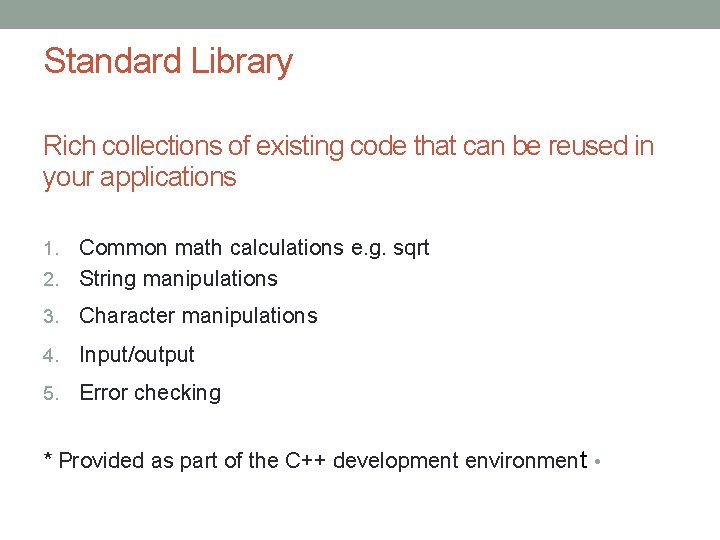
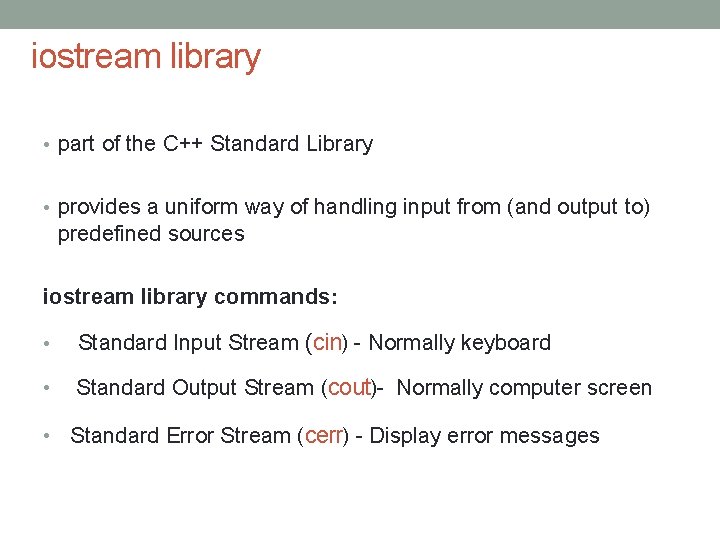
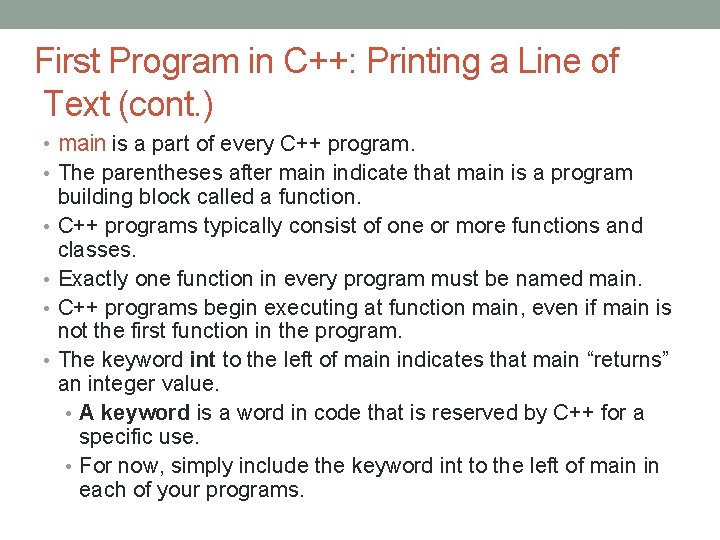
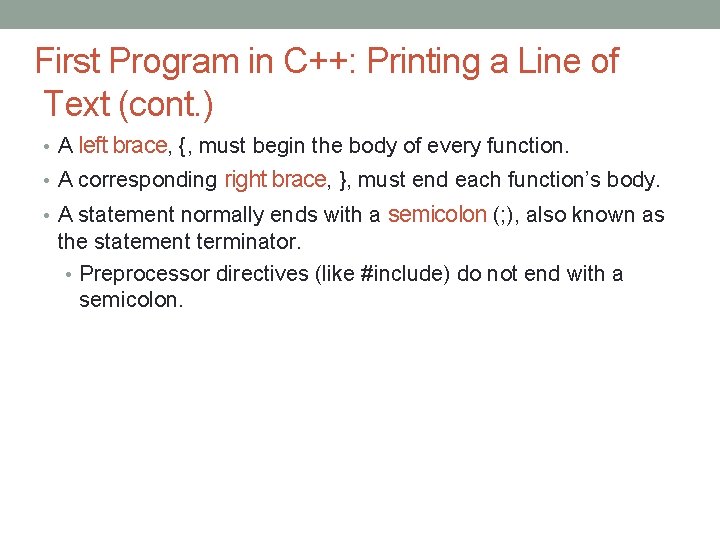
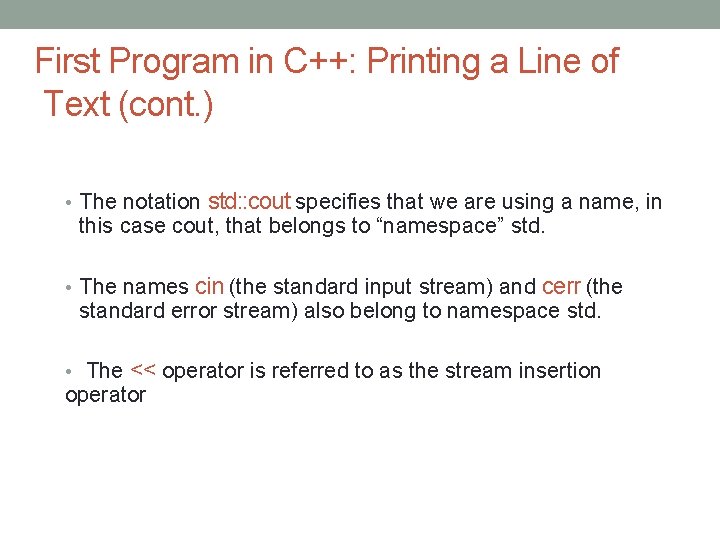
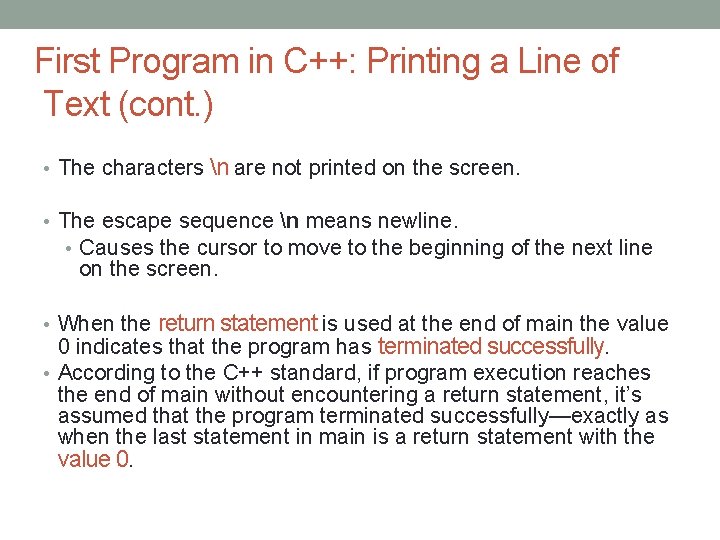
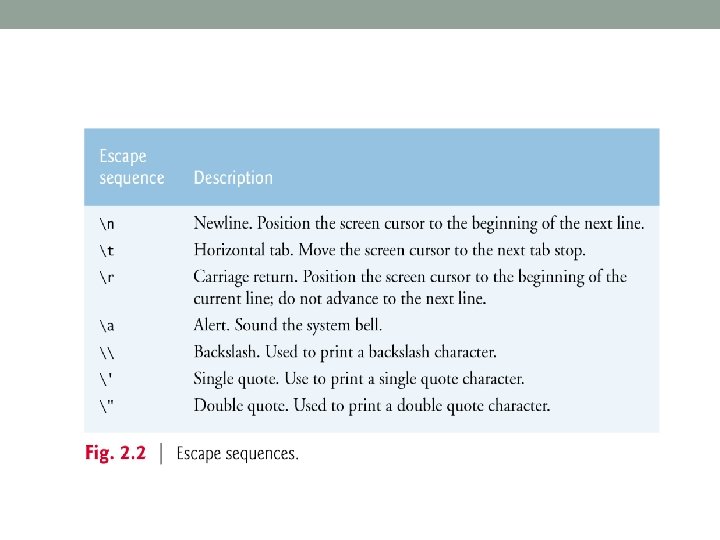
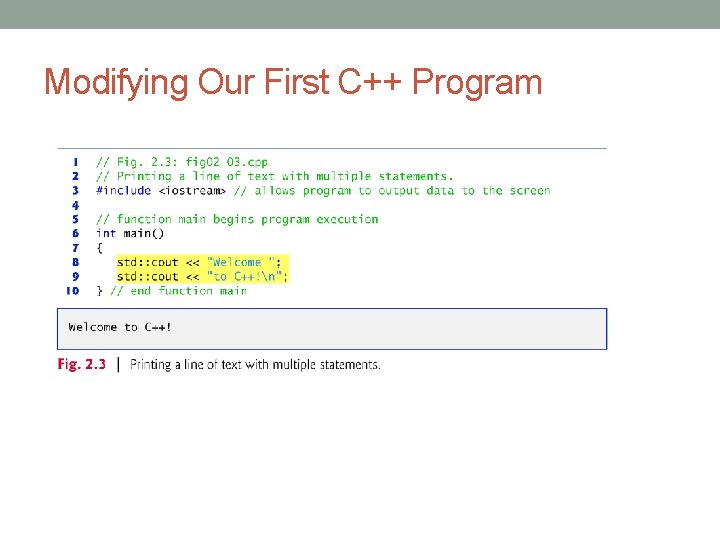
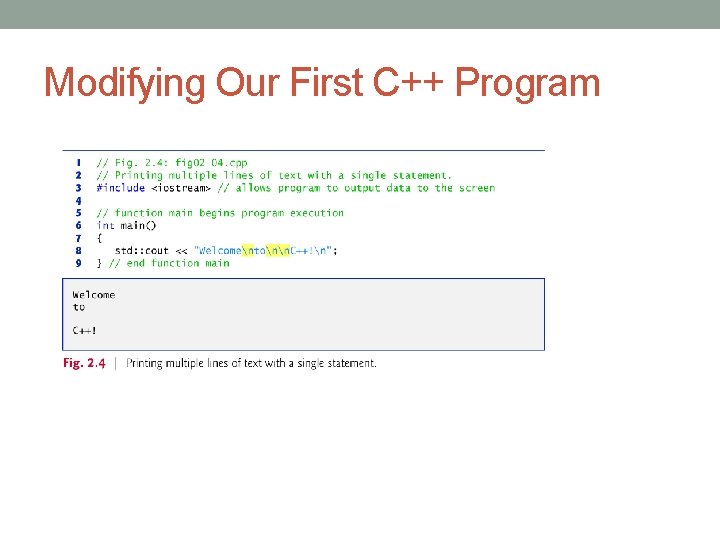
- Slides: 26
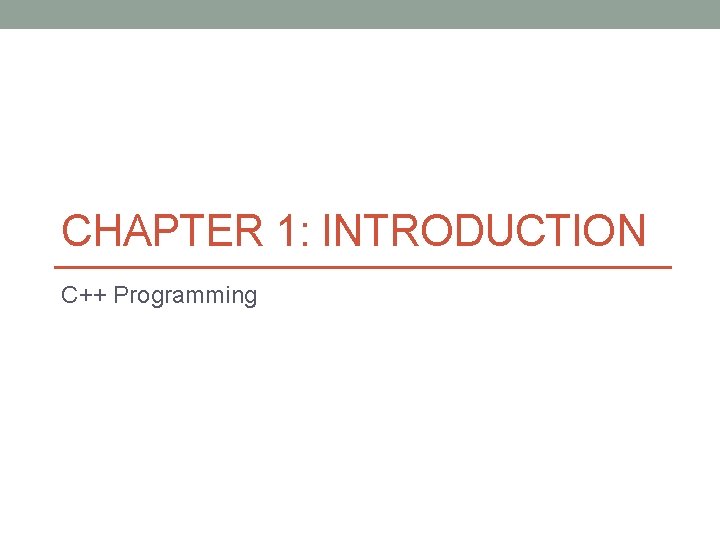
CHAPTER 1: INTRODUCTION C++ Programming
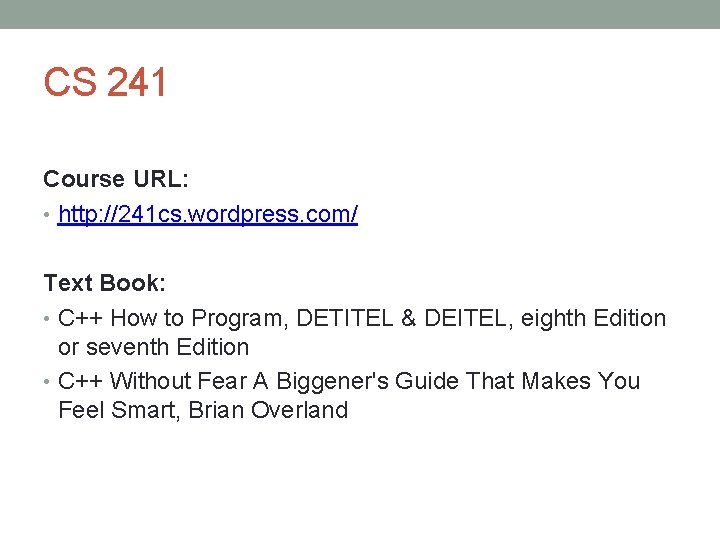
CS 241 Course URL: • http: //241 cs. wordpress. com/ Text Book: • C++ How to Program, DETITEL & DEITEL, eighth Edition or seventh Edition • C++ Without Fear A Biggener's Guide That Makes You Feel Smart, Brian Overland
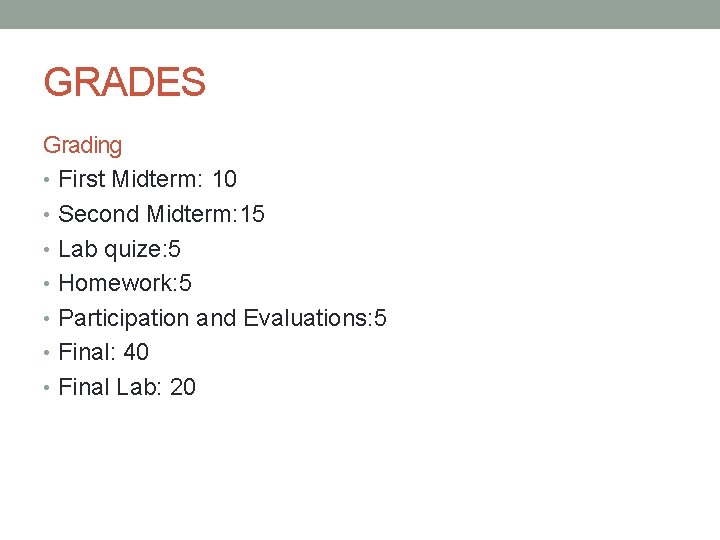
GRADES Grading • First Midterm: 10 • Second Midterm: 15 • Lab quize: 5 • Homework: 5 • Participation and Evaluations: 5 • Final: 40 • Final Lab: 20
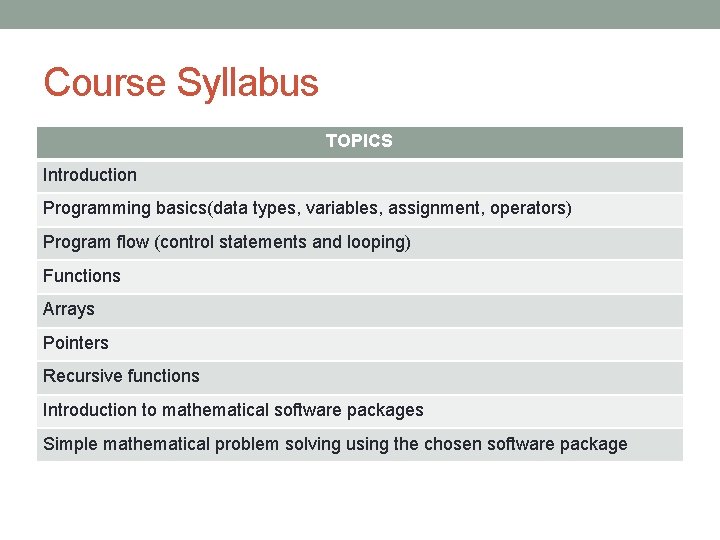
Course Syllabus TOPICS Introduction Programming basics(data types, variables, assignment, operators) Program flow (control statements and looping) Functions Arrays Pointers Recursive functions Introduction to mathematical software packages Simple mathematical problem solving using the chosen software package
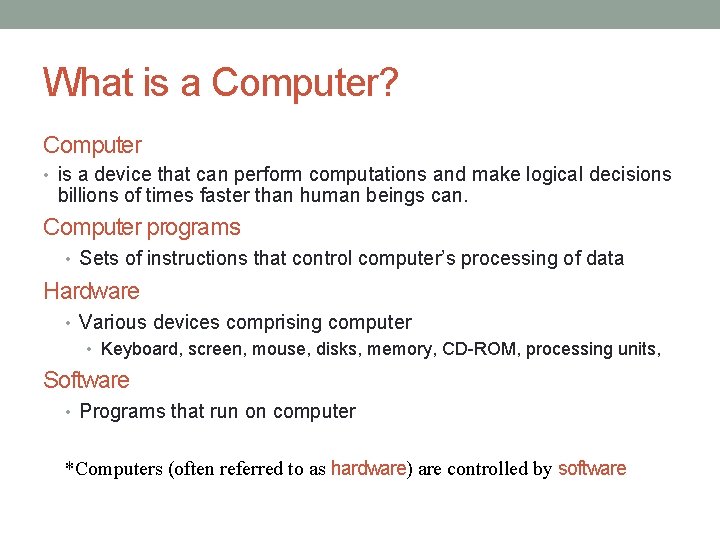
What is a Computer? Computer • is a device that can perform computations and make logical decisions billions of times faster than human beings can. Computer programs • Sets of instructions that control computer’s processing of data Hardware • Various devices comprising computer • Keyboard, screen, mouse, disks, memory, CD-ROM, processing units, Software • Programs that run on computer *Computers (often referred to as hardware) are controlled by software
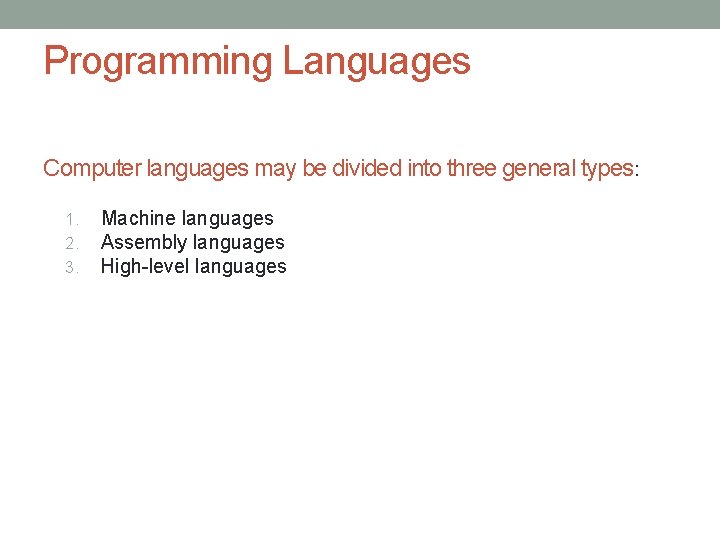
Programming Languages Computer languages may be divided into three general types: 1. 2. 3. Machine languages Assembly languages High-level languages
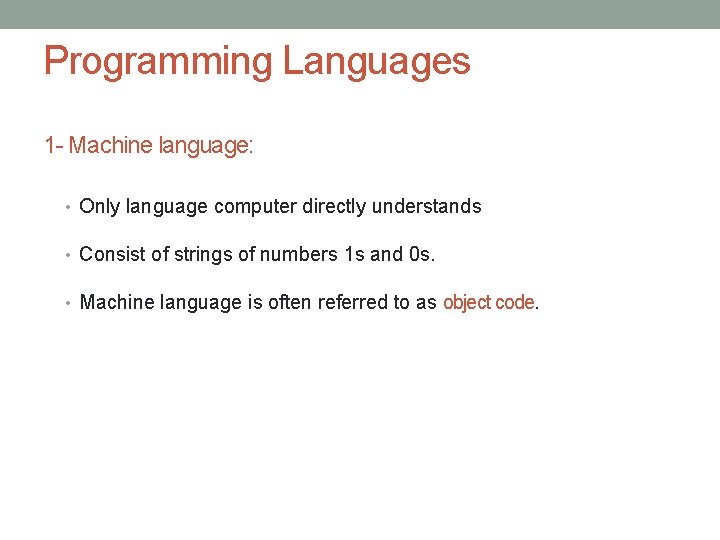
Programming Languages 1 - Machine language: • Only language computer directly understands • Consist of strings of numbers 1 s and 0 s. • Machine language is often referred to as object code.
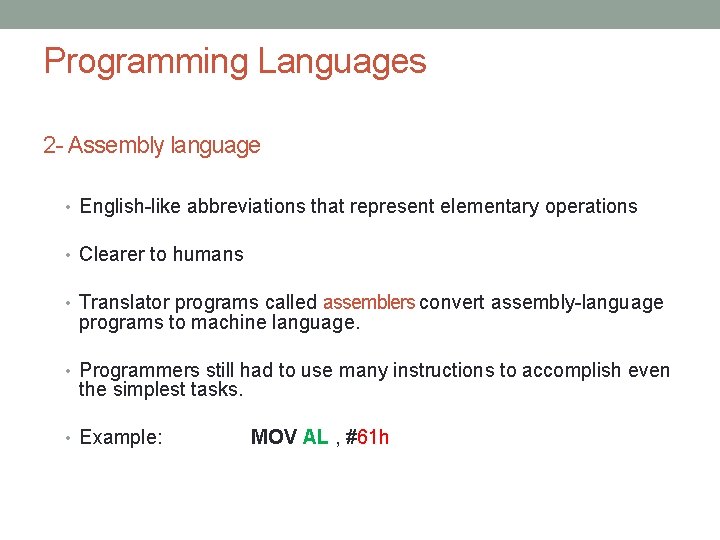
Programming Languages 2 - Assembly language • English-like abbreviations that represent elementary operations • Clearer to humans • Translator programs called assemblers convert assembly-language programs to machine language. • Programmers still had to use many instructions to accomplish even the simplest tasks. • Example: MOV AL , #61 h
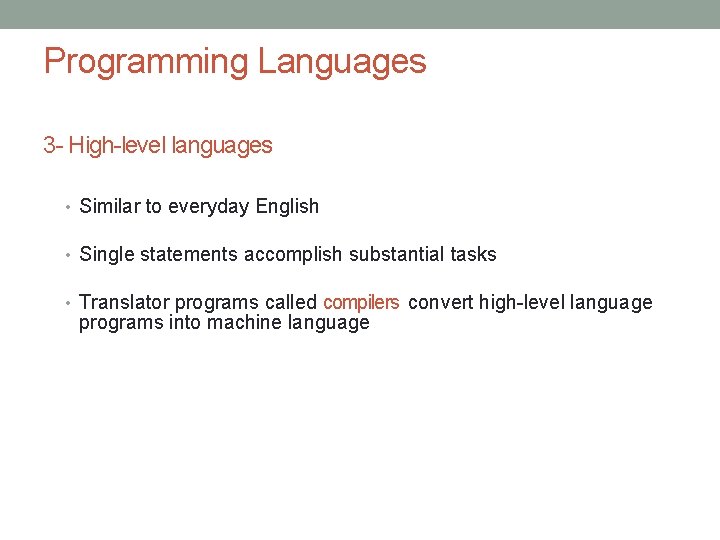
Programming Languages 3 - High-level languages • Similar to everyday English • Single statements accomplish substantial tasks • Translator programs called compilers convert high-level language programs into machine language
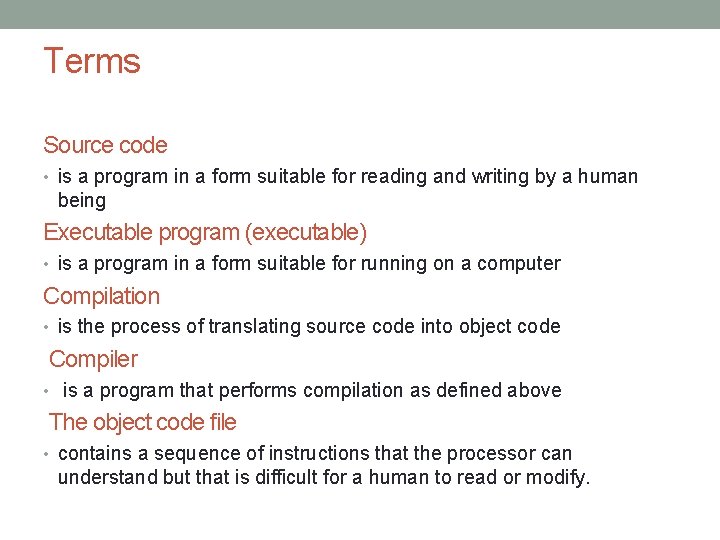
Terms Source code • is a program in a form suitable for reading and writing by a human being Executable program (executable) • is a program in a form suitable for running on a computer Compilation • is the process of translating source code into object code Compiler • is a program that performs compilation as defined above The object code file • contains a sequence of instructions that the processor can understand but that is difficult for a human to read or modify.
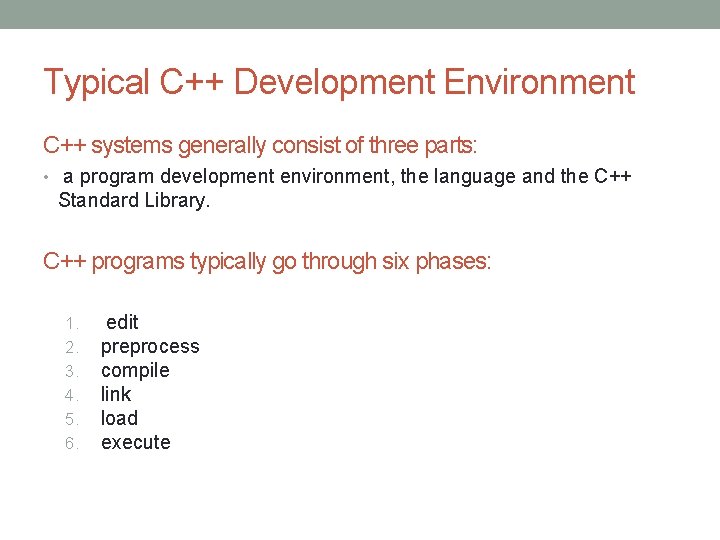
Typical C++ Development Environment C++ systems generally consist of three parts: • a program development environment, the language and the C++ Standard Library. C++ programs typically go through six phases: 1. 2. 3. 4. 5. 6. edit preprocess compile link load execute
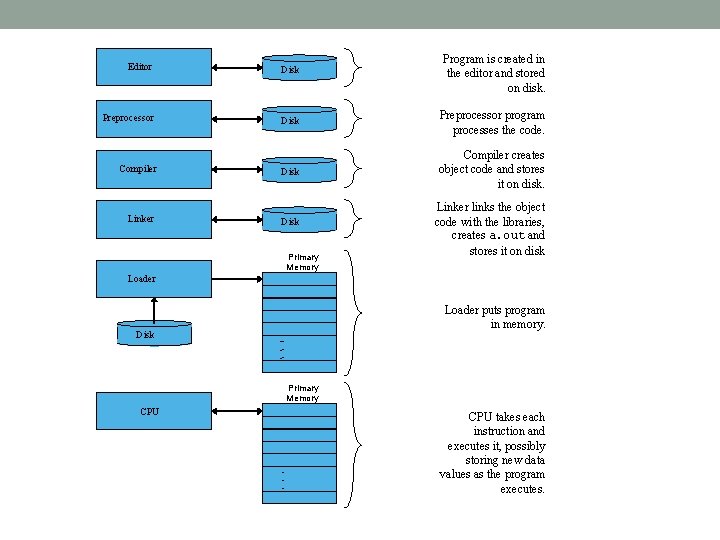
Editor Disk Program is created in the editor and stored on disk. Preprocessor Disk Preprocessor program processes the code. Disk Compiler creates object code and stores it on disk. Compiler Linker Disk Primary Memory Linker links the object code with the libraries, creates a. out and stores it on disk Loader Disk Loader puts program in memory. . . . Primary Memory CPU . . . CPU takes each instruction and executes it, possibly storing new data values as the program executes.
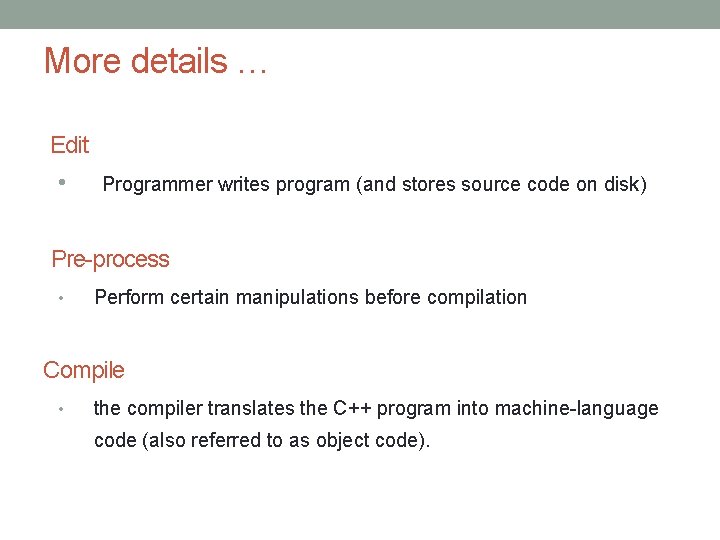
More details … Edit • Programmer writes program (and stores source code on disk) Pre-process • Perform certain manipulations before compilation Compile • the compiler translates the C++ program into machine-language code (also referred to as object code).
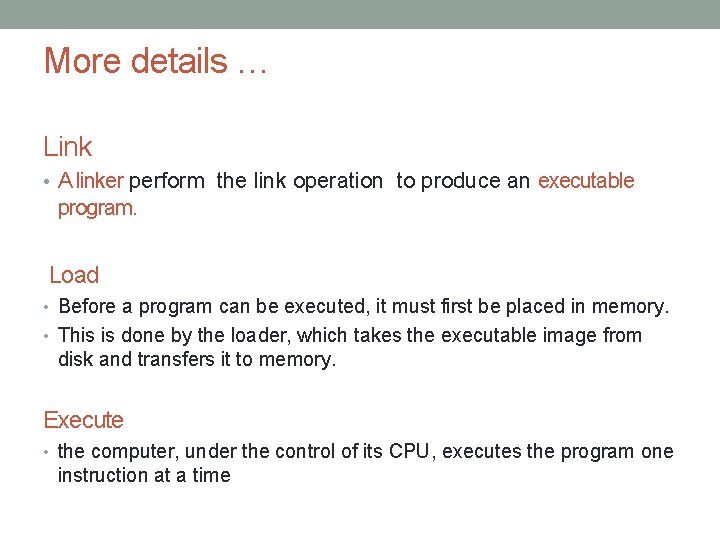
More details … Link • A linker perform the link operation to produce an executable program. Load • Before a program can be executed, it must first be placed in memory. • This is done by the loader, which takes the executable image from disk and transfers it to memory. Execute • the computer, under the control of its CPU, executes the program one instruction at a time
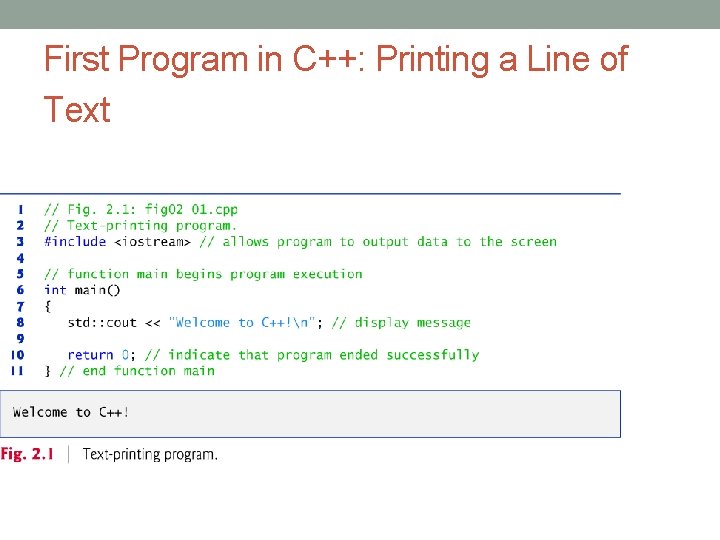
First Program in C++: Printing a Line of Text
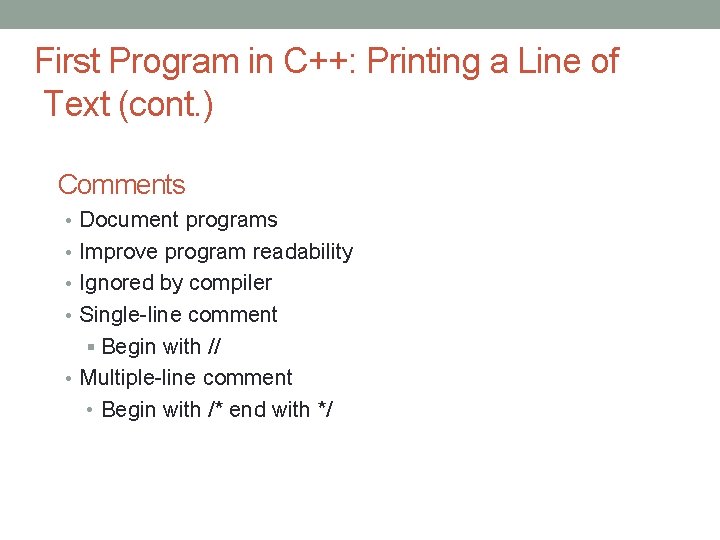
First Program in C++: Printing a Line of Text (cont. ) Comments • Document programs • Improve program readability • Ignored by compiler • Single-line comment § Begin with // • Multiple-line comment • Begin with /* end with */
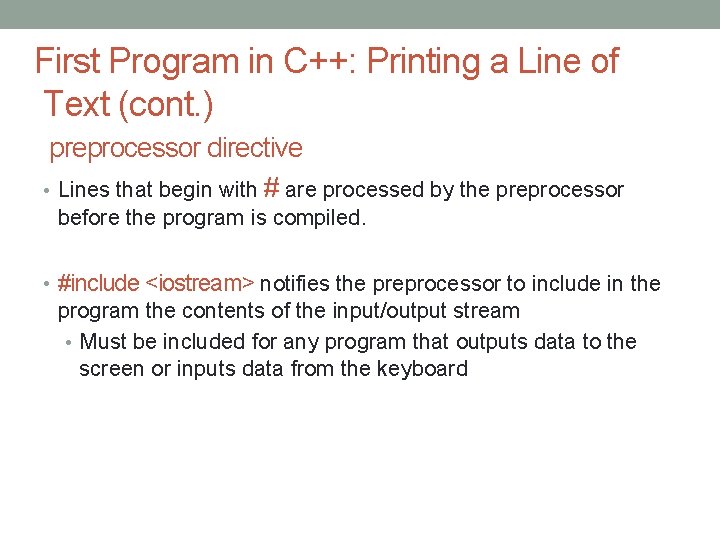
First Program in C++: Printing a Line of Text (cont. ) preprocessor directive • Lines that begin with # are processed by the preprocessor before the program is compiled. • #include <iostream> notifies the preprocessor to include in the program the contents of the input/output stream • Must be included for any program that outputs data to the screen or inputs data from the keyboard
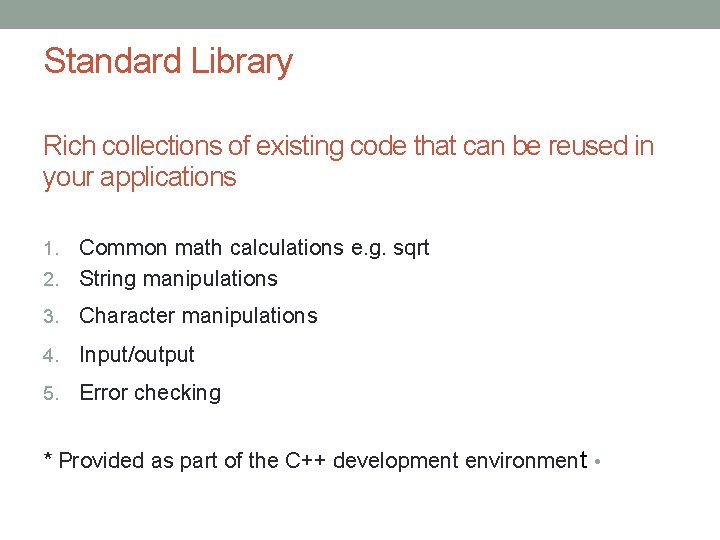
Standard Library Rich collections of existing code that can be reused in your applications Common math calculations e. g. sqrt 2. String manipulations 1. 3. Character manipulations 4. Input/output 5. Error checking * Provided as part of the C++ development environment •
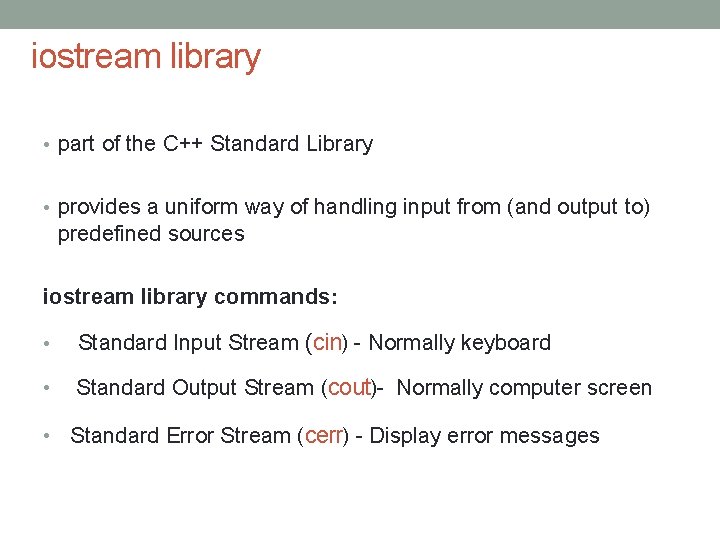
iostream library • part of the C++ Standard Library • provides a uniform way of handling input from (and output to) predefined sources iostream library commands: • Standard Input Stream (cin) - Normally keyboard • Standard Output Stream (cout)- Normally computer screen • Standard Error Stream (cerr) - Display error messages
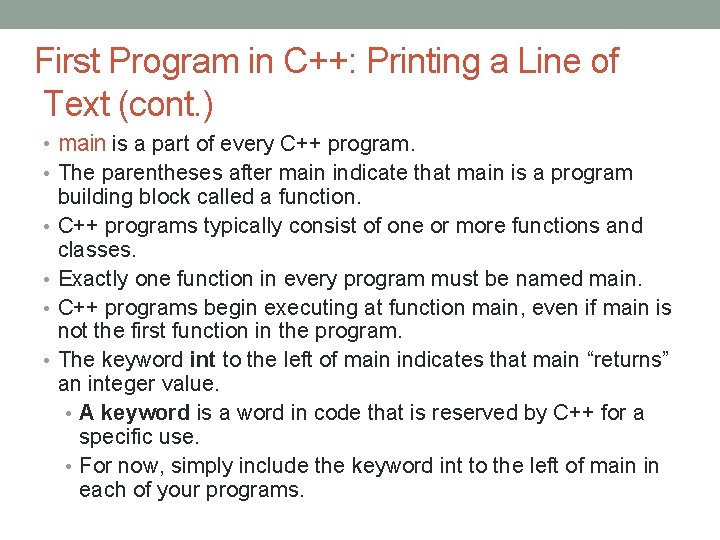
First Program in C++: Printing a Line of Text (cont. ) • main is a part of every C++ program. • The parentheses after main indicate that main is a program • • building block called a function. C++ programs typically consist of one or more functions and classes. Exactly one function in every program must be named main. C++ programs begin executing at function main, even if main is not the first function in the program. The keyword int to the left of main indicates that main “returns” an integer value. • A keyword is a word in code that is reserved by C++ for a specific use. • For now, simply include the keyword int to the left of main in each of your programs.
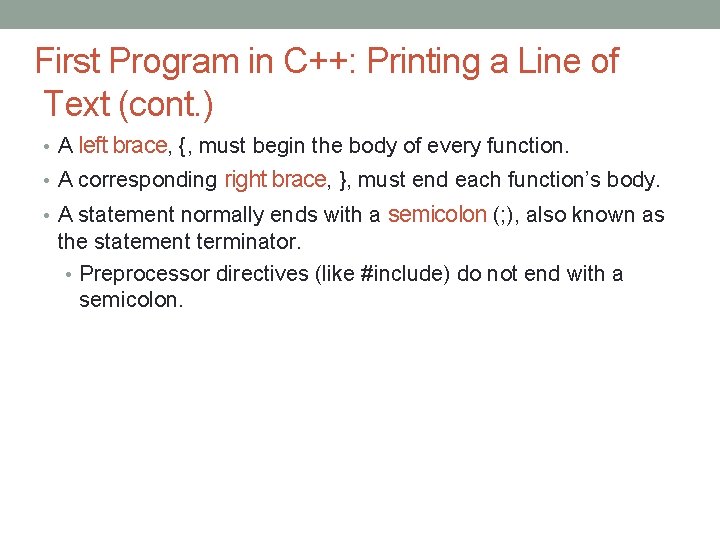
First Program in C++: Printing a Line of Text (cont. ) left brace, {, must begin the body of every function. • A corresponding right brace, }, must end each function’s body. • A statement normally ends with a semicolon (; ), also known as • A the statement terminator. • Preprocessor directives (like #include) do not end with a semicolon.
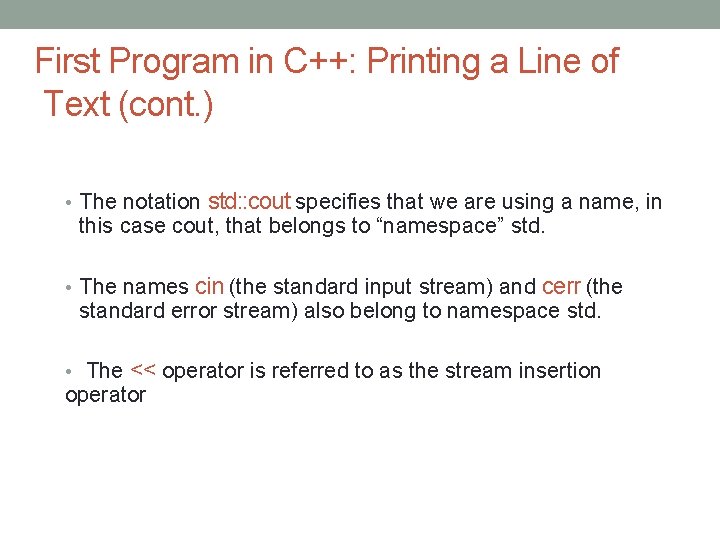
First Program in C++: Printing a Line of Text (cont. ) • The notation std: : cout specifies that we are using a name, in this case cout, that belongs to “namespace” std. • The names cin (the standard input stream) and cerr (the standard error stream) also belong to namespace std. • The << operator is referred to as the stream insertion operator
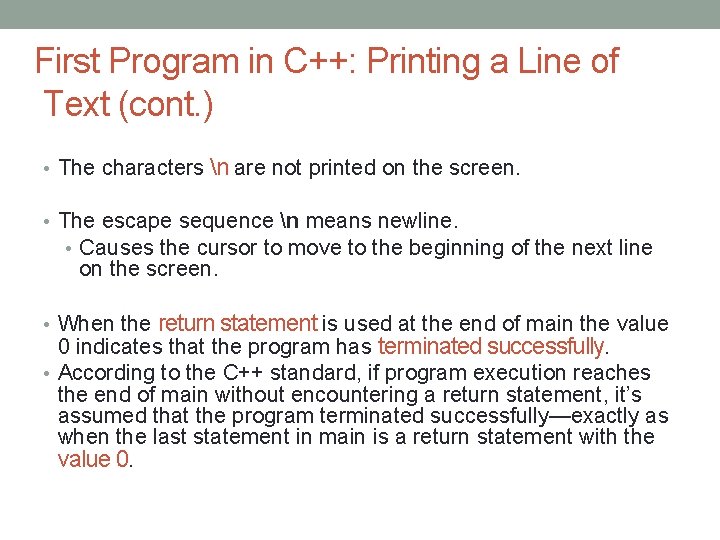
First Program in C++: Printing a Line of Text (cont. ) • The characters n are not printed on the screen. • The escape sequence n means newline. • Causes the cursor to move to the beginning of the next line on the screen. return statement is used at the end of main the value 0 indicates that the program has terminated successfully. • When the • According to the C++ standard, if program execution reaches the end of main without encountering a return statement, it’s assumed that the program terminated successfully—exactly as when the last statement in main is a return statement with the value 0.
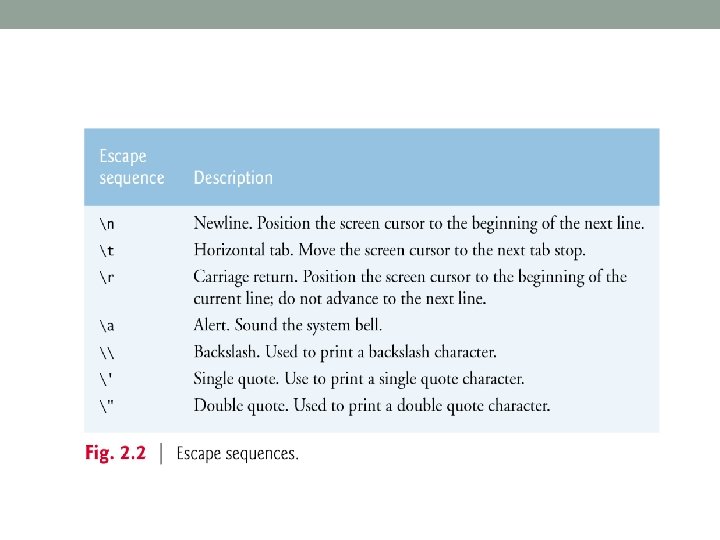
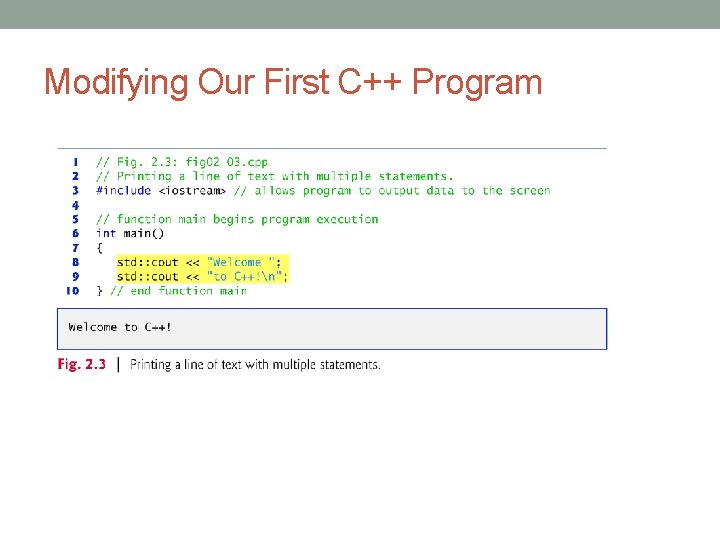
Modifying Our First C++ Program
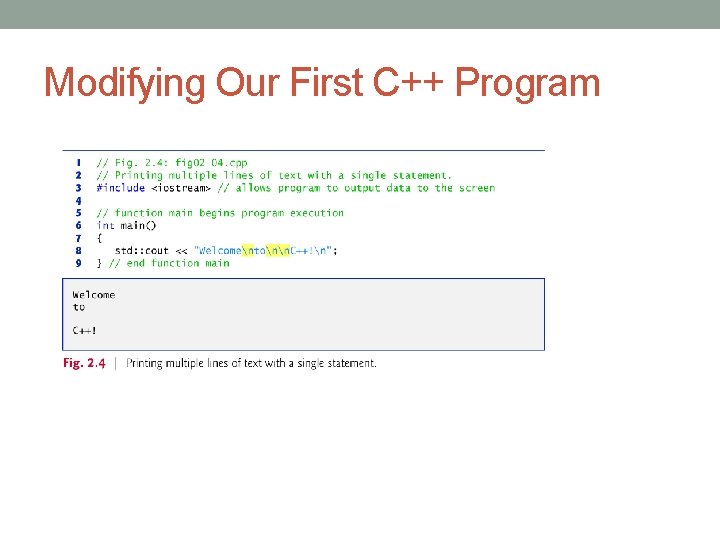
Modifying Our First C++ Program
Computer programming chapter 1
History of python
Chapter 1 introduction to computers and programming
Chapter 1 introduction to computers and programming
Nfpa 241 plan example
Charging by induction
241 bce
Round off to the nearest hundred thousand
Fon 241
Cob 241
Ceng 241
Psyco 241
Cs 241 vector mp
Ceng 241
Chm 241
Shaft drawing example
Mappa concettuale legge 241/90
Vectoral
Legge 241/90 slide
Purdue phys 241
Bio 241
241 in arabic
Disadvantages of cavity wall
Course title and course number
Course interne course externe
Integer programming course
Visual programming course outline