Ch 3 Classes Stepwise Refinement STEP 1 Define
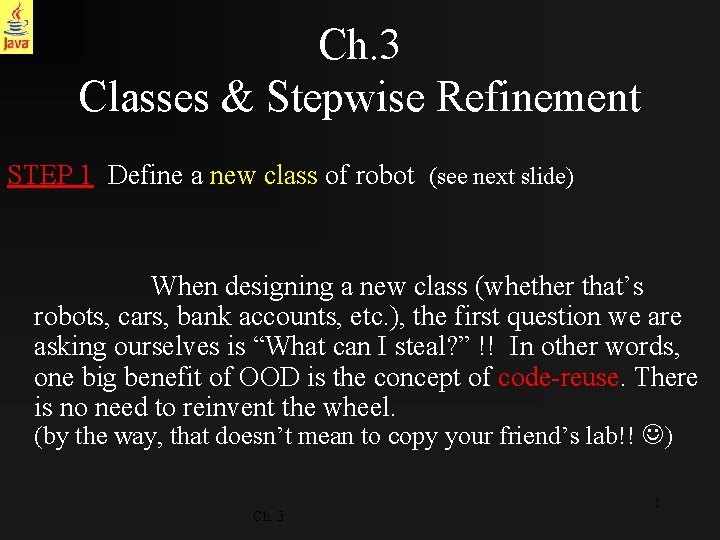
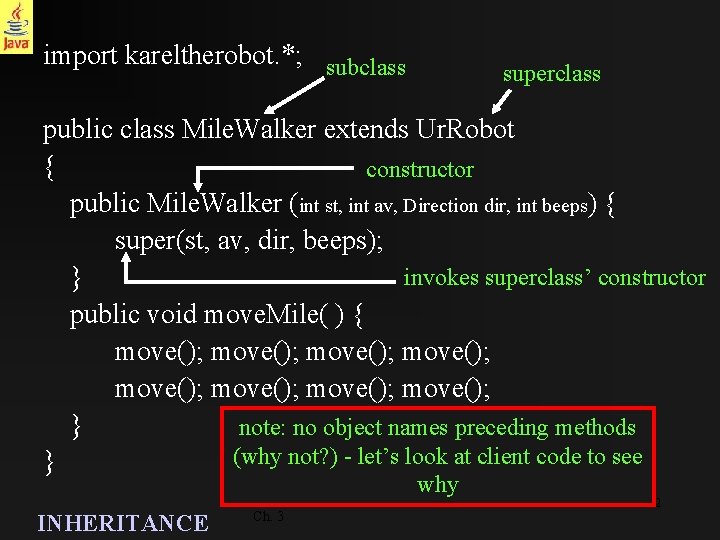
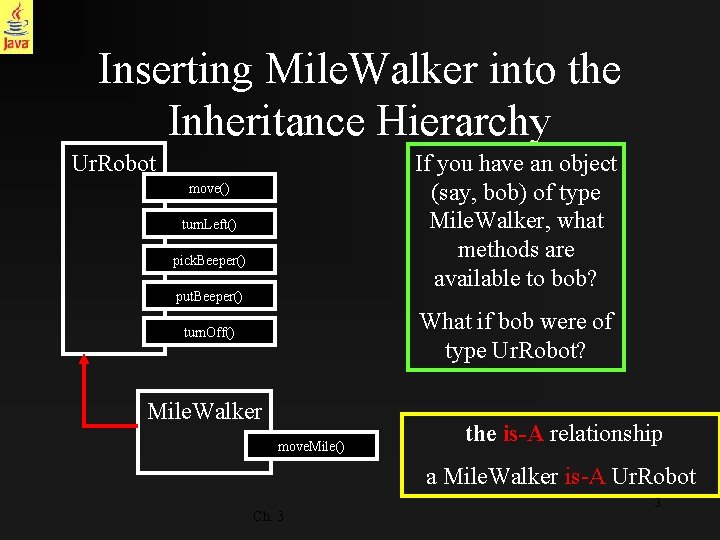
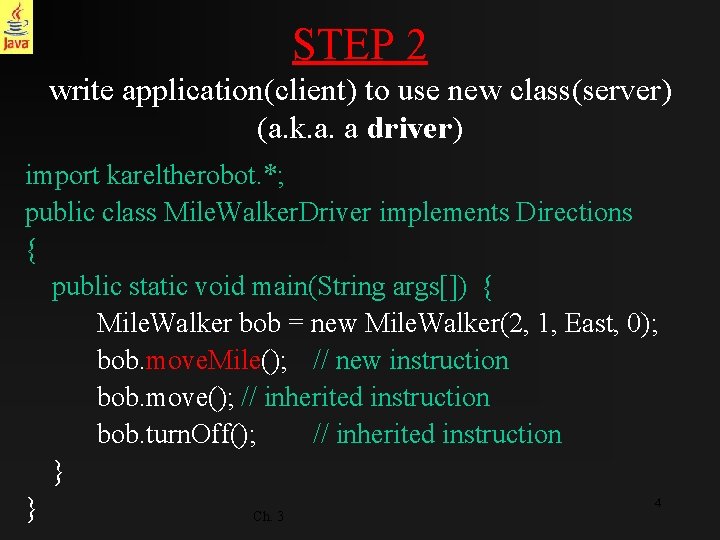
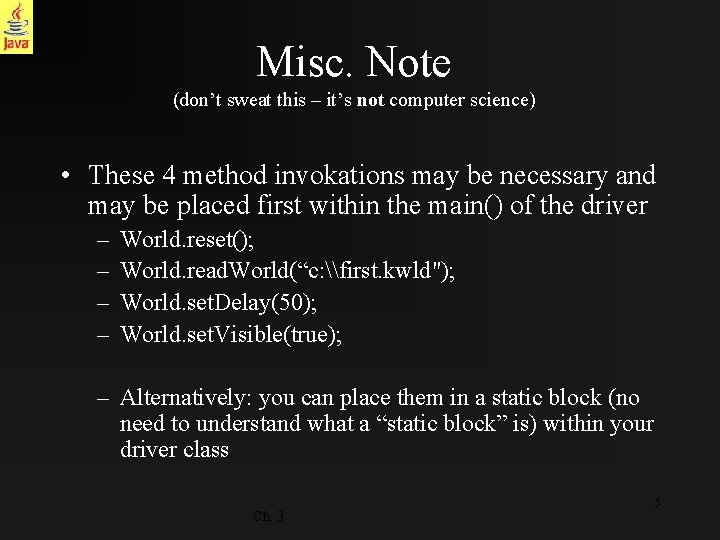
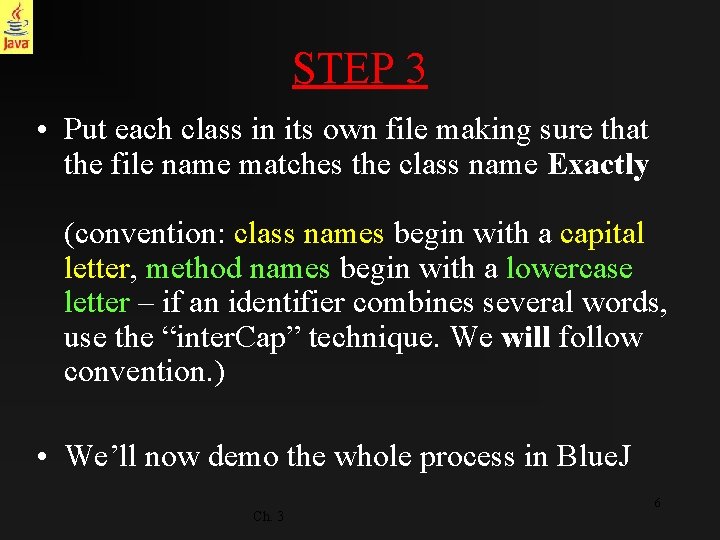
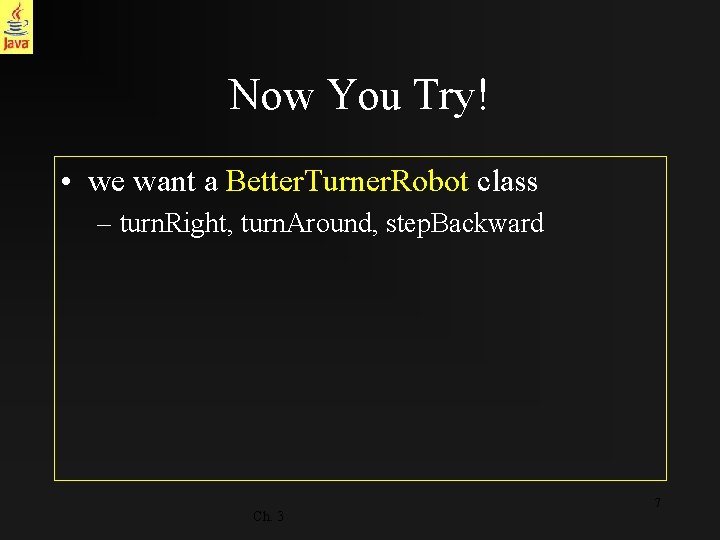
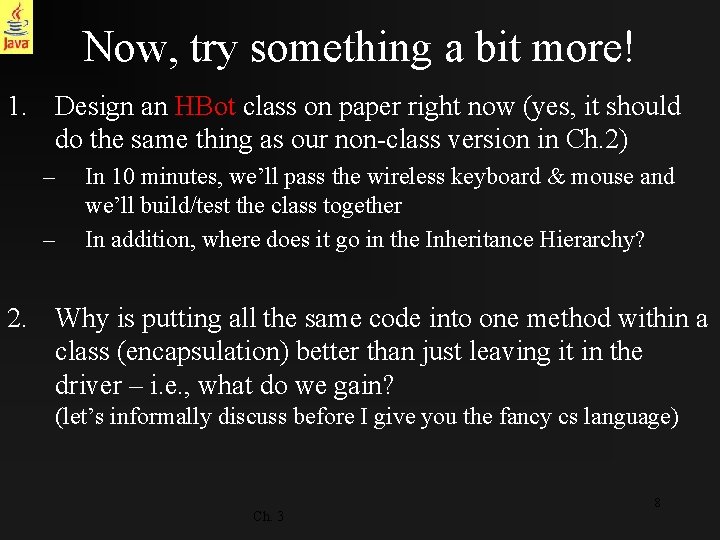
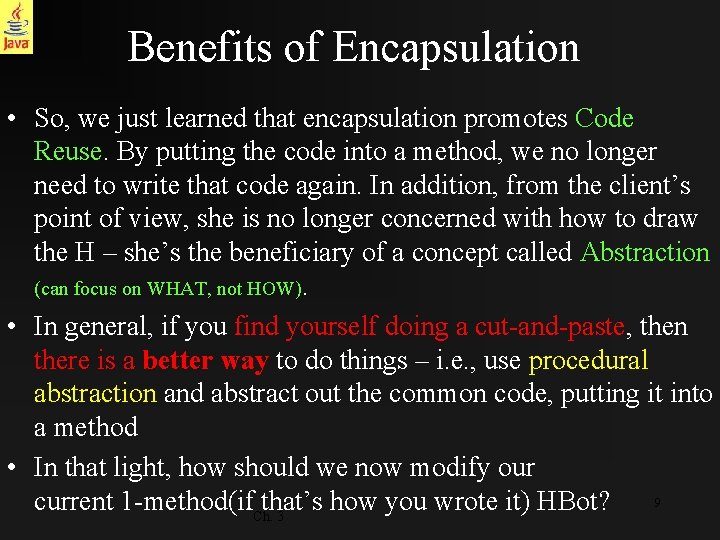
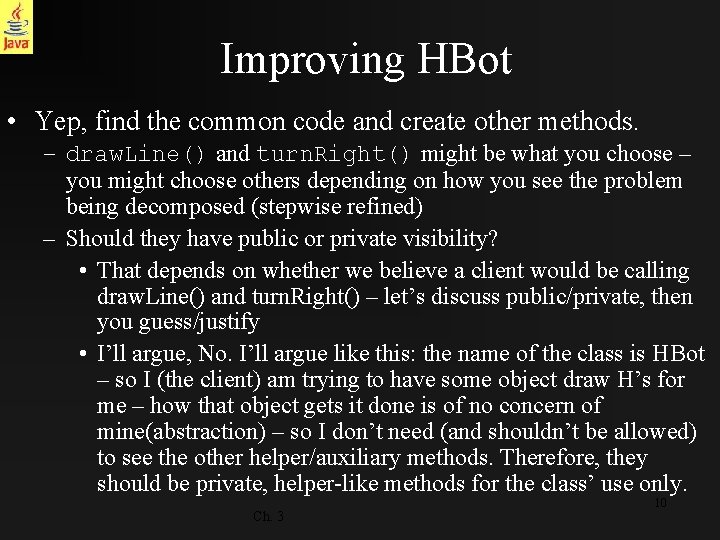
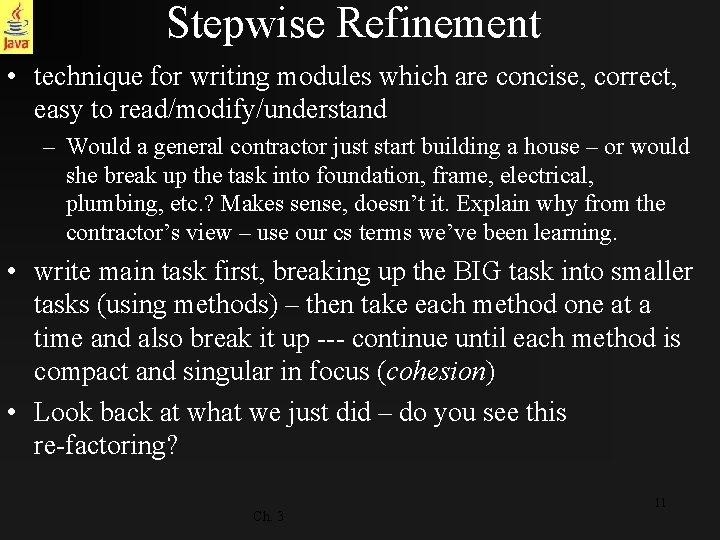
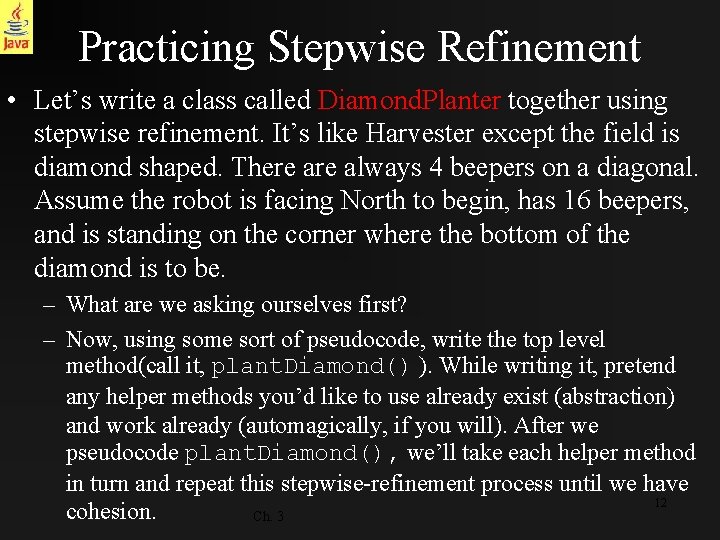
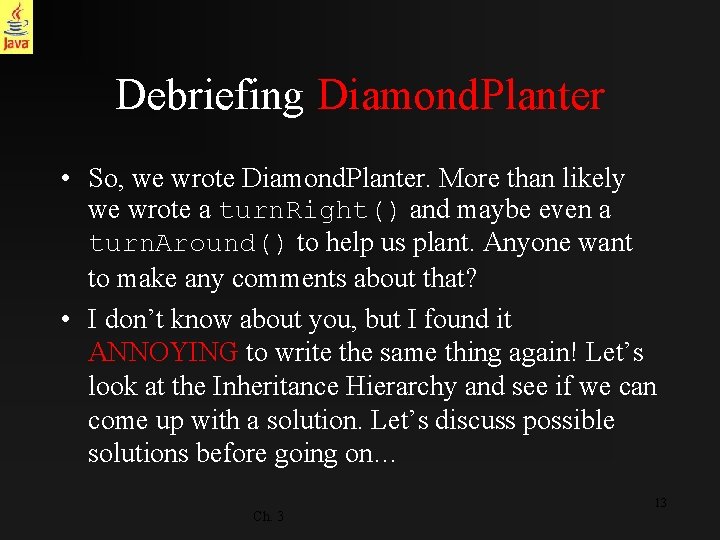
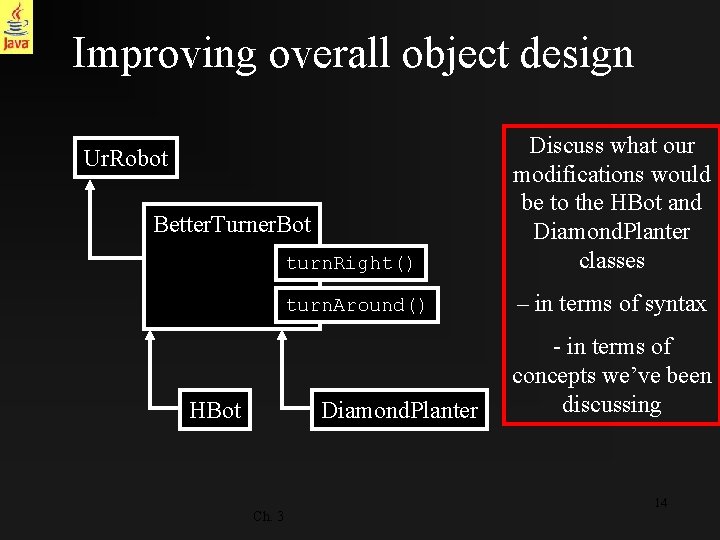
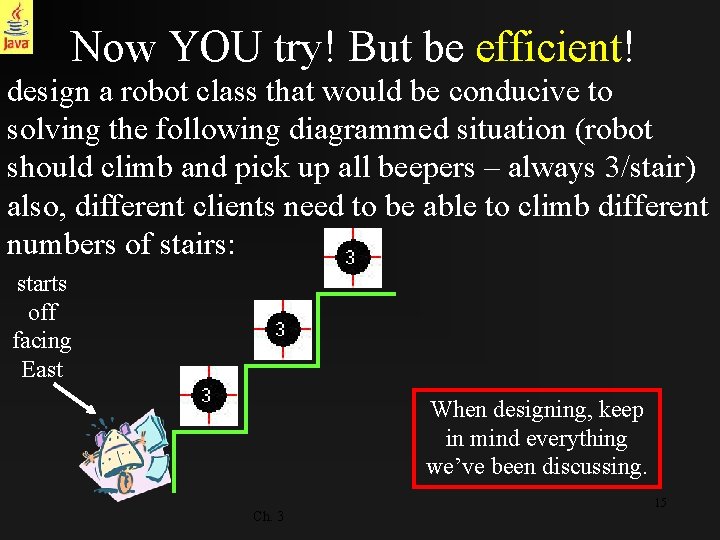
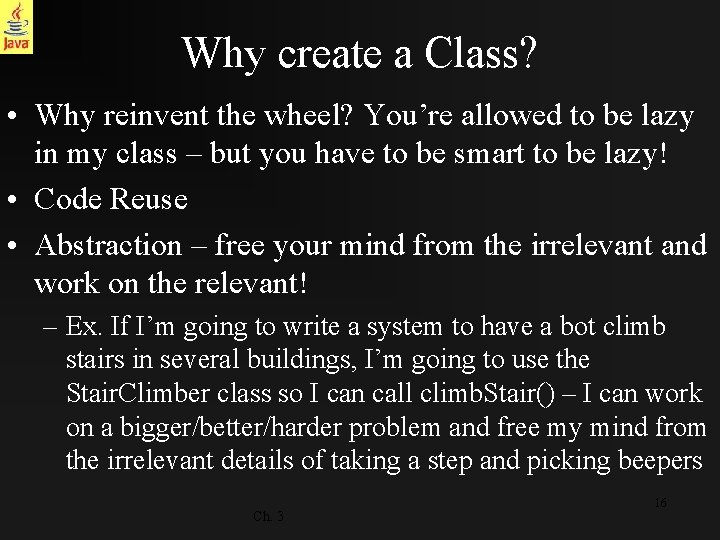
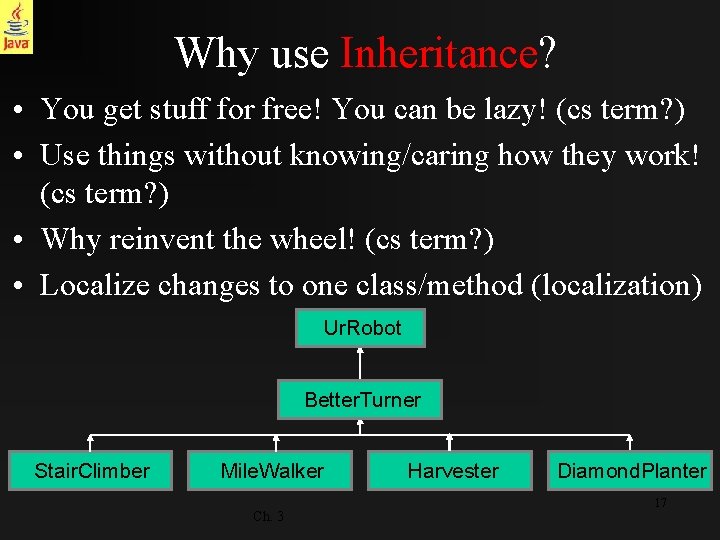
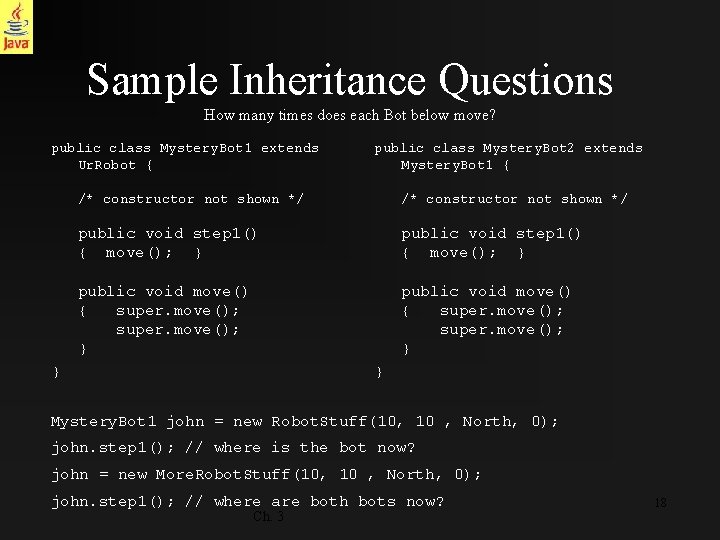
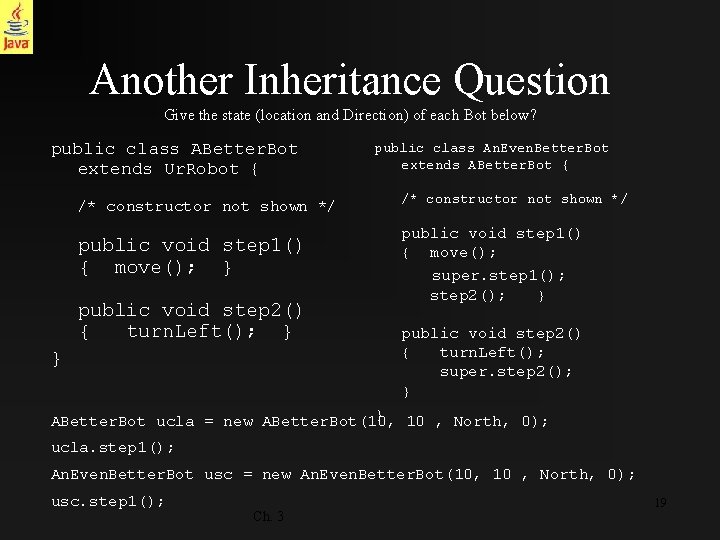
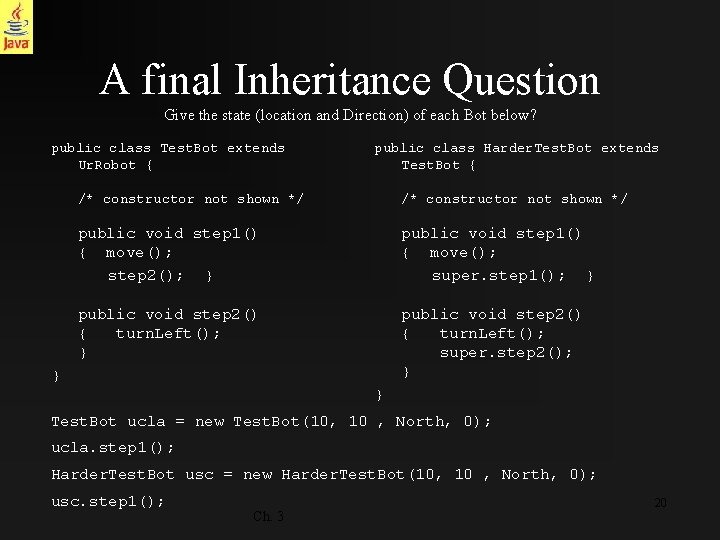
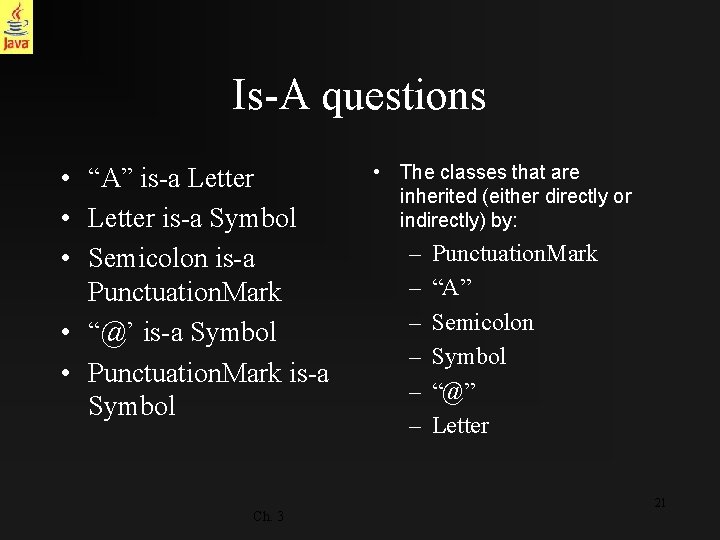
- Slides: 21
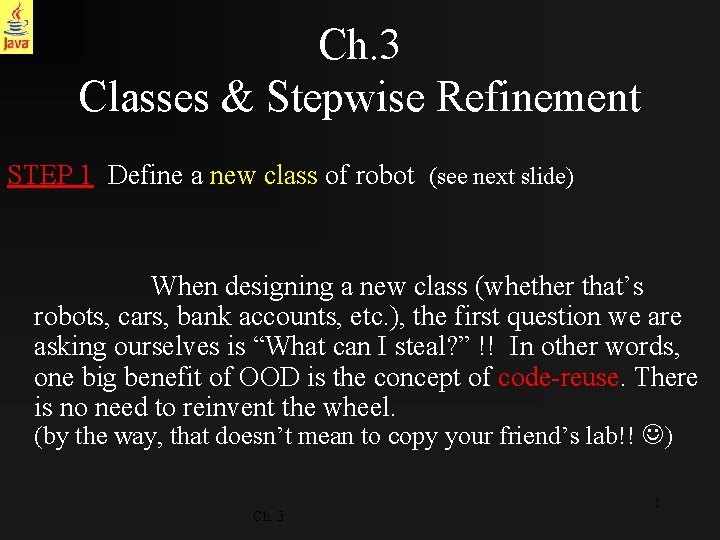
Ch. 3 Classes & Stepwise Refinement STEP 1 Define a new class of robot (see next slide) When designing a new class (whether that’s robots, cars, bank accounts, etc. ), the first question we are asking ourselves is “What can I steal? ” !! In other words, one big benefit of OOD is the concept of code-reuse. There is no need to reinvent the wheel. (by the way, that doesn’t mean to copy your friend’s lab!! ) Ch. 3 1
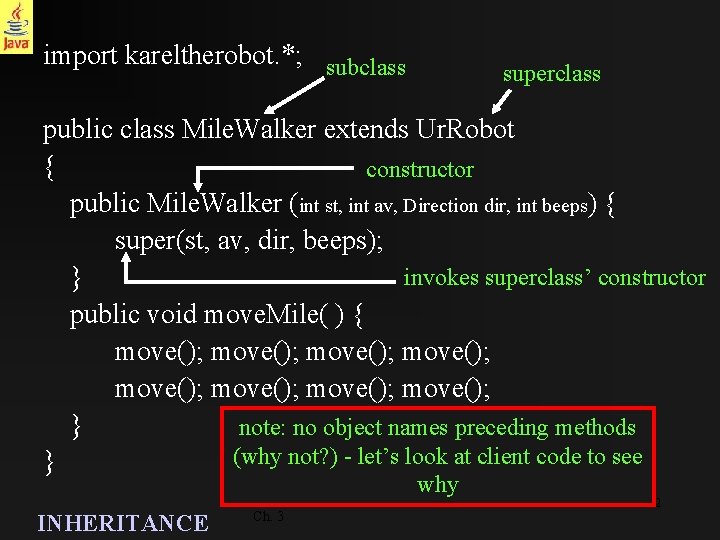
import kareltherobot. *; subclass superclass public class Mile. Walker extends Ur. Robot { constructor public Mile. Walker (int st, int av, Direction dir, int beeps) { super(st, av, dir, beeps); invokes superclass’ constructor } public void move. Mile( ) { move(); move(); } note: no object names preceding methods (why not? ) - let’s look at client code to see } why INHERITANCE Ch. 3 2
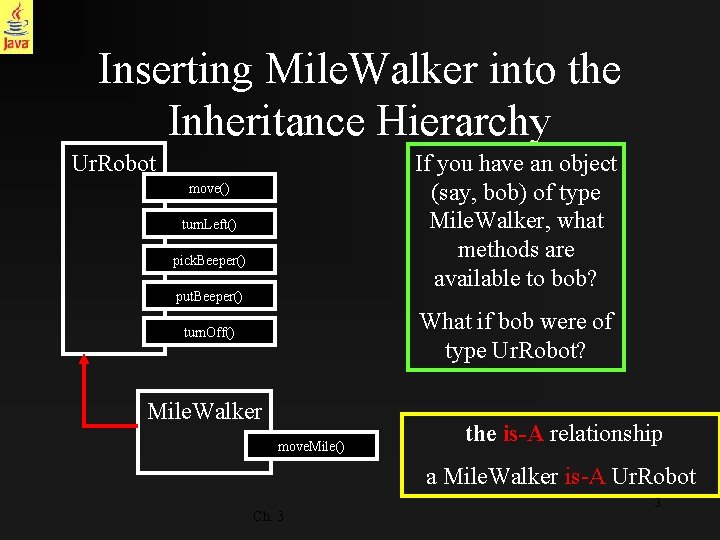
Inserting Mile. Walker into the Inheritance Hierarchy Ur. Robot If you have an object (say, bob) of type Mile. Walker, what methods are available to bob? move() turn. Left() pick. Beeper() put. Beeper() What if bob were of type Ur. Robot? turn. Off() Mile. Walker move. Mile() the is-A relationship a Mile. Walker is-A Ur. Robot Ch. 3 3
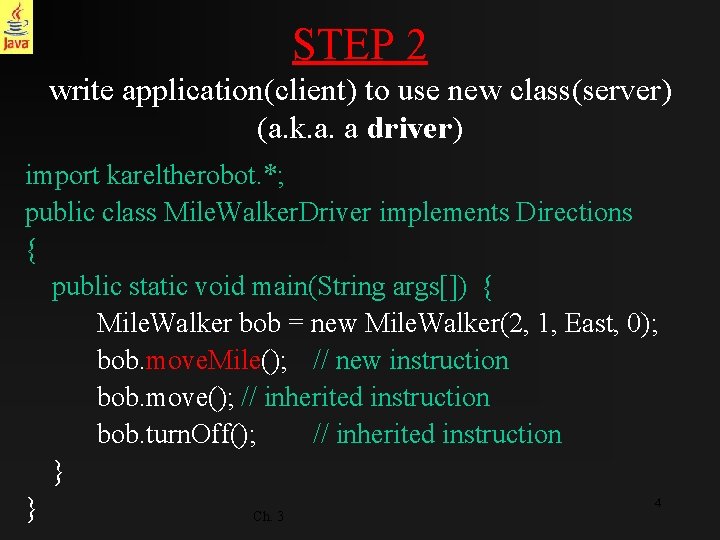
STEP 2 write application(client) to use new class(server) (a. k. a. a driver) import kareltherobot. *; public class Mile. Walker. Driver implements Directions { public static void main(String args[]) { Mile. Walker bob = new Mile. Walker(2, 1, East, 0); bob. move. Mile(); // new instruction bob. move(); // inherited instruction bob. turn. Off(); // inherited instruction } 4 } Ch. 3
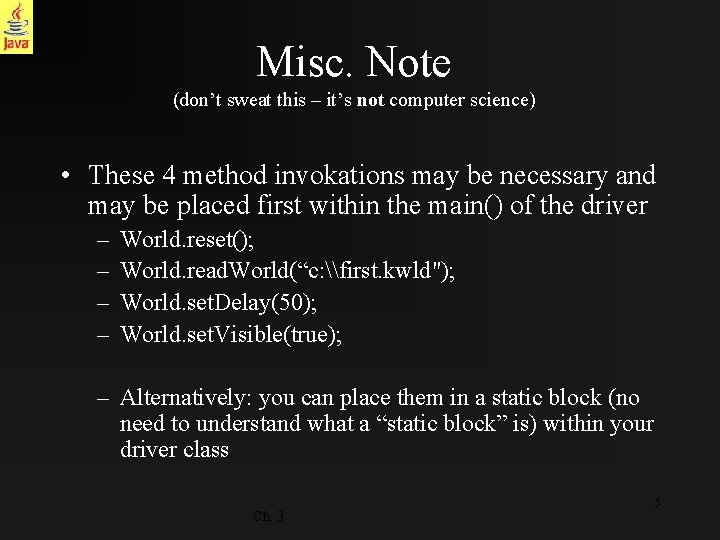
Misc. Note (don’t sweat this – it’s not computer science) • These 4 method invokations may be necessary and may be placed first within the main() of the driver – – World. reset(); World. read. World(“c: \first. kwld"); World. set. Delay(50); World. set. Visible(true); – Alternatively: you can place them in a static block (no need to understand what a “static block” is) within your driver class Ch. 3 5
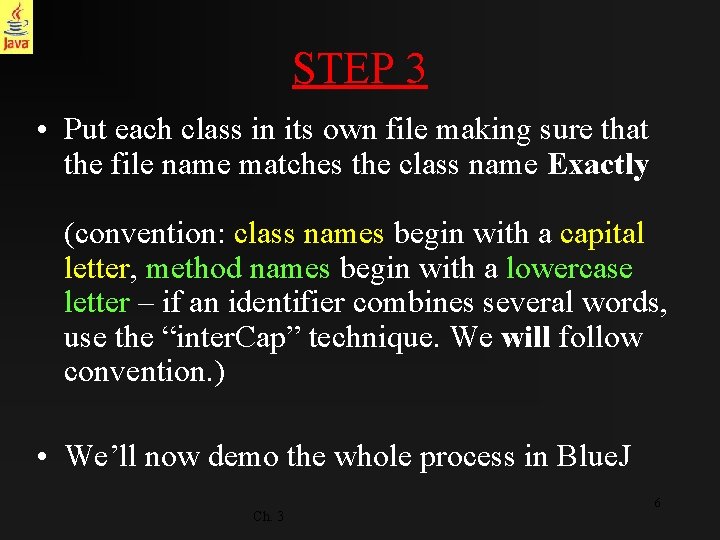
STEP 3 • Put each class in its own file making sure that the file name matches the class name Exactly (convention: class names begin with a capital letter, method names begin with a lowercase letter – if an identifier combines several words, use the “inter. Cap” technique. We will follow convention. ) • We’ll now demo the whole process in Blue. J Ch. 3 6
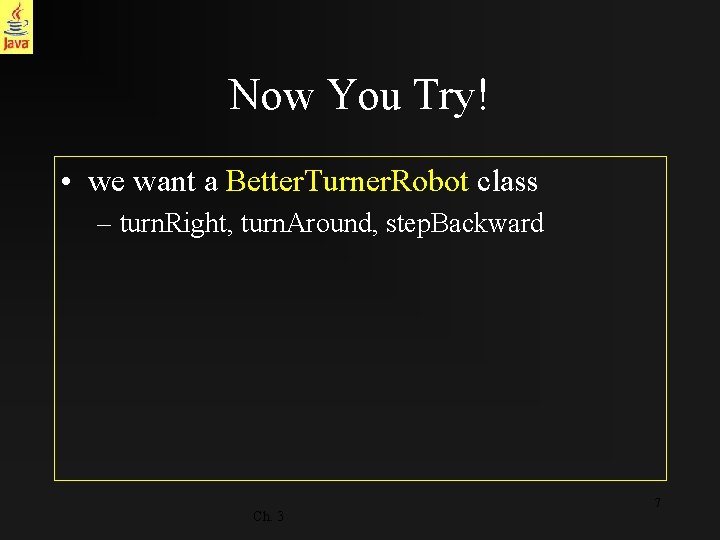
Now You Try! • we want a Better. Turner. Robot class – turn. Right, turn. Around, step. Backward Ch. 3 7
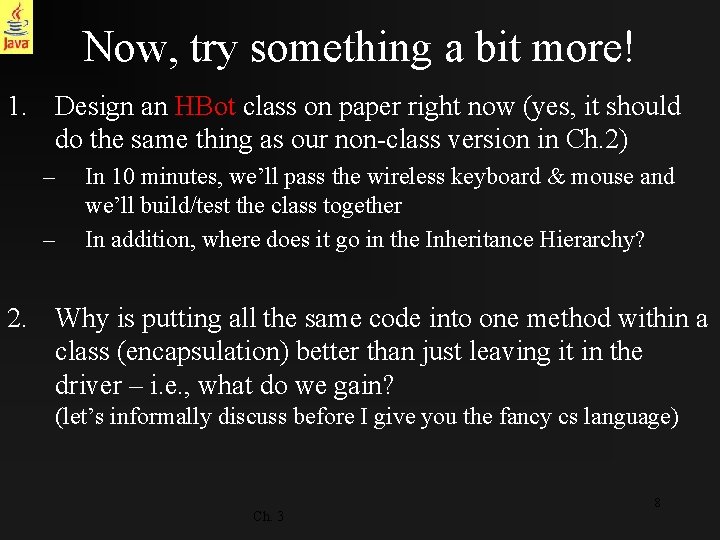
Now, try something a bit more! 1. Design an HBot class on paper right now (yes, it should do the same thing as our non-class version in Ch. 2) – – In 10 minutes, we’ll pass the wireless keyboard & mouse and we’ll build/test the class together In addition, where does it go in the Inheritance Hierarchy? 2. Why is putting all the same code into one method within a class (encapsulation) better than just leaving it in the driver – i. e. , what do we gain? (let’s informally discuss before I give you the fancy cs language) Ch. 3 8
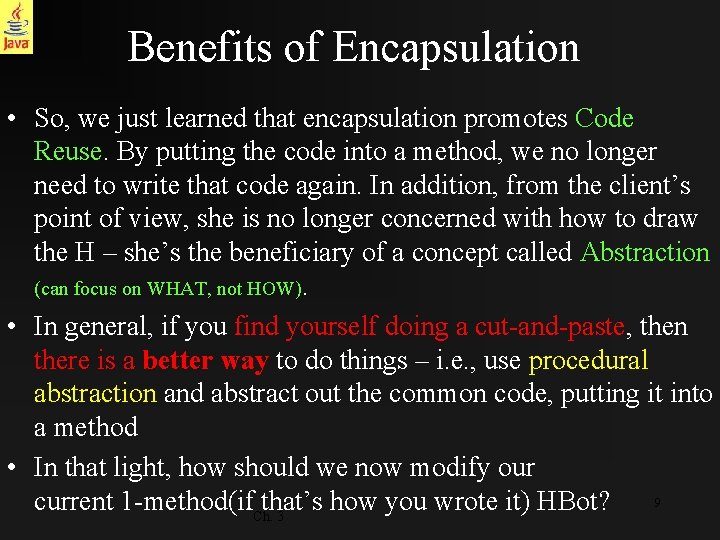
Benefits of Encapsulation • So, we just learned that encapsulation promotes Code Reuse. By putting the code into a method, we no longer need to write that code again. In addition, from the client’s point of view, she is no longer concerned with how to draw the H – she’s the beneficiary of a concept called Abstraction (can focus on WHAT, not HOW). • In general, if you find yourself doing a cut-and-paste, then there is a better way to do things – i. e. , use procedural abstraction and abstract out the common code, putting it into a method • In that light, how should we now modify our 9 current 1 -method(if. Ch. that’s how you wrote it) HBot? 3
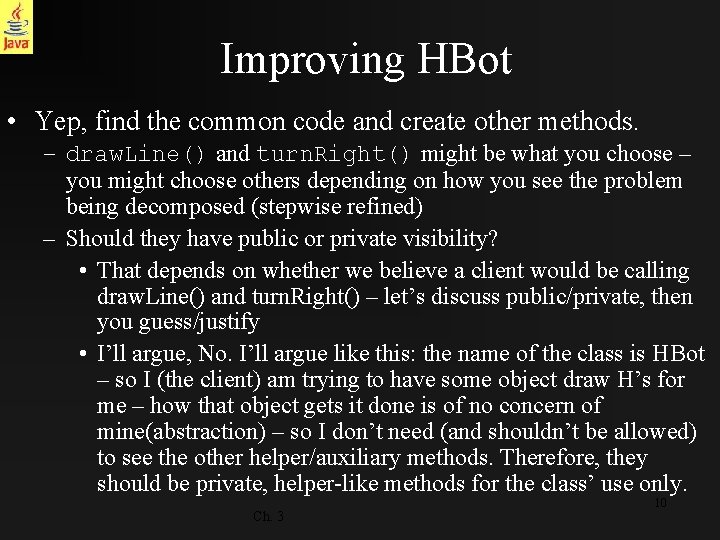
Improving HBot • Yep, find the common code and create other methods. – draw. Line() and turn. Right() might be what you choose – you might choose others depending on how you see the problem being decomposed (stepwise refined) – Should they have public or private visibility? • That depends on whether we believe a client would be calling draw. Line() and turn. Right() – let’s discuss public/private, then you guess/justify • I’ll argue, No. I’ll argue like this: the name of the class is HBot – so I (the client) am trying to have some object draw H’s for me – how that object gets it done is of no concern of mine(abstraction) – so I don’t need (and shouldn’t be allowed) to see the other helper/auxiliary methods. Therefore, they should be private, helper-like methods for the class’ use only. Ch. 3 10
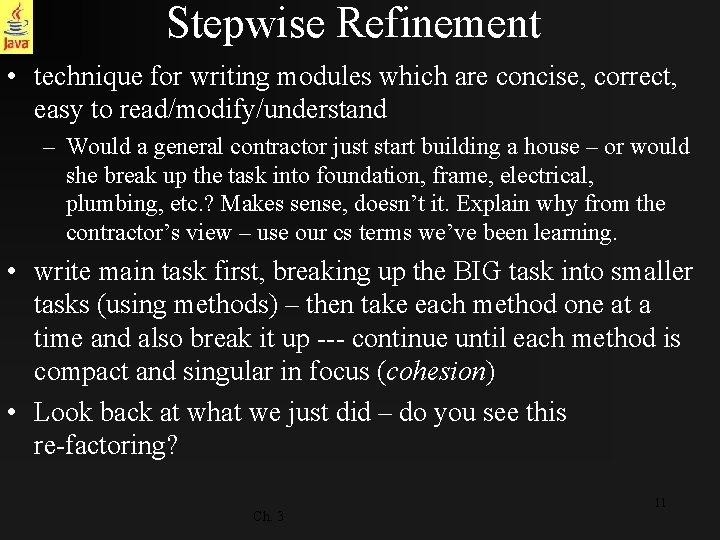
Stepwise Refinement • technique for writing modules which are concise, correct, easy to read/modify/understand – Would a general contractor just start building a house – or would she break up the task into foundation, frame, electrical, plumbing, etc. ? Makes sense, doesn’t it. Explain why from the contractor’s view – use our cs terms we’ve been learning. • write main task first, breaking up the BIG task into smaller tasks (using methods) – then take each method one at a time and also break it up --- continue until each method is compact and singular in focus (cohesion) • Look back at what we just did – do you see this re-factoring? Ch. 3 11
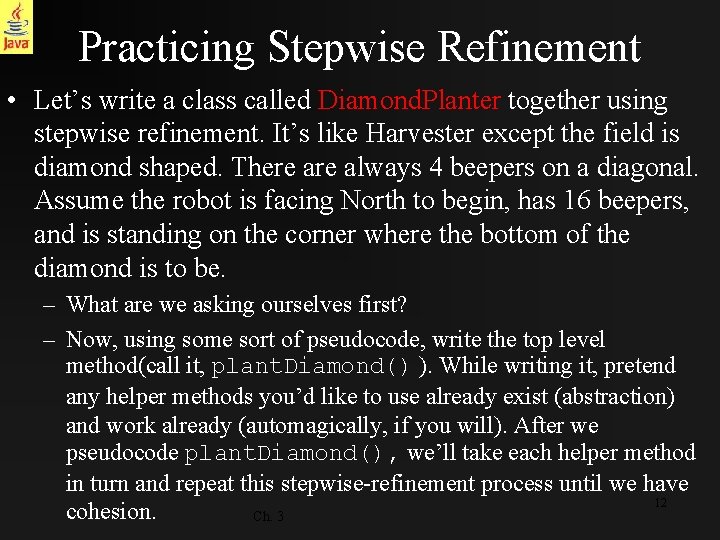
Practicing Stepwise Refinement • Let’s write a class called Diamond. Planter together using stepwise refinement. It’s like Harvester except the field is diamond shaped. There always 4 beepers on a diagonal. Assume the robot is facing North to begin, has 16 beepers, and is standing on the corner where the bottom of the diamond is to be. – What are we asking ourselves first? – Now, using some sort of pseudocode, write the top level method(call it, plant. Diamond() ). While writing it, pretend any helper methods you’d like to use already exist (abstraction) and work already (automagically, if you will). After we pseudocode plant. Diamond(), we’ll take each helper method in turn and repeat this stepwise-refinement process until we have 12 cohesion. Ch. 3
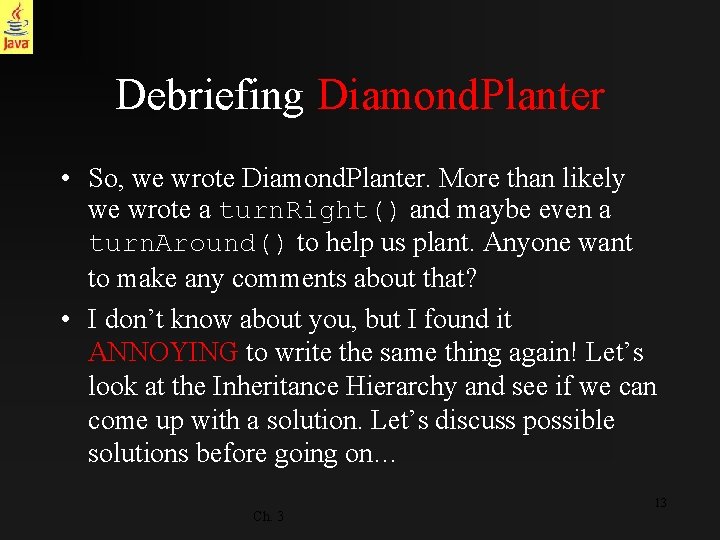
Debriefing Diamond. Planter • So, we wrote Diamond. Planter. More than likely we wrote a turn. Right() and maybe even a turn. Around() to help us plant. Anyone want to make any comments about that? • I don’t know about you, but I found it ANNOYING to write the same thing again! Let’s look at the Inheritance Hierarchy and see if we can come up with a solution. Let’s discuss possible solutions before going on… Ch. 3 13
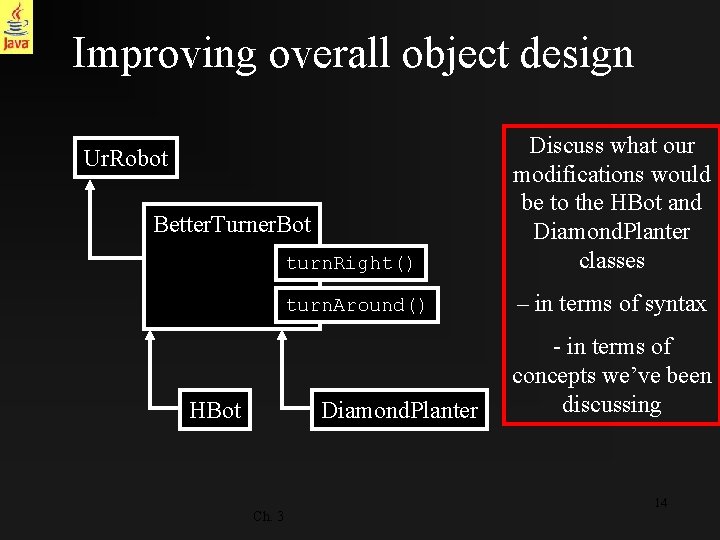
Improving overall object design turn. Right() Discuss what our modifications would be to the HBot and Diamond. Planter classes turn. Around() – in terms of syntax Ur. Robot Better. Turner. Bot HBot Diamond. Planter Ch. 3 - in terms of concepts we’ve been discussing 14
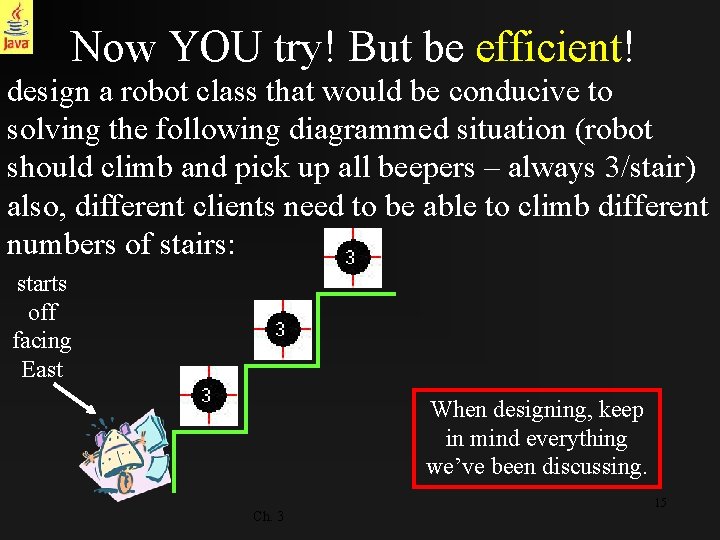
Now YOU try! But be efficient! design a robot class that would be conducive to solving the following diagrammed situation (robot should climb and pick up all beepers – always 3/stair) also, different clients need to be able to climb different numbers of stairs: starts off facing East When designing, keep in mind everything we’ve been discussing. Ch. 3 15
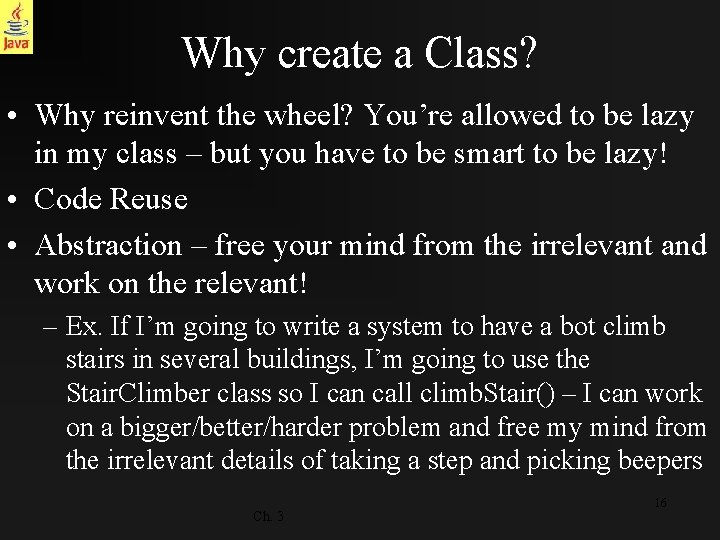
Why create a Class? • Why reinvent the wheel? You’re allowed to be lazy in my class – but you have to be smart to be lazy! • Code Reuse • Abstraction – free your mind from the irrelevant and work on the relevant! – Ex. If I’m going to write a system to have a bot climb stairs in several buildings, I’m going to use the Stair. Climber class so I can call climb. Stair() – I can work on a bigger/better/harder problem and free my mind from the irrelevant details of taking a step and picking beepers Ch. 3 16
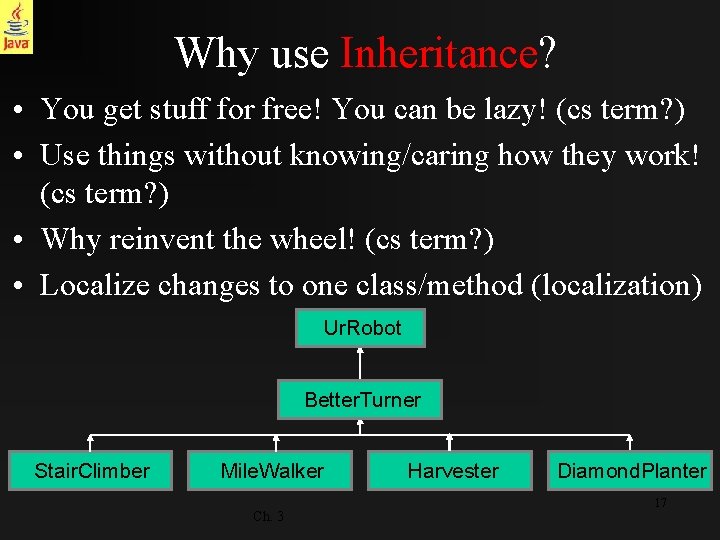
Why use Inheritance? • You get stuff for free! You can be lazy! (cs term? ) • Use things without knowing/caring how they work! (cs term? ) • Why reinvent the wheel! (cs term? ) • Localize changes to one class/method (localization) Ur. Robot Better. Turner Stair. Climber Mile. Walker Ch. 3 Harvester Diamond. Planter 17
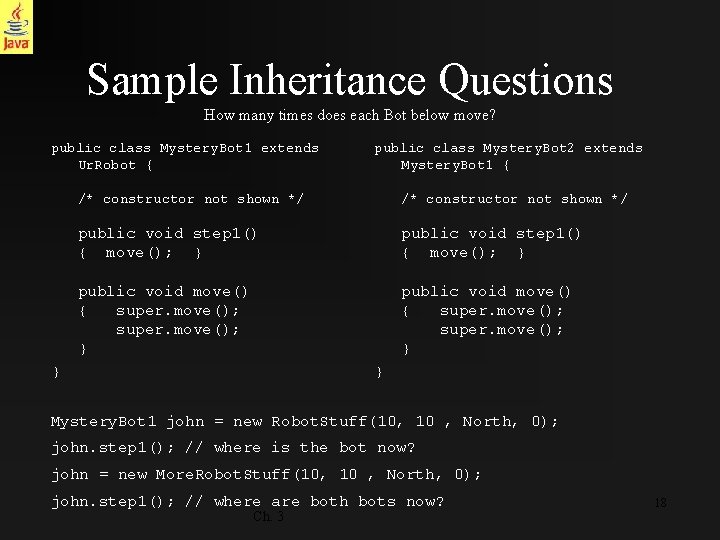
Sample Inheritance Questions How many times does each Bot below move? public class Mystery. Bot 1 extends Ur. Robot { public class Mystery. Bot 2 extends Mystery. Bot 1 { /* constructor not shown */ public void step 1() { move(); } public void move() { super. move(); } } } Mystery. Bot 1 john = new Robot. Stuff(10, 10 , North, 0); john. step 1(); // where is the bot now? john = new More. Robot. Stuff(10, 10 , North, 0); john. step 1(); // where are both bots now? Ch. 3 18
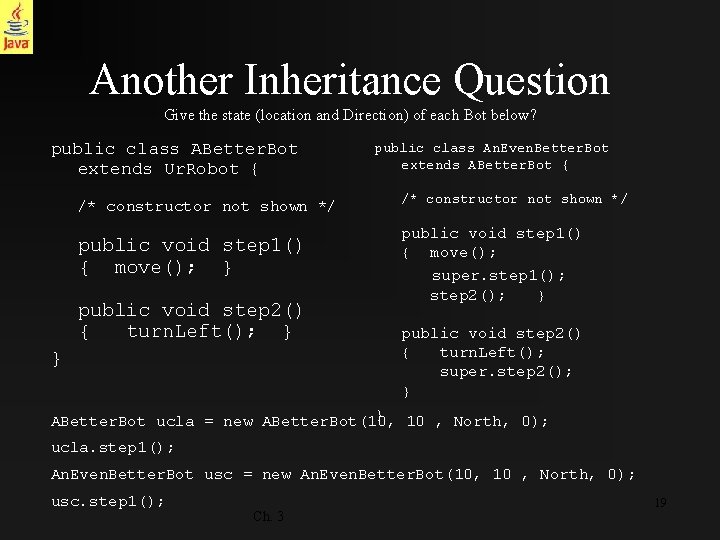
Another Inheritance Question Give the state (location and Direction) of each Bot below? public class ABetter. Bot extends Ur. Robot { /* constructor not shown */ public void step 1() { move(); } public void step 2() { turn. Left(); } } public class An. Even. Better. Bot extends ABetter. Bot { /* constructor not shown */ public void step 1() { move(); super. step 1(); step 2(); } public void step 2() { turn. Left(); super. step 2(); } } 10 , North, 0); ABetter. Bot ucla = new ABetter. Bot(10, ucla. step 1(); An. Even. Better. Bot usc = new An. Even. Better. Bot(10, 10 , North, 0); usc. step 1(); Ch. 3 19
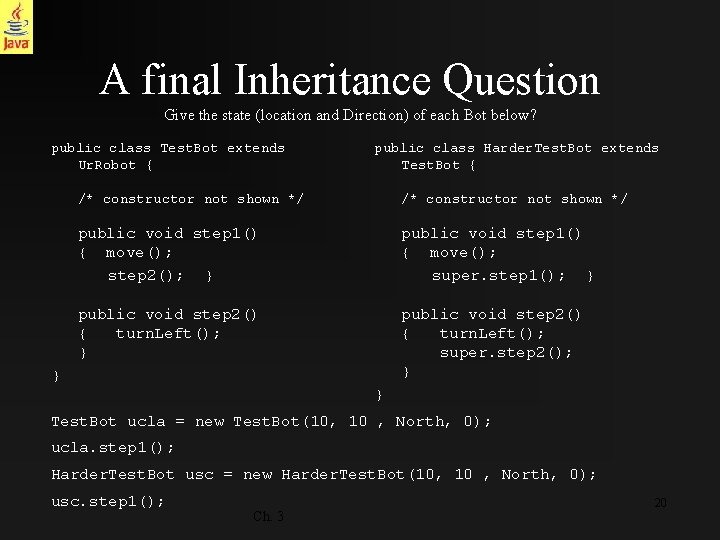
A final Inheritance Question Give the state (location and Direction) of each Bot below? public class Test. Bot extends Ur. Robot { public class Harder. Test. Bot extends Test. Bot { /* constructor not shown */ public void step 1() { move(); step 2(); } public void step 1() { move(); super. step 1(); } public void step 2() { turn. Left(); super. step 2(); } } } Test. Bot ucla = new Test. Bot(10, 10 , North, 0); ucla. step 1(); Harder. Test. Bot usc = new Harder. Test. Bot(10, 10 , North, 0); usc. step 1(); Ch. 3 20
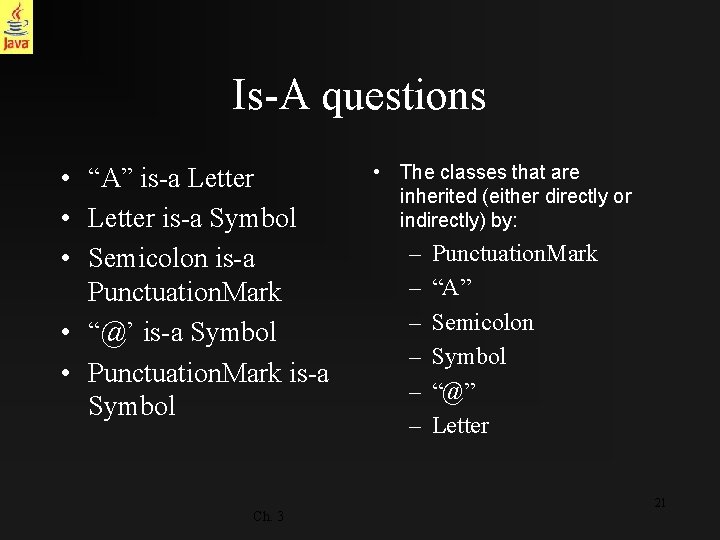
Is-A questions • “A” is-a Letter • Letter is-a Symbol • Semicolon is-a Punctuation. Mark • “@’ is-a Symbol • Punctuation. Mark is-a Symbol Ch. 3 • The classes that are inherited (either directly or indirectly) by: – – – Punctuation. Mark “A” Semicolon Symbol “@” Letter 21
Stepwise refinement definition
Stepwise refinement python
Step 1 step 2 step 3 step 4
Disjunctive topic shift
Stepwise regression minitab
Stepwise airway management
Contoh stepwise
Stepwise regression eviews
Regression anal
Classe e subclasse dos nomes
Pre ap classes vs regular classes
Flush terminal plane
Creating a dinosaur sculpture step by step
Pbpa writing
Persuasive essay sentence starters
Step up step back
Factoring ways
Harder simultaneous equations
Simultaneous equations step by step
How to combine like terms step by step
Steps in photosynthesis
Matlab particle filter example