Arrays ConstructorsDestructors INFSY 440 Lecture 2 Spring 2004
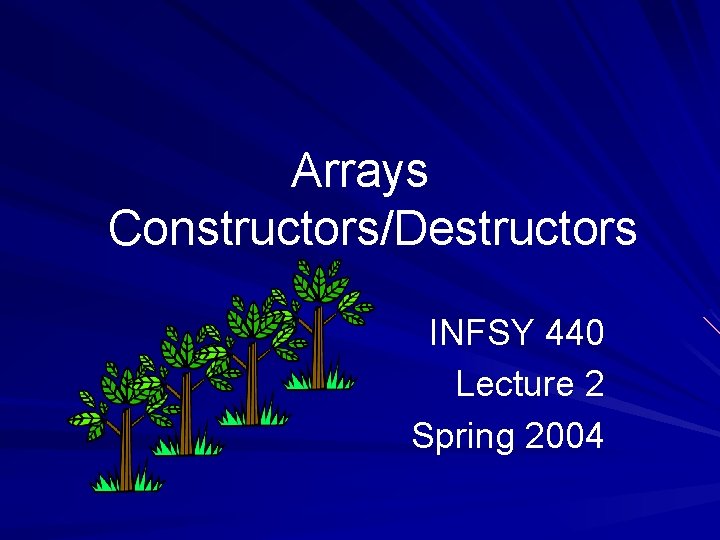
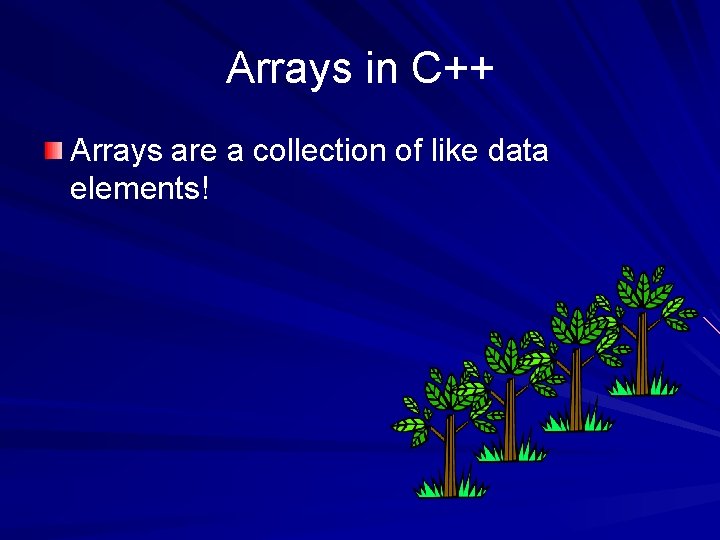
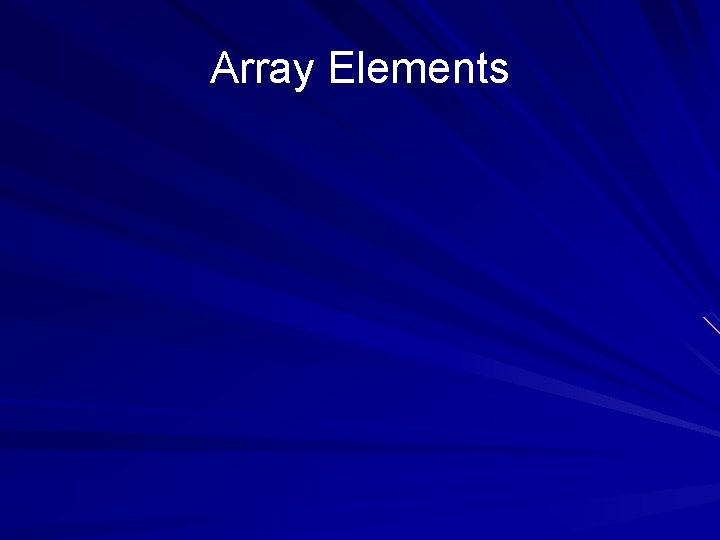
![Arrays int num[5]; Arrays int num[5];](https://slidetodoc.com/presentation_image_h2/fd0546c5e51fdb8c3ba45c1f7f857cb4/image-4.jpg)
![Arrays int num [5]; for (int i = 0; i < 5; i++) { Arrays int num [5]; for (int i = 0; i < 5; i++) {](https://slidetodoc.com/presentation_image_h2/fd0546c5e51fdb8c3ba45c1f7f857cb4/image-5.jpg)
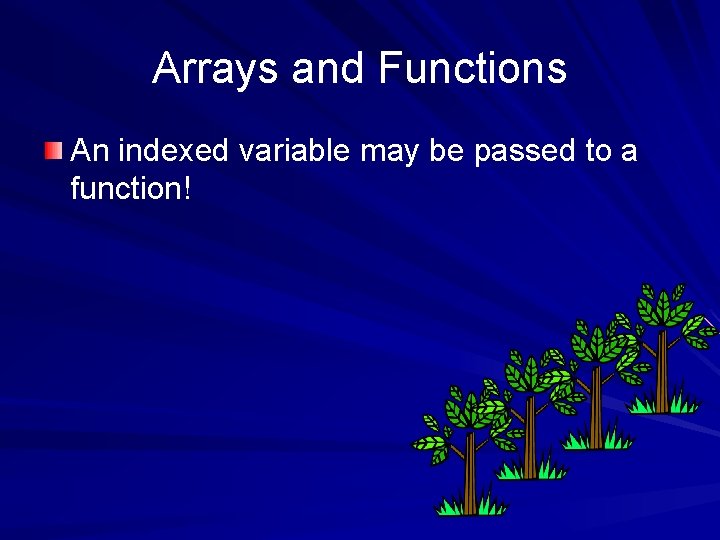
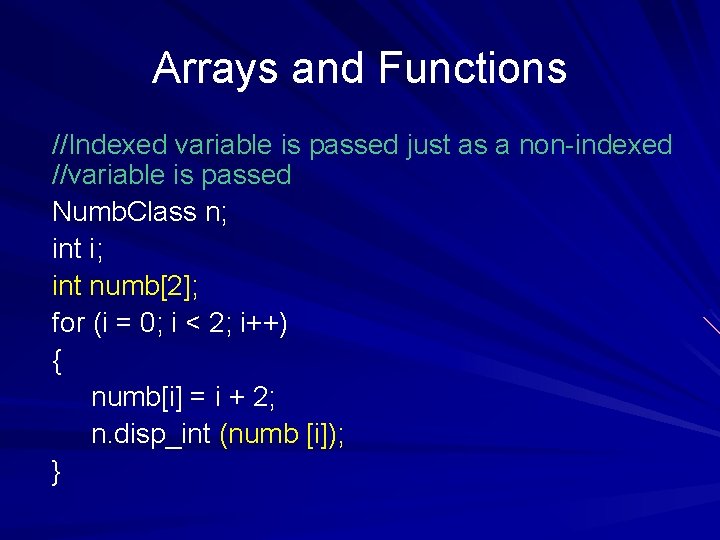
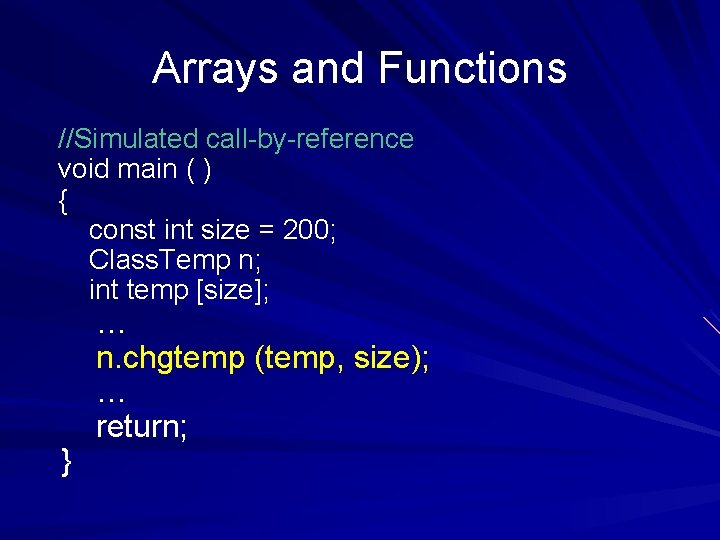
![The class function, chgtemp class Class. Temp { public: void chgtemp (int [ ], The class function, chgtemp class Class. Temp { public: void chgtemp (int [ ],](https://slidetodoc.com/presentation_image_h2/fd0546c5e51fdb8c3ba45c1f7f857cb4/image-9.jpg)
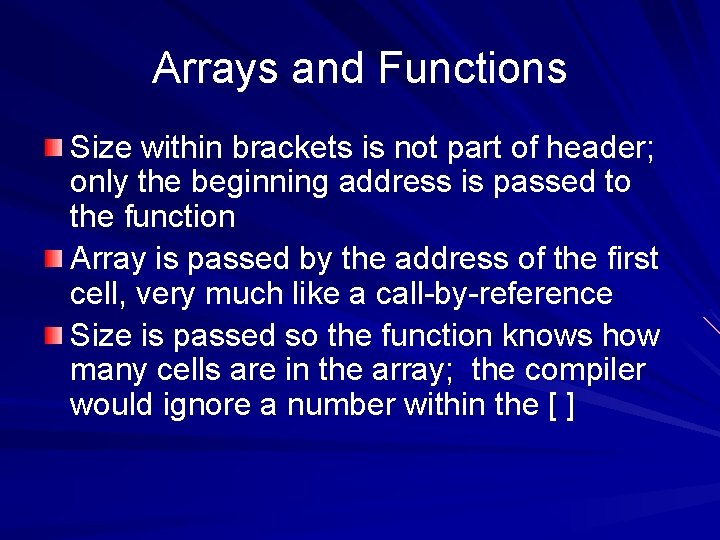
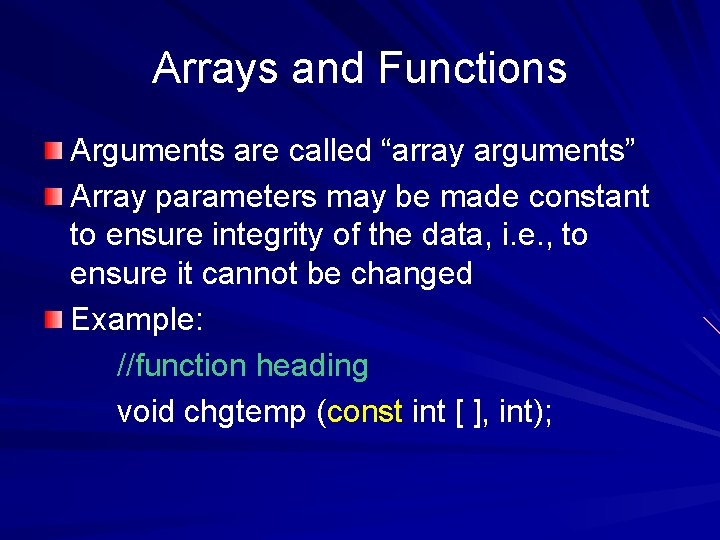
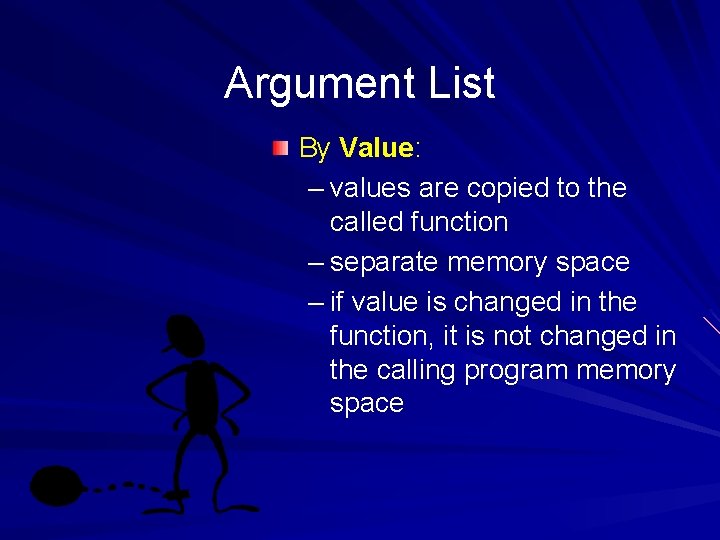
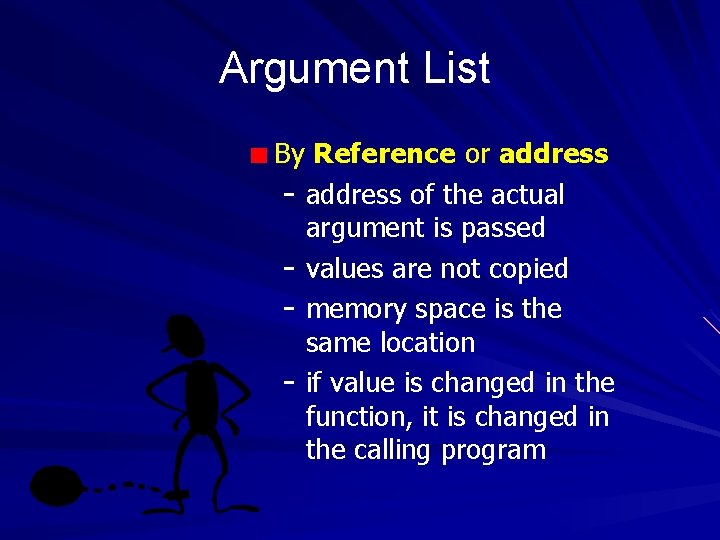
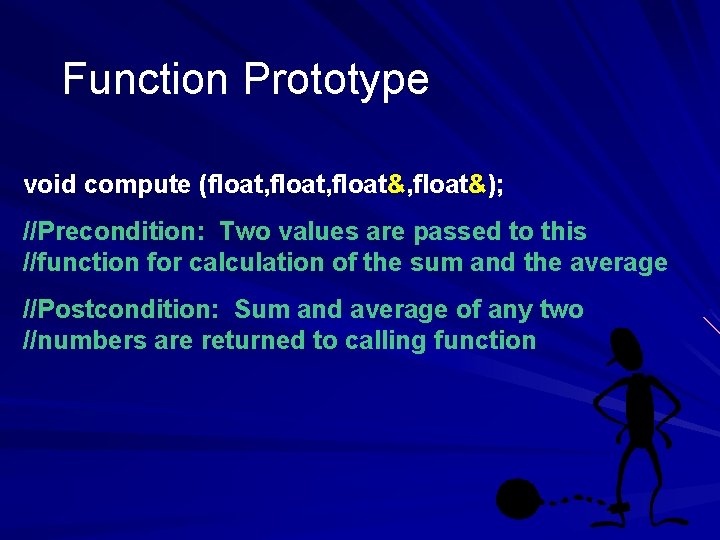
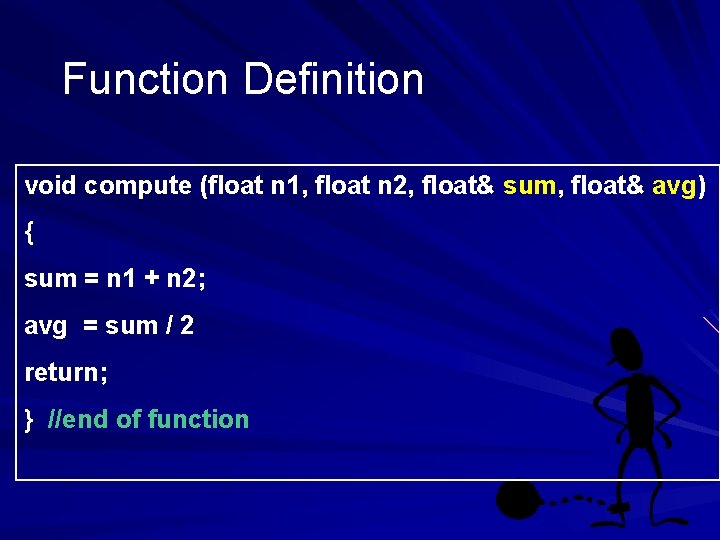
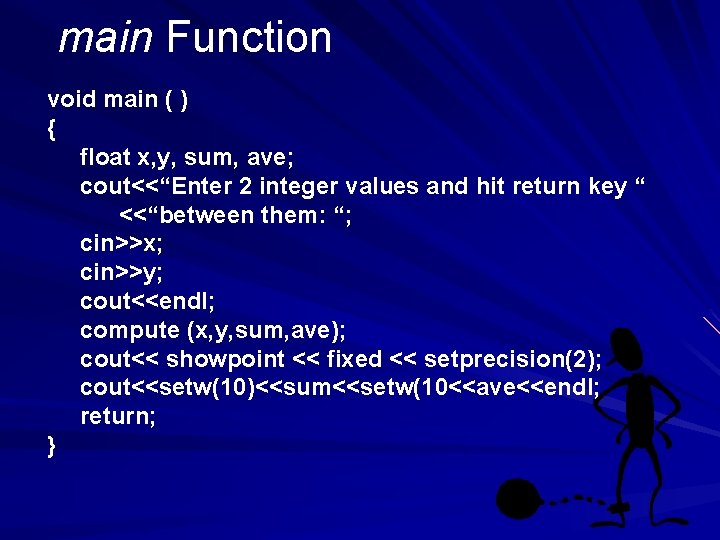
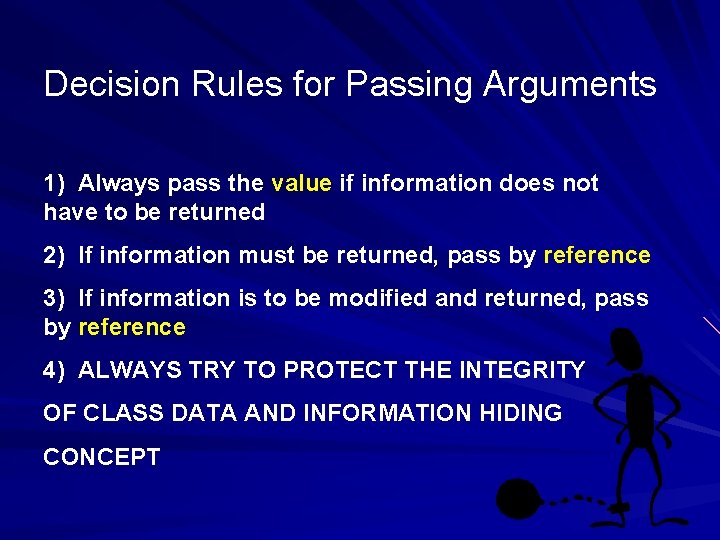
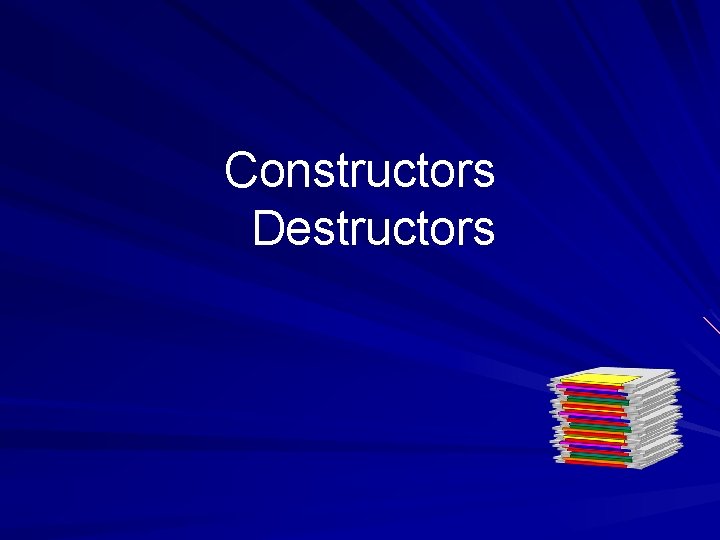
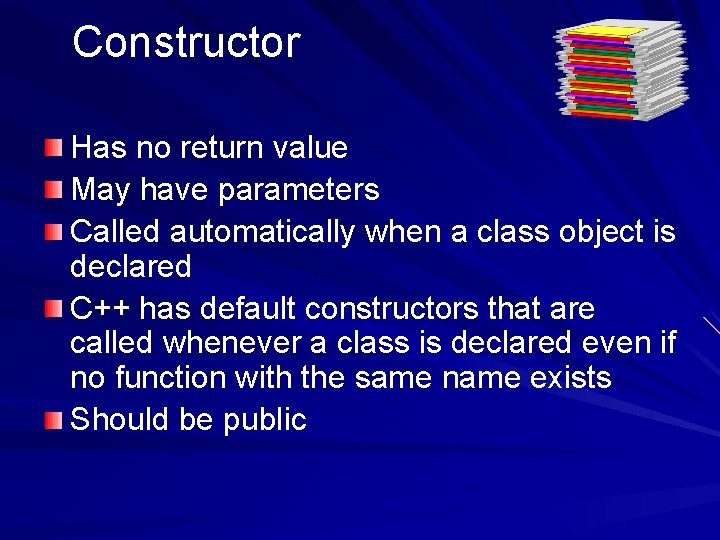
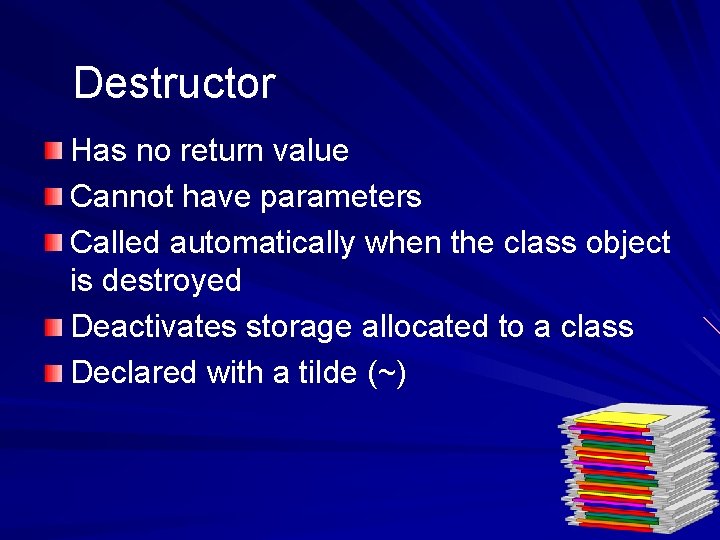
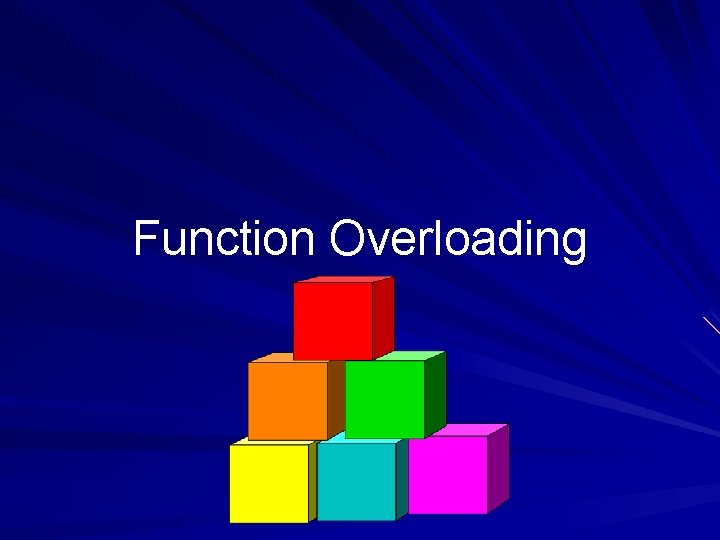
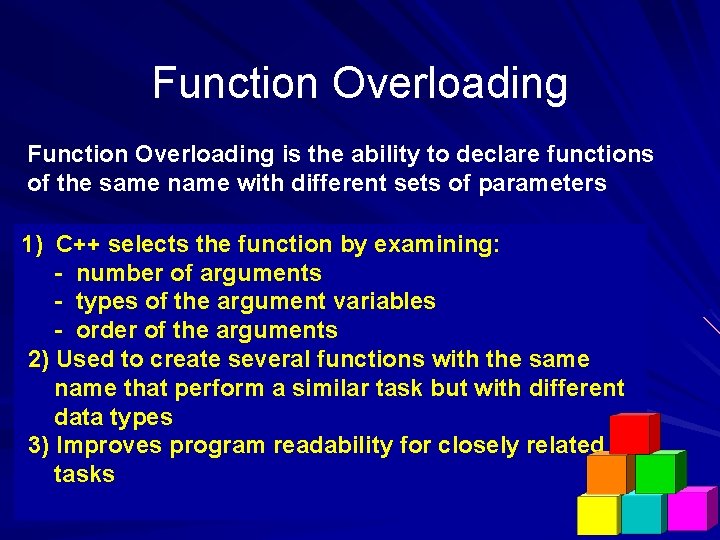
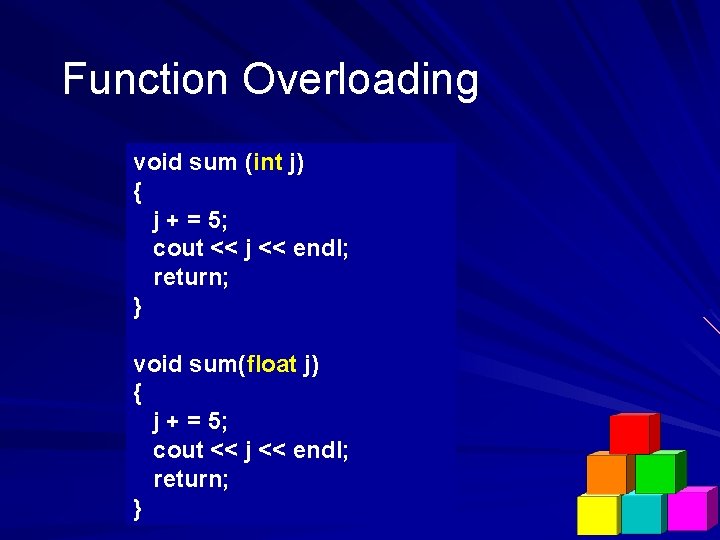
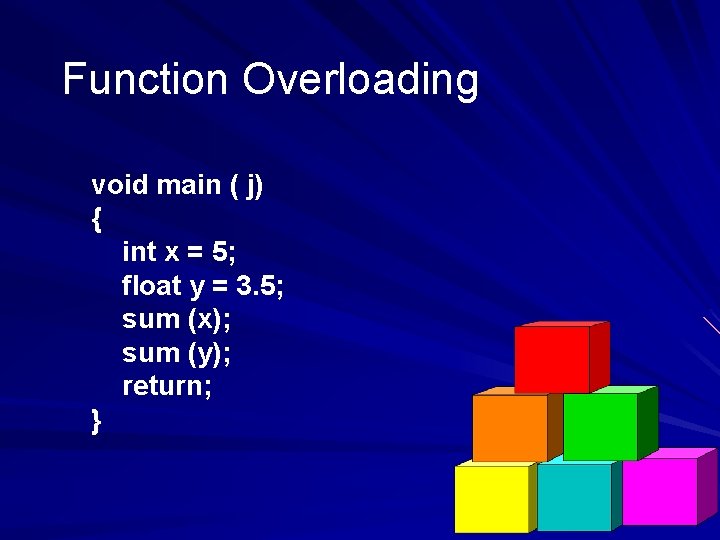
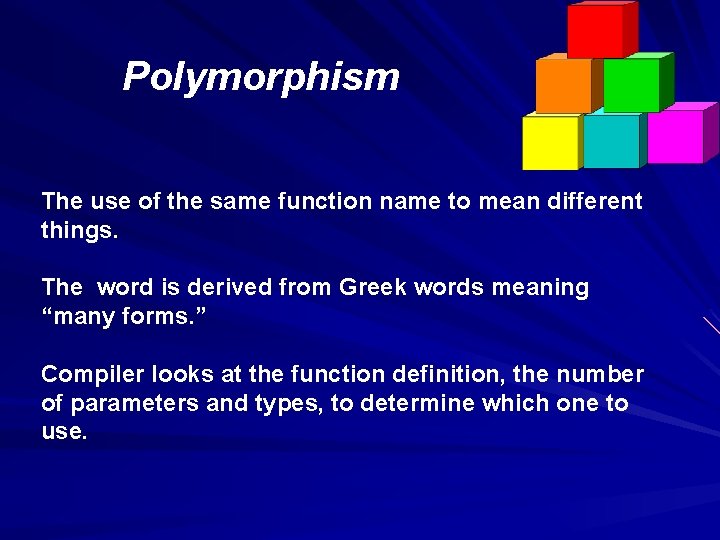
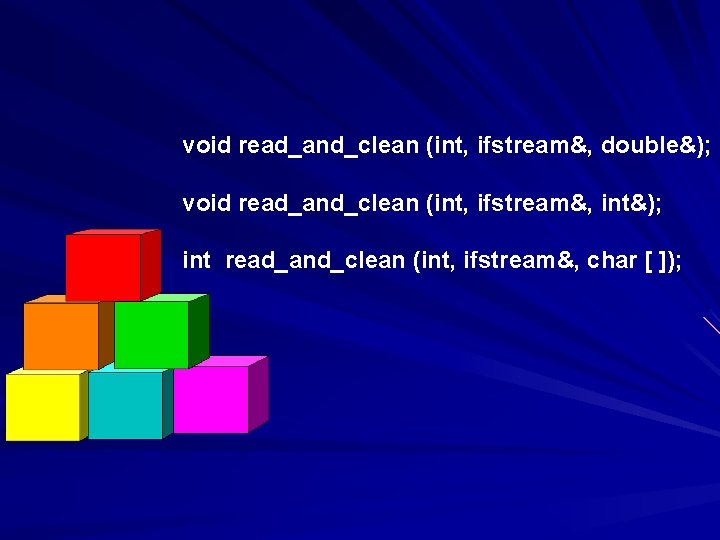
- Slides: 26
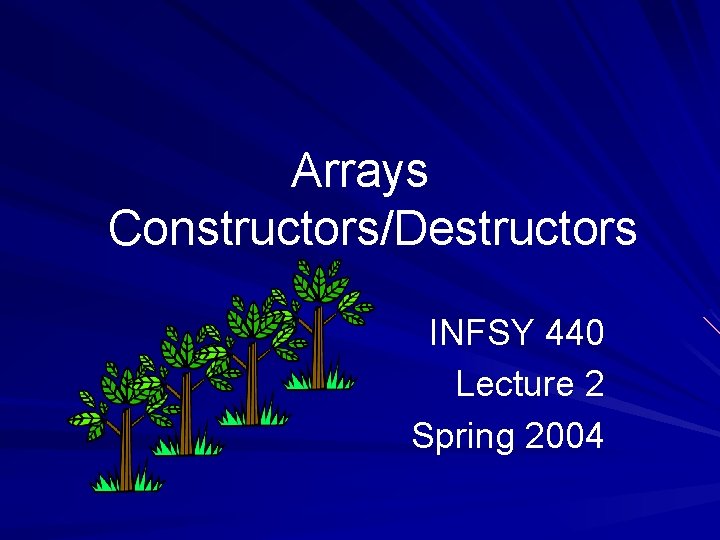
Arrays Constructors/Destructors INFSY 440 Lecture 2 Spring 2004
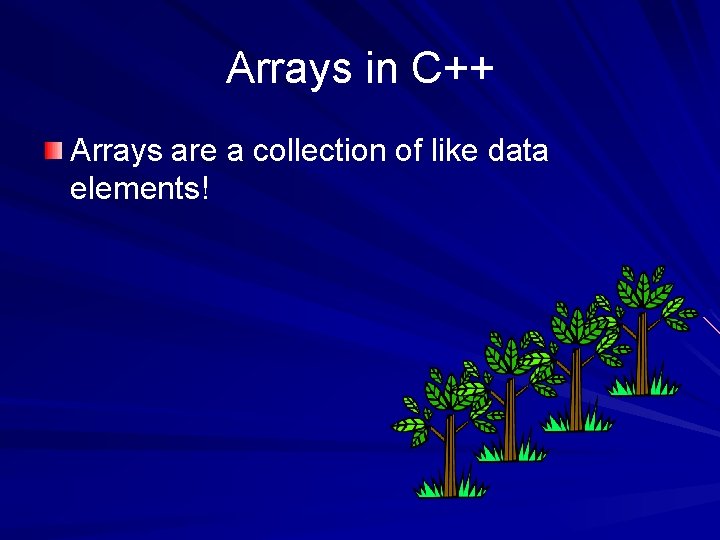
Arrays in C++ Arrays are a collection of like data elements!
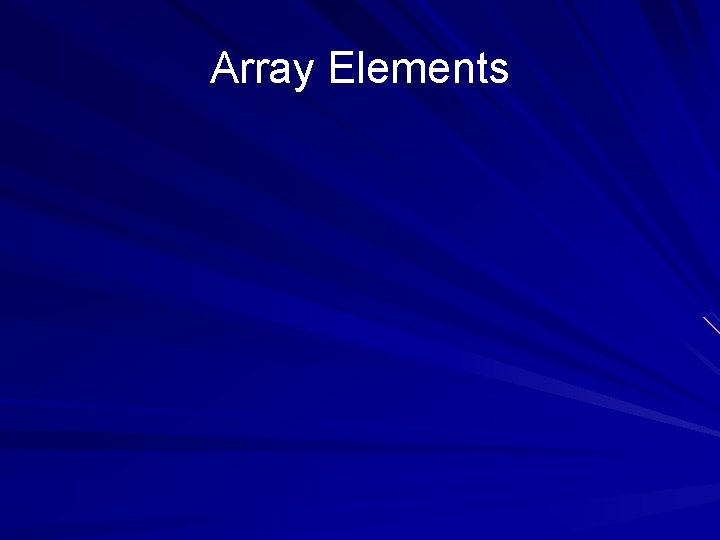
Array Elements
![Arrays int num5 Arrays int num[5];](https://slidetodoc.com/presentation_image_h2/fd0546c5e51fdb8c3ba45c1f7f857cb4/image-4.jpg)
Arrays int num[5];
![Arrays int num 5 for int i 0 i 5 i Arrays int num [5]; for (int i = 0; i < 5; i++) {](https://slidetodoc.com/presentation_image_h2/fd0546c5e51fdb8c3ba45c1f7f857cb4/image-5.jpg)
Arrays int num [5]; for (int i = 0; i < 5; i++) { cin >> num[i]; }
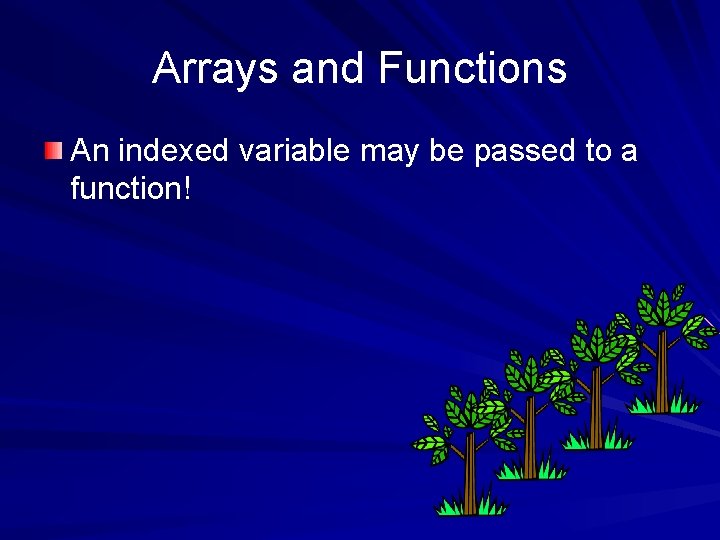
Arrays and Functions An indexed variable may be passed to a function!
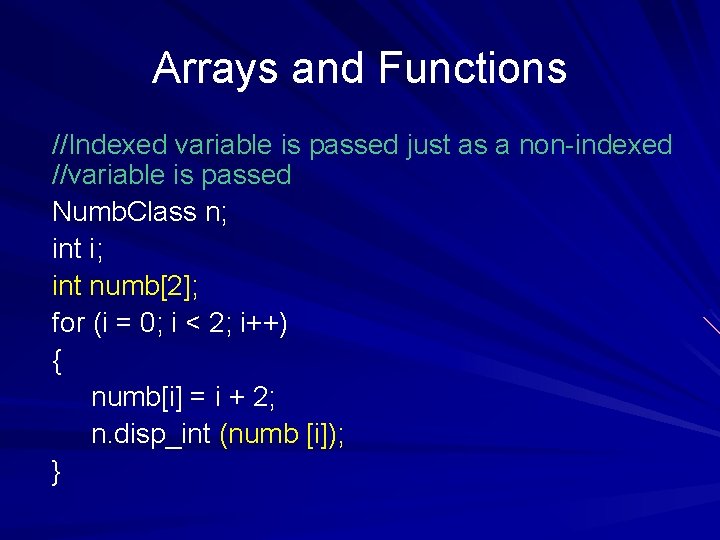
Arrays and Functions //Indexed variable is passed just as a non-indexed //variable is passed Numb. Class n; int i; int numb[2]; for (i = 0; i < 2; i++) { numb[i] = i + 2; n. disp_int (numb [i]); }
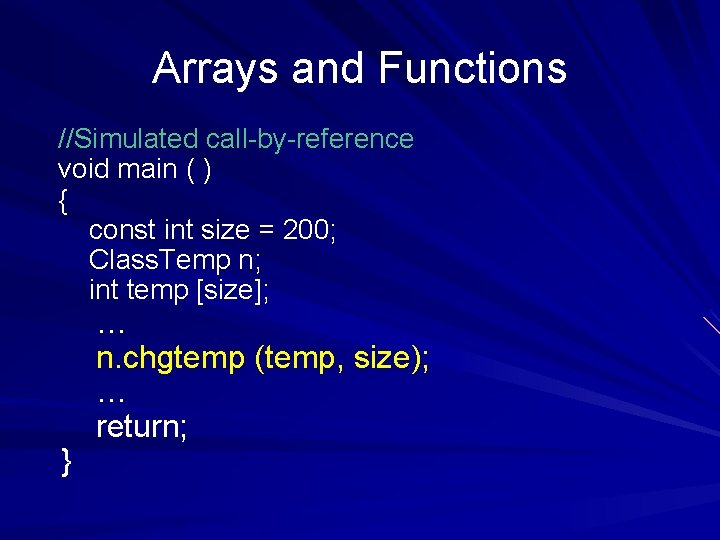
Arrays and Functions //Simulated call-by-reference void main ( ) { const int size = 200; Class. Temp n; int temp [size]; } … n. chgtemp (temp, size); … return;
![The class function chgtemp class Class Temp public void chgtemp int The class function, chgtemp class Class. Temp { public: void chgtemp (int [ ],](https://slidetodoc.com/presentation_image_h2/fd0546c5e51fdb8c3ba45c1f7f857cb4/image-9.jpg)
The class function, chgtemp class Class. Temp { public: void chgtemp (int [ ], int); private: }; void Class. Temp: : chgtemp(int x[ ], int size) { ……
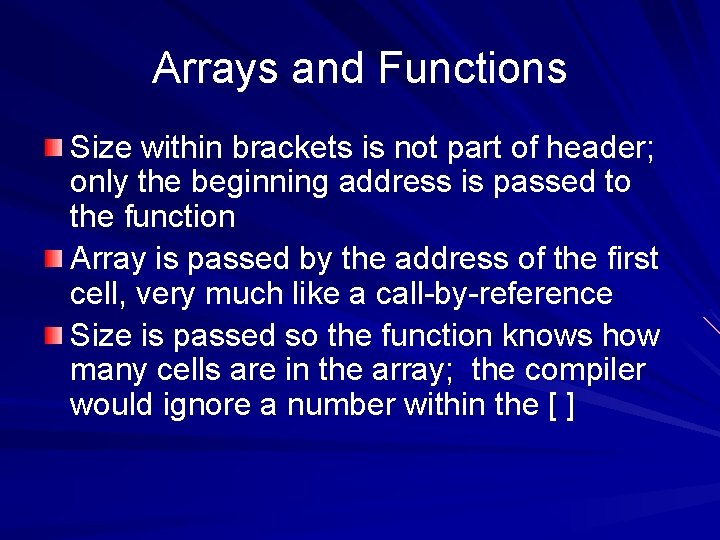
Arrays and Functions Size within brackets is not part of header; only the beginning address is passed to the function Array is passed by the address of the first cell, very much like a call-by-reference Size is passed so the function knows how many cells are in the array; the compiler would ignore a number within the [ ]
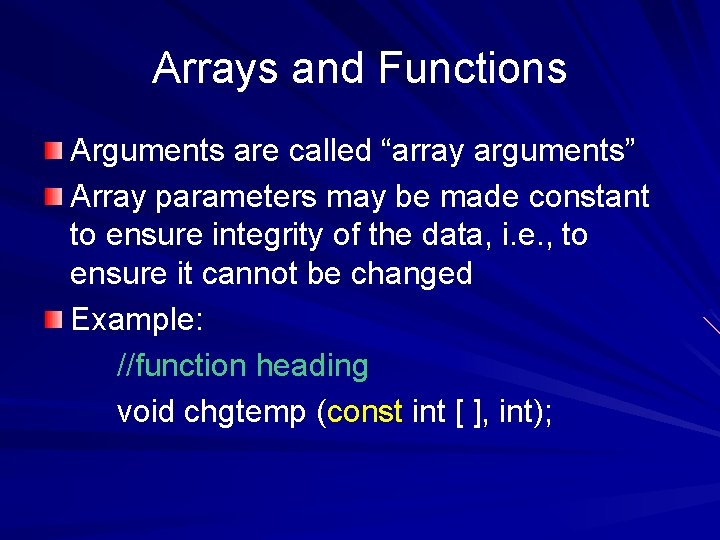
Arrays and Functions Arguments are called “array arguments” Array parameters may be made constant to ensure integrity of the data, i. e. , to ensure it cannot be changed Example: //function heading void chgtemp (const int [ ], int);
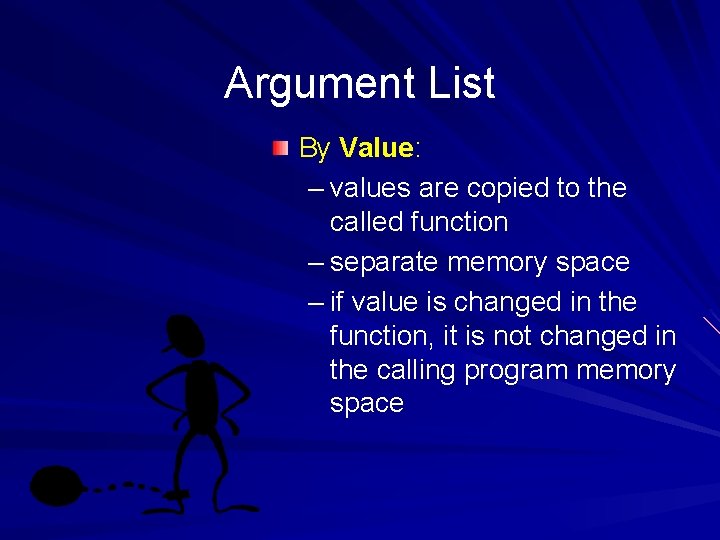
Argument List By Value: – values are copied to the called function – separate memory space – if value is changed in the function, it is not changed in the calling program memory space
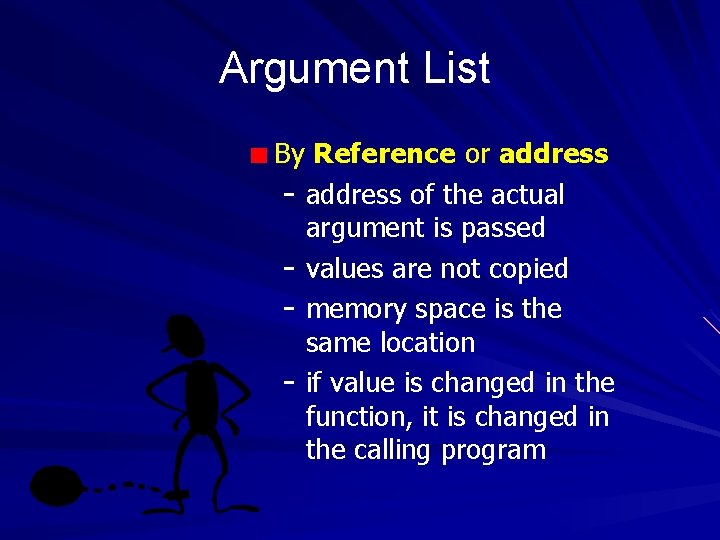
Argument List By Reference or address - address of the actual argument is passed - values are not copied - memory space is the same location - if value is changed in the function, it is changed in the calling program
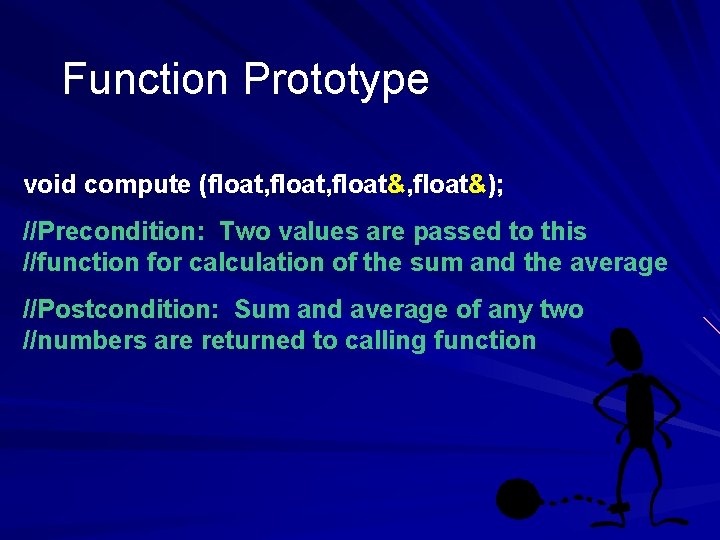
Function Prototype void compute (float, float&, float&); //Precondition: Two values are passed to this //function for calculation of the sum and the average //Postcondition: Sum and average of any two //numbers are returned to calling function
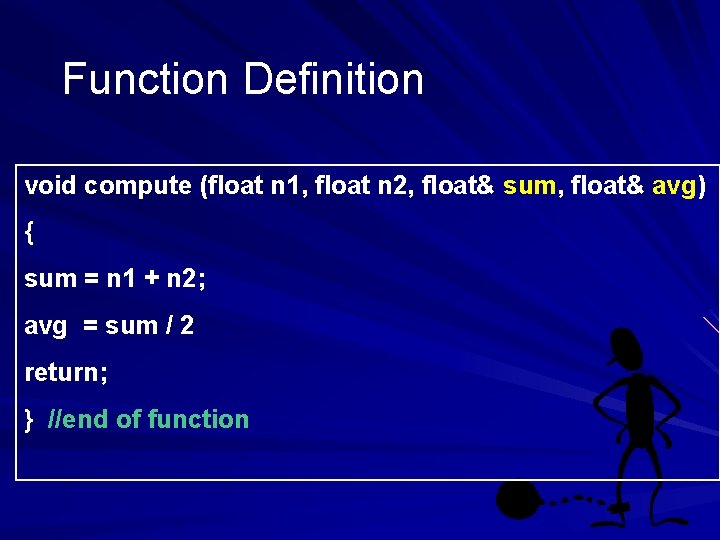
Function Definition void compute (float n 1, float n 2, float& sum, float& avg) { sum = n 1 + n 2; avg = sum / 2 return; } //end of function
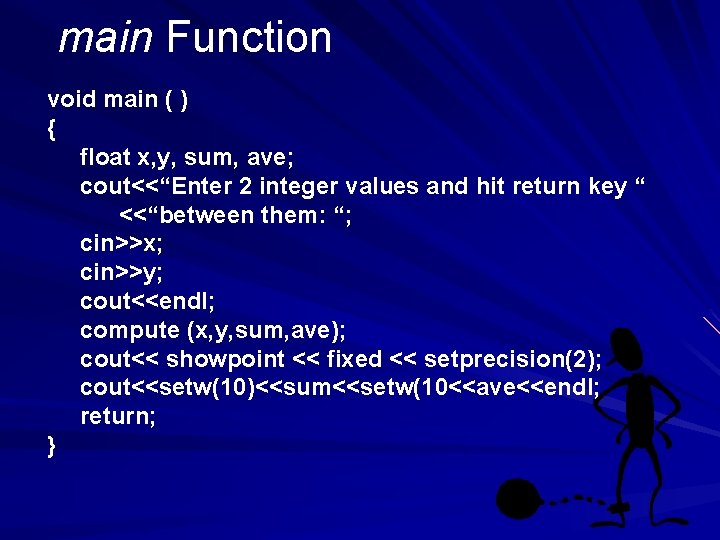
main Function void main ( ) { float x, y, sum, ave; cout<<“Enter 2 integer values and hit return key “ <<“between them: “; cin>>x; cin>>y; cout<<endl; compute (x, y, sum, ave); cout<< showpoint << fixed << setprecision(2); cout<<setw(10)<<sum<<setw(10<<ave<<endl; return; }
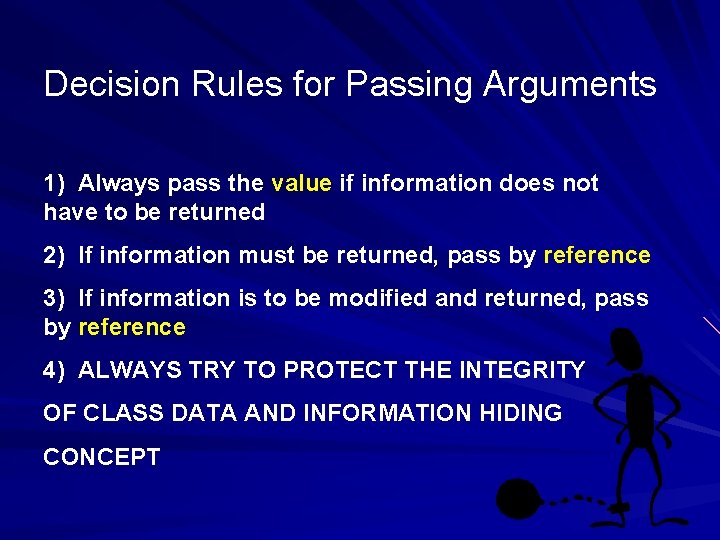
Decision Rules for Passing Arguments 1) Always pass the value if information does not have to be returned 2) If information must be returned, pass by reference 3) If information is to be modified and returned, pass by reference 4) ALWAYS TRY TO PROTECT THE INTEGRITY OF CLASS DATA AND INFORMATION HIDING CONCEPT
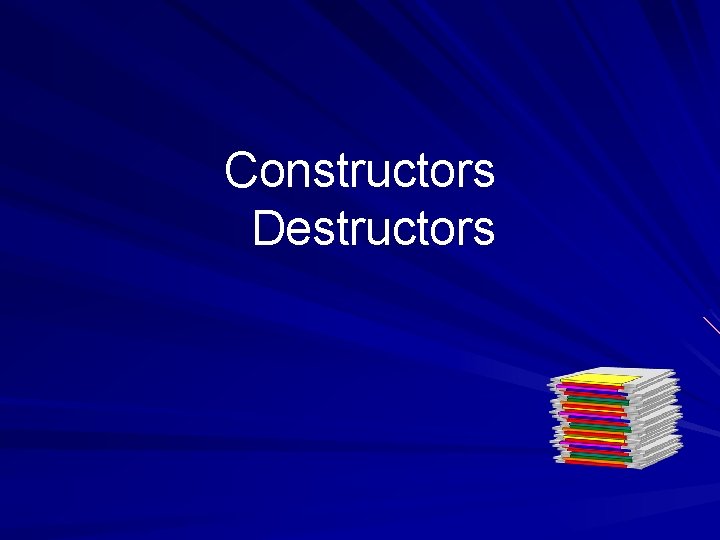
Constructors Destructors
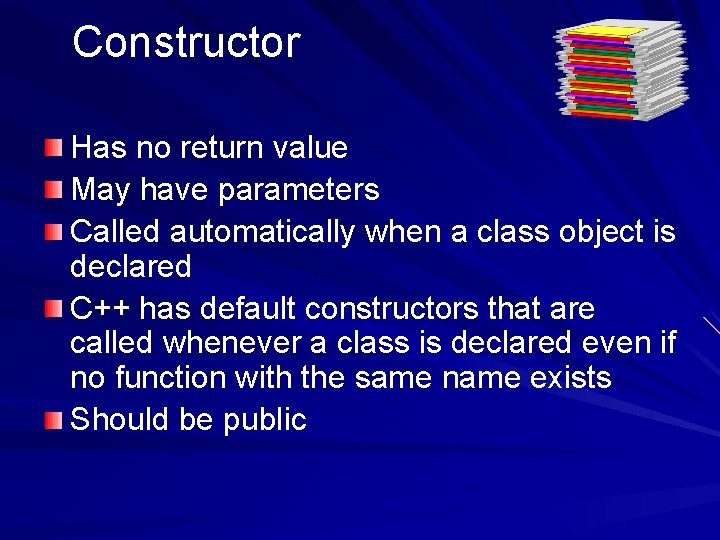
Constructor Has no return value May have parameters Called automatically when a class object is declared C++ has default constructors that are called whenever a class is declared even if no function with the same name exists Should be public
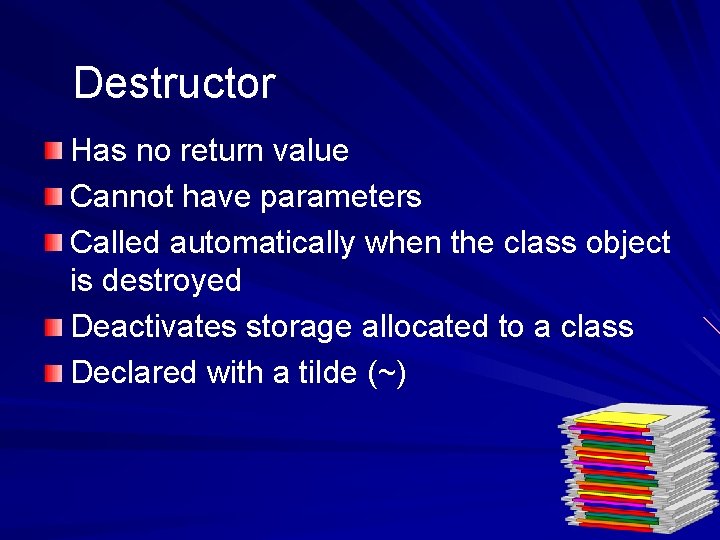
Destructor Has no return value Cannot have parameters Called automatically when the class object is destroyed Deactivates storage allocated to a class Declared with a tilde (~)
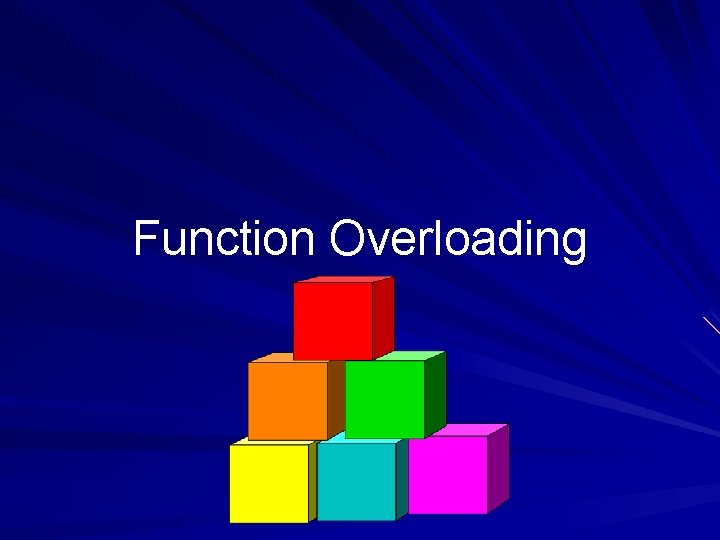
Function Overloading
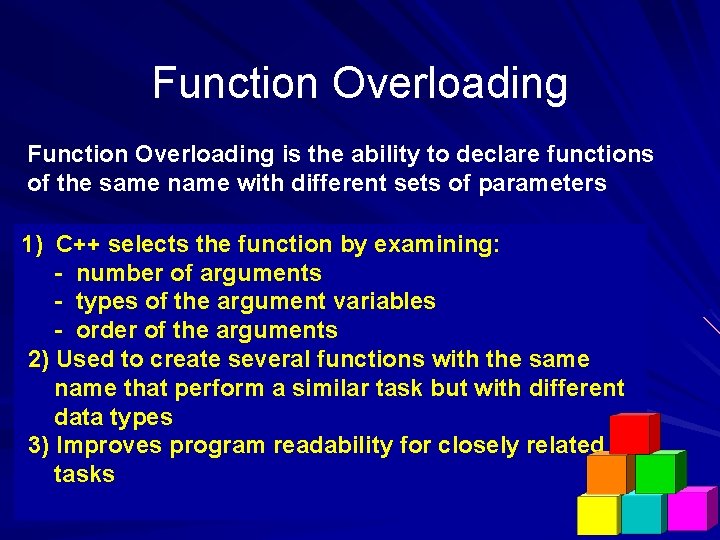
Function Overloading is the ability to declare functions of the same name with different sets of parameters 1) C++ selects the function by examining: - number of arguments - types of the argument variables - order of the arguments 2) Used to create several functions with the same name that perform a similar task but with different data types 3) Improves program readability for closely related tasks
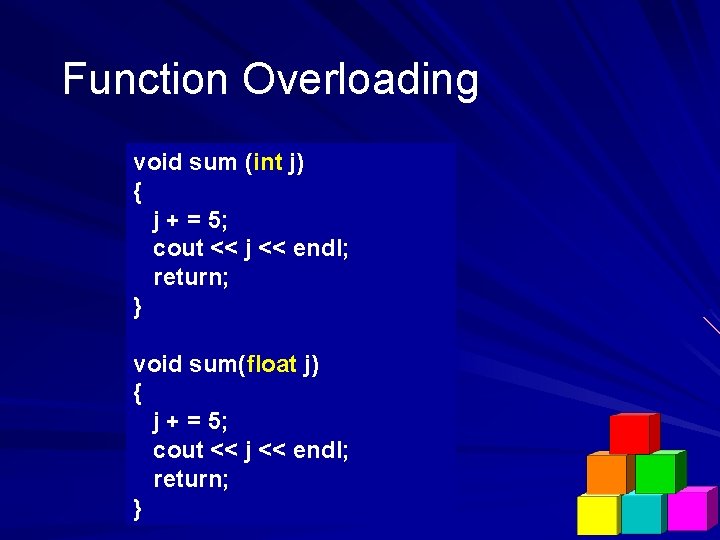
Function Overloading void sum (int j) { j + = 5; cout << j << endl; return; } void sum(float j) { j + = 5; cout << j << endl; return; }
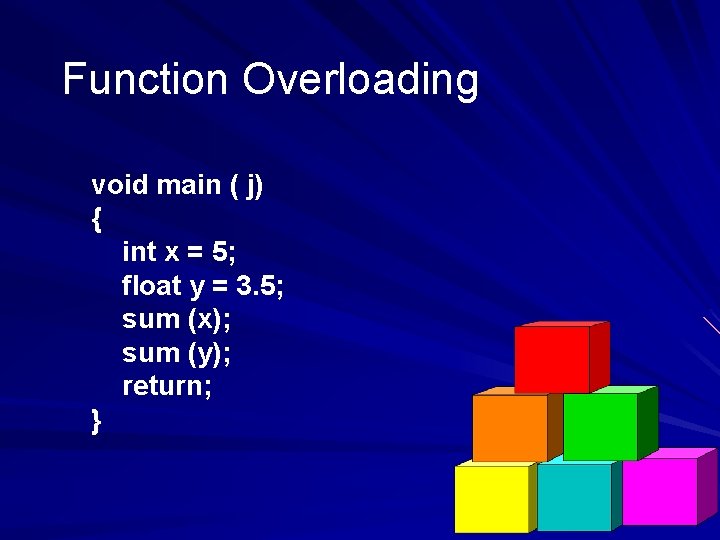
Function Overloading void main ( j) { int x = 5; float y = 3. 5; sum (x); sum (y); return; }
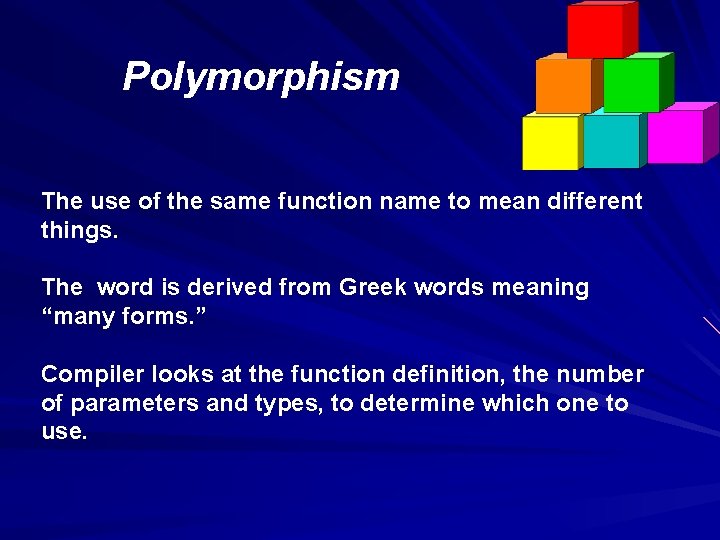
Polymorphism The use of the same function name to mean different things. The word is derived from Greek words meaning “many forms. ” Compiler looks at the function definition, the number of parameters and types, to determine which one to use.
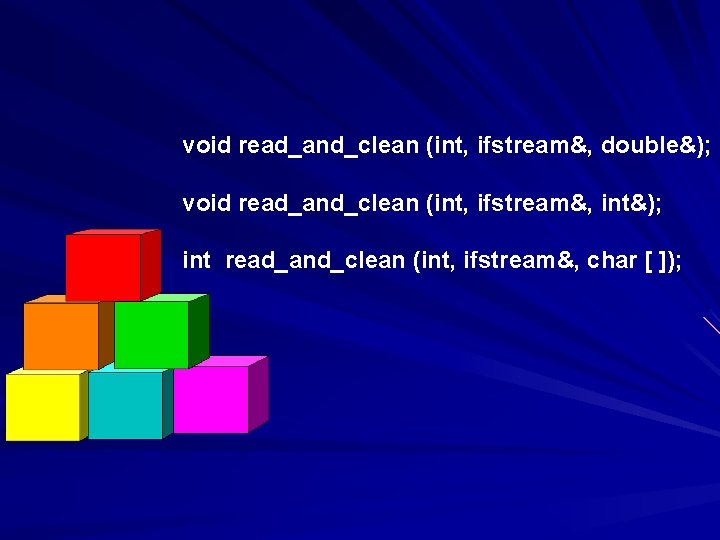
void read_and_clean (int, ifstream&, double&); void read_and_clean (int, ifstream&, int&); int read_and_clean (int, ifstream&, char [ ]);
Infsy
01:640:244 lecture notes - lecture 15: plat, idah, farad
Kim ki duk spring summer fall winter
Months for spring summer fall winter
Sebuah gerbong kereta api mempunyai massa 10.000 kg
Meris 440 review
15-440 cmu
15-440
Ensc 440
Basis risk arises due to
Spravac
25-3/440
Cos 440
Monitor/pimer
Xavier mail delivery robot
Area code 440 location
Ensc 440
Textilexpo
Fin 440
Bola dengan massa 0 440 kg yang bergerak ke timur
Polylite 440-m850
Section 17-4 patterns of evolution
Python parallel arrays
Mips arrays
Dynamic arrays and amortized analysis
Disadvantages of dynamic programming
Partially filled array java