Linked Lists INFSY 440 Spring 2004 Lecture 6
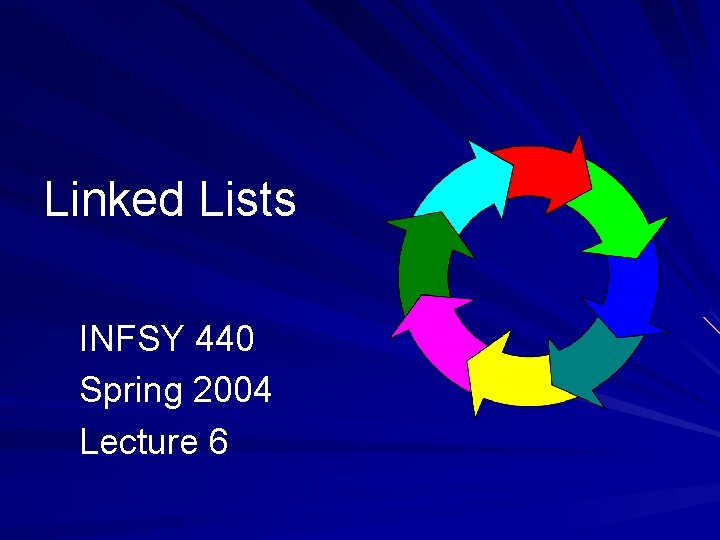
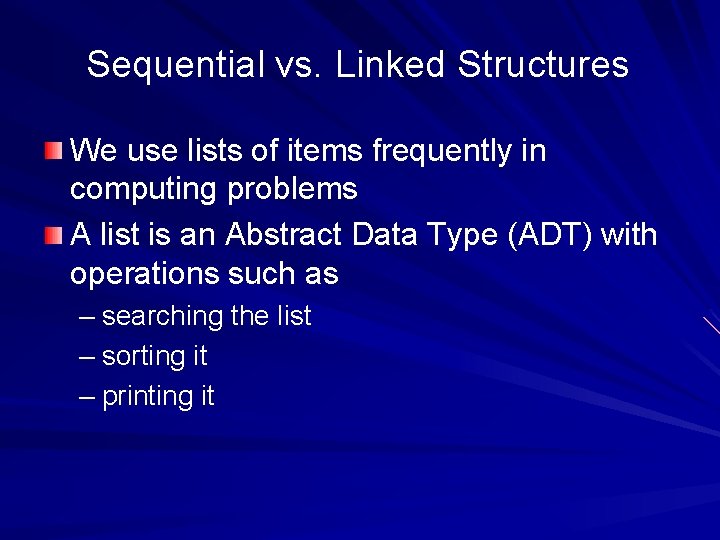
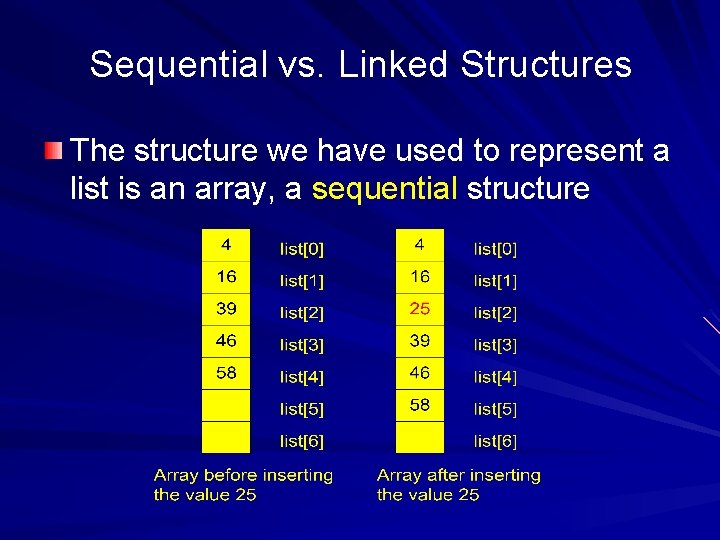
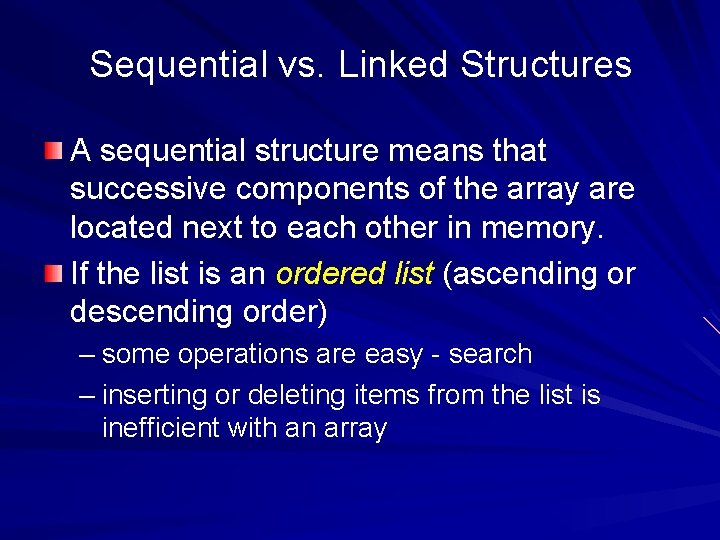
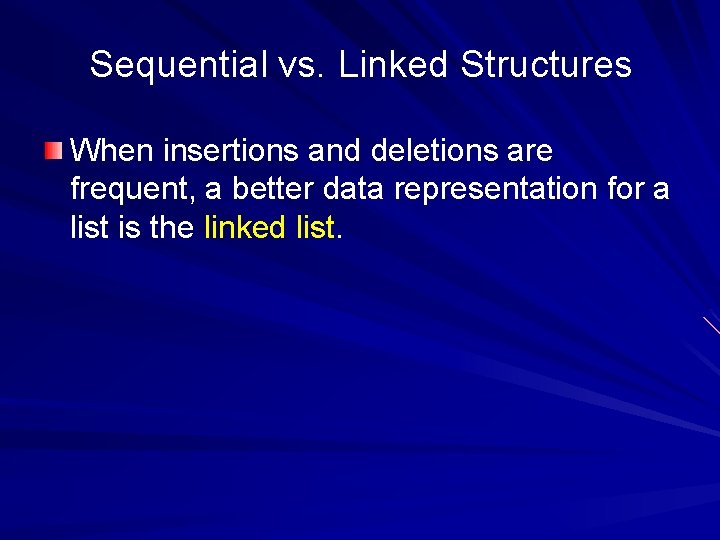
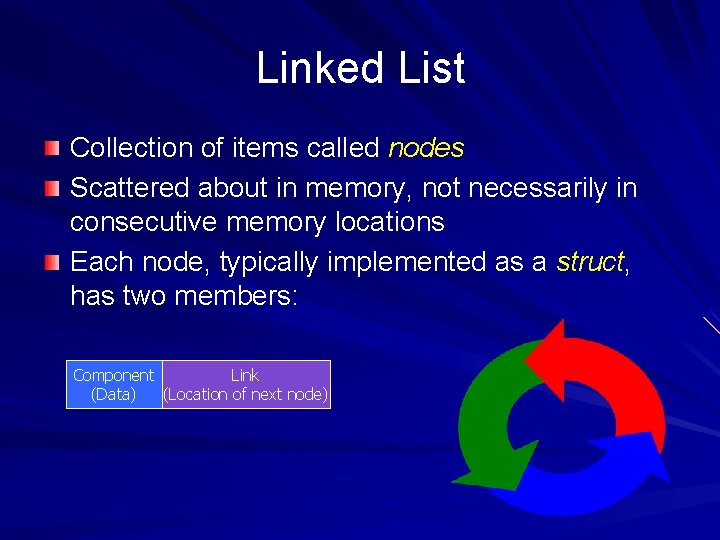
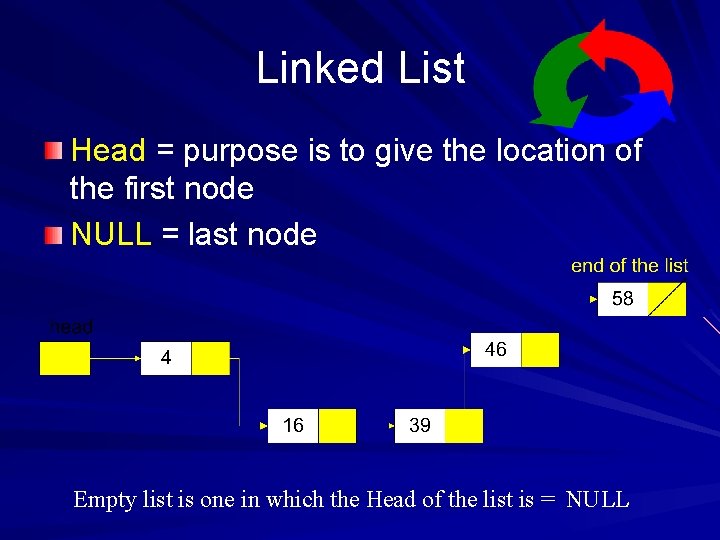
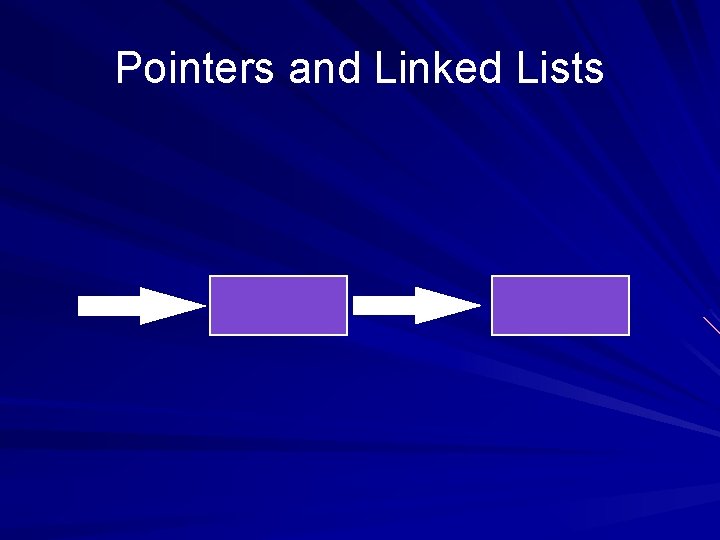
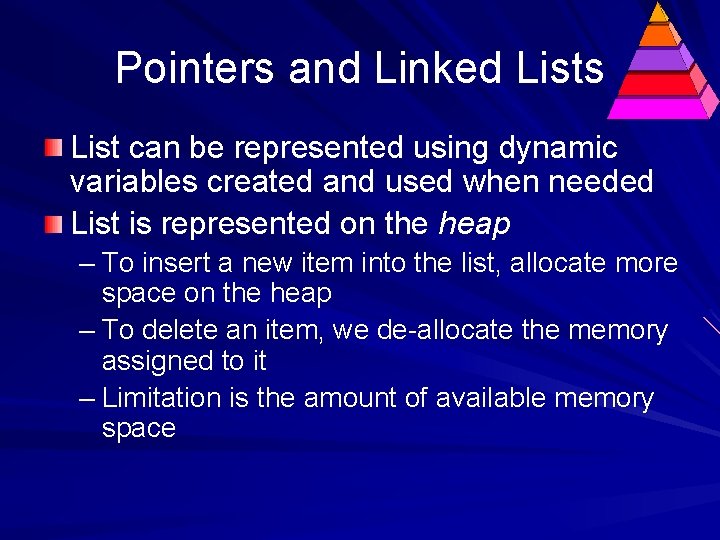
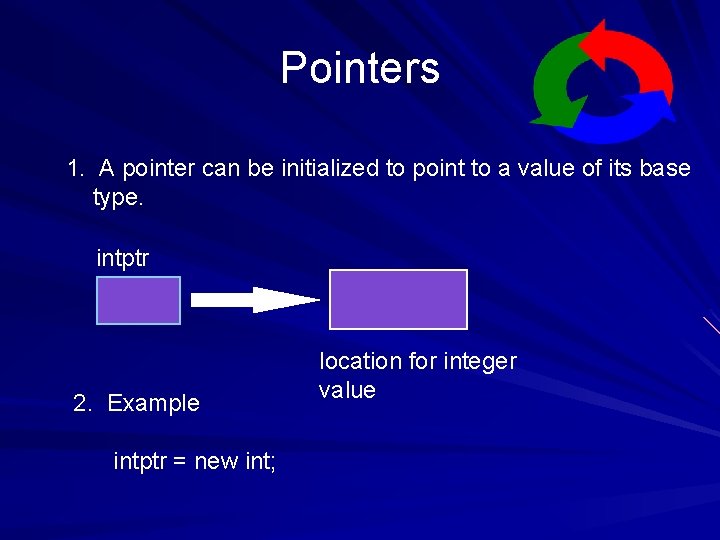
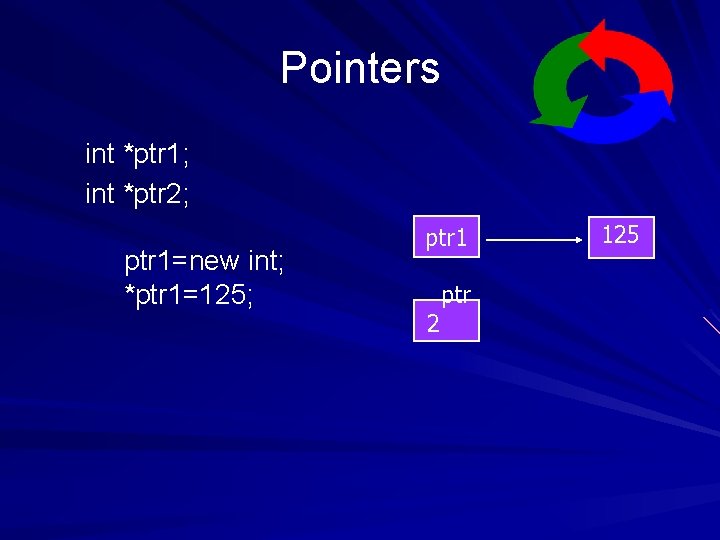
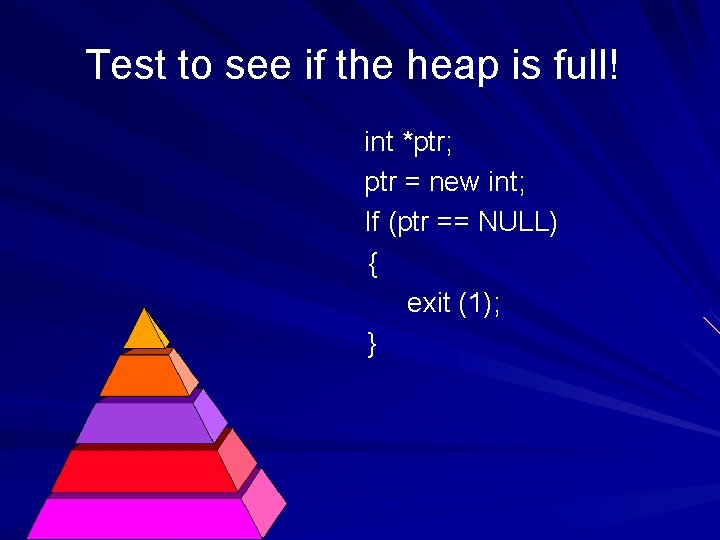
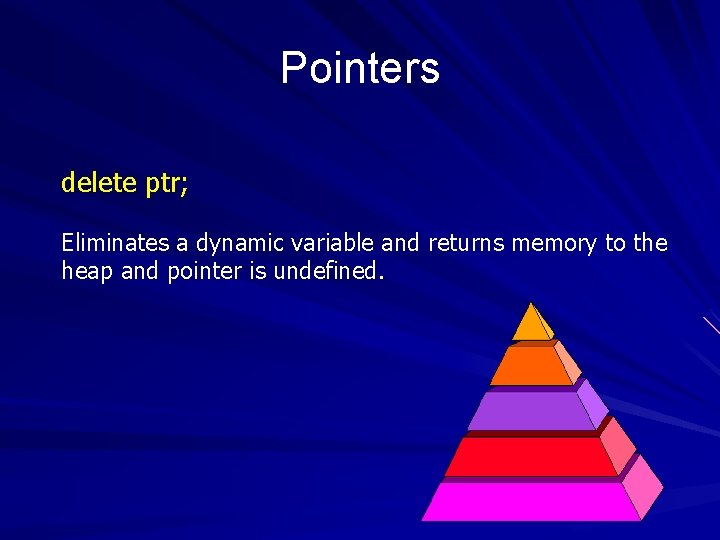
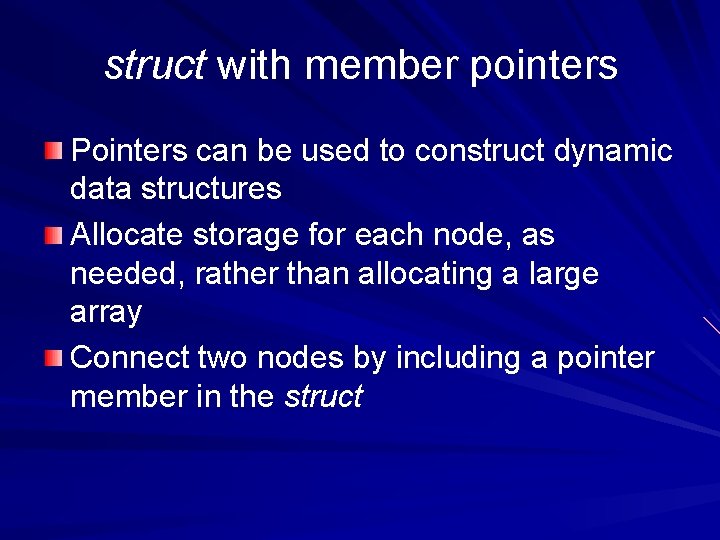
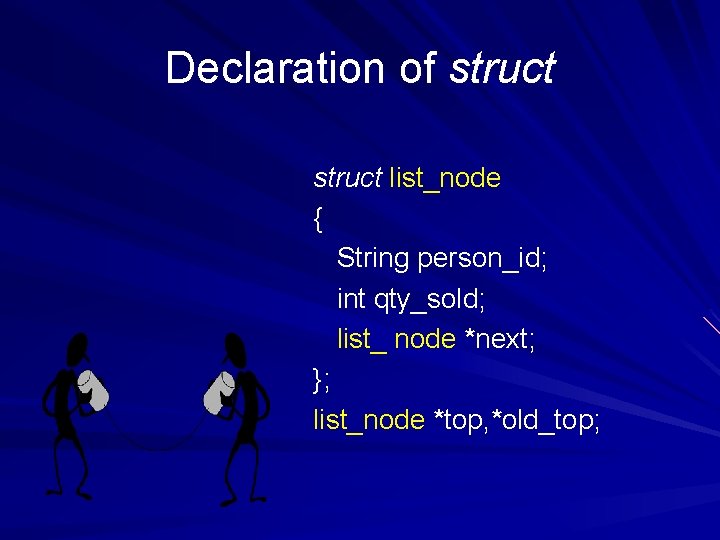
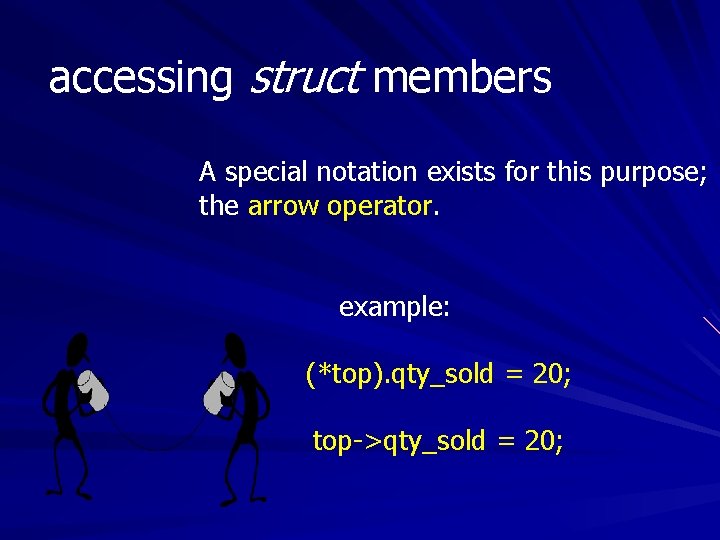
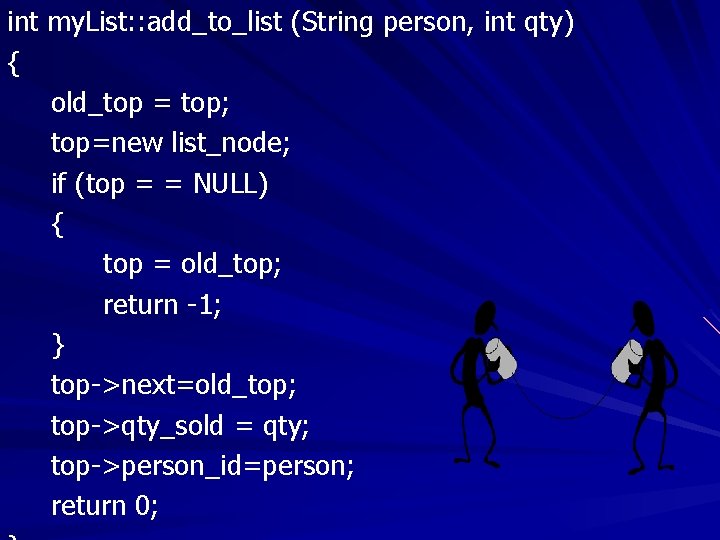
- Slides: 17
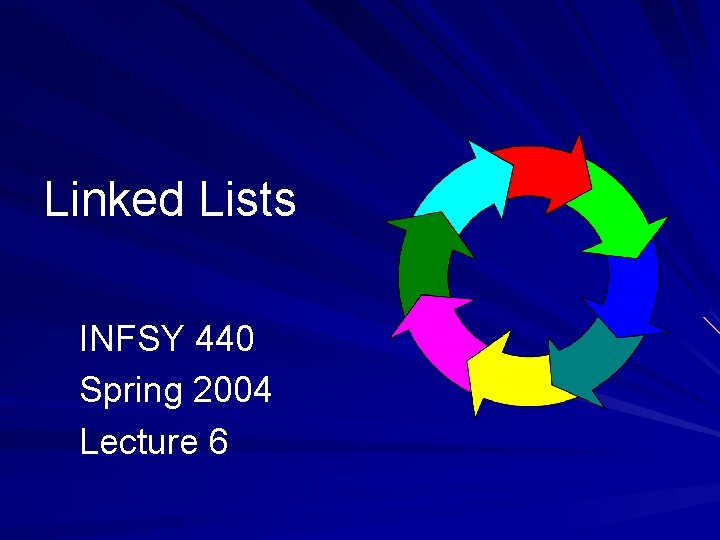
Linked Lists INFSY 440 Spring 2004 Lecture 6
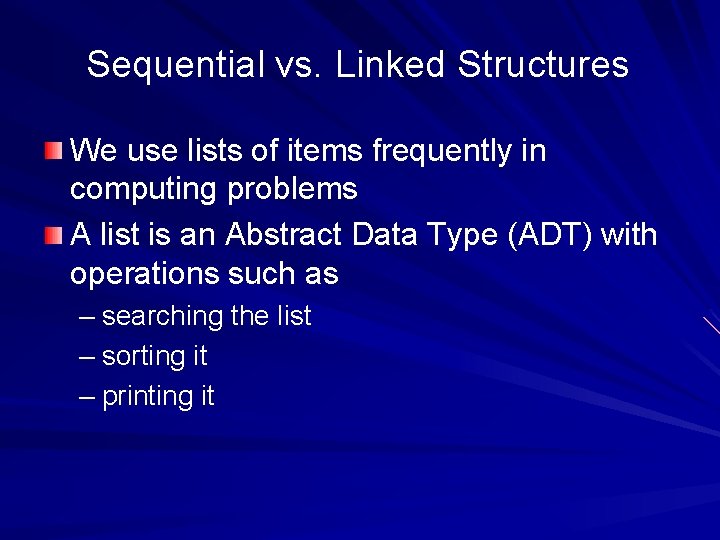
Sequential vs. Linked Structures We use lists of items frequently in computing problems A list is an Abstract Data Type (ADT) with operations such as – searching the list – sorting it – printing it
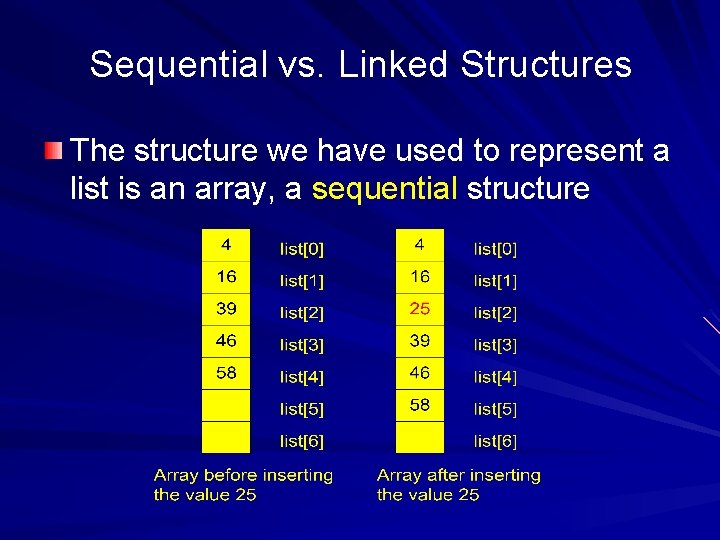
Sequential vs. Linked Structures The structure we have used to represent a list is an array, a sequential structure
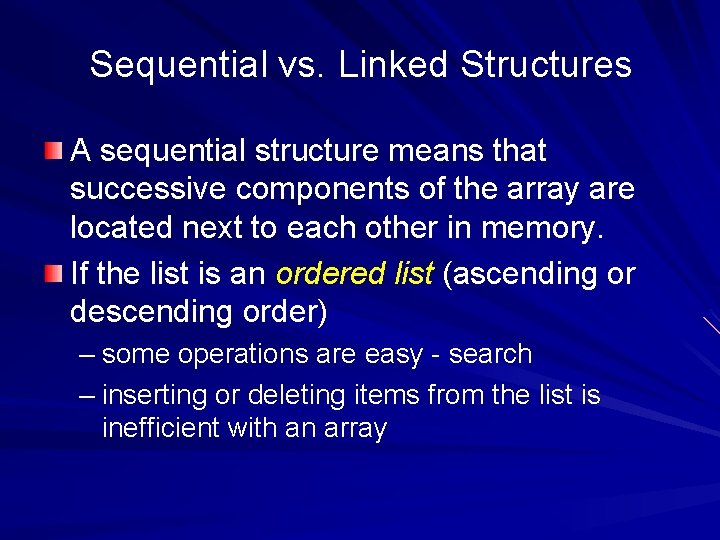
Sequential vs. Linked Structures A sequential structure means that successive components of the array are located next to each other in memory. If the list is an ordered list (ascending or descending order) – some operations are easy - search – inserting or deleting items from the list is inefficient with an array
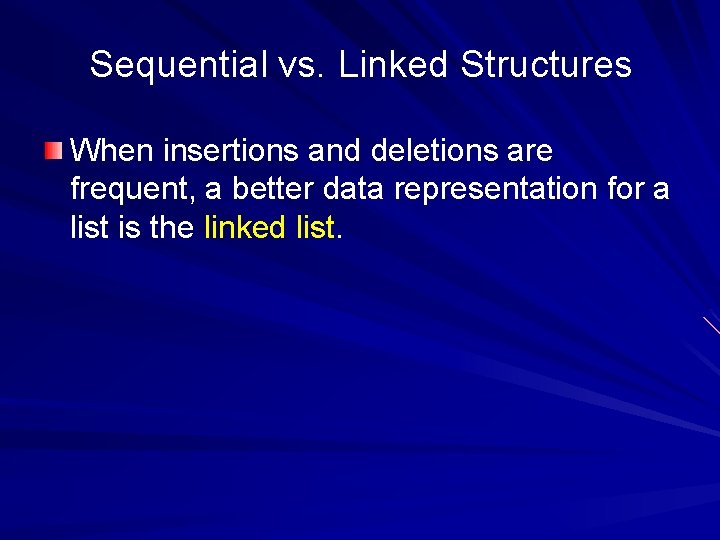
Sequential vs. Linked Structures When insertions and deletions are frequent, a better data representation for a list is the linked list.
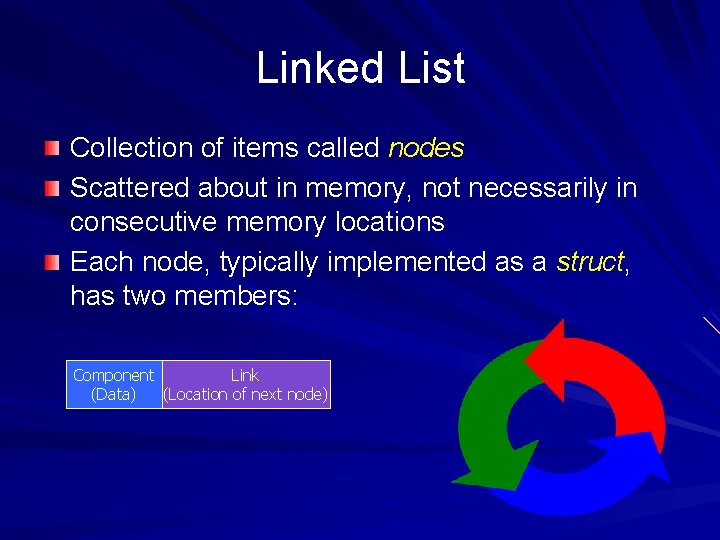
Linked List Collection of items called nodes Scattered about in memory, not necessarily in consecutive memory locations Each node, typically implemented as a struct, has two members: Component Link (Data) (Location of next node)
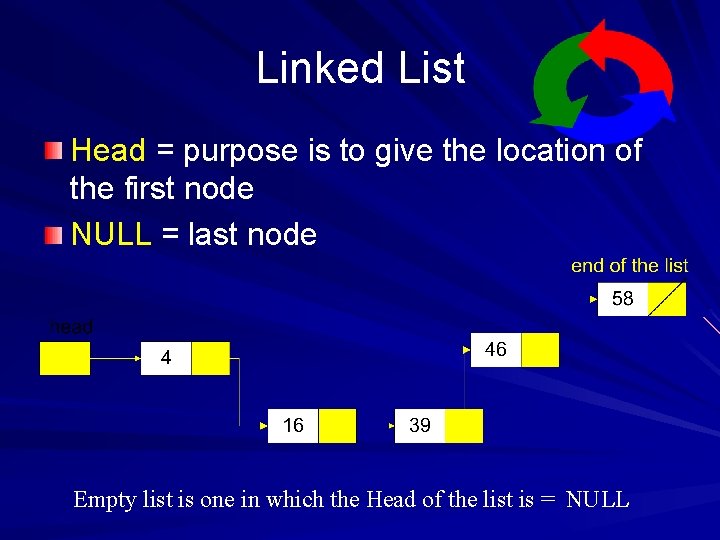
Linked List Head = purpose is to give the location of the first node NULL = last node Empty list is one in which the Head of the list is = NULL
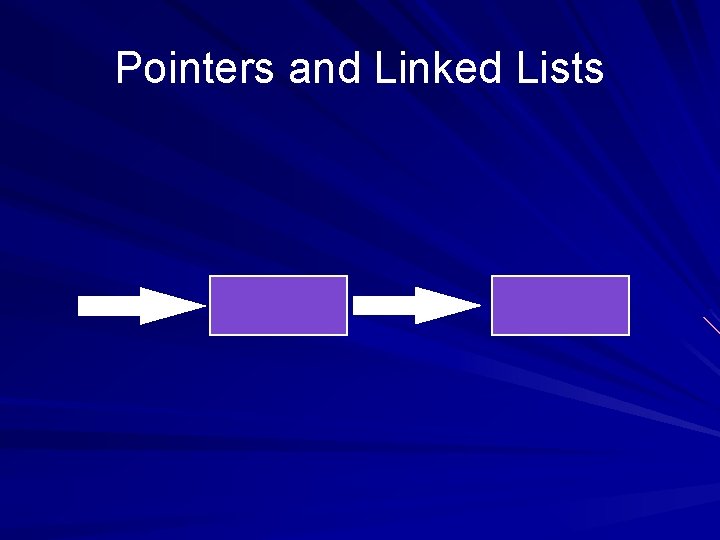
Pointers and Linked Lists
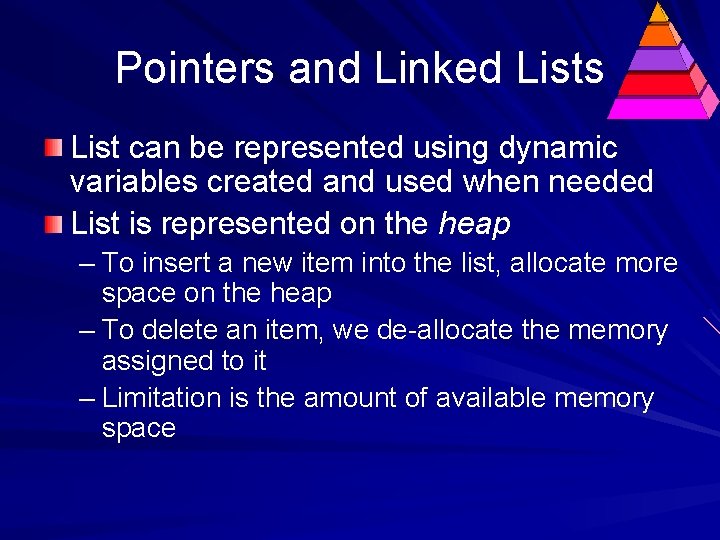
Pointers and Linked Lists List can be represented using dynamic variables created and used when needed List is represented on the heap – To insert a new item into the list, allocate more space on the heap – To delete an item, we de-allocate the memory assigned to it – Limitation is the amount of available memory space
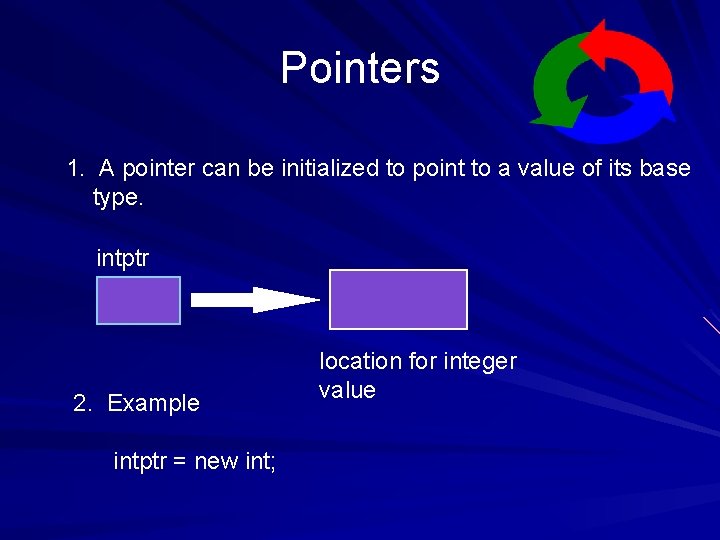
Pointers 1. A pointer can be initialized to point to a value of its base type. intptr 2. Example intptr = new int; location for integer value
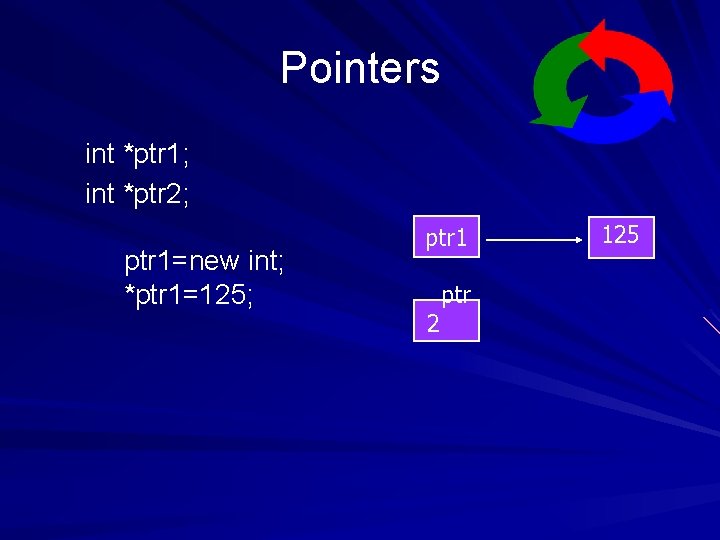
Pointers int *ptr 1; int *ptr 2; ptr 1=new int; *ptr 1=125; ptr 1 ptr 2 125
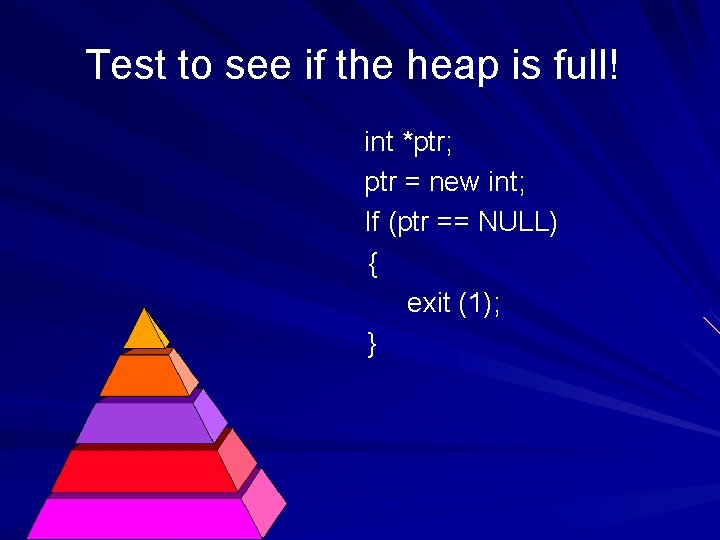
Test to see if the heap is full! int *ptr; ptr = new int; If (ptr == NULL) { exit (1); }
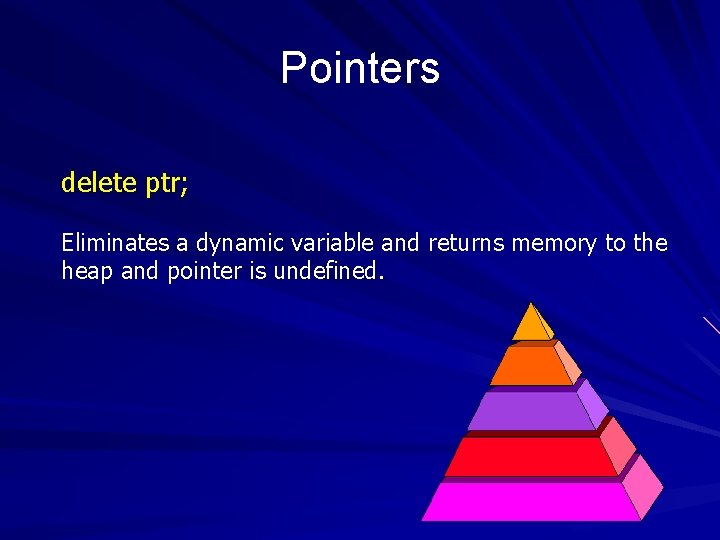
Pointers delete ptr; Eliminates a dynamic variable and returns memory to the heap and pointer is undefined.
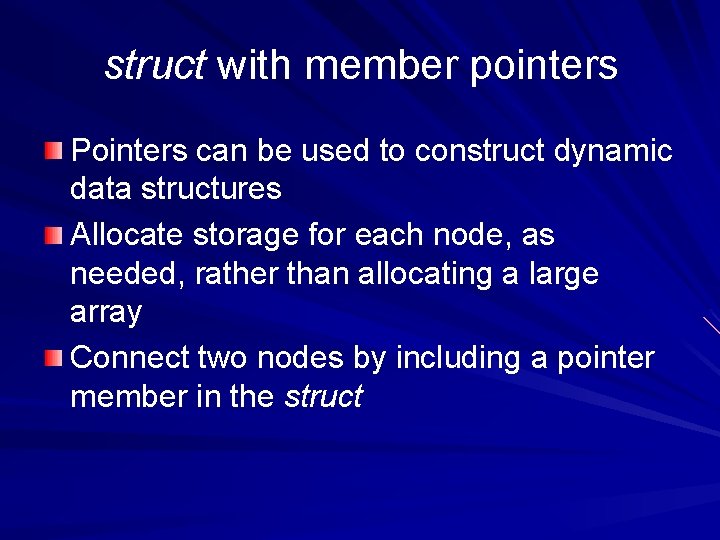
struct with member pointers Pointers can be used to construct dynamic data structures Allocate storage for each node, as needed, rather than allocating a large array Connect two nodes by including a pointer member in the struct
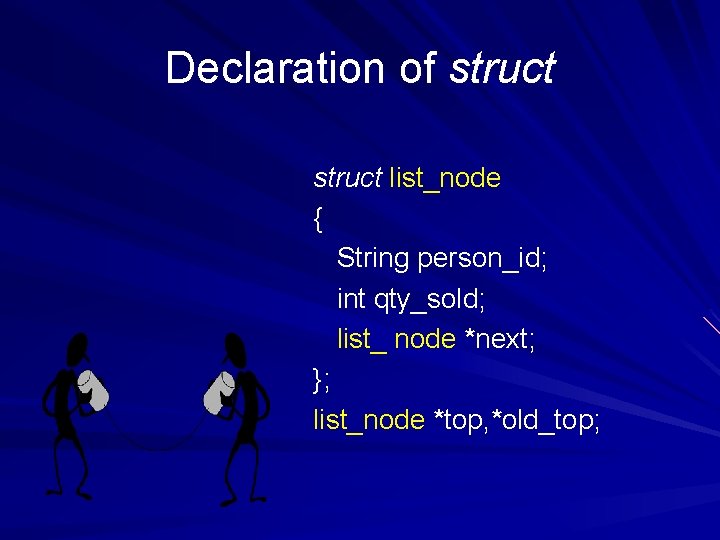
Declaration of struct list_node { String person_id; int qty_sold; list_ node *next; }; list_node *top, *old_top;
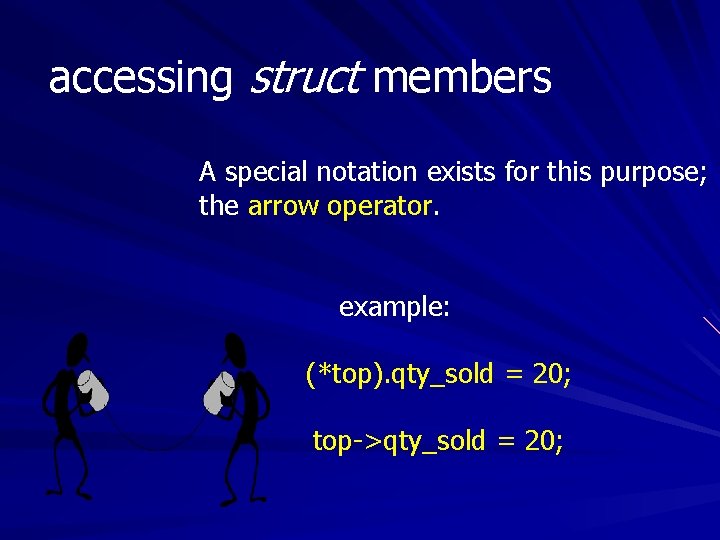
accessing struct members A special notation exists for this purpose; the arrow operator. example: (*top). qty_sold = 20; top->qty_sold = 20;
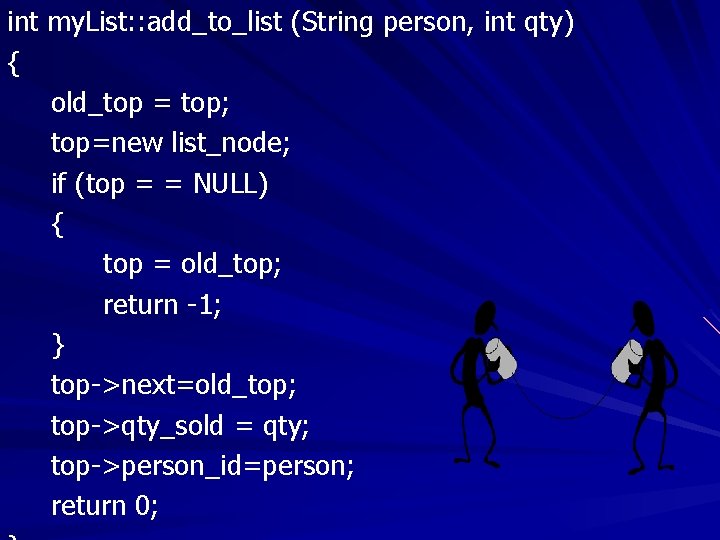
int my. List: : add_to_list (String person, int qty) { old_top = top; top=new list_node; if (top = = NULL) { top = old_top; return -1; } top->next=old_top; top->qty_sold = qty; top->person_id=person; return 0;
Dr ocker
Perbedaan single linked list dan double linked list
Singly vs doubly linked list
Introduction to linked list
01:640:244 lecture notes - lecture 15: plat, idah, farad
Fall season months
Spring, summer, fall, winter... and spring (2003)
Spravac
Cos 440
Piattaforma pimer monitor
Bola dengan massa 0 440 kg yang bergerak ke timur
Xavier mail delivery robot
Area code 440 location
Ensc 440
Xenotest 440
Fin 440
15-440 cmu
Polylite 440-m850