Android Data Storage Shared Preferences Shared Preferences Android
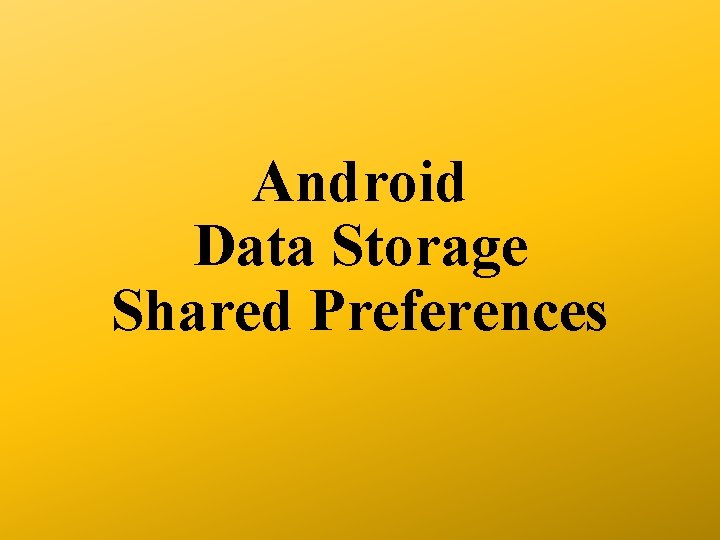
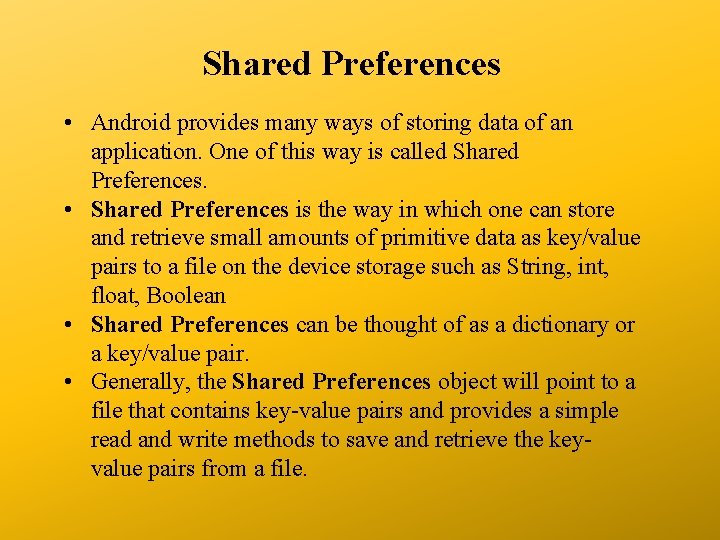
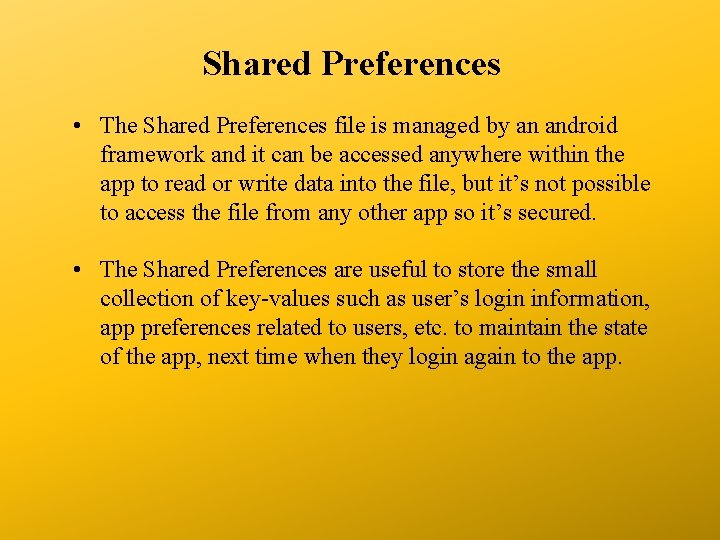
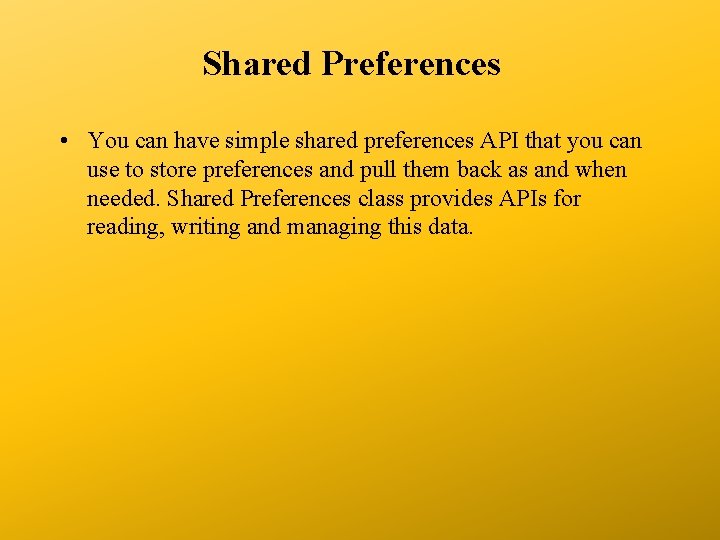
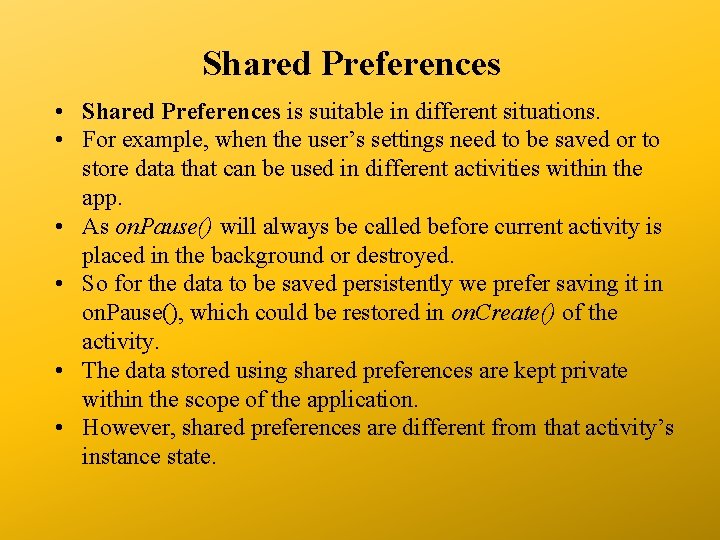
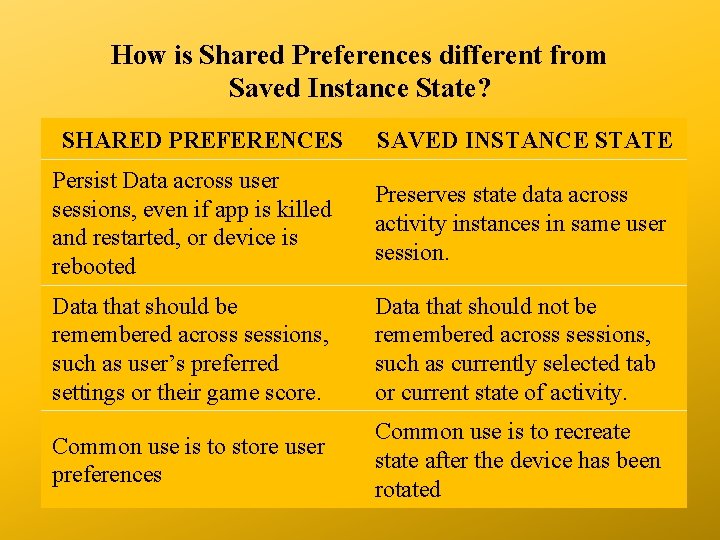
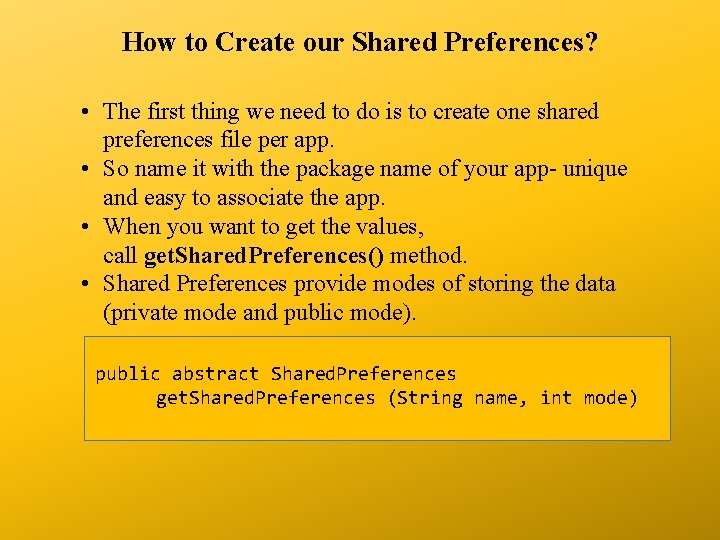
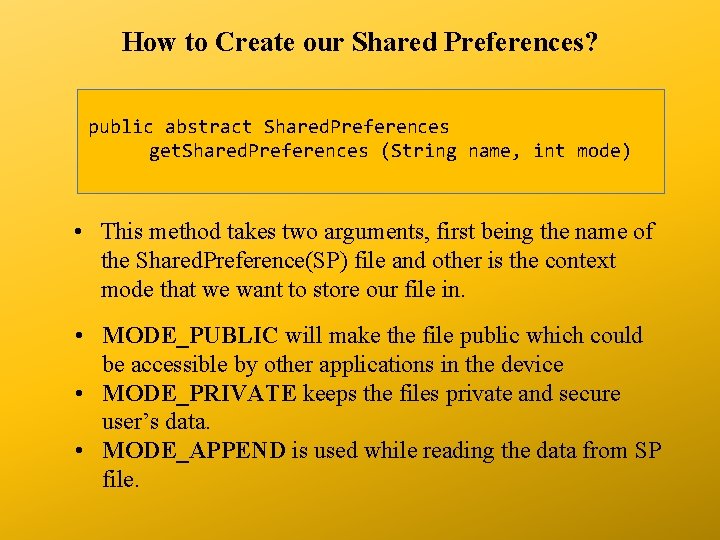
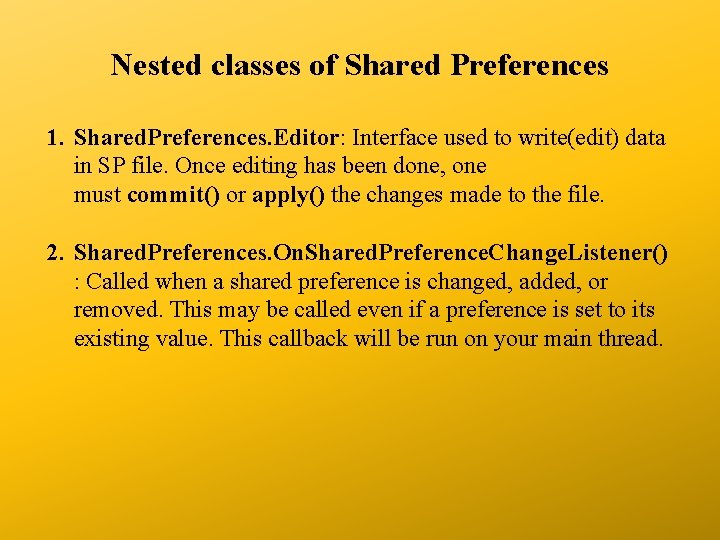
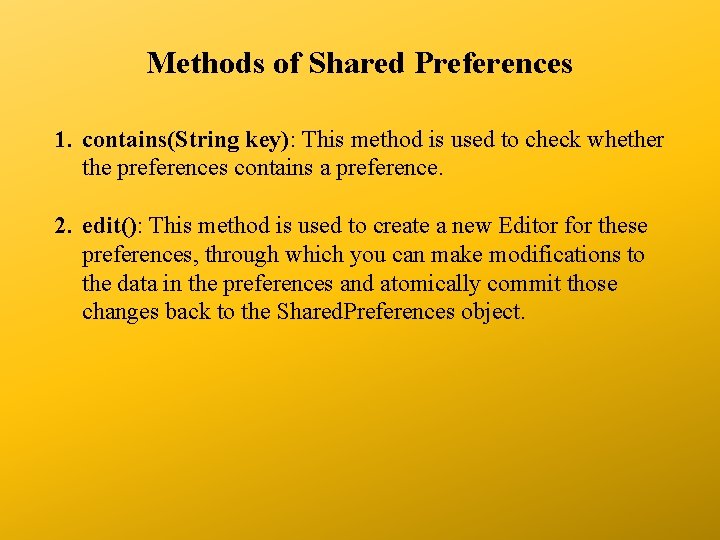
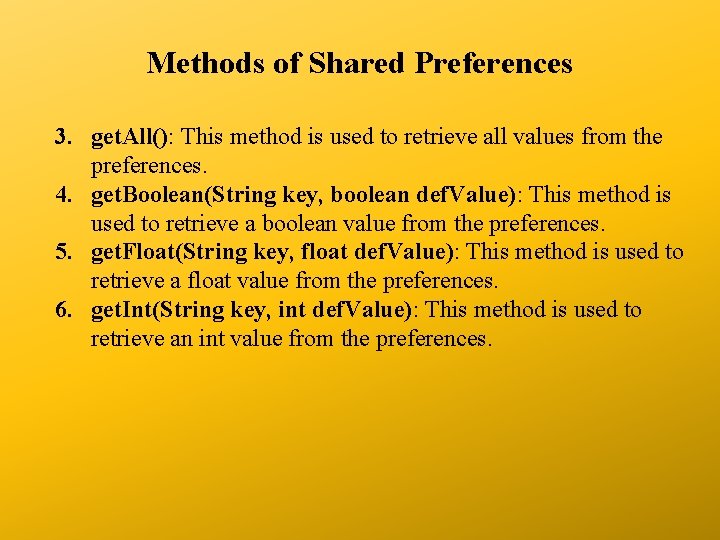
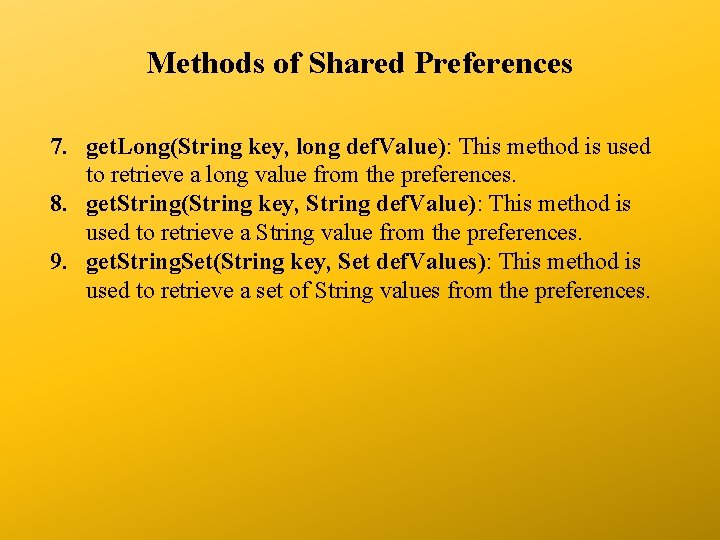
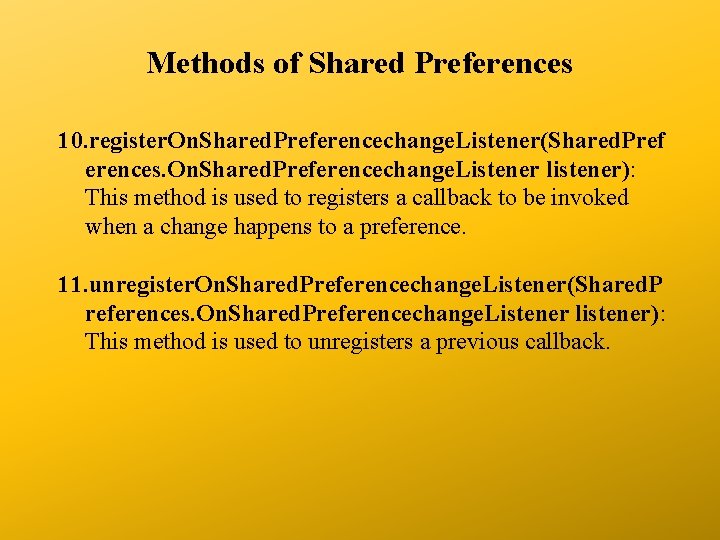
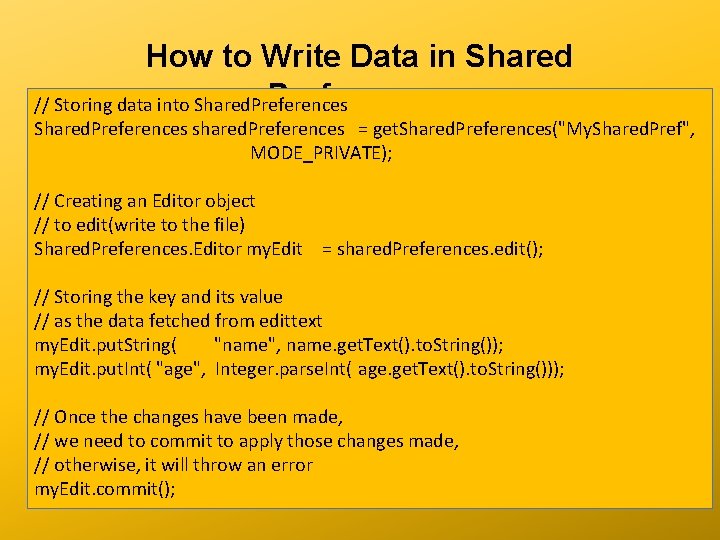
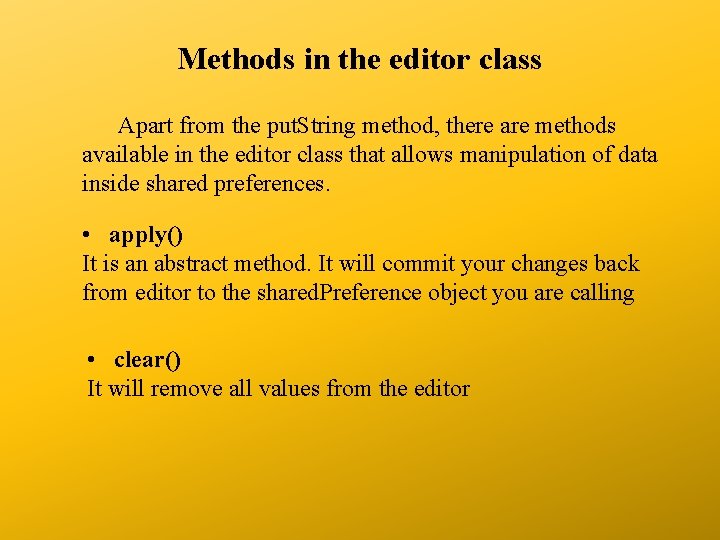
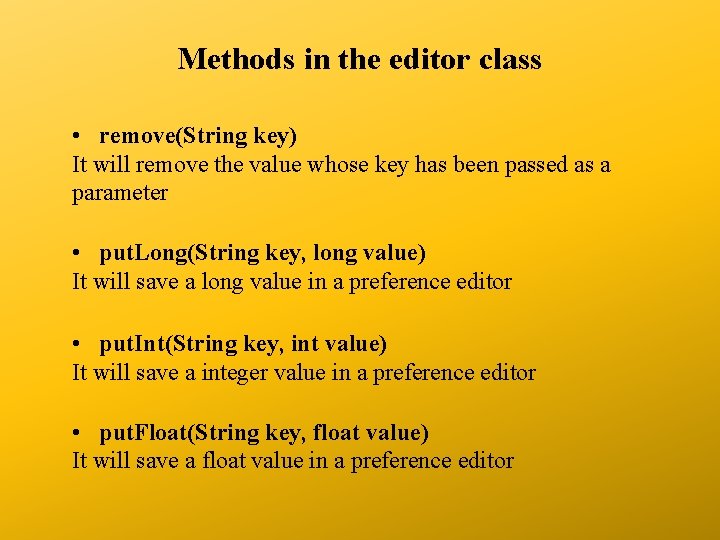
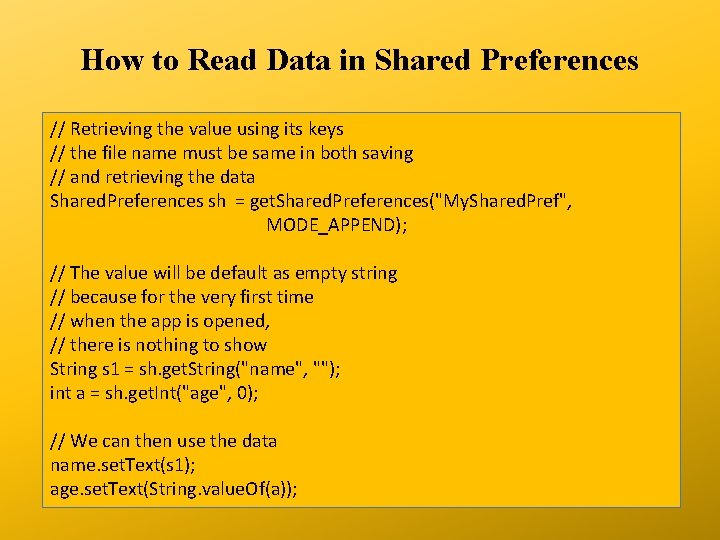
- Slides: 17
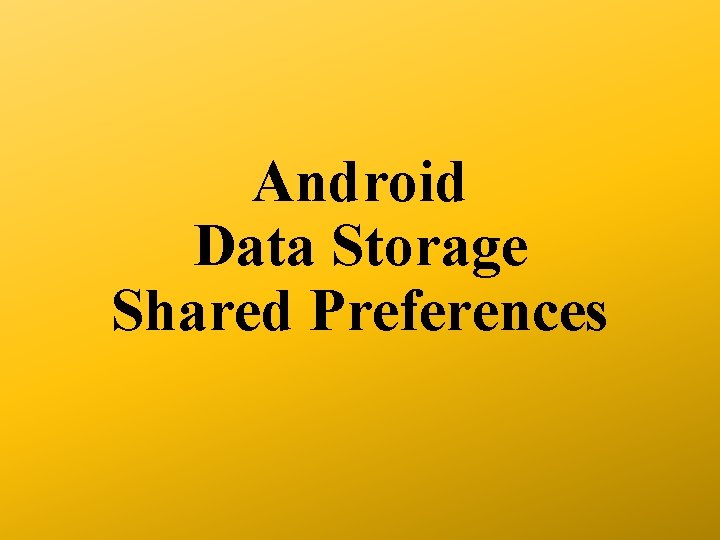
Android Data Storage Shared Preferences
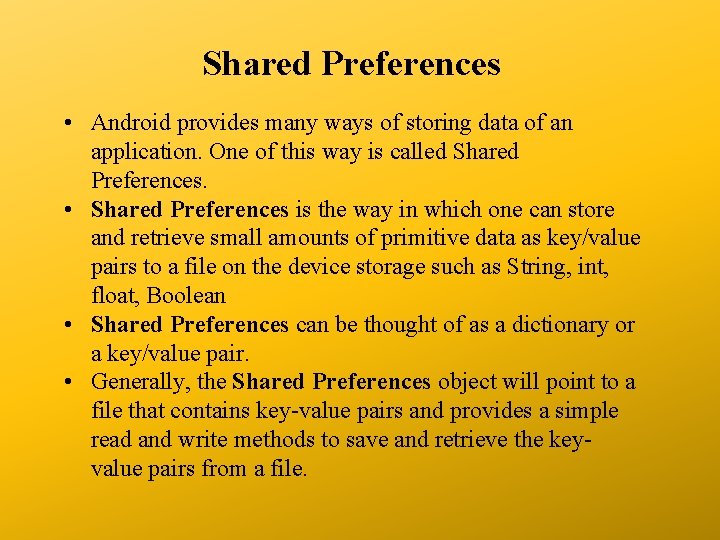
Shared Preferences • Android provides many ways of storing data of an application. One of this way is called Shared Preferences. • Shared Preferences is the way in which one can store and retrieve small amounts of primitive data as key/value pairs to a file on the device storage such as String, int, float, Boolean • Shared Preferences can be thought of as a dictionary or a key/value pair. • Generally, the Shared Preferences object will point to a file that contains key-value pairs and provides a simple read and write methods to save and retrieve the keyvalue pairs from a file.
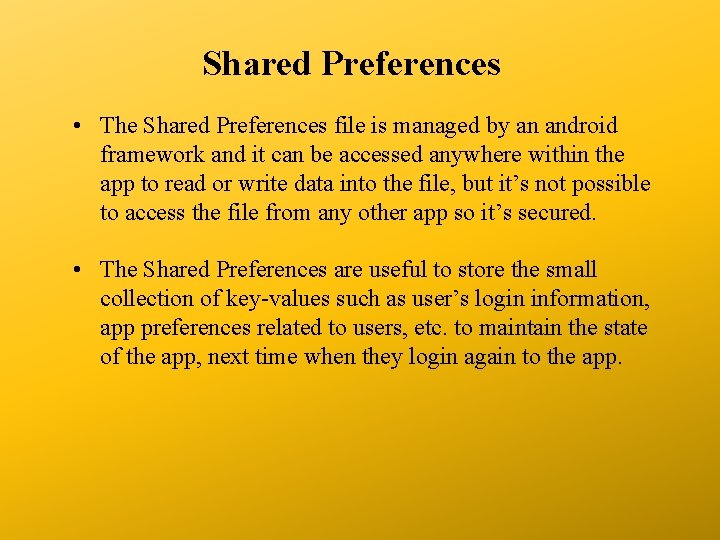
Shared Preferences • The Shared Preferences file is managed by an android framework and it can be accessed anywhere within the app to read or write data into the file, but it’s not possible to access the file from any other app so it’s secured. • The Shared Preferences are useful to store the small collection of key-values such as user’s login information, app preferences related to users, etc. to maintain the state of the app, next time when they login again to the app.
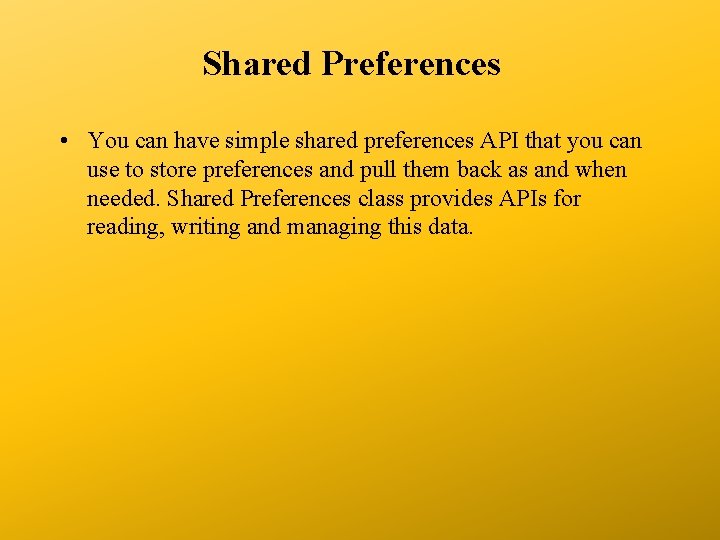
Shared Preferences • You can have simple shared preferences API that you can use to store preferences and pull them back as and when needed. Shared Preferences class provides APIs for reading, writing and managing this data.
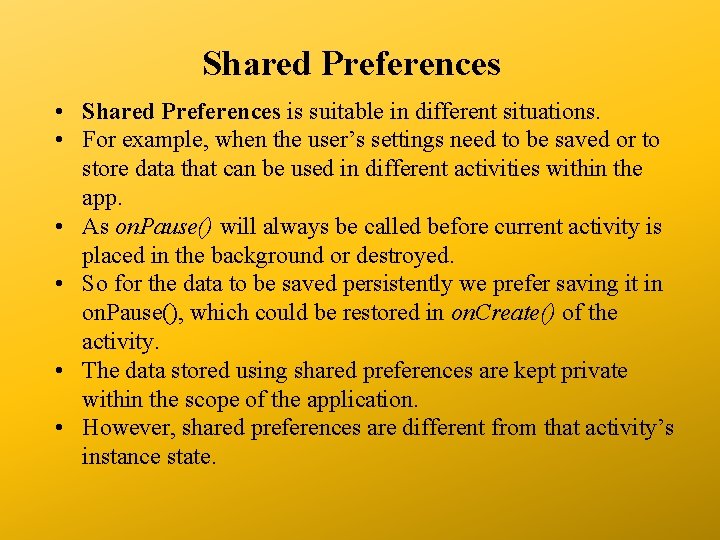
Shared Preferences • Shared Preferences is suitable in different situations. • For example, when the user’s settings need to be saved or to store data that can be used in different activities within the app. • As on. Pause() will always be called before current activity is placed in the background or destroyed. • So for the data to be saved persistently we prefer saving it in on. Pause(), which could be restored in on. Create() of the activity. • The data stored using shared preferences are kept private within the scope of the application. • However, shared preferences are different from that activity’s instance state.
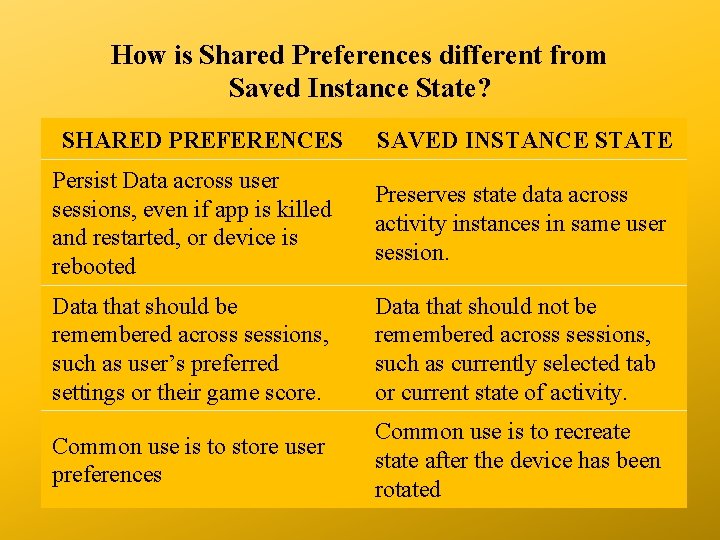
How is Shared Preferences different from Saved Instance State? SHARED PREFERENCES SAVED INSTANCE STATE Persist Data across user sessions, even if app is killed and restarted, or device is rebooted Preserves state data across activity instances in same user session. Data that should be remembered across sessions, such as user’s preferred settings or their game score. Data that should not be remembered across sessions, such as currently selected tab or current state of activity. Common use is to store user preferences Common use is to recreate state after the device has been rotated
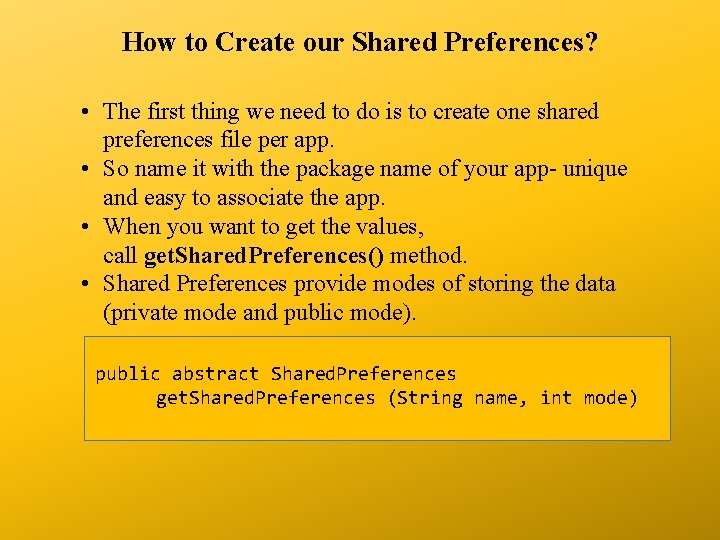
How to Create our Shared Preferences? • The first thing we need to do is to create one shared preferences file per app. • So name it with the package name of your app- unique and easy to associate the app. • When you want to get the values, call get. Shared. Preferences() method. • Shared Preferences provide modes of storing the data (private mode and public mode). public abstract Shared. Preferences get. Shared. Preferences (String name, int mode)
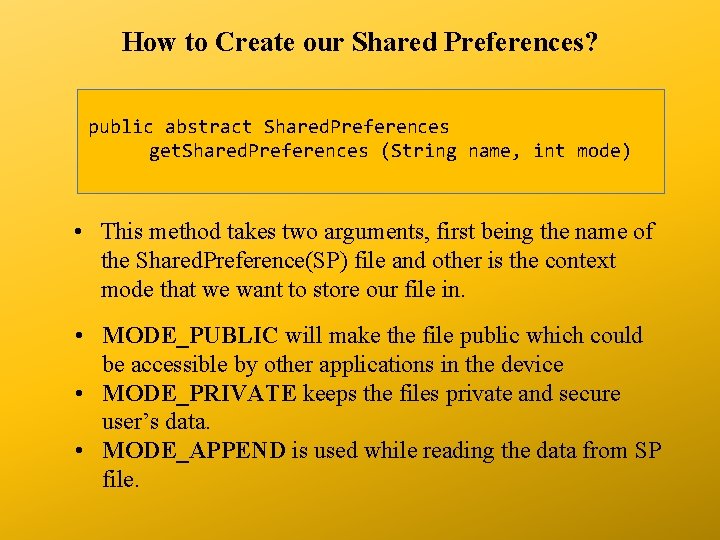
How to Create our Shared Preferences? public abstract Shared. Preferences get. Shared. Preferences (String name, int mode) • This method takes two arguments, first being the name of the Shared. Preference(SP) file and other is the context mode that we want to store our file in. • MODE_PUBLIC will make the file public which could be accessible by other applications in the device • MODE_PRIVATE keeps the files private and secure user’s data. • MODE_APPEND is used while reading the data from SP file.
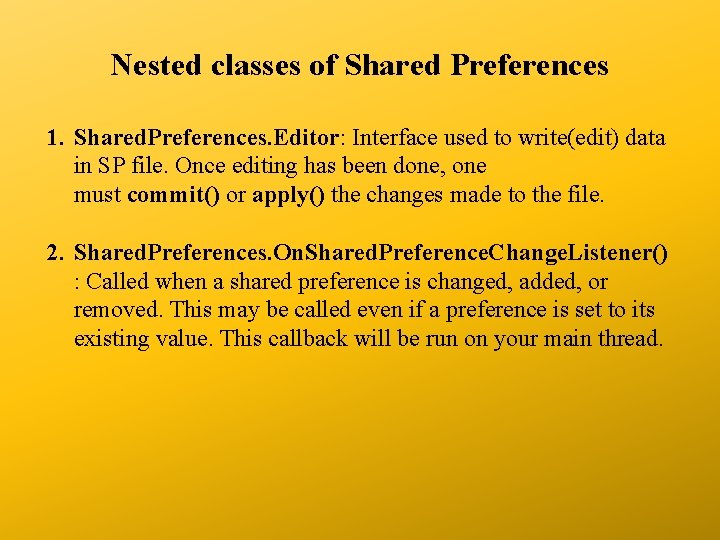
Nested classes of Shared Preferences 1. Shared. Preferences. Editor: Interface used to write(edit) data in SP file. Once editing has been done, one must commit() or apply() the changes made to the file. 2. Shared. Preferences. On. Shared. Preference. Change. Listener() : Called when a shared preference is changed, added, or removed. This may be called even if a preference is set to its existing value. This callback will be run on your main thread.
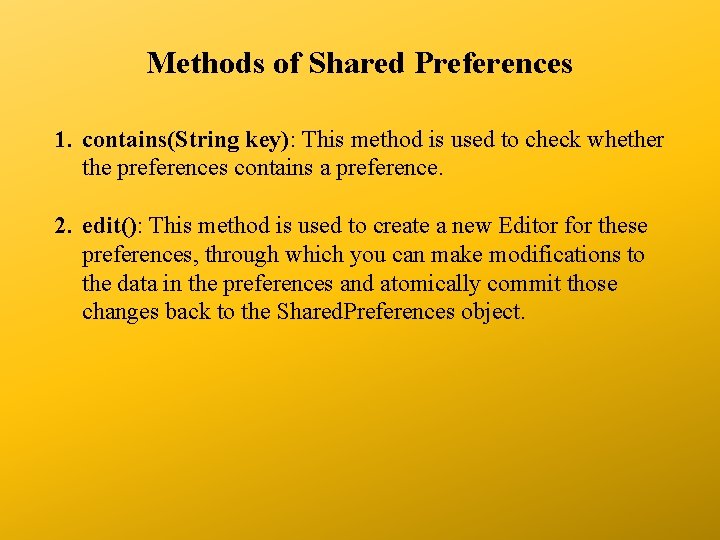
Methods of Shared Preferences 1. contains(String key): This method is used to check whether the preferences contains a preference. 2. edit(): This method is used to create a new Editor for these preferences, through which you can make modifications to the data in the preferences and atomically commit those changes back to the Shared. Preferences object.
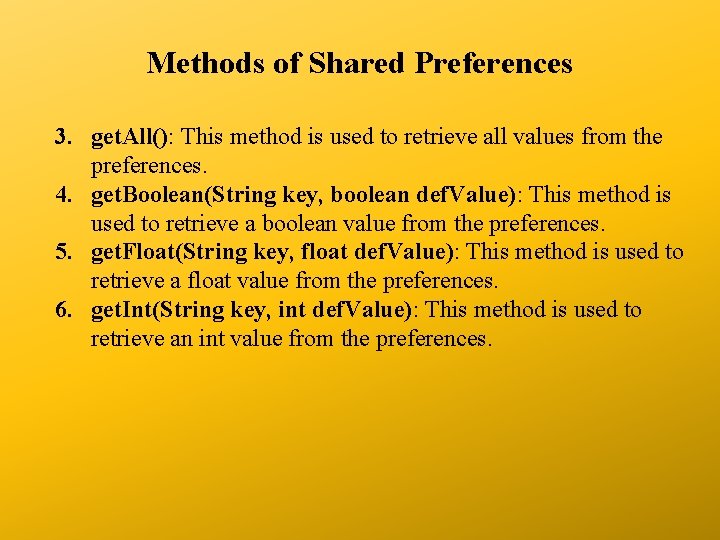
Methods of Shared Preferences 3. get. All(): This method is used to retrieve all values from the preferences. 4. get. Boolean(String key, boolean def. Value): This method is used to retrieve a boolean value from the preferences. 5. get. Float(String key, float def. Value): This method is used to retrieve a float value from the preferences. 6. get. Int(String key, int def. Value): This method is used to retrieve an int value from the preferences.
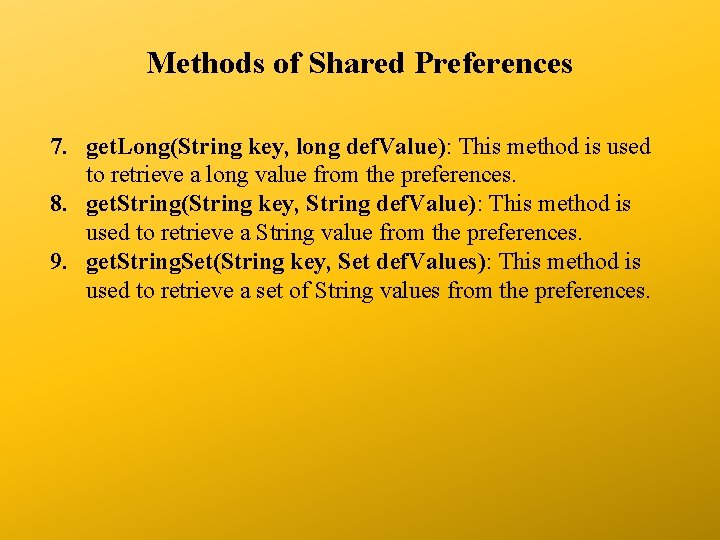
Methods of Shared Preferences 7. get. Long(String key, long def. Value): This method is used to retrieve a long value from the preferences. 8. get. String(String key, String def. Value): This method is used to retrieve a String value from the preferences. 9. get. String. Set(String key, Set def. Values): This method is used to retrieve a set of String values from the preferences.
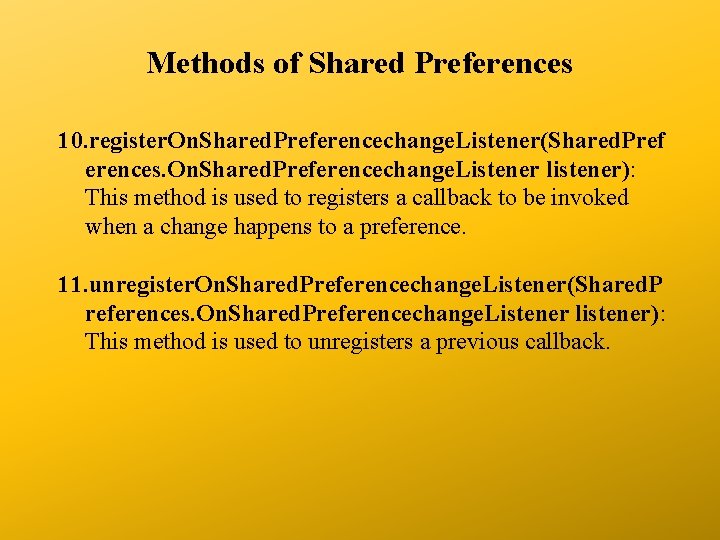
Methods of Shared Preferences 10. register. On. Shared. Preferencechange. Listener(Shared. Pref erences. On. Shared. Preferencechange. Listener listener): This method is used to registers a callback to be invoked when a change happens to a preference. 11. unregister. On. Shared. Preferencechange. Listener(Shared. P references. On. Shared. Preferencechange. Listener listener): This method is used to unregisters a previous callback.
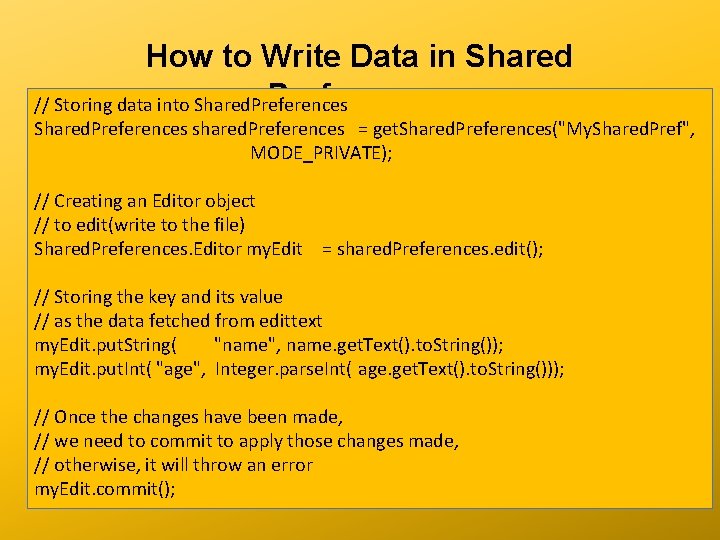
How to Write Data in Shared Preferences // Storing data into Shared. Preferences shared. Preferences = get. Shared. Preferences("My. Shared. Pref", MODE_PRIVATE); // Creating an Editor object // to edit(write to the file) Shared. Preferences. Editor my. Edit = shared. Preferences. edit(); // Storing the key and its value // as the data fetched from edittext my. Edit. put. String( "name", name. get. Text(). to. String()); my. Edit. put. Int( "age", Integer. parse. Int( age. get. Text(). to. String())); // Once the changes have been made, // we need to commit to apply those changes made, // otherwise, it will throw an error my. Edit. commit();
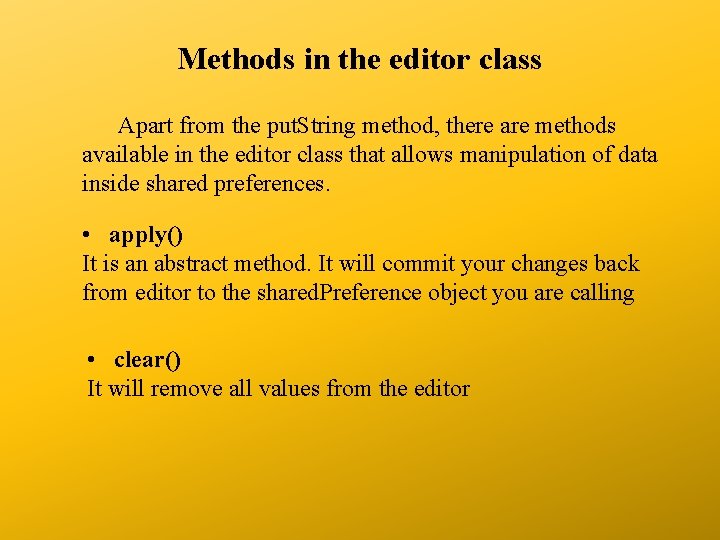
Methods in the editor class Apart from the put. String method, there are methods available in the editor class that allows manipulation of data inside shared preferences. • apply() It is an abstract method. It will commit your changes back from editor to the shared. Preference object you are calling • clear() It will remove all values from the editor
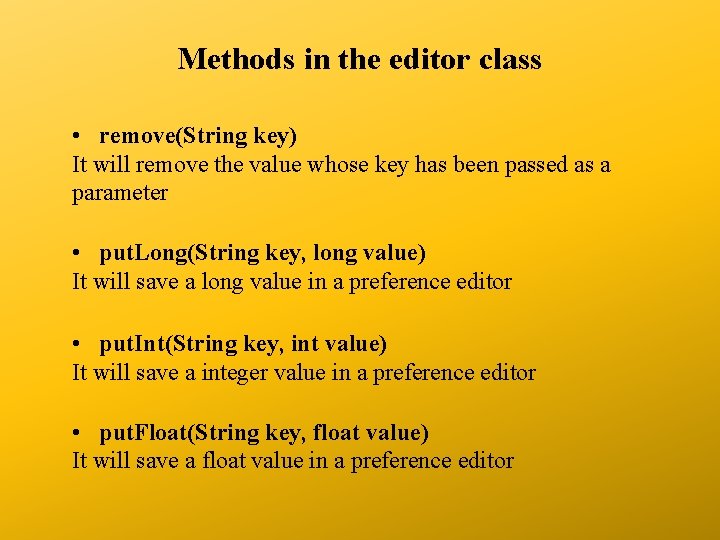
Methods in the editor class • remove(String key) It will remove the value whose key has been passed as a parameter • put. Long(String key, long value) It will save a long value in a preference editor • put. Int(String key, int value) It will save a integer value in a preference editor • put. Float(String key, float value) It will save a float value in a preference editor
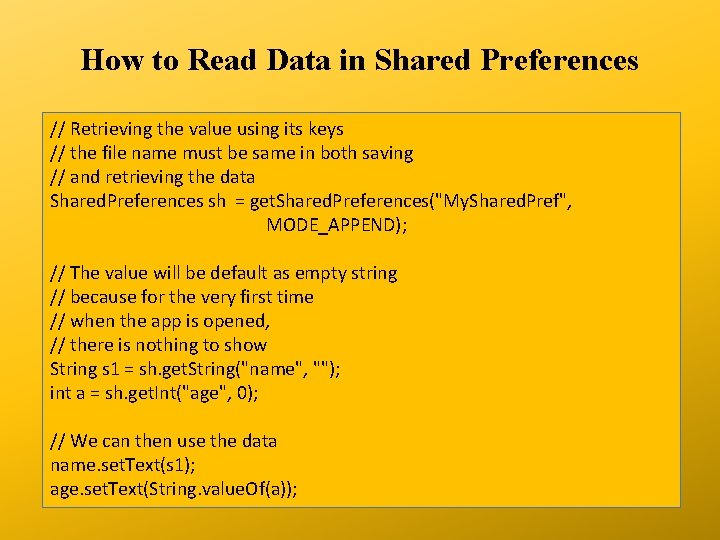
How to Read Data in Shared Preferences // Retrieving the value using its keys // the file name must be same in both saving // and retrieving the data Shared. Preferences sh = get. Shared. Preferences("My. Shared. Pref", MODE_APPEND); // The value will be default as empty string // because for the very first time // when the app is opened, // there is nothing to show String s 1 = sh. get. String("name", ""); int a = sh. get. Int("age", 0); // We can then use the data name. set. Text(s 1); age. set. Text(String. value. Of(a));
Data storage options in android
Primary storage vs secondary storage
Shared storage pool
Nas fiber channel
Bmi html
Storage devices of computer
Secondary storage provides temporary or volatile storage
Unified storage vs traditional storage
Wishes and regrets
Three types of claim
Socioemotional selectivity theory
Platform for privacy preferences project
Generalised system of preferences wto
Career choices and preferences in hrm
Tisc
Evolutionary psychology
Well-behaved preferences
Mendeley desktop preferences