Android Data Storage Your data storage options are
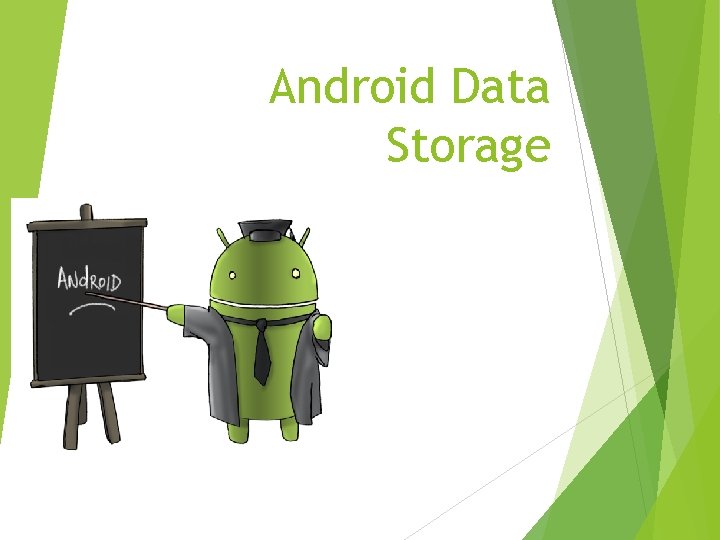
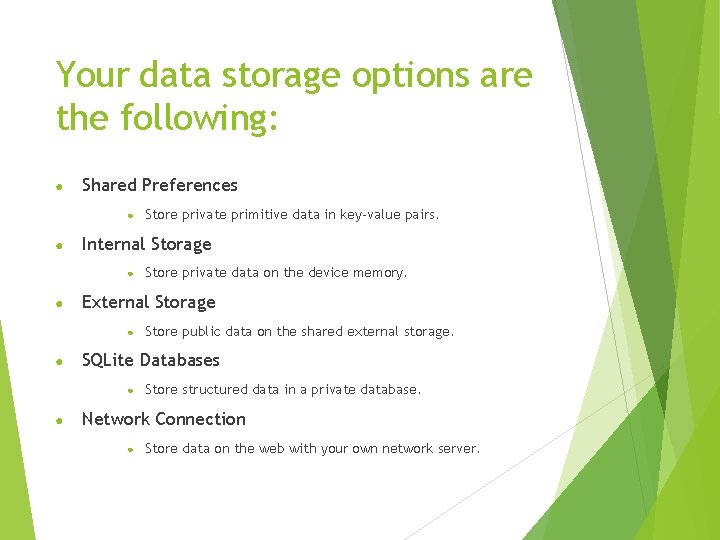
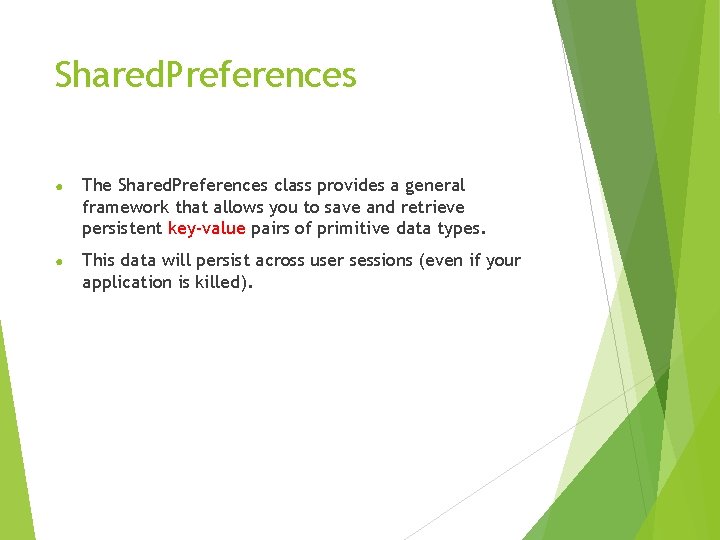
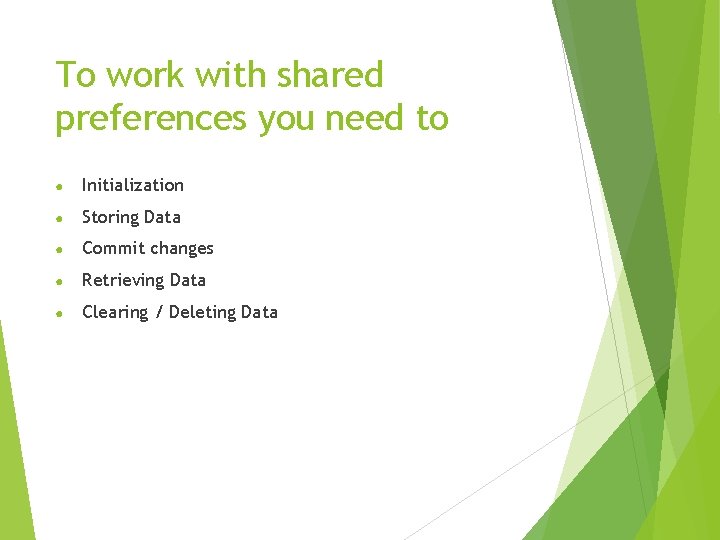
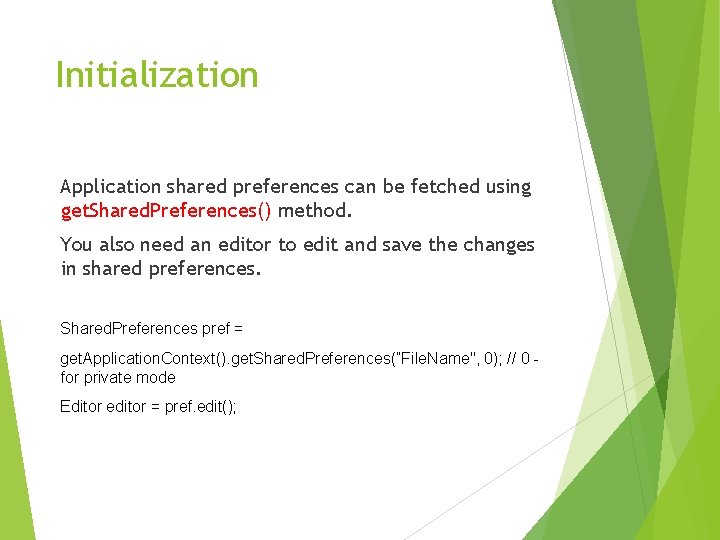
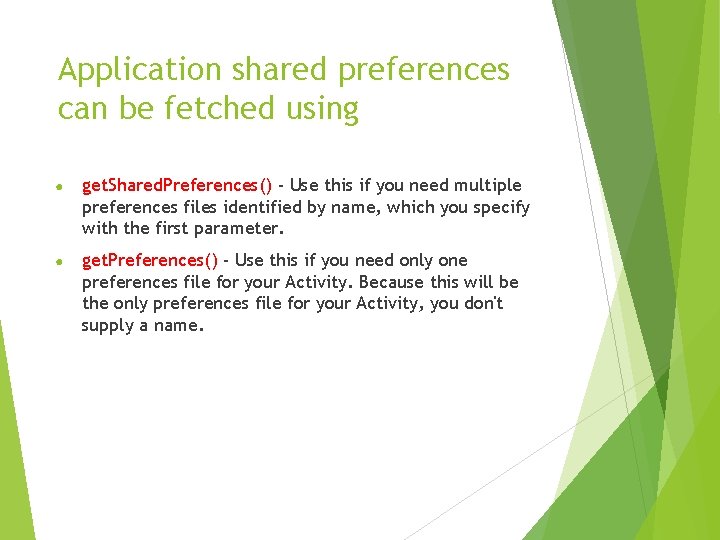
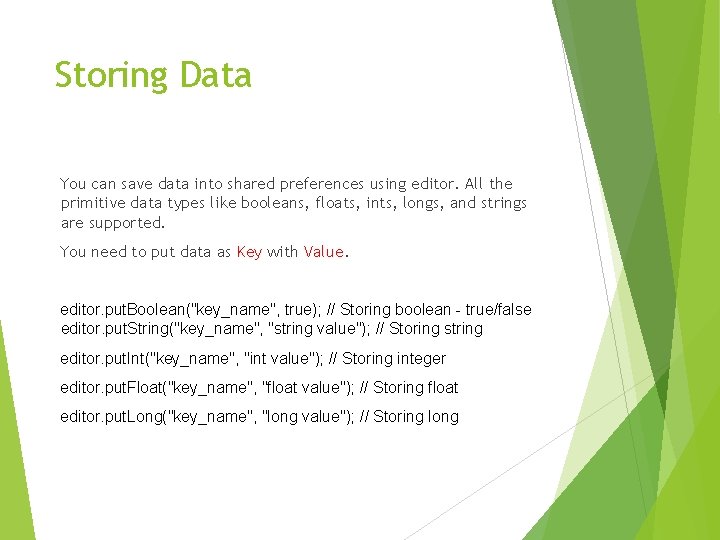
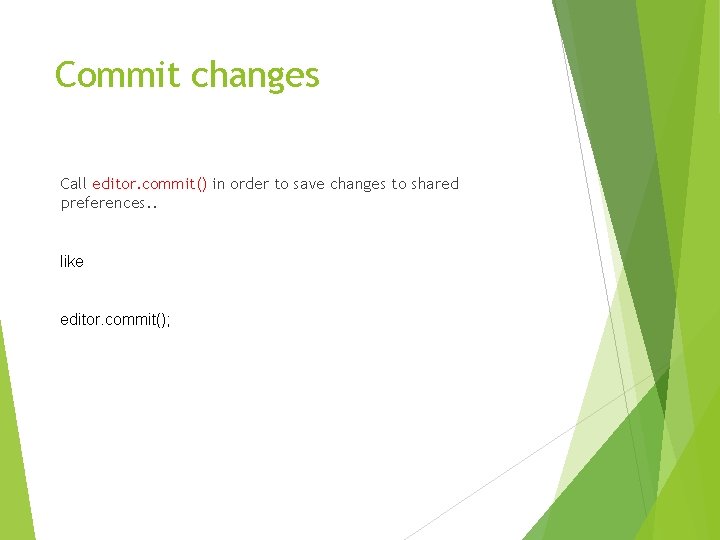
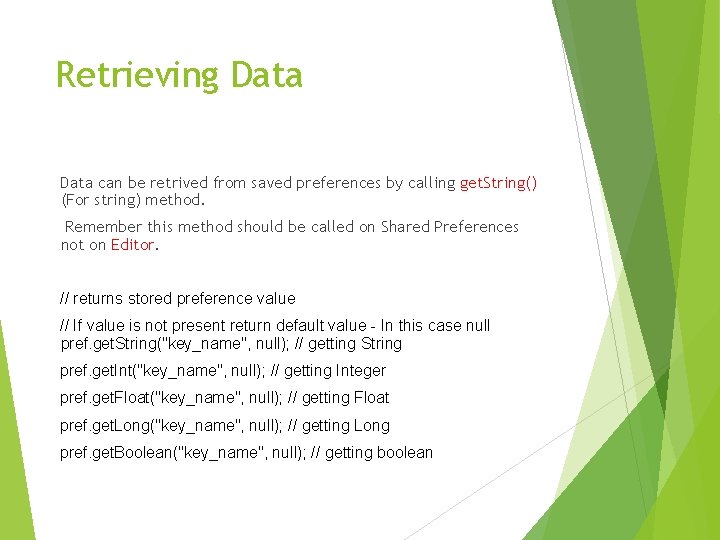
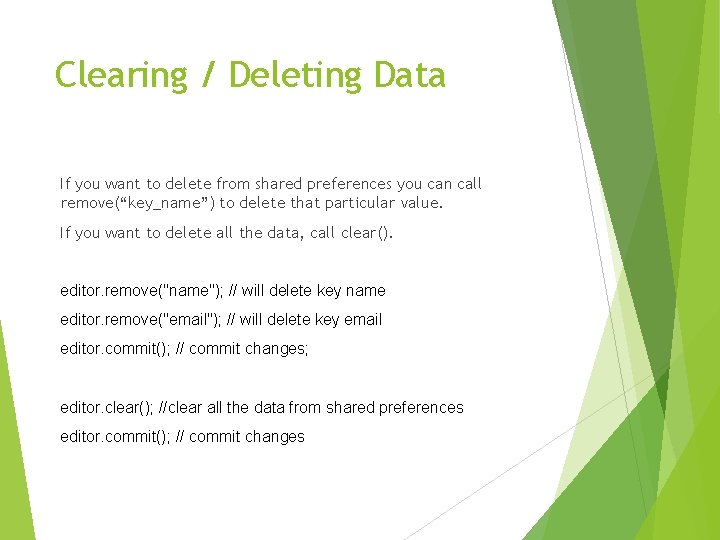
- Slides: 10
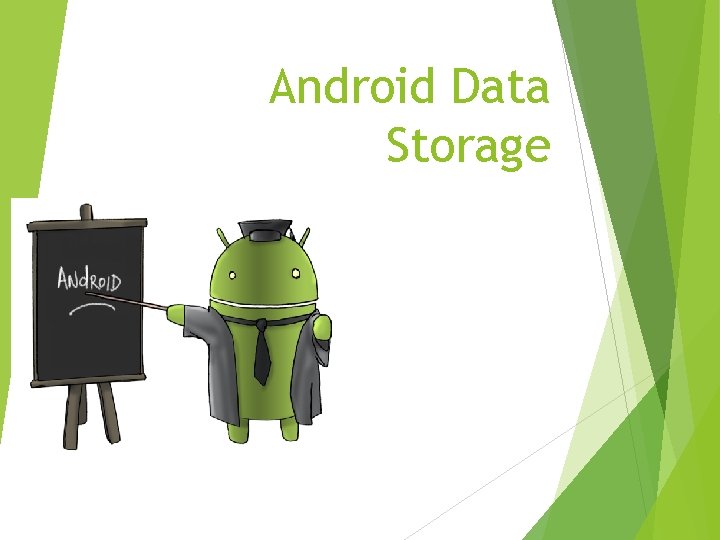
Android Data Storage
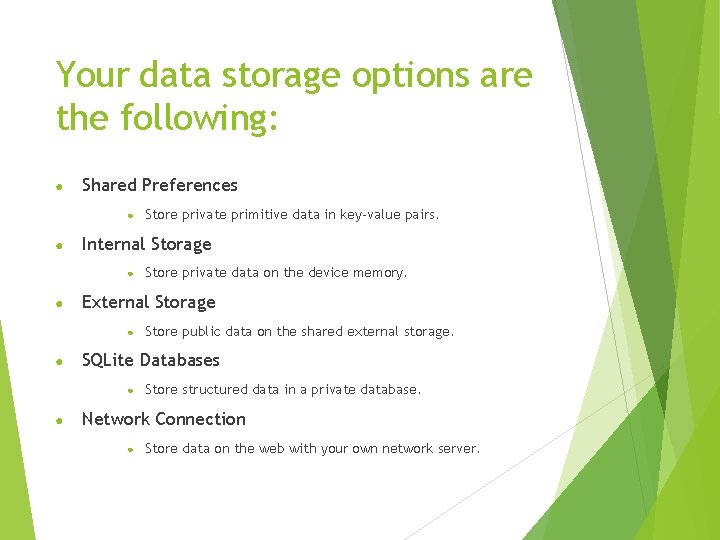
Your data storage options are the following: ● Shared Preferences ● ● Internal Storage ● ● Store public data on the shared external storage. SQLite Databases ● ● Store private data on the device memory. External Storage ● ● Store private primitive data in key-value pairs. Store structured data in a private database. Network Connection ● Store data on the web with your own network server.
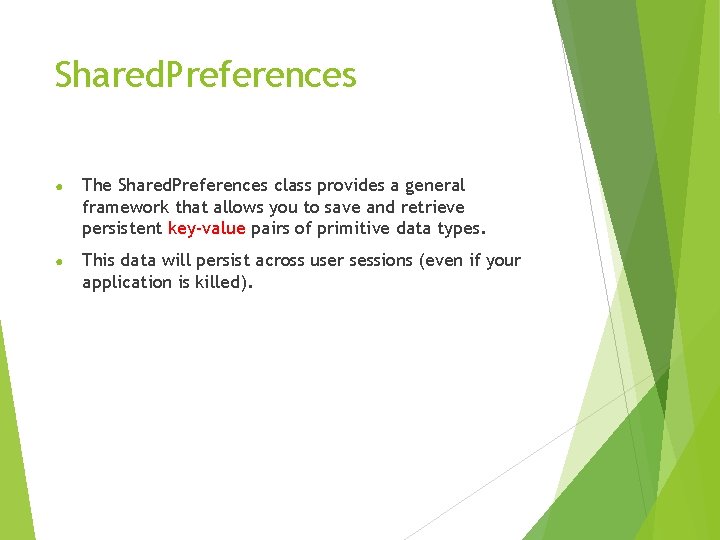
Shared. Preferences ● The Shared. Preferences class provides a general framework that allows you to save and retrieve persistent key-value pairs of primitive data types. ● This data will persist across user sessions (even if your application is killed).
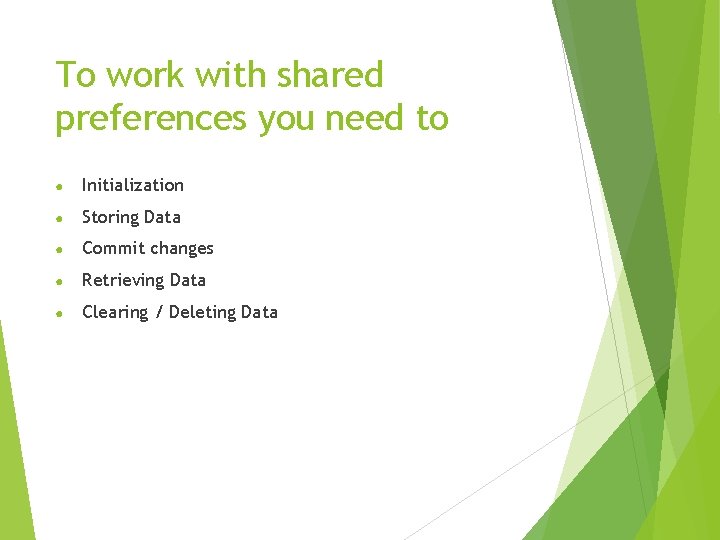
To work with shared preferences you need to ● Initialization ● Storing Data ● Commit changes ● Retrieving Data ● Clearing / Deleting Data
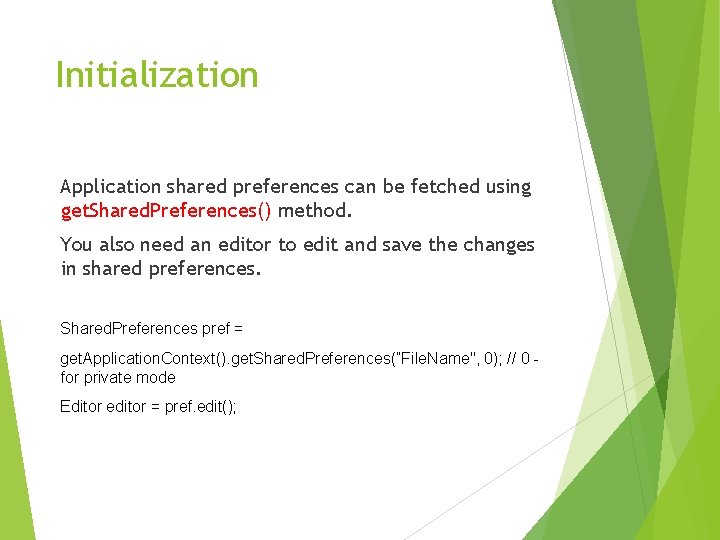
Initialization Application shared preferences can be fetched using get. Shared. Preferences() method. You also need an editor to edit and save the changes in shared preferences. Shared. Preferences pref = get. Application. Context(). get. Shared. Preferences(”File. Name", 0); // 0 for private mode Editor editor = pref. edit();
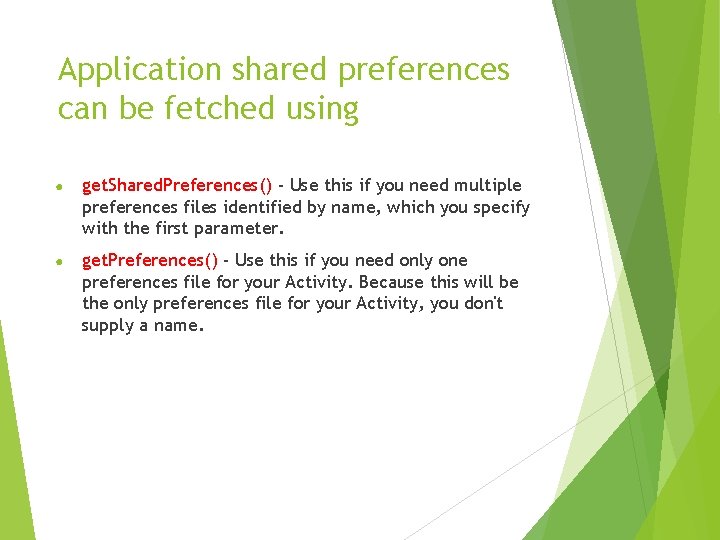
Application shared preferences can be fetched using ● get. Shared. Preferences() - Use this if you need multiple preferences files identified by name, which you specify with the first parameter. ● get. Preferences() - Use this if you need only one preferences file for your Activity. Because this will be the only preferences file for your Activity, you don't supply a name.
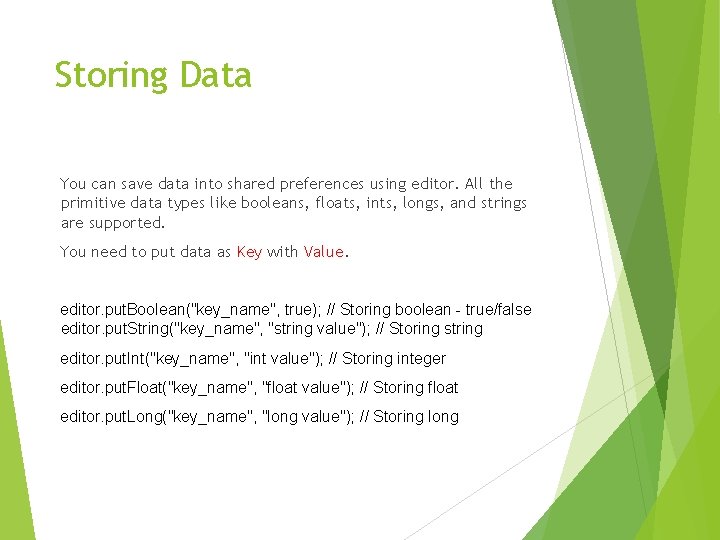
Storing Data You can save data into shared preferences using editor. All the primitive data types like booleans, floats, ints, longs, and strings are supported. You need to put data as Key with Value. editor. put. Boolean("key_name", true); // Storing boolean - true/false editor. put. String("key_name", "string value"); // Storing string editor. put. Int("key_name", "int value"); // Storing integer editor. put. Float("key_name", "float value"); // Storing float editor. put. Long("key_name", "long value"); // Storing long
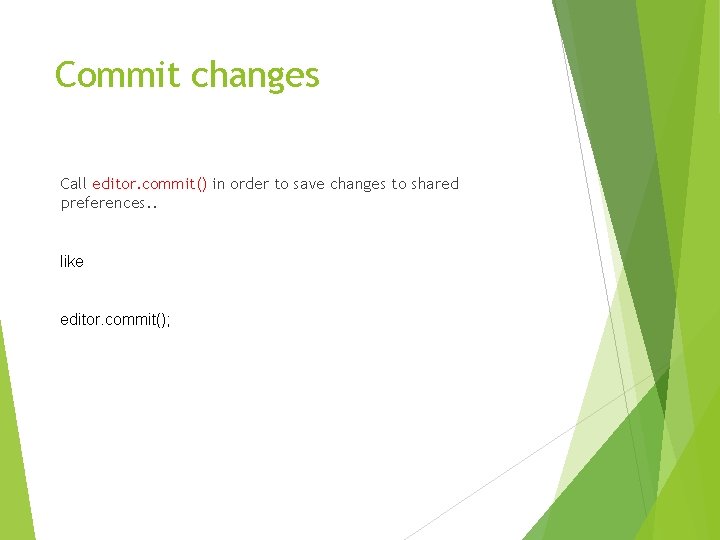
Commit changes Call editor. commit() in order to save changes to shared preferences. . like editor. commit();
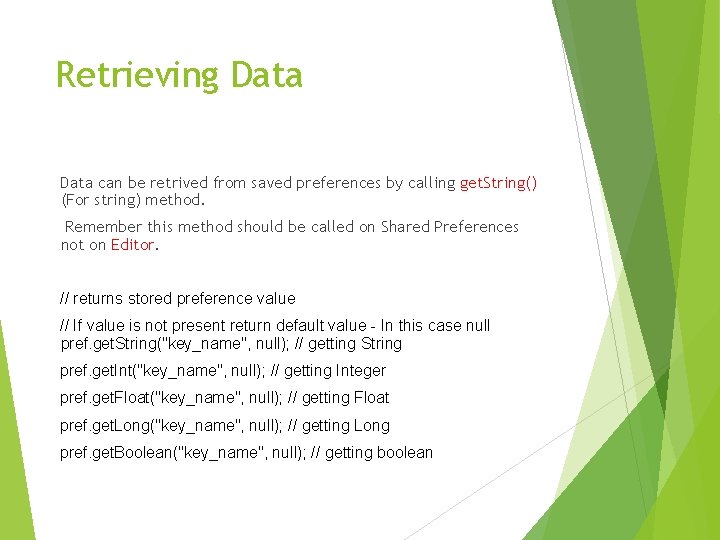
Retrieving Data can be retrived from saved preferences by calling get. String() (For string) method. Remember this method should be called on Shared Preferences not on Editor. // returns stored preference value // If value is not present return default value - In this case null pref. get. String("key_name", null); // getting String pref. get. Int("key_name", null); // getting Integer pref. get. Float("key_name", null); // getting Float pref. get. Long("key_name", null); // getting Long pref. get. Boolean("key_name", null); // getting boolean
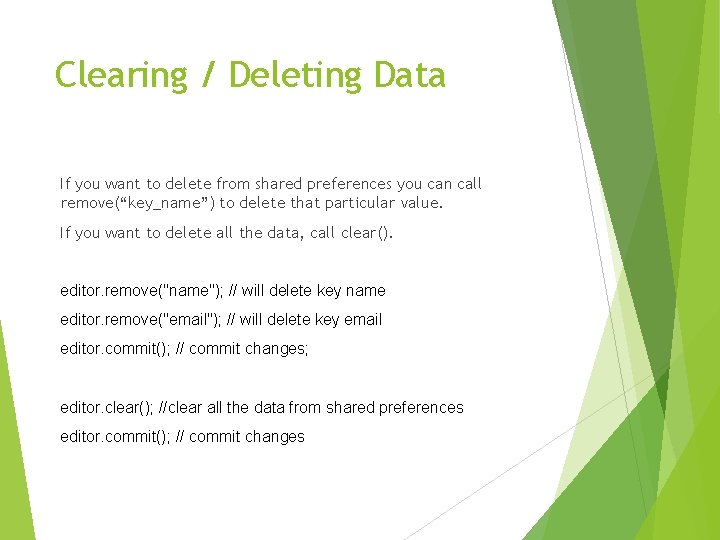
Clearing / Deleting Data If you want to delete from shared preferences you can call remove(“key_name”) to delete that particular value. If you want to delete all the data, call clear(). editor. remove("name"); // will delete key name editor. remove("email"); // will delete key email editor. commit(); // commit changes; editor. clear(); //clear all the data from shared preferences editor. commit(); // commit changes