8 1 class Point private int xcoord int
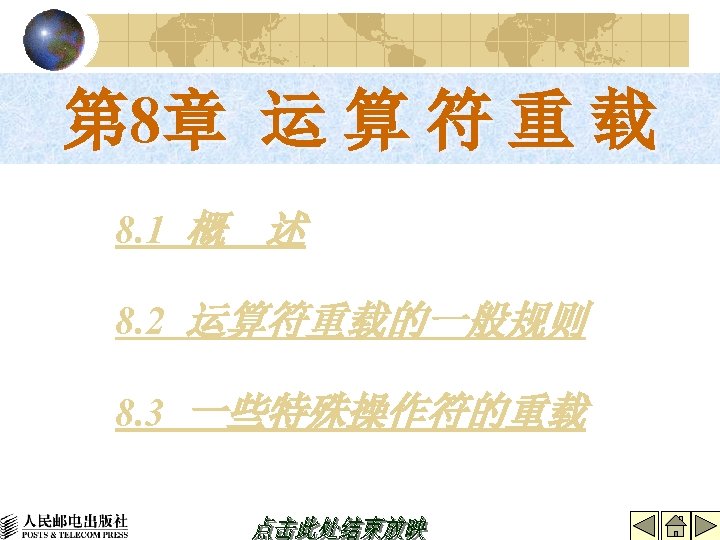
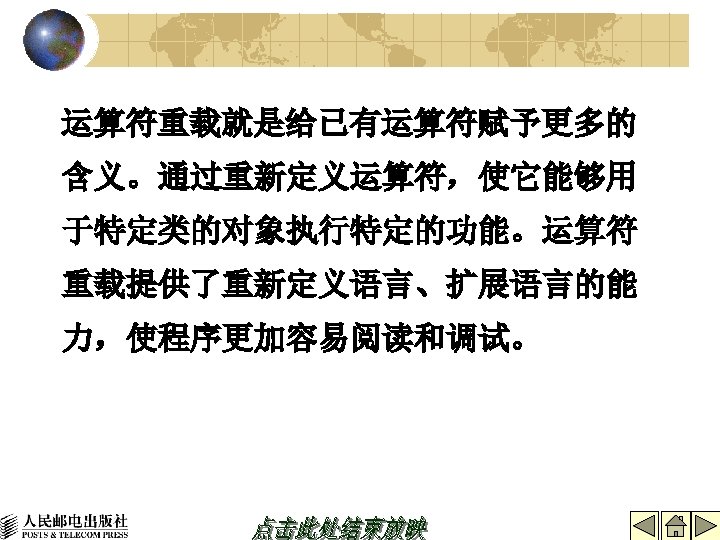
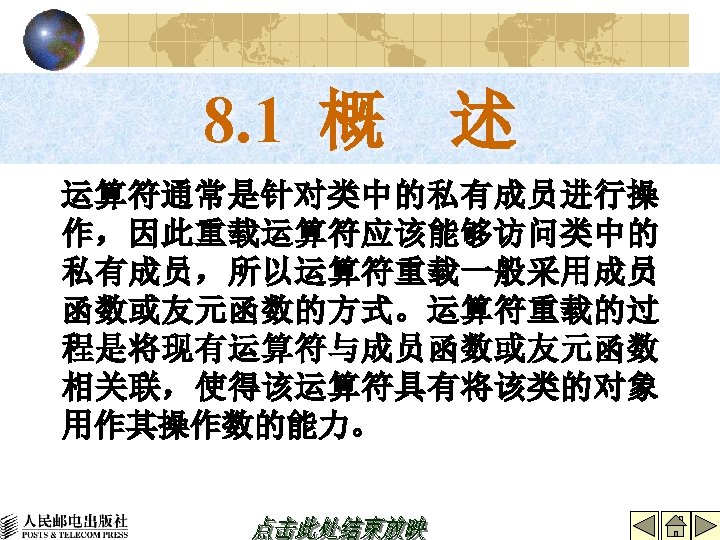
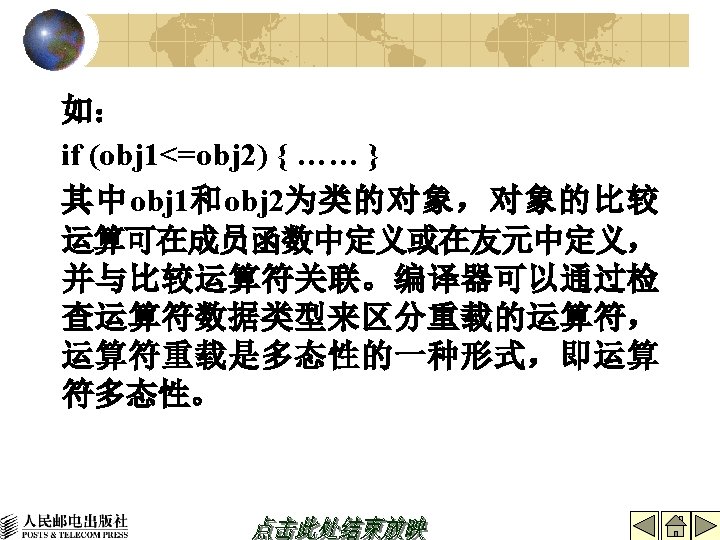
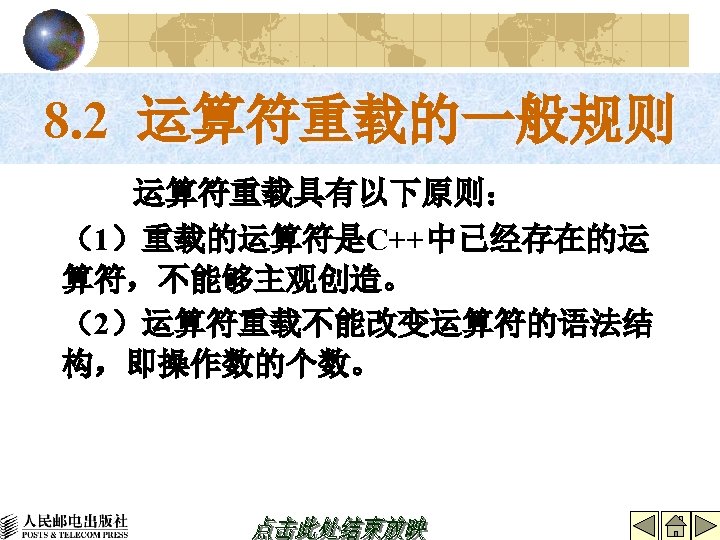
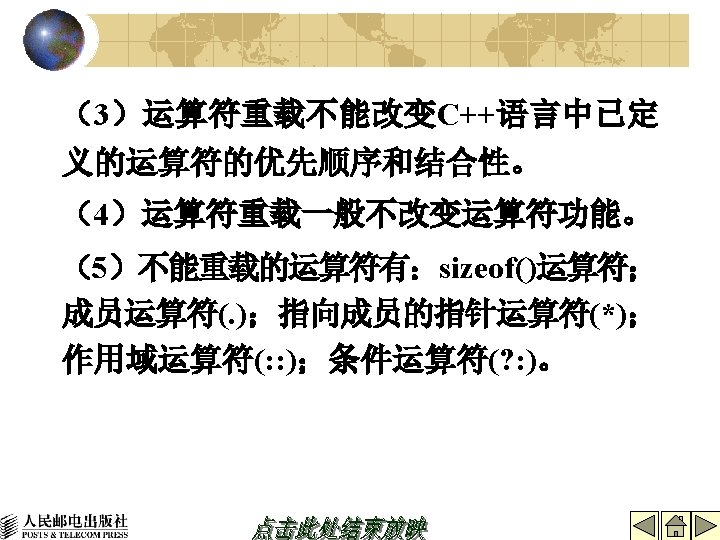
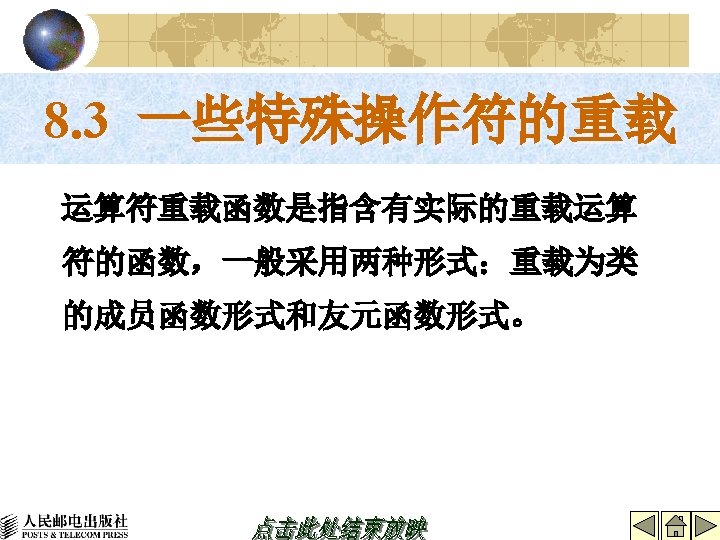
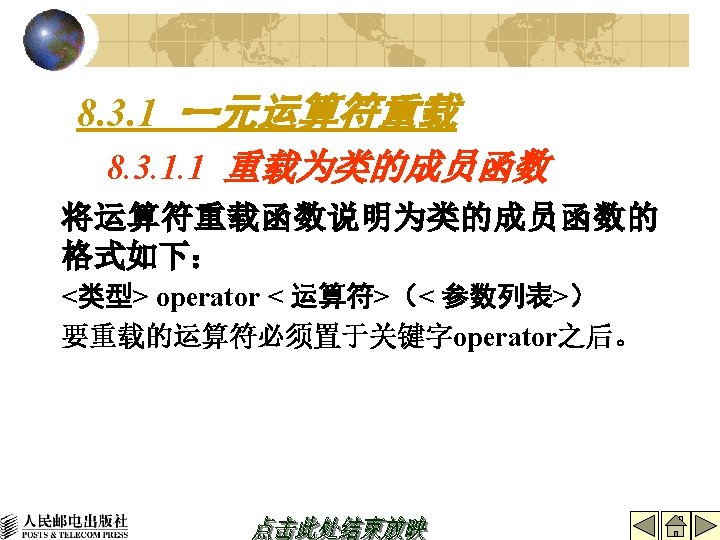
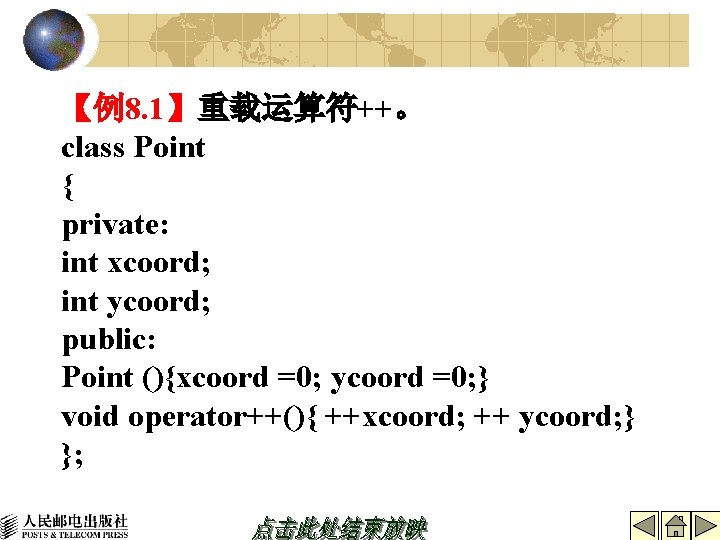
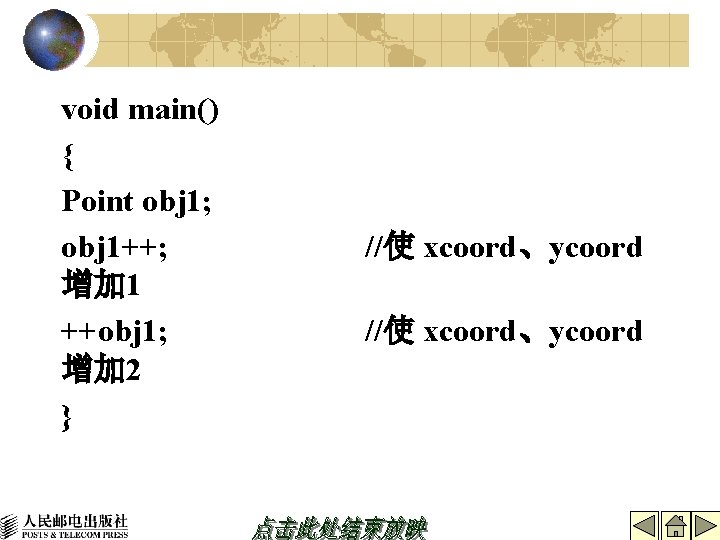
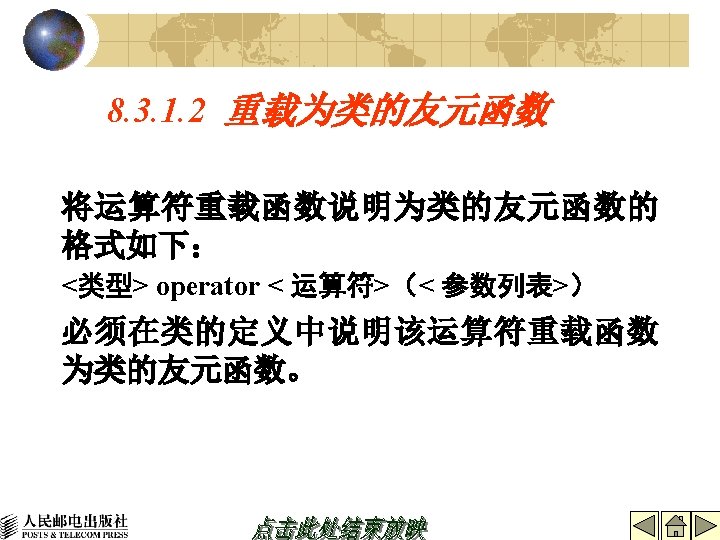
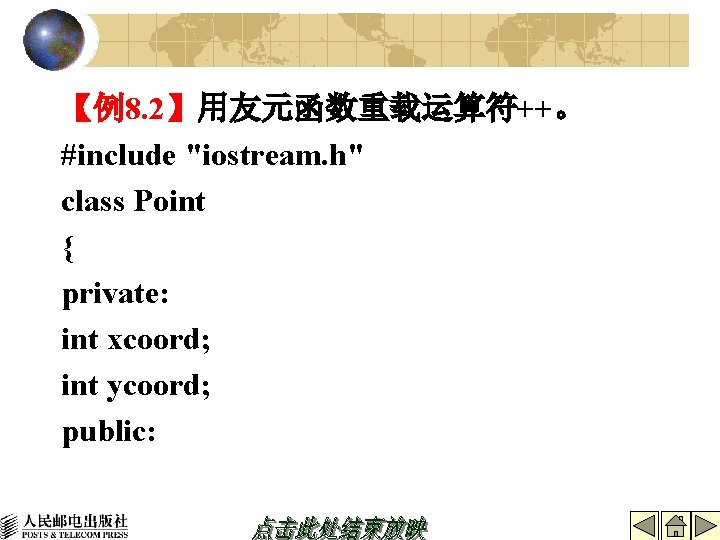
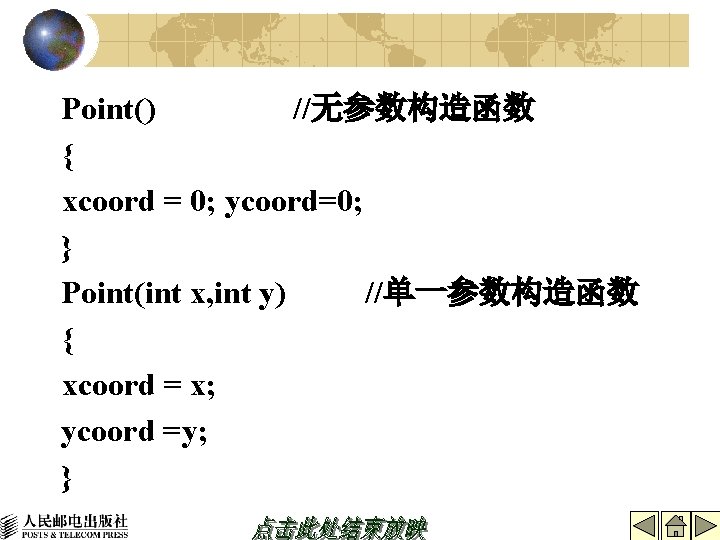
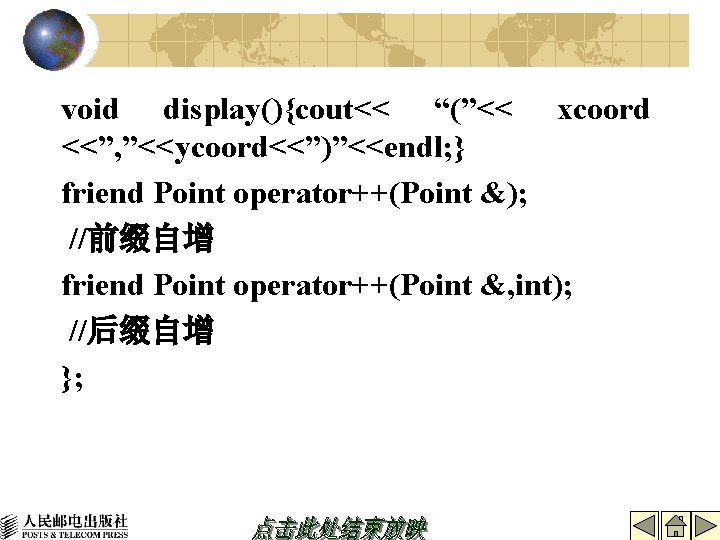
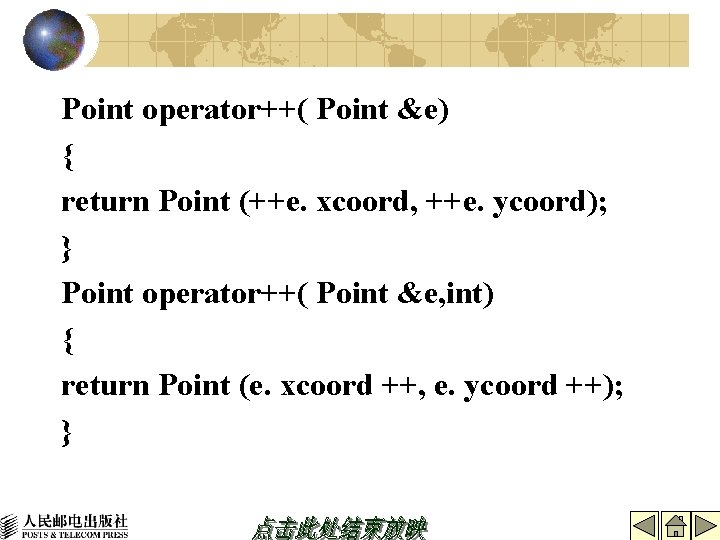
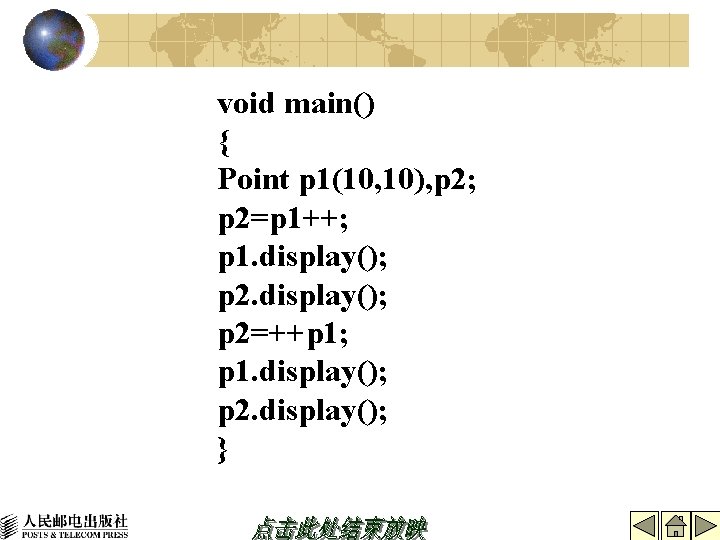
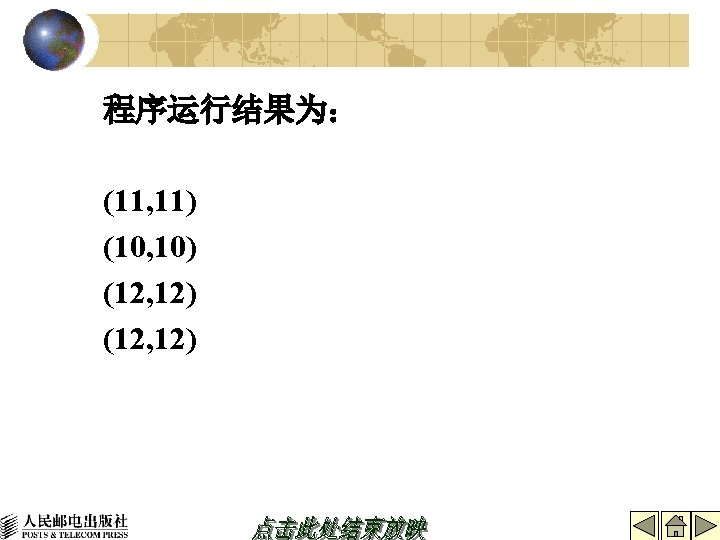
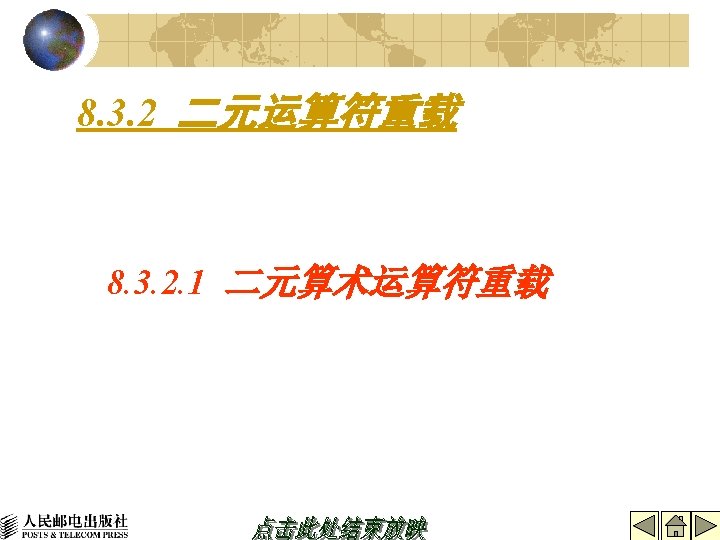
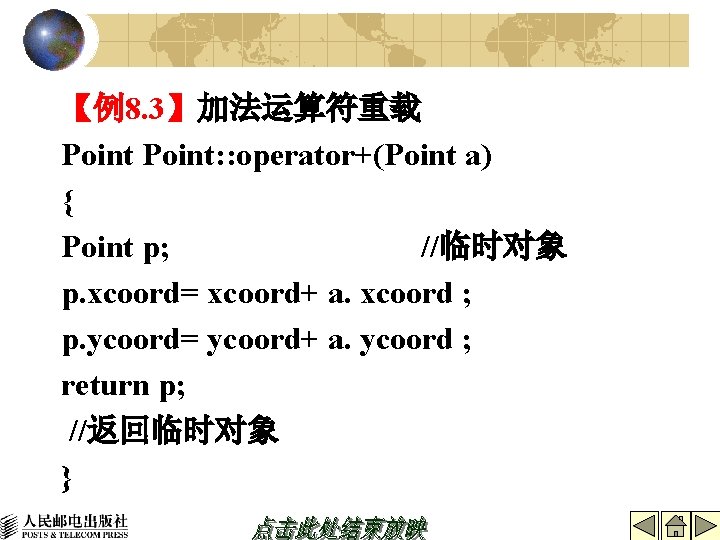
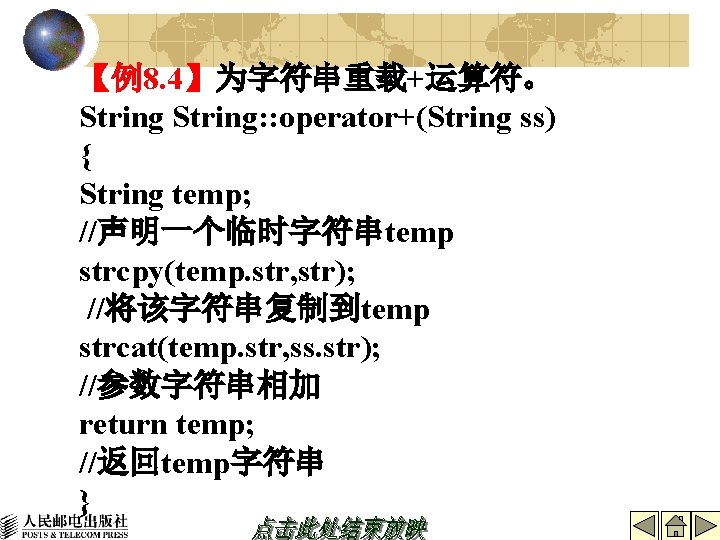
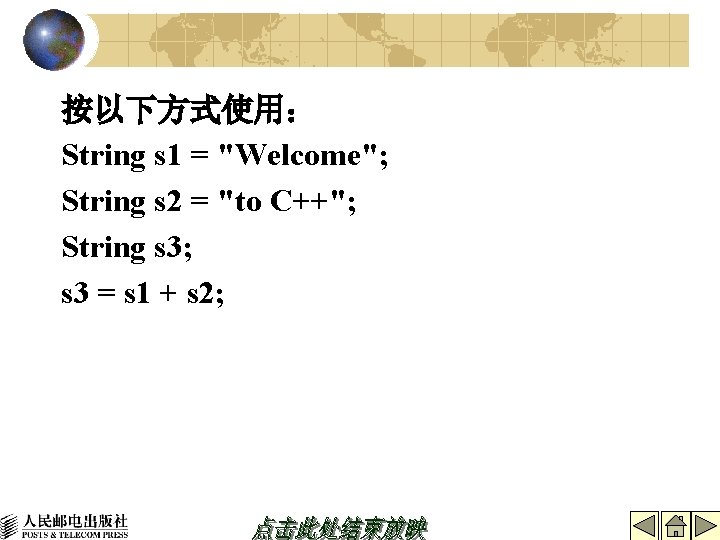
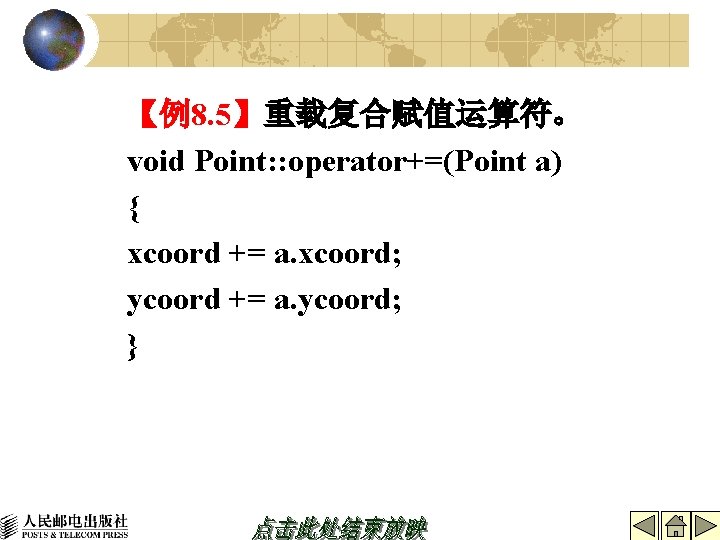
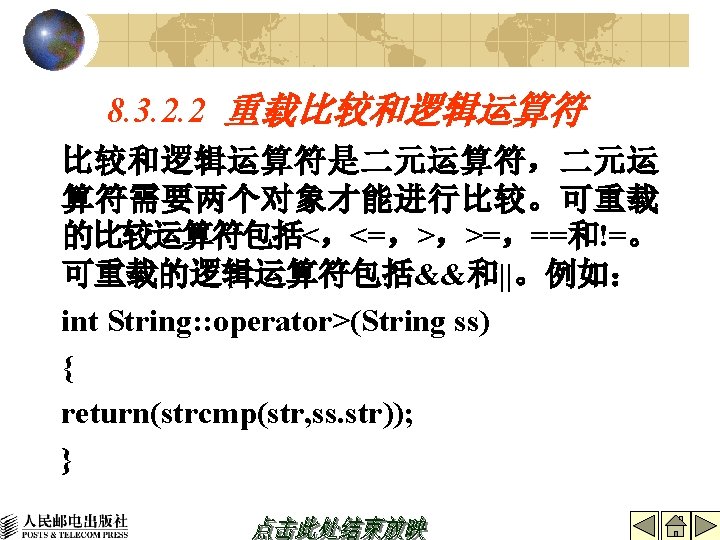
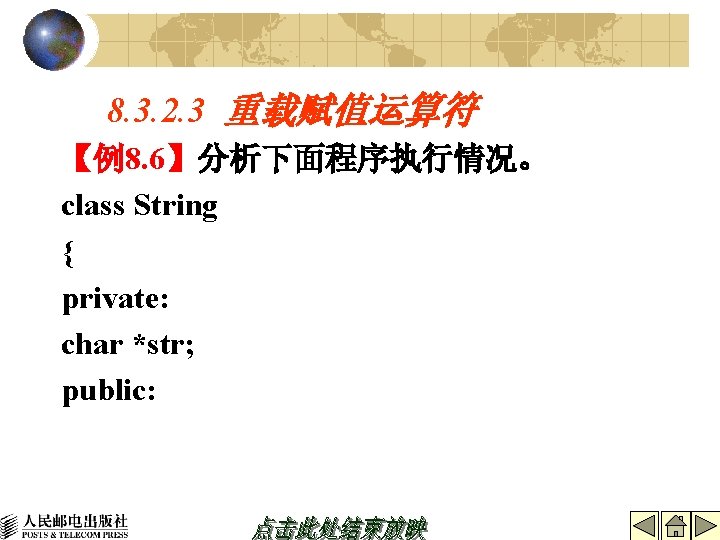
![String(char *s = "") { int length = strlen(s); str = new char[length+1]; strcpy(str, String(char *s = "") { int length = strlen(s); str = new char[length+1]; strcpy(str,](https://slidetodoc.com/presentation_image_h2/592da0f71288eaafee1d37c1e4c510f4/image-25.jpg)
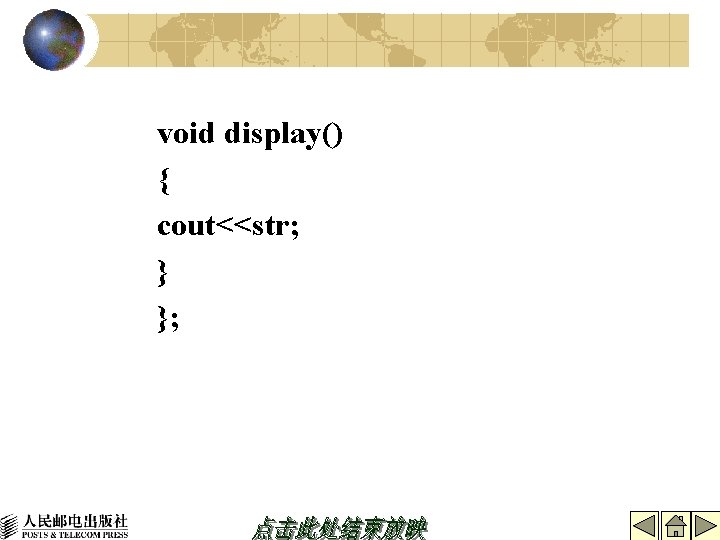
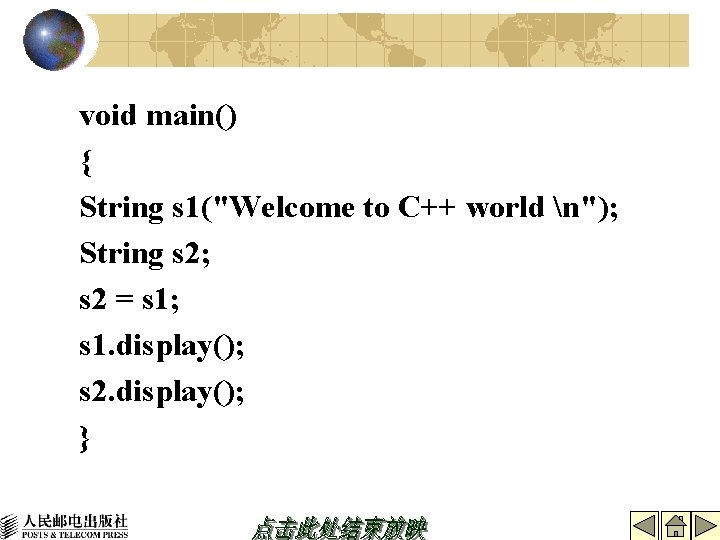
- Slides: 27
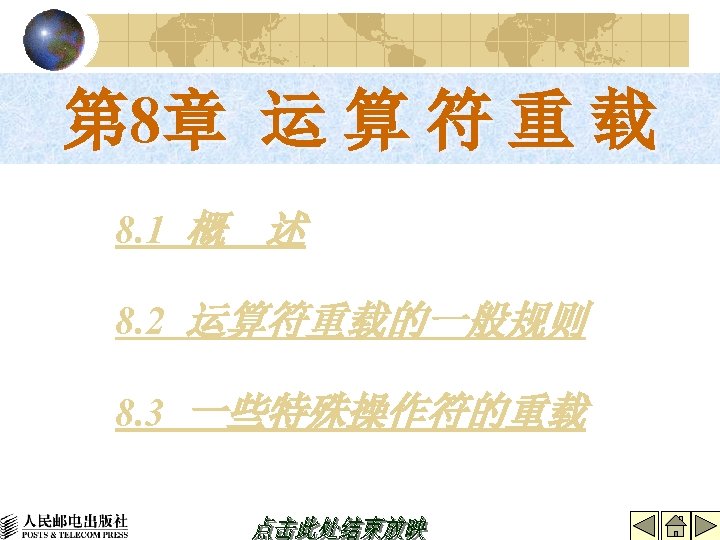
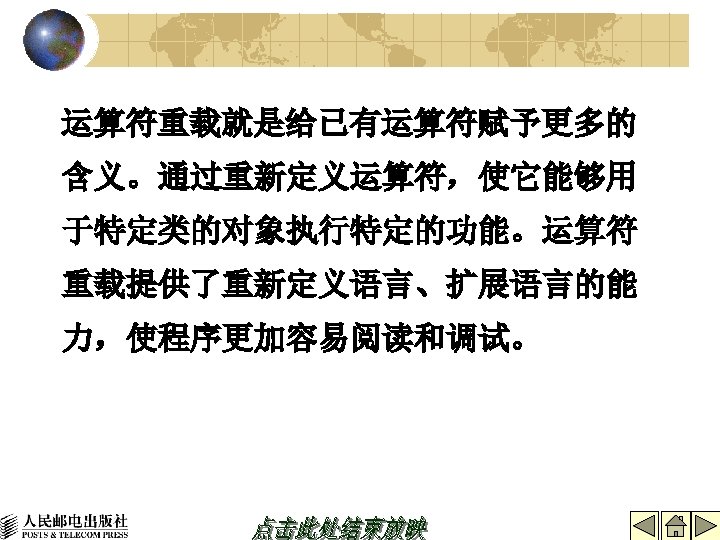
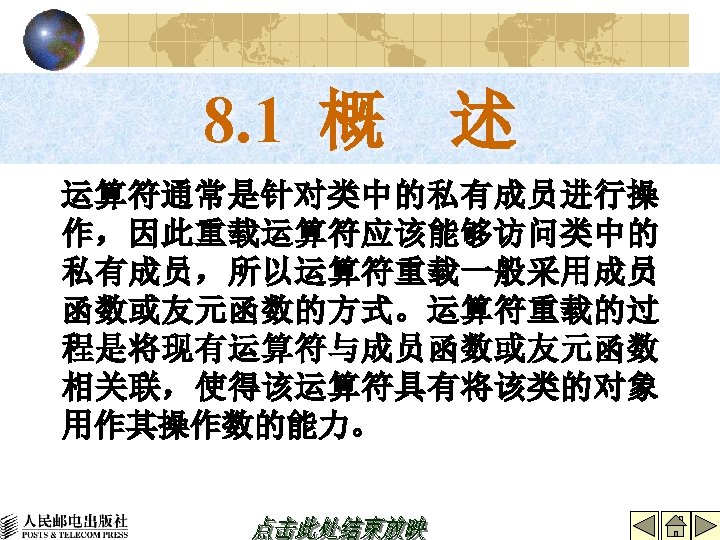
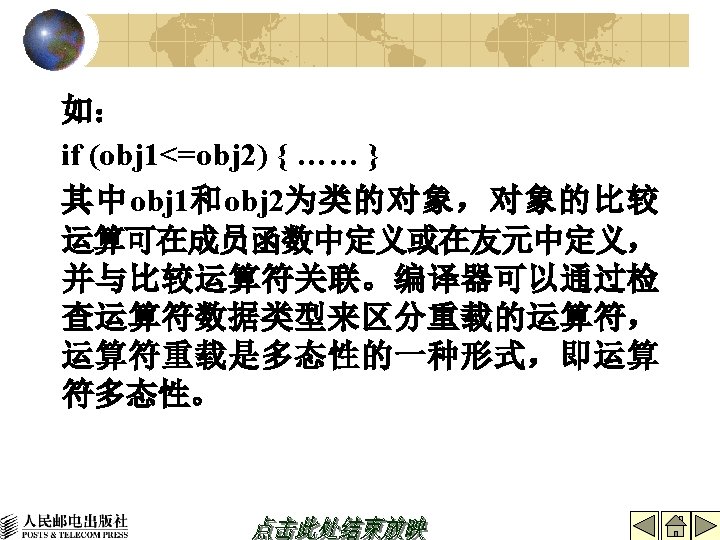
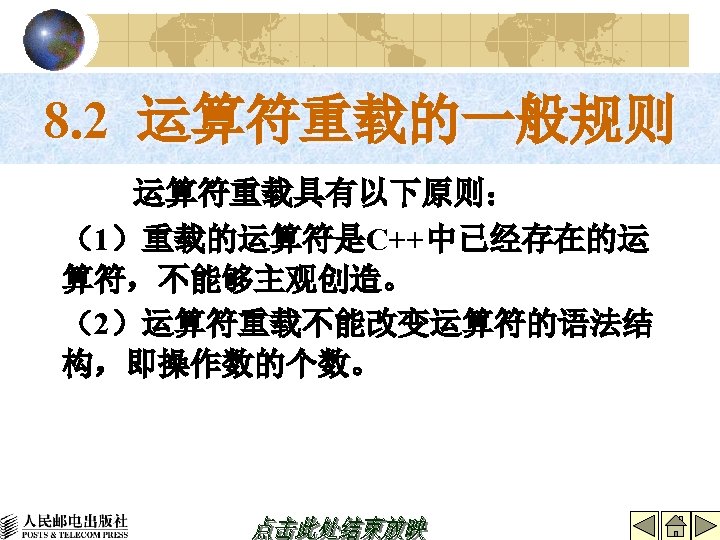
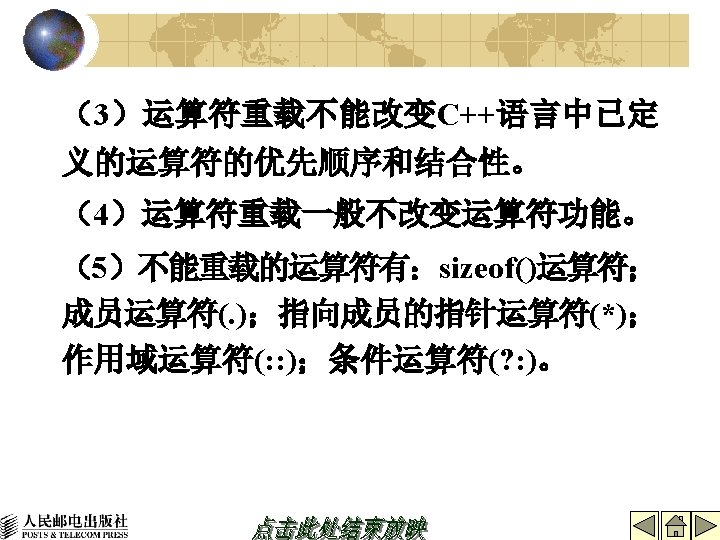
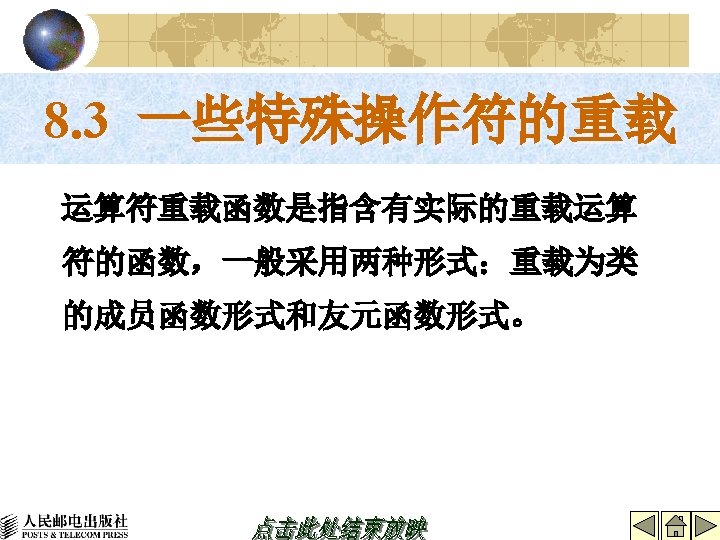
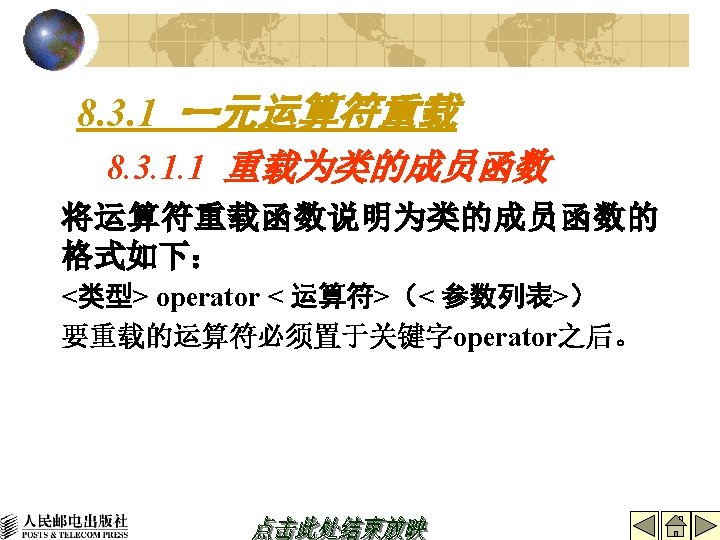
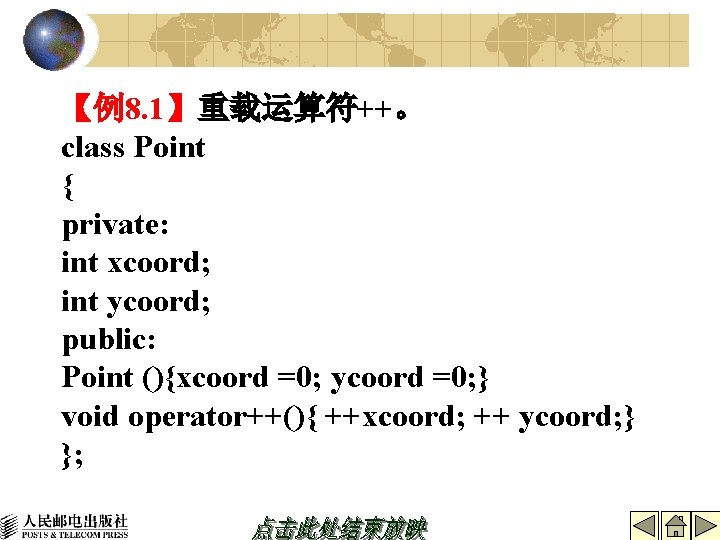
【例8. 1】重载运算符++。 class Point { private: int xcoord; int ycoord; public: Point (){xcoord =0; ycoord =0; } void operator++(){ ++xcoord; ++ ycoord; } };
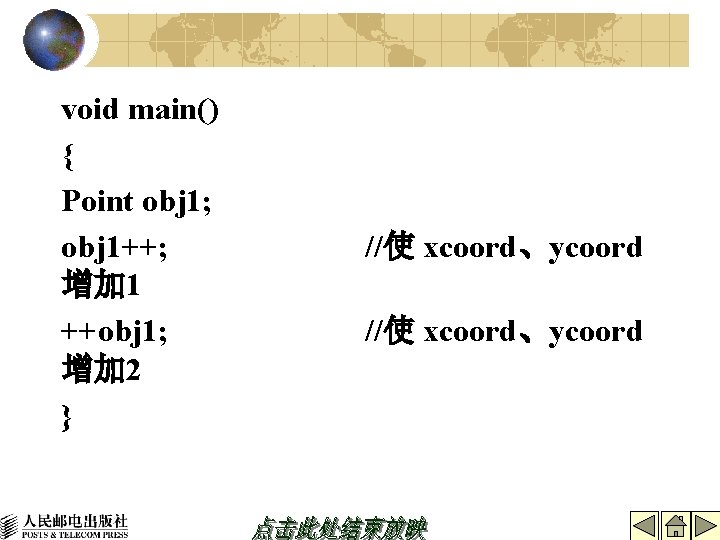
void main() { Point obj 1; obj 1++; 增加 1 ++obj 1; 增加 2 } //使 xcoord、ycoord
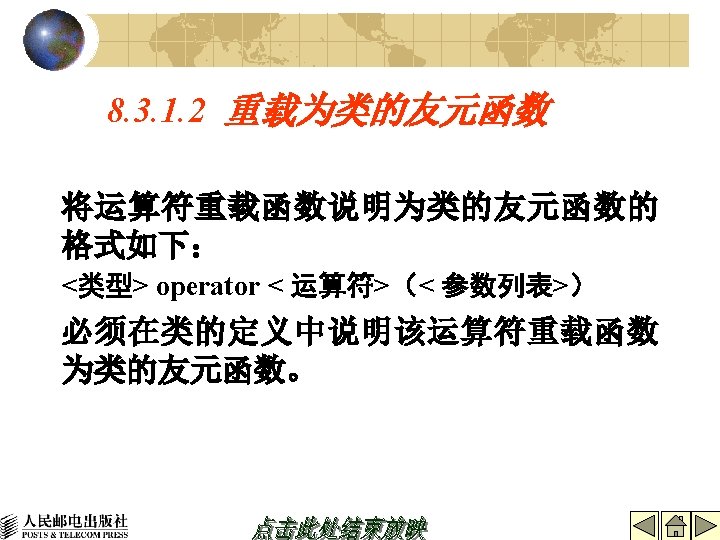
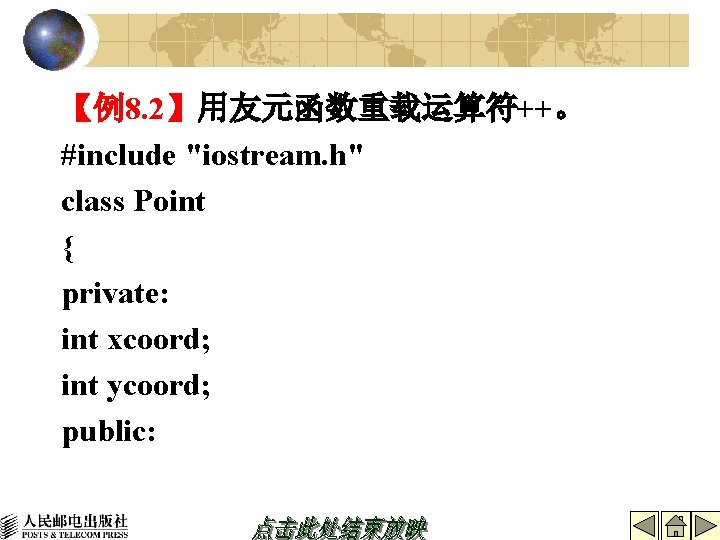
【例8. 2】用友元函数重载运算符++。 #include "iostream. h" class Point { private: int xcoord; int ycoord; public:
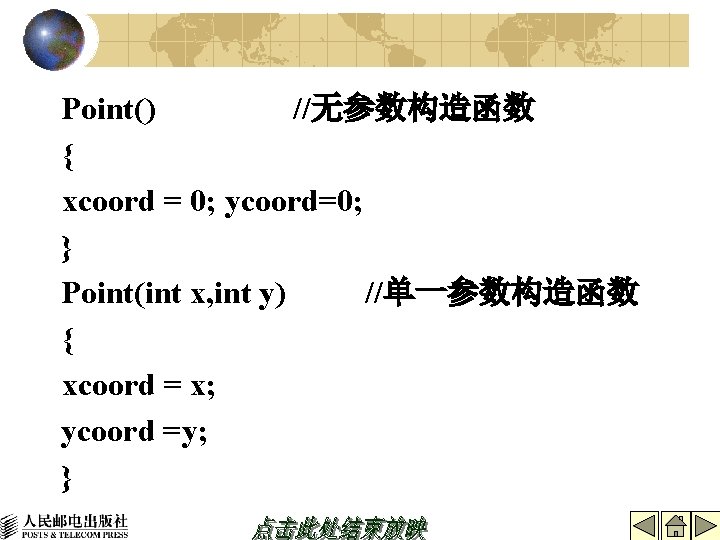
Point() //无参数构造函数 { xcoord = 0; ycoord=0; } Point(int x, int y) //单一参数构造函数 { xcoord = x; ycoord =y; }
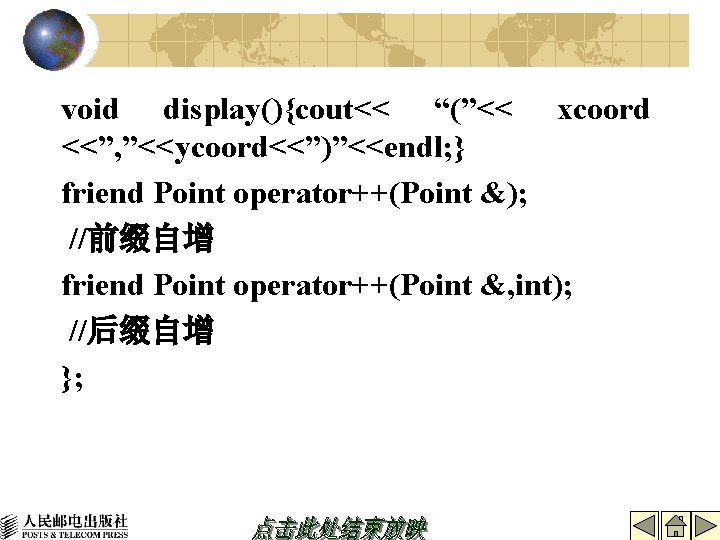
void display(){cout<< “(”<< xcoord <<”, ”<<ycoord<<”)”<<endl; } friend Point operator++(Point &); //前缀自增 friend Point operator++(Point &, int); //后缀自增 };
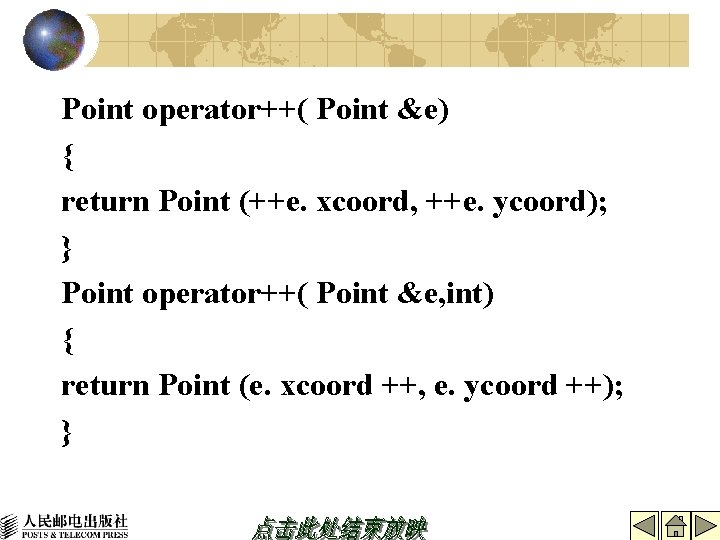
Point operator++( Point &e) { return Point (++e. xcoord, ++e. ycoord); } Point operator++( Point &e, int) { return Point (e. xcoord ++, e. ycoord ++); }
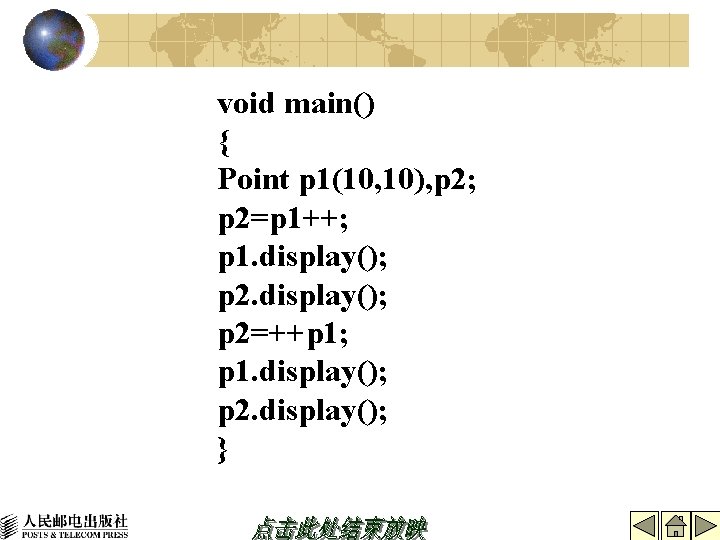
void main() { Point p 1(10, 10), p 2; p 2=p 1++; p 1. display(); p 2=++p 1; p 1. display(); p 2. display(); }
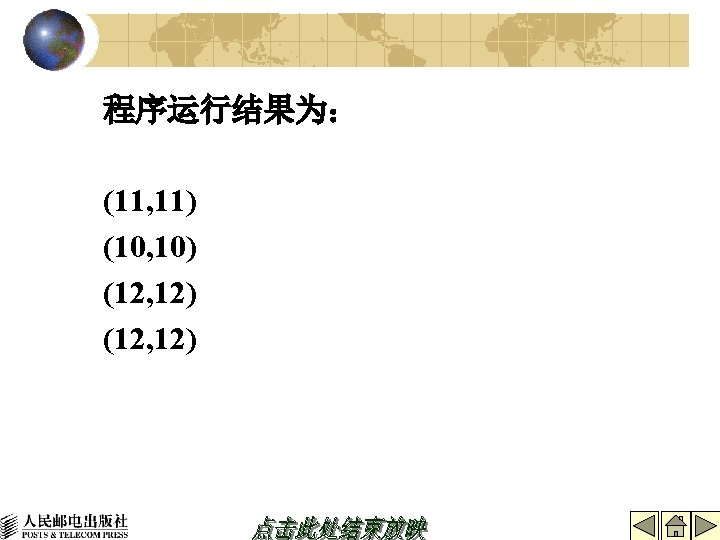
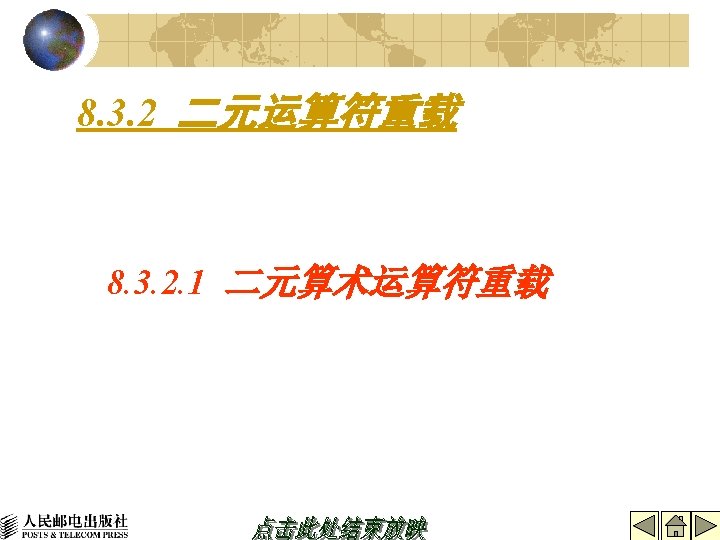
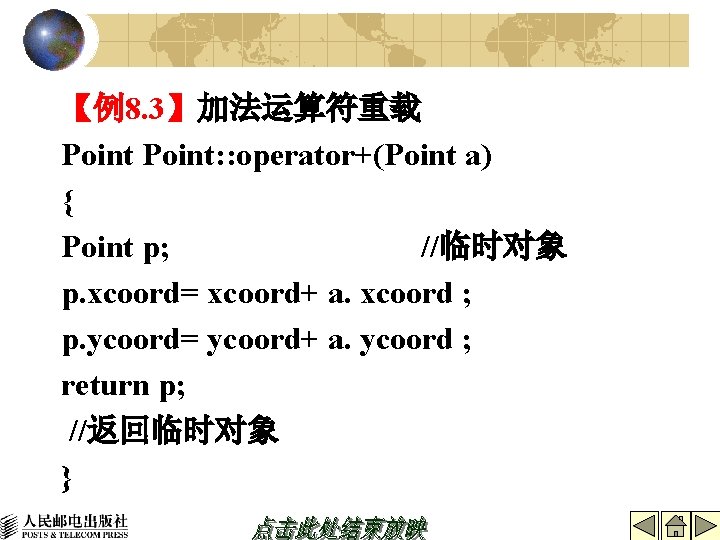
【例8. 3】加法运算符重载 Point: : operator+(Point a) { Point p; //临时对象 p. xcoord= xcoord+ a. xcoord ; p. ycoord= ycoord+ a. ycoord ; return p; //返回临时对象 }
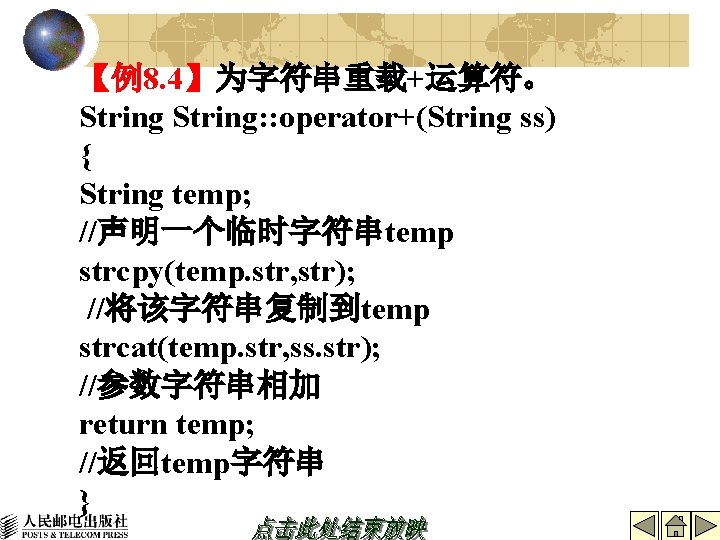
【例8. 4】为字符串重载+运算符。 String: : operator+(String ss) { String temp; //声明一个临时字符串temp strcpy(temp. str, str); //将该字符串复制到temp strcat(temp. str, ss. str); //参数字符串相加 return temp; //返回temp字符串 }
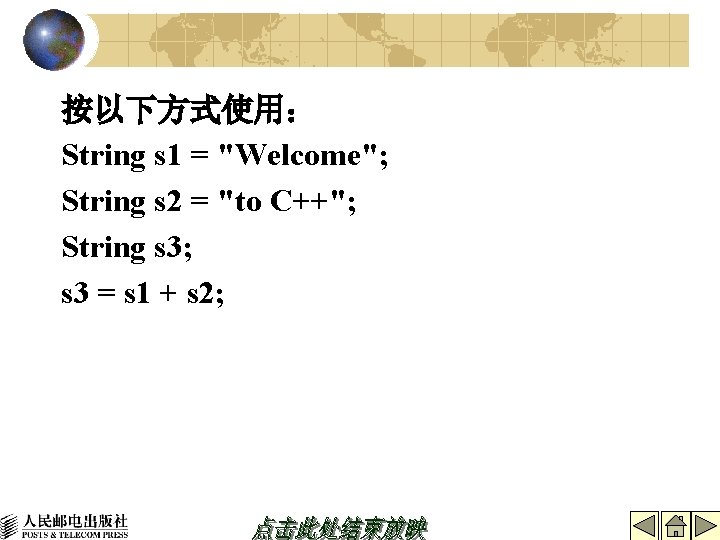
按以下方式使用: String s 1 = "Welcome"; String s 2 = "to C++"; String s 3; s 3 = s 1 + s 2;
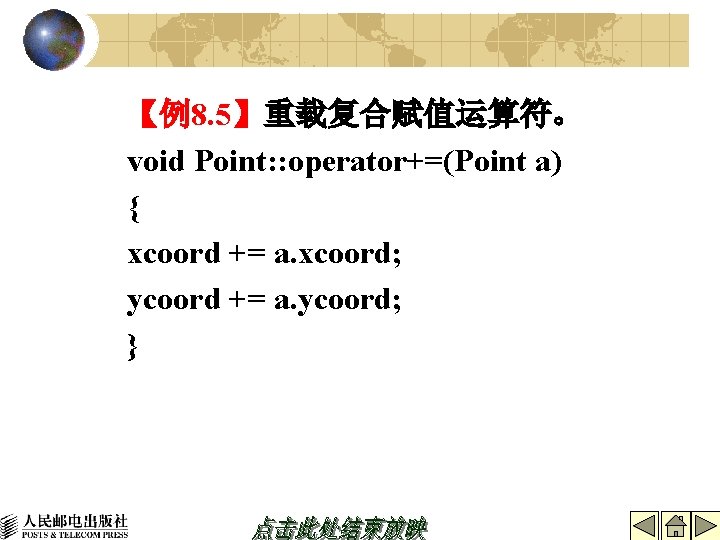
【例8. 5】重载复合赋值运算符。 void Point: : operator+=(Point a) { xcoord += a. xcoord; ycoord += a. ycoord; }
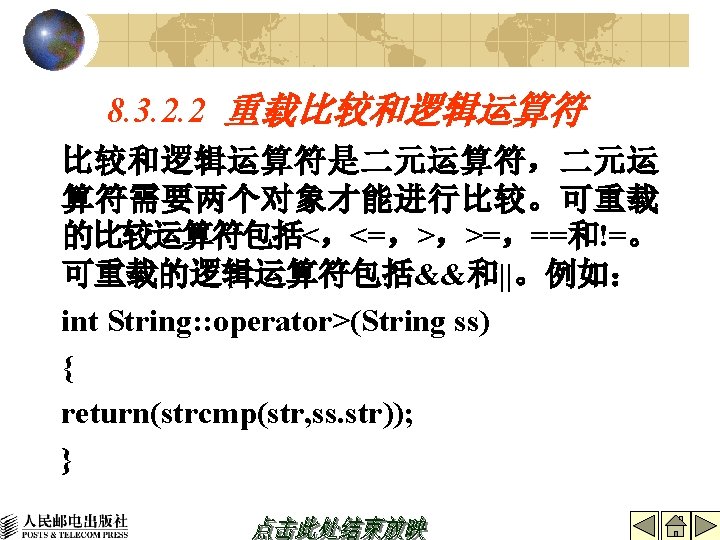
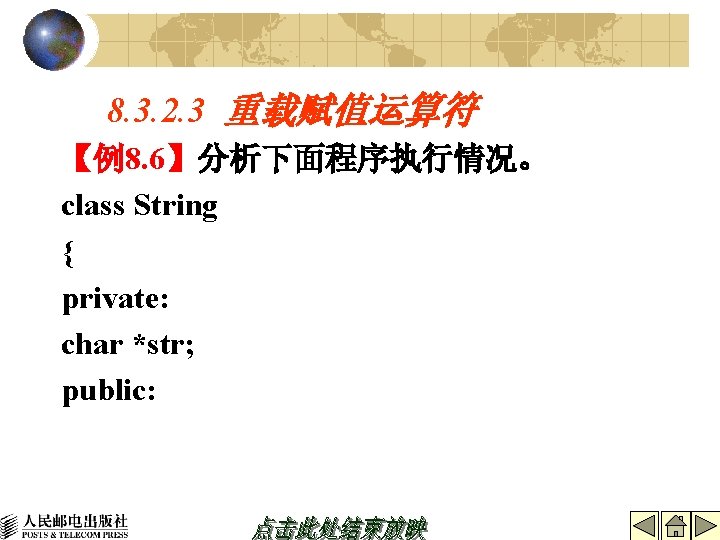
![Stringchar s int length strlens str new charlength1 strcpystr String(char *s = "") { int length = strlen(s); str = new char[length+1]; strcpy(str,](https://slidetodoc.com/presentation_image_h2/592da0f71288eaafee1d37c1e4c510f4/image-25.jpg)
String(char *s = "") { int length = strlen(s); str = new char[length+1]; strcpy(str, s); } ~String() //析构函数 { delete[] str; }
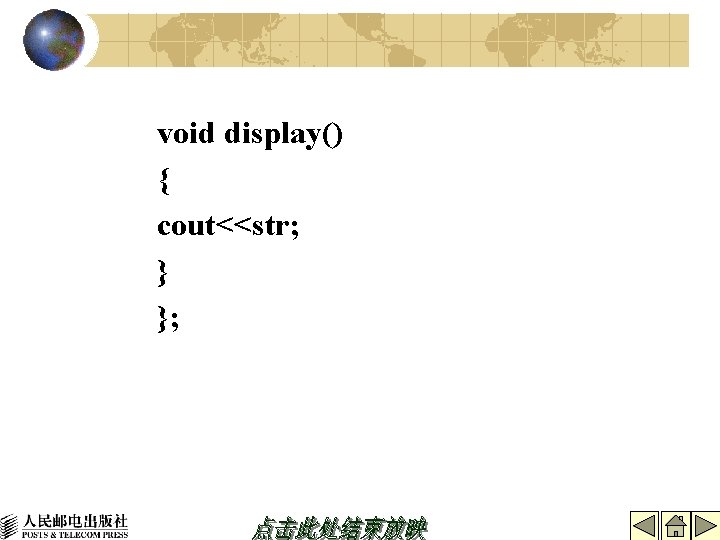
void display() { cout<<str; } };
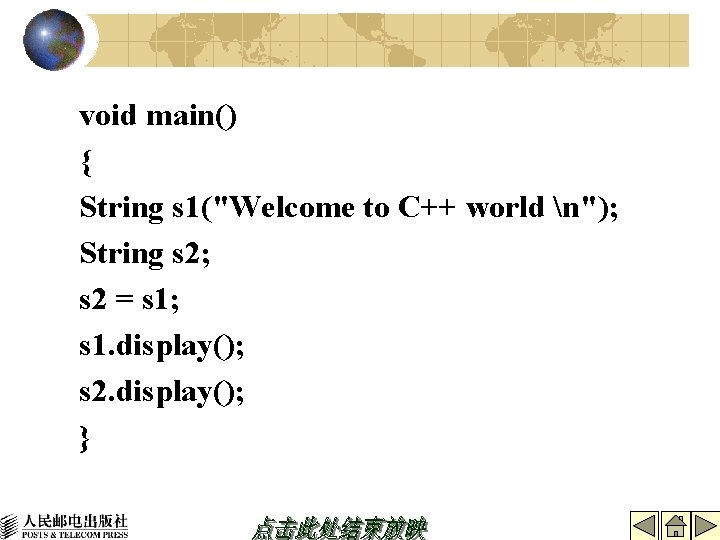
void main() { String s 1("Welcome to C++ world n"); String s 2; s 2 = s 1; s 1. display(); s 2. display(); }
Int sum(int a int n) int sum=0 i
Interface calculator public int add(int a int b)
Int max(int x int y)
Public void drawsquare(int x, int y, int len)
Divideint
Class person string name int age
A { private int x; public a( val) { x = val; } }
Private int
Private int
Private int
Interface myinterface int foo(int x)
Int main(int argc, char** argv)
7팩토리얼
Const int arduino adalah
Int main int num 4
Void swap
Void f(int i) int j=0
Int.max
Int main int argc char argv
Cadet private first class
Public class employee private string name
Private equity as an asset class
Private string[]
Public class plant private string name
Public class animal private string name
Public abstract class fish private void swim
Public class telephone { private string number