Point class Point private int x int y
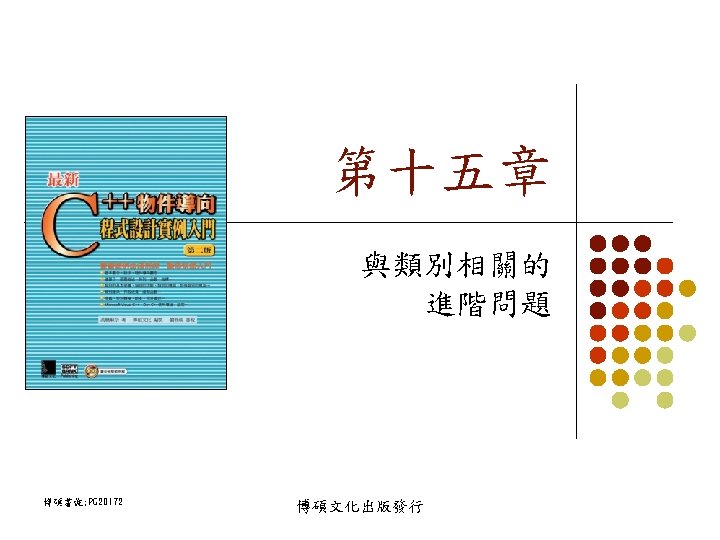
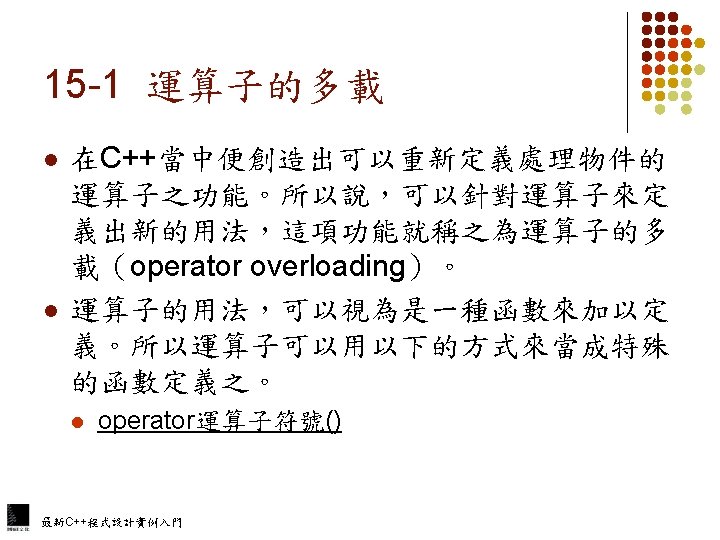
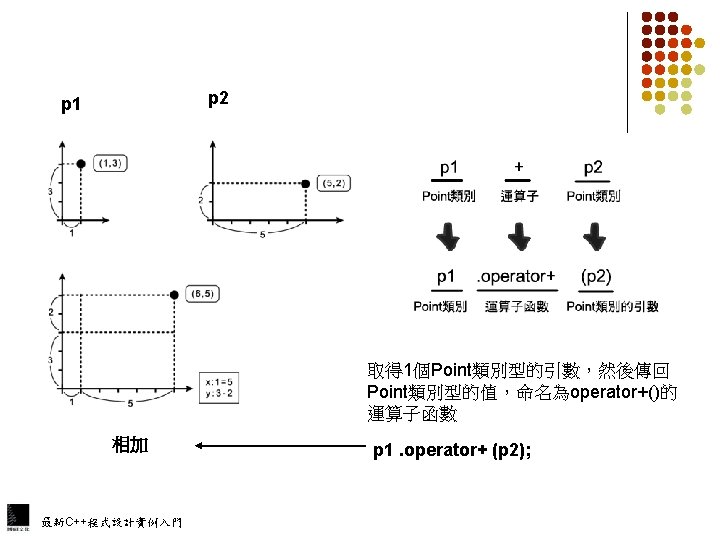
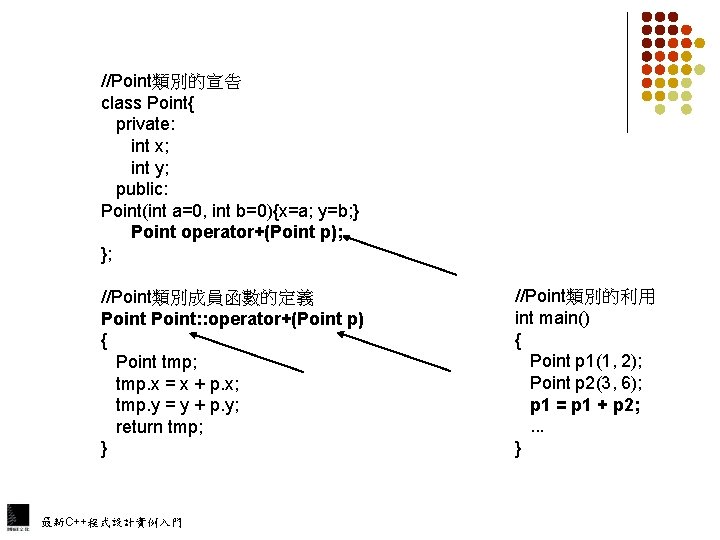
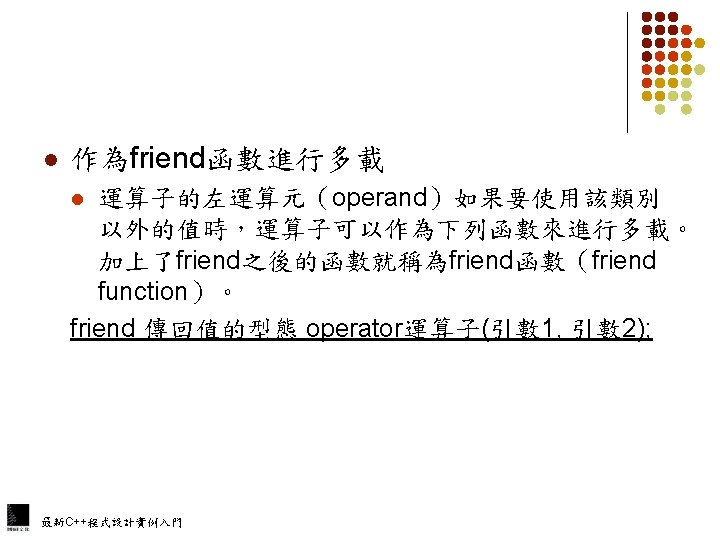
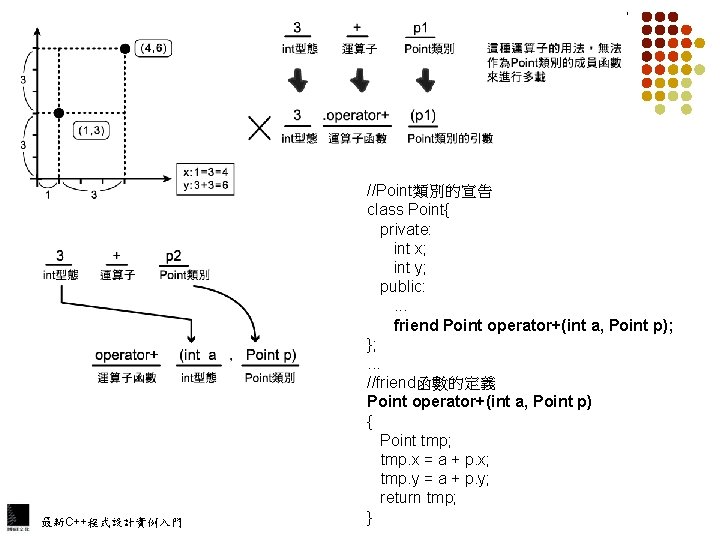
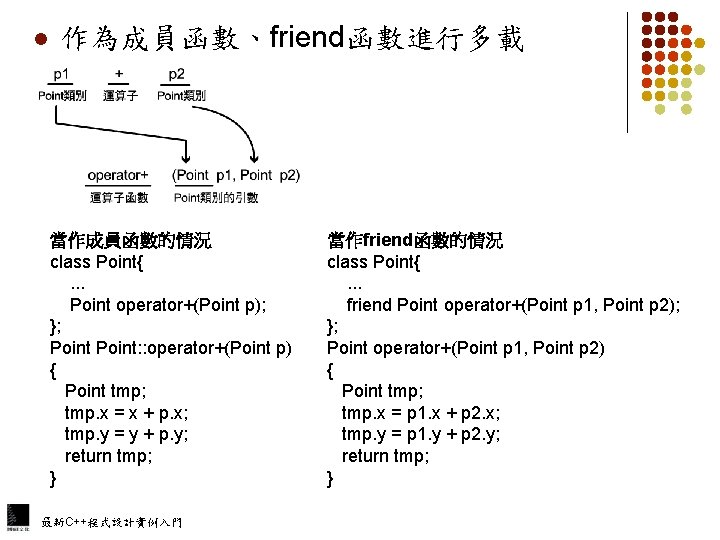
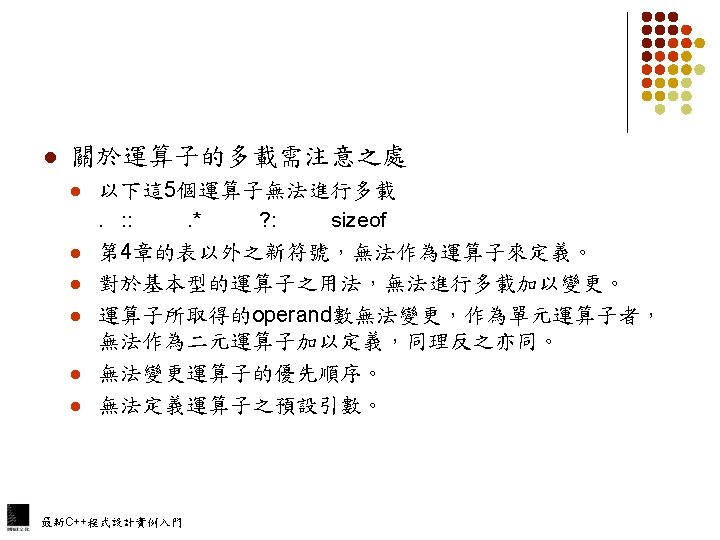
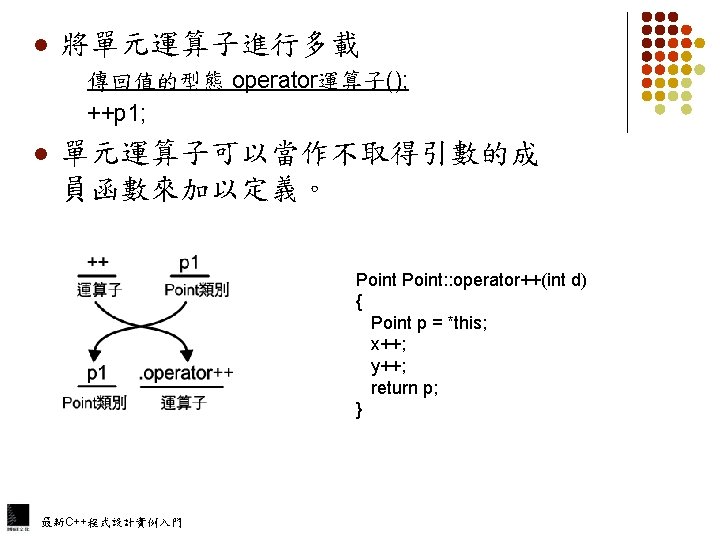
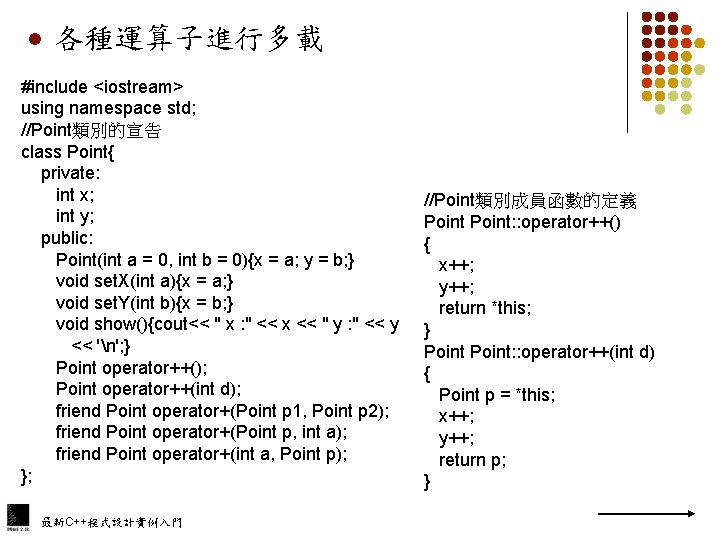
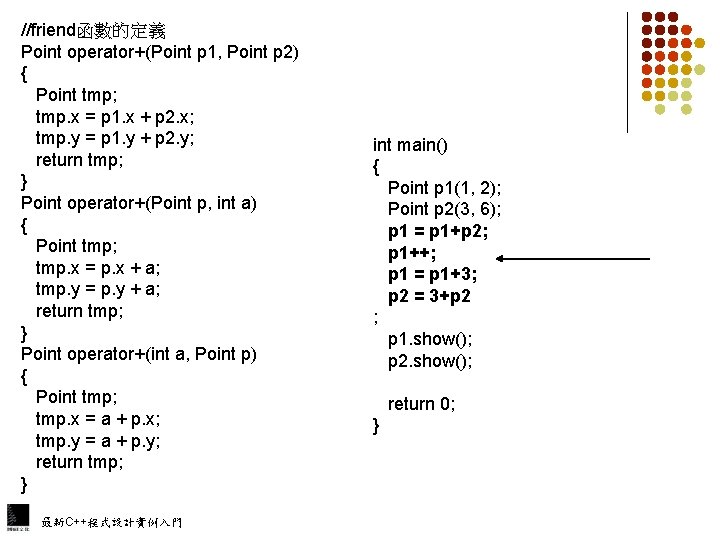
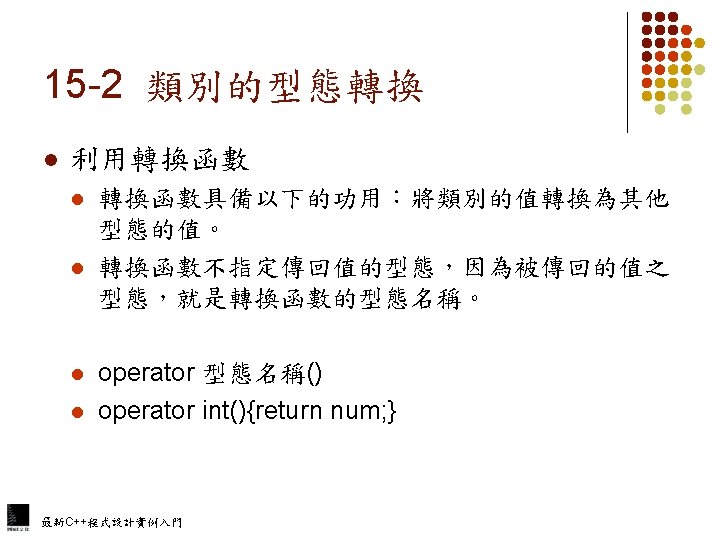
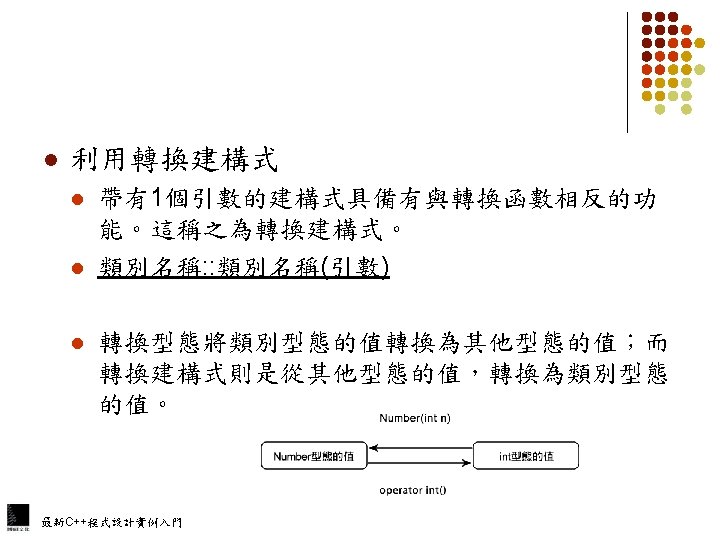
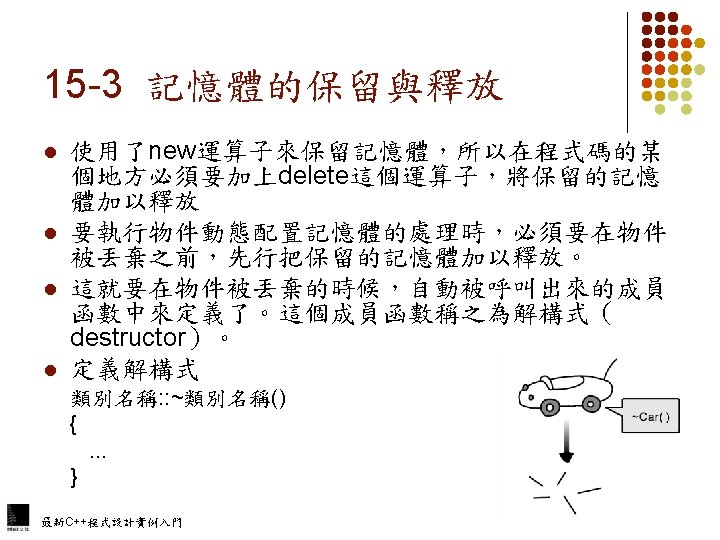
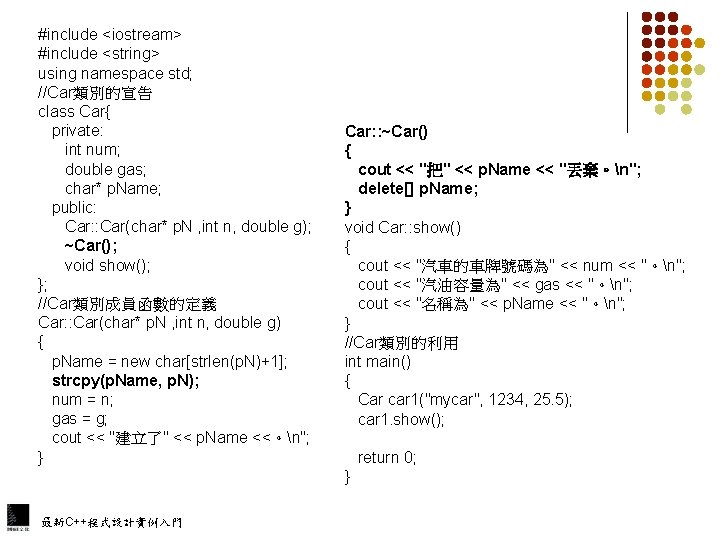
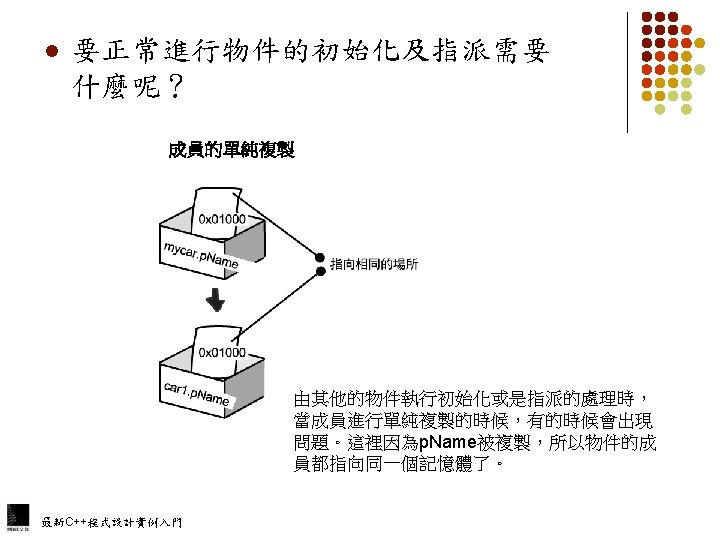
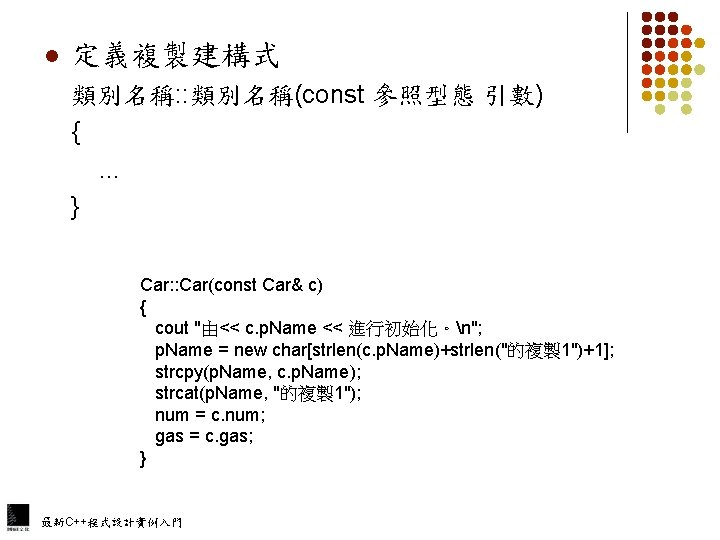
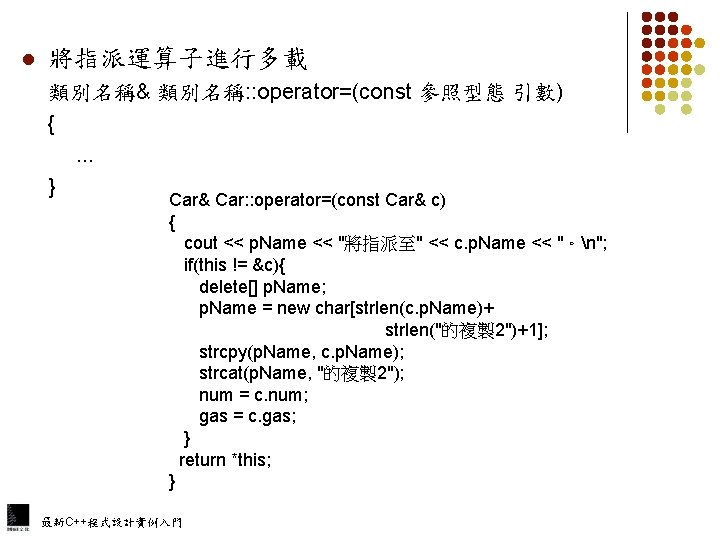
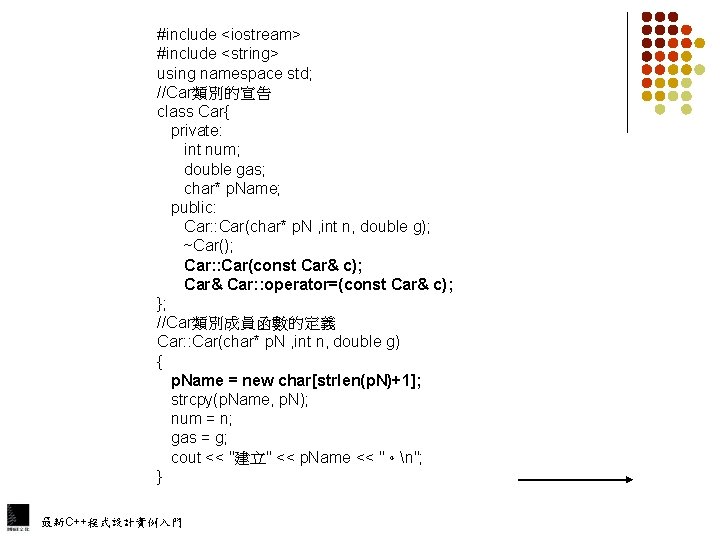
![Car: : ~Car() { cout << "丟棄" << p. Name << "。n"; delete[] p. Car: : ~Car() { cout << "丟棄" << p. Name << "。n"; delete[] p.](https://slidetodoc.com/presentation_image_h2/5c6374a9144499314cf83cae8d80c8d3/image-20.jpg)
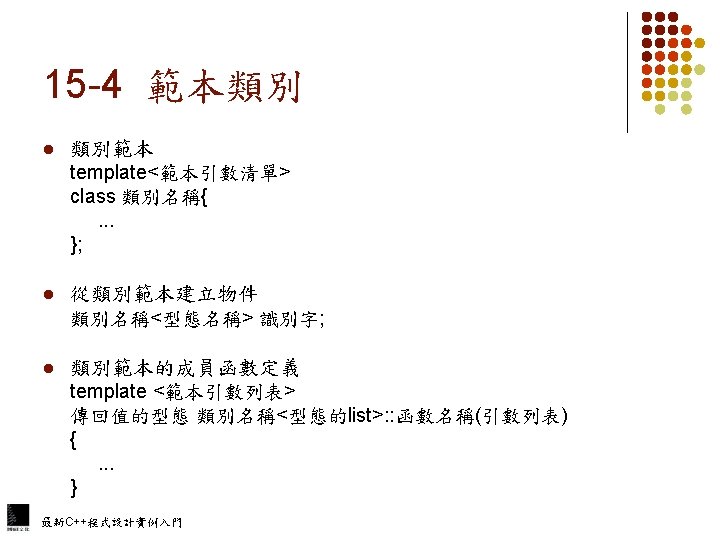
![#include <iostream> using namespace std; //Array類別範本 template <class T> class Array{ private: T data[5]; #include <iostream> using namespace std; //Array類別範本 template <class T> class Array{ private: T data[5];](https://slidetodoc.com/presentation_image_h2/5c6374a9144499314cf83cae8d80c8d3/image-22.jpg)
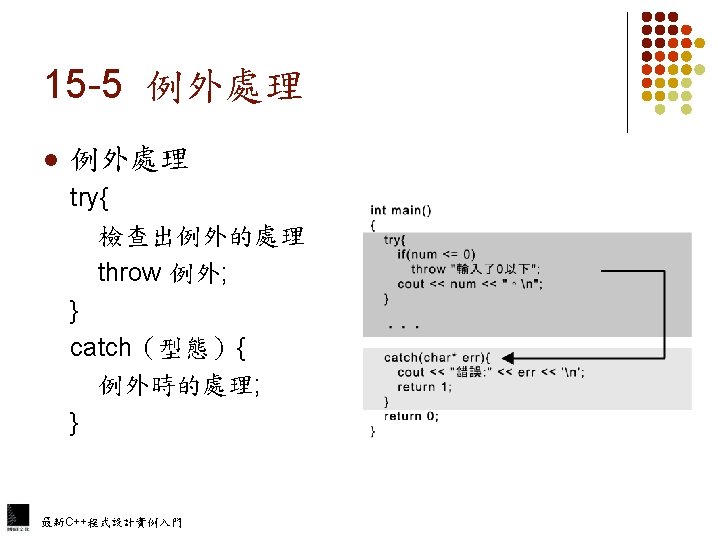
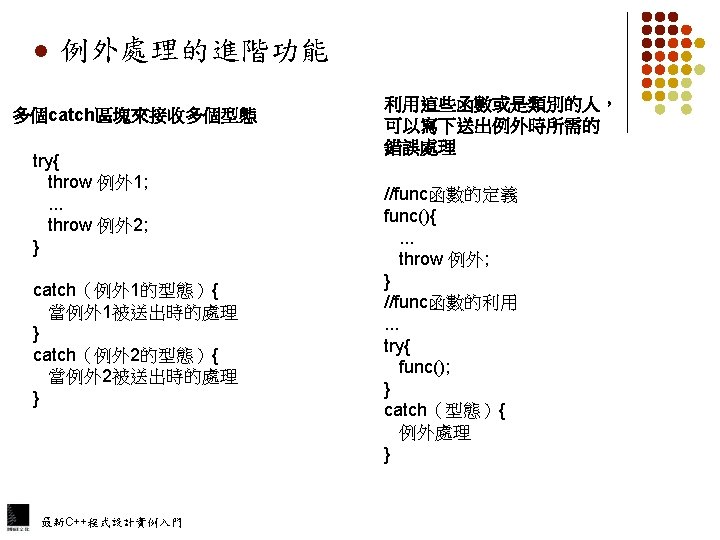
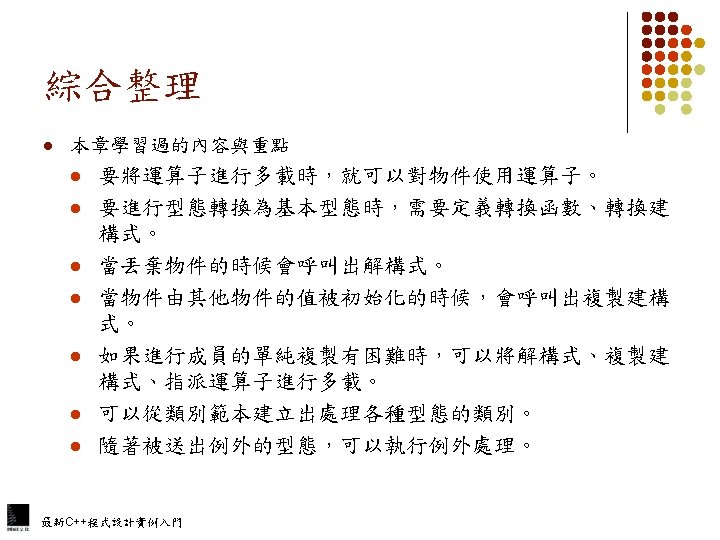
- Slides: 25
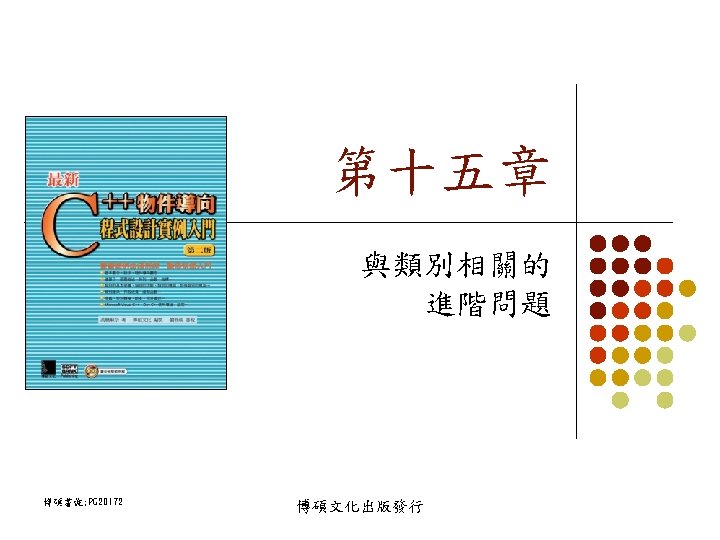
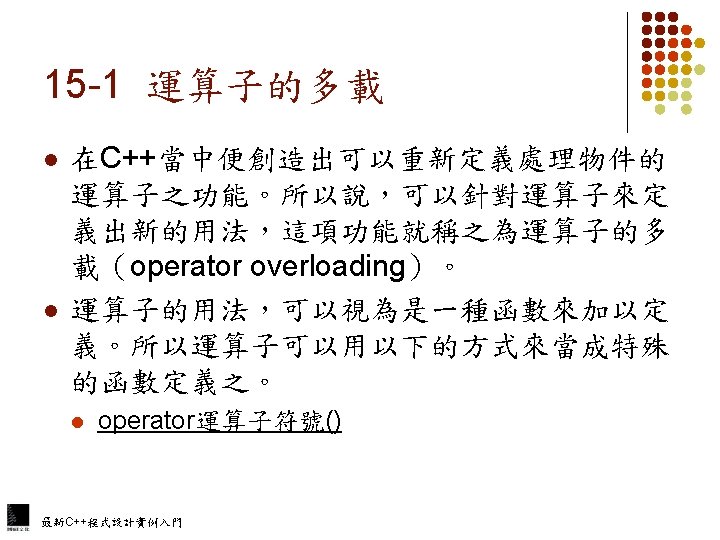
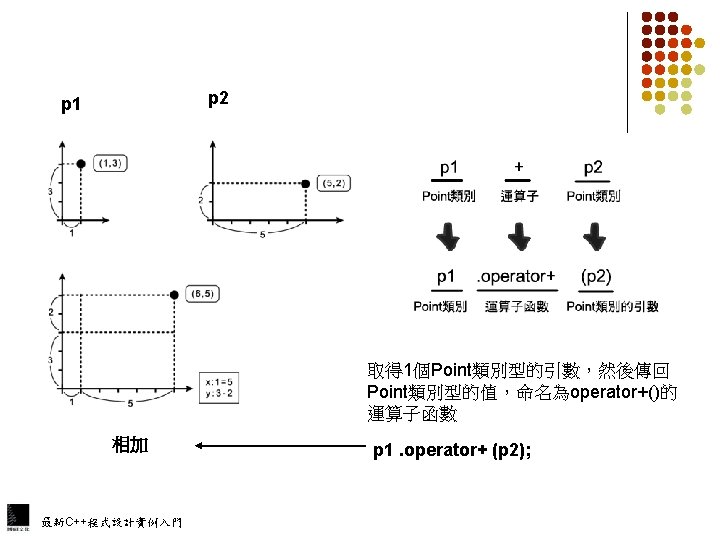
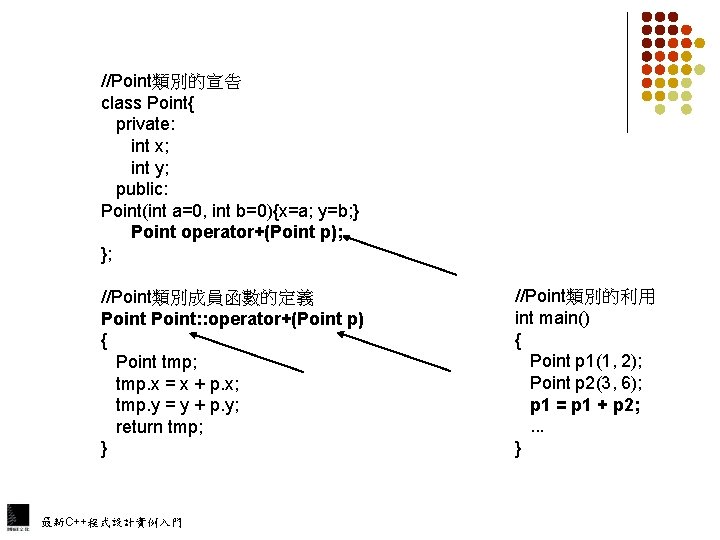
//Point類別的宣告 class Point{ private: int x; int y; public: Point(int a=0, int b=0){x=a; y=b; } Point operator+(Point p); }; //Point類別成員函數的定義 Point: : operator+(Point p) { Point tmp; tmp. x = x + p. x; tmp. y = y + p. y; return tmp; } 最新C++程式設計實例入門 //Point類別的利用 int main() { Point p 1(1, 2); Point p 2(3, 6); p 1 = p 1 + p 2; . . . }
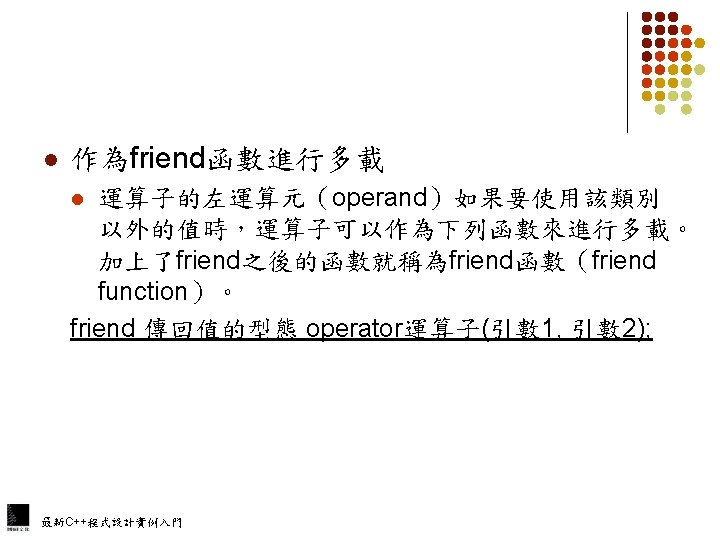
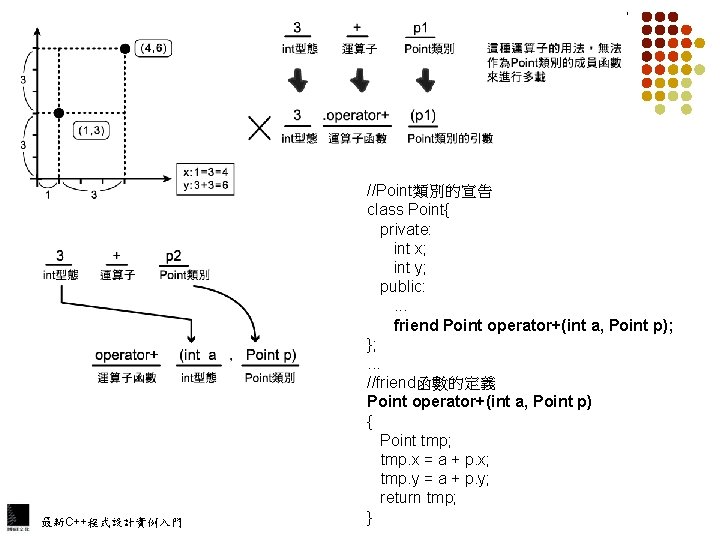
最新C++程式設計實例入門 //Point類別的宣告 class Point{ private: int x; int y; public: . . . friend Point operator+(int a, Point p); }; . . . //friend函數的定義 Point operator+(int a, Point p) { Point tmp; tmp. x = a + p. x; tmp. y = a + p. y; return tmp; }
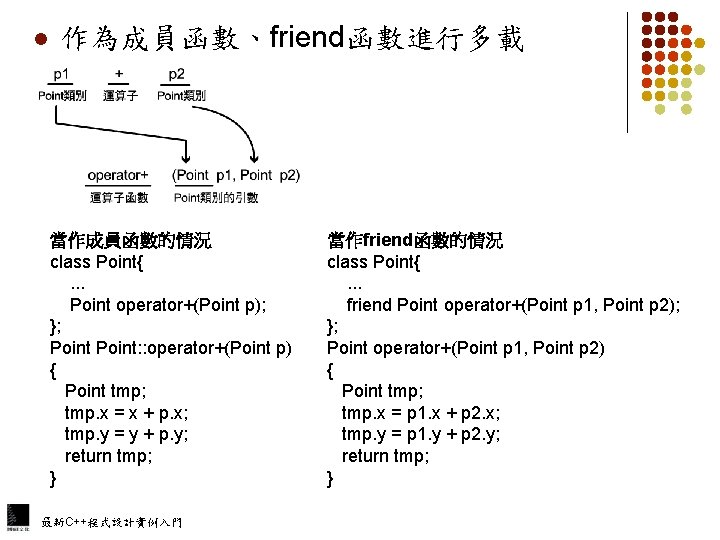
l 作為成員函數、friend函數進行多載 當作成員函數的情況 class Point{. . . Point operator+(Point p); }; Point: : operator+(Point p) { Point tmp; tmp. x = x + p. x; tmp. y = y + p. y; return tmp; } 最新C++程式設計實例入門 當作friend函數的情況 class Point{. . . friend Point operator+(Point p 1, Point p 2); }; Point operator+(Point p 1, Point p 2) { Point tmp; tmp. x = p 1. x + p 2. x; tmp. y = p 1. y + p 2. y; return tmp; }
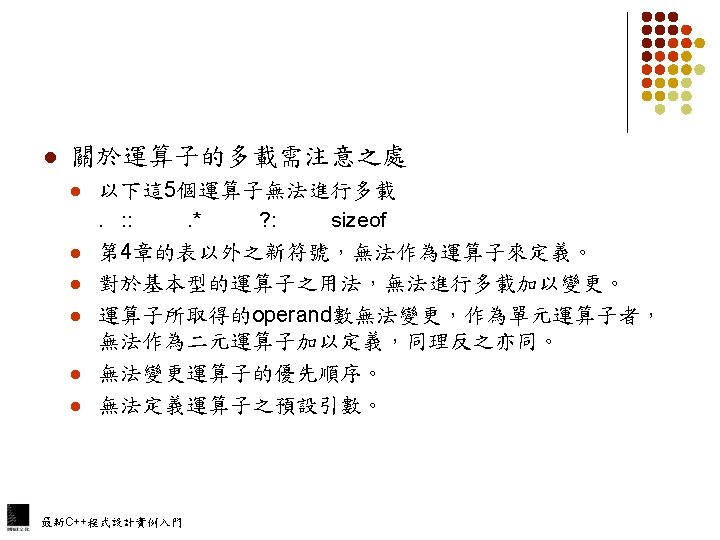
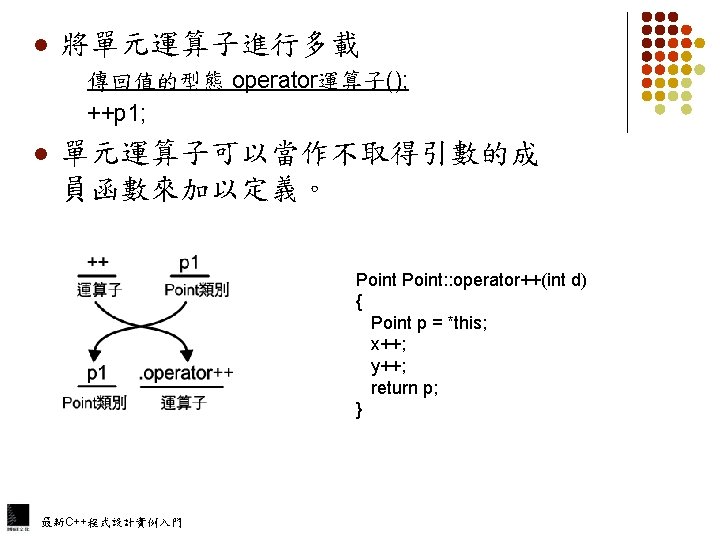
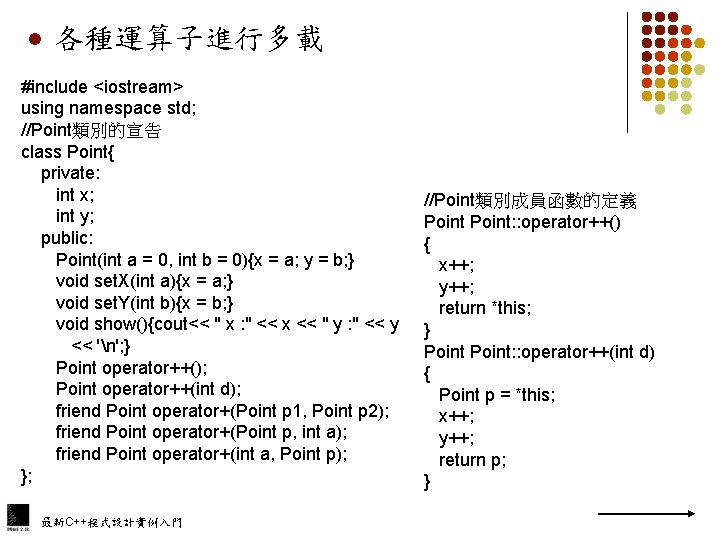
l 各種運算子進行多載 #include <iostream> using namespace std; //Point類別的宣告 class Point{ private: int x; int y; public: Point(int a = 0, int b = 0){x = a; y = b; } void set. X(int a){x = a; } void set. Y(int b){x = b; } void show(){cout<< " x : " << x << " y : " << y << 'n'; } Point operator++(); Point operator++(int d); friend Point operator+(Point p 1, Point p 2); friend Point operator+(Point p, int a); friend Point operator+(int a, Point p); }; 最新C++程式設計實例入門 //Point類別成員函數的定義 Point: : operator++() { x++; y++; return *this; } Point: : operator++(int d) { Point p = *this; x++; y++; return p; }
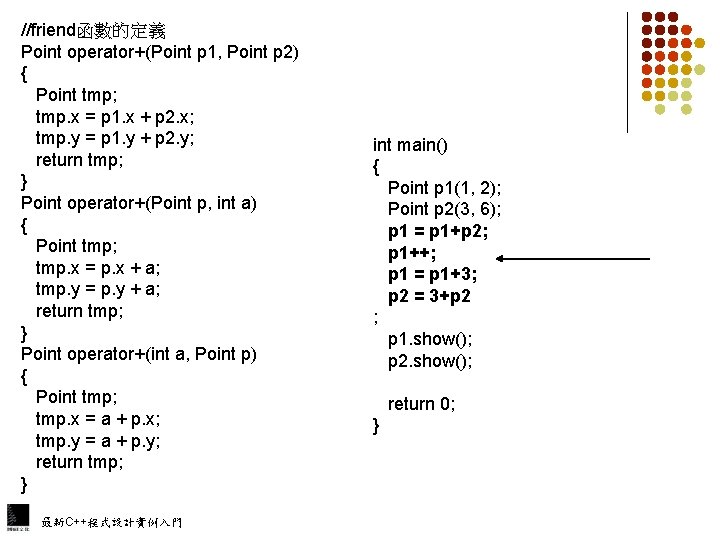
//friend函數的定義 Point operator+(Point p 1, Point p 2) { Point tmp; tmp. x = p 1. x + p 2. x; tmp. y = p 1. y + p 2. y; return tmp; } Point operator+(Point p, int a) { Point tmp; tmp. x = p. x + a; tmp. y = p. y + a; return tmp; } Point operator+(int a, Point p) { Point tmp; tmp. x = a + p. x; tmp. y = a + p. y; return tmp; } 最新C++程式設計實例入門 int main() { Point p 1(1, 2); Point p 2(3, 6); p 1 = p 1+p 2; p 1++; p 1 = p 1+3; p 2 = 3+p 2 ; p 1. show(); p 2. show(); return 0; }
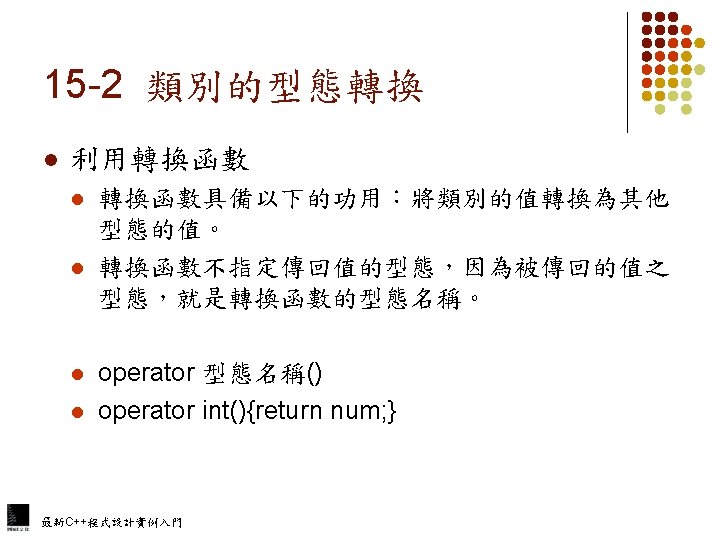
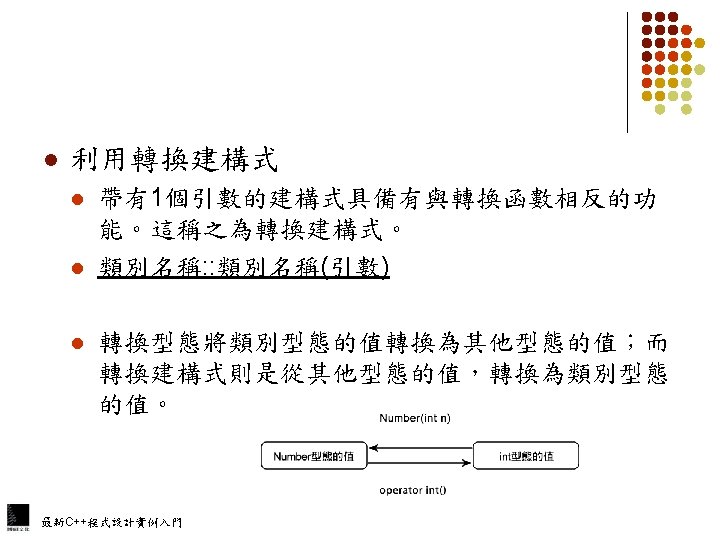
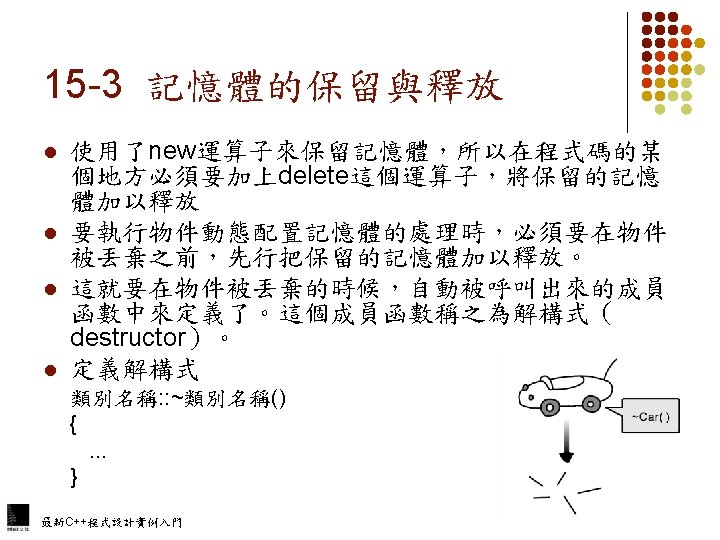
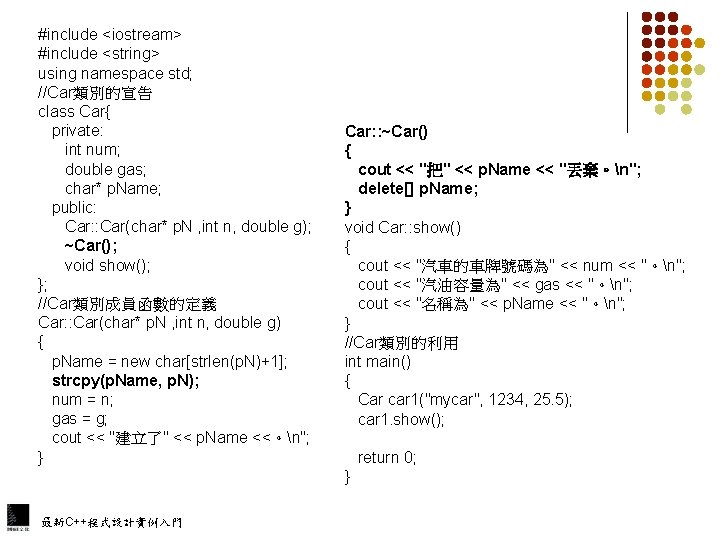
#include <iostream> #include <string> using namespace std; //Car類別的宣告 class Car{ private: int num; double gas; char* p. Name; public: Car: : Car(char* p. N , int n, double g); ~Car(); void show(); }; //Car類別成員函數的定義 Car: : Car(char* p. N , int n, double g) { p. Name = new char[strlen(p. N)+1]; strcpy(p. Name, p. N); num = n; gas = g; cout << "建立了" << p. Name <<。n"; } Car: : ~Car() { cout << "把" << p. Name << "丟棄。n"; delete[] p. Name; } void Car: : show() { cout << "汽車的車牌號碼為" << num << "。n"; cout << "汽油容量為" << gas << "。n"; cout << "名稱為" << p. Name << "。n"; } //Car類別的利用 int main() { Car car 1("mycar", 1234, 25. 5); car 1. show(); return 0; } 最新C++程式設計實例入門
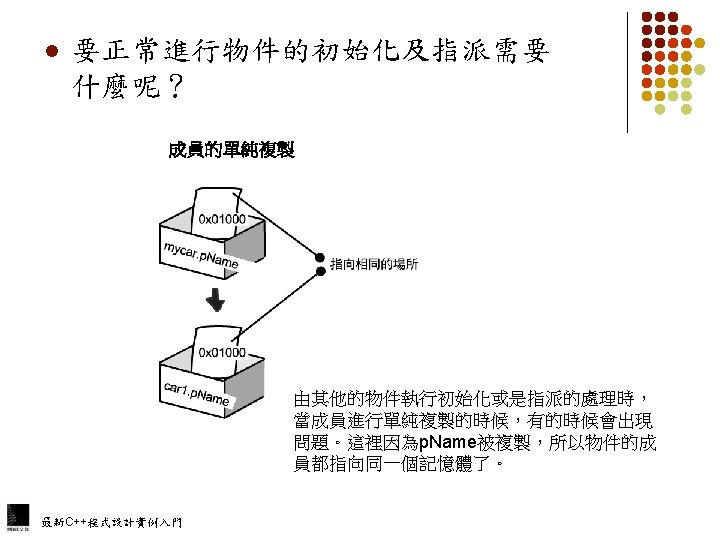
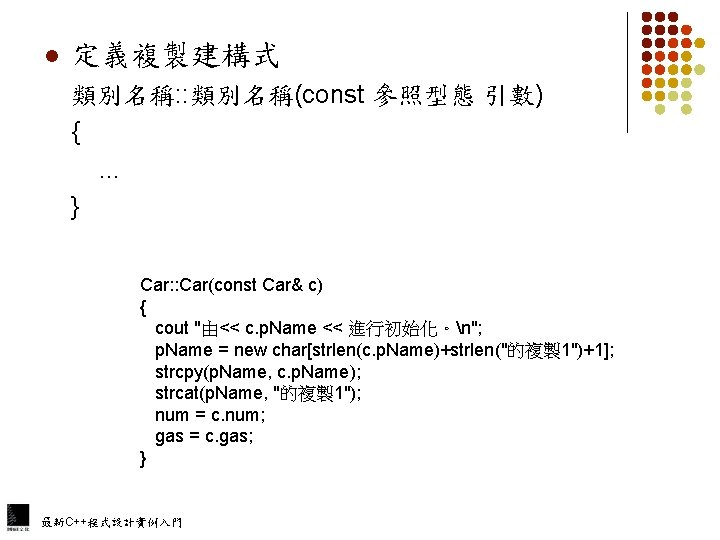
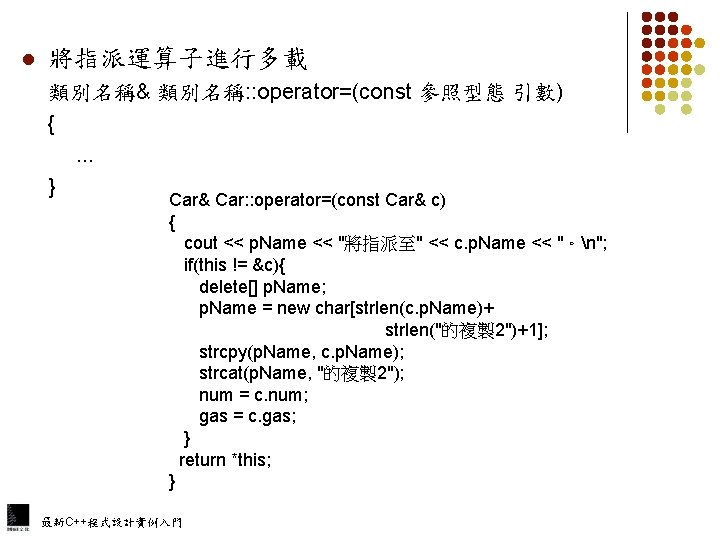
l 將指派運算子進行多載 類別名稱& 類別名稱: : operator=(const 參照型態 引數) {. . . } Car& Car: : operator=(const Car& c) { cout << p. Name << "將指派至" << c. p. Name << "。n"; if(this != &c){ delete[] p. Name; p. Name = new char[strlen(c. p. Name)+ strlen("的複製 2")+1]; strcpy(p. Name, c. p. Name); strcat(p. Name, "的複製 2"); num = c. num; gas = c. gas; } return *this; } 最新C++程式設計實例入門
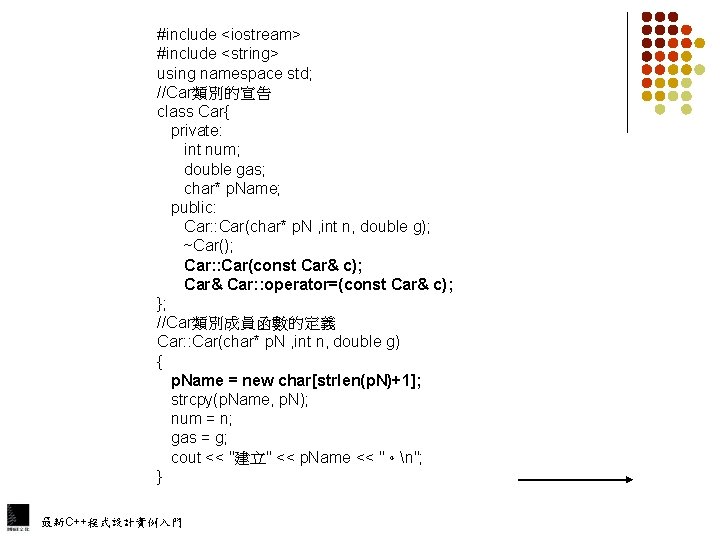
#include <iostream> #include <string> using namespace std; //Car類別的宣告 class Car{ private: int num; double gas; char* p. Name; public: Car: : Car(char* p. N , int n, double g); ~Car(); Car: : Car(const Car& c); Car& Car: : operator=(const Car& c); }; //Car類別成員函數的定義 Car: : Car(char* p. N , int n, double g) { p. Name = new char[strlen(p. N)+1]; strcpy(p. Name, p. N); num = n; gas = g; cout << "建立" << p. Name << "。n"; } 最新C++程式設計實例入門
![Car Car cout 丟棄 p Name n delete p Car: : ~Car() { cout << "丟棄" << p. Name << "。n"; delete[] p.](https://slidetodoc.com/presentation_image_h2/5c6374a9144499314cf83cae8d80c8d3/image-20.jpg)
Car: : ~Car() { cout << "丟棄" << p. Name << "。n"; delete[] p. Name; } Car: : Car(const Car& c) { cout << "用" << c. p. Name << "進行初始化。n"; p. Name = new char[strlen(c. p. Name) + strlen("的複製 1")+1]; strcpy(p. Name, c. p. Name); strcat(p. Name, "的複製 1"); num = c. num; gas = c. gas; } Car& Car: : operator=(const Car& c) { cout << "把" << p. Name << "指派給" << c. p. Name << "。n"; if(this != &c){ delete[] p. Name; p. Name = new char[strlen(c. p. Name)+strlen("的複製 2")+1]; strcpy(p. Name, c. p. Name); strcat(p. Name, "的複製 2"); num = c. num; gas = c. gas; } return *this; } 最新C++程式設計實例入門 int main() { Car mycar("mycar", 1234, 25. 5); Car car 1 = mycar; Car car 2("car 2", 0, 0); car 2 = mycar; return 0; }
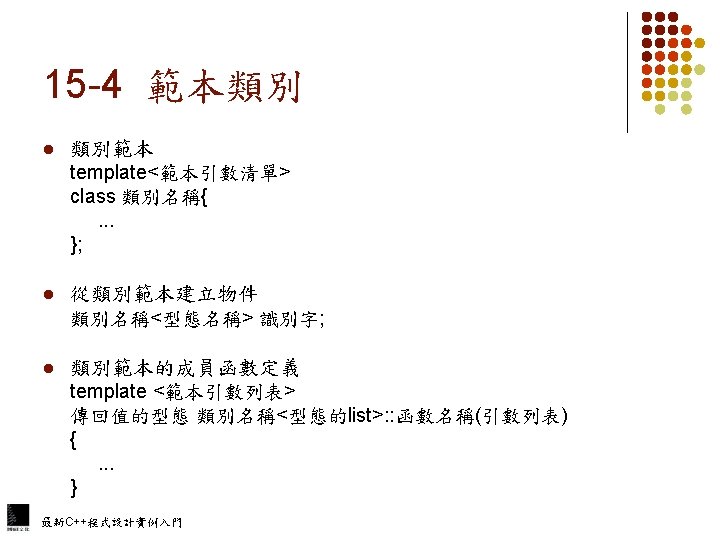
![include iostream using namespace std Array類別範本 template class T class Array private T data5 #include <iostream> using namespace std; //Array類別範本 template <class T> class Array{ private: T data[5];](https://slidetodoc.com/presentation_image_h2/5c6374a9144499314cf83cae8d80c8d3/image-22.jpg)
#include <iostream> using namespace std; //Array類別範本 template <class T> class Array{ private: T data[5]; public: void set. Data(int num, T d); T get. Data(int num); }; template <class T> void Array<T>: : set. Data(int num, T d) { if(num < 0 || num > 4 ) cout << "超過陣列的範圍。n"; else data[num] = d; } template <class T> T Array<T>: : get. Data(int num) { if(num < 0 || num > 4 ){ cout << "超過陣列的範圍。n"; return data[0]; } else return data[num]; } 最新C++程式設計實例入門 int main() { cout << "建立int型態的陣列。n"; Array<int> i_array; i_array. set. Data(0, 80); i_array. set. Data(1, 60); i_array. set. Data(2, 58); i_array. set. Data(3, 77); i_array. set. Data(4, 57); for(int i=0; i<5; i++) cout << i_array. get. Data(i) << 'n'; cout << "建立double型態的陣列。n"; Array<double> d_array; d_array. set. Data(0, 35. 5); d_array. set. Data(1, 45. 6); d_array. set. Data(2, 26. 8); d_array. set. Data(3, 76. 2); d_array. set. Data(4, 85. 5); for(int j=0; j<5; j++) cout << d_array. get. Data(j) << 'n'; return 0; }
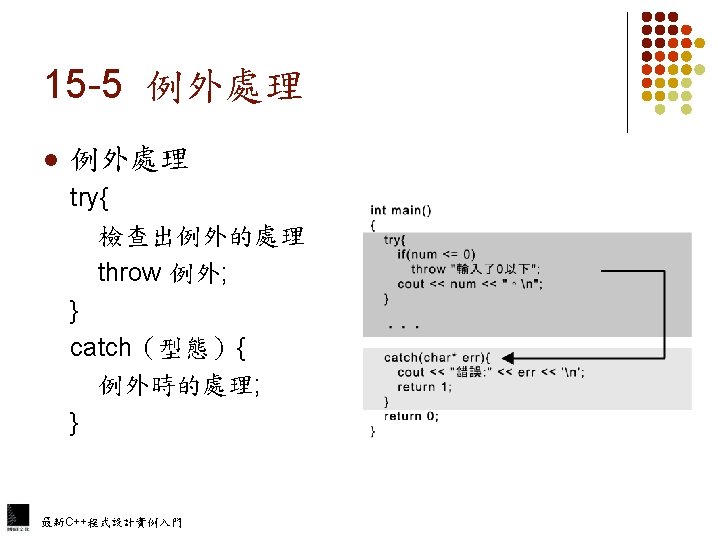
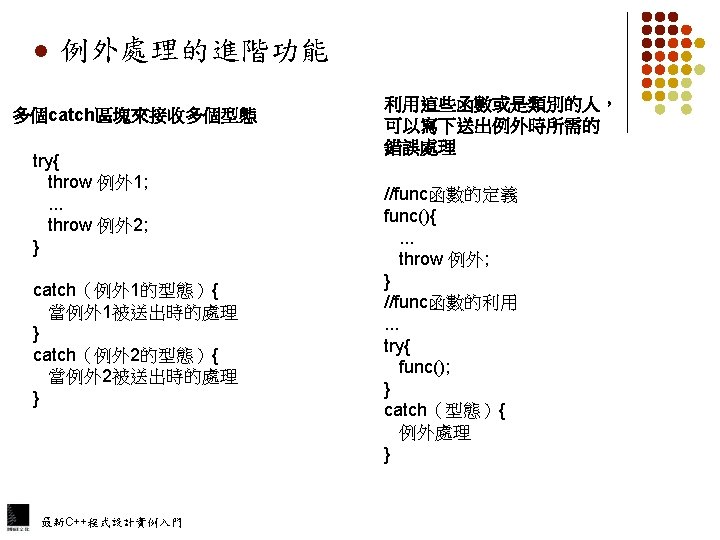
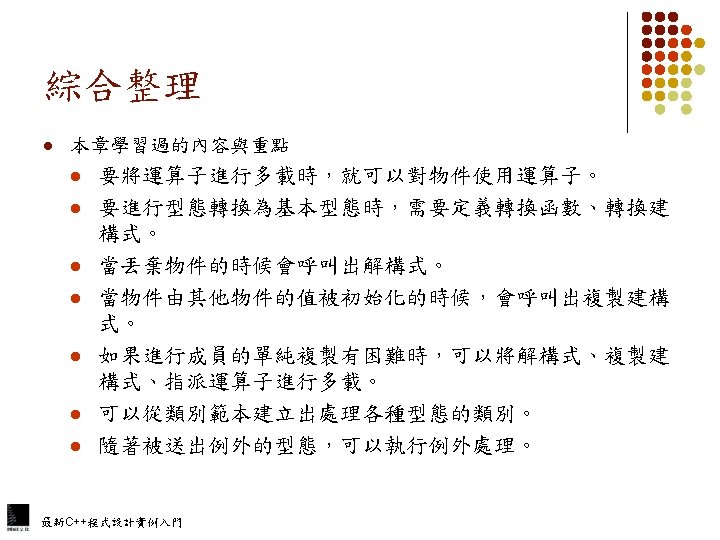