x 86 Programming Computer Architecture CMP Instruction CMP
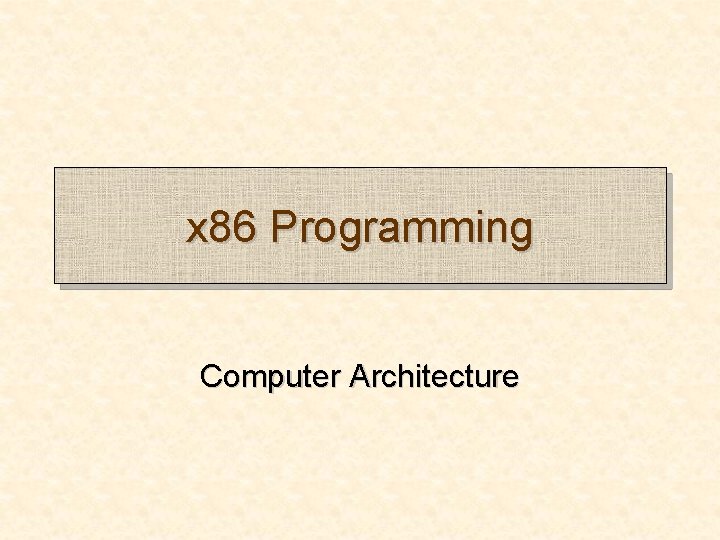
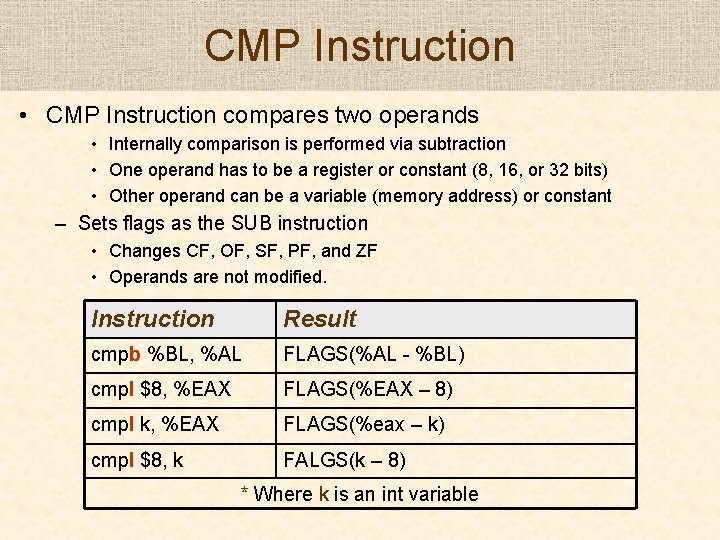
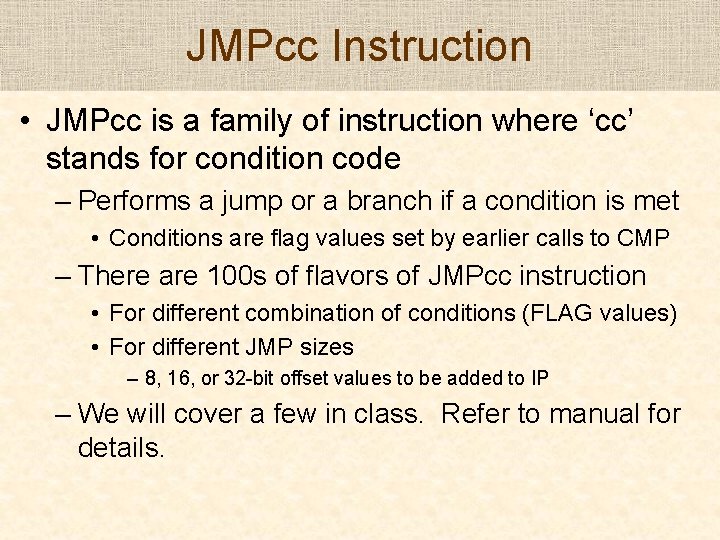
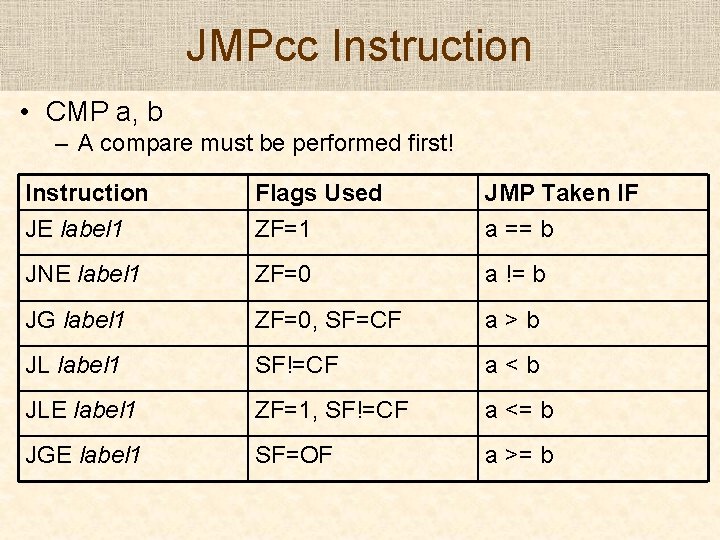
![Example Usage • Convert the following Java code to assembly public static main(String[] args) Example Usage • Convert the following Java code to assembly public static main(String[] args)](https://slidetodoc.com/presentation_image_h2/08e94e9c73abe1514048b17620740334/image-5.jpg)
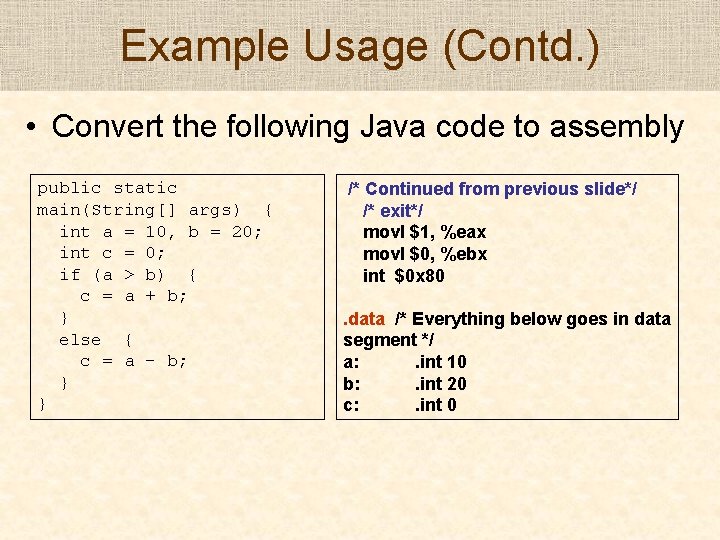
- Slides: 6
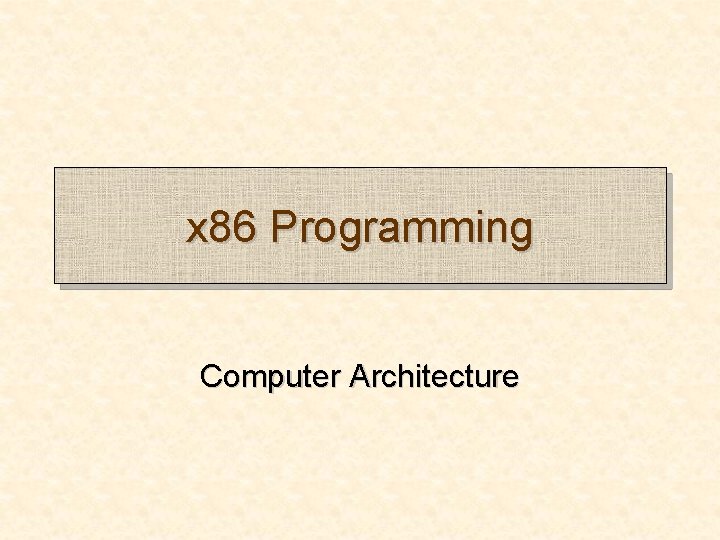
x 86 Programming Computer Architecture
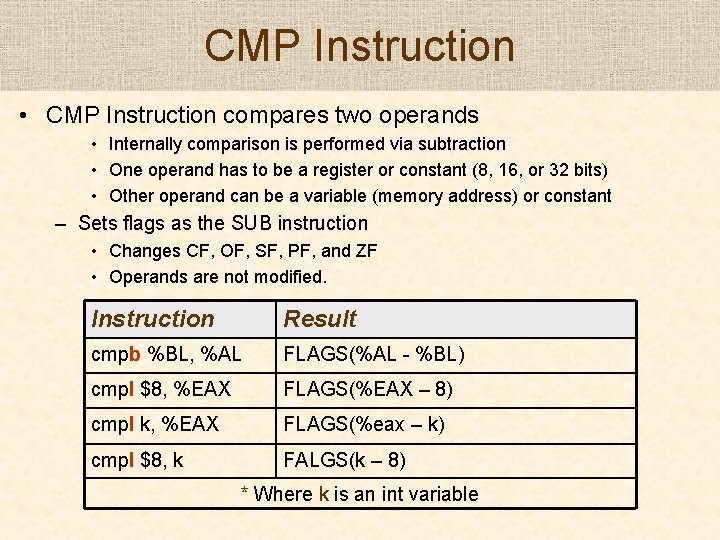
CMP Instruction • CMP Instruction compares two operands • Internally comparison is performed via subtraction • One operand has to be a register or constant (8, 16, or 32 bits) • Other operand can be a variable (memory address) or constant – Sets flags as the SUB instruction • Changes CF, OF, SF, PF, and ZF • Operands are not modified. Instruction Result cmpb %BL, %AL FLAGS(%AL - %BL) cmpl $8, %EAX FLAGS(%EAX – 8) cmpl k, %EAX FLAGS(%eax – k) cmpl $8, k FALGS(k – 8) * Where k is an int variable
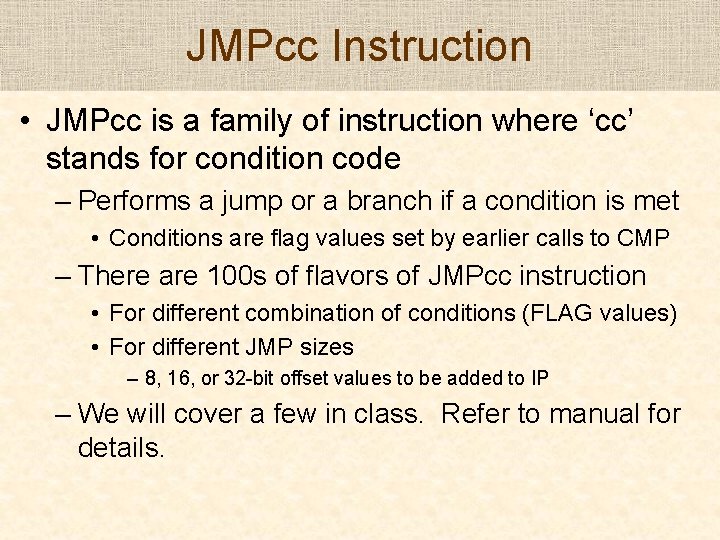
JMPcc Instruction • JMPcc is a family of instruction where ‘cc’ stands for condition code – Performs a jump or a branch if a condition is met • Conditions are flag values set by earlier calls to CMP – There are 100 s of flavors of JMPcc instruction • For different combination of conditions (FLAG values) • For different JMP sizes – 8, 16, or 32 -bit offset values to be added to IP – We will cover a few in class. Refer to manual for details.
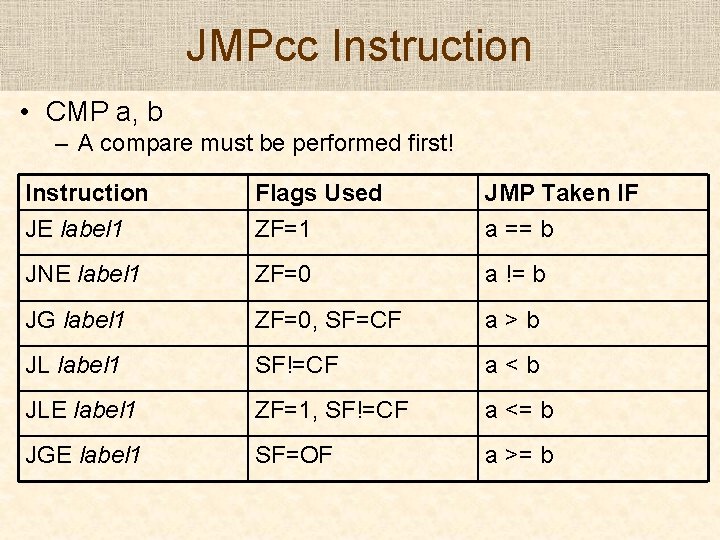
JMPcc Instruction • CMP a, b – A compare must be performed first! Instruction JE label 1 Flags Used ZF=1 JMP Taken IF a == b JNE label 1 ZF=0 a != b JG label 1 ZF=0, SF=CF a>b JL label 1 SF!=CF a<b JLE label 1 ZF=1, SF!=CF a <= b JGE label 1 SF=OF a >= b
![Example Usage Convert the following Java code to assembly public static mainString args Example Usage • Convert the following Java code to assembly public static main(String[] args)](https://slidetodoc.com/presentation_image_h2/08e94e9c73abe1514048b17620740334/image-5.jpg)
Example Usage • Convert the following Java code to assembly public static main(String[] args) { int a = 10, b = 20; int c = 0; if (a > b) { c = a + b; } else { c = a – b; } } . text /* Every thing below goes in Code Segment */. global _start /* Global entry point into program */ _start: /* Indicate where program starts */ movl a, %eax /* eax = a */ cmpl b, %eax /* Set Flags */ JG if. Part /* a > b */ subl b, %eax /* eax -= b */ jmp end. IF /* Done with else */ if. Part: addl b, %eax /* eax += b */ end. IF: movl %eax, c /* c = eax */
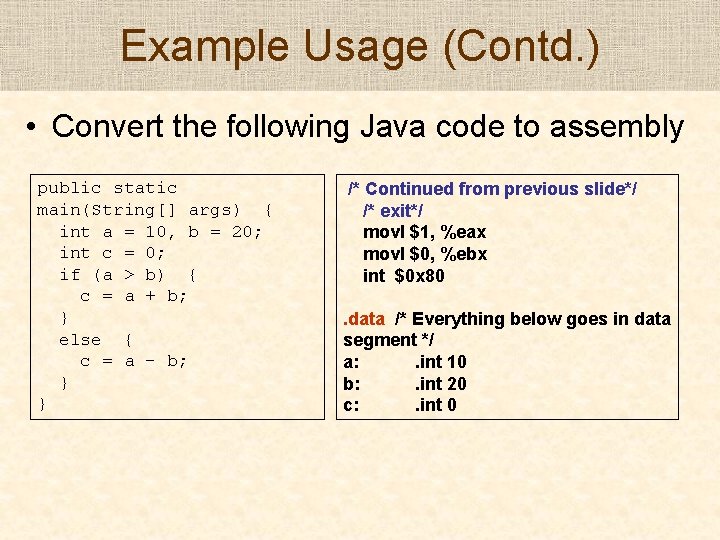
Example Usage (Contd. ) • Convert the following Java code to assembly public static main(String[] args) { int a = 10, b = 20; int c = 0; if (a > b) { c = a + b; } else { c = a – b; } } /* Continued from previous slide*/ /* exit*/ movl $1, %eax movl $0, %ebx int $0 x 80. data /* Everything below goes in data segment */ a: . int 10 b: . int 20 c: . int 0