The Controller Carol Wolf Computer Science Rails generate
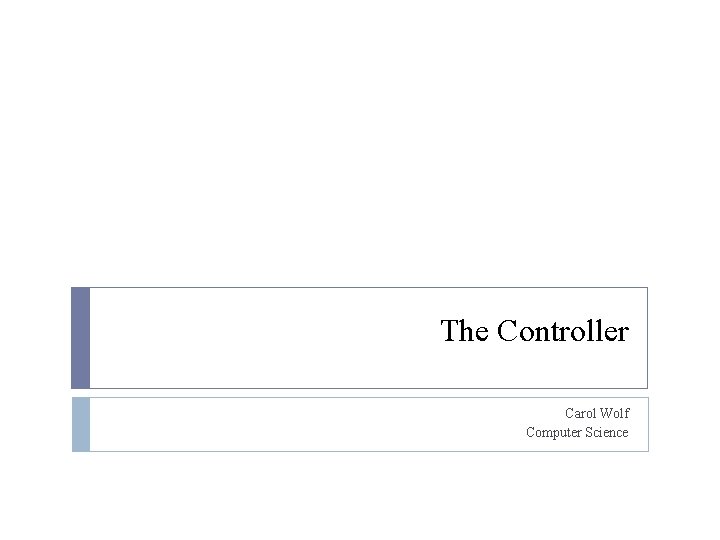
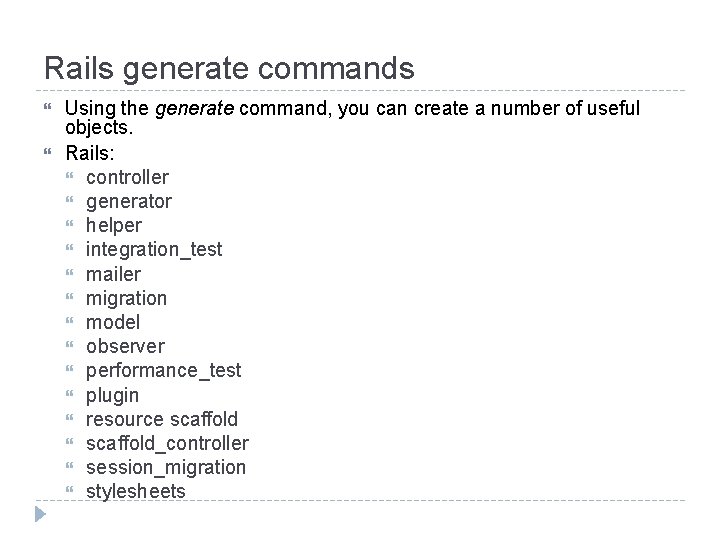
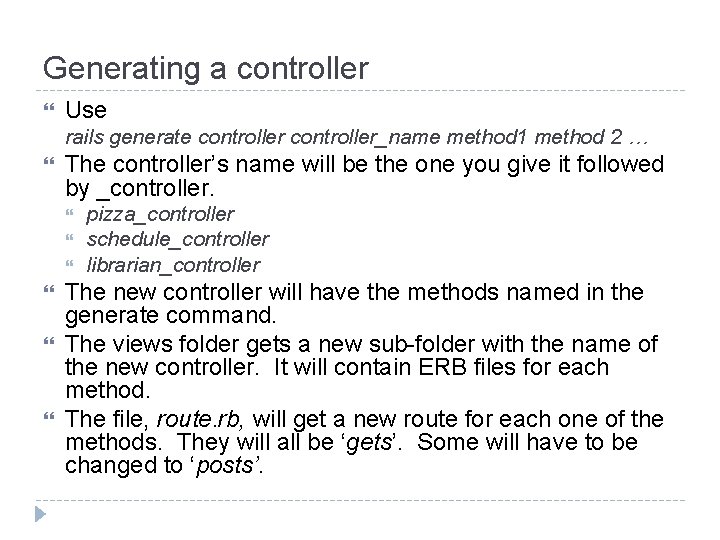
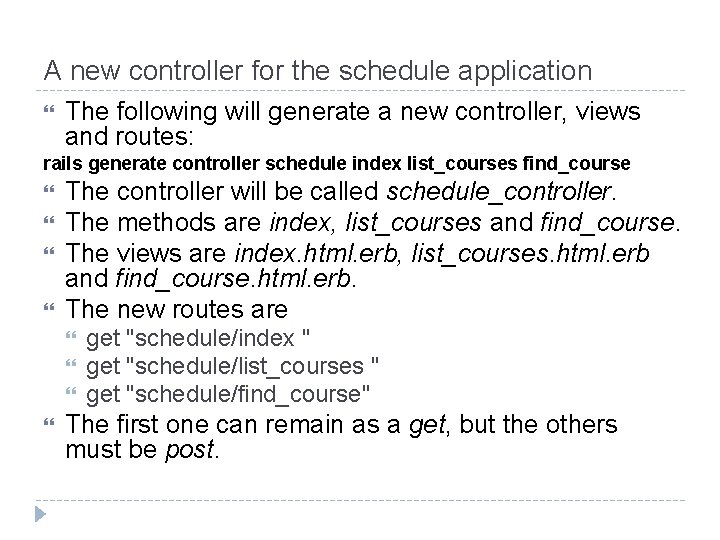
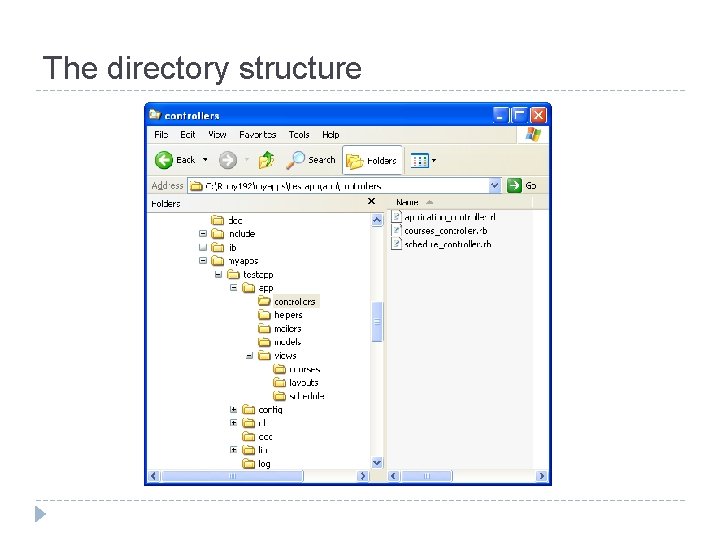
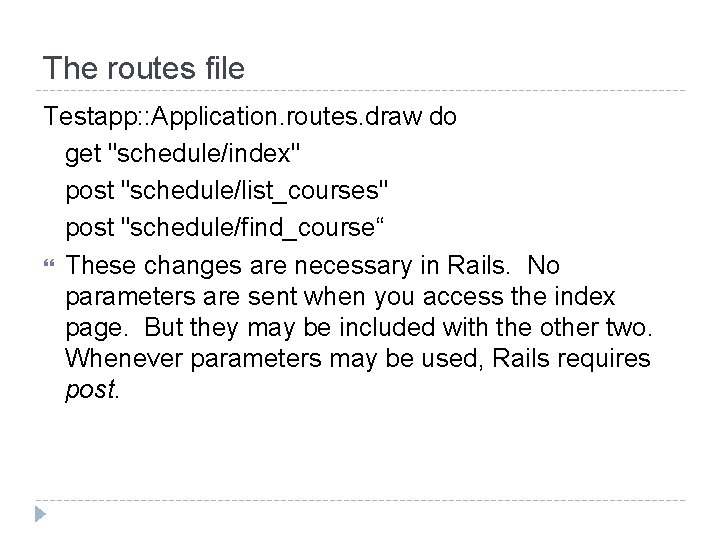
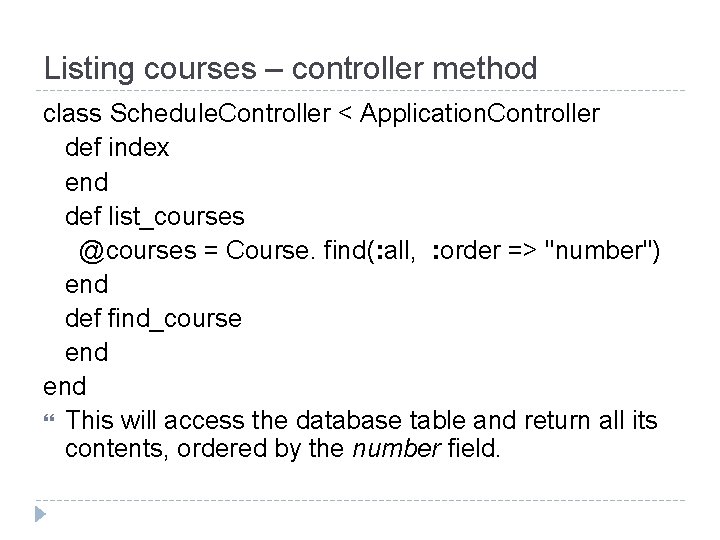
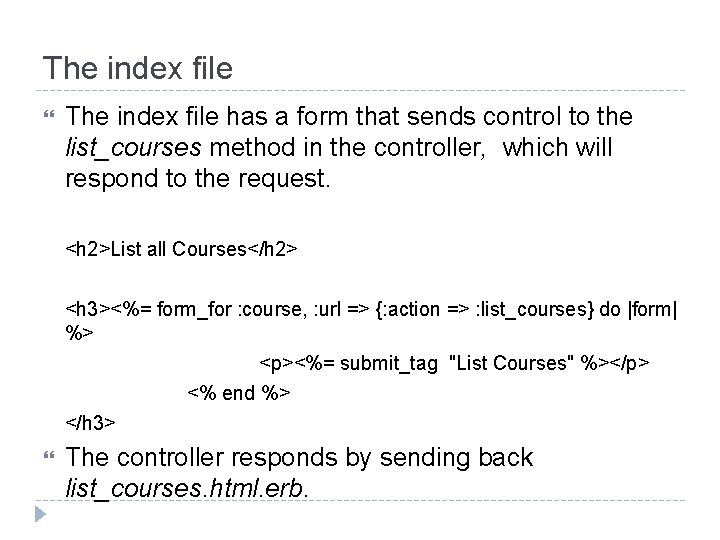
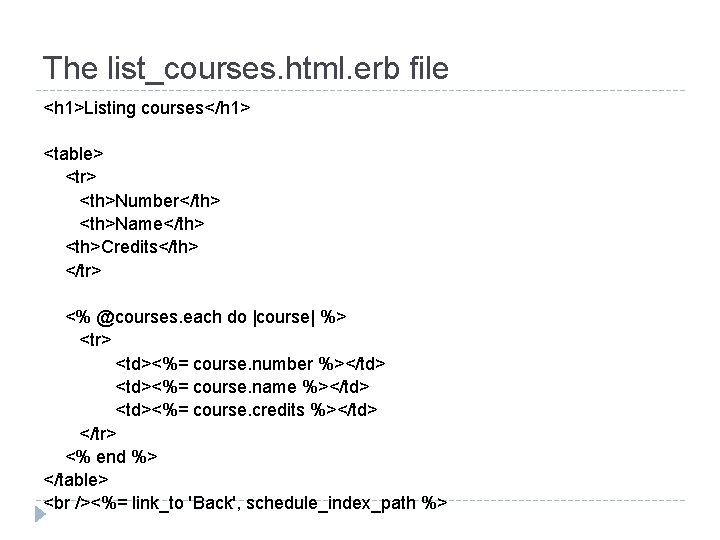
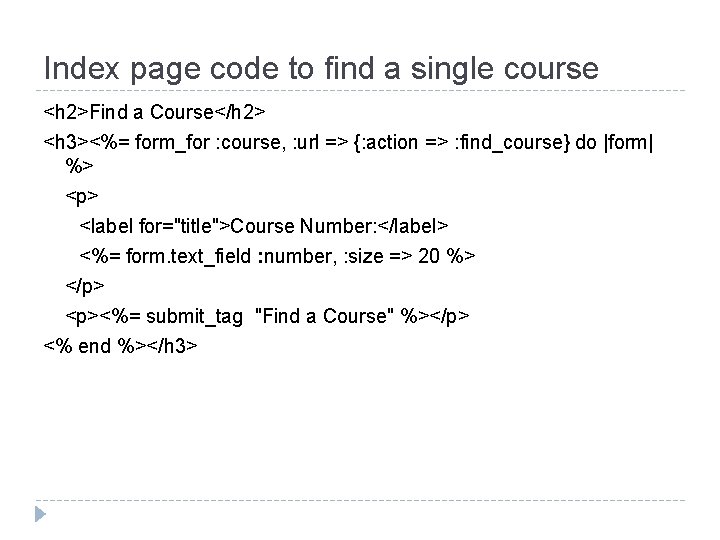
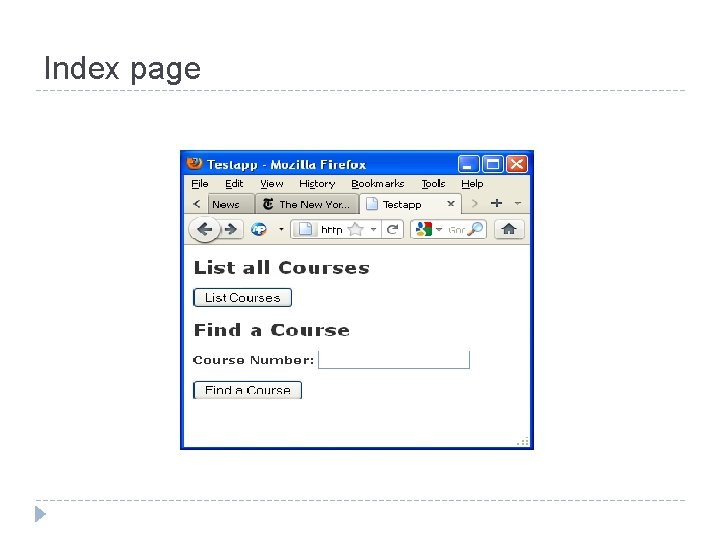
![Controller code for finding a course def find_course @params = params[: course] @course = Controller code for finding a course def find_course @params = params[: course] @course =](https://slidetodoc.com/presentation_image/db6792e8ec90955aa541cdba4263b3da/image-12.jpg)
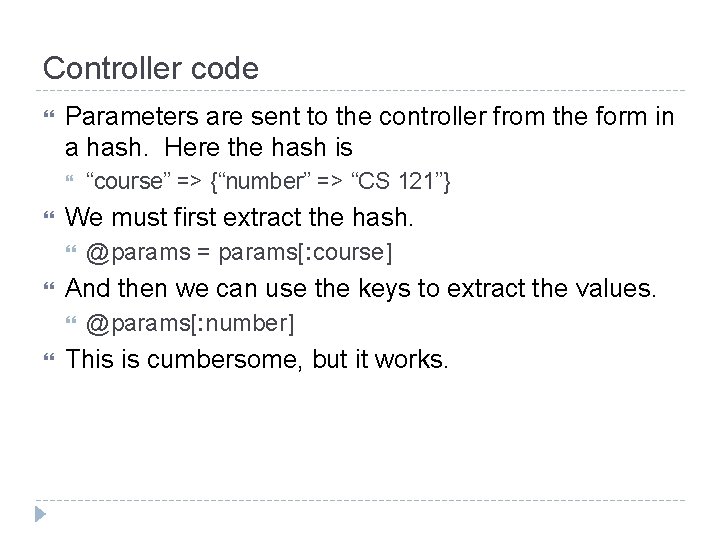
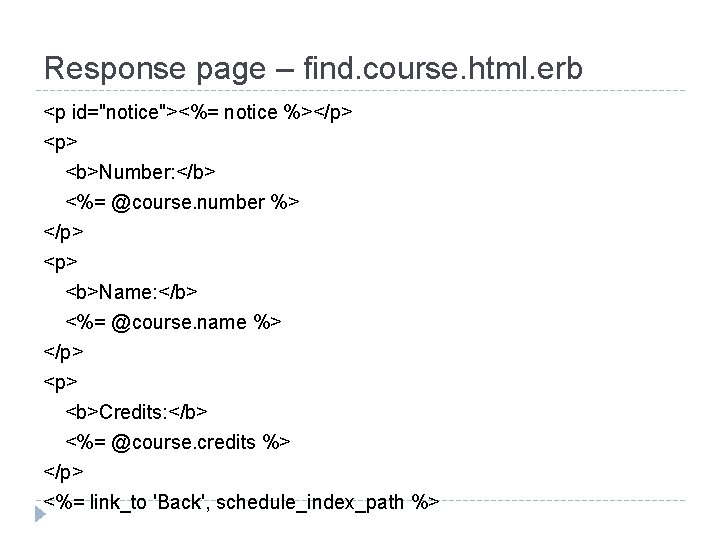
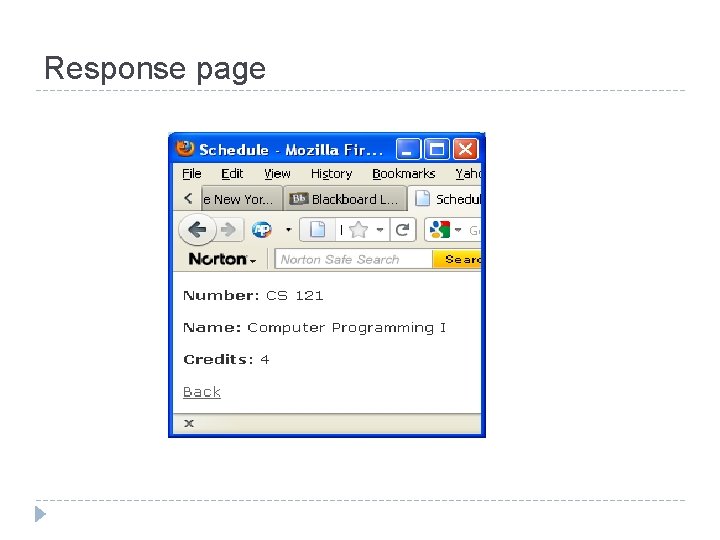
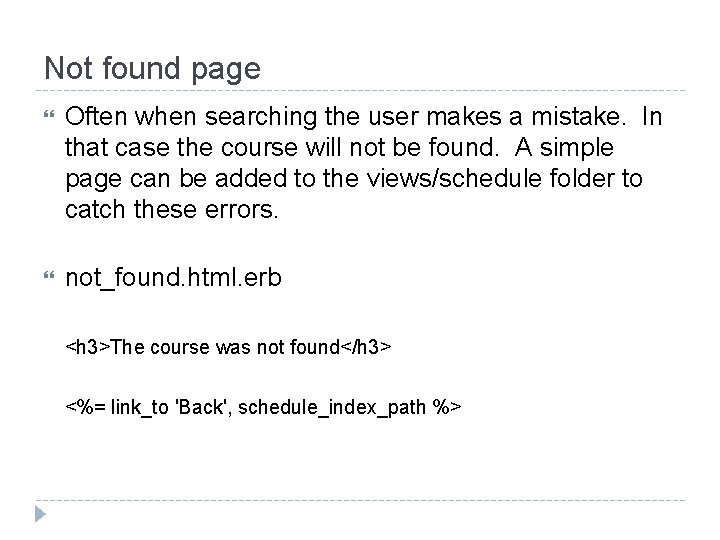
- Slides: 16
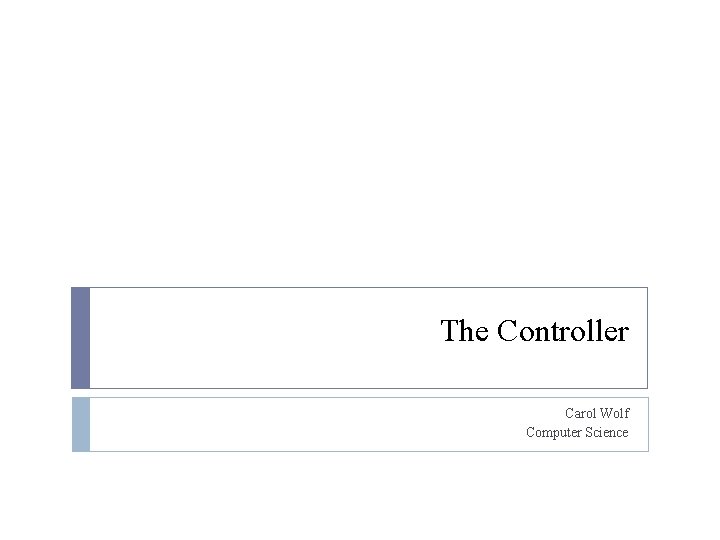
The Controller Carol Wolf Computer Science
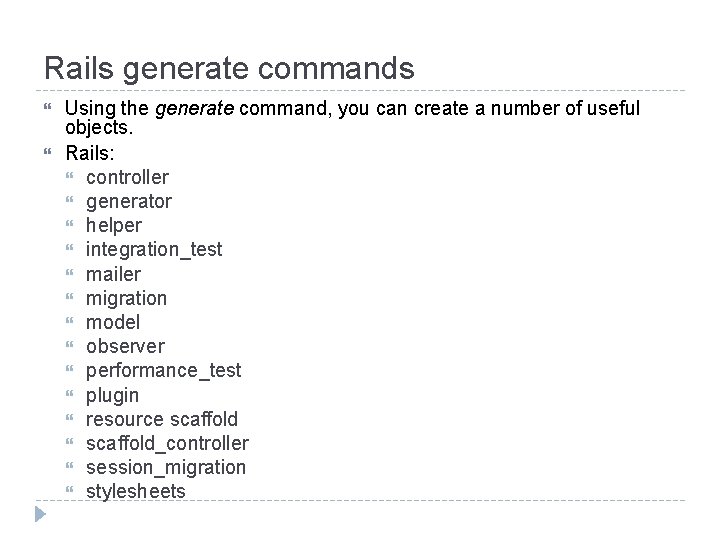
Rails generate commands Using the generate command, you can create a number of useful objects. Rails: controller generator helper integration_test mailer migration model observer performance_test plugin resource scaffold_controller session_migration stylesheets
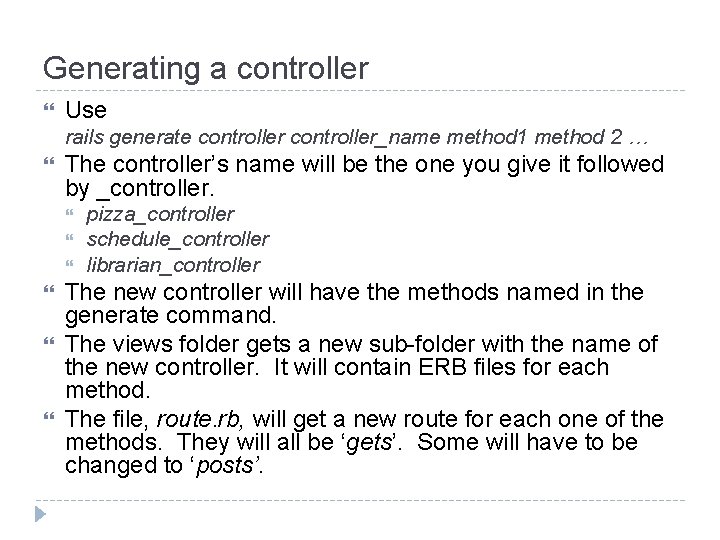
Generating a controller Use rails generate controller_name method 1 method 2 … The controller’s name will be the one you give it followed by _controller. pizza_controller schedule_controller librarian_controller The new controller will have the methods named in the generate command. The views folder gets a new sub-folder with the name of the new controller. It will contain ERB files for each method. The file, route. rb, will get a new route for each one of the methods. They will all be ‘gets’. Some will have to be changed to ‘posts’.
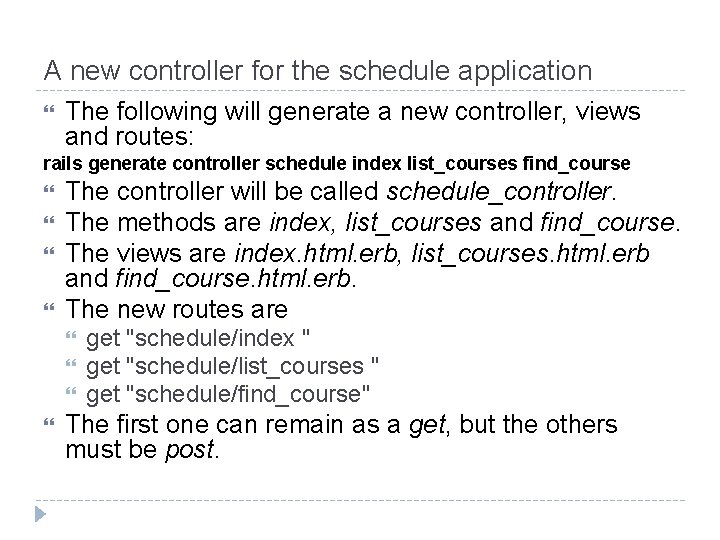
A new controller for the schedule application The following will generate a new controller, views and routes: rails generate controller schedule index list_courses find_course The controller will be called schedule_controller. The methods are index, list_courses and find_course. The views are index. html. erb, list_courses. html. erb and find_course. html. erb. The new routes are get "schedule/index " get "schedule/list_courses " get "schedule/find_course" The first one can remain as a get, but the others must be post.
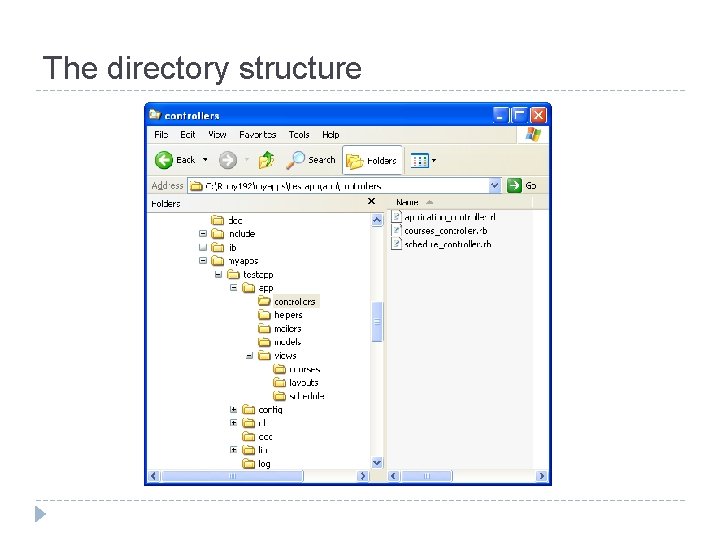
The directory structure
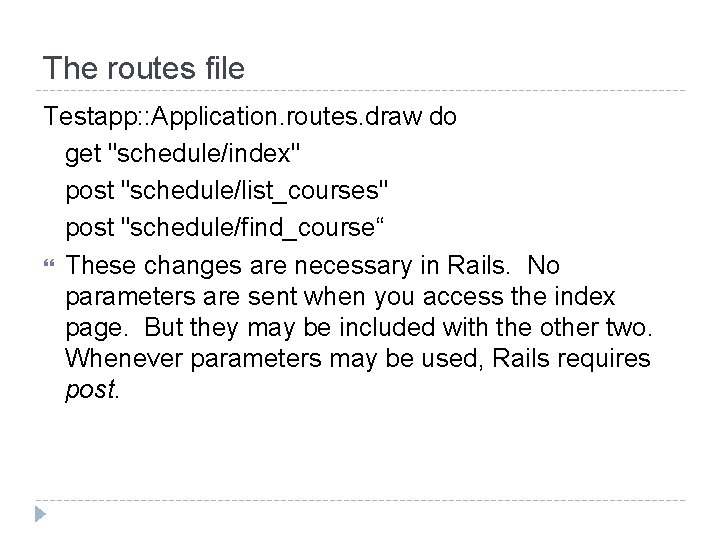
The routes file Testapp: : Application. routes. draw do get "schedule/index" post "schedule/list_courses" post "schedule/find_course“ These changes are necessary in Rails. No parameters are sent when you access the index page. But they may be included with the other two. Whenever parameters may be used, Rails requires post.
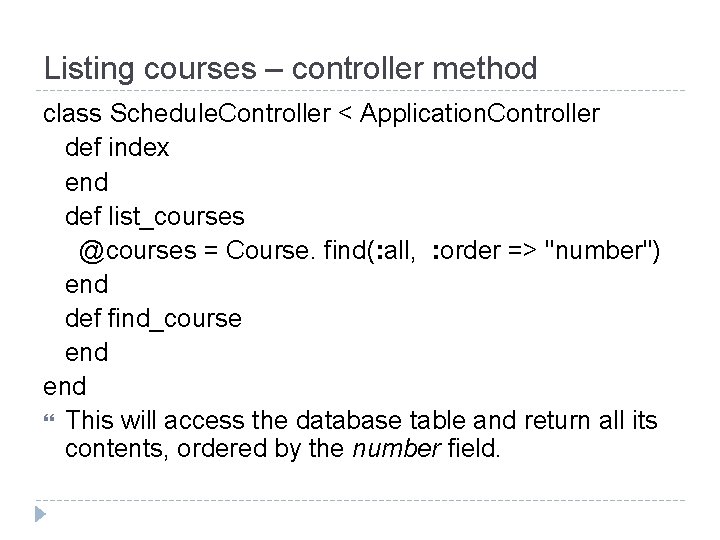
Listing courses – controller method class Schedule. Controller < Application. Controller def index end def list_courses @courses = Course. find(: all, : order => "number") end def find_course end This will access the database table and return all its contents, ordered by the number field.
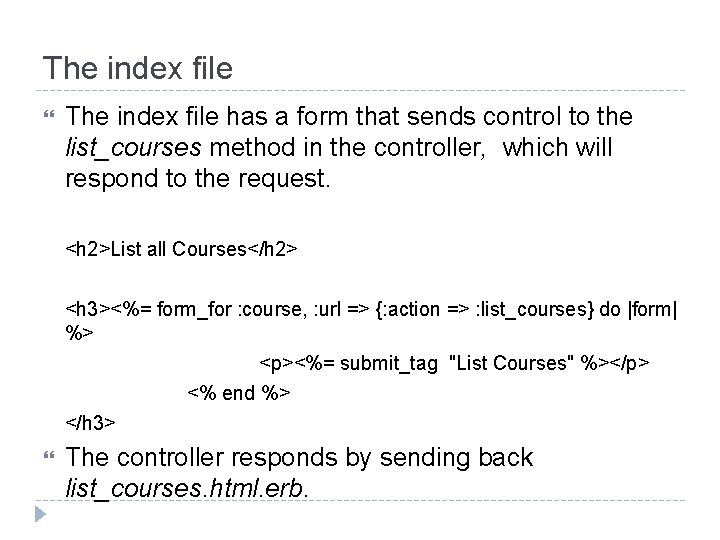
The index file has a form that sends control to the list_courses method in the controller, which will respond to the request. <h 2>List all Courses</h 2> <h 3><%= form_for : course, : url => {: action => : list_courses} do |form| %> <p><%= submit_tag "List Courses" %></p> <% end %> </h 3> The controller responds by sending back list_courses. html. erb.
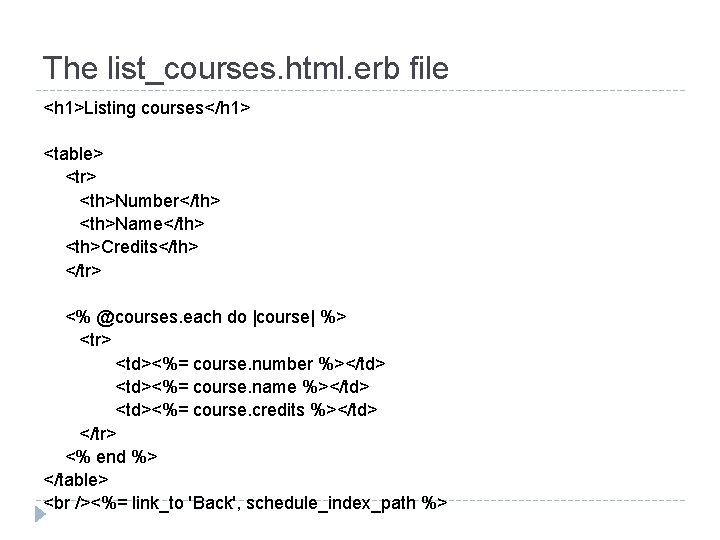
The list_courses. html. erb file <h 1>Listing courses</h 1> <table> <tr> <th>Number</th> <th>Name</th> <th>Credits</th> </tr> <% @courses. each do |course| %> <tr> <td><%= course. number %></td> <td><%= course. name %></td> <td><%= course. credits %></td> </tr> <% end %> </table> <br /><%= link_to 'Back', schedule_index_path %>
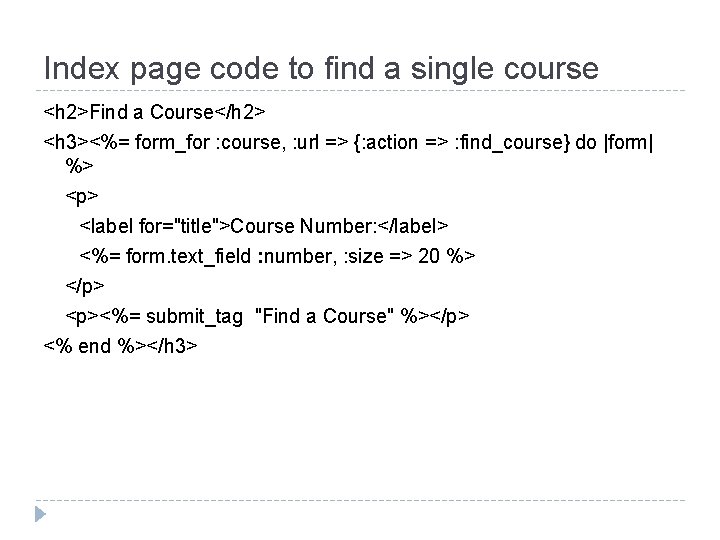
Index page code to find a single course <h 2>Find a Course</h 2> <h 3><%= form_for : course, : url => {: action => : find_course} do |form| %> <p> <label for="title">Course Number: </label> <%= form. text_field : number, : size => 20 %> </p> <p><%= submit_tag "Find a Course" %></p> <% end %></h 3>
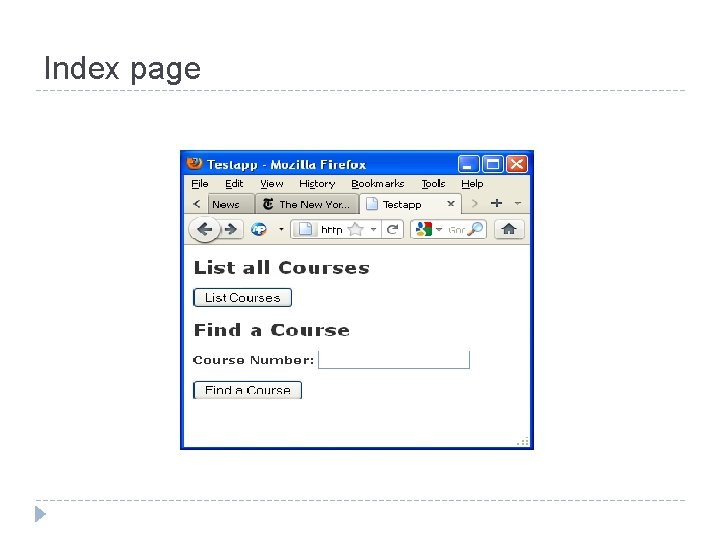
Index page
![Controller code for finding a course def findcourse params params course course Controller code for finding a course def find_course @params = params[: course] @course =](https://slidetodoc.com/presentation_image/db6792e8ec90955aa541cdba4263b3da/image-12.jpg)
Controller code for finding a course def find_course @params = params[: course] @course = Course. find_by_number(@params[: number]) respond_to do |format| if @course != nil format. html else format. html { render : action => "not_found" } end end
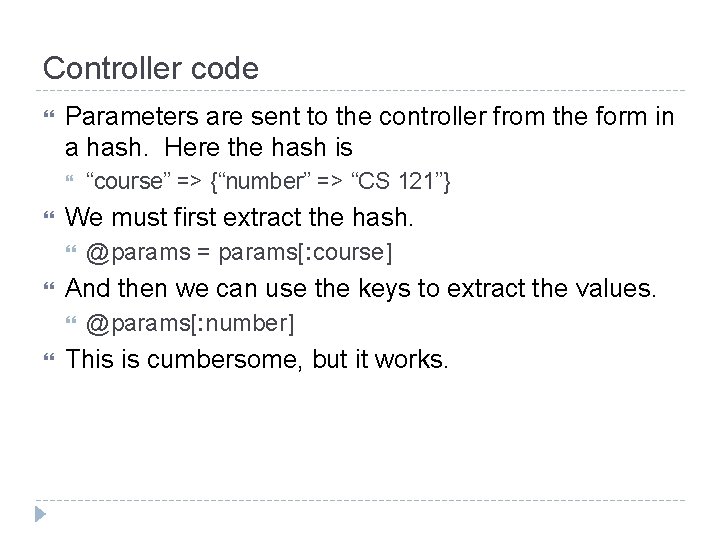
Controller code Parameters are sent to the controller from the form in a hash. Here the hash is We must first extract the hash. @params = params[: course] And then we can use the keys to extract the values. “course” => {“number” => “CS 121”} @params[: number] This is cumbersome, but it works.
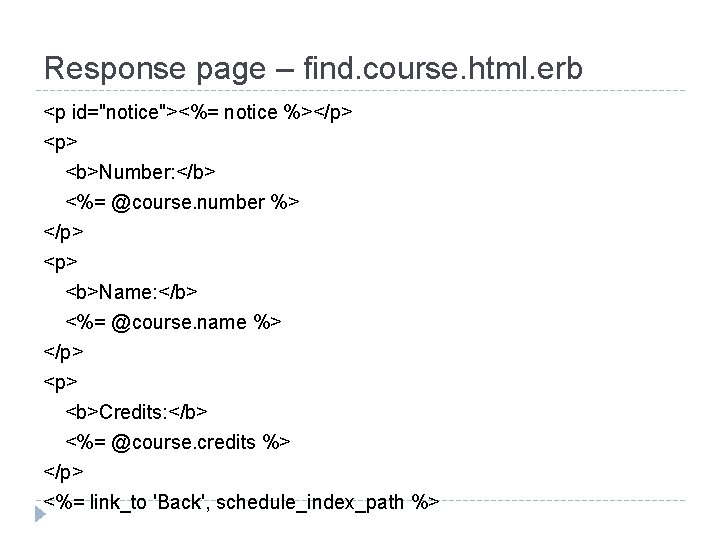
Response page – find. course. html. erb <p id="notice"><%= notice %></p> <b>Number: </b> <%= @course. number %> </p> <b>Name: </b> <%= @course. name %> </p> <b>Credits: </b> <%= @course. credits %> </p> <%= link_to 'Back', schedule_index_path %>
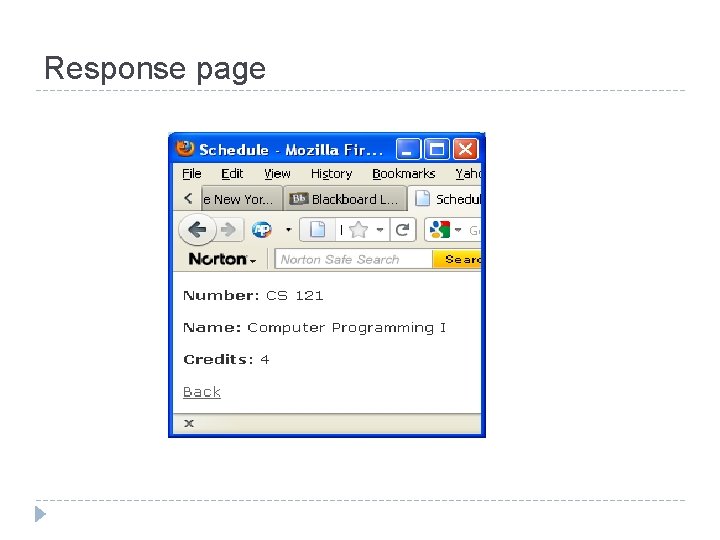
Response page
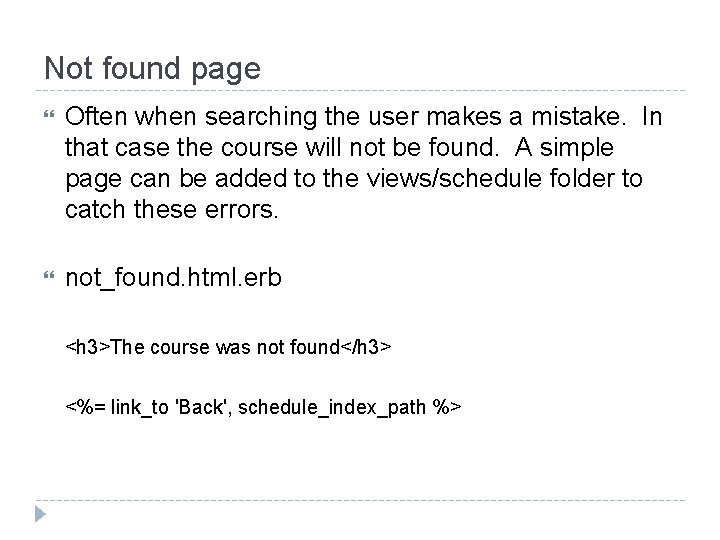
Not found page Often when searching the user makes a mistake. In that case the course will not be found. A simple page can be added to the views/schedule folder to catch these errors. not_found. html. erb <h 3>The course was not found</h 3> <%= link_to 'Back', schedule_index_path %>
Ruby validates_presence_of
Brush wolf vs timber wolf
Konrad wolf judith catherine wolf
Brass wolf
What is your favorite subject what subject
Programmed i/o in computer architecture
Wear on rails
Rails test database
Rails sti vs polymorphic
Seizure precautions bed rails
Ruby gem おすすめ
Transportation engineering
Types of rail section
Versine
Dampeners are used on some fuel rails to
Electrical safety office ghaziabad
Conclusion of comfort devices in nursing