SQL JOIN MULTIPLE RELATION QUERIES Often queries need
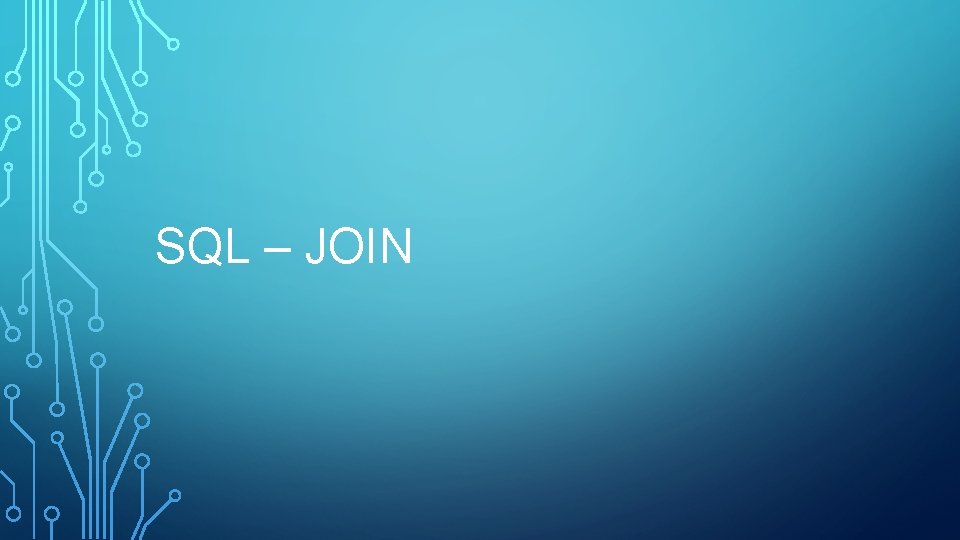
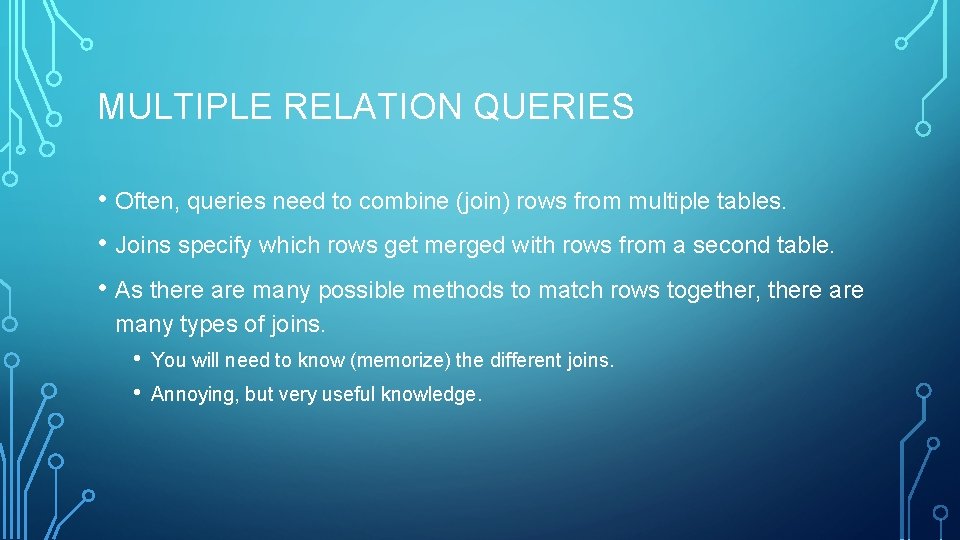
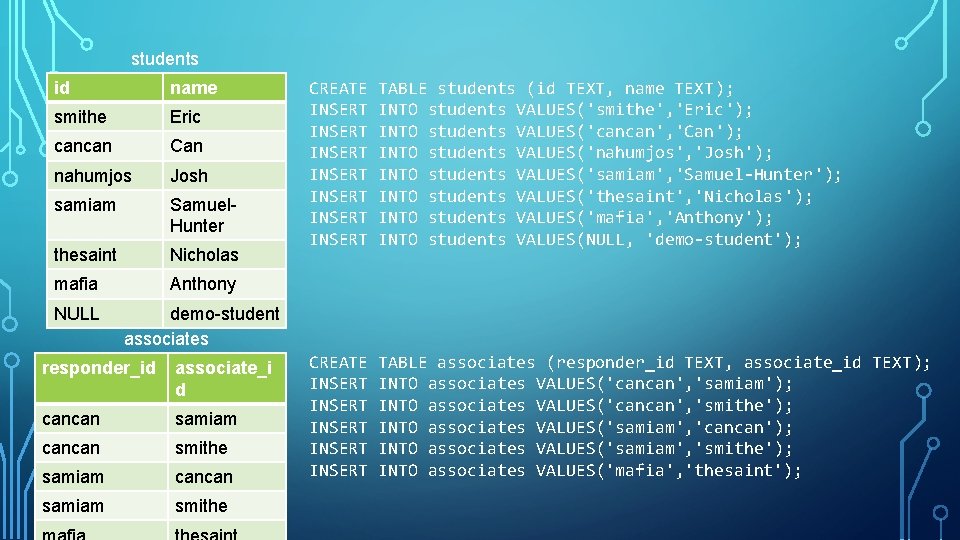
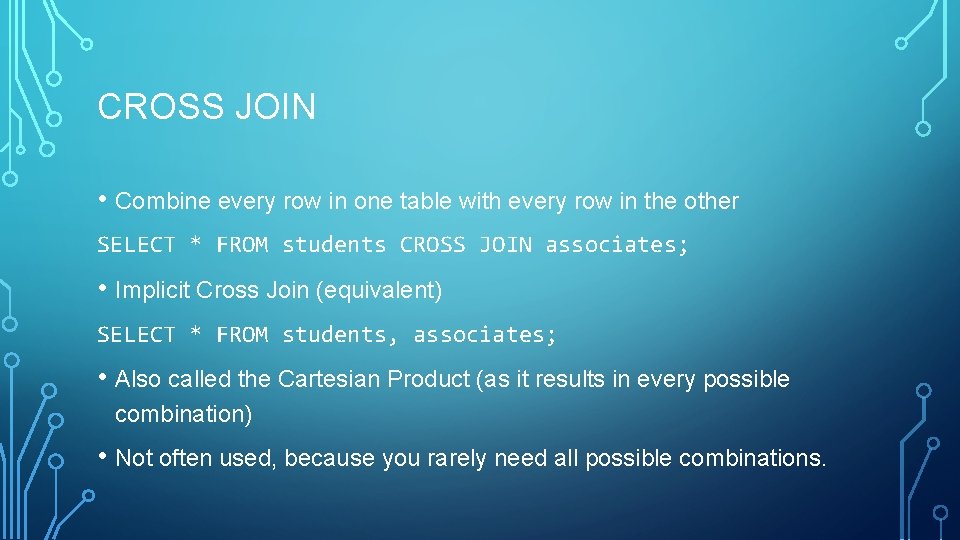
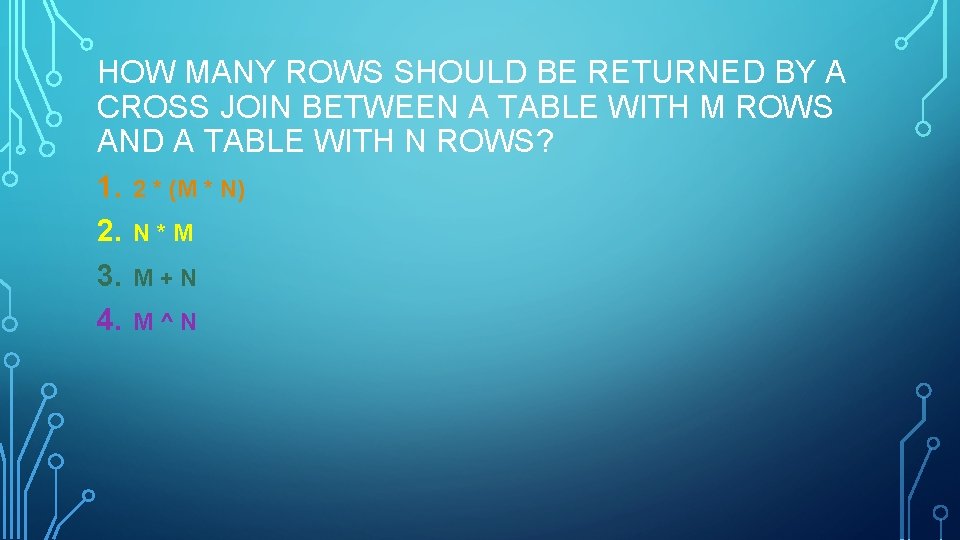
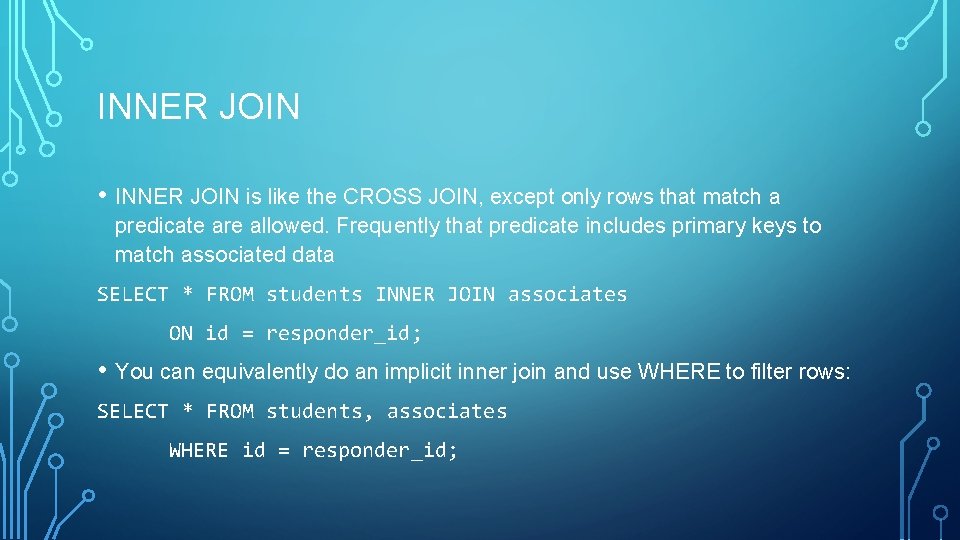
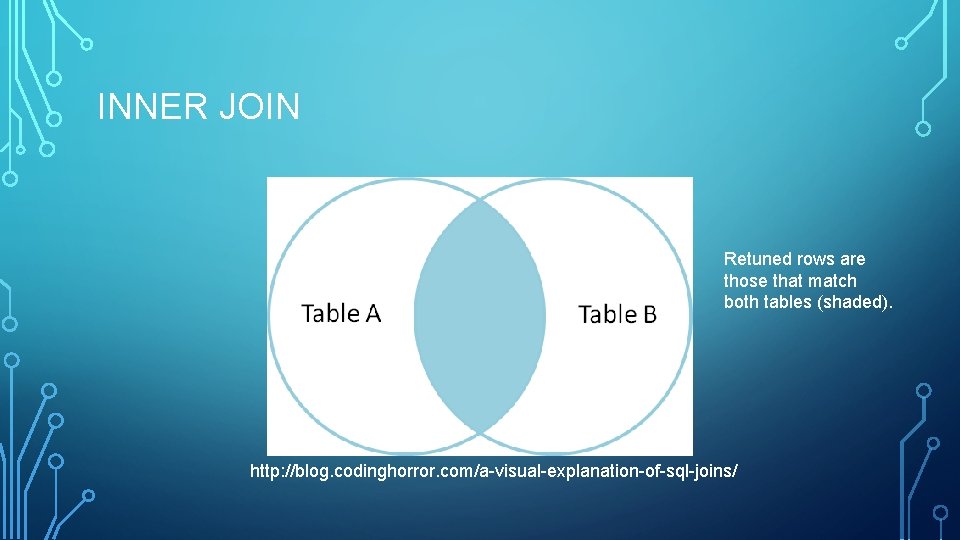
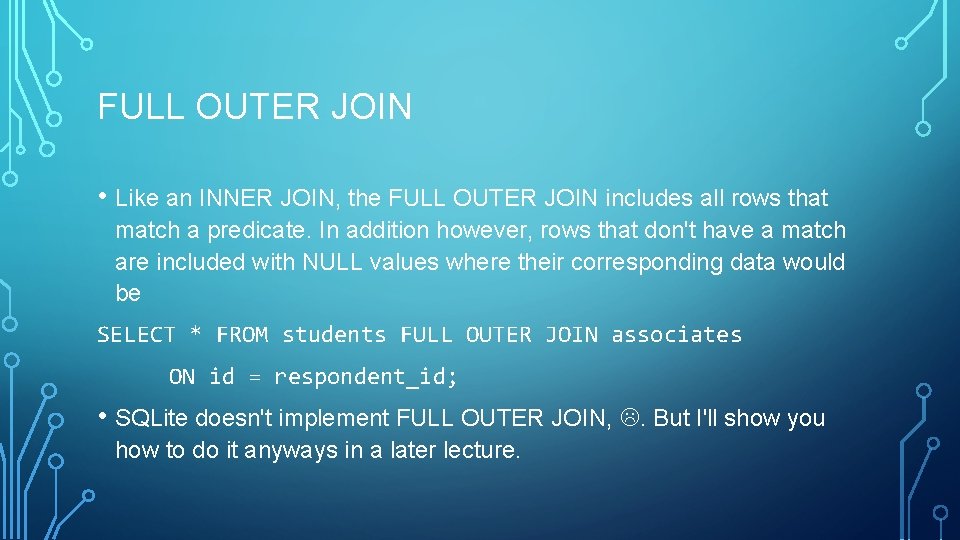
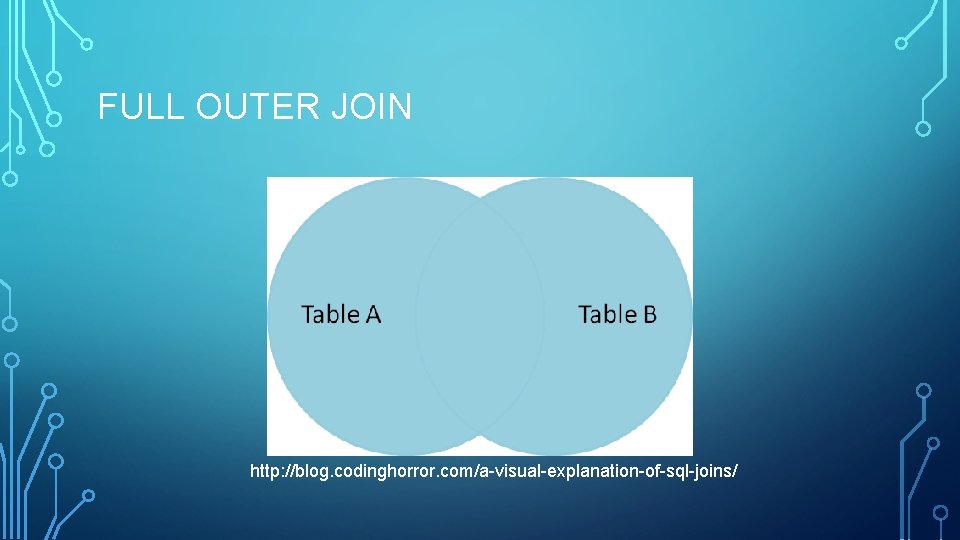
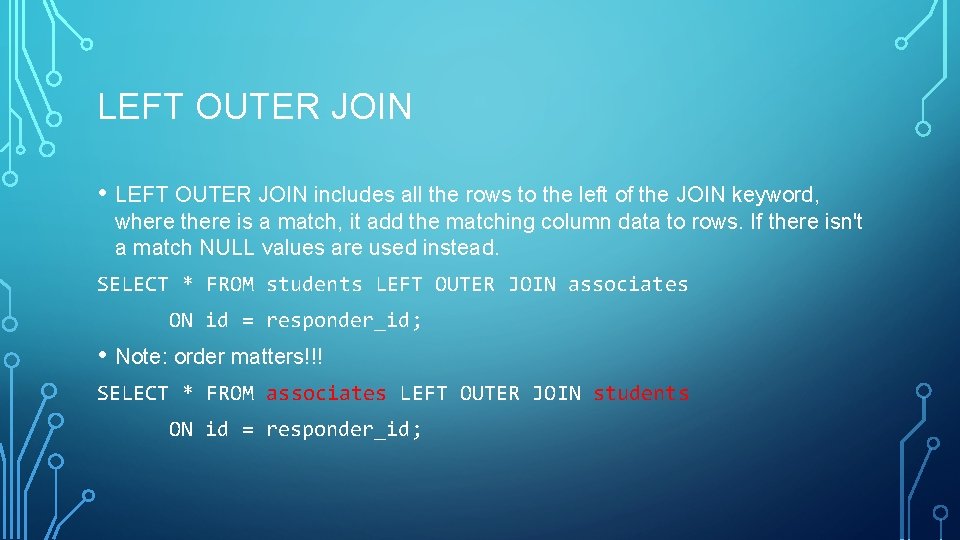
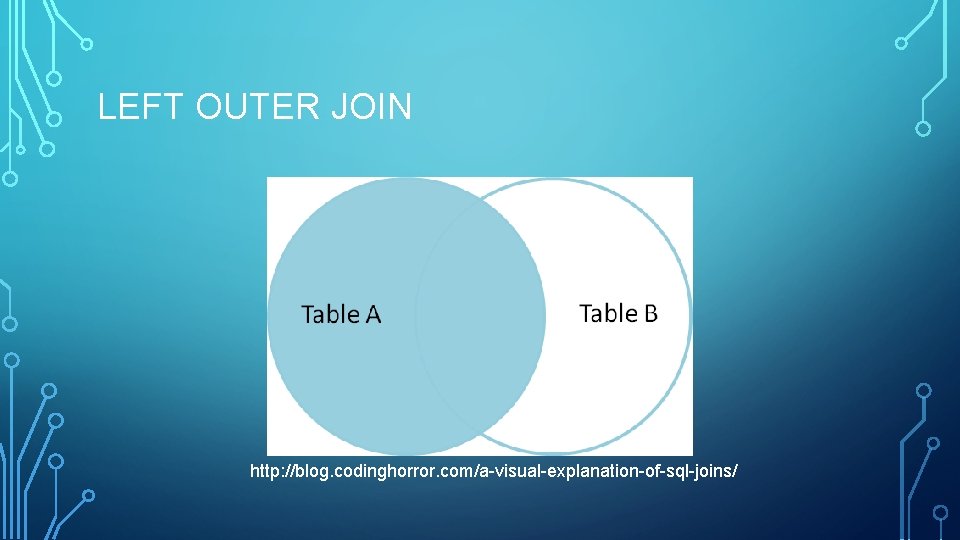
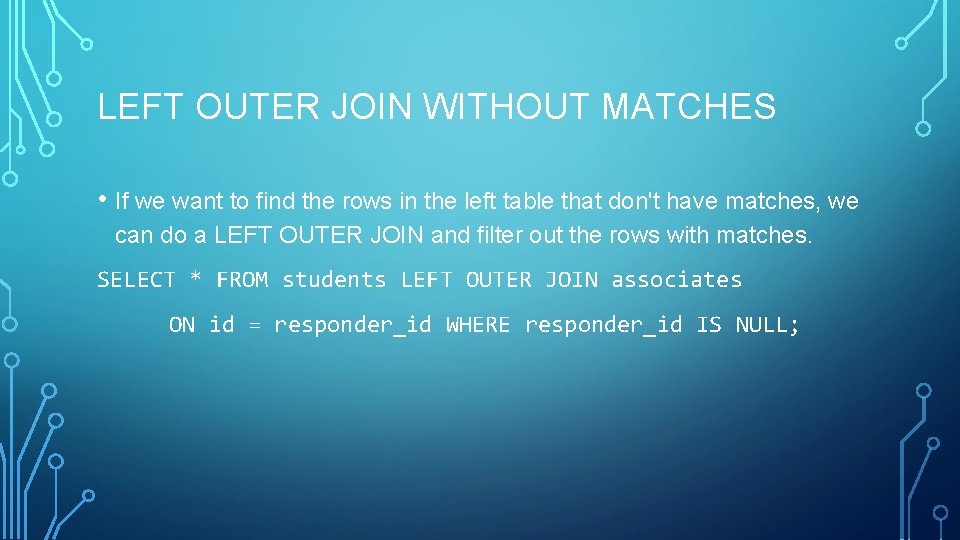
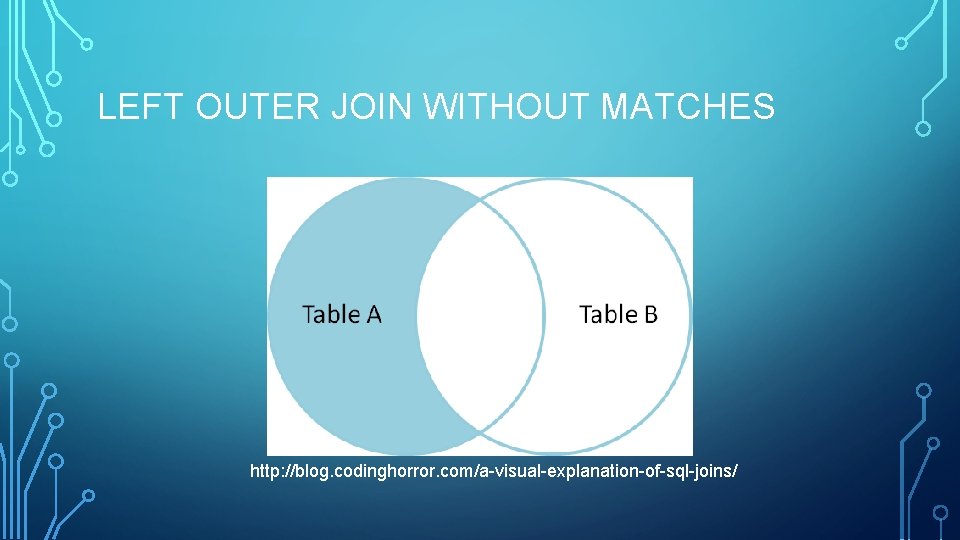
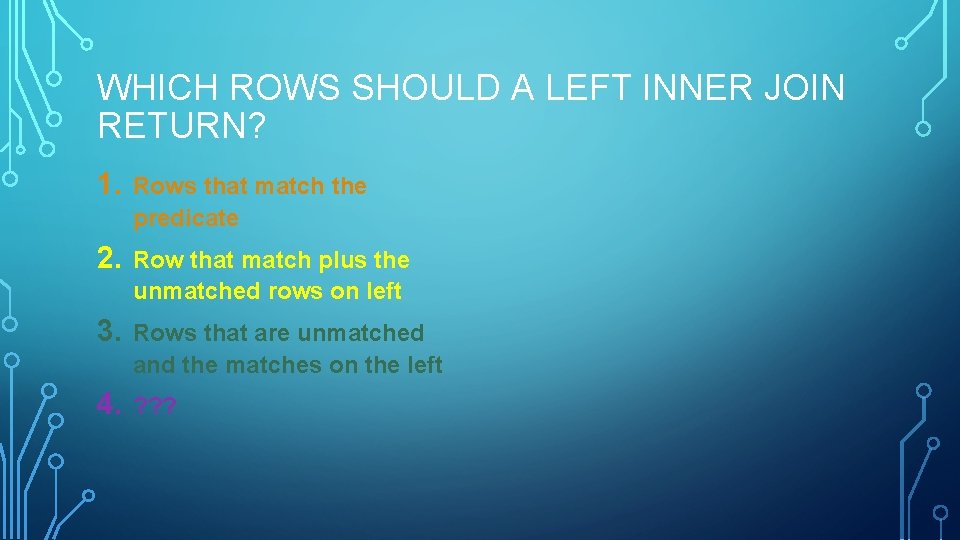
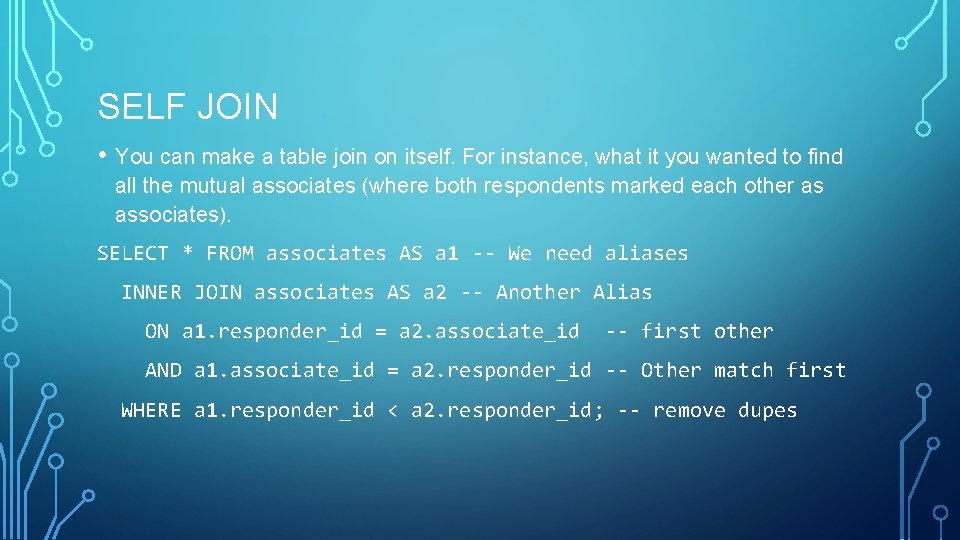
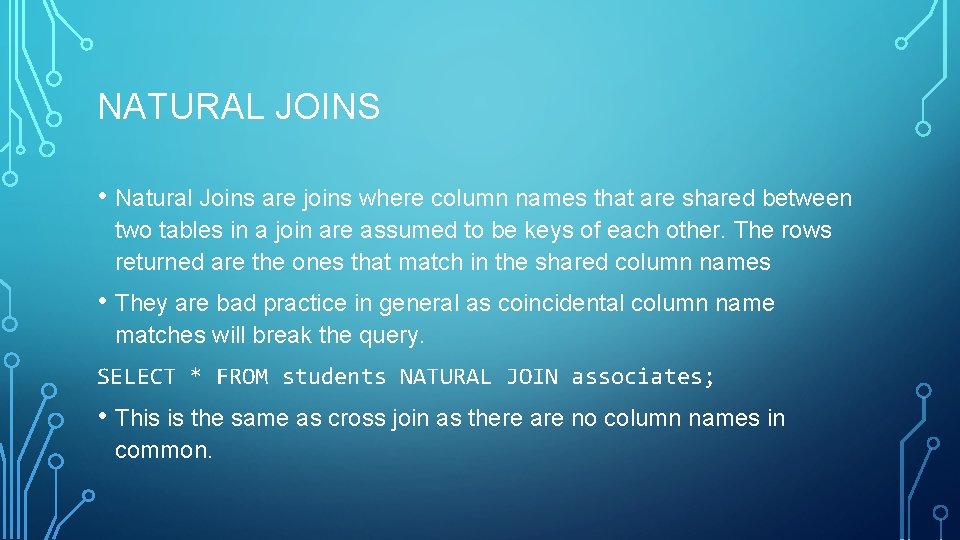
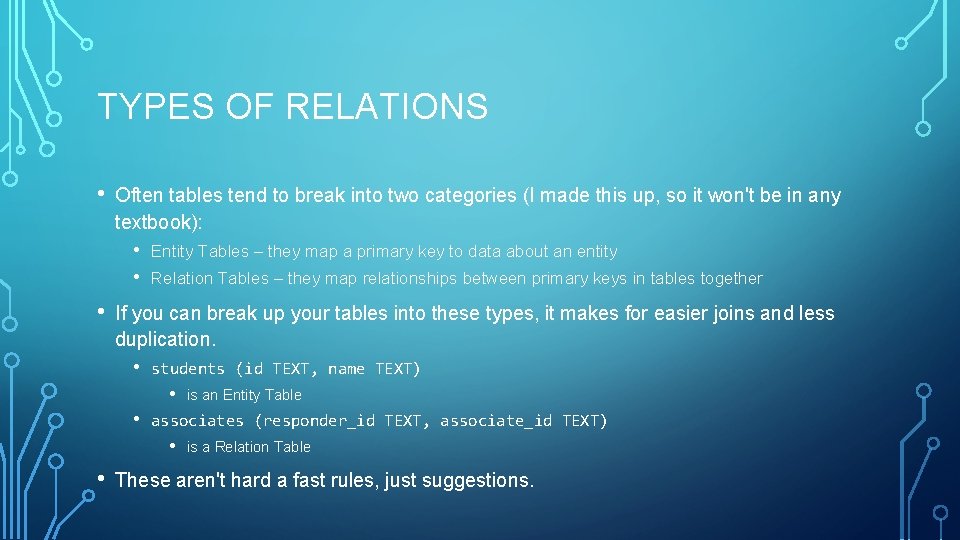
- Slides: 17
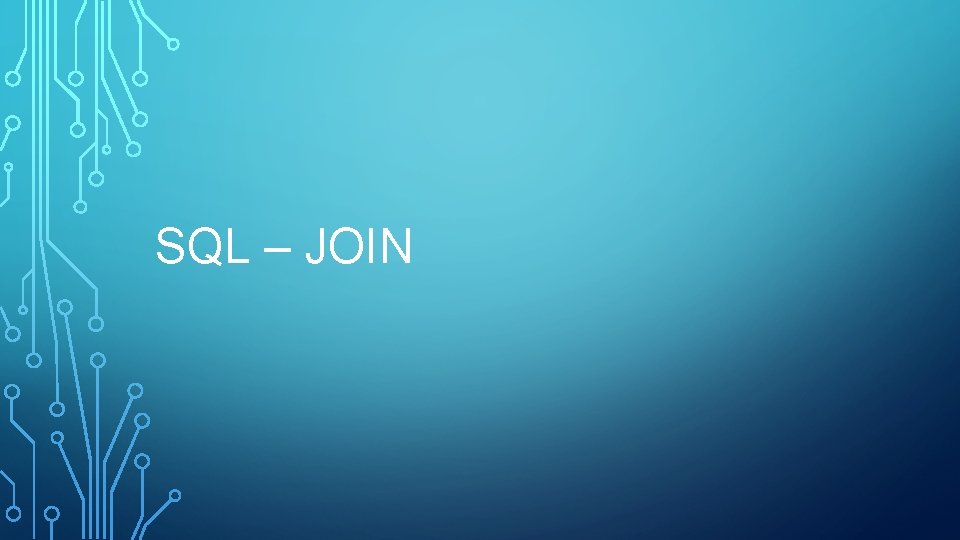
SQL – JOIN
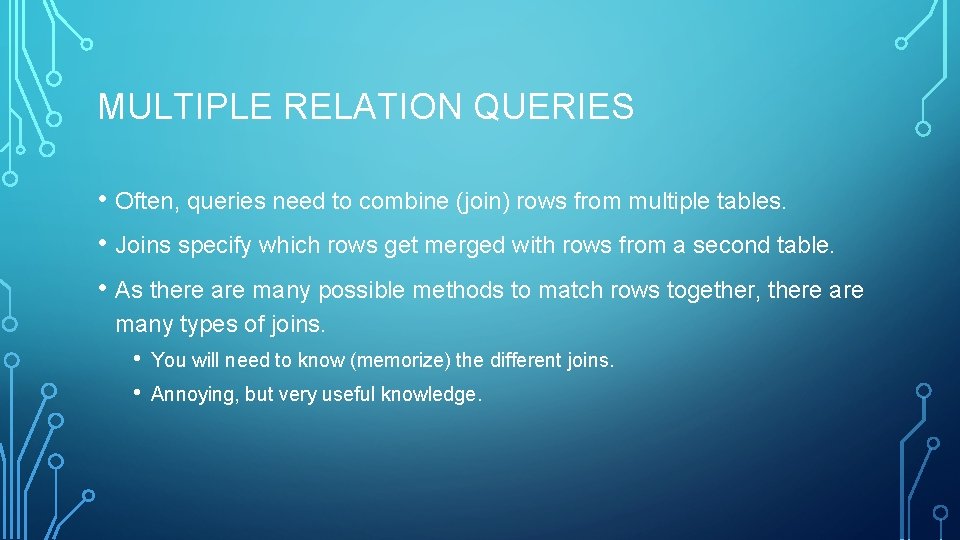
MULTIPLE RELATION QUERIES • Often, queries need to combine (join) rows from multiple tables. • Joins specify which rows get merged with rows from a second table. • As there are many possible methods to match rows together, there are many types of joins. • • You will need to know (memorize) the different joins. Annoying, but very useful knowledge.
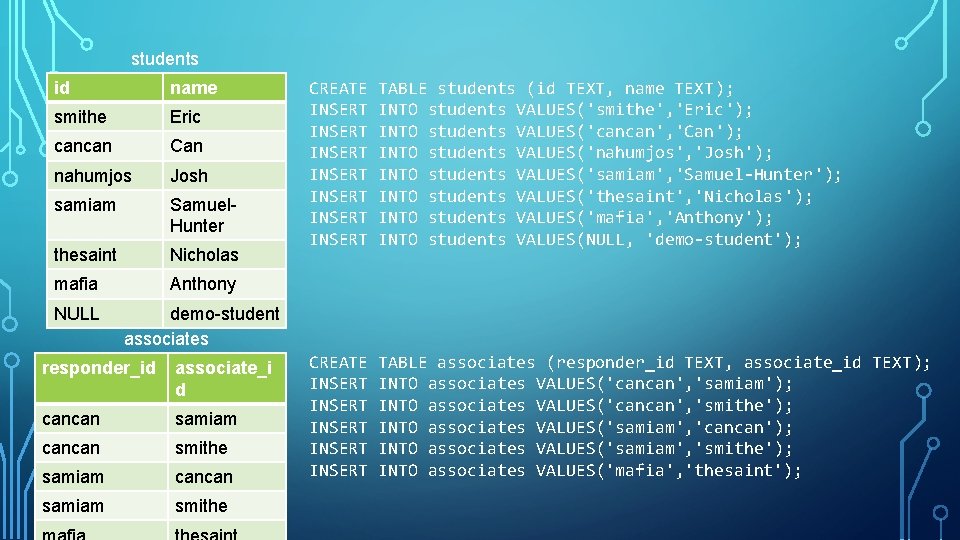
students id name smithe Eric cancan Can nahumjos Josh samiam Samuel. Hunter thesaint Nicholas mafia Anthony NULL demo-student associates responder_id associate_i d cancan samiam cancan smithe samiam cancan samiam smithe CREATE INSERT INSERT TABLE students (id TEXT, name TEXT); INTO students VALUES('smithe', 'Eric'); INTO students VALUES('cancan', 'Can'); INTO students VALUES('nahumjos', 'Josh'); INTO students VALUES('samiam', 'Samuel-Hunter'); INTO students VALUES('thesaint', 'Nicholas'); INTO students VALUES('mafia', 'Anthony'); INTO students VALUES(NULL, 'demo-student'); CREATE INSERT INSERT TABLE associates (responder_id TEXT, associate_id TEXT); INTO associates VALUES('cancan', 'samiam'); INTO associates VALUES('cancan', 'smithe'); INTO associates VALUES('samiam', 'cancan'); INTO associates VALUES('samiam', 'smithe'); INTO associates VALUES('mafia', 'thesaint');
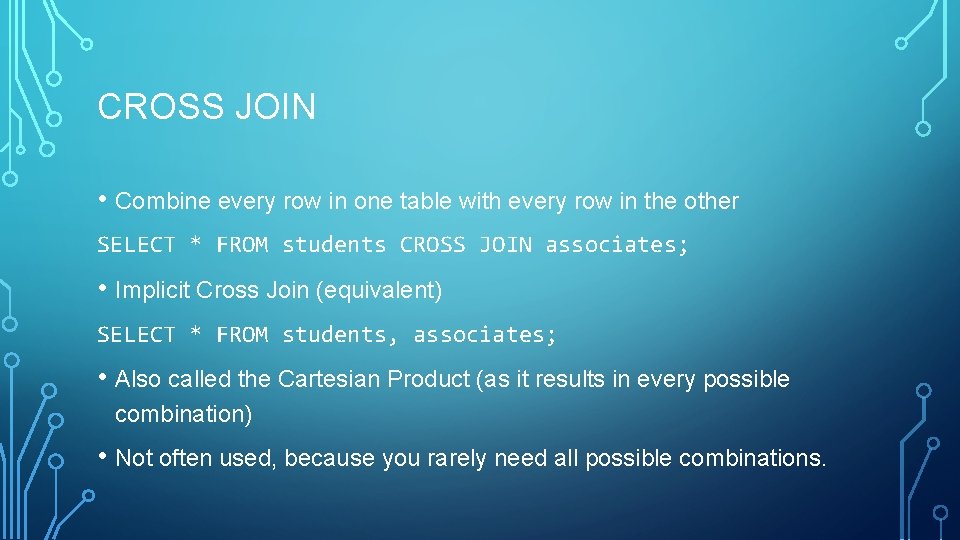
CROSS JOIN • Combine every row in one table with every row in the other SELECT * FROM students CROSS JOIN associates; • Implicit Cross Join (equivalent) SELECT * FROM students, associates; • Also called the Cartesian Product (as it results in every possible combination) • Not often used, because you rarely need all possible combinations.
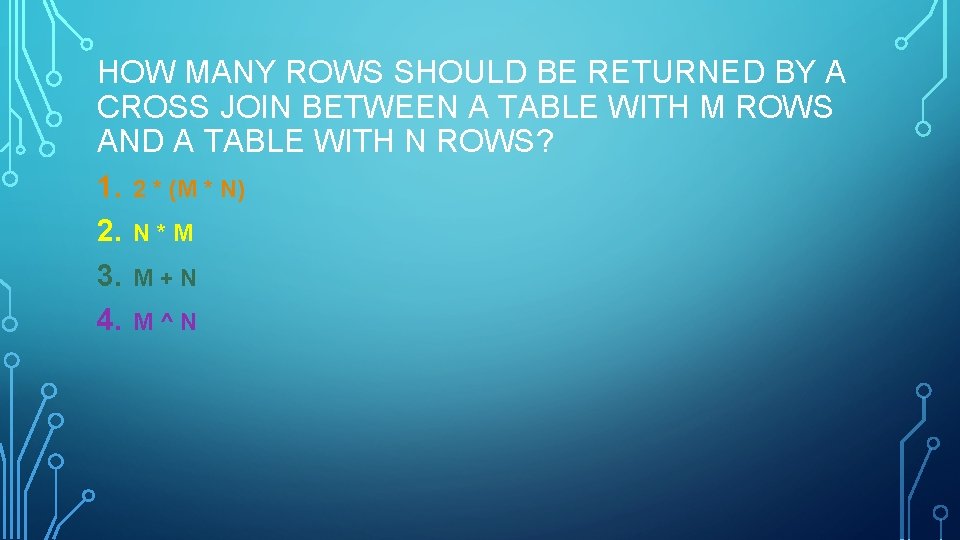
HOW MANY ROWS SHOULD BE RETURNED BY A CROSS JOIN BETWEEN A TABLE WITH M ROWS AND A TABLE WITH N ROWS? 1. 2 * (M * N) 2. N * M 3. M + N 4. M ^ N
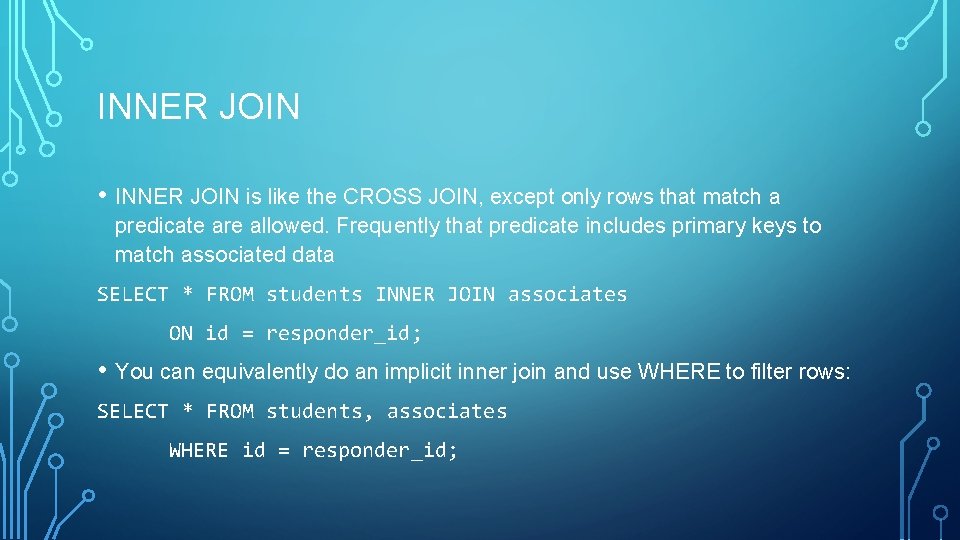
INNER JOIN • INNER JOIN is like the CROSS JOIN, except only rows that match a predicate are allowed. Frequently that predicate includes primary keys to match associated data SELECT * FROM students INNER JOIN associates ON id = responder_id; • You can equivalently do an implicit inner join and use WHERE to filter rows: SELECT * FROM students, associates WHERE id = responder_id;
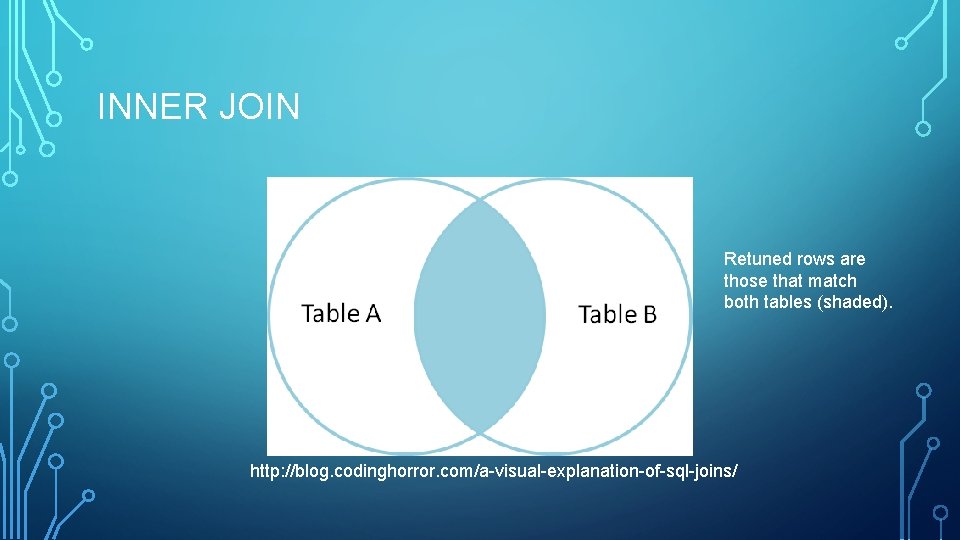
INNER JOIN Retuned rows are those that match both tables (shaded). http: //blog. codinghorror. com/a-visual-explanation-of-sql-joins/
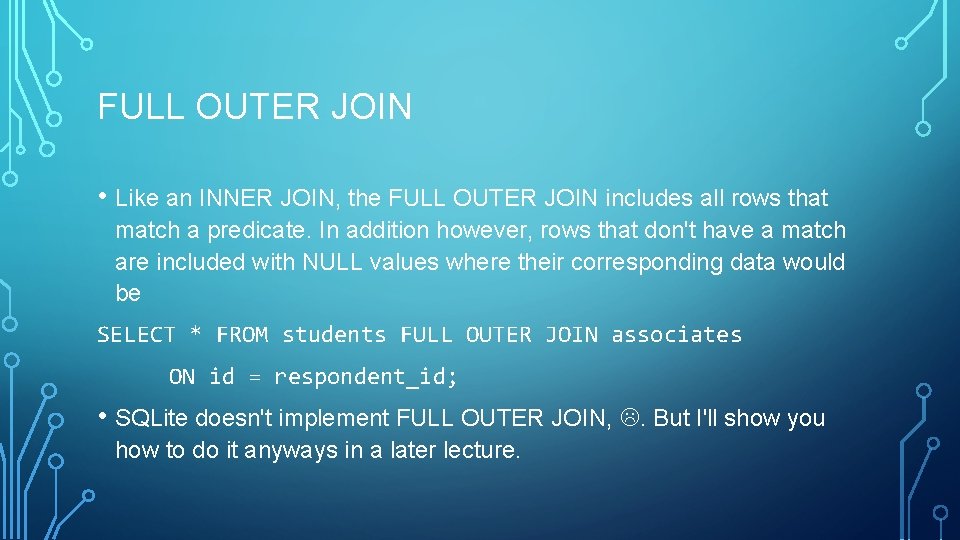
FULL OUTER JOIN • Like an INNER JOIN, the FULL OUTER JOIN includes all rows that match a predicate. In addition however, rows that don't have a match are included with NULL values where their corresponding data would be SELECT * FROM students FULL OUTER JOIN associates ON id = respondent_id; • SQLite doesn't implement FULL OUTER JOIN, . But I'll show you how to do it anyways in a later lecture.
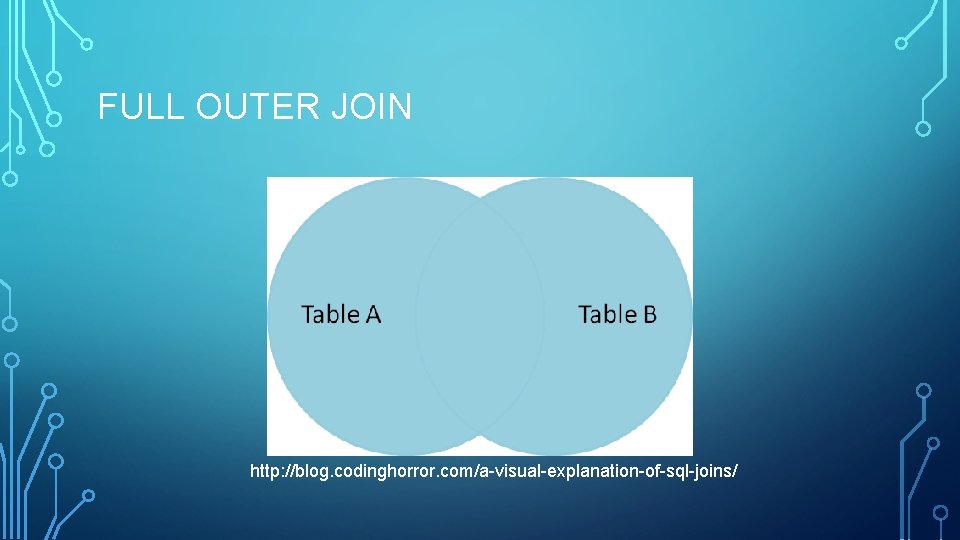
FULL OUTER JOIN http: //blog. codinghorror. com/a-visual-explanation-of-sql-joins/
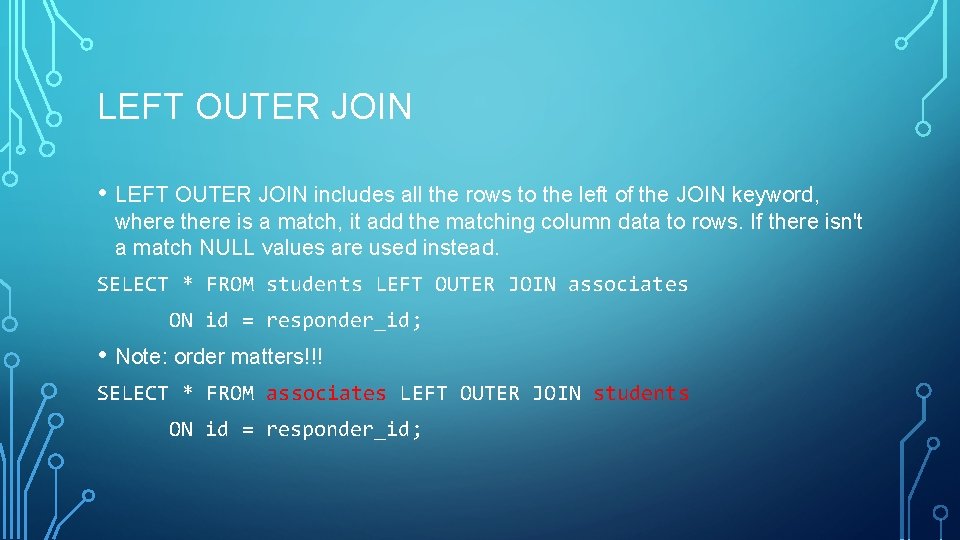
LEFT OUTER JOIN • LEFT OUTER JOIN includes all the rows to the left of the JOIN keyword, where there is a match, it add the matching column data to rows. If there isn't a match NULL values are used instead. SELECT * FROM students LEFT OUTER JOIN associates ON id = responder_id; • Note: order matters!!! SELECT * FROM associates LEFT OUTER JOIN students ON id = responder_id;
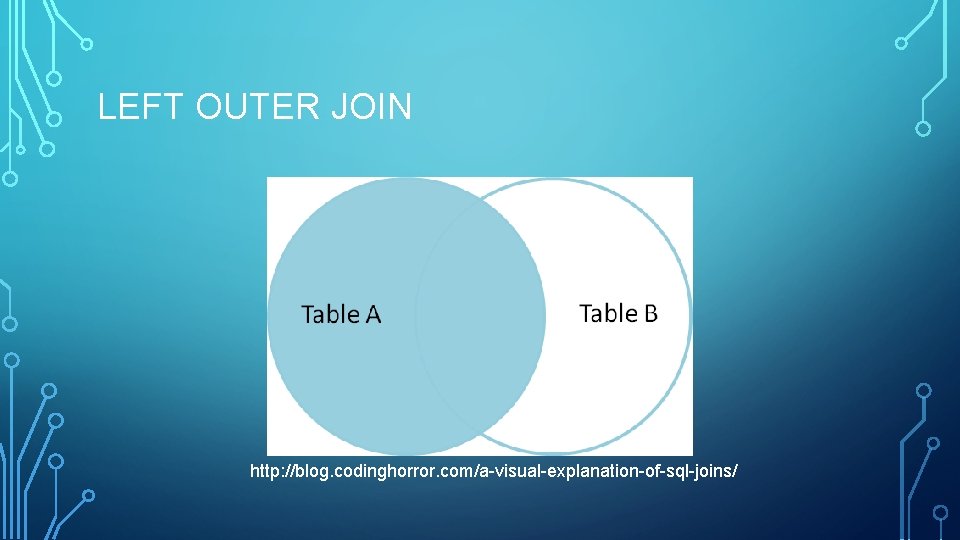
LEFT OUTER JOIN http: //blog. codinghorror. com/a-visual-explanation-of-sql-joins/
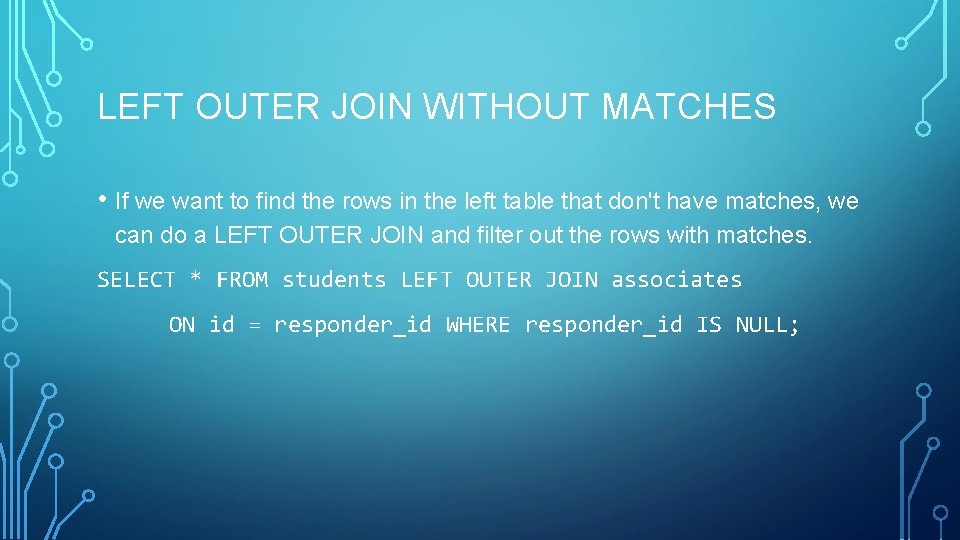
LEFT OUTER JOIN WITHOUT MATCHES • If we want to find the rows in the left table that don't have matches, we can do a LEFT OUTER JOIN and filter out the rows with matches. SELECT * FROM students LEFT OUTER JOIN associates ON id = responder_id WHERE responder_id IS NULL;
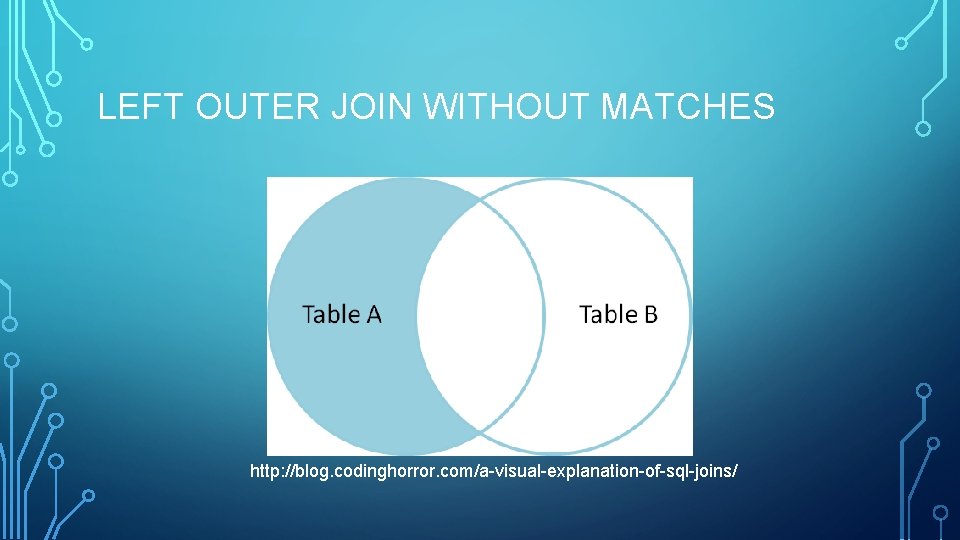
LEFT OUTER JOIN WITHOUT MATCHES http: //blog. codinghorror. com/a-visual-explanation-of-sql-joins/
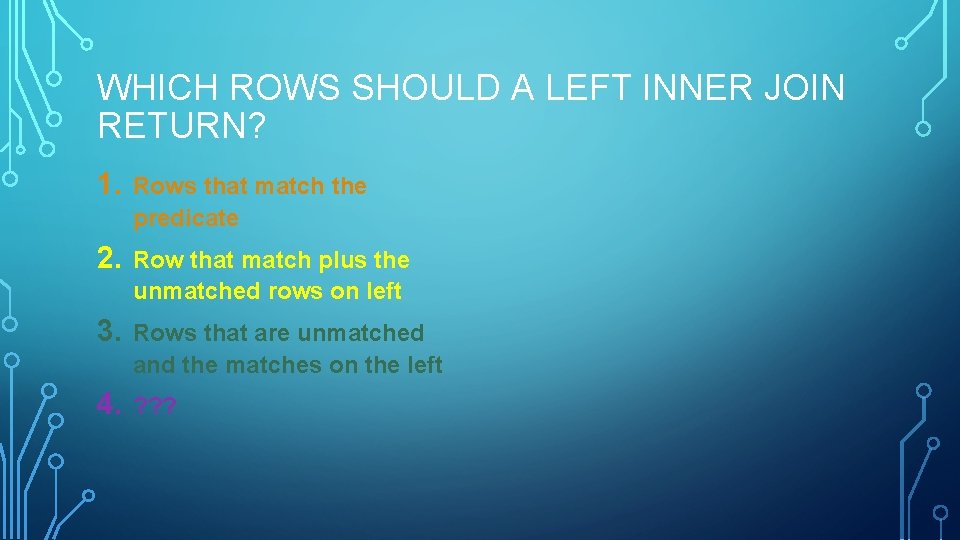
WHICH ROWS SHOULD A LEFT INNER JOIN RETURN? 1. Rows that match the predicate 2. Row that match plus the unmatched rows on left 3. Rows that are unmatched and the matches on the left 4. ? ? ?
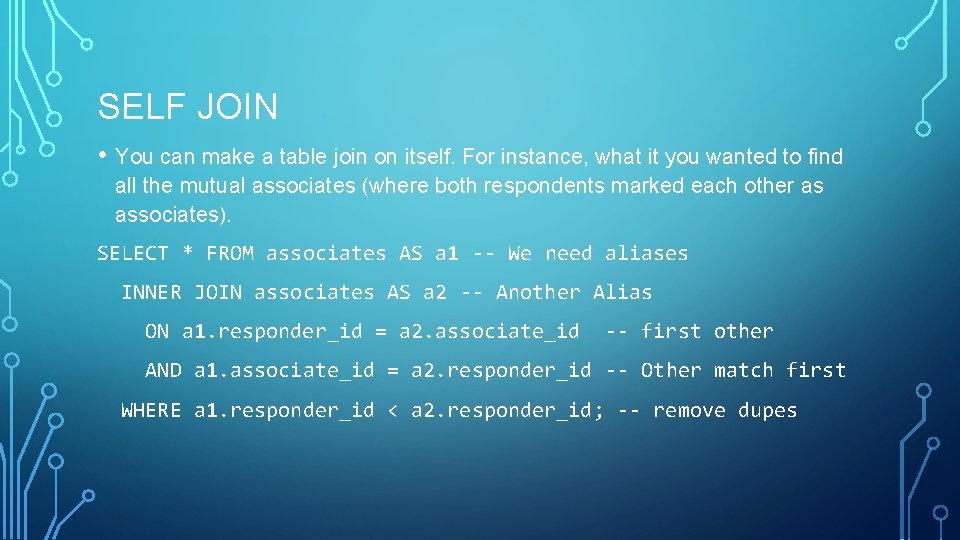
SELF JOIN • You can make a table join on itself. For instance, what it you wanted to find all the mutual associates (where both respondents marked each other as associates). SELECT * FROM associates AS a 1 -- We need aliases INNER JOIN associates AS a 2 -- Another Alias ON a 1. responder_id = a 2. associate_id -- first other AND a 1. associate_id = a 2. responder_id -- Other match first WHERE a 1. responder_id < a 2. responder_id; -- remove dupes
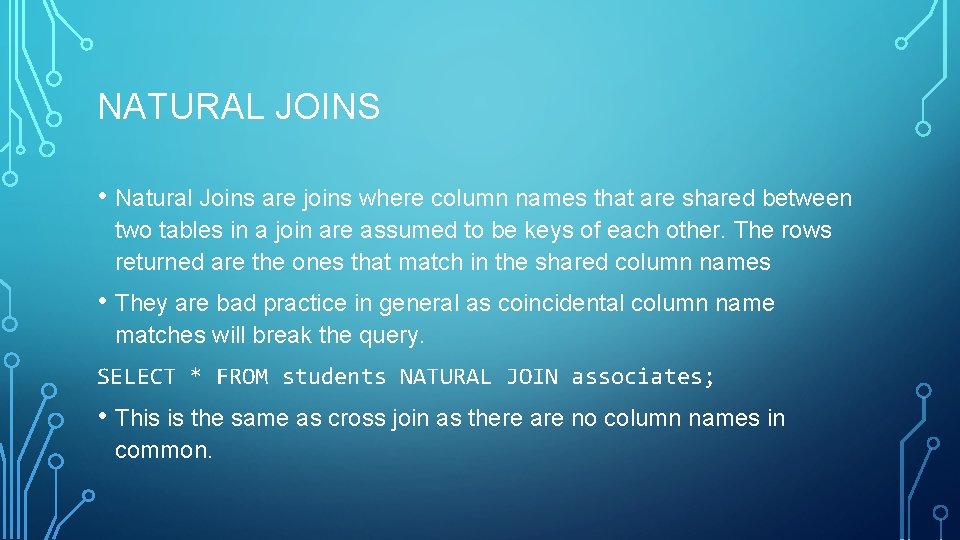
NATURAL JOINS • Natural Joins are joins where column names that are shared between two tables in a join are assumed to be keys of each other. The rows returned are the ones that match in the shared column names • They are bad practice in general as coincidental column name matches will break the query. SELECT * FROM students NATURAL JOIN associates; • This is the same as cross join as there are no column names in common.
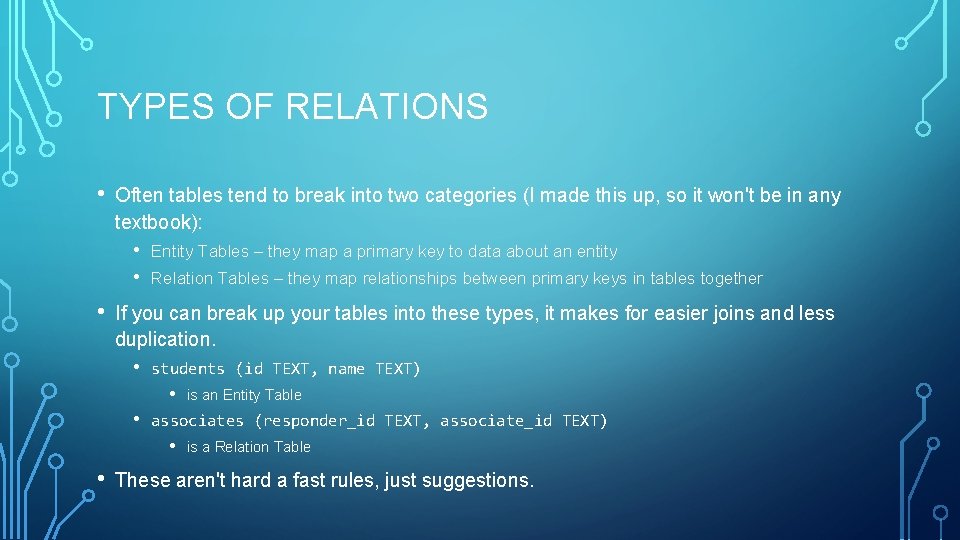
TYPES OF RELATIONS • Often tables tend to break into two categories (I made this up, so it won't be in any textbook): • • • Entity Tables – they map a primary key to data about an entity Relation Tables – they map relationships between primary keys in tables together If you can break up your tables into these types, it makes for easier joins and less duplication. • students (id TEXT, name TEXT) • • associates (responder_id TEXT, associate_id TEXT) • • is an Entity Table is a Relation Table These aren't hard a fast rules, just suggestions.