Sets v Set is an unordered list of
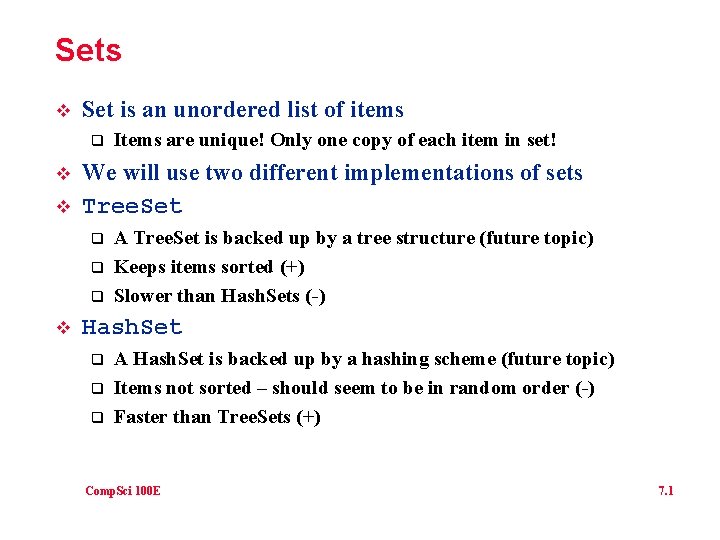
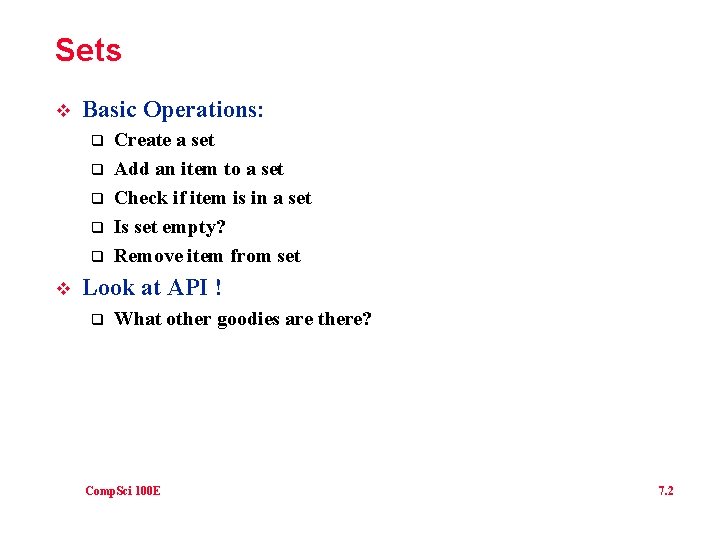
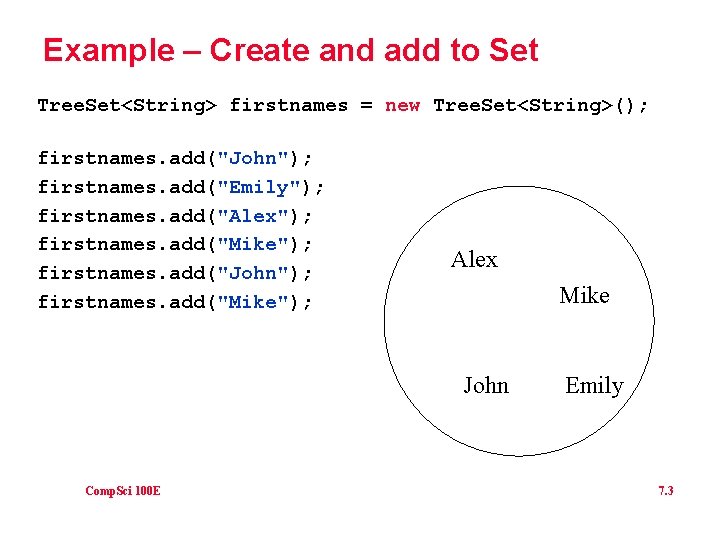
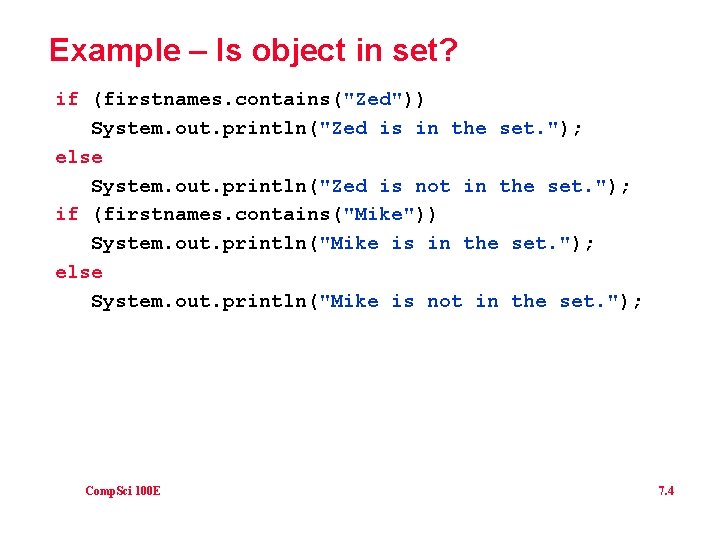
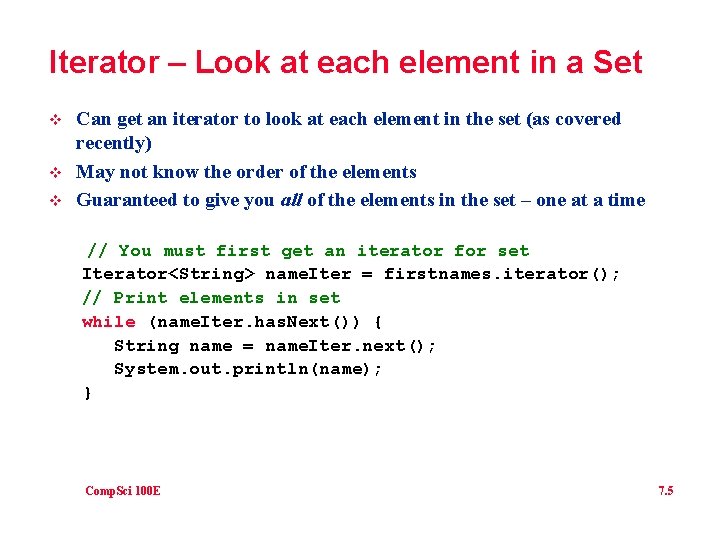
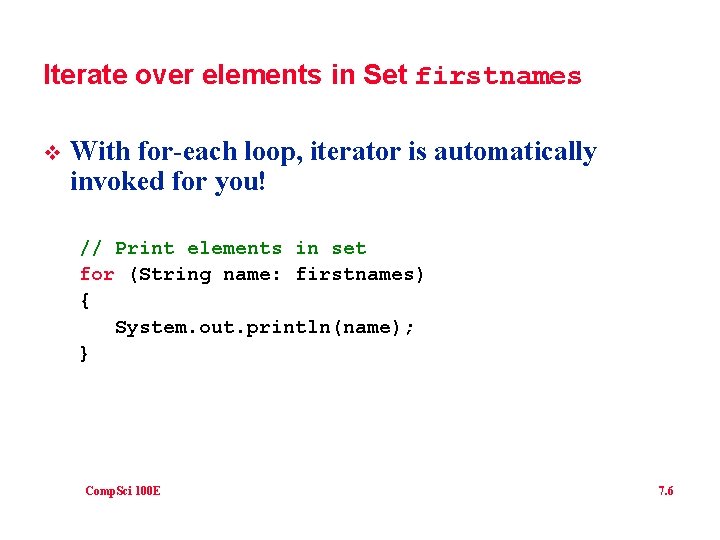
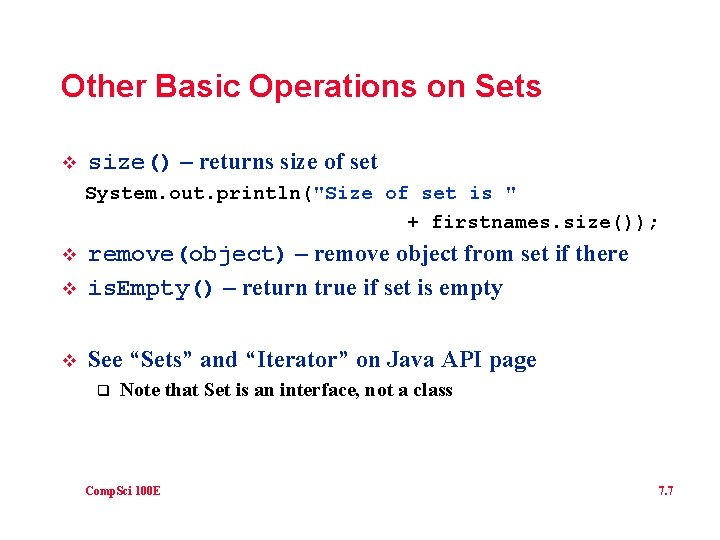
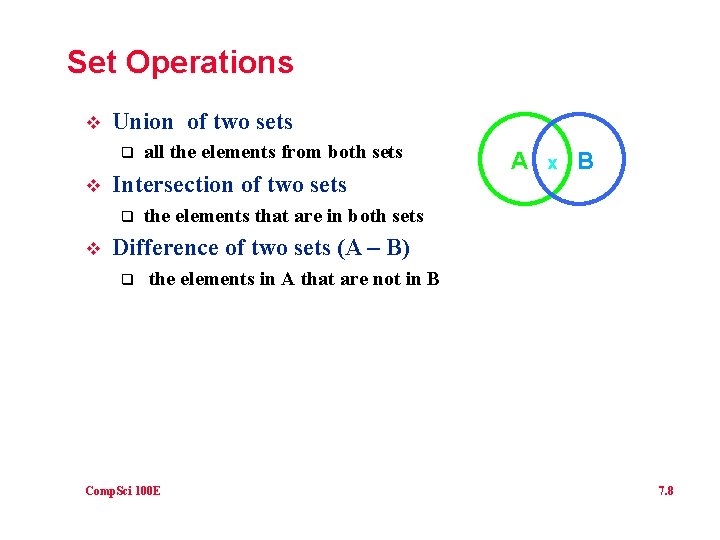
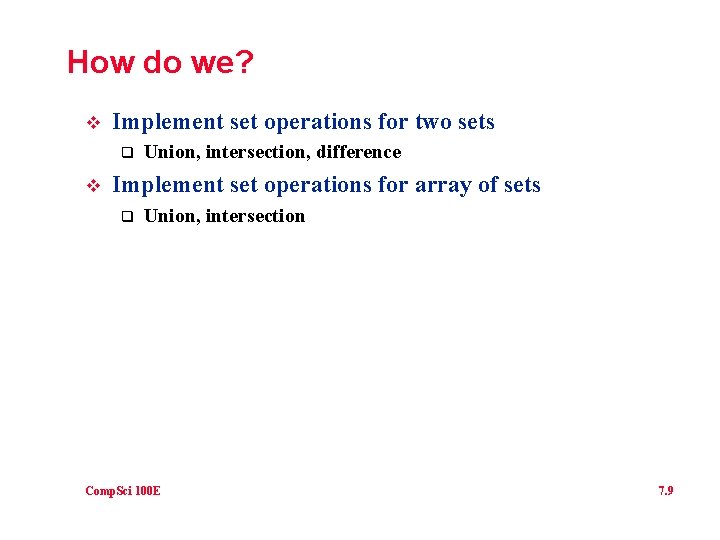
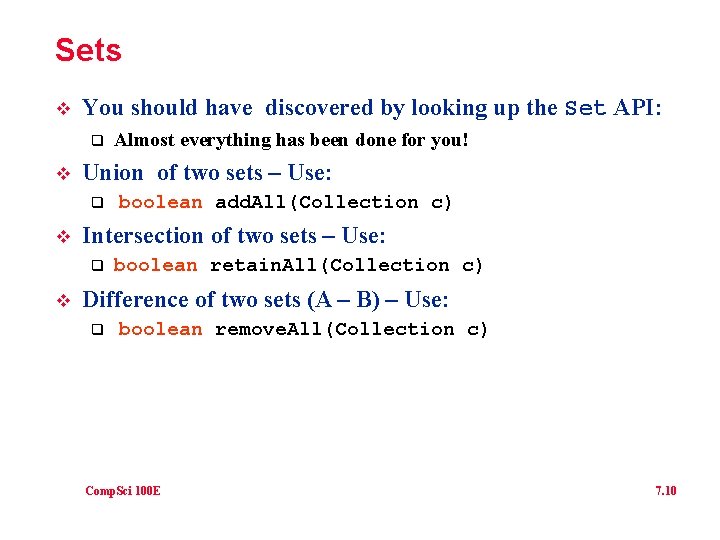
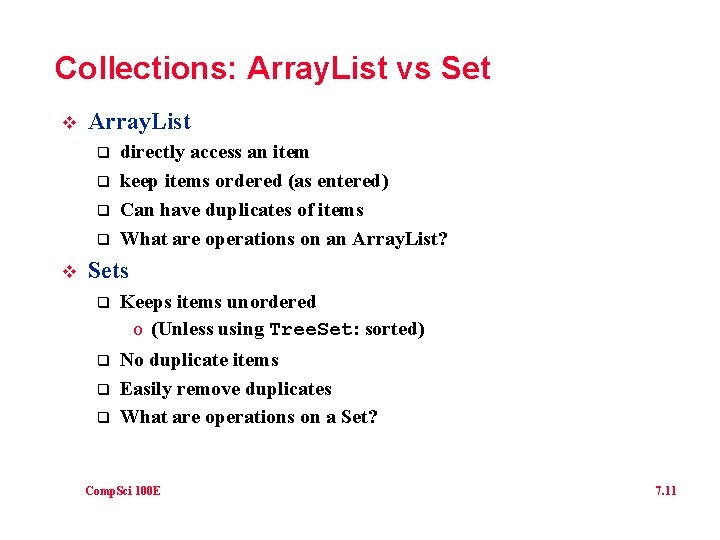
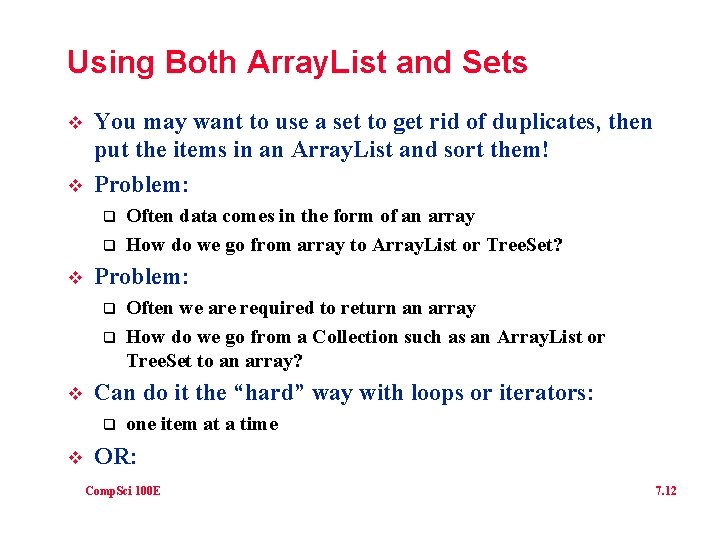
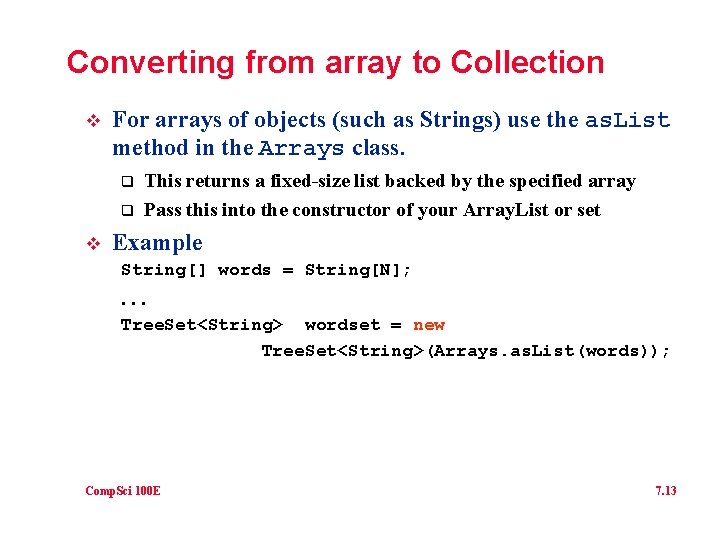
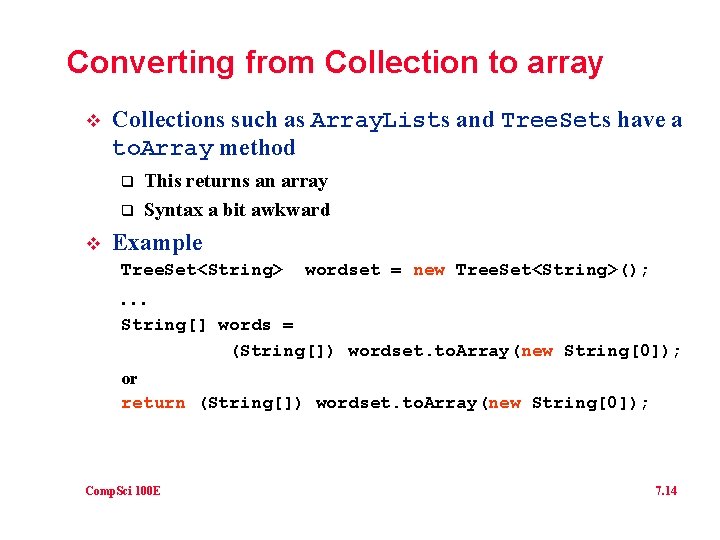
- Slides: 14
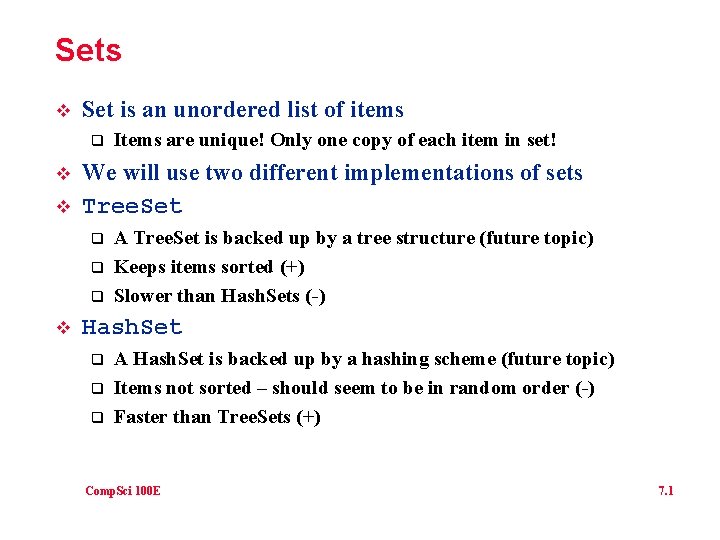
Sets v Set is an unordered list of items q v v We will use two different implementations of sets Tree. Set q q q v Items are unique! Only one copy of each item in set! A Tree. Set is backed up by a tree structure (future topic) Keeps items sorted (+) Slower than Hash. Sets (-) Hash. Set q q q A Hash. Set is backed up by a hashing scheme (future topic) Items not sorted – should seem to be in random order (-) Faster than Tree. Sets (+) Comp. Sci 100 E 7. 1
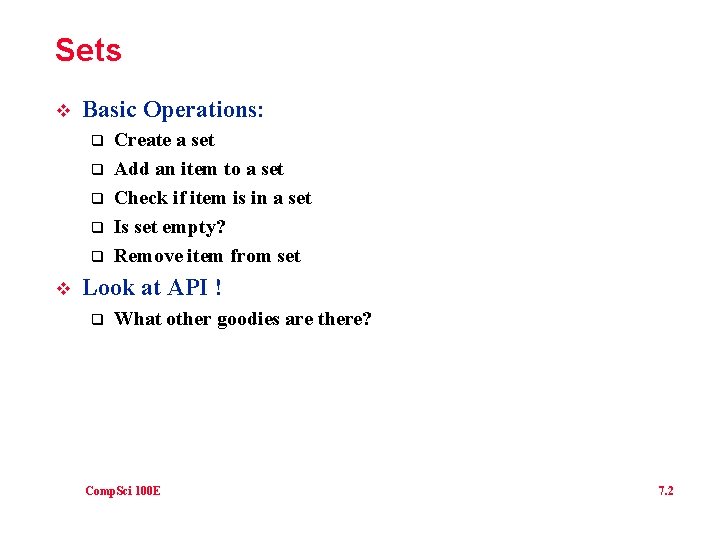
Sets v Basic Operations: q q q v Create a set Add an item to a set Check if item is in a set Is set empty? Remove item from set Look at API ! q What other goodies are there? Comp. Sci 100 E 7. 2
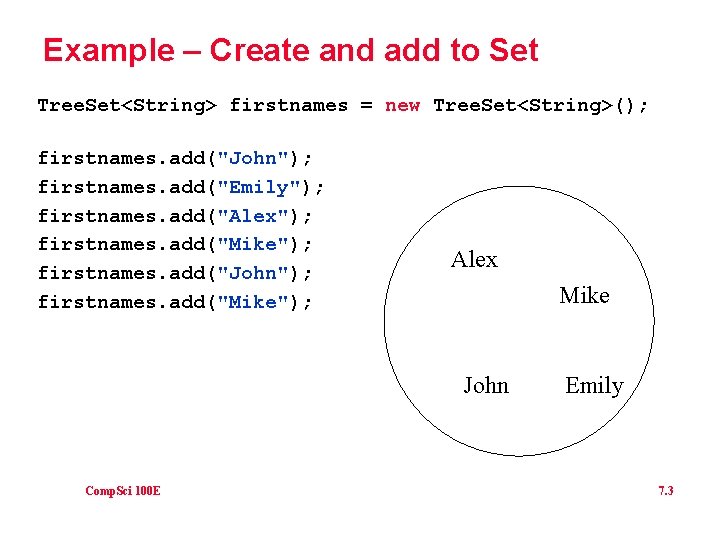
Example – Create and add to Set Tree. Set<String> firstnames = new Tree. Set<String>(); firstnames. add("John"); firstnames. add("Emily"); firstnames. add("Alex"); firstnames. add("Mike"); firstnames. add("John"); firstnames. add("Mike"); Alex Mike John Comp. Sci 100 E Emily 7. 3
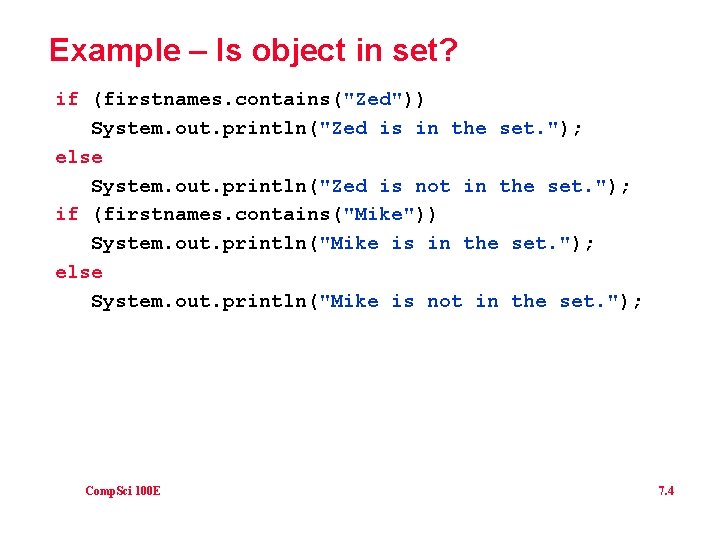
Example – Is object in set? if (firstnames. contains("Zed")) System. out. println("Zed is in the set. "); else System. out. println("Zed is not in the set. "); if (firstnames. contains("Mike")) System. out. println("Mike is in the set. "); else System. out. println("Mike is not in the set. "); Comp. Sci 100 E 7. 4
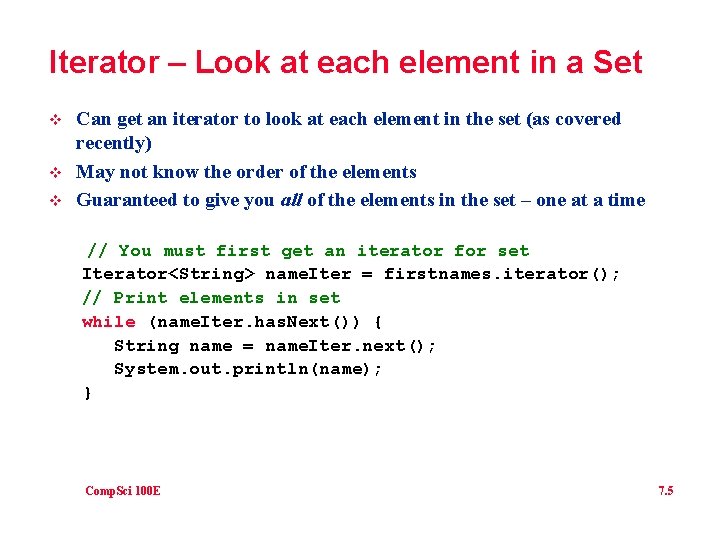
Iterator – Look at each element in a Set v v v Can get an iterator to look at each element in the set (as covered recently) May not know the order of the elements Guaranteed to give you all of the elements in the set – one at a time // You must first get an iterator for set Iterator<String> name. Iter = firstnames. iterator(); // Print elements in set while (name. Iter. has. Next()) { String name = name. Iter. next(); System. out. println(name); } Comp. Sci 100 E 7. 5
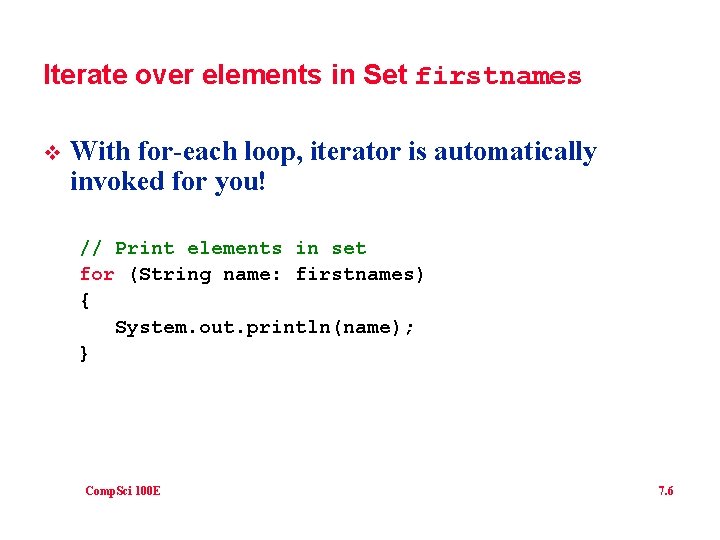
Iterate over elements in Set firstnames v With for-each loop, iterator is automatically invoked for you! // Print elements in set for (String name: firstnames) { System. out. println(name); } Comp. Sci 100 E 7. 6
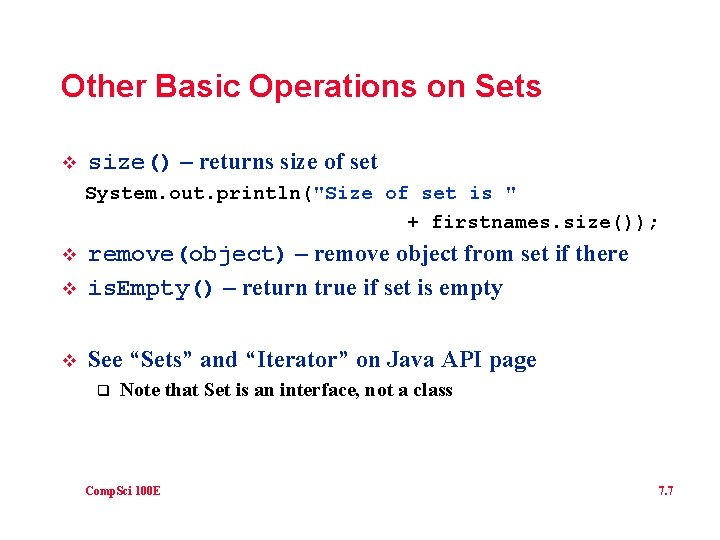
Other Basic Operations on Sets v size() – returns size of set System. out. println("Size of set is " + firstnames. size()); v remove(object) – remove object from set if there is. Empty() – return true if set is empty v See “Sets” and “Iterator” on Java API page v q Note that Set is an interface, not a class Comp. Sci 100 E 7. 7
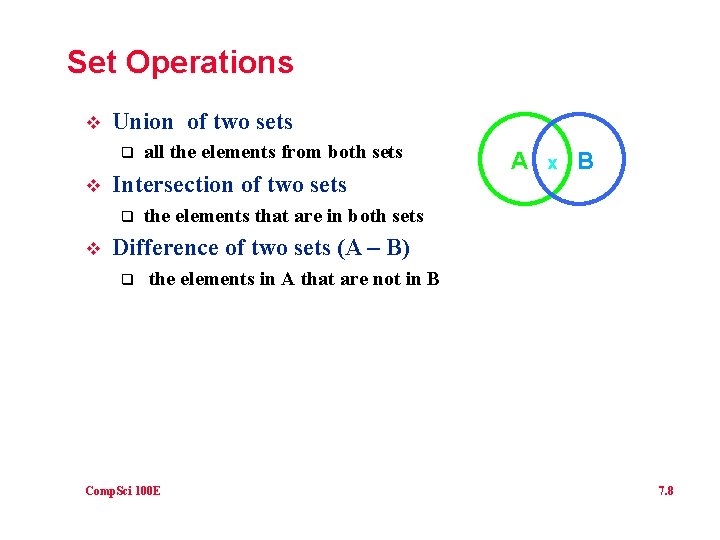
Set Operations v Union of two sets q v Intersection of two sets q v all the elements from both sets A x B the elements that are in both sets Difference of two sets (A – B) q the elements in A that are not in B Comp. Sci 100 E 7. 8
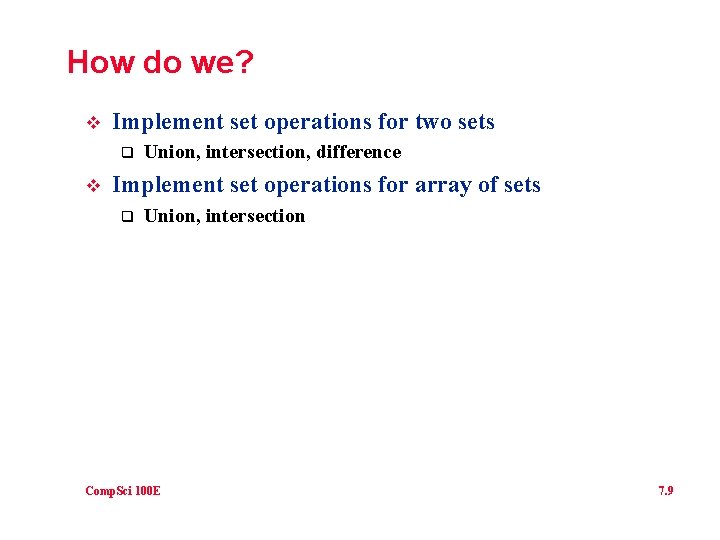
How do we? v Implement set operations for two sets q v Union, intersection, difference Implement set operations for array of sets q Union, intersection Comp. Sci 100 E 7. 9
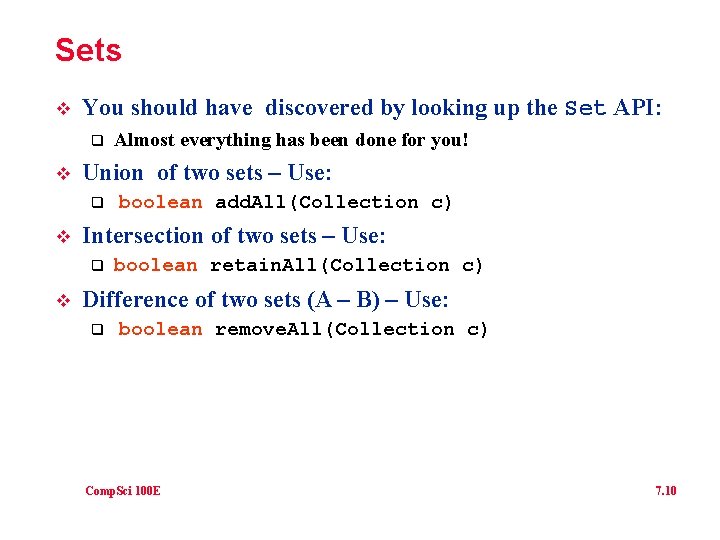
Sets v You should have discovered by looking up the Set API: q v Union of two sets – Use: q v boolean add. All(Collection c) Intersection of two sets – Use: q v Almost everything has been done for you! boolean retain. All(Collection c) Difference of two sets (A – B) – Use: q boolean remove. All(Collection c) Comp. Sci 100 E 7. 10
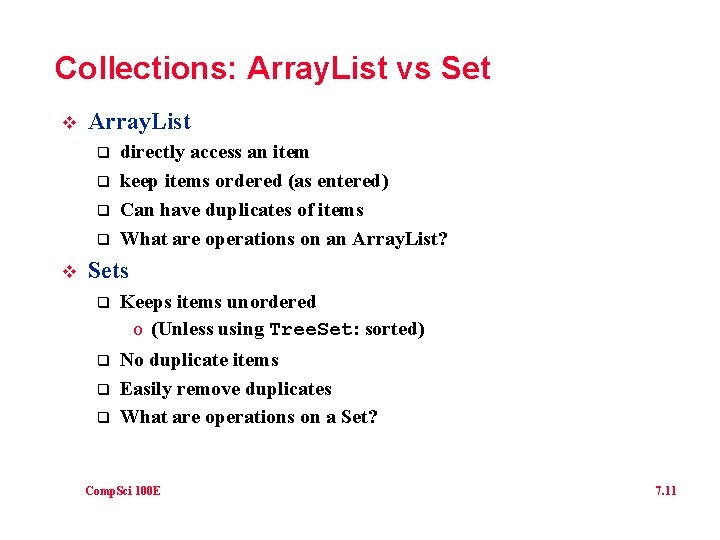
Collections: Array. List vs Set v Array. List q q v directly access an item keep items ordered (as entered) Can have duplicates of items What are operations on an Array. List? Sets q Keeps items unordered o (Unless using Tree. Set: sorted) q No duplicate items Easily remove duplicates What are operations on a Set? q q Comp. Sci 100 E 7. 11
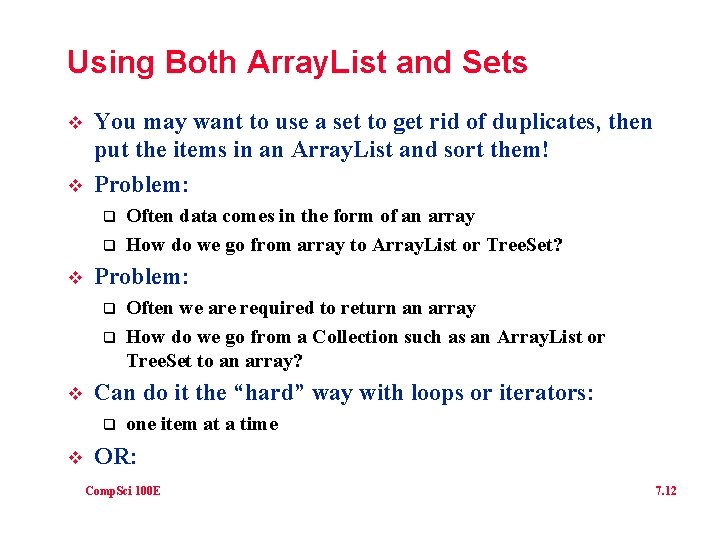
Using Both Array. List and Sets v v You may want to use a set to get rid of duplicates, then put the items in an Array. List and sort them! Problem: q q v Often we are required to return an array How do we go from a Collection such as an Array. List or Tree. Set to an array? Can do it the “hard” way with loops or iterators: q v Often data comes in the form of an array How do we go from array to Array. List or Tree. Set? one item at a time OR: Comp. Sci 100 E 7. 12
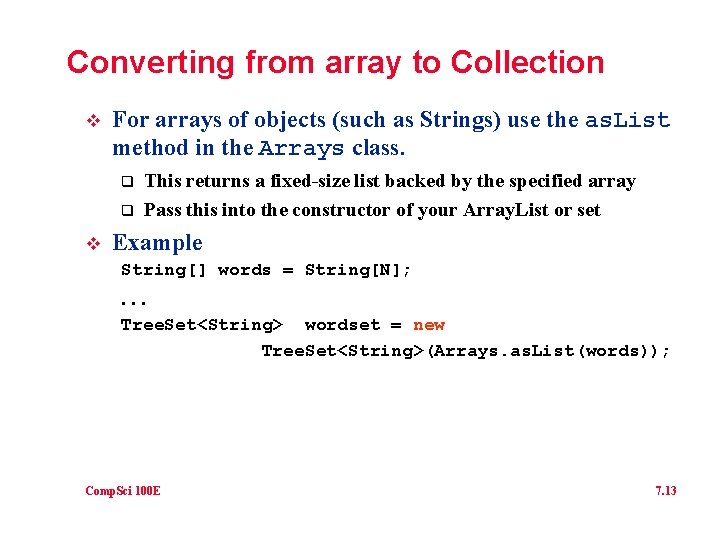
Converting from array to Collection v For arrays of objects (such as Strings) use the as. List method in the Arrays class. q q v This returns a fixed-size list backed by the specified array Pass this into the constructor of your Array. List or set Example String[] words = String[N]; . . . Tree. Set<String> wordset = new Tree. Set<String>(Arrays. as. List(words)); Comp. Sci 100 E 7. 13
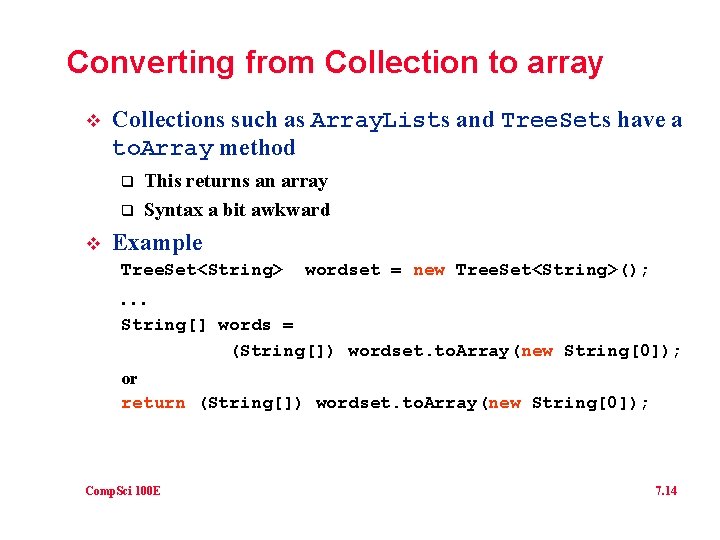
Converting from Collection to array v Collections such as Array. Lists and Tree. Sets have a to. Array method q q v This returns an array Syntax a bit awkward Example Tree. Set<String> wordset = new Tree. Set<String>(); . . . String[] words = (String[]) wordset. to. Array(new String[0]); or return (String[]) wordset. to. Array(new String[0]); Comp. Sci 100 E 7. 14
Hash collision
Unordered collection of objects
Total set awareness set consideration set
Training set validation set test set
Unordered tetrad analysis
Permutations
Unordered stem and leaf plot
Unordered subsets
What is the class width for the given class (28-33)
What set or sets of numbers does 450 belong?
Linked list representation of disjoint sets
Bounded set vs centered set
Crisp set vs fuzzy set
Crisp set vs fuzzy set
Crisp set vs fuzzy set