References Pointers CS1030 Dr Mark L Hornick 1
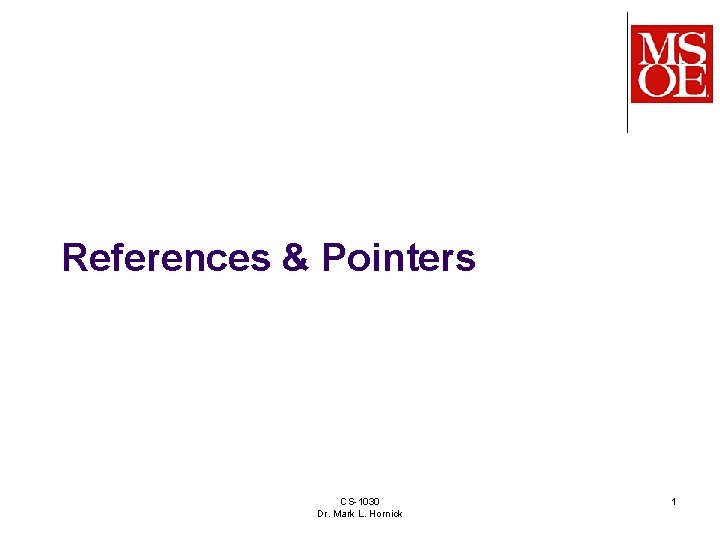
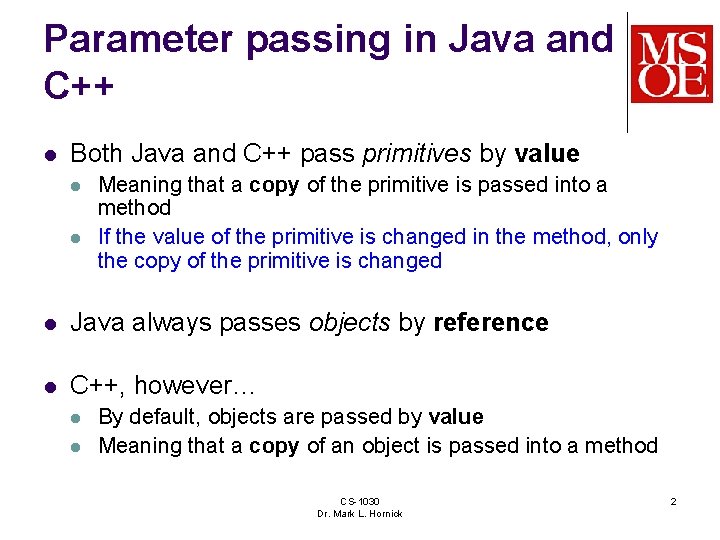
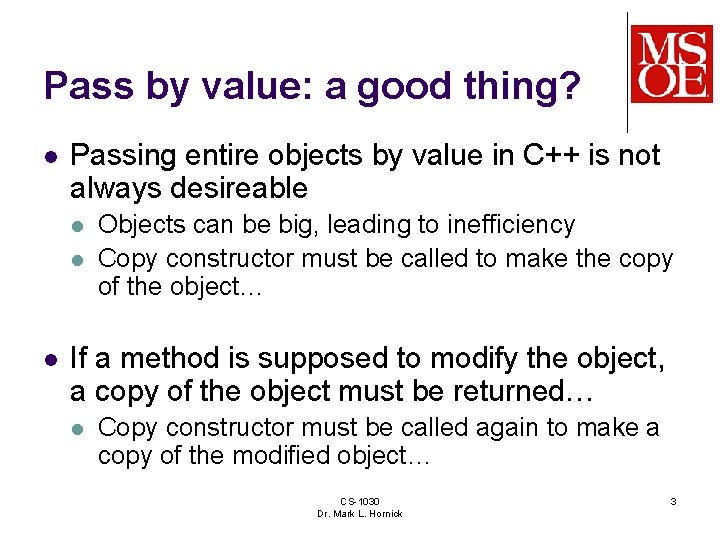
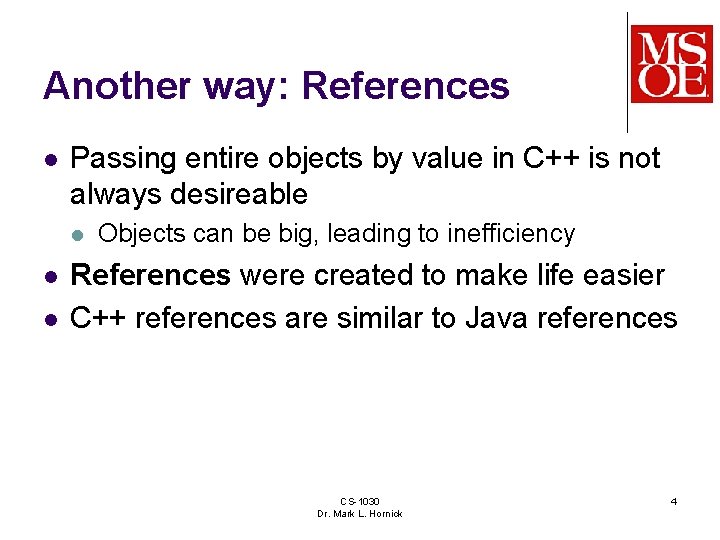
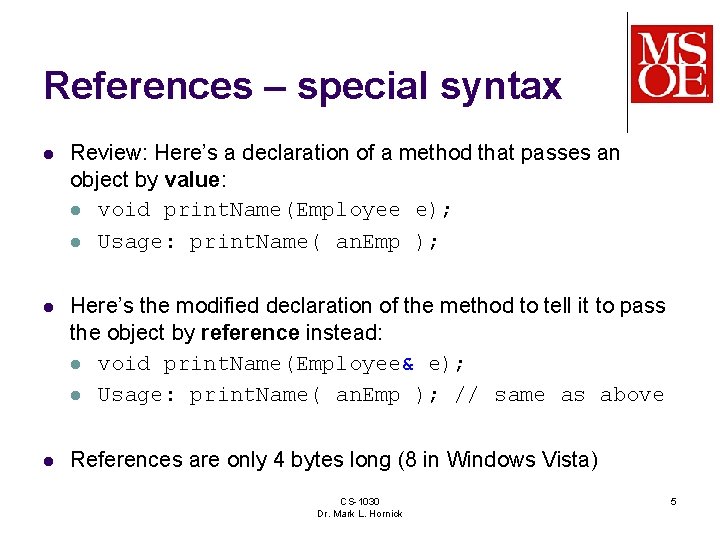
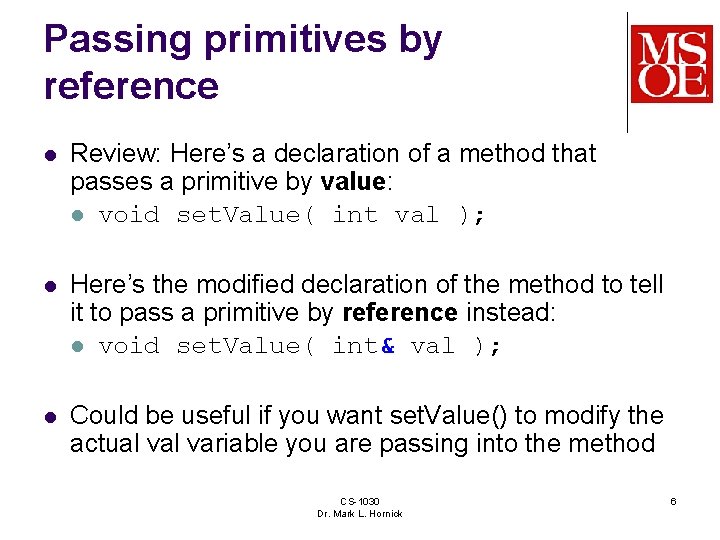
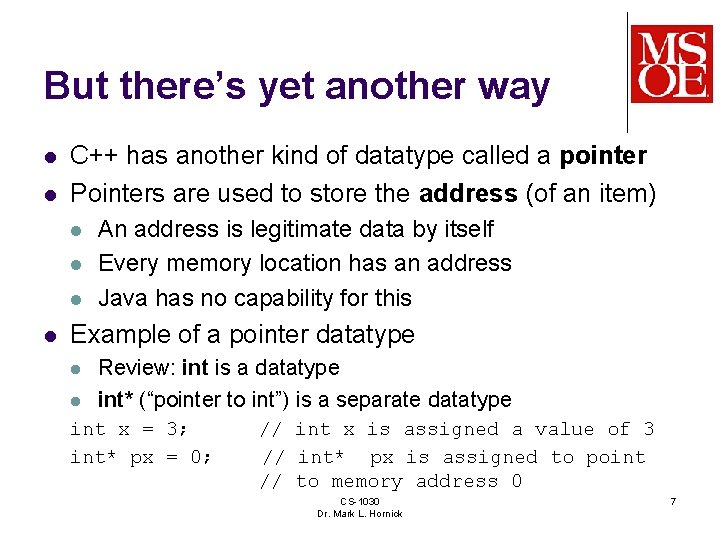
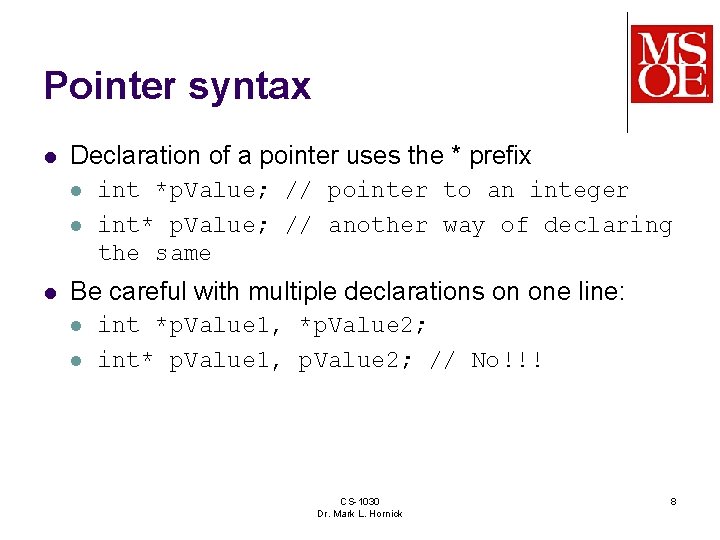
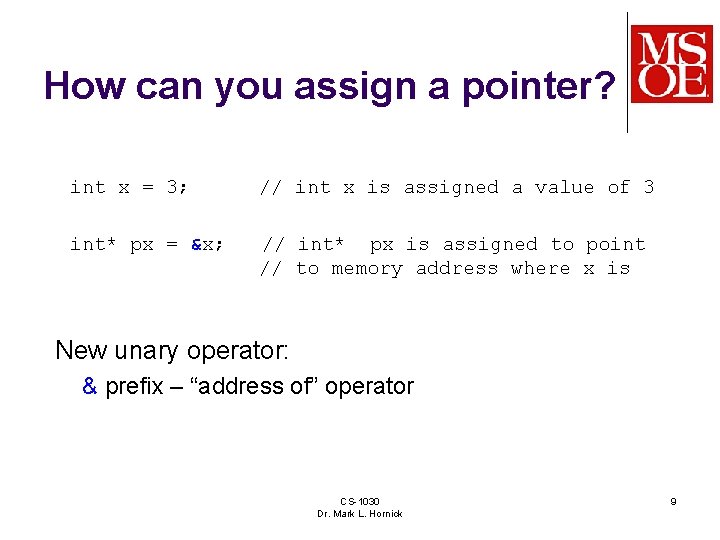
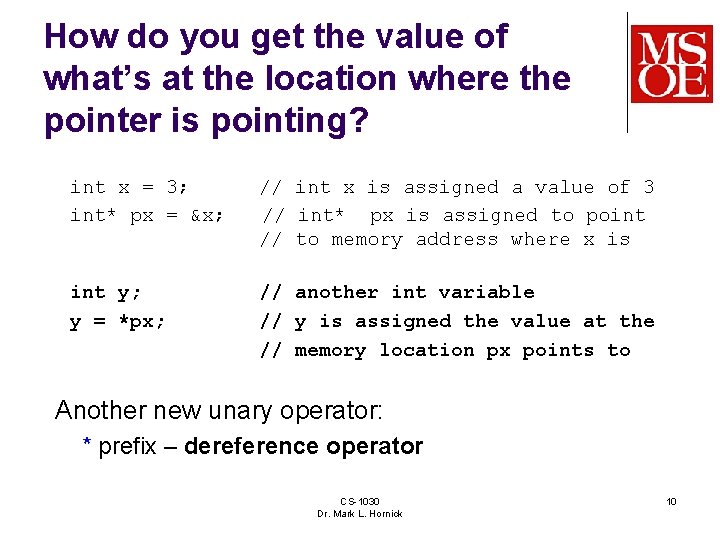
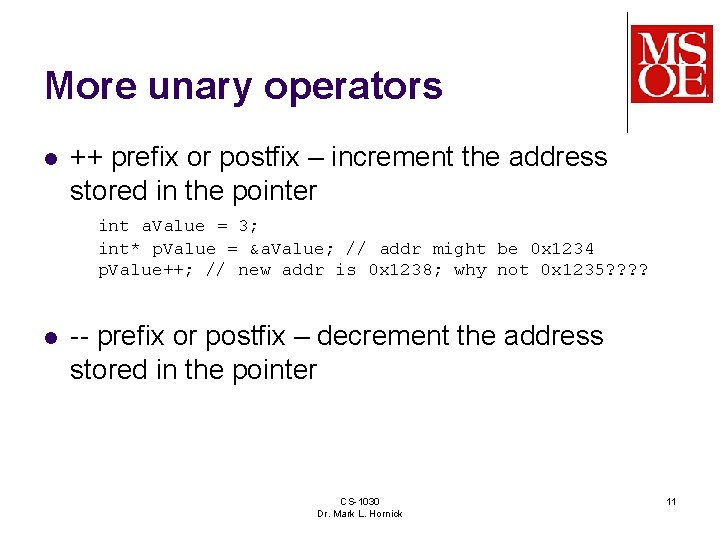
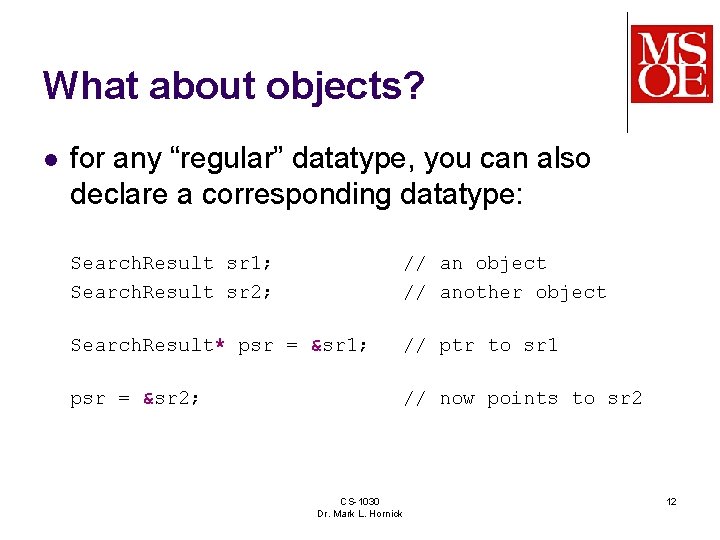
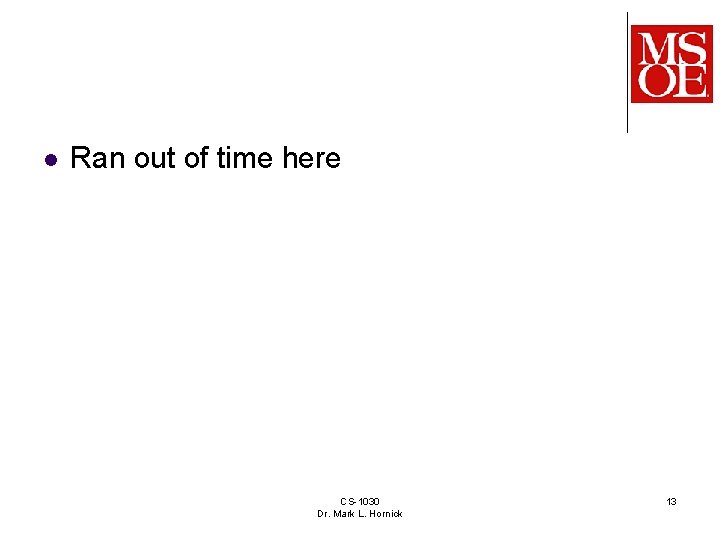
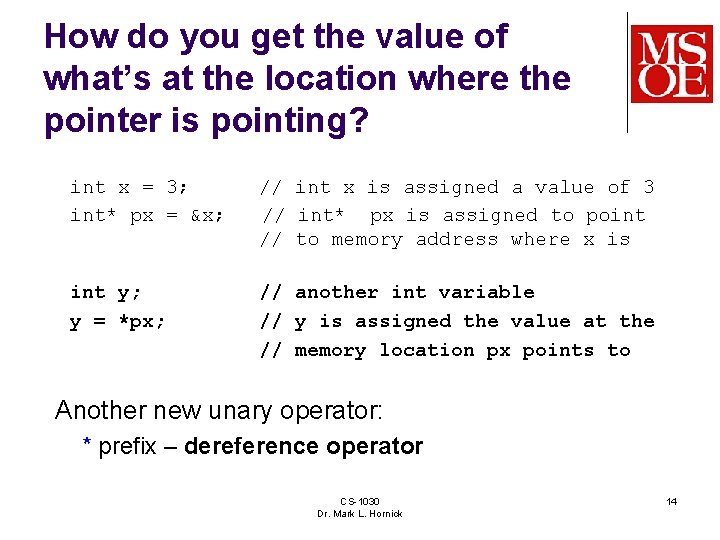
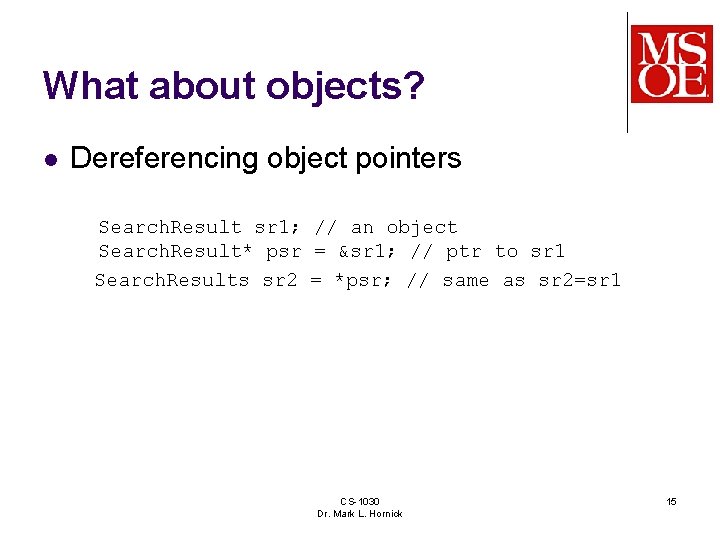
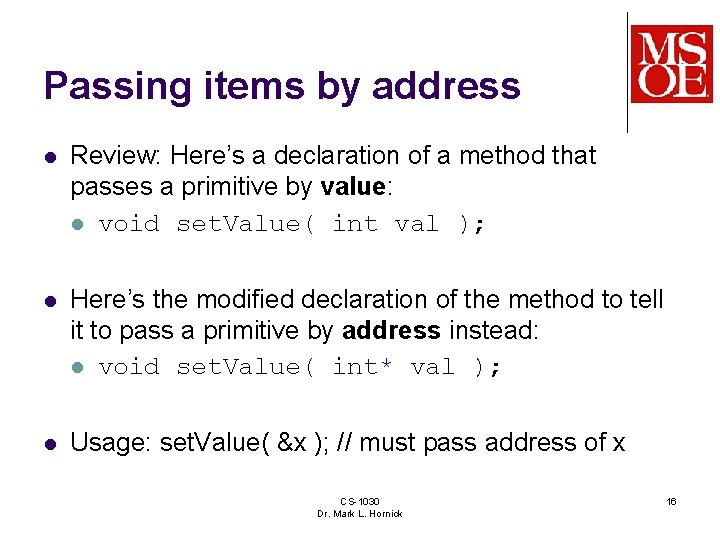
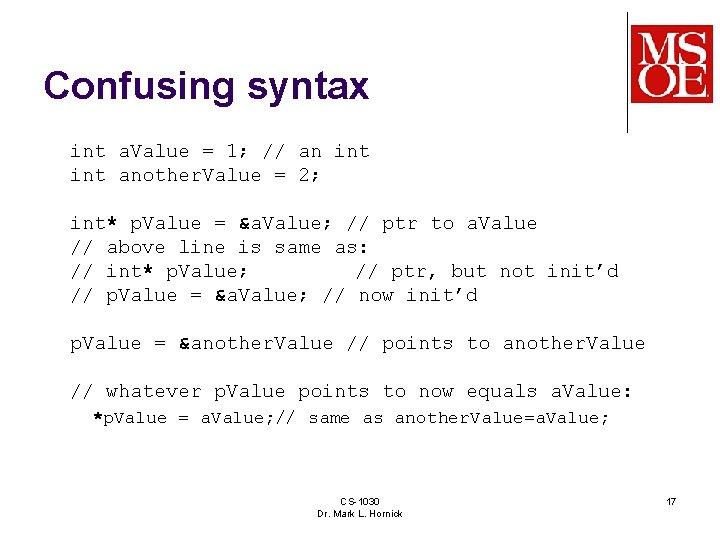
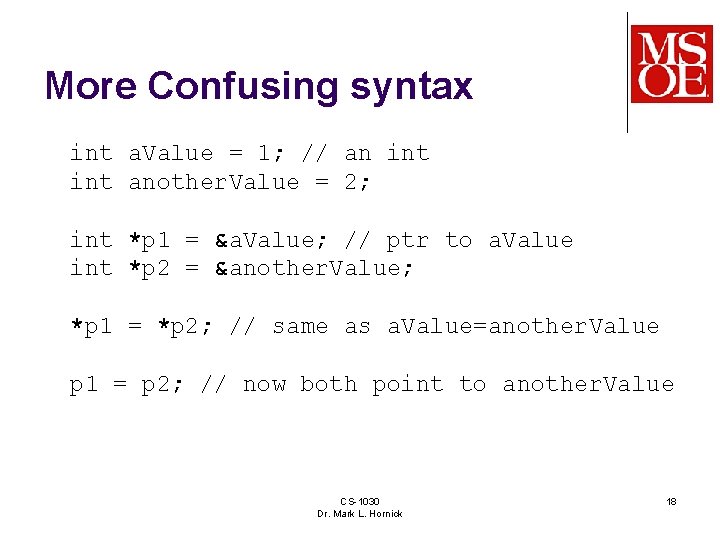
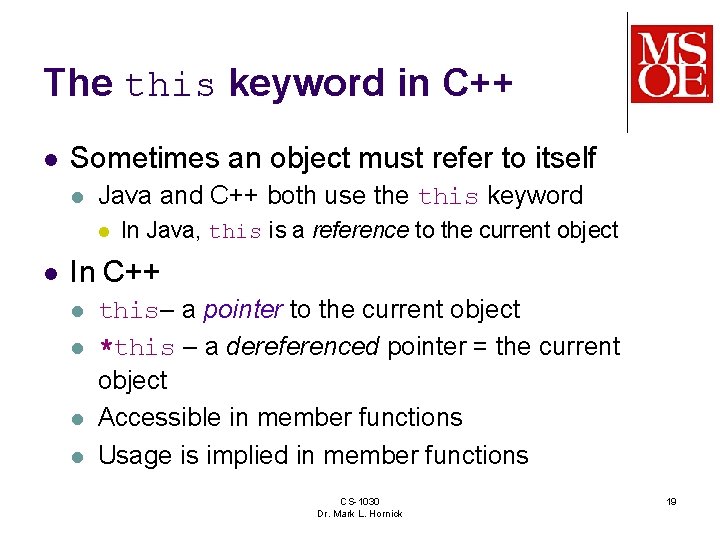
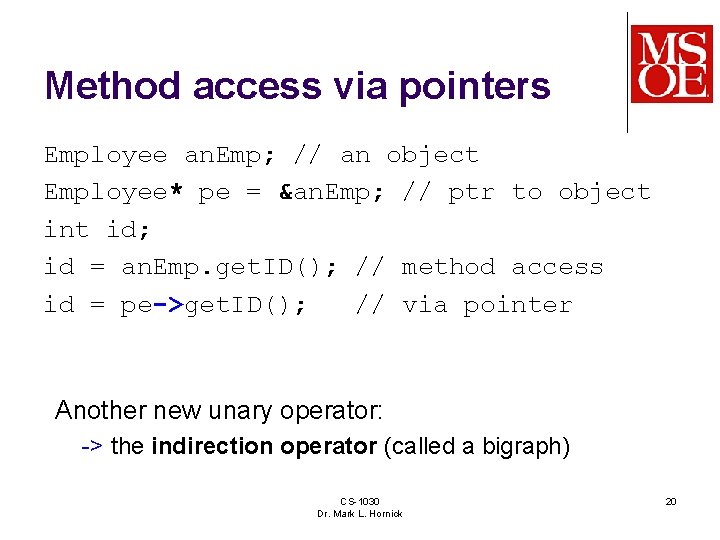
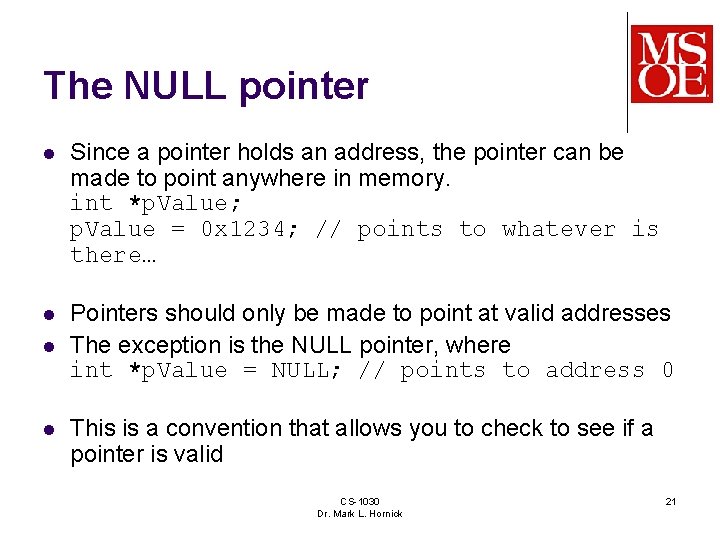
- Slides: 21
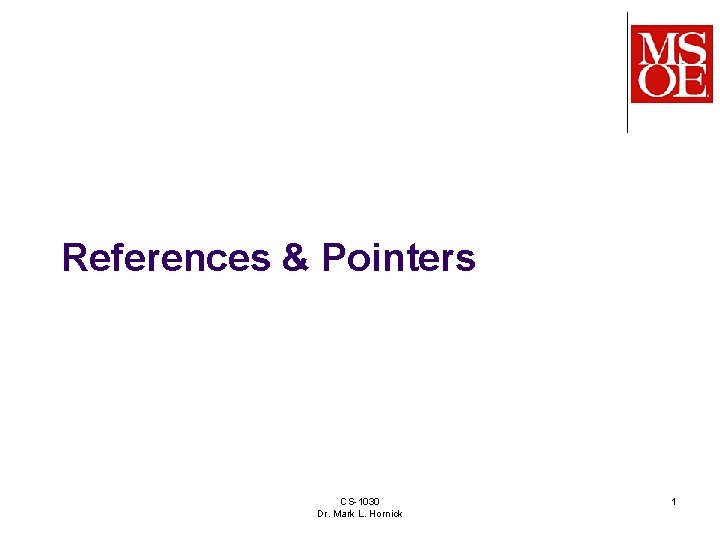
References & Pointers CS-1030 Dr. Mark L. Hornick 1
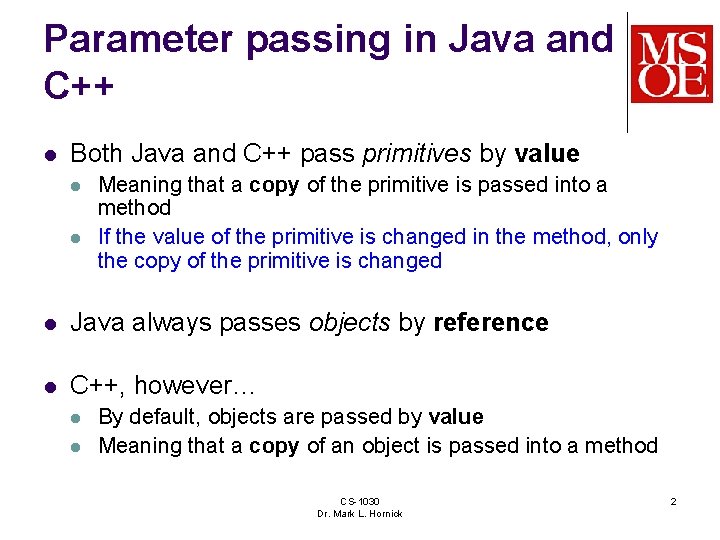
Parameter passing in Java and C++ l Both Java and C++ pass primitives by value l l Meaning that a copy of the primitive is passed into a method If the value of the primitive is changed in the method, only the copy of the primitive is changed l Java always passes objects by reference l C++, however… l l By default, objects are passed by value Meaning that a copy of an object is passed into a method CS-1030 Dr. Mark L. Hornick 2
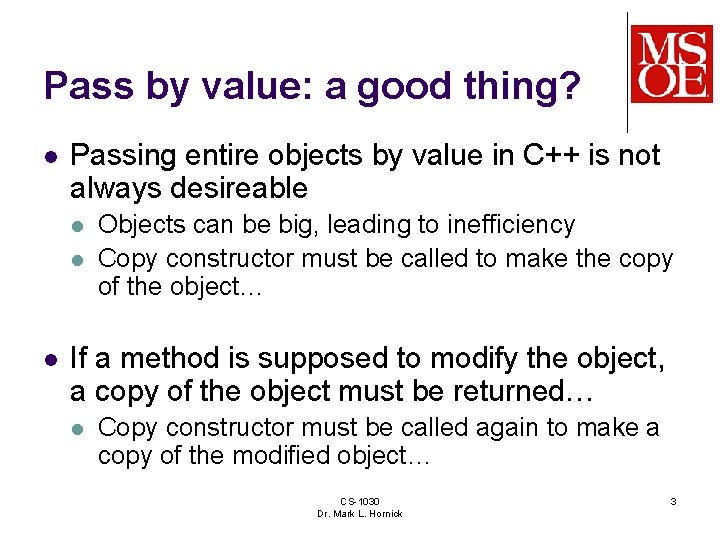
Pass by value: a good thing? l Passing entire objects by value in C++ is not always desireable l l l Objects can be big, leading to inefficiency Copy constructor must be called to make the copy of the object… If a method is supposed to modify the object, a copy of the object must be returned… l Copy constructor must be called again to make a copy of the modified object… CS-1030 Dr. Mark L. Hornick 3
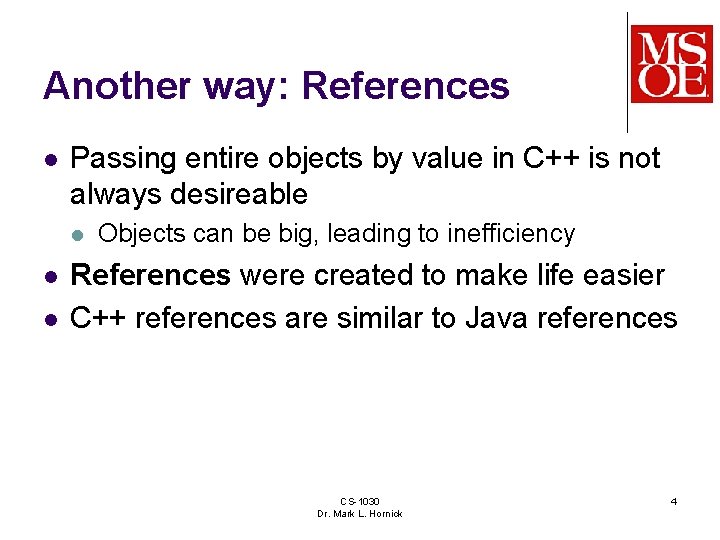
Another way: References l Passing entire objects by value in C++ is not always desireable l l l Objects can be big, leading to inefficiency References were created to make life easier C++ references are similar to Java references CS-1030 Dr. Mark L. Hornick 4
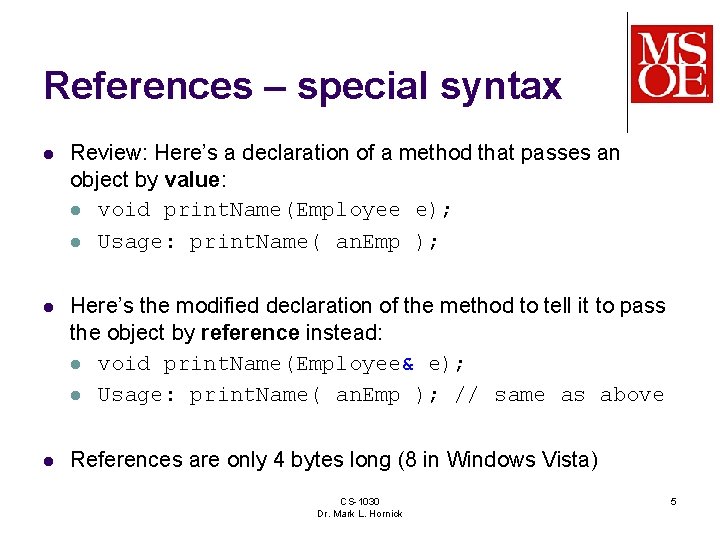
References – special syntax l Review: Here’s a declaration of a method that passes an object by value: l void print. Name(Employee e); l Usage: print. Name( an. Emp ); l Here’s the modified declaration of the method to tell it to pass the object by reference instead: l void print. Name(Employee& e); l Usage: print. Name( an. Emp ); // same as above l References are only 4 bytes long (8 in Windows Vista) CS-1030 Dr. Mark L. Hornick 5
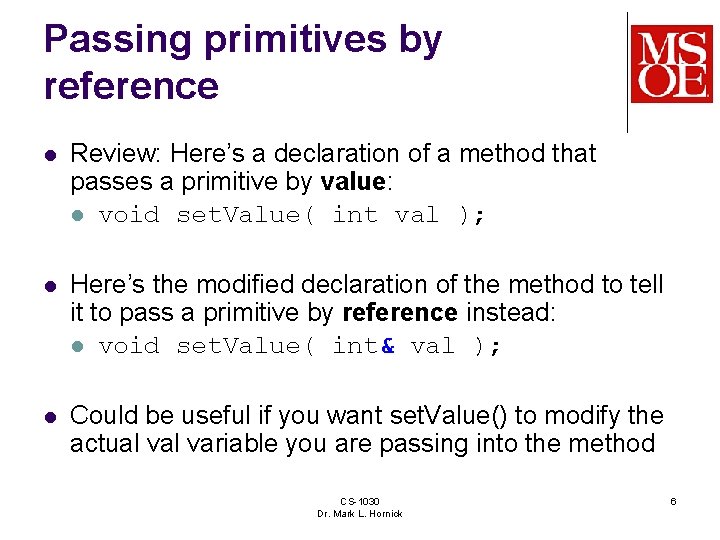
Passing primitives by reference l Review: Here’s a declaration of a method that passes a primitive by value: l void set. Value( int val ); l Here’s the modified declaration of the method to tell it to pass a primitive by reference instead: l void set. Value( int& val ); l Could be useful if you want set. Value() to modify the actual variable you are passing into the method CS-1030 Dr. Mark L. Hornick 6
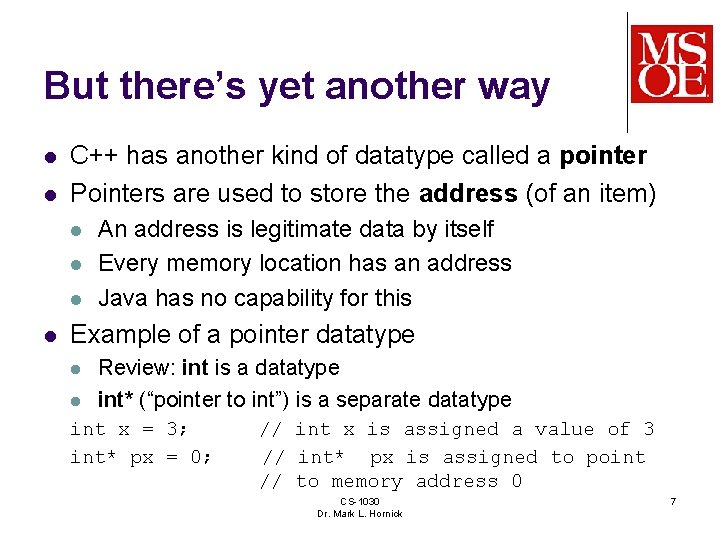
But there’s yet another way l l C++ has another kind of datatype called a pointer Pointers are used to store the address (of an item) l l An address is legitimate data by itself Every memory location has an address Java has no capability for this Example of a pointer datatype l l Review: int is a datatype int* (“pointer to int”) is a separate datatype int x = 3; int* px = 0; // int x is assigned a value of 3 // int* px is assigned to point // to memory address 0 CS-1030 Dr. Mark L. Hornick 7
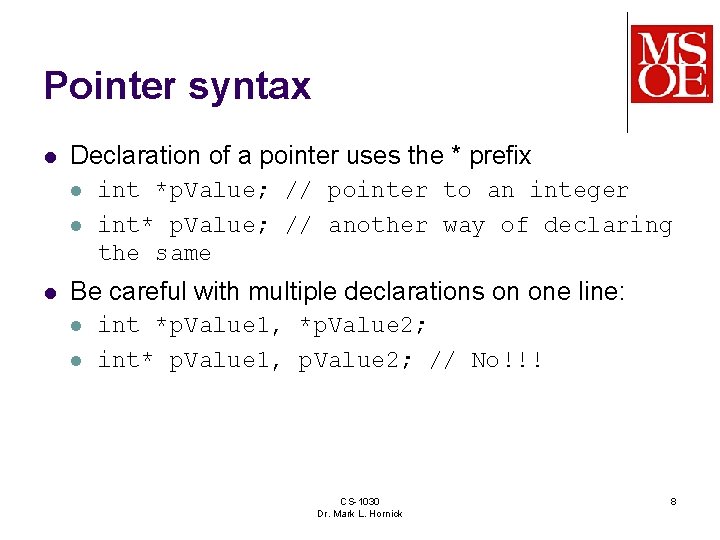
Pointer syntax l Declaration of a pointer uses the * prefix l l l int *p. Value; // pointer to an integer int* p. Value; // another way of declaring the same Be careful with multiple declarations on one line: l l int *p. Value 1, *p. Value 2; int* p. Value 1, p. Value 2; // No!!! CS-1030 Dr. Mark L. Hornick 8
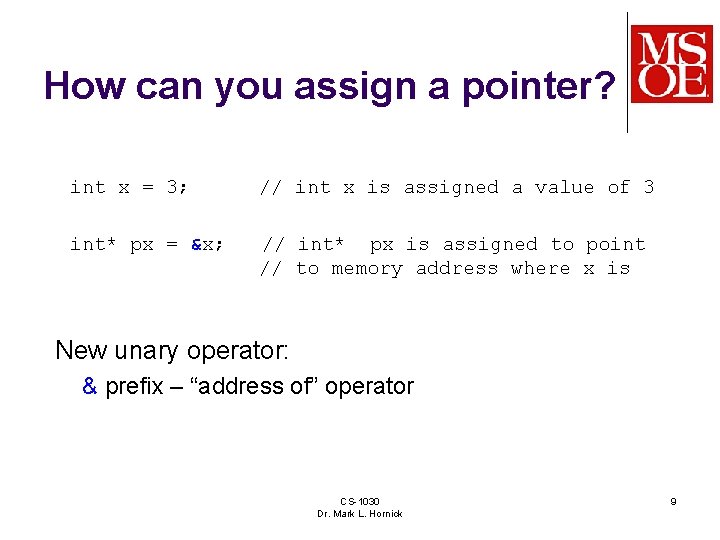
How can you assign a pointer? int x = 3; // int x is assigned a value of 3 int* px = &x; // int* px is assigned to point // to memory address where x is New unary operator: & prefix – “address of” operator CS-1030 Dr. Mark L. Hornick 9
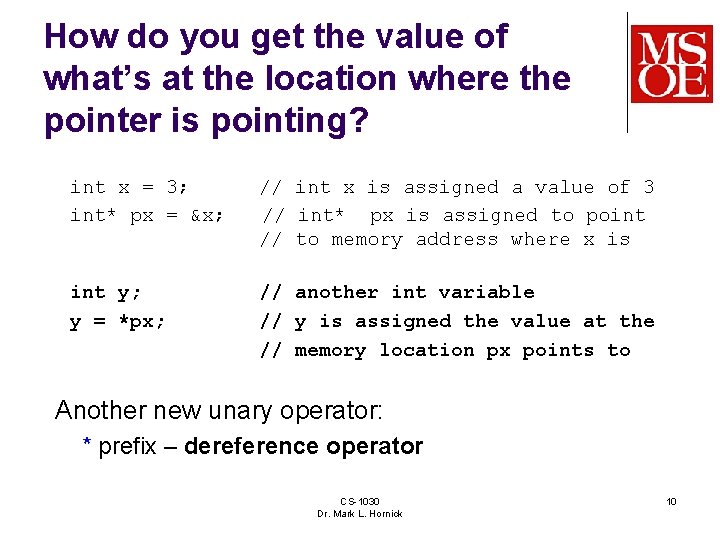
How do you get the value of what’s at the location where the pointer is pointing? int x = 3; int* px = &x; // int x is assigned a value of 3 // int* px is assigned to point // to memory address where x is int y; y = *px; // another int variable // y is assigned the value at the // memory location px points to Another new unary operator: * prefix – dereference operator CS-1030 Dr. Mark L. Hornick 10
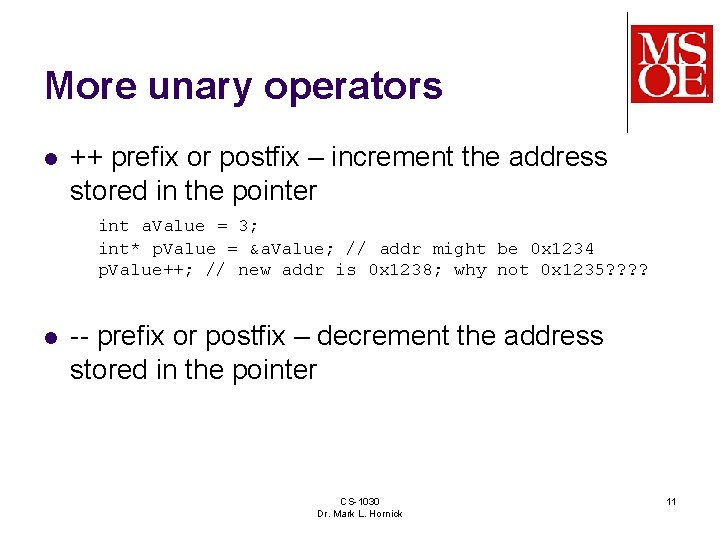
More unary operators l ++ prefix or postfix – increment the address stored in the pointer int a. Value = 3; int* p. Value = &a. Value; // addr might be 0 x 1234 p. Value++; // new addr is 0 x 1238; why not 0 x 1235? ? l -- prefix or postfix – decrement the address stored in the pointer CS-1030 Dr. Mark L. Hornick 11
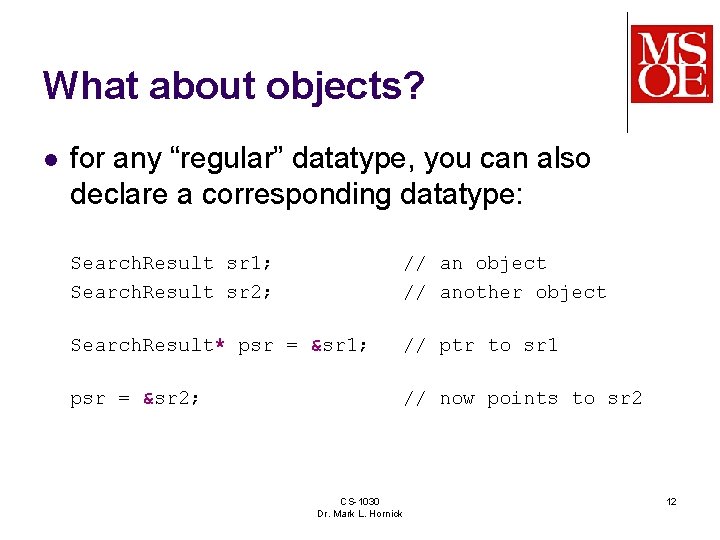
What about objects? l for any “regular” datatype, you can also declare a corresponding datatype: Search. Result sr 1; Search. Result sr 2; // an object // another object Search. Result* psr = &sr 1; // ptr to sr 1 psr = &sr 2; // now points to sr 2 CS-1030 Dr. Mark L. Hornick 12
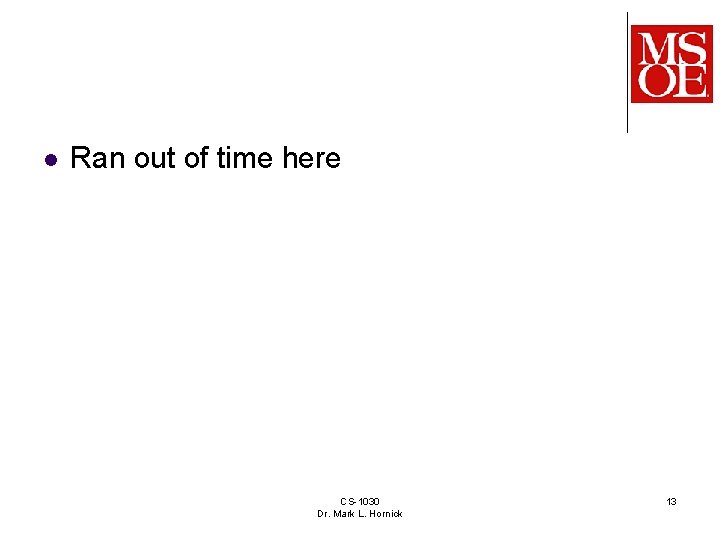
l Ran out of time here CS-1030 Dr. Mark L. Hornick 13
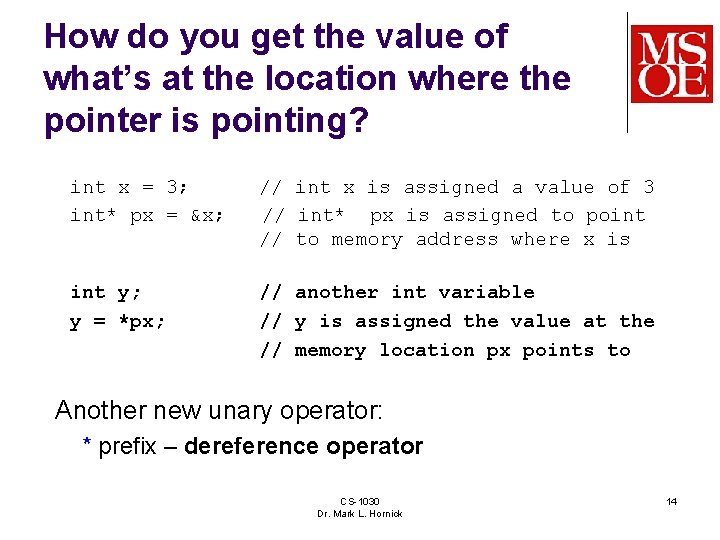
How do you get the value of what’s at the location where the pointer is pointing? int x = 3; int* px = &x; // int x is assigned a value of 3 // int* px is assigned to point // to memory address where x is int y; y = *px; // another int variable // y is assigned the value at the // memory location px points to Another new unary operator: * prefix – dereference operator CS-1030 Dr. Mark L. Hornick 14
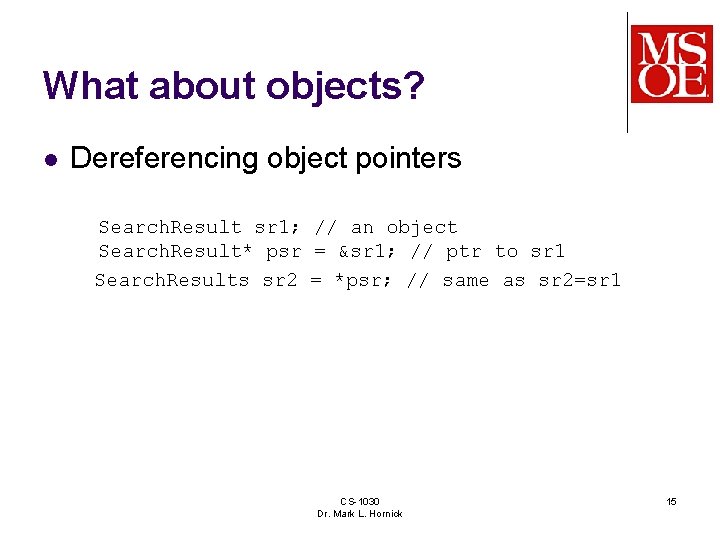
What about objects? l Dereferencing object pointers Search. Result sr 1; // an object Search. Result* psr = &sr 1; // ptr to sr 1 Search. Results sr 2 = *psr; // same as sr 2=sr 1 CS-1030 Dr. Mark L. Hornick 15
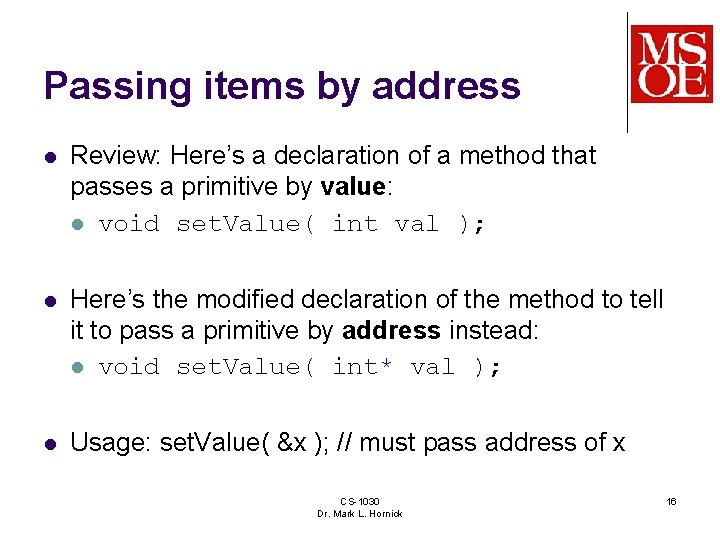
Passing items by address l Review: Here’s a declaration of a method that passes a primitive by value: l void set. Value( int val ); l Here’s the modified declaration of the method to tell it to pass a primitive by address instead: l void set. Value( int* val ); l Usage: set. Value( &x ); // must pass address of x CS-1030 Dr. Mark L. Hornick 16
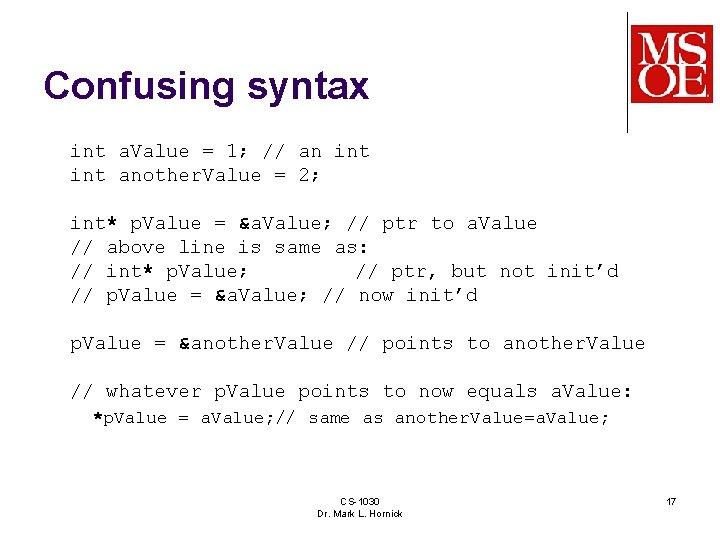
Confusing syntax int a. Value = 1; // an int another. Value = 2; int* p. Value = &a. Value; // ptr to a. Value // above line is same as: // int* p. Value; // ptr, but not init’d // p. Value = &a. Value; // now init’d p. Value = &another. Value // points to another. Value // whatever p. Value points to now equals a. Value: *p. Value = a. Value; // same as another. Value=a. Value; CS-1030 Dr. Mark L. Hornick 17
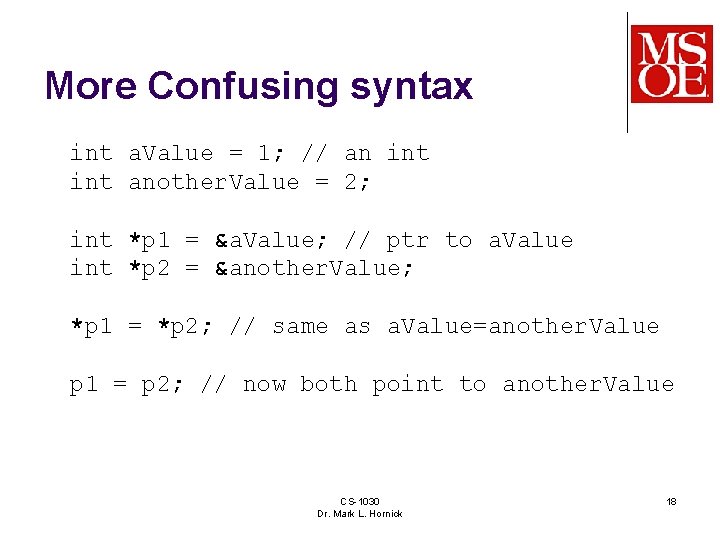
More Confusing syntax int a. Value = 1; // an int another. Value = 2; int *p 1 = &a. Value; // ptr to a. Value int *p 2 = &another. Value; *p 1 = *p 2; // same as a. Value=another. Value p 1 = p 2; // now both point to another. Value CS-1030 Dr. Mark L. Hornick 18
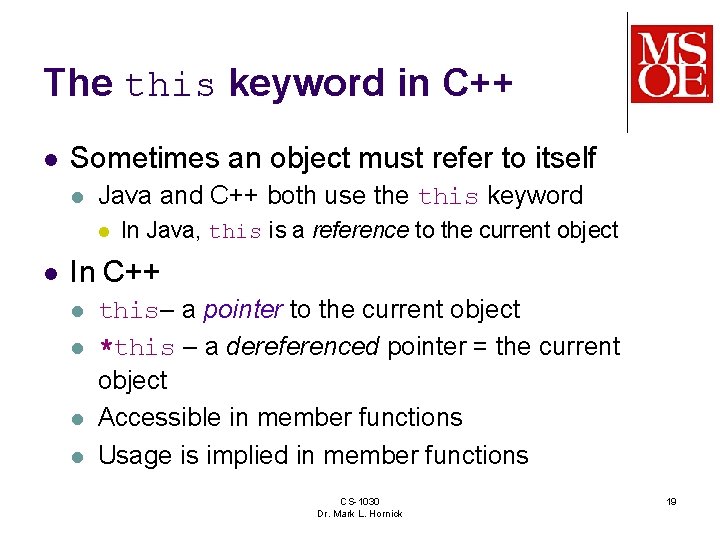
The this keyword in C++ l Sometimes an object must refer to itself l Java and C++ both use this keyword l l In Java, this is a reference to the current object In C++ l l this– a pointer to the current object *this – a dereferenced pointer = the current object Accessible in member functions Usage is implied in member functions CS-1030 Dr. Mark L. Hornick 19
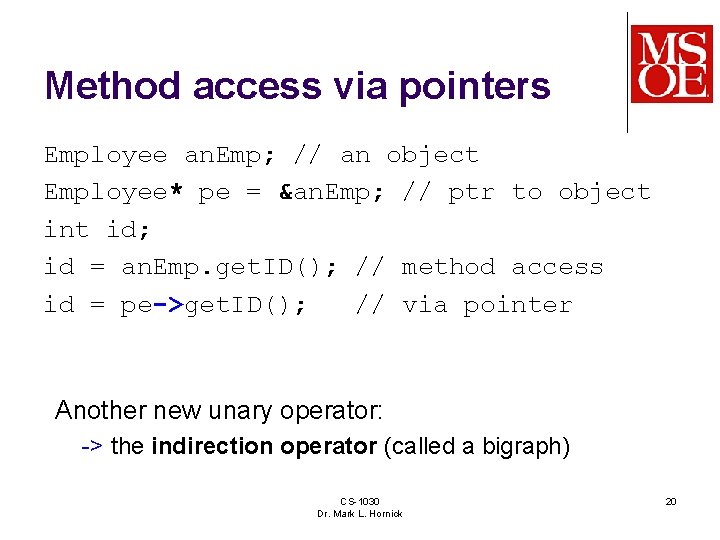
Method access via pointers Employee an. Emp; // an object Employee* pe = &an. Emp; // ptr to object int id; id = an. Emp. get. ID(); // method access id = pe->get. ID(); // via pointer Another new unary operator: -> the indirection operator (called a bigraph) CS-1030 Dr. Mark L. Hornick 20
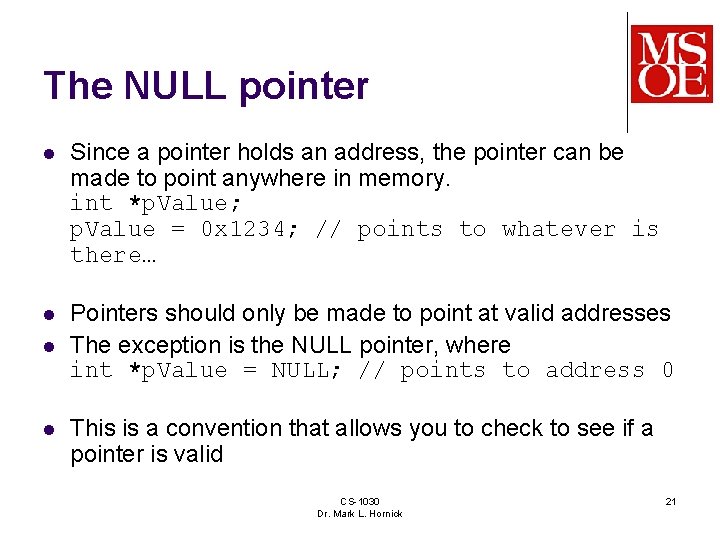
The NULL pointer l Since a pointer holds an address, the pointer can be made to point anywhere in memory. int *p. Value; p. Value = 0 x 1234; // points to whatever is there… l Pointers should only be made to point at valid addresses The exception is the NULL pointer, where int *p. Value = NULL; // points to address 0 l l This is a convention that allows you to check to see if a pointer is valid CS-1030 Dr. Mark L. Hornick 21
& vs * in c
& vs * in c
Swizzling glsl
Skip pointer
9 pointers
Science logbook example
Skip pointers in information retrieval
Pointers and strings
File pointers in c
Pointers are variables that contain as their values
Importance of pointers in c
Hazard pointers
Shuffle left algorithm
Pointers are variables that contain
For skip pointer more skip leads to
I-need-a-few-pointers-98hpou5
Pointers in assembly
Pointers in java
C array of pointers to structs
Converging pointers algorithm
12 pointers
Xkcd pointers