PYTHON DICTIONARY WHAT IS A DICTIONARY A dictionary
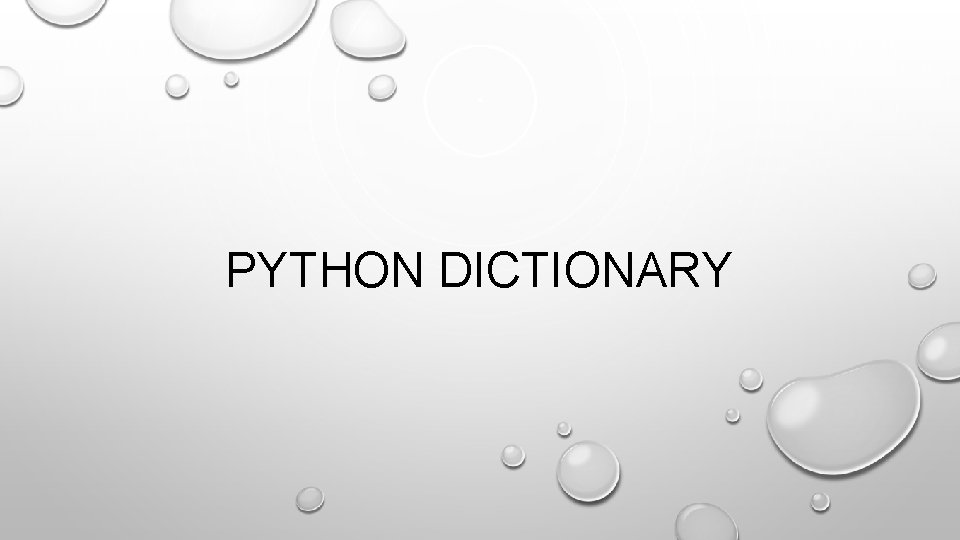
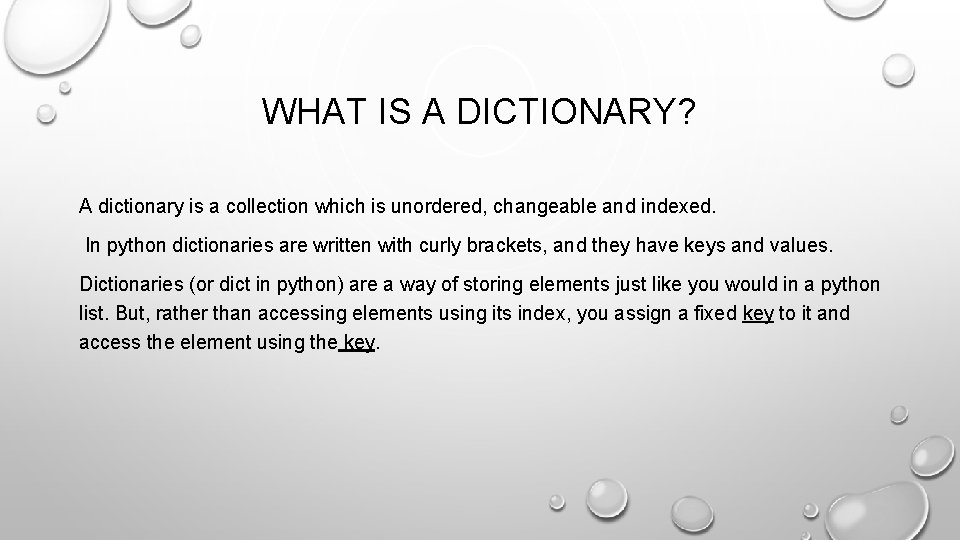
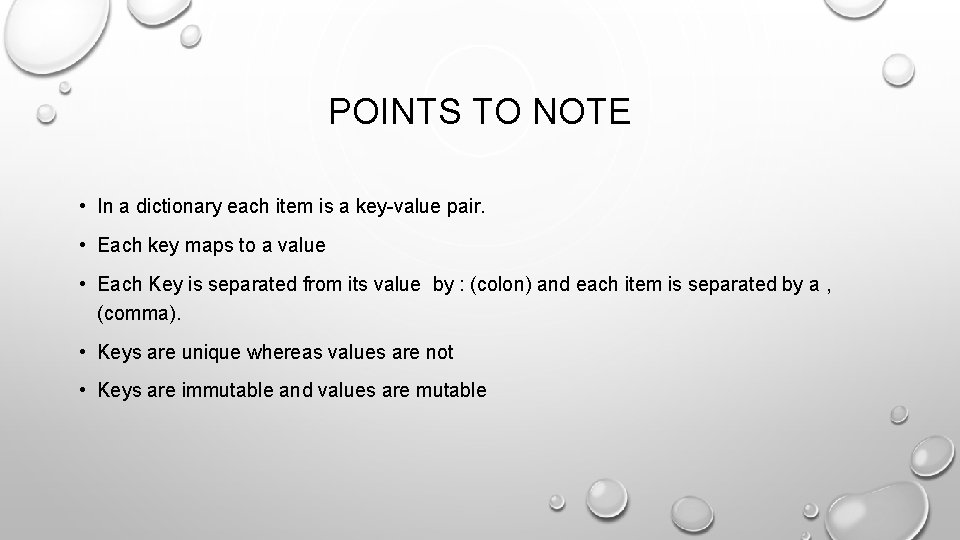
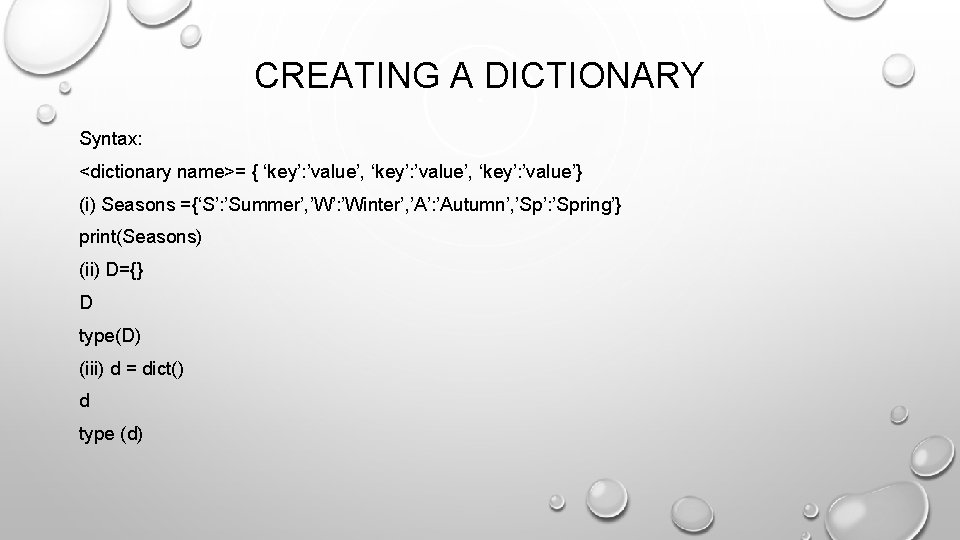
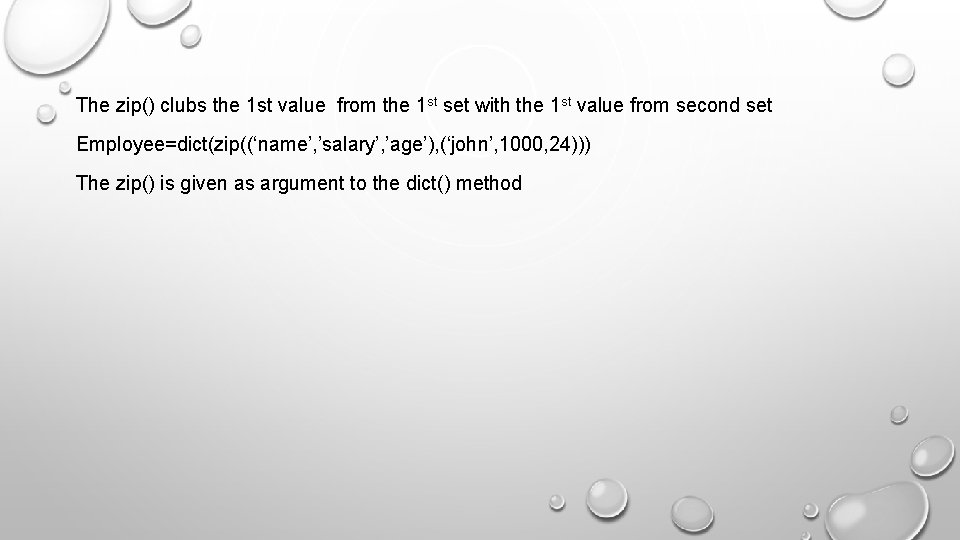
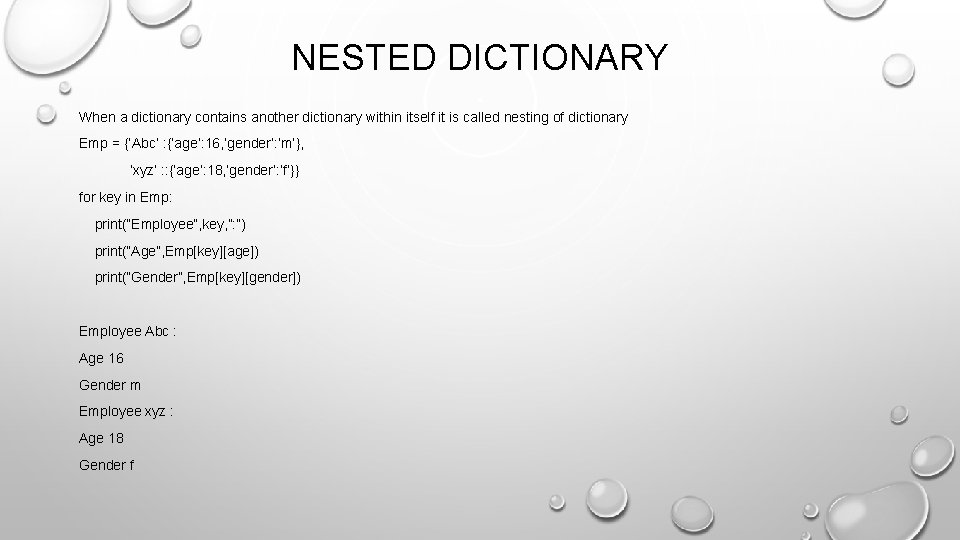
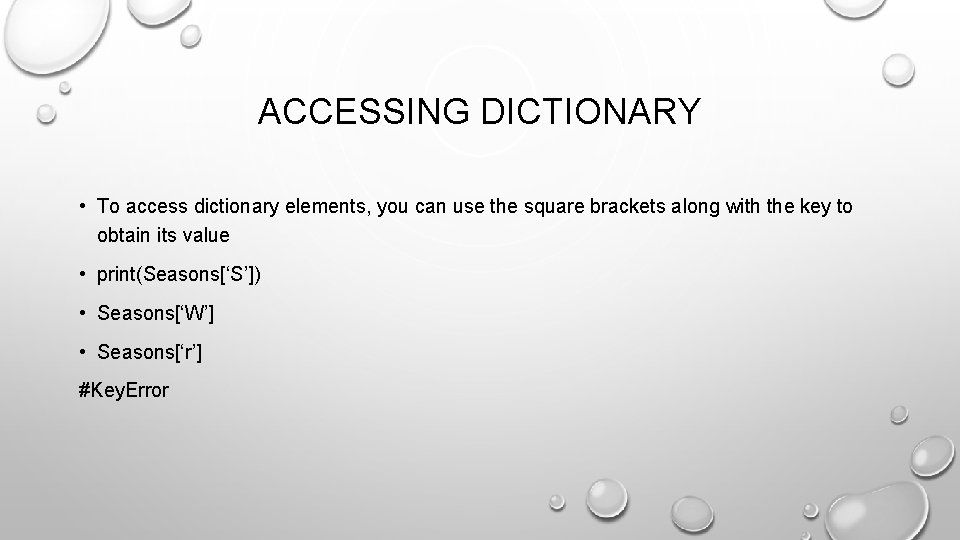
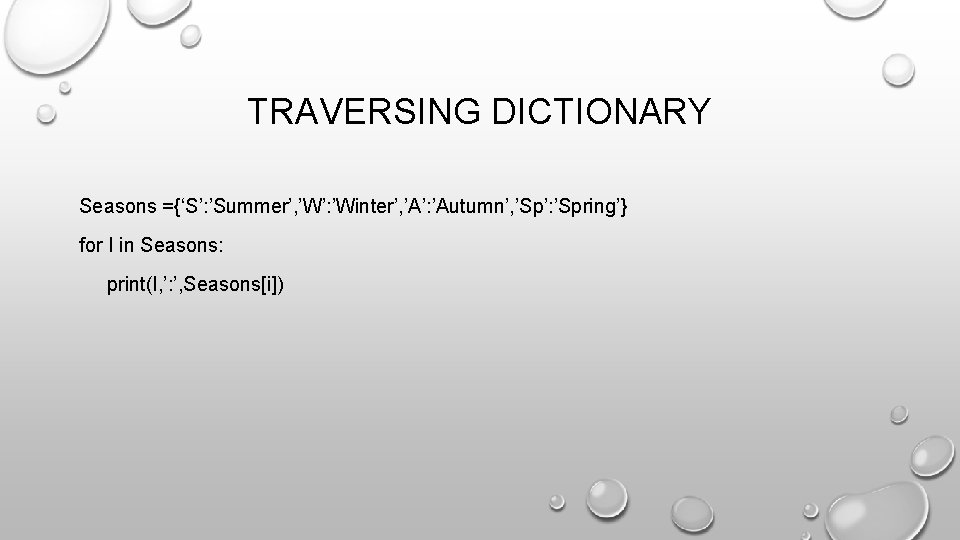
![APPENDING DICTIONARY SYNTAX : <dictionary name >[key]=value Example d={10: ’Ten’, 20: ’Twenty’, 30: ’Thirty’} APPENDING DICTIONARY SYNTAX : <dictionary name >[key]=value Example d={10: ’Ten’, 20: ’Twenty’, 30: ’Thirty’}](https://slidetodoc.com/presentation_image_h2/0fce8ad800a274495137d573875cb0e9/image-9.jpg)
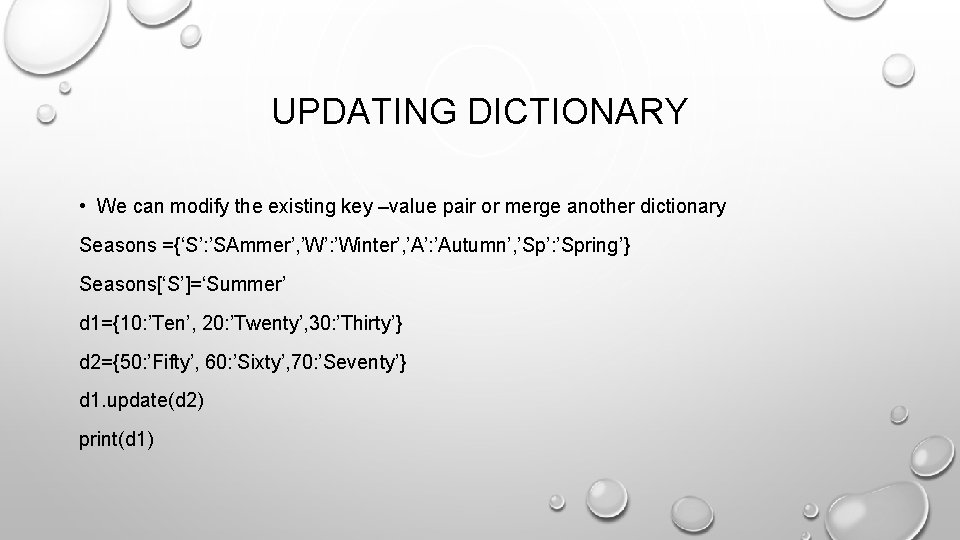
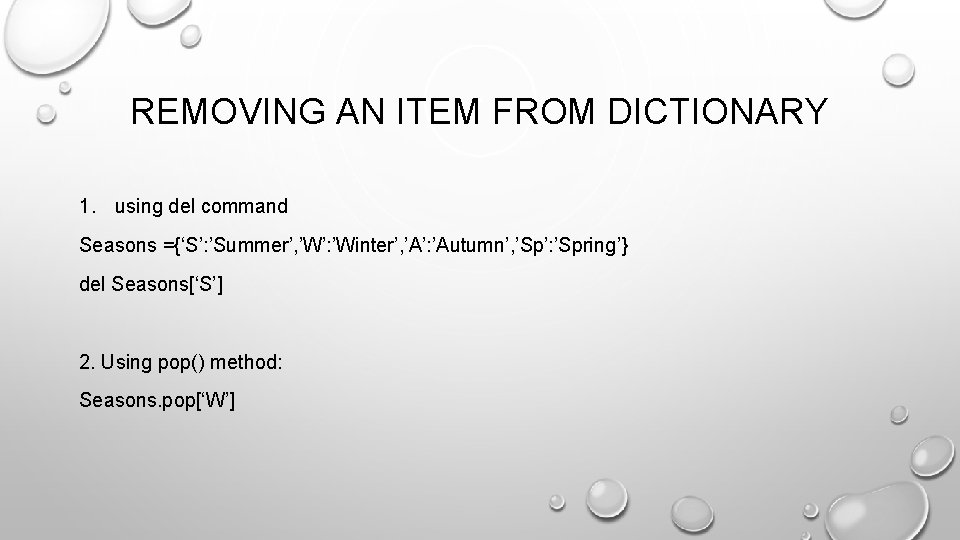
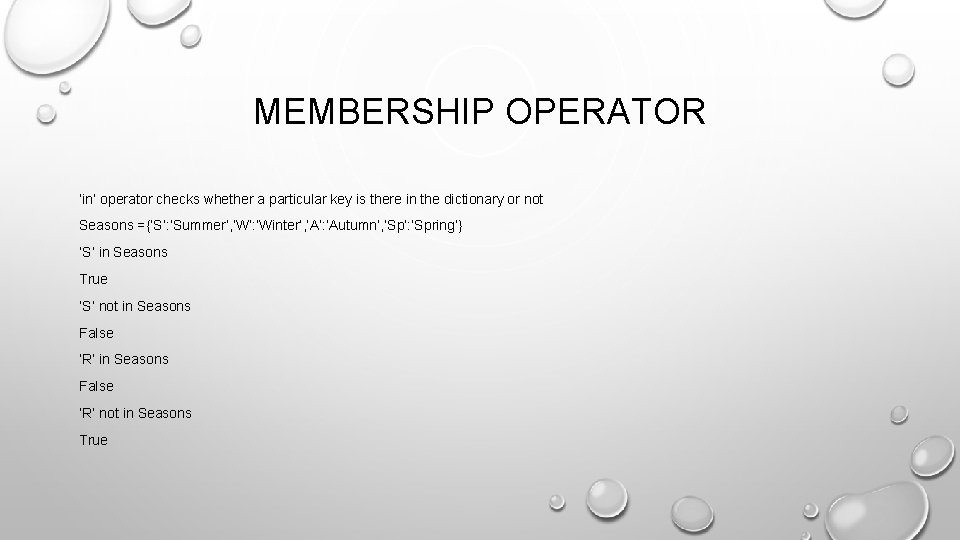
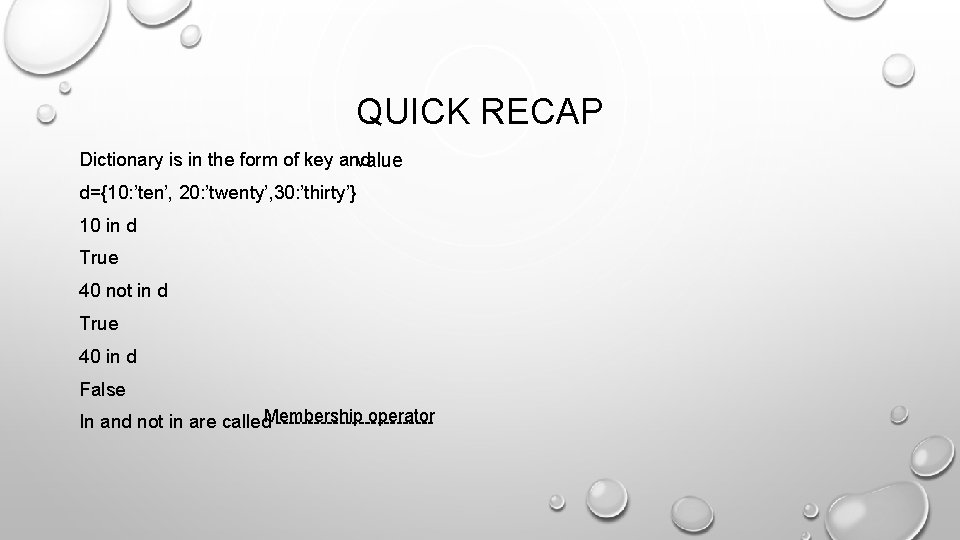
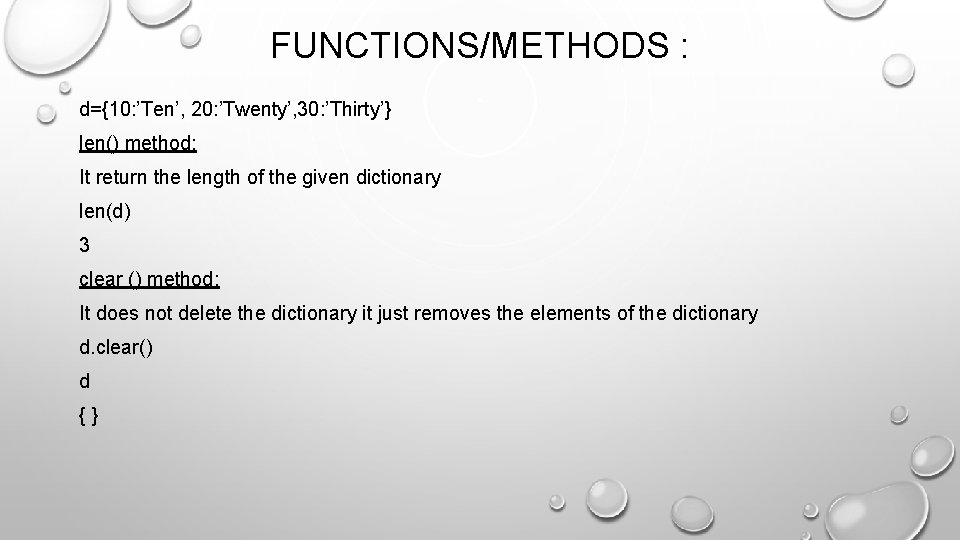
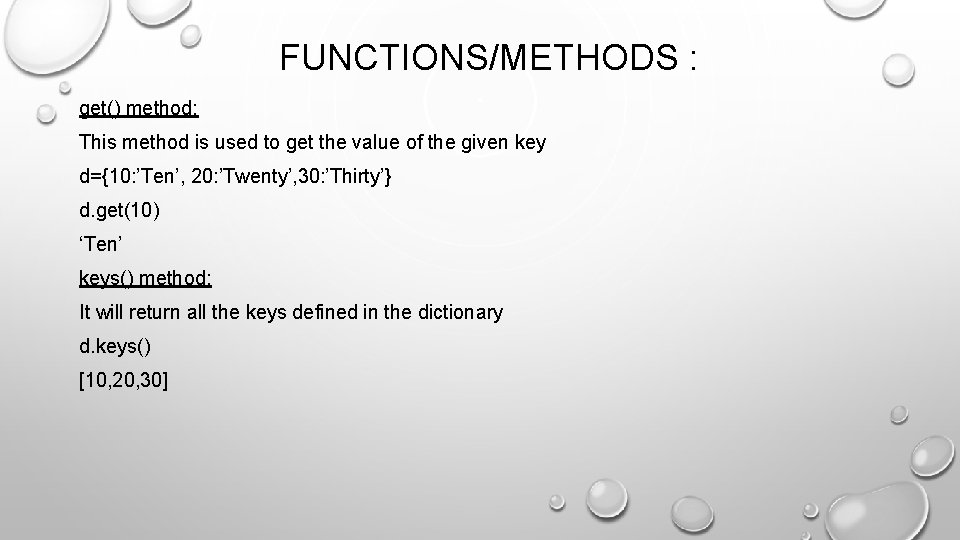
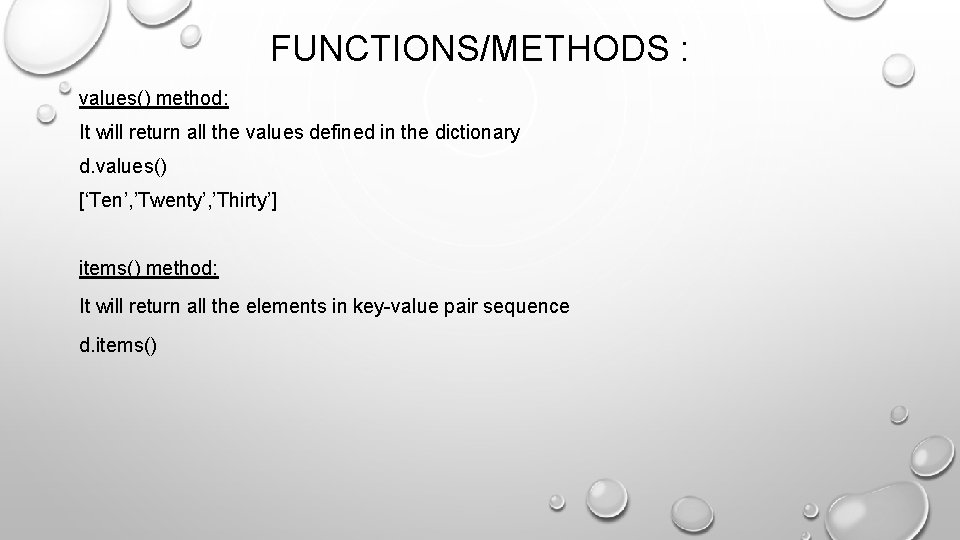
- Slides: 16
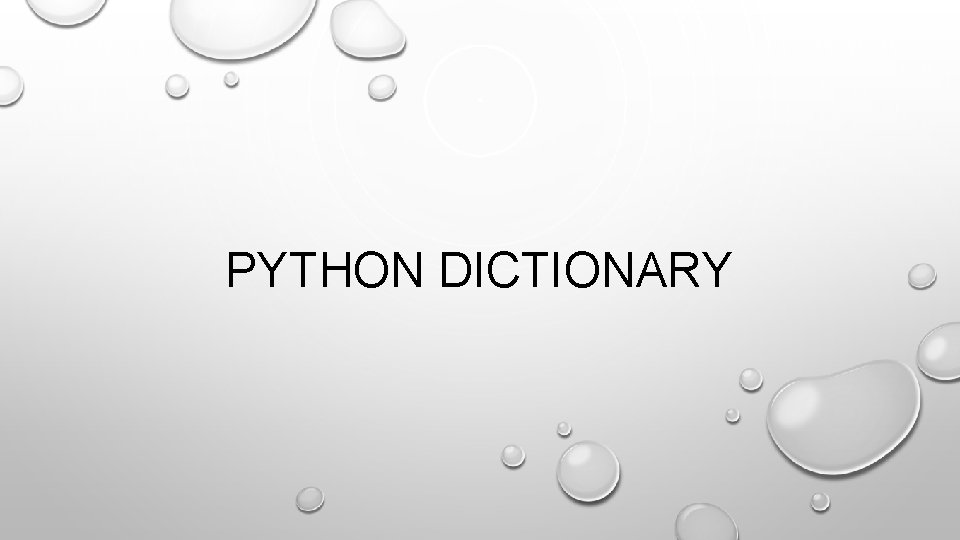
PYTHON DICTIONARY
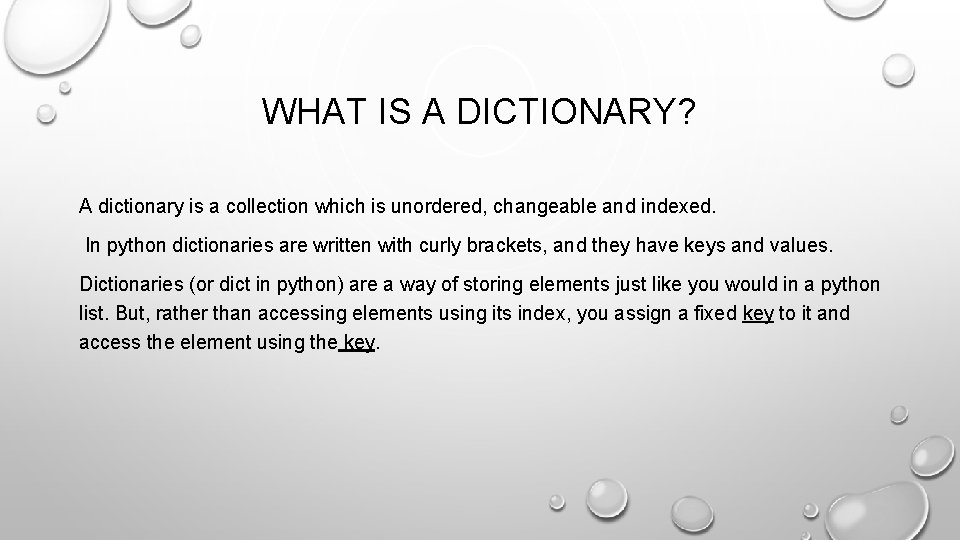
WHAT IS A DICTIONARY? A dictionary is a collection which is unordered, changeable and indexed. In python dictionaries are written with curly brackets, and they have keys and values. Dictionaries (or dict in python) are a way of storing elements just like you would in a python list. But, rather than accessing elements using its index, you assign a fixed key to it and access the element using the key.
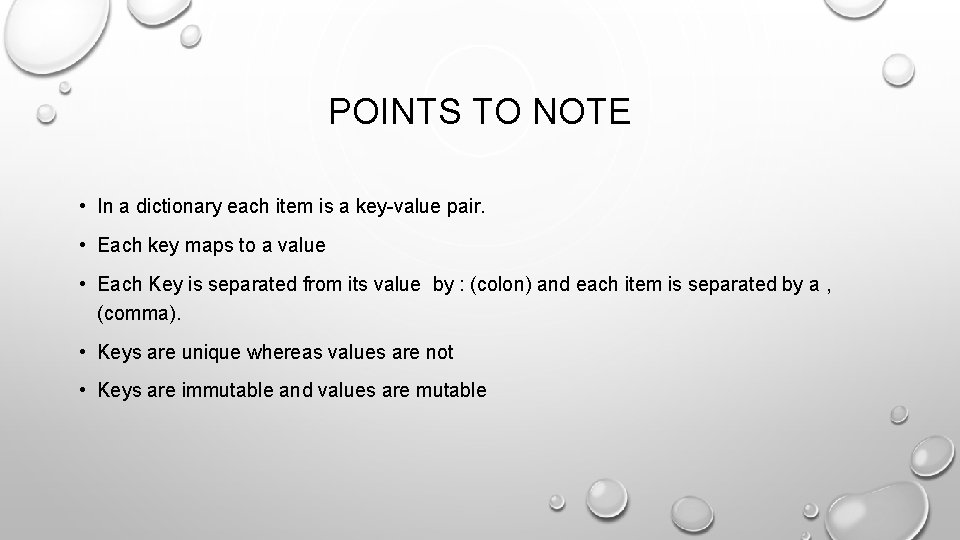
POINTS TO NOTE • In a dictionary each item is a key-value pair. • Each key maps to a value • Each Key is separated from its value by : (colon) and each item is separated by a , (comma). • Keys are unique whereas values are not • Keys are immutable and values are mutable
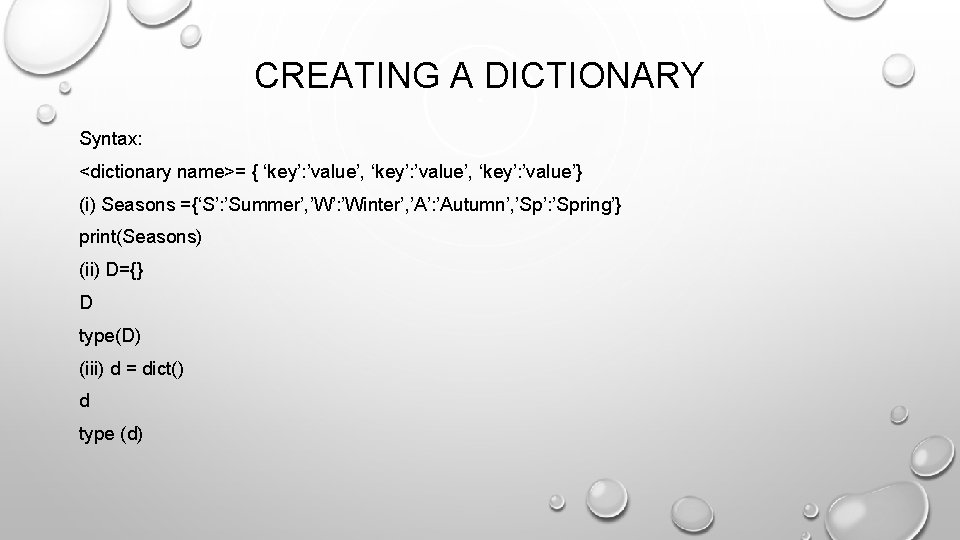
CREATING A DICTIONARY Syntax: <dictionary name>= { ‘key’: ’value’, ‘key’: ’value’} (i) Seasons ={‘S’: ’Summer’, ’W’: ’Winter’, ’A’: ’Autumn’, ’Sp’: ’Spring’} print(Seasons) (ii) D={} D type(D) (iii) d = dict() d type (d)
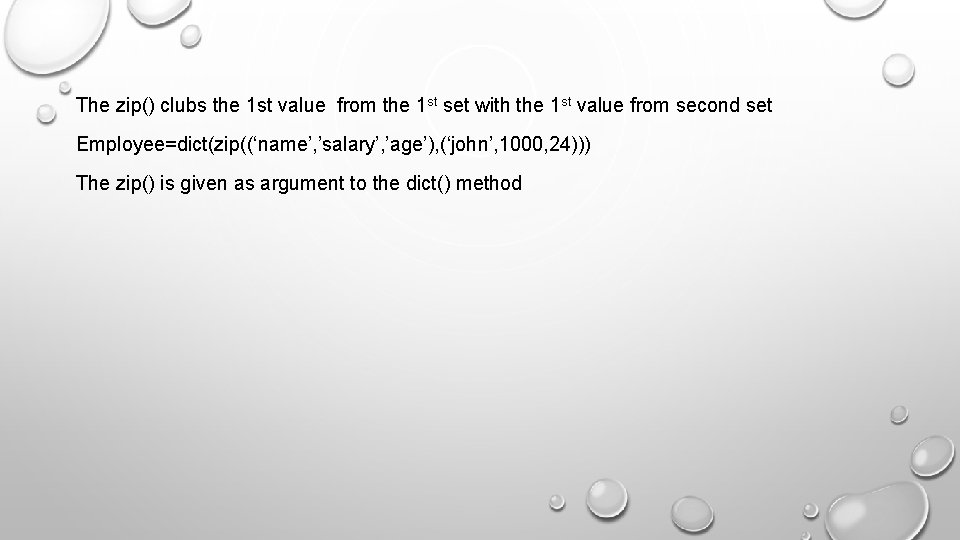
The zip() clubs the 1 st value from the 1 st set with the 1 st value from second set Employee=dict(zip((‘name’, ’salary’, ’age’), (‘john’, 1000, 24))) The zip() is given as argument to the dict() method
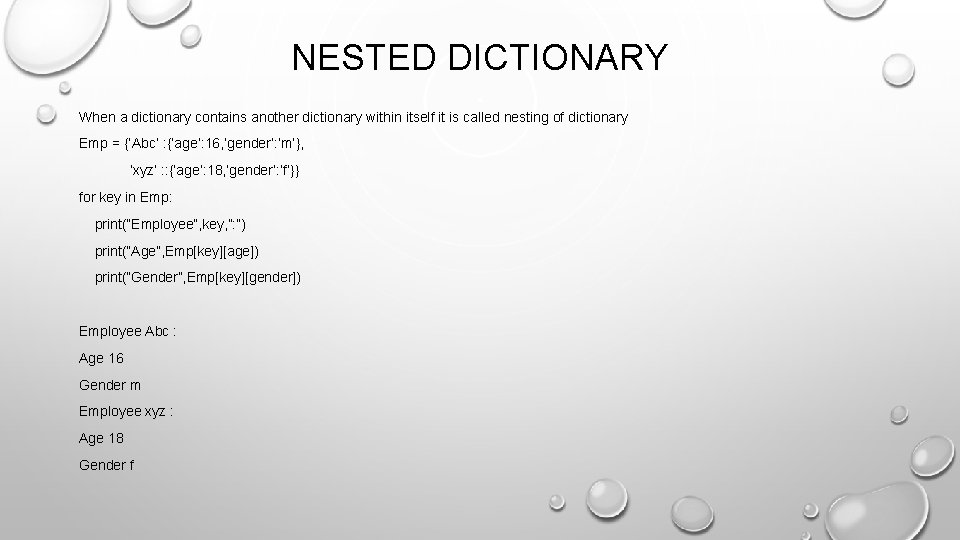
NESTED DICTIONARY When a dictionary contains another dictionary within itself it is called nesting of dictionary Emp = {‘Abc’ : {‘age’: 16, ’gender’: ’m’}, ‘xyz’ : : {‘age’: 18, ’gender’: ’f’}} for key in Emp: print(“Employee”, key, ”: ”) print(“Age”, Emp[key][age]) print(“Gender”, Emp[key][gender]) Employee Abc : Age 16 Gender m Employee xyz : Age 18 Gender f
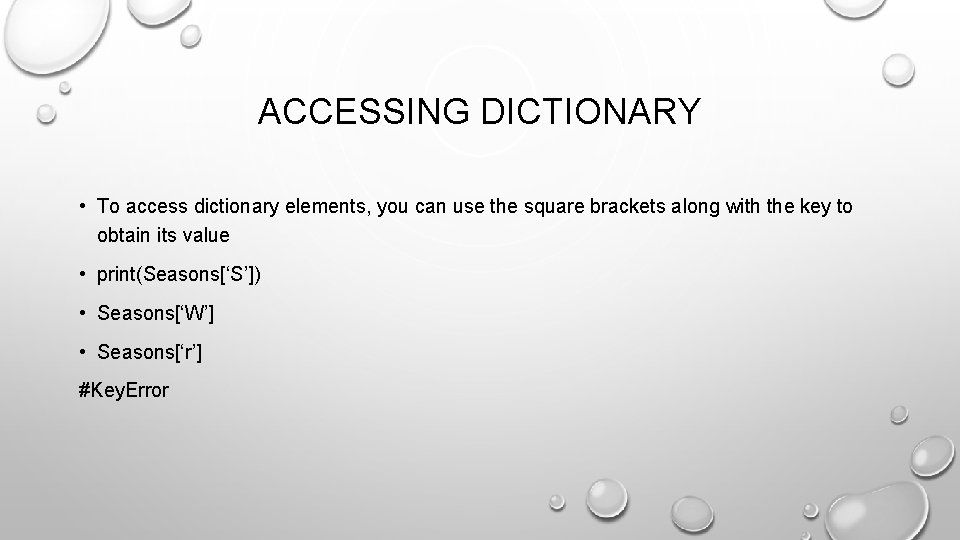
ACCESSING DICTIONARY • To access dictionary elements, you can use the square brackets along with the key to obtain its value • print(Seasons[‘S’]) • Seasons[‘W’] • Seasons[‘r’] #Key. Error
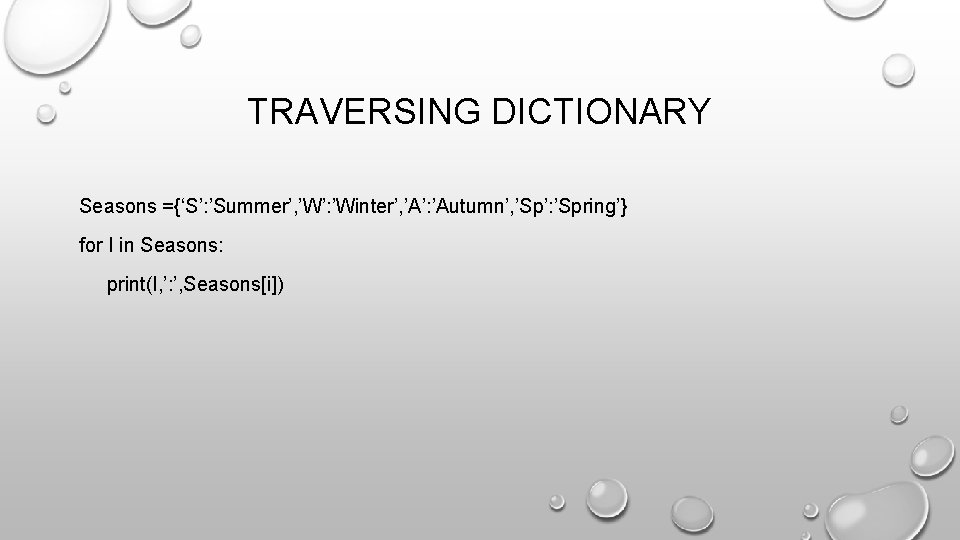
TRAVERSING DICTIONARY Seasons ={‘S’: ’Summer’, ’W’: ’Winter’, ’A’: ’Autumn’, ’Sp’: ’Spring’} for I in Seasons: print(I, ’: ’, Seasons[i])
![APPENDING DICTIONARY SYNTAX dictionary name keyvalue Example d10 Ten 20 Twenty 30 Thirty APPENDING DICTIONARY SYNTAX : <dictionary name >[key]=value Example d={10: ’Ten’, 20: ’Twenty’, 30: ’Thirty’}](https://slidetodoc.com/presentation_image_h2/0fce8ad800a274495137d573875cb0e9/image-9.jpg)
APPENDING DICTIONARY SYNTAX : <dictionary name >[key]=value Example d={10: ’Ten’, 20: ’Twenty’, 30: ’Thirty’} d[40]=‘Forty’ print(d)
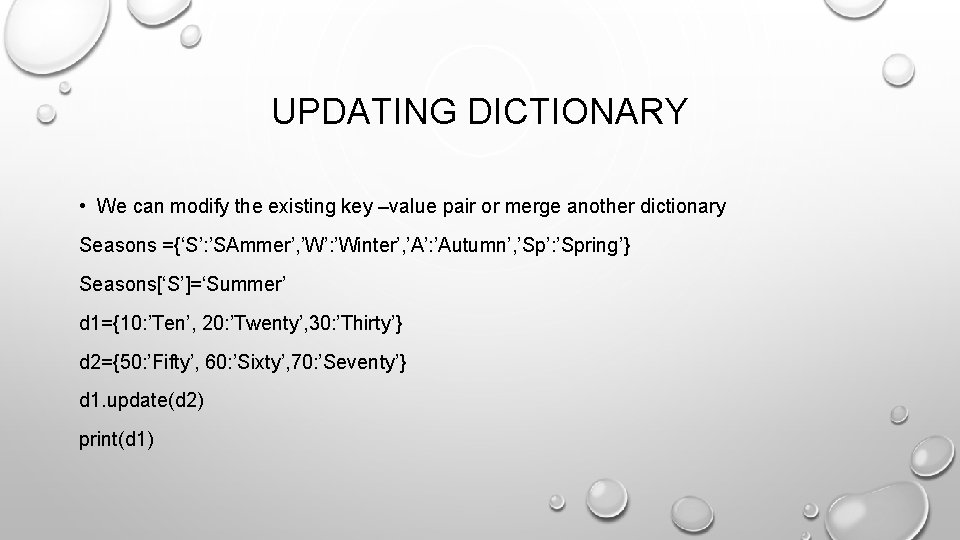
UPDATING DICTIONARY • We can modify the existing key –value pair or merge another dictionary Seasons ={‘S’: ’SAmmer’, ’W’: ’Winter’, ’A’: ’Autumn’, ’Sp’: ’Spring’} Seasons[‘S’]=‘Summer’ d 1={10: ’Ten’, 20: ’Twenty’, 30: ’Thirty’} d 2={50: ’Fifty’, 60: ’Sixty’, 70: ’Seventy’} d 1. update(d 2) print(d 1)
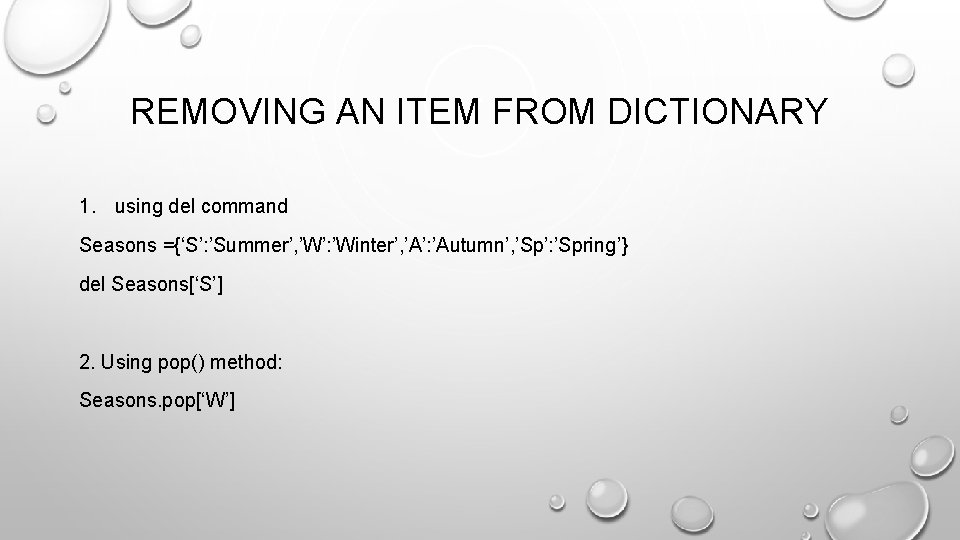
REMOVING AN ITEM FROM DICTIONARY 1. using del command Seasons ={‘S’: ’Summer’, ’W’: ’Winter’, ’A’: ’Autumn’, ’Sp’: ’Spring’} del Seasons[‘S’] 2. Using pop() method: Seasons. pop[‘W’]
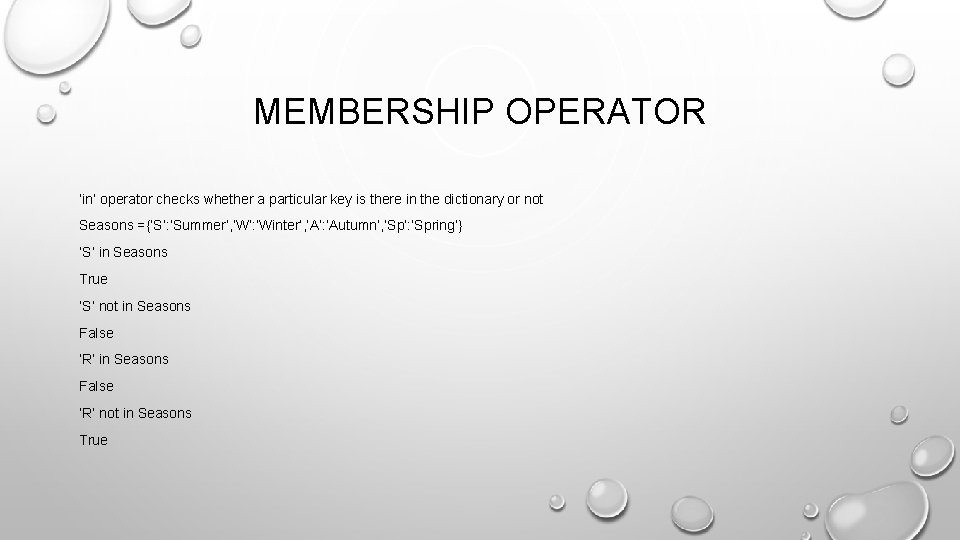
MEMBERSHIP OPERATOR ‘in’ operator checks whether a particular key is there in the dictionary or not Seasons ={‘S’: ’Summer’, ’W’: ’Winter’, ’A’: ’Autumn’, ’Sp’: ’Spring’} ‘S’ in Seasons True ‘S’ not in Seasons False ‘R’ not in Seasons True
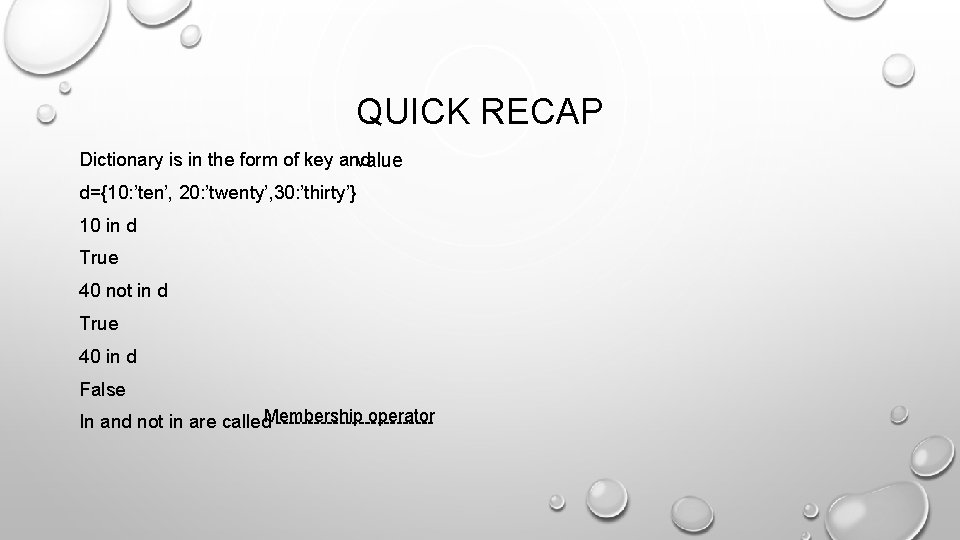
QUICK RECAP Dictionary is in the form of key and value d={10: ’ten’, 20: ’twenty’, 30: ’thirty’} 10 in d True 40 not in d True 40 in d False operator In and not in are called. Membership -------------
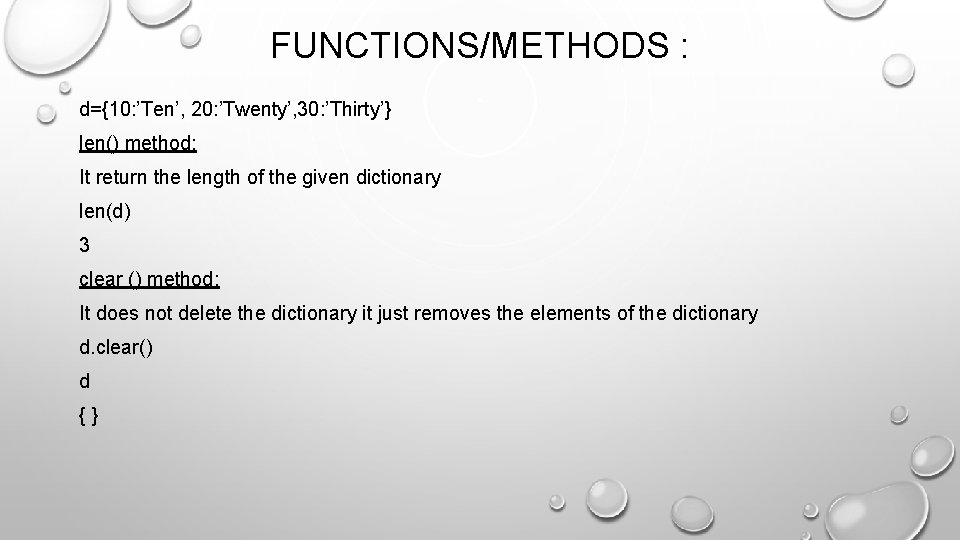
FUNCTIONS/METHODS : d={10: ’Ten’, 20: ’Twenty’, 30: ’Thirty’} len() method: It return the length of the given dictionary len(d) 3 clear () method: It does not delete the dictionary it just removes the elements of the dictionary d. clear() d {}
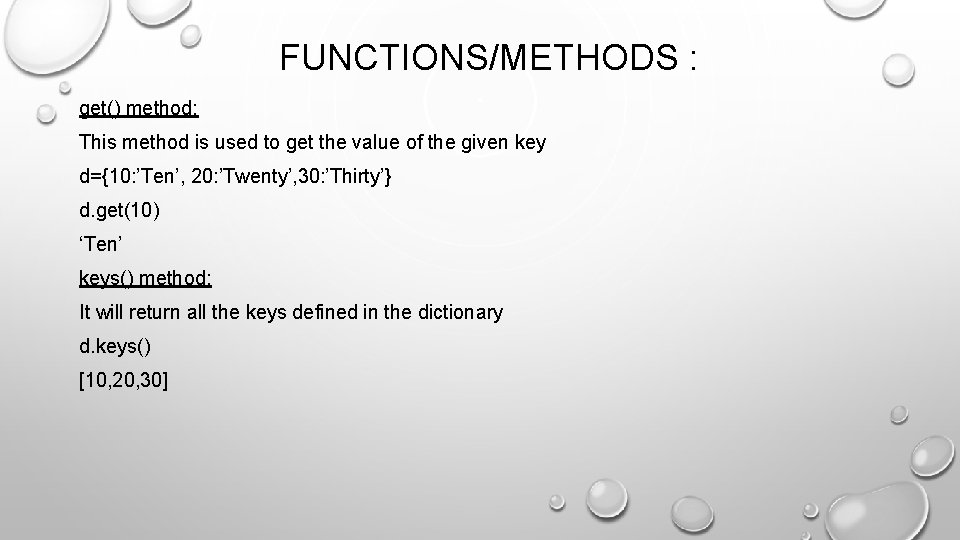
FUNCTIONS/METHODS : get() method: This method is used to get the value of the given key d={10: ’Ten’, 20: ’Twenty’, 30: ’Thirty’} d. get(10) ‘Ten’ keys() method: It will return all the keys defined in the dictionary d. keys() [10, 20, 30]
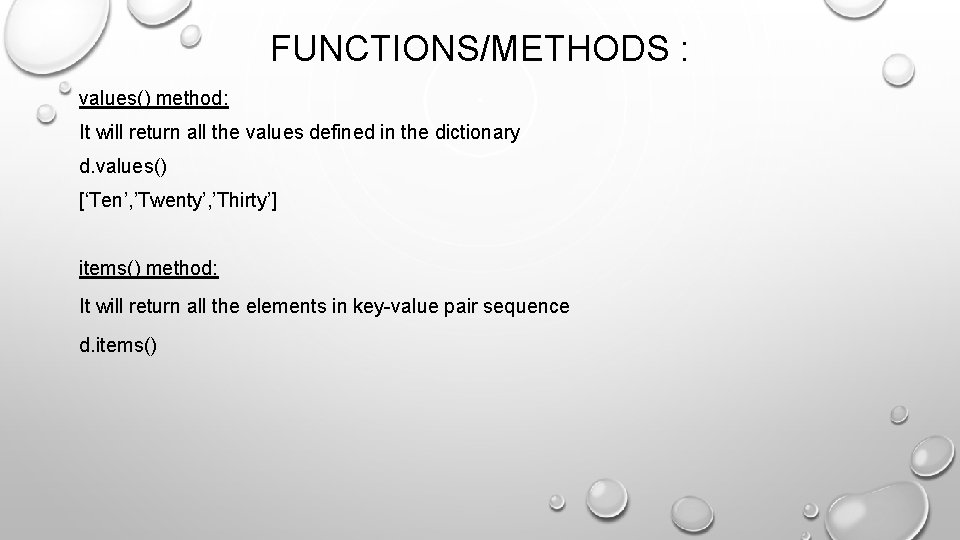
FUNCTIONS/METHODS : values() method: It will return all the values defined in the dictionary d. values() [‘Ten’, ’Twenty’, ’Thirty’] items() method: It will return all the elements in key-value pair sequence d. items()
Dictionaries mutable python
Python difference list tuple
Iso 20022 data dictionary
Remote dictionary server
World law dictionary
Monkey denotation
Jeffrey lee parson
Is the hudson bay saltwater
Permuterm index of x*y*z
Data dictionary example in system analysis and design
Define urban sprawl
Binary dictionary
My mother has bought me a dictionary.
Shafafiya dictionary
Leader definition oxford
Dictionary binary search tree
Deployment diagram of hospital management system