Program Correctness an introduction Program Correctness How do
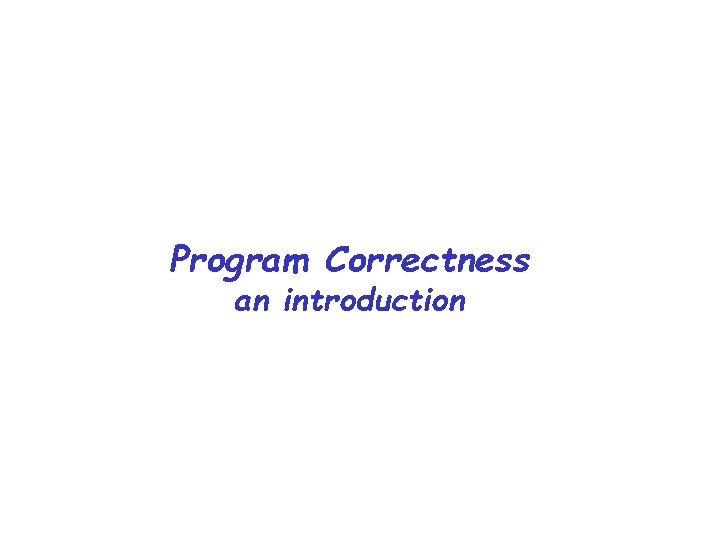
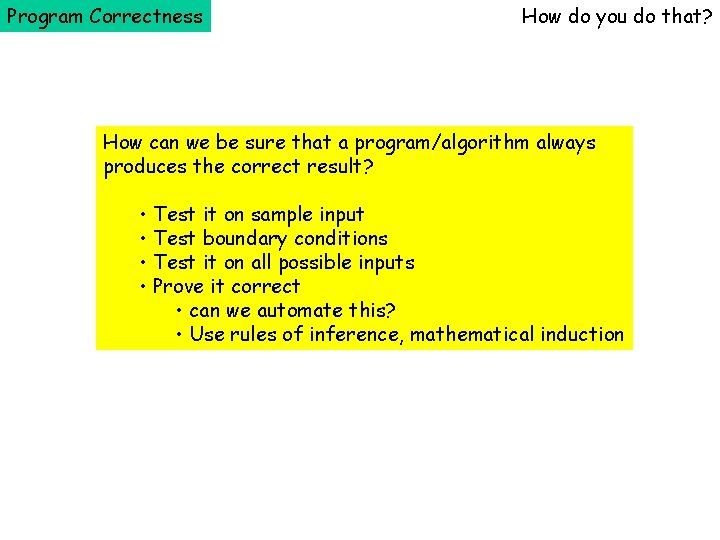
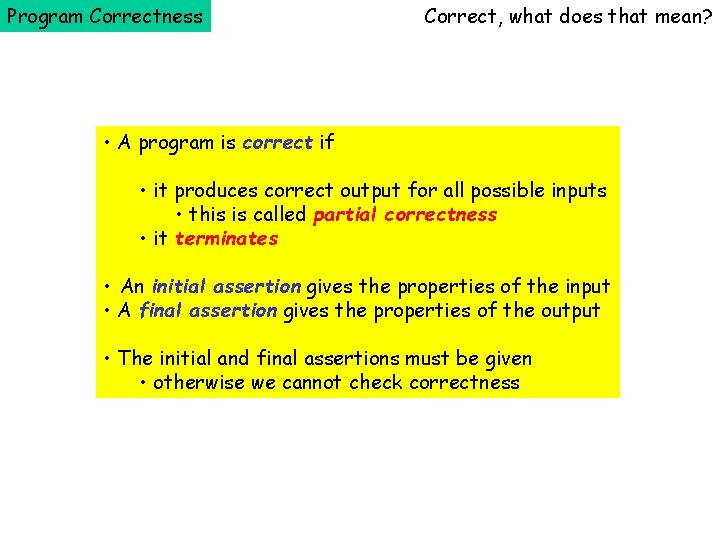
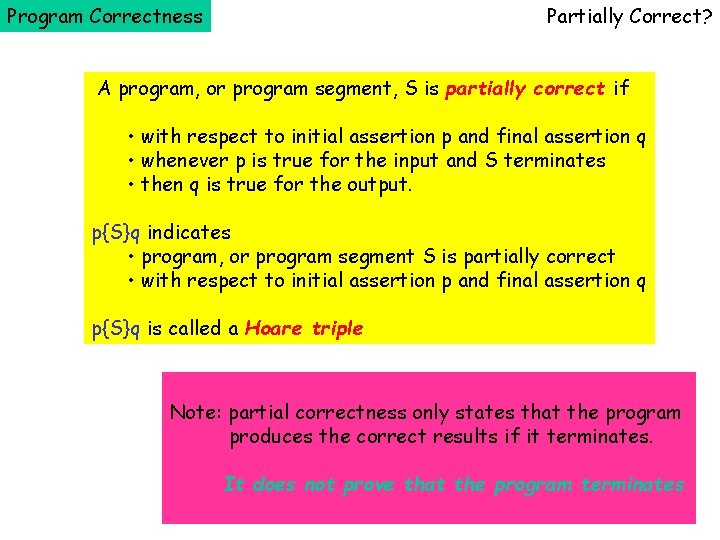
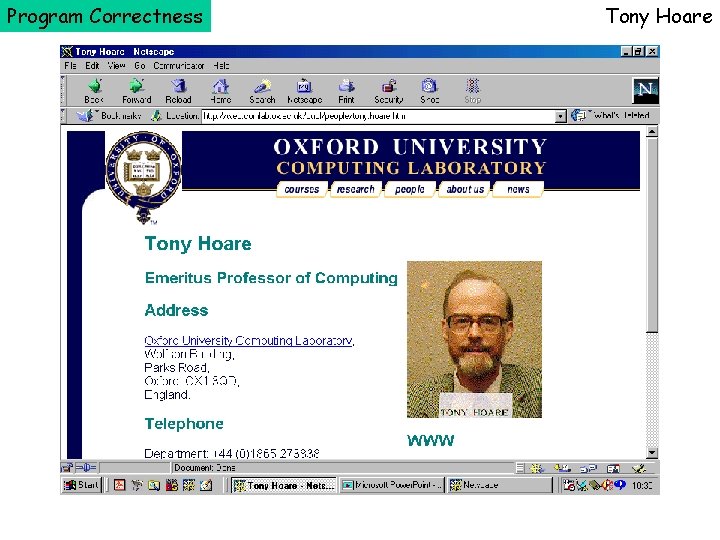
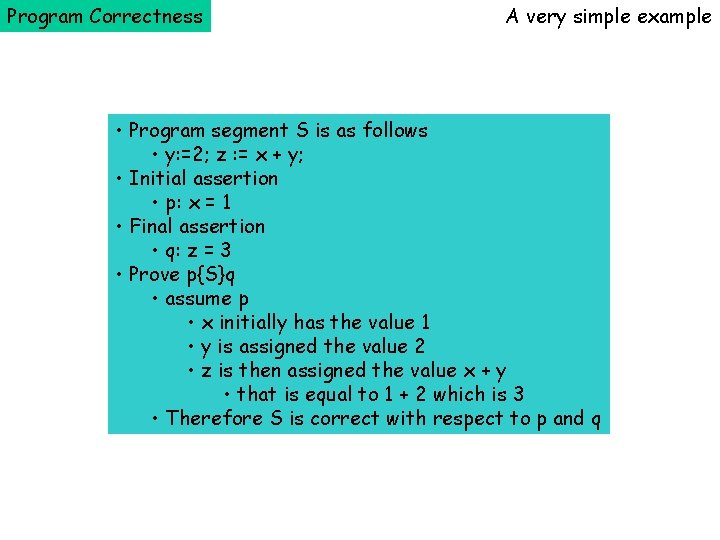
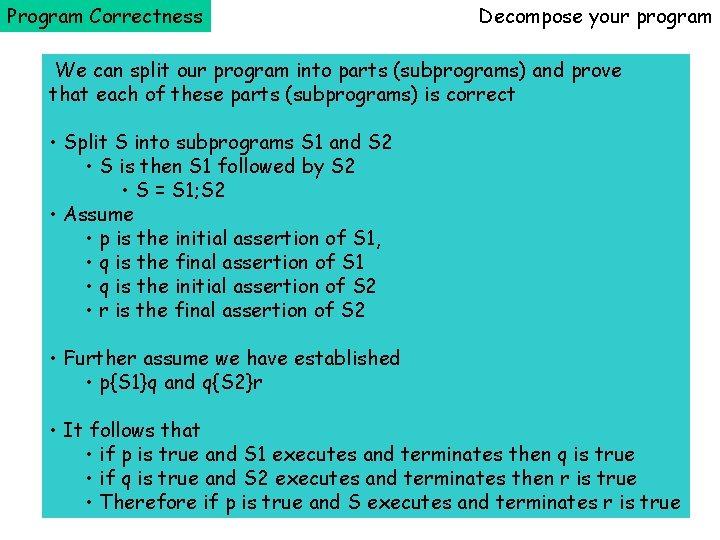
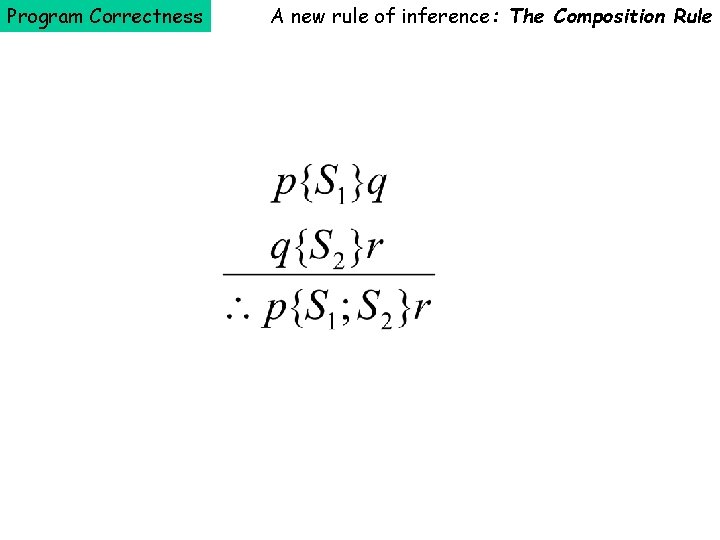
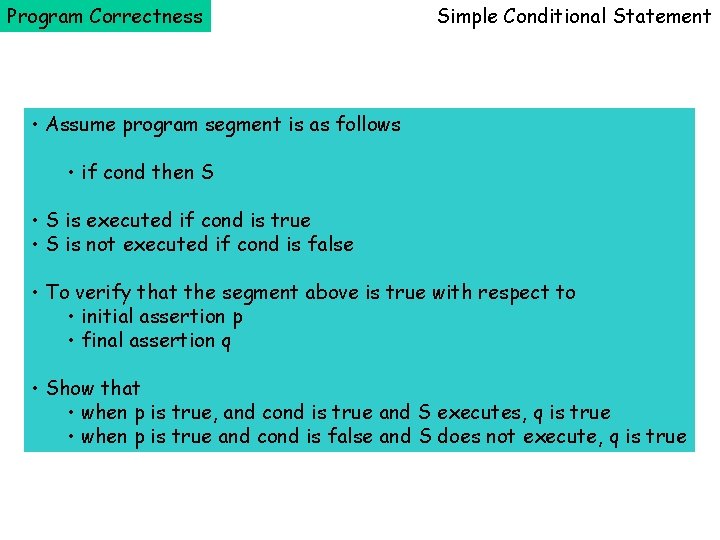
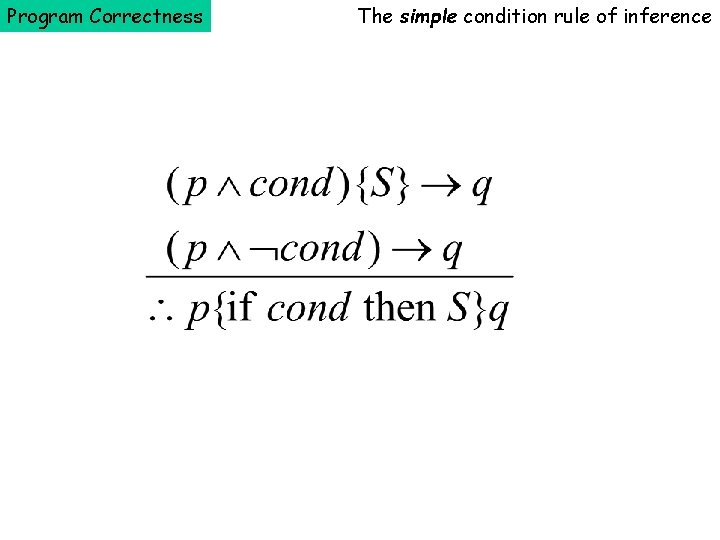
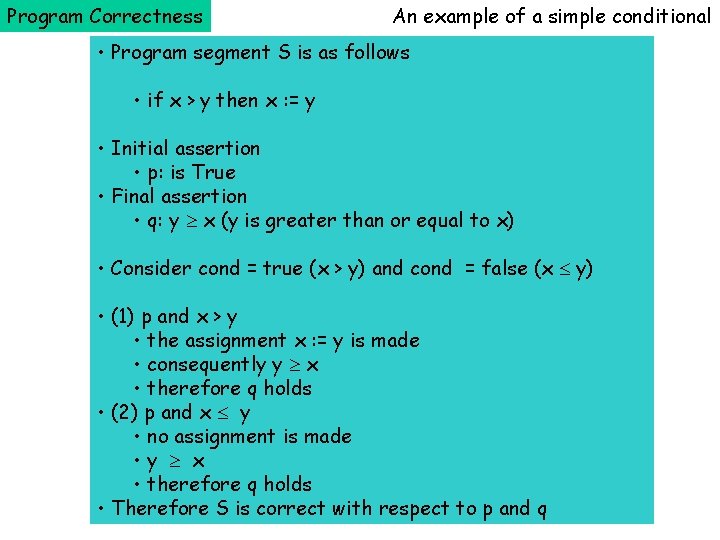
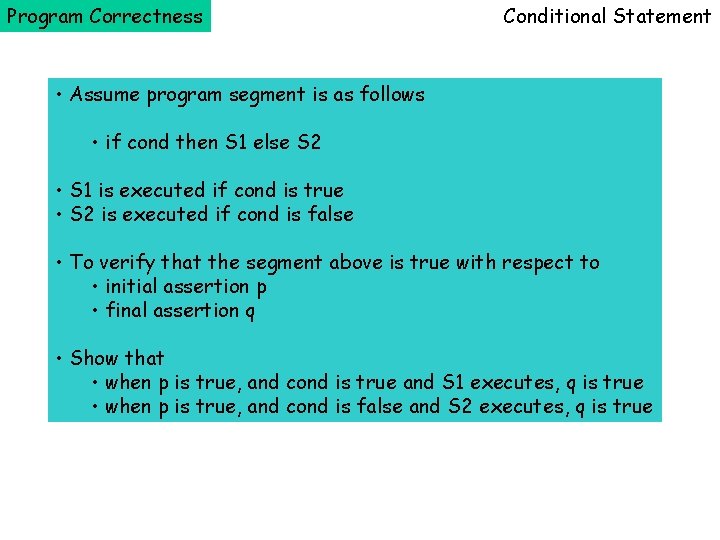
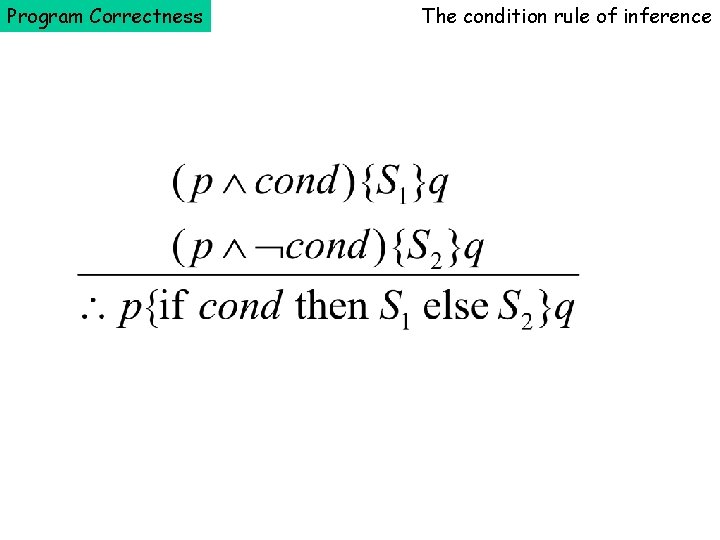
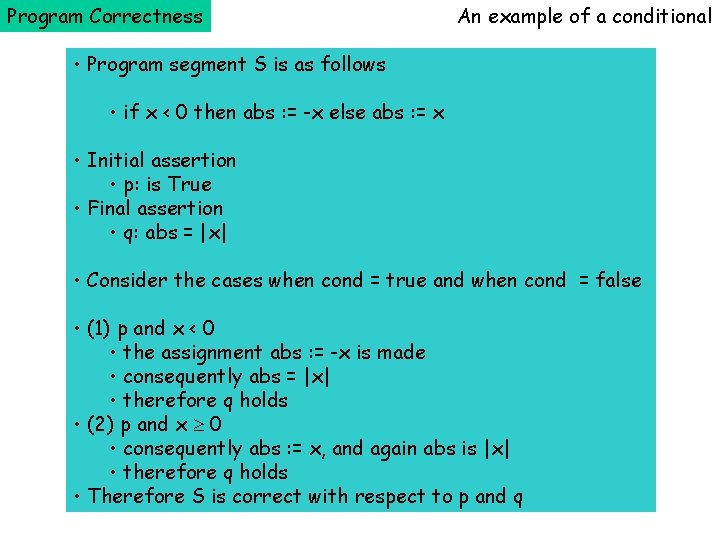
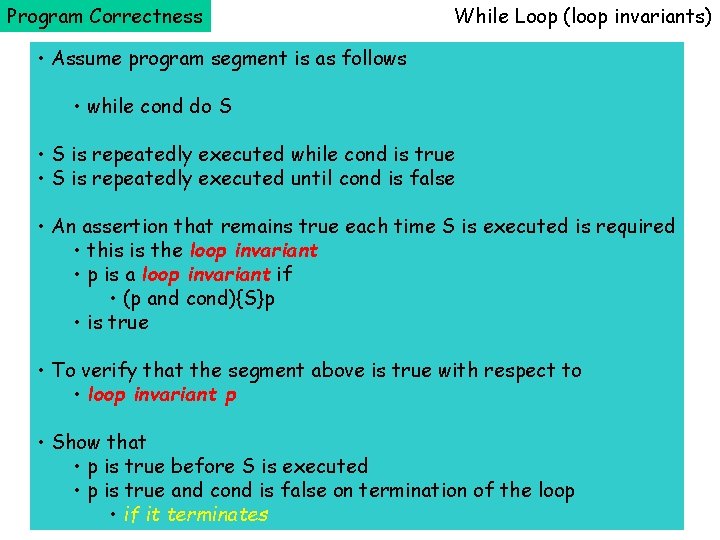
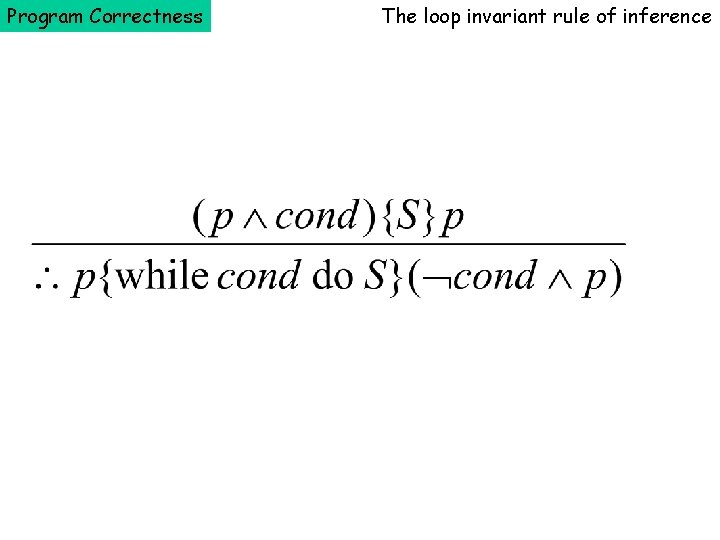
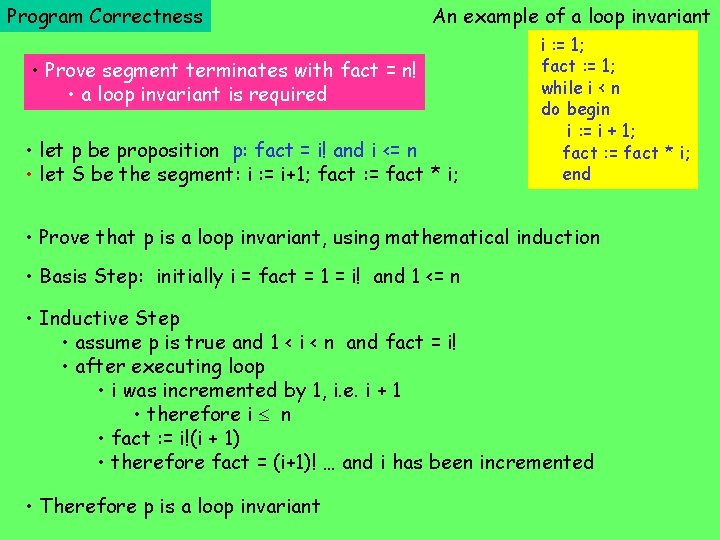
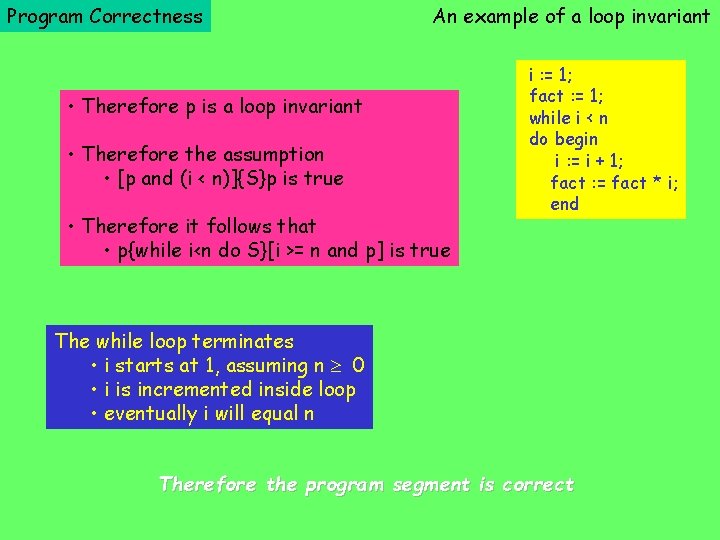
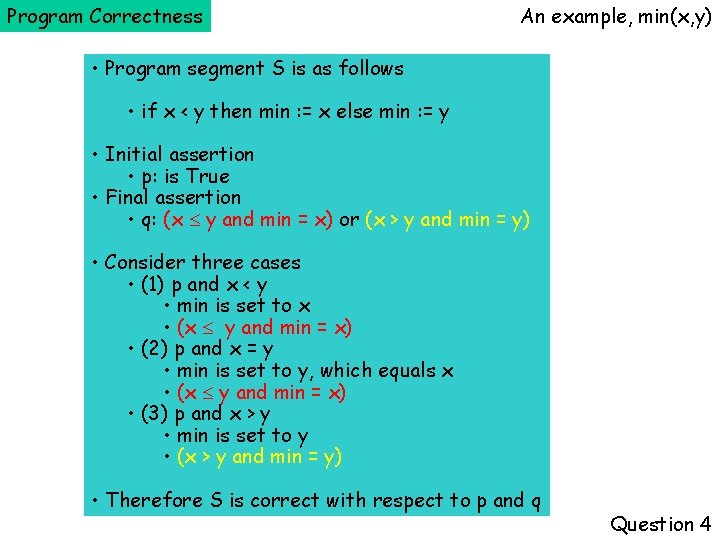
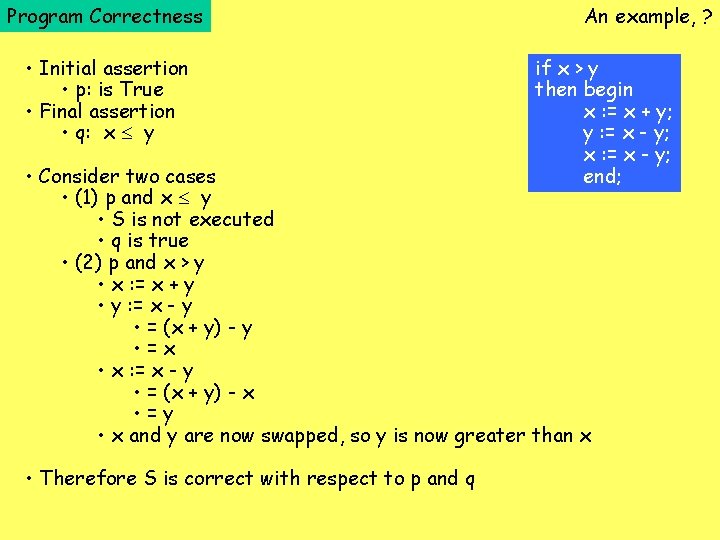
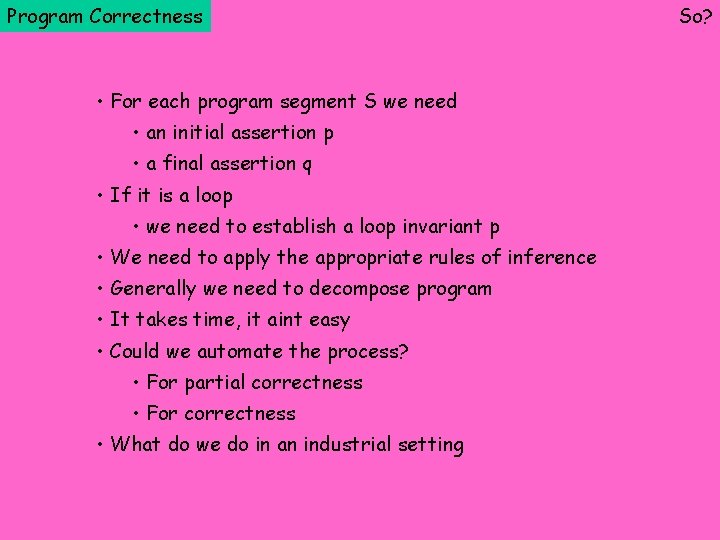
- Slides: 21
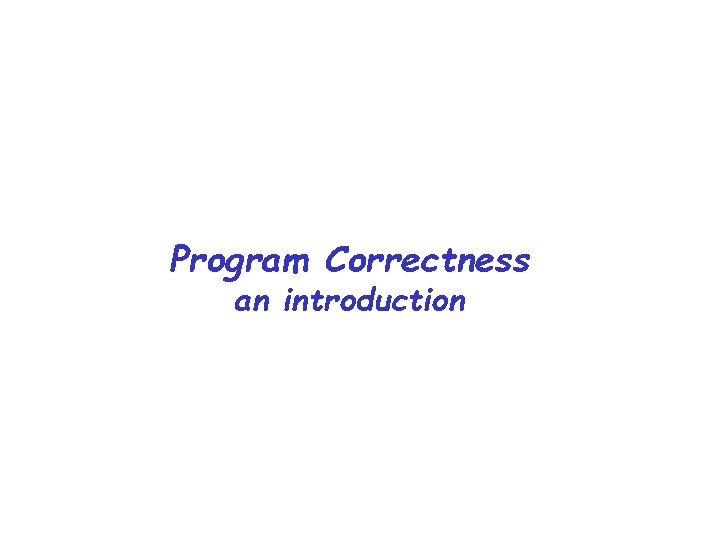
Program Correctness an introduction
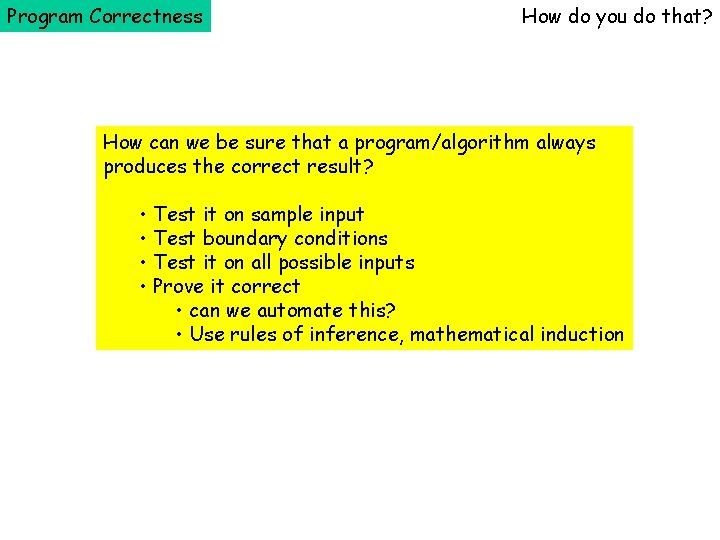
Program Correctness How do you do that? How can we be sure that a program/algorithm always produces the correct result? • Test it on sample input • Test boundary conditions • Test it on all possible inputs • Prove it correct • can we automate this? • Use rules of inference, mathematical induction
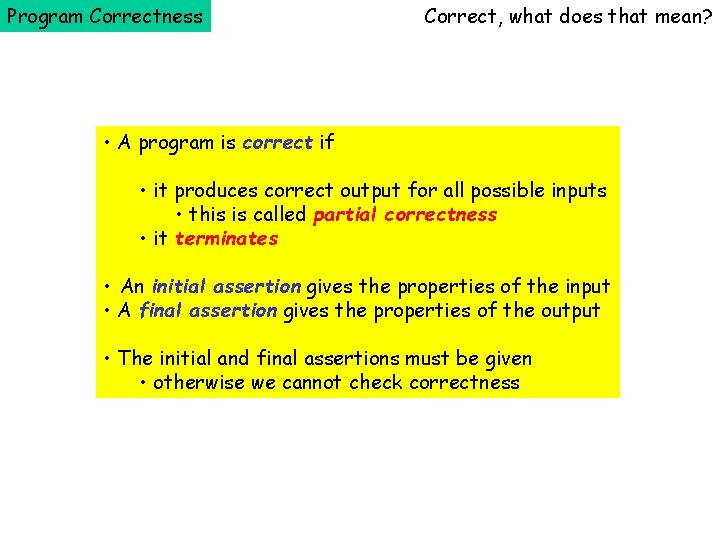
Program Correctness Correct, what does that mean? • A program is correct if • it produces correct output for all possible inputs • this is called partial correctness • it terminates • An initial assertion gives the properties of the input • A final assertion gives the properties of the output • The initial and final assertions must be given • otherwise we cannot check correctness
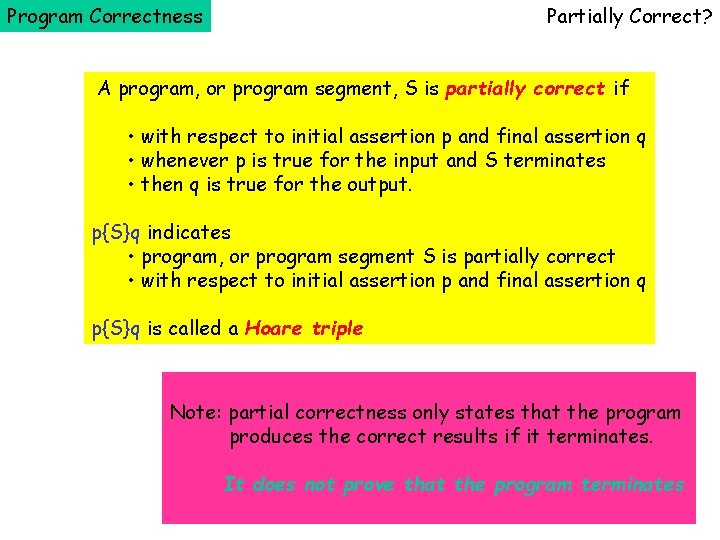
Program Correctness Partially Correct? A program, or program segment, S is partially correct if • with respect to initial assertion p and final assertion q • whenever p is true for the input and S terminates • then q is true for the output. p{S}q indicates • program, or program segment S is partially correct • with respect to initial assertion p and final assertion q p{S}q is called a Hoare triple Note: partial correctness only states that the program produces the correct results if it terminates. It does not prove that the program terminates
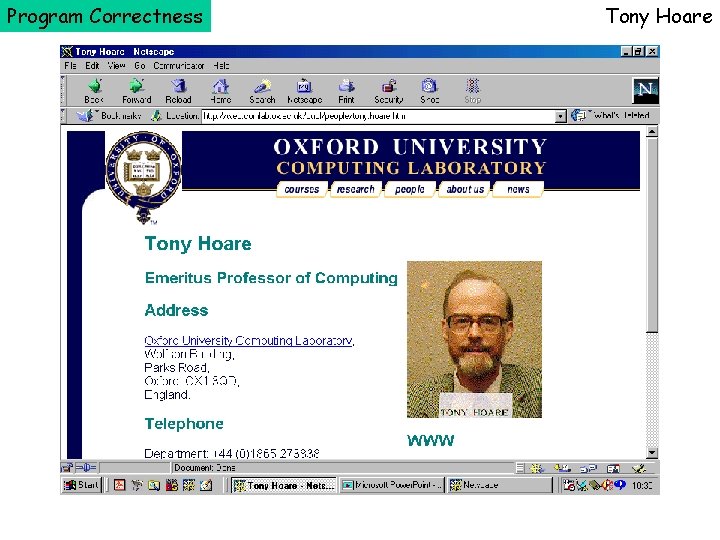
Program Correctness Tony Hoare
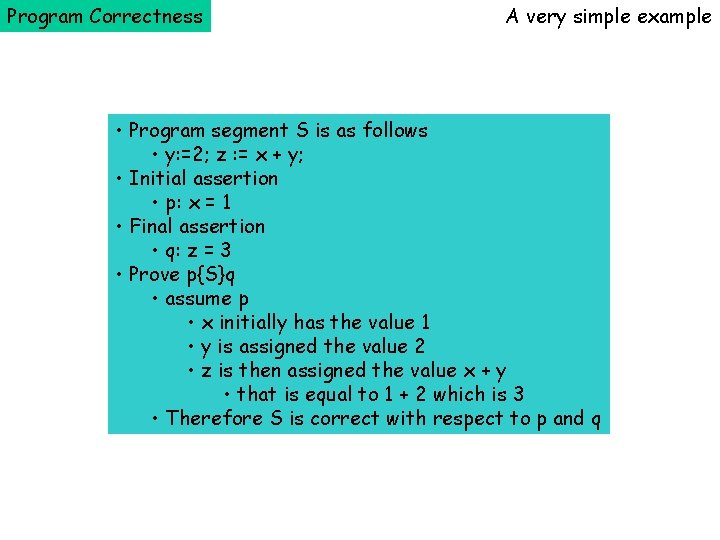
Program Correctness A very simple example • Program segment S is as follows • y: =2; z : = x + y; • Initial assertion • p: x = 1 • Final assertion • q: z = 3 • Prove p{S}q • assume p • x initially has the value 1 • y is assigned the value 2 • z is then assigned the value x + y • that is equal to 1 + 2 which is 3 • Therefore S is correct with respect to p and q
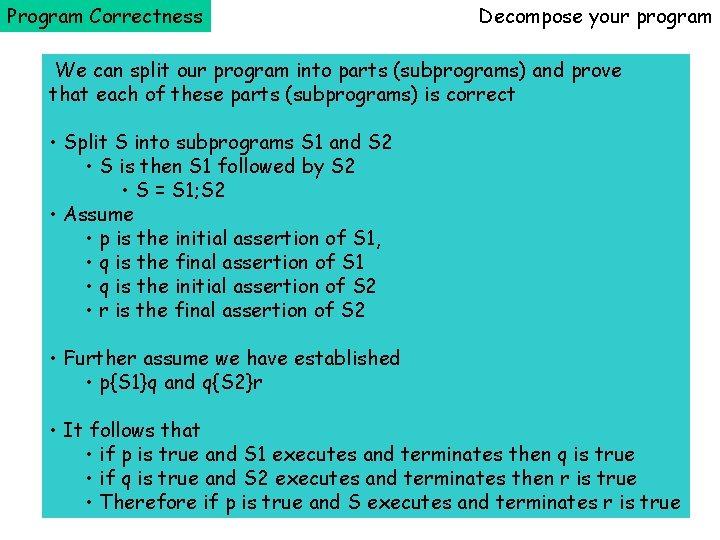
Program Correctness Decompose your program We can split our program into parts (subprograms) and prove that each of these parts (subprograms) is correct • Split S into subprograms S 1 and S 2 • S is then S 1 followed by S 2 • S = S 1; S 2 • Assume • p is the initial assertion of S 1, • q is the final assertion of S 1 • q is the initial assertion of S 2 • r is the final assertion of S 2 • Further assume we have established • p{S 1}q and q{S 2}r • It follows that • if p is true and S 1 executes and terminates then q is true • if q is true and S 2 executes and terminates then r is true • Therefore if p is true and S executes and terminates r is true
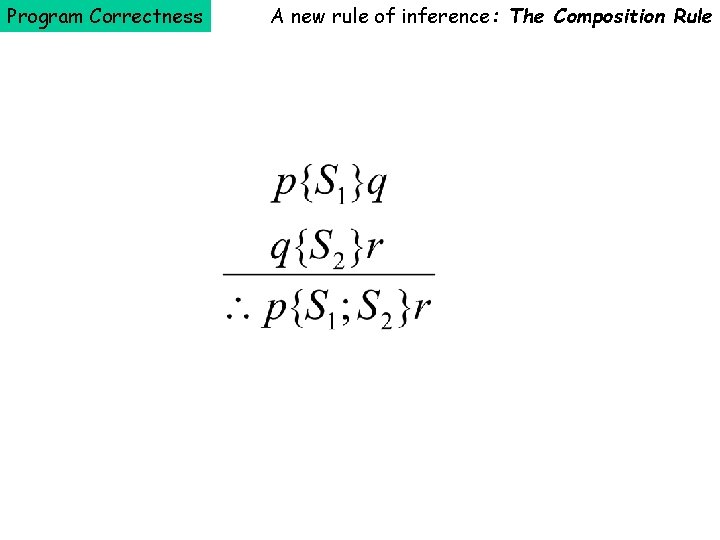
Program Correctness A new rule of inference: The Composition Rule
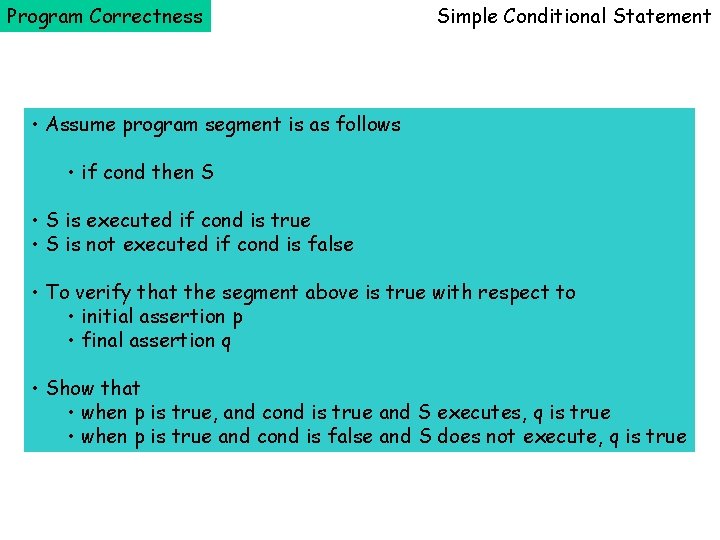
Program Correctness Simple Conditional Statement • Assume program segment is as follows • if cond then S • S is executed if cond is true • S is not executed if cond is false • To verify that the segment above is true with respect to • initial assertion p • final assertion q • Show that • when p is true, and cond is true and S executes, q is true • when p is true and cond is false and S does not execute, q is true
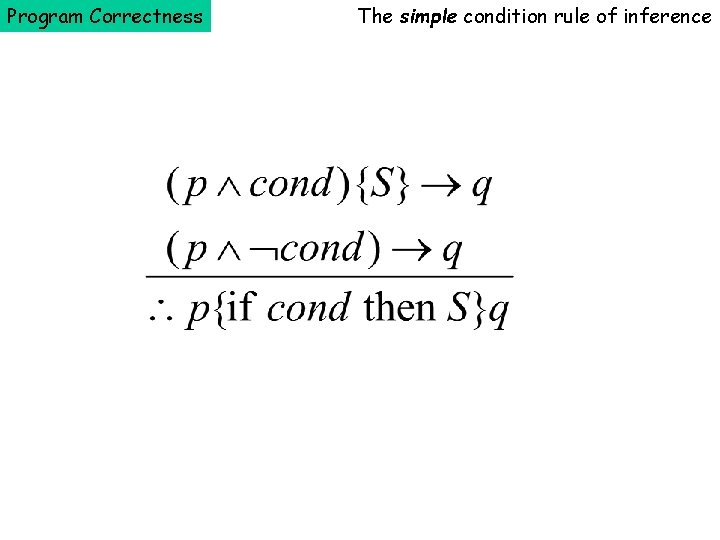
Program Correctness The simple condition rule of inference
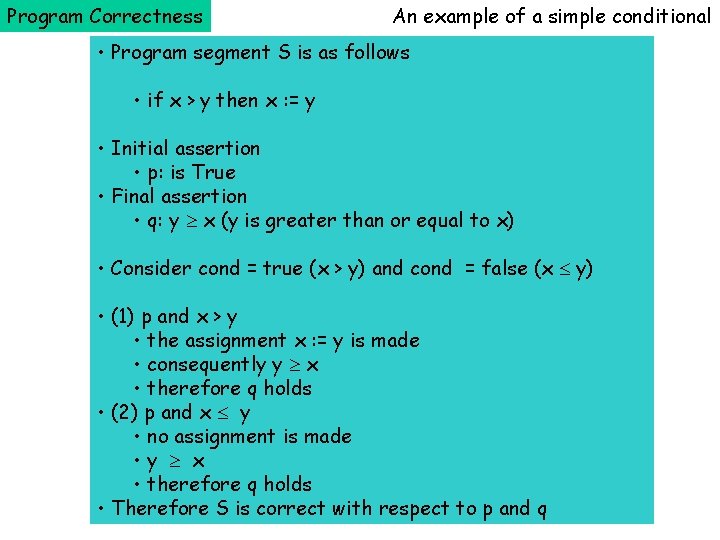
Program Correctness An example of a simple conditional • Program segment S is as follows • if x > y then x : = y • Initial assertion • p: is True • Final assertion • q: y x (y is greater than or equal to x) • Consider cond = true (x > y) and cond = false (x y) • (1) p and x > y • the assignment x : = y is made • consequently y x • therefore q holds • (2) p and x y • no assignment is made • y x • therefore q holds • Therefore S is correct with respect to p and q
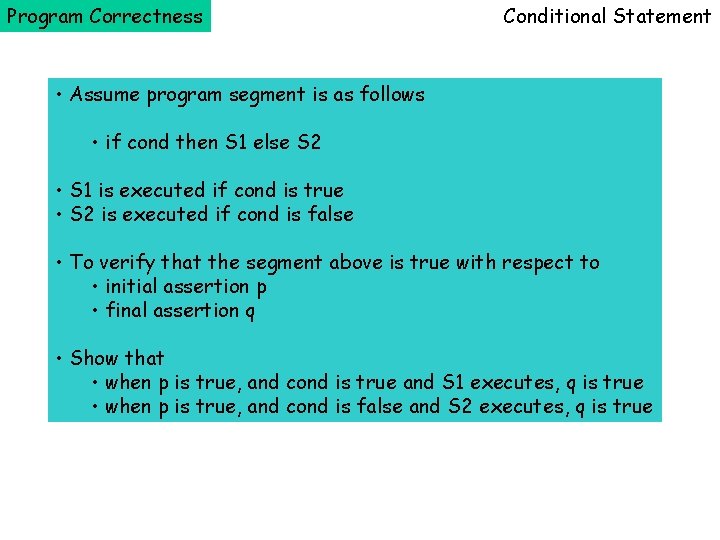
Program Correctness Conditional Statement • Assume program segment is as follows • if cond then S 1 else S 2 • S 1 is executed if cond is true • S 2 is executed if cond is false • To verify that the segment above is true with respect to • initial assertion p • final assertion q • Show that • when p is true, and cond is true and S 1 executes, q is true • when p is true, and cond is false and S 2 executes, q is true
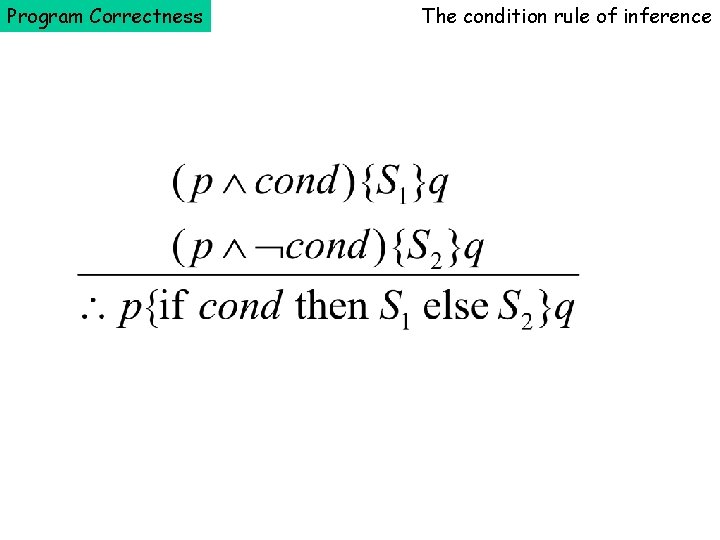
Program Correctness The condition rule of inference
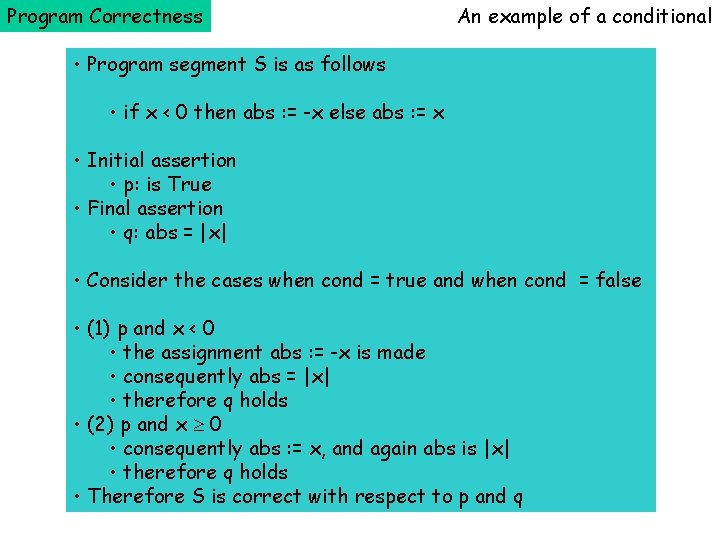
Program Correctness An example of a conditional • Program segment S is as follows • if x < 0 then abs : = -x else abs : = x • Initial assertion • p: is True • Final assertion • q: abs = |x| • Consider the cases when cond = true and when cond = false • (1) p and x < 0 • the assignment abs : = -x is made • consequently abs = |x| • therefore q holds • (2) p and x 0 • consequently abs : = x, and again abs is |x| • therefore q holds • Therefore S is correct with respect to p and q
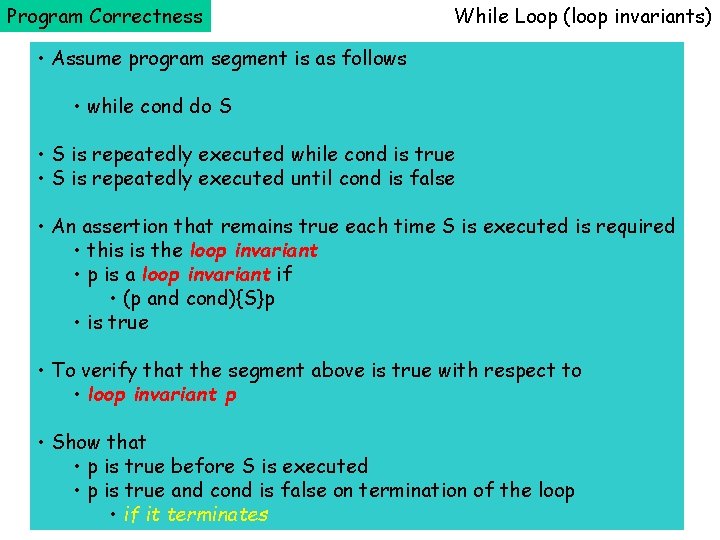
Program Correctness While Loop (loop invariants) • Assume program segment is as follows • while cond do S • S is repeatedly executed while cond is true • S is repeatedly executed until cond is false • An assertion that remains true each time S is executed is required • this is the loop invariant • p is a loop invariant if • (p and cond){S}p • is true • To verify that the segment above is true with respect to • loop invariant p • Show that • p is true before S is executed • p is true and cond is false on termination of the loop • if it terminates
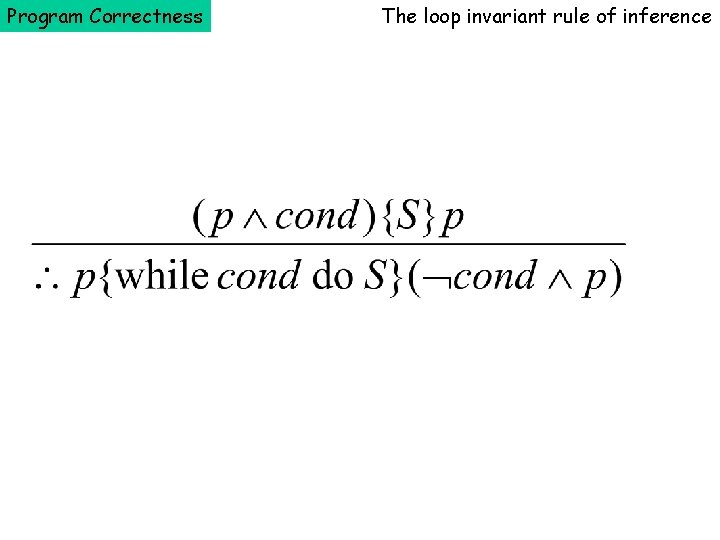
Program Correctness The loop invariant rule of inference
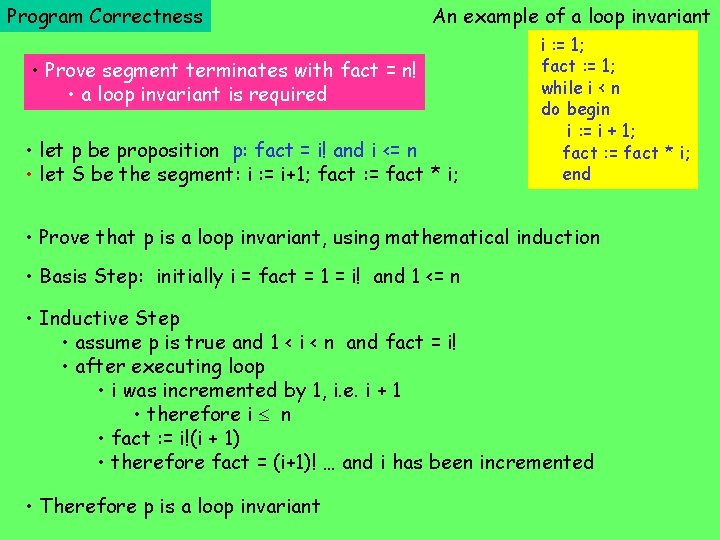
Program Correctness An example of a loop invariant • Prove segment terminates with fact = n! • a loop invariant is required • let p be proposition p: fact = i! and i <= n • let S be the segment: i : = i+1; fact : = fact * i; i : = 1; fact : = 1; while i < n do begin i : = i + 1; fact : = fact * i; end • Prove that p is a loop invariant, using mathematical induction • Basis Step: initially i = fact = 1 = i! and 1 <= n • Inductive Step • assume p is true and 1 < i < n and fact = i! • after executing loop • i was incremented by 1, i. e. i + 1 • therefore i n • fact : = i!(i + 1) • therefore fact = (i+1)! … and i has been incremented • Therefore p is a loop invariant
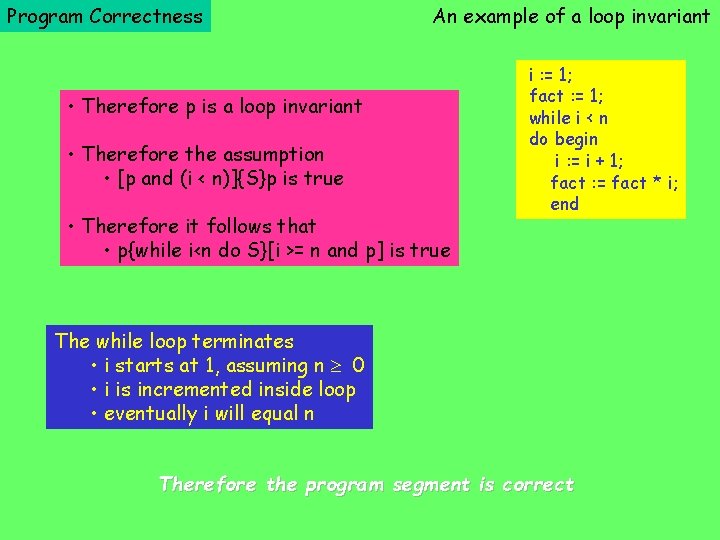
Program Correctness An example of a loop invariant • Therefore p is a loop invariant • Therefore the assumption • [p and (i < n)]{S}p is true • Therefore it follows that • p{while i<n do S}[i >= n and p] is true i : = 1; fact : = 1; while i < n do begin i : = i + 1; fact : = fact * i; end The while loop terminates • i starts at 1, assuming n 0 • i is incremented inside loop • eventually i will equal n Therefore the program segment is correct
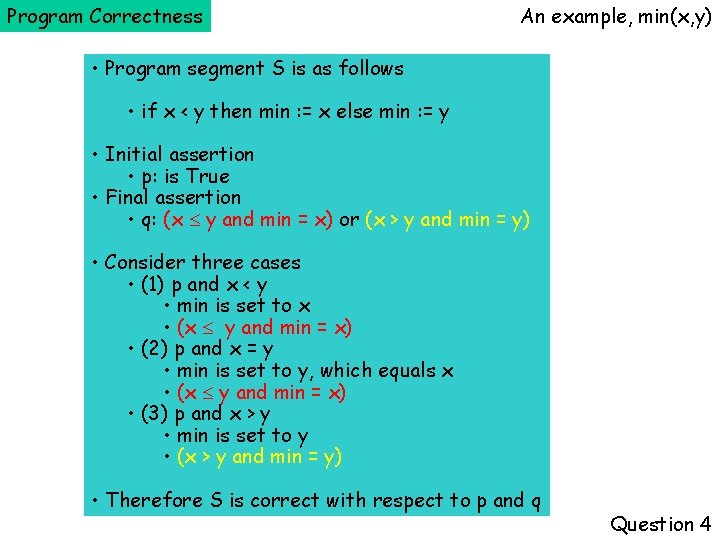
Program Correctness An example, min(x, y) • Program segment S is as follows • if x < y then min : = x else min : = y • Initial assertion • p: is True • Final assertion • q: (x y and min = x) or (x > y and min = y) • Consider three cases • (1) p and x < y • min is set to x • (x y and min = x) • (2) p and x = y • min is set to y, which equals x • (x y and min = x) • (3) p and x > y • min is set to y • (x > y and min = y) • Therefore S is correct with respect to p and q Question 4
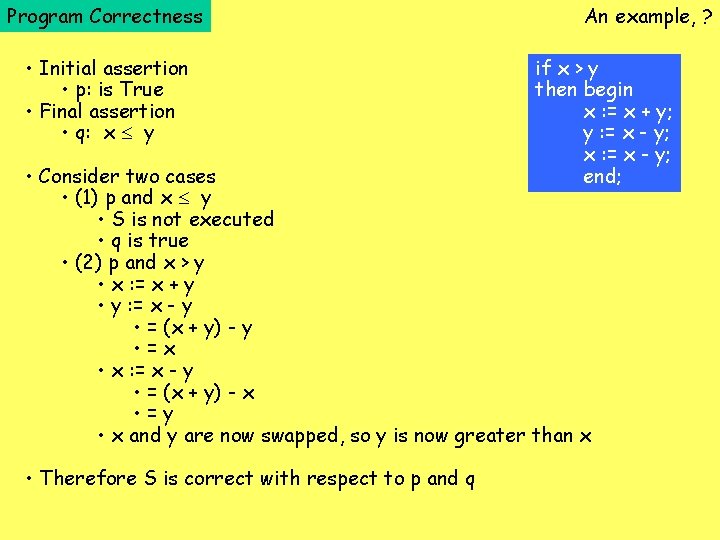
Program Correctness • Initial assertion • p: is True • Final assertion • q: x y An example, ? if x > y then begin x : = x + y; y : = x - y; x : = x - y; end; • Consider two cases • (1) p and x y • S is not executed • q is true • (2) p and x > y • x : = x + y • y : = x - y • = (x + y) - y • =x • x : = x - y • = (x + y) - x • =y • x and y are now swapped, so y is now greater than x • Therefore S is correct with respect to p and q
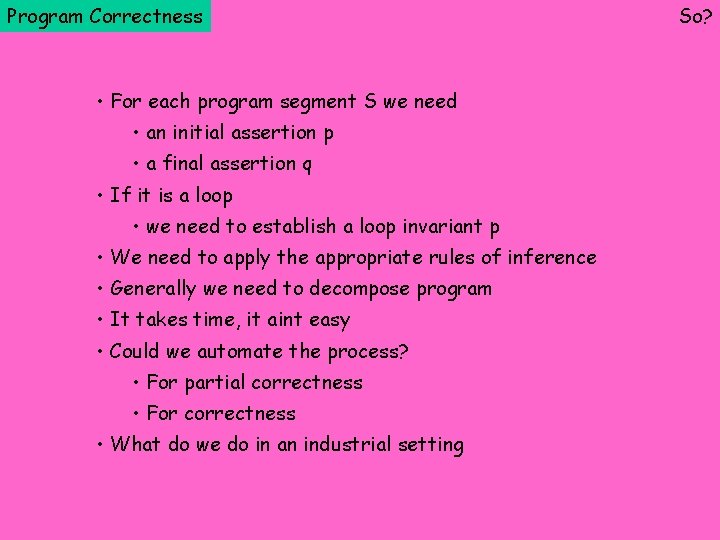
Program Correctness • For each program segment S we need • an initial assertion p • a final assertion q • If it is a loop • we need to establish a loop invariant p • We need to apply the appropriate rules of inference • Generally we need to decompose program • It takes time, it aint easy • Could we automate the process? • For partial correctness • For correctness • What do we do in an industrial setting So?
What is program correctness
Entity integrity ensures correctness of the data in a table
7cs of communication
Principles of communication correctness
Bfs proof of correctness
Divide and conquer
Concreteness in technical writing
7'cs of effective communication
Minterm predicates
Reliability vs correctness
Emotional correctness
Four loopy questions
Loop invariant of bubble sort
Sender's courtesy
Proof of correctness examples
Correctness of fragmentation
Dynamic connectivity problem
Software design principles correctness and robustness
Derived horizontal fragmentation
Functional correctness
Loop invariant induction
Check the correctness of the equation v=u+at