PHP Arrays Functions Array An array can store
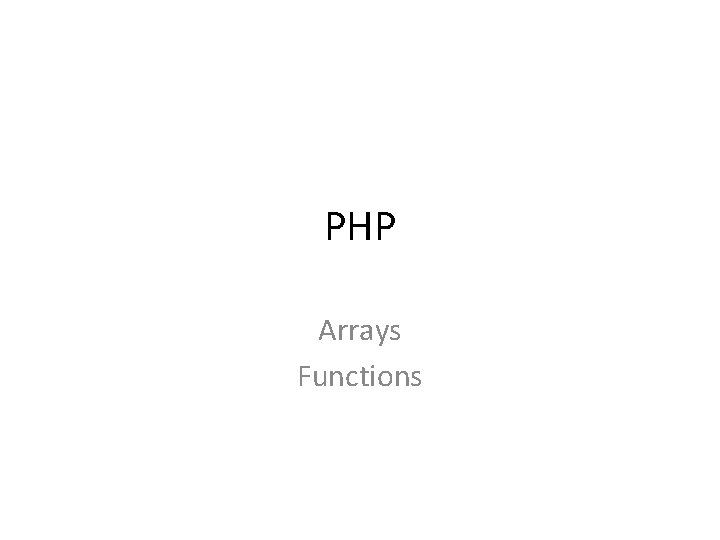
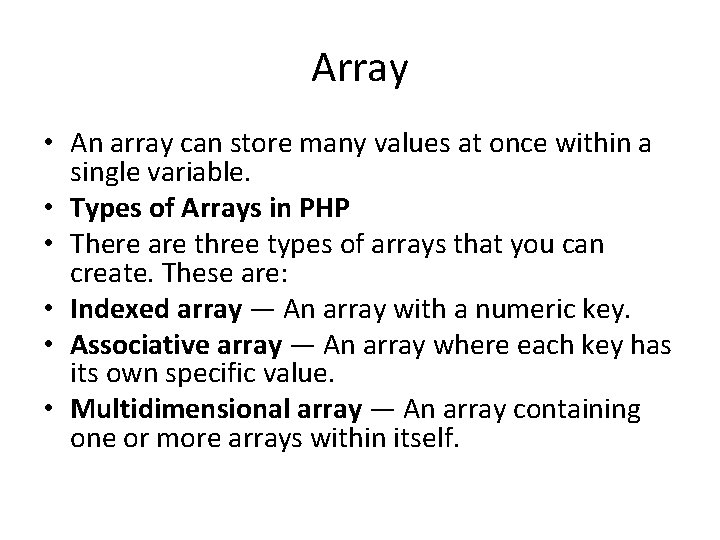
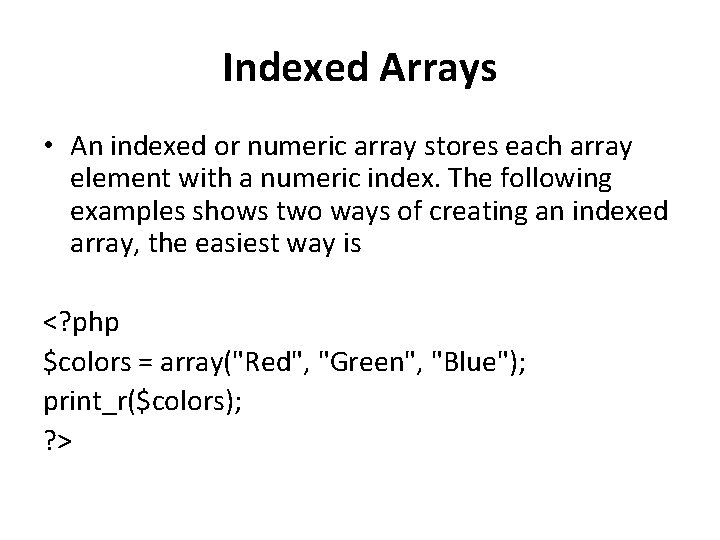
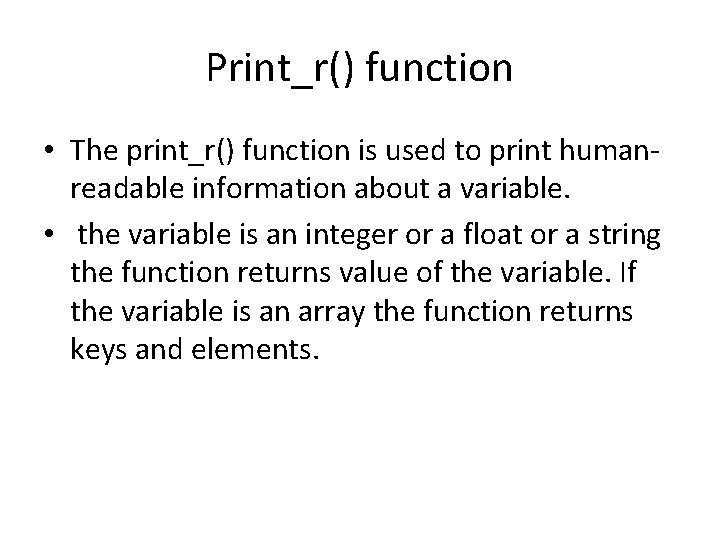
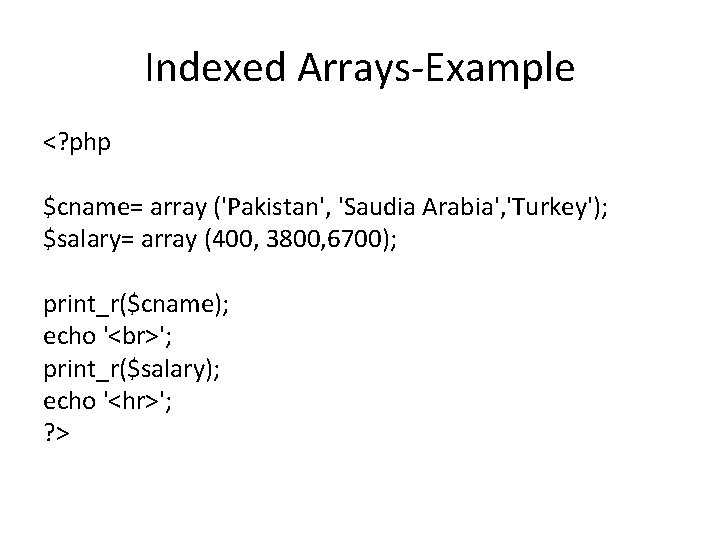
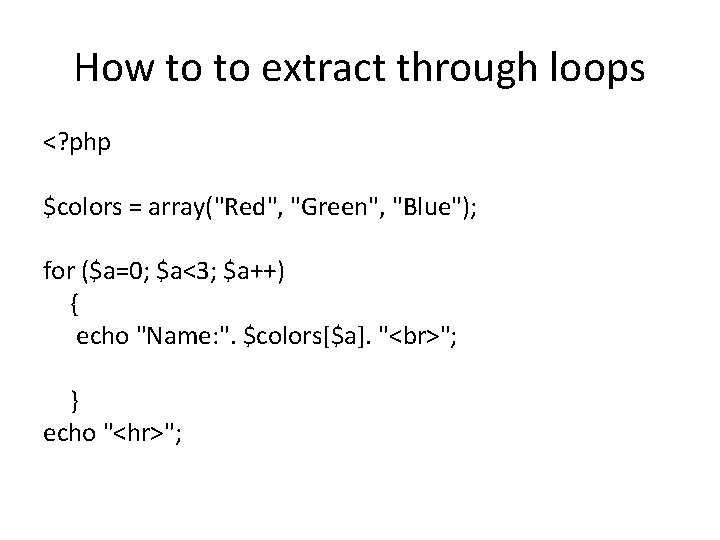
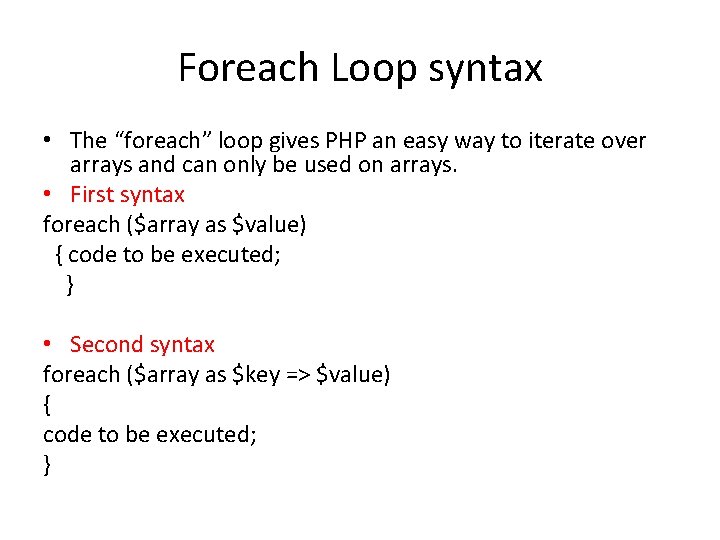
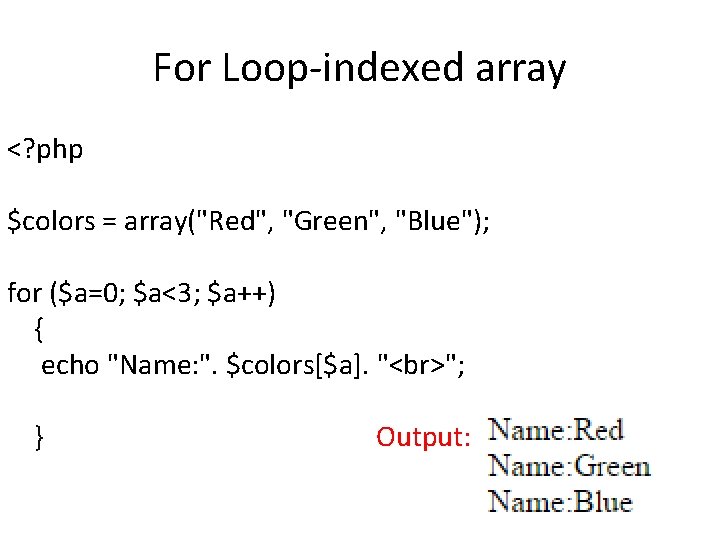
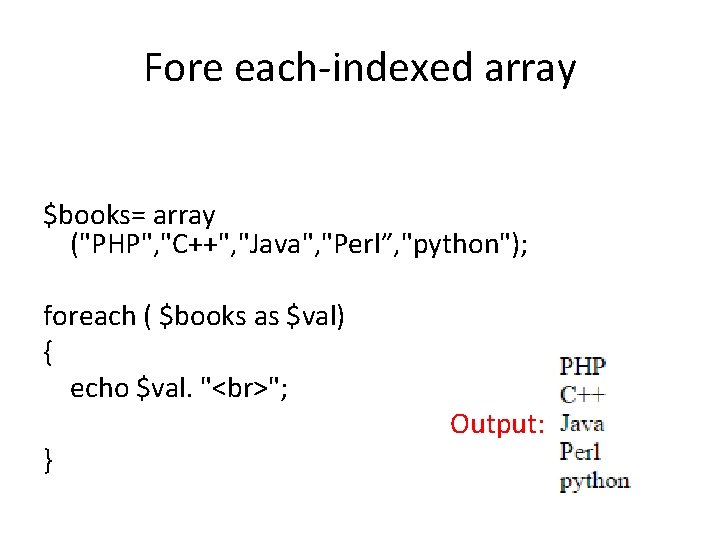
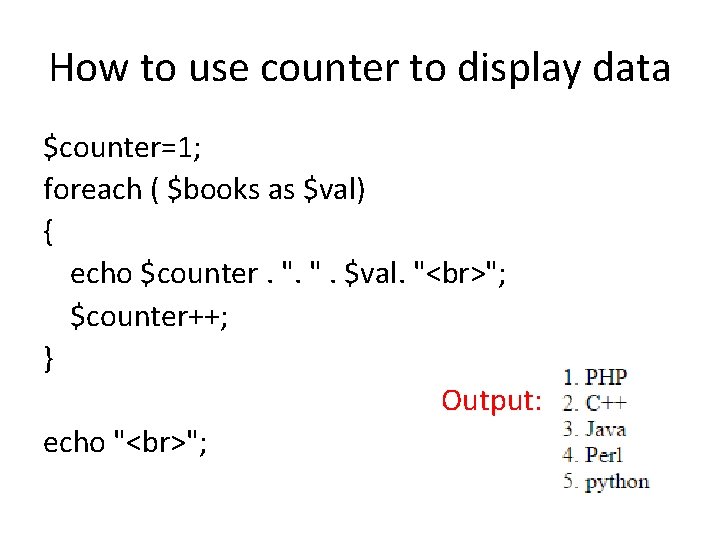
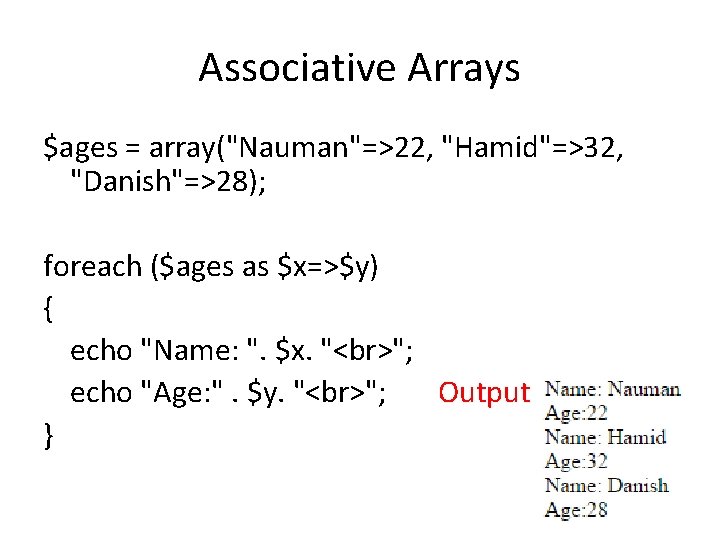
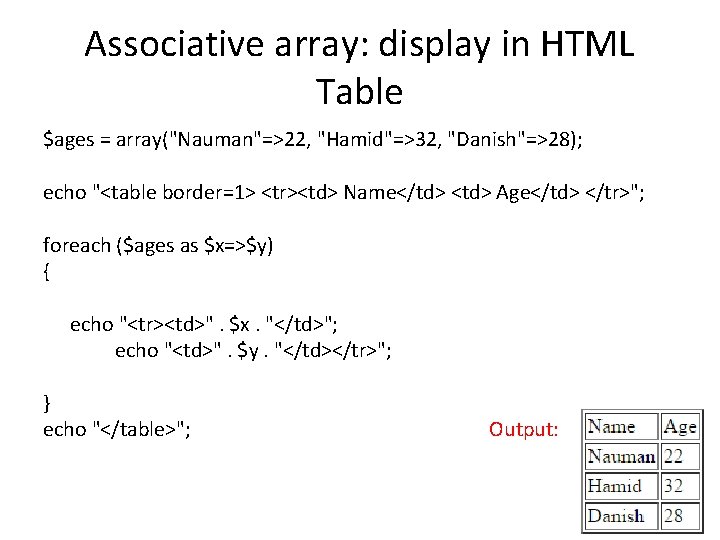
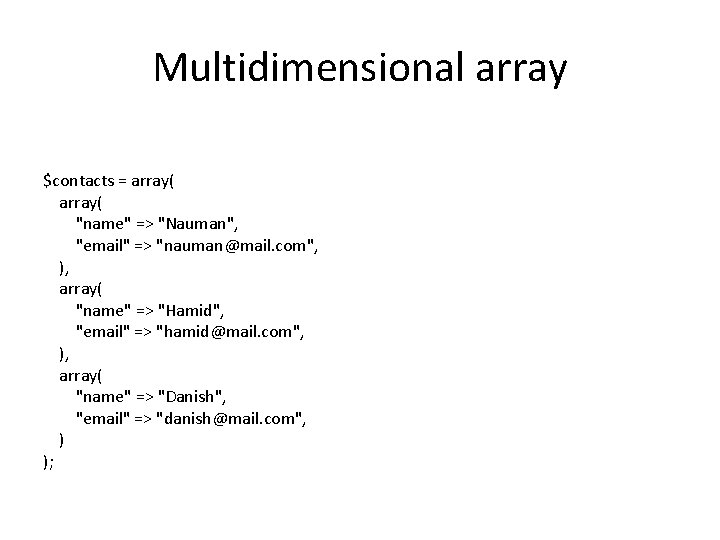
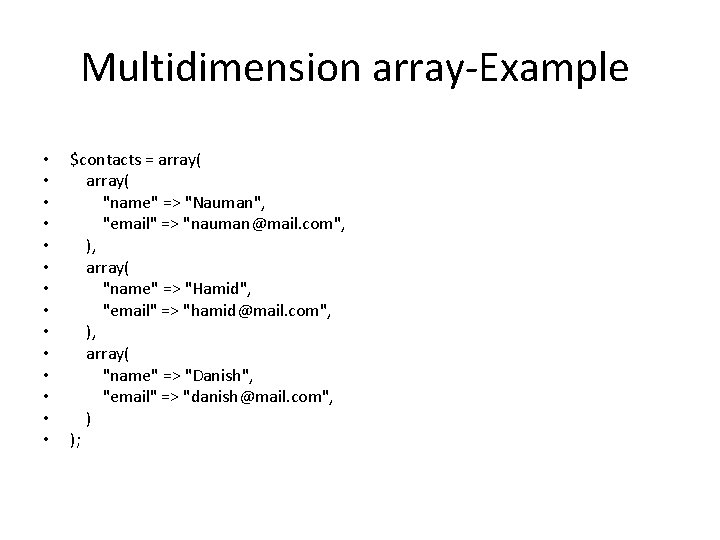
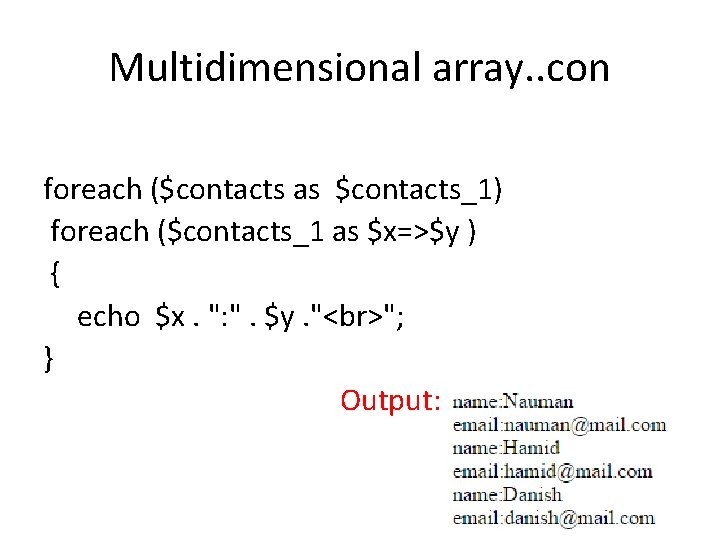
- Slides: 15
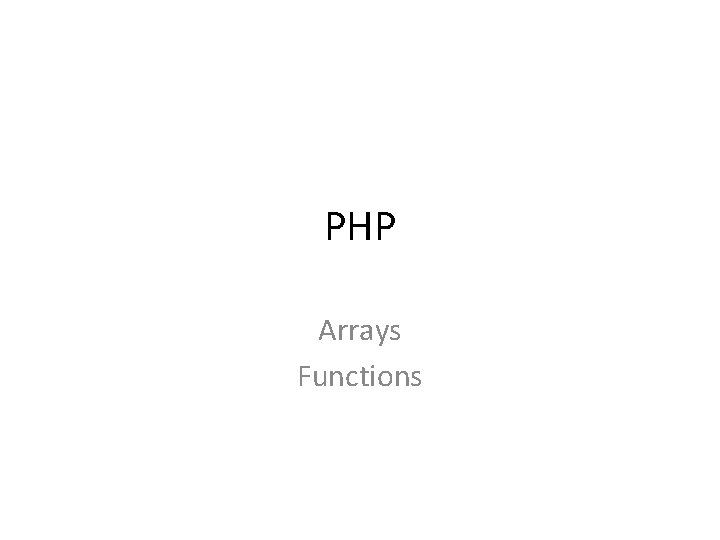
PHP Arrays Functions
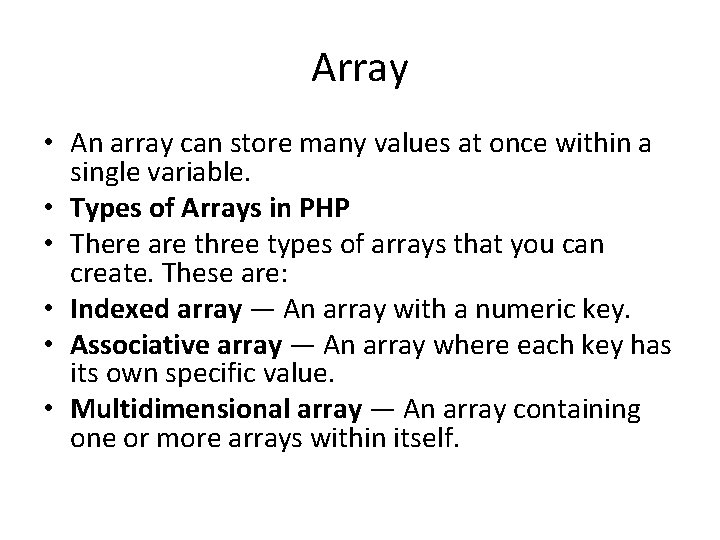
Array • An array can store many values at once within a single variable. • Types of Arrays in PHP • There are three types of arrays that you can create. These are: • Indexed array — An array with a numeric key. • Associative array — An array where each key has its own specific value. • Multidimensional array — An array containing one or more arrays within itself.
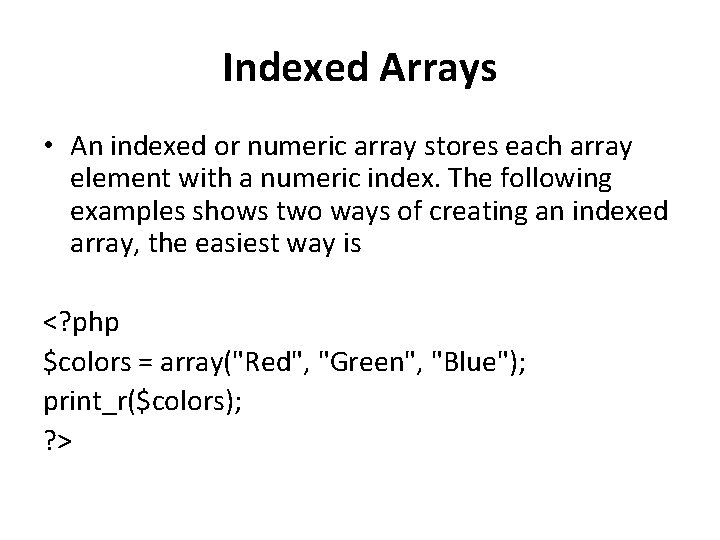
Indexed Arrays • An indexed or numeric array stores each array element with a numeric index. The following examples shows two ways of creating an indexed array, the easiest way is <? php $colors = array("Red", "Green", "Blue"); print_r($colors); ? >
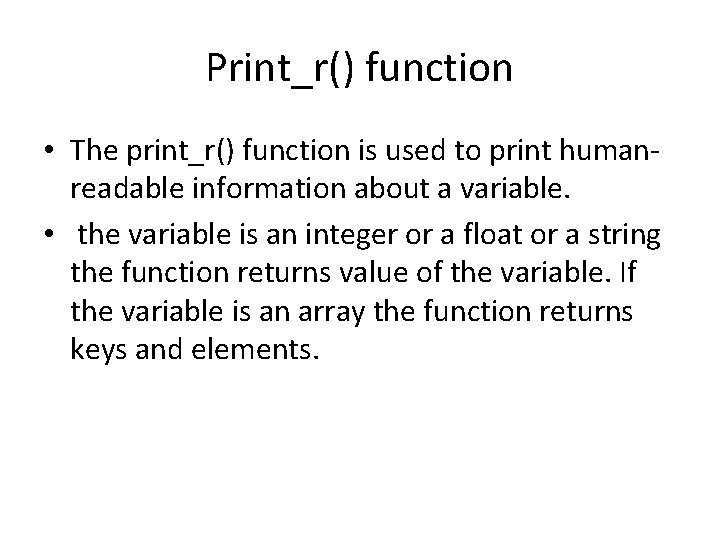
Print_r() function • The print_r() function is used to print humanreadable information about a variable. • the variable is an integer or a float or a string the function returns value of the variable. If the variable is an array the function returns keys and elements.
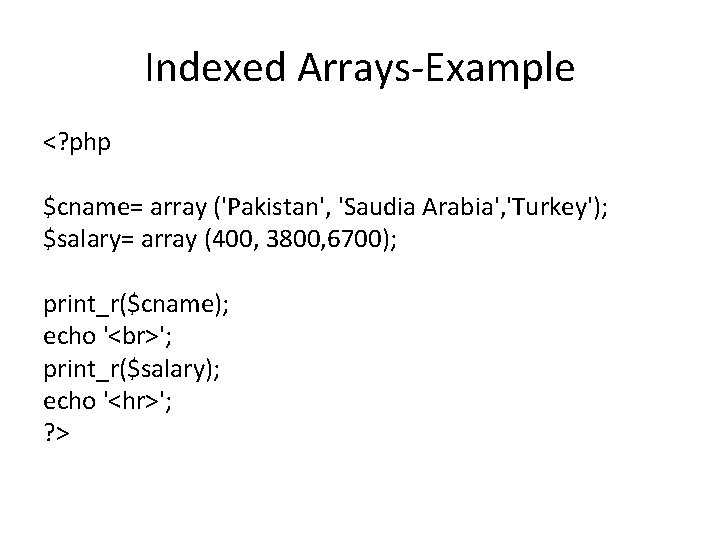
Indexed Arrays-Example <? php $cname= array ('Pakistan', 'Saudia Arabia', 'Turkey'); $salary= array (400, 3800, 6700); print_r($cname); echo ' '; print_r($salary); echo '<hr>'; ? >
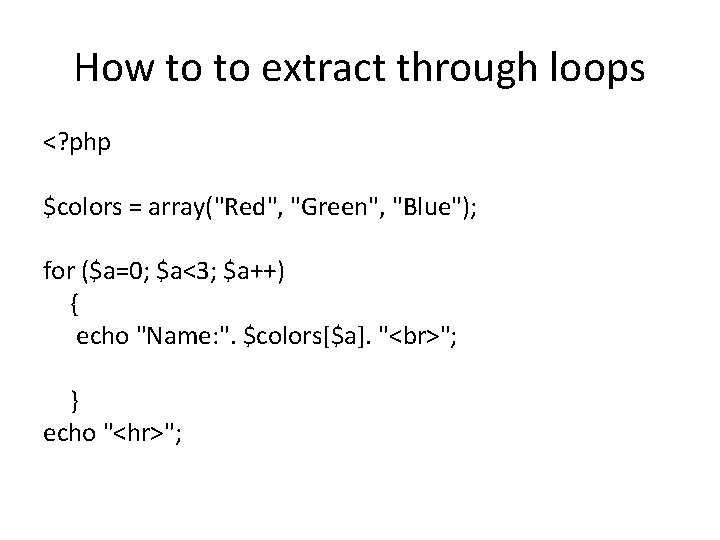
How to to extract through loops <? php $colors = array("Red", "Green", "Blue"); for ($a=0; $a<3; $a++) { echo "Name: ". $colors[$a]. " "; } echo "<hr>";
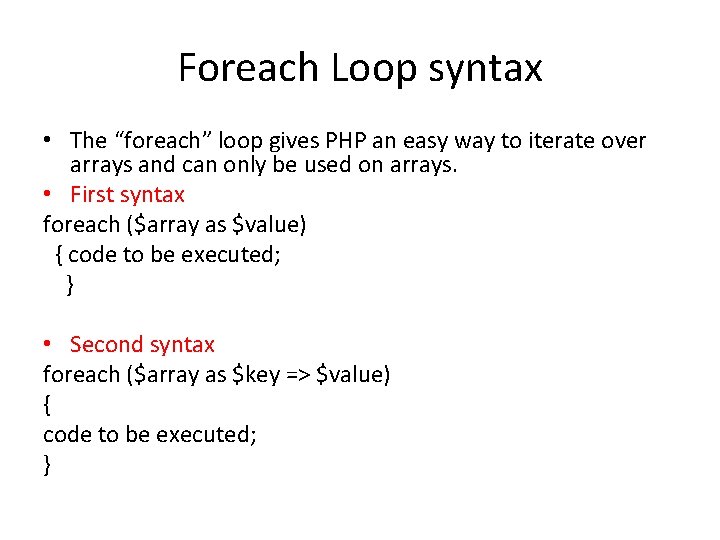
Foreach Loop syntax • The “foreach” loop gives PHP an easy way to iterate over arrays and can only be used on arrays. • First syntax foreach ($array as $value) { code to be executed; } • Second syntax foreach ($array as $key => $value) { code to be executed; }
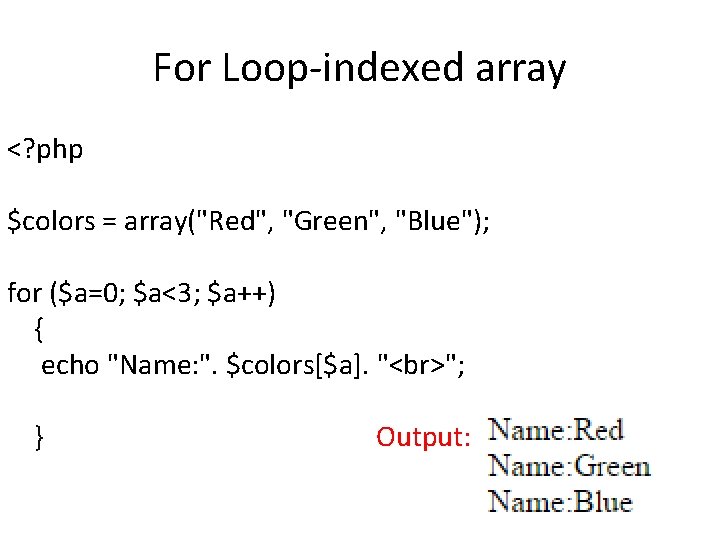
For Loop-indexed array <? php $colors = array("Red", "Green", "Blue"); for ($a=0; $a<3; $a++) { echo "Name: ". $colors[$a]. " "; } Output:
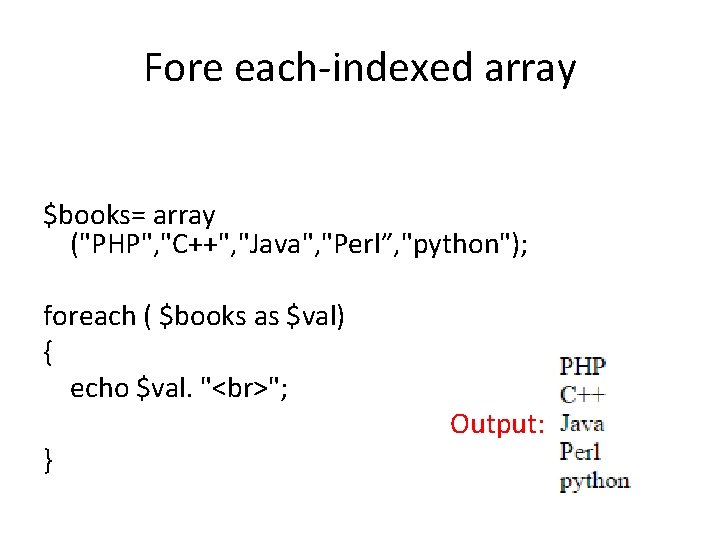
Fore each-indexed array $books= array ("PHP", "C++", "Java", "Perl”, "python"); foreach ( $books as $val) { echo $val. " "; Output: }
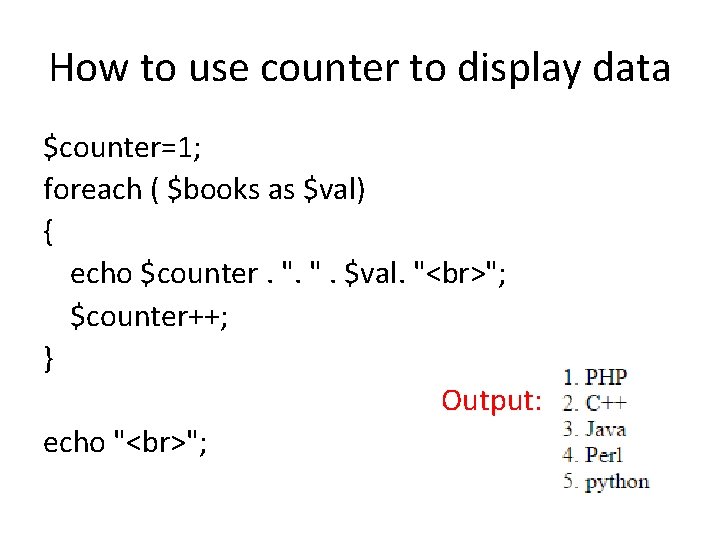
How to use counter to display data $counter=1; foreach ( $books as $val) { echo $counter. ". ". $val. " "; $counter++; } Output: echo " ";
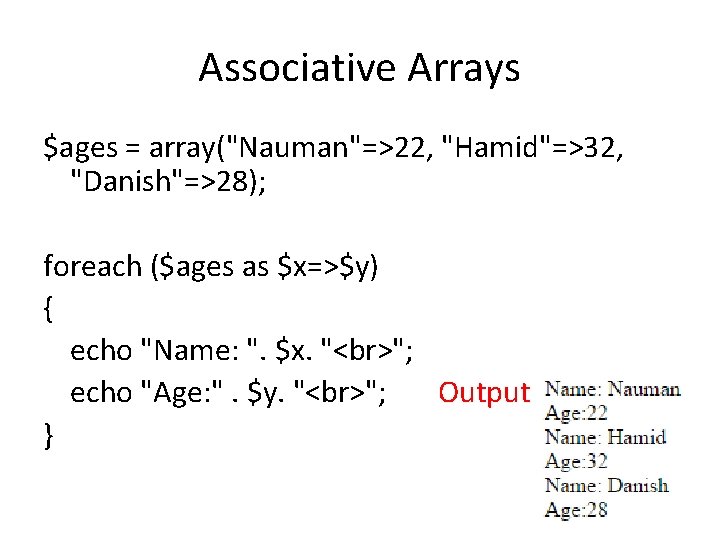
Associative Arrays $ages = array("Nauman"=>22, "Hamid"=>32, "Danish"=>28); foreach ($ages as $x=>$y) { echo "Name: ". $x. " "; echo "Age: ". $y. " "; Output: }
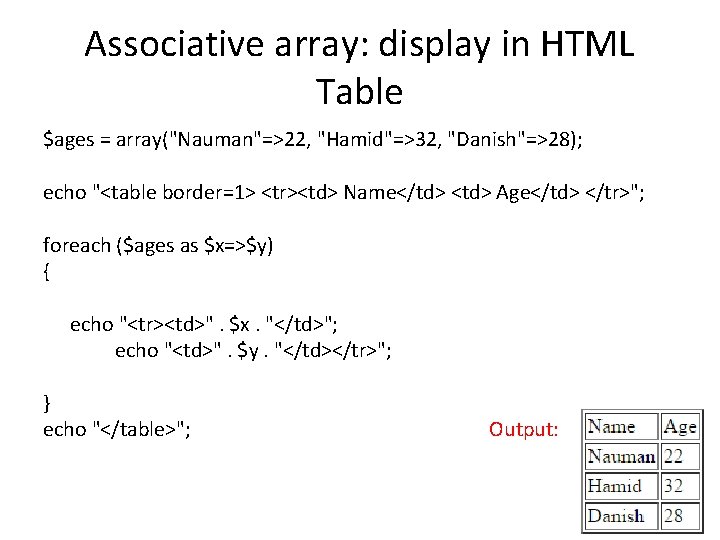
Associative array: display in HTML Table $ages = array("Nauman"=>22, "Hamid"=>32, "Danish"=>28); echo "<table border=1> <tr><td> Name</td> <td> Age</td> </tr>"; foreach ($ages as $x=>$y) { echo "<tr><td>". $x. "</td>"; echo "<td>". $y. "</td></tr>"; } echo "</table>"; Output:
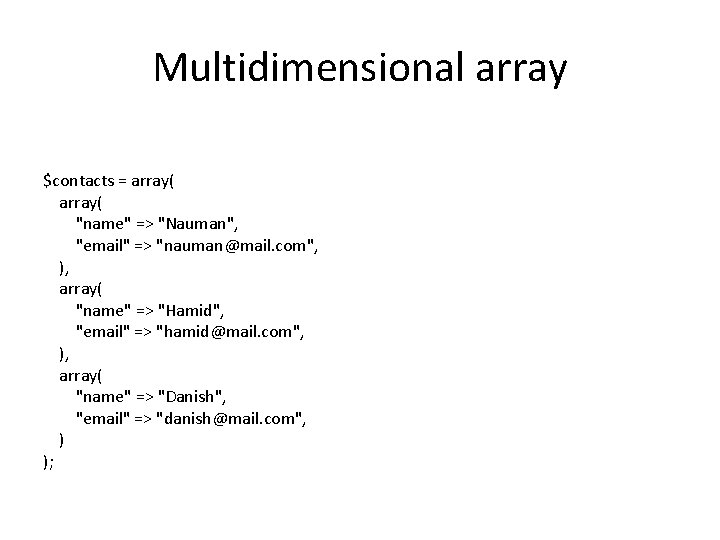
Multidimensional array $contacts = array( "name" => "Nauman", "email" => "nauman@mail. com", ), array( "name" => "Hamid", "email" => "hamid@mail. com", ), array( "name" => "Danish", "email" => "danish@mail. com", ) );
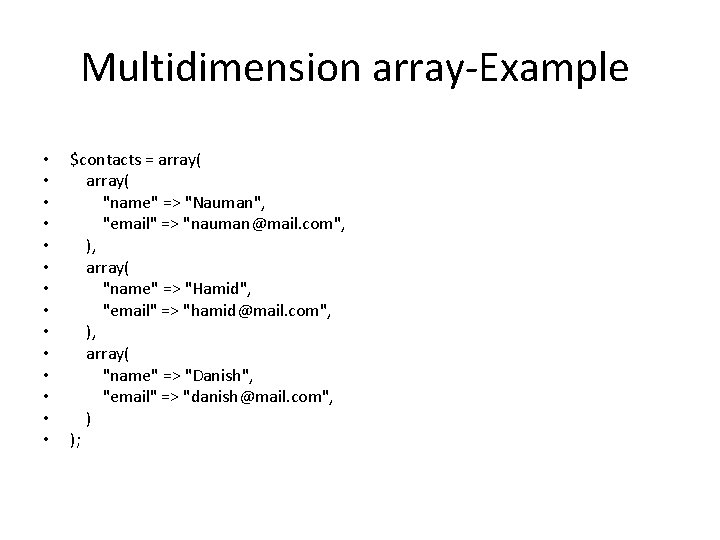
Multidimension array-Example • • • • $contacts = array( "name" => "Nauman", "email" => "nauman@mail. com", ), array( "name" => "Hamid", "email" => "hamid@mail. com", ), array( "name" => "Danish", "email" => "danish@mail. com", ) );
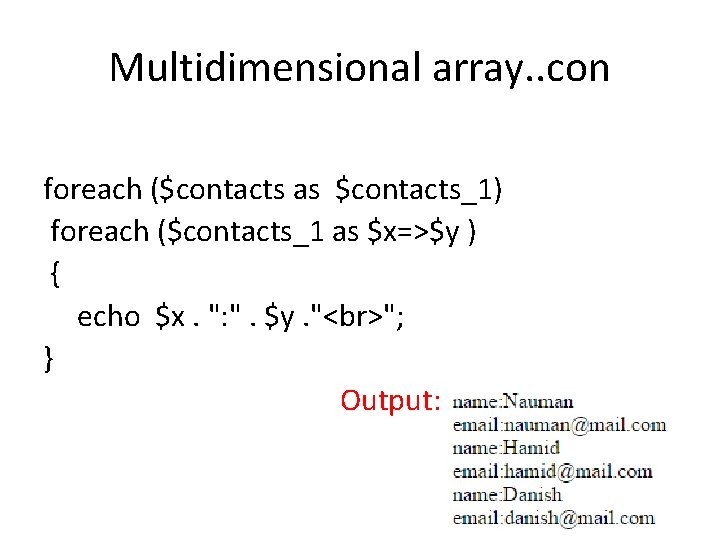
Multidimensional array. . con foreach ($contacts as $contacts_1) foreach ($contacts_1 as $x=>$y ) { echo $x. ": ". $y. " "; } Output:
Array of arrays c++
Php array functions
Row store vs column store
Row store vs column store
Web.facebook.com /profile.php
Php php://input
Php form array
The numerically indexed array of php starts with position
Php array
Php stands for
Array php
Php array definition
Php array average
Parallel array java
Ragged array
Partially filled arrays