Creating PHP Pages Chapter 10 PHP Arrays Arrays
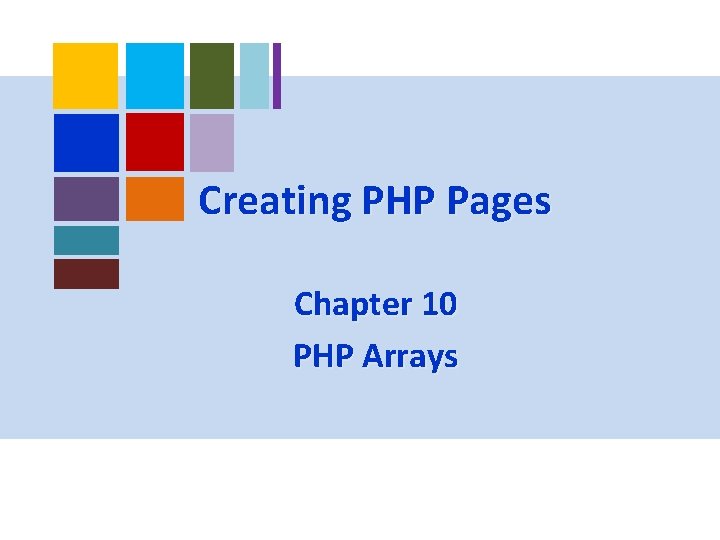
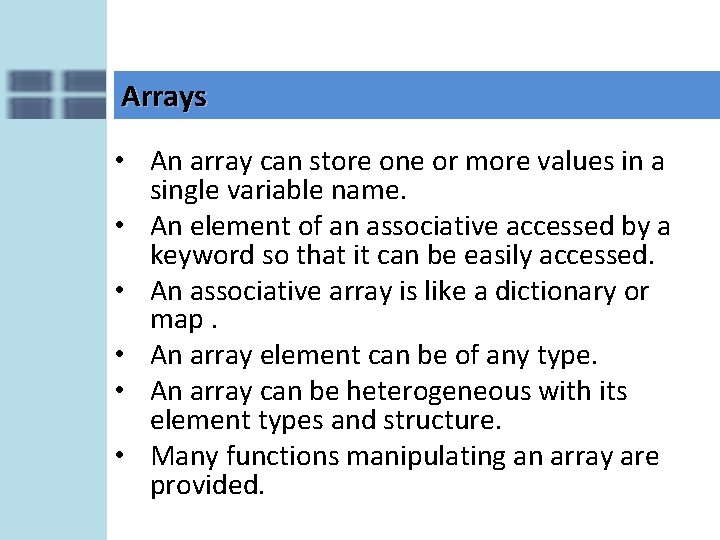
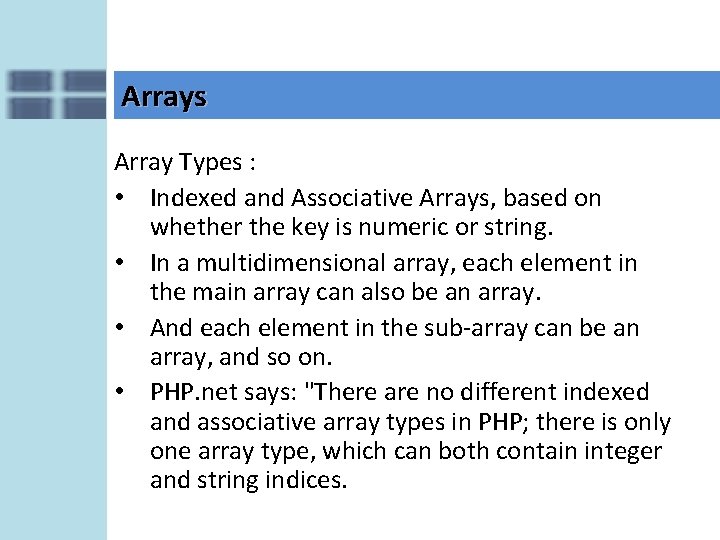
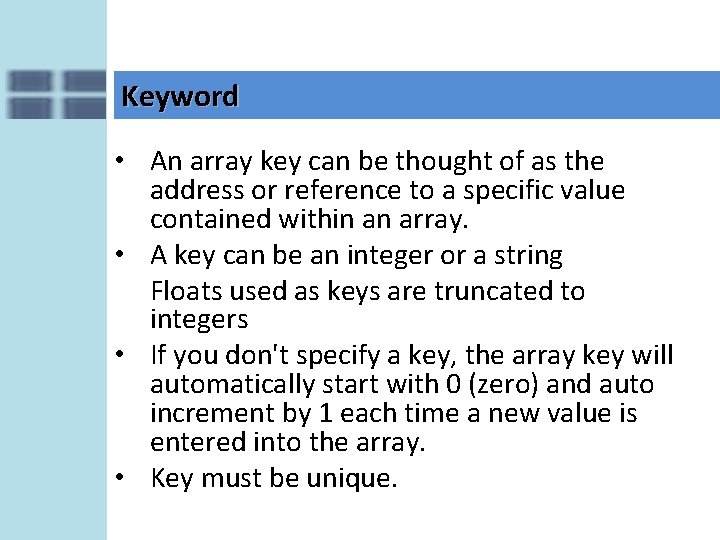
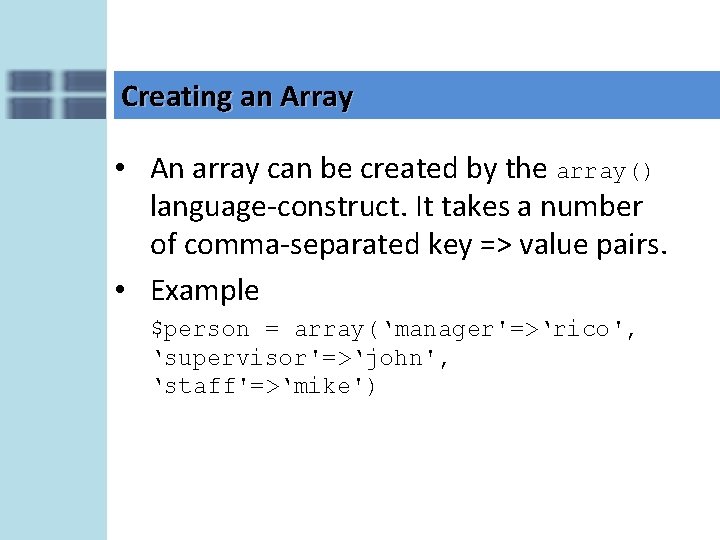
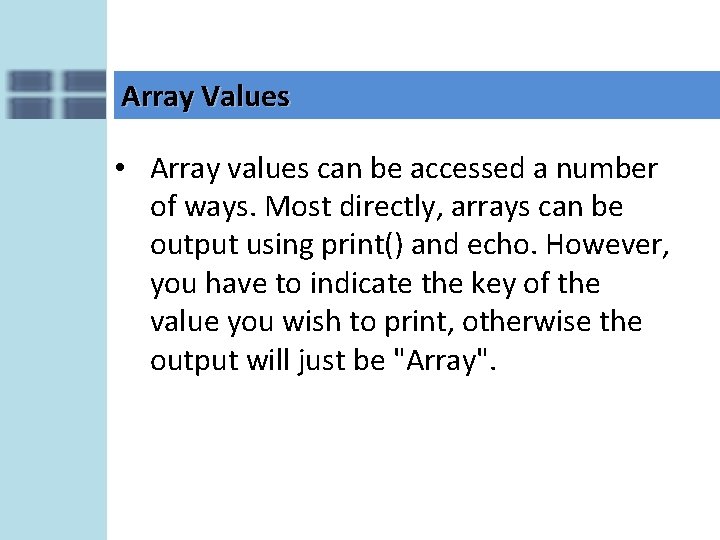
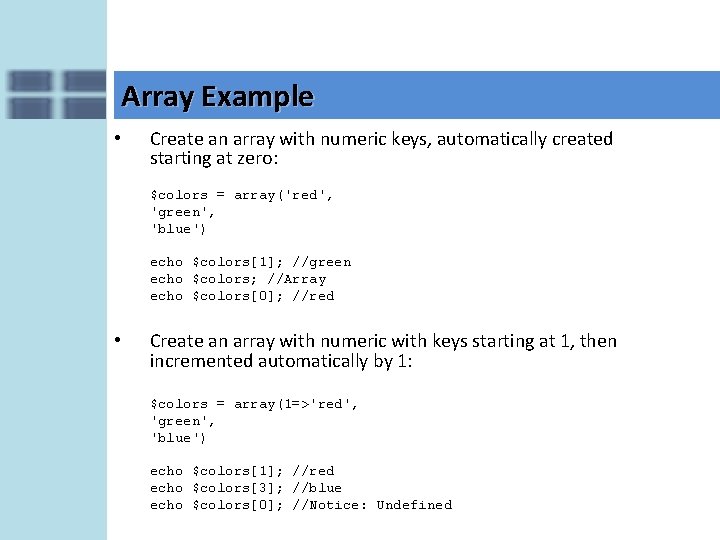
![Indexed Array • Example : $animals = array("dog", "cat", "fish"); echo "$animals[0]n"; echo "$animals[2]n"; Indexed Array • Example : $animals = array("dog", "cat", "fish"); echo "$animals[0]n"; echo "$animals[2]n";](https://slidetodoc.com/presentation_image_h2/8c5ad9aab2002cd500ffaa32c8d33501/image-8.jpg)
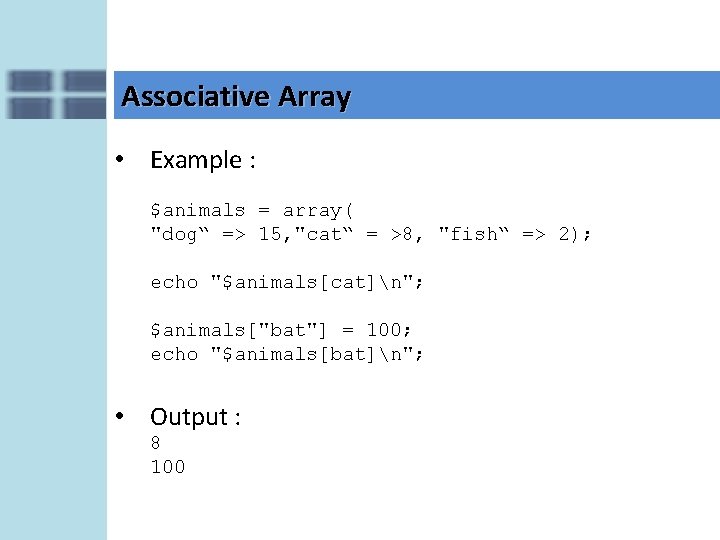
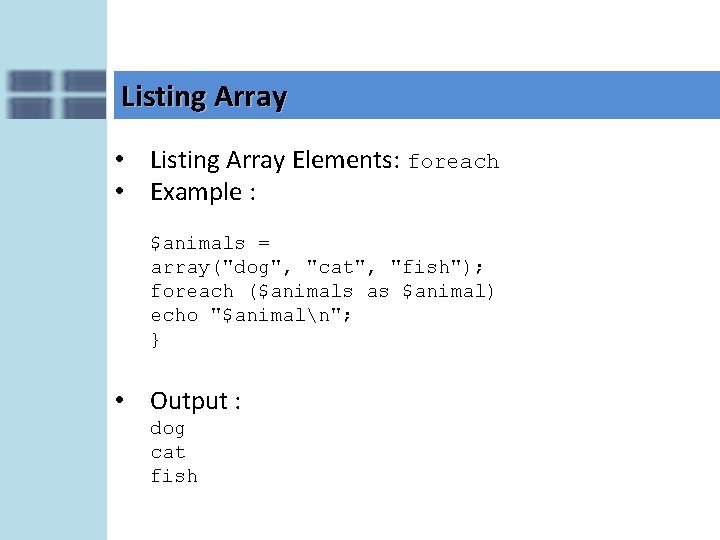
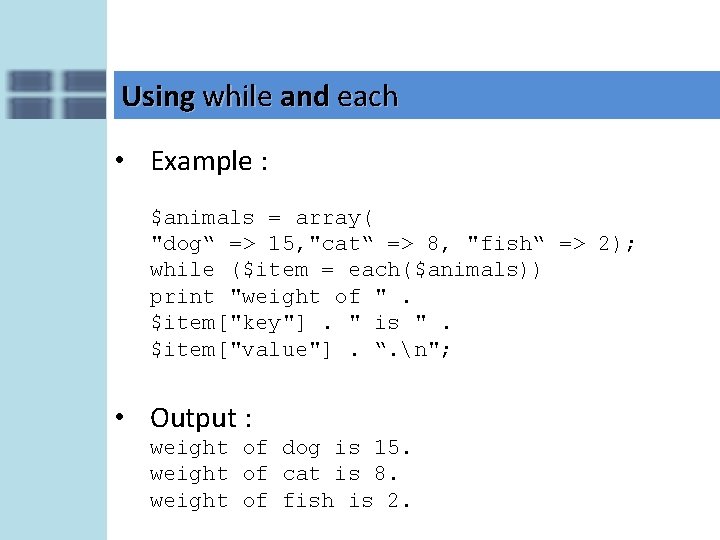
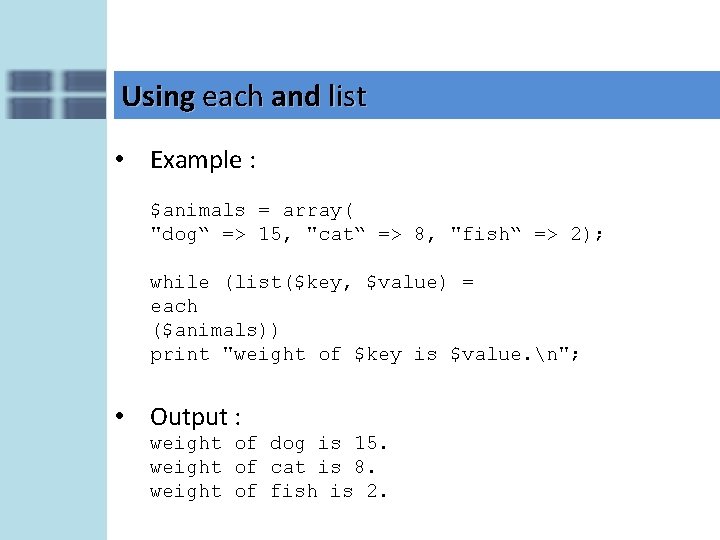
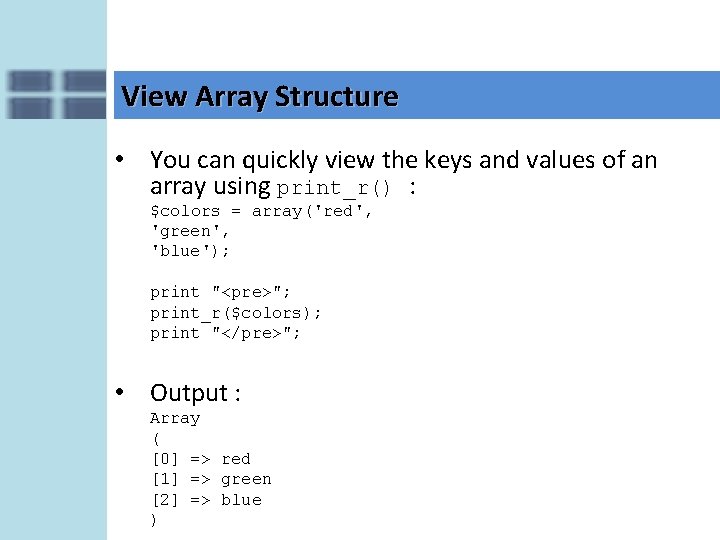
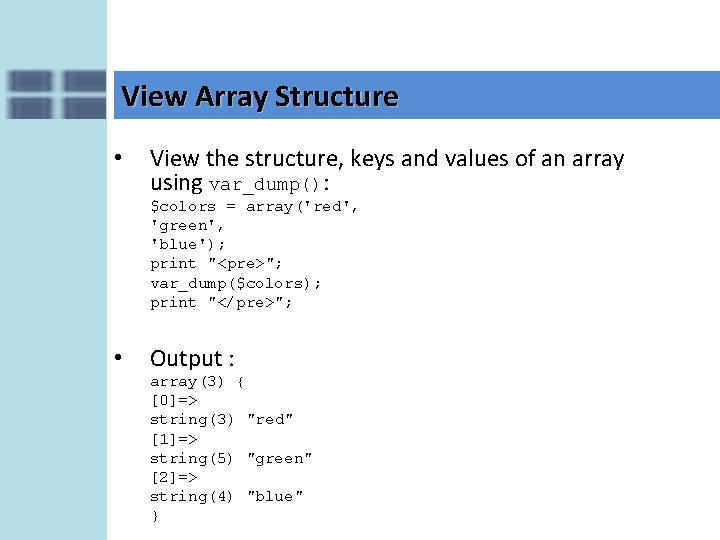
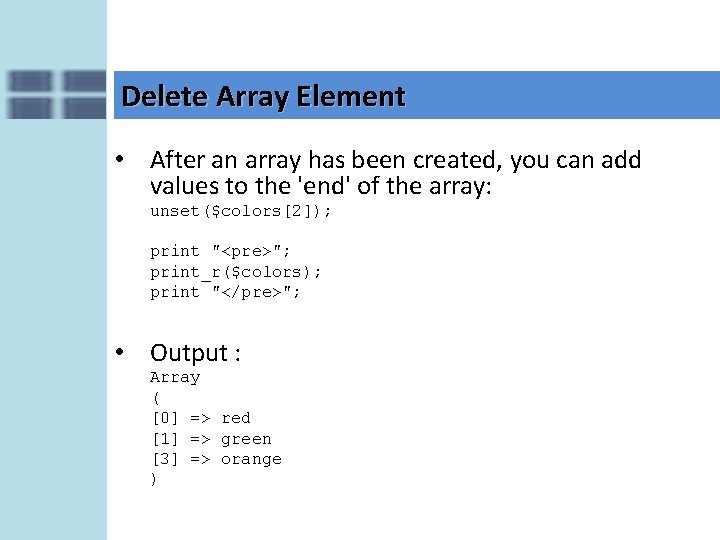
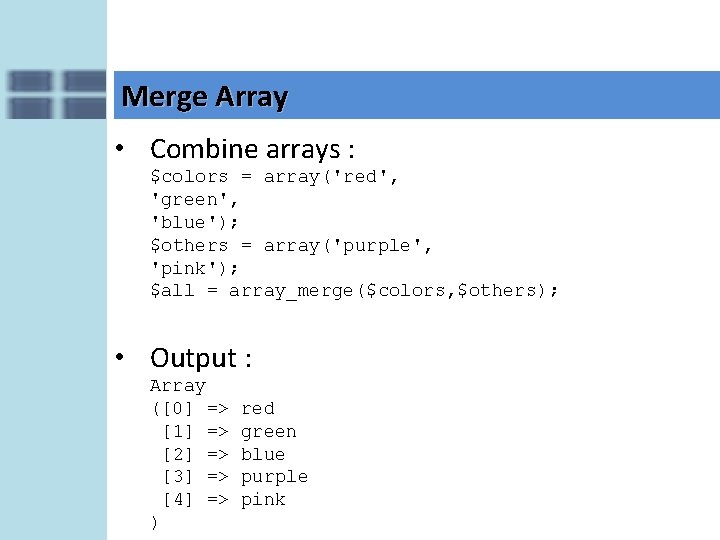
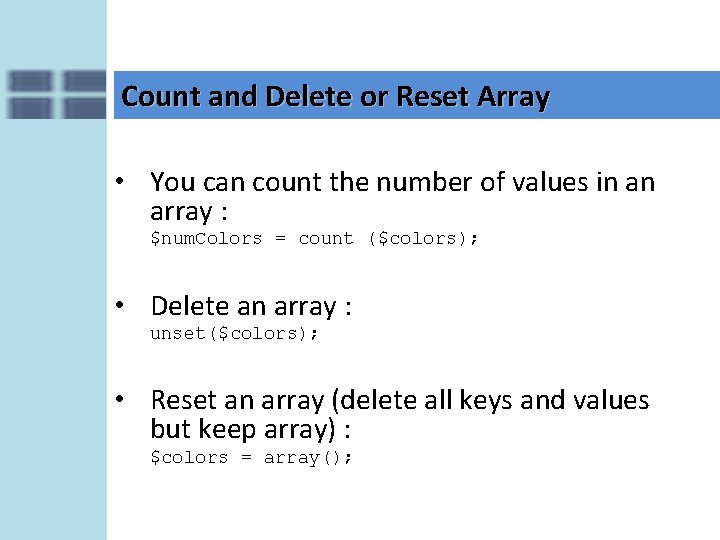
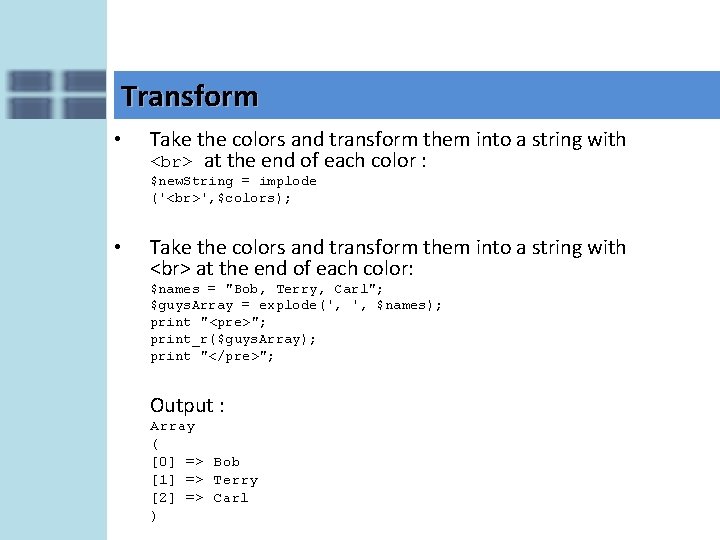
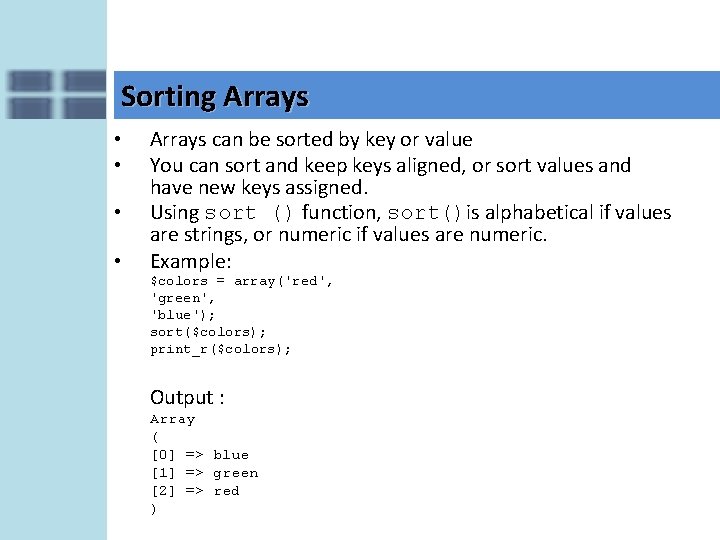
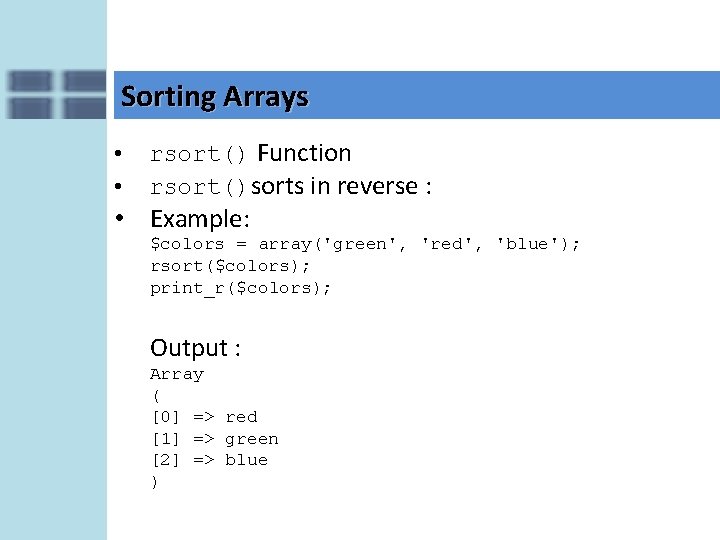
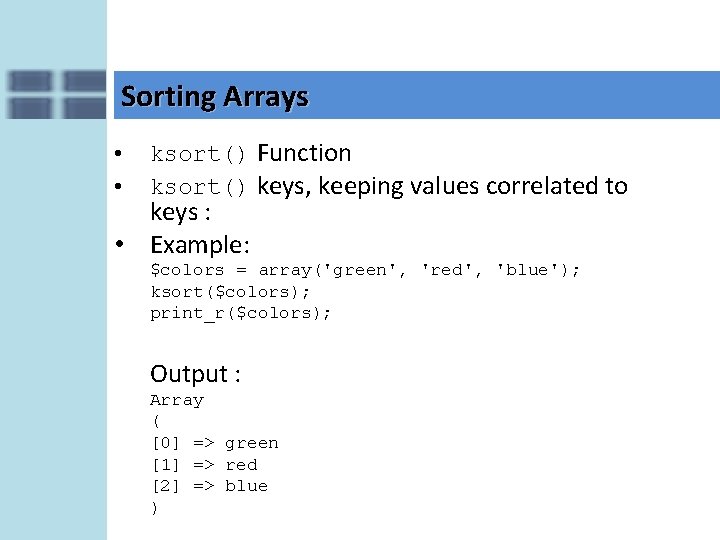
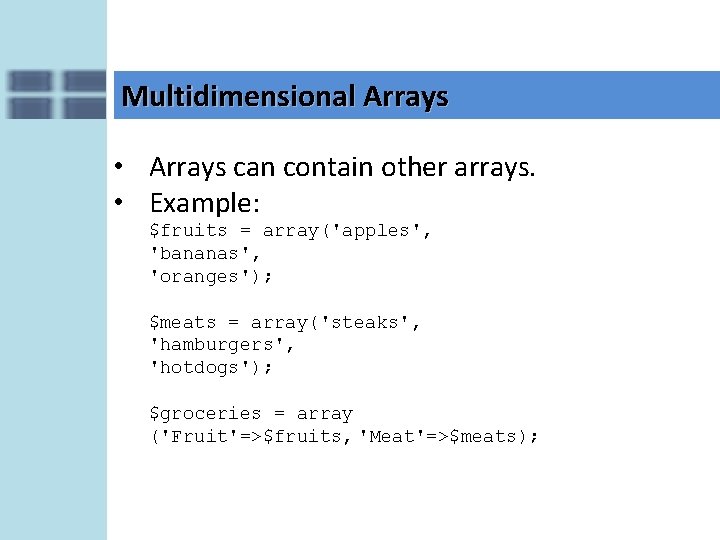
![Multidimensional Arrays • Example: Array ( [Fruit] => Array ( [0] => apples [1] Multidimensional Arrays • Example: Array ( [Fruit] => Array ( [0] => apples [1]](https://slidetodoc.com/presentation_image_h2/8c5ad9aab2002cd500ffaa32c8d33501/image-23.jpg)
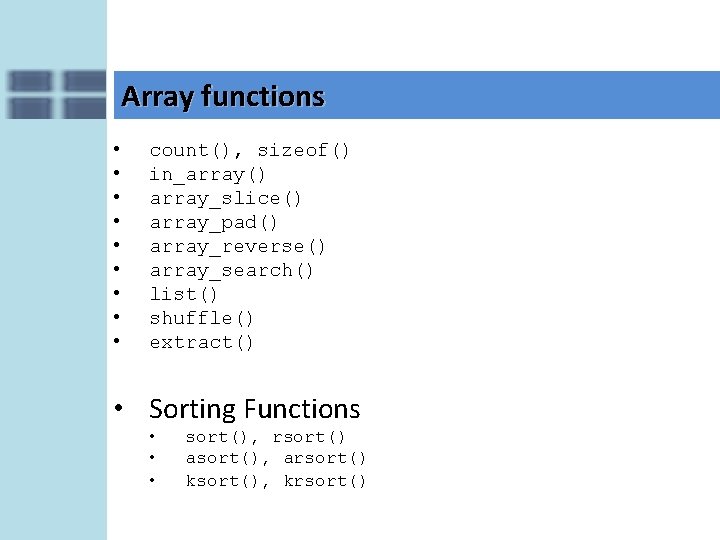
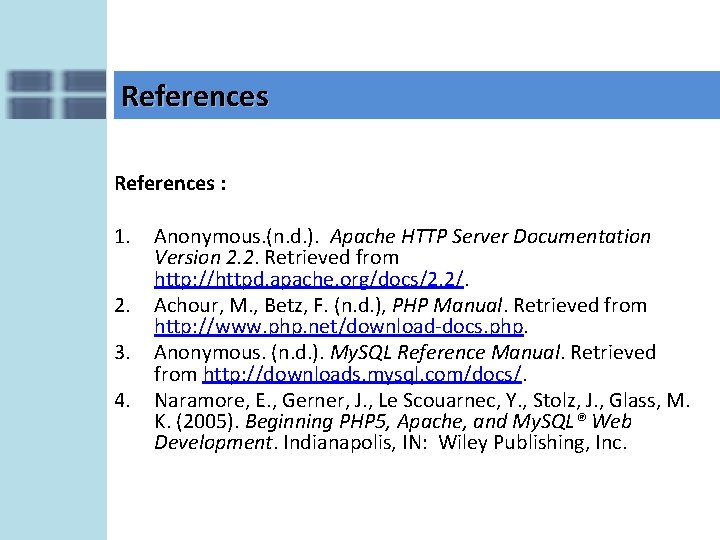
- Slides: 25
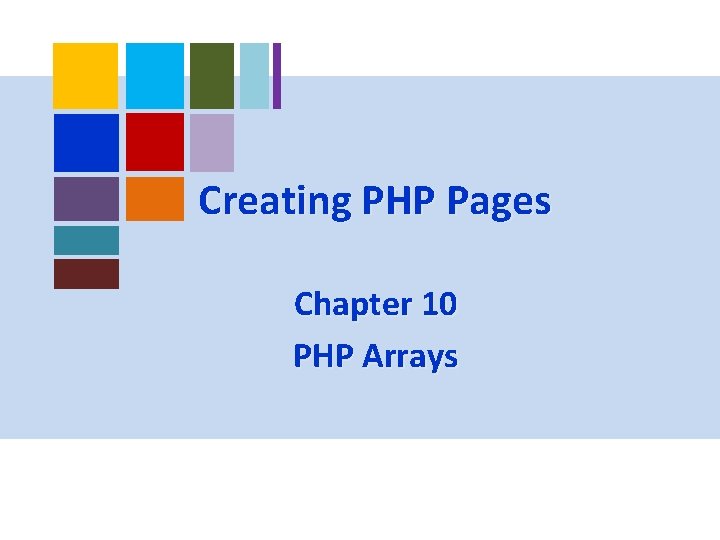
Creating PHP Pages Chapter 10 PHP Arrays
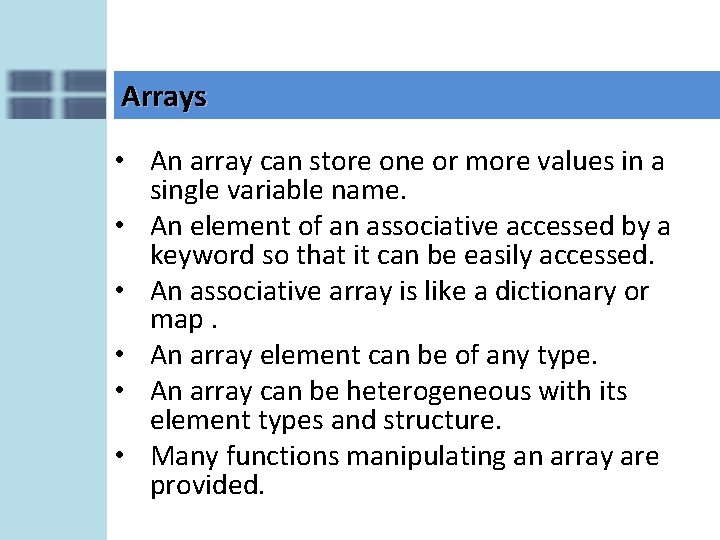
Arrays • An array can store one or more values in a single variable name. • An element of an associative accessed by a keyword so that it can be easily accessed. • An associative array is like a dictionary or map. • An array element can be of any type. • An array can be heterogeneous with its element types and structure. • Many functions manipulating an array are provided.
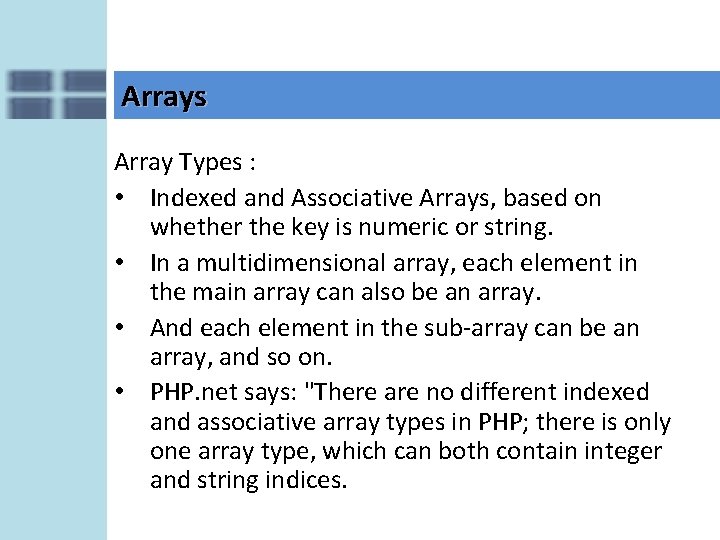
Arrays Array Types : • Indexed and Associative Arrays, based on whether the key is numeric or string. • In a multidimensional array, each element in the main array can also be an array. • And each element in the sub-array can be an array, and so on. • PHP. net says: "There are no different indexed and associative array types in PHP; there is only one array type, which can both contain integer and string indices.
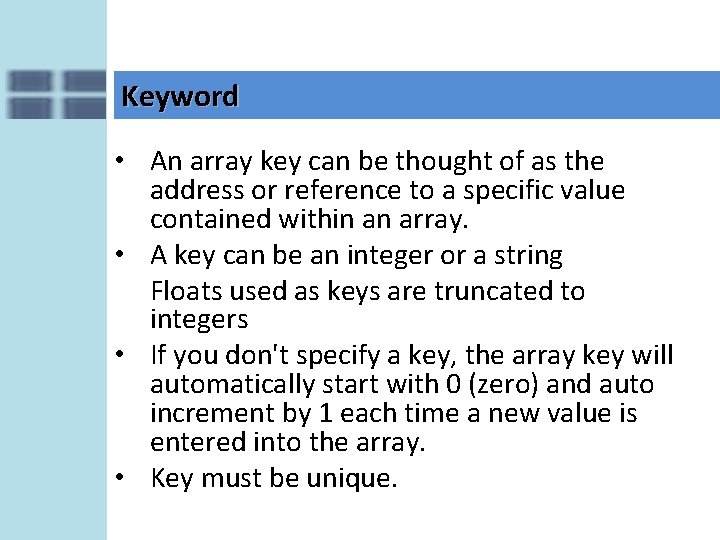
Keyword • An array key can be thought of as the address or reference to a specific value contained within an array. • A key can be an integer or a string Floats used as keys are truncated to integers • If you don't specify a key, the array key will automatically start with 0 (zero) and auto increment by 1 each time a new value is entered into the array. • Key must be unique.
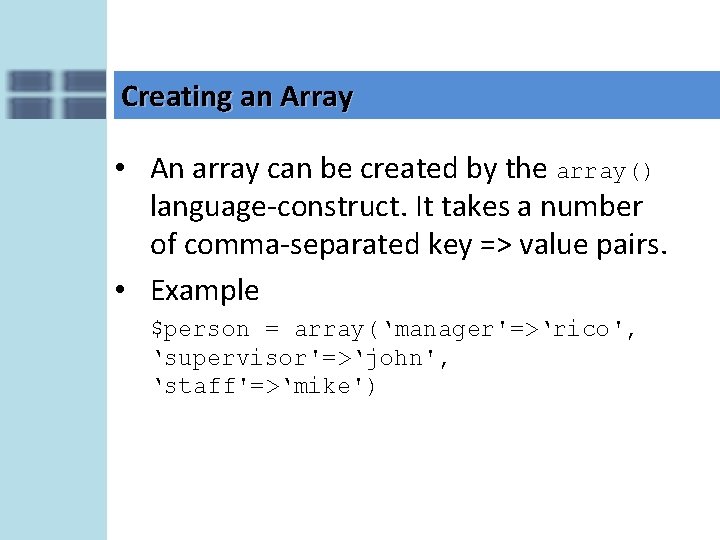
Creating an Array • An array can be created by the array() language-construct. It takes a number of comma-separated key => value pairs. • Example $person = array(‘manager'=>‘rico', ‘supervisor'=>‘john', ‘staff'=>‘mike')
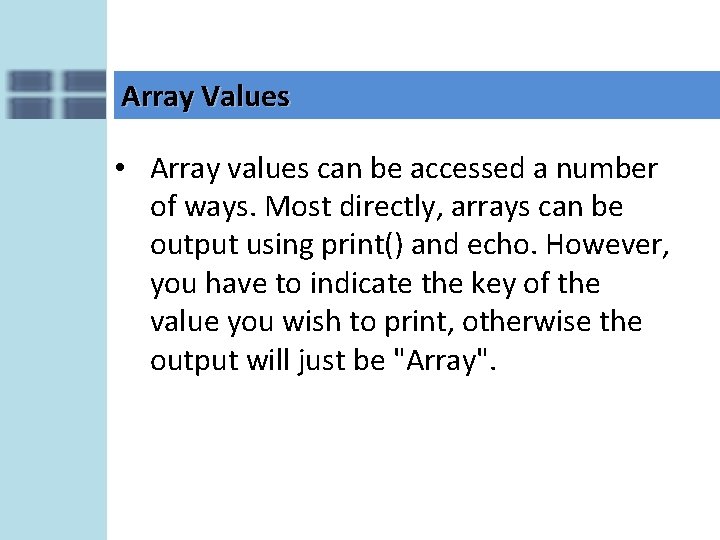
Array Values • Array values can be accessed a number of ways. Most directly, arrays can be output using print() and echo. However, you have to indicate the key of the value you wish to print, otherwise the output will just be "Array".
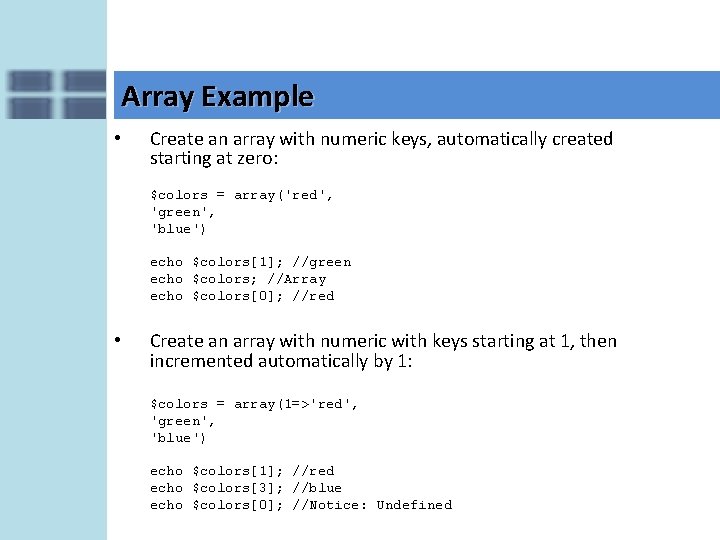
Array Example • Create an array with numeric keys, automatically created starting at zero: $colors = array('red', 'green', 'blue') echo $colors[1]; //green echo $colors; //Array echo $colors[0]; //red • Create an array with numeric with keys starting at 1, then incremented automatically by 1: $colors = array(1=>'red', 'green', 'blue') echo $colors[1]; //red echo $colors[3]; //blue echo $colors[0]; //Notice: Undefined
![Indexed Array Example animals arraydog cat fish echo animals0n echo animals2n Indexed Array • Example : $animals = array("dog", "cat", "fish"); echo "$animals[0]n"; echo "$animals[2]n";](https://slidetodoc.com/presentation_image_h2/8c5ad9aab2002cd500ffaa32c8d33501/image-8.jpg)
Indexed Array • Example : $animals = array("dog", "cat", "fish"); echo "$animals[0]n"; echo "$animals[2]n"; echo "$animalsn"; • Output : dog fish Array
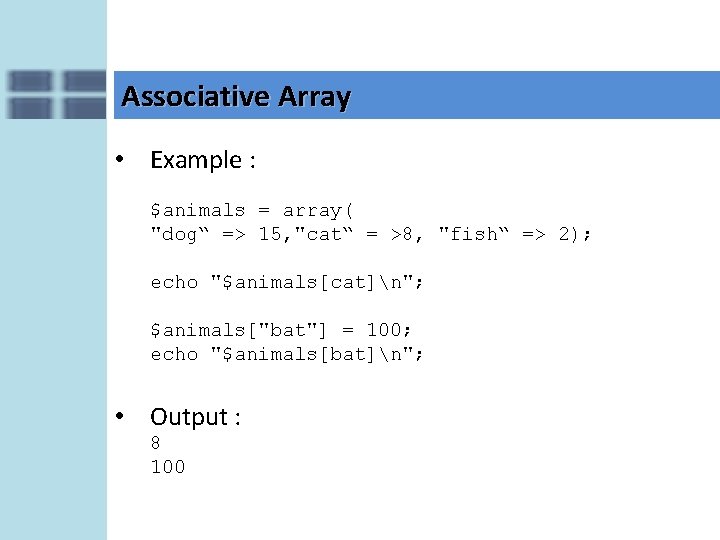
Associative Array • Example : $animals = array( "dog“ => 15, "cat“ = >8, "fish“ => 2); echo "$animals[cat]n"; $animals["bat"] = 100; echo "$animals[bat]n"; • Output : 8 100
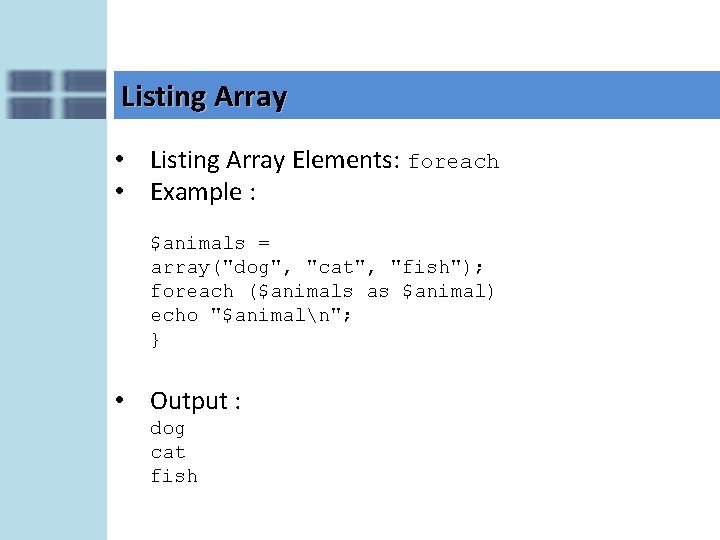
Listing Array • Listing Array Elements: foreach • Example : $animals = array("dog", "cat", "fish"); foreach ($animals as $animal) echo "$animaln"; } • Output : dog cat fish
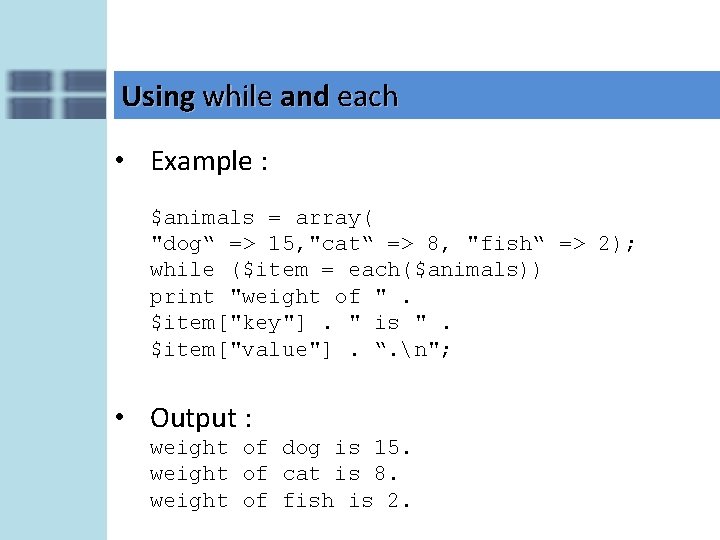
Using while and each • Example : $animals = array( "dog“ => 15, "cat“ => 8, "fish“ => 2); while ($item = each($animals)) print "weight of ". $item["key"]. " is ". $item["value"]. “. n"; • Output : weight of dog is 15. weight of cat is 8. weight of fish is 2.
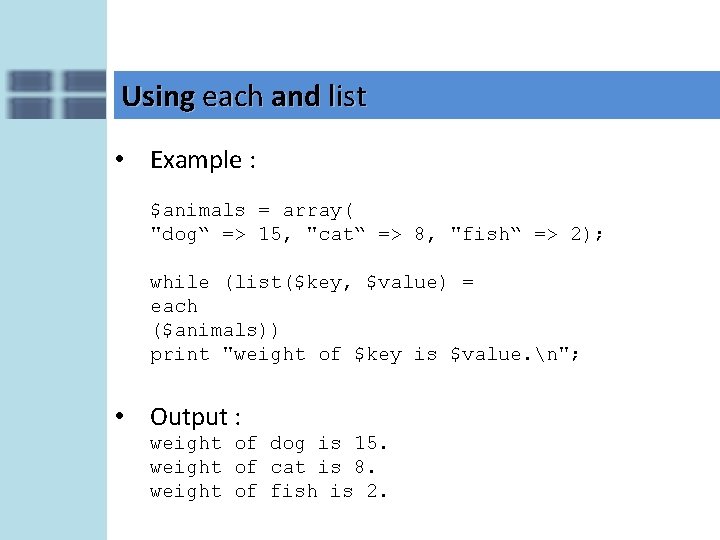
Using each and list • Example : $animals = array( "dog“ => 15, "cat“ => 8, "fish“ => 2); while (list($key, $value) = each ($animals)) print "weight of $key is $value. n"; • Output : weight of dog is 15. weight of cat is 8. weight of fish is 2.
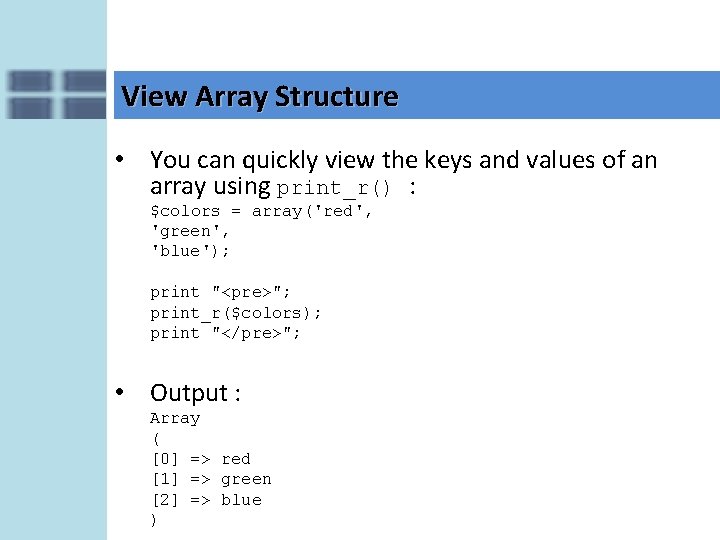
View Array Structure • You can quickly view the keys and values of an array using print_r() : $colors = array('red', 'green', 'blue'); print "<pre>"; print_r($colors); print "</pre>"; • Output : Array ( [0] => red [1] => green [2] => blue )
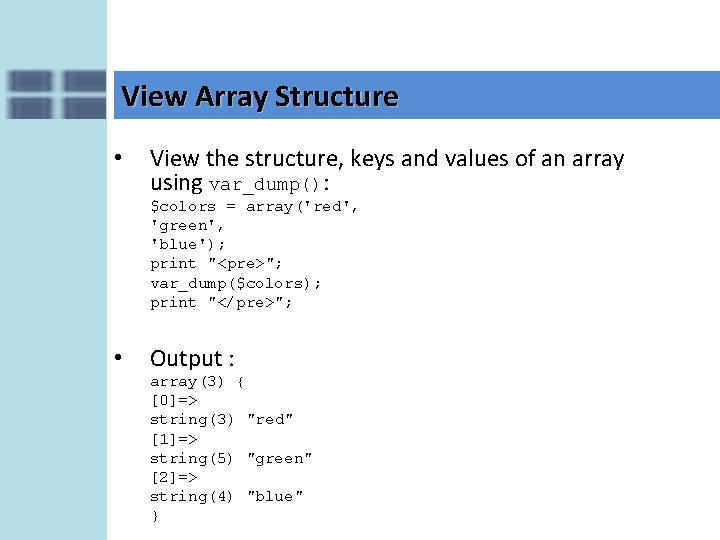
View Array Structure • View the structure, keys and values of an array using var_dump(): $colors = array('red', 'green', 'blue'); print "<pre>"; var_dump($colors); print "</pre>"; • Output : array(3) { [0]=> string(3) "red" [1]=> string(5) "green" [2]=> string(4) "blue" }
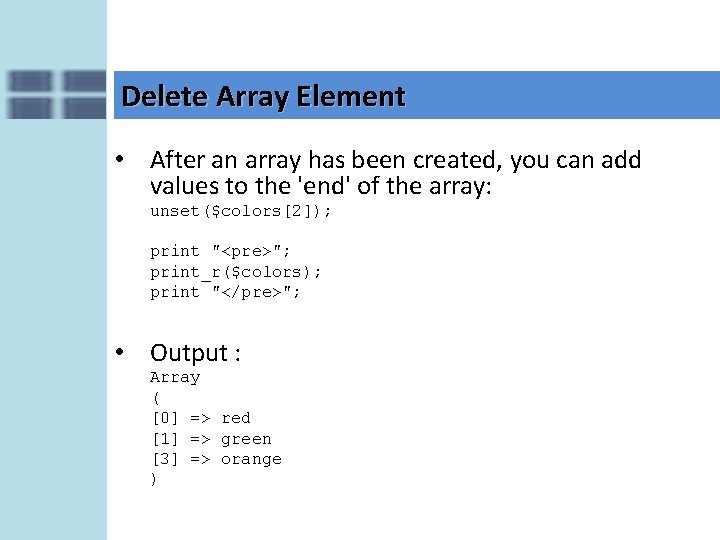
Delete Array Element • After an array has been created, you can add values to the 'end' of the array: unset($colors[2]); print "<pre>"; print_r($colors); print "</pre>"; • Output : Array ( [0] => red [1] => green [3] => orange )
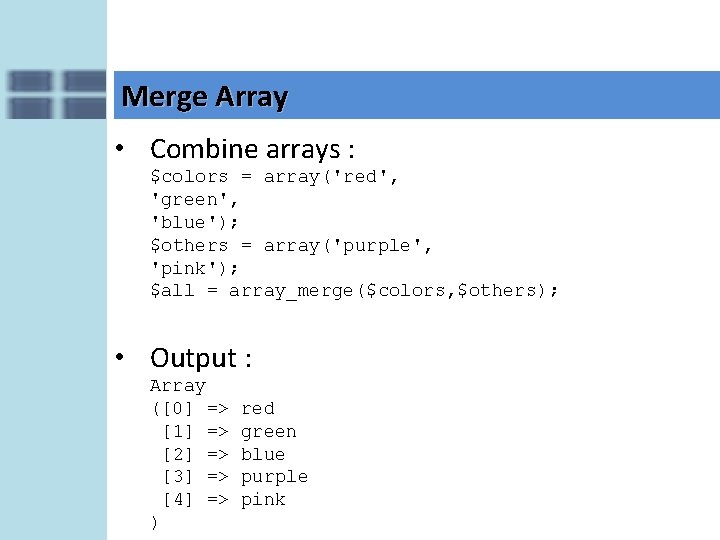
Merge Array • Combine arrays : $colors = array('red', 'green', 'blue'); $others = array('purple', 'pink'); $all = array_merge($colors, $others); • Output : Array ([0] => [1] => [2] => [3] => [4] => ) red green blue purple pink
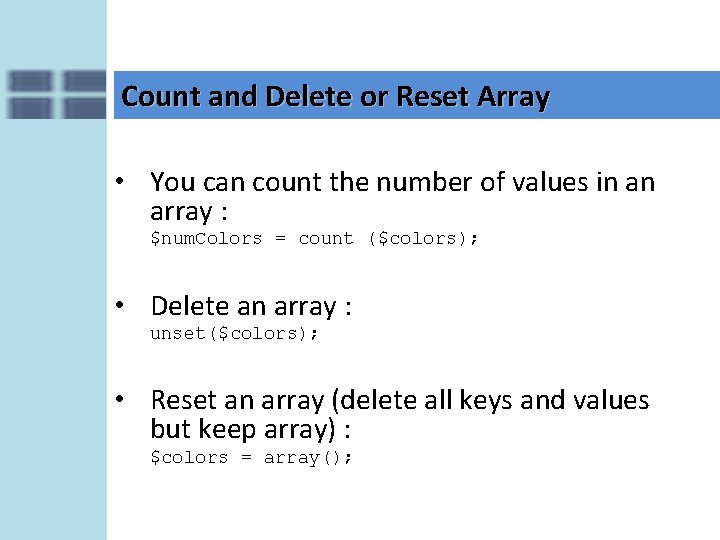
Count and Delete or Reset Array • You can count the number of values in an array : $num. Colors = count ($colors); • Delete an array : unset($colors); • Reset an array (delete all keys and values but keep array) : $colors = array();
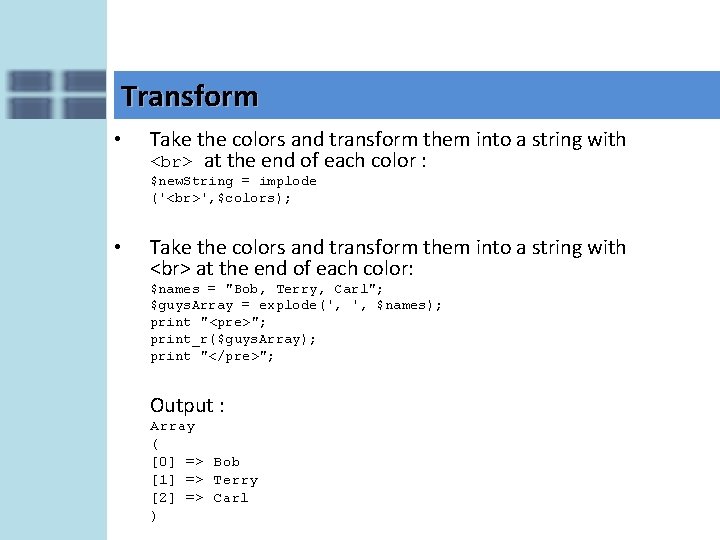
Transform • Take the colors and transform them into a string with at the end of each color : $new. String = implode (' ', $colors); • Take the colors and transform them into a string with at the end of each color: $names = "Bob, Terry, Carl"; $guys. Array = explode(', ', $names); print "<pre>"; print_r($guys. Array); print "</pre>"; Output : Array ( [0] => Bob [1] => Terry [2] => Carl )
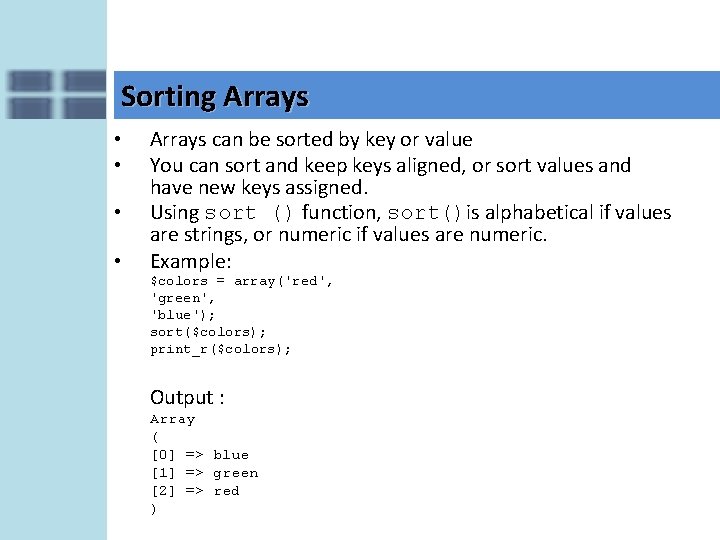
Sorting Arrays • • Arrays can be sorted by key or value You can sort and keep keys aligned, or sort values and have new keys assigned. Using sort () function, sort()is alphabetical if values are strings, or numeric if values are numeric. Example: $colors = array('red', 'green', 'blue'); sort($colors); print_r($colors); Output : Array ( [0] => blue [1] => green [2] => red )
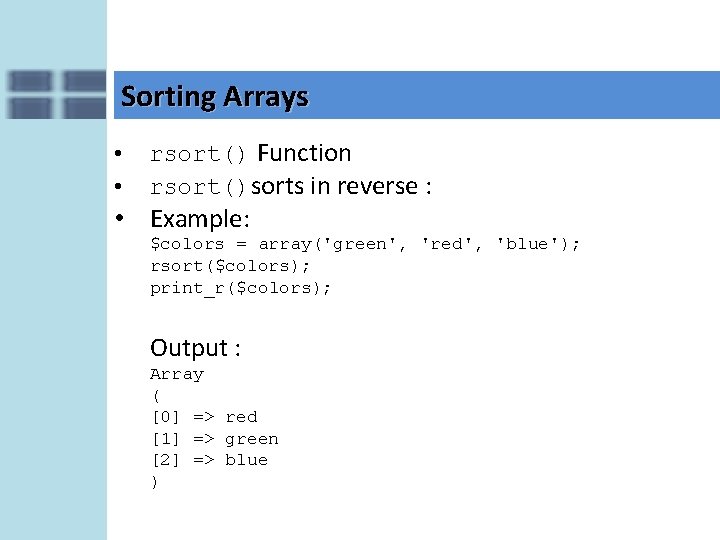
Sorting Arrays • • rsort() Function rsort()sorts in reverse : • Example: $colors = array('green', 'red', 'blue'); rsort($colors); print_r($colors); Output : Array ( [0] => red [1] => green [2] => blue )
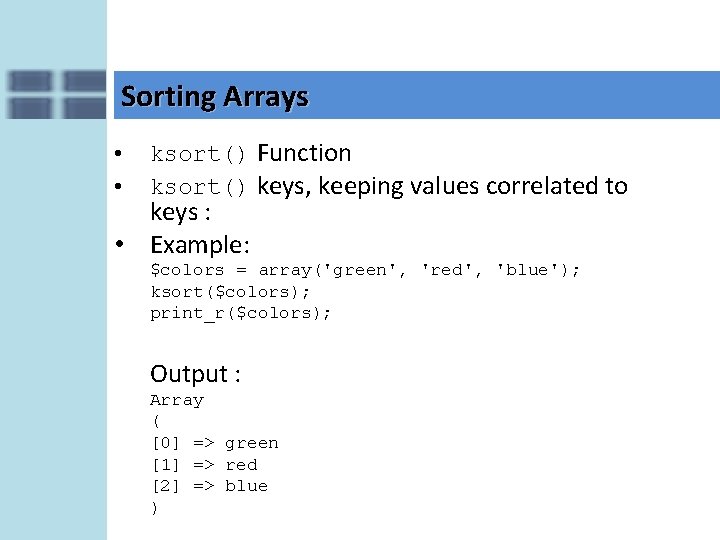
Sorting Arrays • • ksort() Function ksort() keys, keeping values correlated to keys : • Example: $colors = array('green', 'red', 'blue'); ksort($colors); print_r($colors); Output : Array ( [0] => green [1] => red [2] => blue )
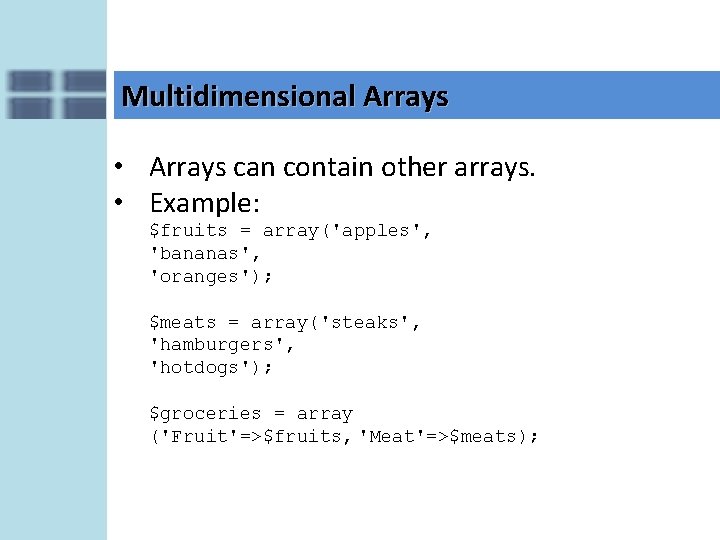
Multidimensional Arrays • Arrays can contain other arrays. • Example: $fruits = array('apples', 'bananas', 'oranges'); $meats = array('steaks', 'hamburgers', 'hotdogs'); $groceries = array ('Fruit'=>$fruits, 'Meat'=>$meats);
![Multidimensional Arrays Example Array Fruit Array 0 apples 1 Multidimensional Arrays • Example: Array ( [Fruit] => Array ( [0] => apples [1]](https://slidetodoc.com/presentation_image_h2/8c5ad9aab2002cd500ffaa32c8d33501/image-23.jpg)
Multidimensional Arrays • Example: Array ( [Fruit] => Array ( [0] => apples [1] => bananas [2] => oranges ) [Meat] => Array ( [0] => steaks [1] => hamburgers [2] => hotdogs ) );
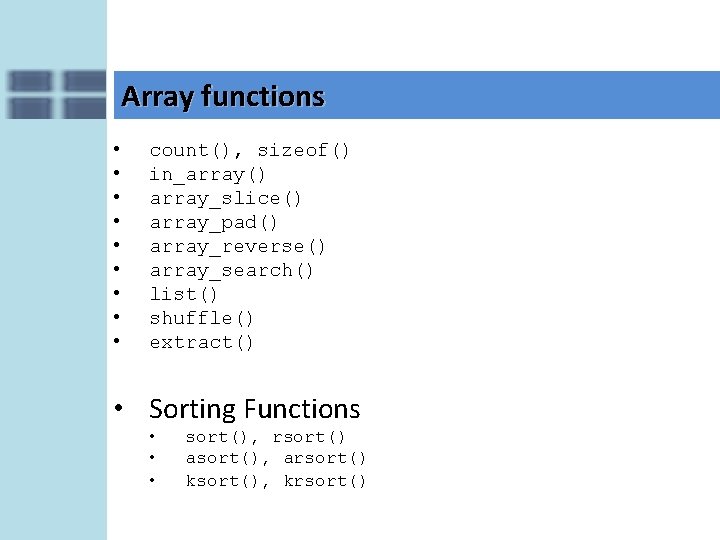
Array functions • • • count(), sizeof() in_array() array_slice() array_pad() array_reverse() array_search() list() shuffle() extract() • Sorting Functions • • • sort(), rsort() asort(), arsort() ksort(), krsort()
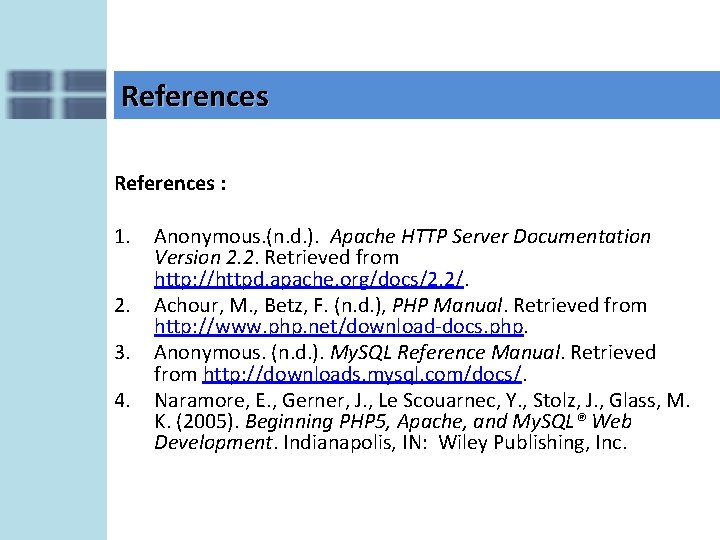
References : 1. 2. 3. 4. Anonymous. (n. d. ). Apache HTTP Server Documentation Version 2. 2. Retrieved from http: //httpd. apache. org/docs/2. 2/. Achour, M. , Betz, F. (n. d. ), PHP Manual. Retrieved from http: //www. php. net/download-docs. php. Anonymous. (n. d. ). My. SQL Reference Manual. Retrieved from http: //downloads. mysql. com/docs/. Naramore, E. , Gerner, J. , Le Scouarnec, Y. , Stolz, J. , Glass, M. K. (2005). Beginning PHP 5, Apache, and My. SQL® Web Development. Indianapolis, IN: Wiley Publishing, Inc.