package jp eclipse surface View import android app
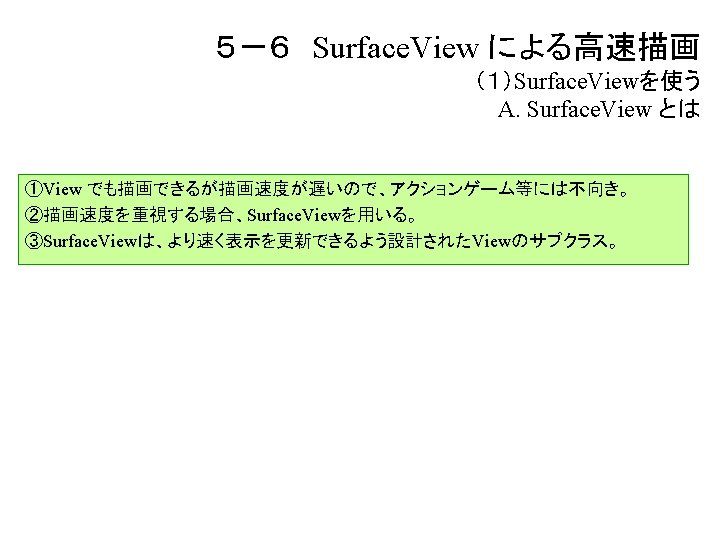
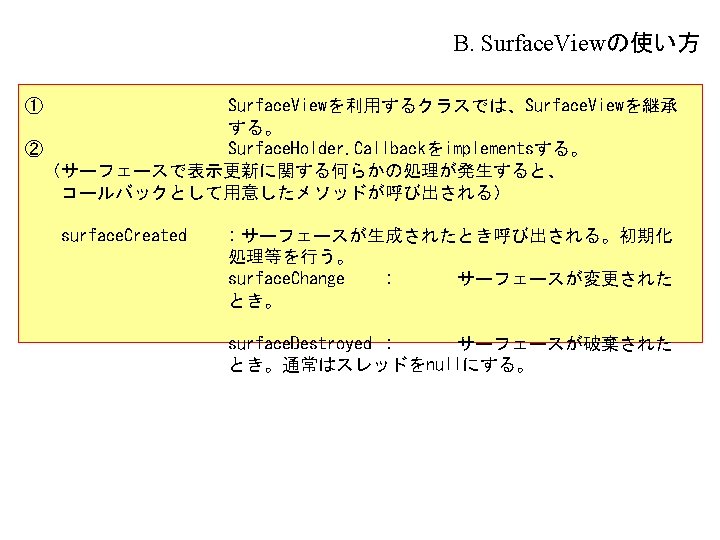
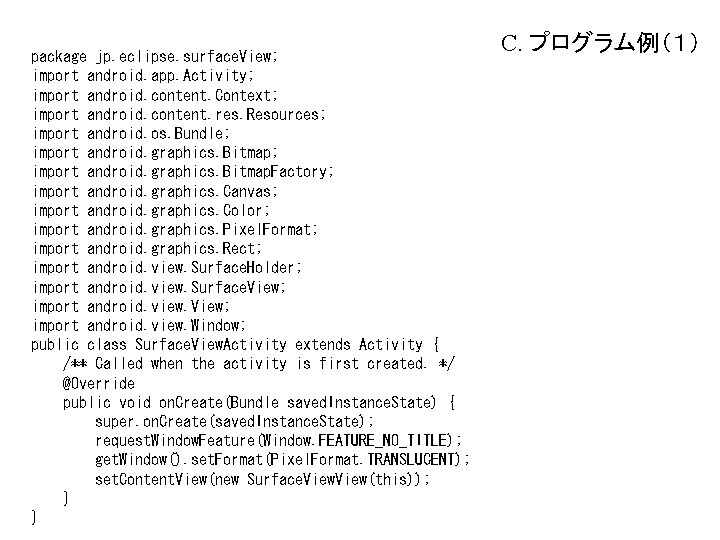
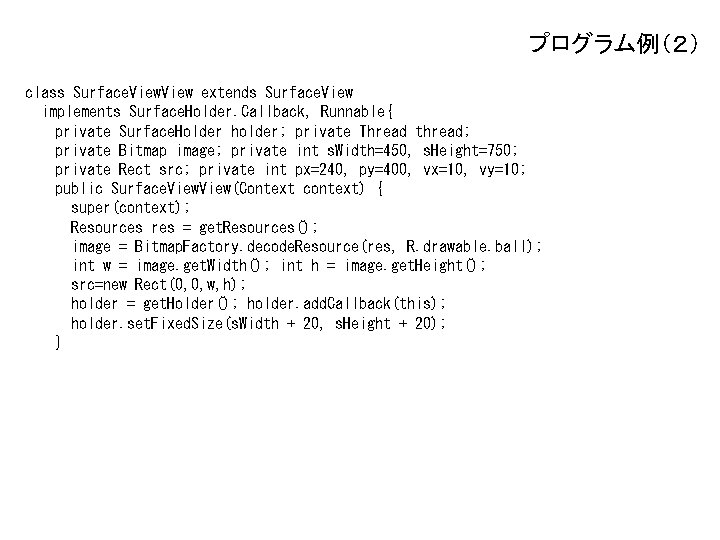
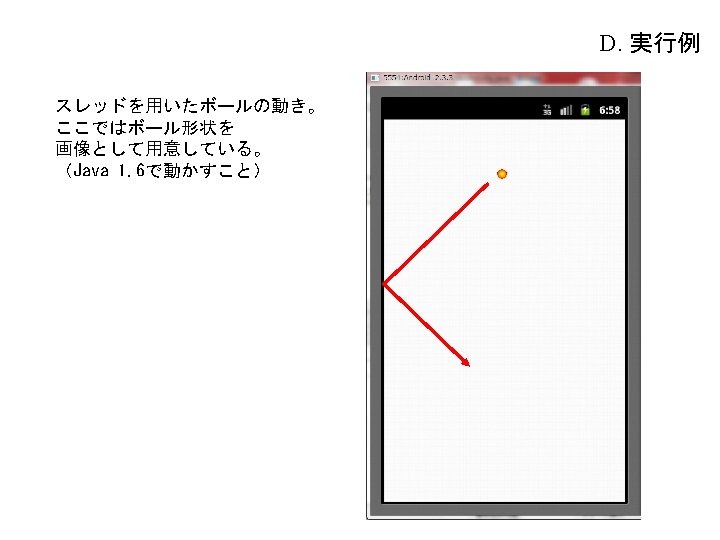
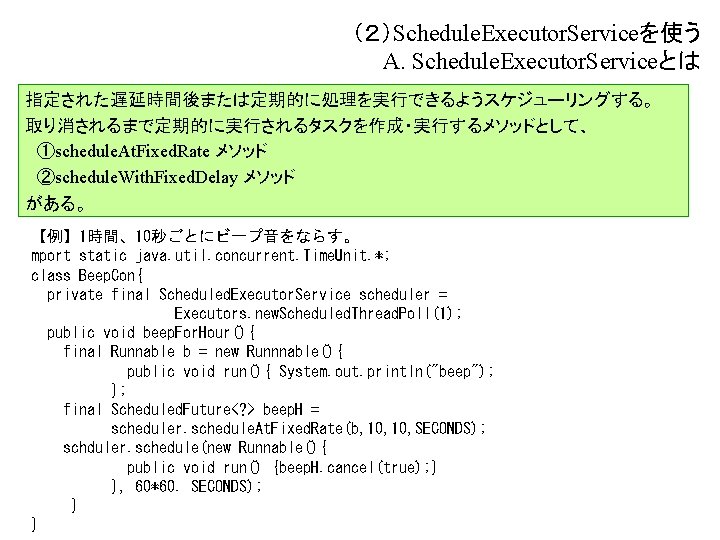
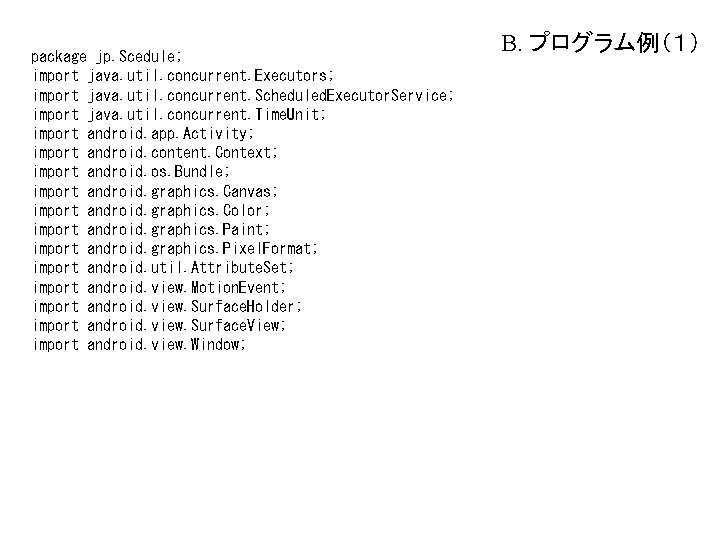
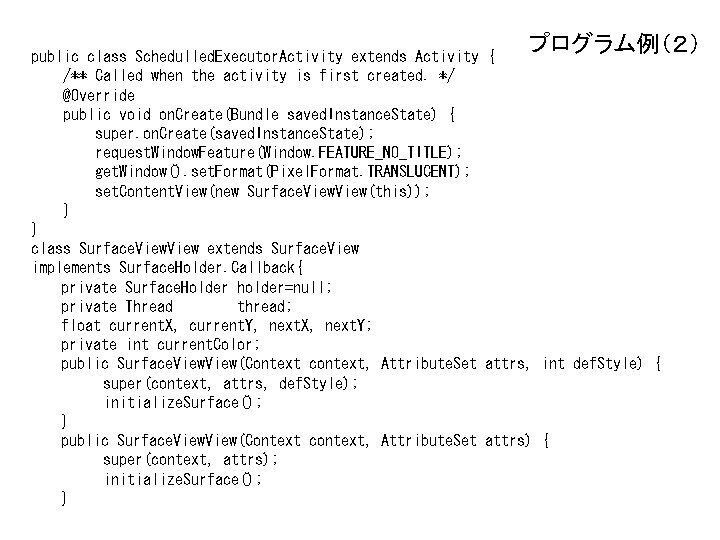
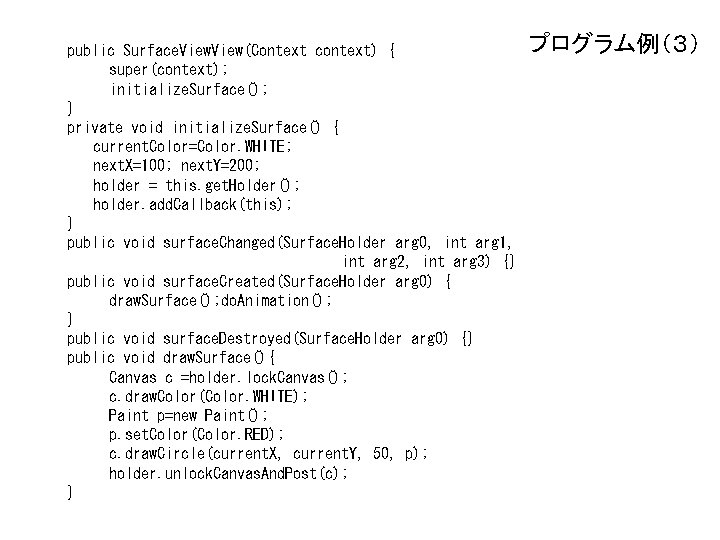
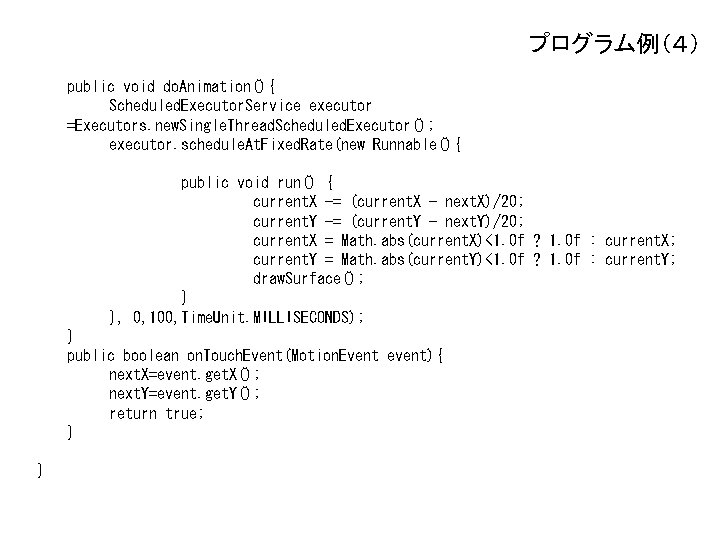
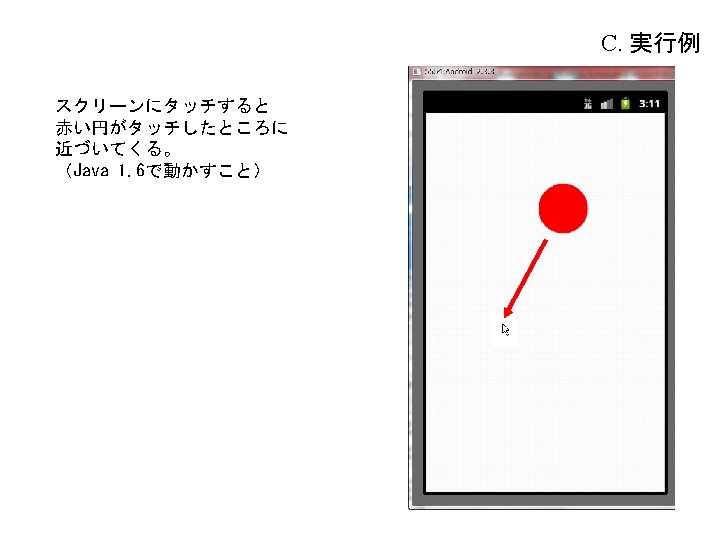
- Slides: 11
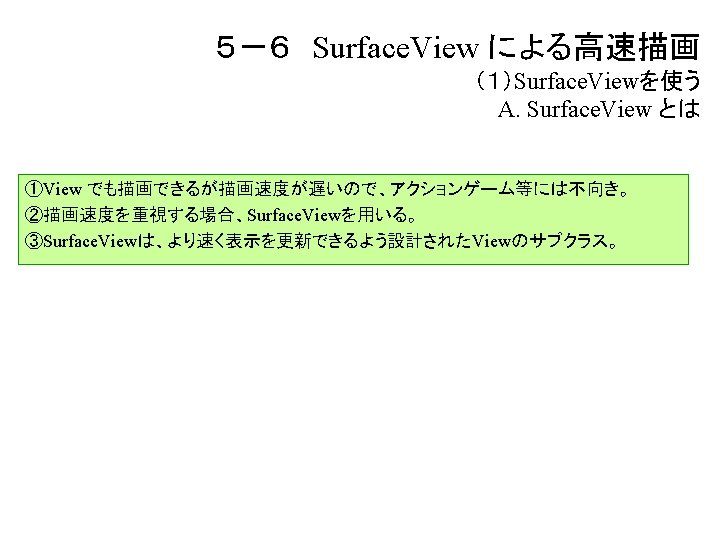
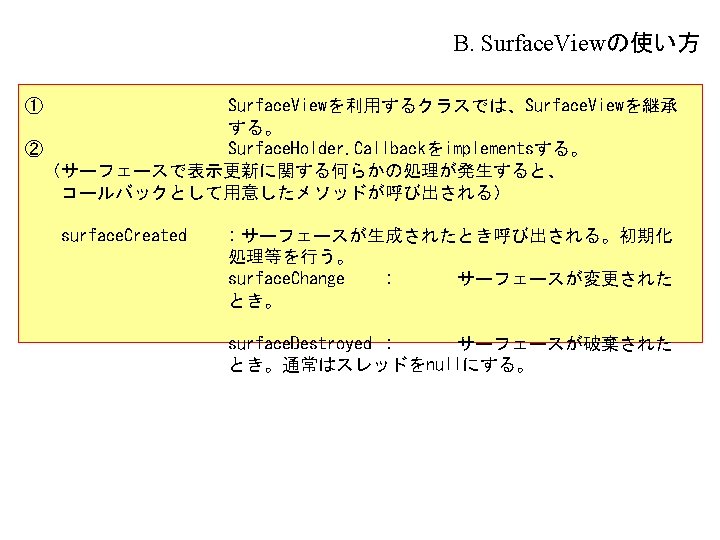
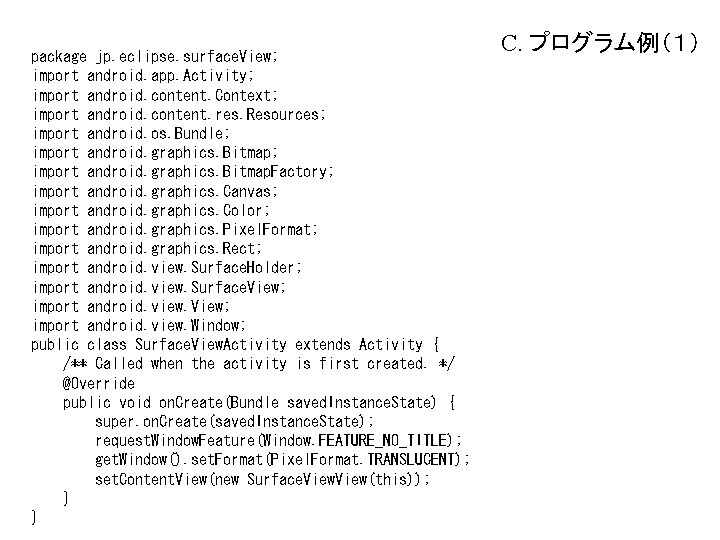
package jp. eclipse. surface. View; import android. app. Activity; import android. content. Context; import android. content. res. Resources; import android. os. Bundle; import android. graphics. Bitmap. Factory; import android. graphics. Canvas; import android. graphics. Color; import android. graphics. Pixel. Format; import android. graphics. Rect; import android. view. Surface. Holder; import android. view. Surface. View; import android. view. Window; public class Surface. View. Activity extends Activity { /** Called when the activity is first created. */ @Override public void on. Create(Bundle saved. Instance. State) { super. on. Create(saved. Instance. State); request. Window. Feature(Window. FEATURE_NO_TITLE); get. Window(). set. Format(Pixel. Format. TRANSLUCENT); set. Content. View(new Surface. View(this)); } } C. プログラム例(1)
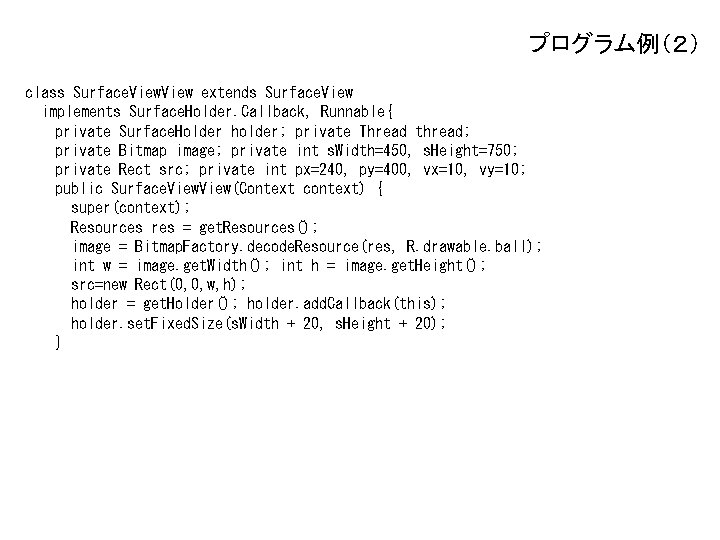
プログラム例(2) class Surface. View extends Surface. View implements Surface. Holder. Callback, Runnable{ private Surface. Holder holder; private Thread thread; private Bitmap image; private int s. Width=450, s. Height=750; private Rect src; private int px=240, py=400, vx=10, vy=10; public Surface. View(Context context) { super(context); Resources res = get. Resources(); image = Bitmap. Factory. decode. Resource(res, R. drawable. ball); int w = image. get. Width(); int h = image. get. Height(); src=new Rect(0, 0, w, h); holder = get. Holder(); holder. add. Callback(this); holder. set. Fixed. Size(s. Width + 20, s. Height + 20); }
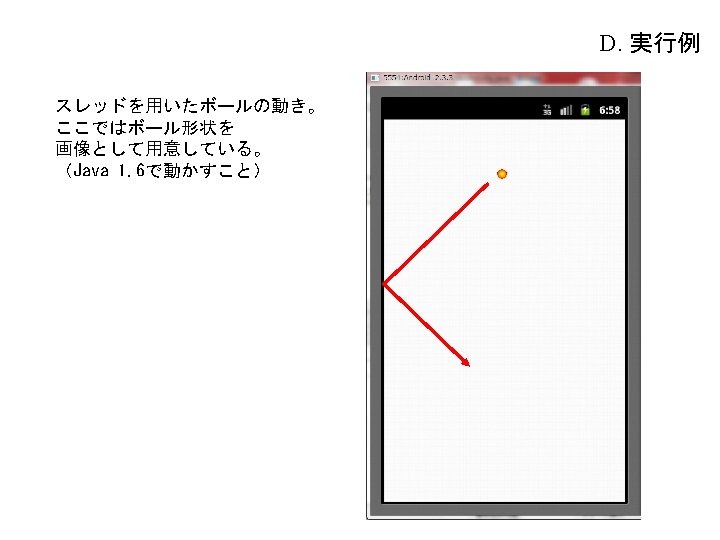
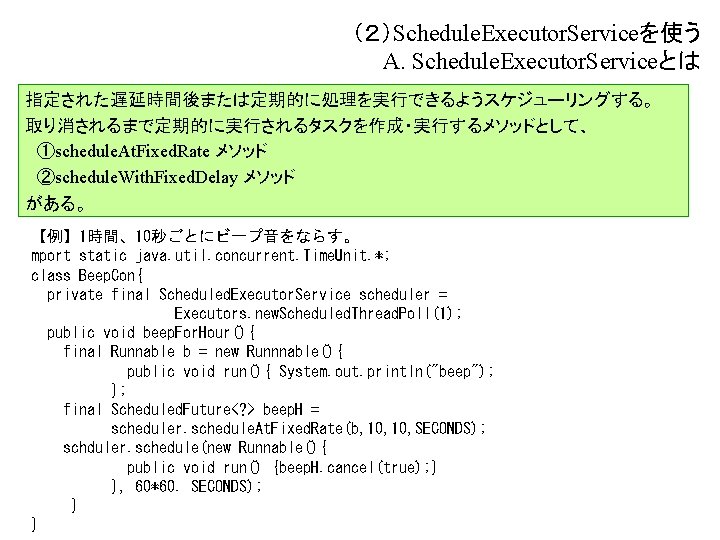
(2)Schedule. Executor. Serviceを使う A. Schedule. Executor. Serviceとは 指定された遅延時間後または定期的に処理を実行できるようスケジューリングする。 取り消されるまで定期的に実行されるタスクを作成・実行するメソッドとして、 ①schedule. At. Fixed. Rate メソッド ②schedule. With. Fixed. Delay メソッド がある。 【例】 1時間、10秒ごとにビープ音をならす。 mport static java. util. concurrent. Time. Unit. *; class Beep. Con{ private final Scheduled. Executor. Service scheduler = Executors. new. Scheduled. Thread. Poll(1); public void beep. For. Hour(){ final Runnable b = new Runnnable(){ public void run(){ System. out. println("beep"); }; final Scheduled. Future<? > beep. H = scheduler. schedule. At. Fixed. Rate(b, 10, SECONDS); schduler. schedule(new Runnable(){ public void run() {beep. H. cancel(true); } }, 60*60. SECONDS); } }
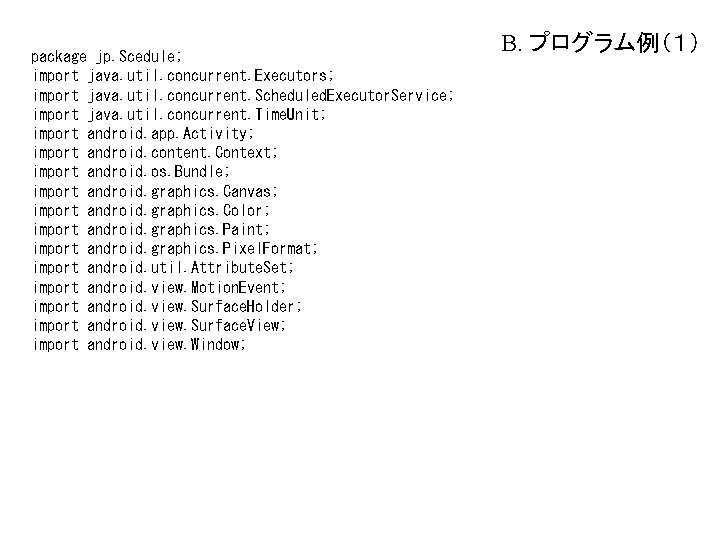
package jp. Scedule; import java. util. concurrent. Executors; import java. util. concurrent. Scheduled. Executor. Service; import java. util. concurrent. Time. Unit; import android. app. Activity; import android. content. Context; import android. os. Bundle; import android. graphics. Canvas; import android. graphics. Color; import android. graphics. Paint; import android. graphics. Pixel. Format; import android. util. Attribute. Set; import android. view. Motion. Event; import android. view. Surface. Holder; import android. view. Surface. View; import android. view. Window; B. プログラム例(1)
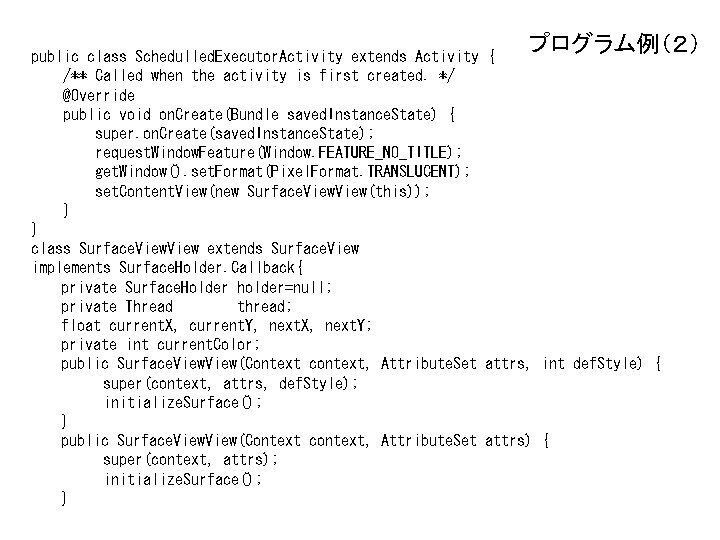
プログラム例(2) public class Schedulled. Executor. Activity extends Activity { /** Called when the activity is first created. */ @Override public void on. Create(Bundle saved. Instance. State) { super. on. Create(saved. Instance. State); request. Window. Feature(Window. FEATURE_NO_TITLE); get. Window(). set. Format(Pixel. Format. TRANSLUCENT); set. Content. View(new Surface. View(this)); } } class Surface. View extends Surface. View implements Surface. Holder. Callback{ private Surface. Holder holder=null; private Thread thread; float current. X, current. Y, next. X, next. Y; private int current. Color; public Surface. View(Context context, Attribute. Set attrs, int def. Style) { super(context, attrs, def. Style); initialize. Surface(); } public Surface. View(Context context, Attribute. Set attrs) { super(context, attrs); initialize. Surface(); }
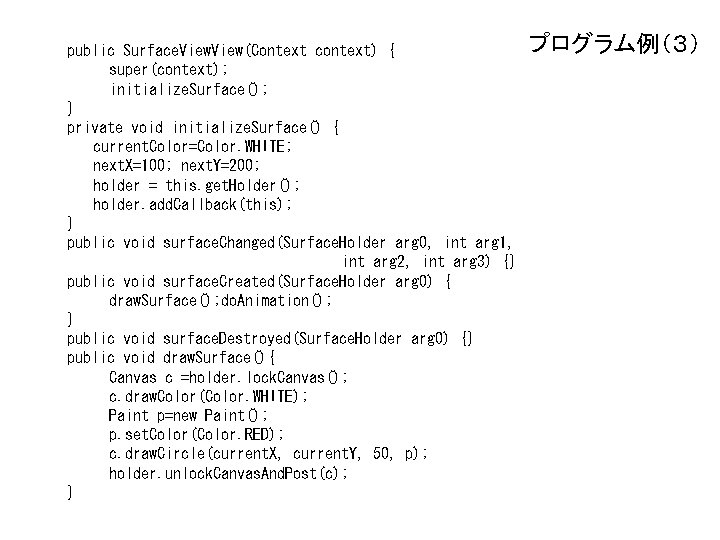
public Surface. View(Context context) { super(context); initialize. Surface(); } private void initialize. Surface() { current. Color=Color. WHITE; next. X=100; next. Y=200; holder = this. get. Holder(); holder. add. Callback(this); } public void surface. Changed(Surface. Holder arg 0, int arg 1, int arg 2, int arg 3) {} public void surface. Created(Surface. Holder arg 0) { draw. Surface(); do. Animation(); } public void surface. Destroyed(Surface. Holder arg 0) {} public void draw. Surface(){ Canvas c =holder. lock. Canvas(); c. draw. Color(Color. WHITE); Paint p=new Paint(); p. set. Color(Color. RED); c. draw. Circle(current. X, current. Y, 50, p); holder. unlock. Canvas. And. Post(c); } プログラム例(3)
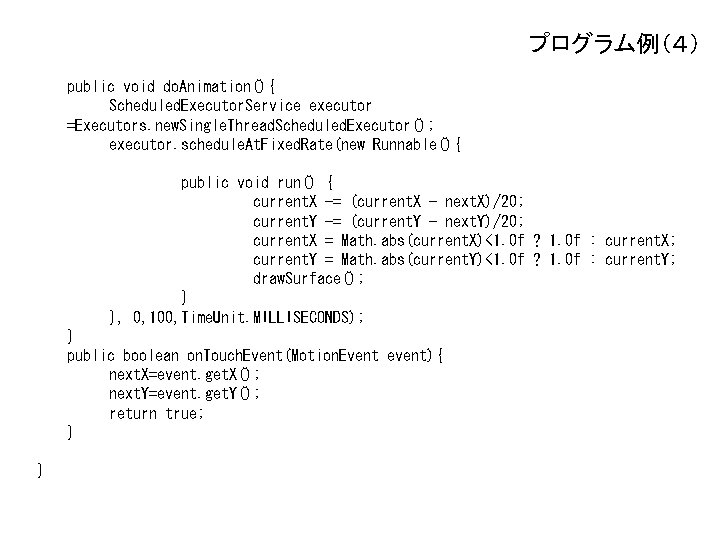
プログラム例(4) public void do. Animation(){ Scheduled. Executor. Service executor =Executors. new. Single. Thread. Scheduled. Executor(); executor. schedule. At. Fixed. Rate(new Runnable(){ public void run() { current. X -= (current. X - next. X)/20; current. Y -= (current. Y - next. Y)/20; current. X = Math. abs(current. X)<1. 0 f ? 1. 0 f : current. X; current. Y = Math. abs(current. Y)<1. 0 f ? 1. 0 f : current. Y; draw. Surface(); } }, 0, 100, Time. Unit. MILLISECONDS); } public boolean on. Touch. Event(Motion. Event event){ next. X=event. get. X(); next. Y=event. get. Y(); return true; } }
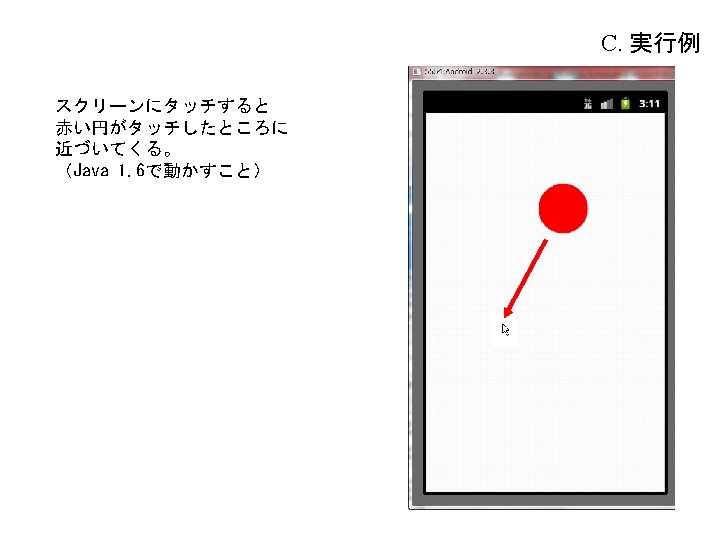
Differentiate between lunar eclipse and solar eclipse
Exo solar lunar
Unity import package characters
Eclipse android programlama
Import java.awt.* import java.applet.*
Import java.io.*
Import numpy as np import matplotlib.pyplot as plt
Import java.awt.event
Ip telephony system architecture
App monetization challenges
Coffee ordering app in android studio
Appmit