Outline Generic classes Generics Inheritance Wild Cards characters
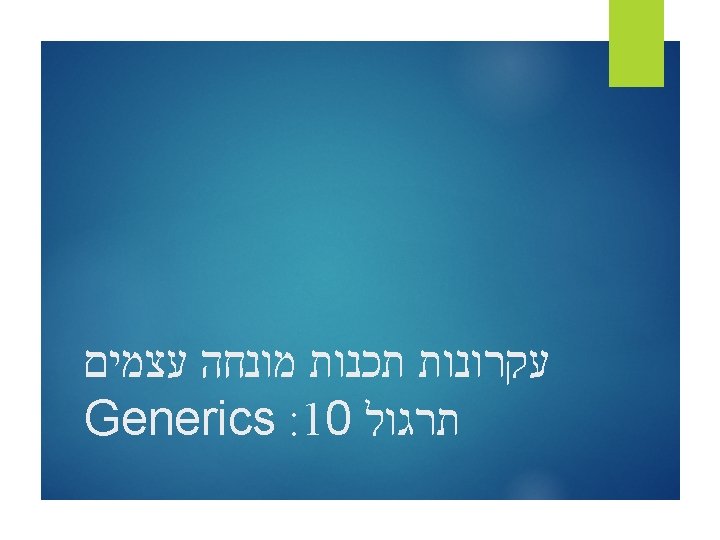
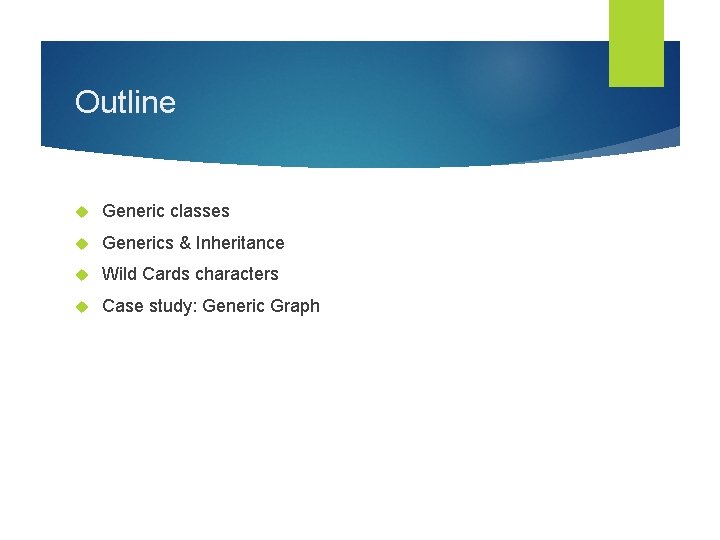
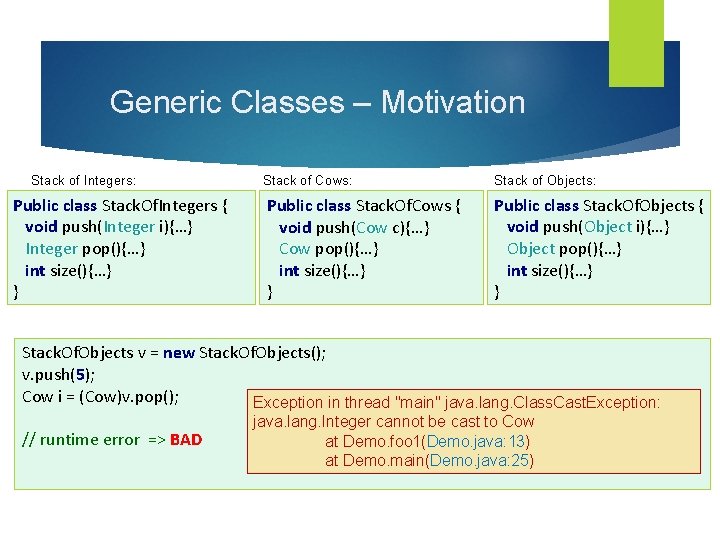
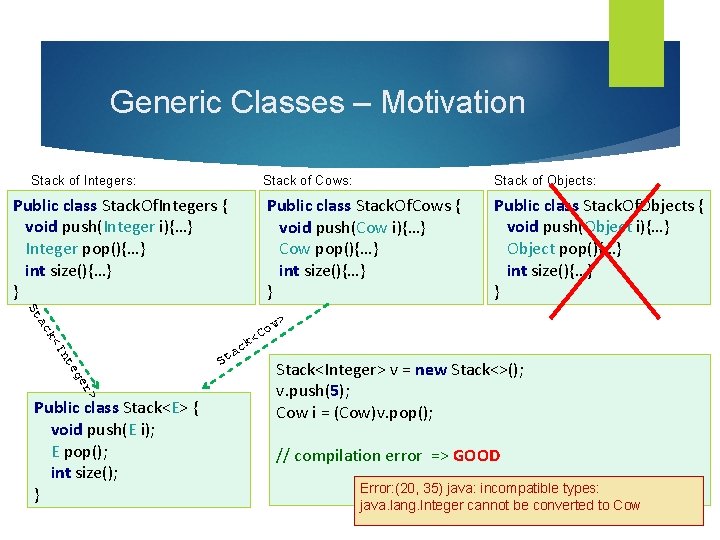
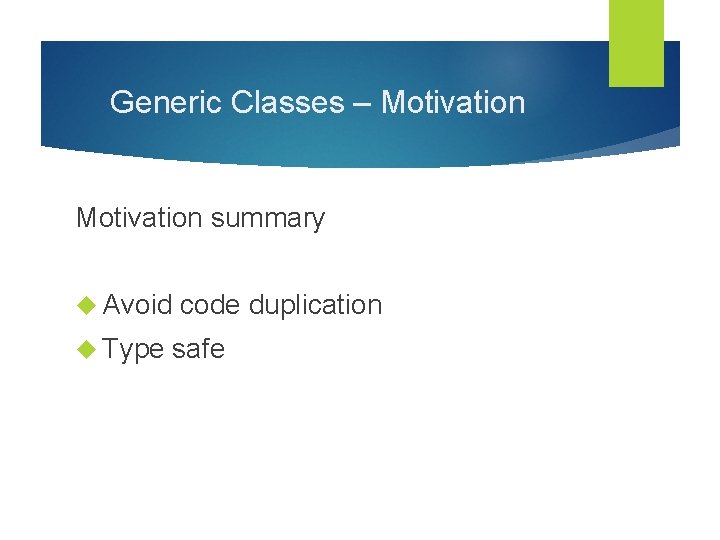
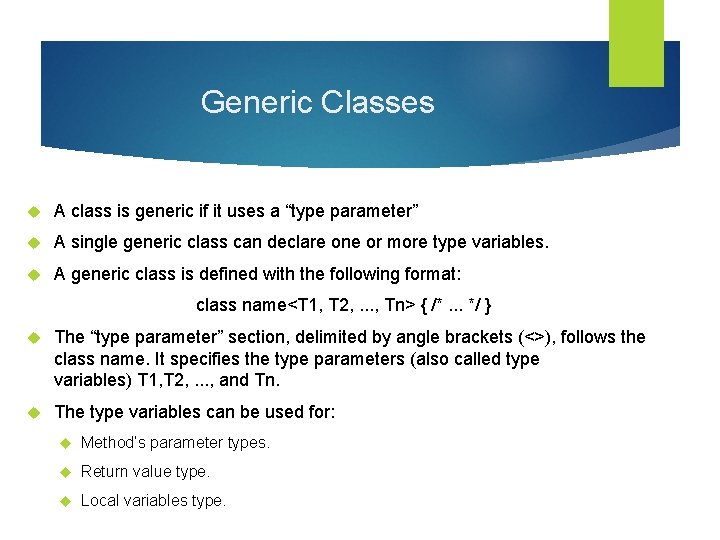
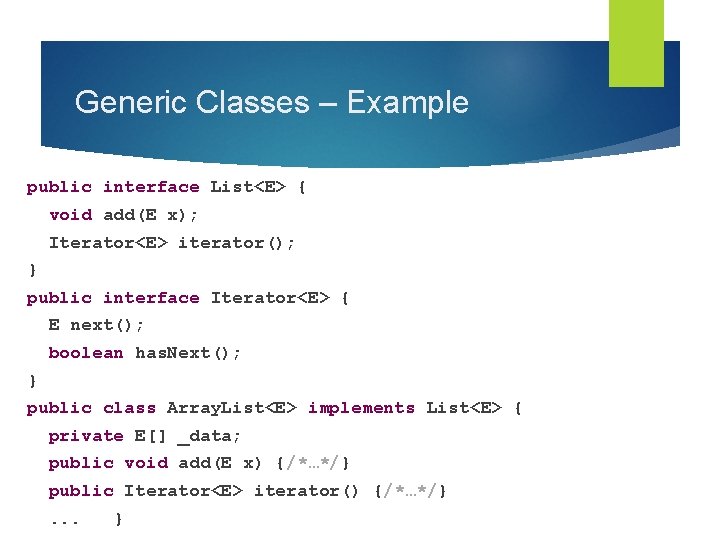
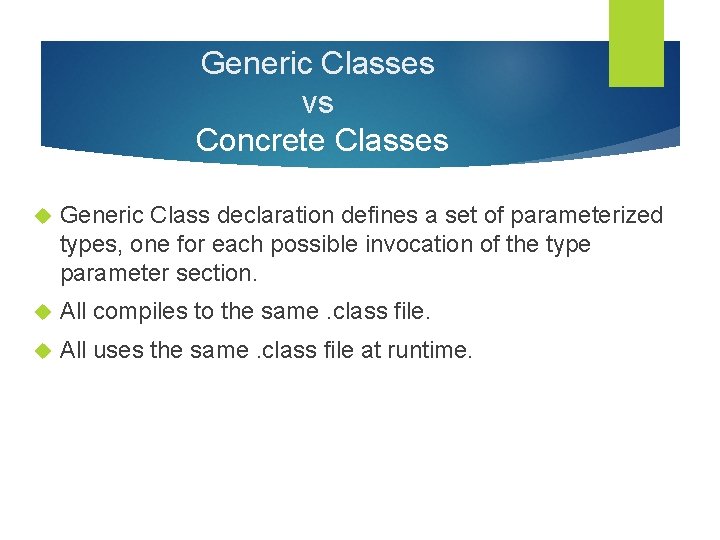
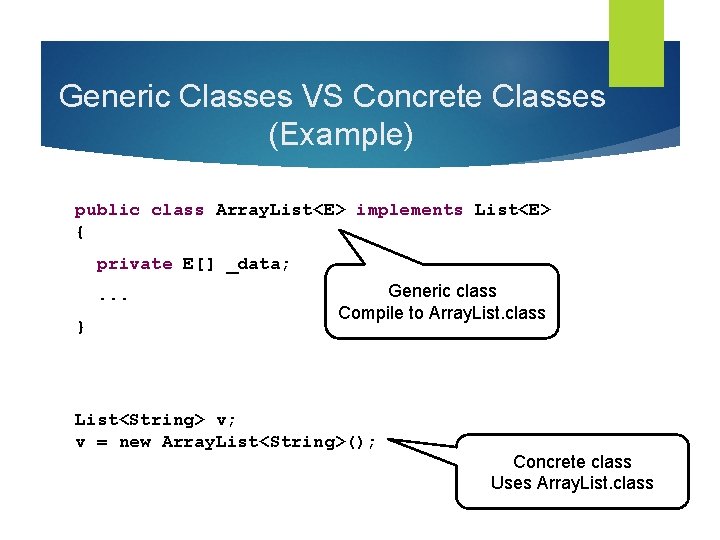
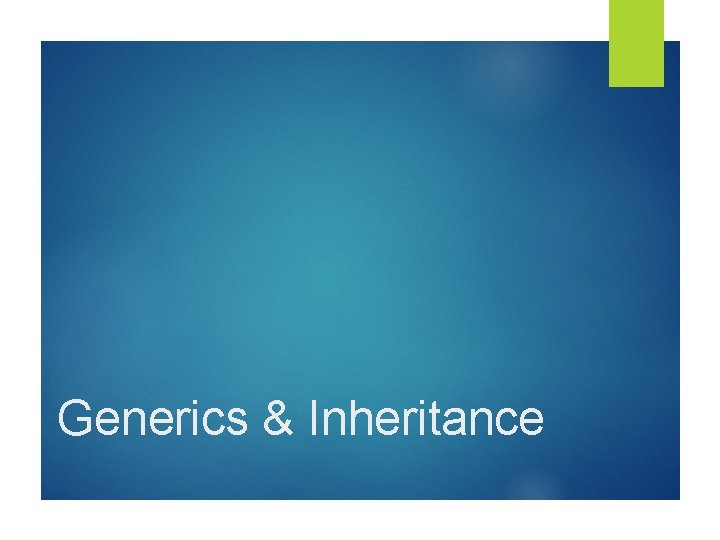
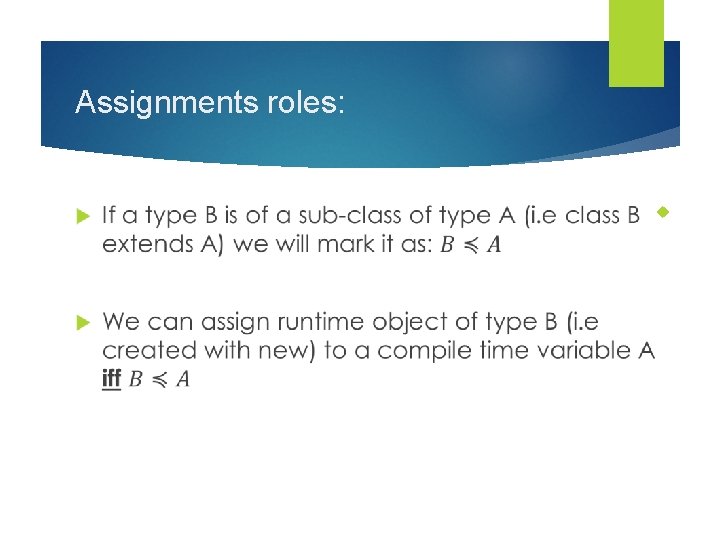
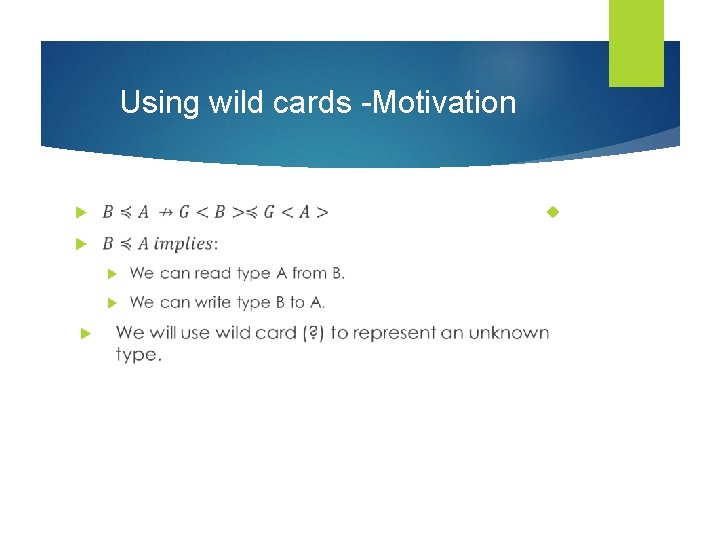
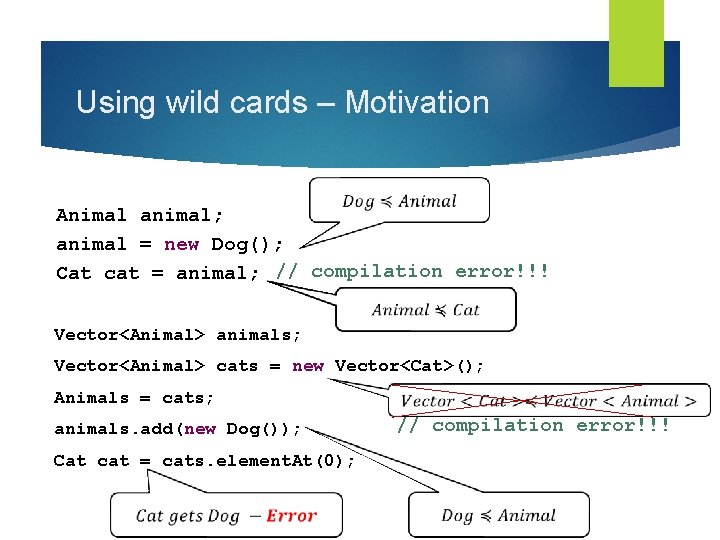
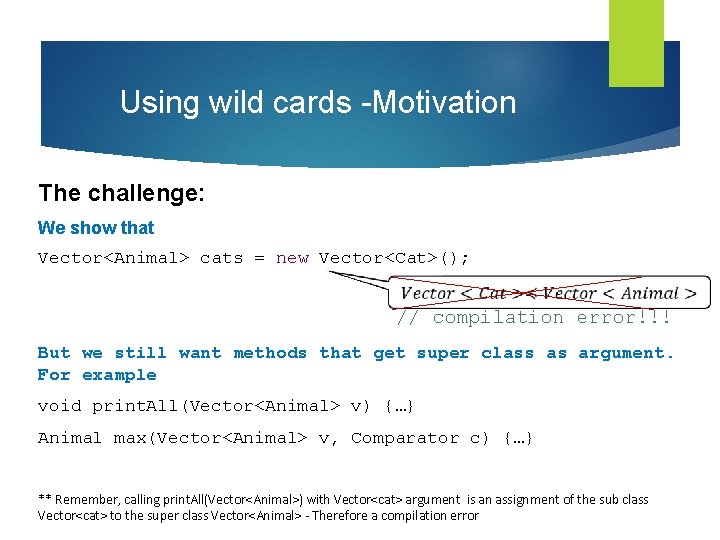
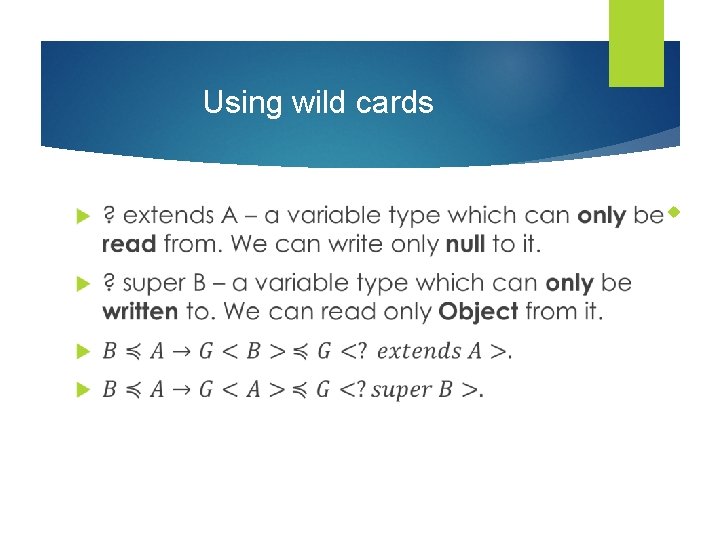
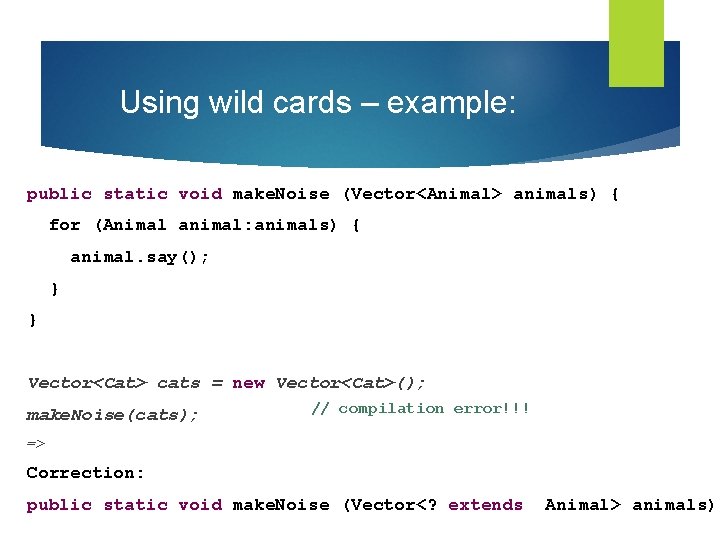
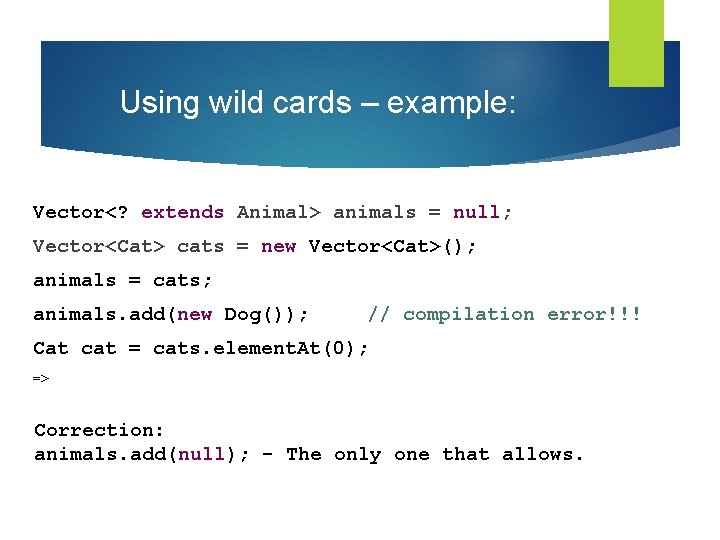
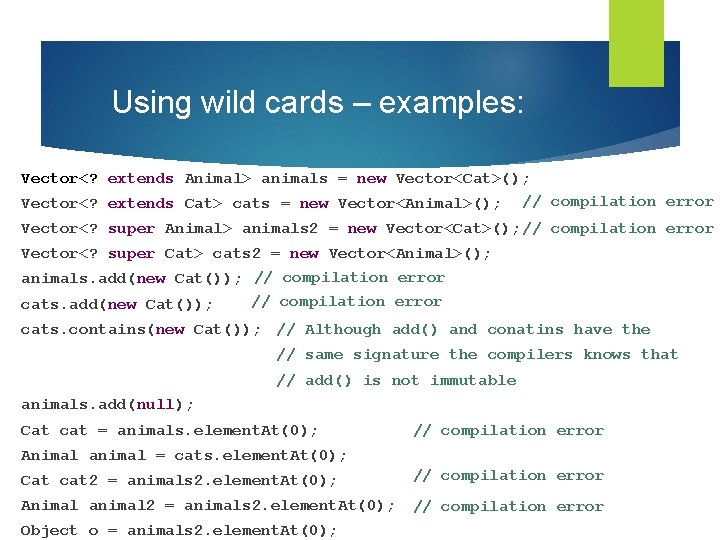
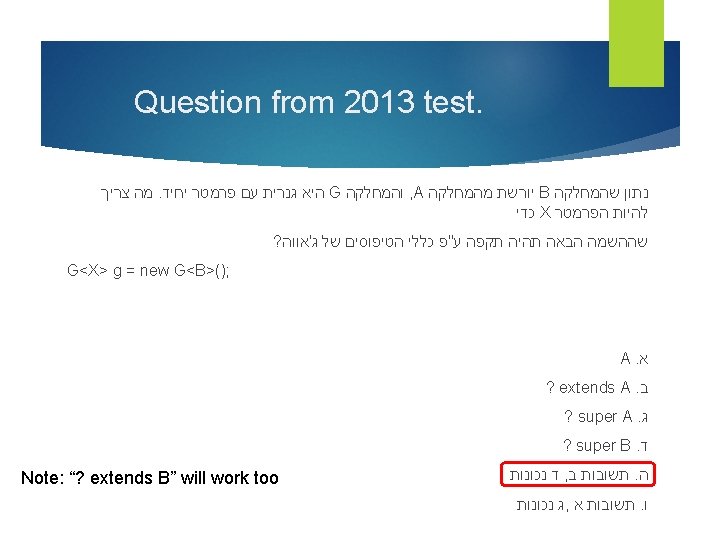
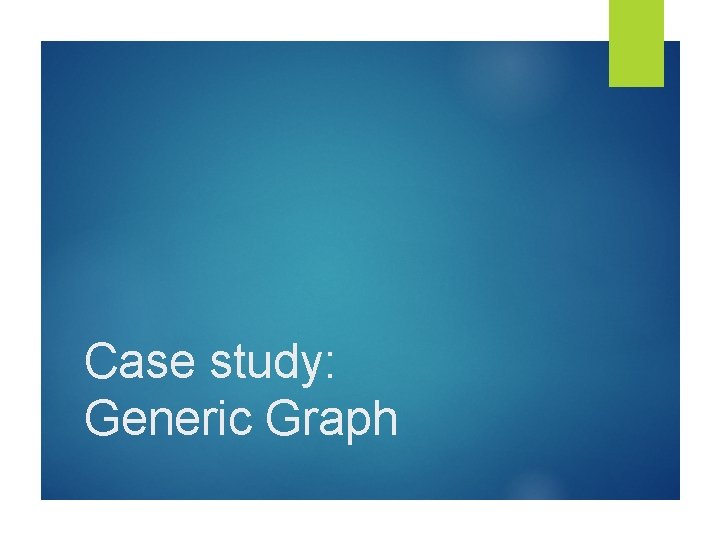
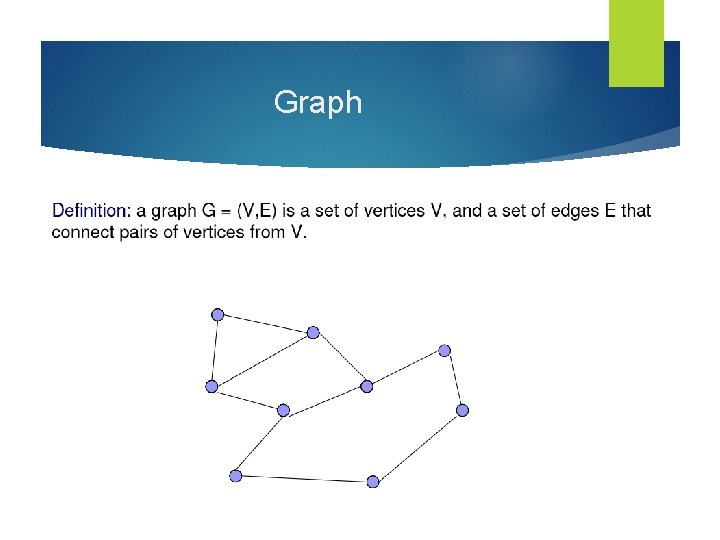
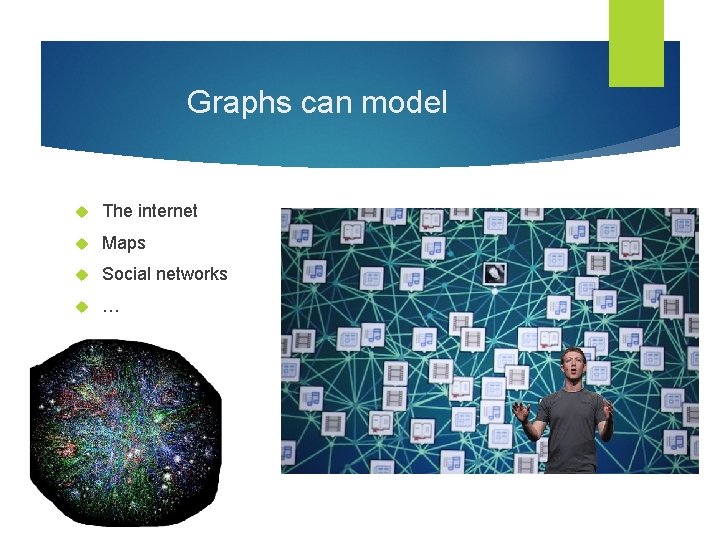
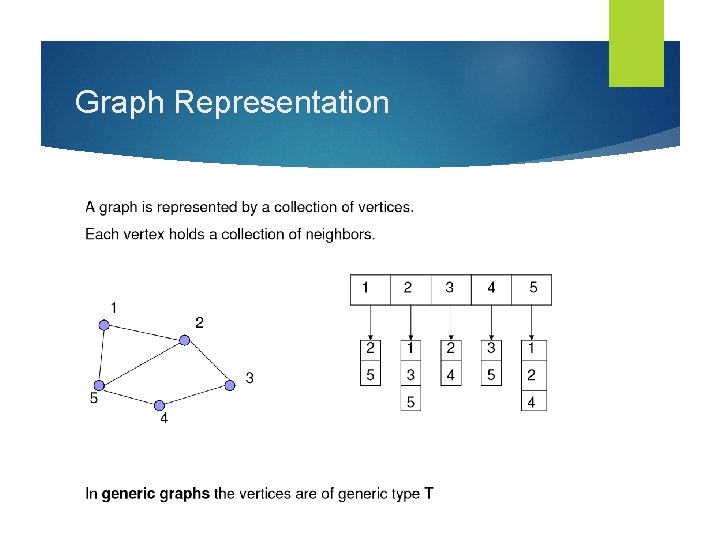
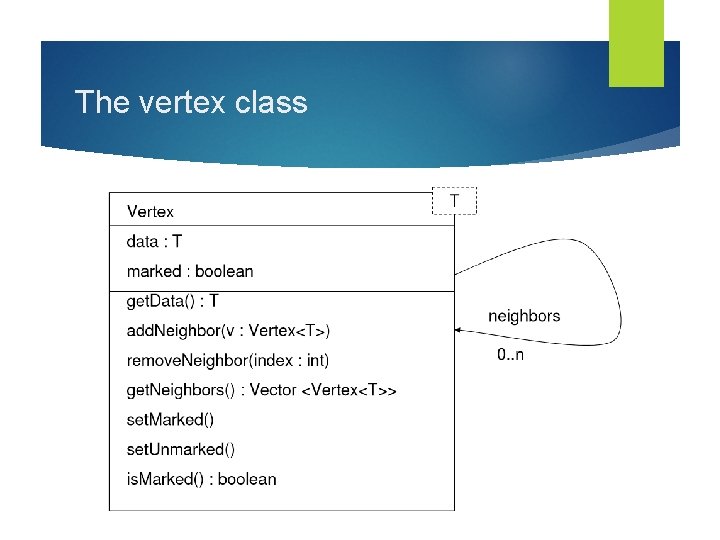
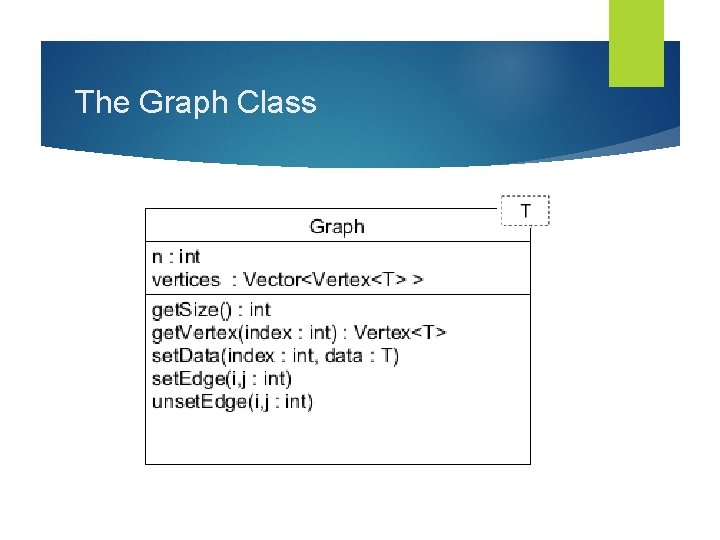
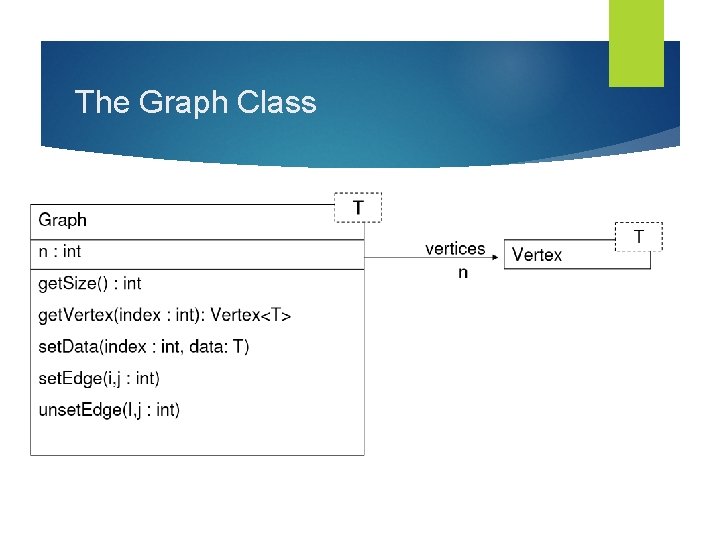
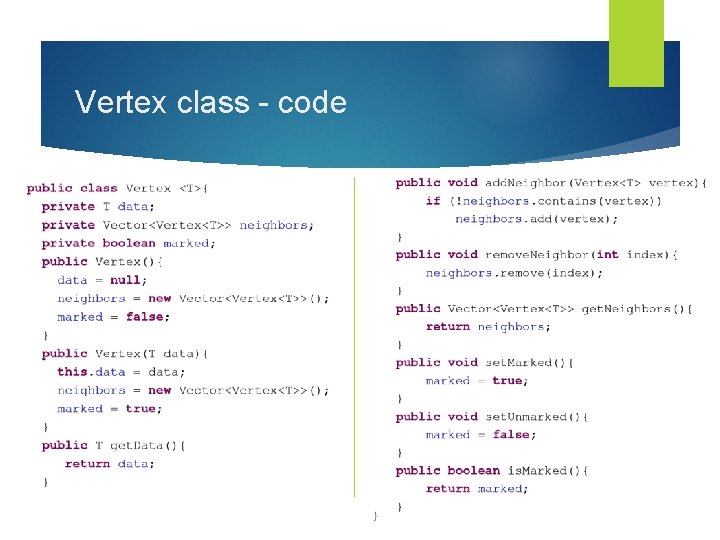
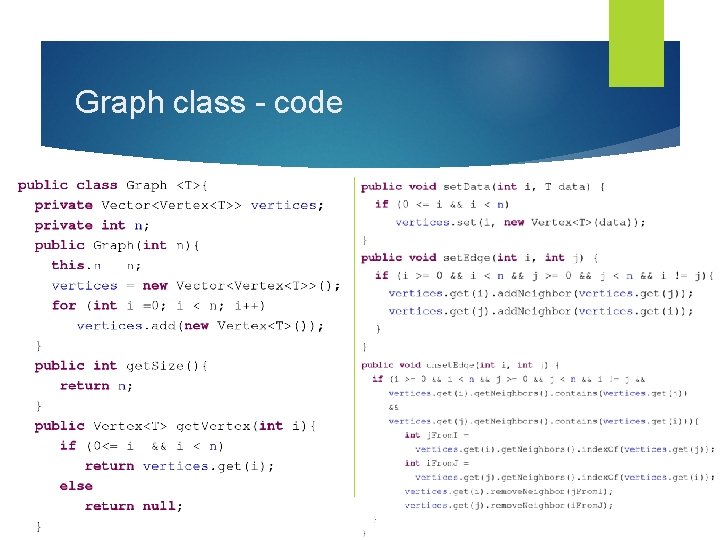
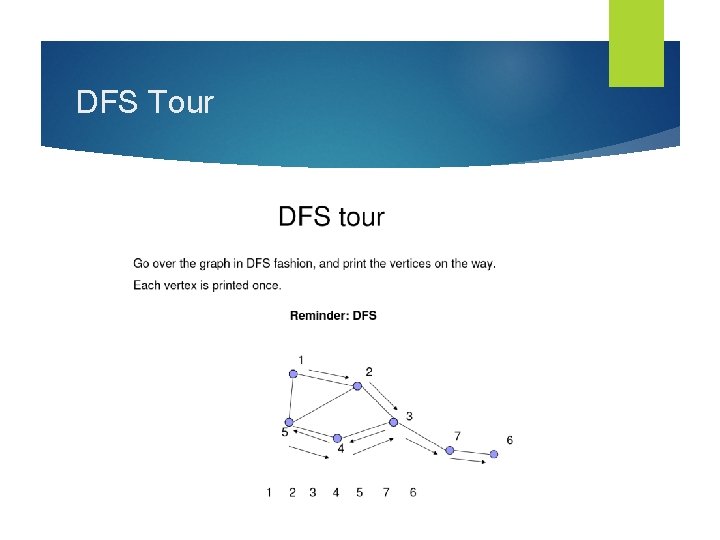
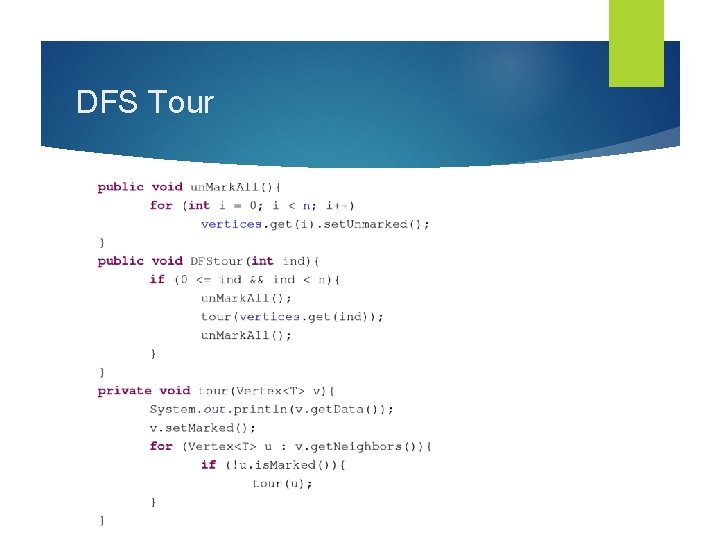
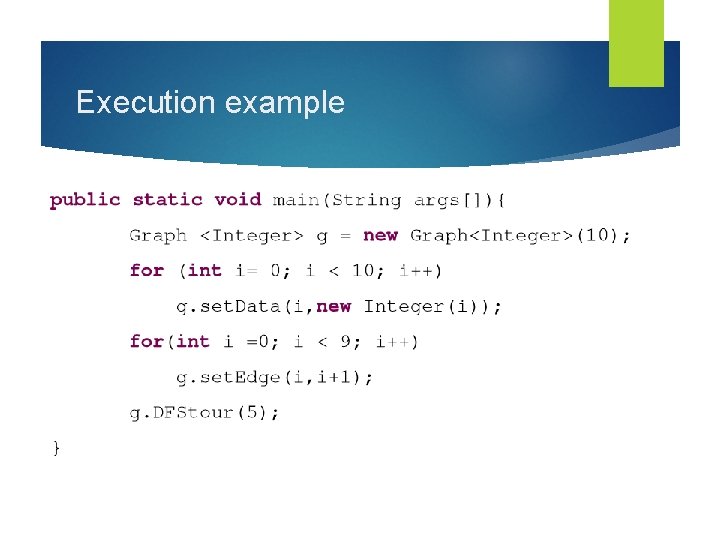
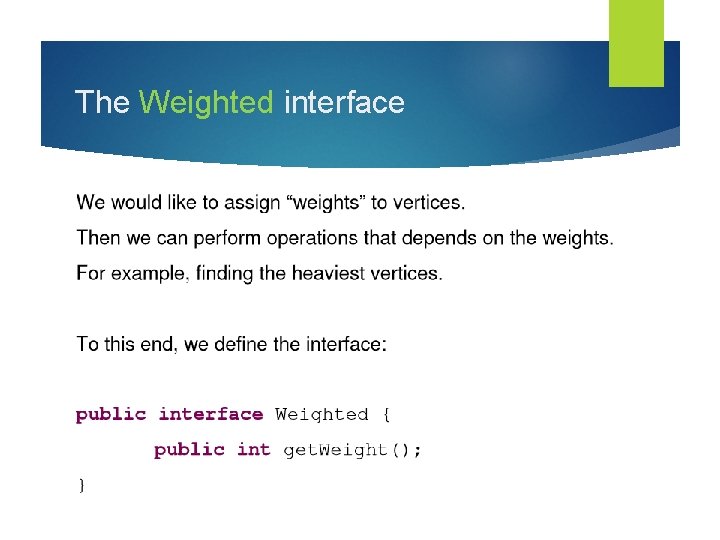
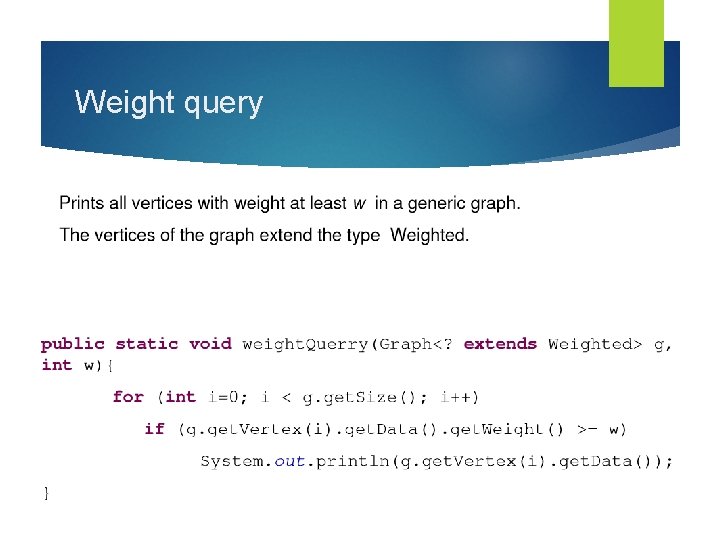
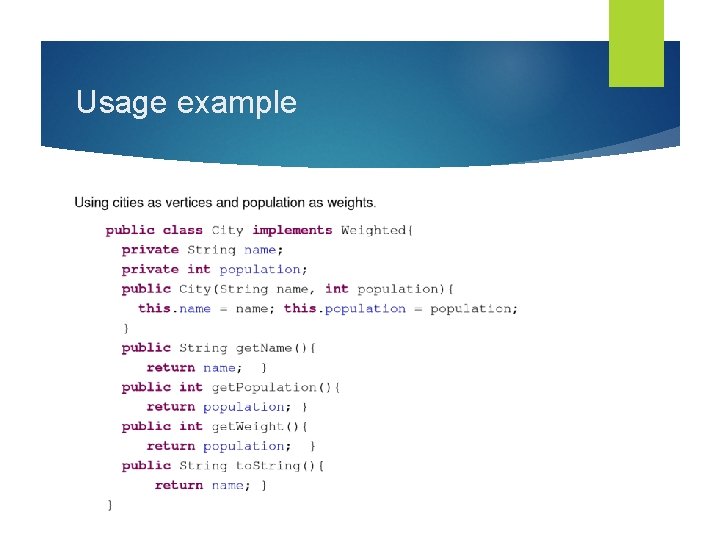
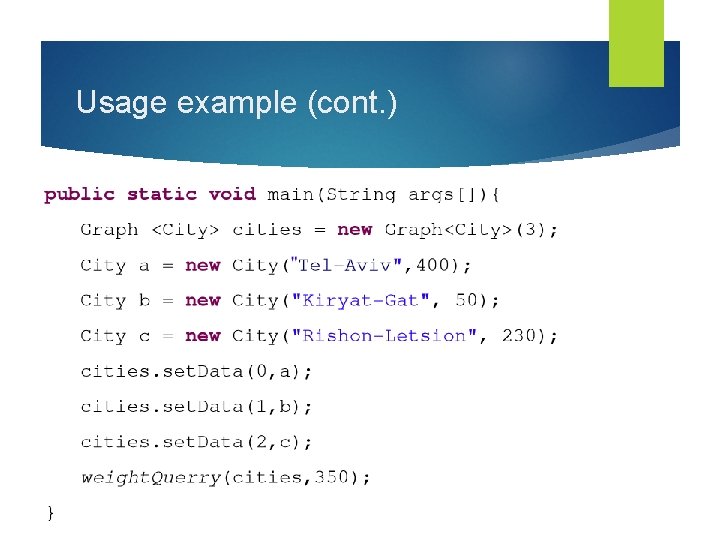
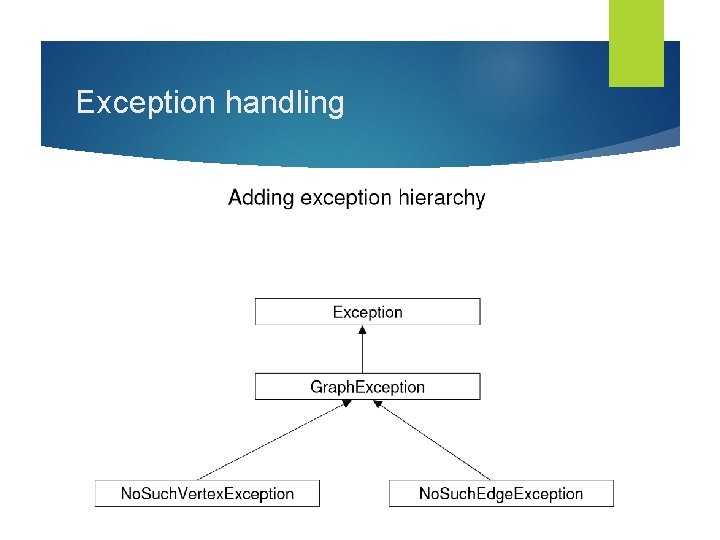
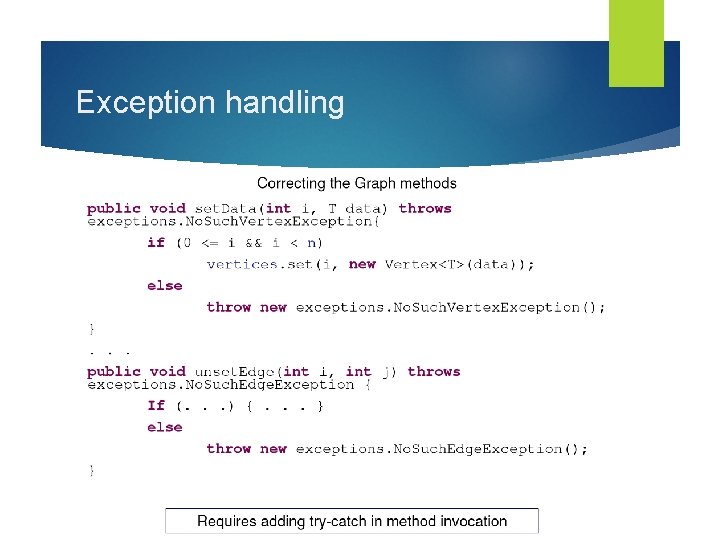
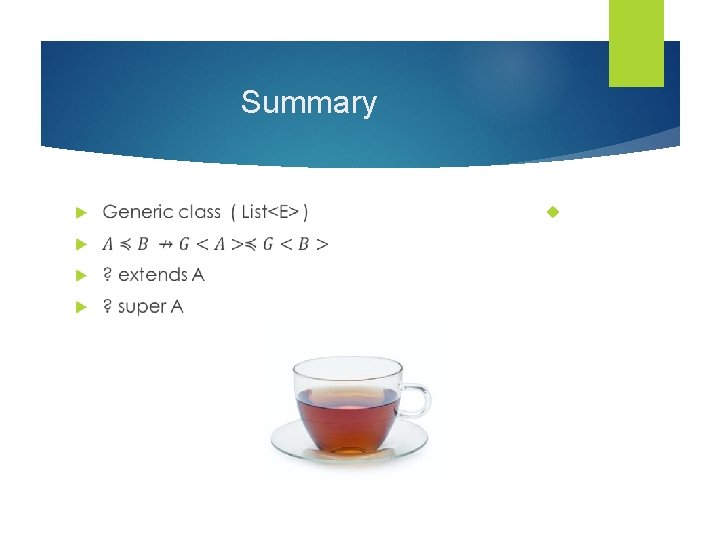
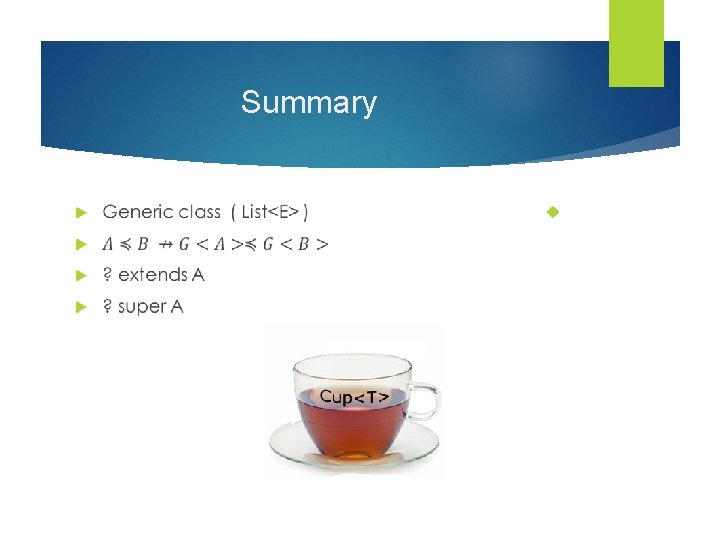
- Slides: 39
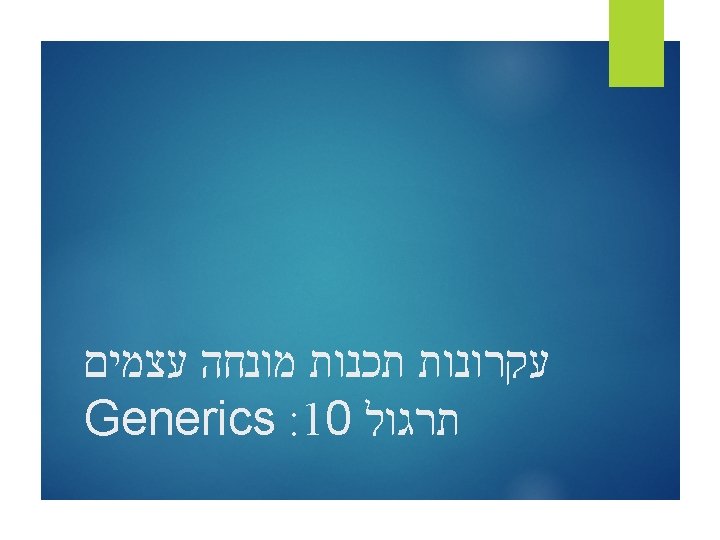
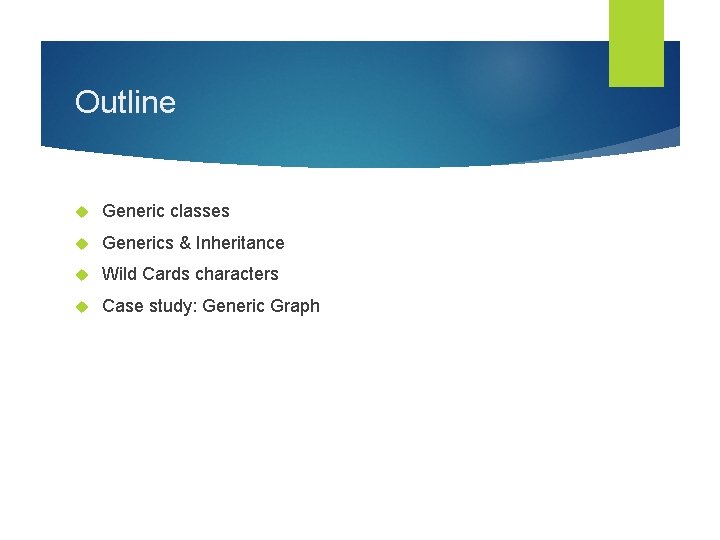
Outline Generic classes Generics & Inheritance Wild Cards characters Case study: Generic Graph
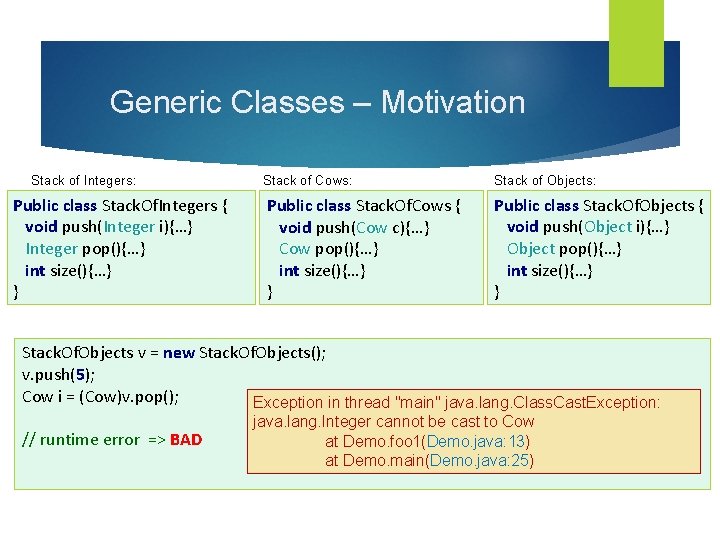
Generic Classes – Motivation Stack of Integers: Public class Stack. Of. Integers { void push(Integer i){…} Integer pop(){…} int size(){…} } Stack of Cows: Stack of Objects: Public class Stack. Of. Cows { void push(Cow c){…} Cow pop(){…} int size(){…} } Public class Stack. Of. Objects { void push(Object i){…} Object pop(){…} int size(){…} } Stack. Of. Objects v = new Stack. Of. Objects(); v. push(5); Cow i = (Cow)v. pop(); Exception in thread "main" java. lang. Class. Cast. Exception: // runtime error => BAD java. lang. Integer cannot be cast to Cow at Demo. foo 1(Demo. java: 13) at Demo. main(Demo. java: 25)
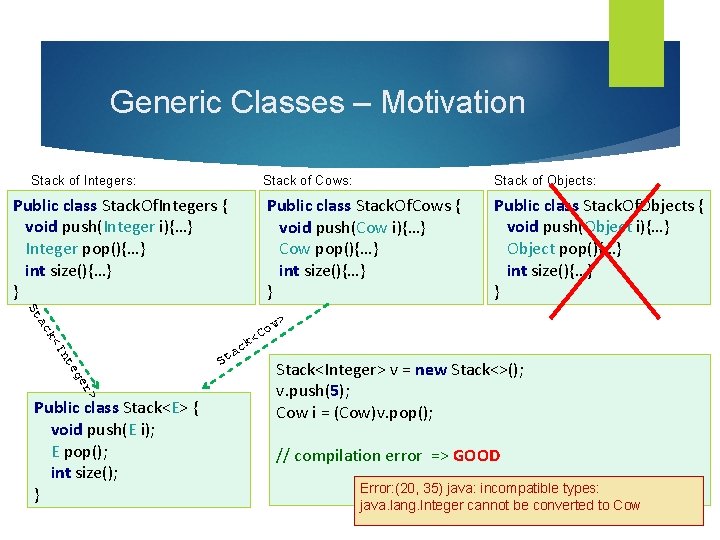
Generic Classes – Motivation Stack of Integers: Public class Stack. Of. Integers { void push(Integer i){…} Integer pop(){…} int size(){…} } St Stack of Objects: Public class Stack. Of. Cows { void push(Cow i){…} Cow pop(){…} int size(){…} } Public class Stack. Of. Objects { void push(Object i){…} Object pop(){…} int size(){…} } > ow C < r> ge te In k< ac Public class Stack<E> { void push(E i); E pop(); int size(); } Stack of Cows: k S c ta Stack<Integer> v = new Stack<>(); v. push(5); Cow i = (Cow)v. pop(); // compilation error => GOOD Error: (20, 35) java: incompatible types: java. lang. Integer cannot be converted to Cow
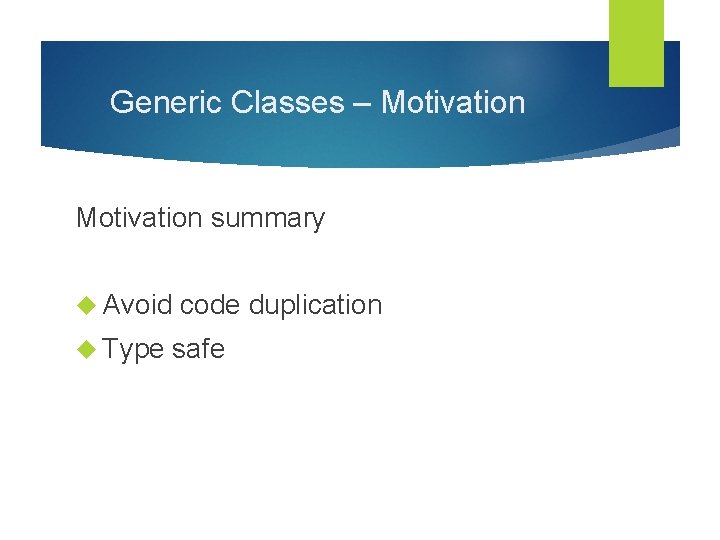
Generic Classes – Motivation summary Avoid Type code duplication safe
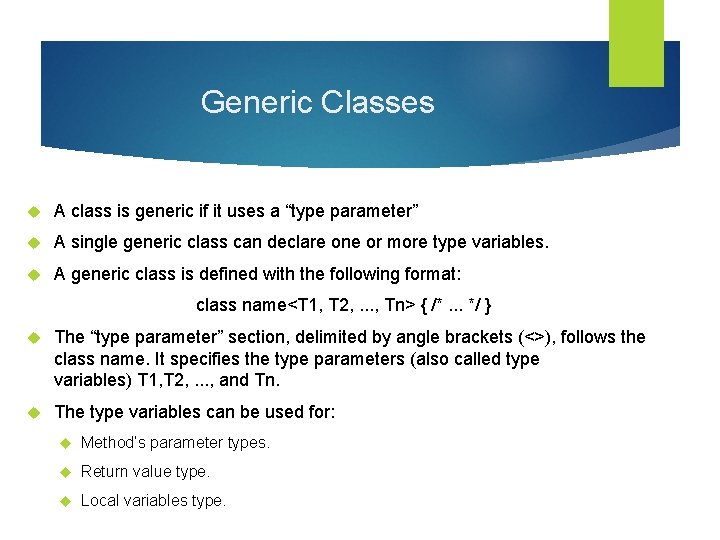
Generic Classes A class is generic if it uses a “type parameter” A single generic class can declare one or more type variables. A generic class is defined with the following format: class name<T 1, T 2, . . . , Tn> { /*. . . */ } The “type parameter” section, delimited by angle brackets (<>), follows the class name. It specifies the type parameters (also called type variables) T 1, T 2, . . . , and Tn. The type variables can be used for: Method’s parameter types. Return value type. Local variables type.
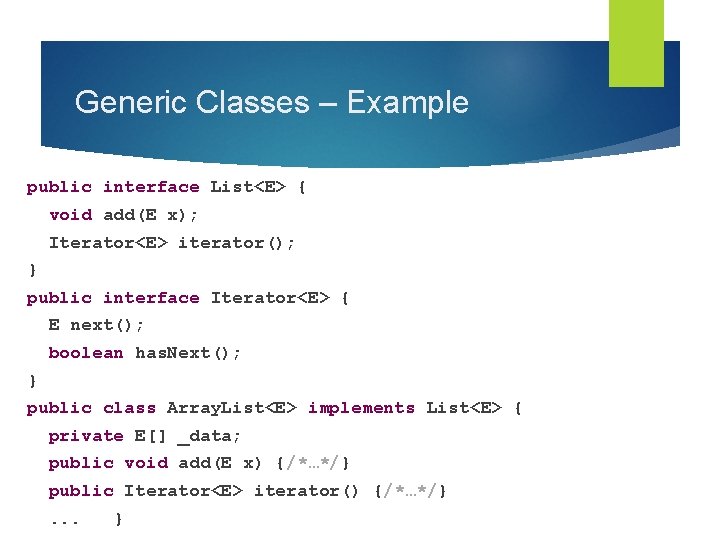
Generic Classes – Example public interface List<E> { void add(E x); Iterator<E> iterator(); } public interface Iterator<E> { E next(); boolean has. Next(); } public class Array. List<E> implements List<E> { private E[] _data; public void add(E x) {/*…*/} public Iterator<E> iterator() {/*…*/}. . . }
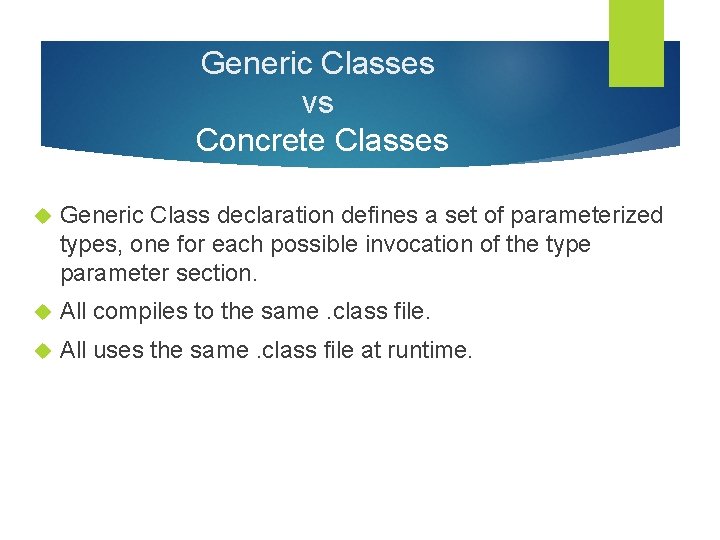
Generic Classes vs Concrete Classes Generic Class declaration defines a set of parameterized types, one for each possible invocation of the type parameter section. All compiles to the same. class file. All uses the same. class file at runtime.
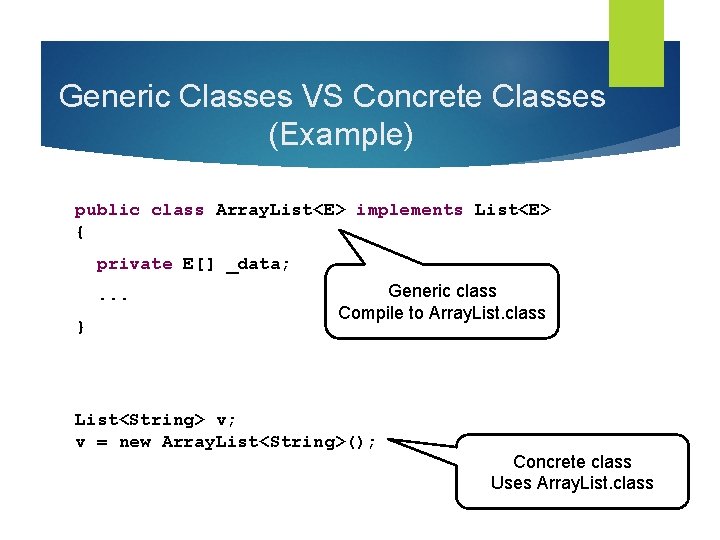
Generic Classes VS Concrete Classes (Example) public class Array. List<E> implements List<E> { private E[] _data; . . . } Generic class Compile to Array. List. class List<String> v; v = new Array. List<String>(); Concrete class Uses Array. List. class
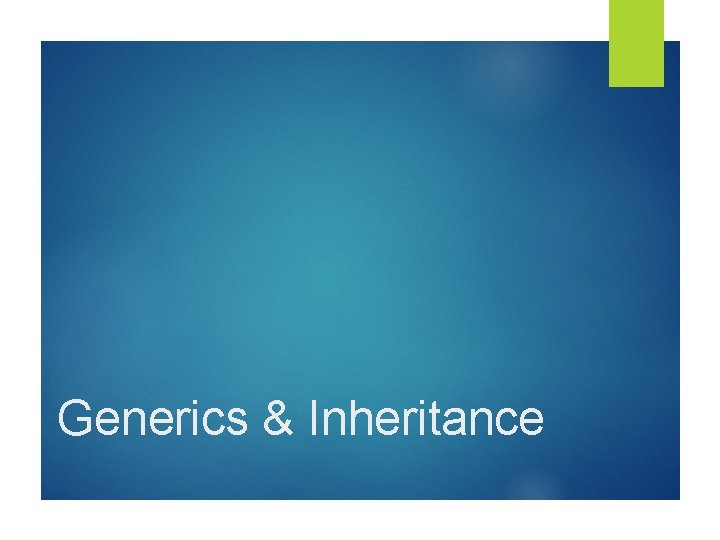
Generics & Inheritance
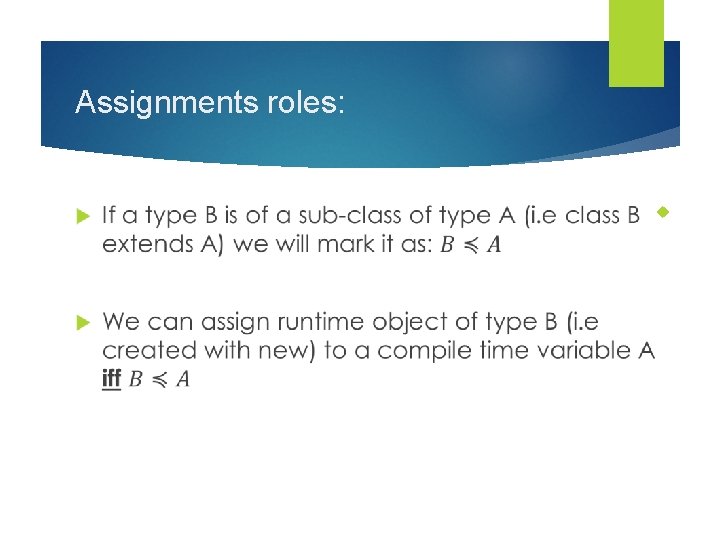
Assignments roles:
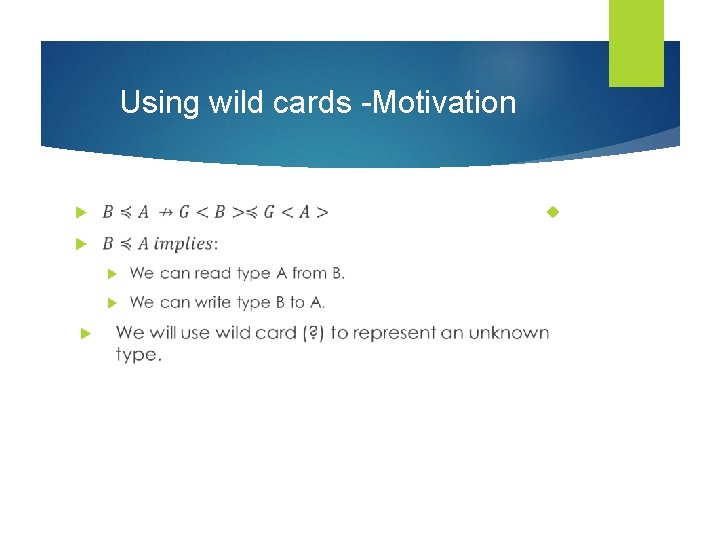
Using wild cards -Motivation
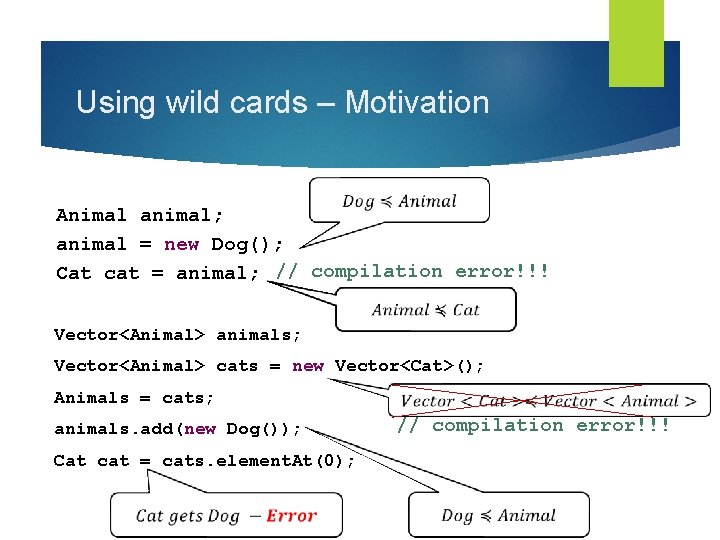
Using wild cards – Motivation Animal animal; animal = new Dog(); Cat cat = animal; // compilation error!!! Vector<Animal> animals; Vector<Animal> cats = new Vector<Cat>(); Animals = cats; animals. add(new Dog()); Cat cat = cats. element. At(0); // compilation error!!!
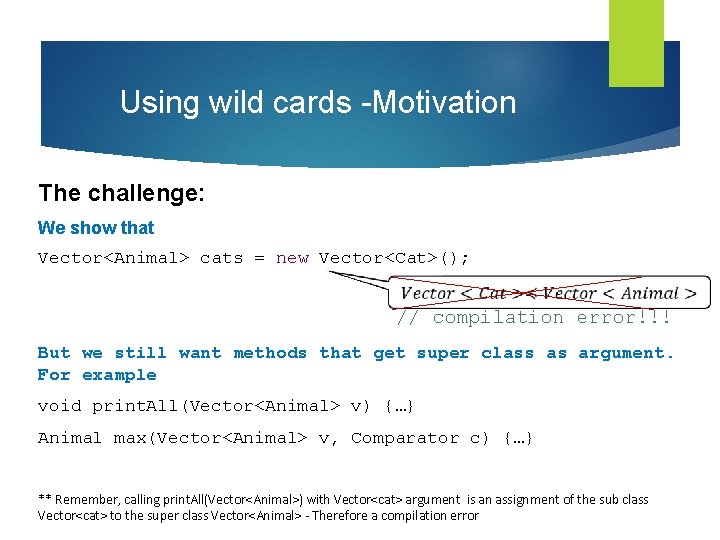
Using wild cards -Motivation The challenge: We show that Vector<Animal> cats = new Vector<Cat>(); // compilation error!!! But we still want methods that get super class as argument. For example void print. All(Vector<Animal> v) {…} Animal max(Vector<Animal> v, Comparator c) {…} ** Remember, calling print. All(Vector<Animal>) with Vector<cat> argument is an assignment of the sub class Vector<cat> to the super class Vector<Animal> - Therefore a compilation error
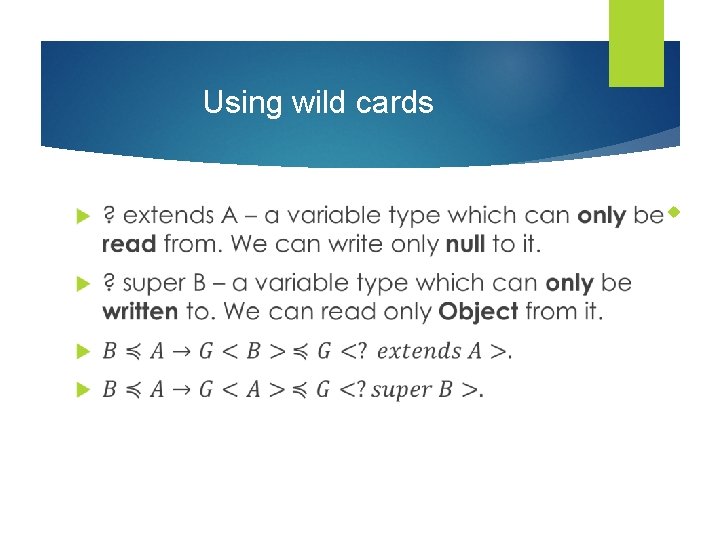
Using wild cards
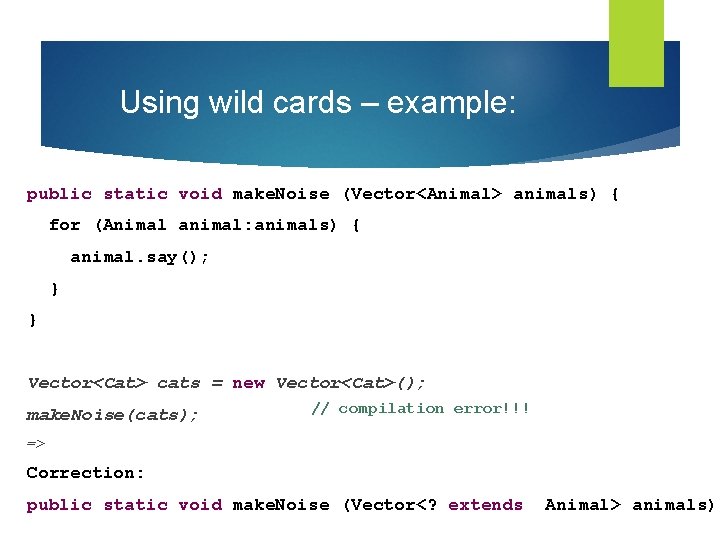
Using wild cards – example: public static void make. Noise (Vector<Animal> animals) { for (Animal animal: animals) { animal. say(); } } Vector<Cat> cats = new Vector<Cat>(); make. Noise(cats); // compilation error!!! => Correction: public static void make. Noise (Vector<? extends Animal> animals)
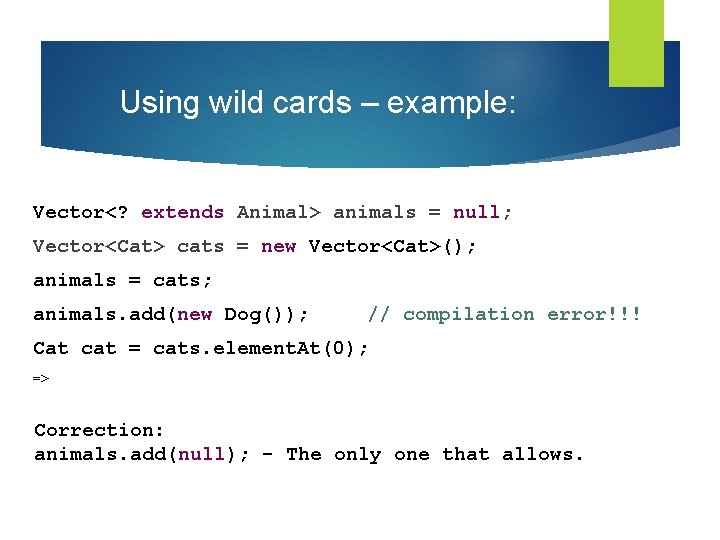
Using wild cards – example: Vector<? extends Animal> animals = null; Vector<Cat> cats = new Vector<Cat>(); animals = cats; animals. add(new Dog()); // compilation error!!! Cat cat = cats. element. At(0); => Correction: animals. add(null); - The only one that allows.
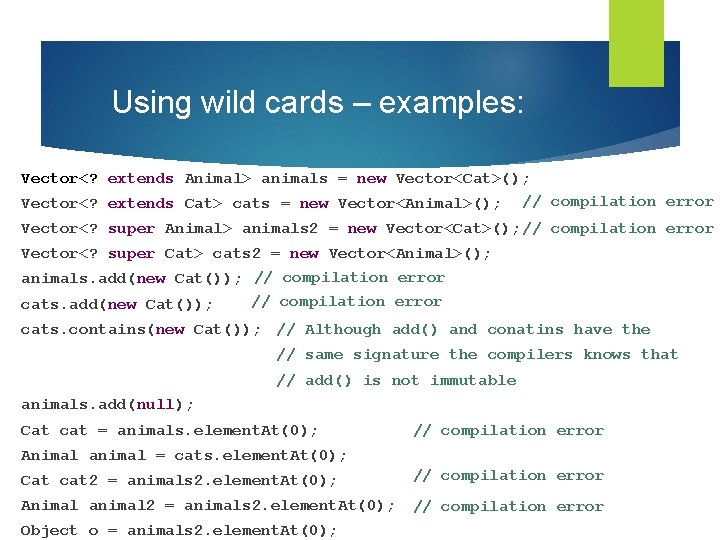
Using wild cards – examples: Vector<? extends Animal> animals = new Vector<Cat>(); Vector<? extends Cat> cats = new Vector<Animal>(); // compilation error Vector<? super Animal> animals 2 = new Vector<Cat>(); // compilation error Vector<? super Cat> cats 2 = new Vector<Animal>(); animals. add(new Cat()); // compilation error cats. add(new Cat()); cats. contains(new Cat()); // Although add() and conatins have the // same signature the compilers knows that // add() is not immutable animals. add(null); Cat cat = animals. element. At(0); // compilation error Animal animal = cats. element. At(0); Cat cat 2 = animals 2. element. At(0); // compilation error Animal animal 2 = animals 2. element. At(0); // compilation error Object o = animals 2. element. At(0);
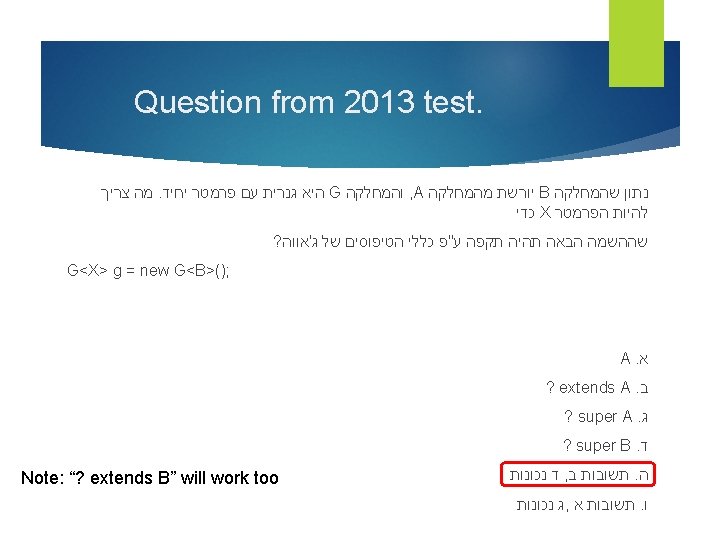
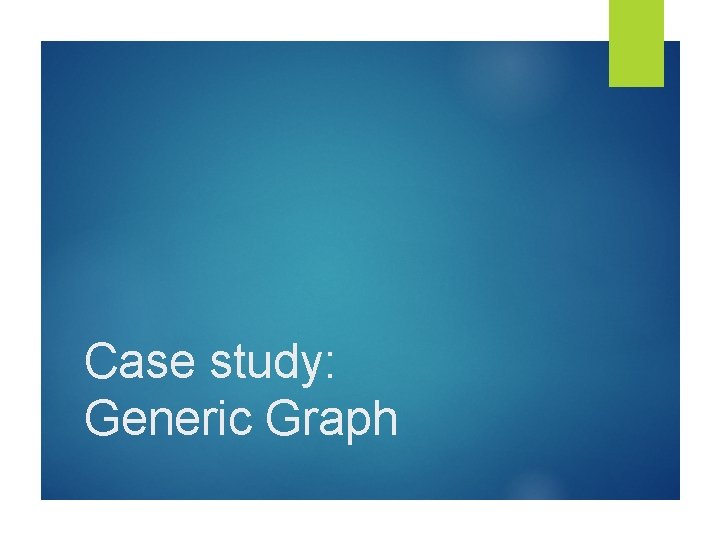
Case study: Generic Graph
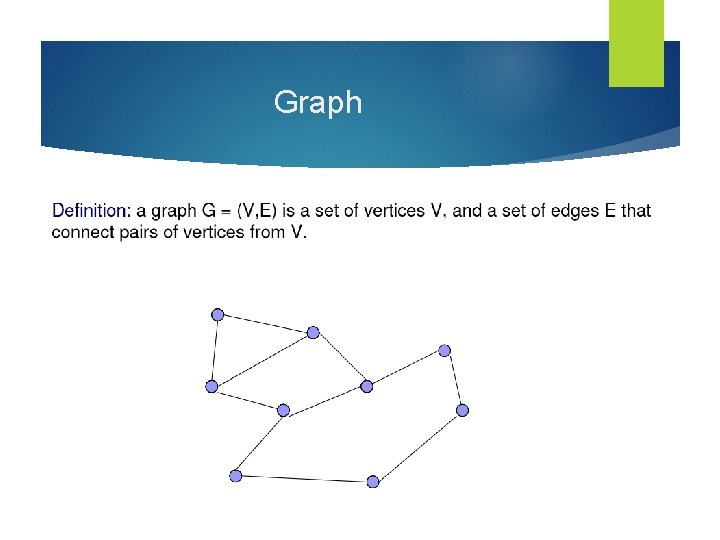
Graph
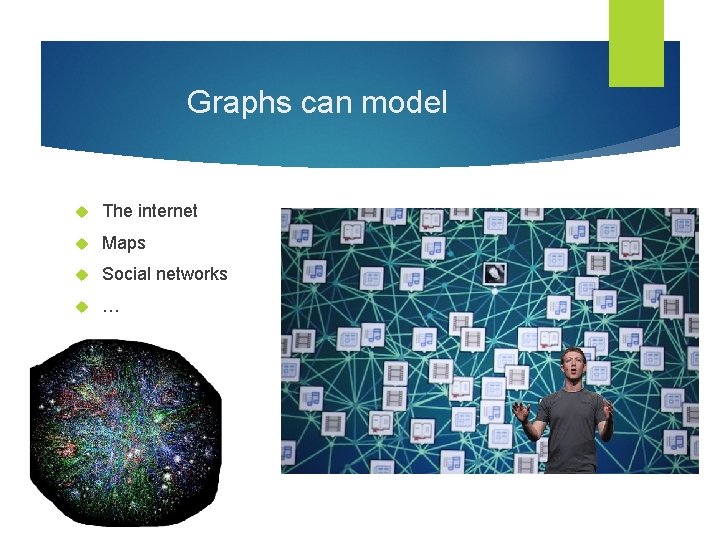
Graphs can model The internet Maps Social networks …
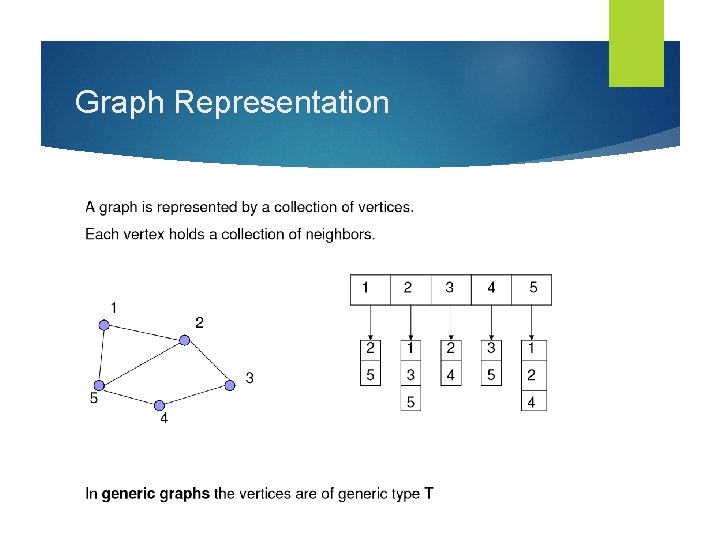
Graph Representation
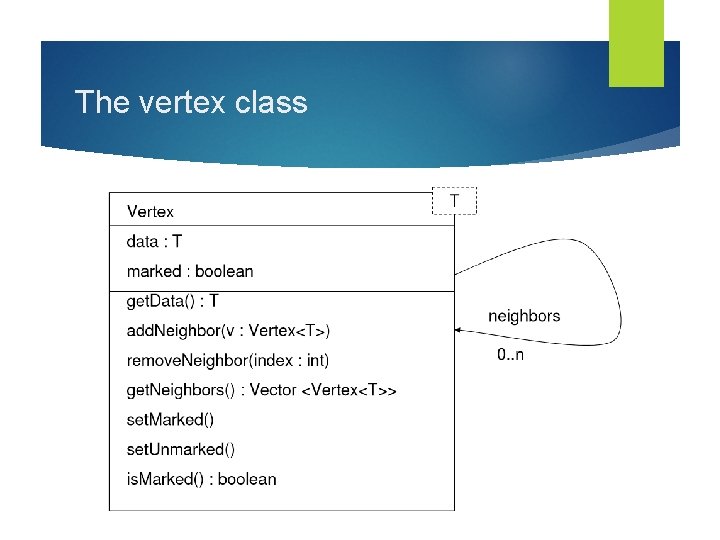
The vertex class
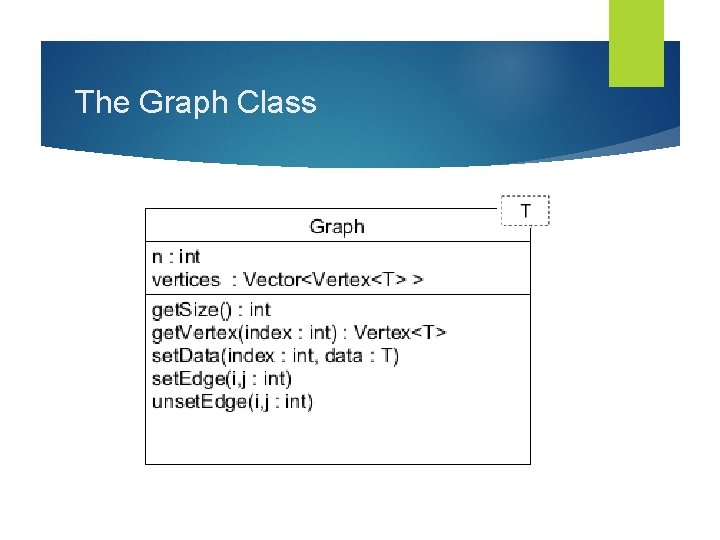
The Graph Class
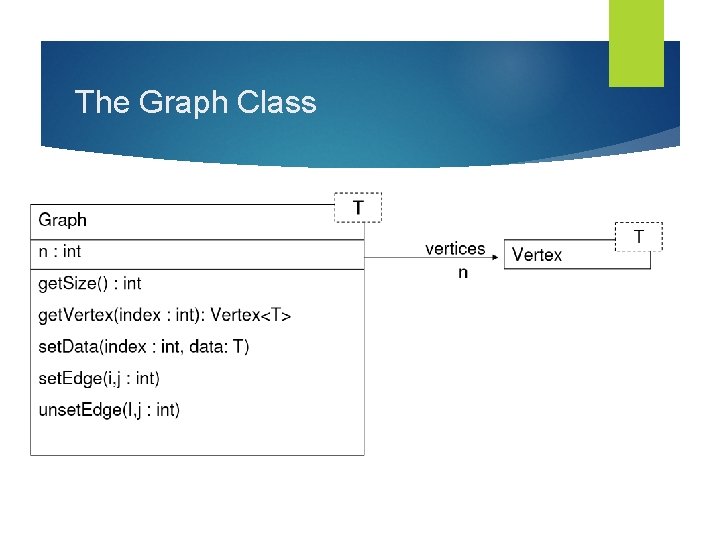
The Graph Class
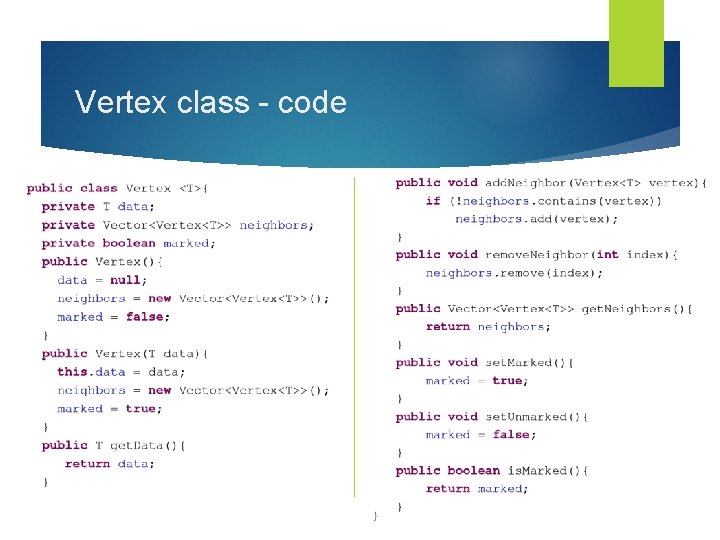
Vertex class - code
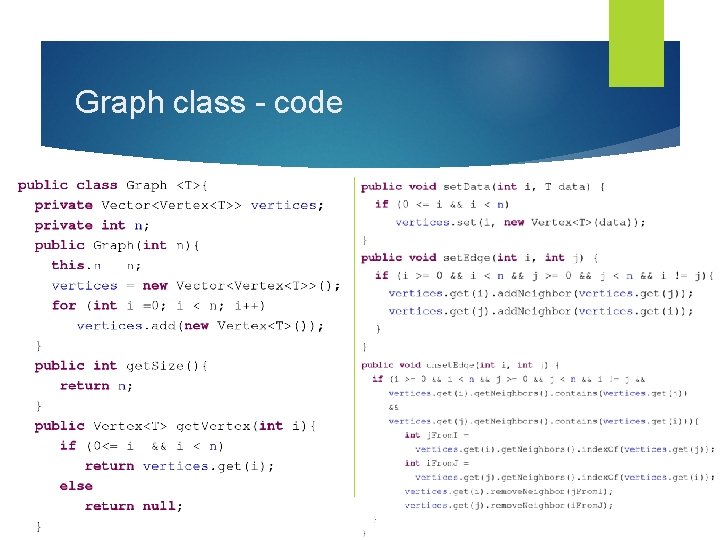
Graph class - code
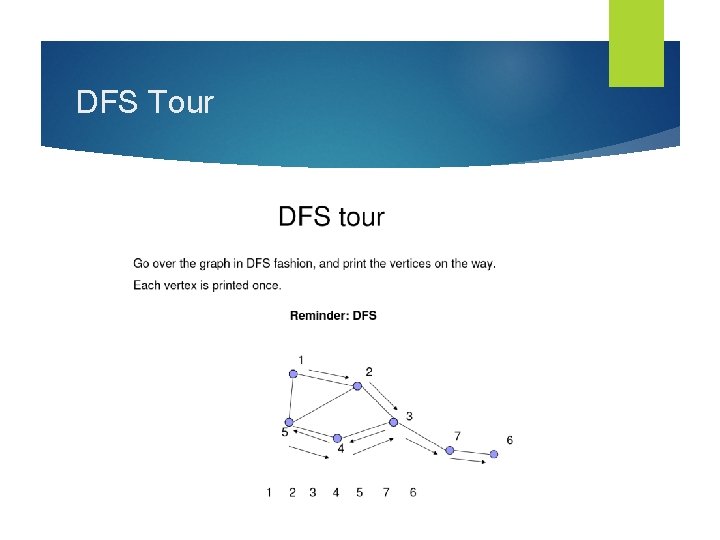
DFS Tour
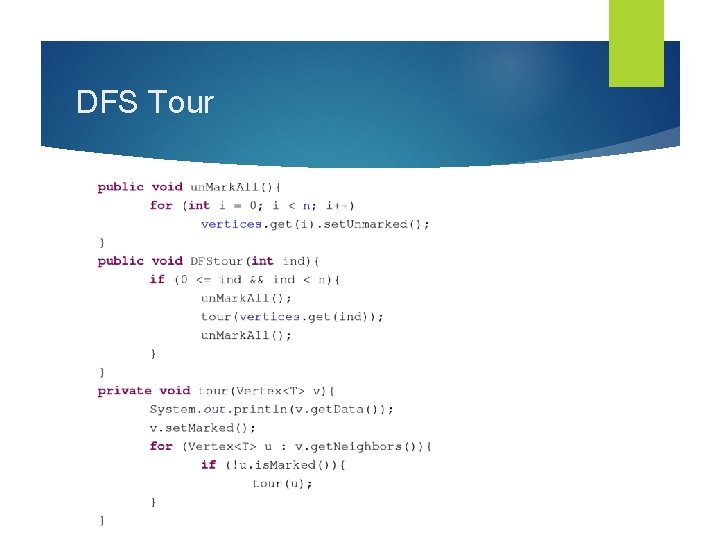
DFS Tour
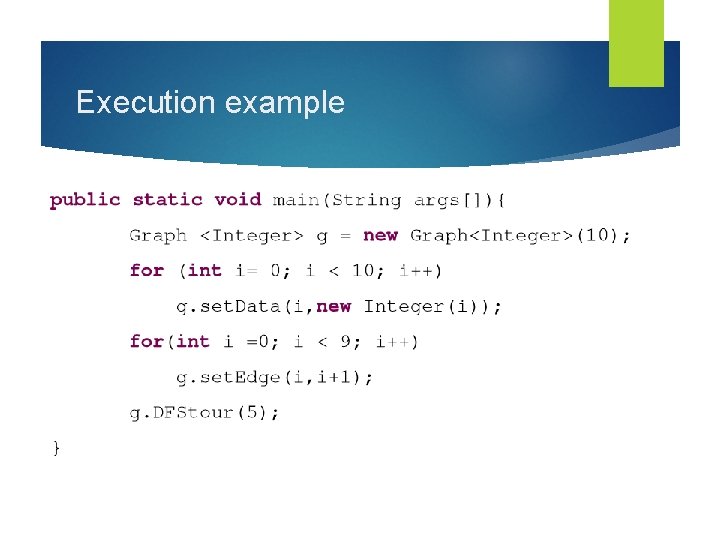
Execution example
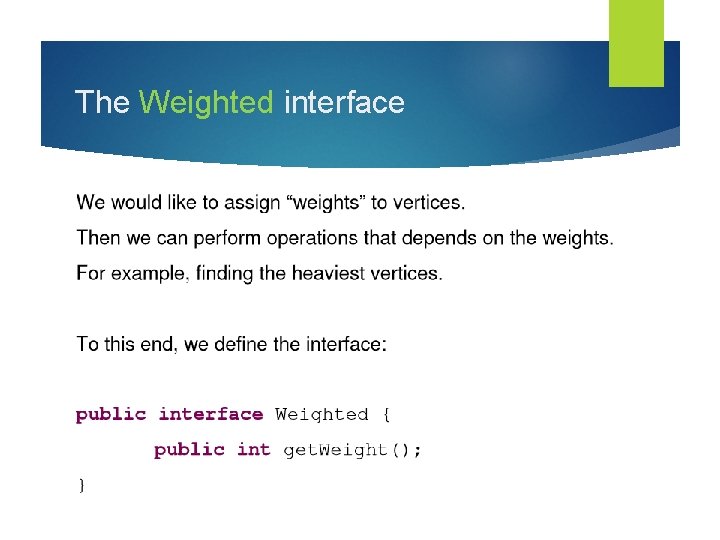
The Weighted interface
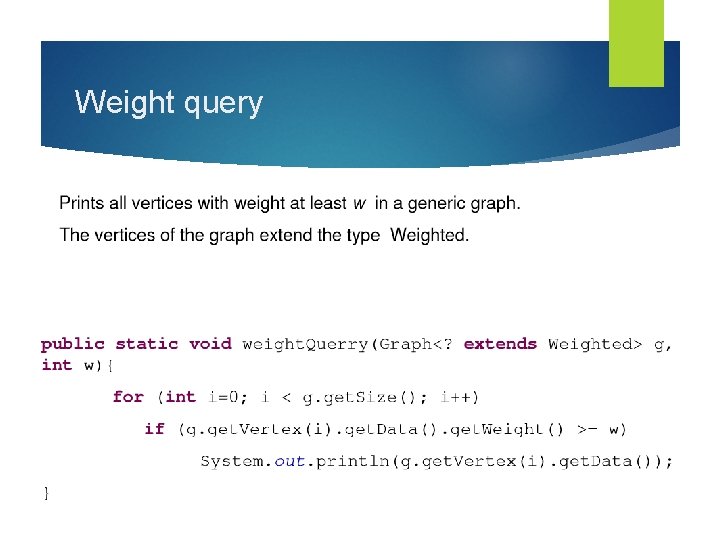
Weight query
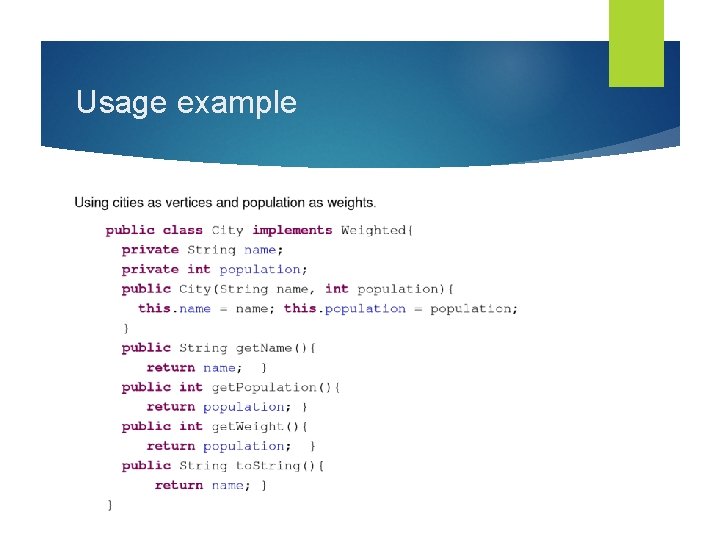
Usage example
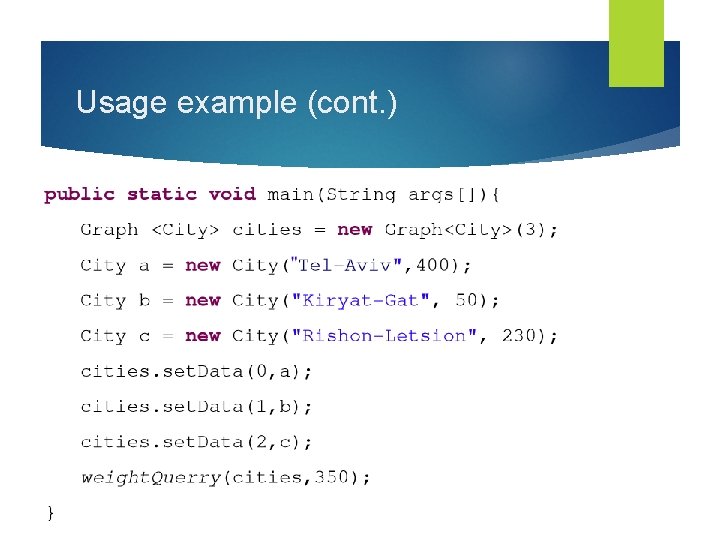
Usage example (cont. )
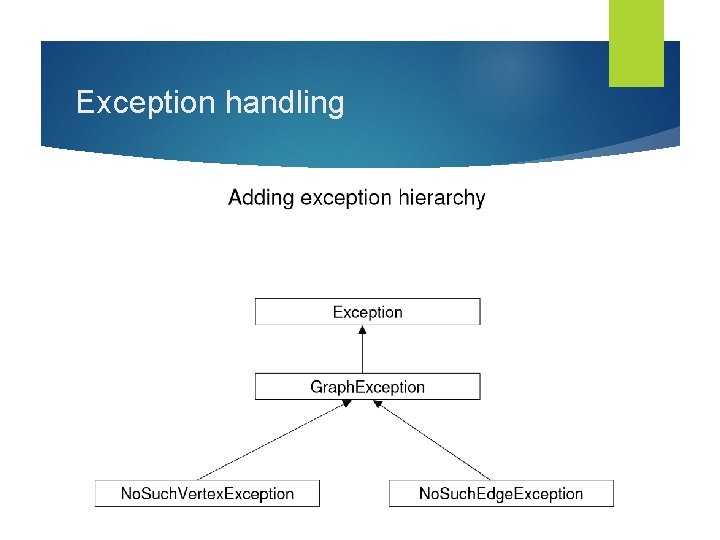
Exception handling
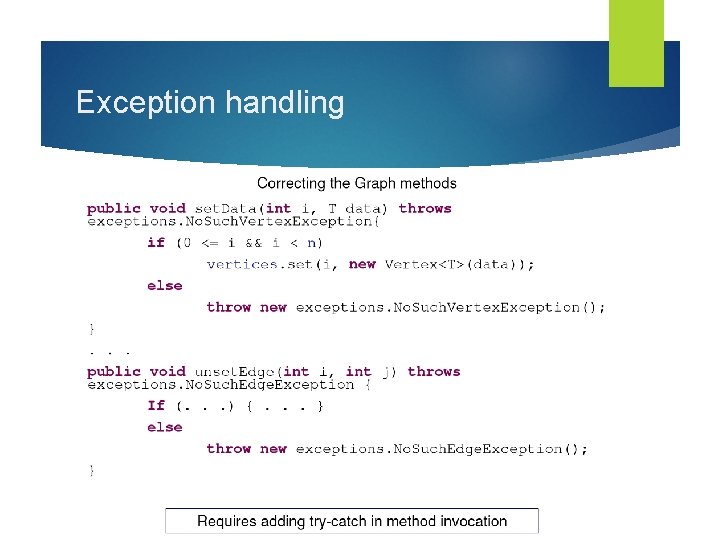
Exception handling
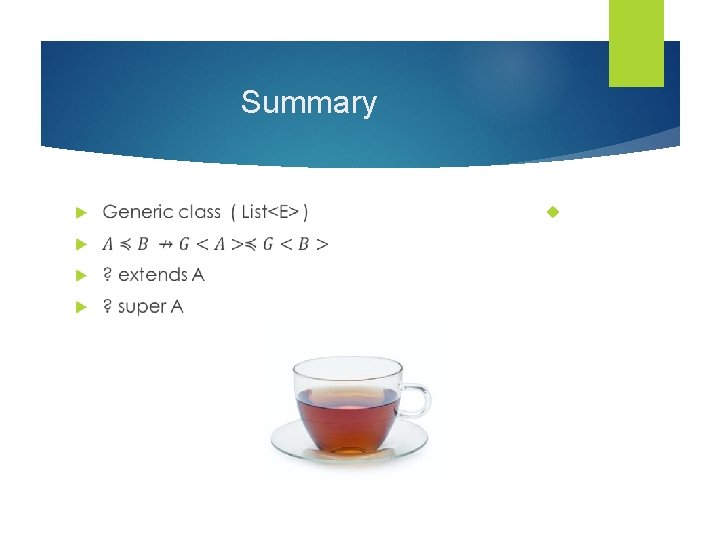
Summary
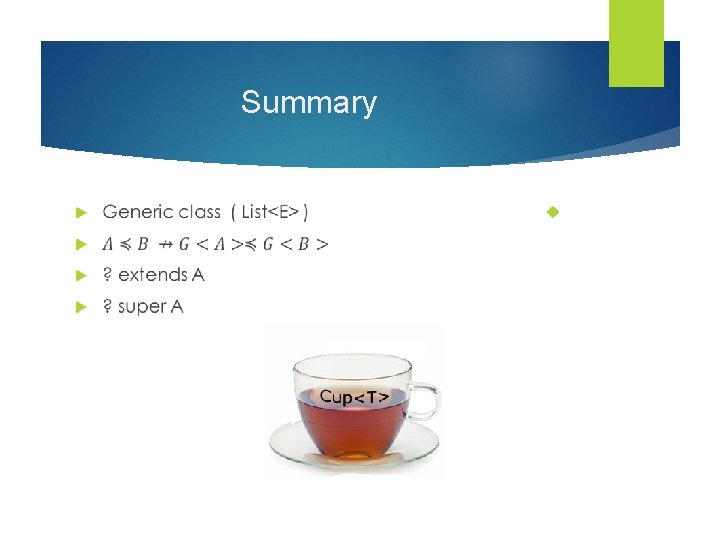
Summary
Wild born
Krakauer into thin air "torrent"
What are source cards
Java generic array
Generics java erklärung
Advantages of generics in java
Java generics array
Using system.collections.generic
Hospitality generics
Kotlinx serialization generics
C generics
Classe e subclasse de palavras exercícios 8 ano
Pre ap classes vs regular classes
What is a quote sandwich examples
Static v dynamic character
Prisma model inheritance
Uml inheritance diagram
Priority inheritance
Donna malayeri
The inheritance of loss summary
Thalassemia trait
Rails sti vs polymorphic
Sex linked inheritance punnett square
Sexlinked inheritance
Qualitative traits vs quantitative traits
Niv proverbs 13
Tujuan inheritance
Inheritance adalah
Sex linked dominant pedigree
Complete dominance pattern of inheritance
Private inheritance
Non nuclear inheritance
Non mendelian inheritance
Human inheritance modern genetics answer key
Modern genetics human inheritance answer key
Modern genetics human inheritance answer key
Epistatic inheritance
Mendel's 3rd law of inheritance
Hemophilia mode of inheritance
Perservefamily/status