More Multithreaded Programming in Java Multimedia Technology Programming
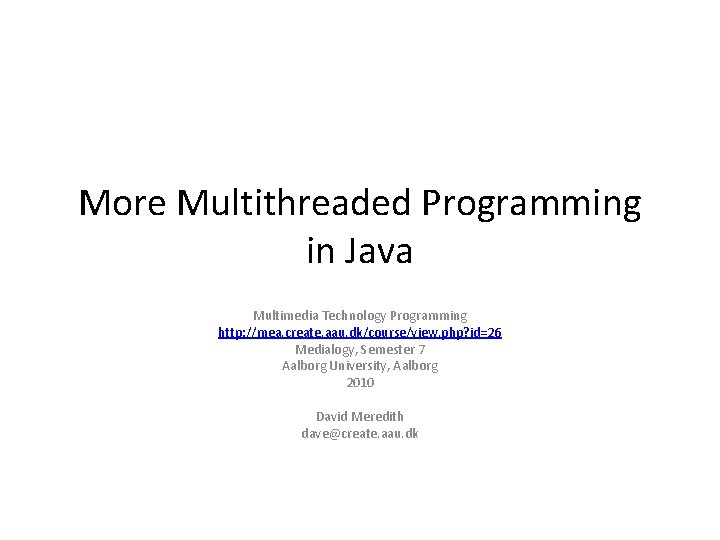
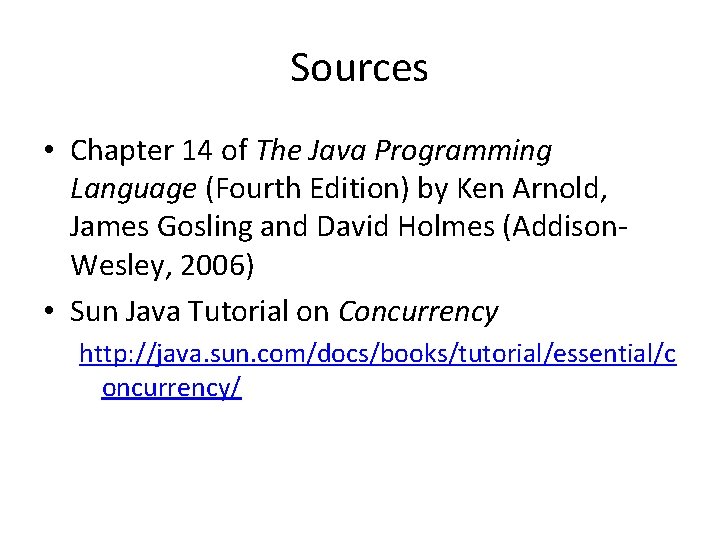
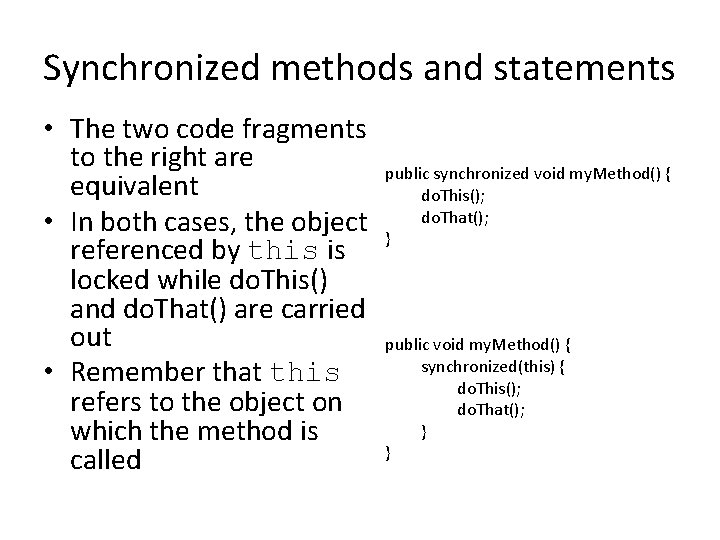
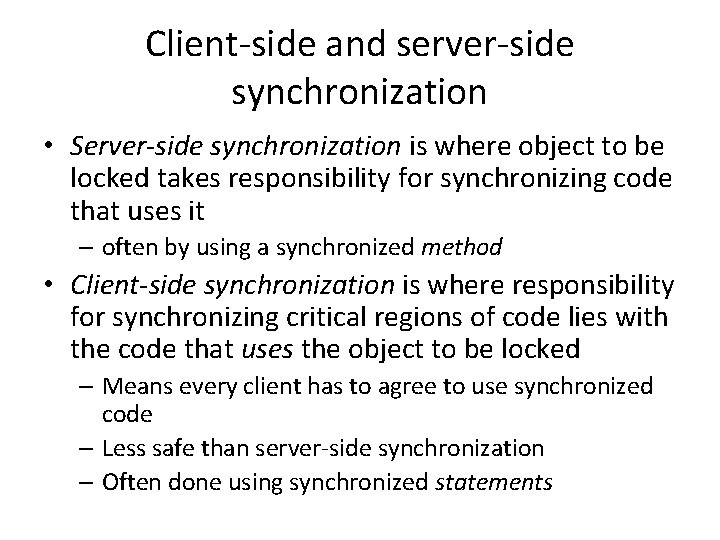
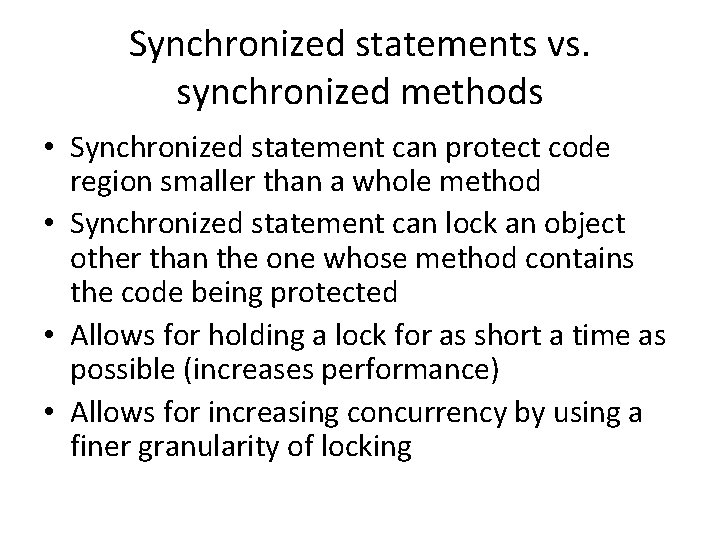
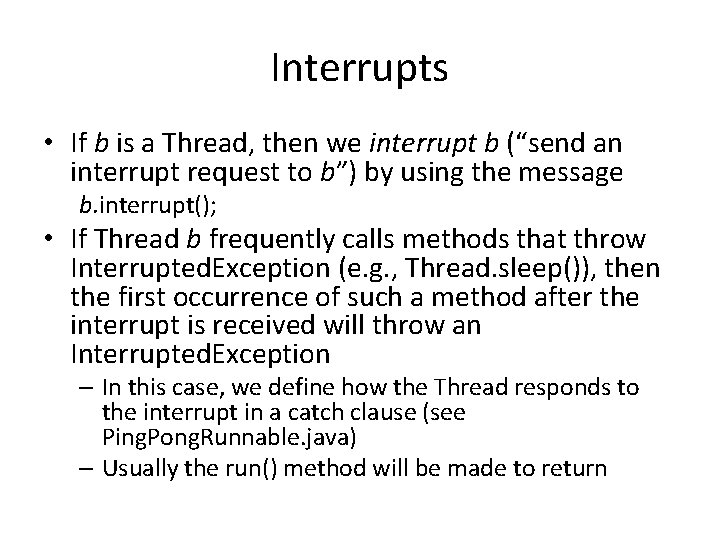
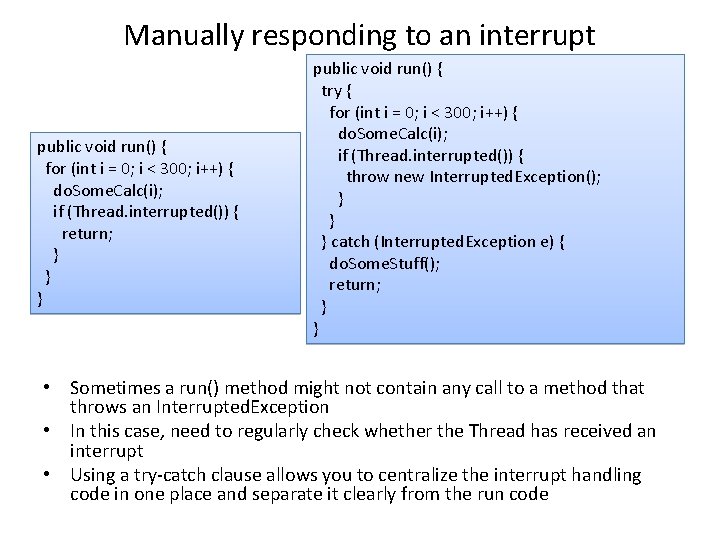
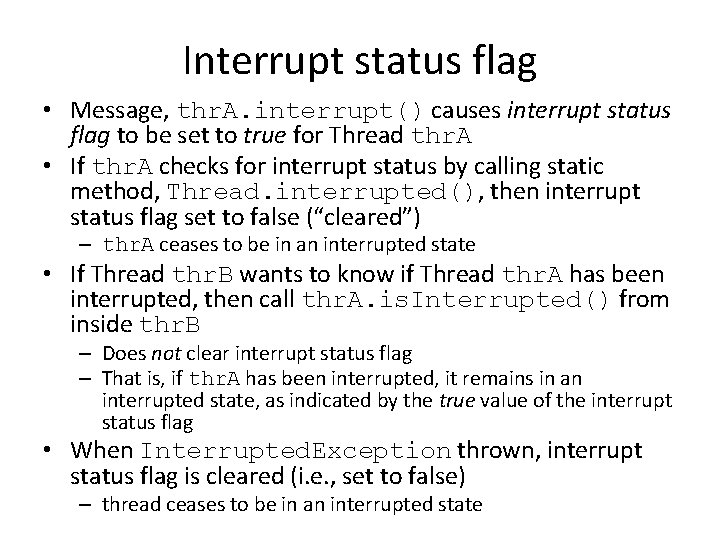
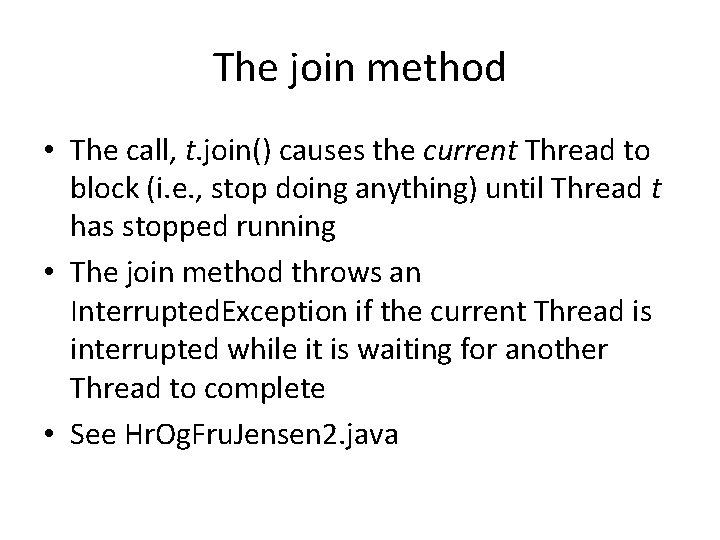
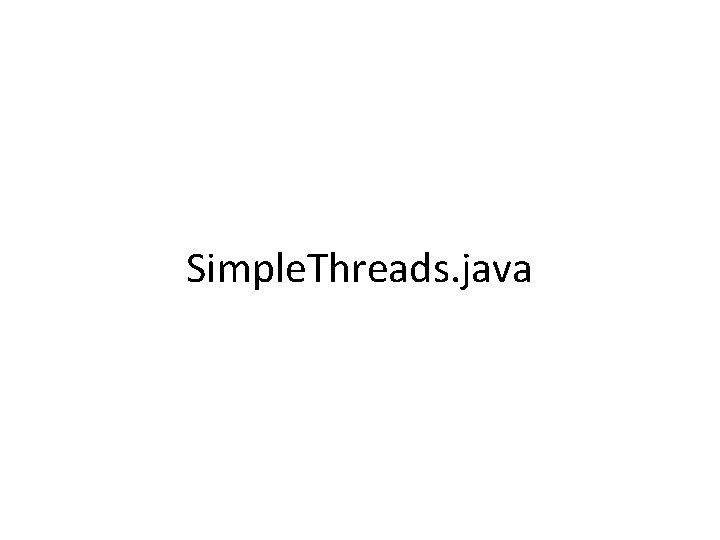
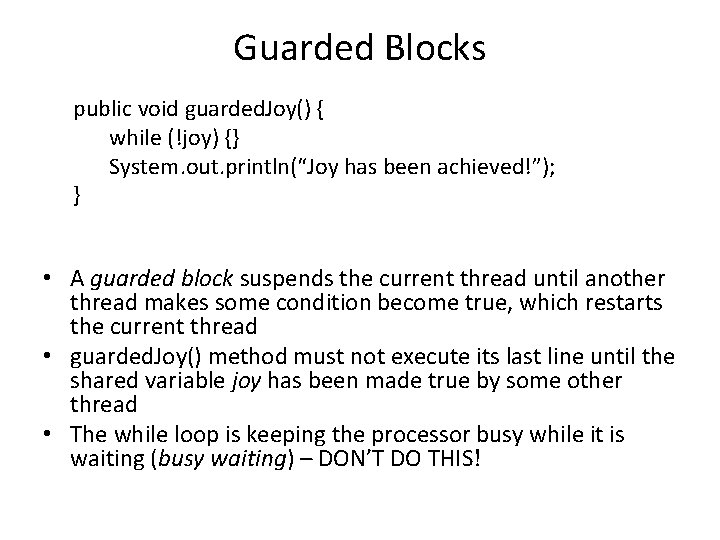
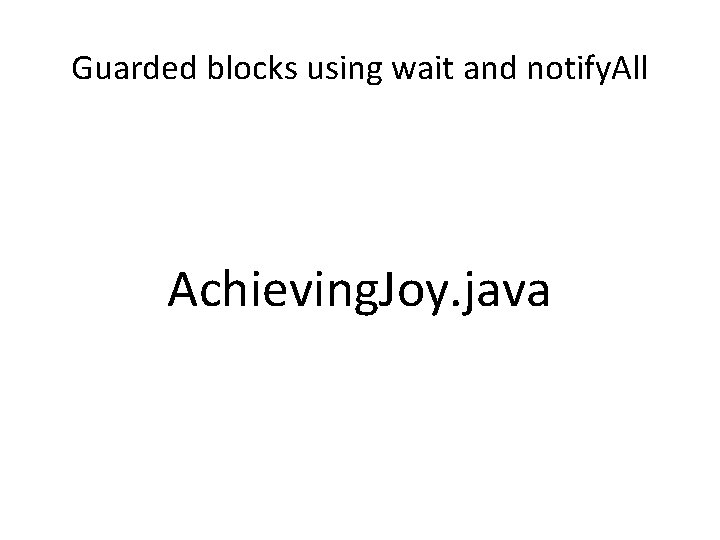
- Slides: 12
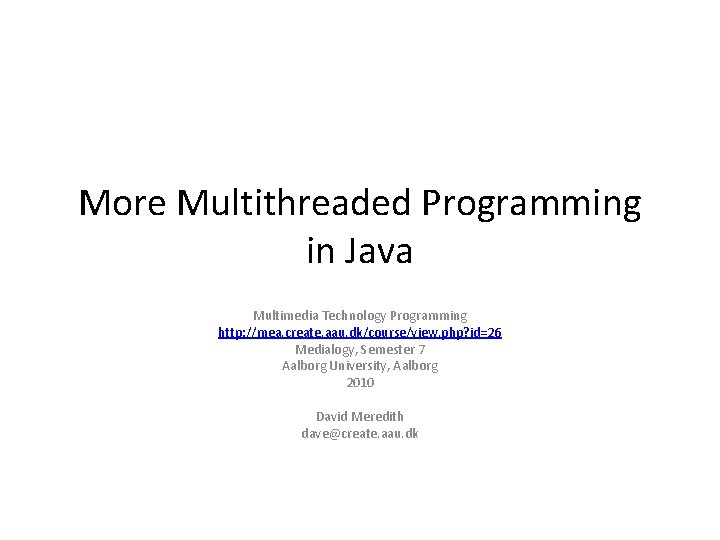
More Multithreaded Programming in Java Multimedia Technology Programming http: //mea. create. aau. dk/course/view. php? id=26 Medialogy, Semester 7 Aalborg University, Aalborg 2010 David Meredith dave@create. aau. dk
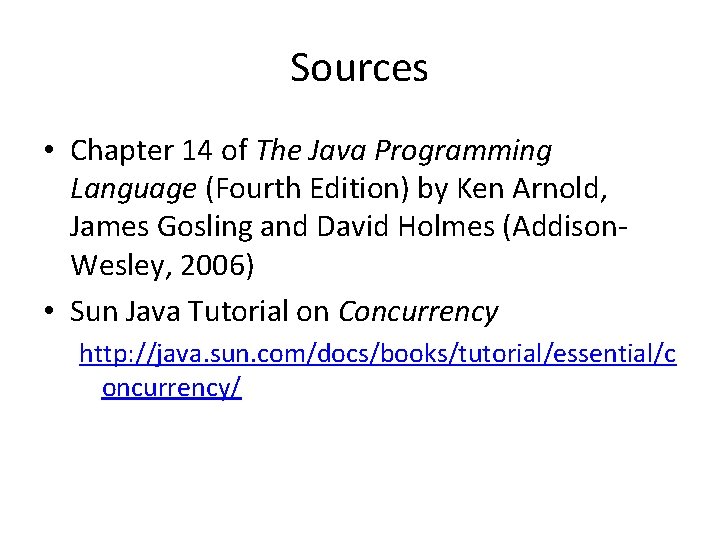
Sources • Chapter 14 of The Java Programming Language (Fourth Edition) by Ken Arnold, James Gosling and David Holmes (Addison. Wesley, 2006) • Sun Java Tutorial on Concurrency http: //java. sun. com/docs/books/tutorial/essential/c oncurrency/
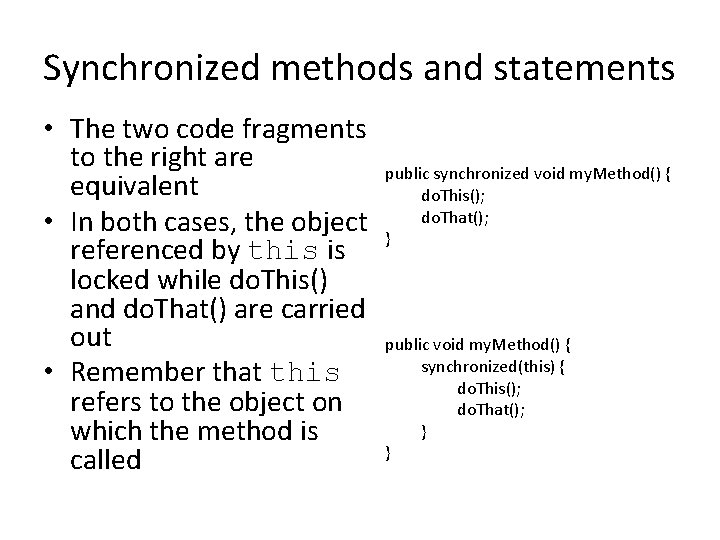
Synchronized methods and statements • The two code fragments to the right are equivalent • In both cases, the object referenced by this is locked while do. This() and do. That() are carried out • Remember that this refers to the object on which the method is called public synchronized void my. Method() { do. This(); do. That(); } public void my. Method() { synchronized(this) { do. This(); do. That(); } }
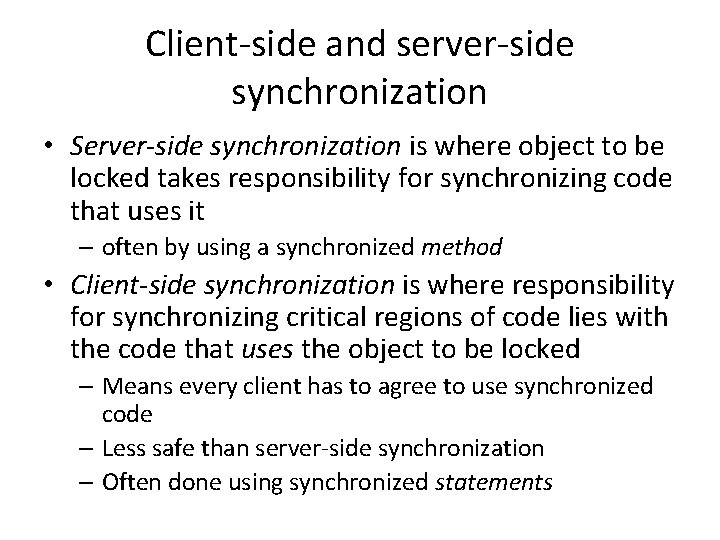
Client-side and server-side synchronization • Server-side synchronization is where object to be locked takes responsibility for synchronizing code that uses it – often by using a synchronized method • Client-side synchronization is where responsibility for synchronizing critical regions of code lies with the code that uses the object to be locked – Means every client has to agree to use synchronized code – Less safe than server-side synchronization – Often done using synchronized statements
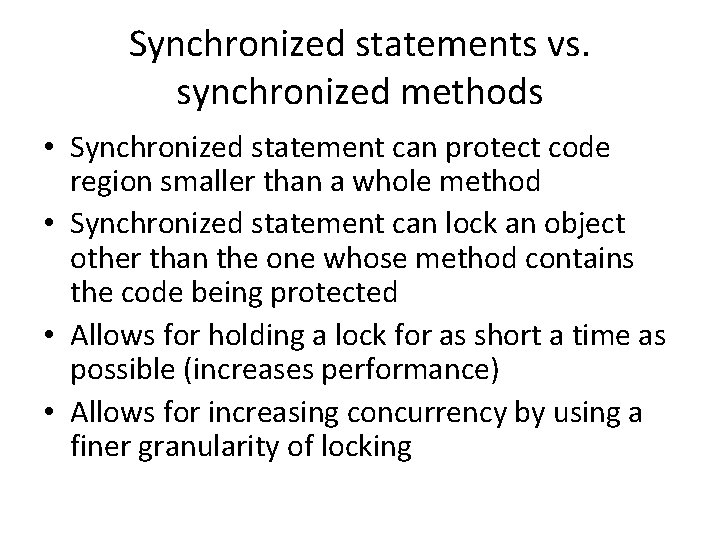
Synchronized statements vs. synchronized methods • Synchronized statement can protect code region smaller than a whole method • Synchronized statement can lock an object other than the one whose method contains the code being protected • Allows for holding a lock for as short a time as possible (increases performance) • Allows for increasing concurrency by using a finer granularity of locking
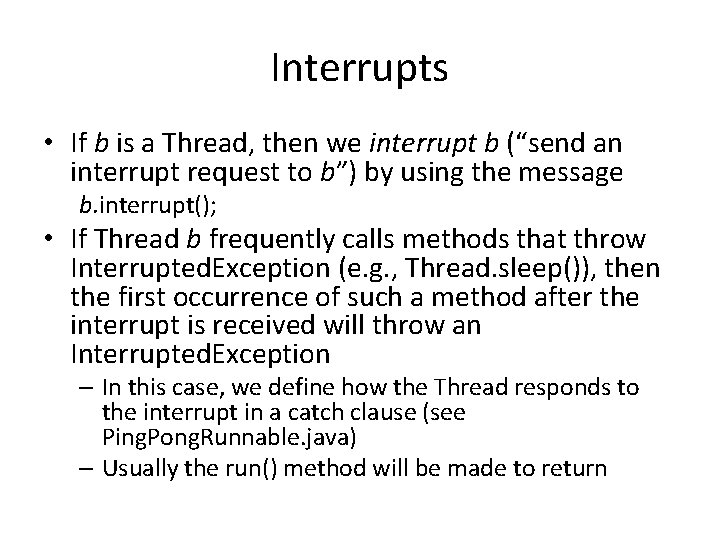
Interrupts • If b is a Thread, then we interrupt b (“send an interrupt request to b”) by using the message b. interrupt(); • If Thread b frequently calls methods that throw Interrupted. Exception (e. g. , Thread. sleep()), then the first occurrence of such a method after the interrupt is received will throw an Interrupted. Exception – In this case, we define how the Thread responds to the interrupt in a catch clause (see Ping. Pong. Runnable. java) – Usually the run() method will be made to return
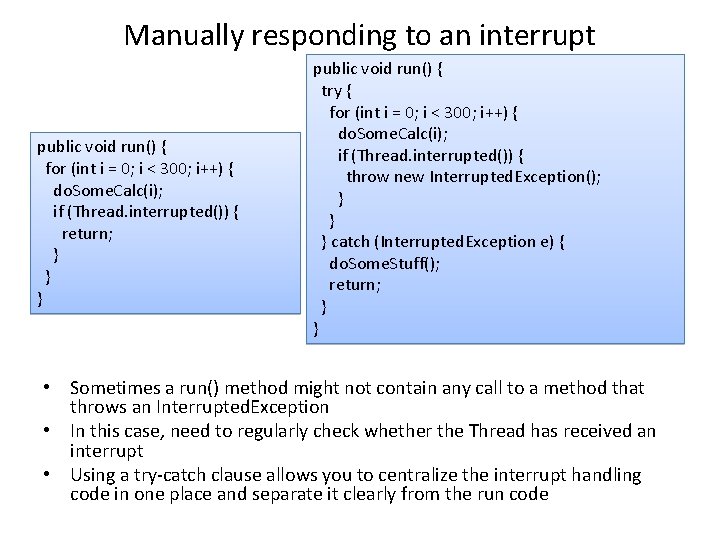
Manually responding to an interrupt public void run() { for (int i = 0; i < 300; i++) { do. Some. Calc(i); if (Thread. interrupted()) { return; } } } public void run() { try { for (int i = 0; i < 300; i++) { do. Some. Calc(i); if (Thread. interrupted()) { throw new Interrupted. Exception(); } } } catch (Interrupted. Exception e) { do. Some. Stuff(); return; } } • Sometimes a run() method might not contain any call to a method that throws an Interrupted. Exception • In this case, need to regularly check whether the Thread has received an interrupt • Using a try-catch clause allows you to centralize the interrupt handling code in one place and separate it clearly from the run code
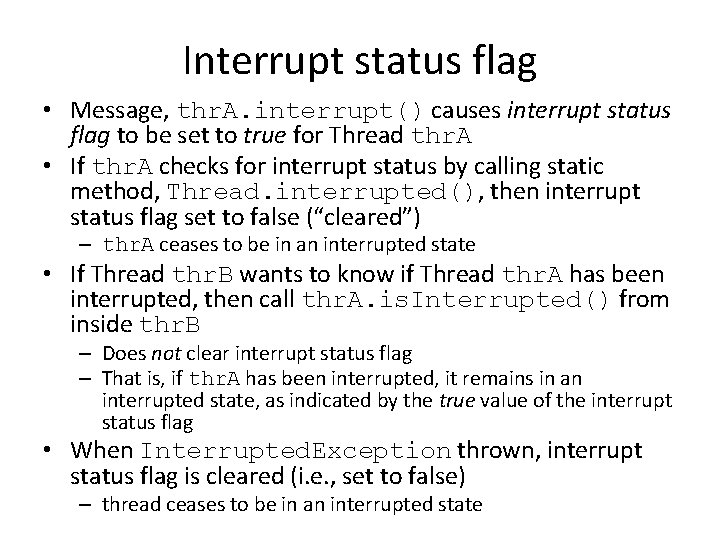
Interrupt status flag • Message, thr. A. interrupt() causes interrupt status flag to be set to true for Thread thr. A • If thr. A checks for interrupt status by calling static method, Thread. interrupted(), then interrupt status flag set to false (“cleared”) – thr. A ceases to be in an interrupted state • If Thread thr. B wants to know if Thread thr. A has been interrupted, then call thr. A. is. Interrupted() from inside thr. B – Does not clear interrupt status flag – That is, if thr. A has been interrupted, it remains in an interrupted state, as indicated by the true value of the interrupt status flag • When Interrupted. Exception thrown, interrupt status flag is cleared (i. e. , set to false) – thread ceases to be in an interrupted state
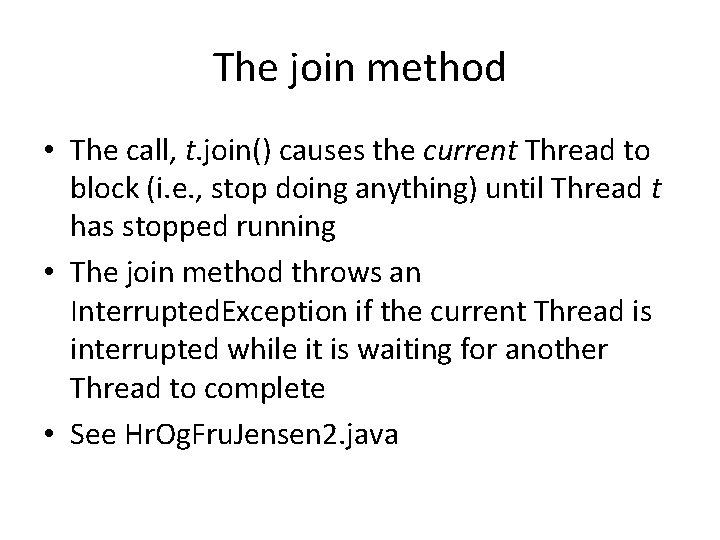
The join method • The call, t. join() causes the current Thread to block (i. e. , stop doing anything) until Thread t has stopped running • The join method throws an Interrupted. Exception if the current Thread is interrupted while it is waiting for another Thread to complete • See Hr. Og. Fru. Jensen 2. java
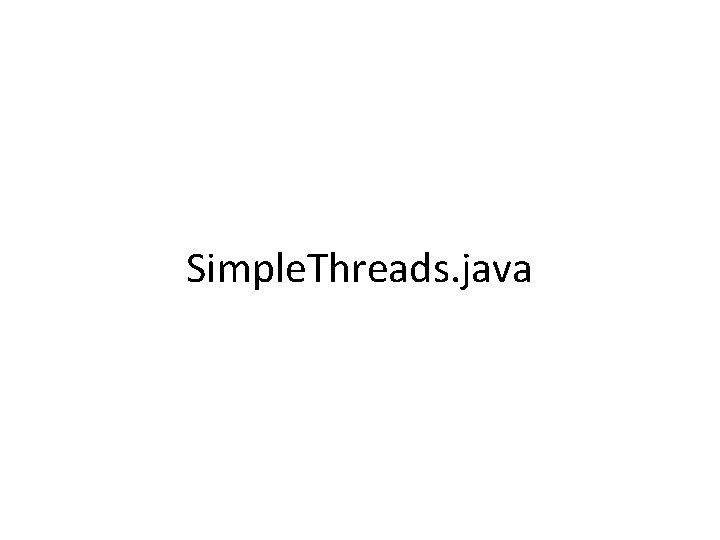
Simple. Threads. java
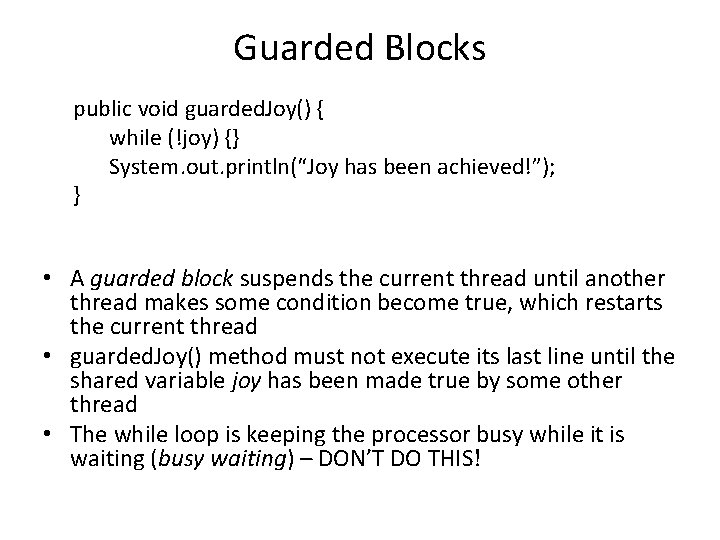
Guarded Blocks public void guarded. Joy() { while (!joy) {} System. out. println(“Joy has been achieved!”); } • A guarded block suspends the current thread until another thread makes some condition become true, which restarts the current thread • guarded. Joy() method must not execute its last line until the shared variable joy has been made true by some other thread • The while loop is keeping the processor busy while it is waiting (busy waiting) – DON’T DO THIS!
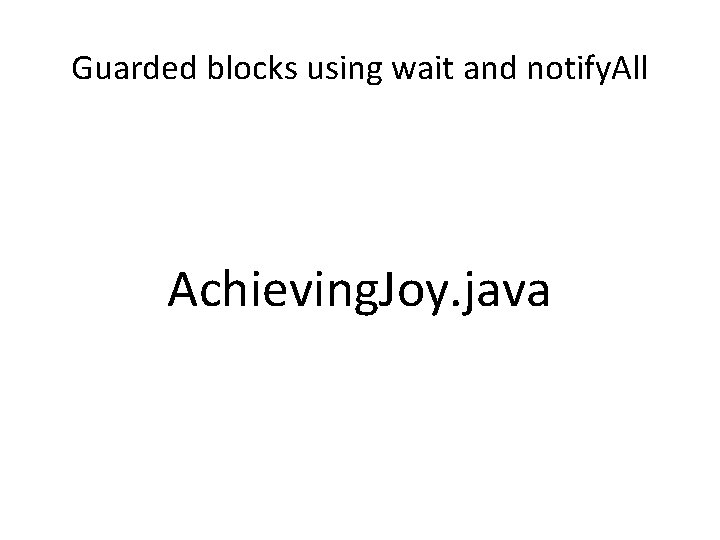
Guarded blocks using wait and notify. All Achieving. Joy. java
More more more i want more more more more we praise you
More more more i want more more more more we praise you
Real time example of multithreading in java
Jumlah level dalam gambar ini adalah . . . .
Multithreaded games
Multithreaded algorithms
Apt multithreaded
Multimedia becomes interactive multimedia when
Hyperactive multimedia examples
Csc253 interactive multimedia
Esa multimedia.esa.int./multimedia/virtual-tour-iss
Define multimedia technology
Iat 265