More Control Structures if and switch Chap 8
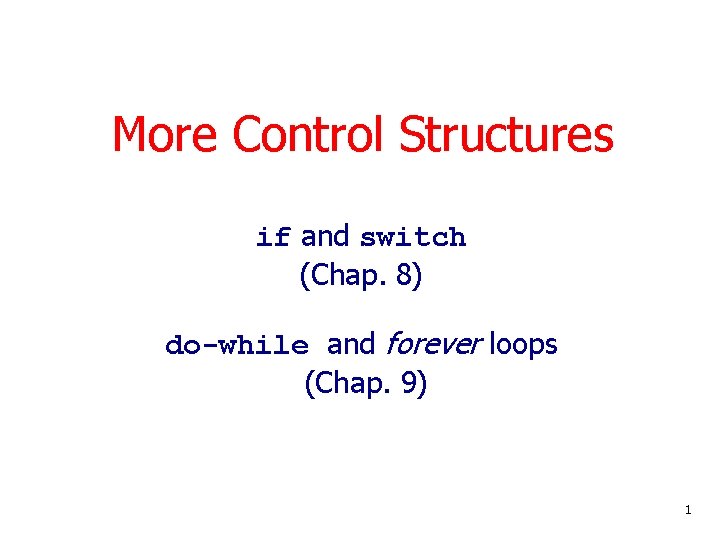
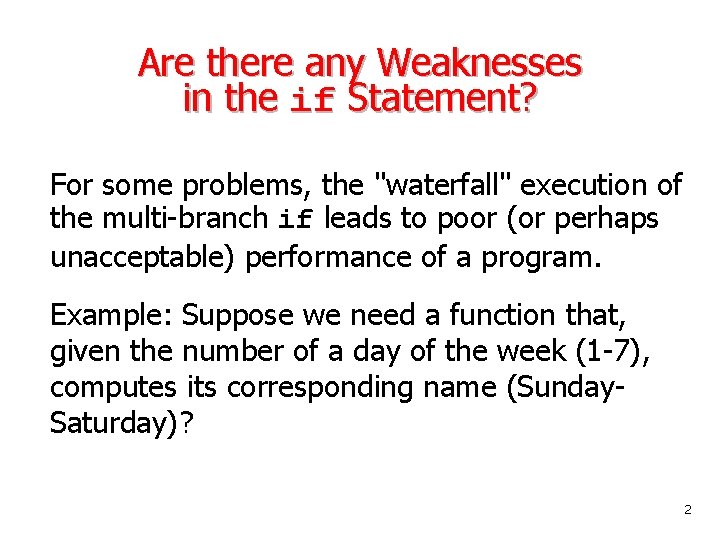
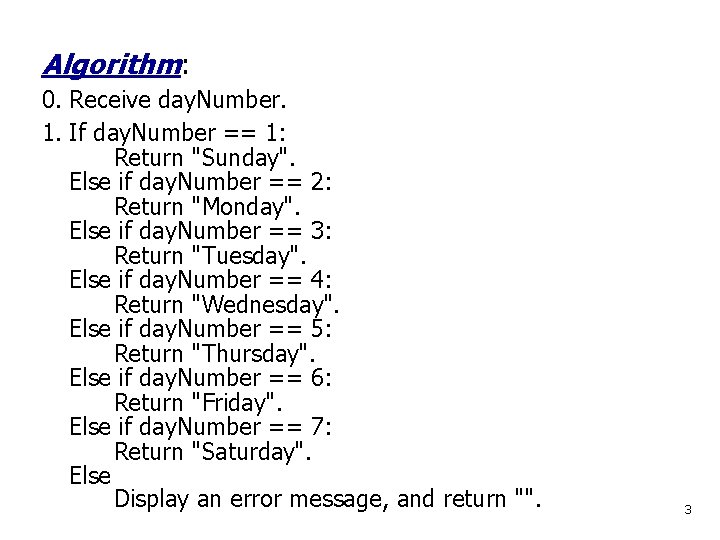
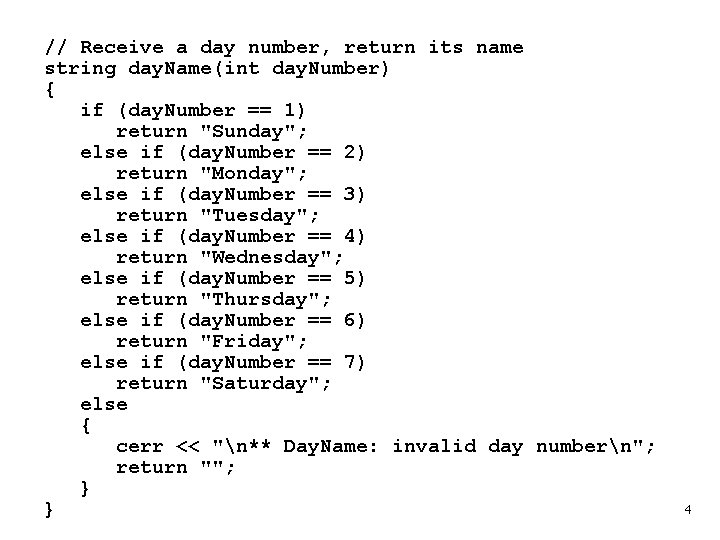
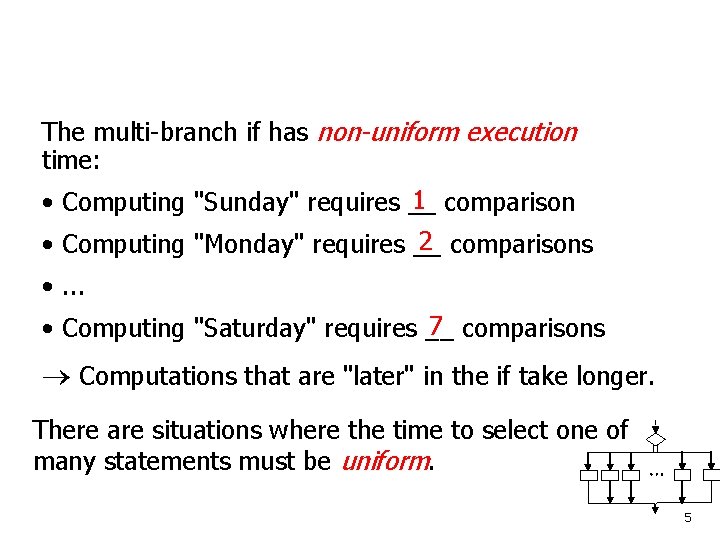
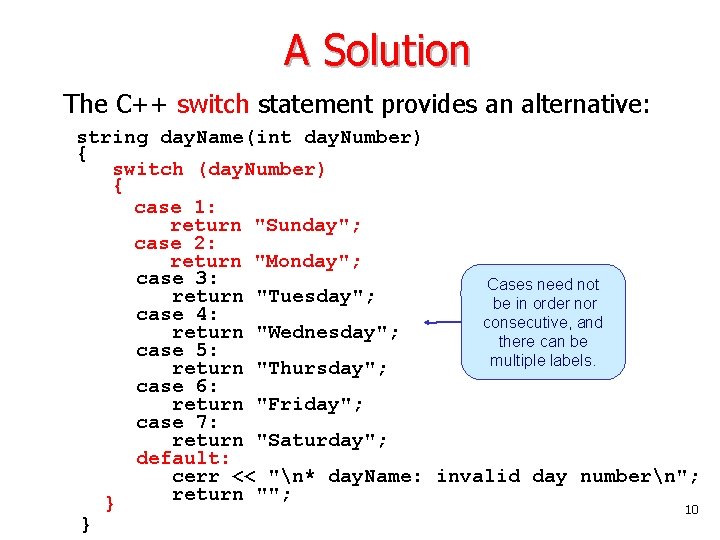
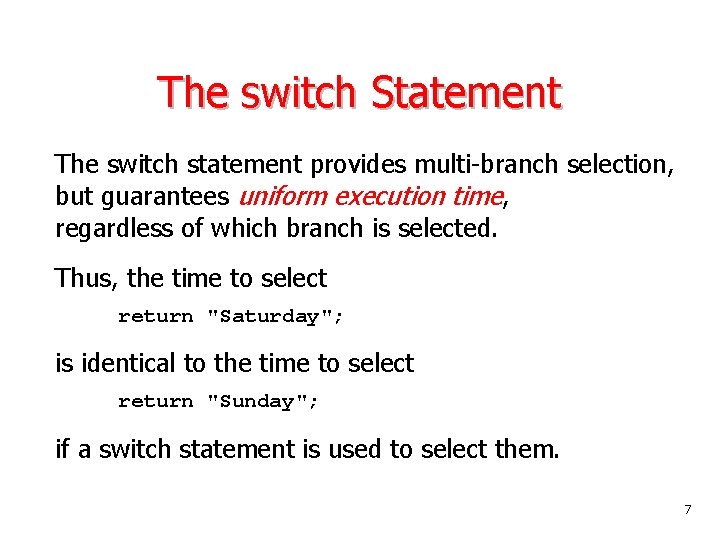
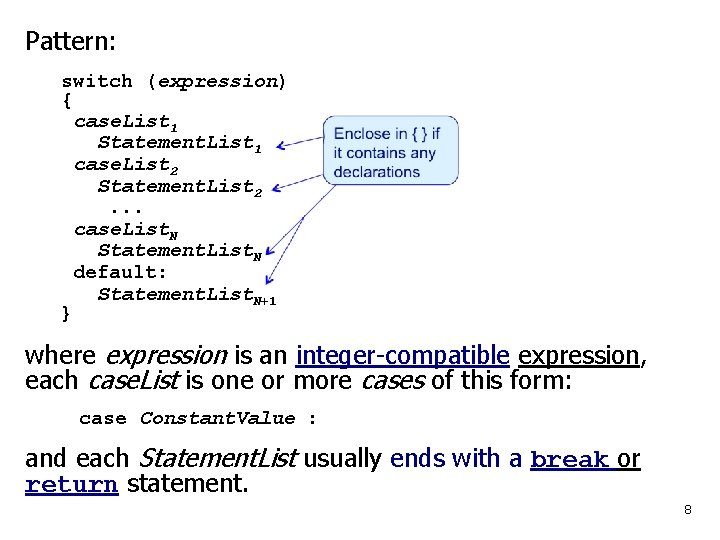
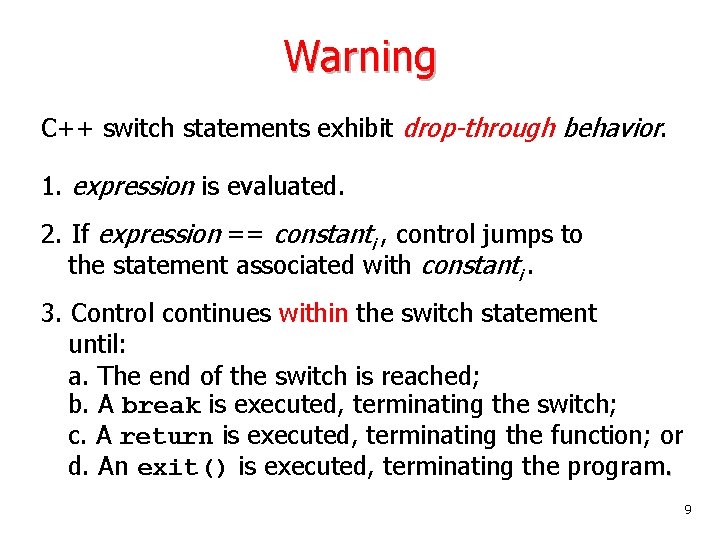
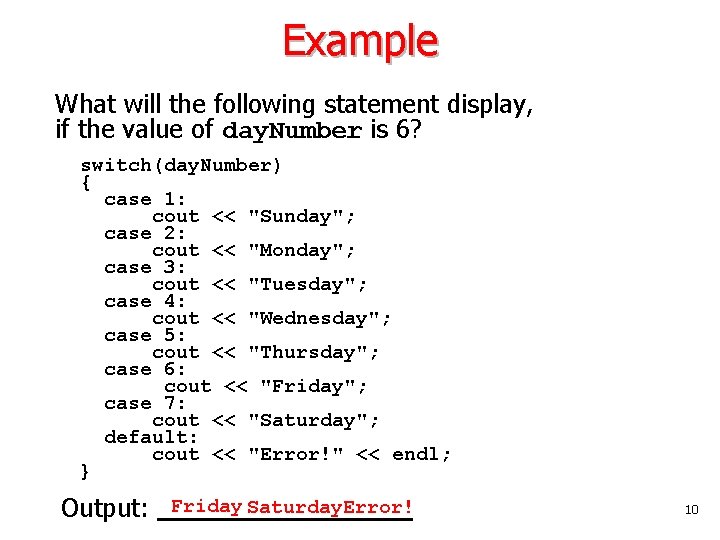
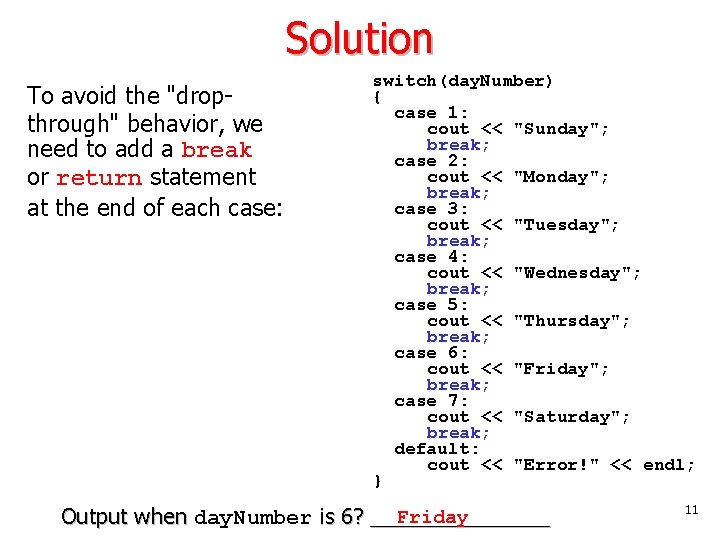
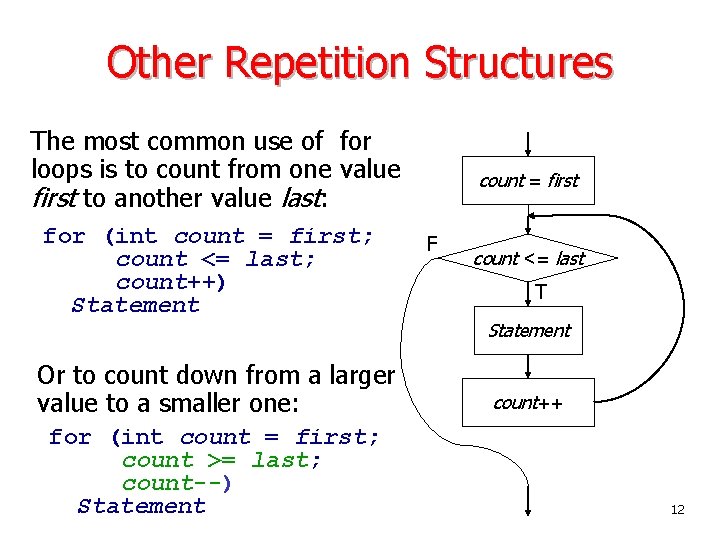
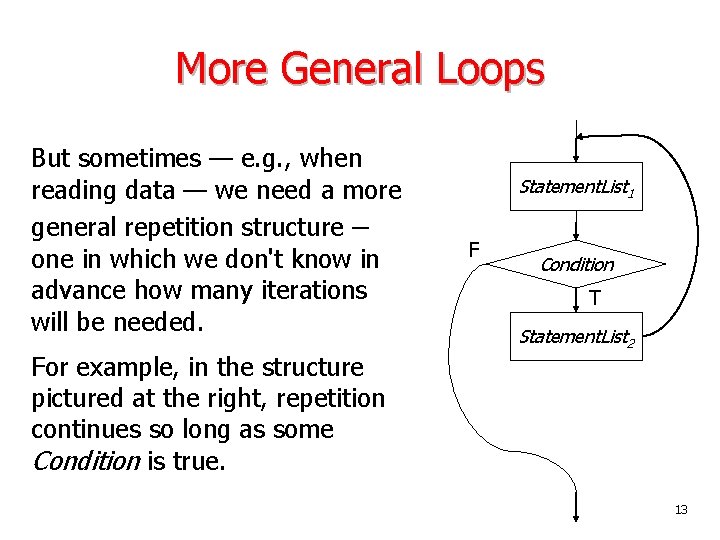
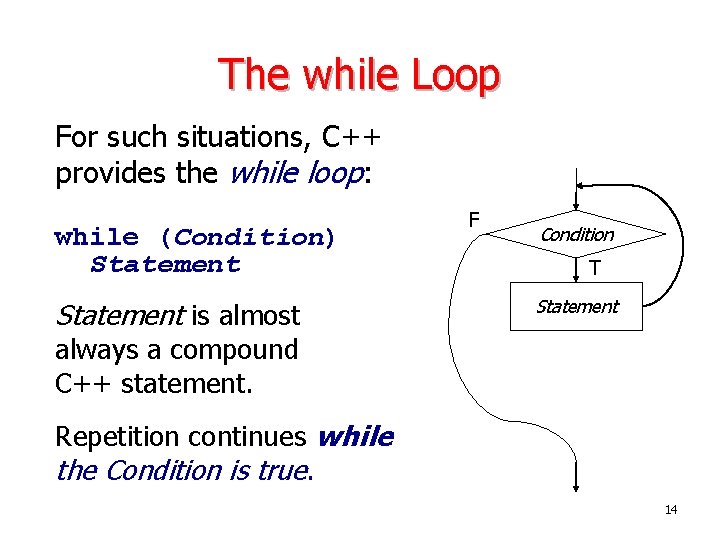
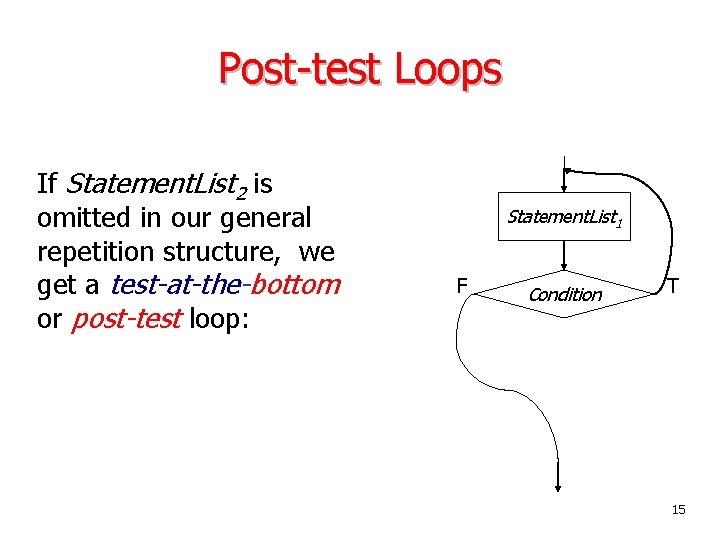
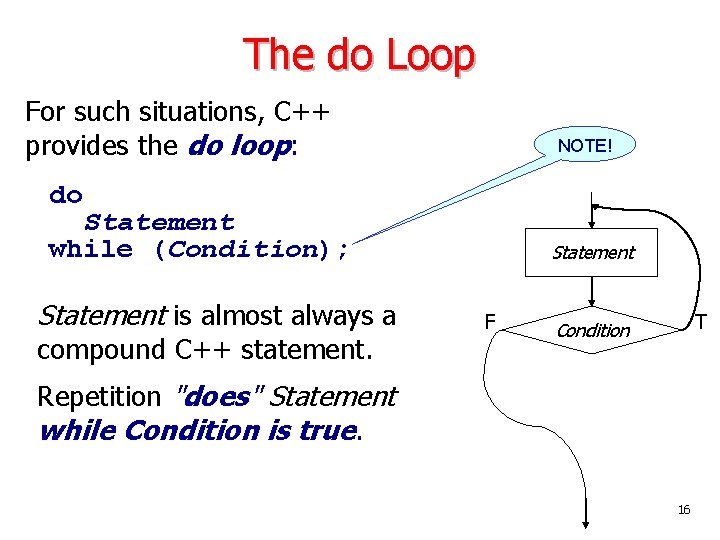
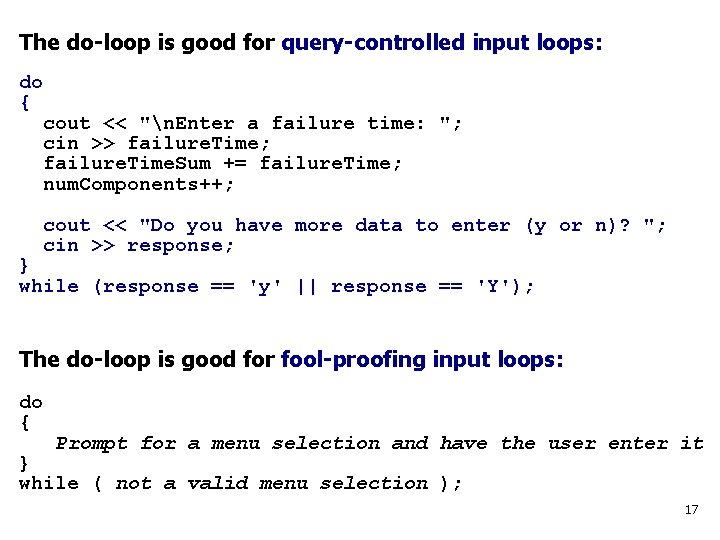
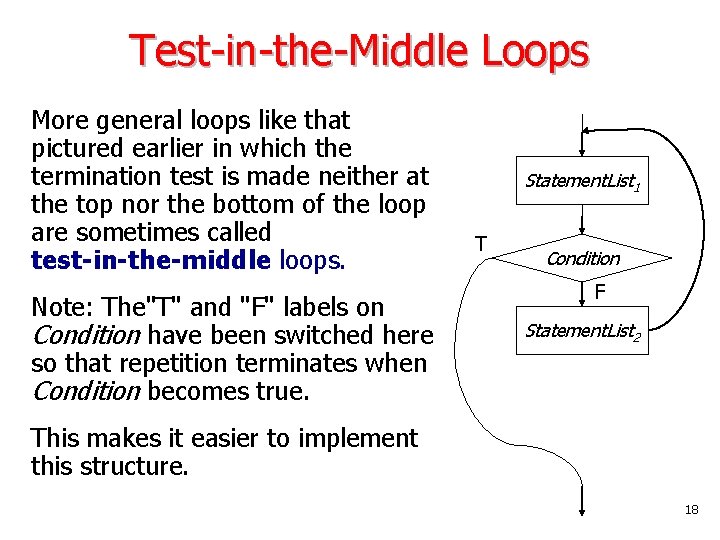
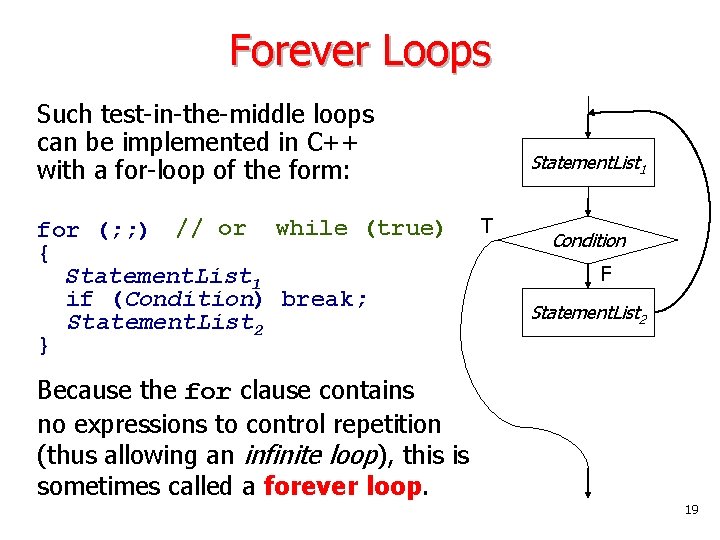
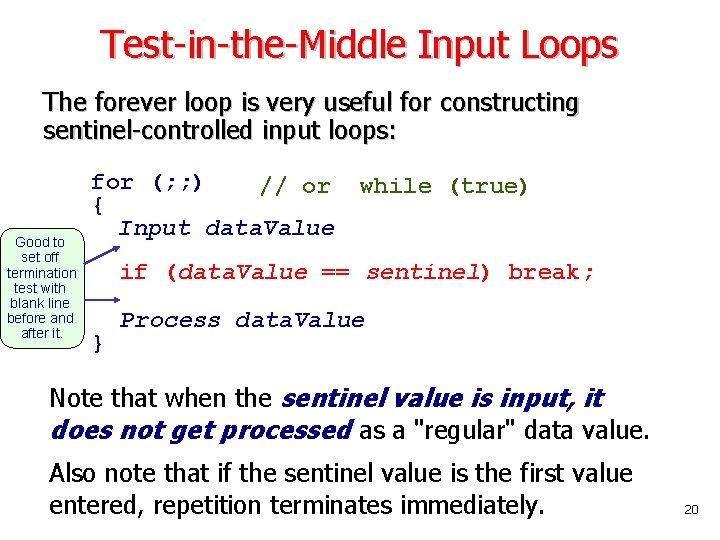
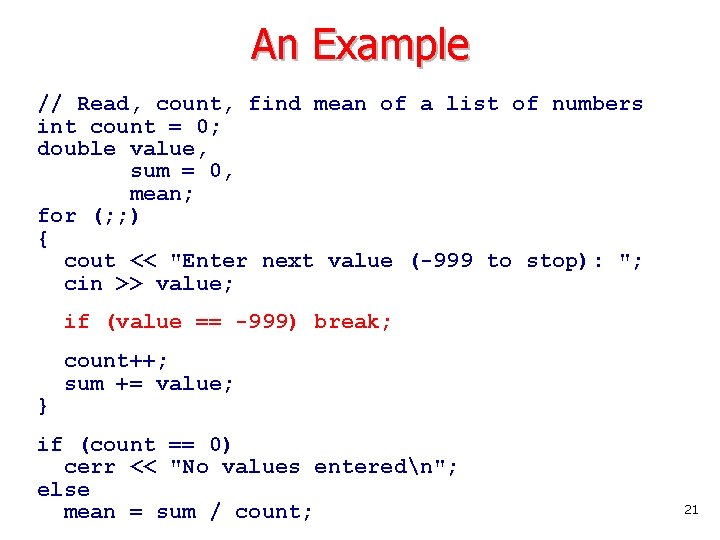
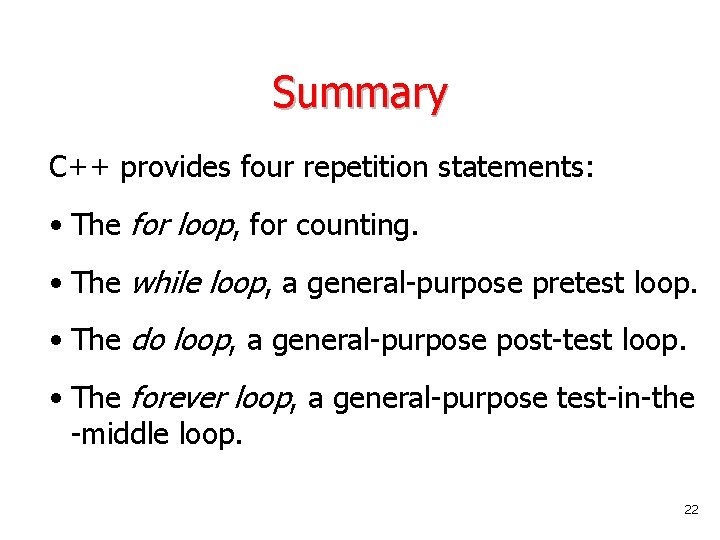
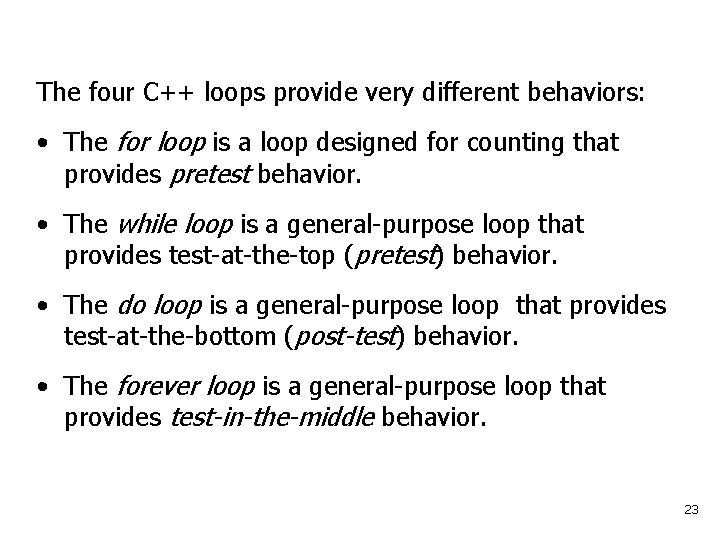
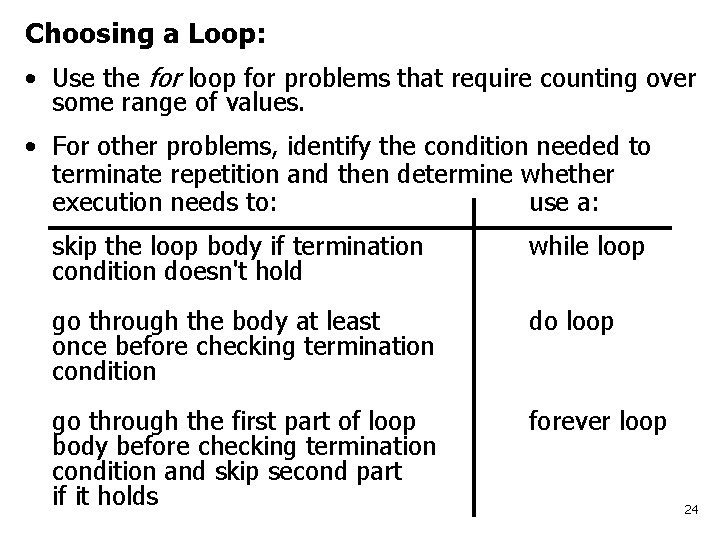
- Slides: 24
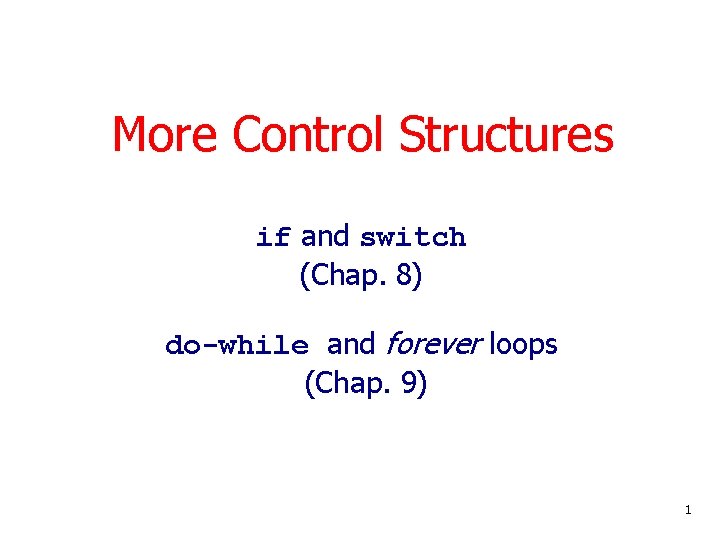
More Control Structures if and switch (Chap. 8) do-while and forever loops (Chap. 9) 1
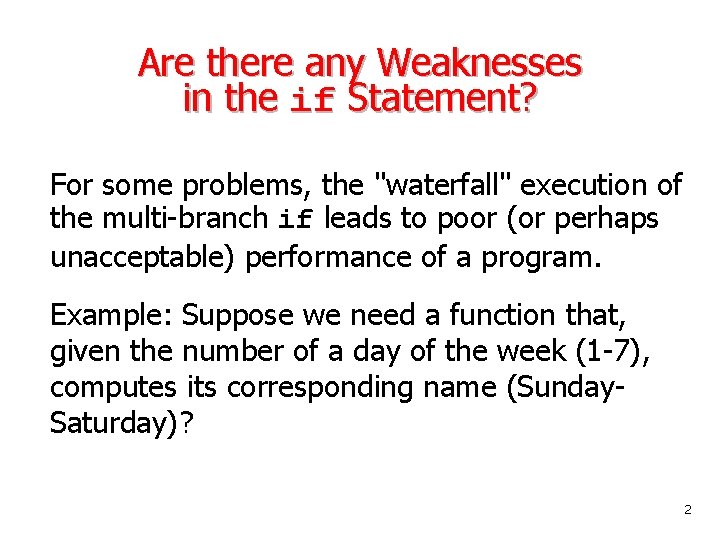
Are there any Weaknesses in the if Statement? For some problems, the "waterfall" execution of the multi-branch if leads to poor (or perhaps unacceptable) performance of a program. Example: Suppose we need a function that, given the number of a day of the week (1 -7), computes its corresponding name (Sunday. Saturday)? 2
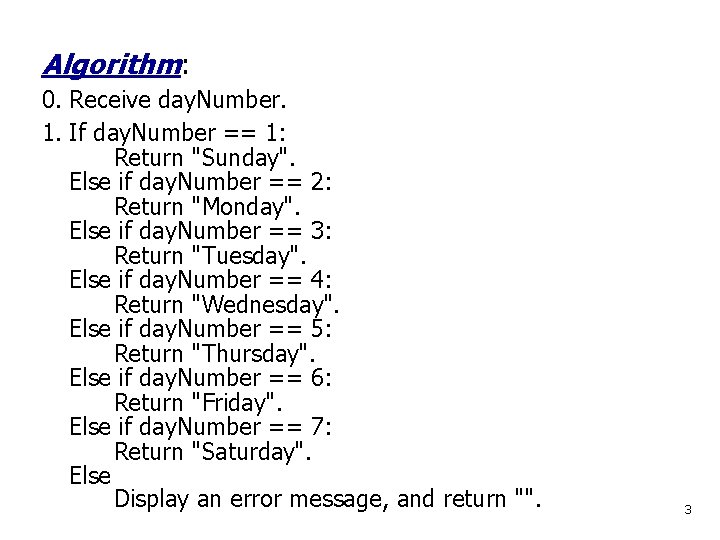
Algorithm: 0. Receive day. Number. 1. If day. Number == 1: Return "Sunday". Else if day. Number == 2: Return "Monday". Else if day. Number == 3: Return "Tuesday". Else if day. Number == 4: Return "Wednesday". Else if day. Number == 5: Return "Thursday". Else if day. Number == 6: Return "Friday". Else if day. Number == 7: Return "Saturday". Else Display an error message, and return "". 3
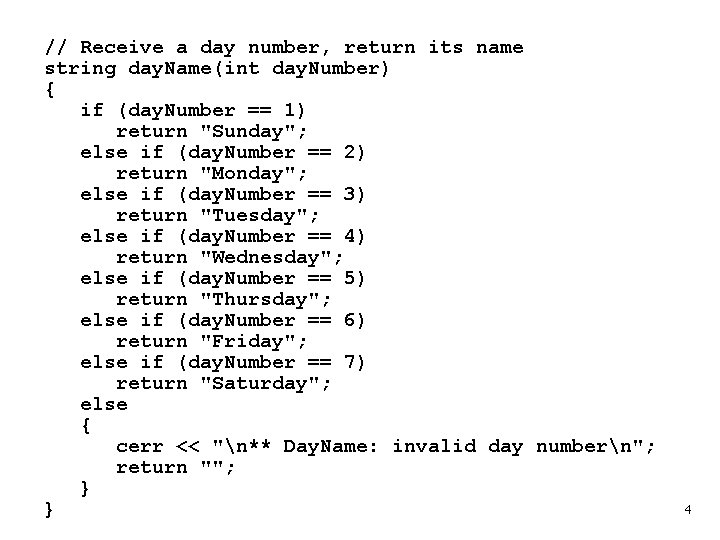
// Receive a day number, return its name string day. Name(int day. Number) { if (day. Number == 1) return "Sunday"; else if (day. Number == 2) return "Monday"; else if (day. Number == 3) return "Tuesday"; else if (day. Number == 4) return "Wednesday"; else if (day. Number == 5) return "Thursday"; else if (day. Number == 6) return "Friday"; else if (day. Number == 7) return "Saturday"; else { cerr << "n** Day. Name: invalid day numbern"; return ""; } } 4
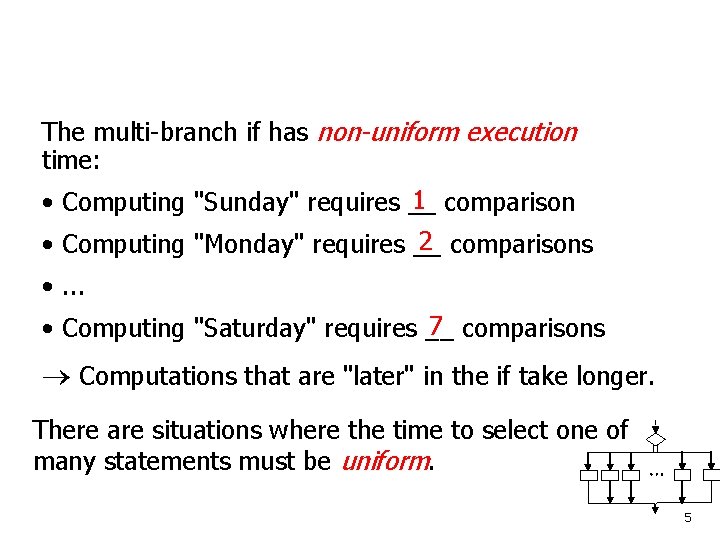
The multi-branch if has non-uniform execution time: 1 comparison • Computing "Sunday" requires __ 2 comparisons • Computing "Monday" requires __ • . . . 7 comparisons • Computing "Saturday" requires __ ® Computations that are "later" in the if take longer. There are situations where the time to select one of many statements must be uniform. 5
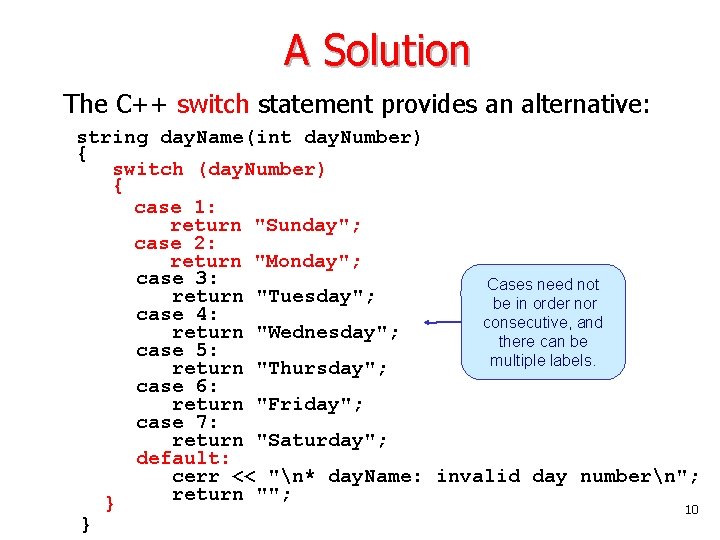
A Solution The C++ switch statement provides an alternative: string day. Name(int day. Number) { switch (day. Number) { case 1: return "Sunday"; case 2: return "Monday"; case 3: Cases need not return "Tuesday"; be in order nor case 4: consecutive, and return "Wednesday"; there can be case 5: multiple labels. return "Thursday"; case 6: return "Friday"; case 7: return "Saturday"; default: cerr << "n* day. Name: invalid day numbern"; return ""; } 10 }
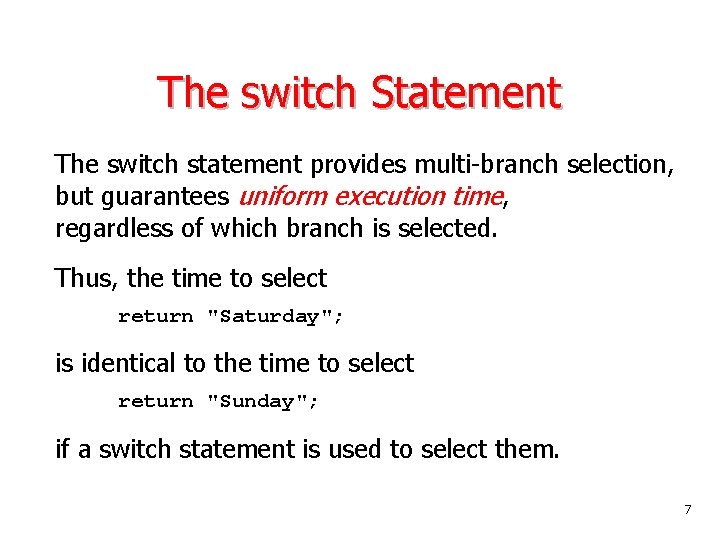
The switch Statement The switch statement provides multi-branch selection, but guarantees uniform execution time, regardless of which branch is selected. Thus, the time to select return "Saturday"; is identical to the time to select return "Sunday"; if a switch statement is used to select them. 7
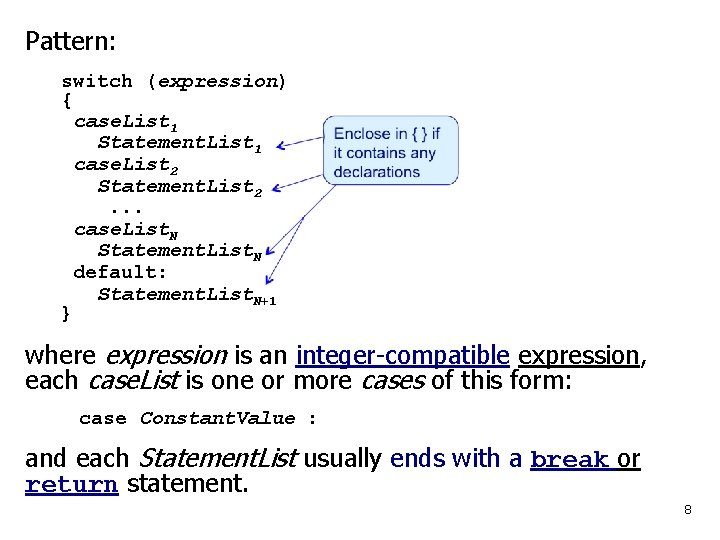
Pattern: switch (expression) { case. List 1 Statement. List 1 case. List 2 Statement. List 2 . . . case. List. N Statement. List. N default: Statement. List. N+1 } where expression is an integer-compatible expression, each case. List is one or more cases of this form: case Constant. Value : and each Statement. List usually ends with a break or return statement. 8
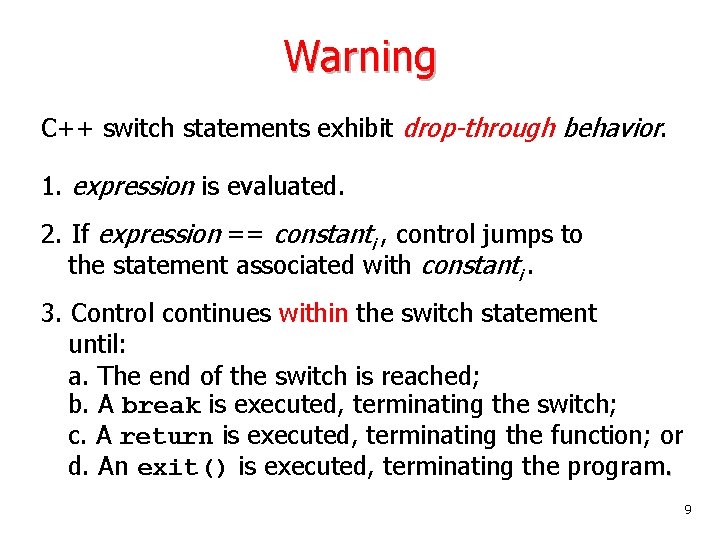
Warning C++ switch statements exhibit drop-through behavior. 1. expression is evaluated. 2. If expression == constanti , control jumps to the statement associated with constanti. 3. Control continues within the switch statement until: a. The end of the switch is reached; b. A break is executed, terminating the switch; c. A return is executed, terminating the function; or d. An exit() is executed, terminating the program. 9
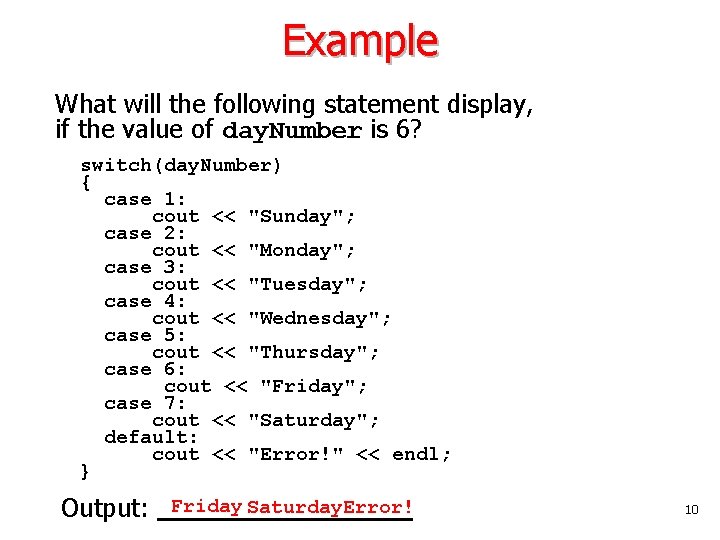
Example What will the following statement display, if the value of day. Number is 6? switch(day. Number) { case 1: cout << "Sunday"; case 2: cout << "Monday"; case 3: cout << "Tuesday"; case 4: cout << "Wednesday"; case 5: cout << "Thursday"; case 6: cout << "Friday"; case 7: cout << "Saturday"; default: cout << "Error!" << endl; } Friday Saturday. Error! Output: _________ 10
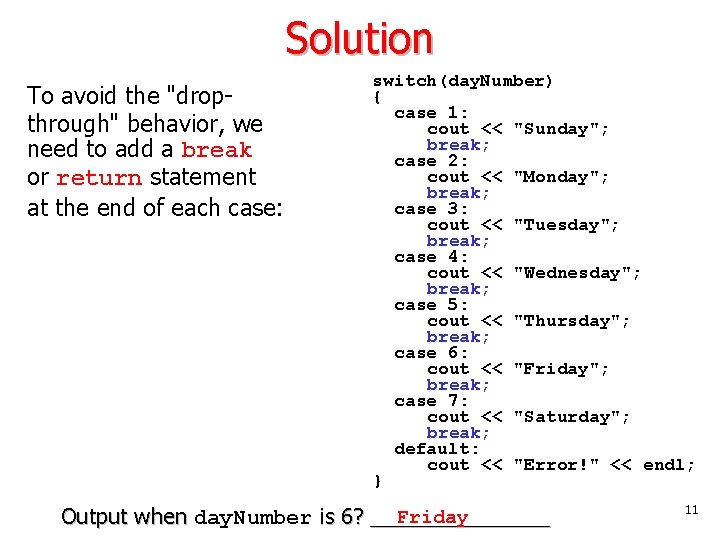
Solution To avoid the "dropthrough" behavior, we need to add a break or return statement at the end of each case: switch(day. Number) { case 1: cout << "Sunday"; break; case 2: cout << "Monday"; break; case 3: cout << "Tuesday"; break; case 4: cout << "Wednesday"; break; case 5: cout << "Thursday"; break; case 6: cout << "Friday"; break; case 7: cout << "Saturday"; break; default: cout << "Error!" << endl; } Friday Output when day. Number is 6? ________ 11
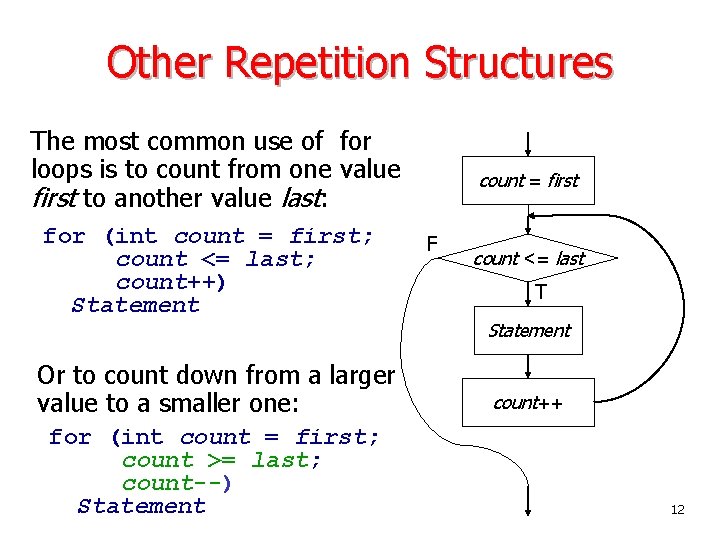
Other Repetition Structures The most common use of for loops is to count from one value first to another value last: for (int count = first; count <= last; count++) Statement Or to count down from a larger value to a smaller one: for (int count = first; count >= last; count--) Statement count = first F count <= last T Statement count++ 12
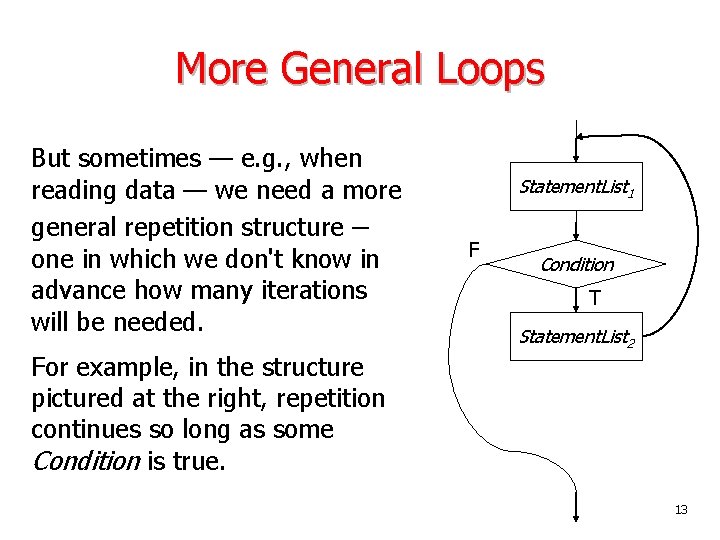
More General Loops But sometimes — e. g. , when reading data — we need a more general repetition structure one in which we don't know in advance how many iterations will be needed. For example, in the structure pictured at the right, repetition continues so long as some Condition is true. Statement. List 1 F Condition T Statement. List 2 13
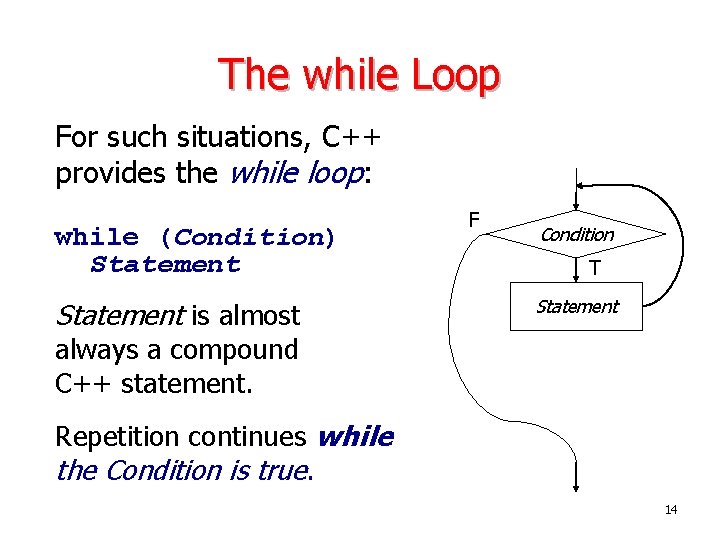
The while Loop For such situations, C++ provides the while loop: while (Condition) Statement is almost F Condition T Statement always a compound C++ statement. Repetition continues while the Condition is true. 14
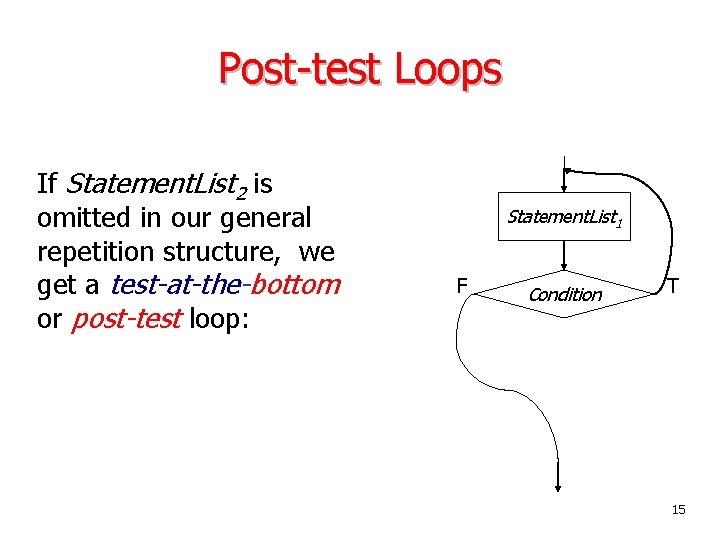
Post-test Loops If Statement. List 2 is omitted in our general repetition structure, we get a test-at-the-bottom or post-test loop: Statement. List 1 F Condition T 15
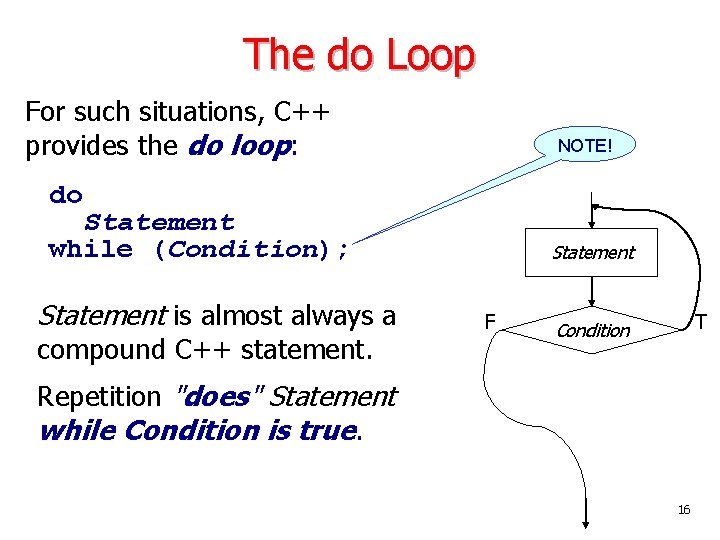
The do Loop For such situations, C++ provides the do loop: NOTE! do Statement while (Condition); Statement is almost always a compound C++ statement. Statement F T Condition Repetition "does" Statement while Condition is true. 16
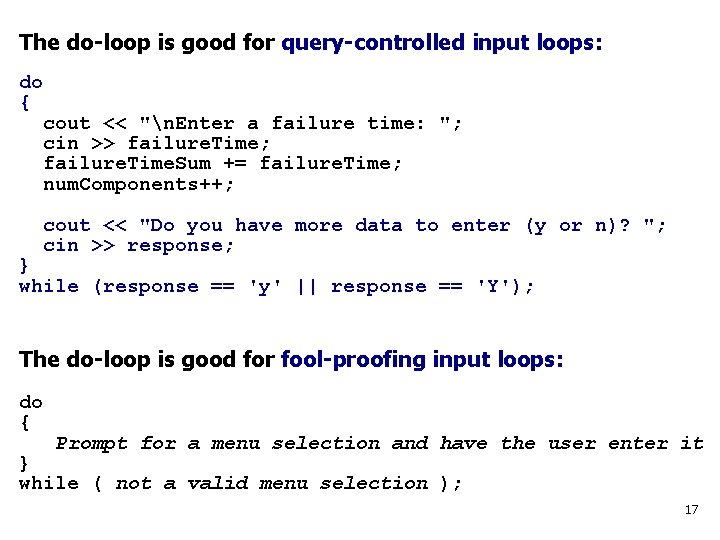
The do-loop is good for query-controlled input loops: do { cout << "n. Enter a failure time: "; cin >> failure. Time; failure. Time. Sum += failure. Time; num. Components++; cout << "Do you have more data to enter (y or n)? "; cin >> response; } while (response == 'y' || response == 'Y'); The do-loop is good for fool-proofing input loops: do { Prompt for a menu selection and have the user enter it } while ( not a valid menu selection ); 17
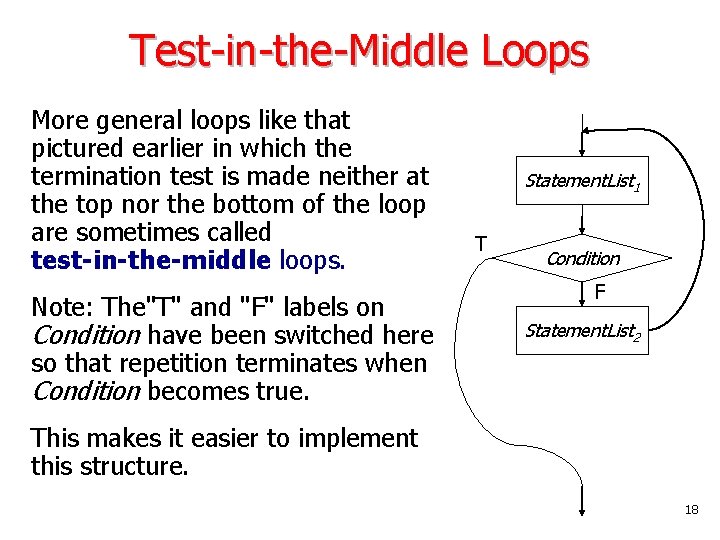
Test-in-the-Middle Loops More general loops like that pictured earlier in which the termination test is made neither at the top nor the bottom of the loop are sometimes called test-in-the-middle loops. Note: The"T" and "F" labels on Condition have been switched here so that repetition terminates when Condition becomes true. Statement. List 1 T Condition F Statement. List 2 This makes it easier to implement this structure. 18
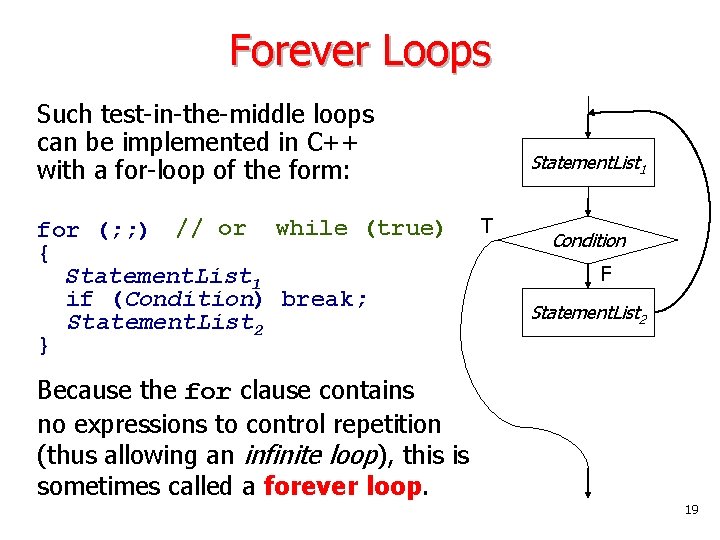
Forever Loops Such test-in-the-middle loops can be implemented in C++ with a for-loop of the form: for (; ; ) // or while (true) { Statement. List 1 if (Condition) break; Statement. List 2 } Statement. List 1 T Condition F Statement. List 2 Because the for clause contains no expressions to control repetition (thus allowing an infinite loop), this is sometimes called a forever loop. 19
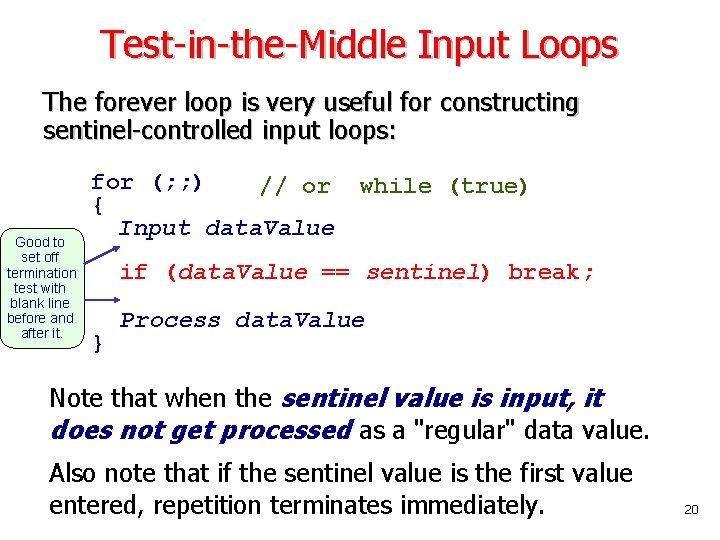
Test-in-the-Middle Input Loops The forever loop is very useful for constructing sentinel-controlled input loops: Good to set off termination test with blank line before and after it. for (; ; ) // or while (true) { Input data. Value if (data. Value == sentinel) break; } Process data. Value Note that when the sentinel value is input, it does not get processed as a "regular" data value. Also note that if the sentinel value is the first value entered, repetition terminates immediately. 20
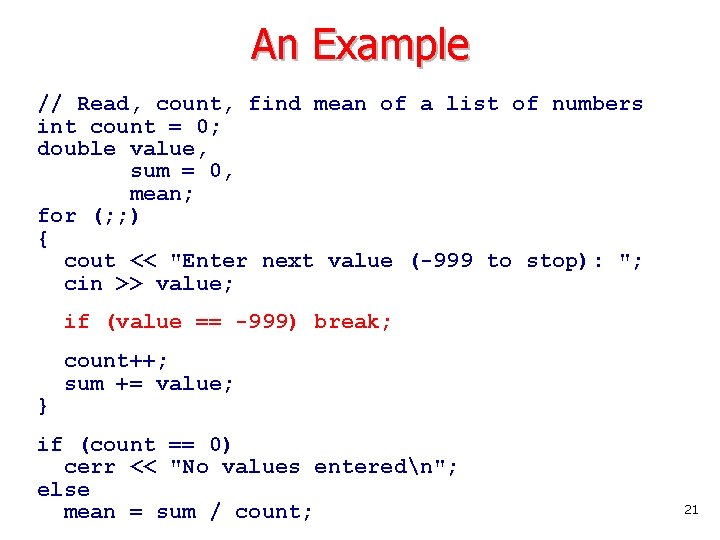
An Example // Read, count, find mean of a list of numbers int count = 0; double value, sum = 0, mean; for (; ; ) { cout << "Enter next value (-999 to stop): "; cin >> value; if (value == -999) break; count++; sum += value; } if (count == 0) cerr << "No values enteredn"; else mean = sum / count; 21
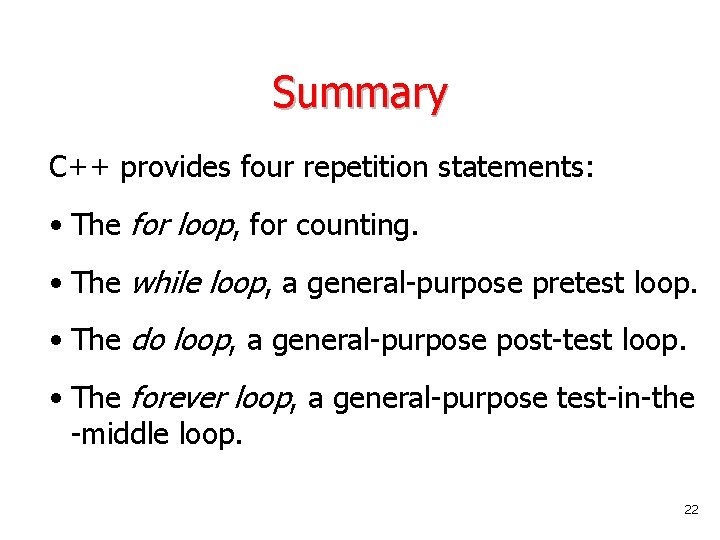
Summary C++ provides four repetition statements: • The for loop, for counting. • The while loop, a general-purpose pretest loop. • The do loop, a general-purpose post-test loop. • The forever loop, a general-purpose test-in-the -middle loop. 22
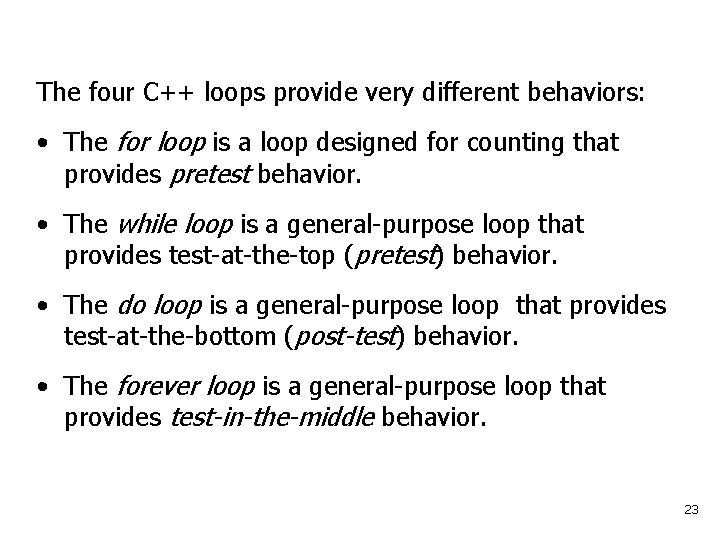
The four C++ loops provide very different behaviors: • The for loop is a loop designed for counting that provides pretest behavior. • The while loop is a general-purpose loop that provides test-at-the-top (pretest) behavior. • The do loop is a general-purpose loop that provides test-at-the-bottom (post-test) behavior. • The forever loop is a general-purpose loop that provides test-in-the-middle behavior. 23
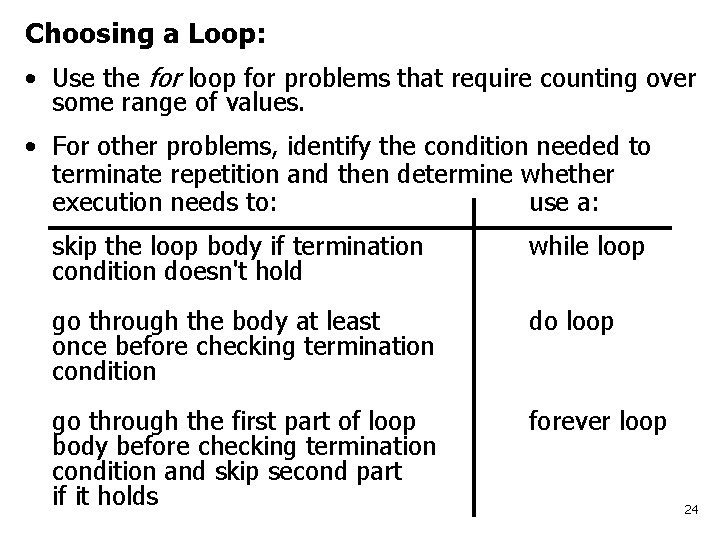
Choosing a Loop: • Use the for loop for problems that require counting over some range of values. • For other problems, identify the condition needed to terminate repetition and then determine whether execution needs to: use a: skip the loop body if termination condition doesn't hold while loop go through the body at least once before checking termination condition do loop go through the first part of loop body before checking termination condition and skip second part if it holds forever loop 24
More more more i want more more more more we praise you
More more more i want more more more more we praise you
Chap chap slide
In a banyan switch micro switch
Go switch 70 series
Homologous structures example
Chapter 25 spicy riddles
Human history becomes more and more a race
Hardware and control structures
Chapter 1 clothing
Passion chap 6
Bank run chap 11
Autocorrelation in econometrics
Kstn chap 18
Family control ch3
The origin of species webtoon
Satisfying needs 6
Types of evolution
Find the passage
If thy right hand offend thee
Chapter 1 learning about children
Rivalry 1 ch 6
System engineer chap 1
Chap tree
Tree switch