Merge Sorting Muhannad Alanazi 201502704 Hassan Alrashid 201502913
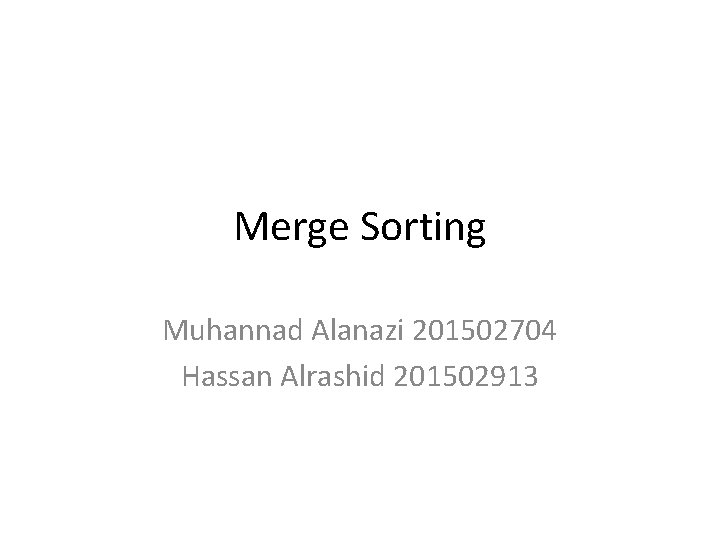
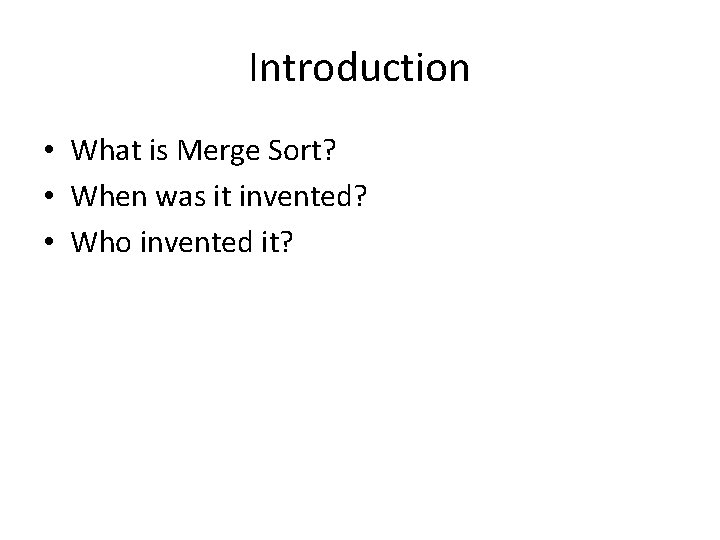
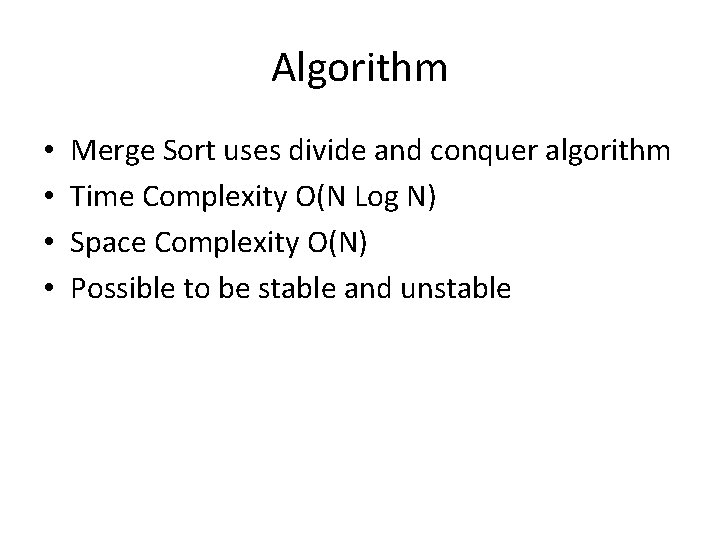
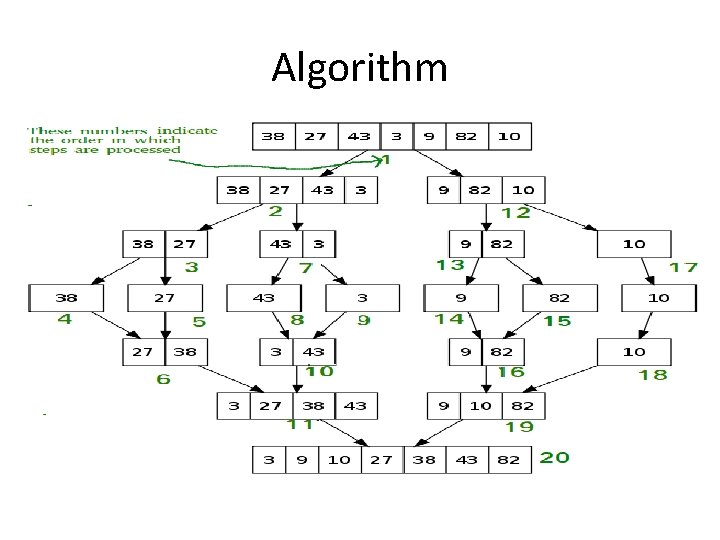
![Code public void merge. Sort (int[] list, int low. Index, int high. Index) { Code public void merge. Sort (int[] list, int low. Index, int high. Index) {](https://slidetodoc.com/presentation_image_h2/5d0240dae8f5ba0ab11fba2409587af7/image-5.jpg)
![Code // Merges two subarrays of arr[]. // First subarray is arr[l. . m] Code // Merges two subarrays of arr[]. // First subarray is arr[l. . m]](https://slidetodoc.com/presentation_image_h2/5d0240dae8f5ba0ab11fba2409587af7/image-6.jpg)
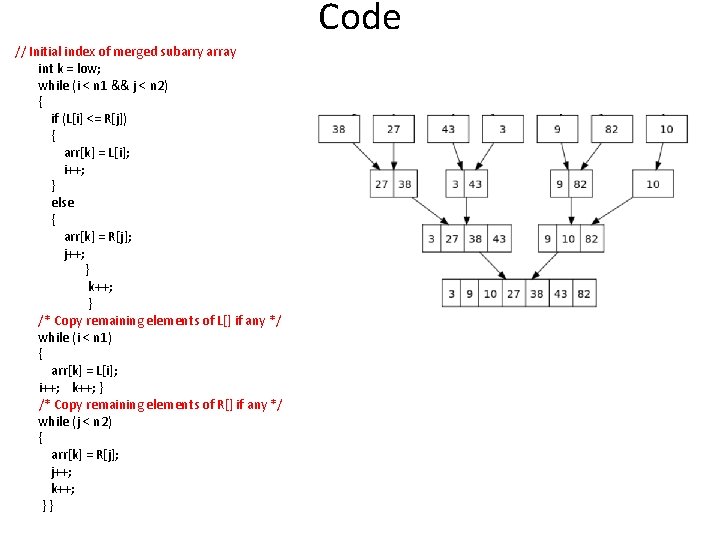
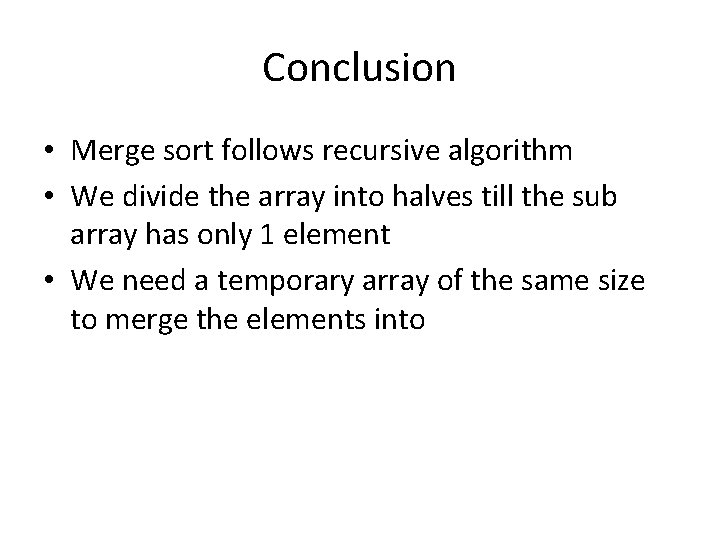
- Slides: 8
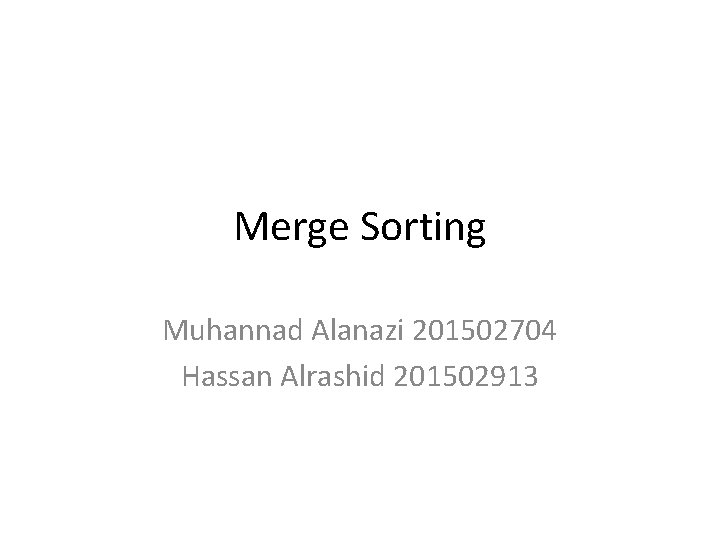
Merge Sorting Muhannad Alanazi 201502704 Hassan Alrashid 201502913
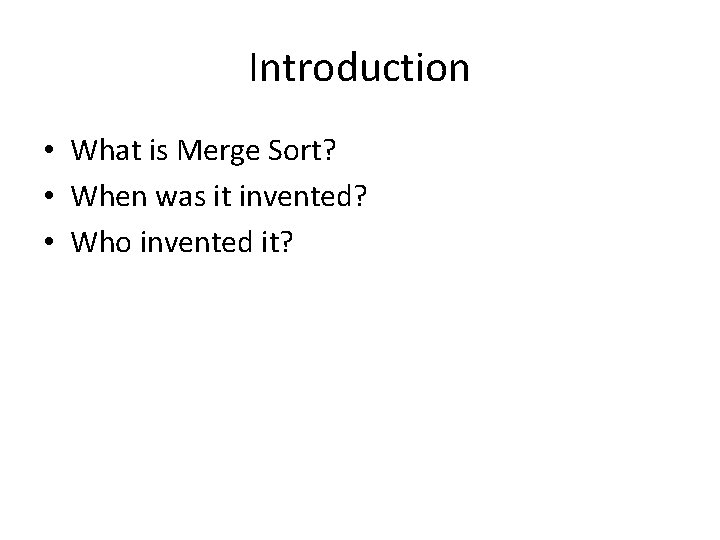
Introduction • What is Merge Sort? • When was it invented? • Who invented it?
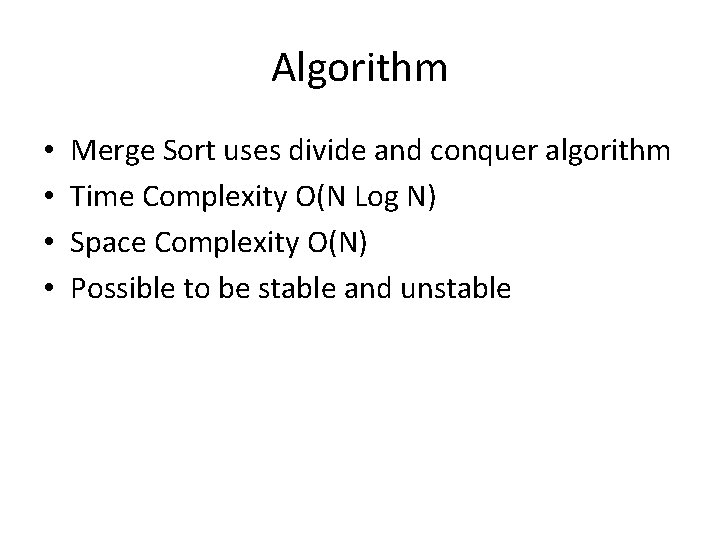
Algorithm • • Merge Sort uses divide and conquer algorithm Time Complexity O(N Log N) Space Complexity O(N) Possible to be stable and unstable
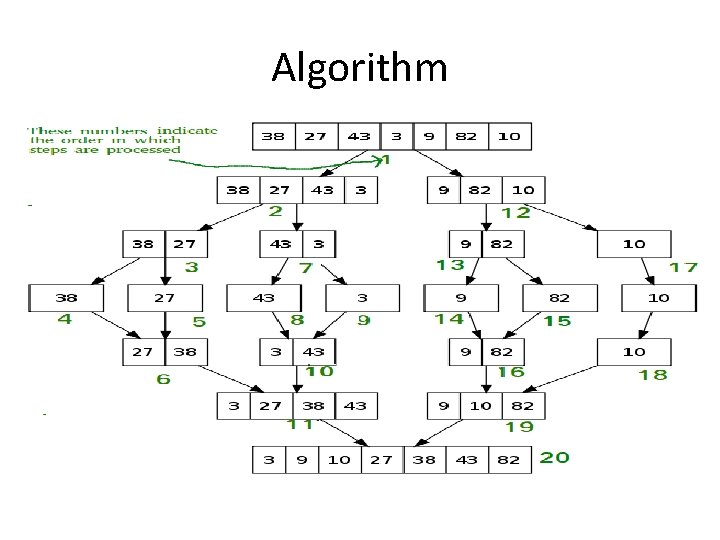
Algorithm
![Code public void merge Sort int list int low Index int high Index Code public void merge. Sort (int[] list, int low. Index, int high. Index) {](https://slidetodoc.com/presentation_image_h2/5d0240dae8f5ba0ab11fba2409587af7/image-5.jpg)
Code public void merge. Sort (int[] list, int low. Index, int high. Index) { if (low. Index == high. Index) return; else { int mid. Index = (low. Index + high. Index) / 2; merge. Sort(list, low. Index, mid. Index); merge. Sort(list, mid. Index + 1, high. Index); merge(list, low. Index, mid. Index, high. Index); } }
![Code Merges two subarrays of arr First subarray is arrl m Code // Merges two subarrays of arr[]. // First subarray is arr[l. . m]](https://slidetodoc.com/presentation_image_h2/5d0240dae8f5ba0ab11fba2409587af7/image-6.jpg)
Code // Merges two subarrays of arr[]. // First subarray is arr[l. . m] // Second subarray is arr[m+1. . r] void merge(int arr[], int low, int m, int high) { // Find sizes of two subarrays to be merged int n 1 = m - low + 1; int n 2 = high - m; /* Create temp arrays */ int L[] = new int [n 1]; int R[] = new int [n 2]; /*Copy data to temp arrays*/ for (int i=0; i<n 1; ++i) L[i] = arr[low + i]; for (int j=0; j<n 2; ++j) R[j] = arr[m + 1+ j]; /* Merge the temp arrays */ // Initial indexes of first and second subarrays int i = 0, j = 0;
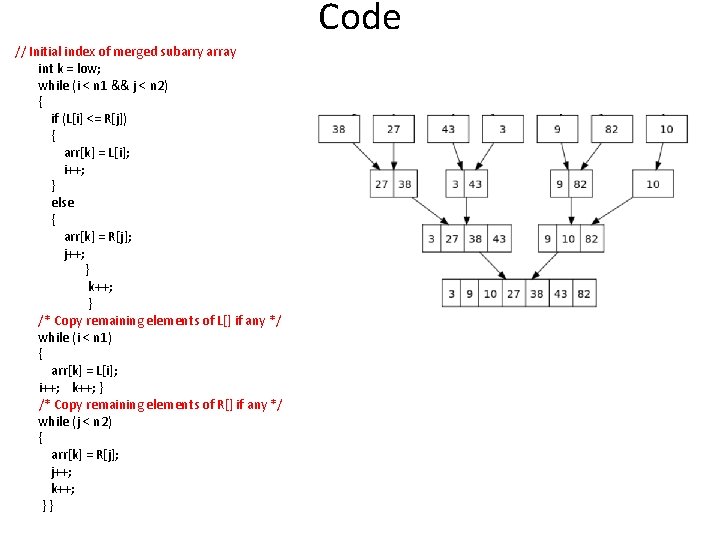
Code // Initial index of merged subarry array int k = low; while (i < n 1 && j < n 2) { if (L[i] <= R[j]) { arr[k] = L[i]; i++; } else { arr[k] = R[j]; j++; } k++; } /* Copy remaining elements of L[] if any */ while (i < n 1) { arr[k] = L[i]; i++; k++; } /* Copy remaining elements of R[] if any */ while (j < n 2) { arr[k] = R[j]; j++; k++; }}
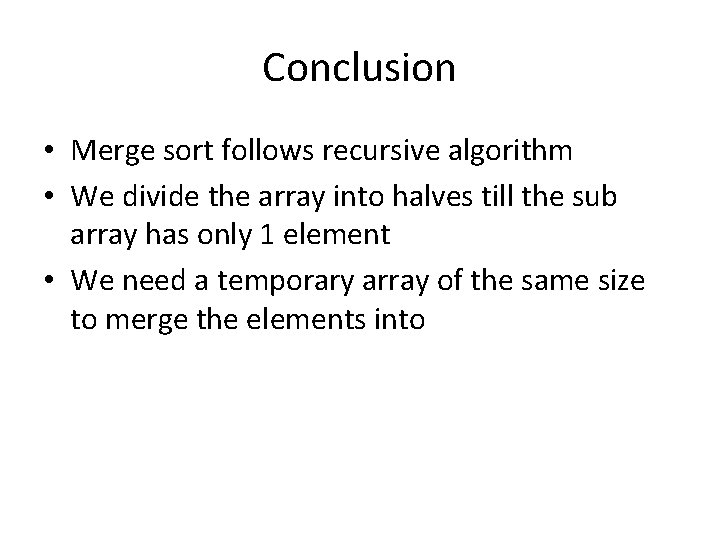
Conclusion • Merge sort follows recursive algorithm • We divide the array into halves till the sub array has only 1 element • We need a temporary array of the same size to merge the elements into