Lecture 7 Designing Classes CS 140 Dick Steflik
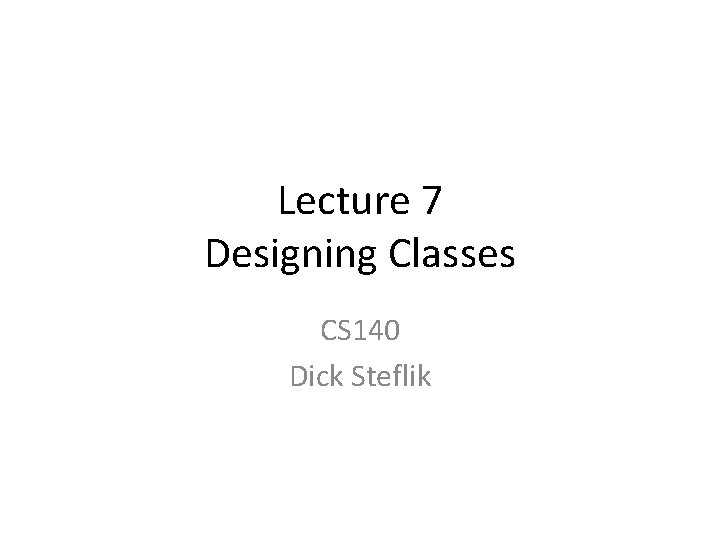
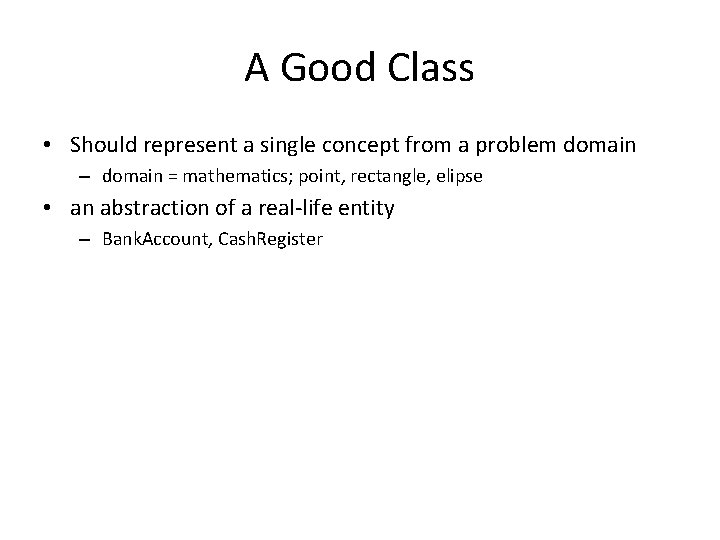
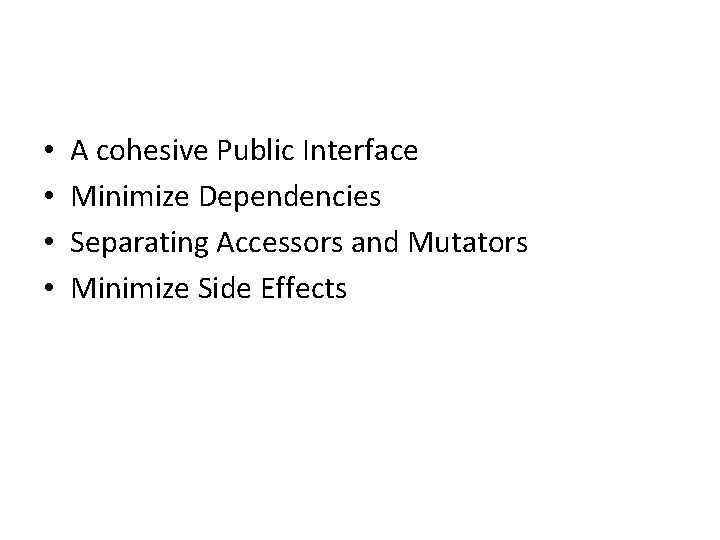
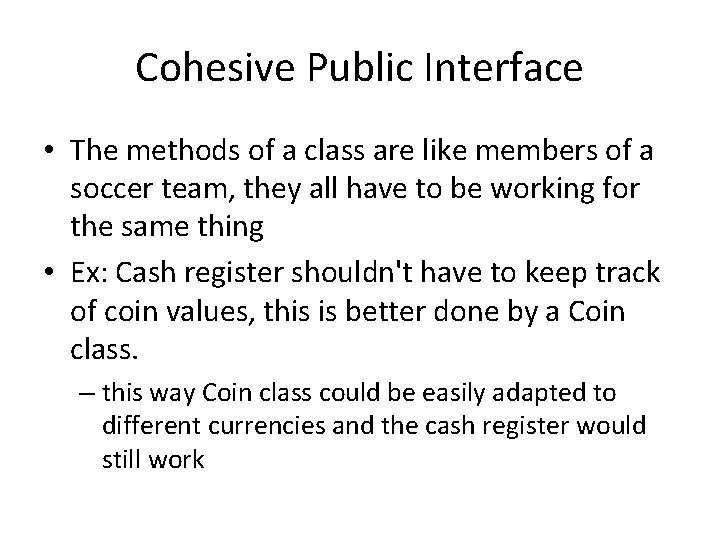
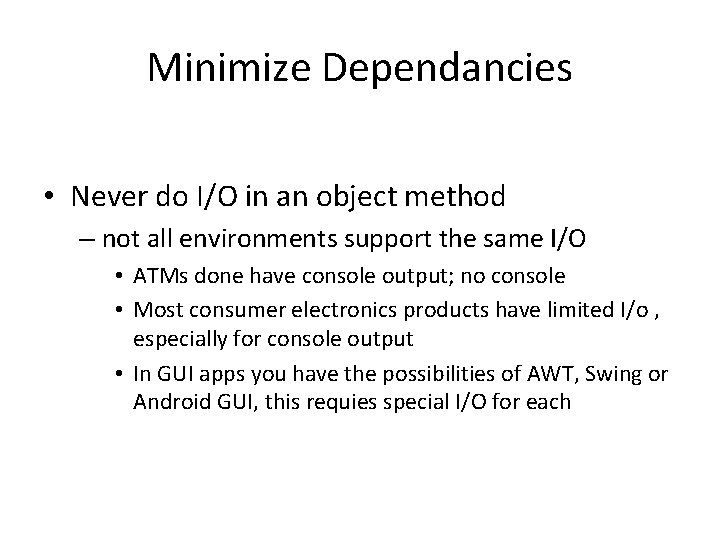
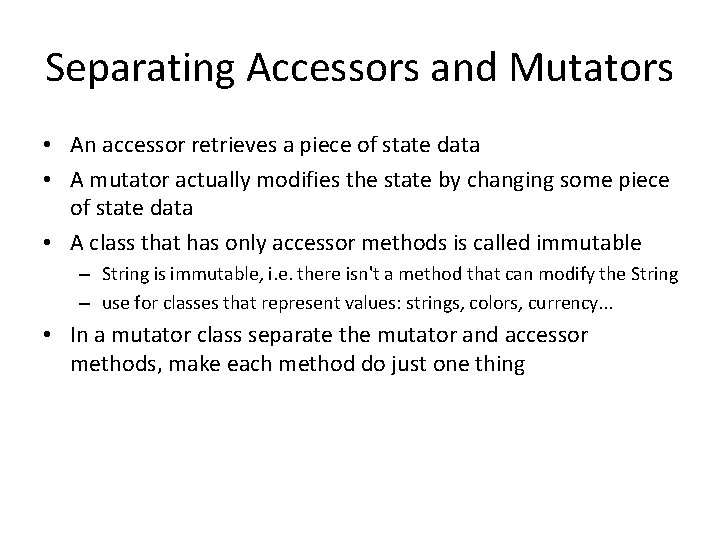
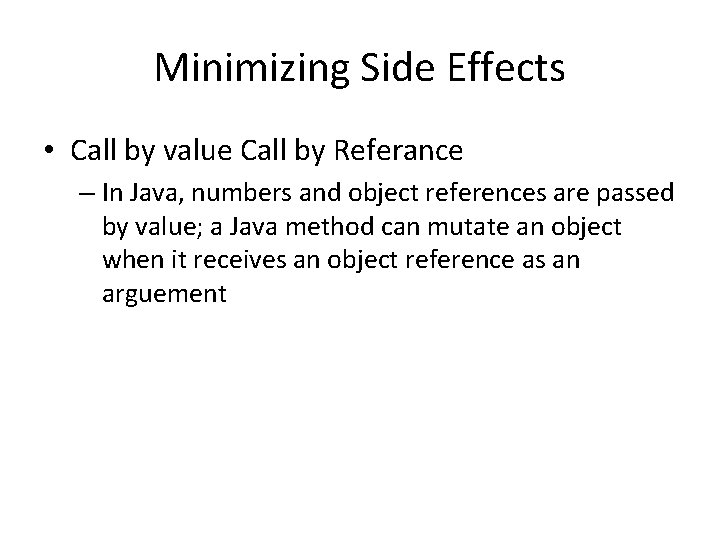
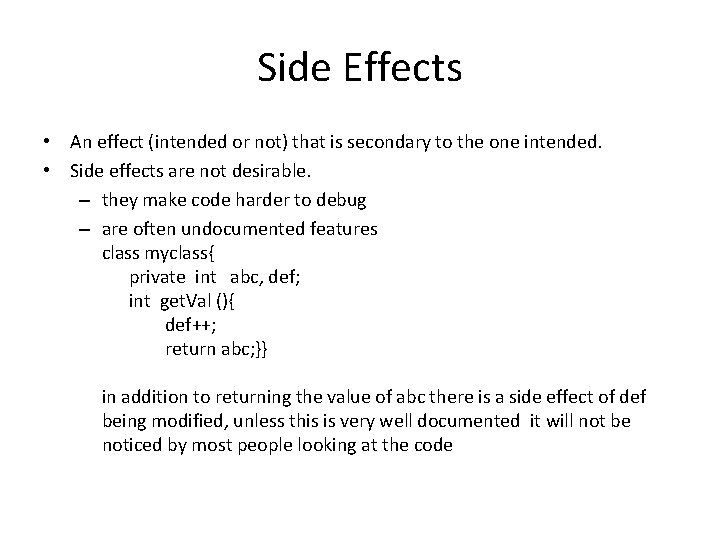
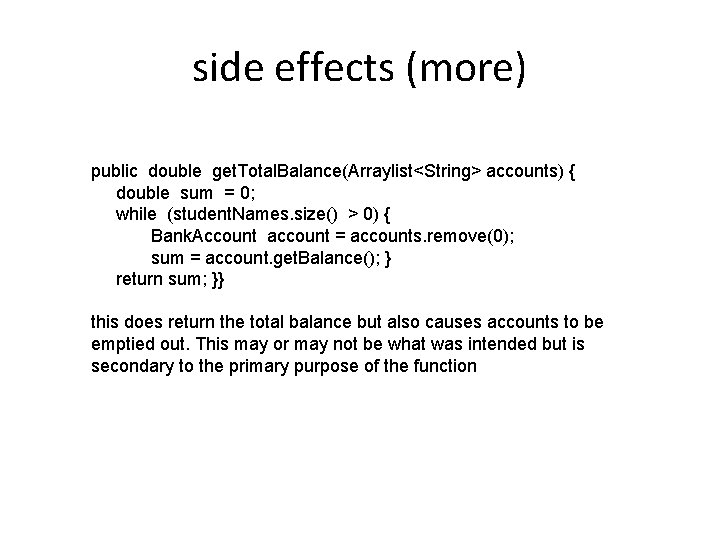
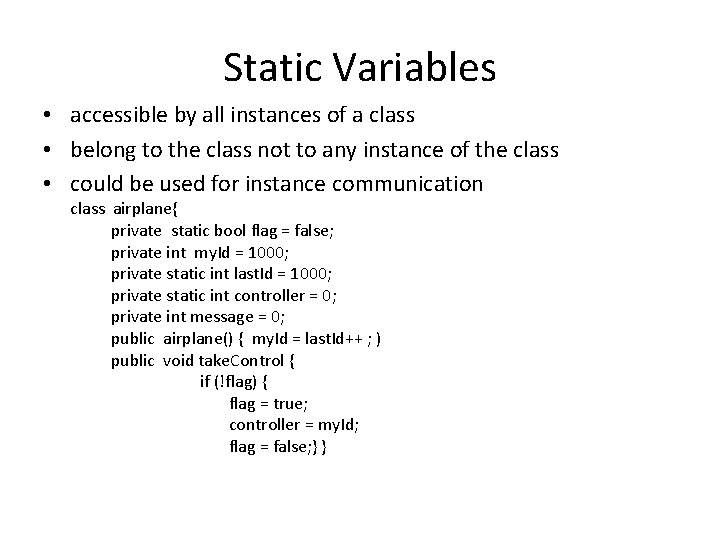
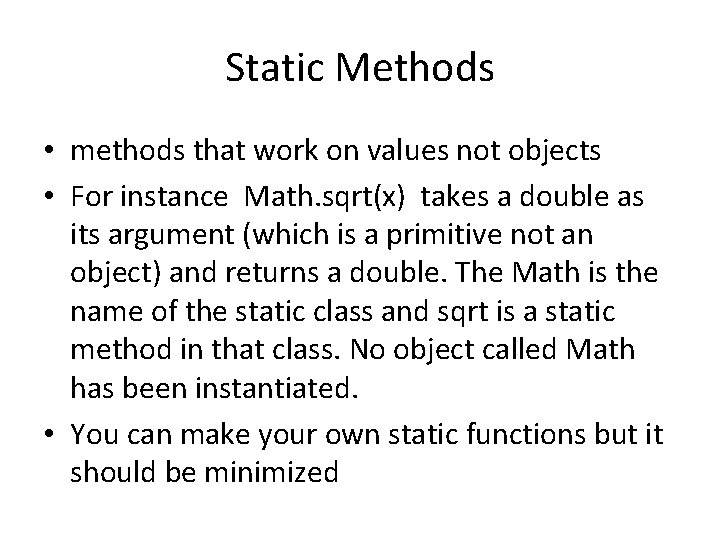
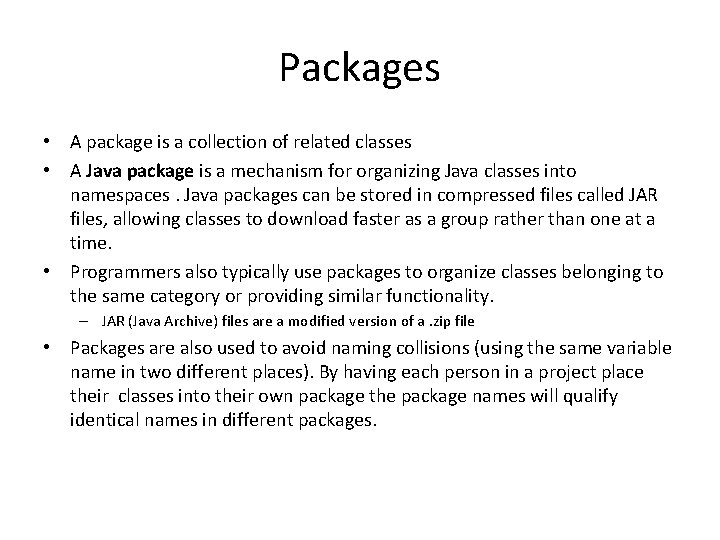
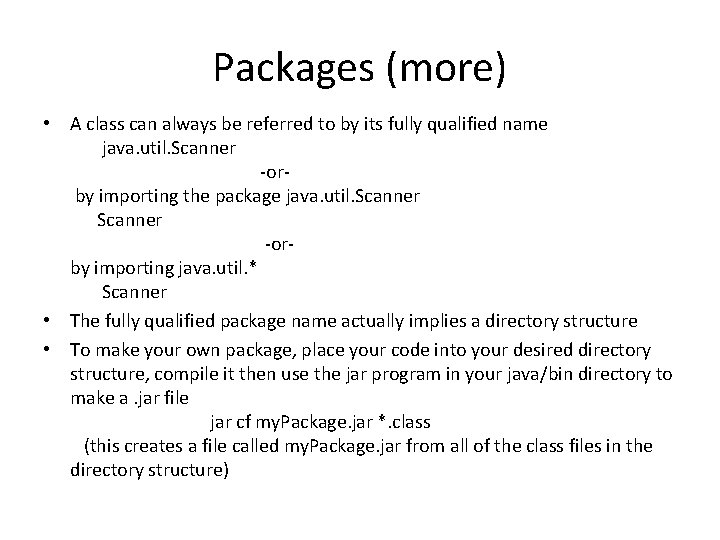
- Slides: 13
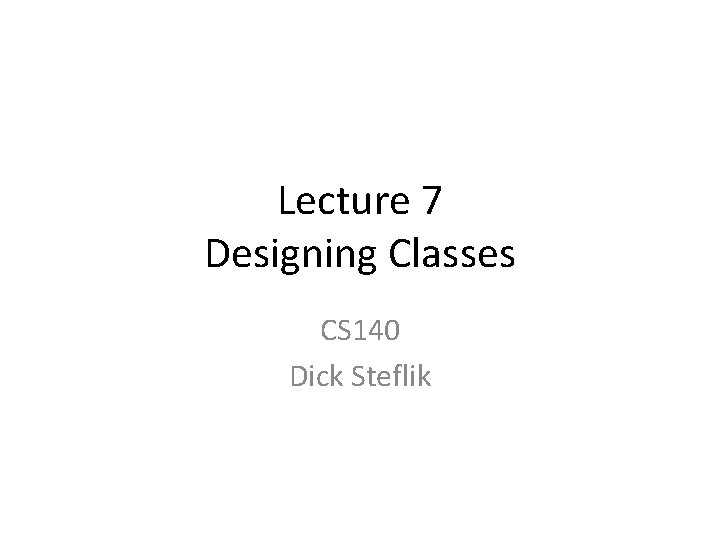
Lecture 7 Designing Classes CS 140 Dick Steflik
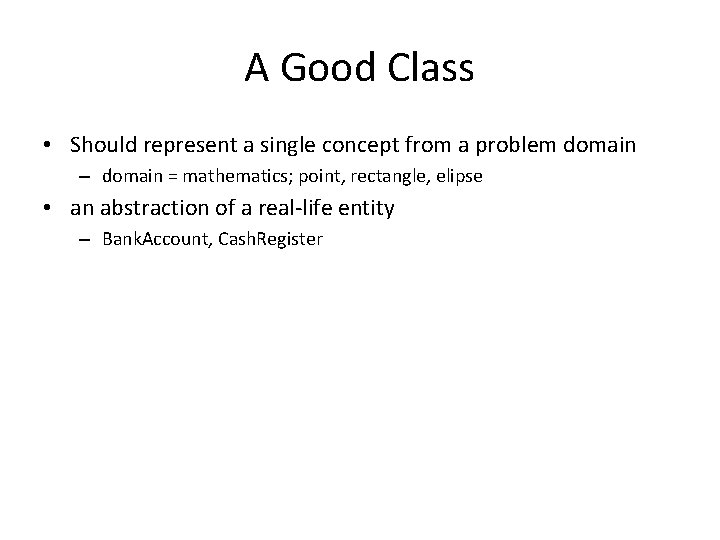
A Good Class • Should represent a single concept from a problem domain – domain = mathematics; point, rectangle, elipse • an abstraction of a real-life entity – Bank. Account, Cash. Register
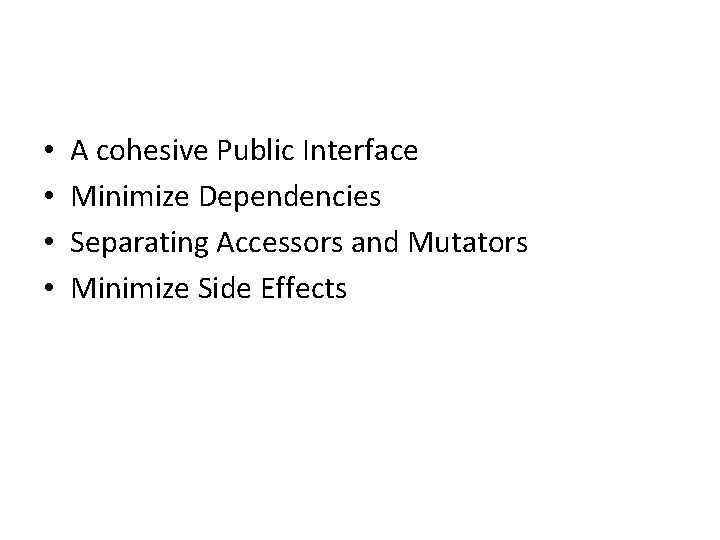
• • A cohesive Public Interface Minimize Dependencies Separating Accessors and Mutators Minimize Side Effects
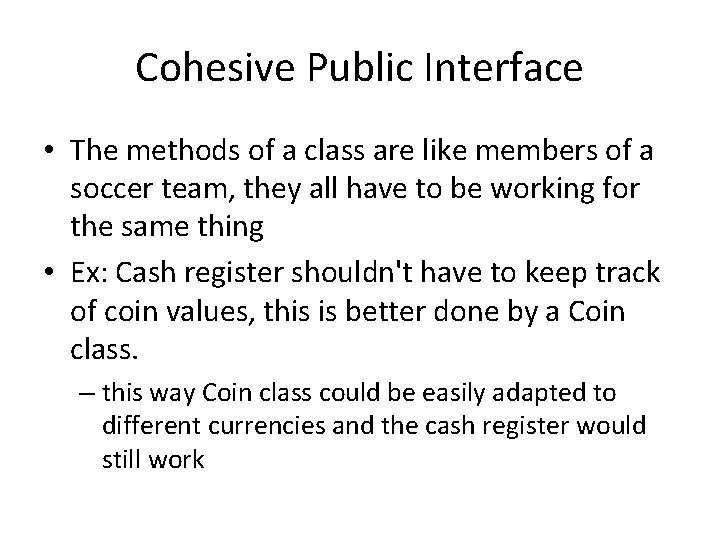
Cohesive Public Interface • The methods of a class are like members of a soccer team, they all have to be working for the same thing • Ex: Cash register shouldn't have to keep track of coin values, this is better done by a Coin class. – this way Coin class could be easily adapted to different currencies and the cash register would still work
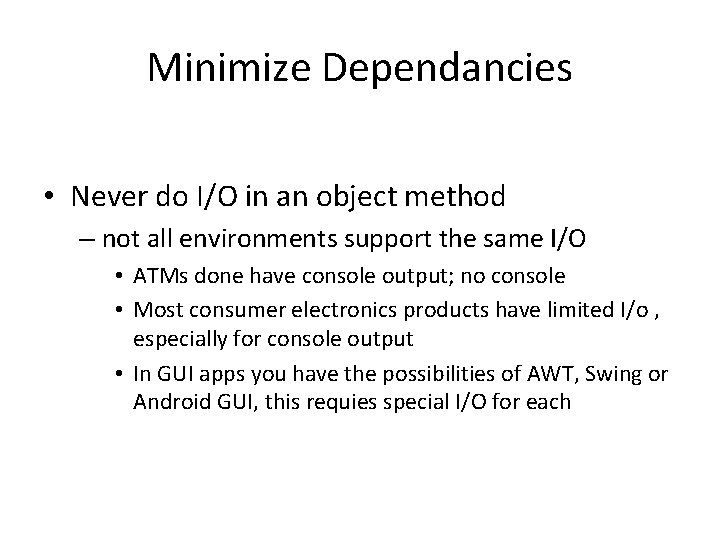
Minimize Dependancies • Never do I/O in an object method – not all environments support the same I/O • ATMs done have console output; no console • Most consumer electronics products have limited I/o , especially for console output • In GUI apps you have the possibilities of AWT, Swing or Android GUI, this requies special I/O for each
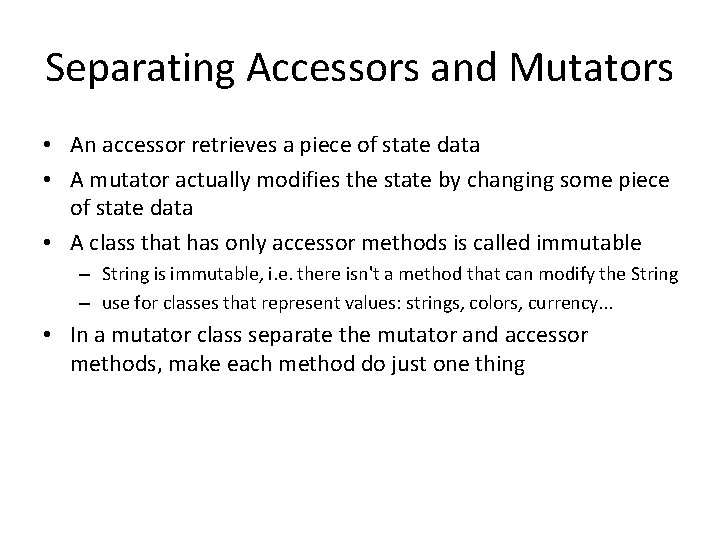
Separating Accessors and Mutators • An accessor retrieves a piece of state data • A mutator actually modifies the state by changing some piece of state data • A class that has only accessor methods is called immutable – String is immutable, i. e. there isn't a method that can modify the String – use for classes that represent values: strings, colors, currency. . . • In a mutator class separate the mutator and accessor methods, make each method do just one thing
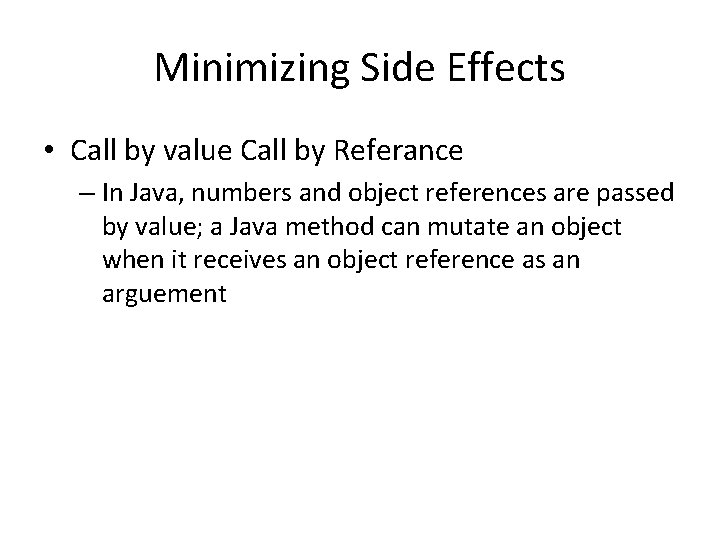
Minimizing Side Effects • Call by value Call by Referance – In Java, numbers and object references are passed by value; a Java method can mutate an object when it receives an object reference as an arguement
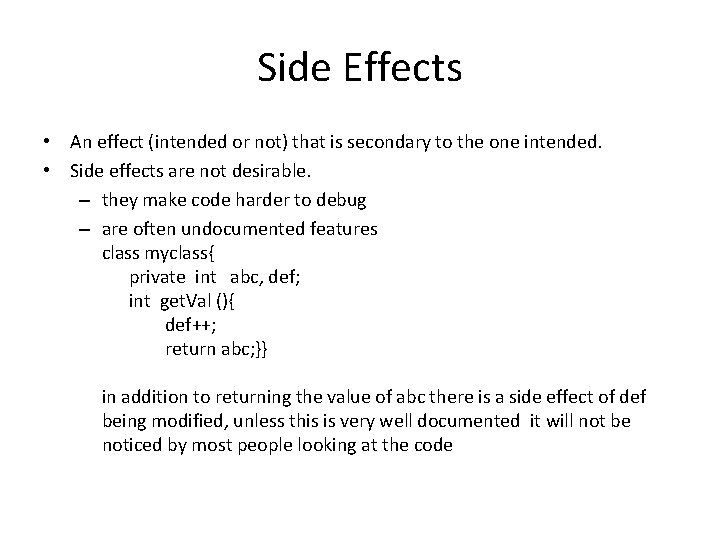
Side Effects • An effect (intended or not) that is secondary to the one intended. • Side effects are not desirable. – they make code harder to debug – are often undocumented features class myclass{ private int abc, def; int get. Val (){ def++; return abc; }} in addition to returning the value of abc there is a side effect of def being modified, unless this is very well documented it will not be noticed by most people looking at the code
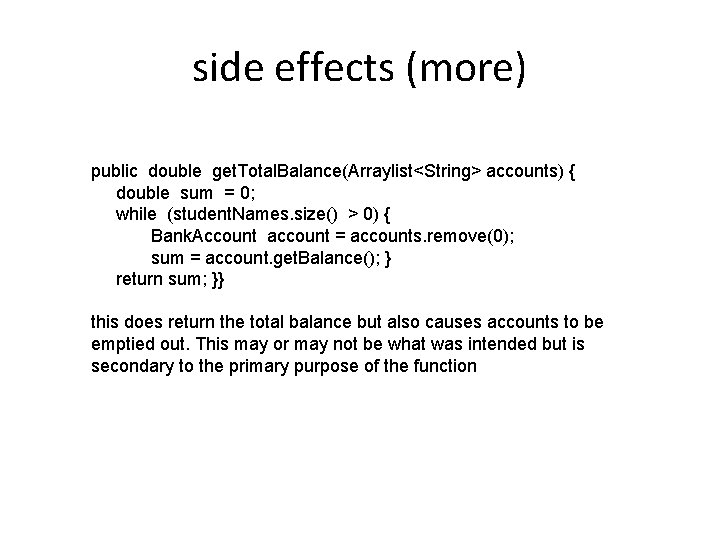
side effects (more) public double get. Total. Balance(Arraylist<String> accounts) { double sum = 0; while (student. Names. size() > 0) { Bank. Account account = accounts. remove(0); sum = account. get. Balance(); } return sum; }} this does return the total balance but also causes accounts to be emptied out. This may or may not be what was intended but is secondary to the primary purpose of the function
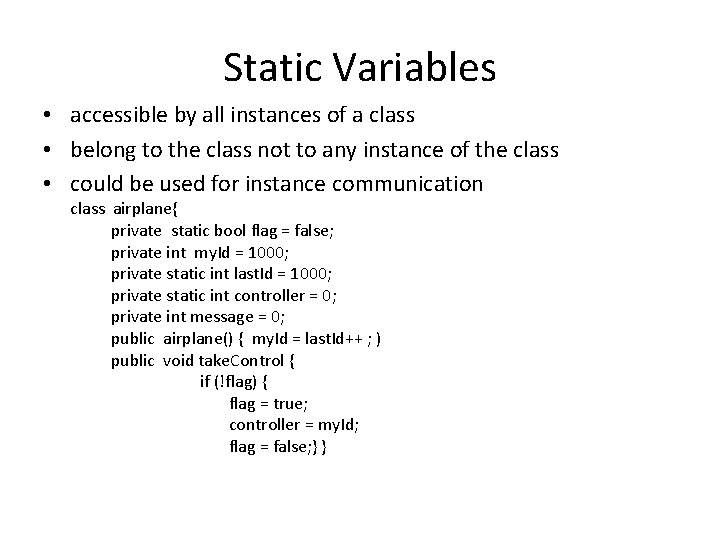
Static Variables • accessible by all instances of a class • belong to the class not to any instance of the class • could be used for instance communication class airplane{ private static bool flag = false; private int my. Id = 1000; private static int last. Id = 1000; private static int controller = 0; private int message = 0; public airplane() { my. Id = last. Id++ ; ) public void take. Control { if (!flag) { flag = true; controller = my. Id; flag = false; } }
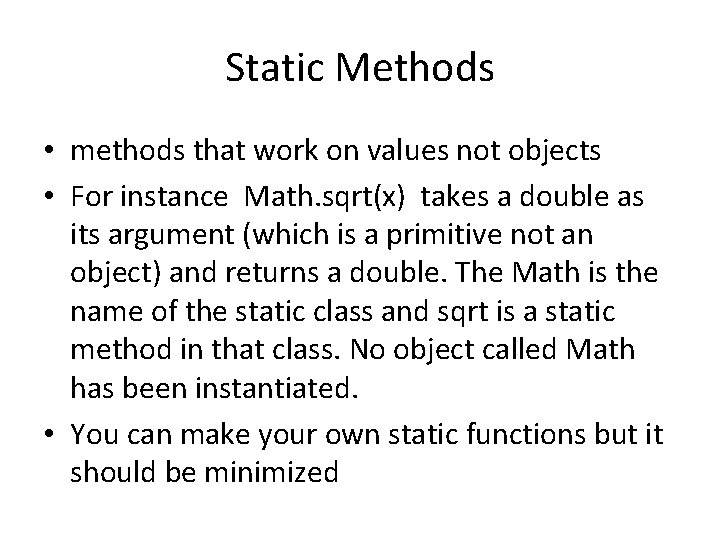
Static Methods • methods that work on values not objects • For instance Math. sqrt(x) takes a double as its argument (which is a primitive not an object) and returns a double. The Math is the name of the static class and sqrt is a static method in that class. No object called Math has been instantiated. • You can make your own static functions but it should be minimized
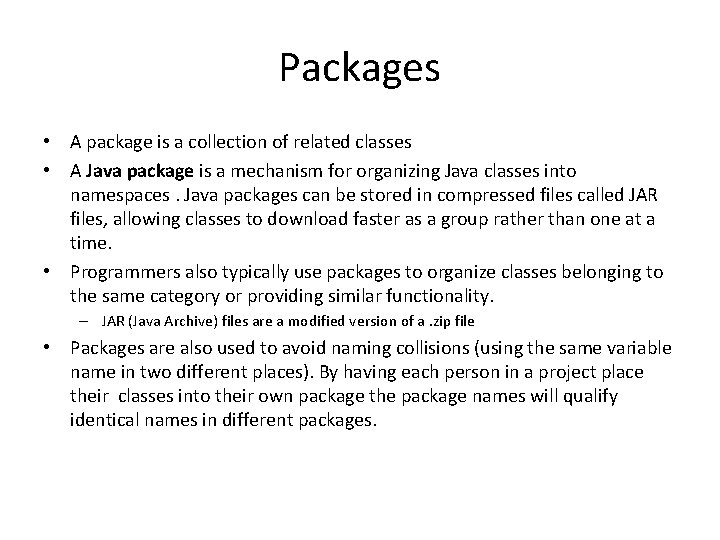
Packages • A package is a collection of related classes • A Java package is a mechanism for organizing Java classes into namespaces. Java packages can be stored in compressed files called JAR files, allowing classes to download faster as a group rather than one at a time. • Programmers also typically use packages to organize classes belonging to the same category or providing similar functionality. – JAR (Java Archive) files are a modified version of a. zip file • Packages are also used to avoid naming collisions (using the same variable name in two different places). By having each person in a project place their classes into their own package the package names will qualify identical names in different packages.
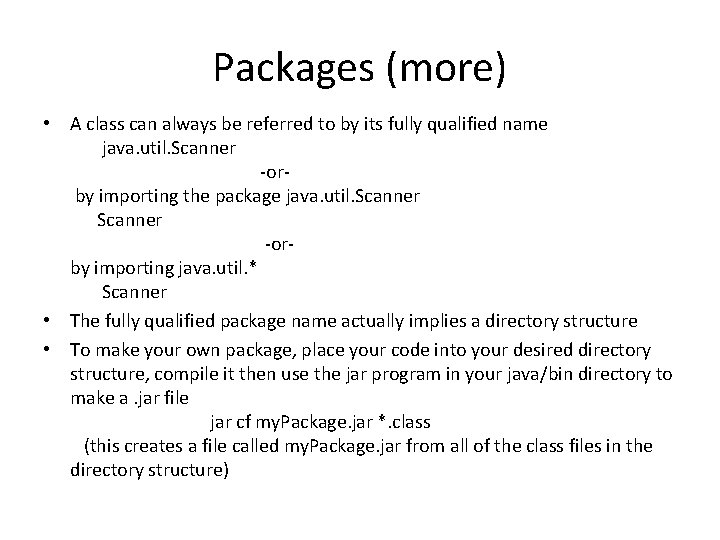
Packages (more) • A class can always be referred to by its fully qualified name java. util. Scanner -orby importing the package java. util. Scanner -orby importing java. util. * Scanner • The fully qualified package name actually implies a directory structure • To make your own package, place your code into your desired directory structure, compile it then use the jar program in your java/bin directory to make a. jar file jar cf my. Package. jar *. class (this creates a file called my. Package. jar from all of the class files in the directory structure)
Mary poppins robert stevenson
Solidworks
Classe e subclasse do que
Pre ap classes vs regular classes
01:640:244 lecture notes - lecture 15: plat, idah, farad
Dick kullendal
Dick houtman
Paraphrase the following sentences.
Dick cramer
Dick rasenberg
Nm interlock indigent fund
Api plc
Design model
Sigmar polke bunnies