Events and Event Handling CS 140 Dick Steflik
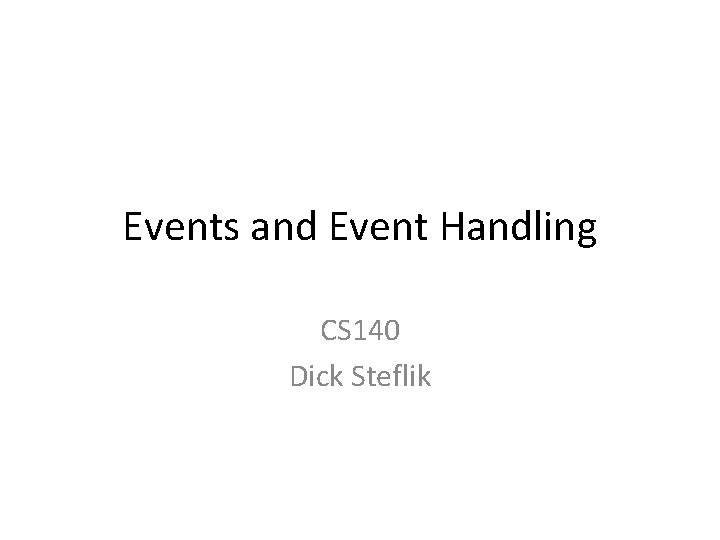
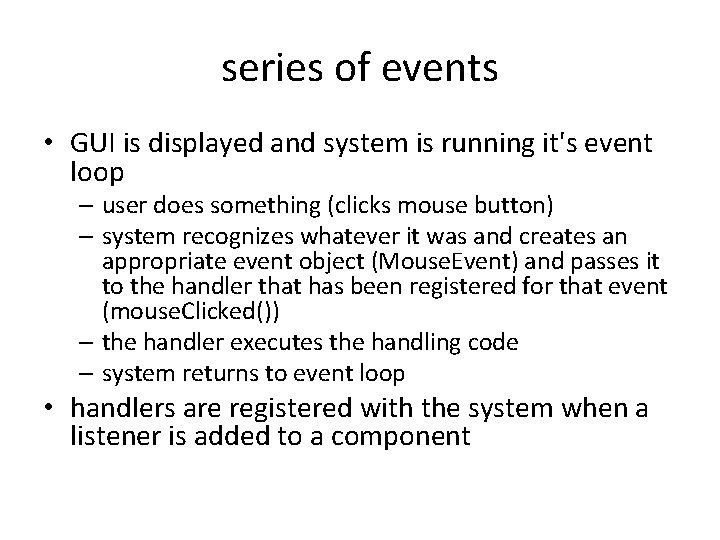
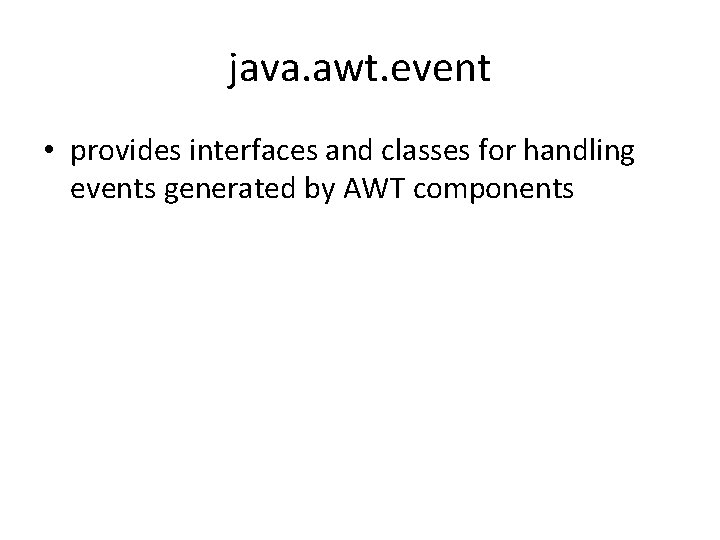
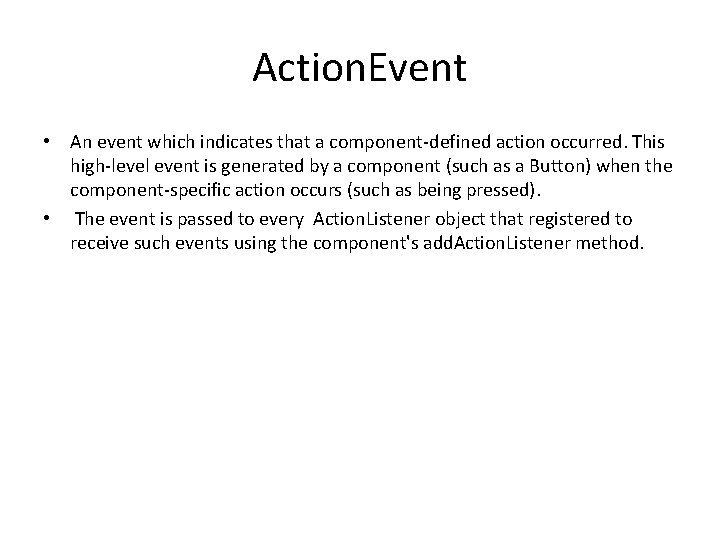
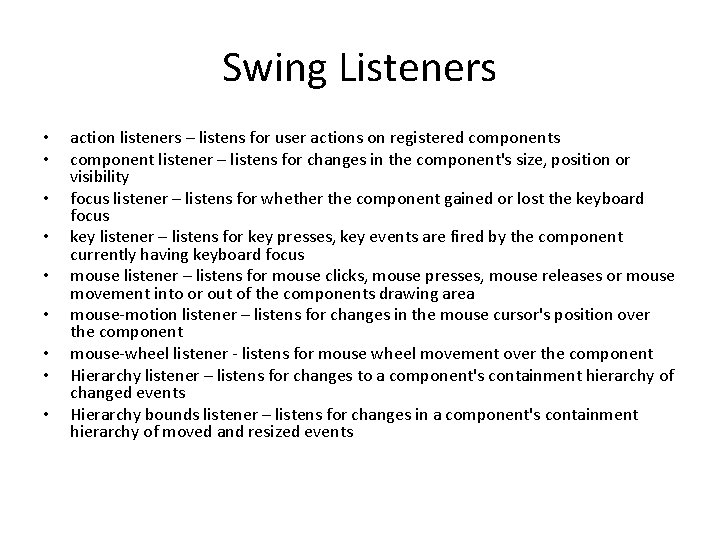
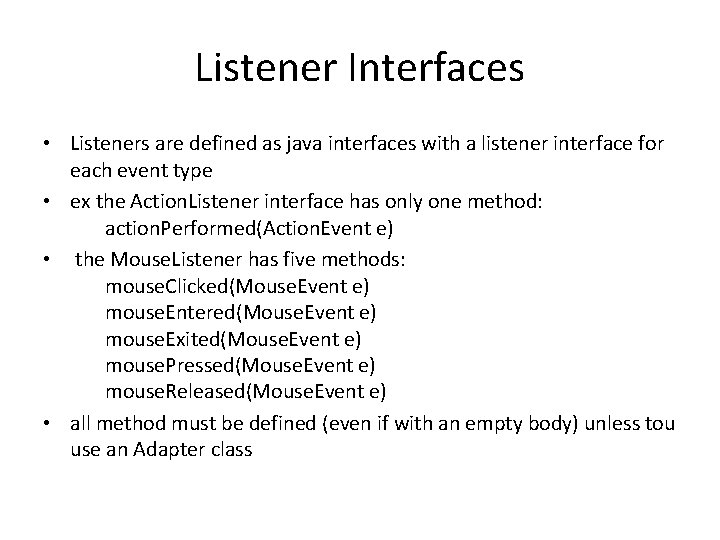
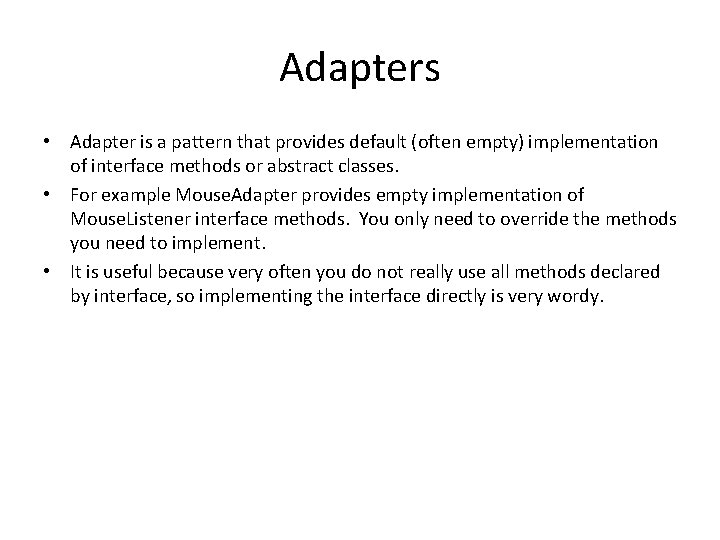
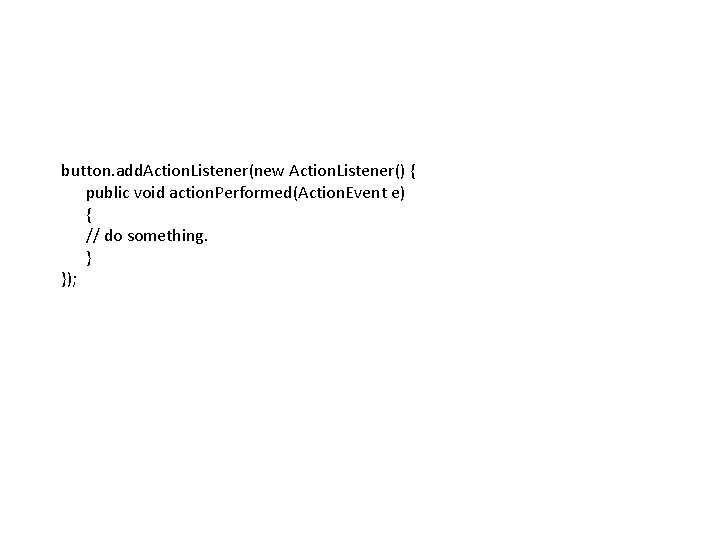
- Slides: 8
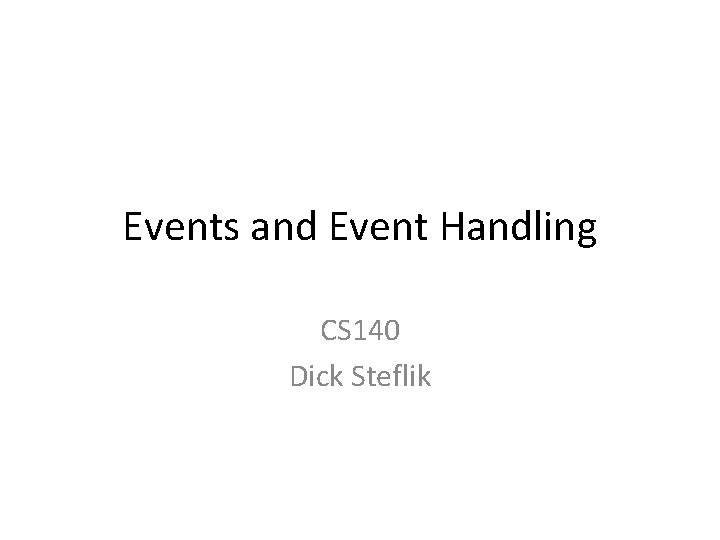
Events and Event Handling CS 140 Dick Steflik
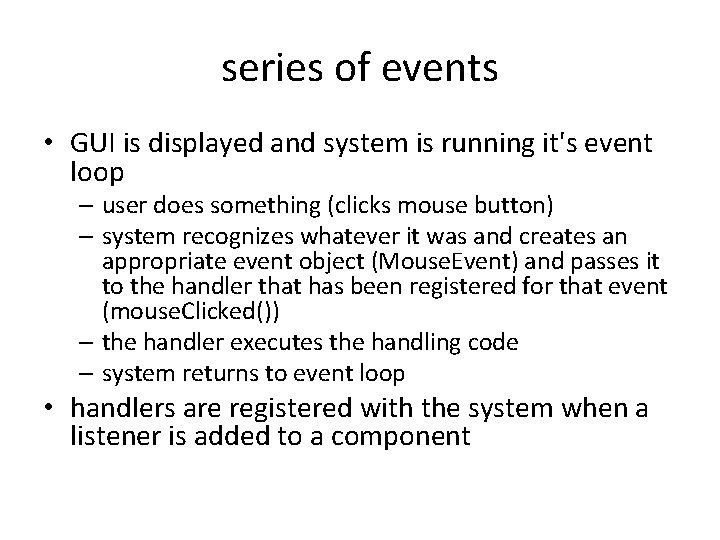
series of events • GUI is displayed and system is running it's event loop – user does something (clicks mouse button) – system recognizes whatever it was and creates an appropriate event object (Mouse. Event) and passes it to the handler that has been registered for that event (mouse. Clicked()) – the handler executes the handling code – system returns to event loop • handlers are registered with the system when a listener is added to a component
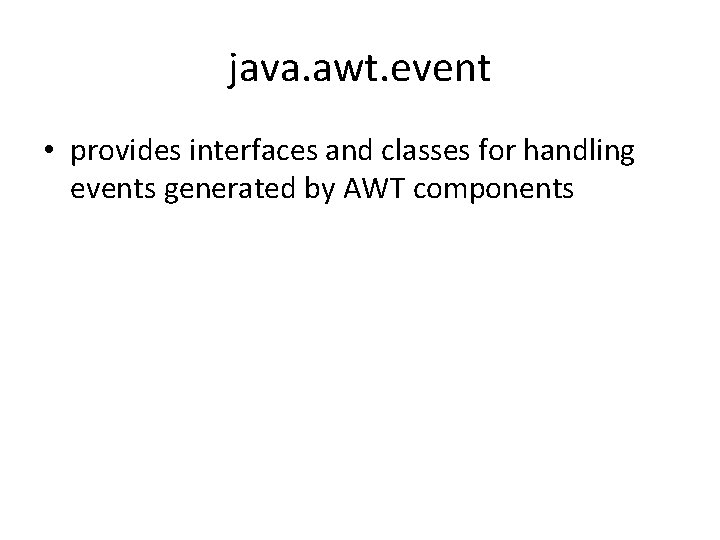
java. awt. event • provides interfaces and classes for handling events generated by AWT components
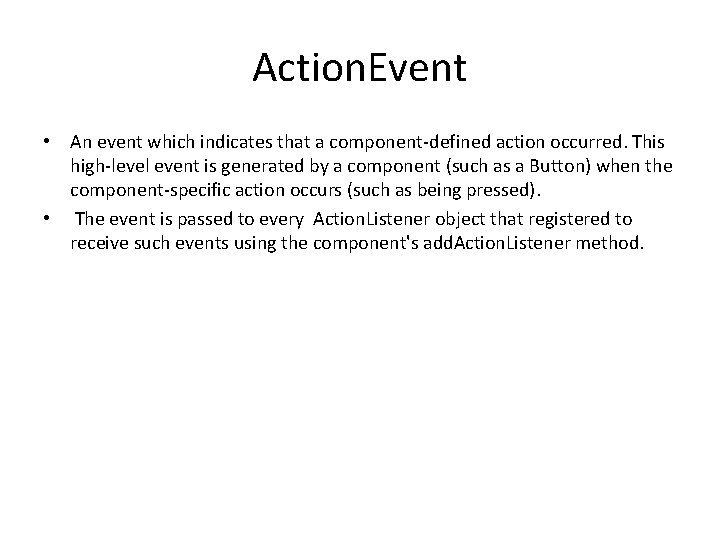
Action. Event • An event which indicates that a component-defined action occurred. This high-level event is generated by a component (such as a Button) when the component-specific action occurs (such as being pressed). • The event is passed to every Action. Listener object that registered to receive such events using the component's add. Action. Listener method.
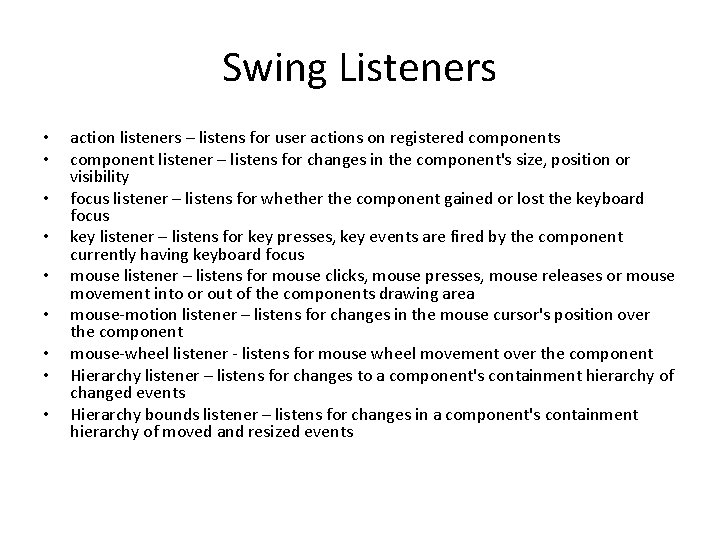
Swing Listeners • • • action listeners – listens for user actions on registered components component listener – listens for changes in the component's size, position or visibility focus listener – listens for whether the component gained or lost the keyboard focus key listener – listens for key presses, key events are fired by the component currently having keyboard focus mouse listener – listens for mouse clicks, mouse presses, mouse releases or mouse movement into or out of the components drawing area mouse-motion listener – listens for changes in the mouse cursor's position over the component mouse-wheel listener - listens for mouse wheel movement over the component Hierarchy listener – listens for changes to a component's containment hierarchy of changed events Hierarchy bounds listener – listens for changes in a component's containment hierarchy of moved and resized events
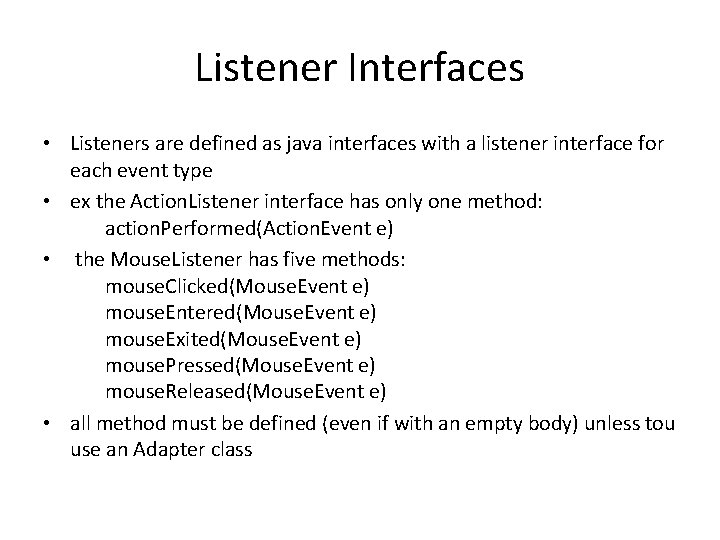
Listener Interfaces • Listeners are defined as java interfaces with a listener interface for each event type • ex the Action. Listener interface has only one method: action. Performed(Action. Event e) • the Mouse. Listener has five methods: mouse. Clicked(Mouse. Event e) mouse. Entered(Mouse. Event e) mouse. Exited(Mouse. Event e) mouse. Pressed(Mouse. Event e) mouse. Released(Mouse. Event e) • all method must be defined (even if with an empty body) unless tou use an Adapter class
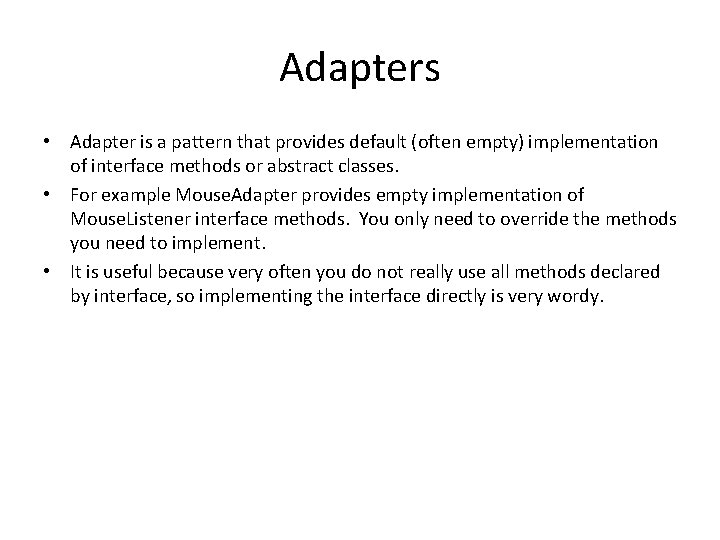
Adapters • Adapter is a pattern that provides default (often empty) implementation of interface methods or abstract classes. • For example Mouse. Adapter provides empty implementation of Mouse. Listener interface methods. You only need to override the methods you need to implement. • It is useful because very often you do not really use all methods declared by interface, so implementing the interface directly is very wordy.
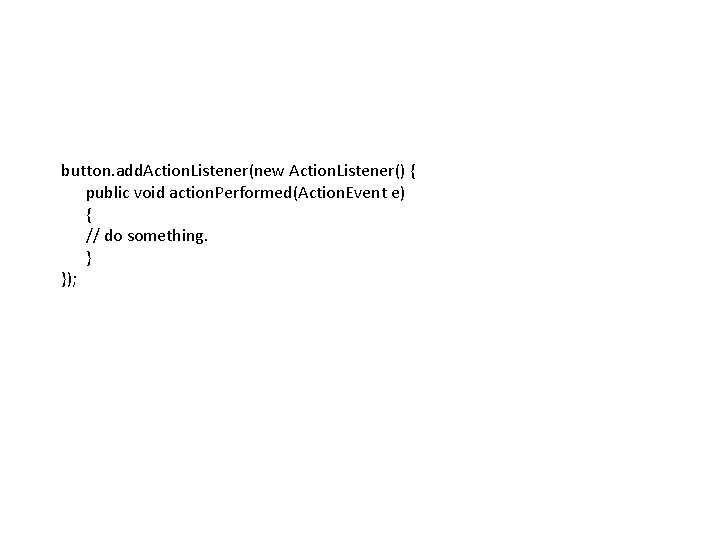
button. add. Action. Listener(new Action. Listener() { public void action. Performed(Action. Event e) { // do something. } });