Lecture 13 Heaps Container Adapters priorityqueue impl with
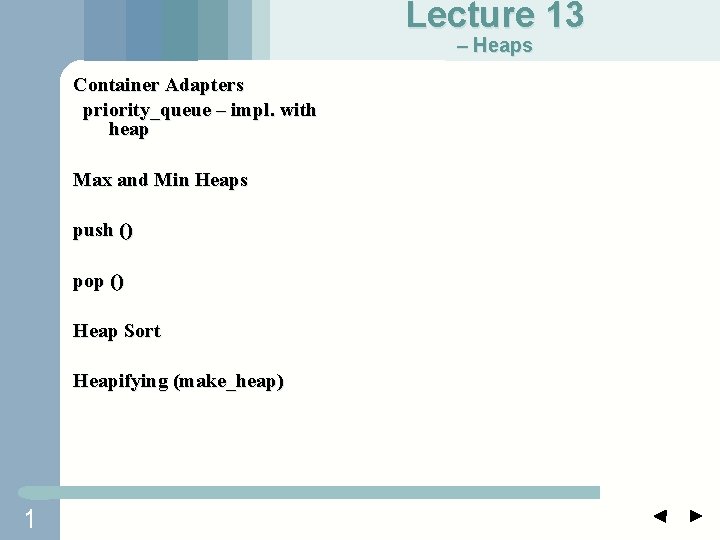
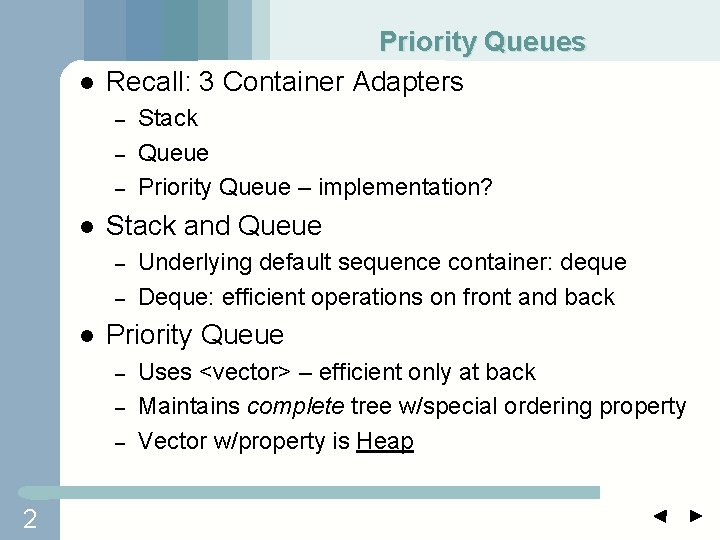
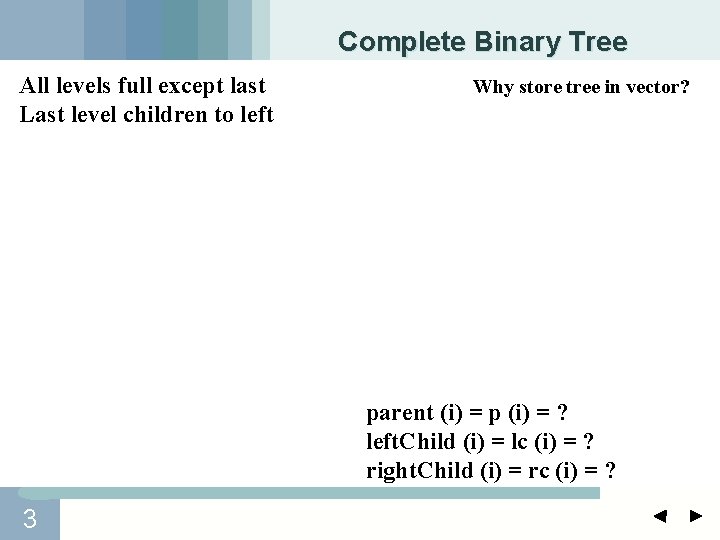
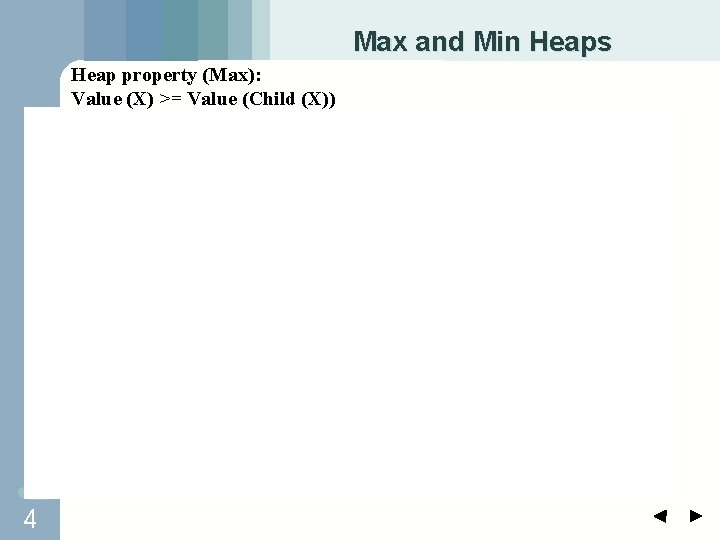
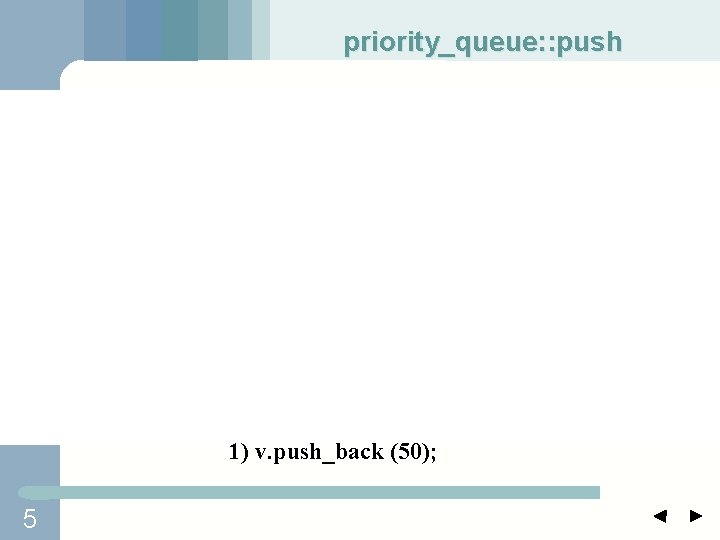
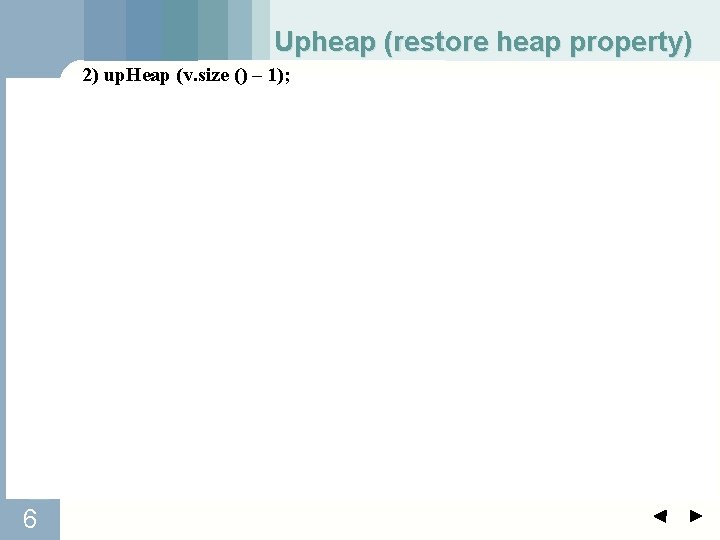
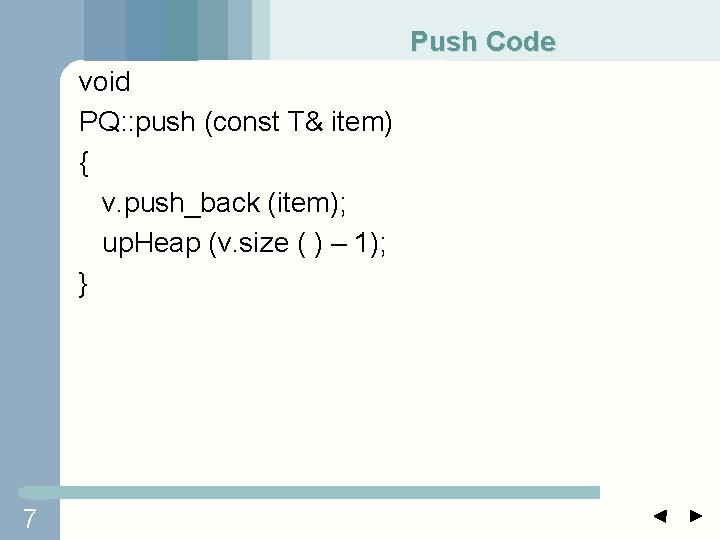
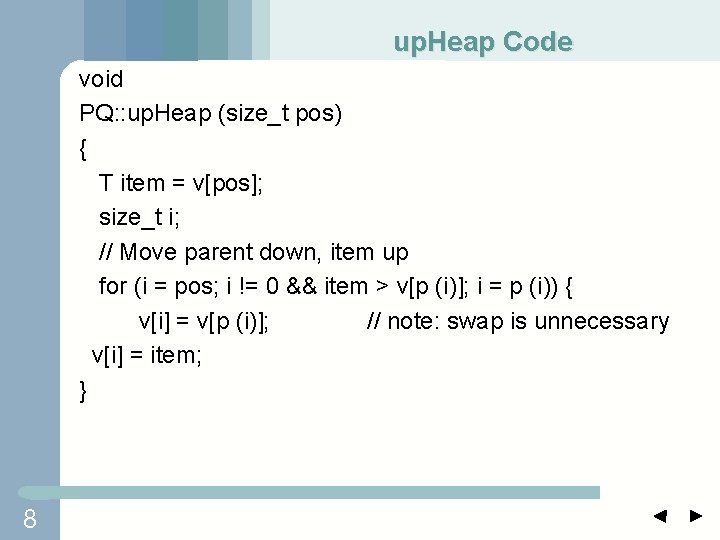
![priority_queue: : pop 18 v[0] = v. back (); v. pop_back (); 9 priority_queue: : pop 18 v[0] = v. back (); v. pop_back (); 9](https://slidetodoc.com/presentation_image/3cfed5468249bcffecbf8c66ea27a40b/image-9.jpg)
![Downheap Compare v[i] with max child Move max child up if necessary 10 Downheap Compare v[i] with max child Move max child up if necessary 10](https://slidetodoc.com/presentation_image/3cfed5468249bcffecbf8c66ea27a40b/image-10.jpg)
![Pop void PQ: : pop () { // Overwrite top element with last v[0] Pop void PQ: : pop () { // Overwrite top element with last v[0]](https://slidetodoc.com/presentation_image/3cfed5468249bcffecbf8c66ea27a40b/image-11.jpg)
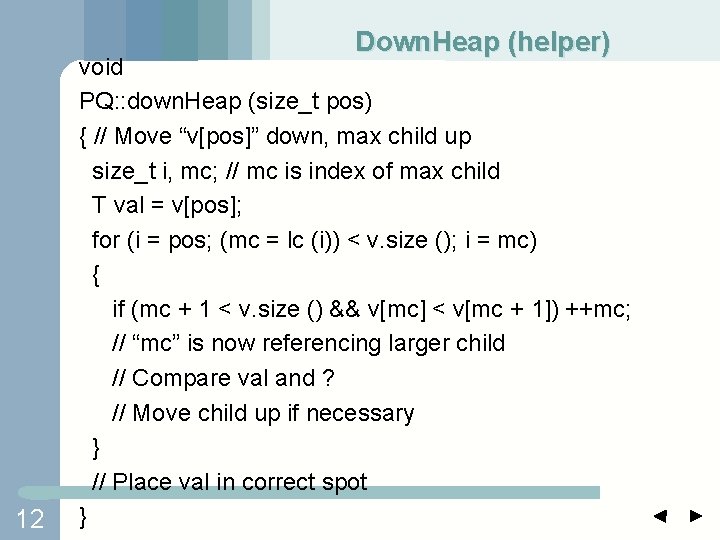
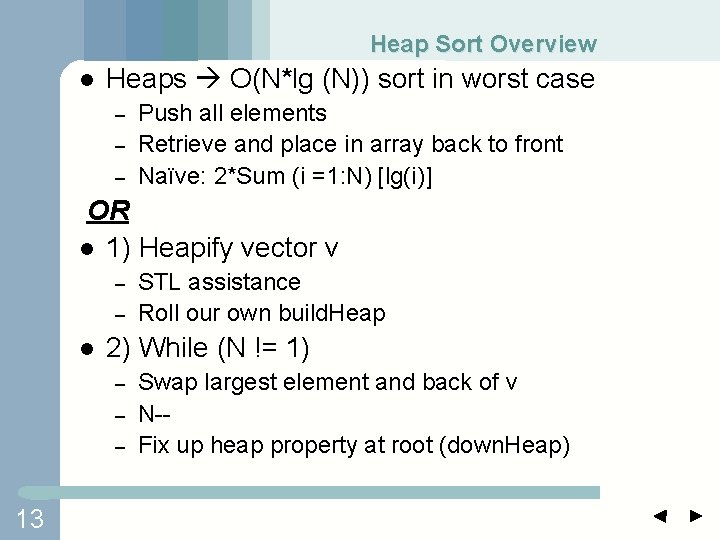
![Make_Heap (STL) int arr[] = { 50, 20, 75, 35, 25 }; make_heap (arr, Make_Heap (STL) int arr[] = { 50, 20, 75, 35, 25 }; make_heap (arr,](https://slidetodoc.com/presentation_image/3cfed5468249bcffecbf8c66ea27a40b/image-14.jpg)
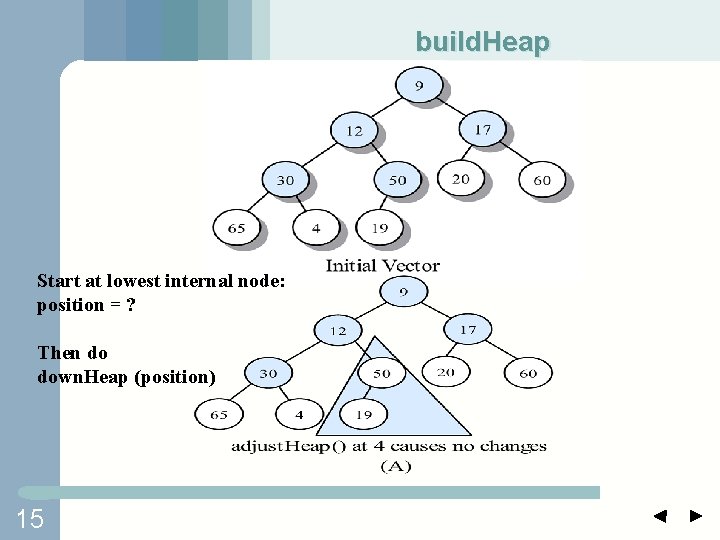
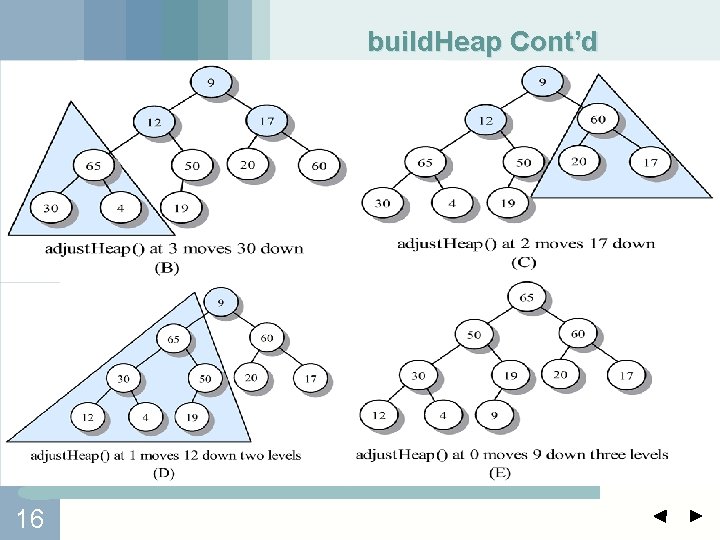
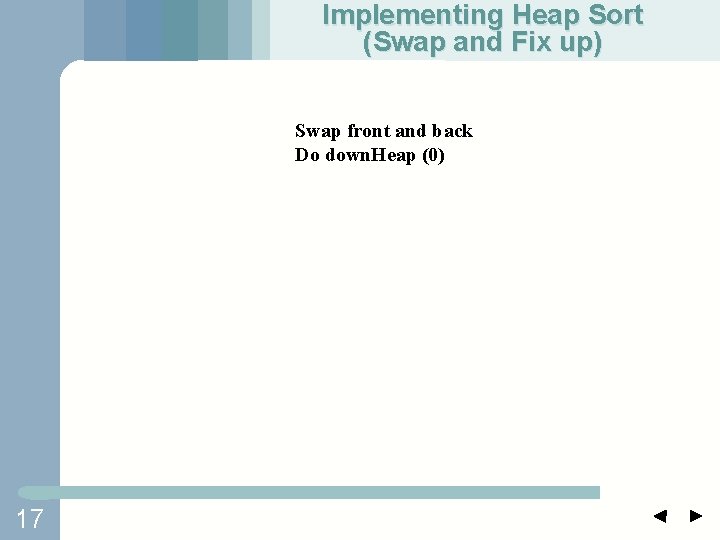
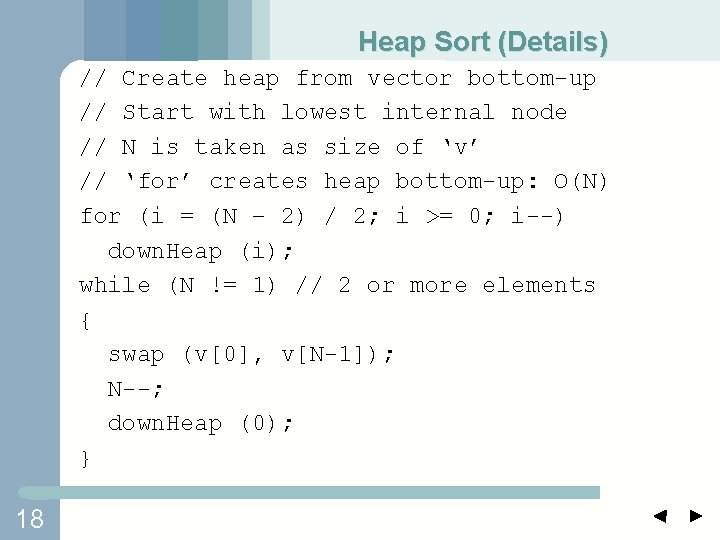
- Slides: 18
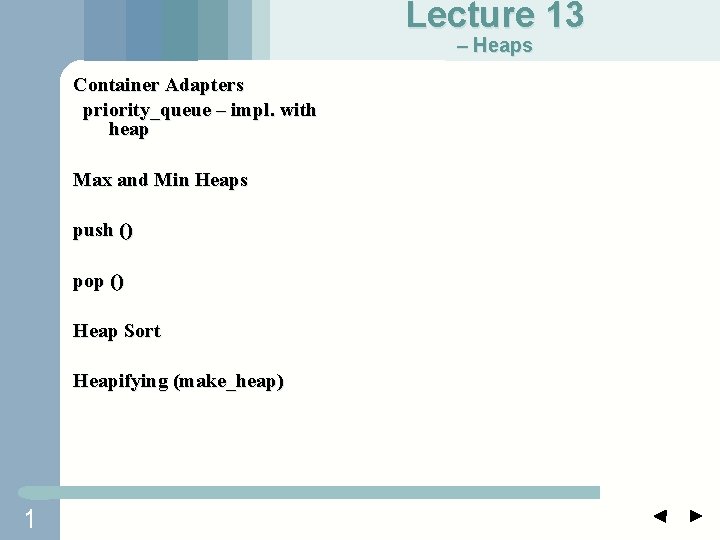
Lecture 13 – Heaps Container Adapters priority_queue – impl. with heap Max and Min Heaps push () pop () Heap Sort Heapifying (make_heap) 1
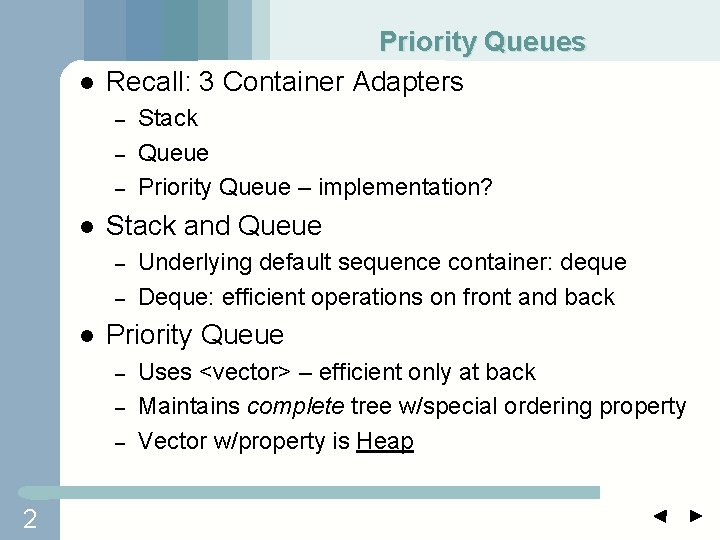
l Priority Queues Recall: 3 Container Adapters – – – l Stack and Queue – – l Underlying default sequence container: deque Deque: efficient operations on front and back Priority Queue – – – 2 Stack Queue Priority Queue – implementation? Uses <vector> – efficient only at back Maintains complete tree w/special ordering property Vector w/property is Heap
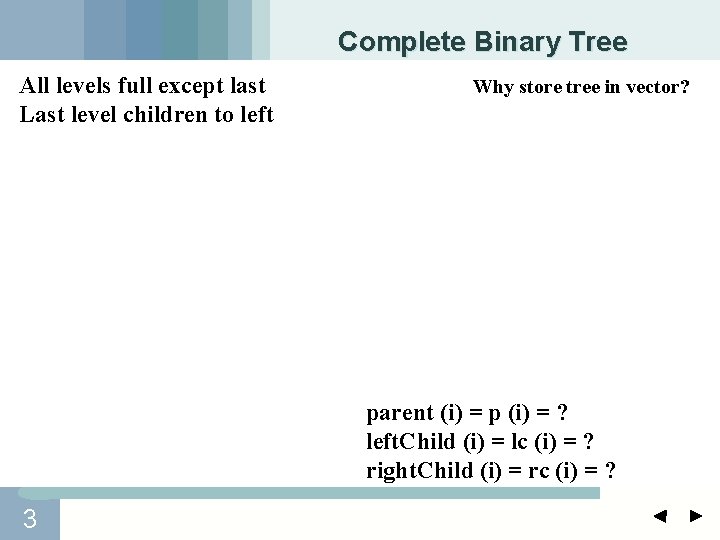
Complete Binary Tree All levels full except last Last level children to left Why store tree in vector? parent (i) = p (i) = ? left. Child (i) = lc (i) = ? right. Child (i) = rc (i) = ? 3
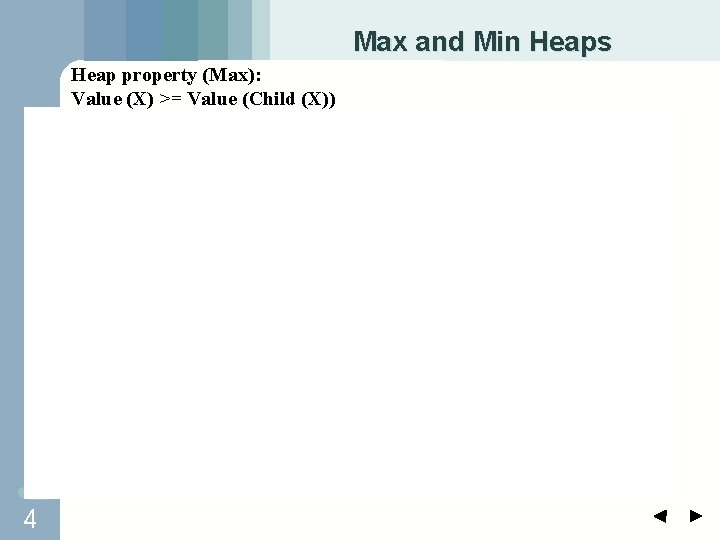
Max and Min Heaps Heap property (Max): Value (X) >= Value (Child (X)) 4
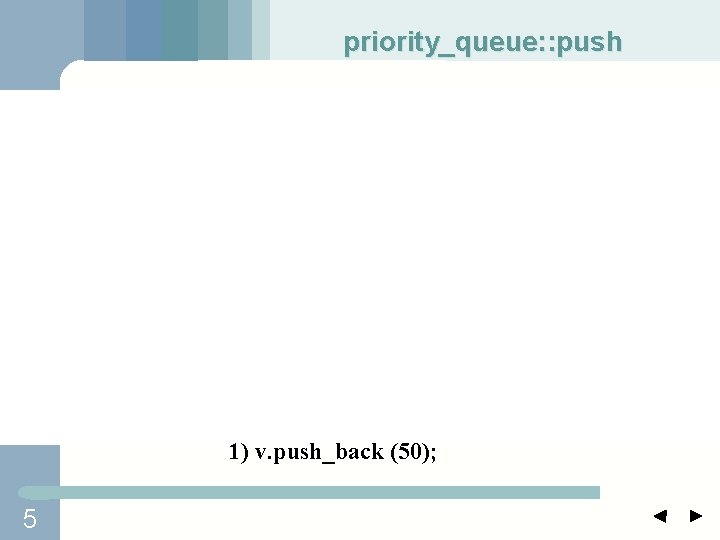
priority_queue: : push 1) v. push_back (50); 5
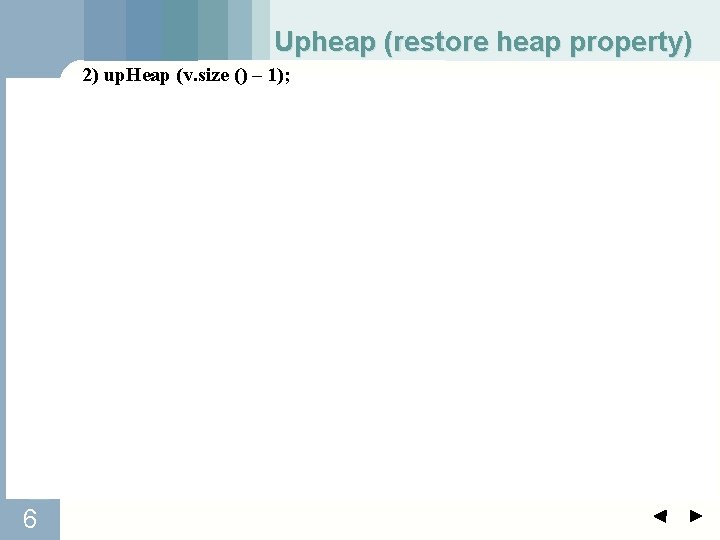
Upheap (restore heap property) 2) up. Heap (v. size () – 1); 6
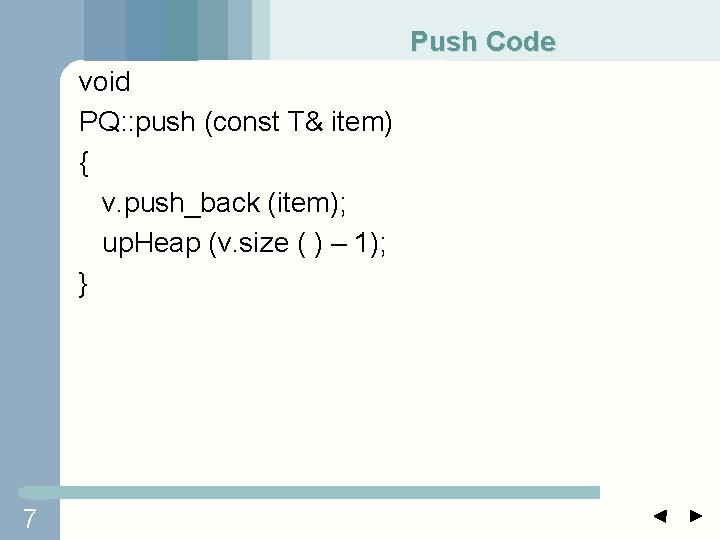
Push Code void PQ: : push (const T& item) { v. push_back (item); up. Heap (v. size ( ) – 1); } 7
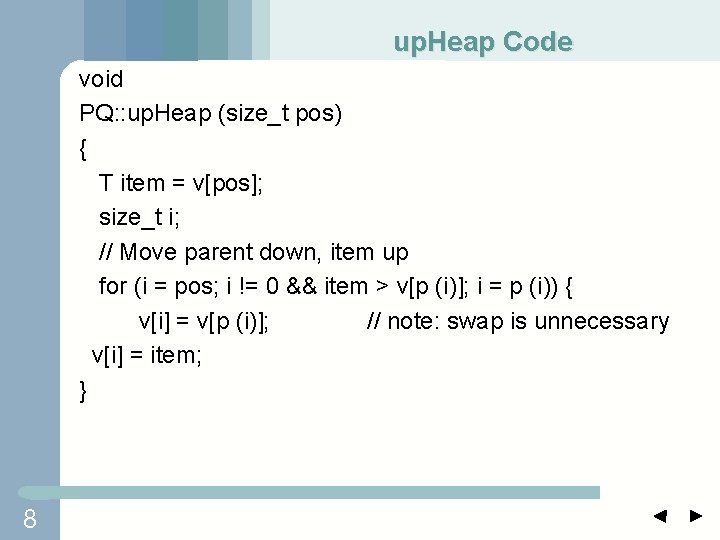
up. Heap Code void PQ: : up. Heap (size_t pos) { T item = v[pos]; size_t i; // Move parent down, item up for (i = pos; i != 0 && item > v[p (i)]; i = p (i)) { v[i] = v[p (i)]; // note: swap is unnecessary v[i] = item; } 8
![priorityqueue pop 18 v0 v back v popback 9 priority_queue: : pop 18 v[0] = v. back (); v. pop_back (); 9](https://slidetodoc.com/presentation_image/3cfed5468249bcffecbf8c66ea27a40b/image-9.jpg)
priority_queue: : pop 18 v[0] = v. back (); v. pop_back (); 9
![Downheap Compare vi with max child Move max child up if necessary 10 Downheap Compare v[i] with max child Move max child up if necessary 10](https://slidetodoc.com/presentation_image/3cfed5468249bcffecbf8c66ea27a40b/image-10.jpg)
Downheap Compare v[i] with max child Move max child up if necessary 10
![Pop void PQ pop Overwrite top element with last v0 Pop void PQ: : pop () { // Overwrite top element with last v[0]](https://slidetodoc.com/presentation_image/3cfed5468249bcffecbf8c66ea27a40b/image-11.jpg)
Pop void PQ: : pop () { // Overwrite top element with last v[0] = v. back (); v. pop_back (); // O (1) // Move elem down to proper place down. Heap (0); } 11
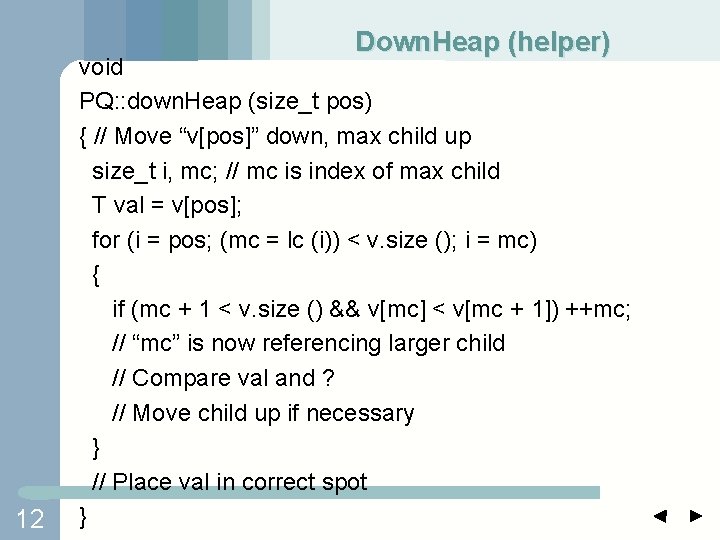
Down. Heap (helper) 12 void PQ: : down. Heap (size_t pos) { // Move “v[pos]” down, max child up size_t i, mc; // mc is index of max child T val = v[pos]; for (i = pos; (mc = lc (i)) < v. size (); i = mc) { if (mc + 1 < v. size () && v[mc] < v[mc + 1]) ++mc; // “mc” is now referencing larger child // Compare val and ? // Move child up if necessary } // Place val in correct spot }
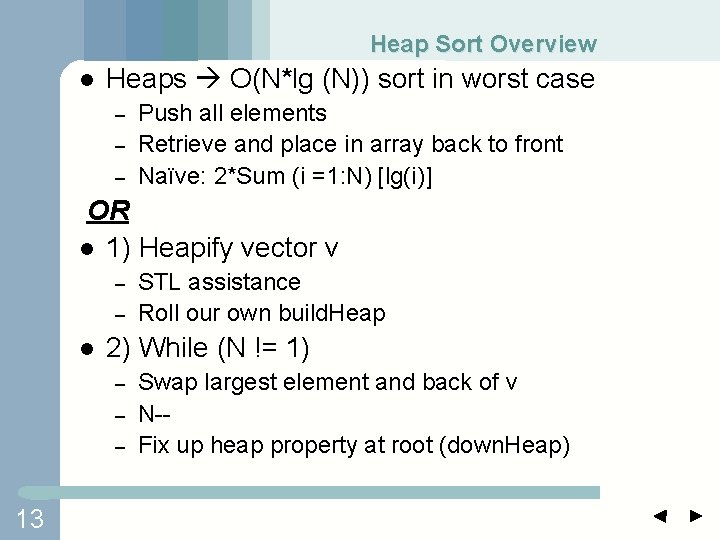
Heap Sort Overview l Heaps O(N*lg (N)) sort in worst case – – – Push all elements Retrieve and place in array back to front Naïve: 2*Sum (i =1: N) [lg(i)] OR l 1) Heapify vector v – – l 2) While (N != 1) – – – 13 STL assistance Roll our own build. Heap Swap largest element and back of v N-Fix up heap property at root (down. Heap)
![MakeHeap STL int arr 50 20 75 35 25 makeheap arr Make_Heap (STL) int arr[] = { 50, 20, 75, 35, 25 }; make_heap (arr,](https://slidetodoc.com/presentation_image/3cfed5468249bcffecbf8c66ea27a40b/image-14.jpg)
Make_Heap (STL) int arr[] = { 50, 20, 75, 35, 25 }; make_heap (arr, arr + 5); // in STL // Details later on impl. - <algorithm> 14
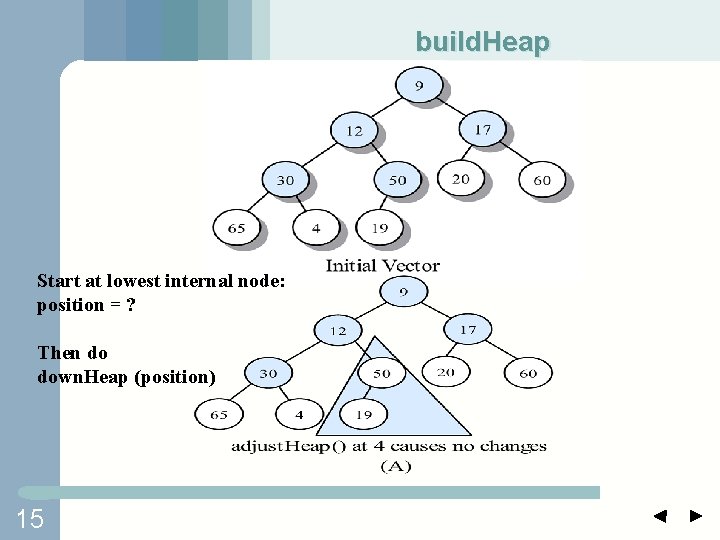
build. Heap Start at lowest internal node: position = ? Then do down. Heap (position) 15
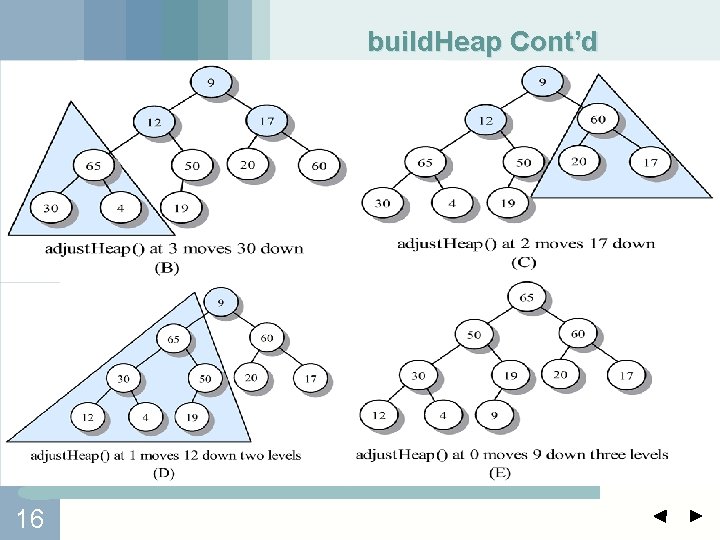
build. Heap Cont’d 16
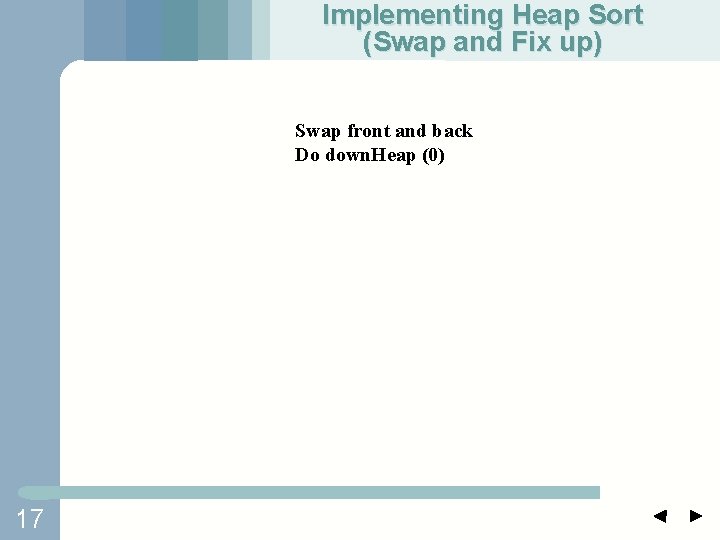
Implementing Heap Sort (Swap and Fix up) Swap front and back Do down. Heap (0) 17
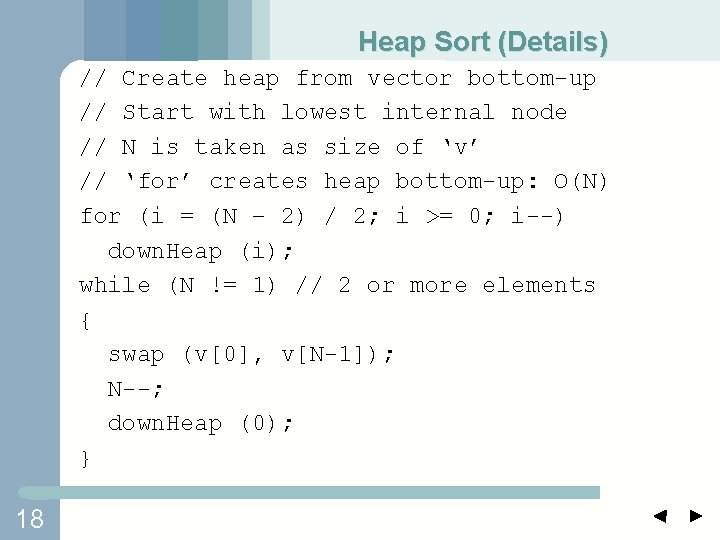
Heap Sort (Details) // Create heap from vector bottom-up // Start with lowest internal node // N is taken as size of ‘v’ // ‘for’ creates heap bottom-up: O(N) for (i = (N – 2) / 2; i >= 0; i--) down. Heap (i); while (N != 1) // 2 or more elements { swap (v[0], v[N-1]); N--; down. Heap (0); } 18
Impl pattern
Waste management plan sample philippines
Connectors and adapters definition
"integration adapters" sap or oracle or erp
Ado integration adapters
01:640:244 lecture notes - lecture 15: plat, idah, farad
Skew heaps
Tom heaps
Mean ?
Soft heaps of kaplan and zwick uses
Heaps of love ice cream
Geetika tewari
Scan text from image
Lazy binomial heap
Reheap up and reheap down
Rough terrain container handler
Dry bulk container
Ctms container
Sheeted bulk container