Java Remote Method Invocation RMI Slides for CSCI
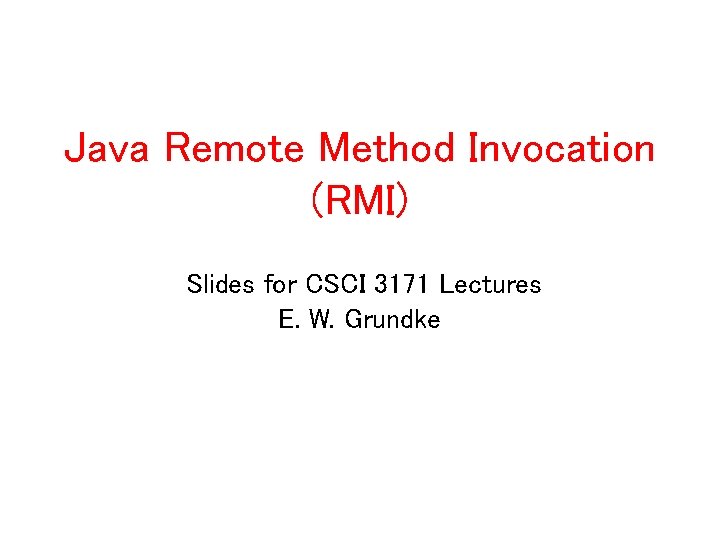
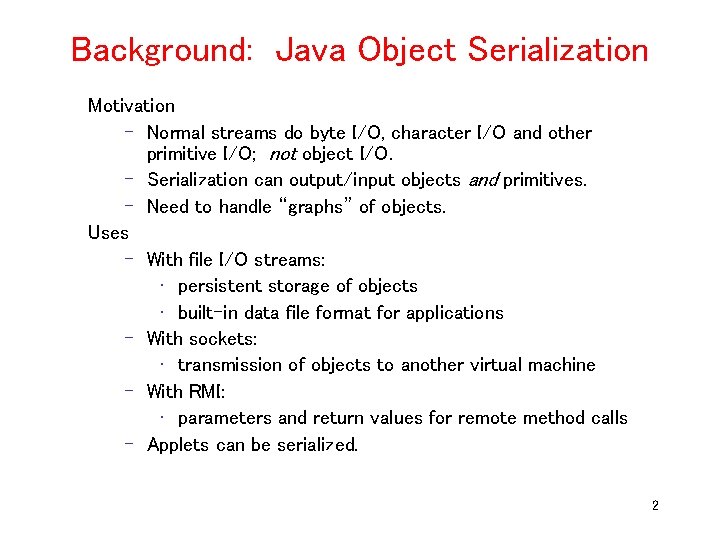
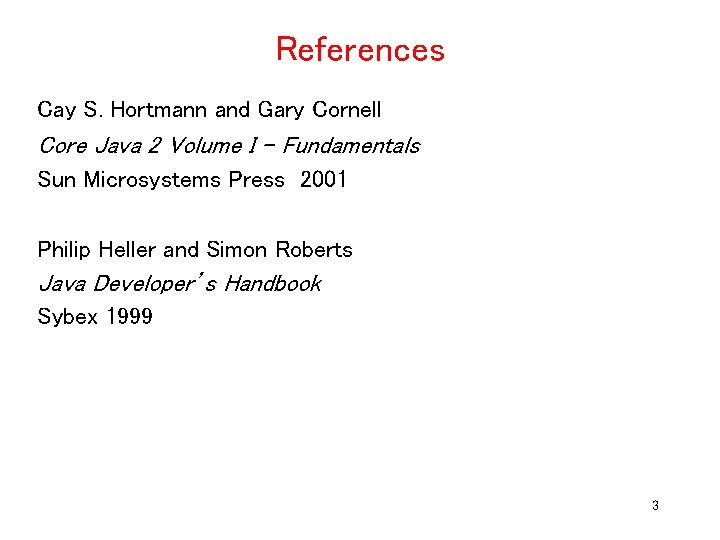
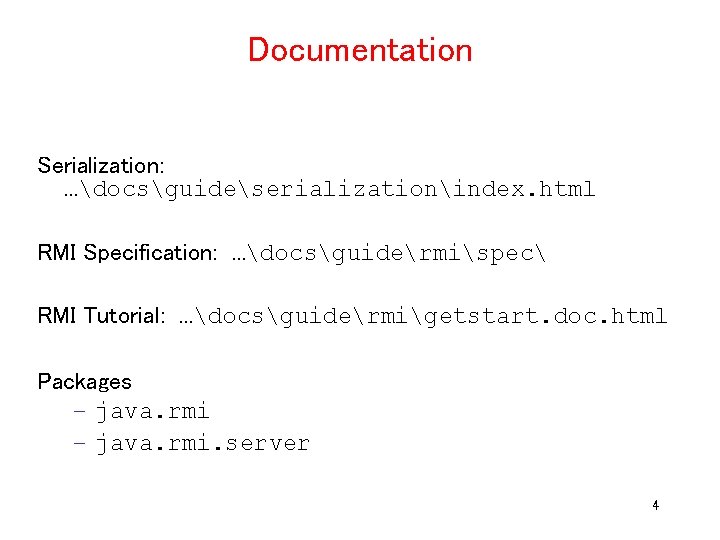
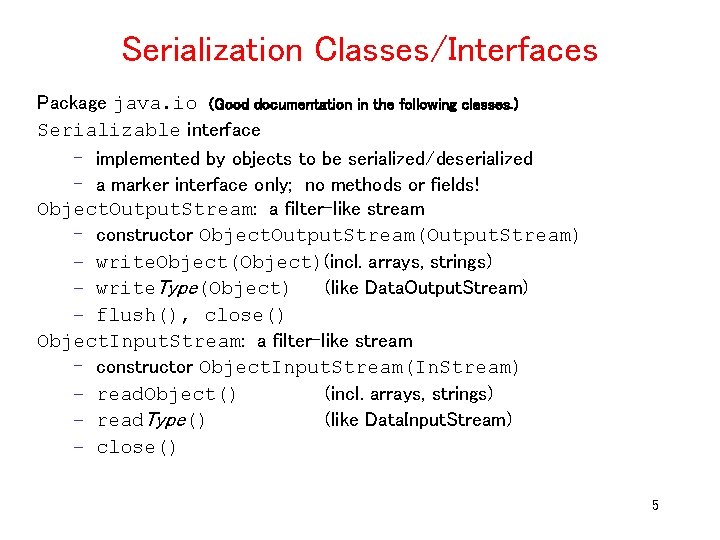
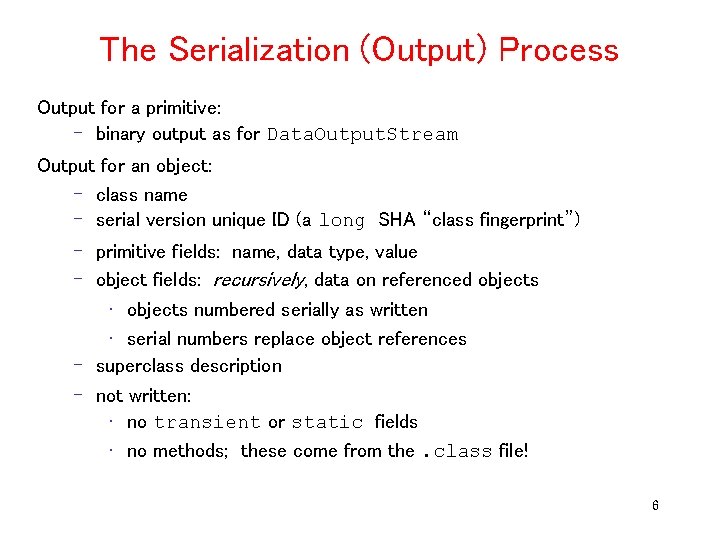
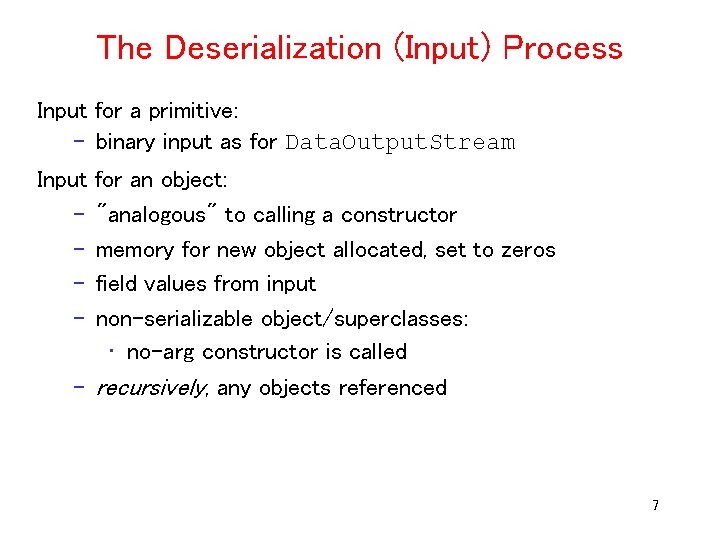
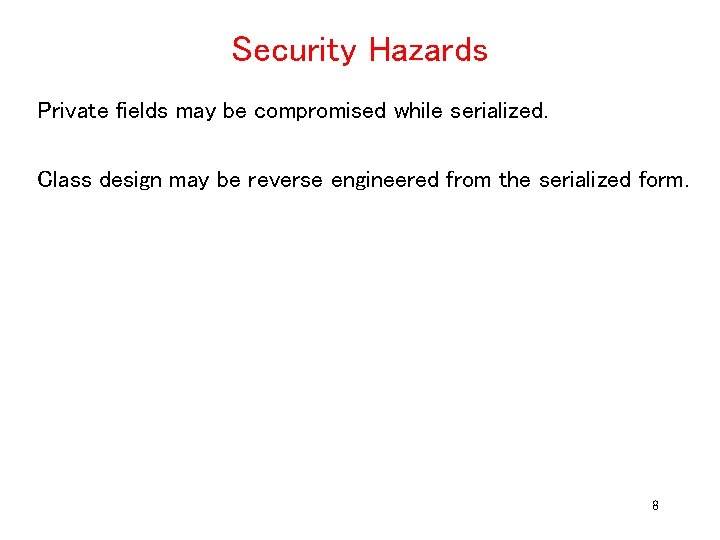
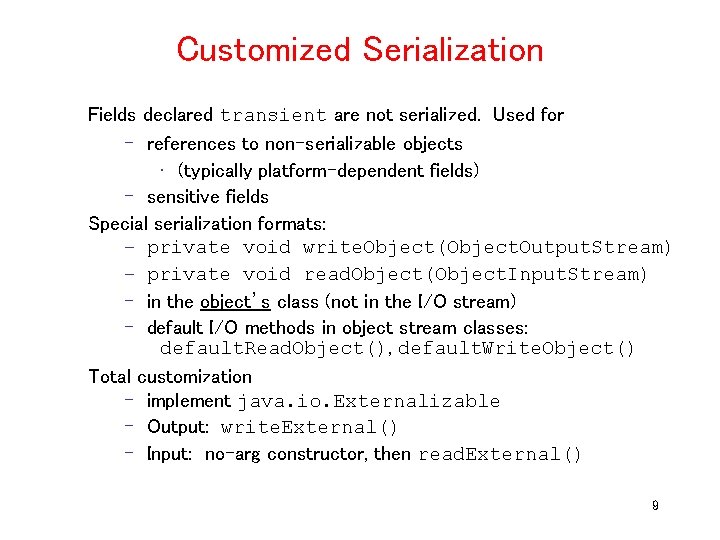
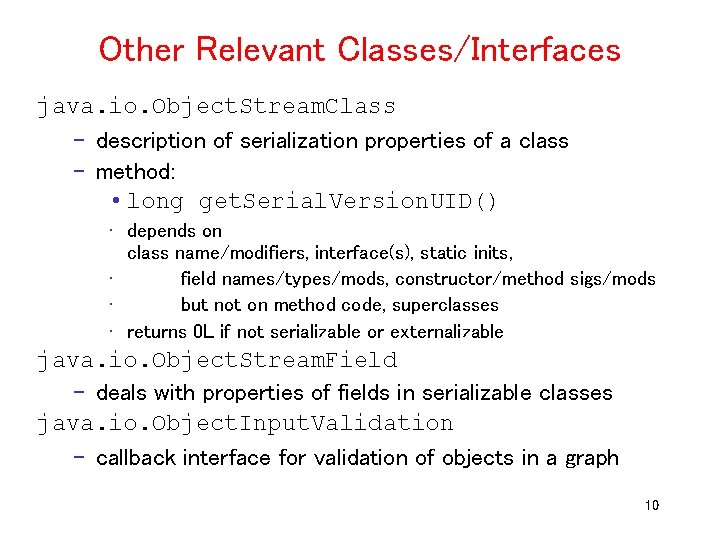
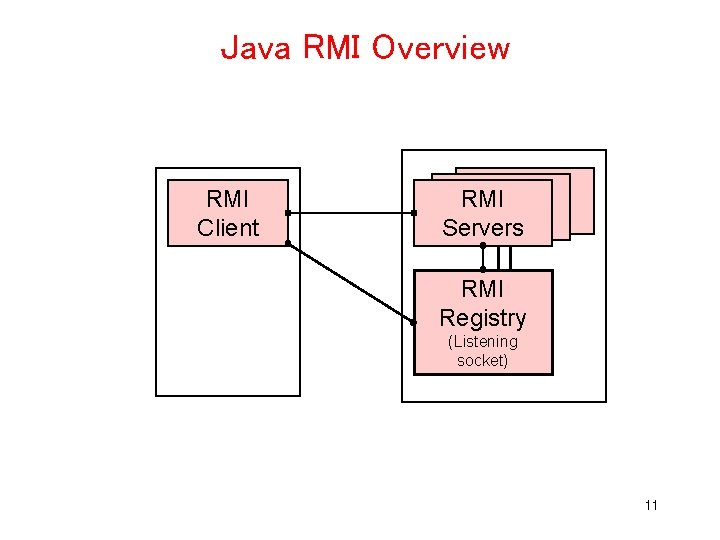
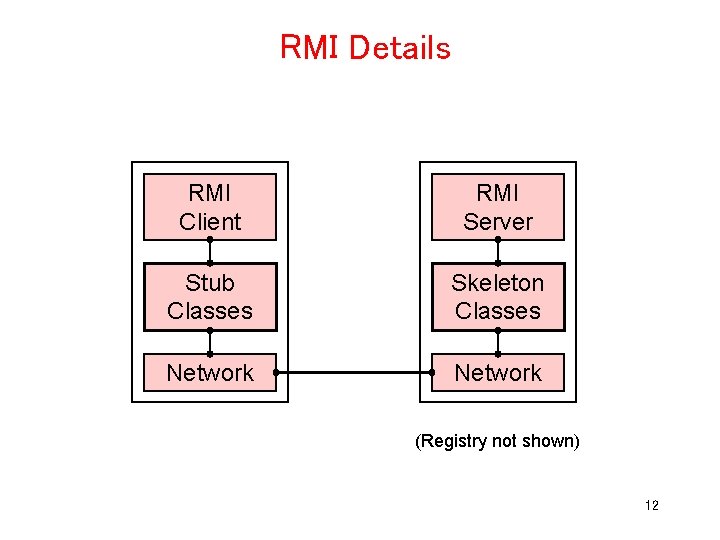
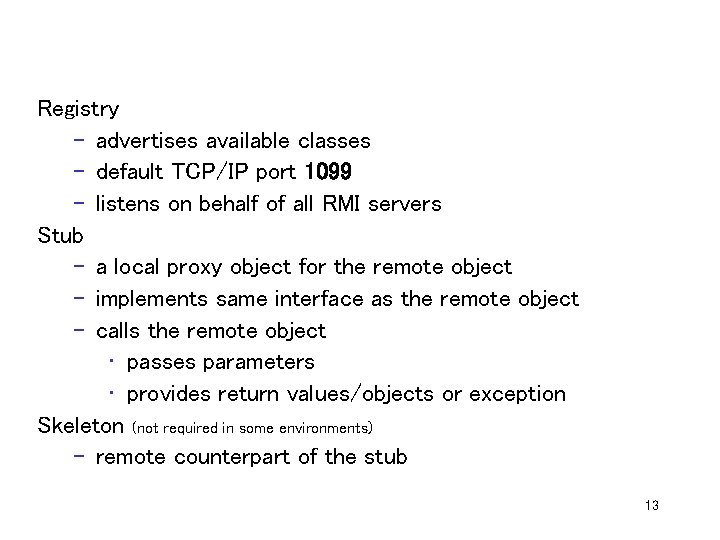
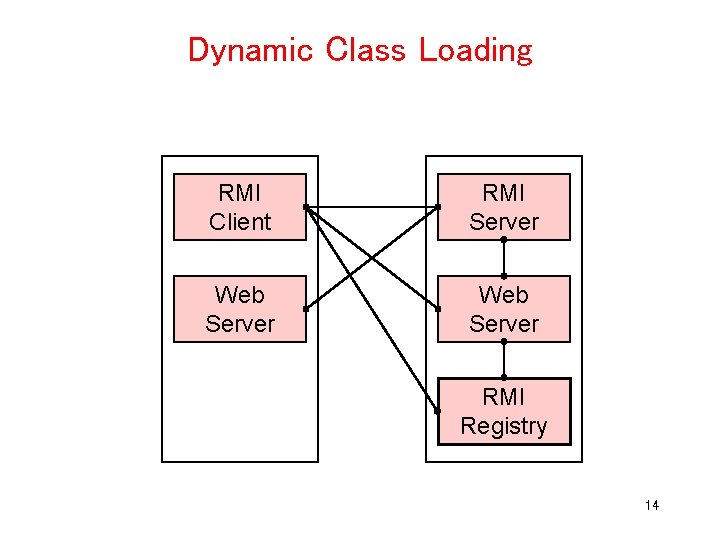
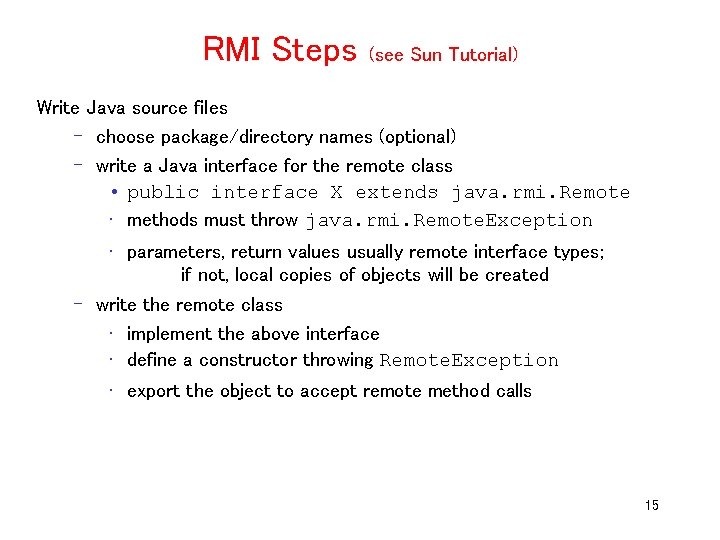
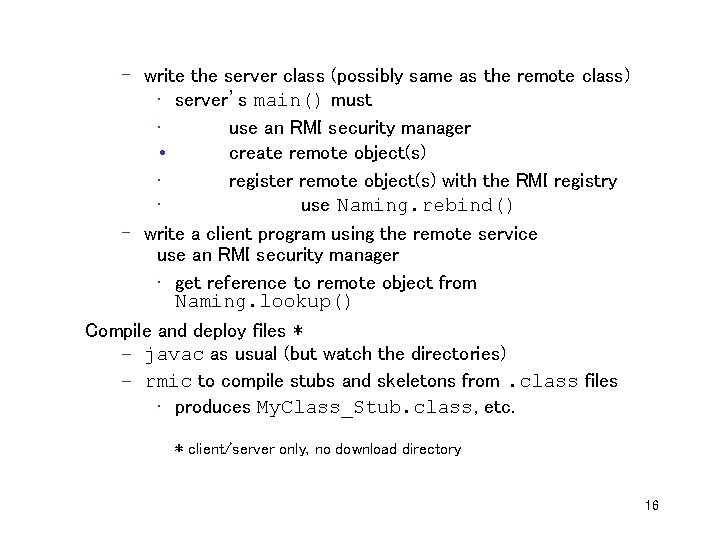
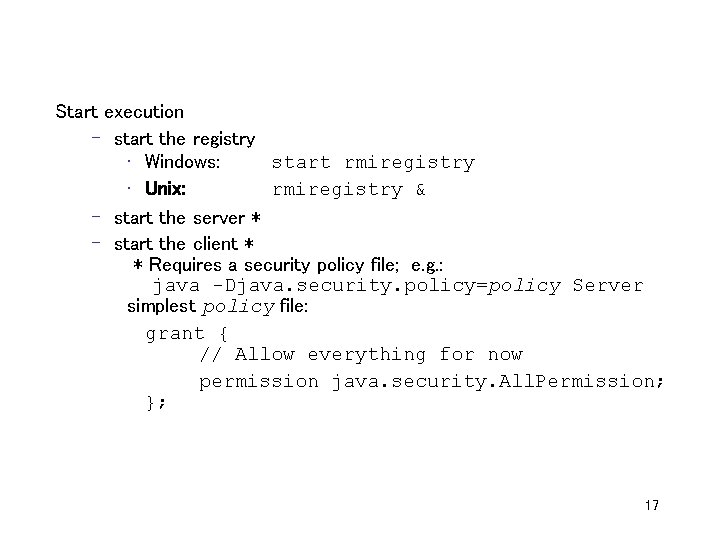
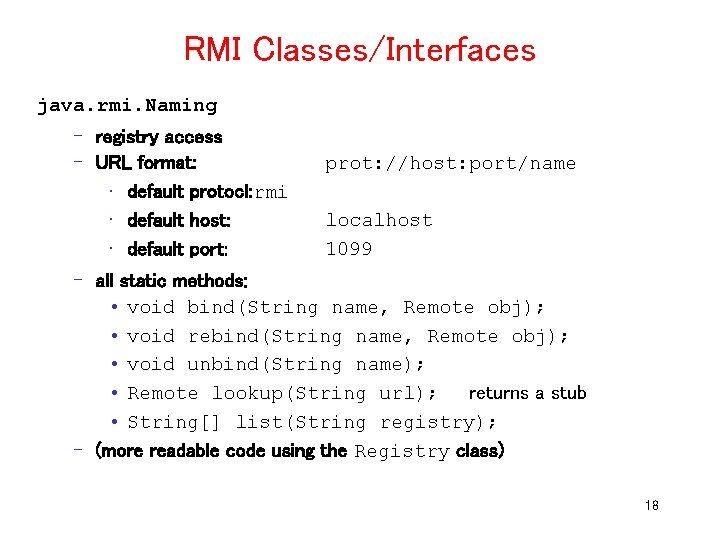
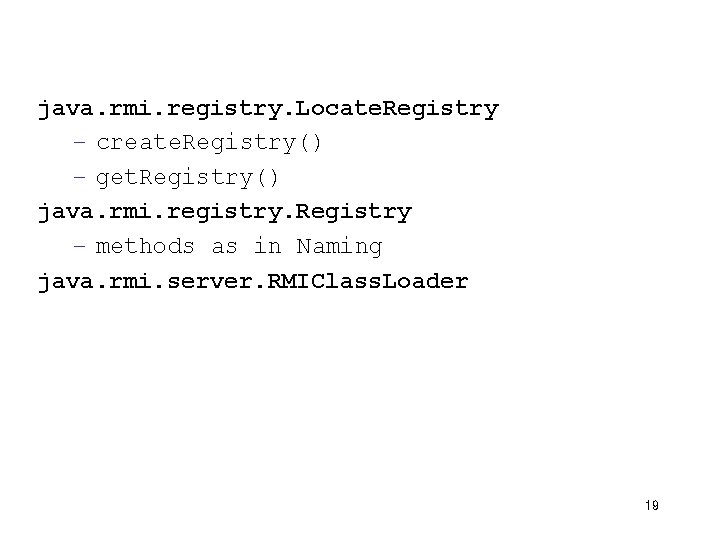
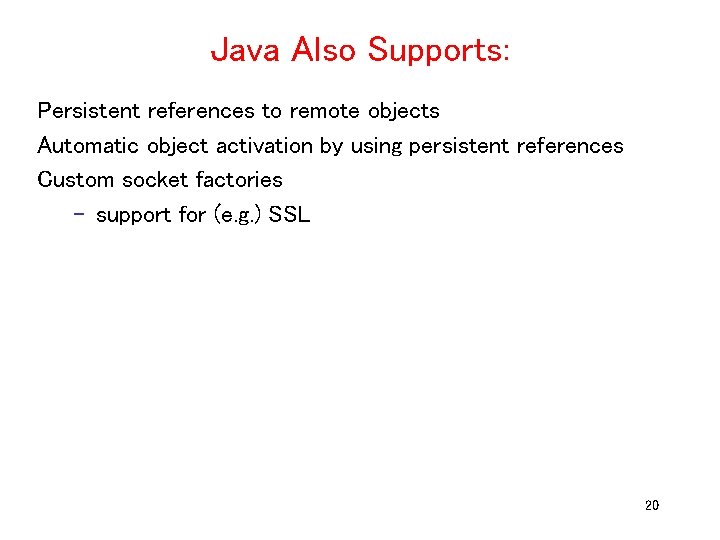
- Slides: 20
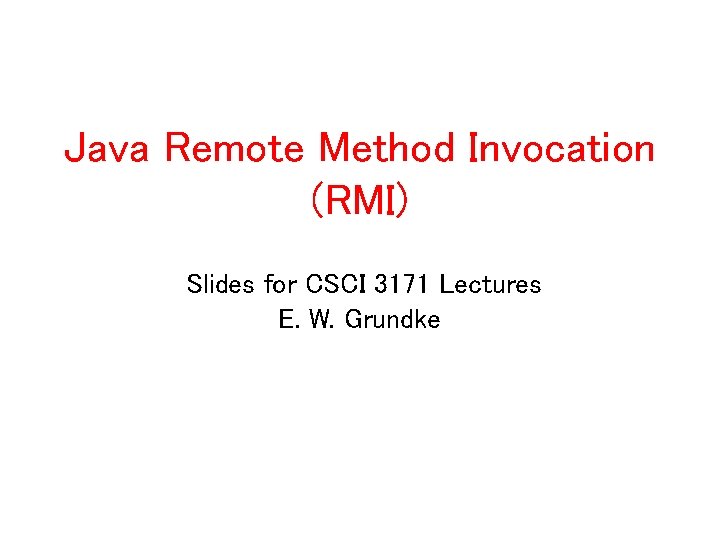
Java Remote Method Invocation (RMI) Slides for CSCI 3171 Lectures E. W. Grundke
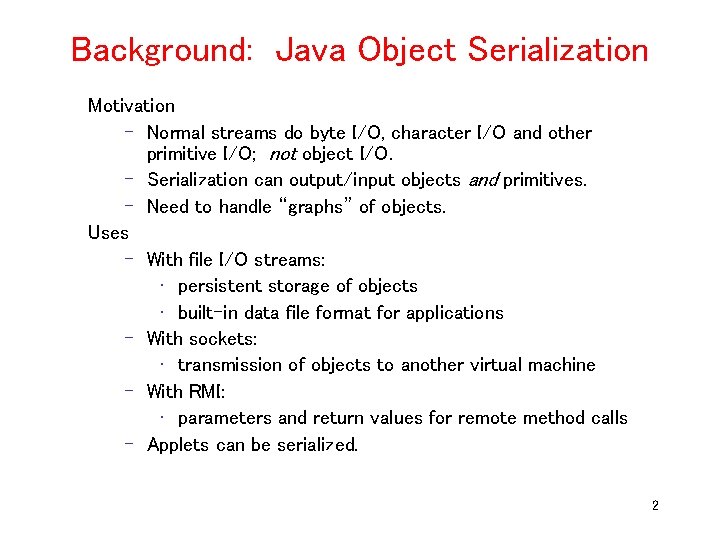
Background: Java Object Serialization Motivation – Normal streams do byte I/O, character I/O and other primitive I/O; not object I/O. – Serialization can output/input objects and primitives. – Need to handle “graphs” of objects. Uses – With file I/O streams: • persistent storage of objects • built-in data file format for applications – With sockets: • transmission of objects to another virtual machine – With RMI: • parameters and return values for remote method calls – Applets can be serialized. 2
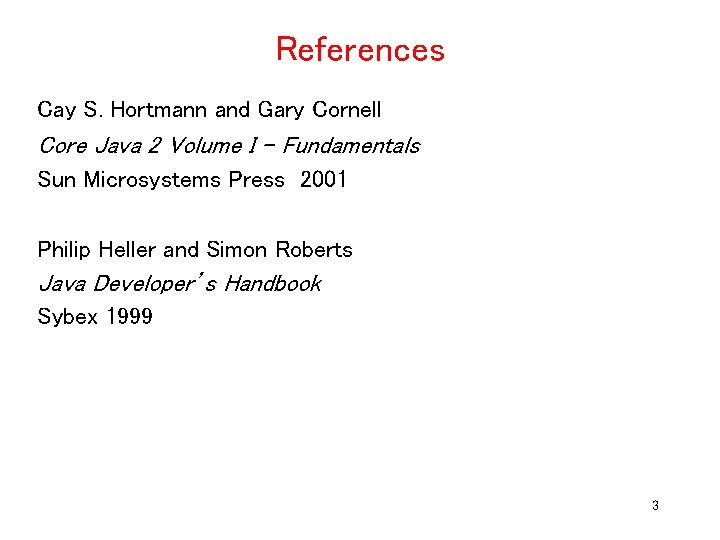
References Cay S. Hortmann and Gary Cornell Core Java 2 Volume I – Fundamentals Sun Microsystems Press 2001 Philip Heller and Simon Roberts Java Developer’s Handbook Sybex 1999 3
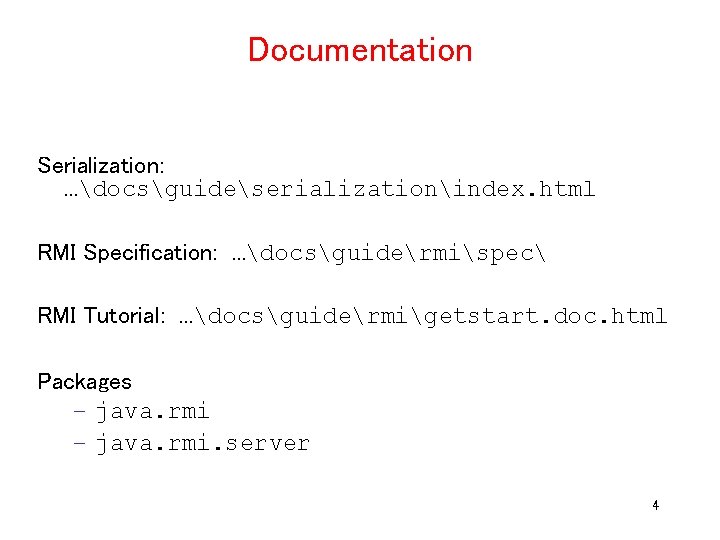
Documentation Serialization: …docsguideserializationindex. html RMI Specification: …docsguidermispec RMI Tutorial: …docsguidermigetstart. doc. html Packages – java. rmi. server 4
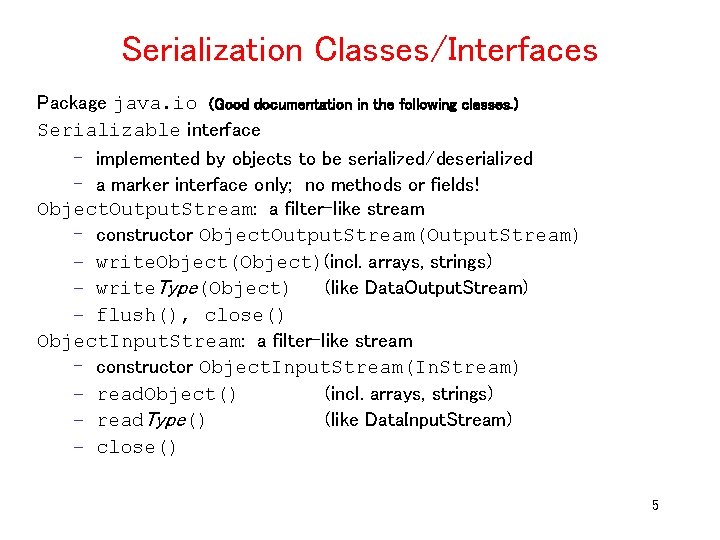
Serialization Classes/Interfaces Package java. io (Good documentation in the following classes. ) Serializable interface – implemented by objects to be serialized/deserialized – a marker interface only; no methods or fields! Object. Output. Stream: a filter-like stream – constructor Object. Output. Stream(Output. Stream) – write. Object(Object)(incl. arrays, strings) – write. Type(Object) (like Data. Output. Stream) – flush(), close() Object. Input. Stream: a filter-like stream – constructor Object. Input. Stream(In. Stream) – read. Object() (incl. arrays, strings) – read. Type() (like Data. Input. Stream) – close() 5
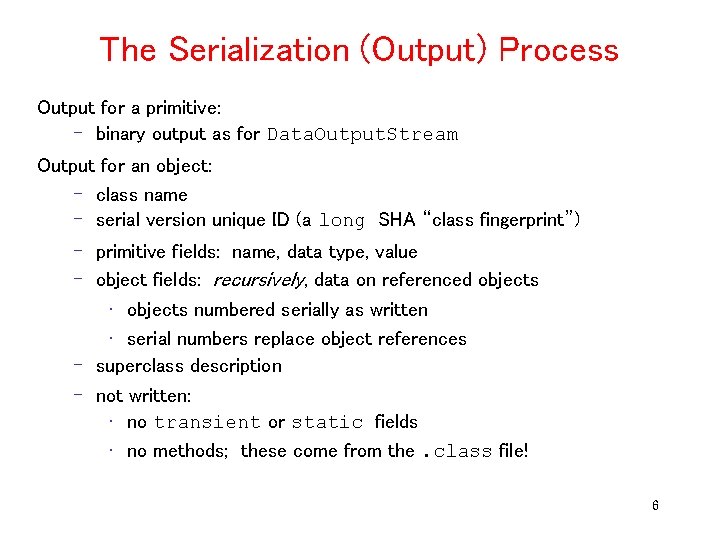
The Serialization (Output) Process Output for a primitive: – binary output as for Data. Output. Stream Output for an object: – class name – serial version unique ID (a long SHA “class fingerprint”) – primitive fields: name, data type, value – object fields: recursively, data on referenced objects • objects numbered serially as written • serial numbers replace object references – superclass description – not written: • no transient or static fields • no methods; these come from the. class file! 6
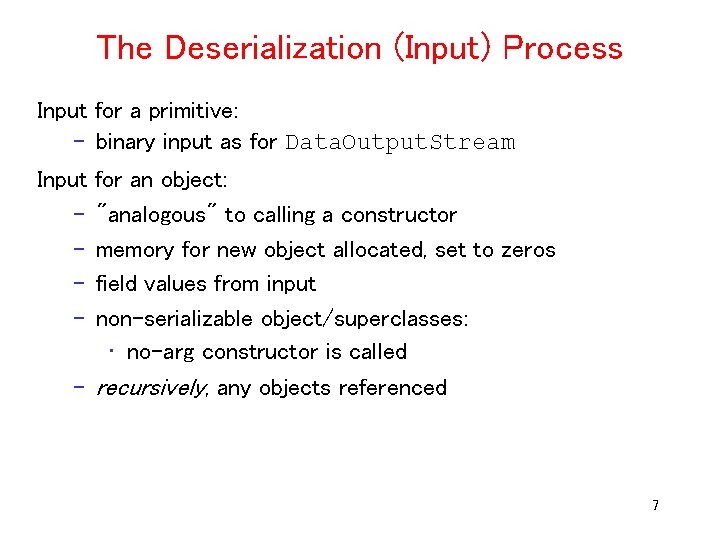
The Deserialization (Input) Process Input for a primitive: – binary input as for Data. Output. Stream Input – – for an object: "analogous" to calling a constructor memory for new object allocated, set to zeros field values from input non-serializable object/superclasses: • no-arg constructor is called – recursively, any objects referenced 7
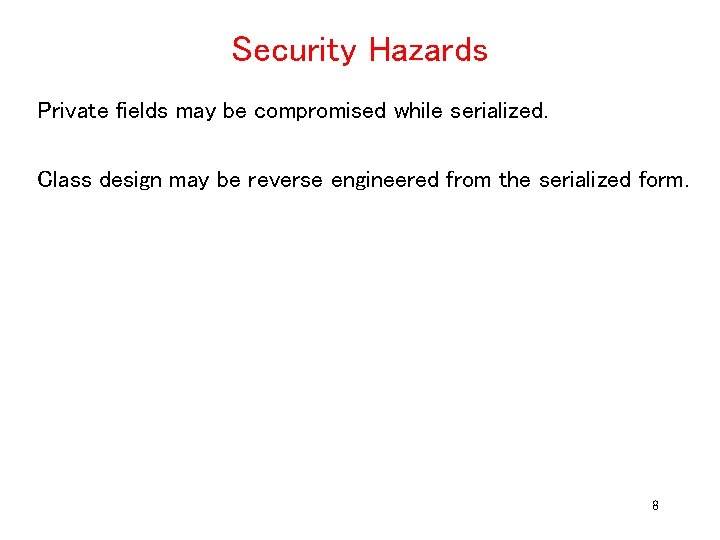
Security Hazards Private fields may be compromised while serialized. Class design may be reverse engineered from the serialized form. 8
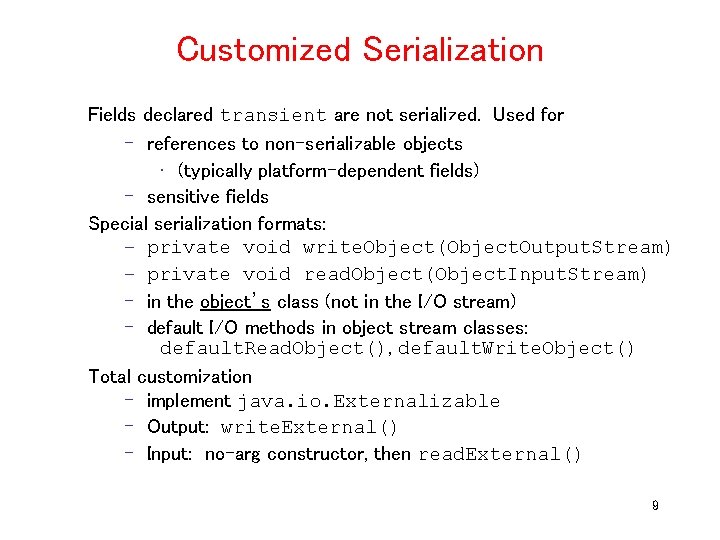
Customized Serialization Fields declared transient are not serialized. Used for – references to non-serializable objects • (typically platform-dependent fields) – sensitive fields Special serialization formats: – private void write. Object(Object. Output. Stream) – private void read. Object(Object. Input. Stream) – in the object’s class (not in the I/O stream) – default I/O methods in object stream classes: default. Read. Object(), default. Write. Object() Total customization – implement java. io. Externalizable – Output: write. External() – Input: no-arg constructor, then read. External() 9
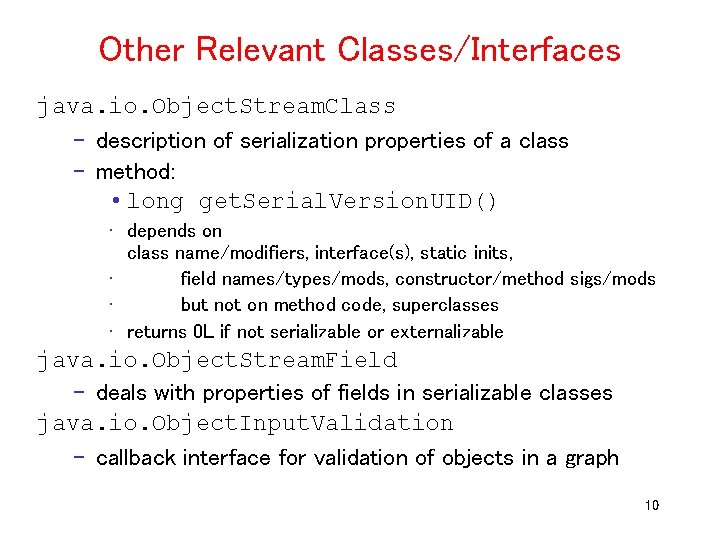
Other Relevant Classes/Interfaces java. io. Object. Stream. Class – description of serialization properties of a class – method: • long get. Serial. Version. UID() • depends on class name/modifiers, interface(s), static inits, • field names/types/mods, constructor/method sigs/mods • but not on method code, superclasses • returns 0 L if not serializable or externalizable java. io. Object. Stream. Field – deals with properties of fields in serializable classes java. io. Object. Input. Validation – callback interface for validation of objects in a graph 10
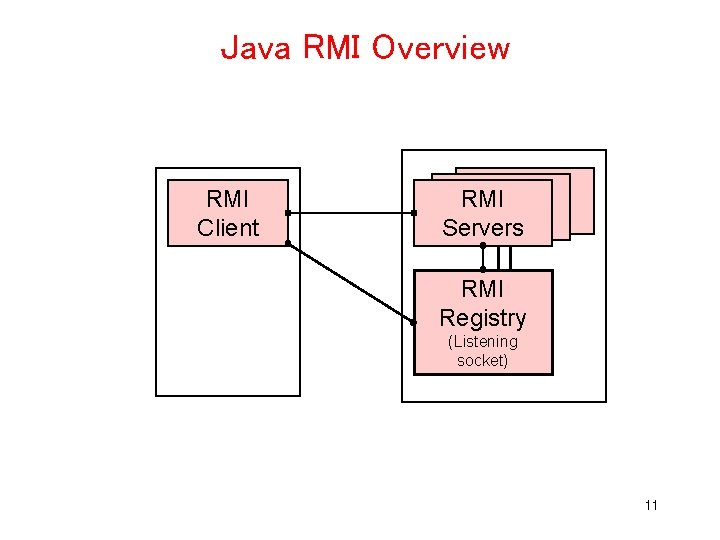
Java RMI Overview RMI Client RMI RMI Servers RMI Registry (Listening socket) 11
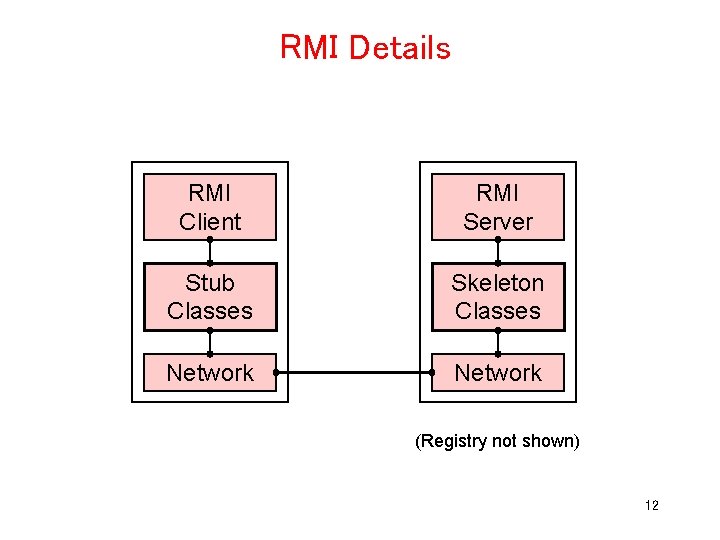
RMI Details RMI Client RMI Server Stub Classes Skeleton Classes Network (Registry not shown) 12
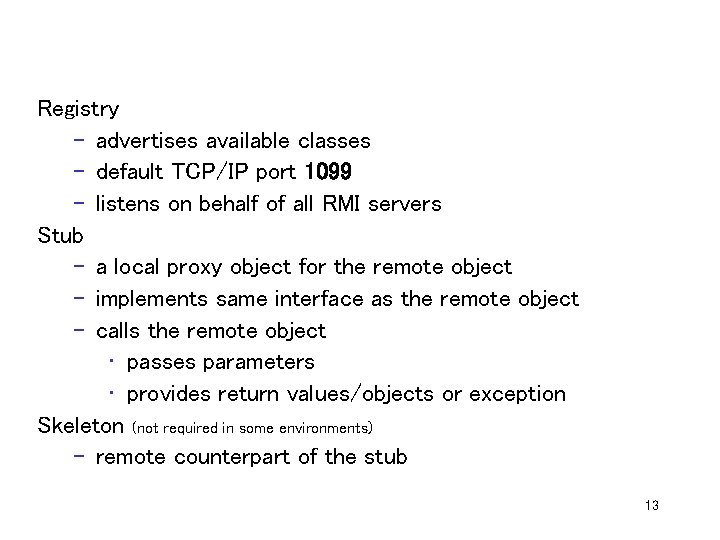
Registry – advertises available classes – default TCP/IP port 1099 – listens on behalf of all RMI servers Stub – a local proxy object for the remote object – implements same interface as the remote object – calls the remote object • passes parameters • provides return values/objects or exception Skeleton (not required in some environments) – remote counterpart of the stub 13
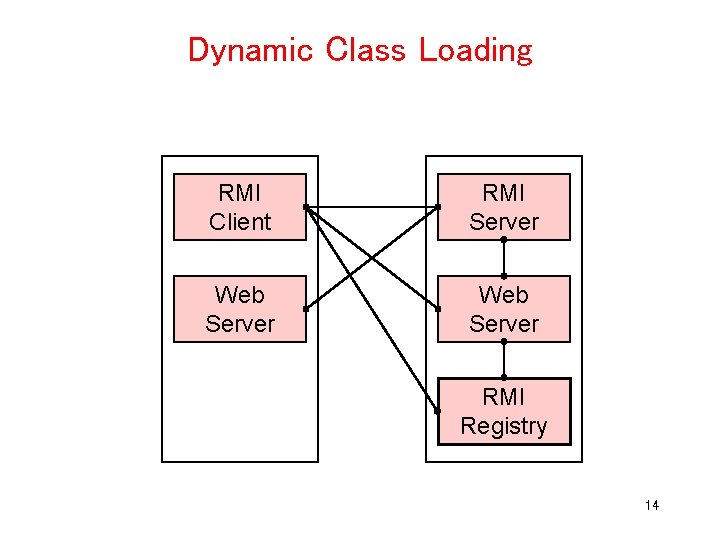
Dynamic Class Loading RMI Client RMI Server Web Server RMI Registry 14
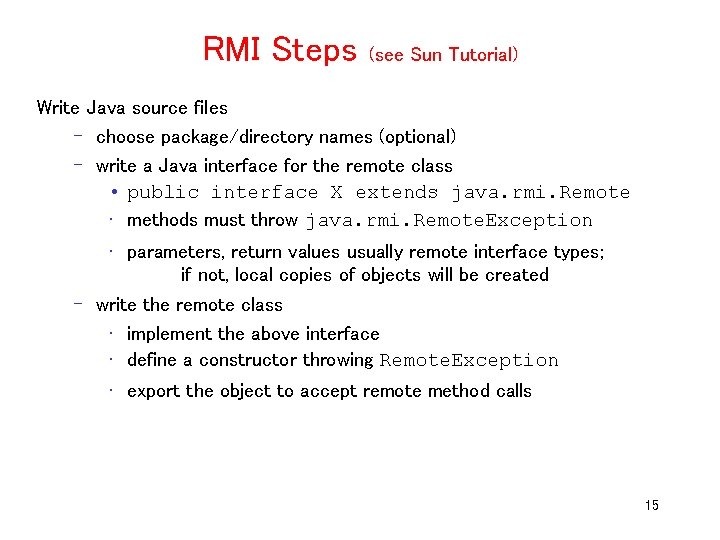
RMI Steps (see Sun Tutorial) Write Java source files – choose package/directory names (optional) – write a Java interface for the remote class • public interface X extends java. rmi. Remote • methods must throw java. rmi. Remote. Exception • parameters, return values usually remote interface types; if not, local copies of objects will be created – write the remote class • implement the above interface • define a constructor throwing Remote. Exception • export the object to accept remote method calls 15
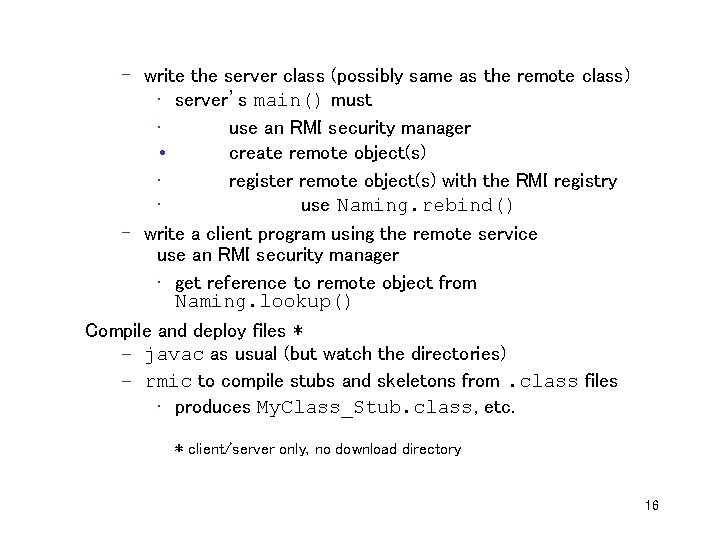
– write the server class (possibly same as the remote class) • server’s main() must • use an RMI security manager • create remote object(s) • register remote object(s) with the RMI registry • use Naming. rebind() – write a client program using the remote service use an RMI security manager • get reference to remote object from Naming. lookup() Compile and deploy files * – javac as usual (but watch the directories) – rmic to compile stubs and skeletons from. class files • produces My. Class_Stub. class, etc. * client/server only, no download directory 16
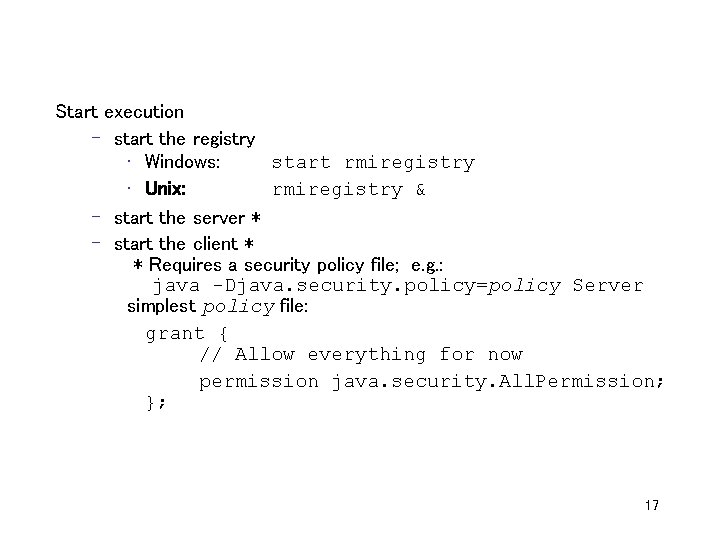
Start execution – start the registry • Windows: start rmiregistry • Unix: rmiregistry & – start the server * – start the client * * Requires a security policy file; e. g. : java -Djava. security. policy=policy Server simplest policy file: grant { // Allow everything for now permission java. security. All. Permission; }; 17
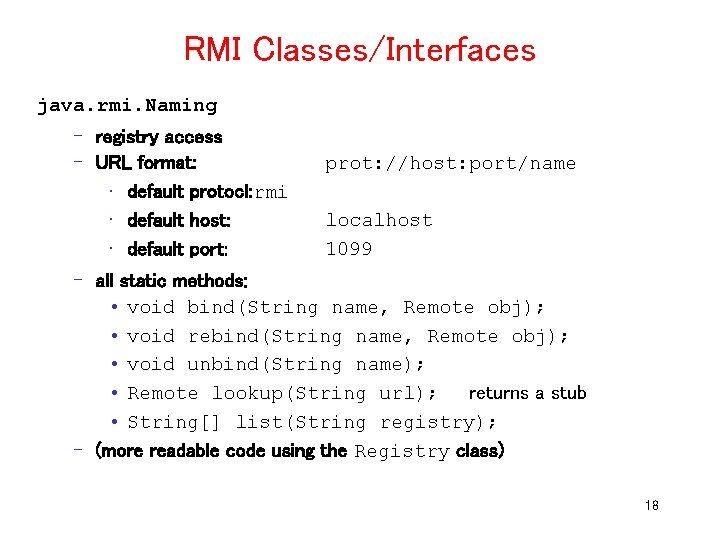
RMI Classes/Interfaces java. rmi. Naming – registry access – URL format: • default protocl: rmi • default host: • default port: prot: //host: port/name localhost 1099 – all static methods: • void bind(String name, Remote obj); • void rebind(String name, Remote obj); • void unbind(String name); • Remote lookup(String url); returns a stub • String[] list(String registry); – (more readable code using the Registry class) 18
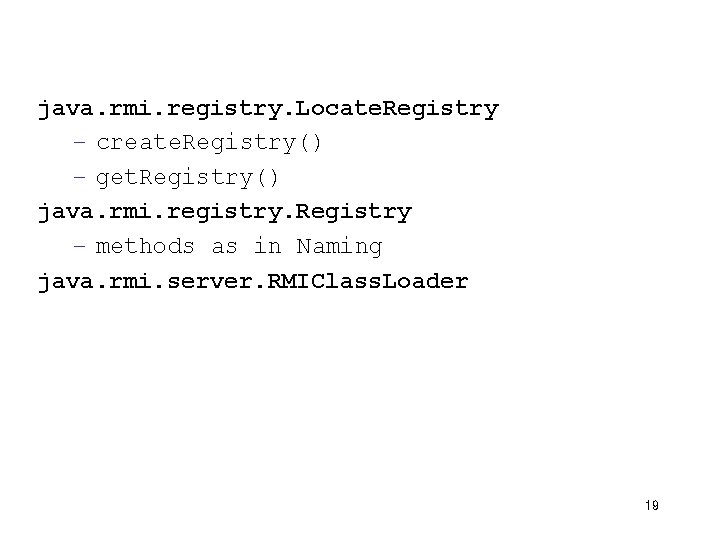
java. rmi. registry. Locate. Registry – create. Registry() – get. Registry() java. rmi. registry. Registry – methods as in Naming java. rmi. server. RMIClass. Loader 19
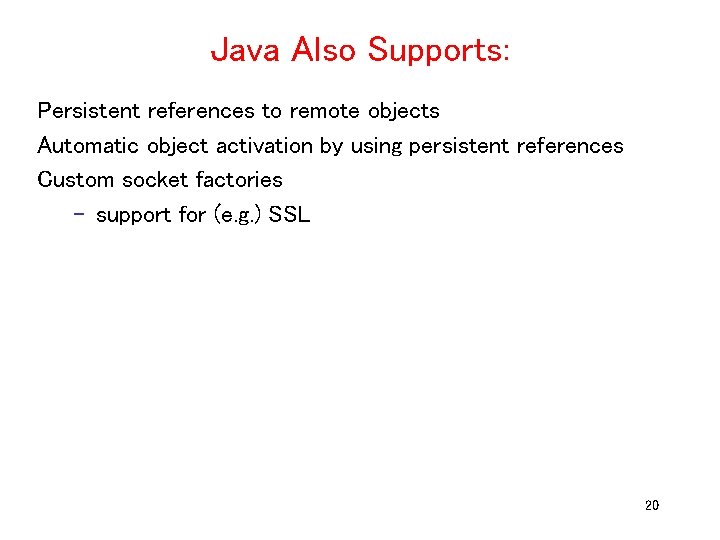
Java Also Supports: Persistent references to remote objects Automatic object activation by using persistent references Custom socket factories – support for (e. g. ) SSL 20
Rmi in java
Distributed objects and remote invocation
Distributed objects and remote invocation
Distributed objects and remote invocation
Www.rmic.somee.com
Java.rmi.server.codebase
Rmi performance
Javatpoint ejb
Rmi java
Rmi rpc
Interfaz java
Java rmi
Procedure call
Southville invocation
Questions about paradise lost
Rotaract pledge and invocation
Anaphora figure of speech
Eagle court of honor invocation
The odyssey invocation
Pediatric in tagalog
Biba model example