Remote Method Invocation RMI ClientServer Communication Sockets Remote
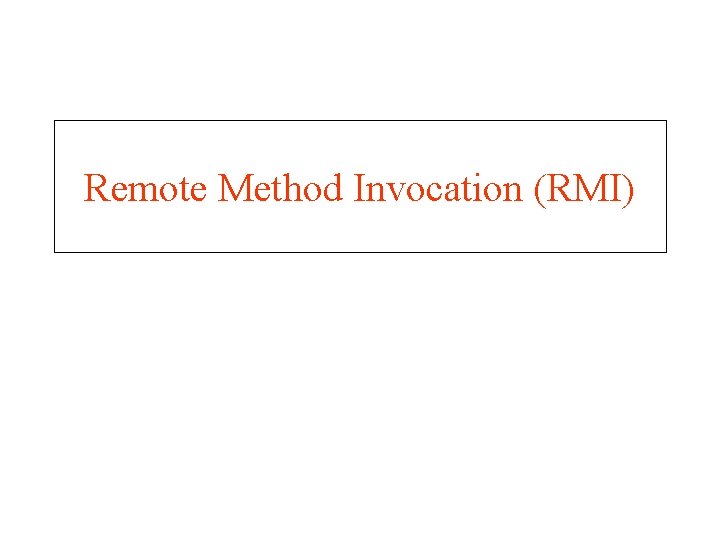
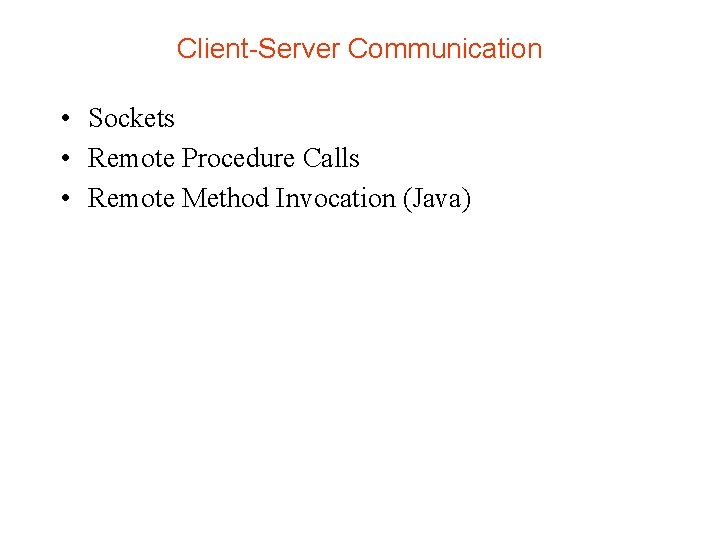
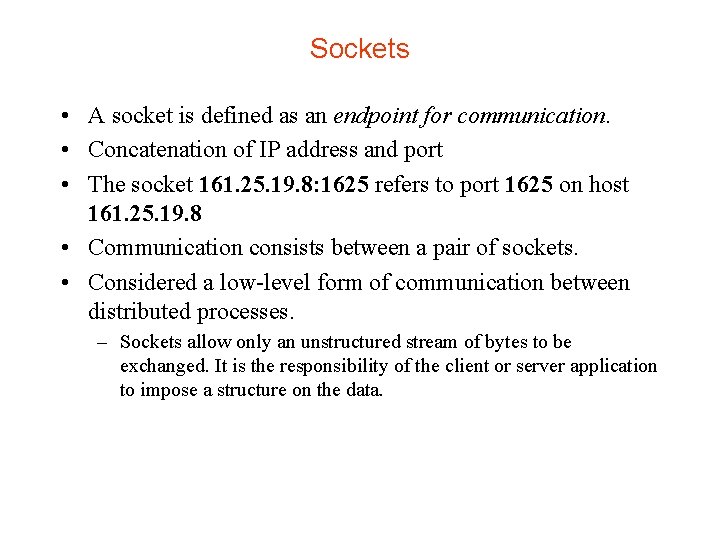
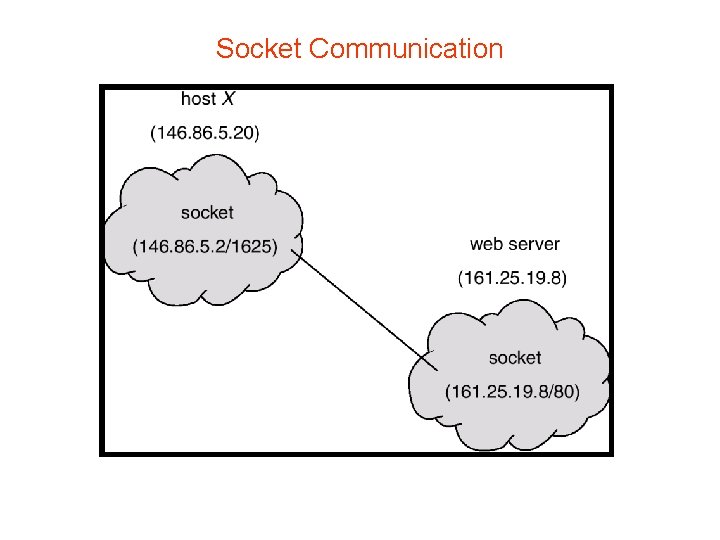
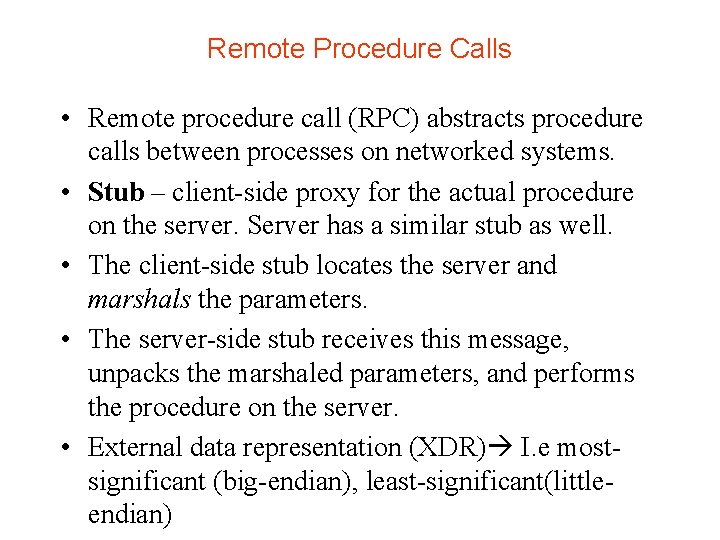
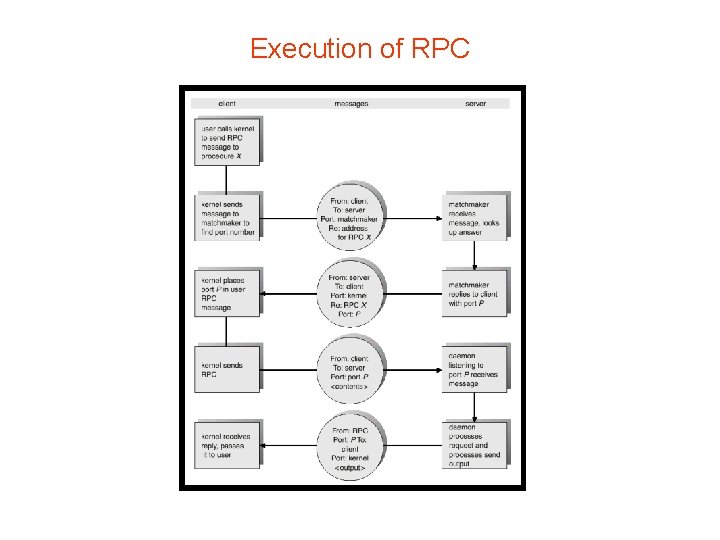
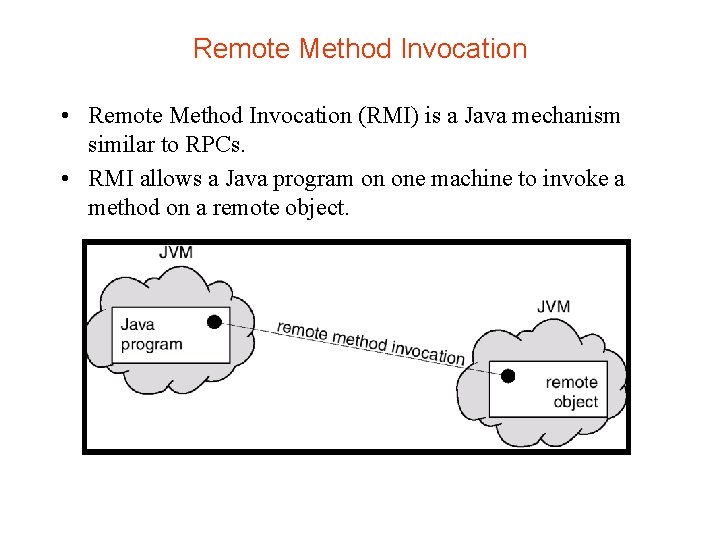
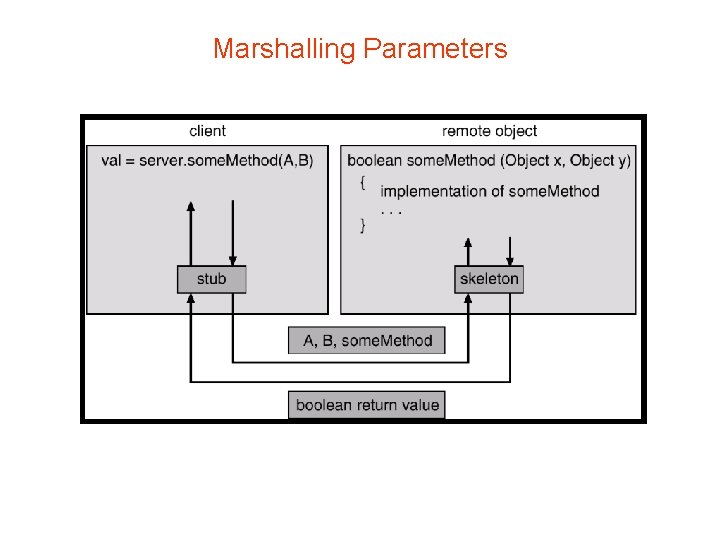
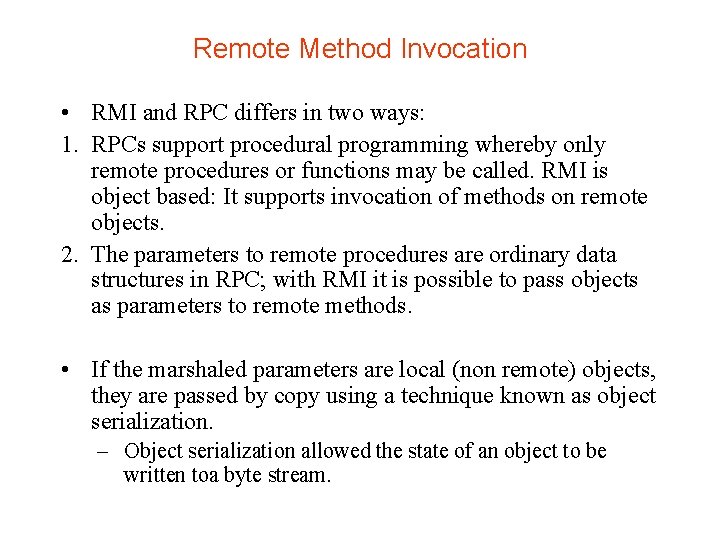
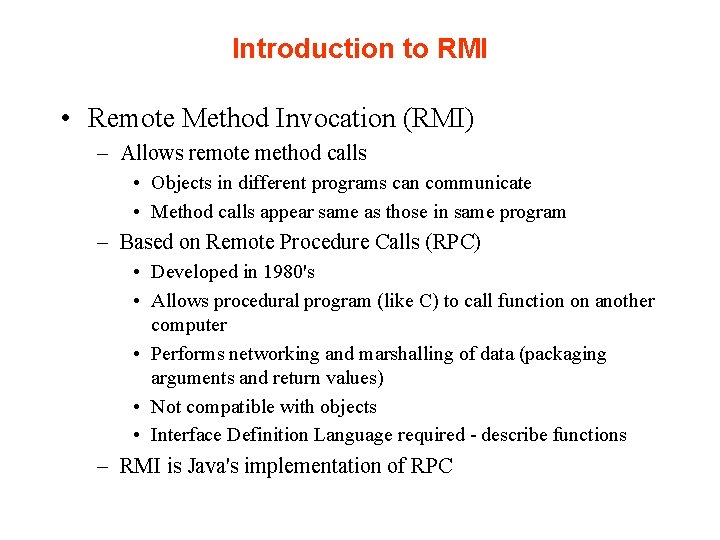
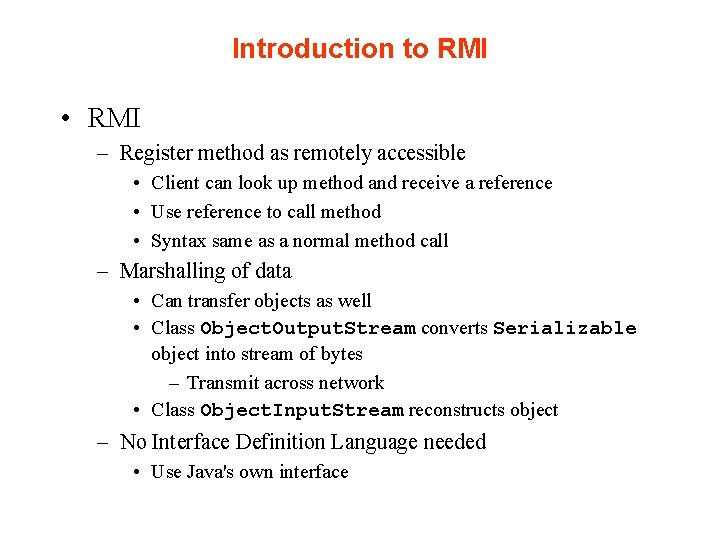
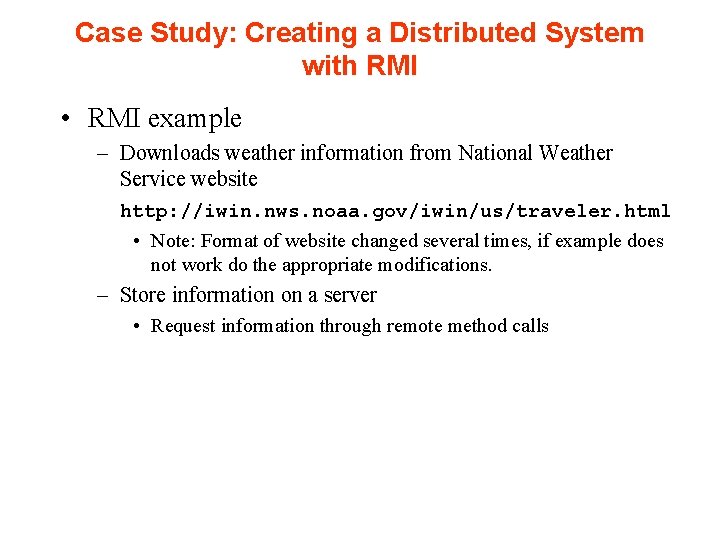
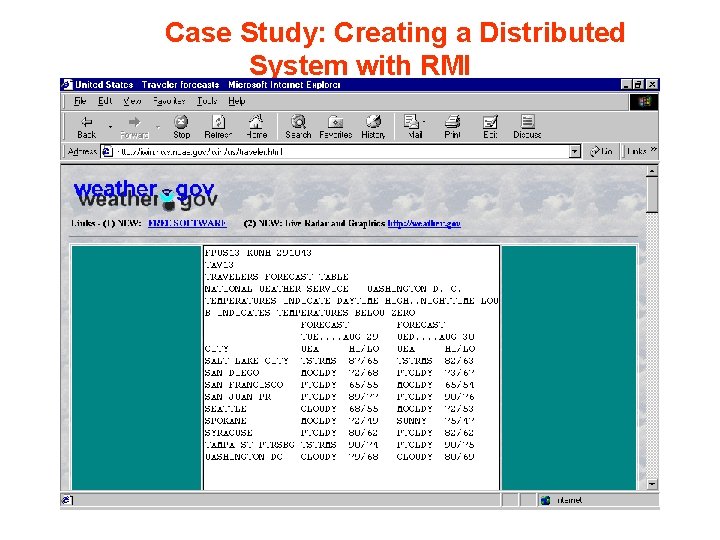
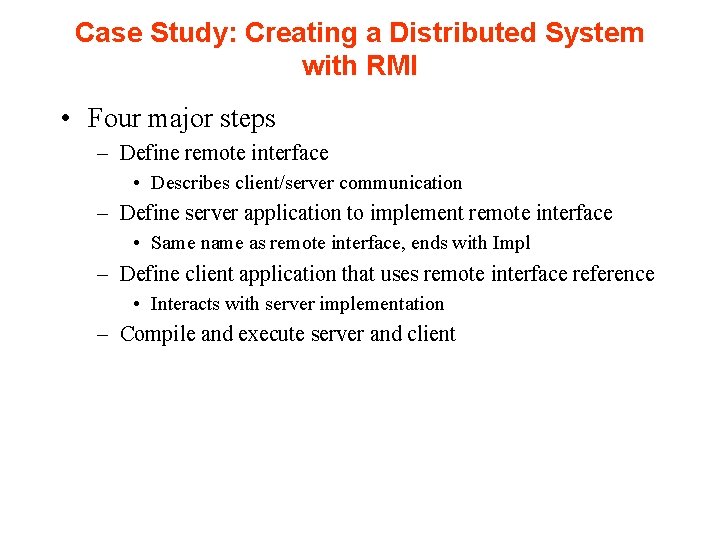
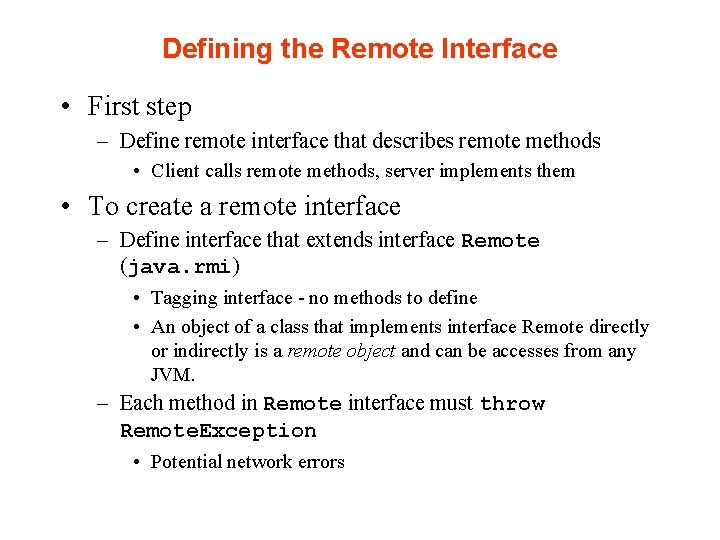
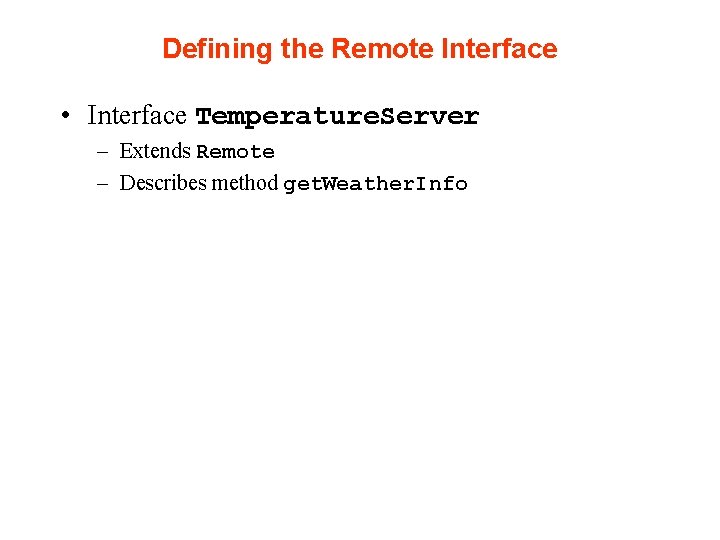
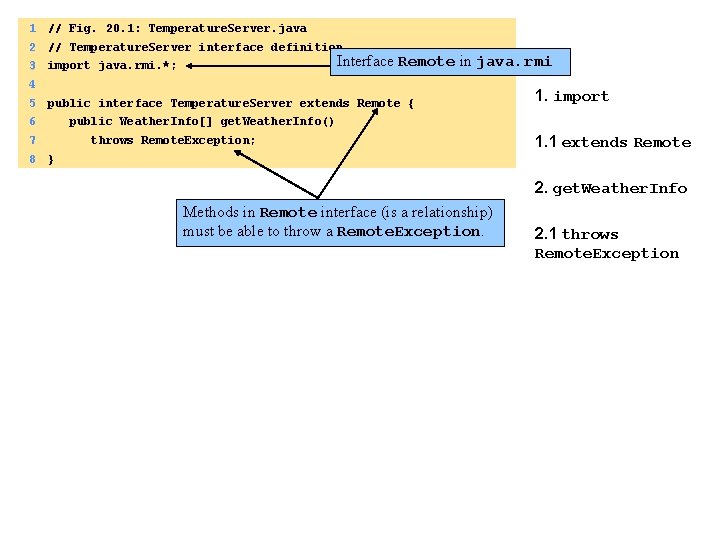
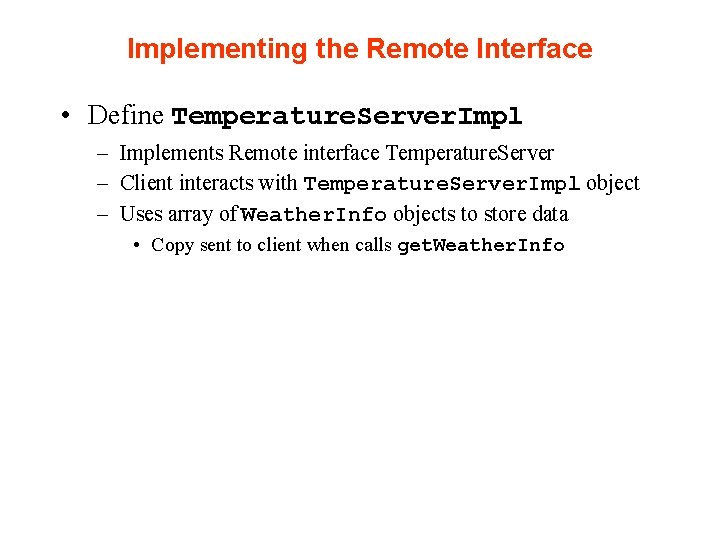
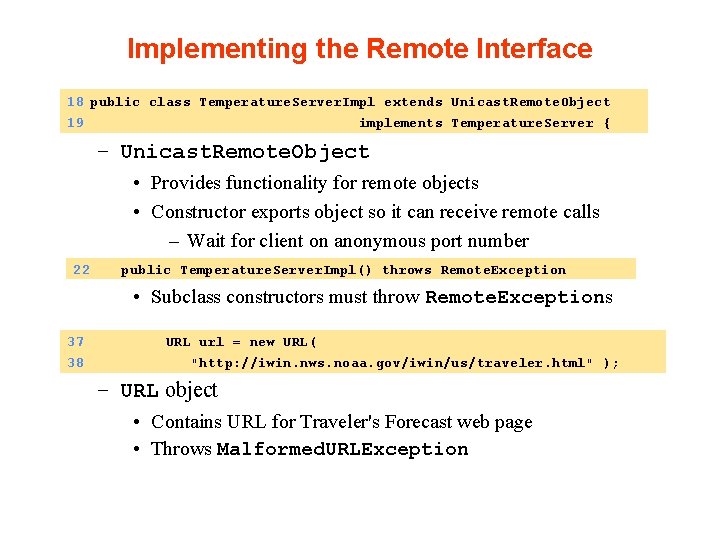
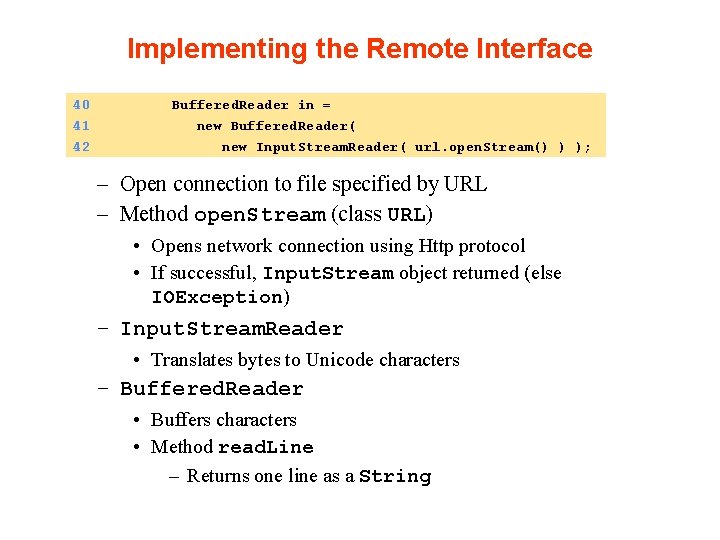
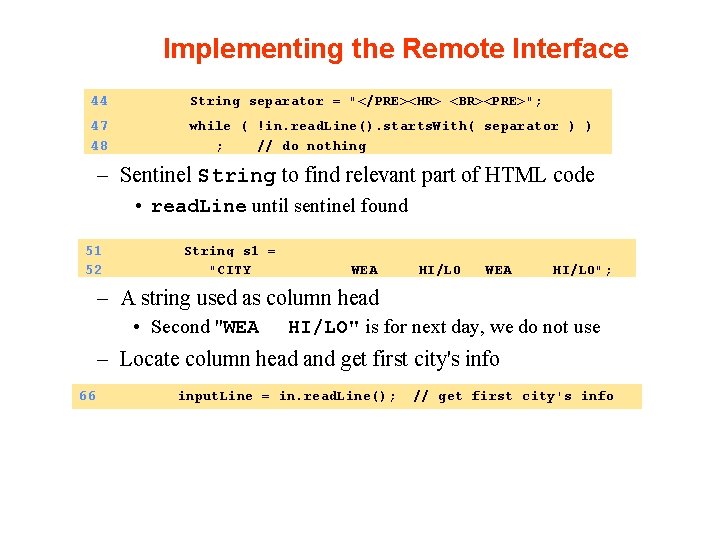
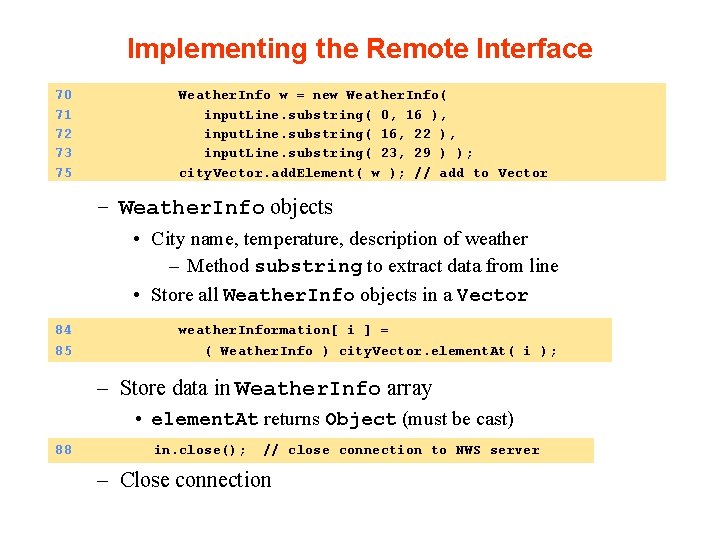
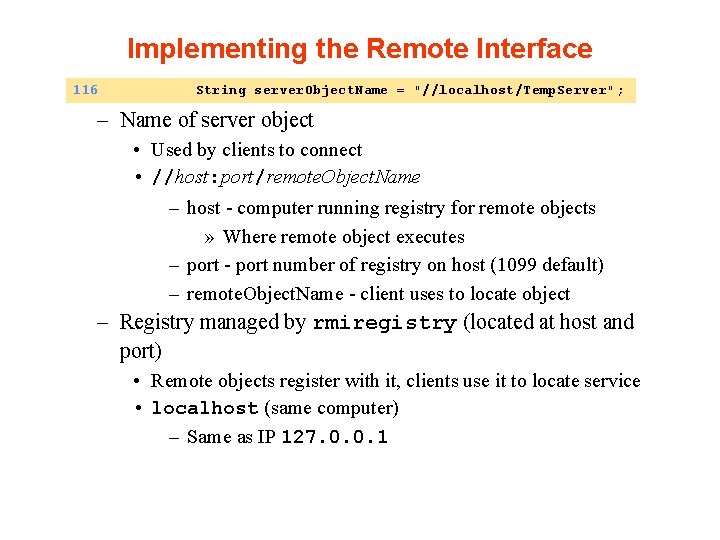
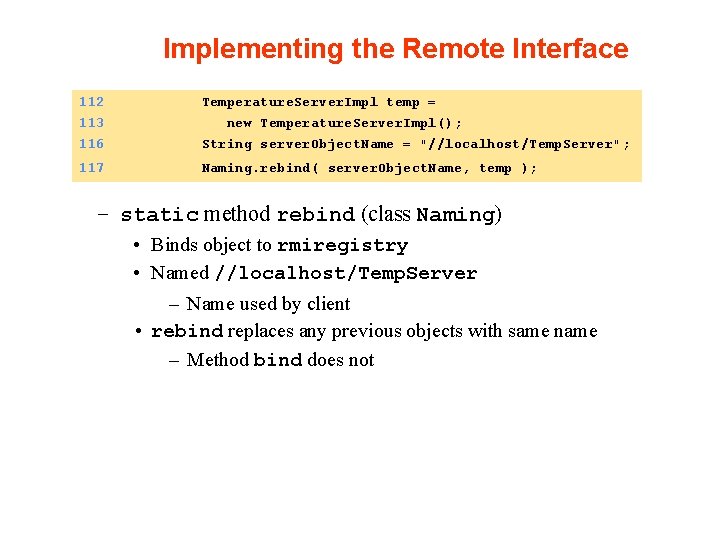
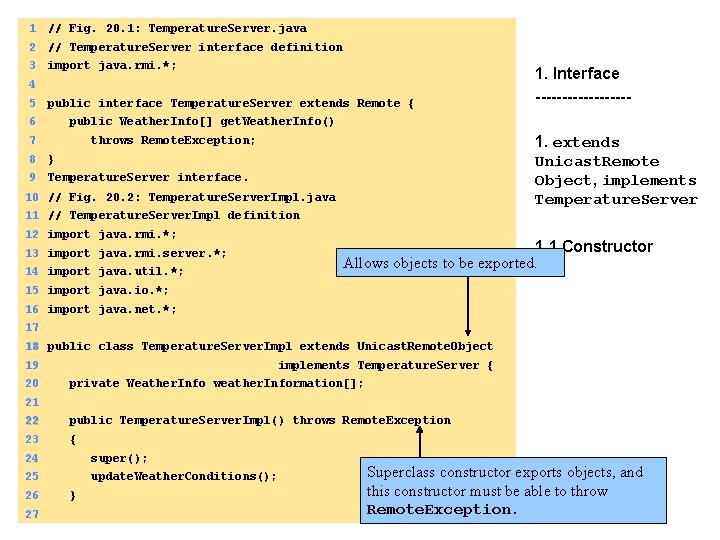
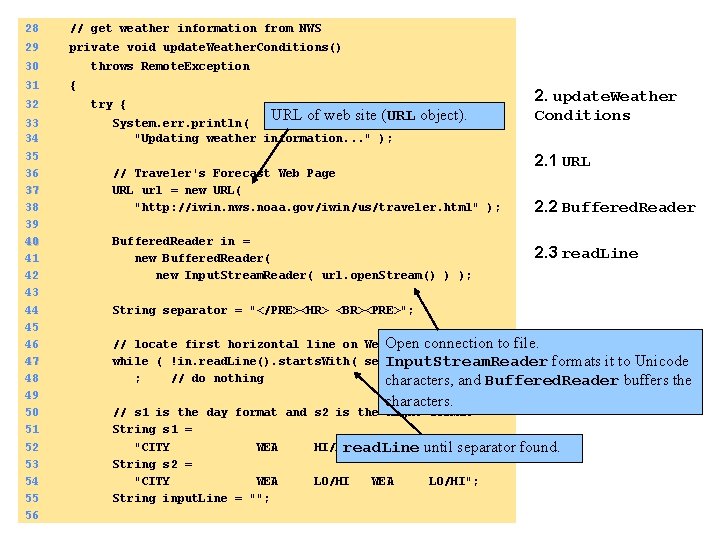
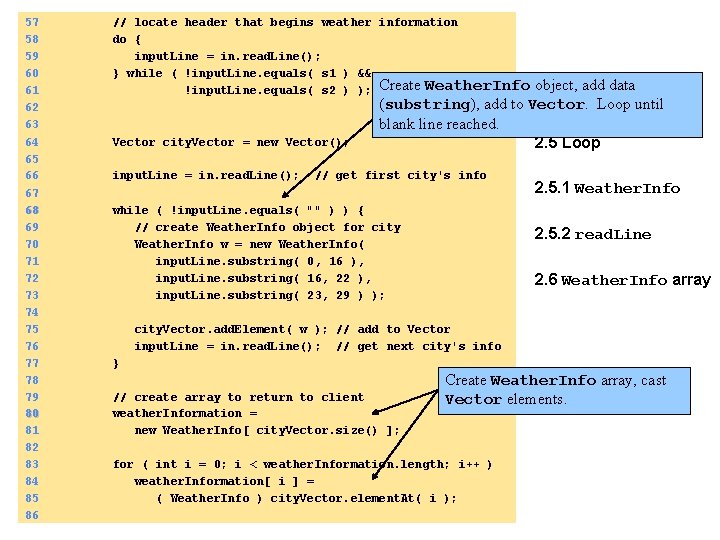
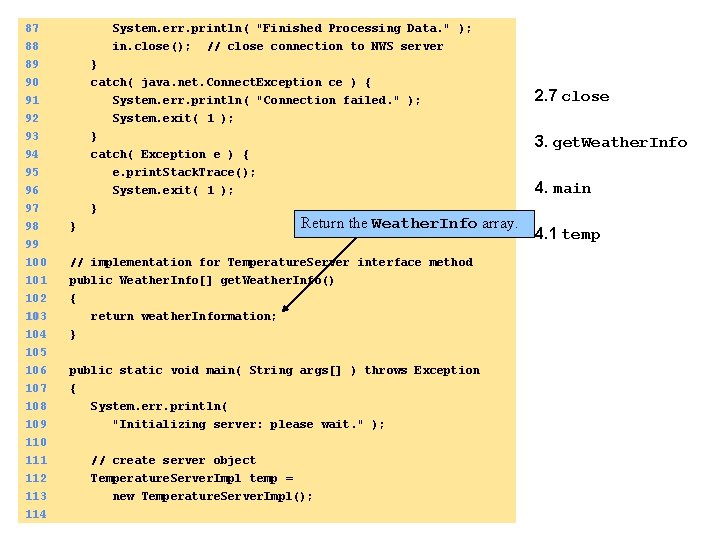
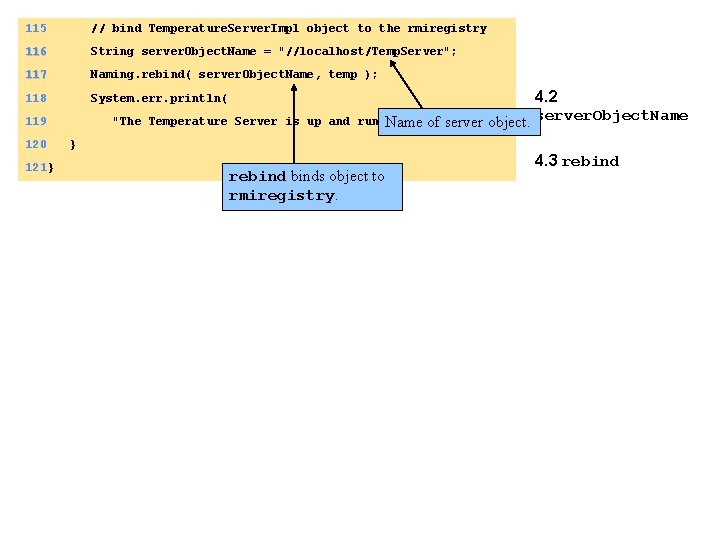
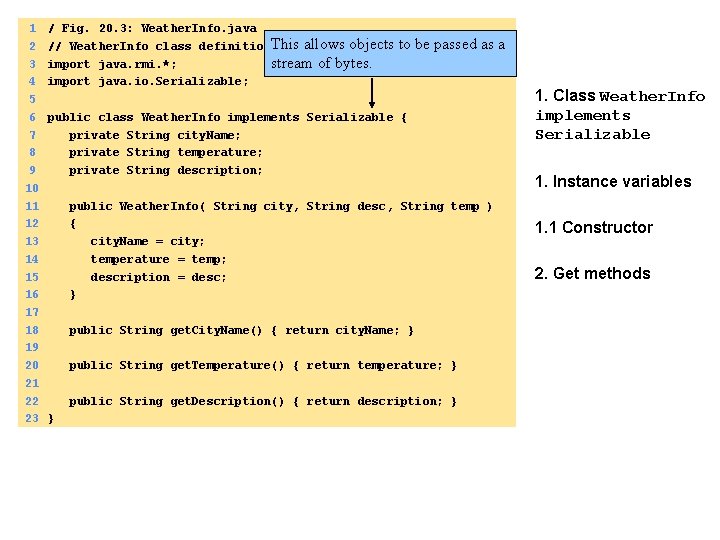
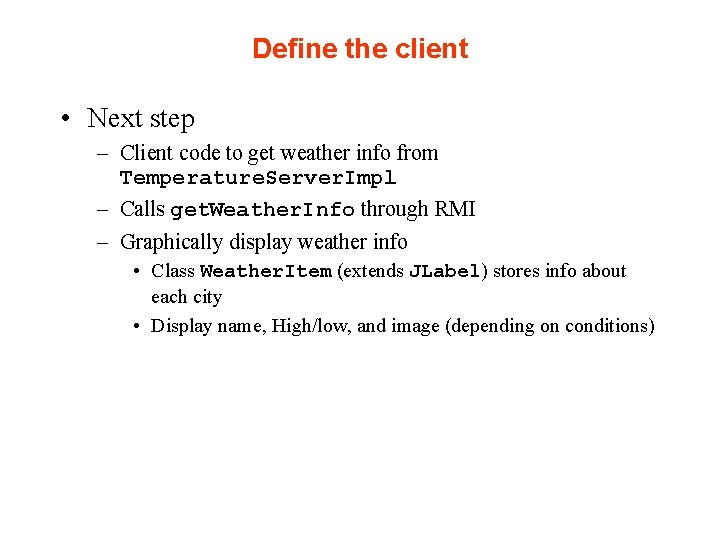
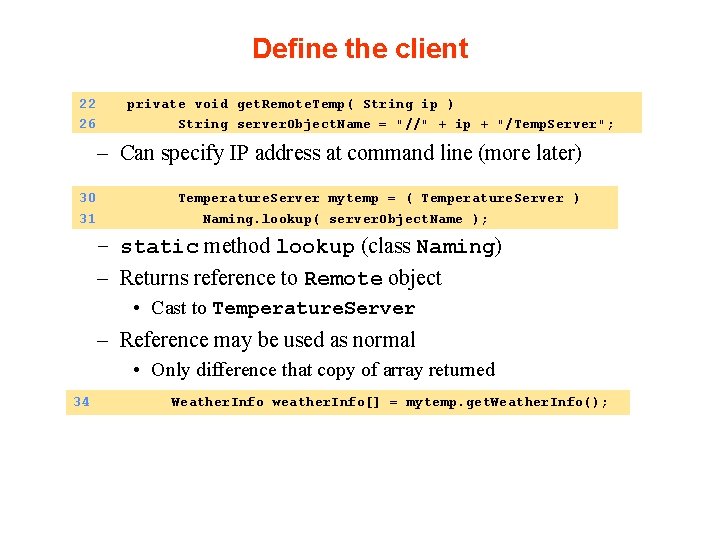
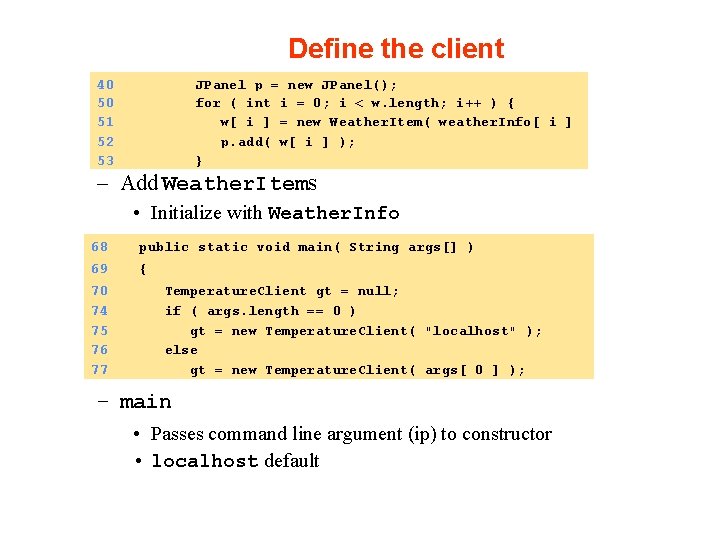
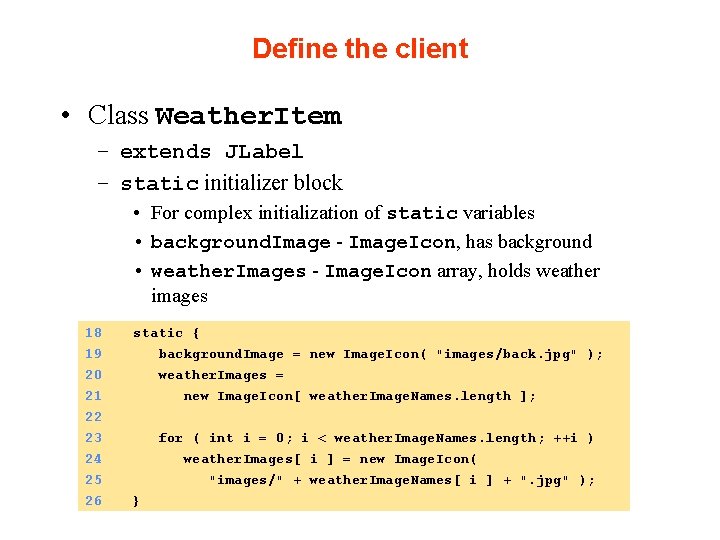
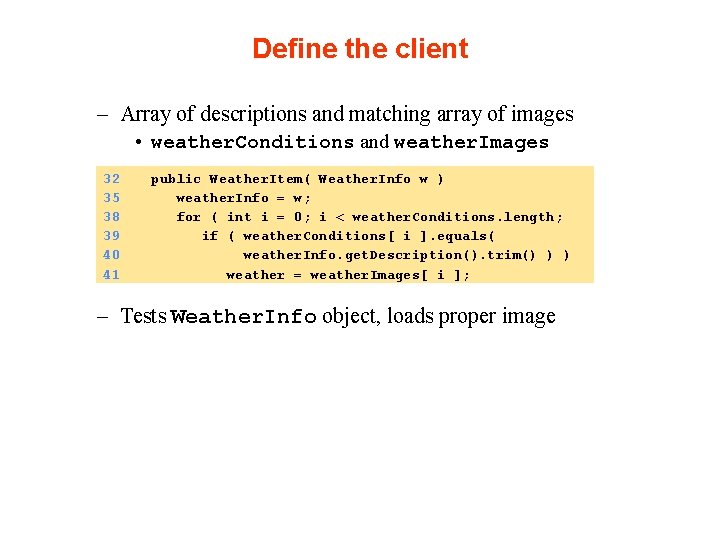
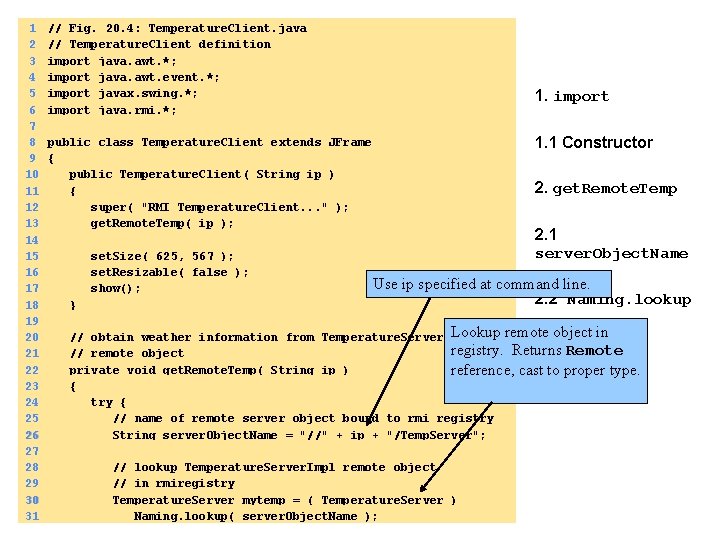
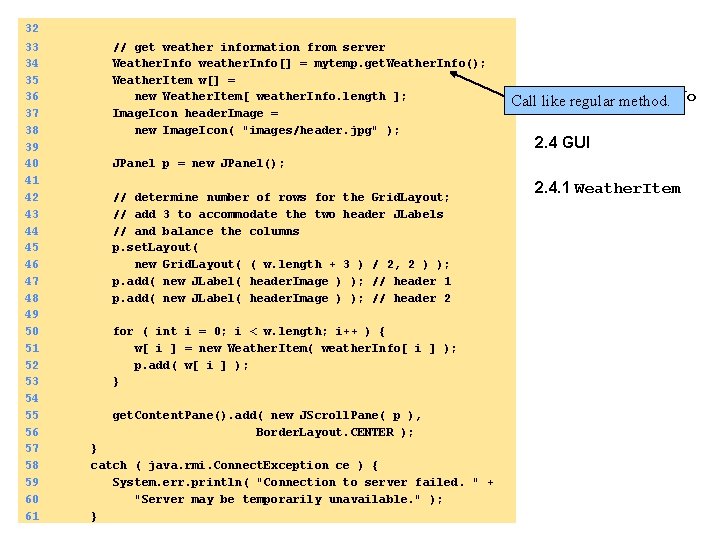
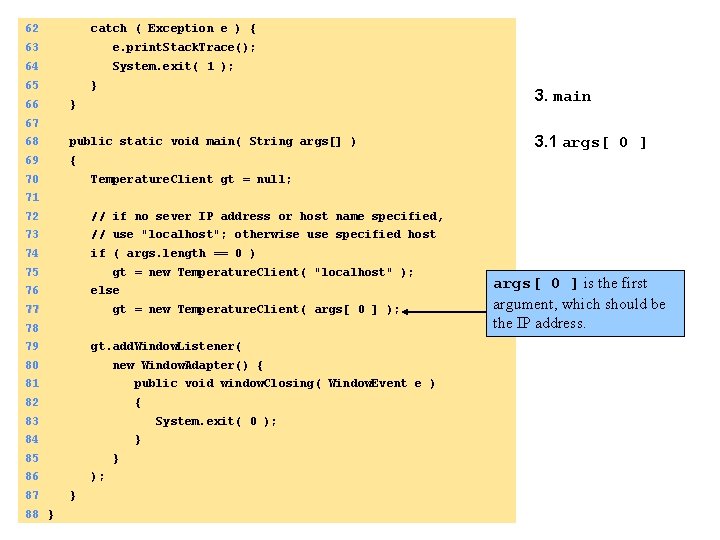
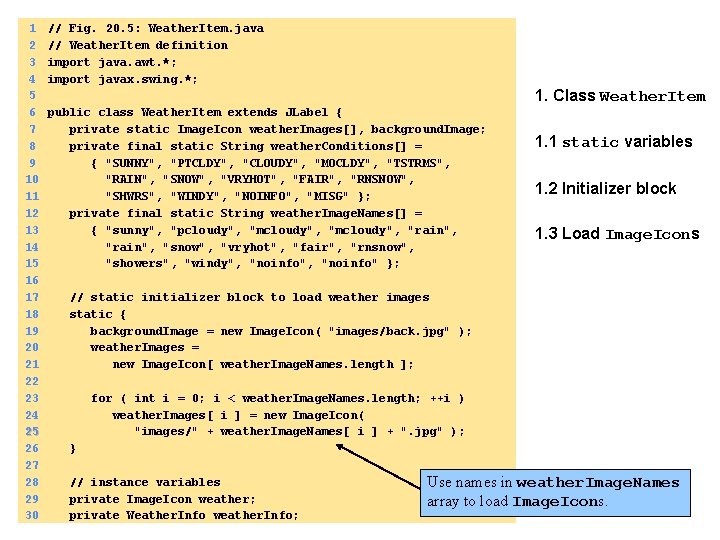
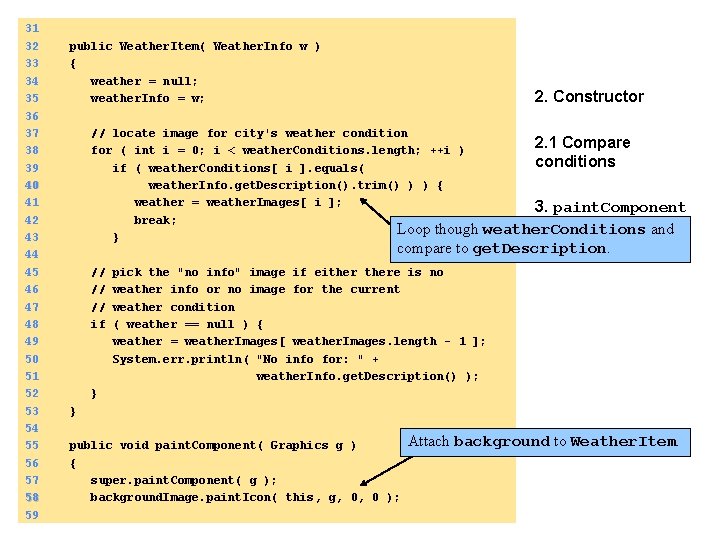
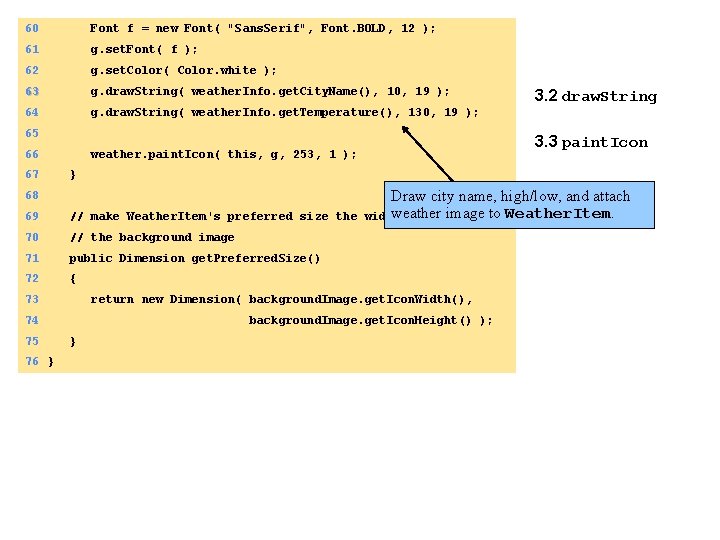
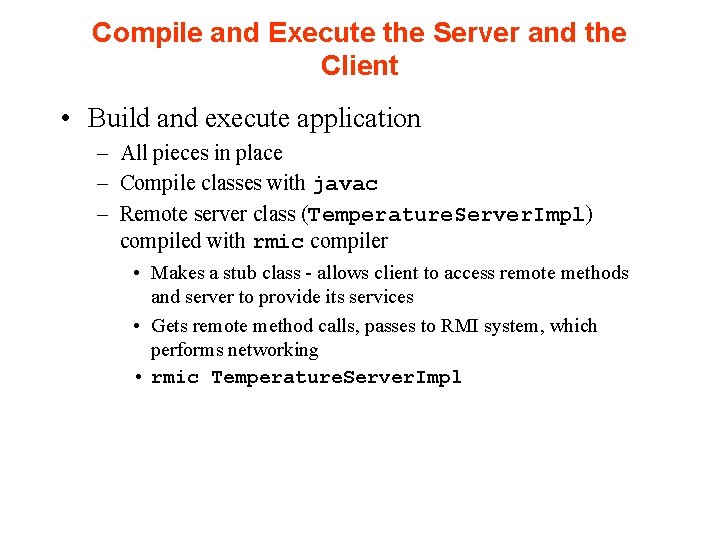
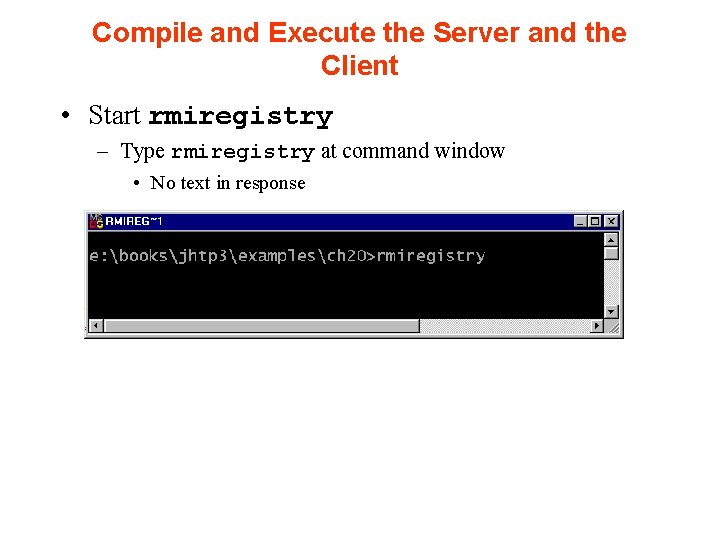
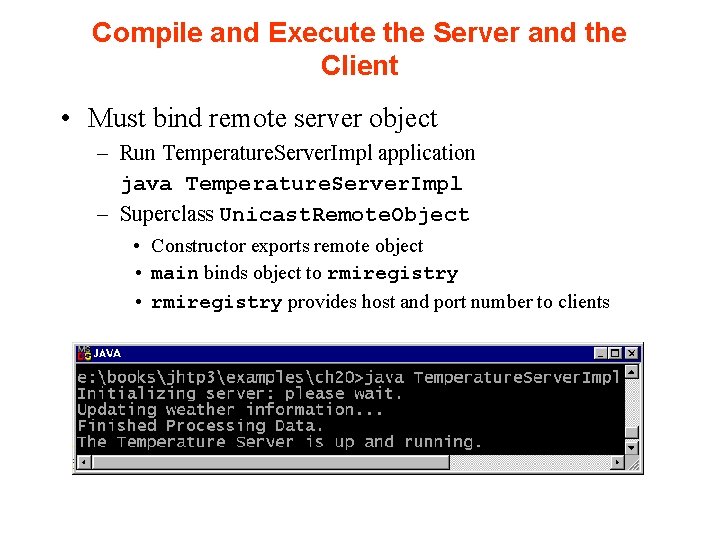
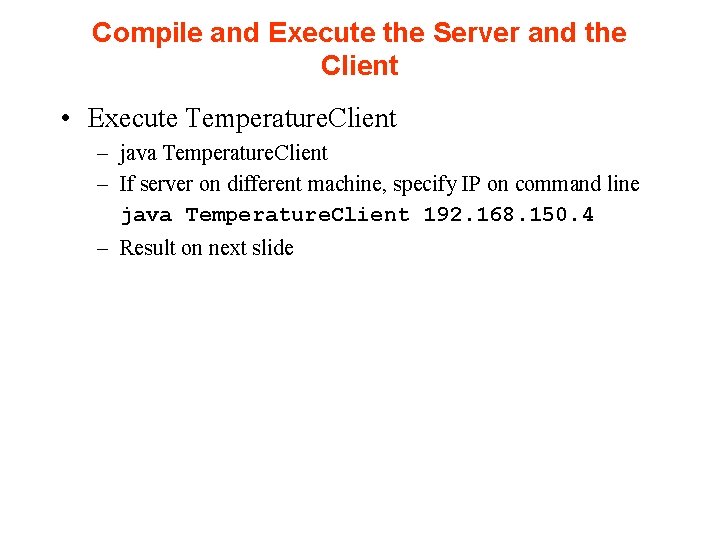
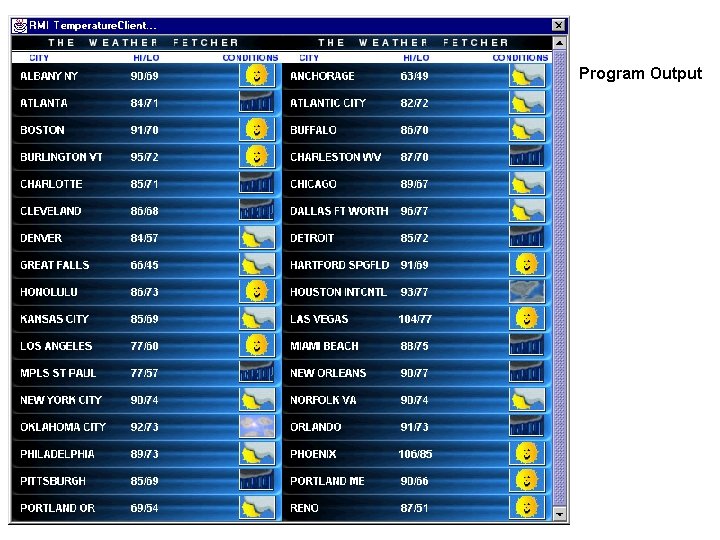
- Slides: 46
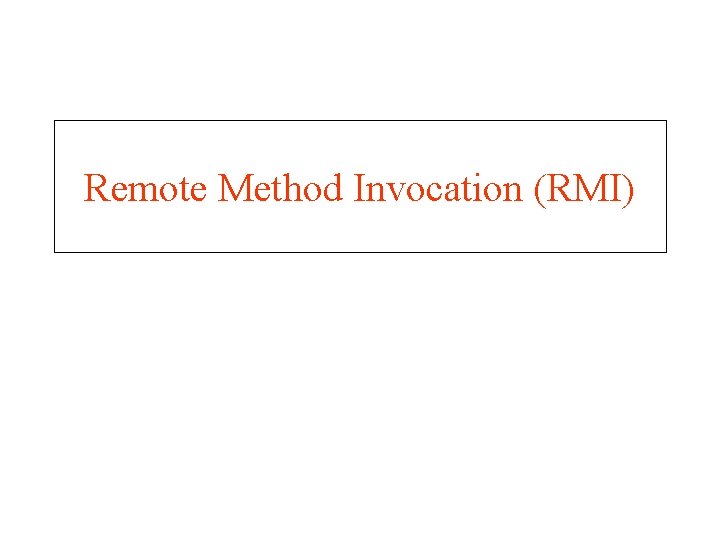
Remote Method Invocation (RMI)
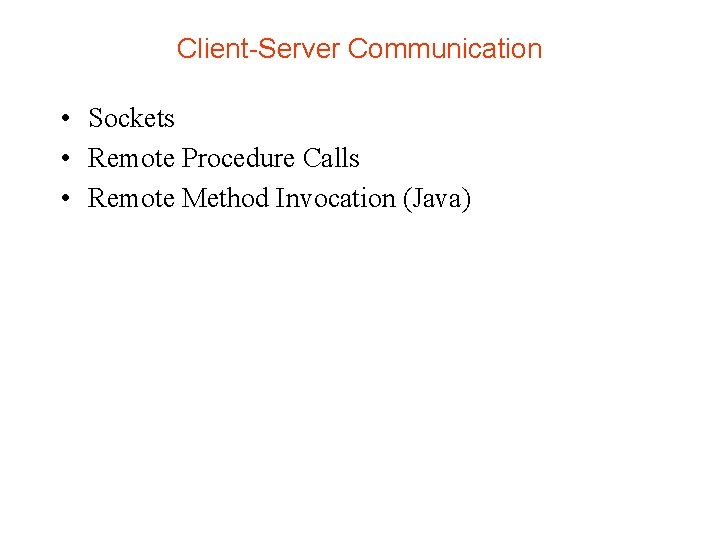
Client-Server Communication • Sockets • Remote Procedure Calls • Remote Method Invocation (Java)
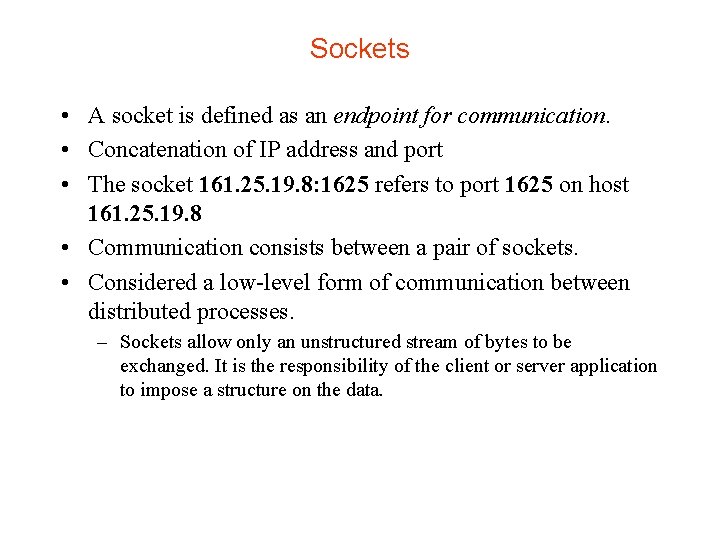
Sockets • A socket is defined as an endpoint for communication. • Concatenation of IP address and port • The socket 161. 25. 19. 8: 1625 refers to port 1625 on host 161. 25. 19. 8 • Communication consists between a pair of sockets. • Considered a low-level form of communication between distributed processes. – Sockets allow only an unstructured stream of bytes to be exchanged. It is the responsibility of the client or server application to impose a structure on the data.
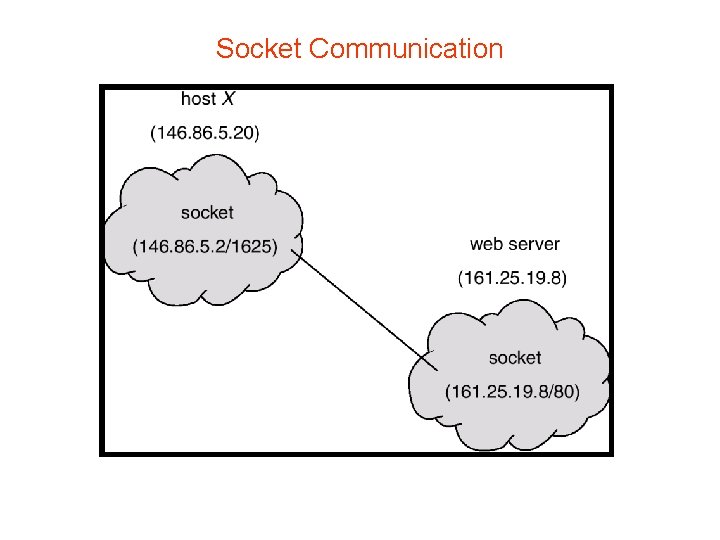
Socket Communication
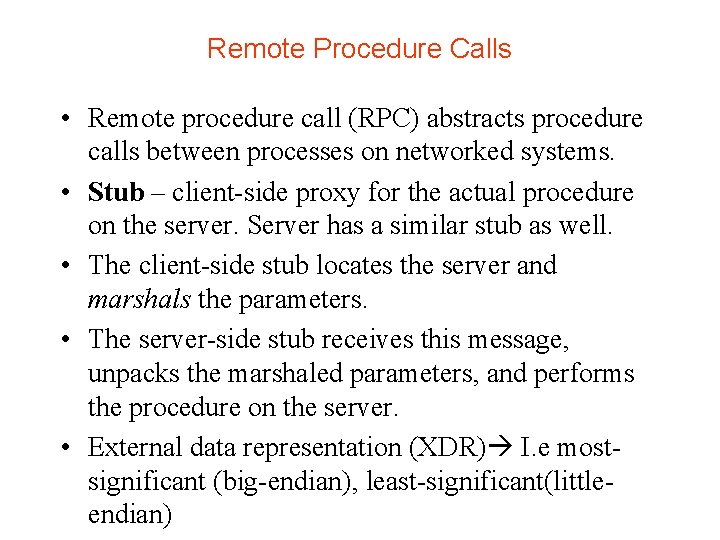
Remote Procedure Calls • Remote procedure call (RPC) abstracts procedure calls between processes on networked systems. • Stub – client-side proxy for the actual procedure on the server. Server has a similar stub as well. • The client-side stub locates the server and marshals the parameters. • The server-side stub receives this message, unpacks the marshaled parameters, and performs the procedure on the server. • External data representation (XDR) I. e mostsignificant (big-endian), least-significant(littleendian)
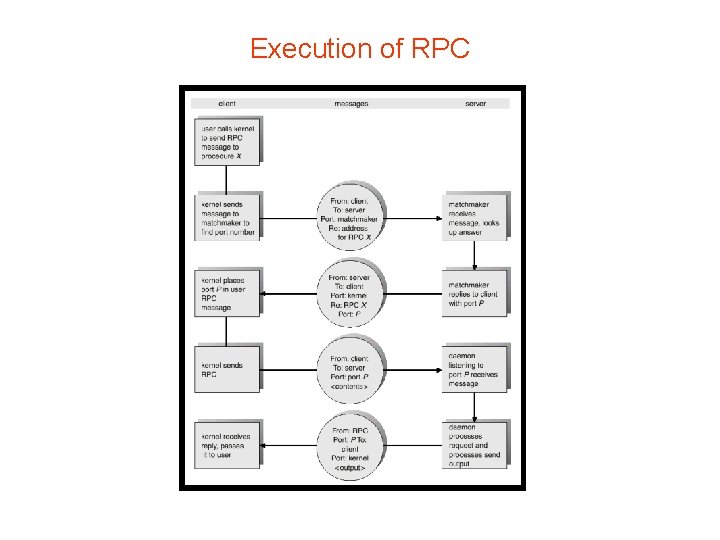
Execution of RPC
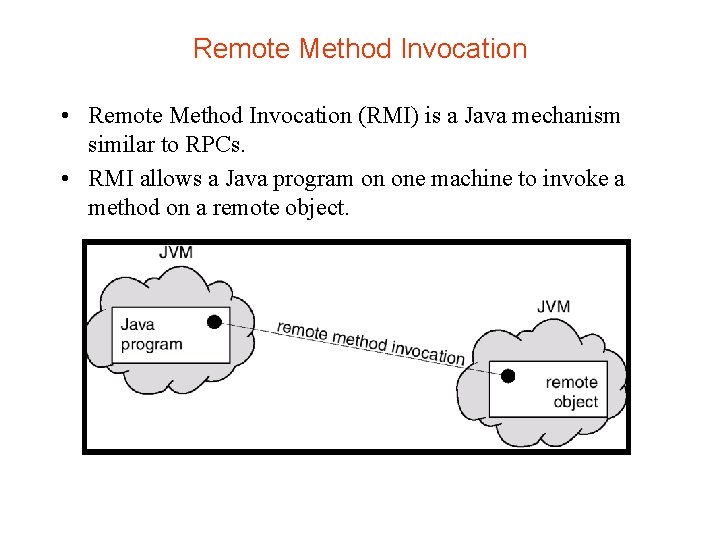
Remote Method Invocation • Remote Method Invocation (RMI) is a Java mechanism similar to RPCs. • RMI allows a Java program on one machine to invoke a method on a remote object.
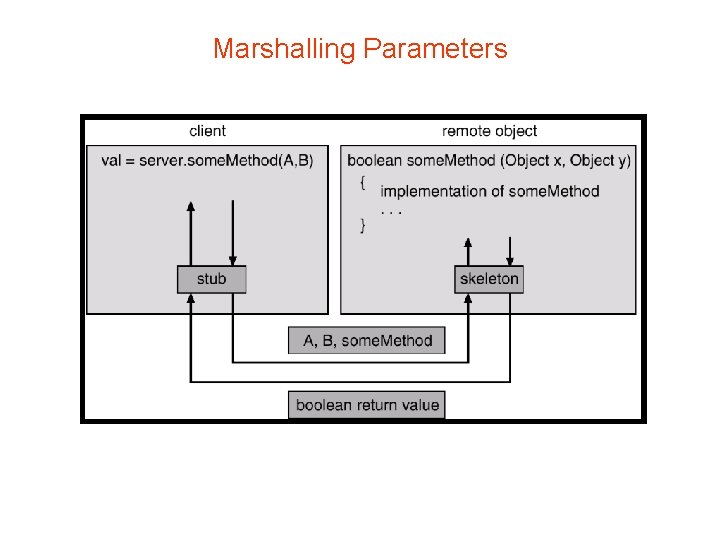
Marshalling Parameters
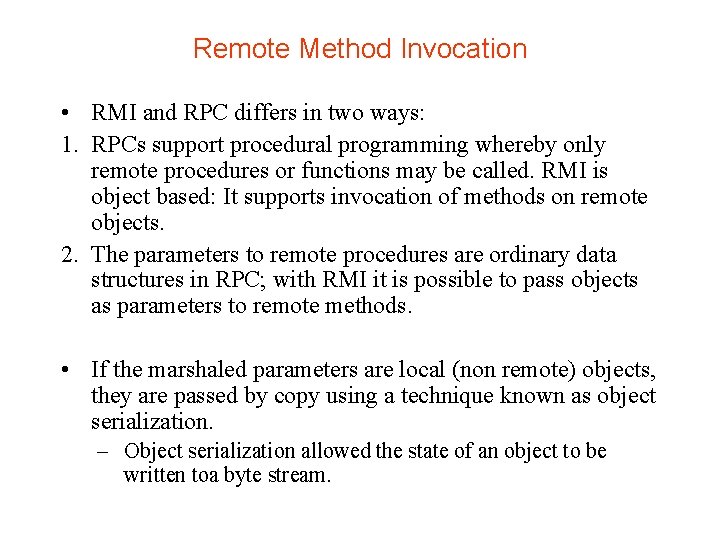
Remote Method Invocation • RMI and RPC differs in two ways: 1. RPCs support procedural programming whereby only remote procedures or functions may be called. RMI is object based: It supports invocation of methods on remote objects. 2. The parameters to remote procedures are ordinary data structures in RPC; with RMI it is possible to pass objects as parameters to remote methods. • If the marshaled parameters are local (non remote) objects, they are passed by copy using a technique known as object serialization. – Object serialization allowed the state of an object to be written toa byte stream.
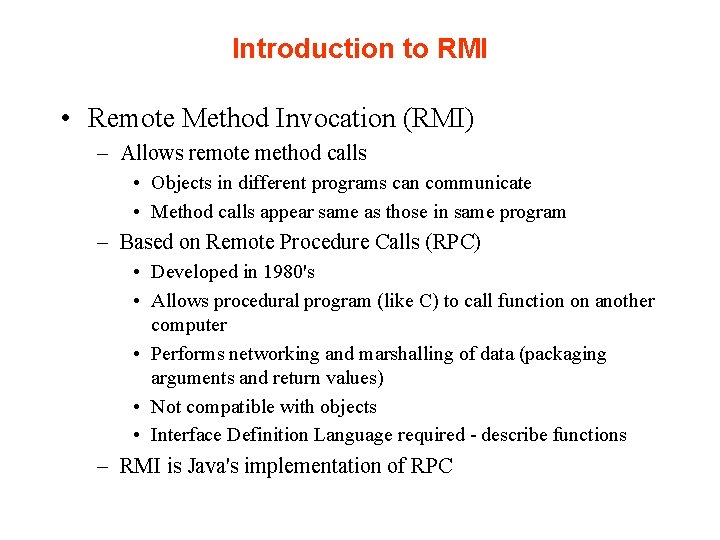
Introduction to RMI • Remote Method Invocation (RMI) – Allows remote method calls • Objects in different programs can communicate • Method calls appear same as those in same program – Based on Remote Procedure Calls (RPC) • Developed in 1980's • Allows procedural program (like C) to call function on another computer • Performs networking and marshalling of data (packaging arguments and return values) • Not compatible with objects • Interface Definition Language required - describe functions – RMI is Java's implementation of RPC
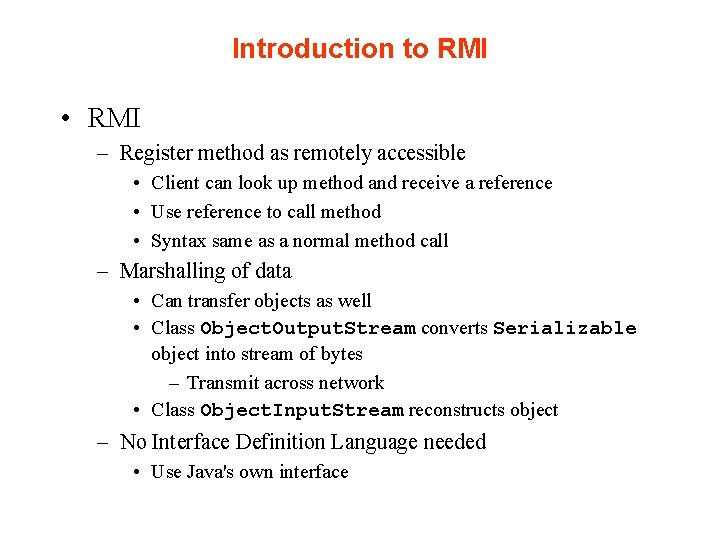
Introduction to RMI • RMI – Register method as remotely accessible • Client can look up method and receive a reference • Use reference to call method • Syntax same as a normal method call – Marshalling of data • Can transfer objects as well • Class Object. Output. Stream converts Serializable object into stream of bytes – Transmit across network • Class Object. Input. Stream reconstructs object – No Interface Definition Language needed • Use Java's own interface
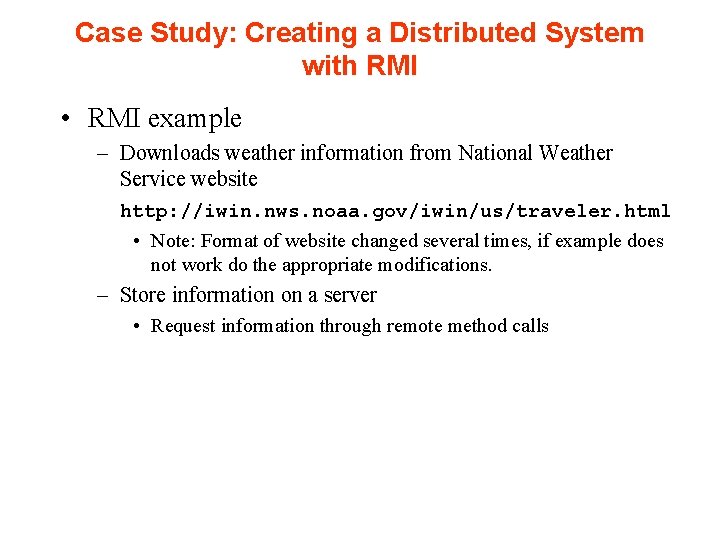
Case Study: Creating a Distributed System with RMI • RMI example – Downloads weather information from National Weather Service website http: //iwin. nws. noaa. gov/iwin/us/traveler. html • Note: Format of website changed several times, if example does not work do the appropriate modifications. – Store information on a server • Request information through remote method calls
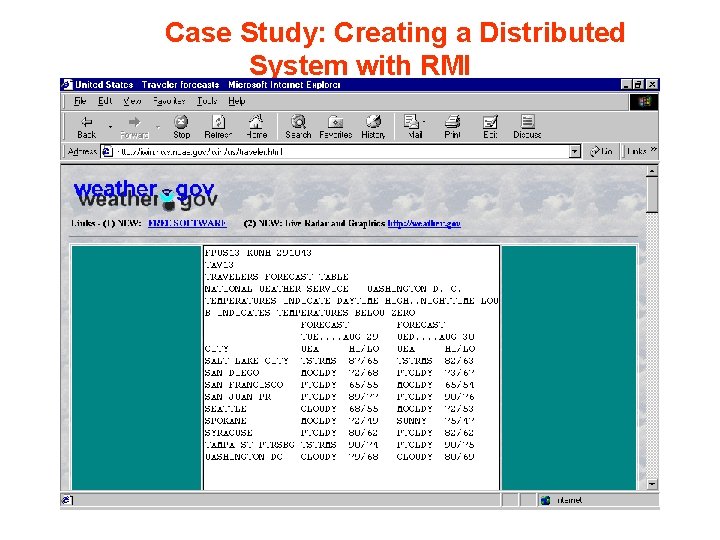
Case Study: Creating a Distributed System with RMI
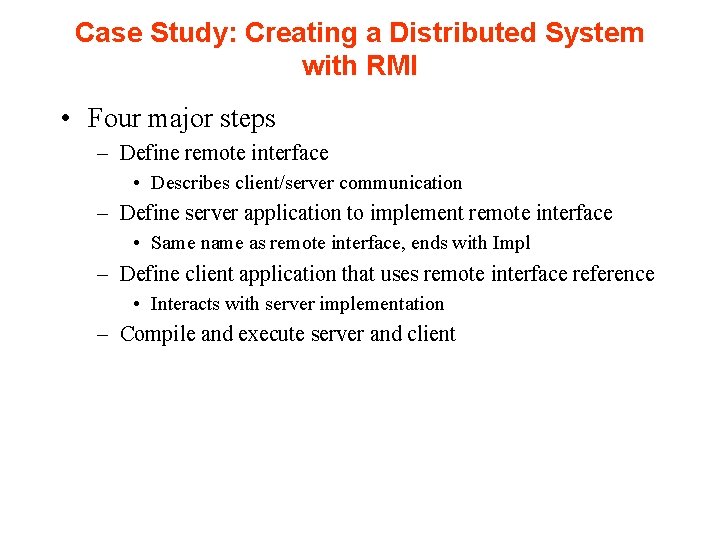
Case Study: Creating a Distributed System with RMI • Four major steps – Define remote interface • Describes client/server communication – Define server application to implement remote interface • Same name as remote interface, ends with Impl – Define client application that uses remote interface reference • Interacts with server implementation – Compile and execute server and client
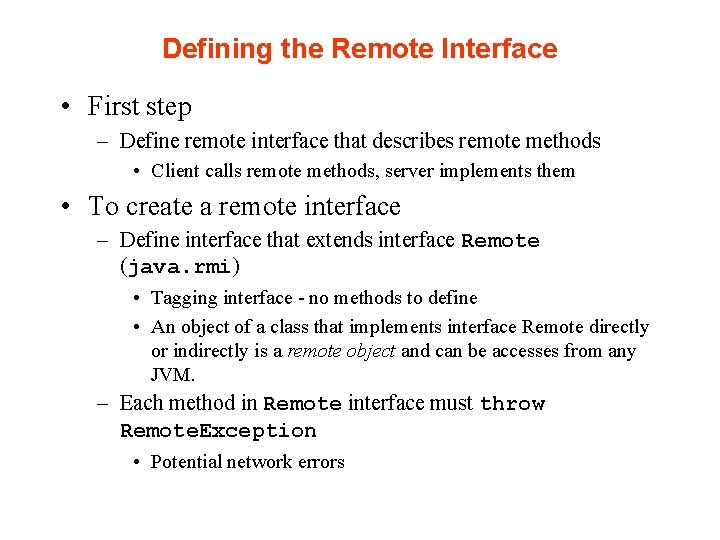
Defining the Remote Interface • First step – Define remote interface that describes remote methods • Client calls remote methods, server implements them • To create a remote interface – Define interface that extends interface Remote (java. rmi) • Tagging interface - no methods to define • An object of a class that implements interface Remote directly or indirectly is a remote object and can be accesses from any JVM. – Each method in Remote interface must throw Remote. Exception • Potential network errors
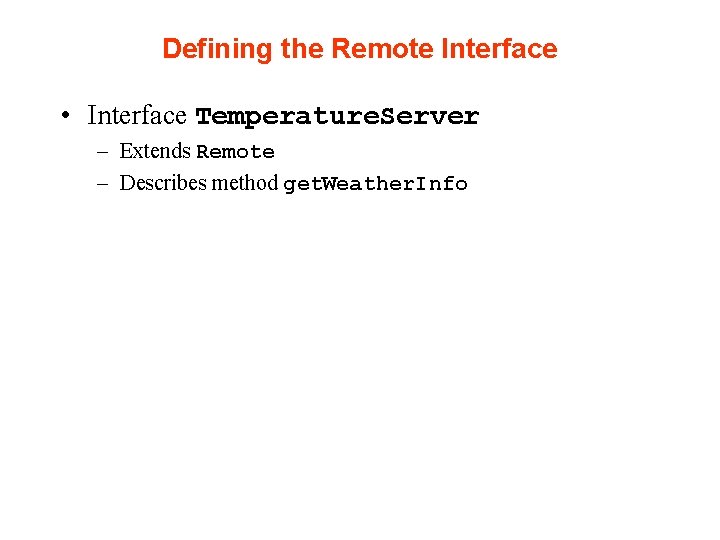
Defining the Remote Interface • Interface Temperature. Server – Extends Remote – Describes method get. Weather. Info
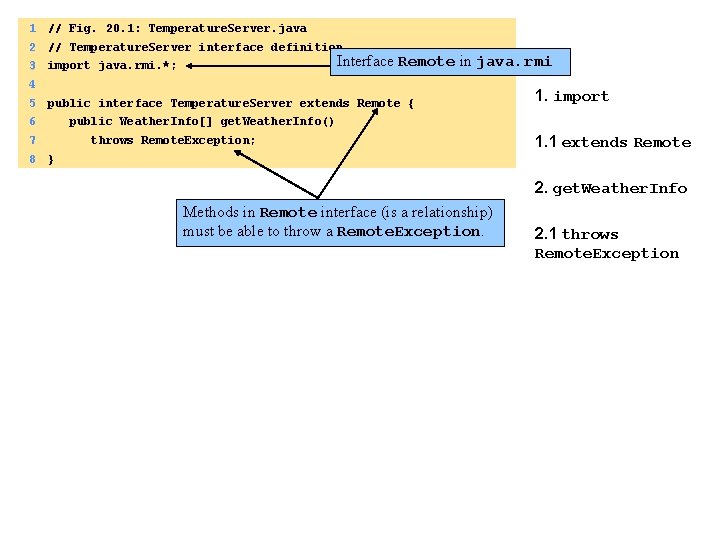
1 // Fig. 20. 1: Temperature. Server. java 2 // Temperature. Server interface definition Interface Remote in java. rmi 3 import java. rmi. *; 4 5 public interface Temperature. Server extends Remote { 6 public Weather. Info[] get. Weather. Info() 7 8 1. import throws Remote. Exception; 1. 1 extends Remote } 2. get. Weather. Info Methods in Remote interface (is a relationship) must be able to throw a Remote. Exception. 2. 1 throws Remote. Exception
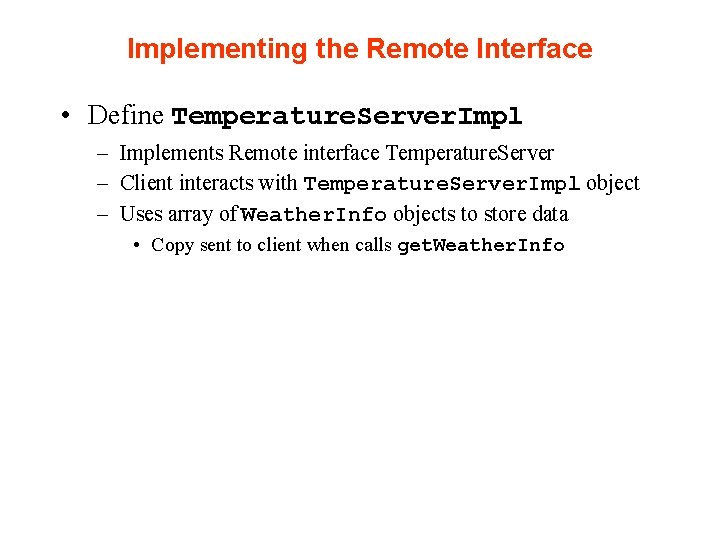
Implementing the Remote Interface • Define Temperature. Server. Impl – Implements Remote interface Temperature. Server – Client interacts with Temperature. Server. Impl object – Uses array of Weather. Info objects to store data • Copy sent to client when calls get. Weather. Info
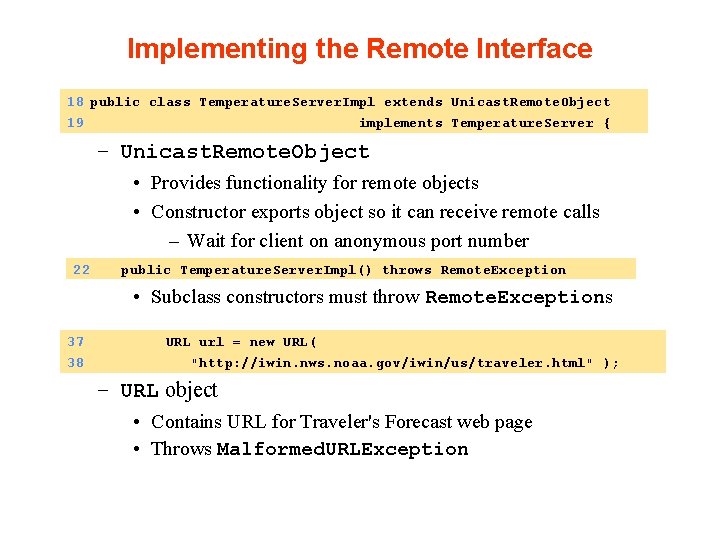
Implementing the Remote Interface 18 public class Temperature. Server. Impl extends Unicast. Remote. Object 19 implements Temperature. Server { – Unicast. Remote. Object • Provides functionality for remote objects • Constructor exports object so it can receive remote calls – Wait for client on anonymous port number 22 public Temperature. Server. Impl() throws Remote. Exception • Subclass constructors must throw Remote. Exceptions 37 38 URL url = new URL( "http: //iwin. nws. noaa. gov/iwin/us/traveler. html" ); – URL object • Contains URL for Traveler's Forecast web page • Throws Malformed. URLException
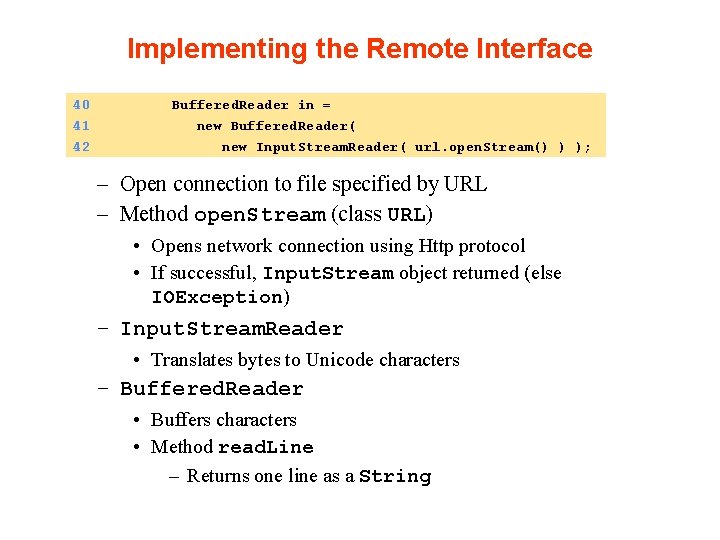
Implementing the Remote Interface 40 41 42 Buffered. Reader in = new Buffered. Reader( new Input. Stream. Reader( url. open. Stream() ) ); – Open connection to file specified by URL – Method open. Stream (class URL) • Opens network connection using Http protocol • If successful, Input. Stream object returned (else IOException) – Input. Stream. Reader • Translates bytes to Unicode characters – Buffered. Reader • Buffers characters • Method read. Line – Returns one line as a String
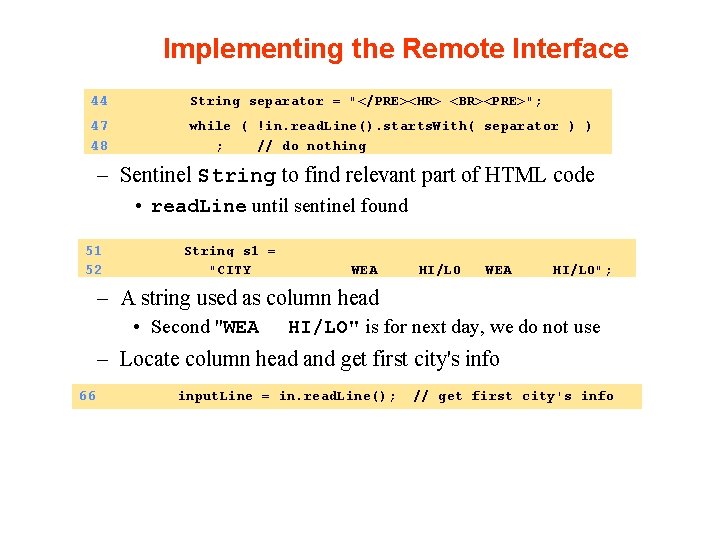
Implementing the Remote Interface 44 String separator = "</PRE><HR> <BR><PRE>"; 47 48 while ( !in. read. Line(). starts. With( separator ) ) ; // do nothing – Sentinel String to find relevant part of HTML code • read. Line until sentinel found 51 52 String s 1 = "CITY WEA HI/LO"; – A string used as column head • Second "WEA HI/LO" is for next day, we do not use – Locate column head and get first city's info 66 input. Line = in. read. Line(); // get first city's info
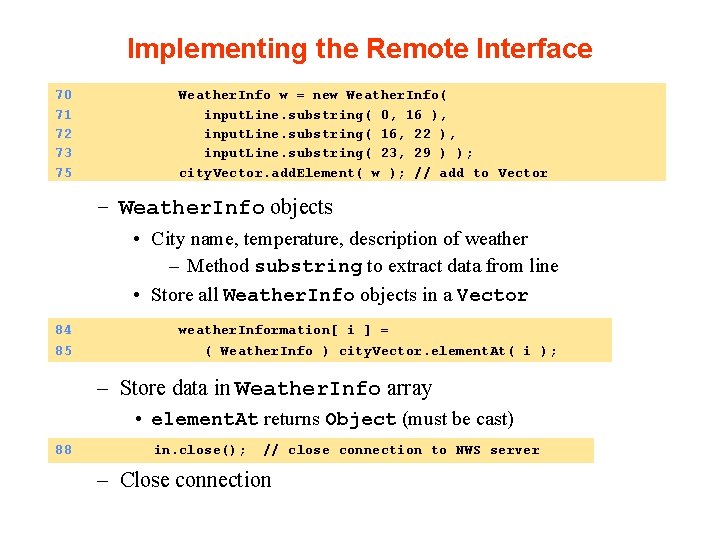
Implementing the Remote Interface 70 71 72 73 75 Weather. Info w = new Weather. Info( input. Line. substring( 0, 16 ), input. Line. substring( 16, 22 ), input. Line. substring( 23, 29 ) ); city. Vector. add. Element( w ); // add to Vector – Weather. Info objects • City name, temperature, description of weather – Method substring to extract data from line • Store all Weather. Info objects in a Vector 84 85 weather. Information[ i ] = ( Weather. Info ) city. Vector. element. At( i ); – Store data in Weather. Info array • element. At returns Object (must be cast) 88 in. close(); // close connection to NWS server – Close connection
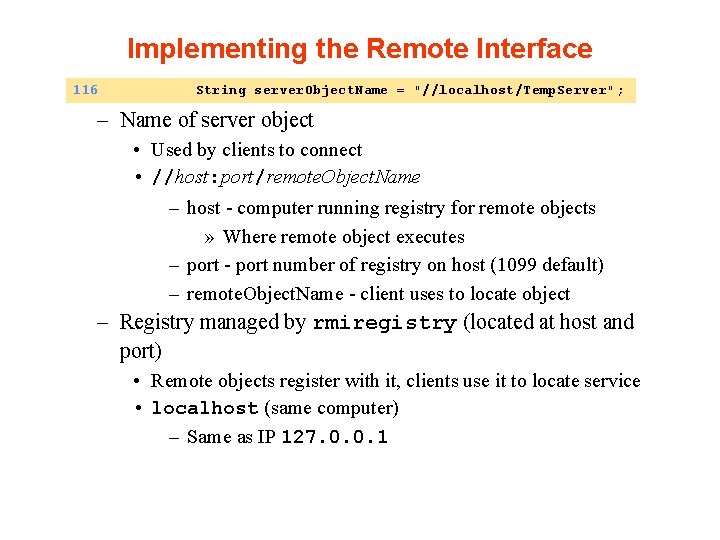
Implementing the Remote Interface 116 String server. Object. Name = "//localhost/Temp. Server"; – Name of server object • Used by clients to connect • //host: port/remote. Object. Name – host - computer running registry for remote objects » Where remote object executes – port - port number of registry on host (1099 default) – remote. Object. Name - client uses to locate object – Registry managed by rmiregistry (located at host and port) • Remote objects register with it, clients use it to locate service • localhost (same computer) – Same as IP 127. 0. 0. 1
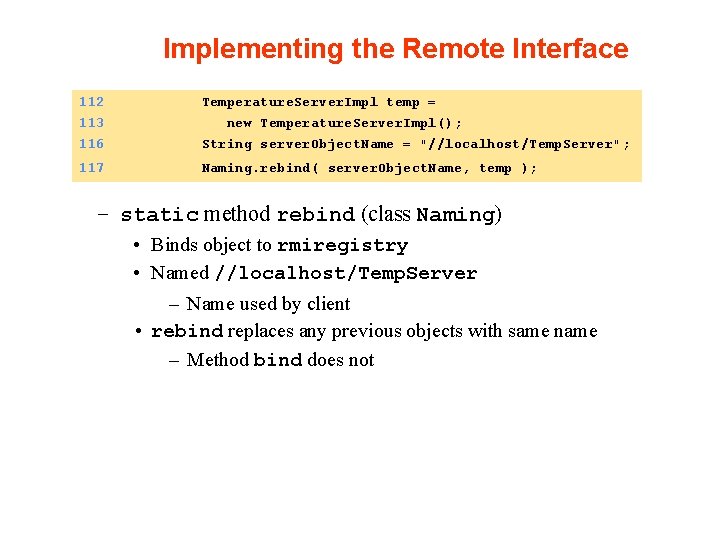
Implementing the Remote Interface 112 Temperature. Server. Impl temp = 113 116 new Temperature. Server. Impl(); String server. Object. Name = "//localhost/Temp. Server"; 117 Naming. rebind( server. Object. Name, temp ); – static method rebind (class Naming) • Binds object to rmiregistry • Named //localhost/Temp. Server – Name used by client • rebind replaces any previous objects with same name – Method bind does not
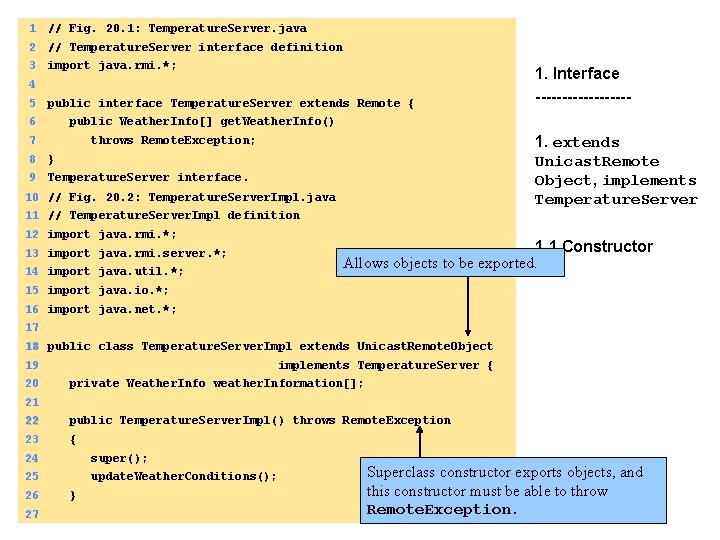
1 // Fig. 20. 1: Temperature. Server. java 2 // Temperature. Server interface definition 3 import java. rmi. *; 4 5 public interface Temperature. Server extends Remote { 6 1. Interface --------- public Weather. Info[] get. Weather. Info() 7 1. extends Unicast. Remote Object, implements Temperature. Server throws Remote. Exception; 8 } 9 Temperature. Server interface. 10 // Fig. 20. 2: Temperature. Server. Impl. java 11 // Temperature. Server. Impl definition 12 import java. rmi. *; 1. 1 Constructor Allows objects to be exported. 13 import java. rmi. server. *; 14 import java. util. *; 15 import java. io. *; 16 import java. net. *; 17 18 public class Temperature. Server. Impl extends Unicast. Remote. Object 19 20 implements Temperature. Server { private Weather. Info weather. Information[]; 21 22 public Temperature. Server. Impl() throws Remote. Exception 23 { 24 super(); 25 update. Weather. Conditions(); 26 27 } Superclass constructor exports objects, and this constructor must be able to throw Remote. Exception.
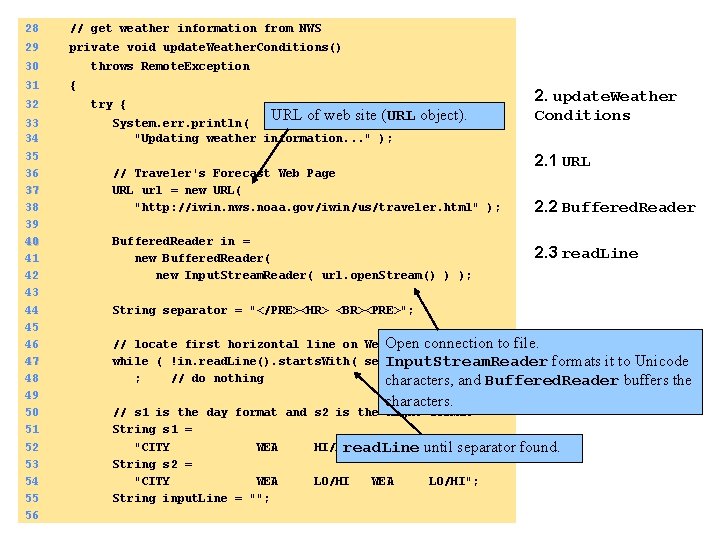
28 // get weather information from NWS 29 private void update. Weather. Conditions() 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 throws Remote. Exception { try { URL of web site (URL object). System. err. println( "Updating weather information. . . " ); // Traveler's Forecast Web Page URL url = new URL( "http: //iwin. nws. noaa. gov/iwin/us/traveler. html" ); Buffered. Reader in = new Buffered. Reader( new Input. Stream. Reader( url. open. Stream() ) ); 2. update. Weather Conditions 2. 1 URL 2. 2 Buffered. Reader 2. 3 read. Line String separator = "</PRE><HR> <BR><PRE>"; // locate first horizontal line on Web. Open page connection to file. while ( !in. read. Line(). starts. With( separator ) ) Input. Stream. Reader formats it to Unicode ; // do nothing characters, and Buffered. Reader buffers the characters. // s 1 is the day format and s 2 is the night format String s 1 = "CITY WEA HI/LO"; read. Line until separator String s 2 = "CITY WEA LO/HI"; String input. Line = ""; found.
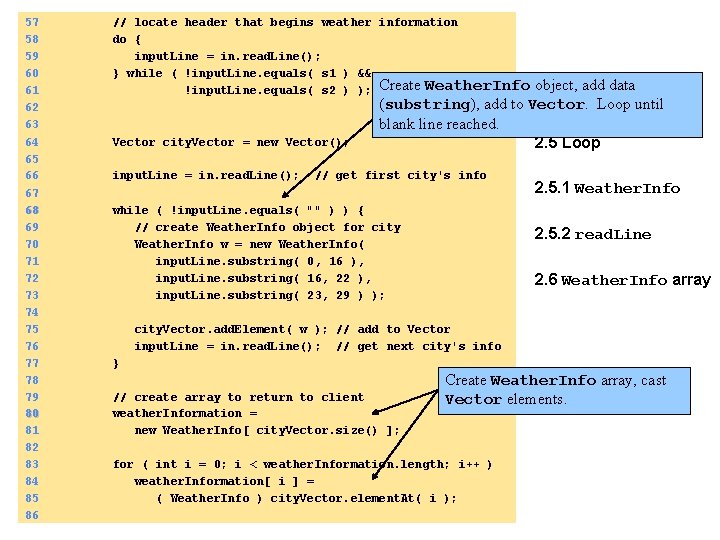
57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 // locate header that begins weather information do { input. Line = in. read. Line(); } while ( !input. Line. equals( s 1 ) && !input. Line. equals( s 2 ) ); Create Weather. Info Vector city. Vector = new Vector(); input. Line = in. read. Line(); object, add data 2. 4 Locate header (substring), add to Vector. Loop until blank line reached. 2. 5 Loop // get first city's info while ( !input. Line. equals( "" ) ) { // create Weather. Info object for city Weather. Info w = new Weather. Info( input. Line. substring( 0, 16 ), input. Line. substring( 16, 22 ), input. Line. substring( 23, 29 ) ); 2. 5. 1 Weather. Info 2. 5. 2 read. Line 2. 6 Weather. Info array city. Vector. add. Element( w ); // add to Vector input. Line = in. read. Line(); // get next city's info } // create array to return to client weather. Information = new Weather. Info[ city. Vector. size() ]; Create Weather. Info array, cast Vector elements. for ( int i = 0; i < weather. Information. length; i++ ) weather. Information[ i ] = ( Weather. Info ) city. Vector. element. At( i );
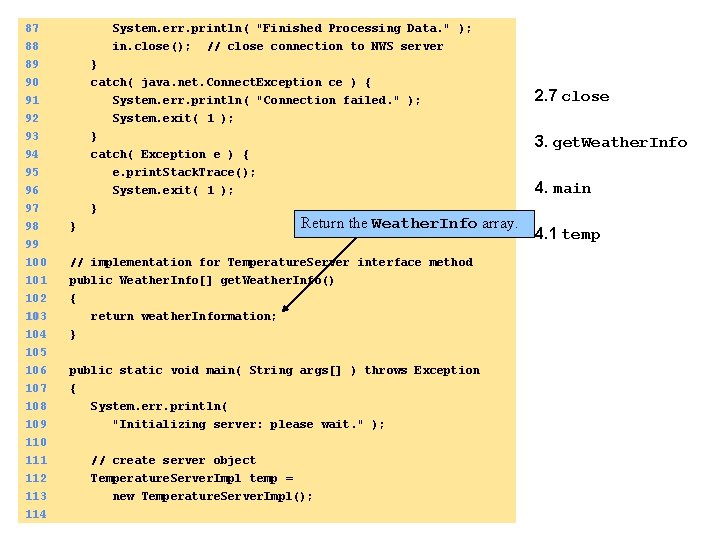
87 88 89 90 System. err. println( "Finished Processing Data. " ); in. close(); // close connection to NWS server } catch( java. net. Connect. Exception ce ) { 91 92 System. err. println( "Connection failed. " ); System. exit( 1 ); 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 } catch( Exception e ) { e. print. Stack. Trace(); 3. get. Weather. Info 4. main System. exit( 1 ); } } 2. 7 close Return the Weather. Info array. // implementation for Temperature. Server interface method public Weather. Info[] get. Weather. Info() { return weather. Information; } public static void main( String args[] ) throws Exception { System. err. println( "Initializing server: please wait. " ); // create server object Temperature. Server. Impl temp = new Temperature. Server. Impl(); 4. 1 temp
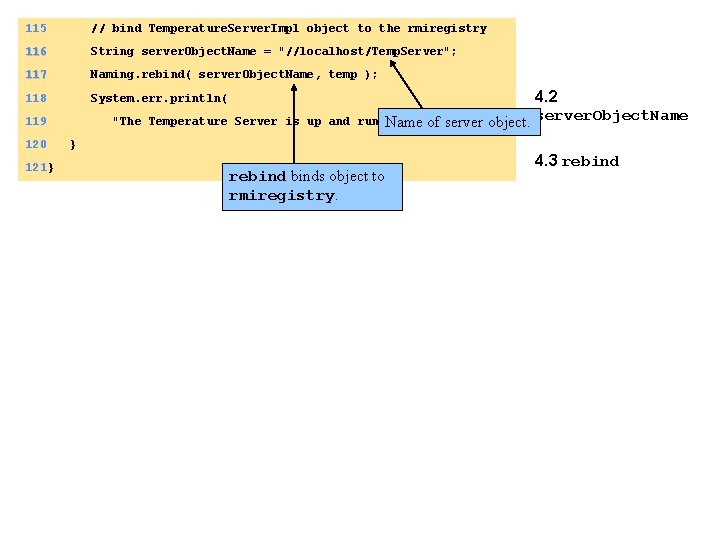
115 // bind Temperature. Server. Impl object to the rmiregistry 116 String server. Object. Name = "//localhost/Temp. Server"; 117 Naming. rebind( server. Object. Name, temp ); 118 System. err. println( 119 120 121 } "The Temperature Server is up and 4. 2 running. " ); server object. server. Object. Name of } rebinds object to rmiregistry. 4. 3 rebind
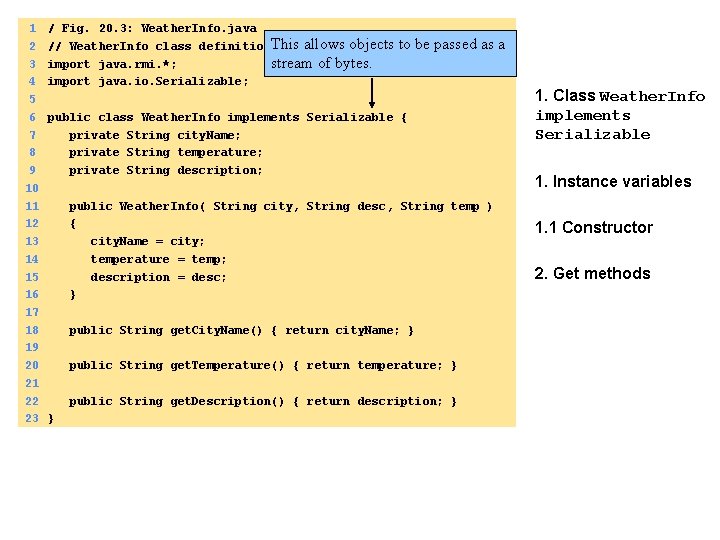
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 / Fig. 20. 3: Weather. Info. java // Weather. Info class definition. This allows objects import java. rmi. *; stream of bytes. import java. io. Serializable; to be passed as a public class Weather. Info implements Serializable { private String city. Name; private String temperature; private String description; public Weather. Info( String city, String desc, String temp ) { city. Name = city; temperature = temp; description = desc; } public String get. City. Name() { return city. Name; } public String get. Temperature() { return temperature; } public String get. Description() { return description; } } 1. Class Weather. Info implements Serializable 1. Instance variables 1. 1 Constructor 2. Get methods
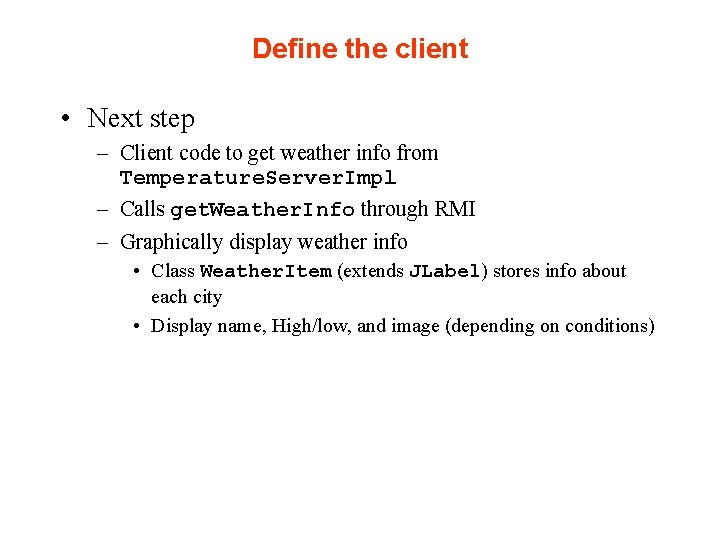
Define the client • Next step – Client code to get weather info from Temperature. Server. Impl – Calls get. Weather. Info through RMI – Graphically display weather info • Class Weather. Item (extends JLabel) stores info about each city • Display name, High/low, and image (depending on conditions)
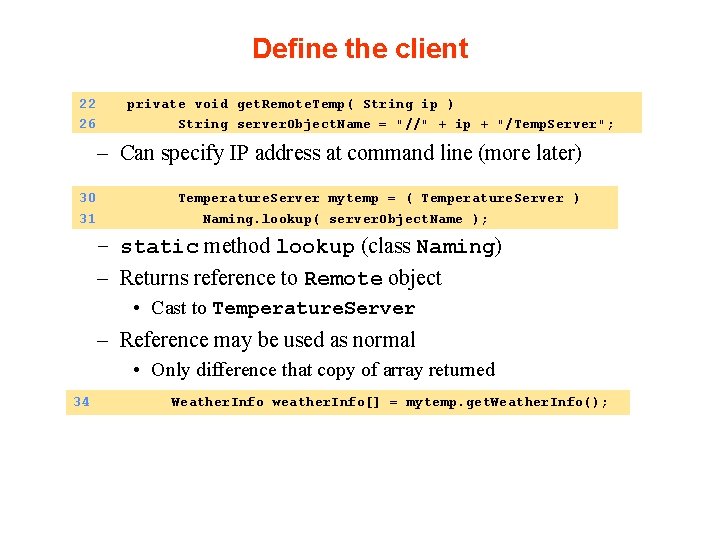
Define the client 22 26 private void get. Remote. Temp( String ip ) String server. Object. Name = "//" + ip + "/Temp. Server"; – Can specify IP address at command line (more later) 30 31 Temperature. Server mytemp = ( Temperature. Server ) Naming. lookup( server. Object. Name ); – static method lookup (class Naming) – Returns reference to Remote object • Cast to Temperature. Server – Reference may be used as normal • Only difference that copy of array returned 34 Weather. Info weather. Info[] = mytemp. get. Weather. Info();
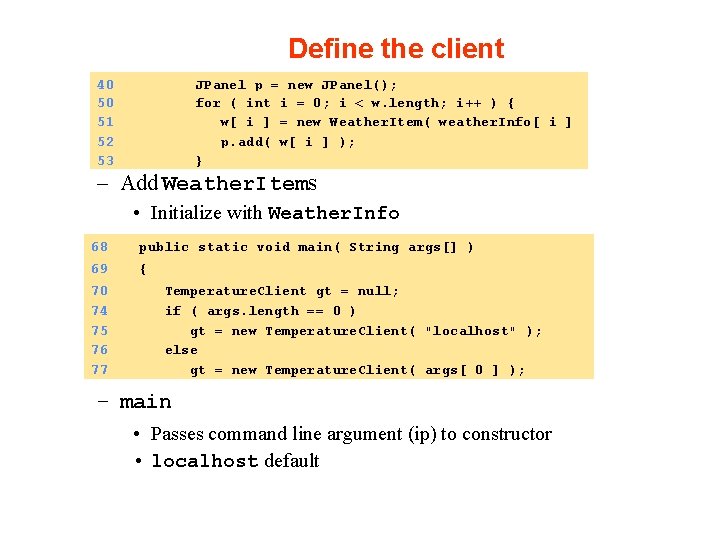
Define the client 40 50 51 ); 52 53 JPanel p = new JPanel(); for ( int i = 0; i < w. length; i++ ) { w[ i ] = new Weather. Item( weather. Info[ i ] p. add( w[ i ] ); } – Add Weather. Items • Initialize with Weather. Info 68 public static void main( String args[] ) 69 { 70 74 75 76 77 Temperature. Client gt = null; if ( args. length == 0 ) gt = new Temperature. Client( "localhost" ); else gt = new Temperature. Client( args[ 0 ] ); – main • Passes command line argument (ip) to constructor • localhost default
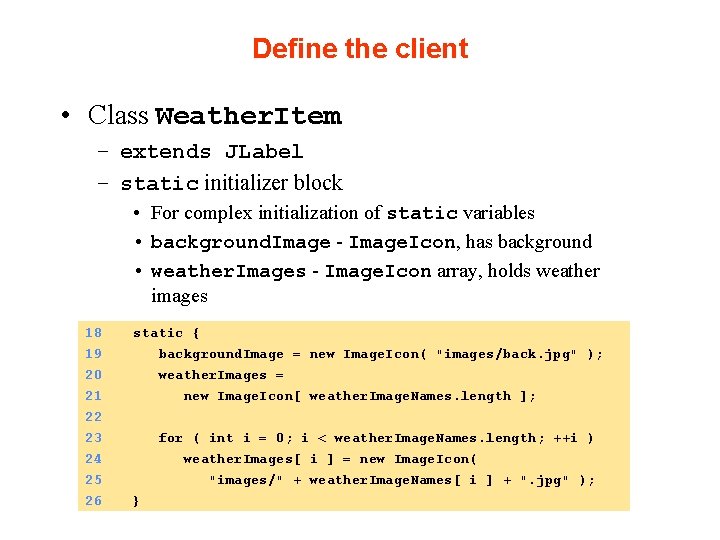
Define the client • Class Weather. Item – extends JLabel – static initializer block • For complex initialization of static variables • background. Image - Image. Icon, has background • weather. Images - Image. Icon array, holds weather images 18 static { 19 20 background. Image = new Image. Icon( "images/back. jpg" ); weather. Images = 21 new Image. Icon[ weather. Image. Names. length ]; 22 23 for ( int i = 0; i < weather. Image. Names. length; ++i ) 24 25 weather. Images[ i ] = new Image. Icon( "images/" + weather. Image. Names[ i ] + ". jpg" ); 26 }
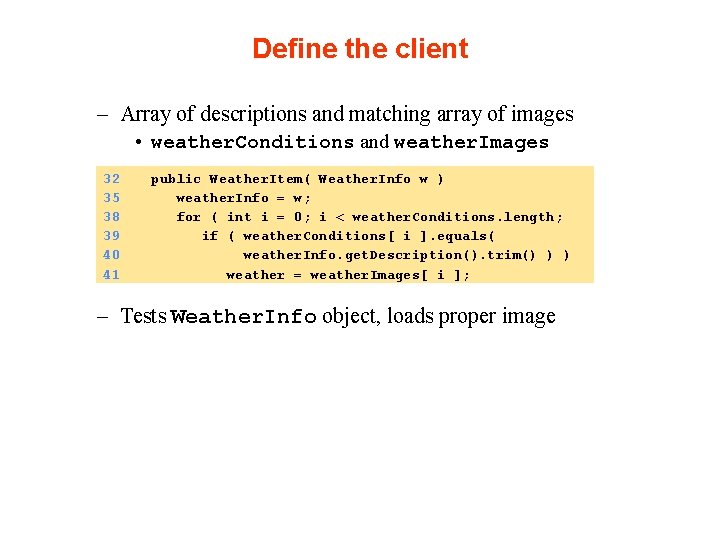
Define the client – Array of descriptions and matching array of images • weather. Conditions and weather. Images 32 public Weather. Item( Weather. Info w ) 35 weather. Info = w; 38 for ( int i = 0; i < weather. Conditions. length; ++i 39 ) if ( weather. Conditions[ i ]. equals( 40 weather. Info. get. Description(). trim() ) ) { 41 weather = weather. Images[ i ]; – Tests Weather. Info object, loads proper image
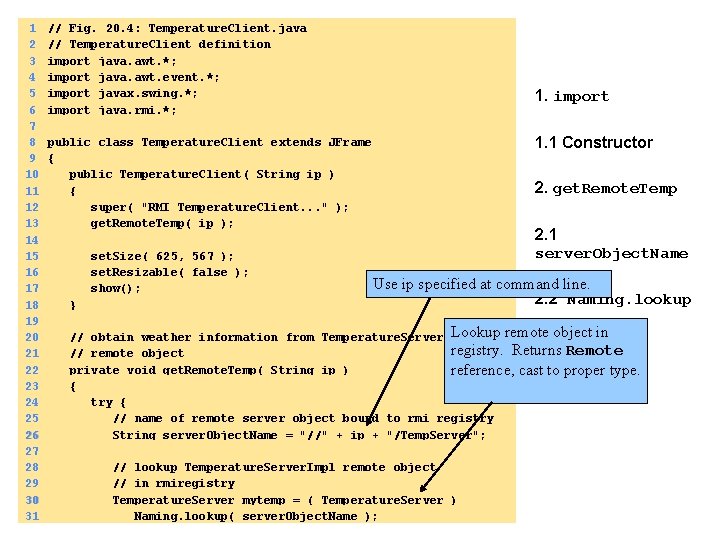
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 // Fig. 20. 4: Temperature. Client. java // Temperature. Client definition import java. awt. *; import java. awt. event. *; import javax. swing. *; import java. rmi. *; 1. import 1. 1 Constructor public class Temperature. Client extends JFrame { public Temperature. Client( String ip ) { super( "RMI Temperature. Client. . . " ); get. Remote. Temp( ip ); set. Size( 625, 567 ); set. Resizable( false ); show(); } 2. get. Remote. Temp 2. 1 server. Object. Name Use ip specified at command line. 2. 2 Naming. lookup Lookup remote object in // obtain weather information from Temperature. Server. Impl registry. Returns Remote // remote object private void get. Remote. Temp( String ip ) reference, cast to proper type. { try { // name of remote server object bound to rmi registry String server. Object. Name = "//" + ip + "/Temp. Server"; // lookup Temperature. Server. Impl remote object // in rmiregistry Temperature. Server mytemp = ( Temperature. Server ) Naming. lookup( server. Object. Name );
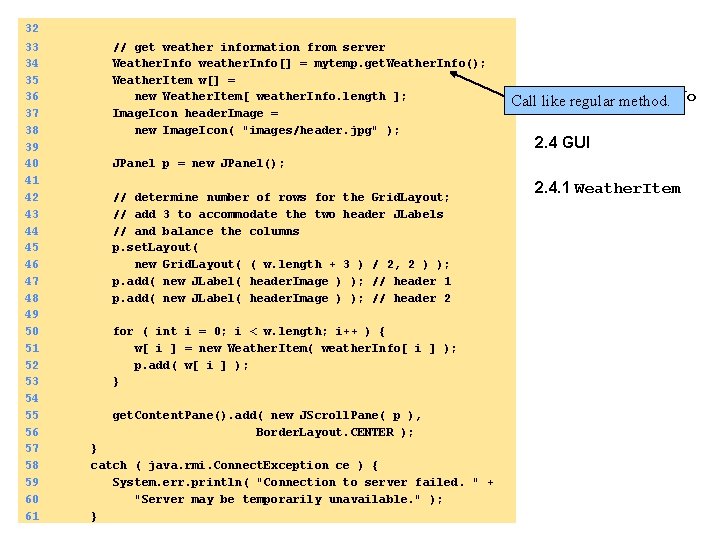
32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 // get weather information from server Weather. Info weather. Info[] = mytemp. get. Weather. Info(); Weather. Item w[] = new Weather. Item[ weather. Info. length ]; Image. Icon header. Image = new Image. Icon( "images/header. jpg" ); Call 2. 3 likeget. Weather. Info regular method. 2. 4 GUI JPanel p = new JPanel(); // determine number of rows for the Grid. Layout; // add 3 to accommodate the two header JLabels // and balance the columns p. set. Layout( new Grid. Layout( ( w. length + 3 ) / 2, 2 ) ); p. add( new JLabel( header. Image ) ); // header 1 p. add( new JLabel( header. Image ) ); // header 2 for ( int i = 0; i < w. length; i++ ) { w[ i ] = new Weather. Item( weather. Info[ i ] ); p. add( w[ i ] ); } get. Content. Pane(). add( new JScroll. Pane( p ), Border. Layout. CENTER ); } catch ( java. rmi. Connect. Exception ce ) { System. err. println( "Connection to server failed. " + "Server may be temporarily unavailable. " ); } 2. 4. 1 Weather. Item
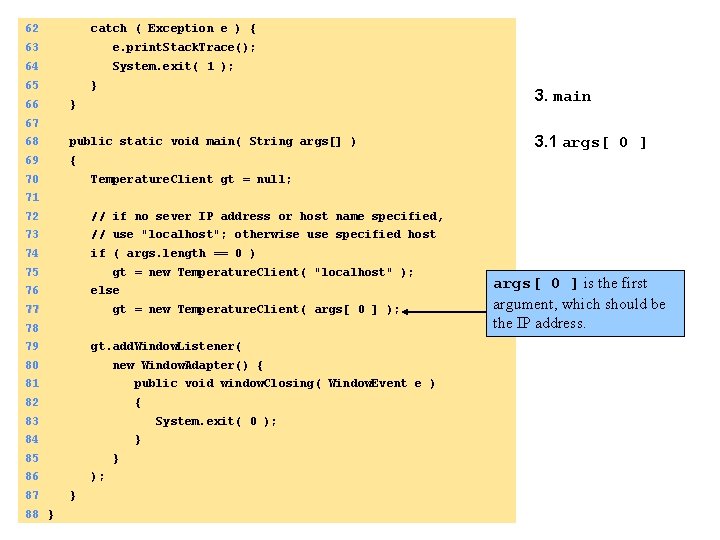
62 catch ( Exception e ) { 63 e. print. Stack. Trace(); 64 System. exit( 1 ); 65 } } 3. main 68 public static void main( String args[] ) 3. 1 args[ 0 ] 69 { 66 67 70 Temperature. Client gt = null; 71 72 // if no sever IP address or host name specified, 73 // use "localhost"; otherwise use specified host 74 if ( args. length == 0 ) 75 gt = new Temperature. Client( "localhost" ); 76 else 77 gt = new Temperature. Client( args[ 0 ] ); 78 79 gt. add. Window. Listener( 80 new Window. Adapter() { 81 public void window. Closing( Window. Event e ) 82 { 83 System. exit( 0 ); 84 } 85 } 86 87 88 } ); } args[ 0 ] is the first argument, which should be the IP address.
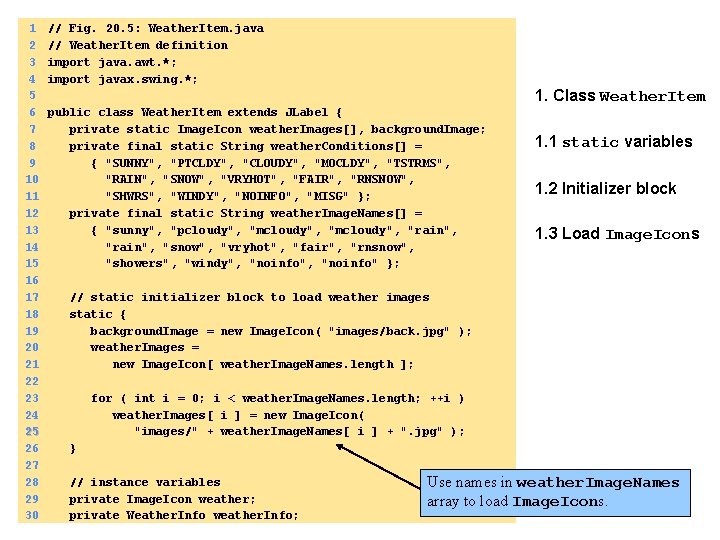
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 // Fig. 20. 5: Weather. Item. java // Weather. Item definition import java. awt. *; import javax. swing. *; 1. Class Weather. Item public class Weather. Item extends JLabel { private static Image. Icon weather. Images[], background. Image; private final static String weather. Conditions[] = { "SUNNY", "PTCLDY", "CLOUDY", "MOCLDY", "TSTRMS", "RAIN", "SNOW", "VRYHOT", "FAIR", "RNSNOW", "SHWRS", "WINDY", "NOINFO", "MISG" }; private final static String weather. Image. Names[] = { "sunny", "pcloudy", "mcloudy", "rain", "snow", "vryhot", "fair", "rnsnow", "showers", "windy", "noinfo" }; 1. 1 static variables 1. 2 Initializer block 1. 3 Load Image. Icons // static initializer block to load weather images static { background. Image = new Image. Icon( "images/back. jpg" ); weather. Images = new Image. Icon[ weather. Image. Names. length ]; for ( int i = 0; i < weather. Image. Names. length; ++i ) weather. Images[ i ] = new Image. Icon( "images/" + weather. Image. Names[ i ] + ". jpg" ); } // instance variables private Image. Icon weather; private Weather. Info weather. Info; Use names in weather. Image. Names array to load Image. Icons.
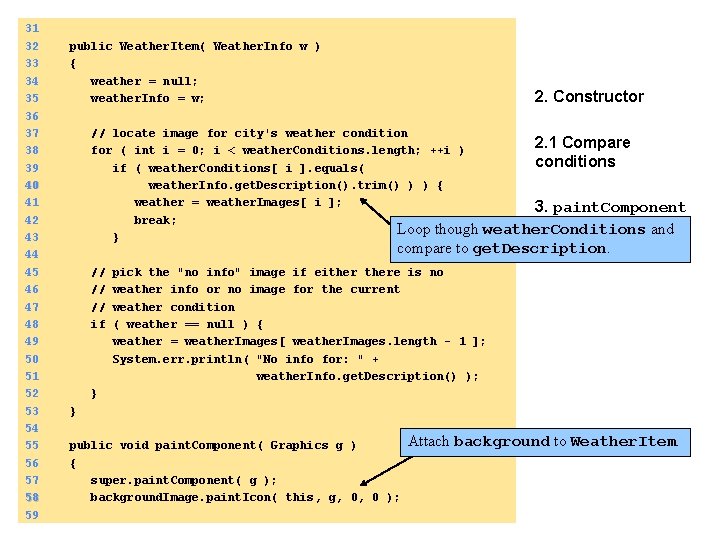
31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 public Weather. Item( Weather. Info w ) { weather = null; weather. Info = w; 2. Constructor // locate image for city's weather condition for ( int i = 0; i < weather. Conditions. length; ++i ) if ( weather. Conditions[ i ]. equals( weather. Info. get. Description(). trim() ) ) { weather = weather. Images[ i ]; break; Loop though } 2. 1 Compare conditions 3. paint. Component weather. Conditions and compare to get. Description. 3. 1 paint. Icon // // // if pick the "no info" image if eithere is no weather info or no image for the current weather condition ( weather == null ) { weather = weather. Images[ weather. Images. length - 1 ]; System. err. println( "No info for: " + weather. Info. get. Description() ); } } public void paint. Component( Graphics g ) { super. paint. Component( g ); background. Image. paint. Icon( this, g, 0, 0 ); Attach background to Weather. Item.
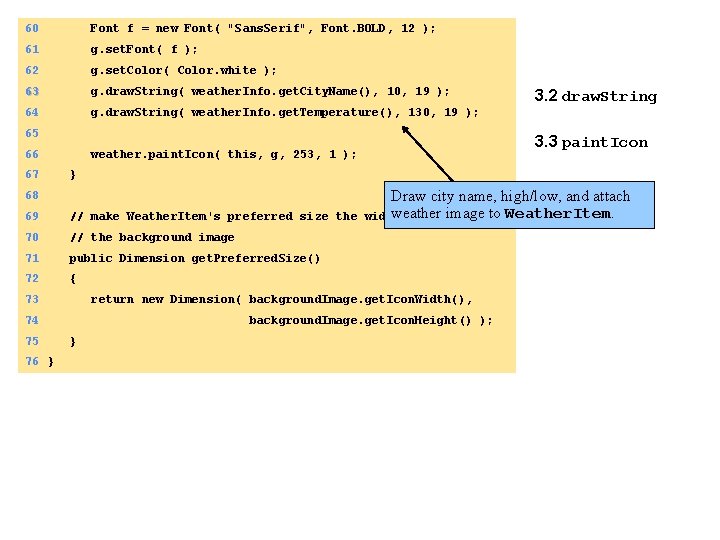
60 Font f = new Font( "Sans. Serif", Font. BOLD, 12 ); 61 g. set. Font( f ); 62 g. set. Color( Color. white ); 63 g. draw. String( weather. Info. get. City. Name(), 10, 19 ); 64 g. draw. String( weather. Info. get. Temperature(), 130, 19 ); 65 66 67 } 69 // make Weather. Item's preferred size the 70 // the background image 71 public Dimension get. Preferred. Size() 72 { 73 Draw city name, high/low, and attach weather image of to Weather. Item. width and height return new Dimension( background. Image. get. Icon. Width(), 74 76 } 3. 3 paint. Icon weather. paint. Icon( this, g, 253, 1 ); 68 75 3. 2 draw. String background. Image. get. Icon. Height() ); }
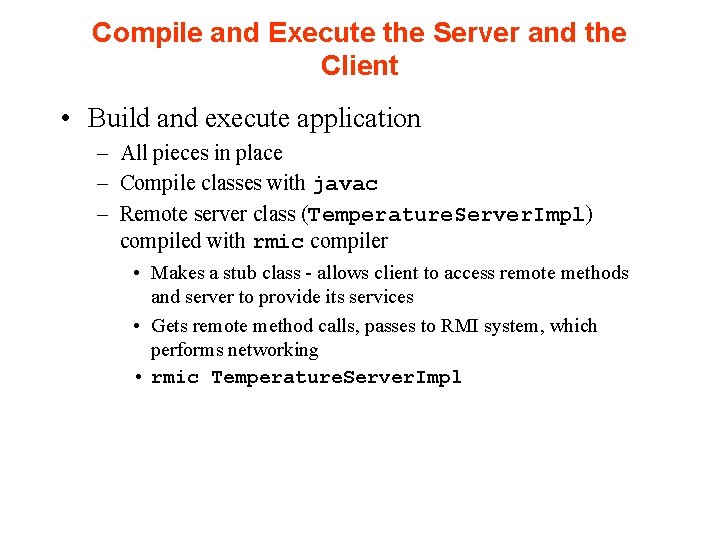
Compile and Execute the Server and the Client • Build and execute application – All pieces in place – Compile classes with javac – Remote server class (Temperature. Server. Impl) compiled with rmic compiler • Makes a stub class - allows client to access remote methods and server to provide its services • Gets remote method calls, passes to RMI system, which performs networking • rmic Temperature. Server. Impl
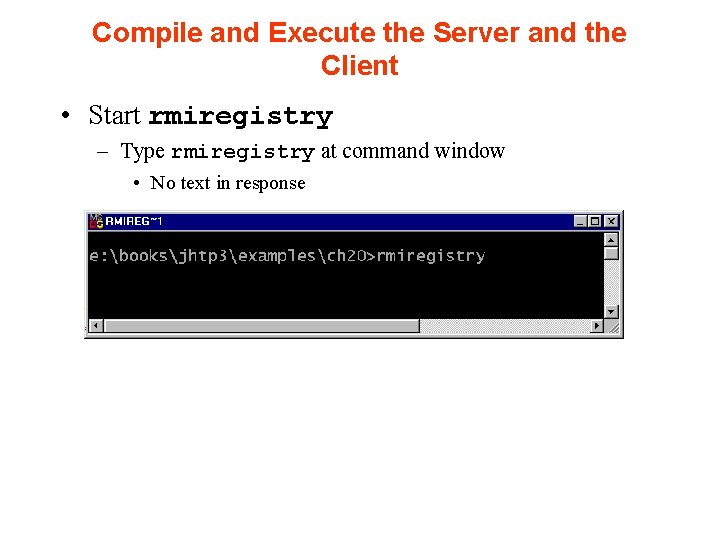
Compile and Execute the Server and the Client • Start rmiregistry – Type rmiregistry at command window • No text in response
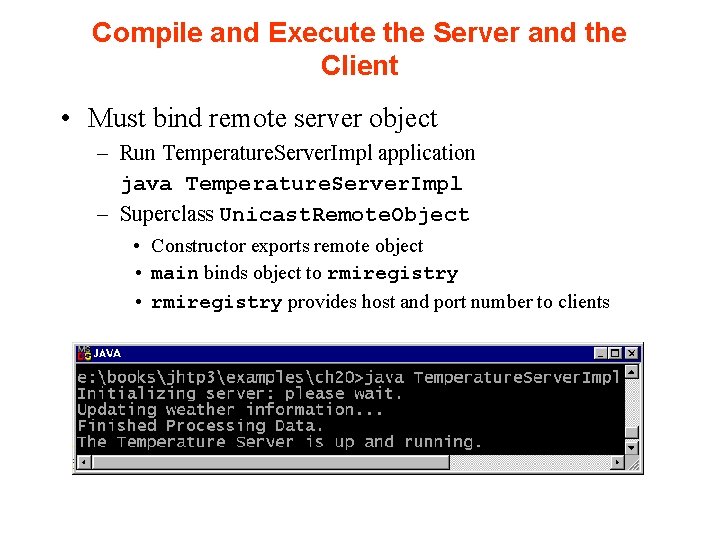
Compile and Execute the Server and the Client • Must bind remote server object – Run Temperature. Server. Impl application java Temperature. Server. Impl – Superclass Unicast. Remote. Object • Constructor exports remote object • main binds object to rmiregistry • rmiregistry provides host and port number to clients
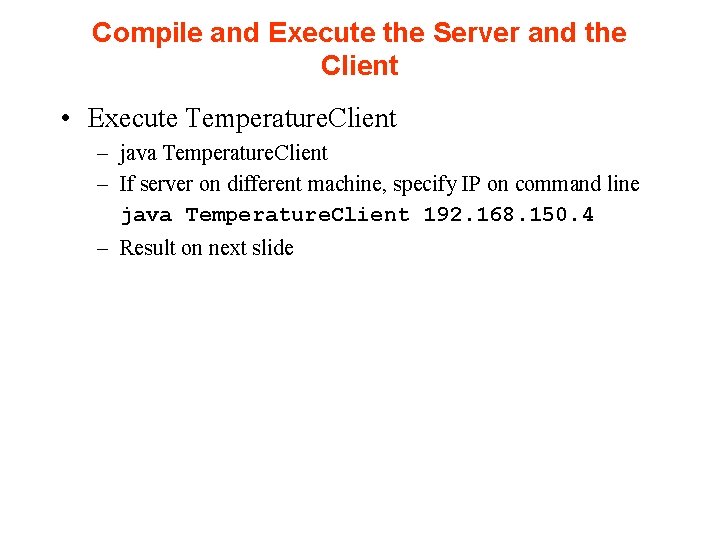
Compile and Execute the Server and the Client • Execute Temperature. Client – java Temperature. Client – If server on different machine, specify IP on command line java Temperature. Client 192. 168. 150. 4 – Result on next slide
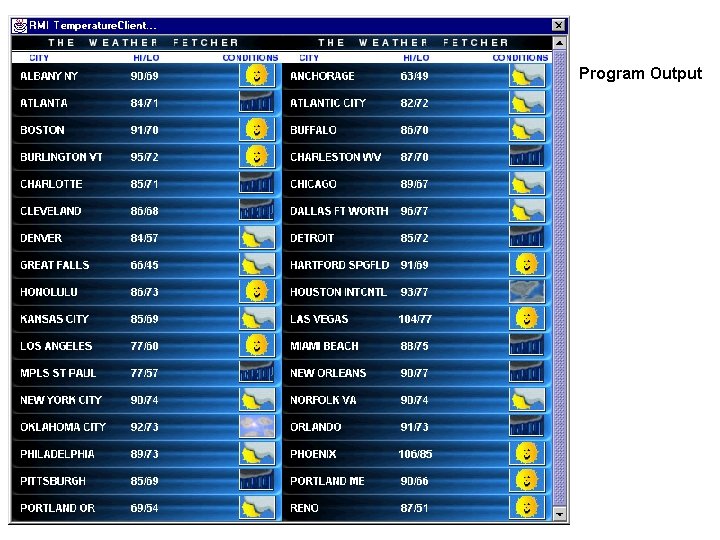
Program Output