Introduction to Java Server Faces Aaron Zeckoski azeckoskigmail
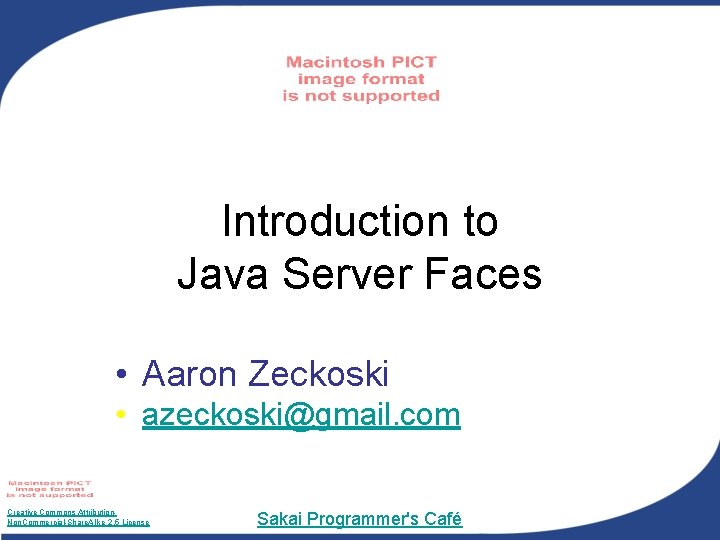
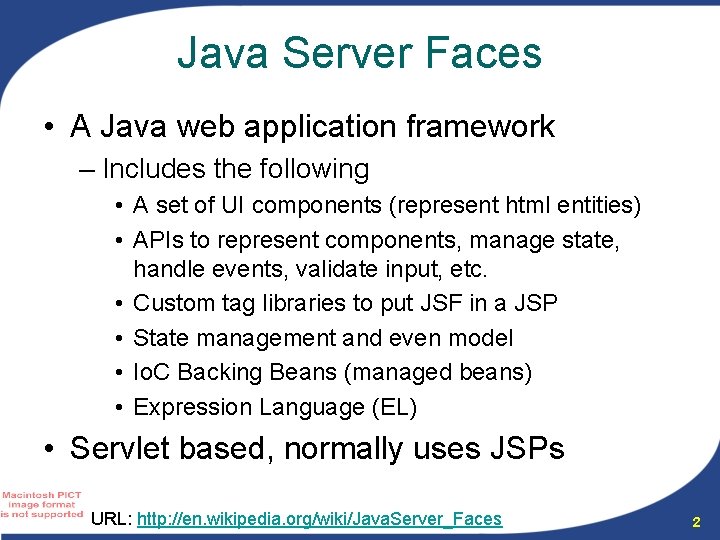
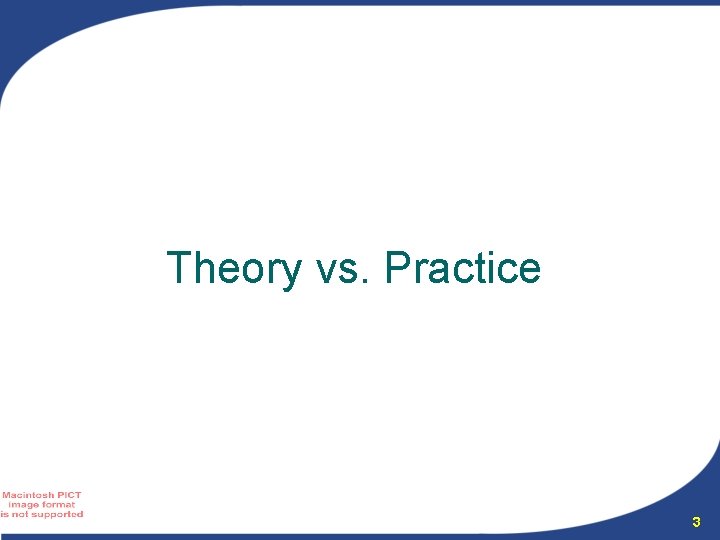
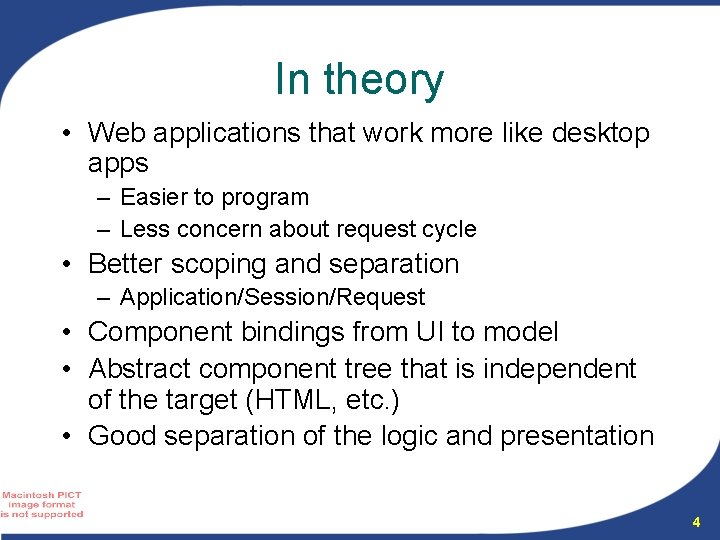
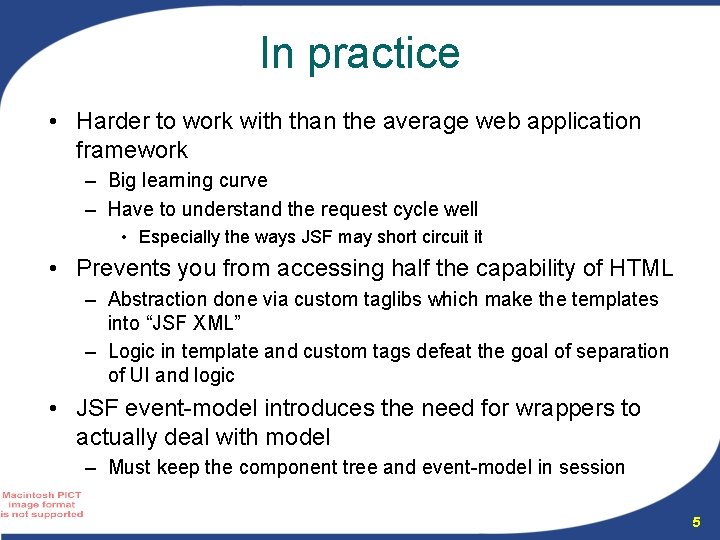
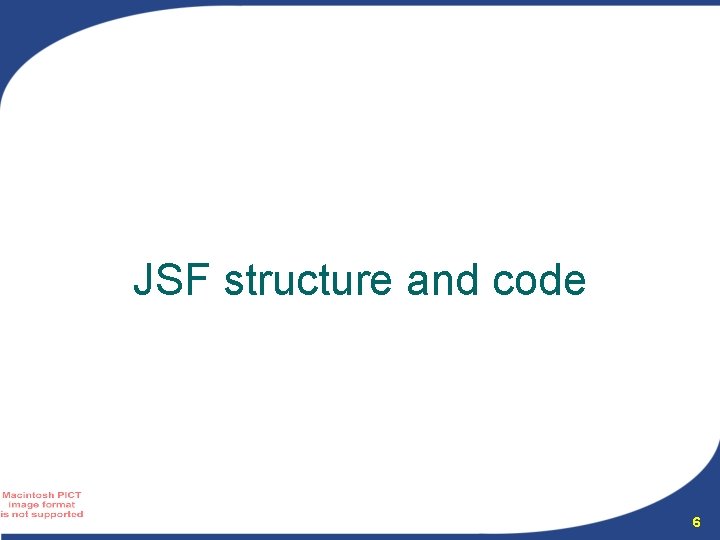
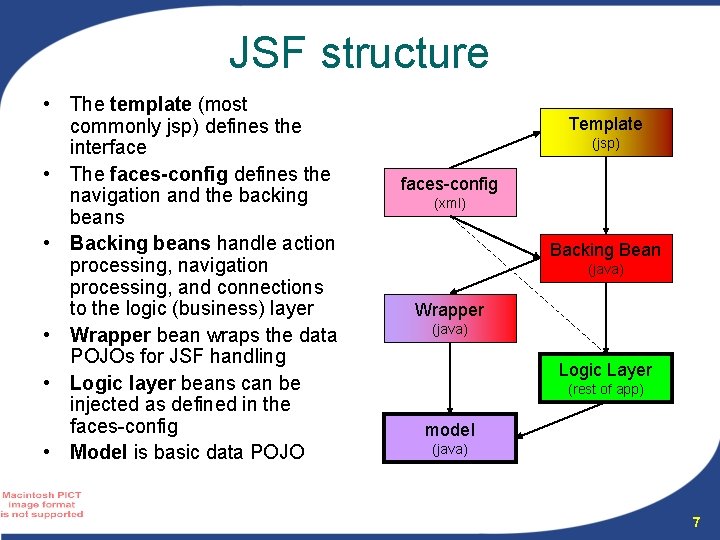
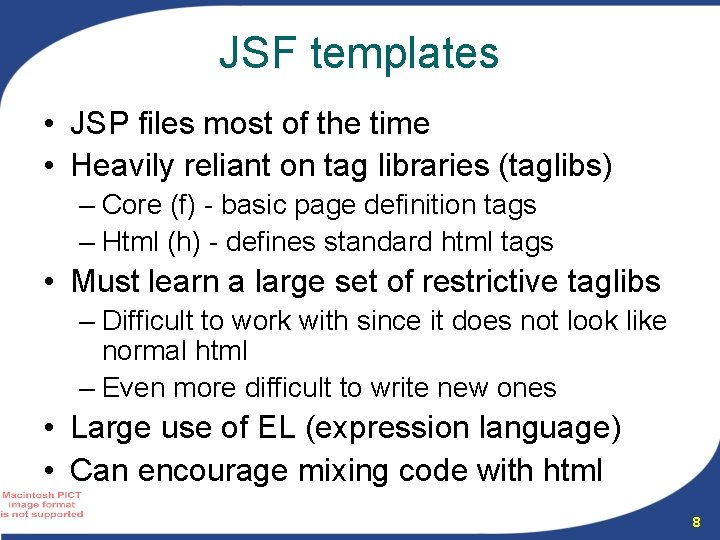
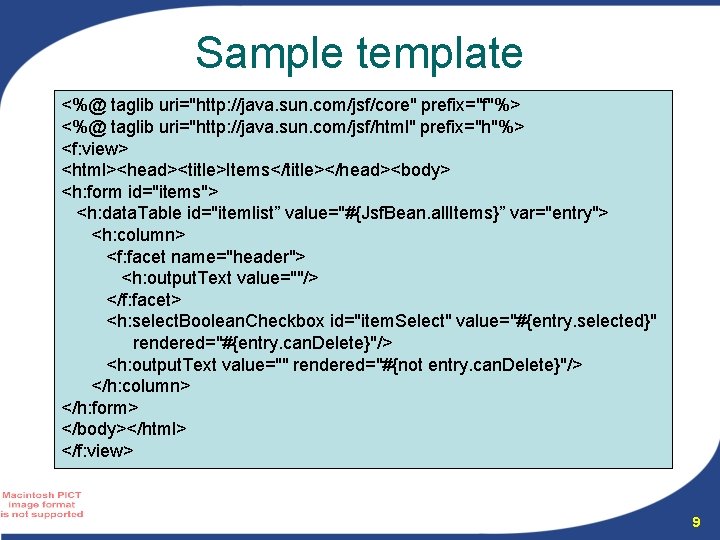
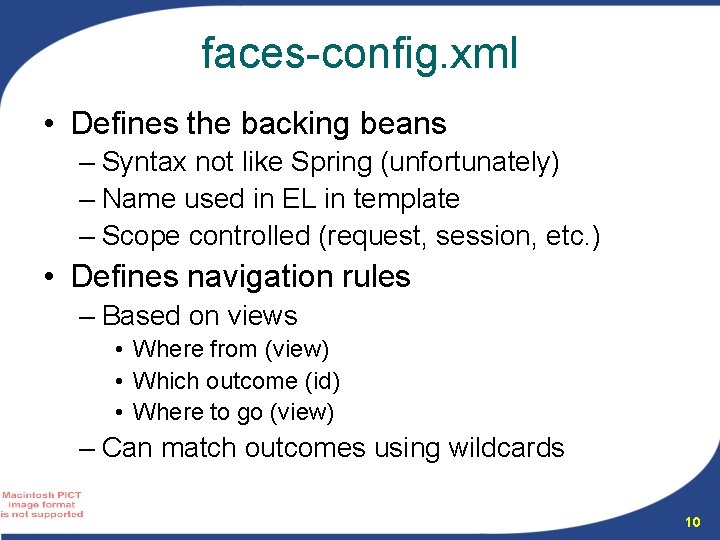
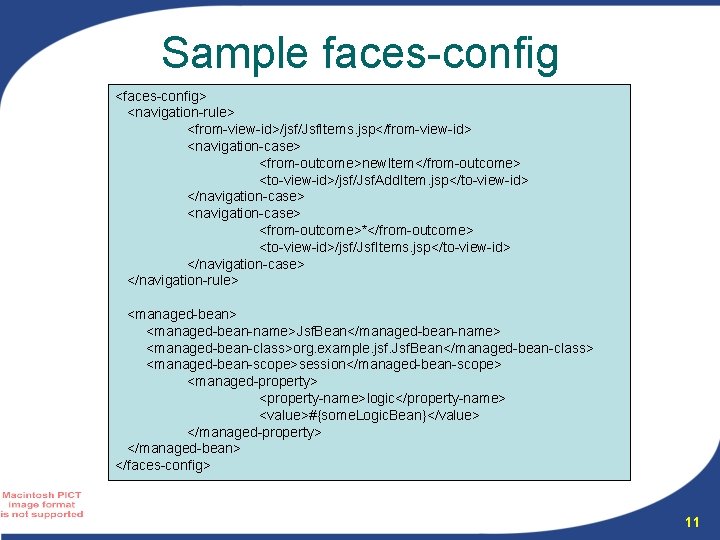
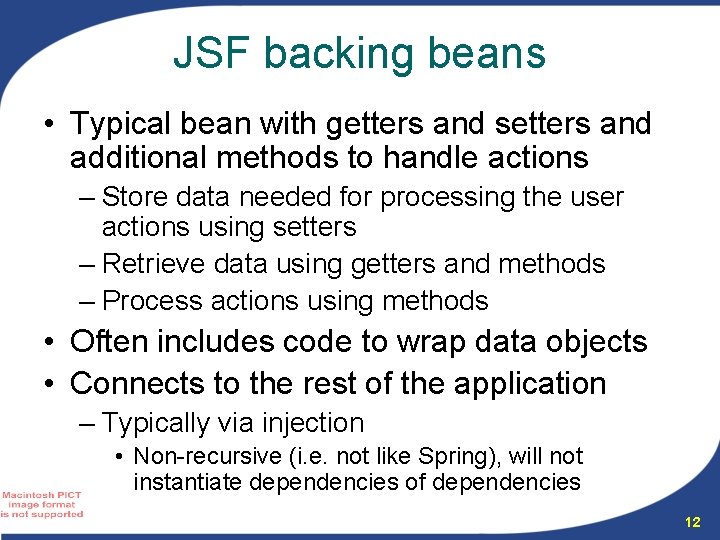
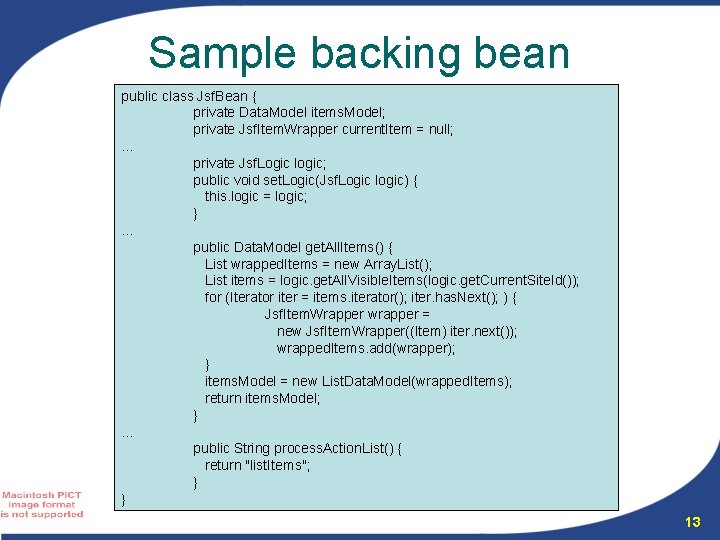
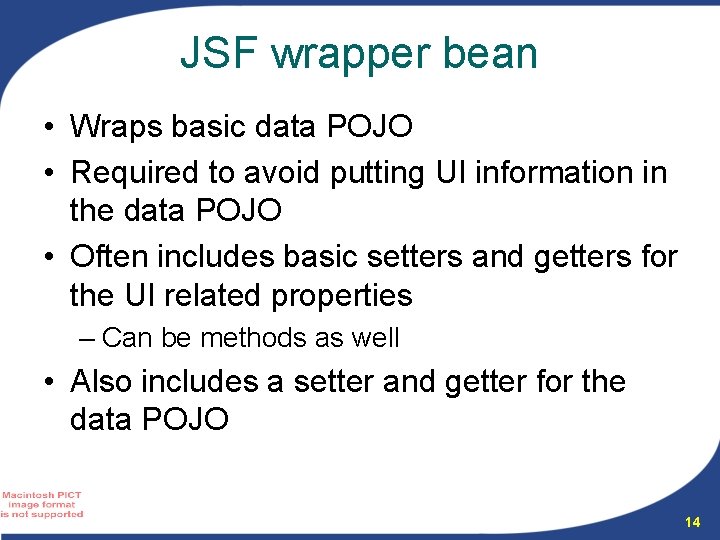
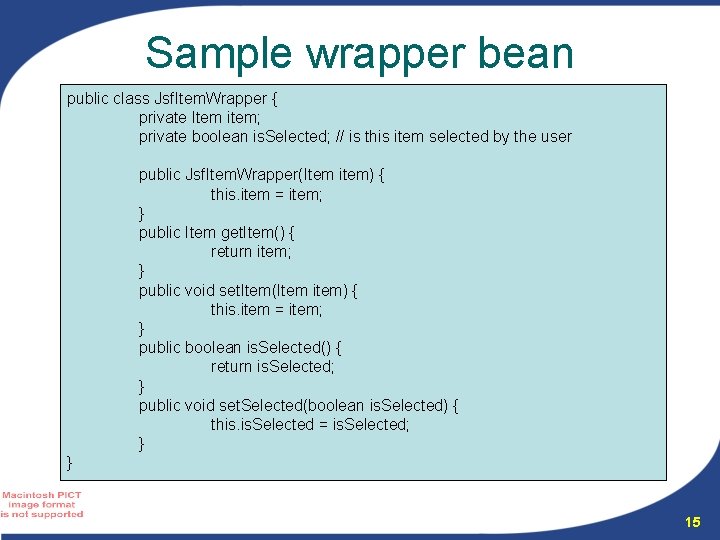
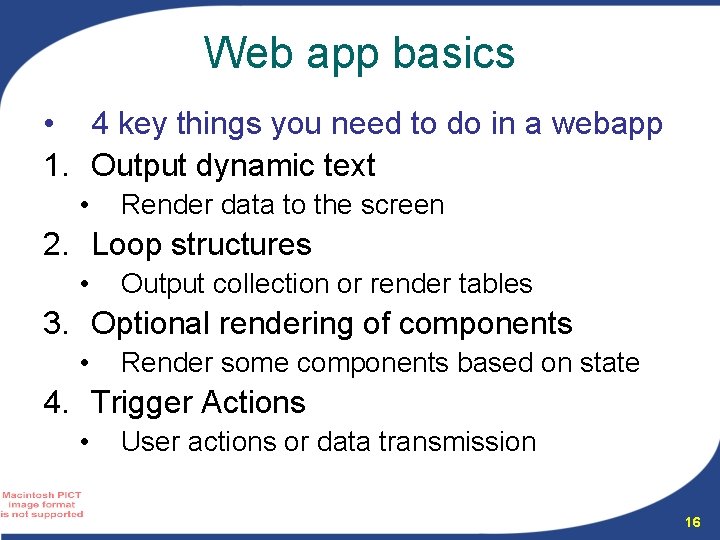
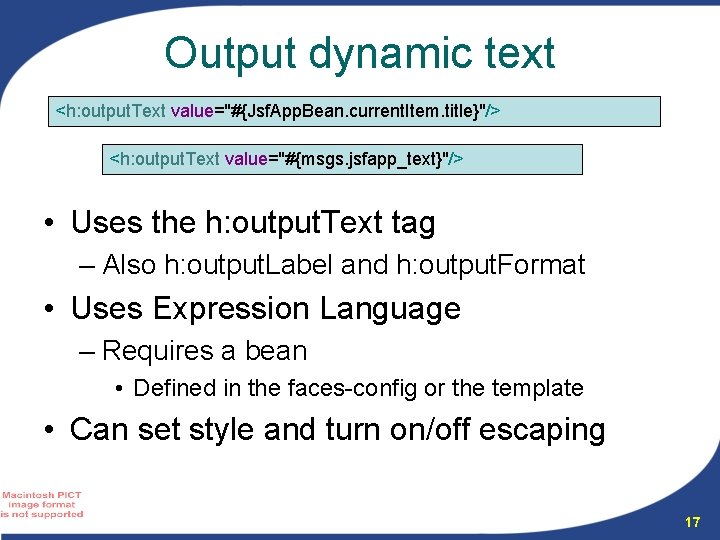
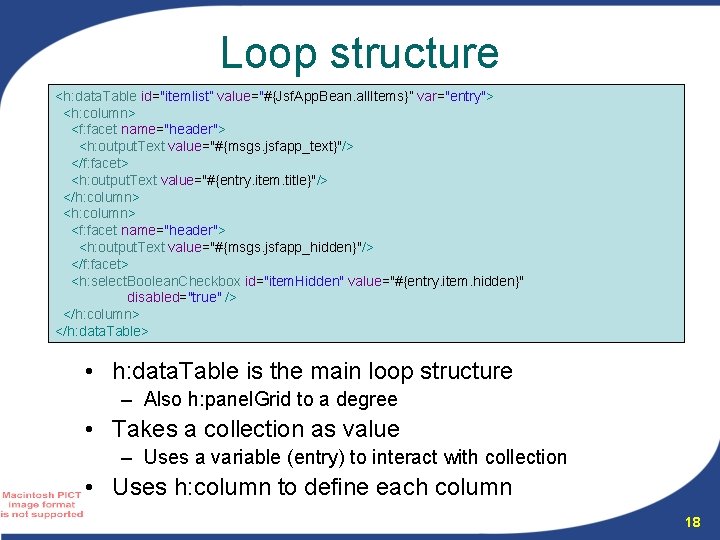
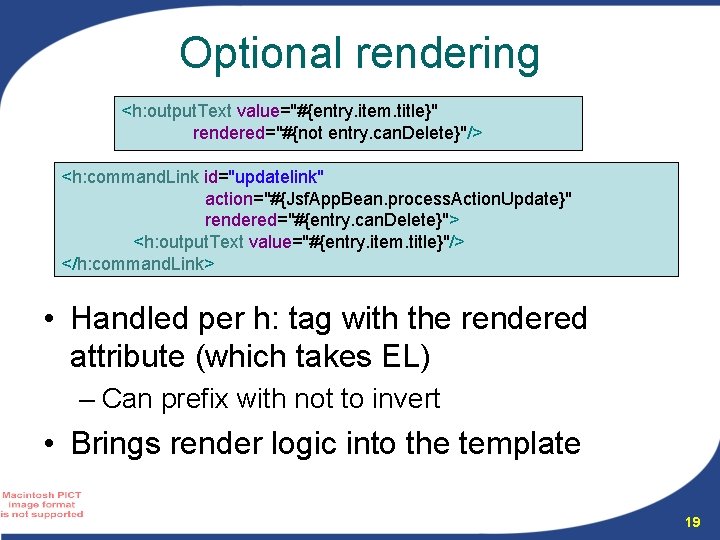
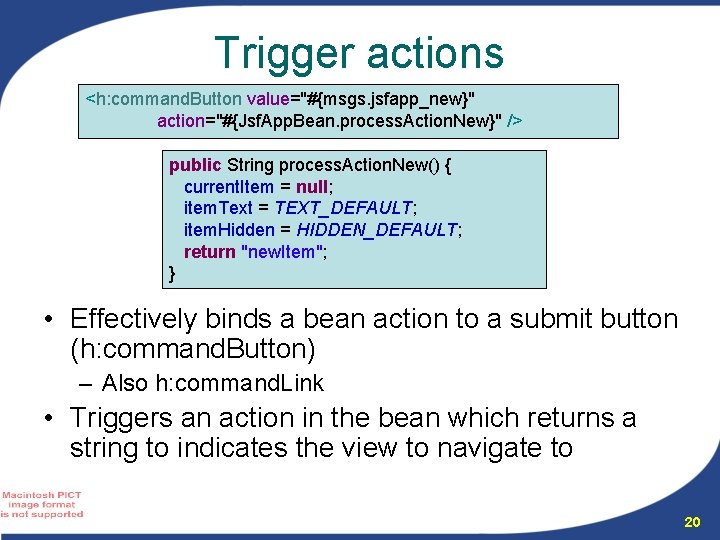
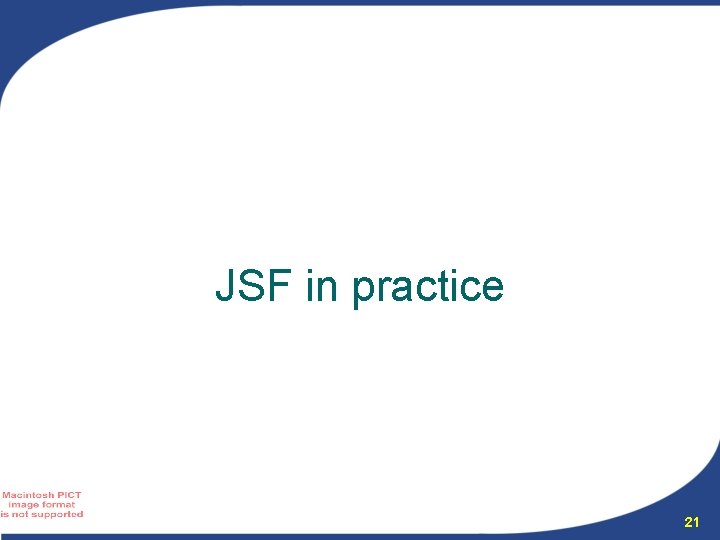
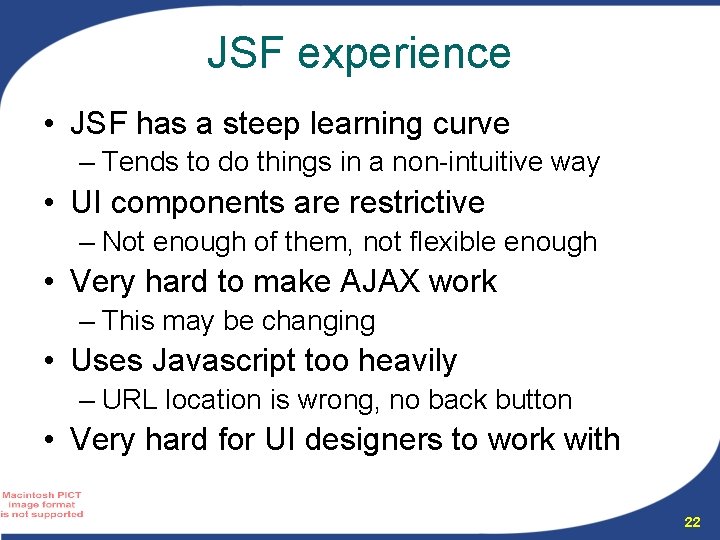
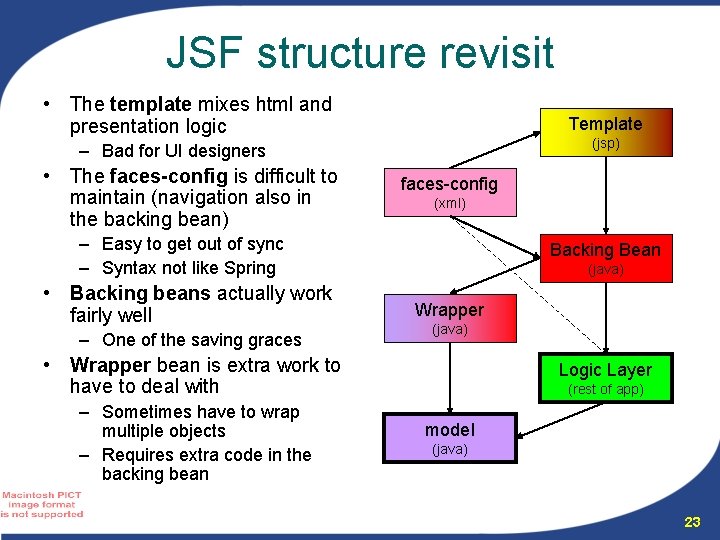
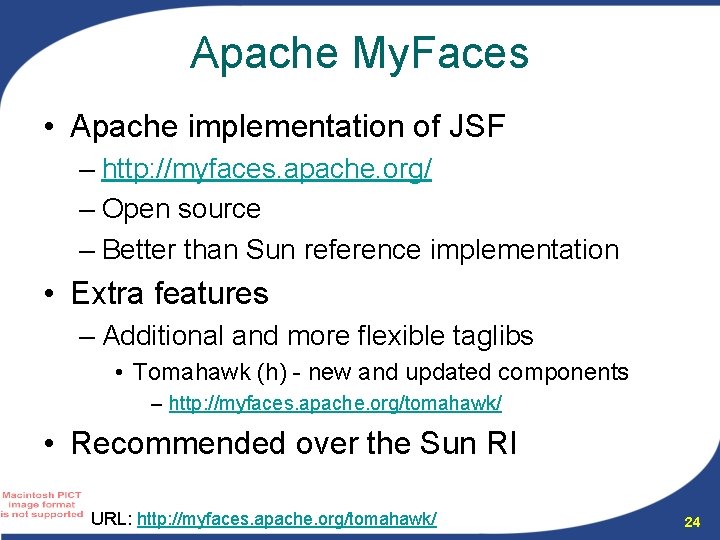
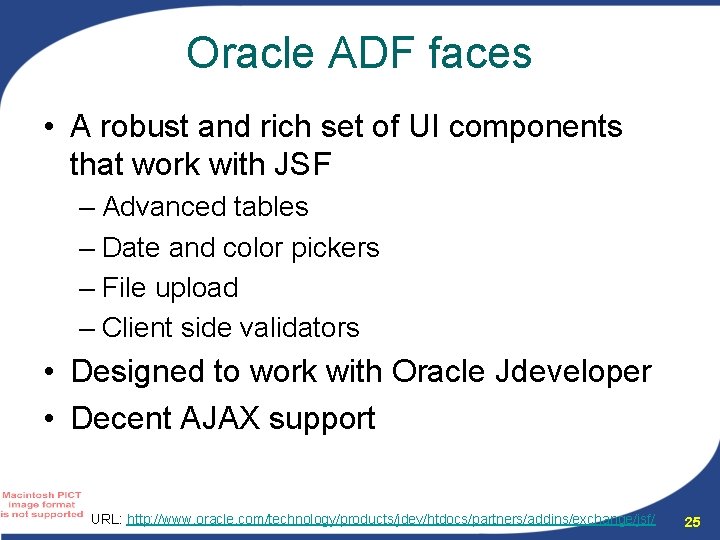
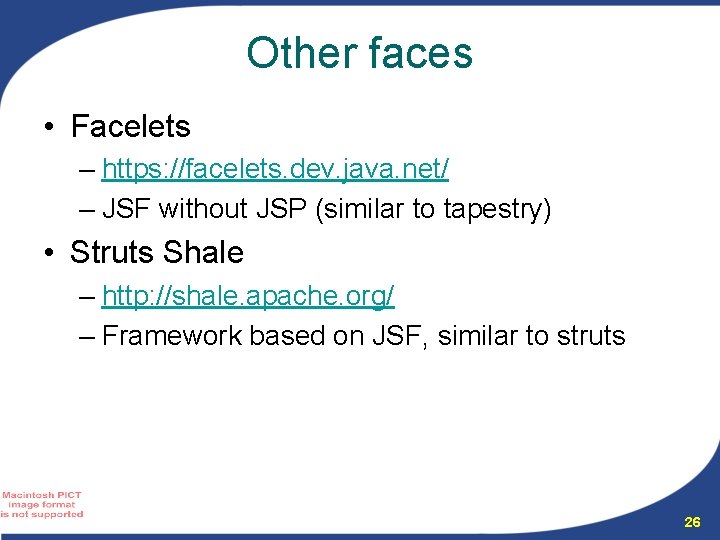
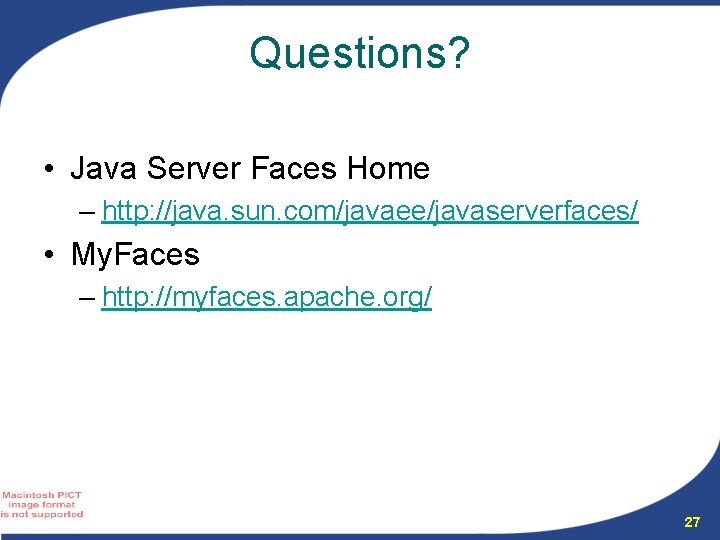
- Slides: 27
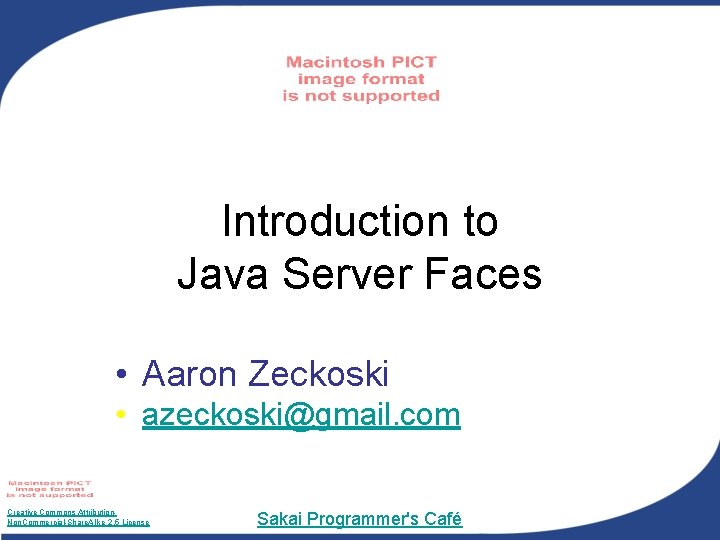
Introduction to Java Server Faces • Aaron Zeckoski • azeckoski@gmail. com Creative Commons Attribution. Non. Commercial-Share. Alike 2. 5 License Sakai Programmer's Café
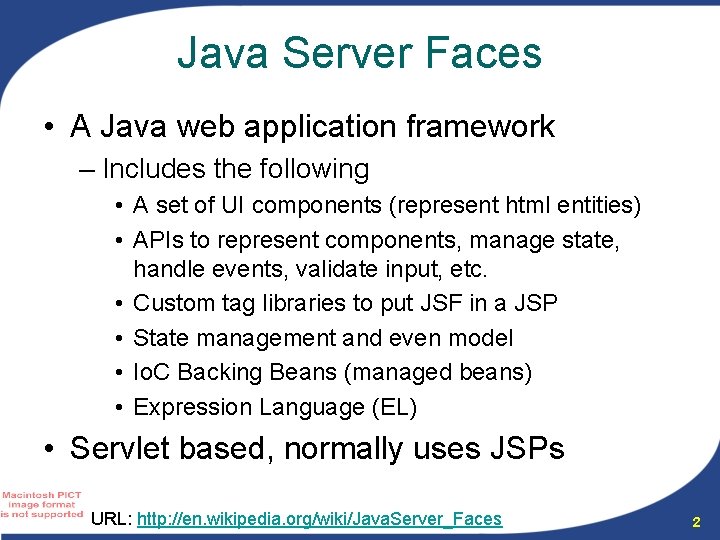
Java Server Faces • A Java web application framework – Includes the following • A set of UI components (represent html entities) • APIs to represent components, manage state, handle events, validate input, etc. • Custom tag libraries to put JSF in a JSP • State management and even model • Io. C Backing Beans (managed beans) • Expression Language (EL) • Servlet based, normally uses JSPs URL: http: //en. wikipedia. org/wiki/Java. Server_Faces 2
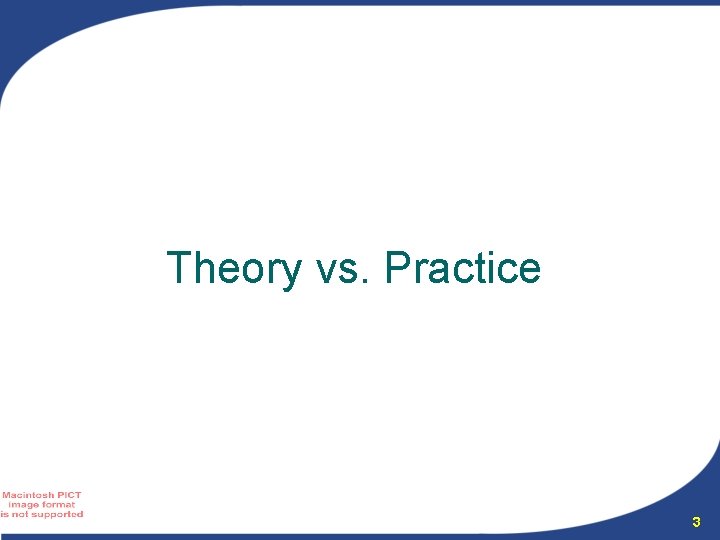
Theory vs. Practice 3
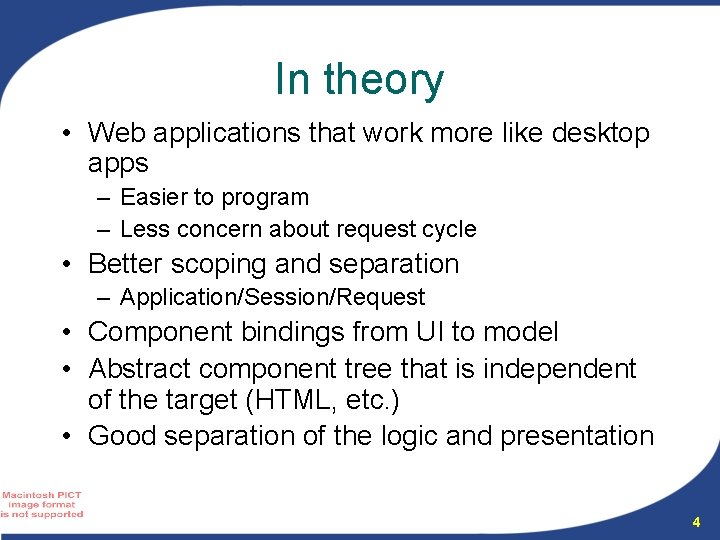
In theory • Web applications that work more like desktop apps – Easier to program – Less concern about request cycle • Better scoping and separation – Application/Session/Request • Component bindings from UI to model • Abstract component tree that is independent of the target (HTML, etc. ) • Good separation of the logic and presentation 4
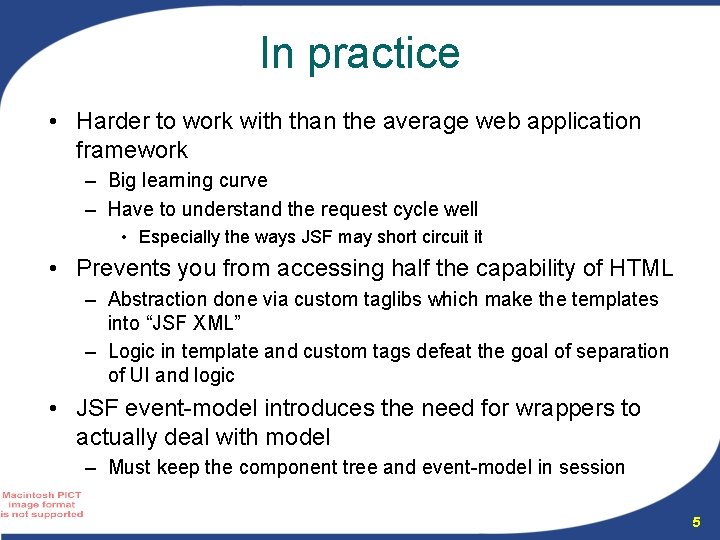
In practice • Harder to work with than the average web application framework – Big learning curve – Have to understand the request cycle well • Especially the ways JSF may short circuit it • Prevents you from accessing half the capability of HTML – Abstraction done via custom taglibs which make the templates into “JSF XML” – Logic in template and custom tags defeat the goal of separation of UI and logic • JSF event-model introduces the need for wrappers to actually deal with model – Must keep the component tree and event-model in session 5
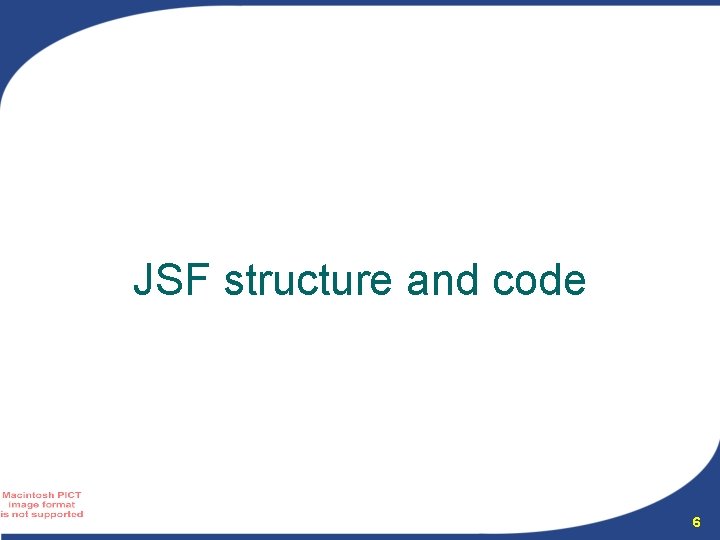
JSF structure and code 6
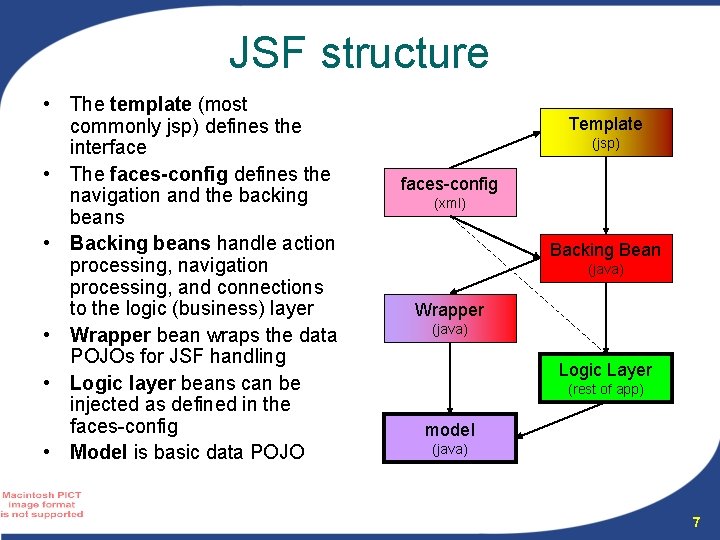
JSF structure • The template (most commonly jsp) defines the interface • The faces-config defines the navigation and the backing beans • Backing beans handle action processing, navigation processing, and connections to the logic (business) layer • Wrapper bean wraps the data POJOs for JSF handling • Logic layer beans can be injected as defined in the faces-config • Model is basic data POJO Template (jsp) faces-config (xml) Backing Bean (java) Wrapper (java) Logic Layer (rest of app) model (java) 7
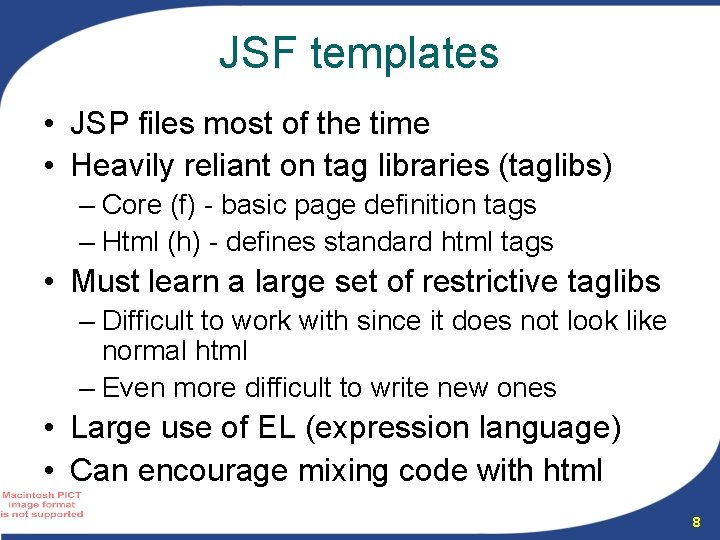
JSF templates • JSP files most of the time • Heavily reliant on tag libraries (taglibs) – Core (f) - basic page definition tags – Html (h) - defines standard html tags • Must learn a large set of restrictive taglibs – Difficult to work with since it does not look like normal html – Even more difficult to write new ones • Large use of EL (expression language) • Can encourage mixing code with html 8
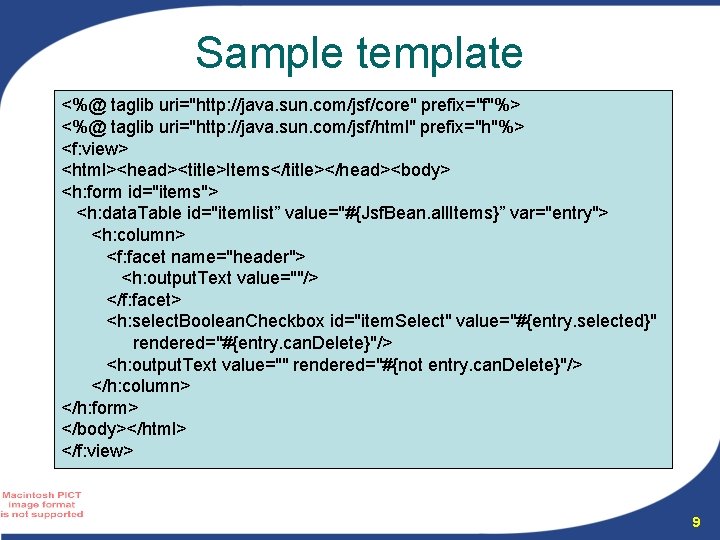
Sample template <%@ taglib uri="http: //java. sun. com/jsf/core" prefix="f"%> <%@ taglib uri="http: //java. sun. com/jsf/html" prefix="h"%> <f: view> <html><head><title>Items</title></head><body> <h: form id="items"> <h: data. Table id="itemlist” value="#{Jsf. Bean. all. Items}” var="entry"> <h: column> <f: facet name="header"> <h: output. Text value=""/> </f: facet> <h: select. Boolean. Checkbox id="item. Select" value="#{entry. selected}" rendered="#{entry. can. Delete}"/> <h: output. Text value="" rendered="#{not entry. can. Delete}"/> </h: column> </h: form> </body></html> </f: view> 9
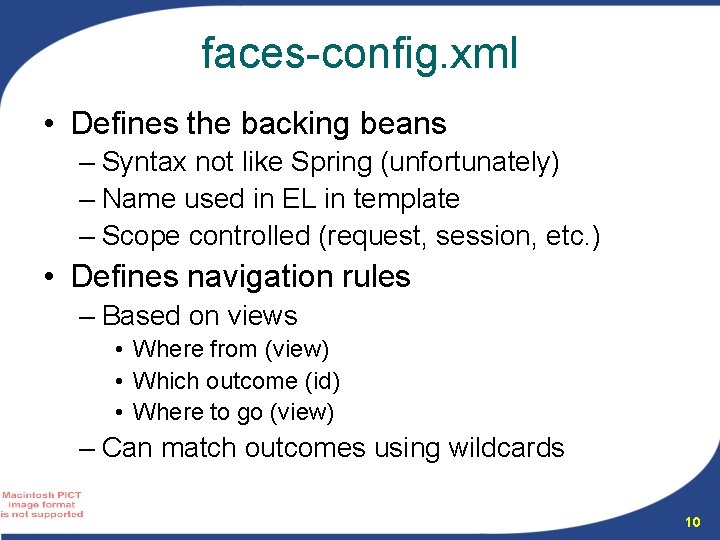
faces-config. xml • Defines the backing beans – Syntax not like Spring (unfortunately) – Name used in EL in template – Scope controlled (request, session, etc. ) • Defines navigation rules – Based on views • Where from (view) • Which outcome (id) • Where to go (view) – Can match outcomes using wildcards 10
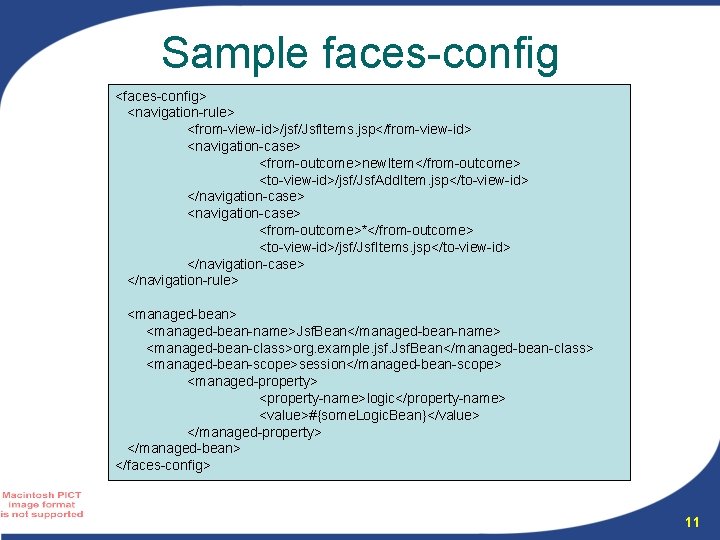
Sample faces-config <faces-config> <navigation-rule> <from-view-id>/jsf/Jsf. Items. jsp</from-view-id> <navigation-case> <from-outcome>new. Item</from-outcome> <to-view-id>/jsf/Jsf. Add. Item. jsp</to-view-id> </navigation-case> <from-outcome>*</from-outcome> <to-view-id>/jsf/Jsf. Items. jsp</to-view-id> </navigation-case> </navigation-rule> <managed-bean-name>Jsf. Bean</managed-bean-name> <managed-bean-class>org. example. jsf. Jsf. Bean</managed-bean-class> <managed-bean-scope>session</managed-bean-scope> <managed-property> <property-name>logic</property-name> <value>#{some. Logic. Bean}</value> </managed-property> </managed-bean> </faces-config> 11
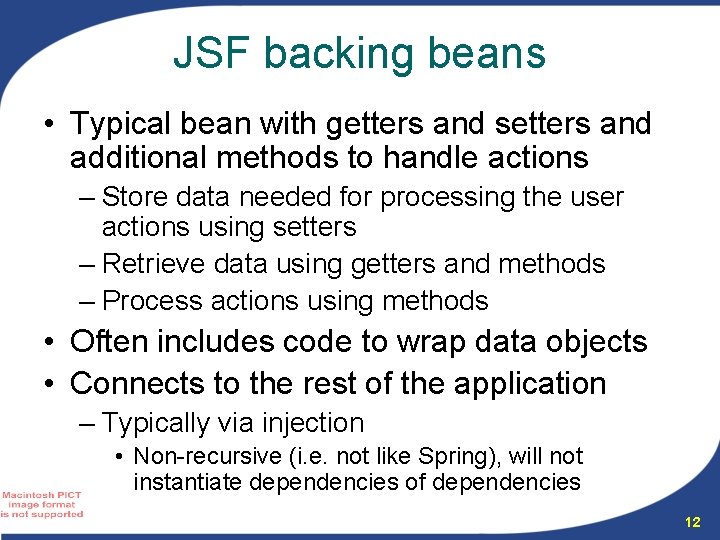
JSF backing beans • Typical bean with getters and setters and additional methods to handle actions – Store data needed for processing the user actions using setters – Retrieve data using getters and methods – Process actions using methods • Often includes code to wrap data objects • Connects to the rest of the application – Typically via injection • Non-recursive (i. e. not like Spring), will not instantiate dependencies of dependencies 12
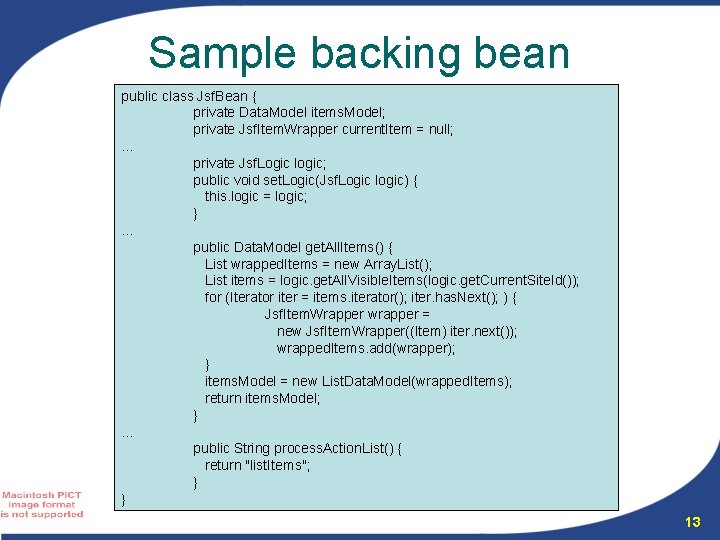
Sample backing bean public class Jsf. Bean { private Data. Model items. Model; private Jsf. Item. Wrapper current. Item = null; . . . private Jsf. Logic logic; public void set. Logic(Jsf. Logic logic) { this. logic = logic; }. . . public Data. Model get. All. Items() { List wrapped. Items = new Array. List(); List items = logic. get. All. Visible. Items(logic. get. Current. Site. Id()); for (Iterator iter = items. iterator(); iter. has. Next(); ) { Jsf. Item. Wrapper wrapper = new Jsf. Item. Wrapper((Item) iter. next()); wrapped. Items. add(wrapper); } items. Model = new List. Data. Model(wrapped. Items); return items. Model; }. . . public String process. Action. List() { return "list. Items"; } } 13
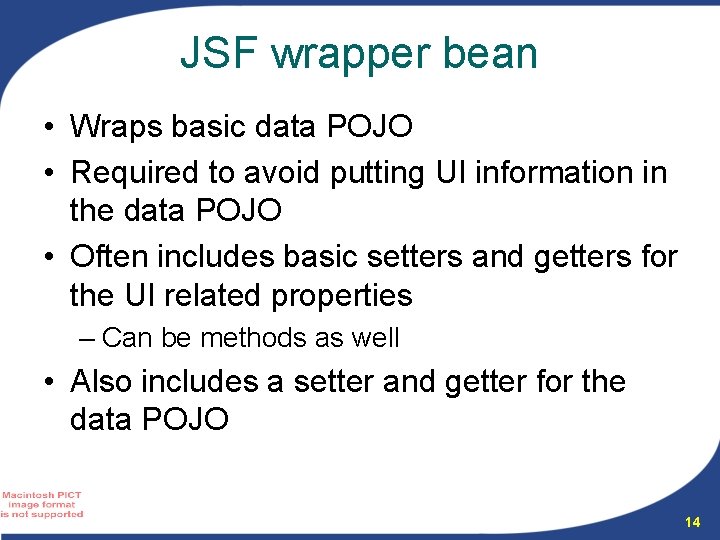
JSF wrapper bean • Wraps basic data POJO • Required to avoid putting UI information in the data POJO • Often includes basic setters and getters for the UI related properties – Can be methods as well • Also includes a setter and getter for the data POJO 14
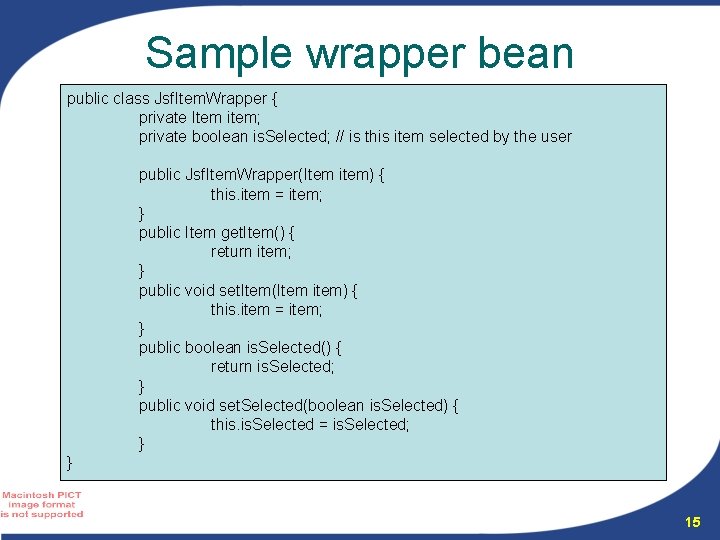
Sample wrapper bean public class Jsf. Item. Wrapper { private Item item; private boolean is. Selected; // is this item selected by the user public Jsf. Item. Wrapper(Item item) { this. item = item; } public Item get. Item() { return item; } public void set. Item(Item item) { this. item = item; } public boolean is. Selected() { return is. Selected; } public void set. Selected(boolean is. Selected) { this. Selected = is. Selected; } } 15
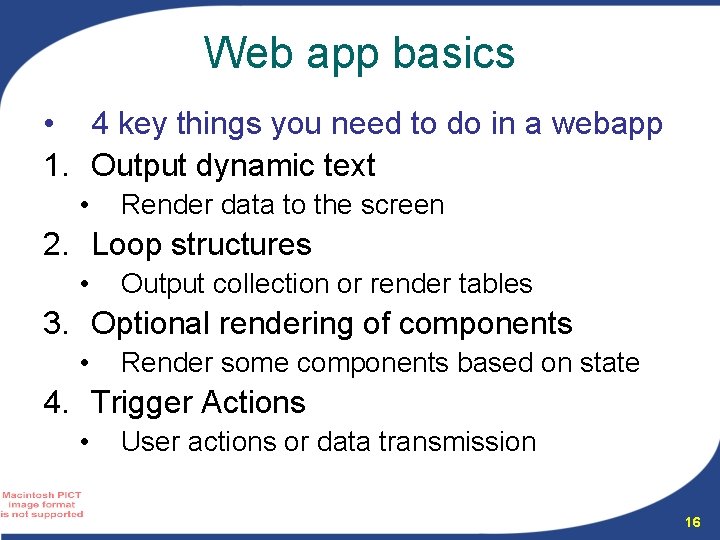
Web app basics • 4 key things you need to do in a webapp 1. Output dynamic text • Render data to the screen 2. Loop structures • Output collection or render tables 3. Optional rendering of components • Render some components based on state 4. Trigger Actions • User actions or data transmission 16
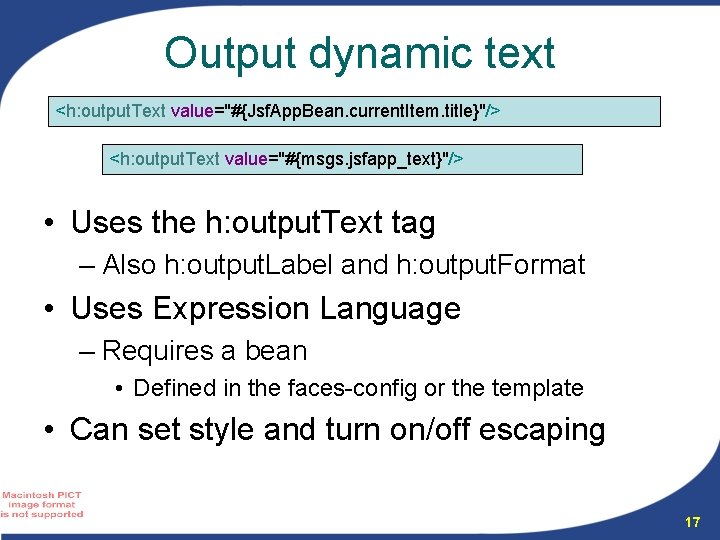
Output dynamic text <h: output. Text value="#{Jsf. App. Bean. current. Item. title}"/> <h: output. Text value="#{msgs. jsfapp_text}"/> • Uses the h: output. Text tag – Also h: output. Label and h: output. Format • Uses Expression Language – Requires a bean • Defined in the faces-config or the template • Can set style and turn on/off escaping 17
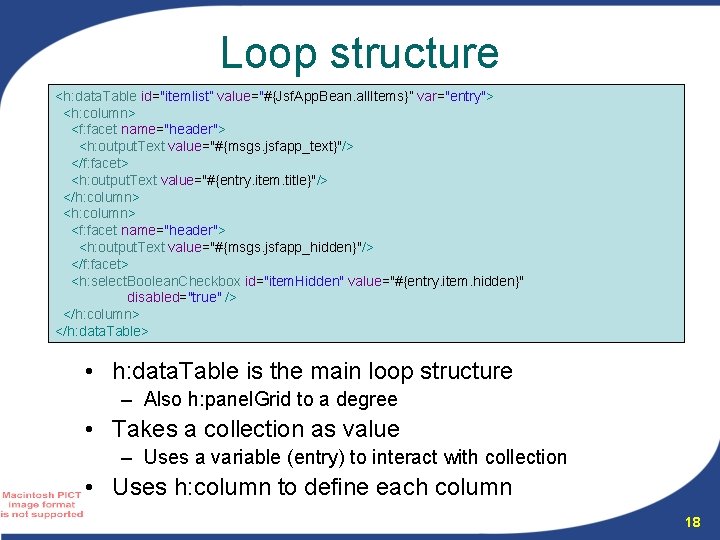
Loop structure <h: data. Table id="itemlist” value="#{Jsf. App. Bean. all. Items}” var="entry"> <h: column> <f: facet name="header"> <h: output. Text value="#{msgs. jsfapp_text}"/> </f: facet> <h: output. Text value="#{entry. item. title}"/> </h: column> <f: facet name="header"> <h: output. Text value="#{msgs. jsfapp_hidden}"/> </f: facet> <h: select. Boolean. Checkbox id="item. Hidden" value="#{entry. item. hidden}" disabled="true" /> </h: column> </h: data. Table> • h: data. Table is the main loop structure – Also h: panel. Grid to a degree • Takes a collection as value – Uses a variable (entry) to interact with collection • Uses h: column to define each column 18
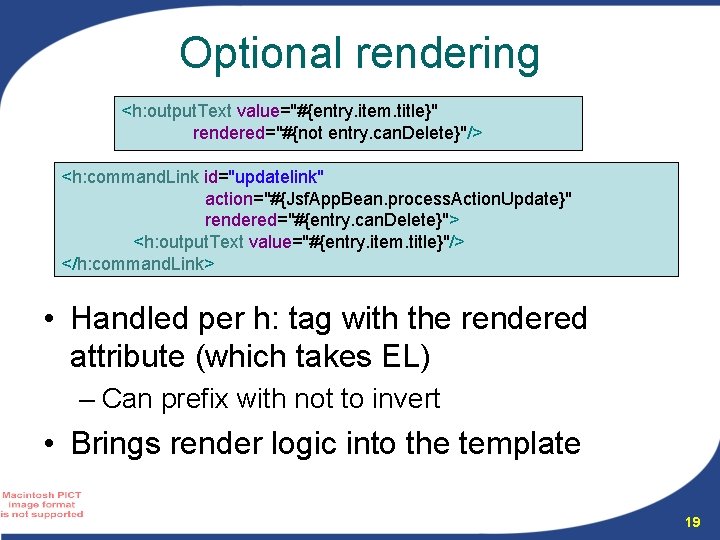
Optional rendering <h: output. Text value="#{entry. item. title}" rendered="#{not entry. can. Delete}"/> <h: command. Link id="updatelink" action="#{Jsf. App. Bean. process. Action. Update}" rendered="#{entry. can. Delete}"> <h: output. Text value="#{entry. item. title}"/> </h: command. Link> • Handled per h: tag with the rendered attribute (which takes EL) – Can prefix with not to invert • Brings render logic into the template 19
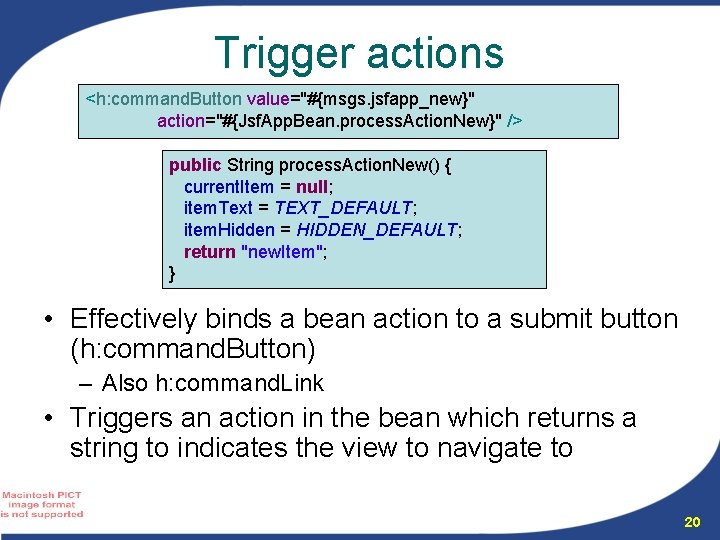
Trigger actions <h: command. Button value="#{msgs. jsfapp_new}" action="#{Jsf. App. Bean. process. Action. New}" /> public String process. Action. New() { current. Item = null; item. Text = TEXT_DEFAULT; item. Hidden = HIDDEN_DEFAULT; return "new. Item"; } • Effectively binds a bean action to a submit button (h: command. Button) – Also h: command. Link • Triggers an action in the bean which returns a string to indicates the view to navigate to 20
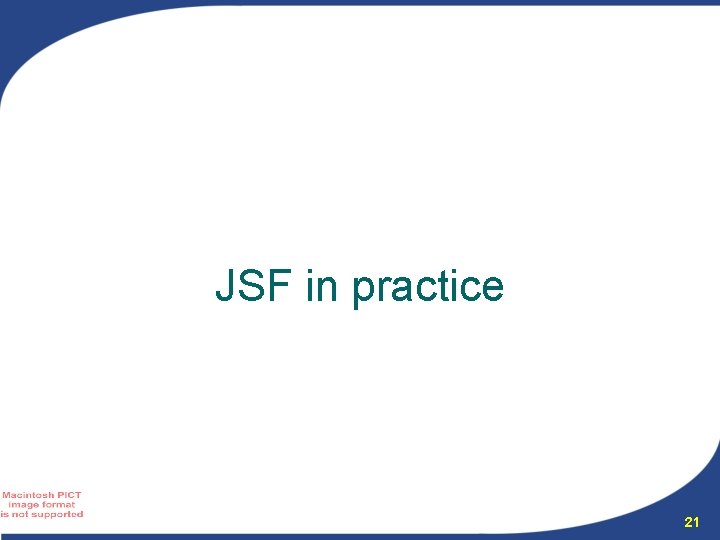
JSF in practice 21
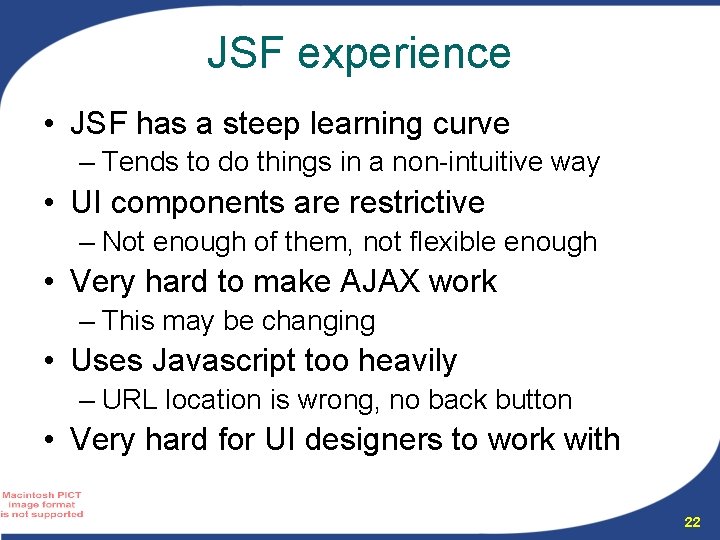
JSF experience • JSF has a steep learning curve – Tends to do things in a non-intuitive way • UI components are restrictive – Not enough of them, not flexible enough • Very hard to make AJAX work – This may be changing • Uses Javascript too heavily – URL location is wrong, no back button • Very hard for UI designers to work with 22
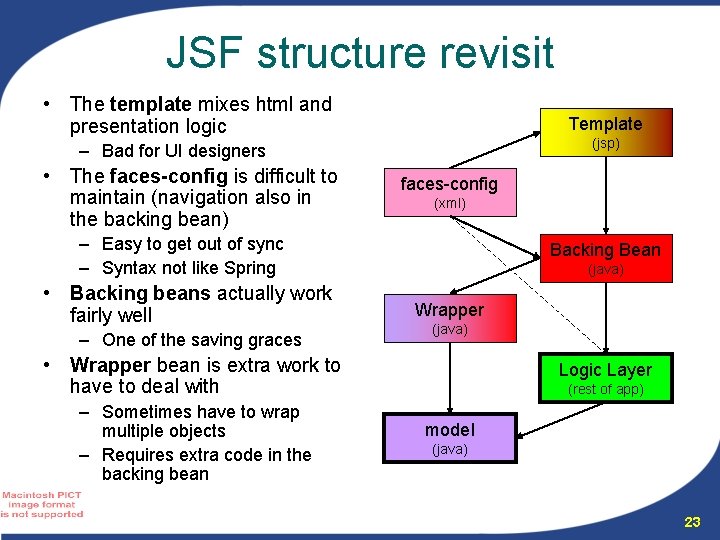
JSF structure revisit • The template mixes html and presentation logic Template (jsp) – Bad for UI designers • The faces-config is difficult to maintain (navigation also in the backing bean) faces-config (xml) – Easy to get out of sync – Syntax not like Spring • Backing beans actually work fairly well – One of the saving graces Backing Bean (java) Wrapper (java) • Wrapper bean is extra work to have to deal with – Sometimes have to wrap multiple objects – Requires extra code in the backing bean Logic Layer (rest of app) model (java) 23
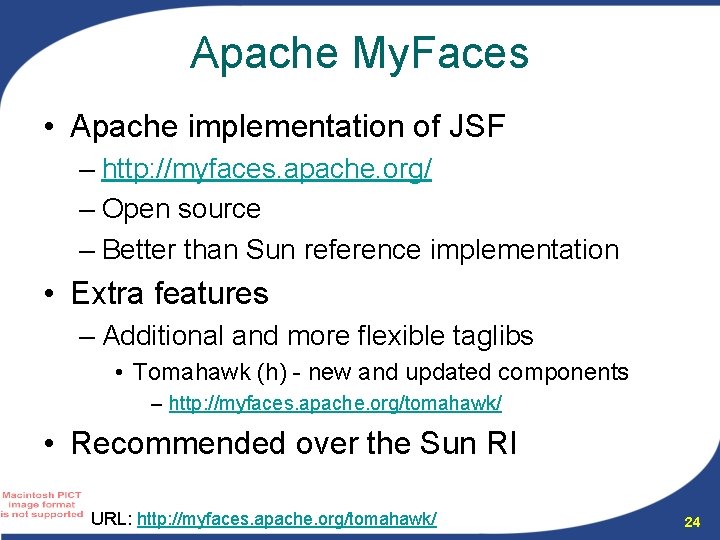
Apache My. Faces • Apache implementation of JSF – http: //myfaces. apache. org/ – Open source – Better than Sun reference implementation • Extra features – Additional and more flexible taglibs • Tomahawk (h) - new and updated components – http: //myfaces. apache. org/tomahawk/ • Recommended over the Sun RI URL: http: //myfaces. apache. org/tomahawk/ 24
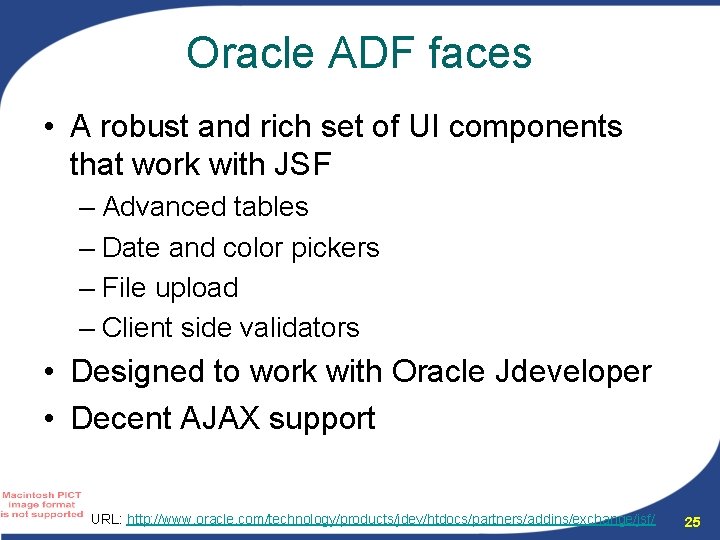
Oracle ADF faces • A robust and rich set of UI components that work with JSF – Advanced tables – Date and color pickers – File upload – Client side validators • Designed to work with Oracle Jdeveloper • Decent AJAX support URL: http: //www. oracle. com/technology/products/jdev/htdocs/partners/addins/exchange/jsf/ 25
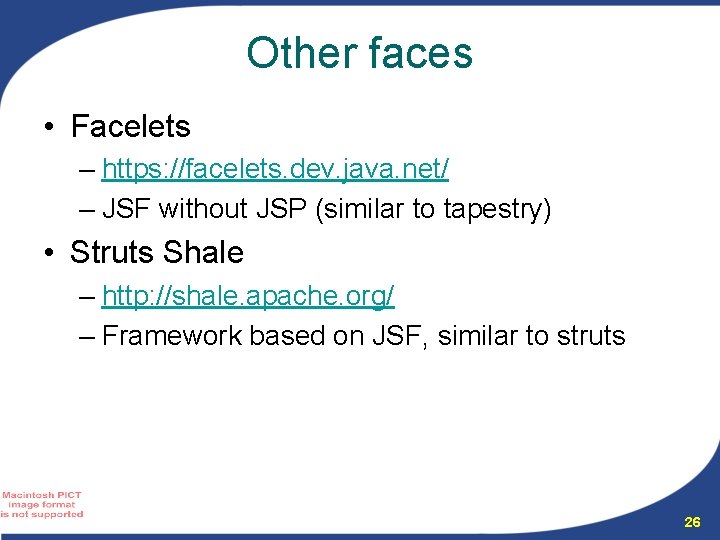
Other faces • Facelets – https: //facelets. dev. java. net/ – JSF without JSP (similar to tapestry) • Struts Shale – http: //shale. apache. org/ – Framework based on JSF, similar to struts 26
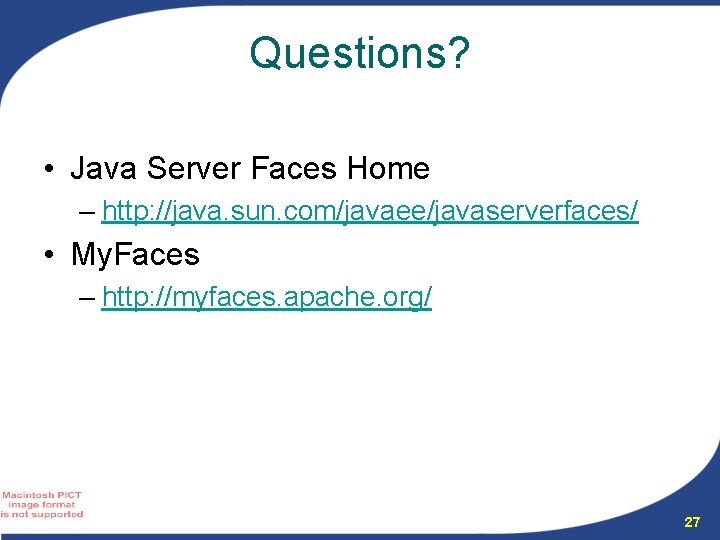
Questions? • Java Server Faces Home – http: //java. sun. com/javaee/javaserverfaces/ • My. Faces – http: //myfaces. apache. org/ 27
6 oblong faces
Server faces
Java server pages tutorial
Java telegram bot
Java irc
Java client server tutorial
Java.rmi.server.codebase
Lập trình socket giao tiếp tcp client/server java
Knock knock server java
Jsf sample
Java server pages
Java server pages
Jsp o que é
Java number
Import java.util.*
Java applet swing
Import.java.util.scanner
Import java.io.* import java.util.*
Java gcd
Import java.util.random;
Import java.io.* in java
Import java.util.
Java import java.io.*
Perbedaan java swing dan awt
Import java.awt.event.*
Language
Ejb remote vs local
Introduction to server side programming