INHERITANCE EXTENDING A CLASS Java allows you to
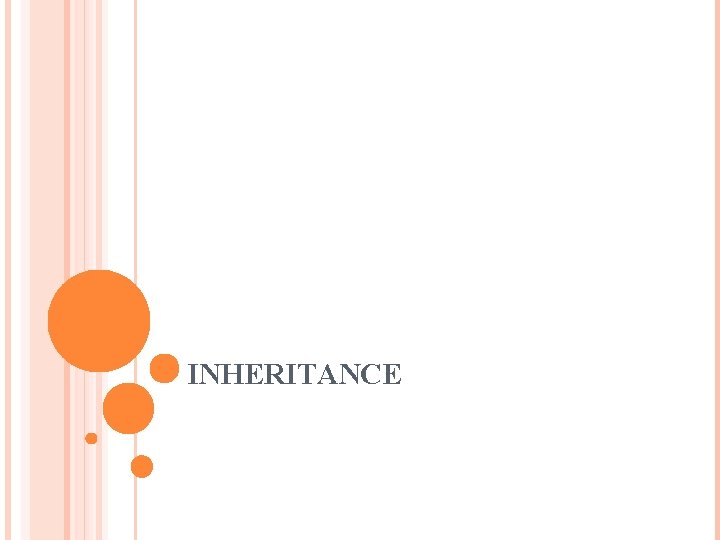
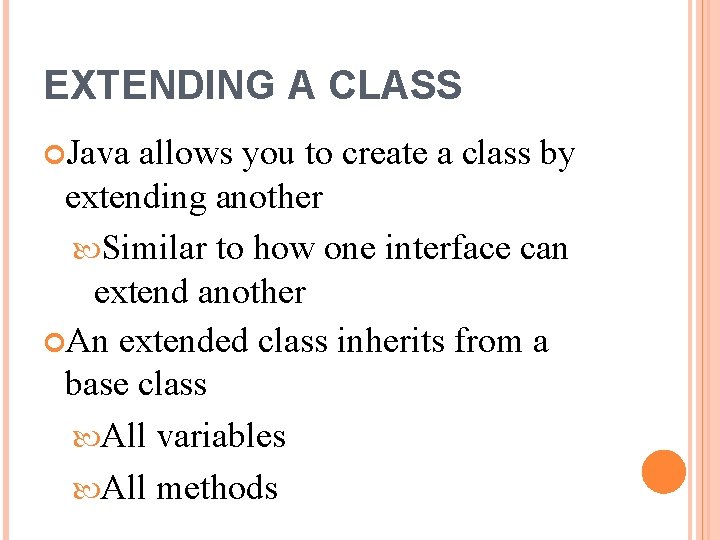
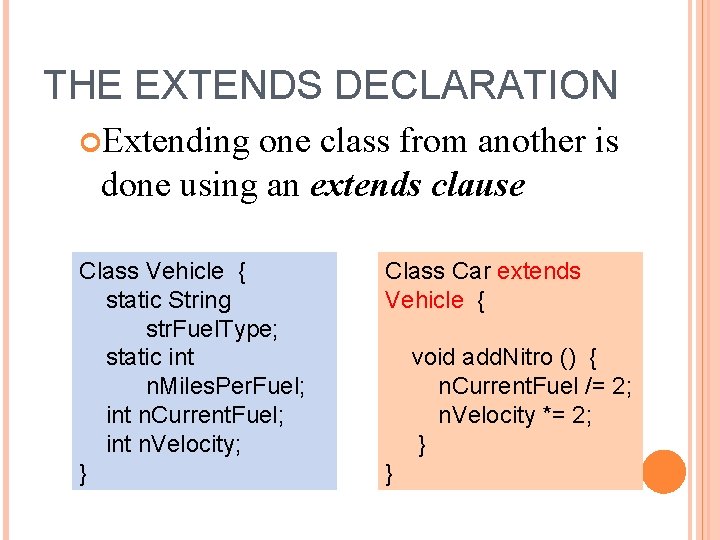
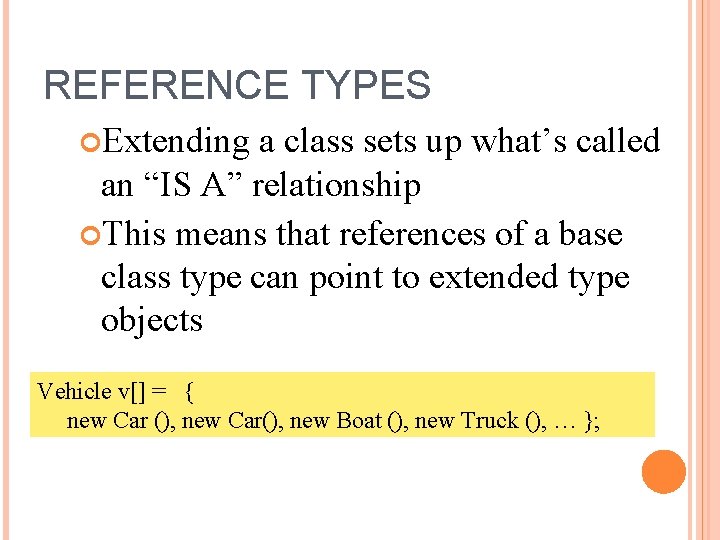
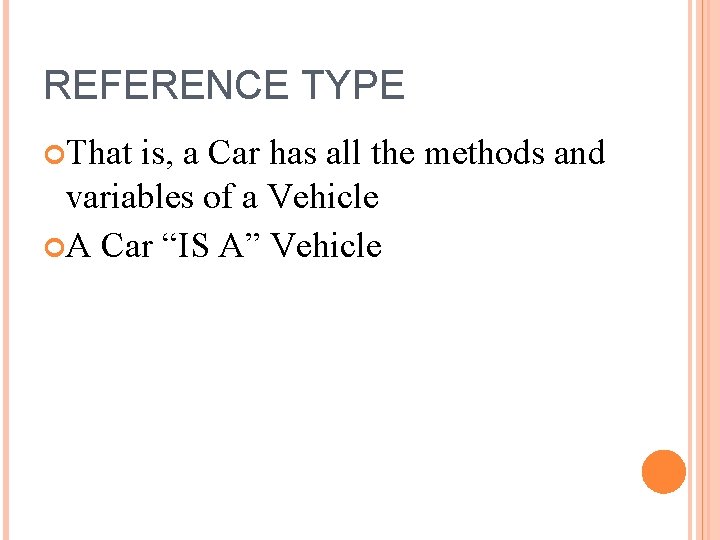
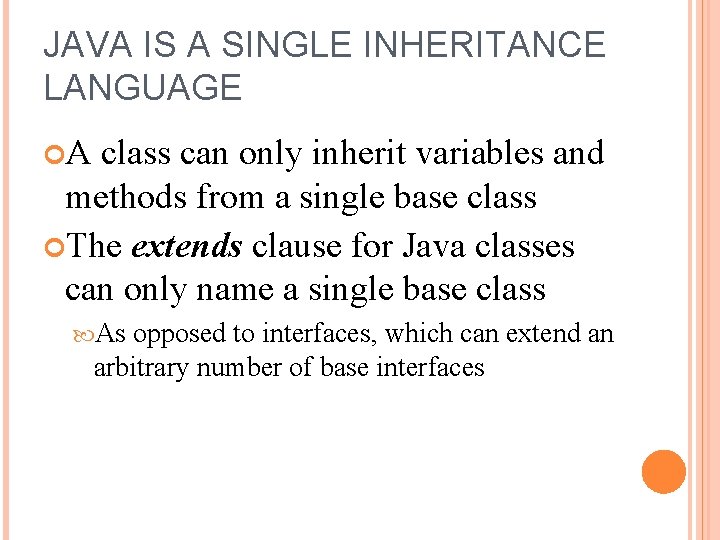
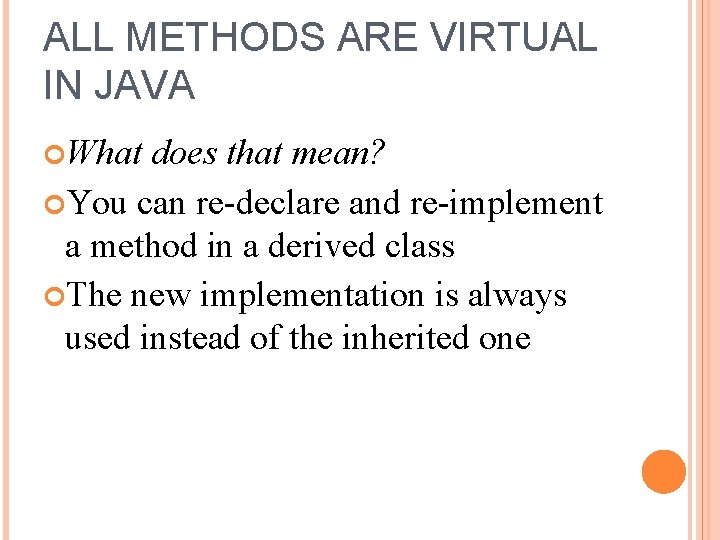
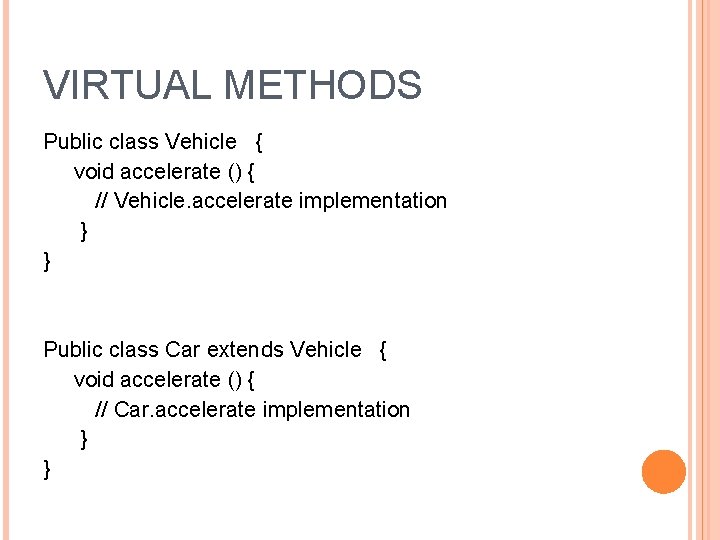
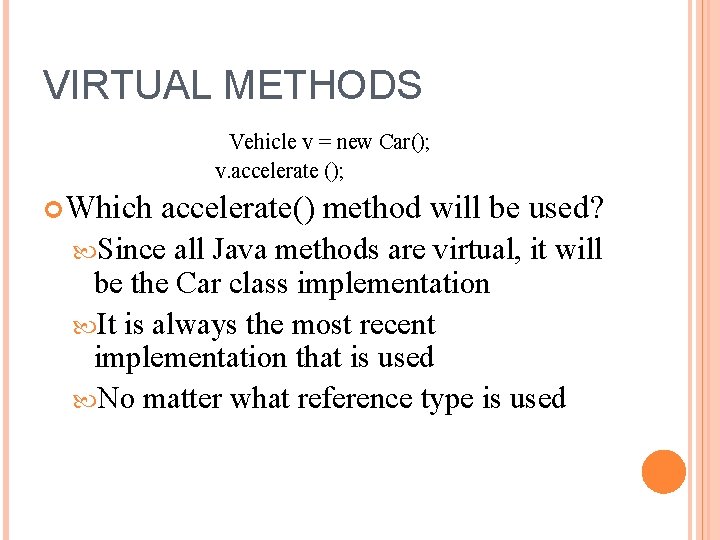
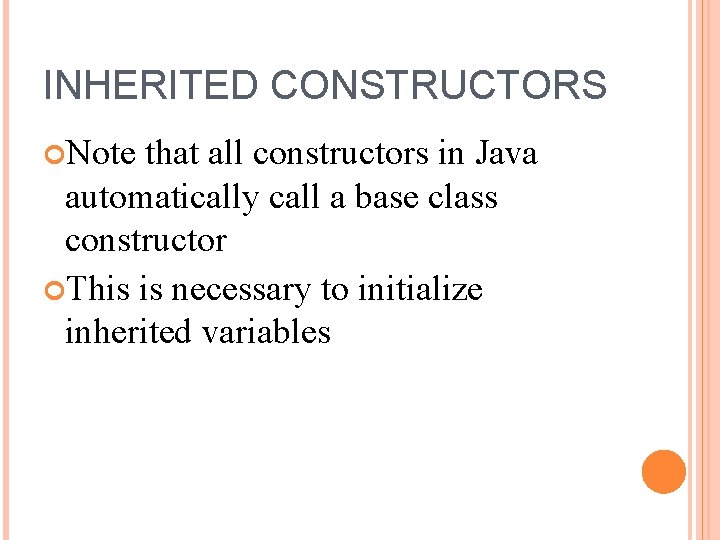
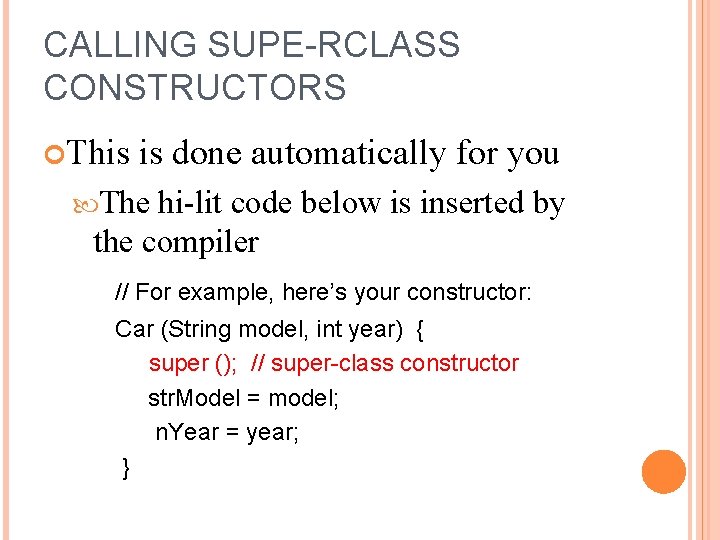
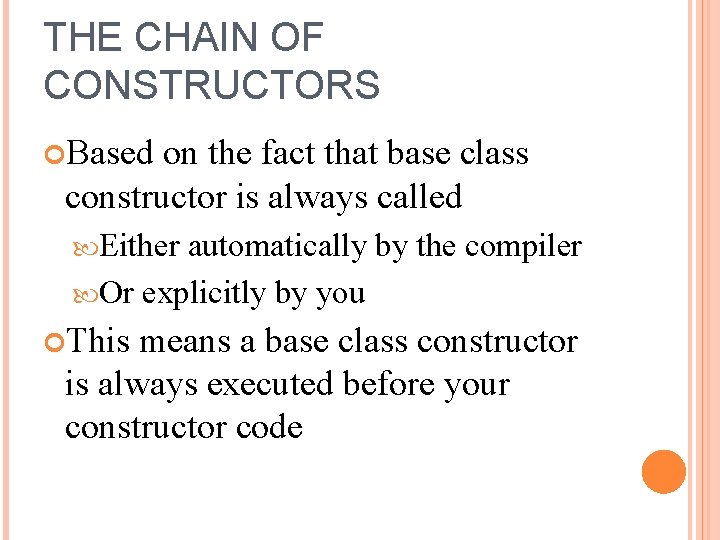
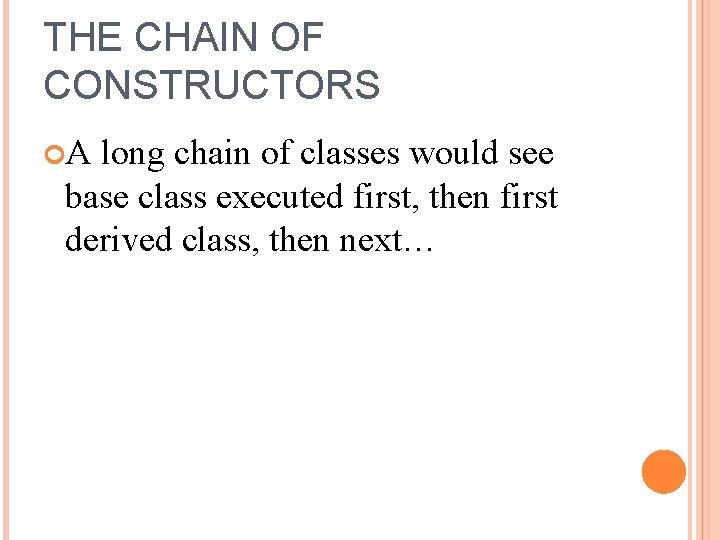
![Public class Constructor. Chain { public static void main (String[] args) { D d Public class Constructor. Chain { public static void main (String[] args) { D d](https://slidetodoc.com/presentation_image_h2/b5a6b06031ed0394a3e4cec13ed1a8bb/image-14.jpg)
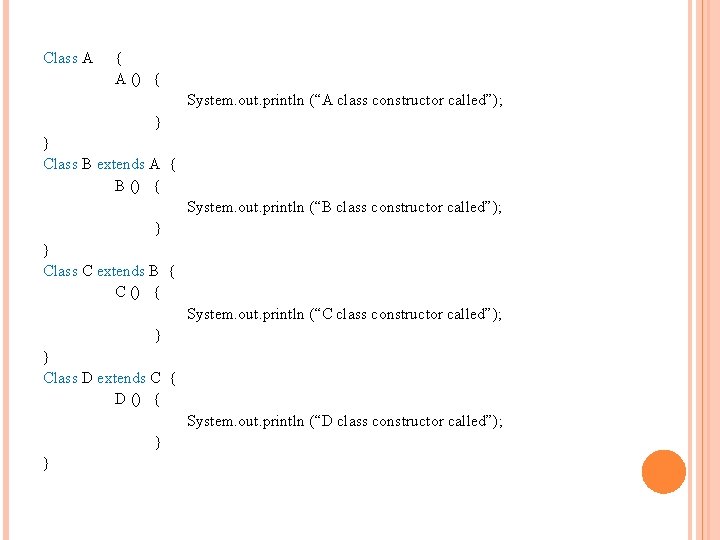
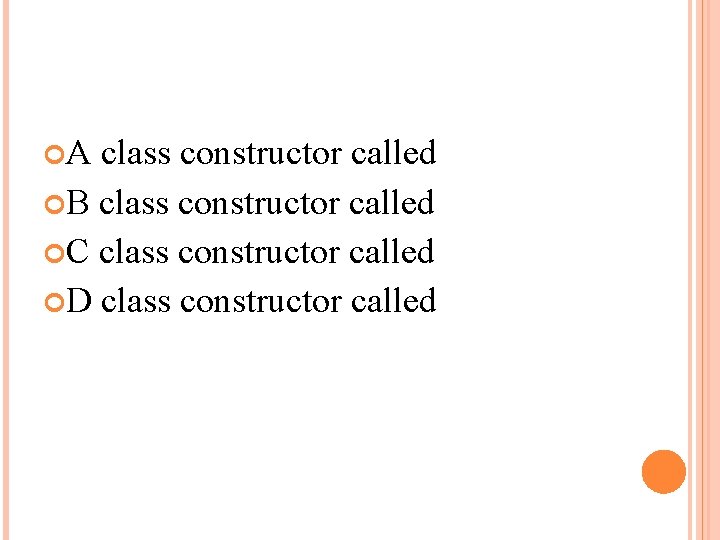
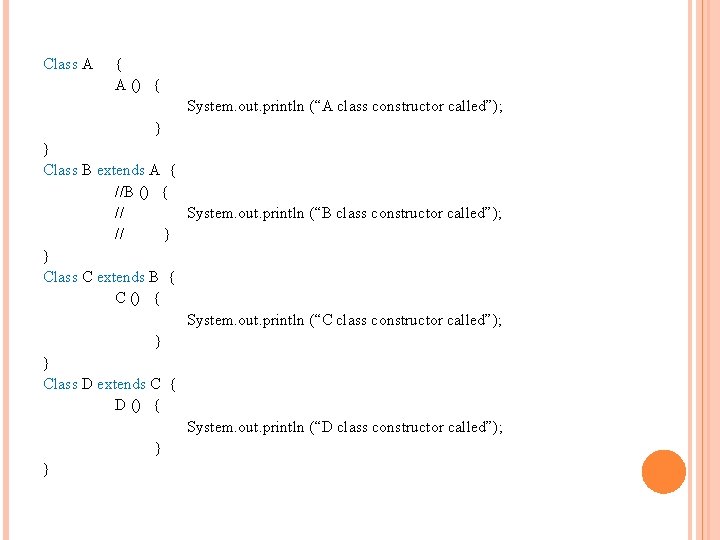
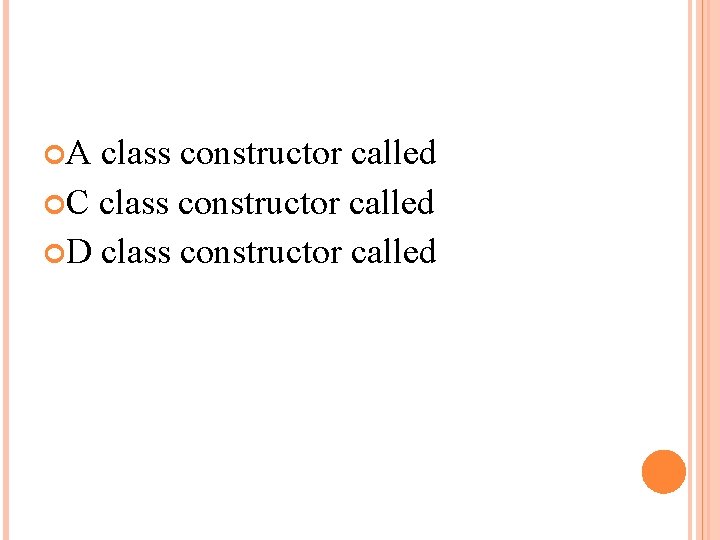
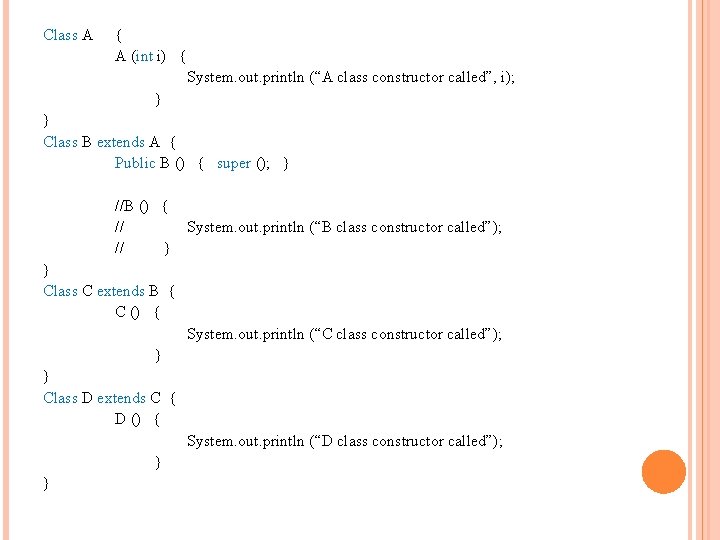
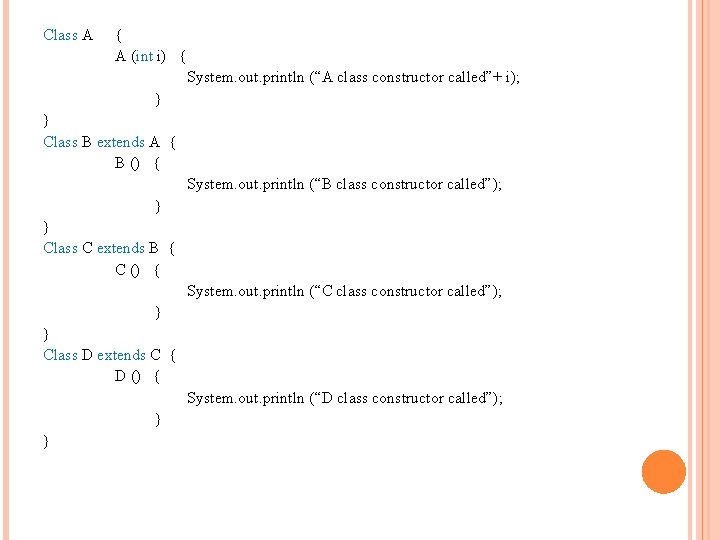
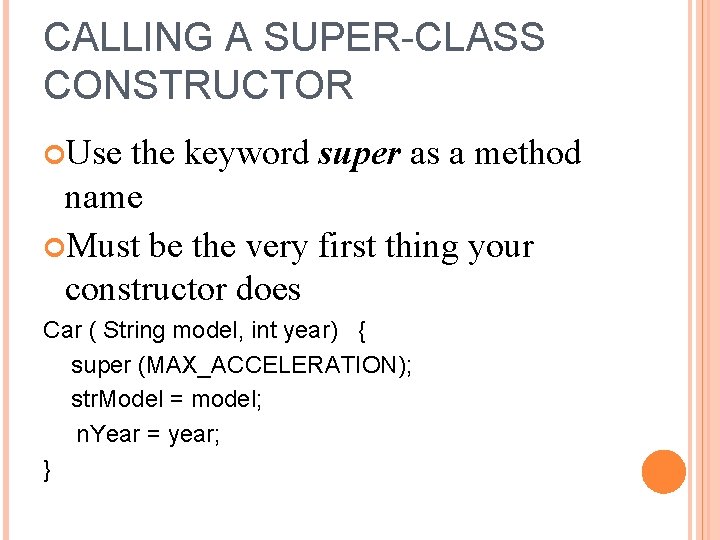
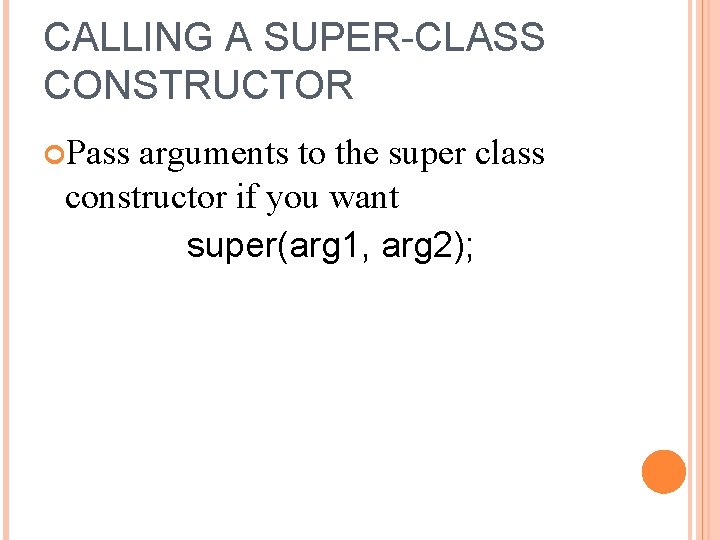
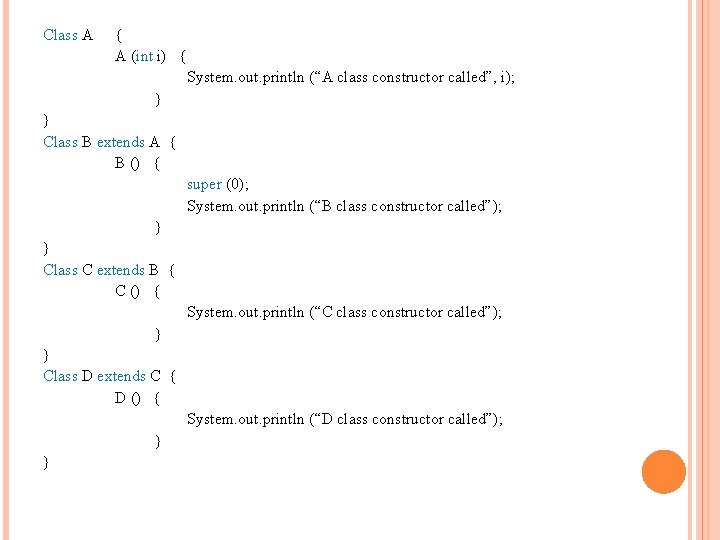
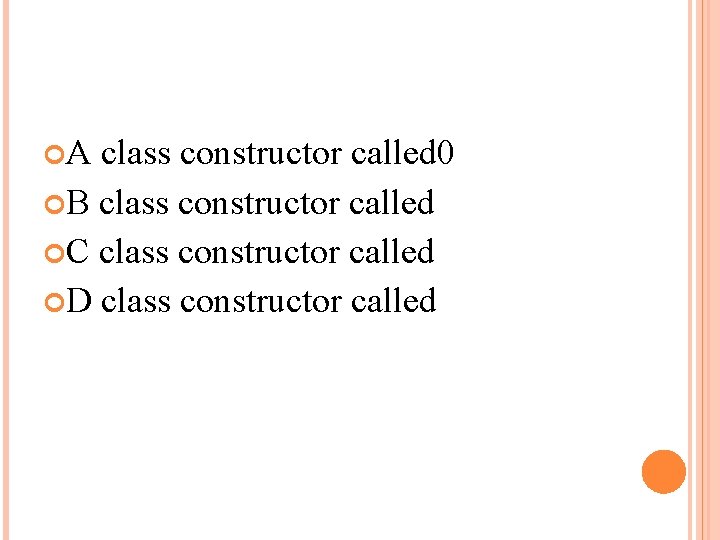
- Slides: 24
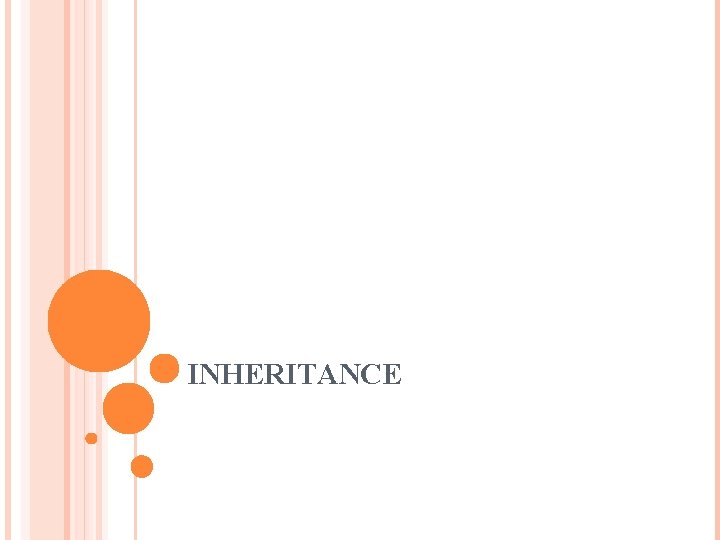
INHERITANCE
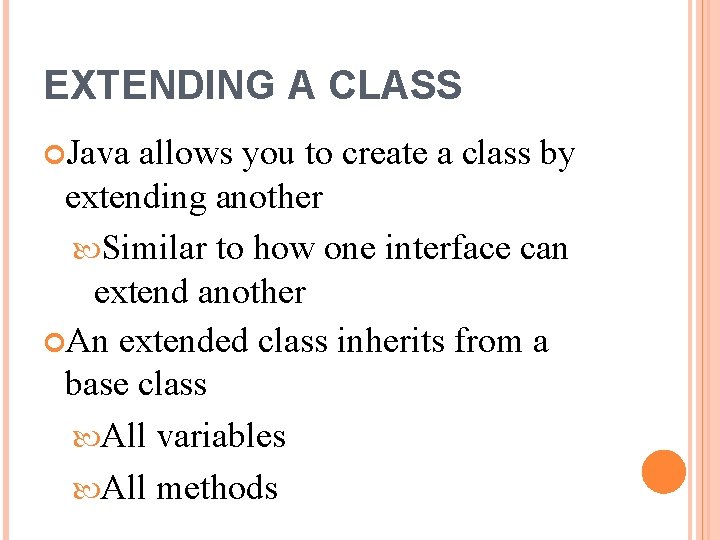
EXTENDING A CLASS Java allows you to create a class by extending another Similar to how one interface can extend another An extended class inherits from a base class All variables All methods
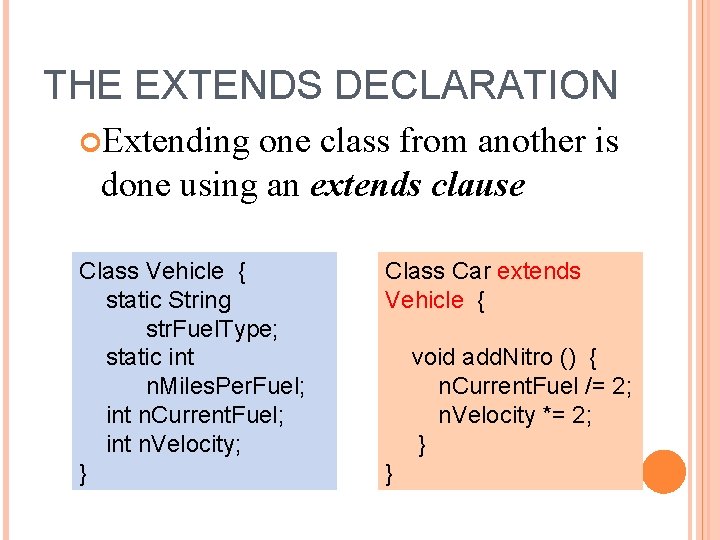
THE EXTENDS DECLARATION Extending one class from another is done using an extends clause Class Vehicle { static String str. Fuel. Type; static int n. Miles. Per. Fuel; int n. Current. Fuel; int n. Velocity; } Class Car extends Vehicle { void add. Nitro () { n. Current. Fuel /= 2; n. Velocity *= 2; } }
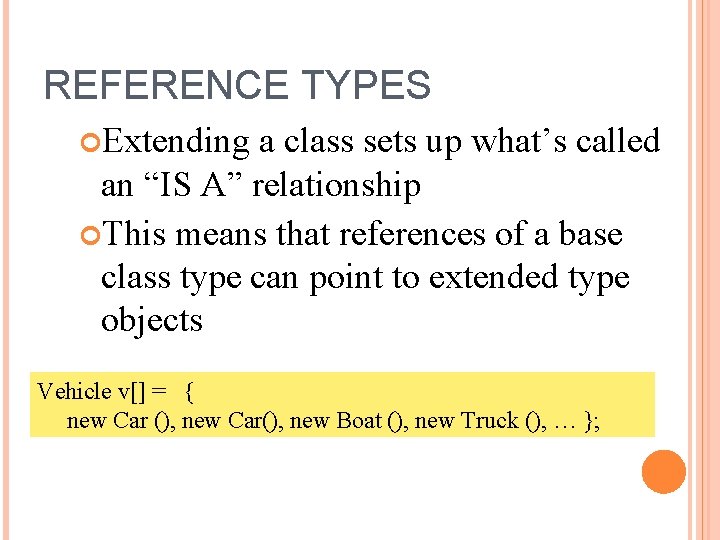
REFERENCE TYPES Extending a class sets up what’s called an “IS A” relationship This means that references of a base class type can point to extended type objects Vehicle v[] = { new Car (), new Car(), new Boat (), new Truck (), … };
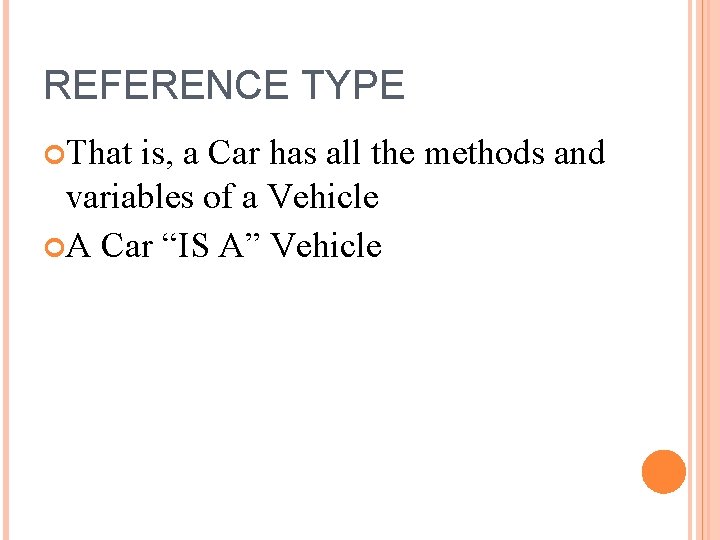
REFERENCE TYPE That is, a Car has all the methods and variables of a Vehicle A Car “IS A” Vehicle
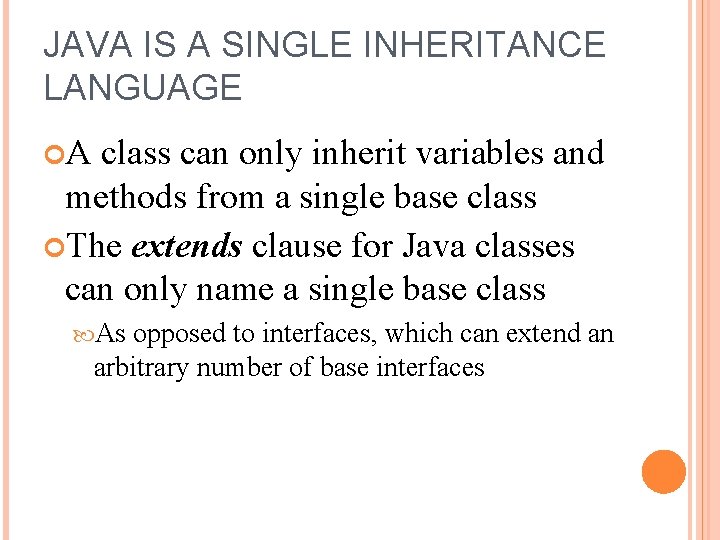
JAVA IS A SINGLE INHERITANCE LANGUAGE A class can only inherit variables and methods from a single base class The extends clause for Java classes can only name a single base class As opposed to interfaces, which can extend an arbitrary number of base interfaces
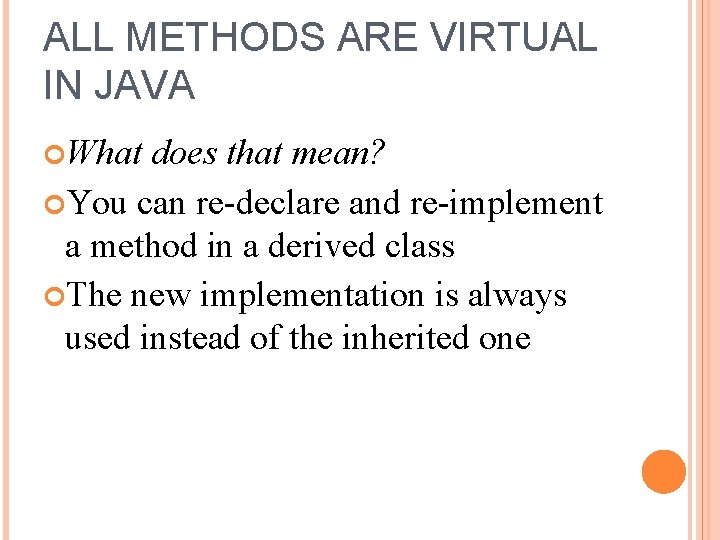
ALL METHODS ARE VIRTUAL IN JAVA What does that mean? You can re-declare and re-implement a method in a derived class The new implementation is always used instead of the inherited one
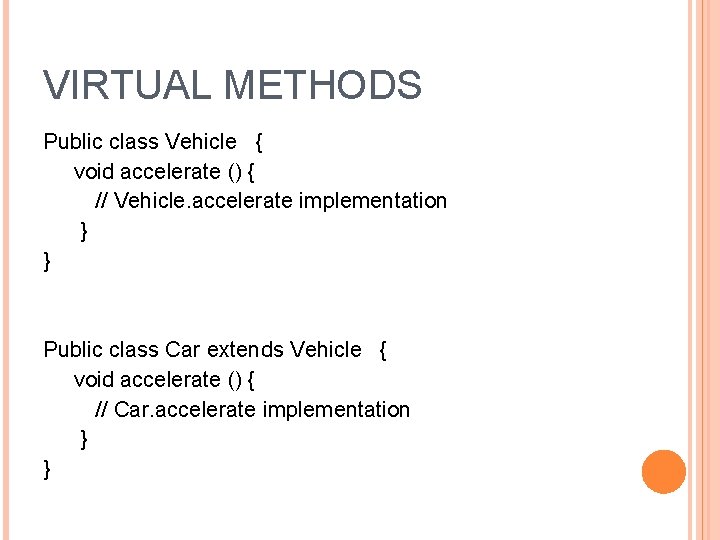
VIRTUAL METHODS Public class Vehicle { void accelerate () { // Vehicle. accelerate implementation } } Public class Car extends Vehicle { void accelerate () { // Car. accelerate implementation } }
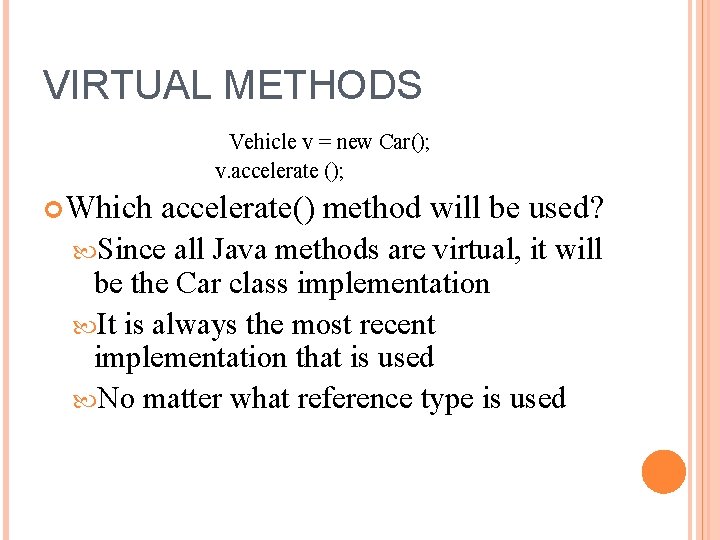
VIRTUAL METHODS Vehicle v = new Car(); v. accelerate (); Which accelerate() method will be used? Since all Java methods are virtual, it will be the Car class implementation It is always the most recent implementation that is used No matter what reference type is used
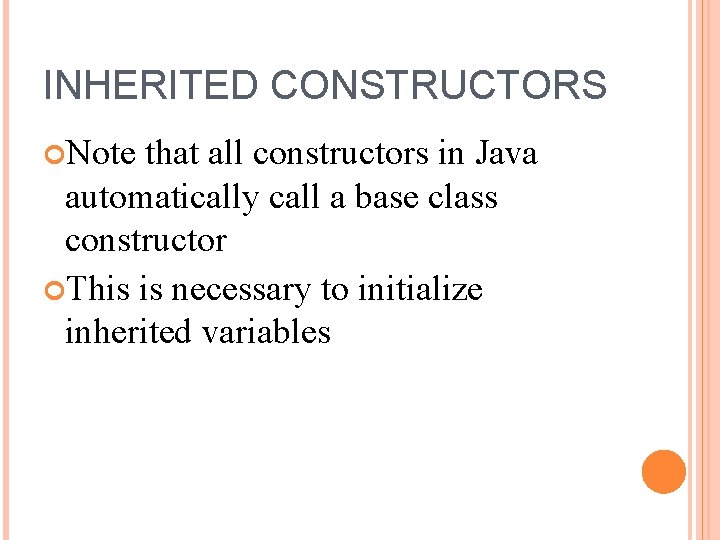
INHERITED CONSTRUCTORS Note that all constructors in Java automatically call a base class constructor This is necessary to initialize inherited variables
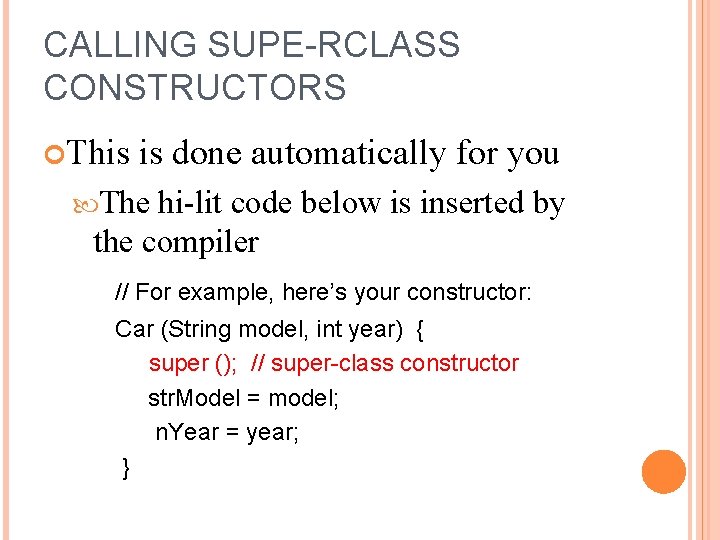
CALLING SUPE-RCLASS CONSTRUCTORS This is done automatically for you The hi-lit code below is inserted by the compiler // For example, here’s your constructor: Car (String model, int year) { super (); // super-class constructor str. Model = model; n. Year = year; }
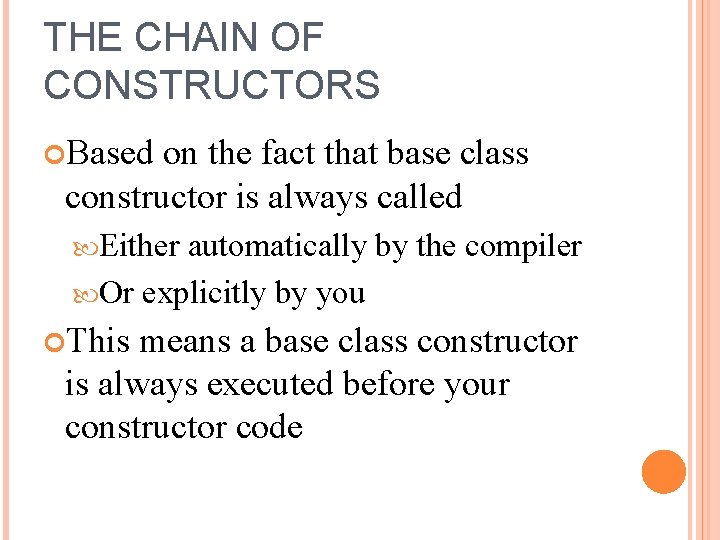
THE CHAIN OF CONSTRUCTORS Based on the fact that base class constructor is always called Either automatically by the compiler Or explicitly by you This means a base class constructor is always executed before your constructor code
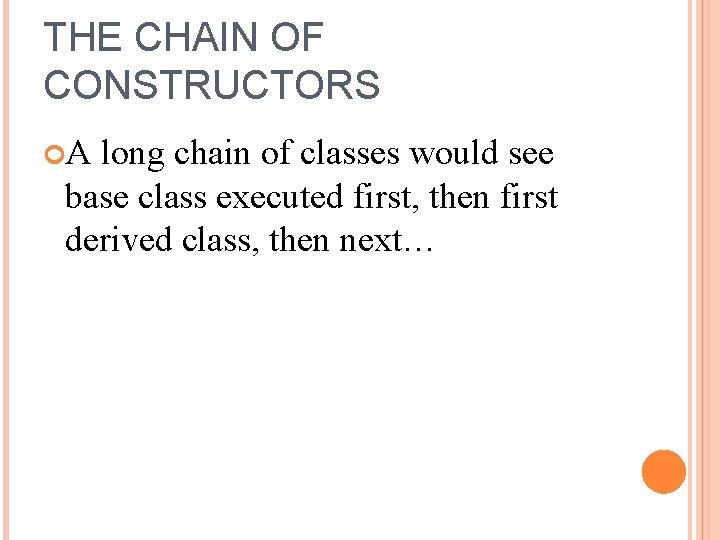
THE CHAIN OF CONSTRUCTORS A long chain of classes would see base class executed first, then first derived class, then next…
![Public class Constructor Chain public static void main String args D d Public class Constructor. Chain { public static void main (String[] args) { D d](https://slidetodoc.com/presentation_image_h2/b5a6b06031ed0394a3e4cec13ed1a8bb/image-14.jpg)
Public class Constructor. Chain { public static void main (String[] args) { D d = new D (); try { System. in. read (); } catch (java. io. IOException ioe) { } } }
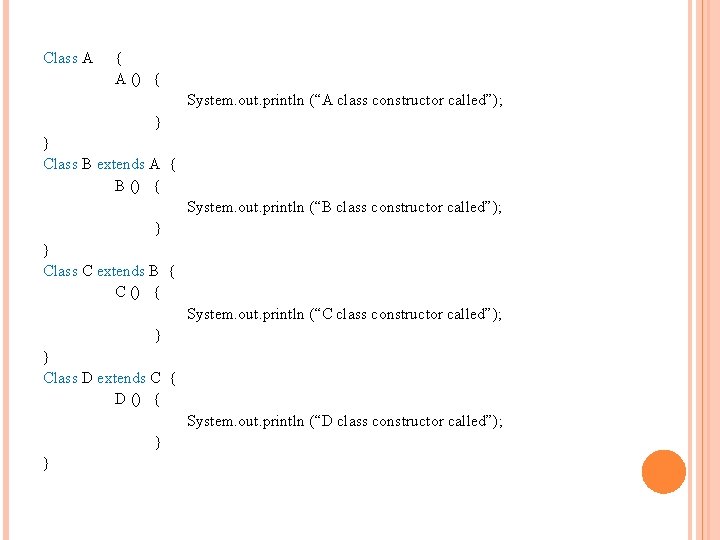
Class A { A () { System. out. println (“A class constructor called”); } } Class B extends A { B () { System. out. println (“B class constructor called”); } } Class C extends B { C () { System. out. println (“C class constructor called”); } } Class D extends C { D () { System. out. println (“D class constructor called”); } }
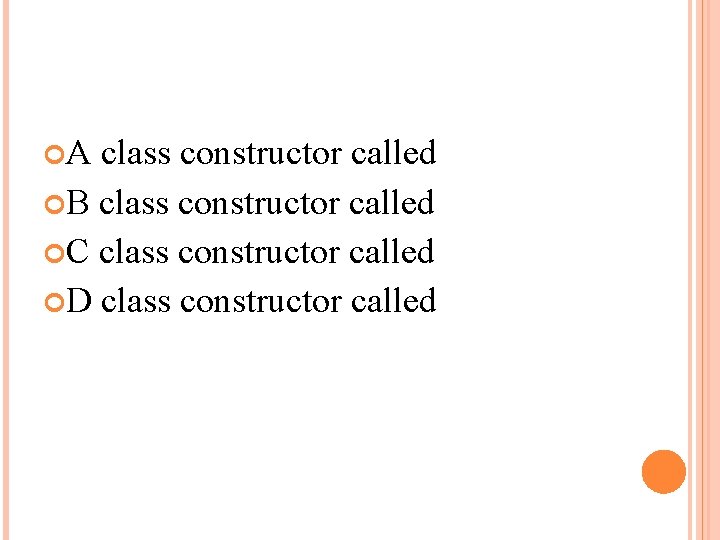
A class constructor called B class constructor called C class constructor called D class constructor called
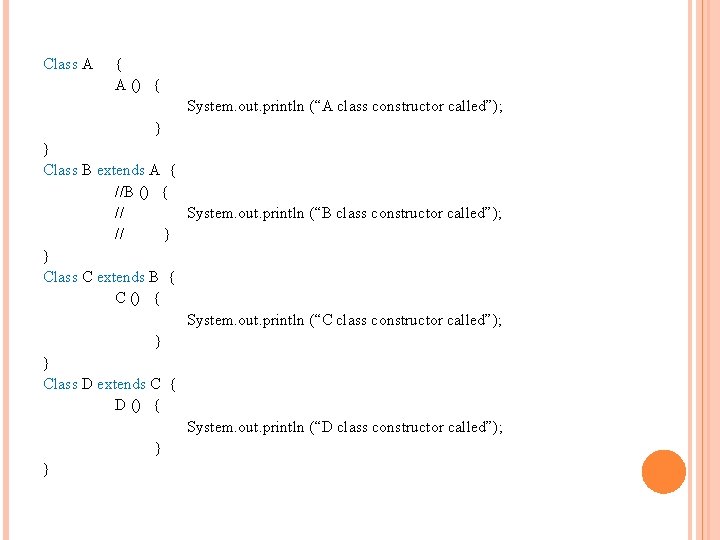
Class A { A () { System. out. println (“A class constructor called”); } } Class B extends A { //B () { // System. out. println (“B class constructor called”); // } } Class C extends B { C () { System. out. println (“C class constructor called”); } } Class D extends C { D () { System. out. println (“D class constructor called”); } }
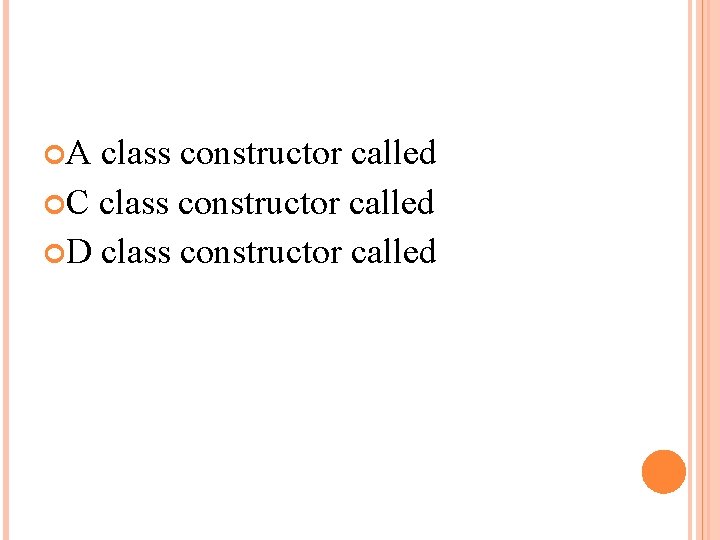
A class constructor called C class constructor called D class constructor called
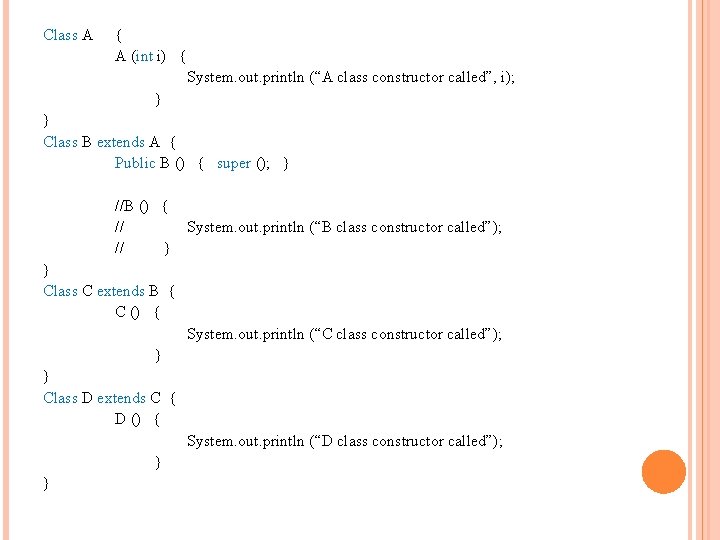
Class A { A (int i) { System. out. println (“A class constructor called”, i); } } Class B extends A { Public B () { super (); } //B () { // System. out. println (“B class constructor called”); // } } Class C extends B { C () { System. out. println (“C class constructor called”); } } Class D extends C { D () { System. out. println (“D class constructor called”); } }
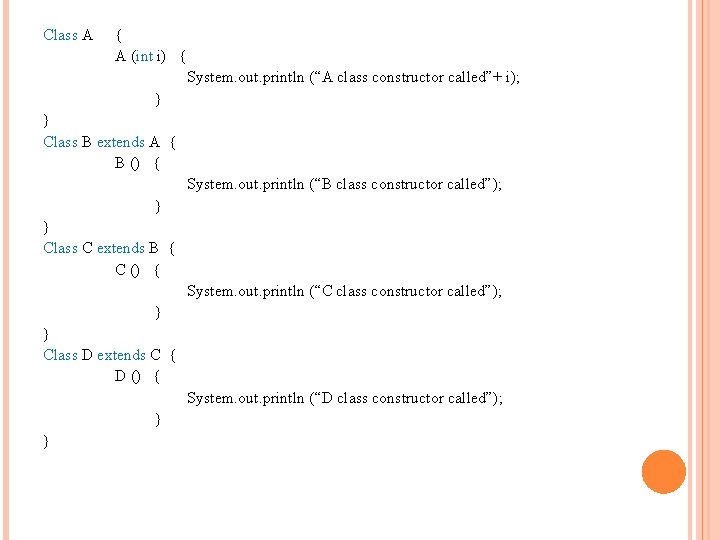
Class A { A (int i) { System. out. println (“A class constructor called”+ i); } } Class B extends A { B () { System. out. println (“B class constructor called”); } } Class C extends B { C () { System. out. println (“C class constructor called”); } } Class D extends C { D () { System. out. println (“D class constructor called”); } }
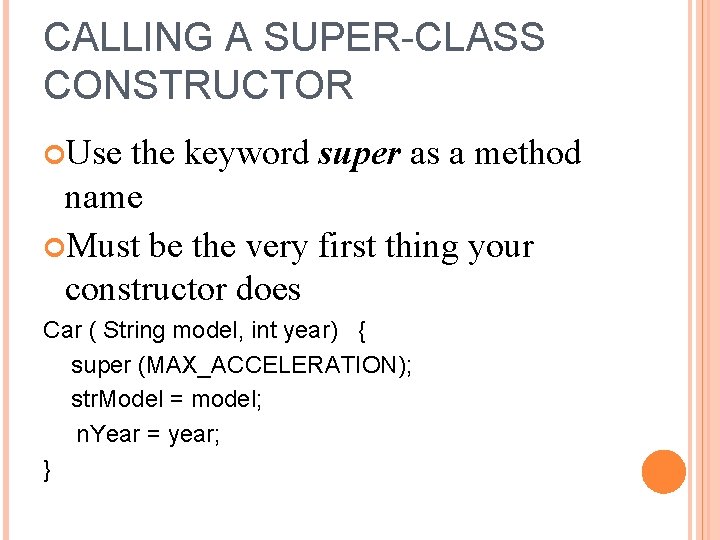
CALLING A SUPER-CLASS CONSTRUCTOR Use the keyword super as a method name Must be the very first thing your constructor does Car ( String model, int year) { super (MAX_ACCELERATION); str. Model = model; n. Year = year; }
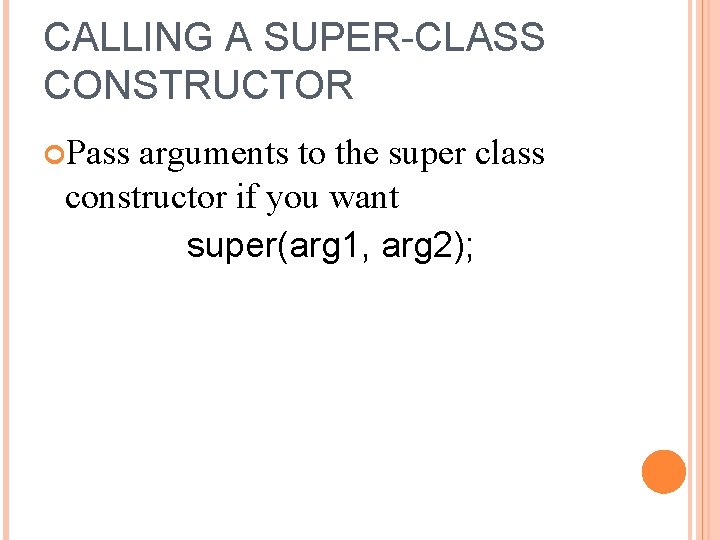
CALLING A SUPER-CLASS CONSTRUCTOR Pass arguments to the super class constructor if you want super(arg 1, arg 2);
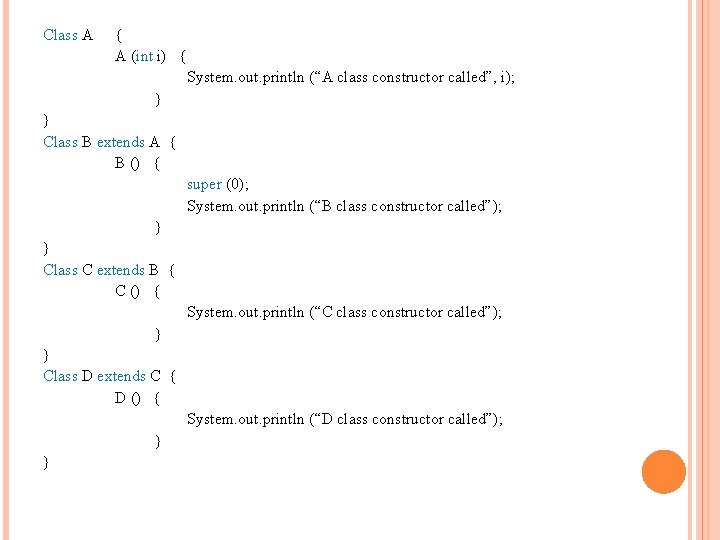
Class A { A (int i) { System. out. println (“A class constructor called”, i); } } Class B extends A { B () { super (0); System. out. println (“B class constructor called”); } } Class C extends B { C () { System. out. println (“C class constructor called”); } } Class D extends C { D () { System. out. println (“D class constructor called”); } }
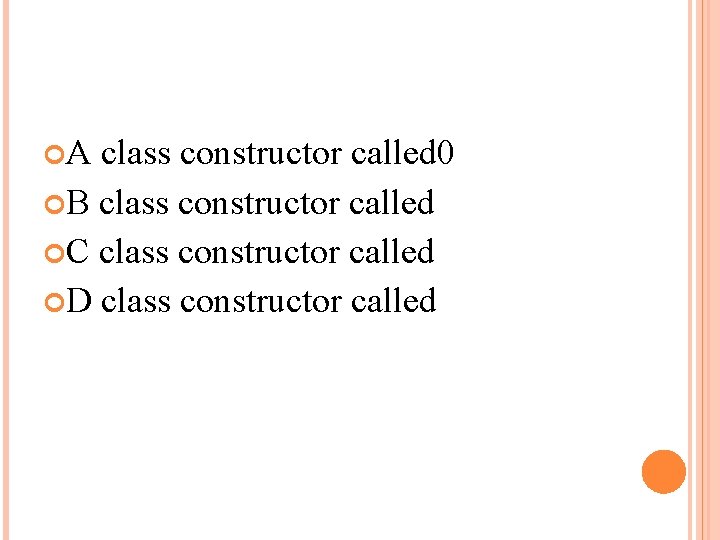
A class constructor called 0 B class constructor called C class constructor called D class constructor called
Inheritance allows
Elements of spoken word poetry
Hierarchical inheritance in java
Advantages and disadvantages of inheritance in java
Savings account java
Type of inheritance
Contoh program inheritance java
Java inheritance lab exercise
Java definition
Extending x-bar theory to functional categories
Emerging proficient extending
La=2πrh
Extending oblivious transfers efficiently
Chapter 7 extending mendelian genetics answer key
Uv xray
Uv spectra of dienes
Extending mendelian genetics answer key
Section 1 chromosomes and phenotype study guide a
A high point of land extending into water
Problem 8-3 extending amounts across the work sheet
Very large land mass
X bar theory practice
What are landforms
Dps tps
Parallelogram desk