1 Chapter 10 Inheritance 2 Inheritance allows a
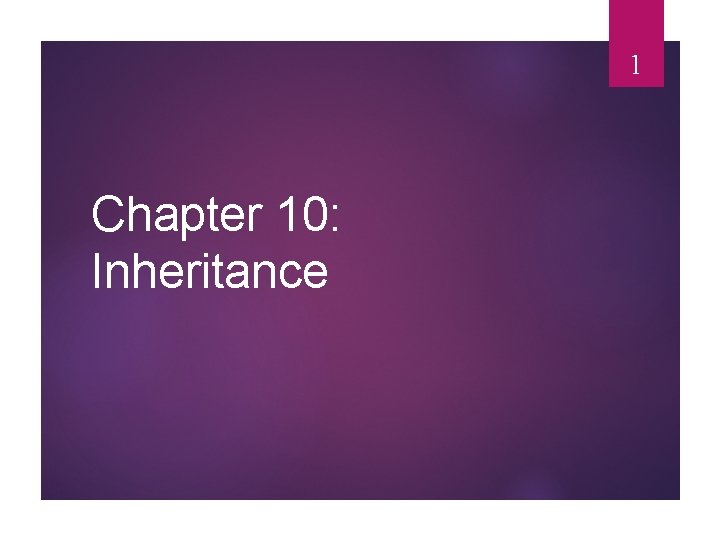
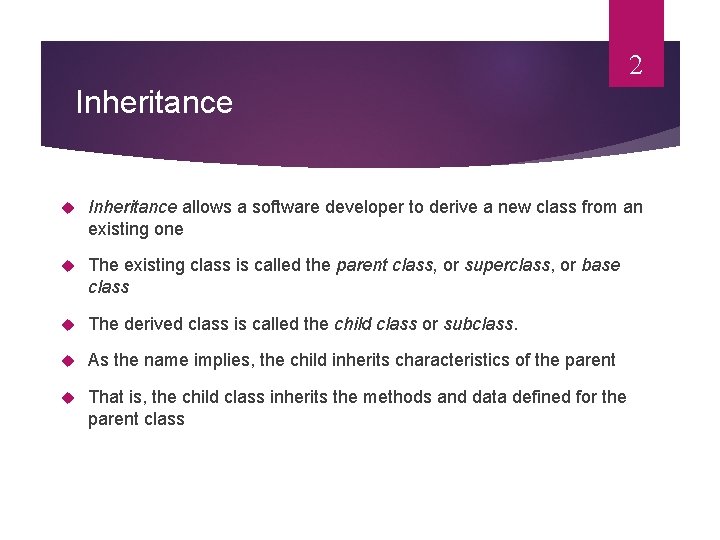
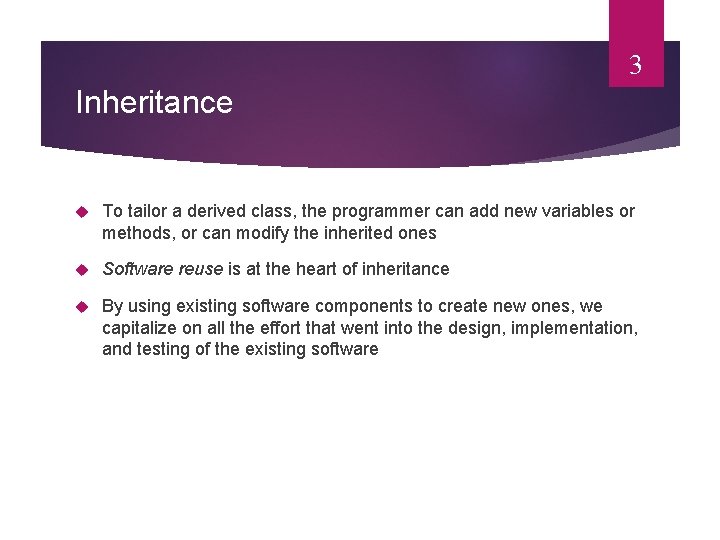
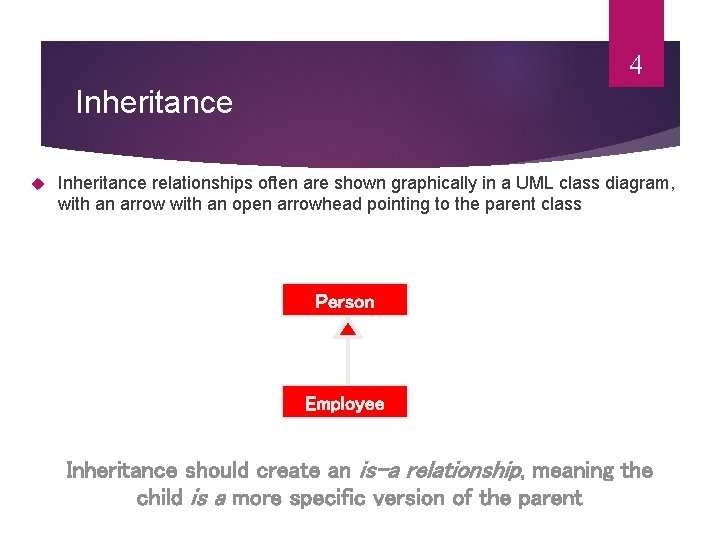
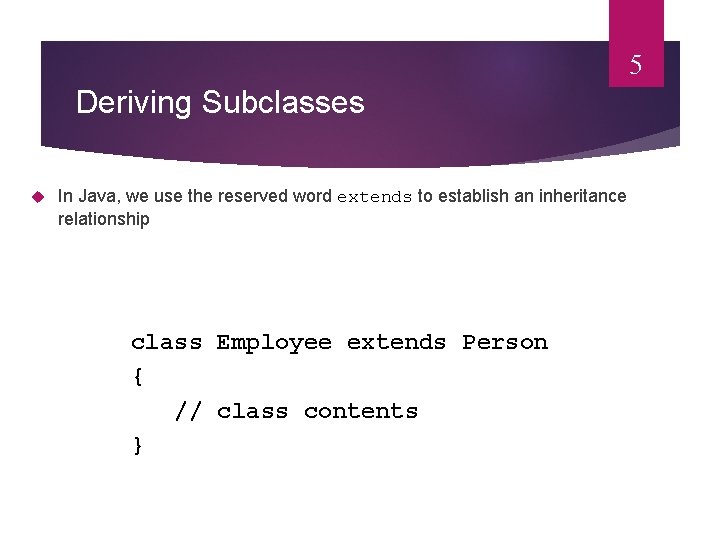
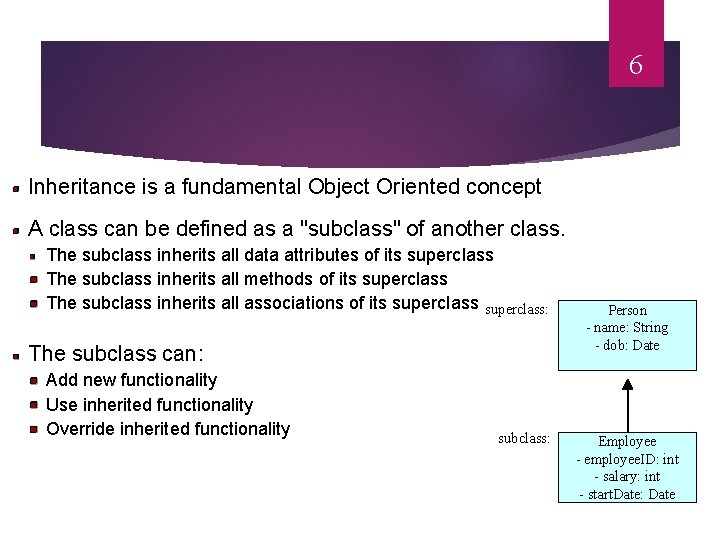
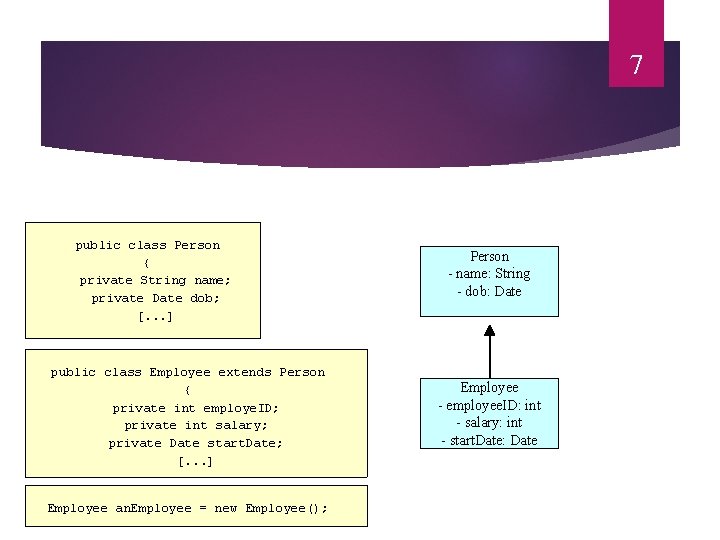
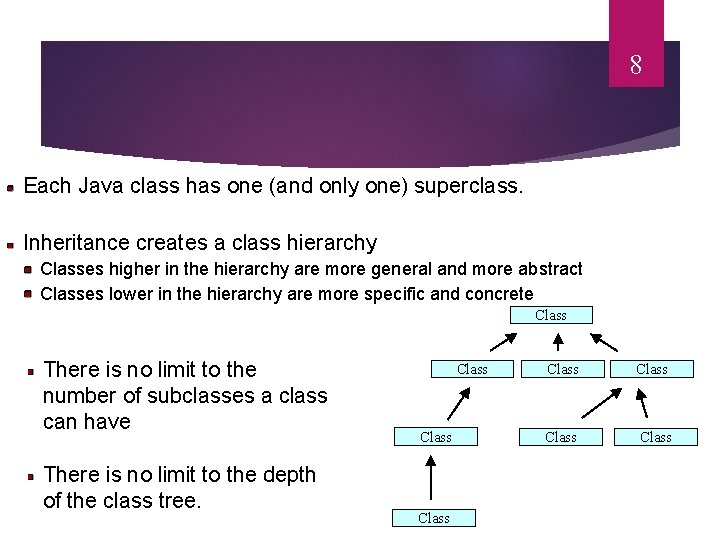
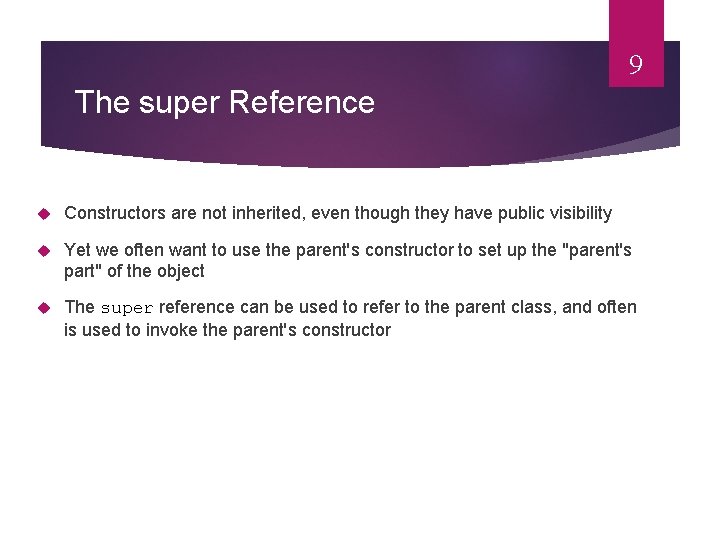
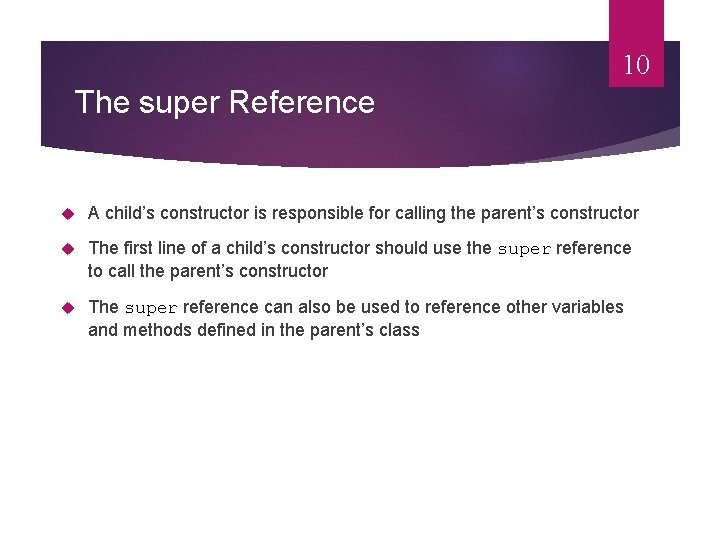
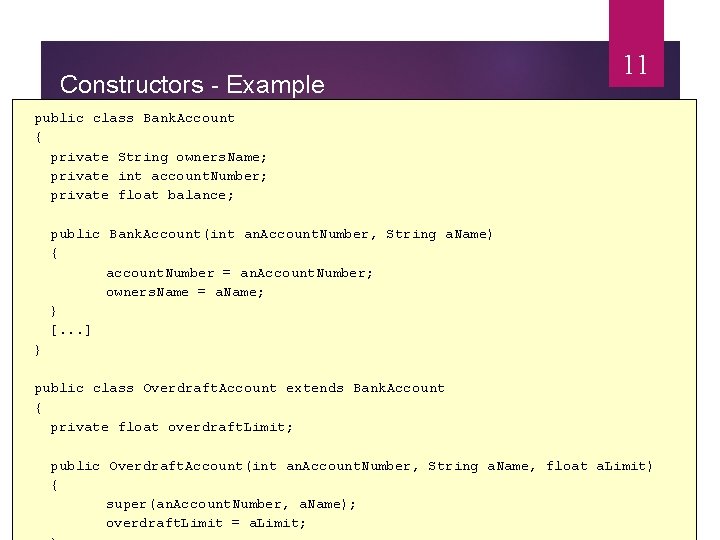
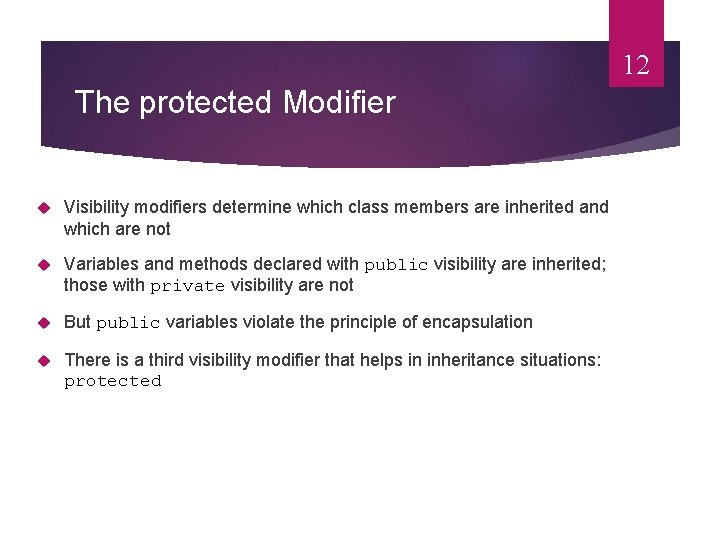
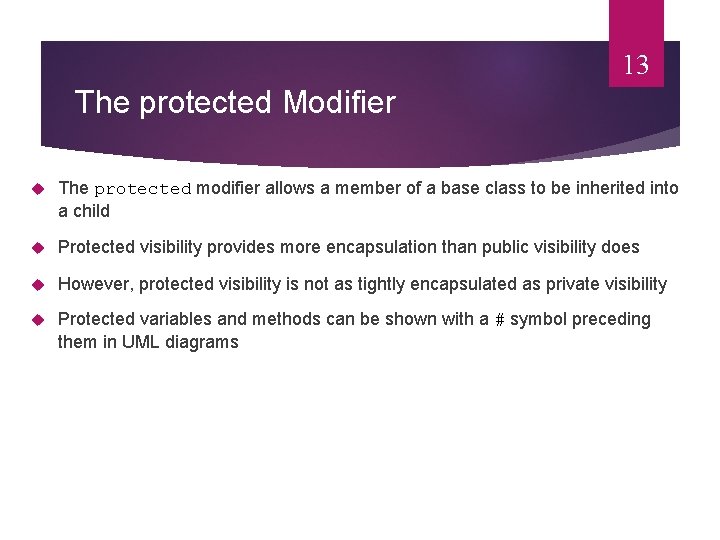
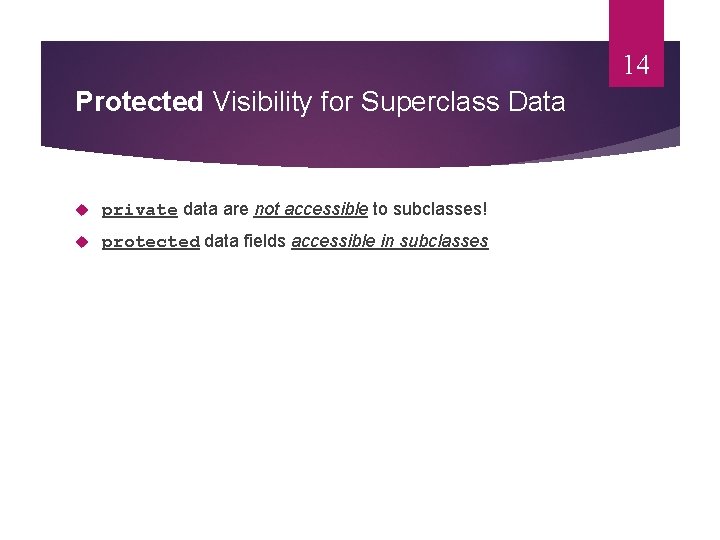
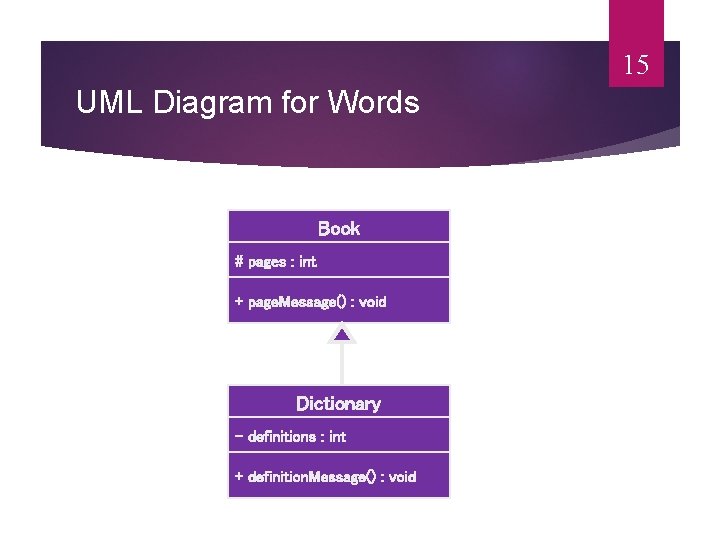
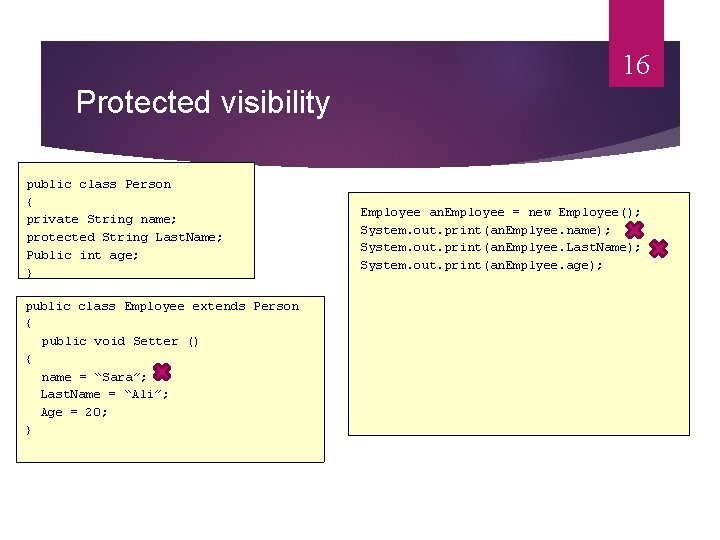
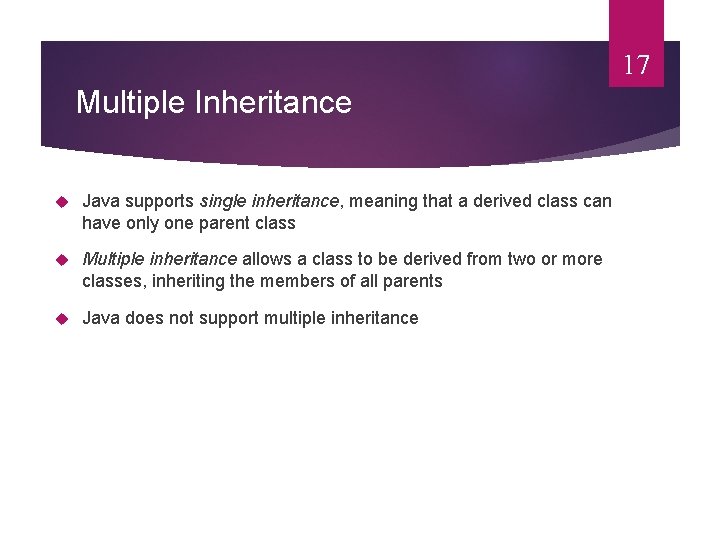
- Slides: 17
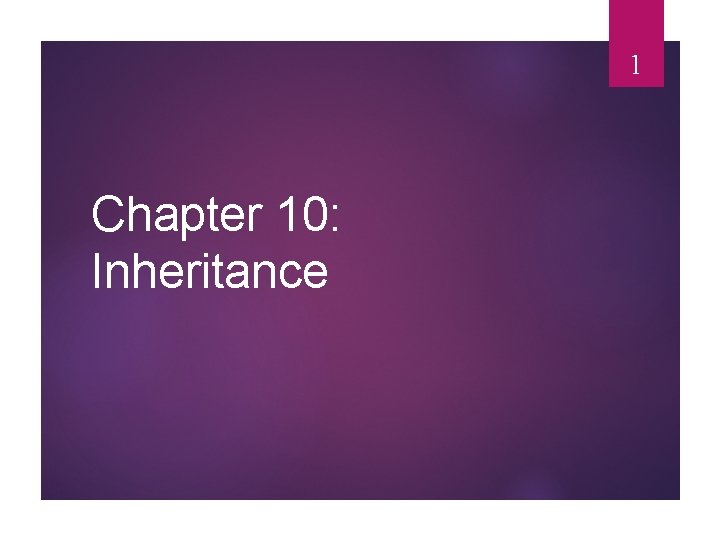
1 Chapter 10: Inheritance
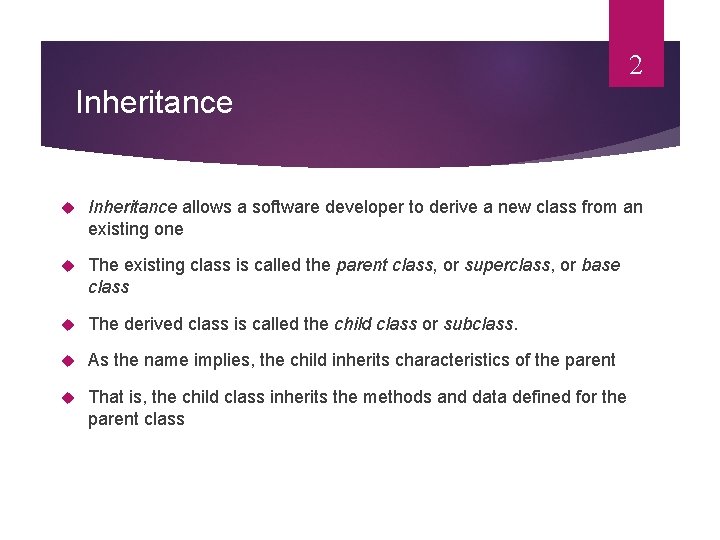
2 Inheritance allows a software developer to derive a new class from an existing one The existing class is called the parent class, or superclass, or base class The derived class is called the child class or subclass. As the name implies, the child inherits characteristics of the parent That is, the child class inherits the methods and data defined for the parent class
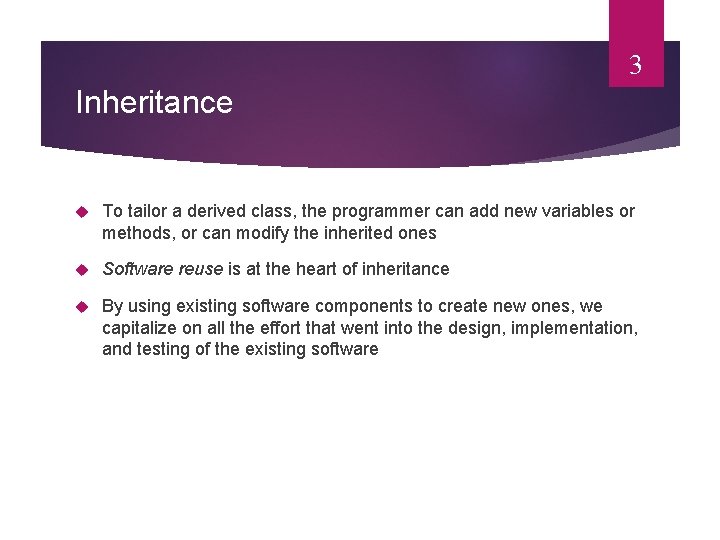
3 Inheritance To tailor a derived class, the programmer can add new variables or methods, or can modify the inherited ones Software reuse is at the heart of inheritance By using existing software components to create new ones, we capitalize on all the effort that went into the design, implementation, and testing of the existing software
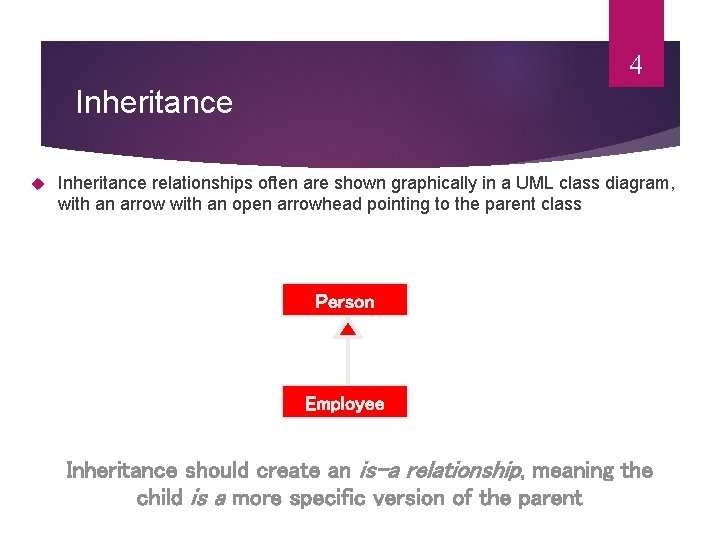
4 Inheritance relationships often are shown graphically in a UML class diagram, with an arrow with an open arrowhead pointing to the parent class Person Employee Inheritance should create an is-a relationship, meaning the child is a more specific version of the parent
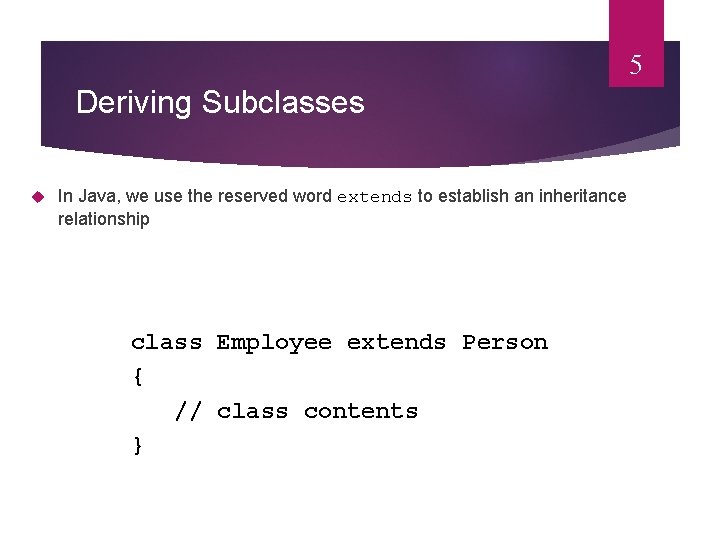
5 Deriving Subclasses In Java, we use the reserved word extends to establish an inheritance relationship class Employee extends Person { // class contents }
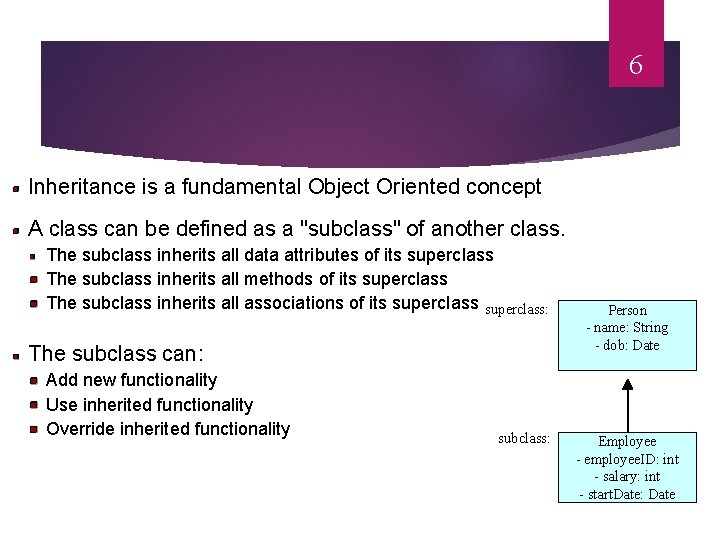
6 Inheritance is a fundamental Object Oriented concept A class can be defined as a "subclass" of another class. The subclass inherits all data attributes of its superclass The subclass inherits all methods of its superclass The subclass inherits all associations of its superclass: The subclass can: Add new functionality Use inherited functionality Override inherited functionality subclass: Person - name: String - dob: Date Employee - employee. ID: int - salary: int - start. Date: Date
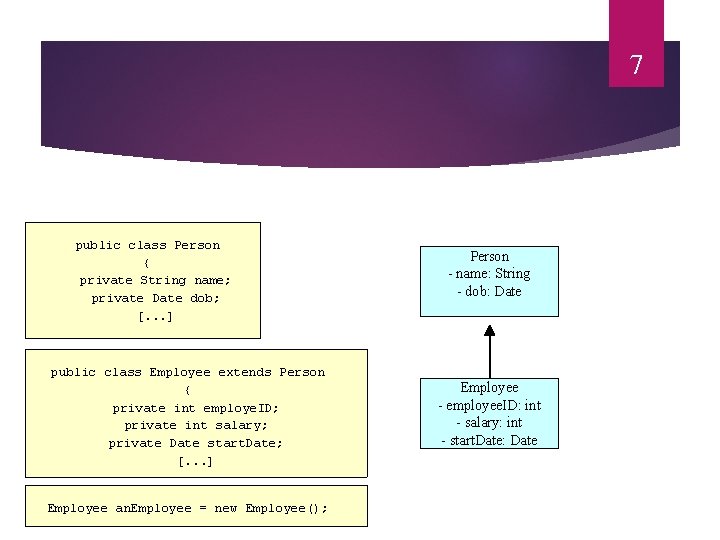
7 public class Person { private String name; private Date dob; [. . . ] public class Employee extends Person { private int employe. ID; private int salary; private Date start. Date; [. . . ] Employee an. Employee = new Employee(); Person - name: String - dob: Date Employee - employee. ID: int - salary: int - start. Date: Date
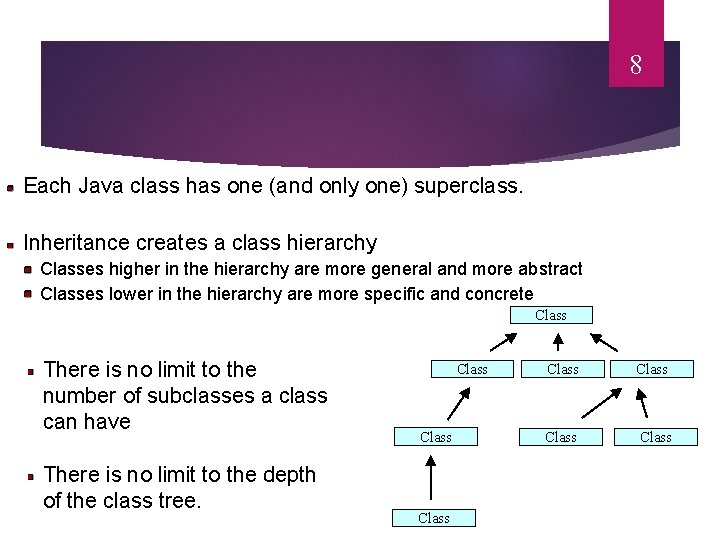
8 Each Java class has one (and only one) superclass. Inheritance creates a class hierarchy Classes higher in the hierarchy are more general and more abstract Classes lower in the hierarchy are more specific and concrete Class There is no limit to the number of subclasses a class can have There is no limit to the depth of the class tree. Class Class
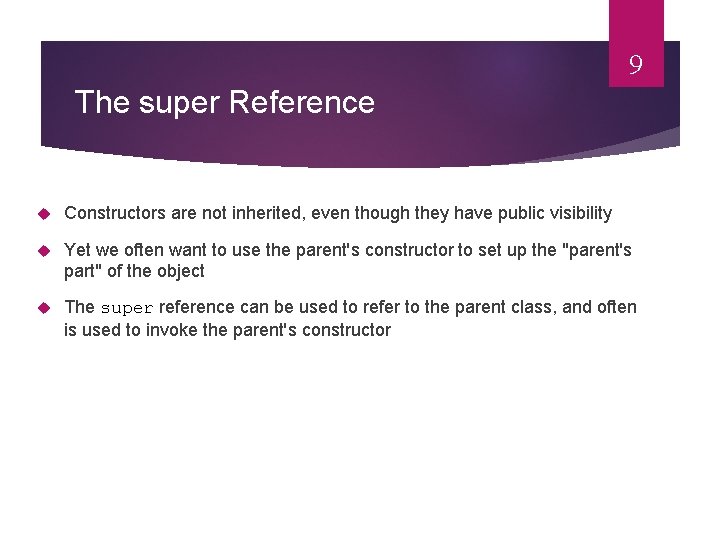
9 The super Reference Constructors are not inherited, even though they have public visibility Yet we often want to use the parent's constructor to set up the "parent's part" of the object The super reference can be used to refer to the parent class, and often is used to invoke the parent's constructor
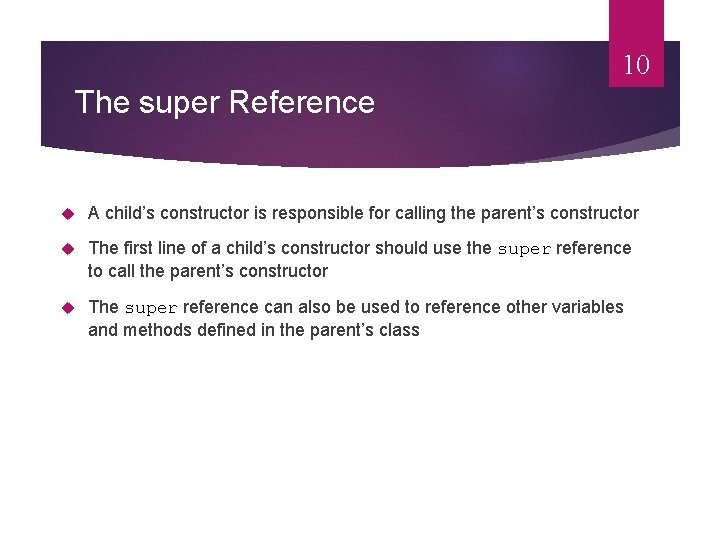
10 The super Reference A child’s constructor is responsible for calling the parent’s constructor The first line of a child’s constructor should use the super reference to call the parent’s constructor The super reference can also be used to reference other variables and methods defined in the parent’s class
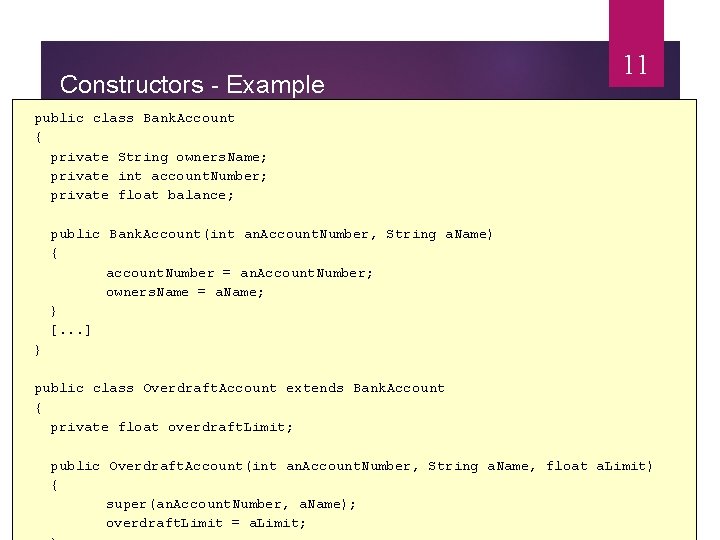
Constructors - Example 11 public class Bank. Account { private String owners. Name; private int account. Number; private float balance; public Bank. Account(int an. Account. Number, String a. Name) { account. Number = an. Account. Number; owners. Name = a. Name; } [. . . ] } public class Overdraft. Account extends Bank. Account { private float overdraft. Limit; public Overdraft. Account(int an. Account. Number, String a. Name, float a. Limit) { super(an. Account. Number, a. Name); overdraft. Limit = a. Limit;
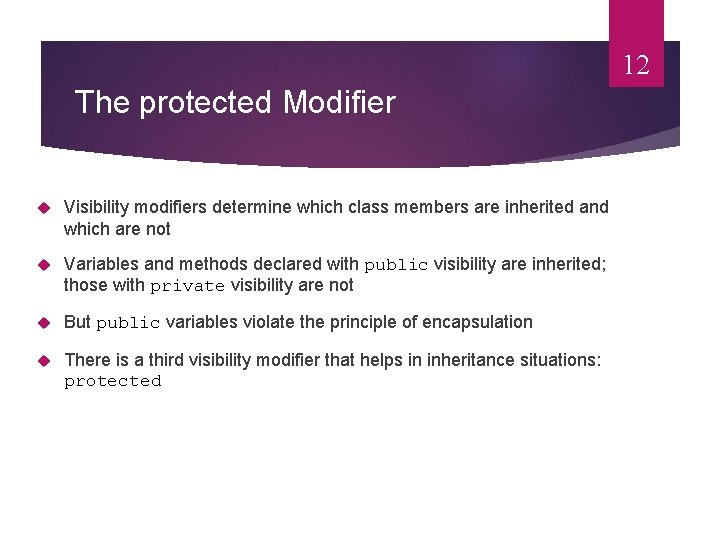
12 The protected Modifier Visibility modifiers determine which class members are inherited and which are not Variables and methods declared with public visibility are inherited; those with private visibility are not But public variables violate the principle of encapsulation There is a third visibility modifier that helps in inheritance situations: protected
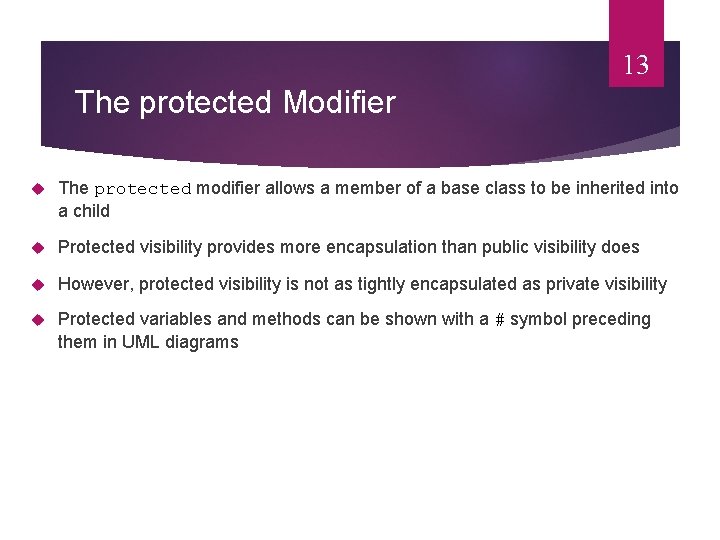
13 The protected Modifier The protected modifier allows a member of a base class to be inherited into a child Protected visibility provides more encapsulation than public visibility does However, protected visibility is not as tightly encapsulated as private visibility Protected variables and methods can be shown with a # symbol preceding them in UML diagrams
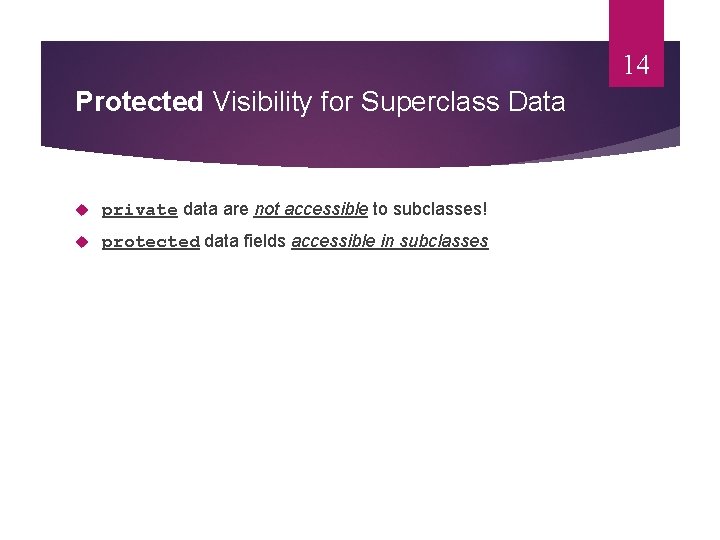
14 Protected Visibility for Superclass Data private data are not accessible to subclasses! protected data fields accessible in subclasses
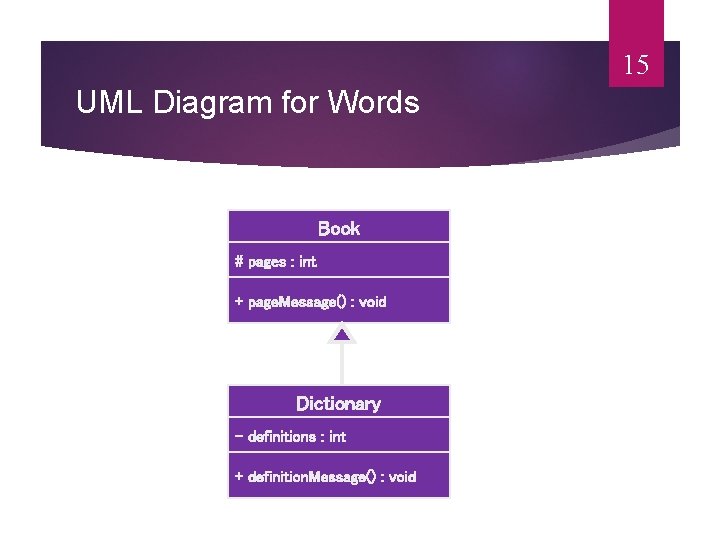
15 UML Diagram for Words Book # pages : int + page. Message() : void Dictionary - definitions : int + definition. Message() : void
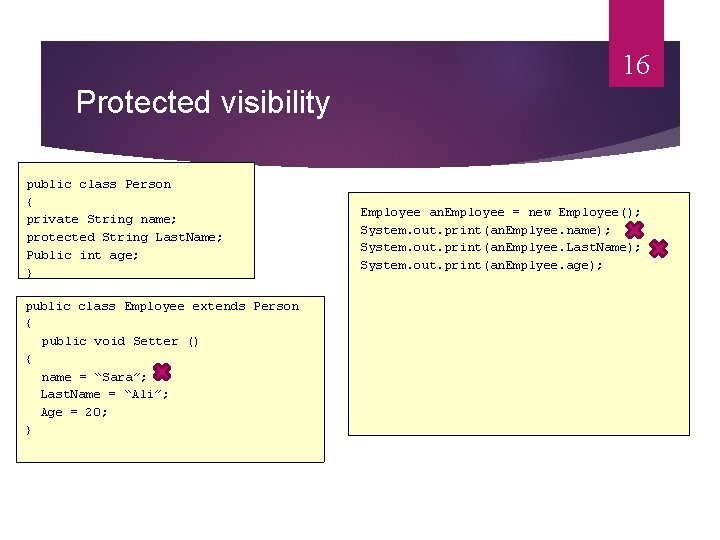
16 Protected visibility public class Person { private String name; protected String Last. Name; Public int age; } public class Employee extends Person { public void Setter () { name = “Sara”; Last. Name = “Ali”; Age = 20; } Employee an. Employee = new Employee(); System. out. print(an. Emplyee. name); System. out. print(an. Emplyee. Last. Name); System. out. print(an. Emplyee. age);
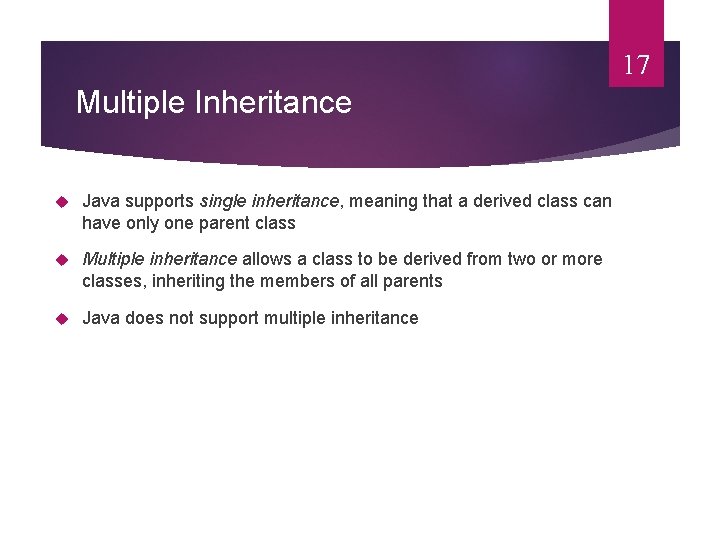
17 Multiple Inheritance Java supports single inheritance, meaning that a derived class can have only one parent class Multiple inheritance allows a class to be derived from two or more classes, inheriting the members of all parents Java does not support multiple inheritance
Inheritance allows
Section 2 complex patterns of inheritance
Chapter 16 the molecular basis of inheritance
Chromosomal theory of inheritance
Chapter 11 complex inheritance and human heredity test
A gene locus is
Chapter 16: the molecular basis of inheritance
Chapter 15 the chromosomal basis of inheritance
The chromosomal basis of inheritance chapter 15
Ap biology chapter 15
Chapter 11 section 1 basic patterns of human inheritance
Chapter 11 section 1 basic patterns of human inheritance
Chapter 13-the molecular basis of inheritance
Chapter 9 patterns of inheritance
Chapter 15 the chromosomal basis of inheritance
The first trial of a controlled experiment allows
Trackball and thumbwheels
Office technology allows you to