IAT 800 Polygons Transformations and Arrays Oct 1
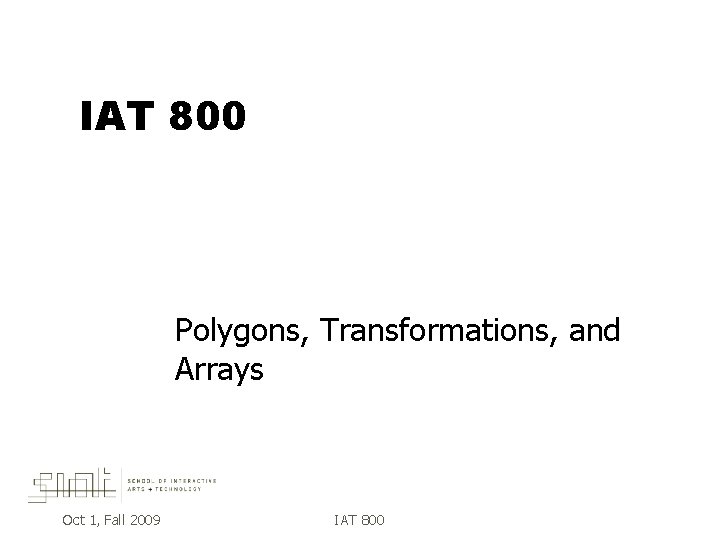
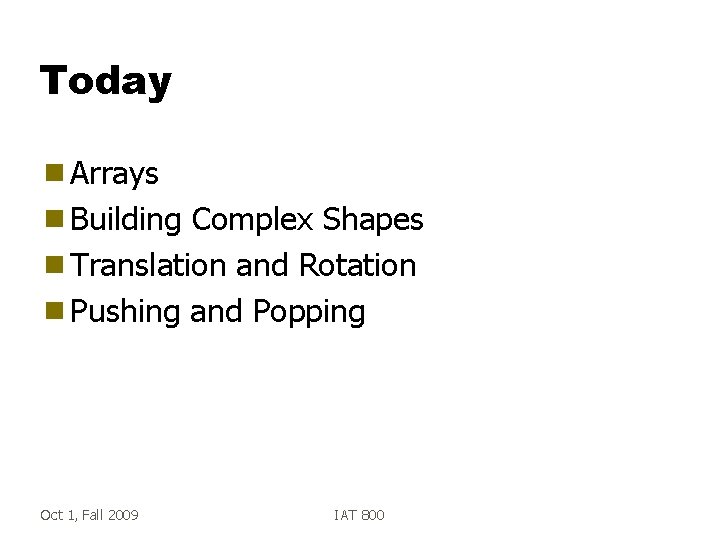
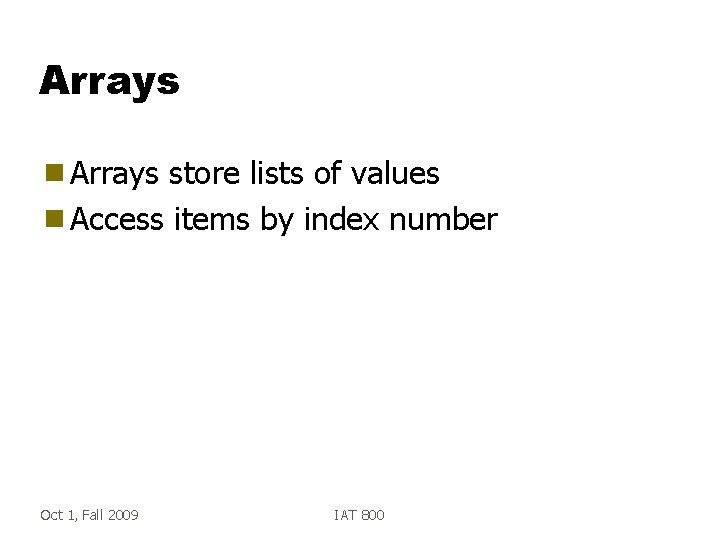
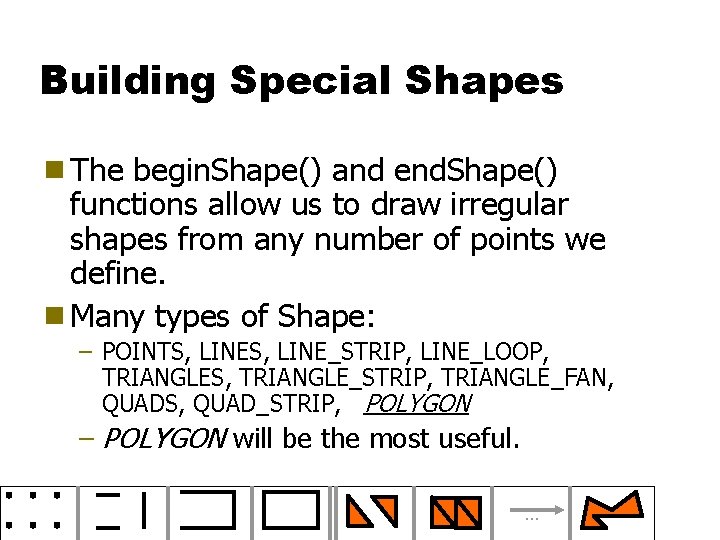
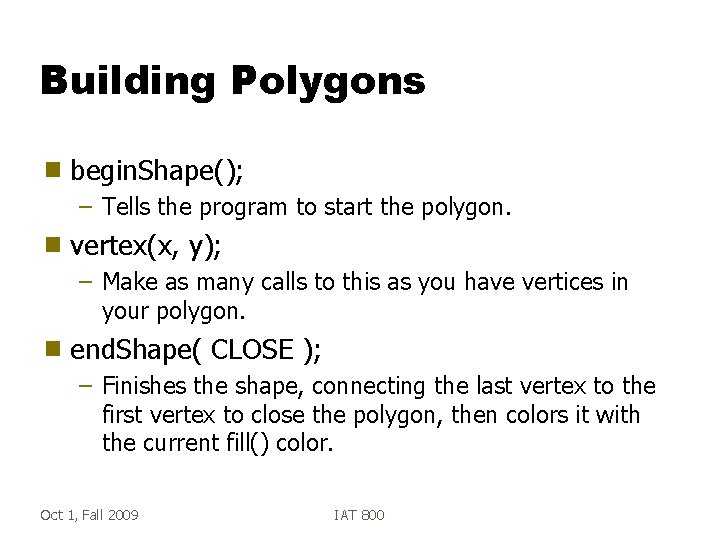
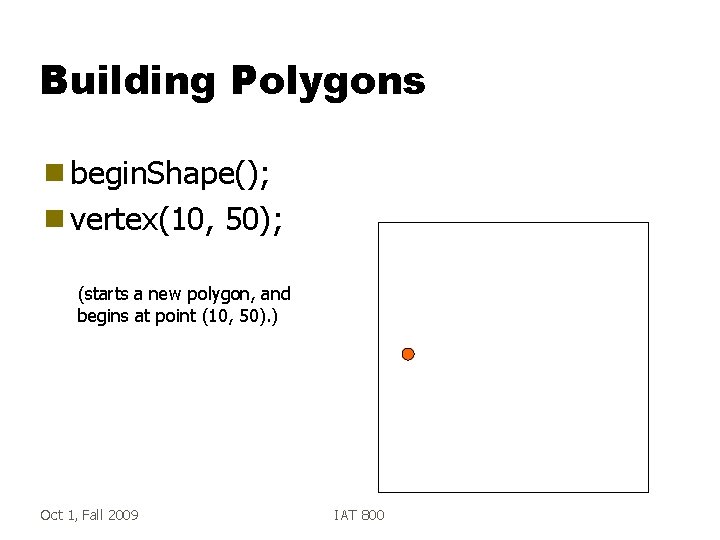
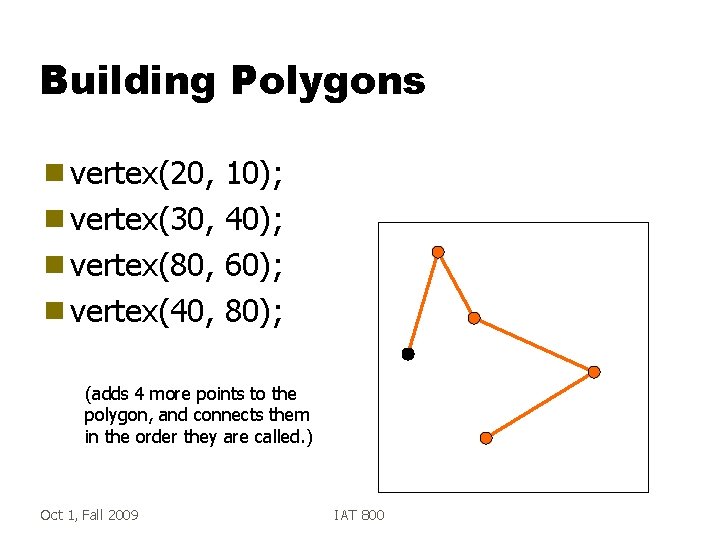
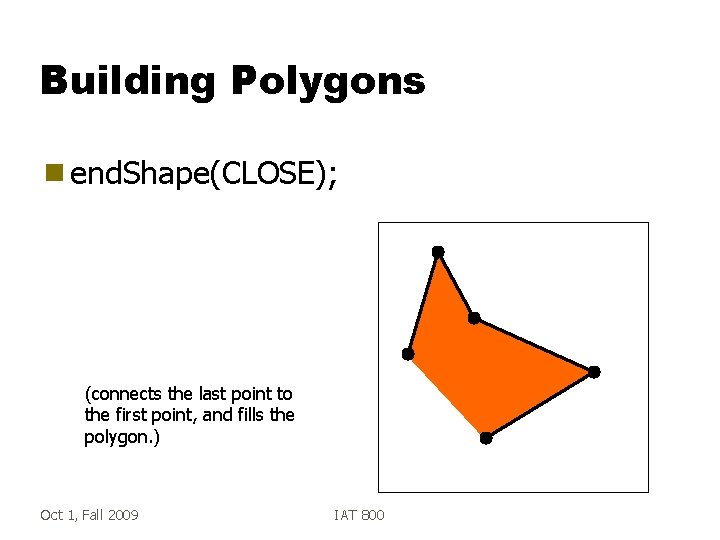
![Let’s Use Arrays g Let’s store the points that we’re drawing. int[] xvals = Let’s Use Arrays g Let’s store the points that we’re drawing. int[] xvals =](https://slidetodoc.com/presentation_image/e81a9af25da7f922c1b58d7c546bc442/image-9.jpg)
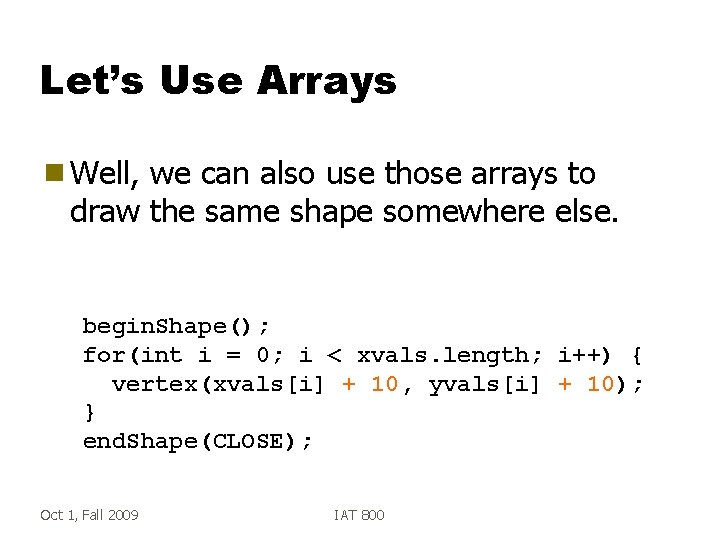
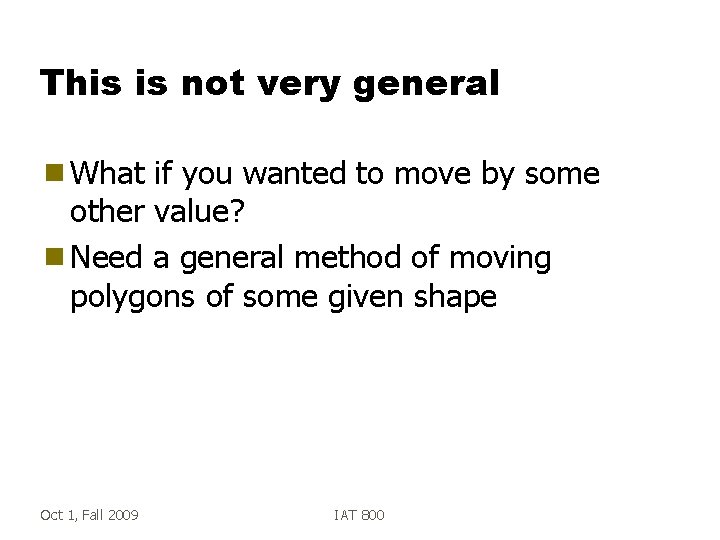
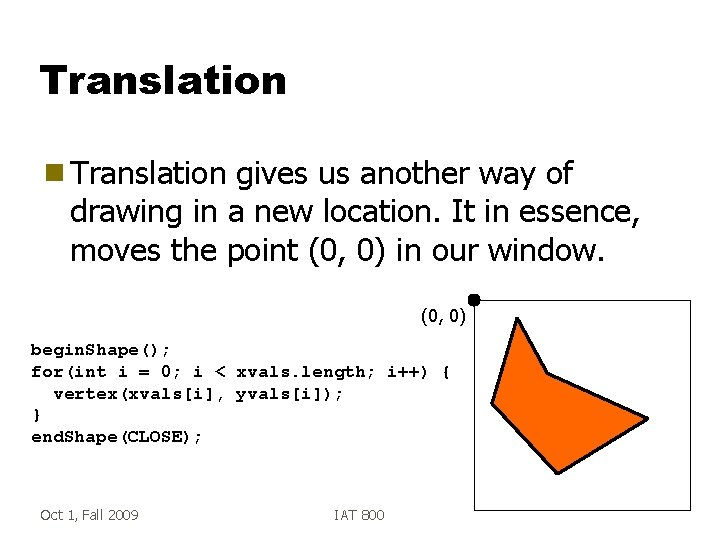
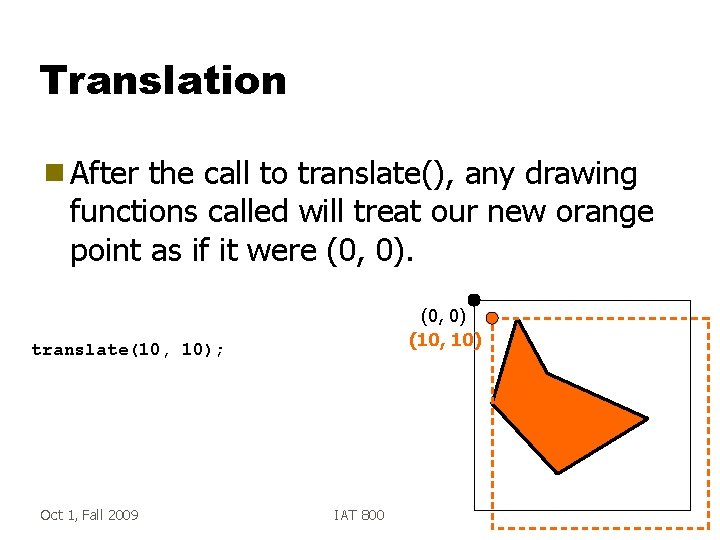
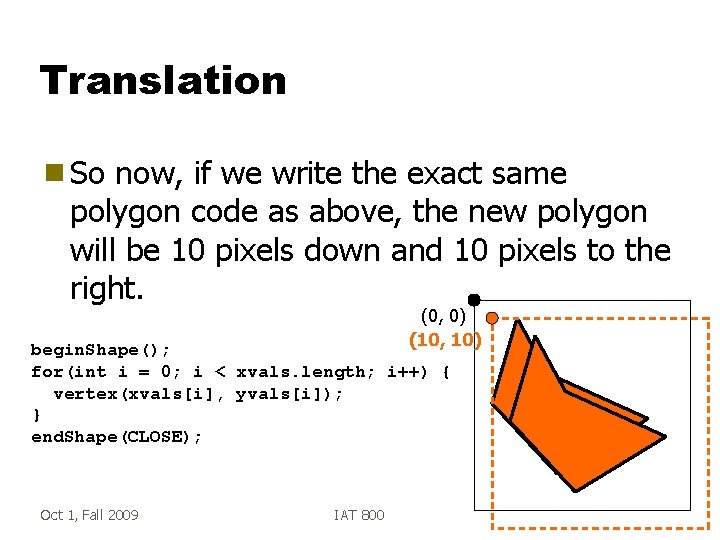
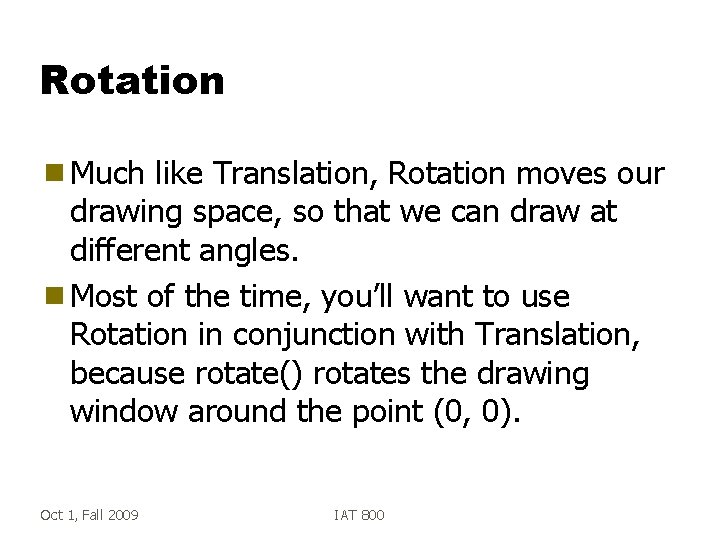
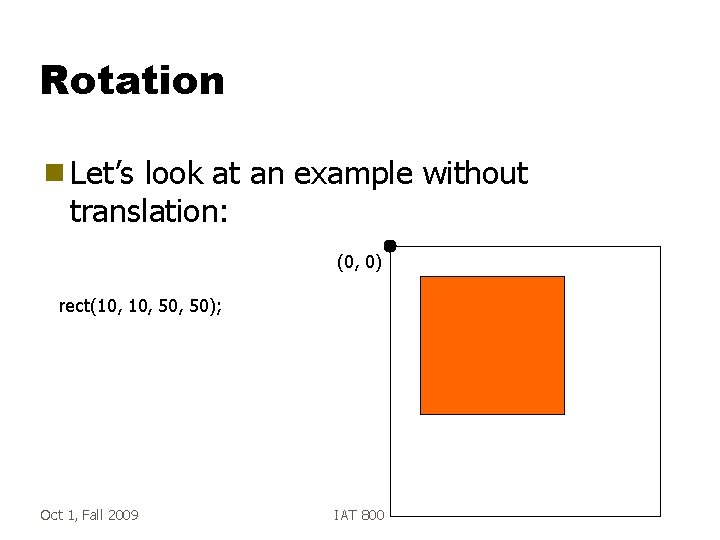
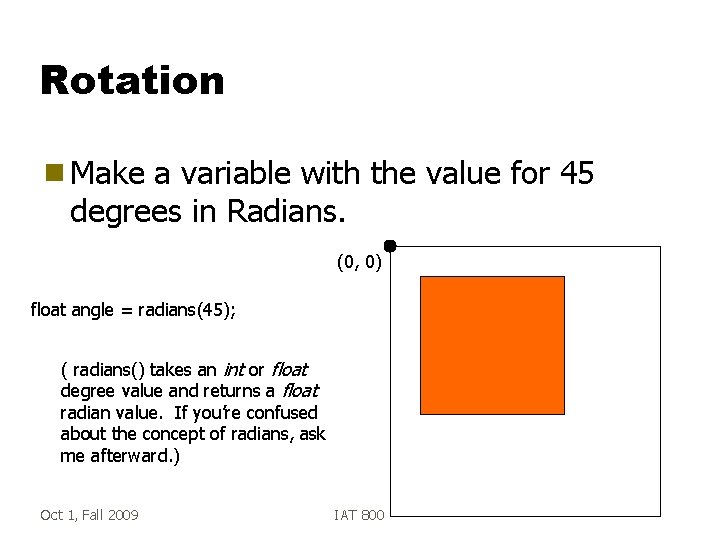
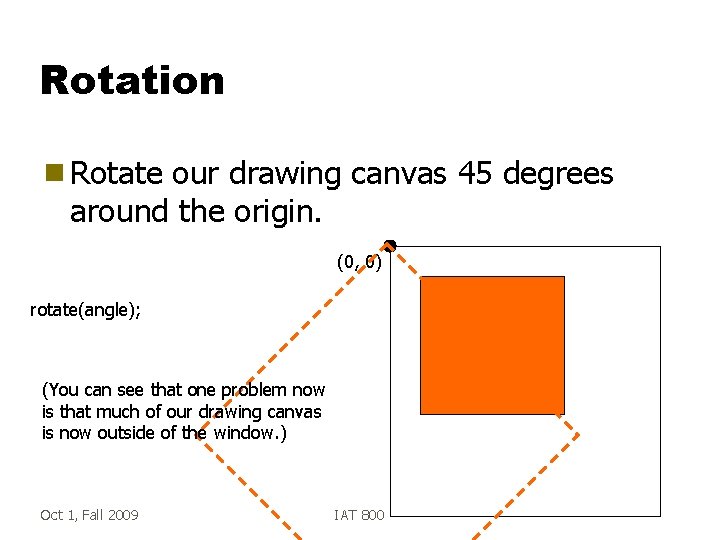
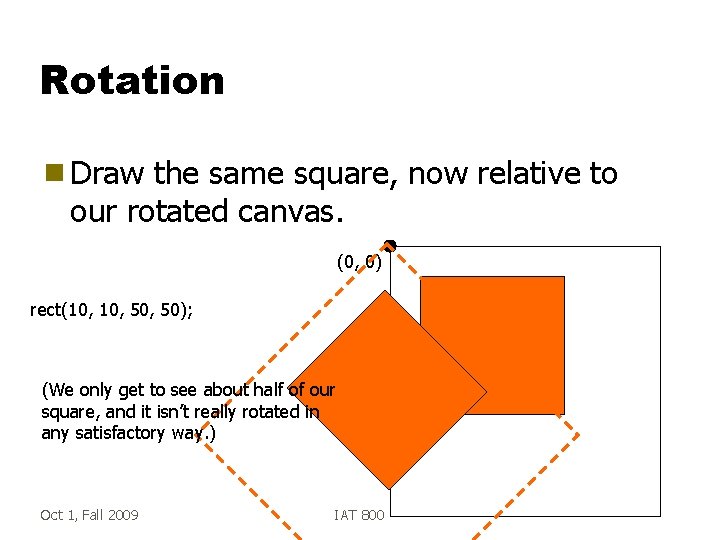
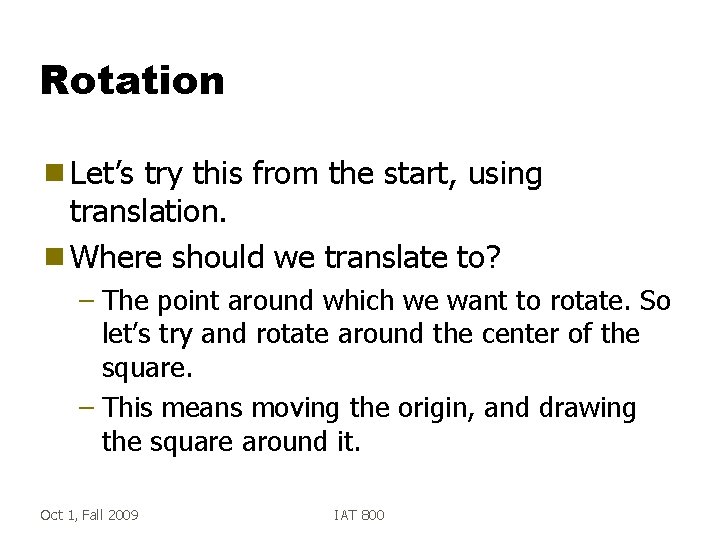
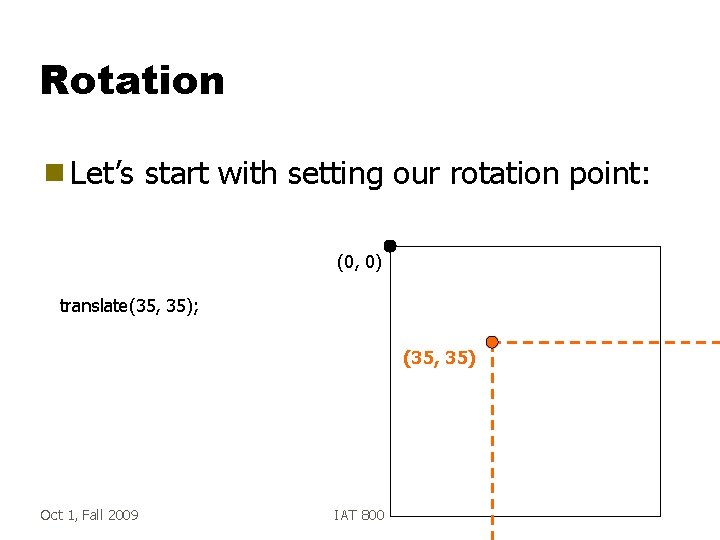
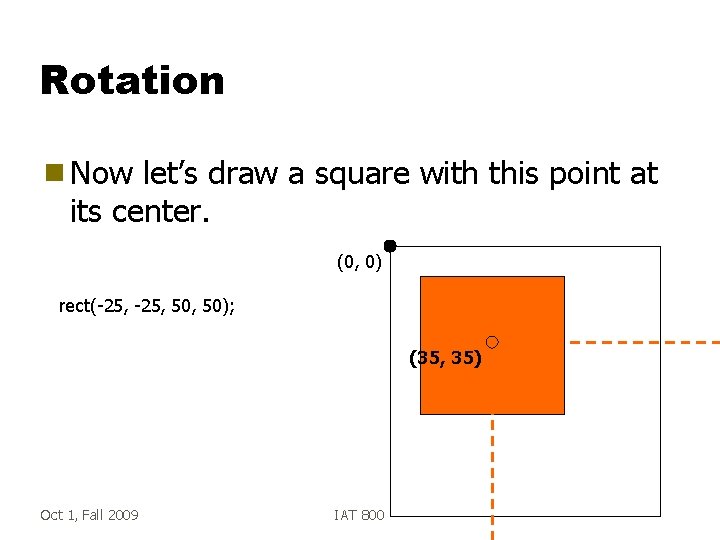
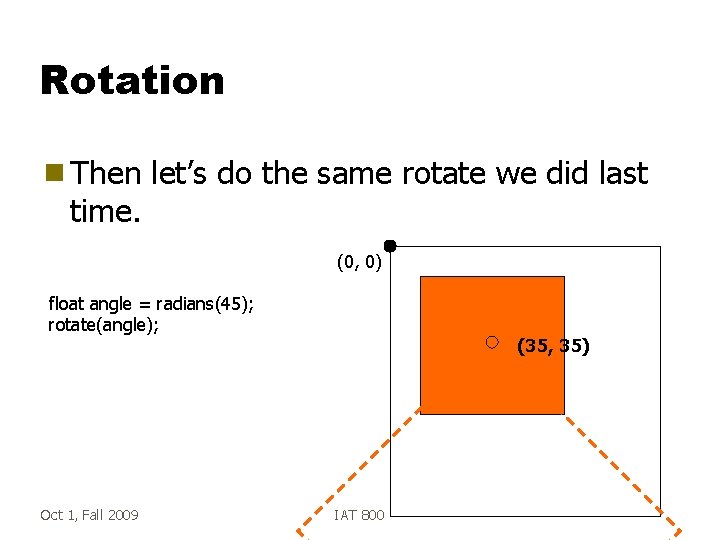
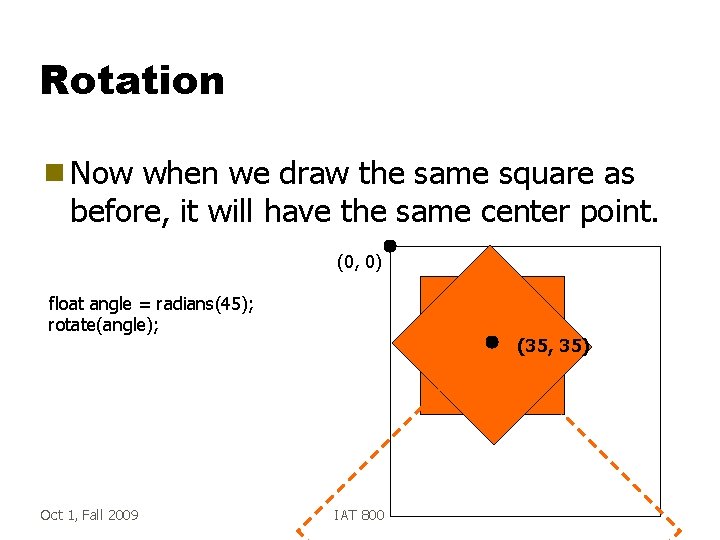
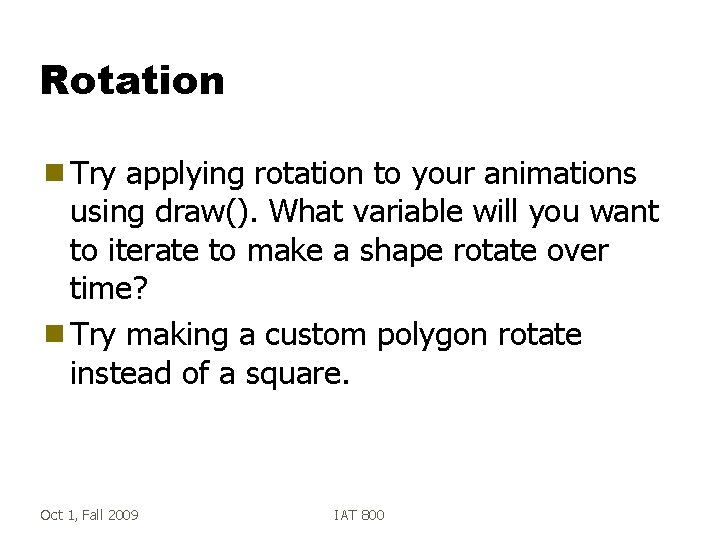
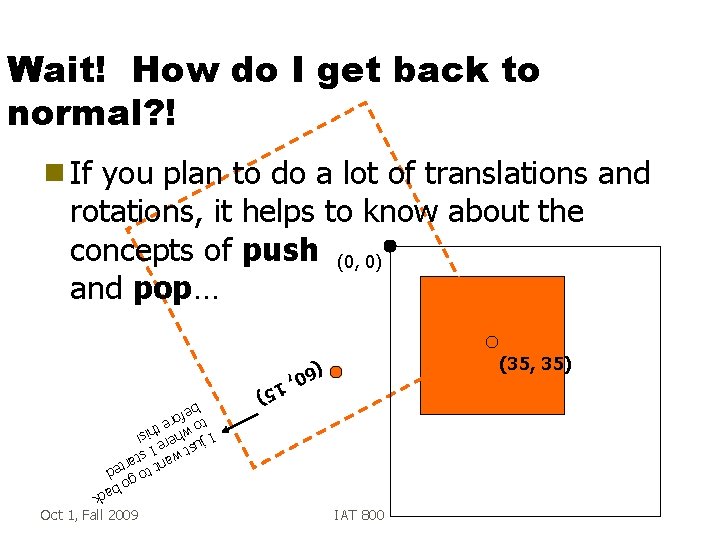
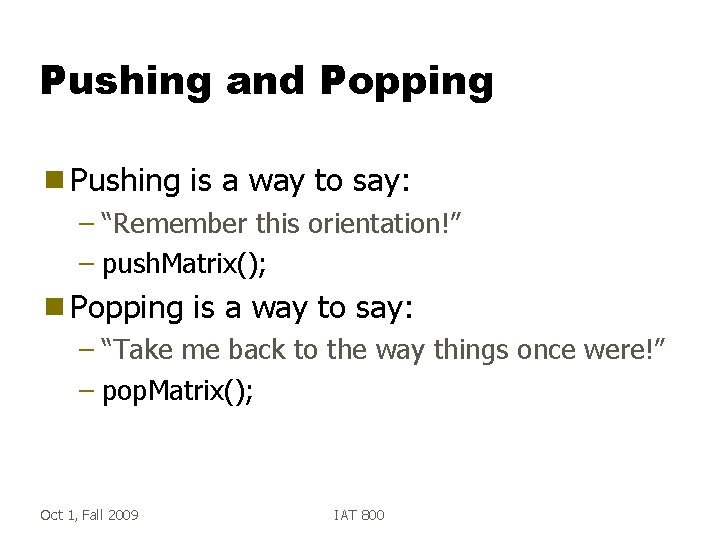
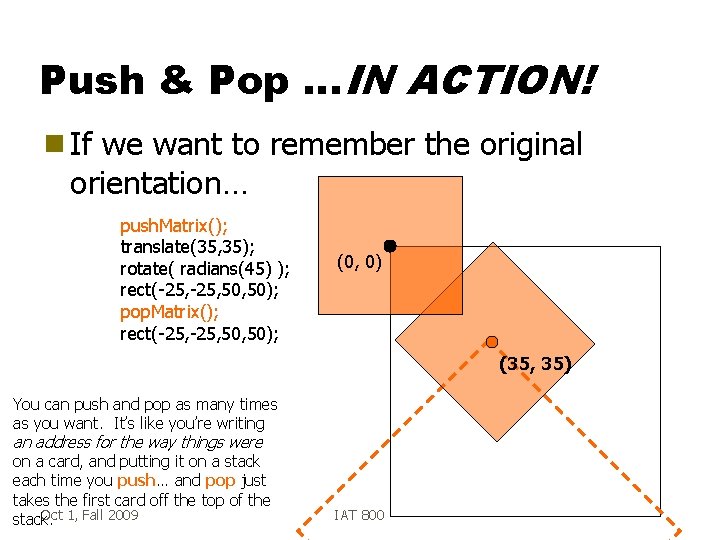
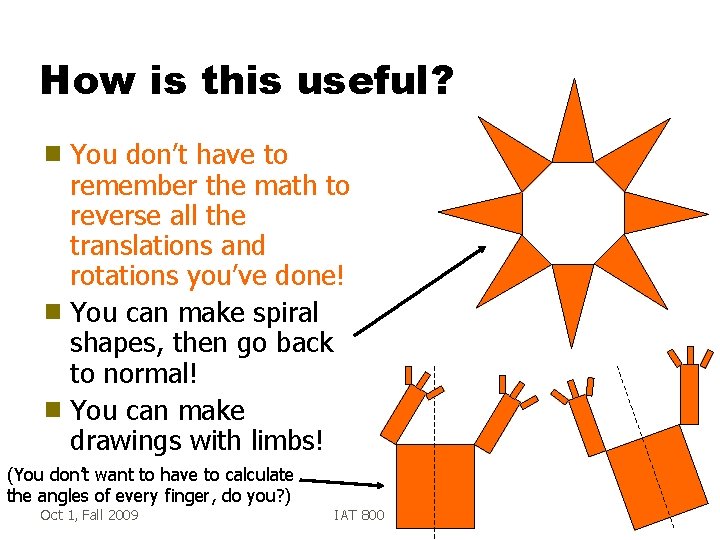
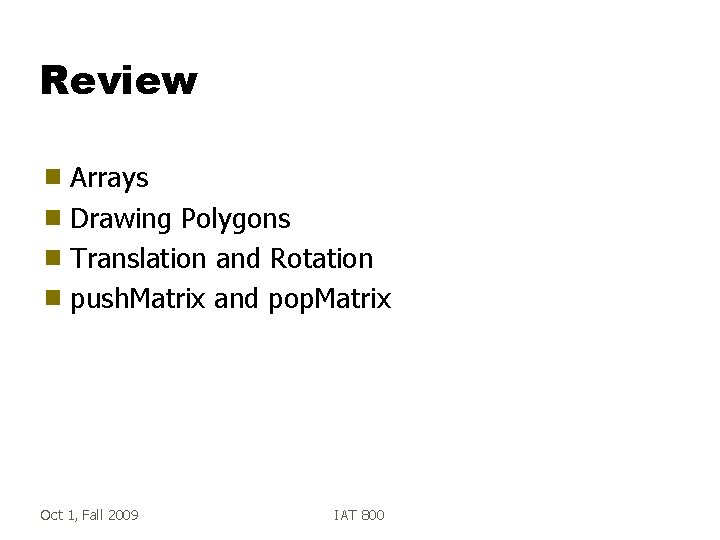
- Slides: 30
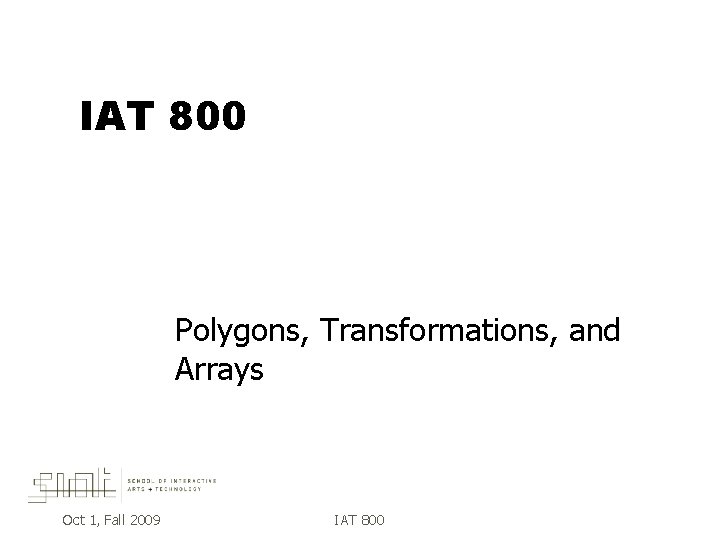
IAT 800 Polygons, Transformations, and Arrays Oct 1, Fall 2009 IAT 800
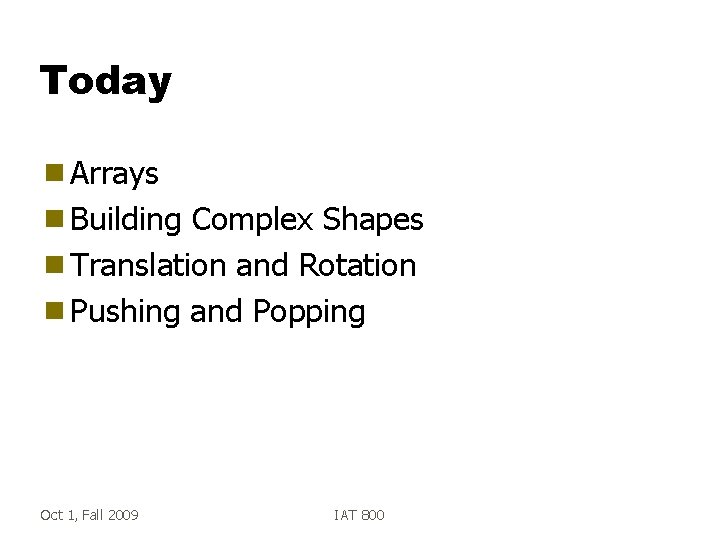
Today g Arrays g Building Complex Shapes g Translation and Rotation g Pushing and Popping Oct 1, Fall 2009 IAT 800
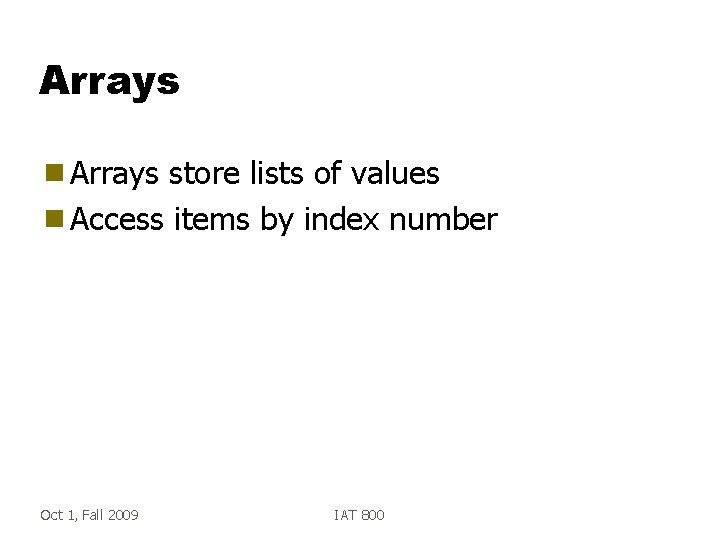
Arrays g Arrays store lists of values g Access items by index number Oct 1, Fall 2009 IAT 800
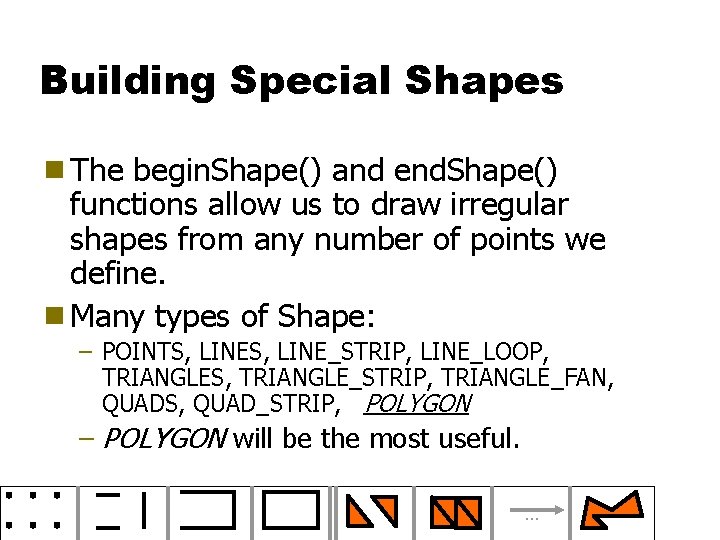
Building Special Shapes g The begin. Shape() and end. Shape() functions allow us to draw irregular shapes from any number of points we define. g Many types of Shape: – POINTS, LINE_STRIP, LINE_LOOP, TRIANGLES, TRIANGLE_STRIP, TRIANGLE_FAN, QUADS, QUAD_STRIP, POLYGON – POLYGON will be the most useful. Oct 1, Fall 2009 IAT 800 …
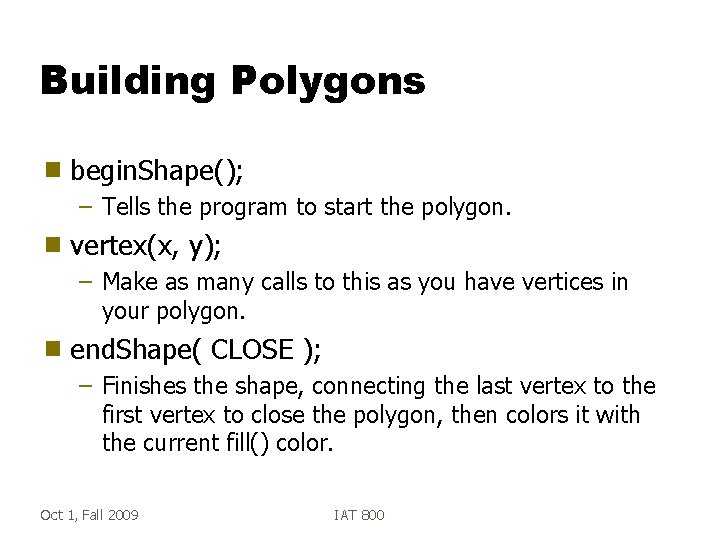
Building Polygons g begin. Shape(); – Tells the program to start the polygon. g vertex(x, y); – Make as many calls to this as you have vertices in your polygon. g end. Shape( CLOSE ); – Finishes the shape, connecting the last vertex to the first vertex to close the polygon, then colors it with the current fill() color. Oct 1, Fall 2009 IAT 800
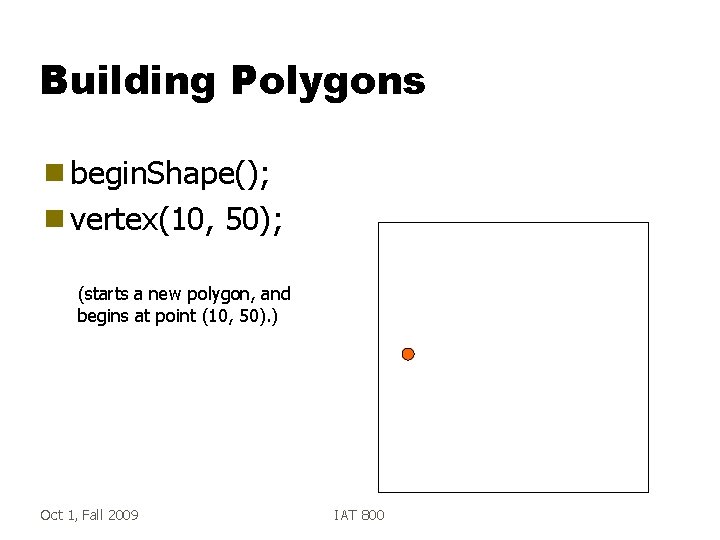
Building Polygons g begin. Shape(); g vertex(10, 50); (starts a new polygon, and begins at point (10, 50). ) Oct 1, Fall 2009 IAT 800
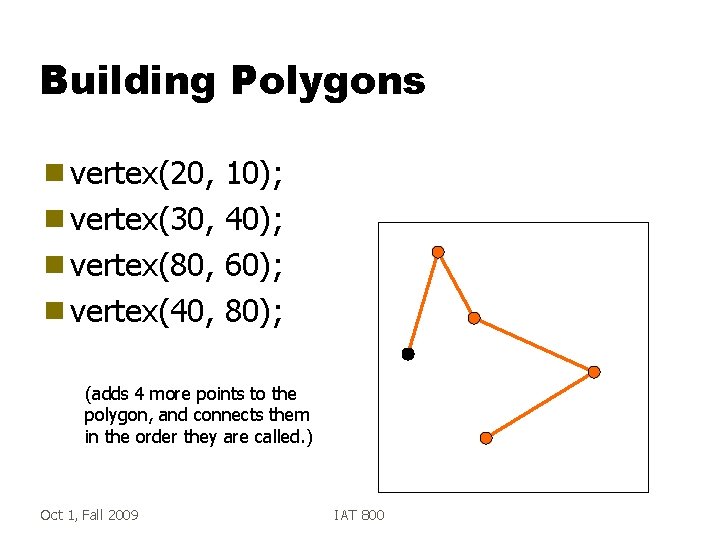
Building Polygons g vertex(20, 10); g vertex(30, 40); g vertex(80, 60); g vertex(40, 80); (adds 4 more points to the polygon, and connects them in the order they are called. ) Oct 1, Fall 2009 IAT 800
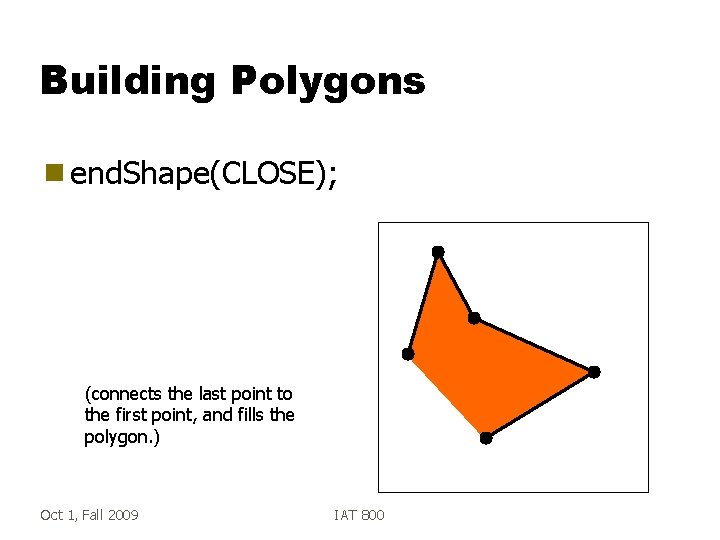
Building Polygons g end. Shape(CLOSE); (connects the last point to the first point, and fills the polygon. ) Oct 1, Fall 2009 IAT 800
![Lets Use Arrays g Lets store the points that were drawing int xvals Let’s Use Arrays g Let’s store the points that we’re drawing. int[] xvals =](https://slidetodoc.com/presentation_image/e81a9af25da7f922c1b58d7c546bc442/image-9.jpg)
Let’s Use Arrays g Let’s store the points that we’re drawing. int[] xvals = {10, 20, 30, 80, 40}; int[] yvals = {50, 10, 40, 60, 80}; begin. Shape(); for(int i = 0; i < xvals. length; i++) { vertex(xvals[i], yvals[i]); } end. Shape(CLOSE); Oct 1, Fall 2009 IAT 800
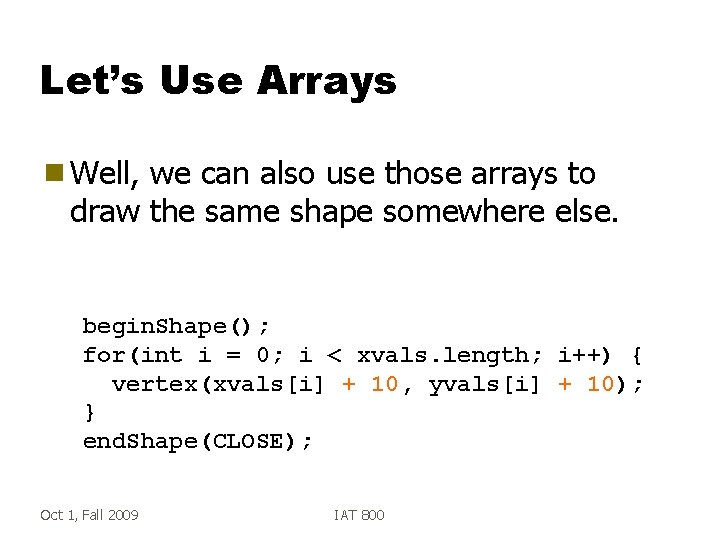
Let’s Use Arrays g Well, we can also use those arrays to draw the same shape somewhere else. begin. Shape(); for(int i = 0; i < xvals. length; i++) { vertex(xvals[i] + 10, yvals[i] + 10); } end. Shape(CLOSE); Oct 1, Fall 2009 IAT 800
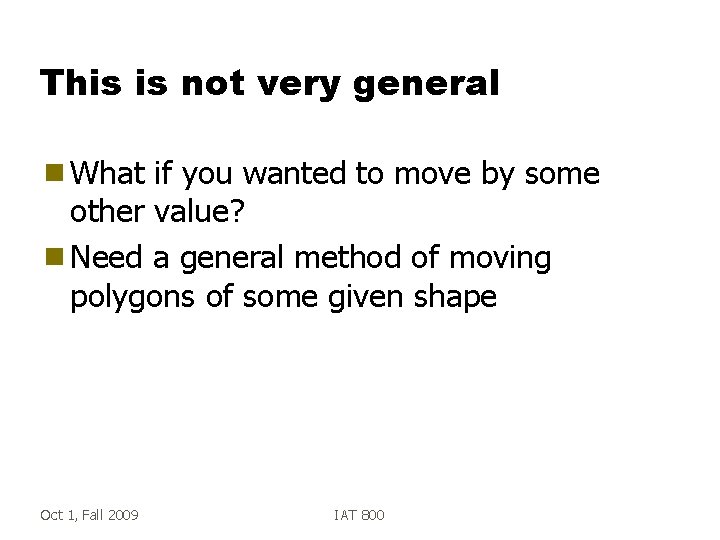
This is not very general g What if you wanted to move by some other value? g Need a general method of moving polygons of some given shape Oct 1, Fall 2009 IAT 800
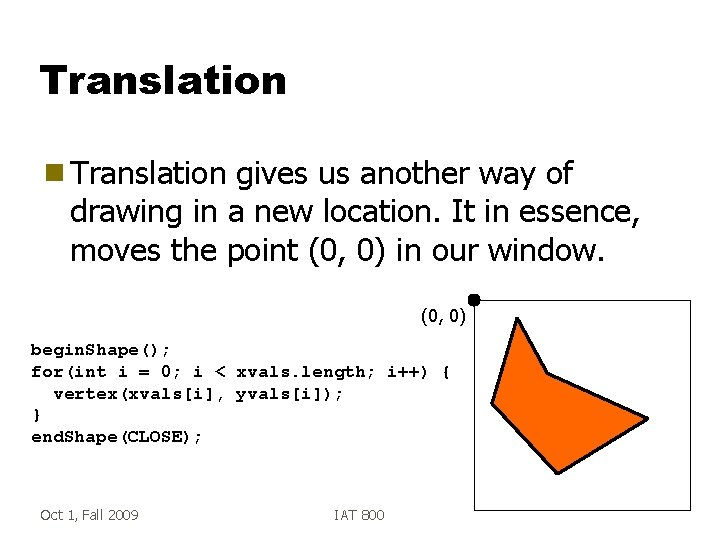
Translation gives us another way of drawing in a new location. It in essence, moves the point (0, 0) in our window. (0, 0) begin. Shape(); for(int i = 0; i < xvals. length; i++) { vertex(xvals[i], yvals[i]); } end. Shape(CLOSE); Oct 1, Fall 2009 IAT 800
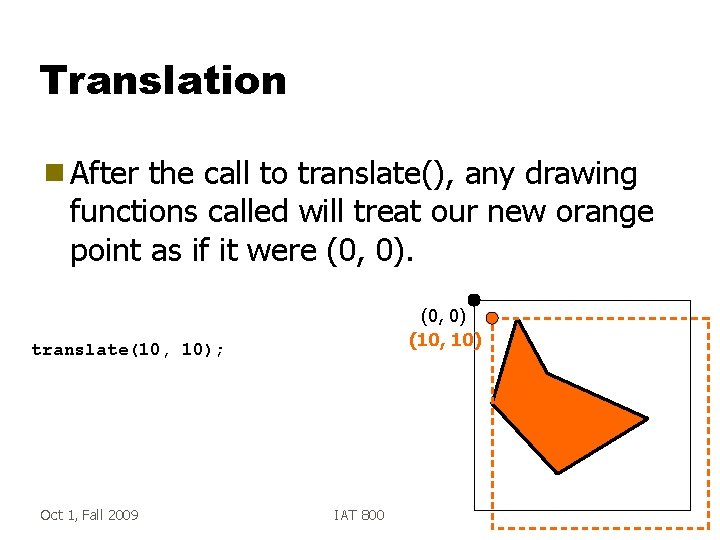
Translation g After the call to translate(), any drawing functions called will treat our new orange point as if it were (0, 0) (10, 10) translate(10, 10); Oct 1, Fall 2009 IAT 800
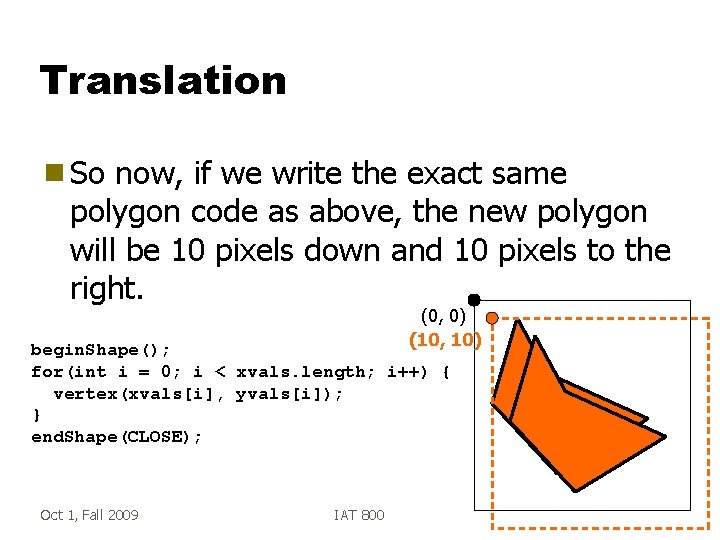
Translation g So now, if we write the exact same polygon code as above, the new polygon will be 10 pixels down and 10 pixels to the right. (0, 0) (10, 10) begin. Shape(); for(int i = 0; i < xvals. length; i++) { vertex(xvals[i], yvals[i]); } end. Shape(CLOSE); Oct 1, Fall 2009 IAT 800
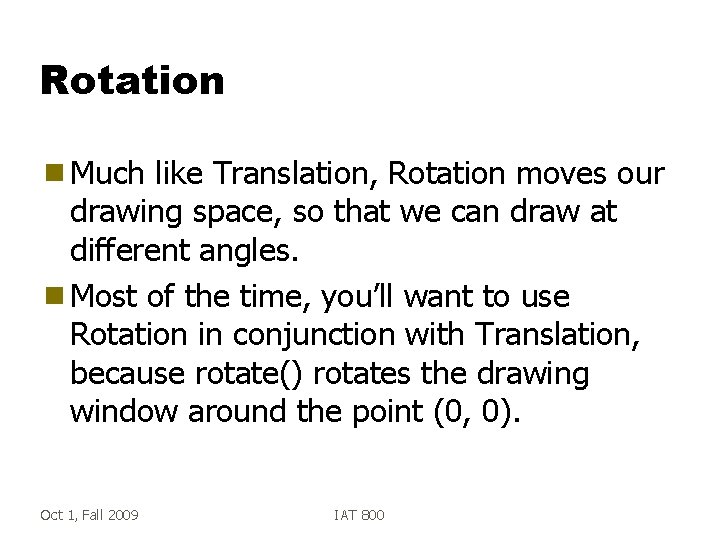
Rotation g Much like Translation, Rotation moves our drawing space, so that we can draw at different angles. g Most of the time, you’ll want to use Rotation in conjunction with Translation, because rotate() rotates the drawing window around the point (0, 0). Oct 1, Fall 2009 IAT 800
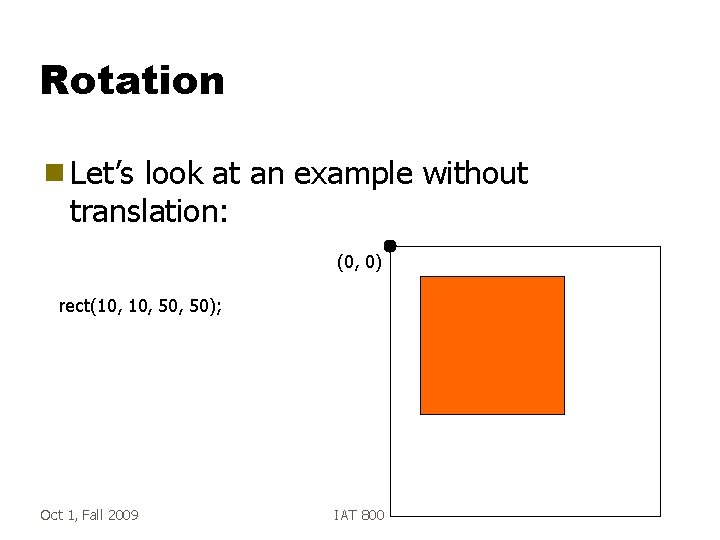
Rotation g Let’s look at an example without translation: (0, 0) rect(10, 50, 50); Oct 1, Fall 2009 IAT 800
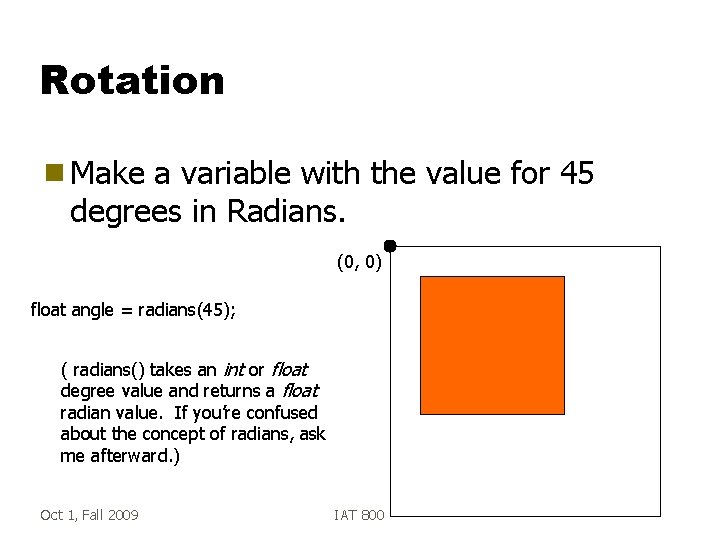
Rotation g Make a variable with the value for 45 degrees in Radians. (0, 0) float angle = radians(45); ( radians() takes an int or float degree value and returns a float radian value. If you’re confused about the concept of radians, ask me afterward. ) Oct 1, Fall 2009 IAT 800
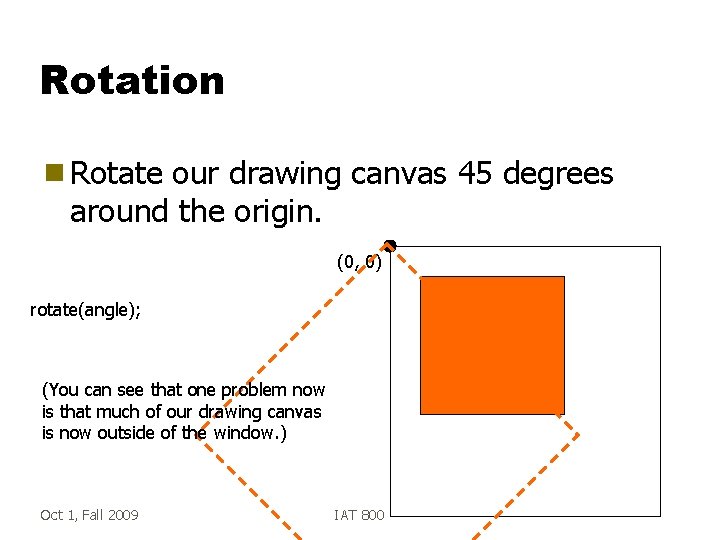
Rotation g Rotate our drawing canvas 45 degrees around the origin. (0, 0) rotate(angle); (You can see that one problem now is that much of our drawing canvas is now outside of the window. ) Oct 1, Fall 2009 IAT 800
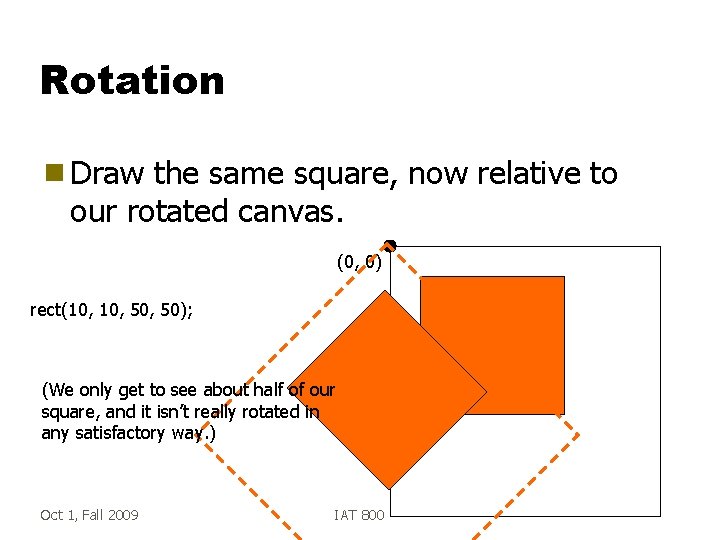
Rotation g Draw the same square, now relative to our rotated canvas. (0, 0) rect(10, 50, 50); (We only get to see about half of our square, and it isn’t really rotated in any satisfactory way. ) Oct 1, Fall 2009 IAT 800
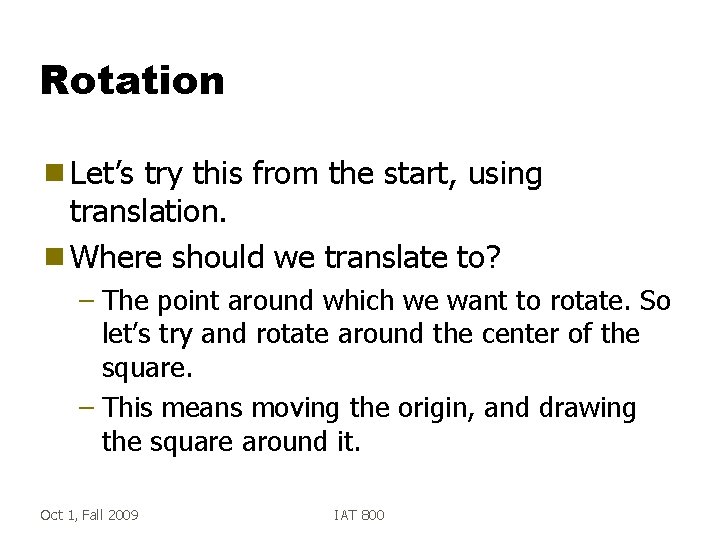
Rotation g Let’s try this from the start, using translation. g Where should we translate to? – The point around which we want to rotate. So let’s try and rotate around the center of the square. – This means moving the origin, and drawing the square around it. Oct 1, Fall 2009 IAT 800
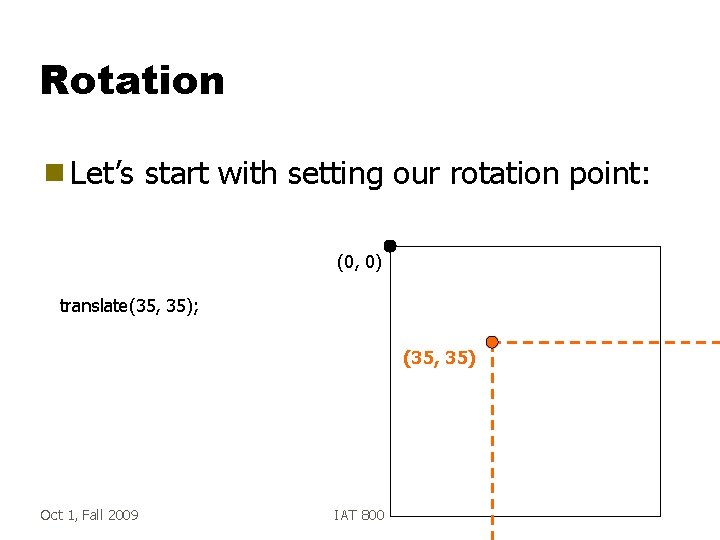
Rotation g Let’s start with setting our rotation point: (0, 0) translate(35, 35); (35, 35) Oct 1, Fall 2009 IAT 800
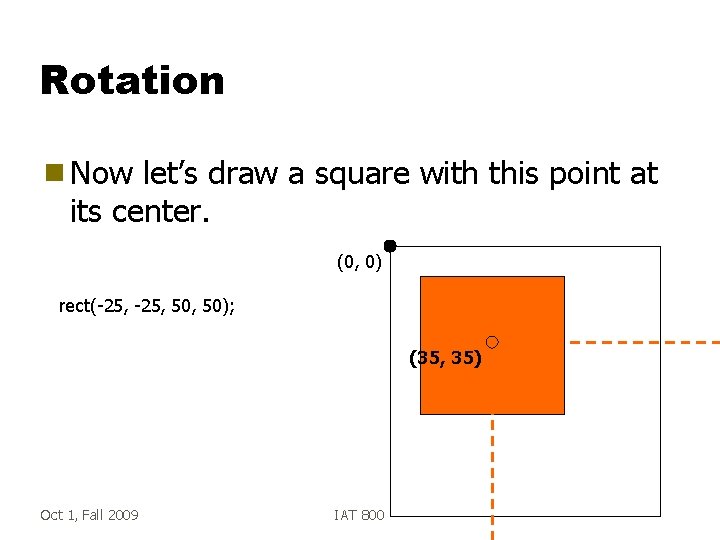
Rotation g Now let’s draw a square with this point at its center. (0, 0) rect(-25, 50, 50); (35, 35) Oct 1, Fall 2009 IAT 800
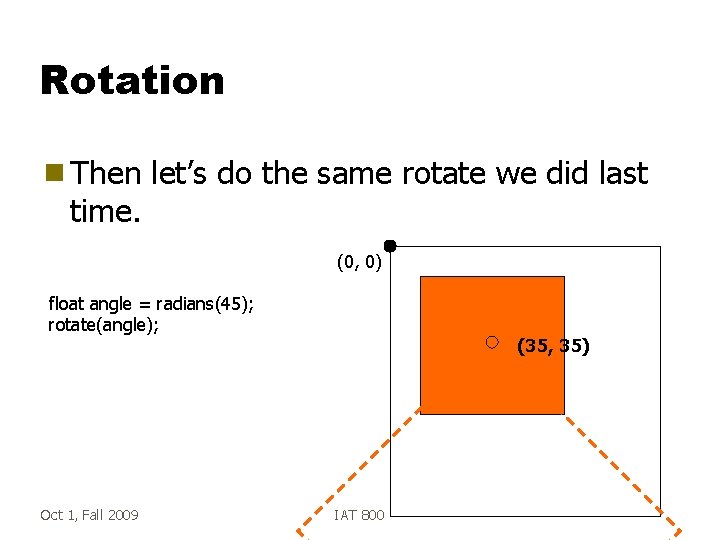
Rotation g Then time. let’s do the same rotate we did last (0, 0) float angle = radians(45); rotate(angle); Oct 1, Fall 2009 (35, 35) IAT 800
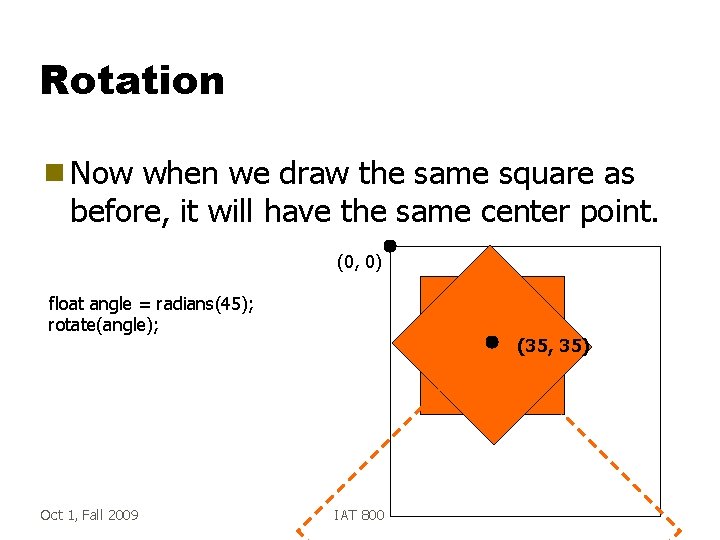
Rotation g Now when we draw the same square as before, it will have the same center point. (0, 0) float angle = radians(45); rotate(angle); Oct 1, Fall 2009 (35, 35) IAT 800
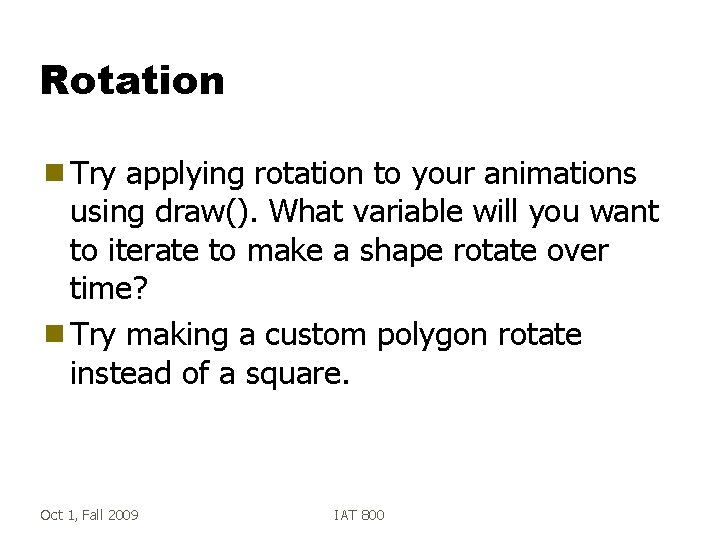
Rotation g Try applying rotation to your animations using draw(). What variable will you want to iterate to make a shape rotate over time? g Try making a custom polygon rotate instead of a square. Oct 1, Fall 2009 IAT 800
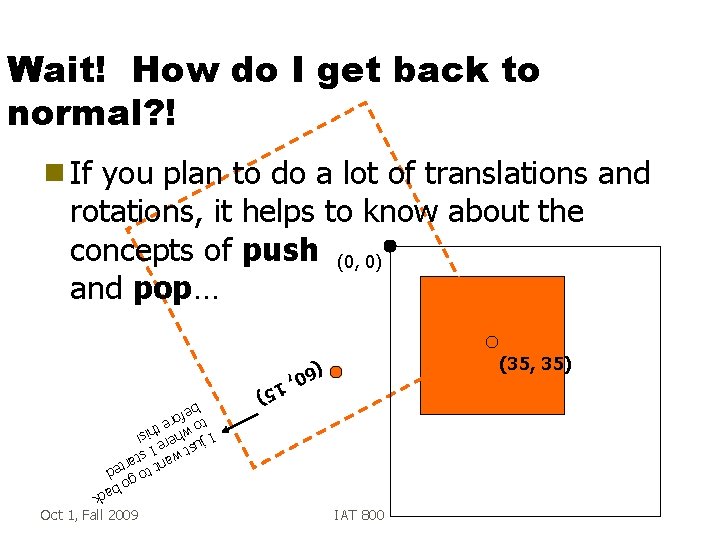
Wait! How do I get back to normal? ! g If you plan to do a lot of translations and rotations, it helps to know about the concepts of push (0, 0) and pop… (6 0, 15 ) ck ba go to ted r nt wa I sta st e I ju her his! w to ore t f be Oct 1, Fall 2009 (35, 35) IAT 800
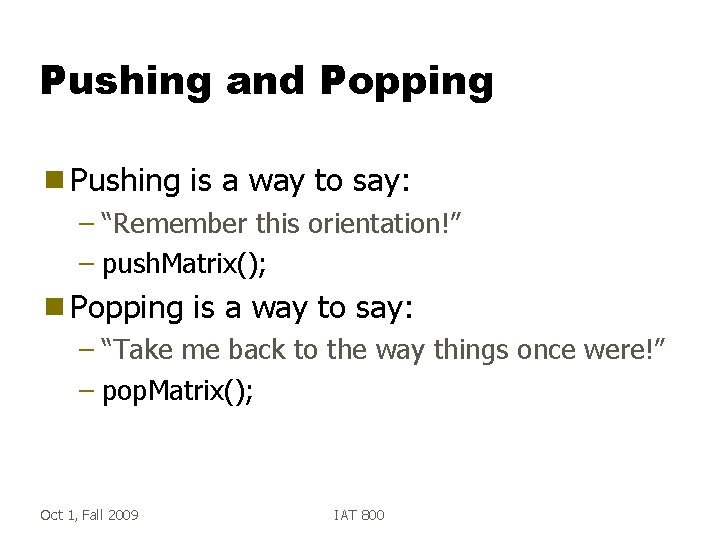
Pushing and Popping g Pushing is a way to say: – “Remember this orientation!” – push. Matrix(); g Popping is a way to say: – “Take me back to the way things once were!” – pop. Matrix(); Oct 1, Fall 2009 IAT 800
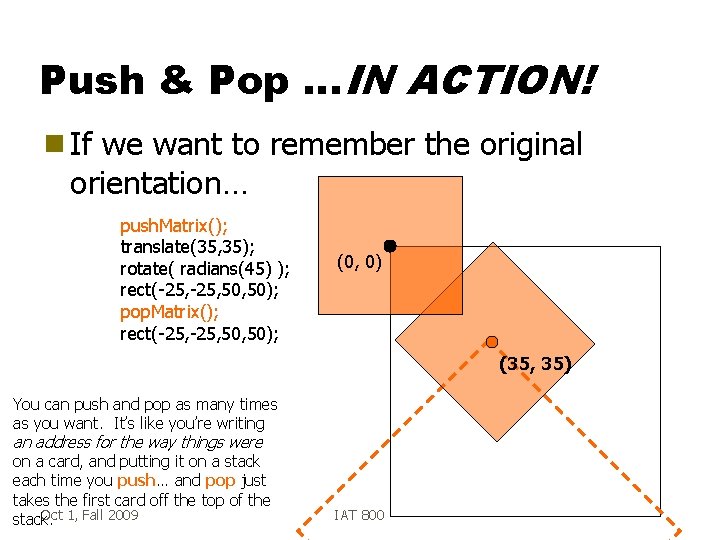
Push & Pop …IN ACTION! g If we want to remember the original orientation… push. Matrix(); translate(35, 35); rotate( radians(45) ); rect(-25, 50, 50); pop. Matrix(); rect(-25, 50, 50); (0, 0) (35, 35) You can push and pop as many times as you want. It’s like you’re writing an address for the way things were on a card, and putting it on a stack each time you push… and pop just takes the first card off the top of the Oct 1, Fall 2009 stack. IAT 800
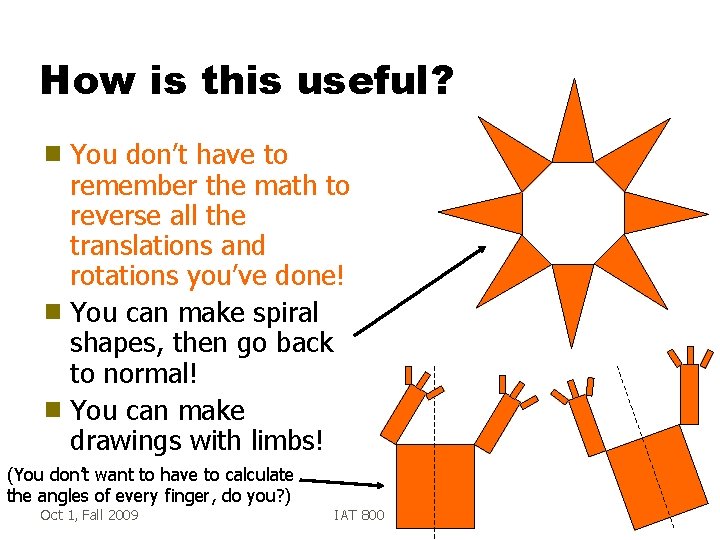
How is this useful? You don’t have to remember the math to reverse all the translations and rotations you’ve done! g You can make spiral shapes, then go back to normal! g You can make drawings with limbs! g (You don’t want to have to calculate the angles of every finger, do you? ) Oct 1, Fall 2009 IAT 800
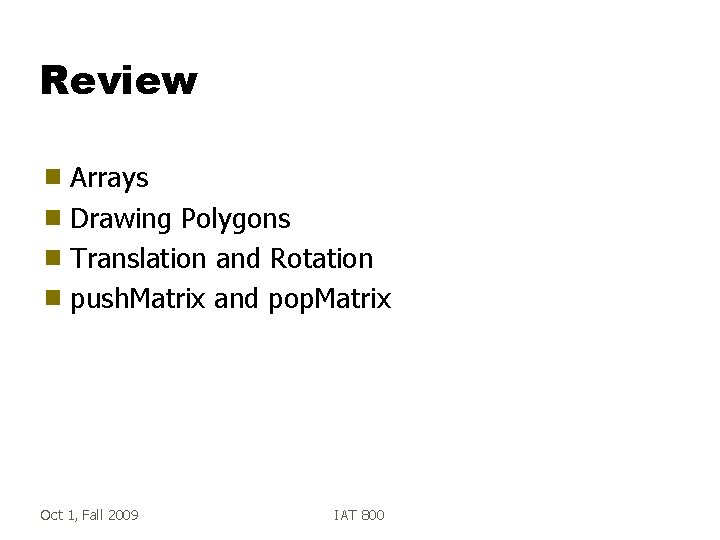
Review Arrays g Drawing Polygons g Translation and Rotation g push. Matrix and pop. Matrix g Oct 1, Fall 2009 IAT 800
What makes shapes similar
800+200+400
Dynamic arrays and amortized analysis
Searching and sorting arrays in c++
Advantages and disadvantages of array over linked list
Parallel arrays
Array of arrays c++
Ragged array
潘仁義
Parallel arrays
Why do we need arrays?
Arrays unidimensionales en java
Arreglos bidimensionales java
Arrays mips
Polynomial representation using array in c
Assembly array of strings
Global arrays in c
Computer science arrays
Arrays visual basic
Python find index of max
How many arrays in 24
Arrays in pascal examples
Mips dynamic array
Creating arrays matlab
Adt of array
Partially filled array java
Redundant arrays of independent disks
Python list of arrays
Arrays
Day 3: arrays
Raid redundant array of inexpensive disks