Generic SearchingSorting n n n Generic search and
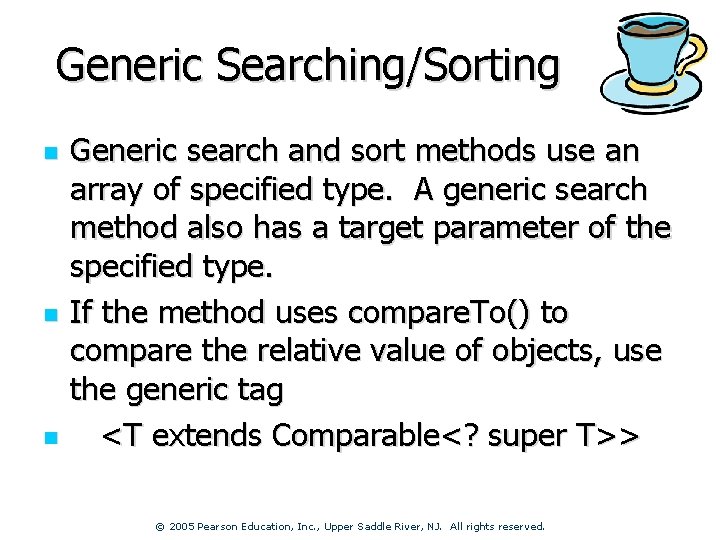
![Generic Selection Sort public static <T extends Comparable<? super T>> void selection. Sort(T[] arr) Generic Selection Sort public static <T extends Comparable<? super T>> void selection. Sort(T[] arr)](https://slidetodoc.com/presentation_image_h2/4a7728ac8d77876ddc2abe98a0652acc/image-2.jpg)
![Generic Selection Sort (concluded) // j traverses from arr[pass+1] to arr[n-1] for (j = Generic Selection Sort (concluded) // j traverses from arr[pass+1] to arr[n-1] for (j =](https://slidetodoc.com/presentation_image_h2/4a7728ac8d77876ddc2abe98a0652acc/image-3.jpg)
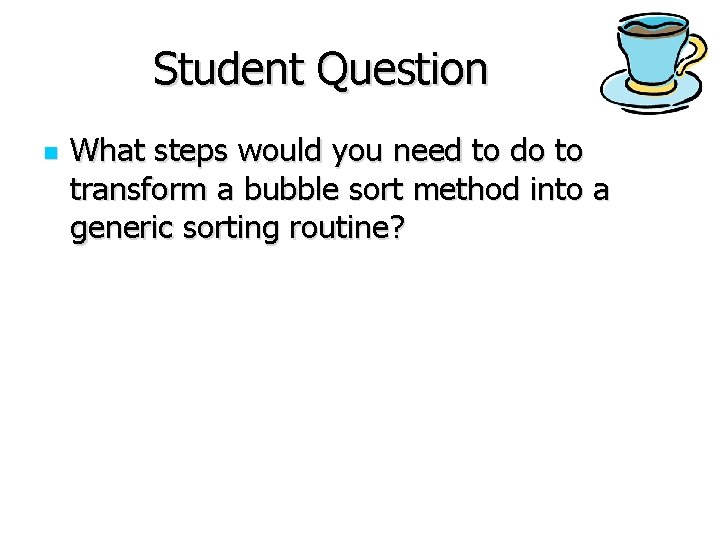
![Generic bin. Search() public static <T extends Comparable<? super T>> int bin. Search(T[] arr, Generic bin. Search() public static <T extends Comparable<? super T>> int bin. Search(T[] arr,](https://slidetodoc.com/presentation_image_h2/4a7728ac8d77876ddc2abe98a0652acc/image-5.jpg)
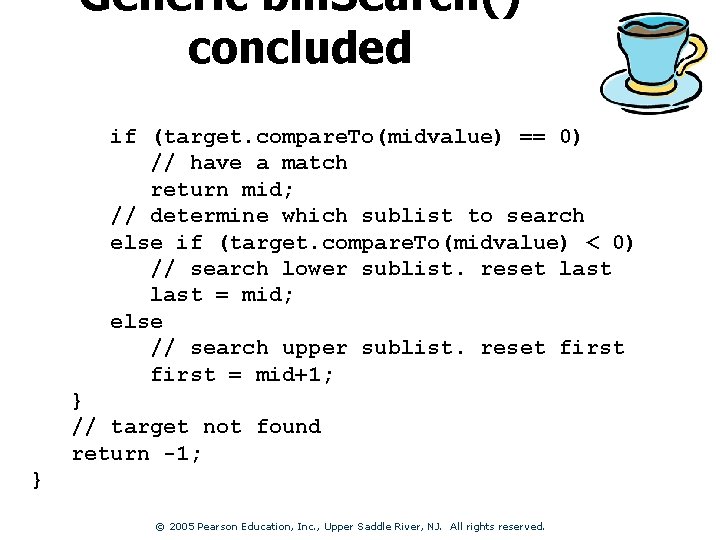
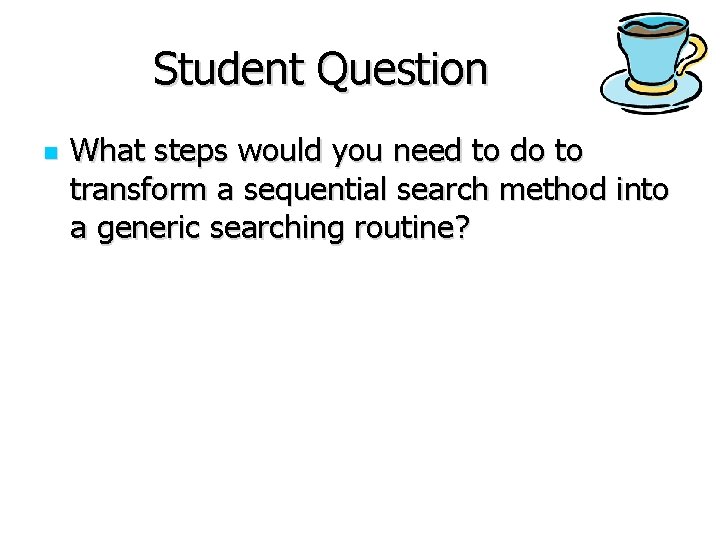
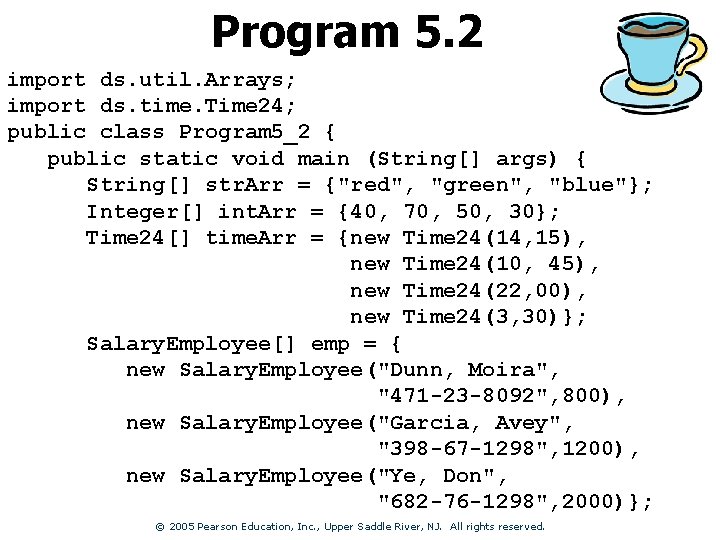
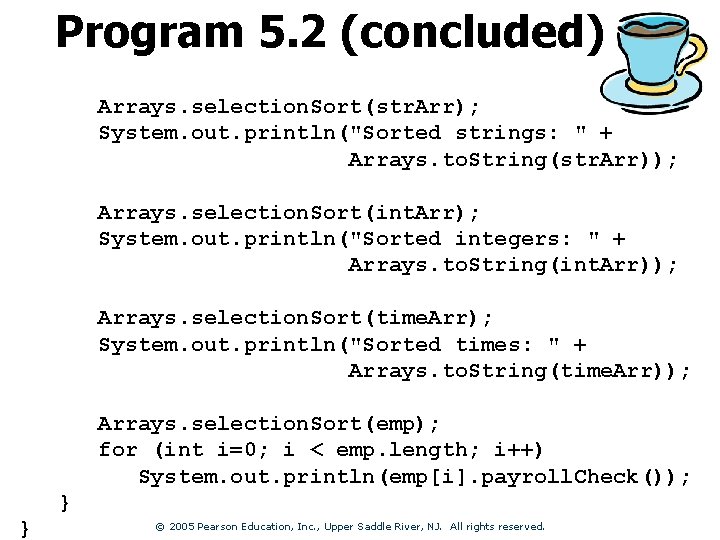
![Program 5. 2 Run: Sorted strings: [blue, green, red] Sorted integers: [30, 40, 50, Program 5. 2 Run: Sorted strings: [blue, green, red] Sorted integers: [30, 40, 50,](https://slidetodoc.com/presentation_image_h2/4a7728ac8d77876ddc2abe98a0652acc/image-10.jpg)
- Slides: 10
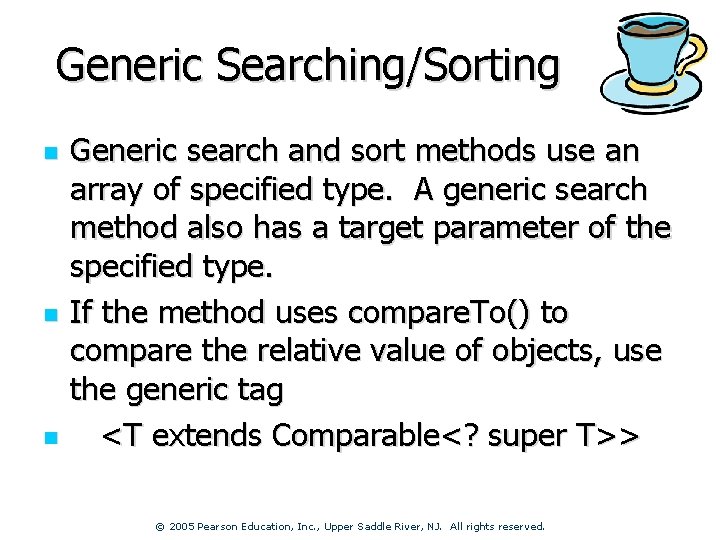
Generic Searching/Sorting n n n Generic search and sort methods use an array of specified type. A generic search method also has a target parameter of the specified type. If the method uses compare. To() to compare the relative value of objects, use the generic tag <T extends Comparable<? super T>> © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
![Generic Selection Sort public static T extends Comparable super T void selection SortT arr Generic Selection Sort public static <T extends Comparable<? super T>> void selection. Sort(T[] arr)](https://slidetodoc.com/presentation_image_h2/4a7728ac8d77876ddc2abe98a0652acc/image-2.jpg)
Generic Selection Sort public static <T extends Comparable<? super T>> void selection. Sort(T[] arr) { // index of smallest element in the sublist int small. Index; int pass, j, n = arr. length; T temp; // pass has the range 0 to n-2 for (pass = 0; pass < n-1; pass++) { // scan the sublist starting at index pass small. Index = pass; © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
![Generic Selection Sort concluded j traverses from arrpass1 to arrn1 for j Generic Selection Sort (concluded) // j traverses from arr[pass+1] to arr[n-1] for (j =](https://slidetodoc.com/presentation_image_h2/4a7728ac8d77876ddc2abe98a0652acc/image-3.jpg)
Generic Selection Sort (concluded) // j traverses from arr[pass+1] to arr[n-1] for (j = pass+1; j < n; j++) // if smaller element found, assign small. Index // to that position if (arr[j]. compare. To(arr[small. Index]) < 0) small. Index = j; // swap the next smallest element and arr[pass] temp = arr[pass]; arr[pass] = arr[small. Index]; arr[small. Index] = temp; } } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
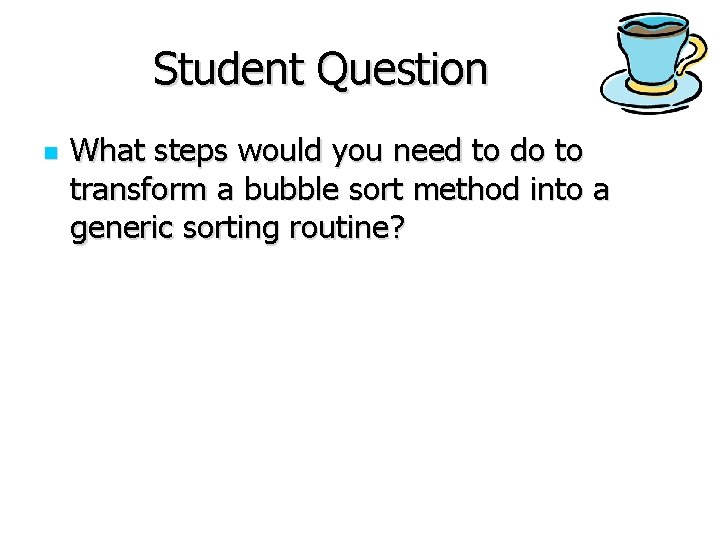
Student Question n What steps would you need to do to transform a bubble sort method into a generic sorting routine?
![Generic bin Search public static T extends Comparable super T int bin SearchT arr Generic bin. Search() public static <T extends Comparable<? super T>> int bin. Search(T[] arr,](https://slidetodoc.com/presentation_image_h2/4a7728ac8d77876ddc2abe98a0652acc/image-5.jpg)
Generic bin. Search() public static <T extends Comparable<? super T>> int bin. Search(T[] arr, int first, int last, T target) { // index of the midpoint mid; // object that is assigned arr[mid] T midvalue; // save original value of last int orig. Last = last; // test for nonempty sublist while (first < last) { mid = (first+last)/2; midvalue = arr[mid]; © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
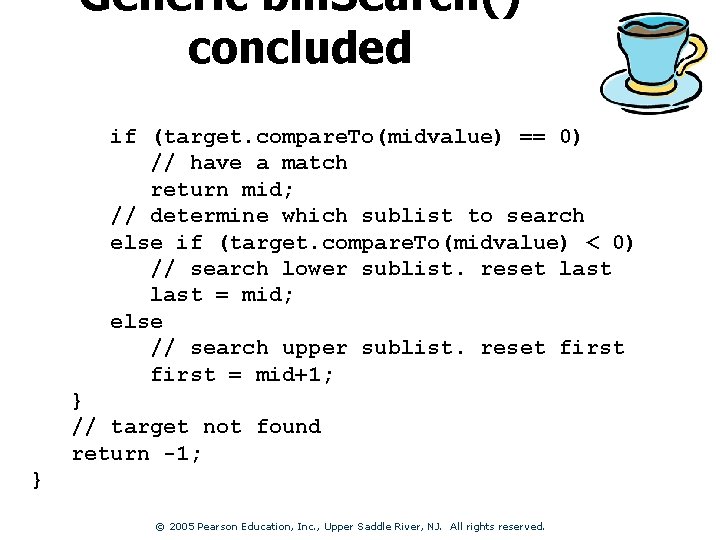
Generic bin. Search() concluded if (target. compare. To(midvalue) == 0) // have a match return mid; // determine which sublist to search else if (target. compare. To(midvalue) < 0) // search lower sublist. reset last = mid; else // search upper sublist. reset first = mid+1; } // target not found return -1; } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
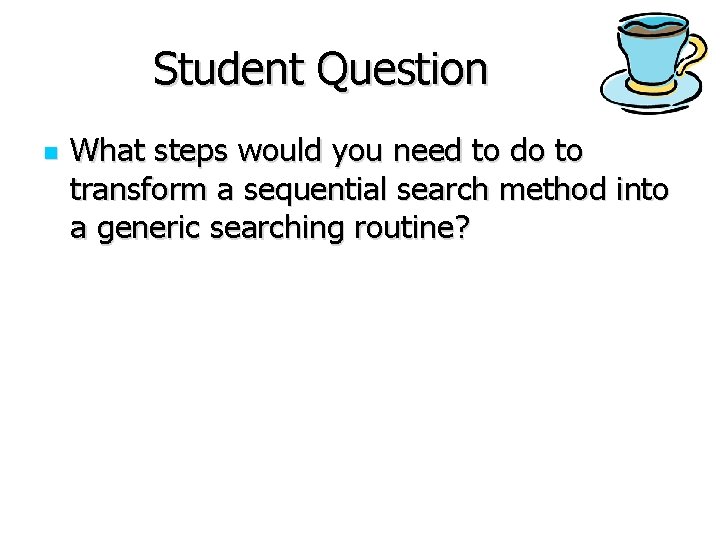
Student Question n What steps would you need to do to transform a sequential search method into a generic searching routine?
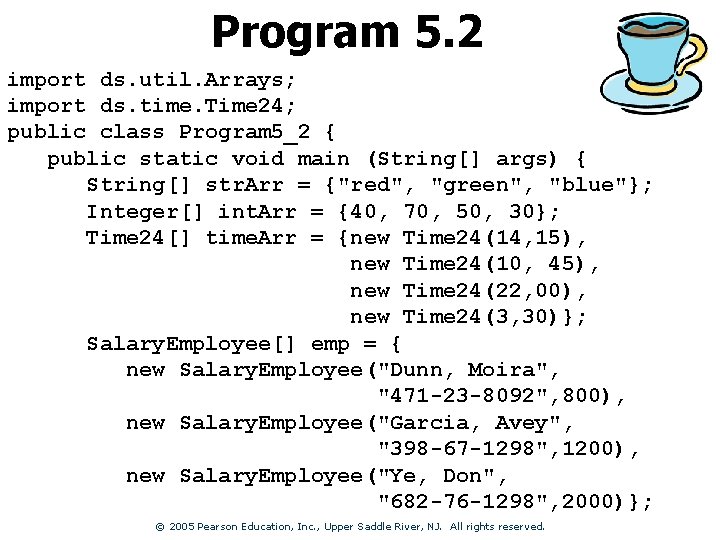
Program 5. 2 import ds. util. Arrays; import ds. time. Time 24; public class Program 5_2 { public static void main (String[] args) { String[] str. Arr = {"red", "green", "blue"}; Integer[] int. Arr = {40, 70, 50, 30}; Time 24[] time. Arr = {new Time 24(14, 15), new Time 24(10, 45), new Time 24(22, 00), new Time 24(3, 30)}; Salary. Employee[] emp = { new Salary. Employee("Dunn, Moira", "471 -23 -8092", 800), new Salary. Employee("Garcia, Avey", "398 -67 -1298", 1200), new Salary. Employee("Ye, Don", "682 -76 -1298", 2000)}; © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
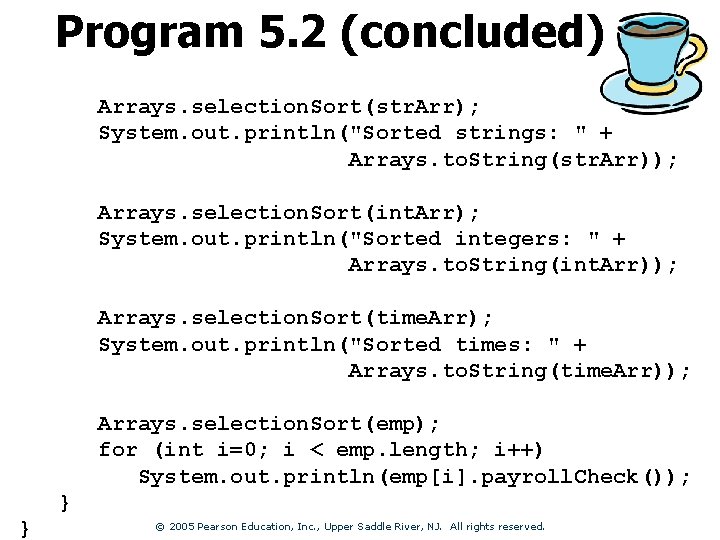
Program 5. 2 (concluded) Arrays. selection. Sort(str. Arr); System. out. println("Sorted strings: " + Arrays. to. String(str. Arr)); Arrays. selection. Sort(int. Arr); System. out. println("Sorted integers: " + Arrays. to. String(int. Arr)); Arrays. selection. Sort(time. Arr); System. out. println("Sorted times: " + Arrays. to. String(time. Arr)); Arrays. selection. Sort(emp); for (int i=0; i < emp. length; i++) System. out. println(emp[i]. payroll. Check()); } } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
![Program 5 2 Run Sorted strings blue green red Sorted integers 30 40 50 Program 5. 2 Run: Sorted strings: [blue, green, red] Sorted integers: [30, 40, 50,](https://slidetodoc.com/presentation_image_h2/4a7728ac8d77876ddc2abe98a0652acc/image-10.jpg)
Program 5. 2 Run: Sorted strings: [blue, green, red] Sorted integers: [30, 40, 50, 70] Sorted times: [3: 30, 10: 45, 14: 15, 22: 00] Pay Garcia, Avey (398 -67 -1298) $1200. 00 Pay Dunn, Moira (471 -23 -8092) $800. 00 Pay Ye, Don (682 -76 -1298) $2000. 00 © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.