Functional Programming Lecture 2 Functions Muffy Calder Function
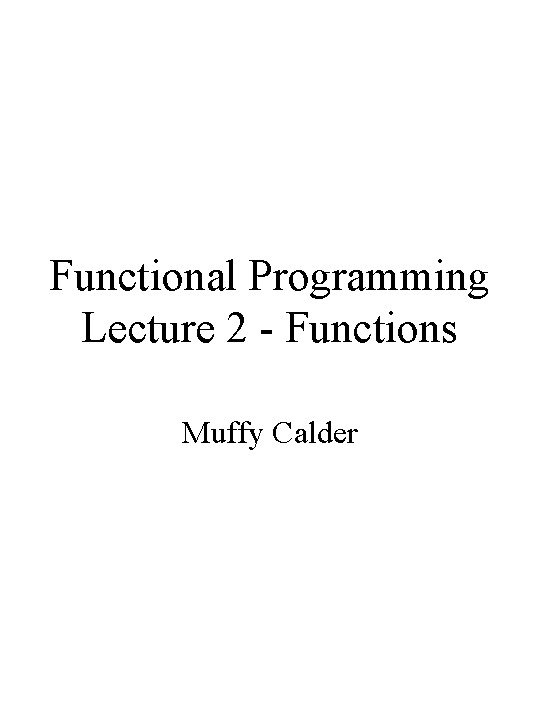
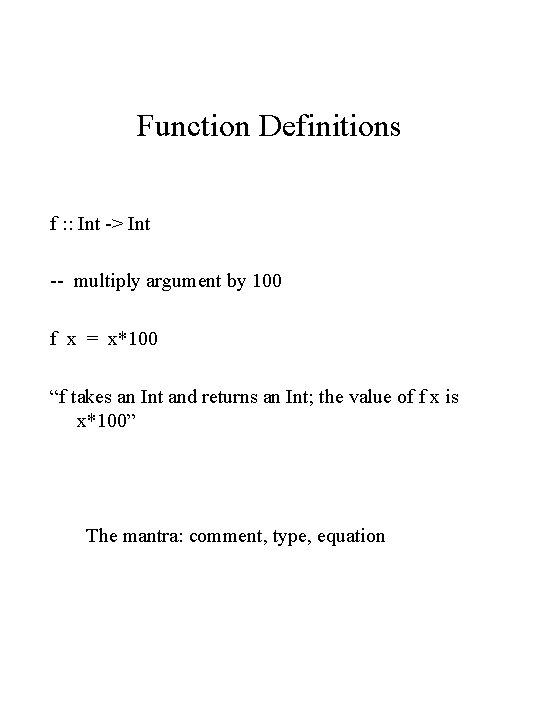
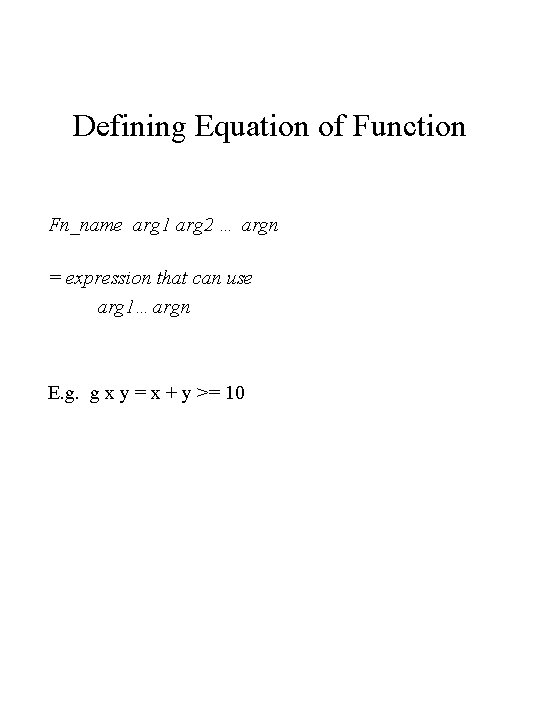
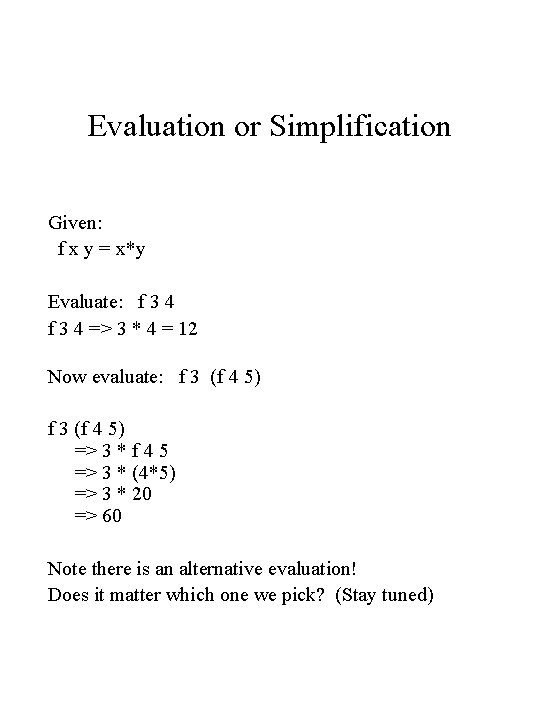
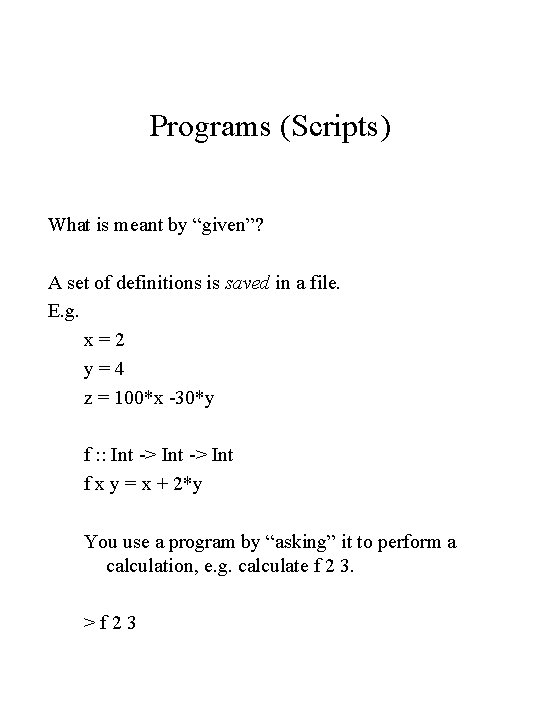
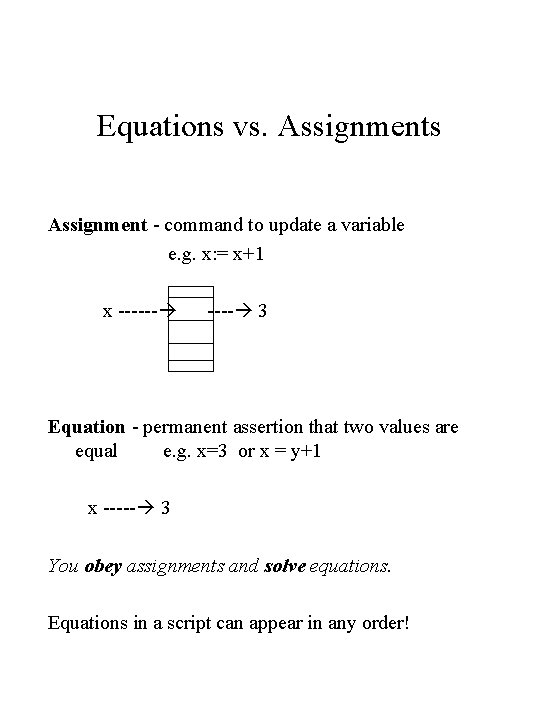
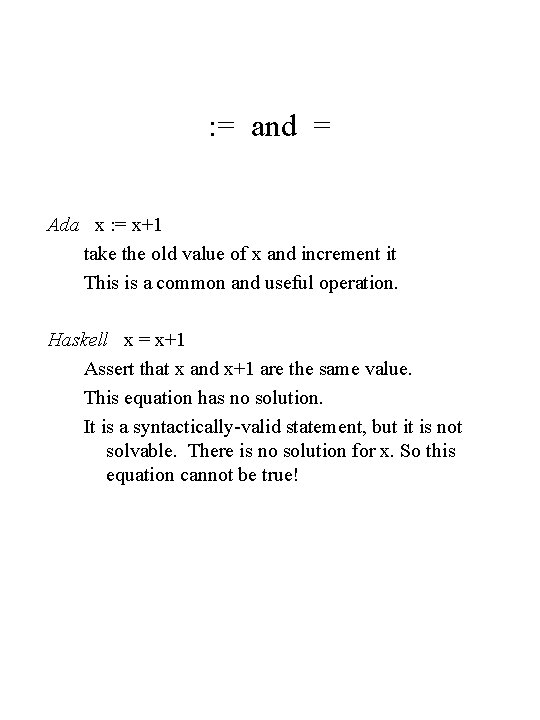
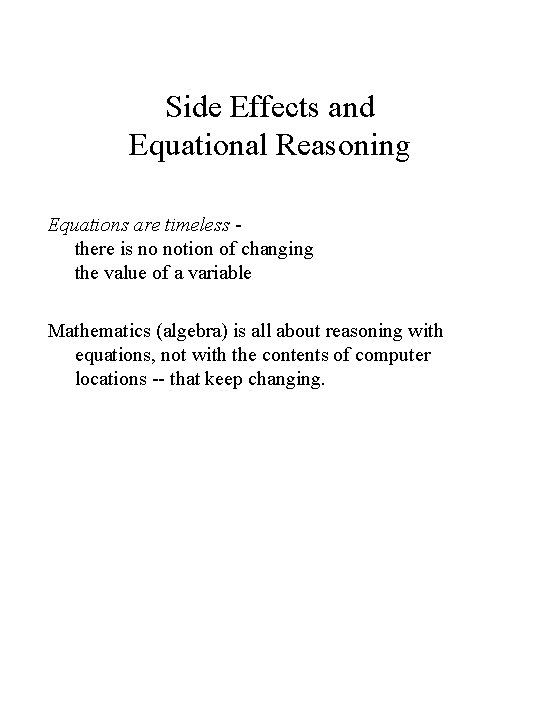
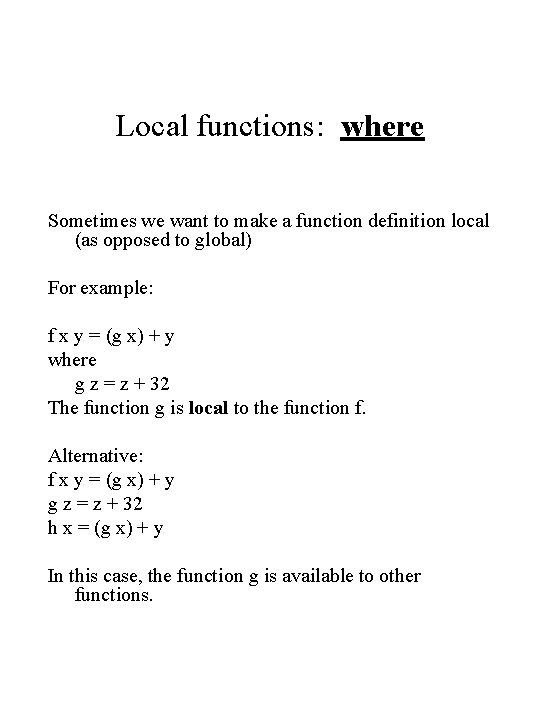
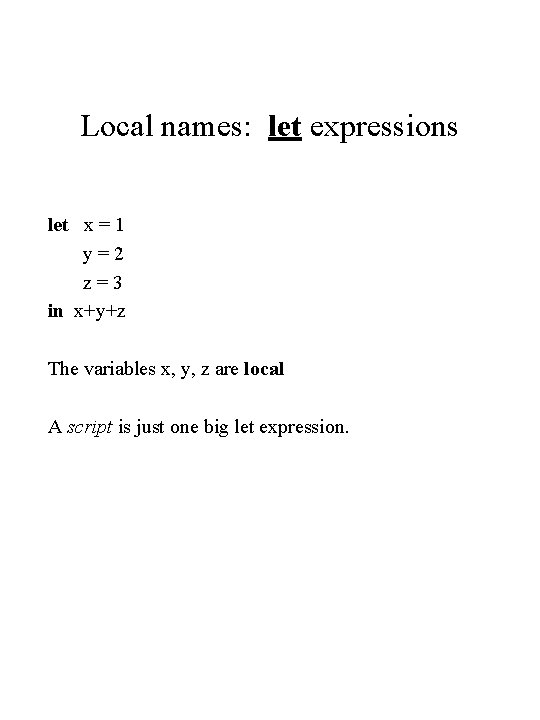
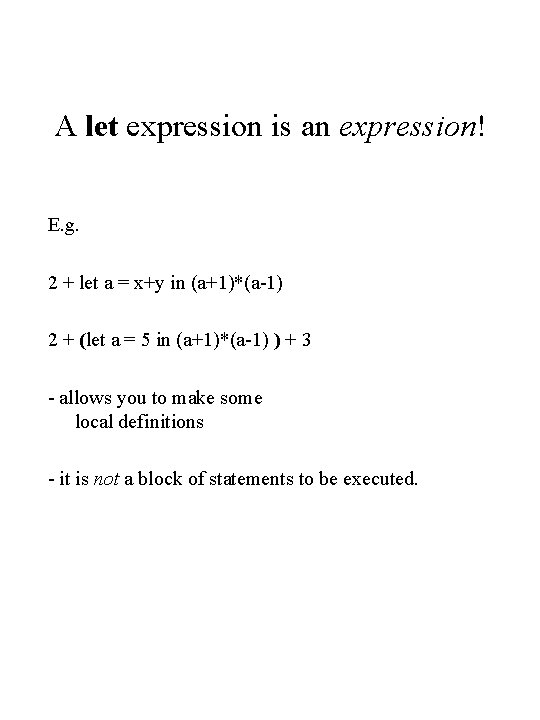
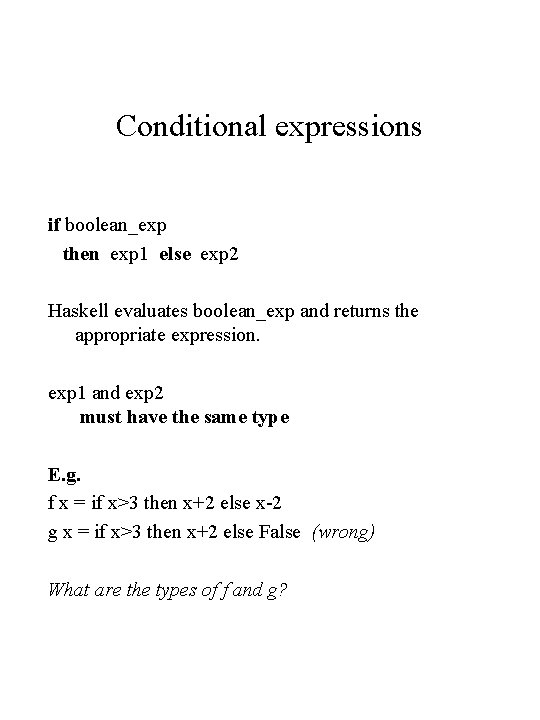
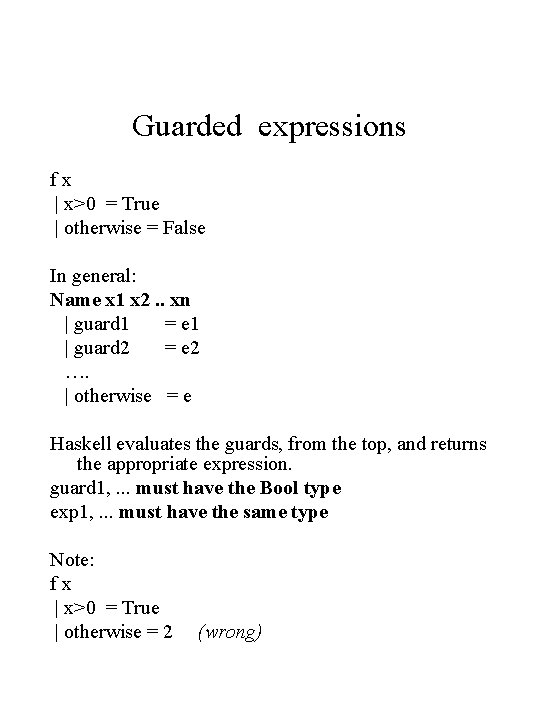
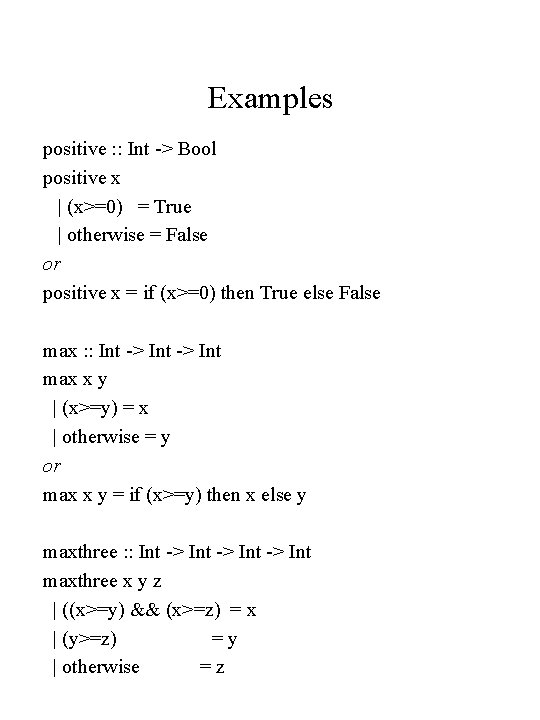
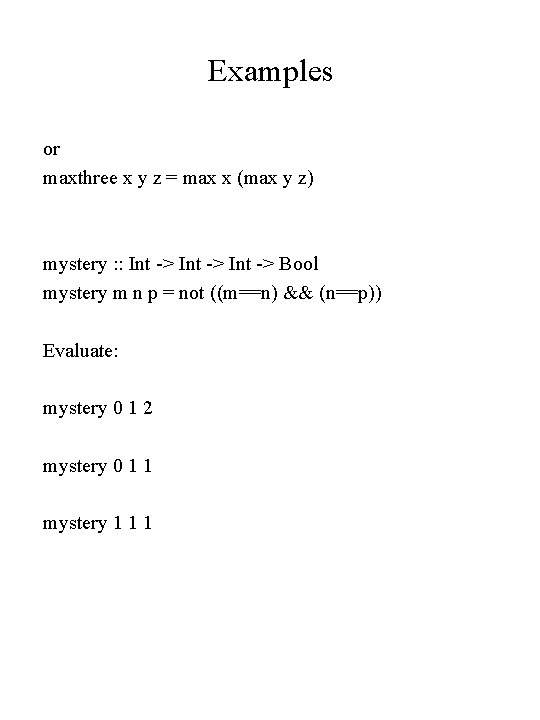
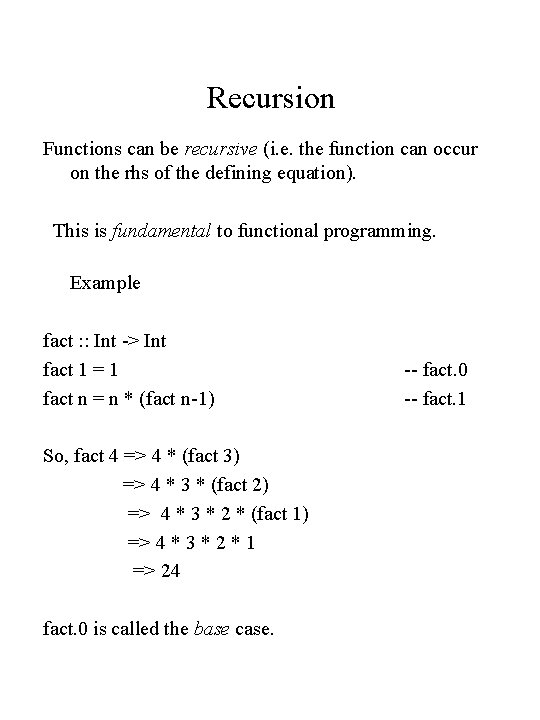
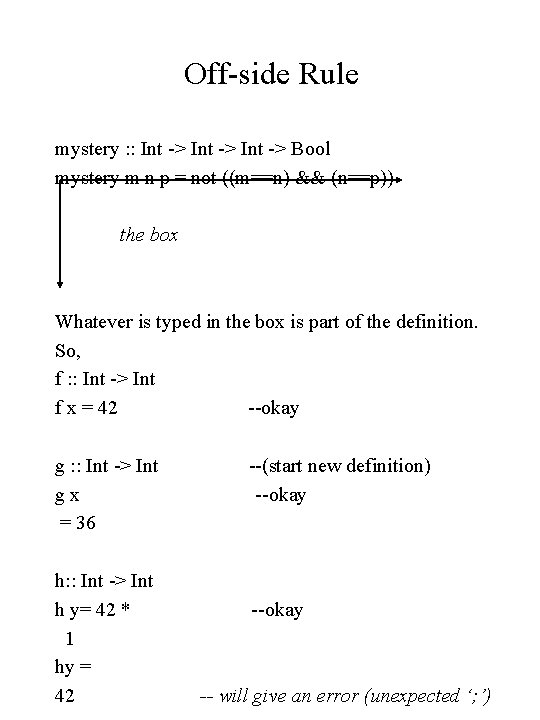
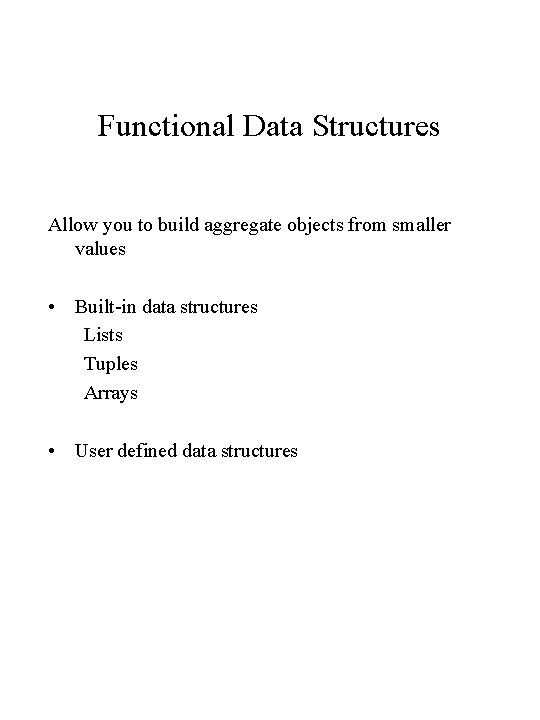
- Slides: 18
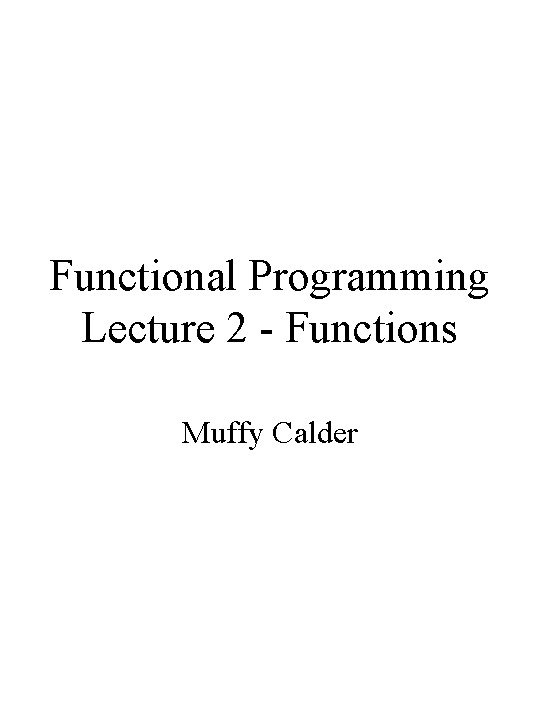
Functional Programming Lecture 2 - Functions Muffy Calder
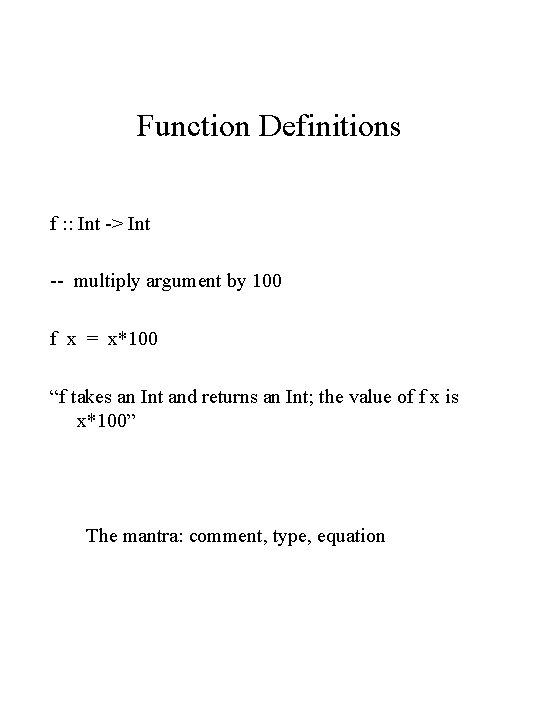
Function Definitions f : : Int -> Int -- multiply argument by 100 f x = x*100 “f takes an Int and returns an Int; the value of f x is x*100” The mantra: comment, type, equation
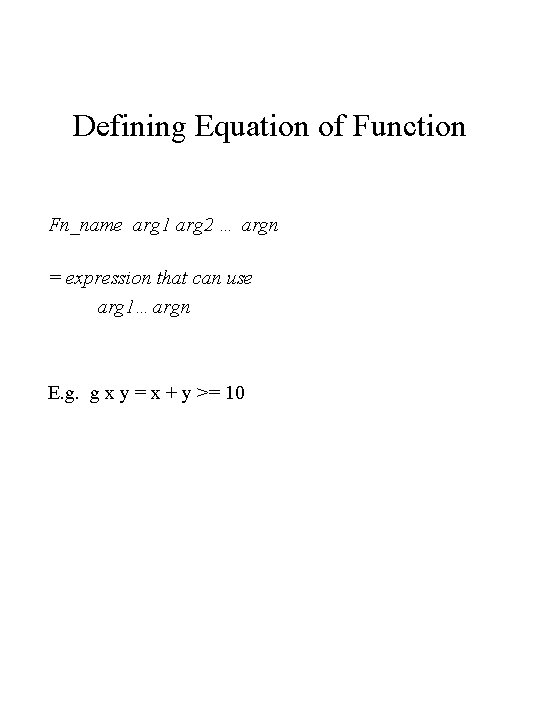
Defining Equation of Function Fn_name arg 1 arg 2 … argn = expression that can use arg 1…argn E. g. g x y = x + y >= 10
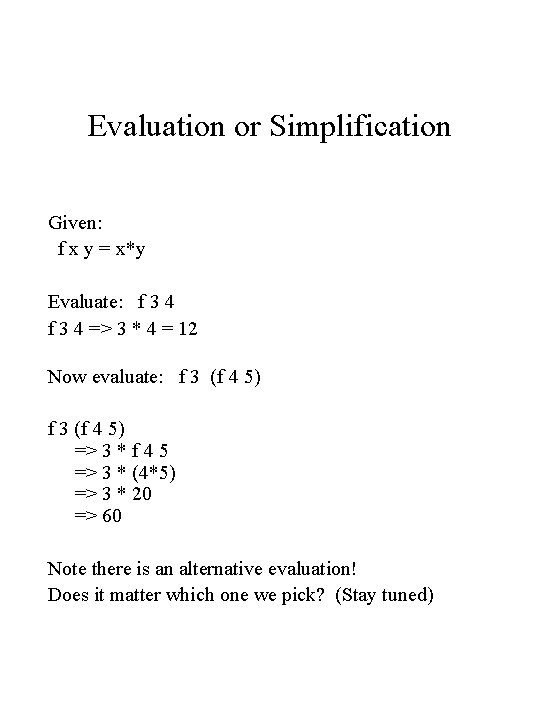
Evaluation or Simplification Given: f x y = x*y Evaluate: f 3 4 => 3 * 4 = 12 Now evaluate: f 3 (f 4 5) => 3 * f 4 5 => 3 * (4*5) => 3 * 20 => 60 Note there is an alternative evaluation! Does it matter which one we pick? (Stay tuned)
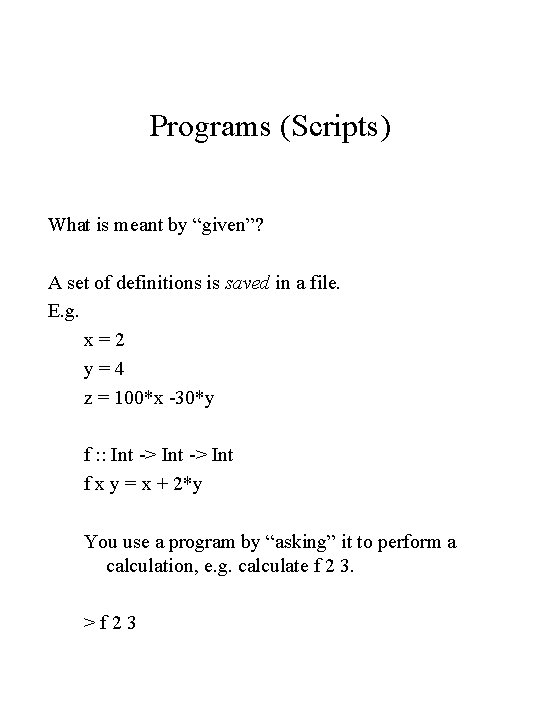
Programs (Scripts) What is meant by “given”? A set of definitions is saved in a file. E. g. x=2 y=4 z = 100*x -30*y f : : Int -> Int f x y = x + 2*y You use a program by “asking” it to perform a calculation, e. g. calculate f 2 3. >f 23
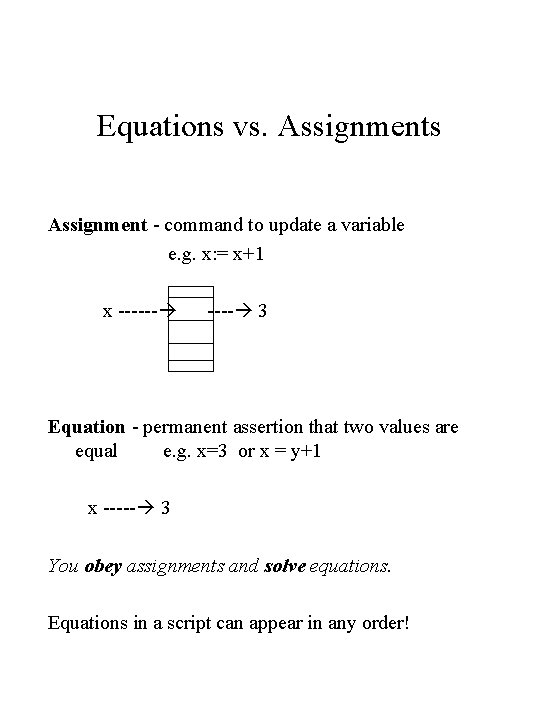
Equations vs. Assignments Assignment - command to update a variable e. g. x: = x+1 x ------ 3 Equation - permanent assertion that two values are equal e. g. x=3 or x = y+1 x ----- 3 You obey assignments and solve equations. Equations in a script can appear in any order!
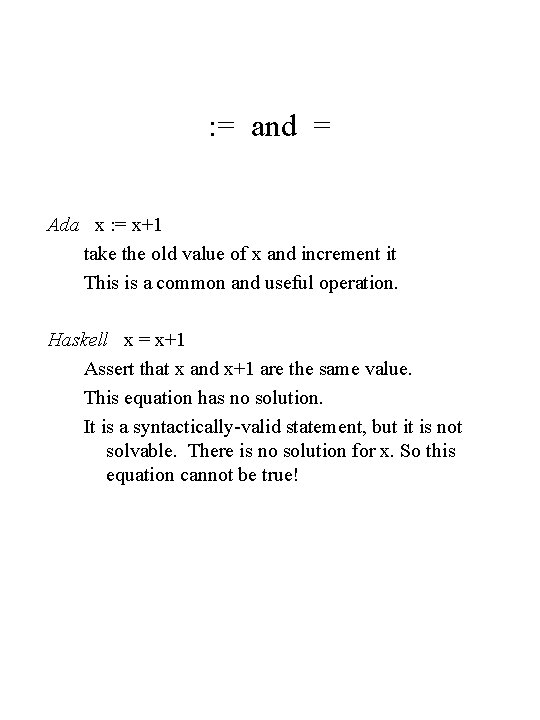
: = and = Ada x : = x+1 take the old value of x and increment it This is a common and useful operation. Haskell x = x+1 Assert that x and x+1 are the same value. This equation has no solution. It is a syntactically-valid statement, but it is not solvable. There is no solution for x. So this equation cannot be true!
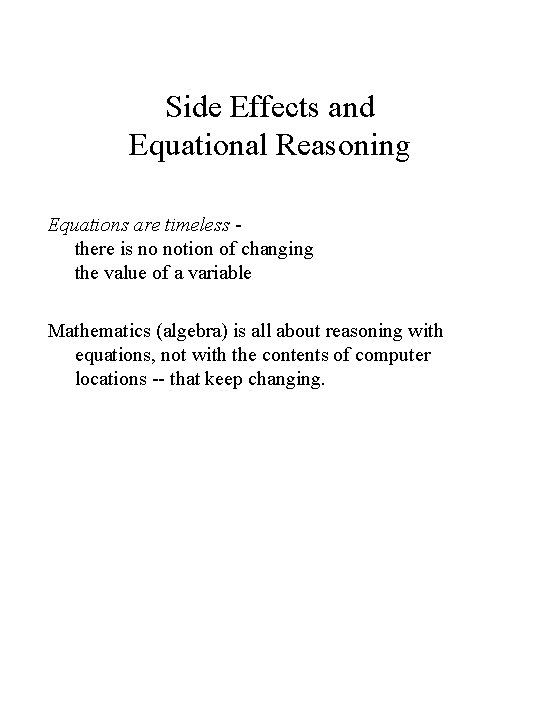
Side Effects and Equational Reasoning Equations are timeless there is no notion of changing the value of a variable Mathematics (algebra) is all about reasoning with equations, not with the contents of computer locations -- that keep changing.
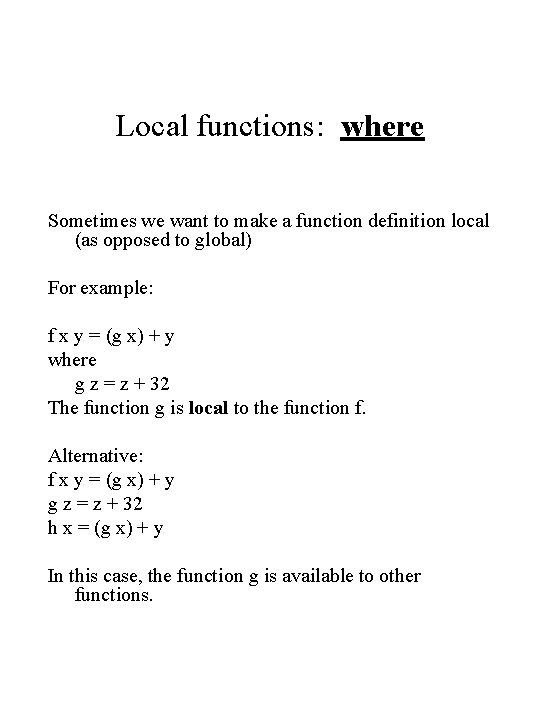
Local functions: where Sometimes we want to make a function definition local (as opposed to global) For example: f x y = (g x) + y where g z = z + 32 The function g is local to the function f. Alternative: f x y = (g x) + y g z = z + 32 h x = (g x) + y In this case, the function g is available to other functions.
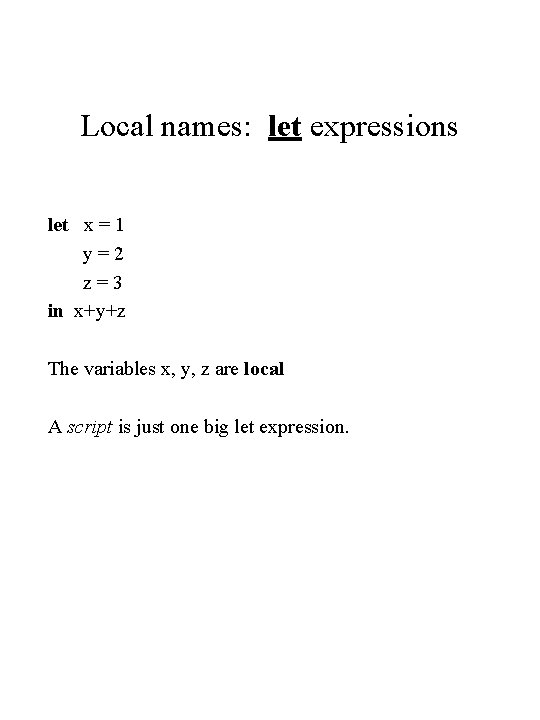
Local names: let expressions let x = 1 y=2 z=3 in x+y+z The variables x, y, z are local A script is just one big let expression.
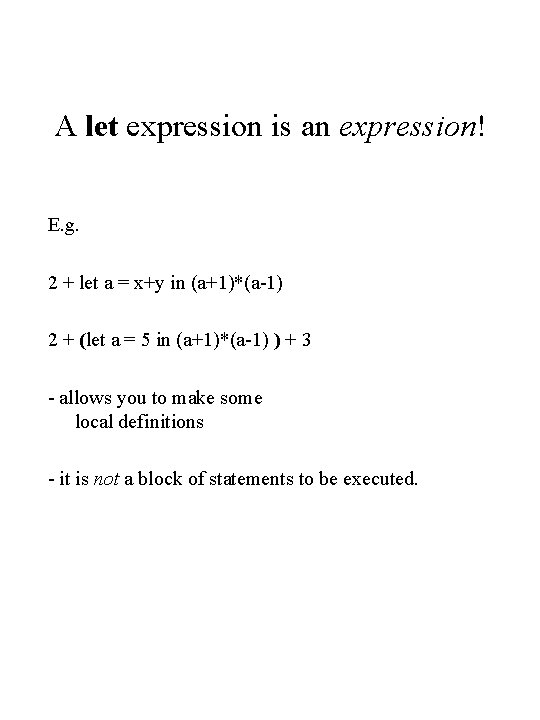
A let expression is an expression! E. g. 2 + let a = x+y in (a+1)*(a-1) 2 + (let a = 5 in (a+1)*(a-1) ) + 3 - allows you to make some local definitions - it is not a block of statements to be executed.
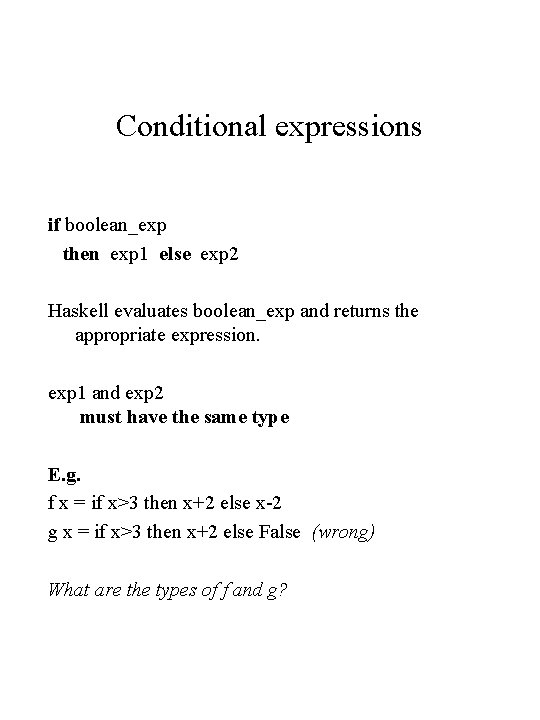
Conditional expressions if boolean_exp then exp 1 else exp 2 Haskell evaluates boolean_exp and returns the appropriate expression. exp 1 and exp 2 must have the same type E. g. f x = if x>3 then x+2 else x-2 g x = if x>3 then x+2 else False (wrong) What are the types of f and g?
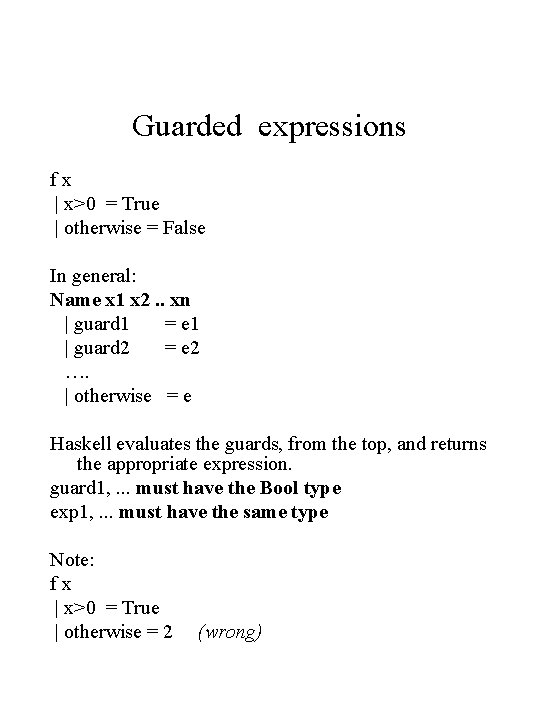
Guarded expressions fx | x>0 = True | otherwise = False In general: Name x 1 x 2. . xn | guard 1 = e 1 | guard 2 = e 2 …. | otherwise = e Haskell evaluates the guards, from the top, and returns the appropriate expression. guard 1, . . . must have the Bool type exp 1, . . . must have the same type Note: fx | x>0 = True | otherwise = 2 (wrong)
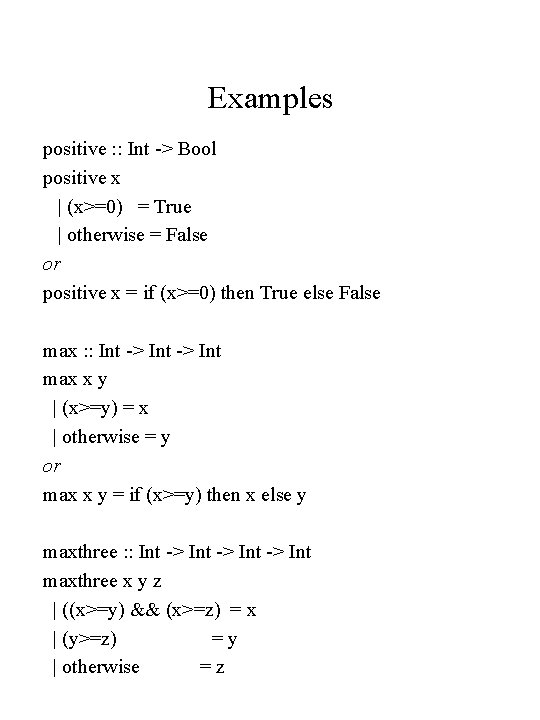
Examples positive : : Int -> Bool positive x | (x>=0) = True | otherwise = False or positive x = if (x>=0) then True else False max : : Int -> Int max x y | (x>=y) = x | otherwise = y or max x y = if (x>=y) then x else y maxthree : : Int -> Int maxthree x y z | ((x>=y) && (x>=z) = x | (y>=z) =y | otherwise =z
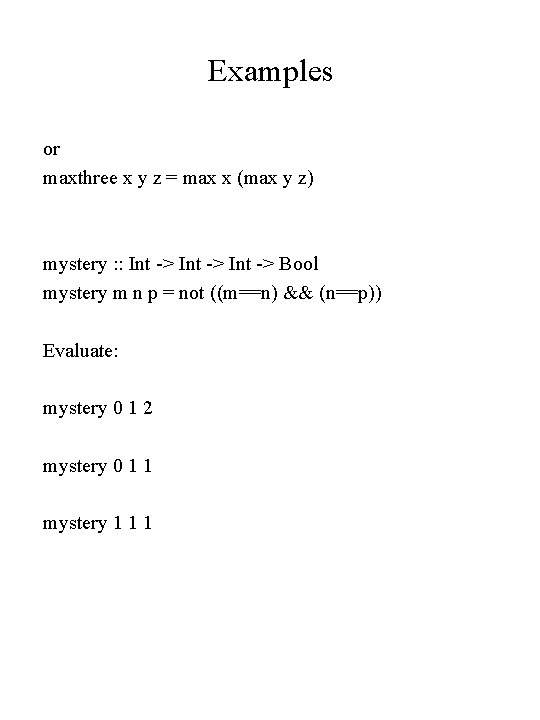
Examples or maxthree x y z = max x (max y z) mystery : : Int -> Bool mystery m n p = not ((m==n) && (n==p)) Evaluate: mystery 0 1 2 mystery 0 1 1 mystery 1 1 1
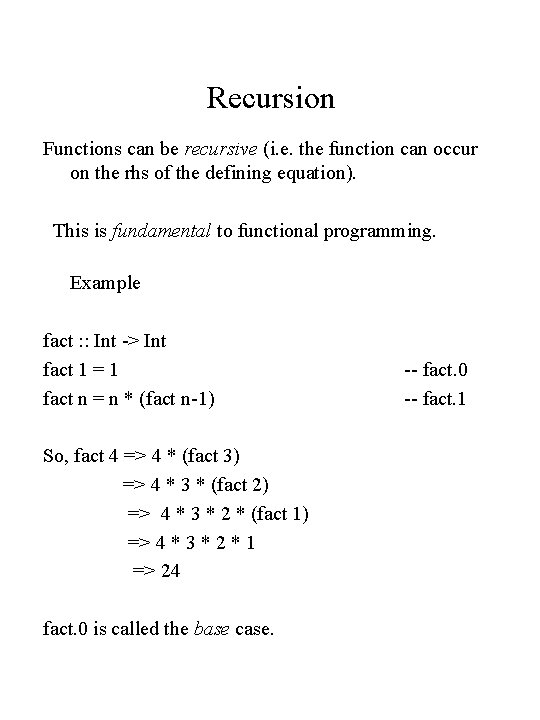
Recursion Functions can be recursive (i. e. the function can occur on the rhs of the defining equation). This is fundamental to functional programming. Example fact : : Int -> Int fact 1 = 1 fact n = n * (fact n-1) So, fact 4 => 4 * (fact 3) => 4 * 3 * (fact 2) => 4 * 3 * 2 * (fact 1) => 4 * 3 * 2 * 1 => 24 fact. 0 is called the base case. -- fact. 0 -- fact. 1
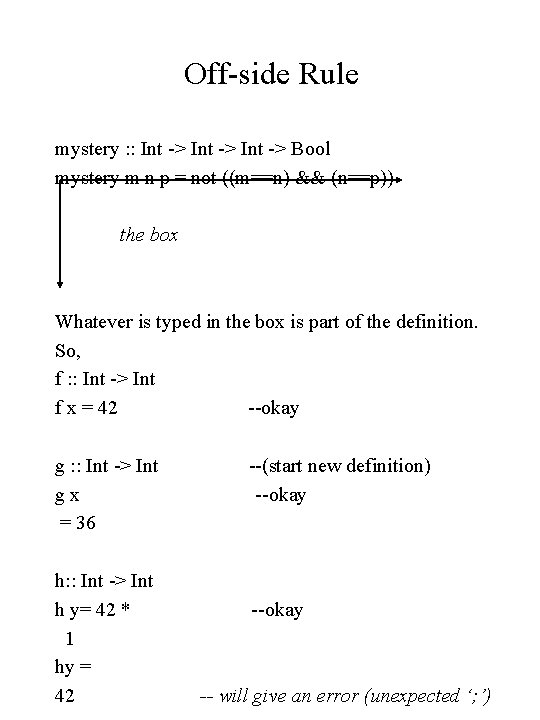
Off-side Rule mystery : : Int -> Bool mystery m n p = not ((m==n) && (n==p)) the box Whatever is typed in the box is part of the definition. So, f : : Int -> Int f x = 42 --okay g : : Int -> Int gx = 36 h: : Int -> Int h y= 42 * 1 hy = 42 --(start new definition) --okay -- will give an error (unexpected ‘; ’)
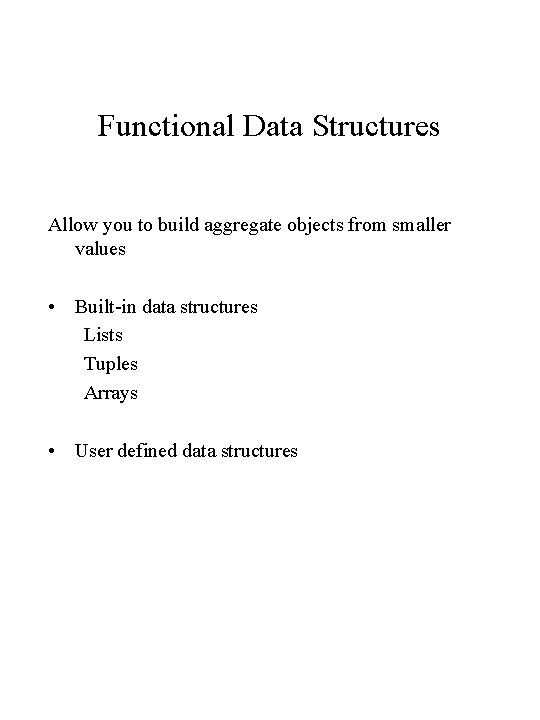
Functional Data Structures Allow you to build aggregate objects from smaller values • Built-in data structures Lists Tuples Arrays • User defined data structures
01:640:244 lecture notes - lecture 15: plat, idah, farad
Calder mobiles and stabiles
Alexander calder mobile-stabile
Alexander calder stabile-mobile
Alexander calder wire portraits
Brad calder
Calder wire portraits
Cabeza y cola de alexander calder
Esculturas estables
Azure calder
Alexander calder medusa
Cascading flowers calder
Alexander calder medium
C programming lecture
Distal shoe appliance
Non functional plasma enzymes
Functional and non functional plasma enzymes
Functional and non functional
Procedural functional object oriented