Dijkstras Algorithm and Heuristic Graph Search David Johnson
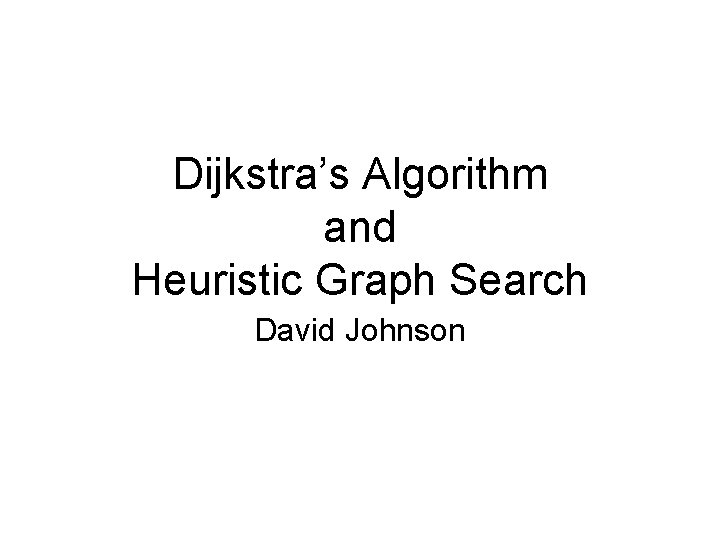
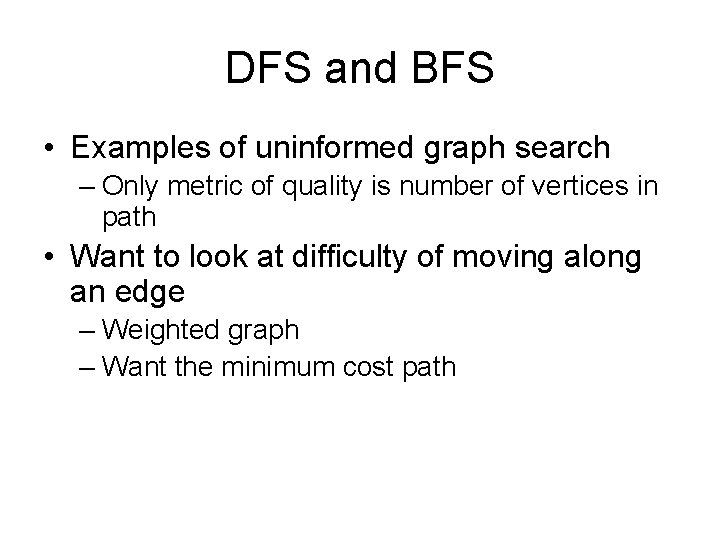
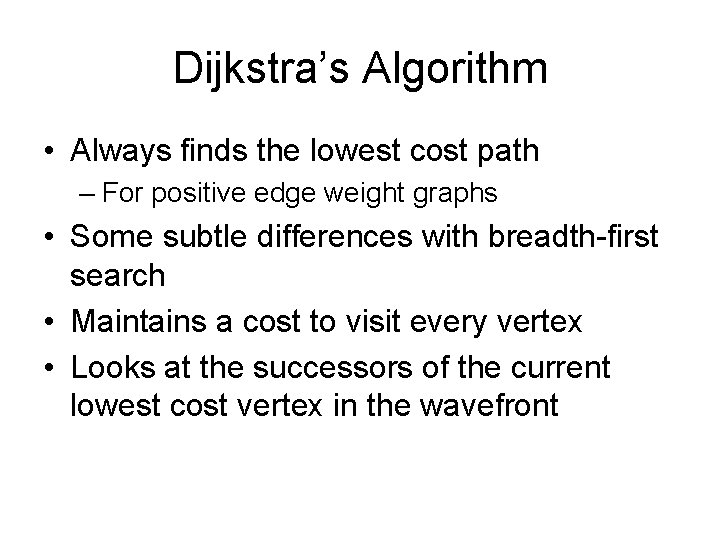
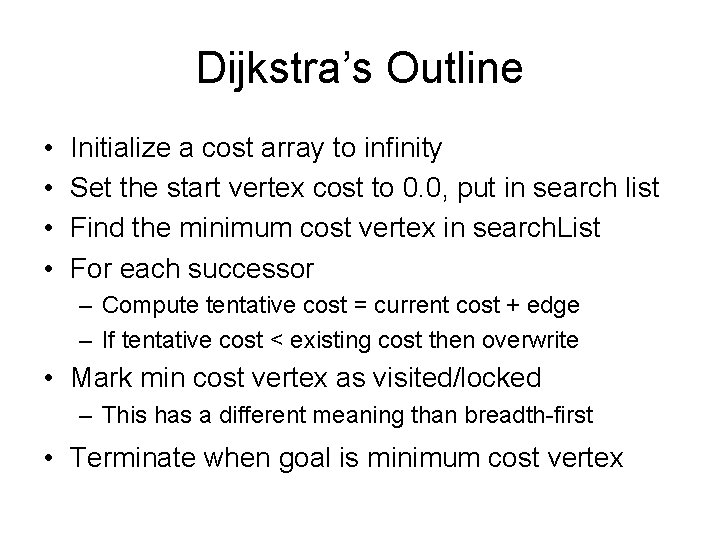
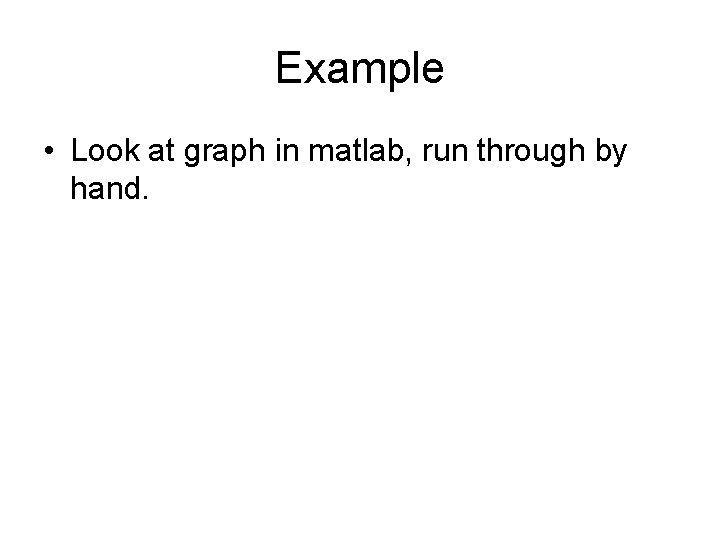
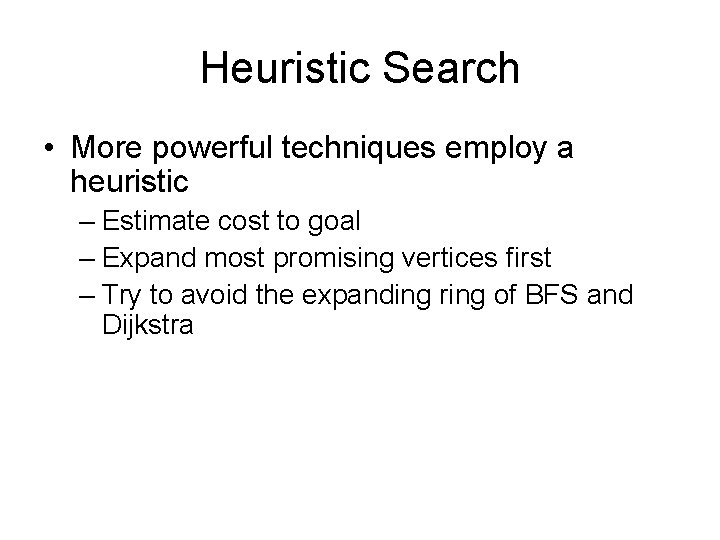
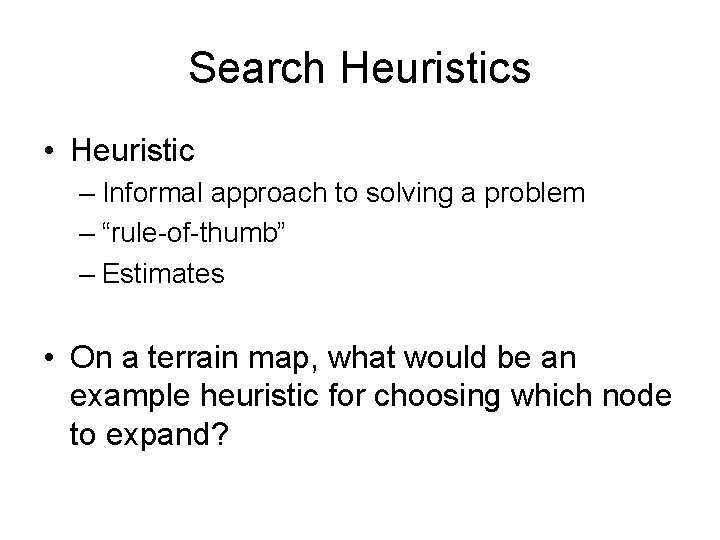
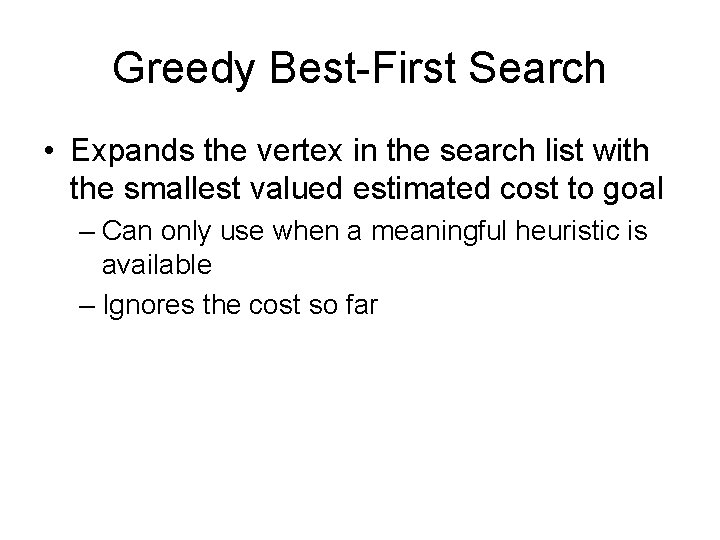
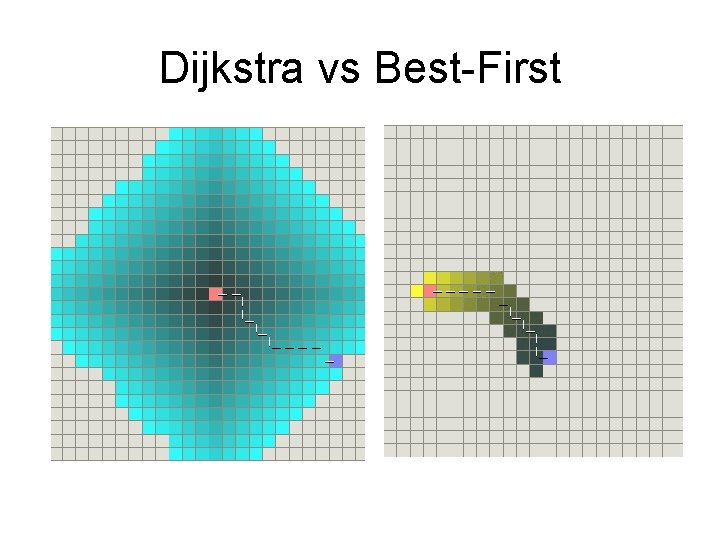
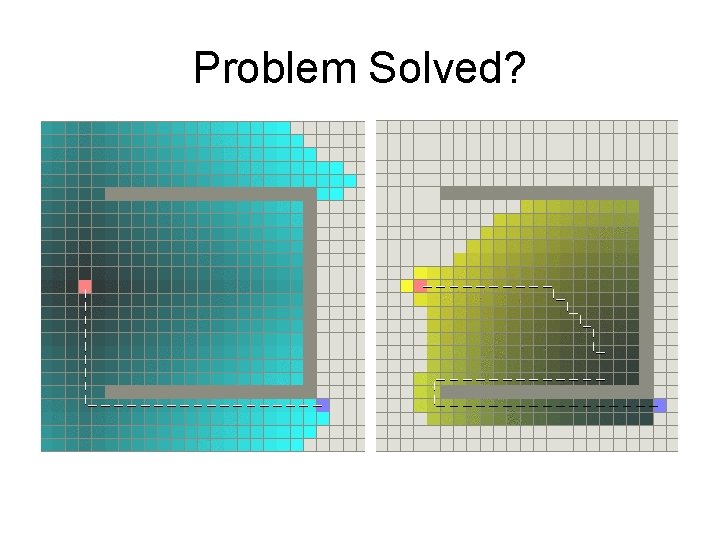
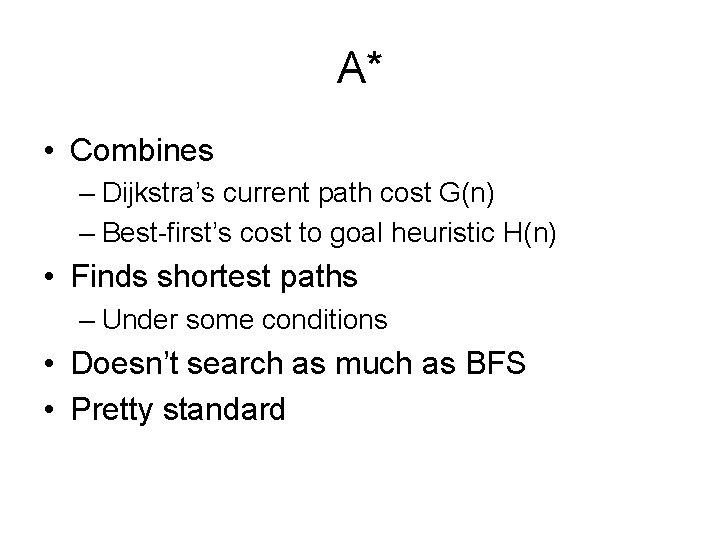
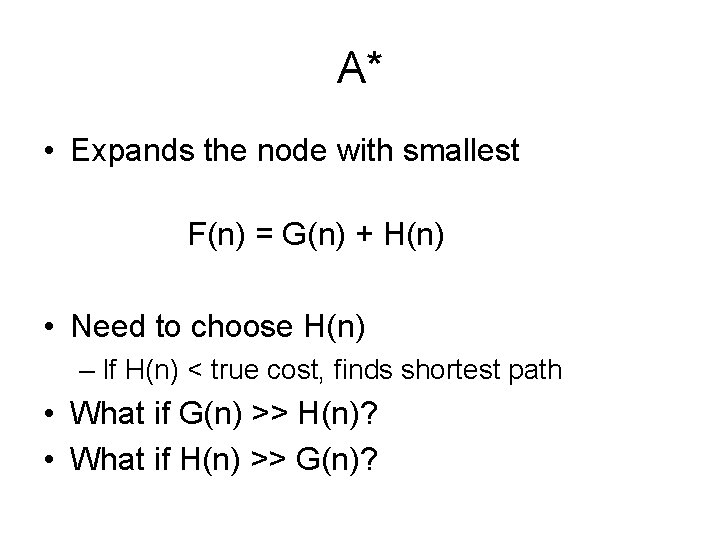
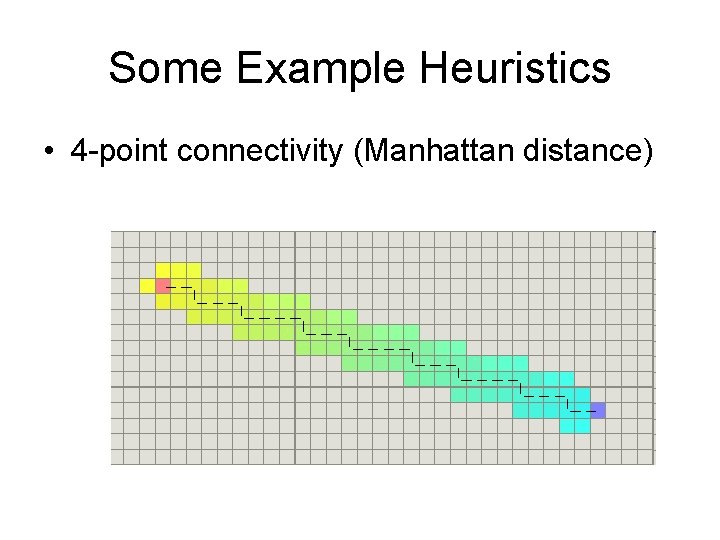
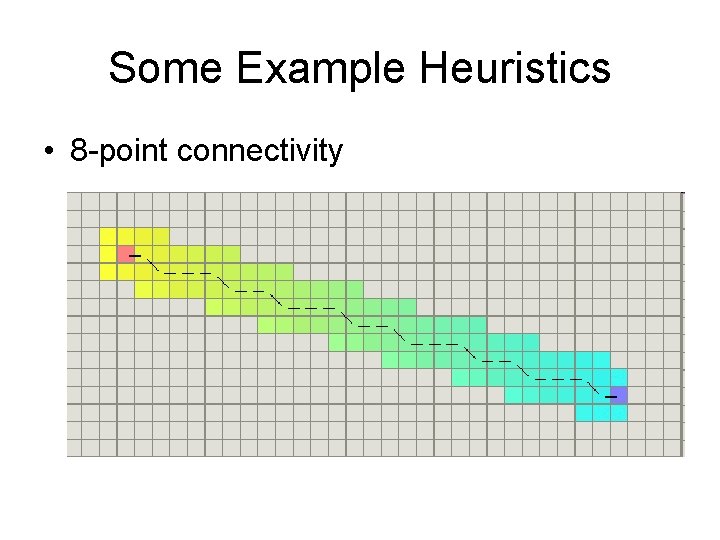
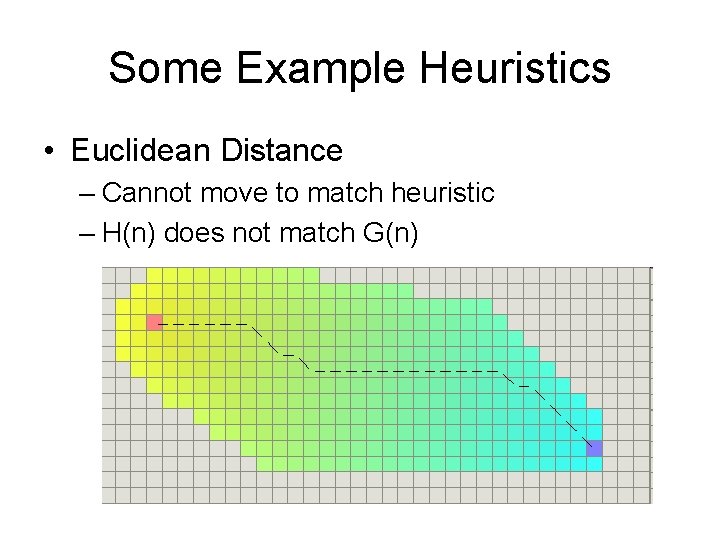
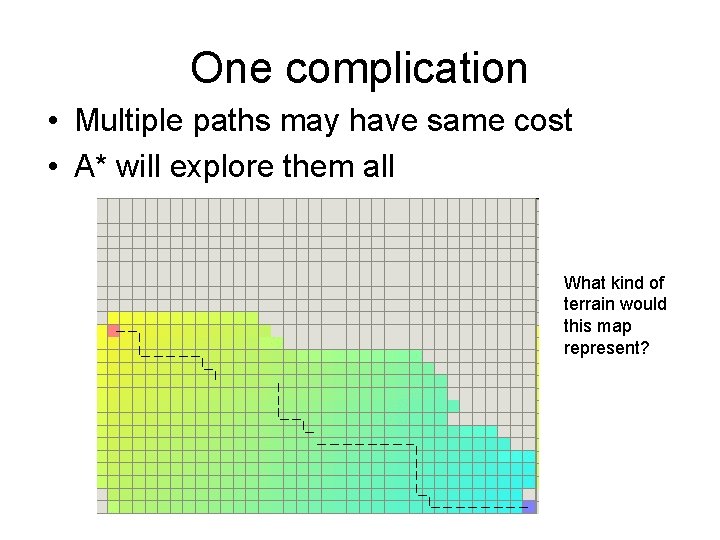
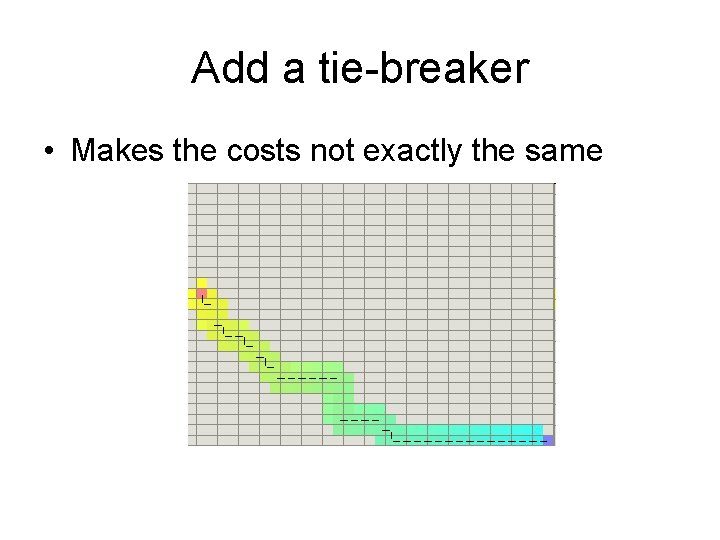
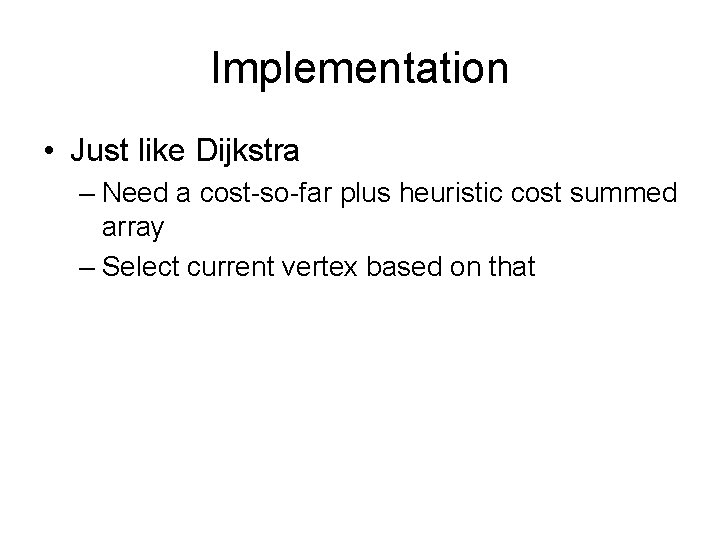
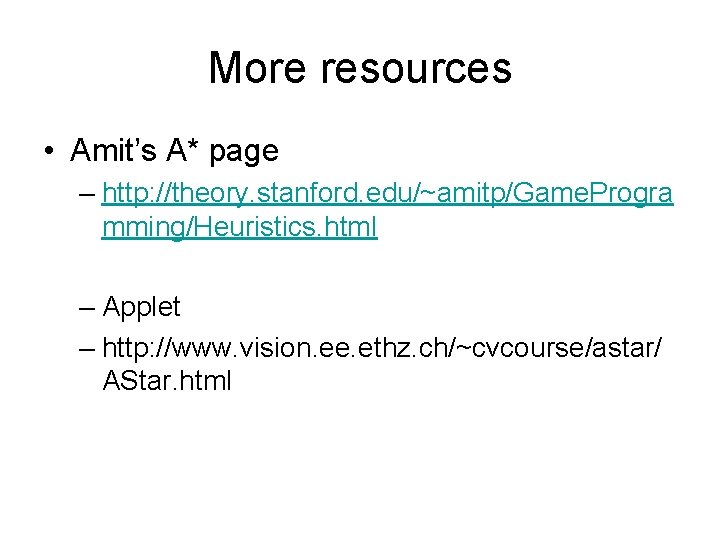
- Slides: 19
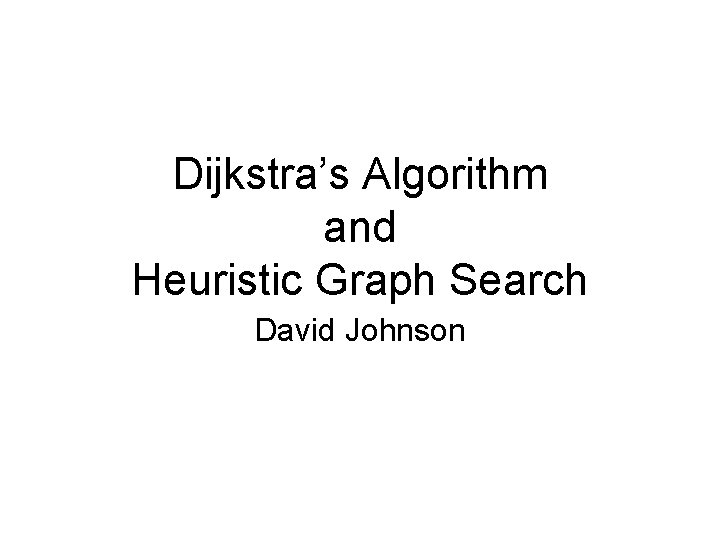
Dijkstra’s Algorithm and Heuristic Graph Search David Johnson
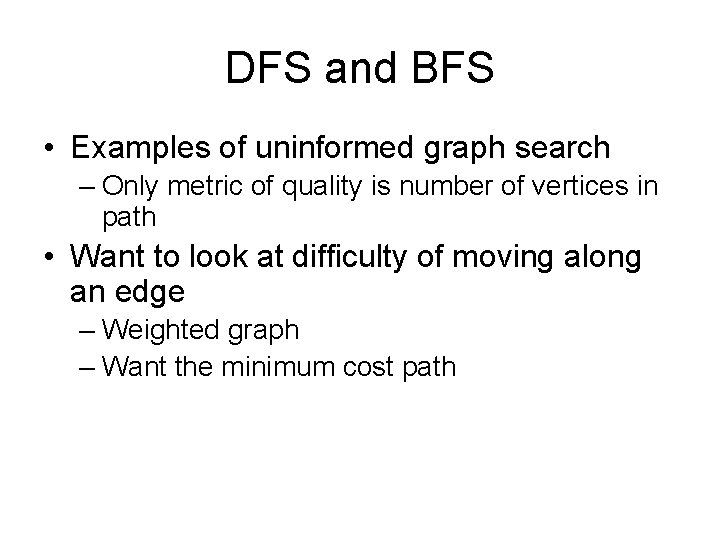
DFS and BFS • Examples of uninformed graph search – Only metric of quality is number of vertices in path • Want to look at difficulty of moving along an edge – Weighted graph – Want the minimum cost path
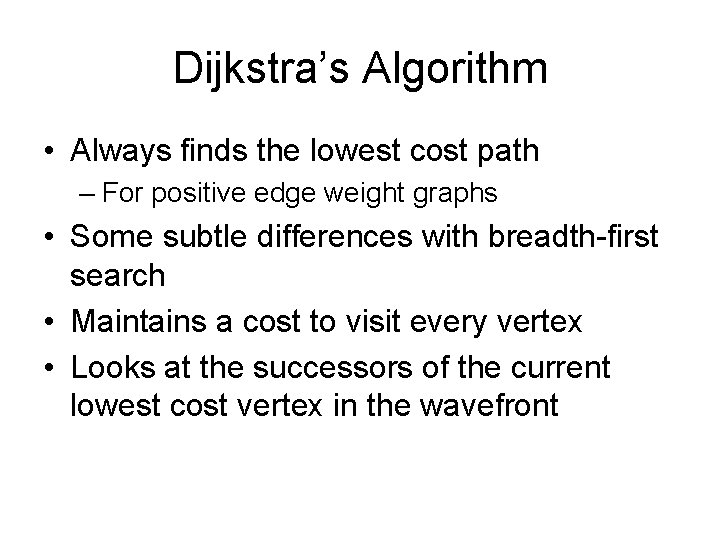
Dijkstra’s Algorithm • Always finds the lowest cost path – For positive edge weight graphs • Some subtle differences with breadth-first search • Maintains a cost to visit every vertex • Looks at the successors of the current lowest cost vertex in the wavefront
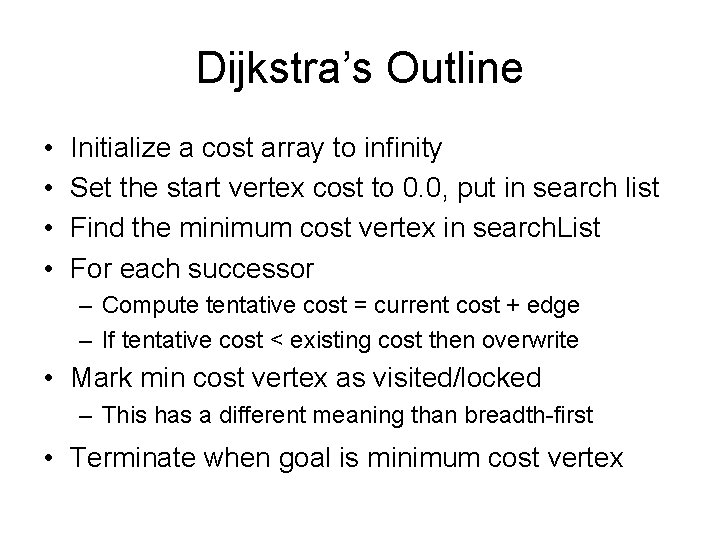
Dijkstra’s Outline • • Initialize a cost array to infinity Set the start vertex cost to 0. 0, put in search list Find the minimum cost vertex in search. List For each successor – Compute tentative cost = current cost + edge – If tentative cost < existing cost then overwrite • Mark min cost vertex as visited/locked – This has a different meaning than breadth-first • Terminate when goal is minimum cost vertex
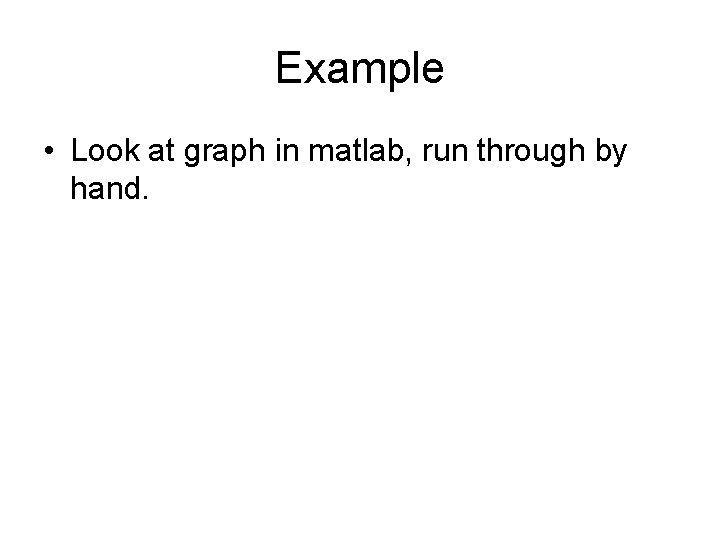
Example • Look at graph in matlab, run through by hand.
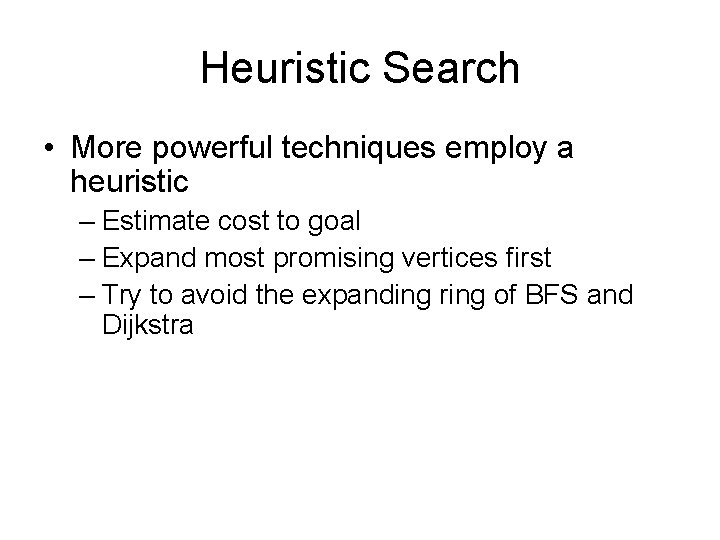
Heuristic Search • More powerful techniques employ a heuristic – Estimate cost to goal – Expand most promising vertices first – Try to avoid the expanding ring of BFS and Dijkstra
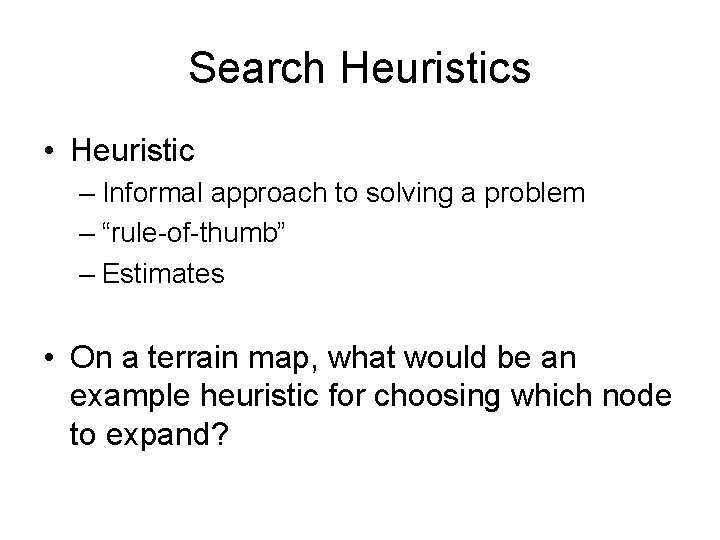
Search Heuristics • Heuristic – Informal approach to solving a problem – “rule-of-thumb” – Estimates • On a terrain map, what would be an example heuristic for choosing which node to expand?
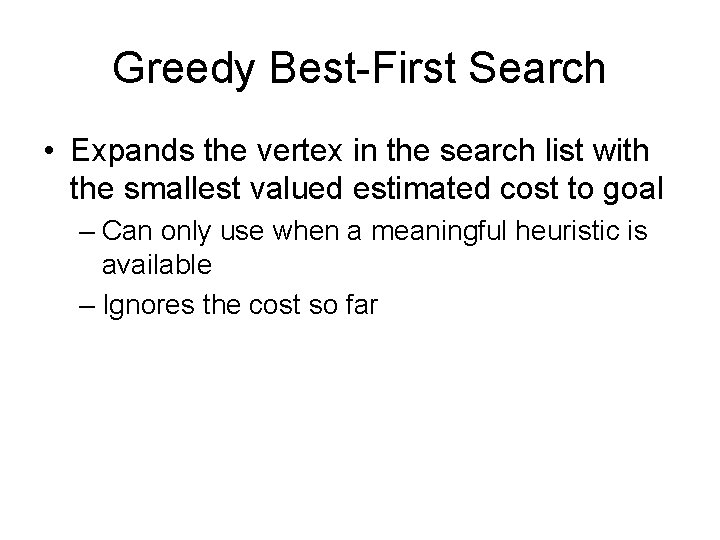
Greedy Best-First Search • Expands the vertex in the search list with the smallest valued estimated cost to goal – Can only use when a meaningful heuristic is available – Ignores the cost so far
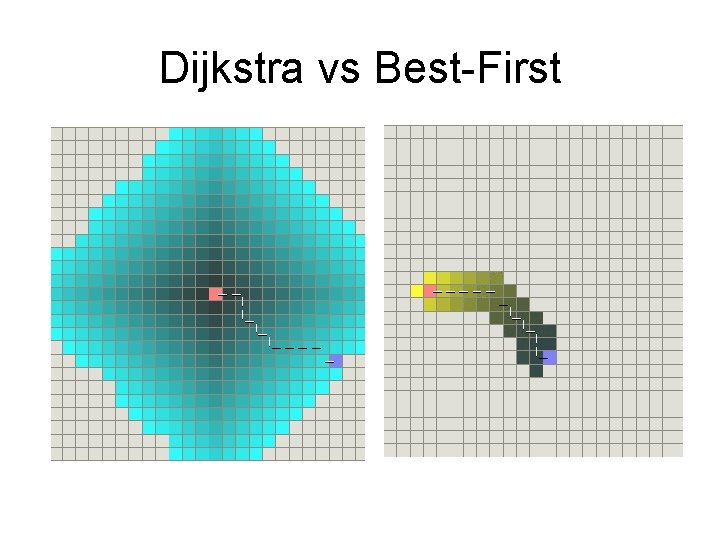
Dijkstra vs Best-First
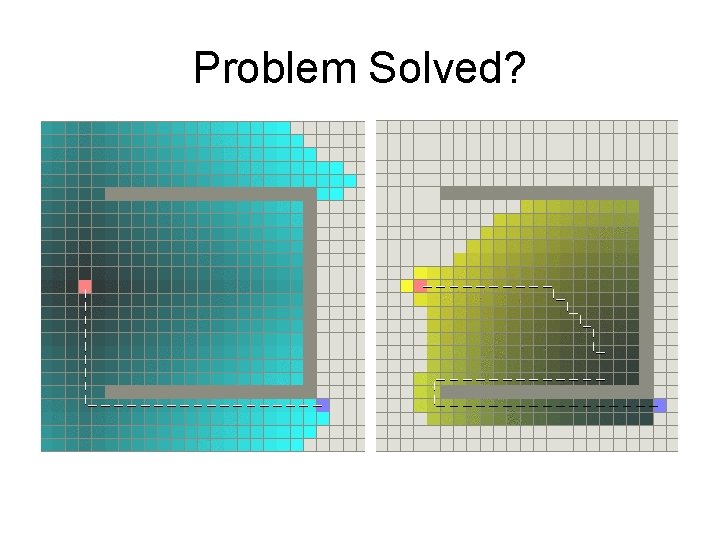
Problem Solved?
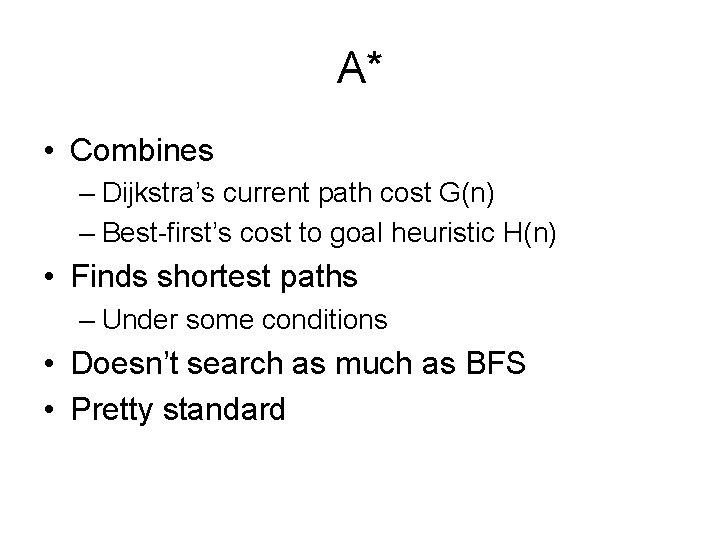
A* • Combines – Dijkstra’s current path cost G(n) – Best-first’s cost to goal heuristic H(n) • Finds shortest paths – Under some conditions • Doesn’t search as much as BFS • Pretty standard
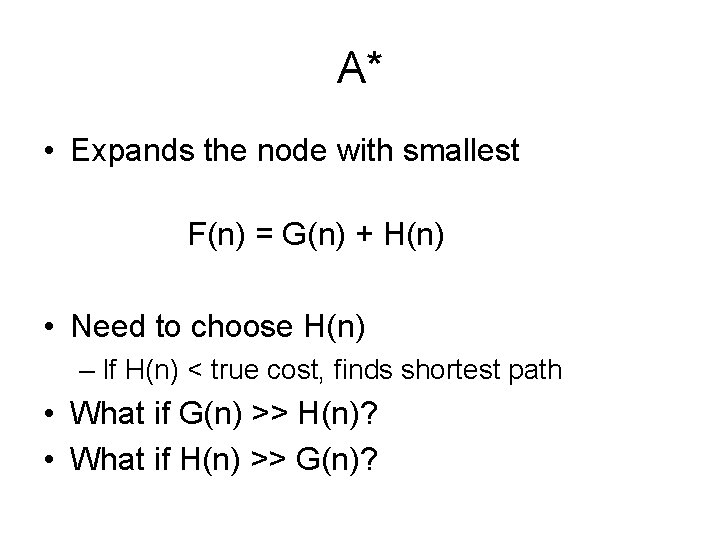
A* • Expands the node with smallest F(n) = G(n) + H(n) • Need to choose H(n) – If H(n) < true cost, finds shortest path • What if G(n) >> H(n)? • What if H(n) >> G(n)?
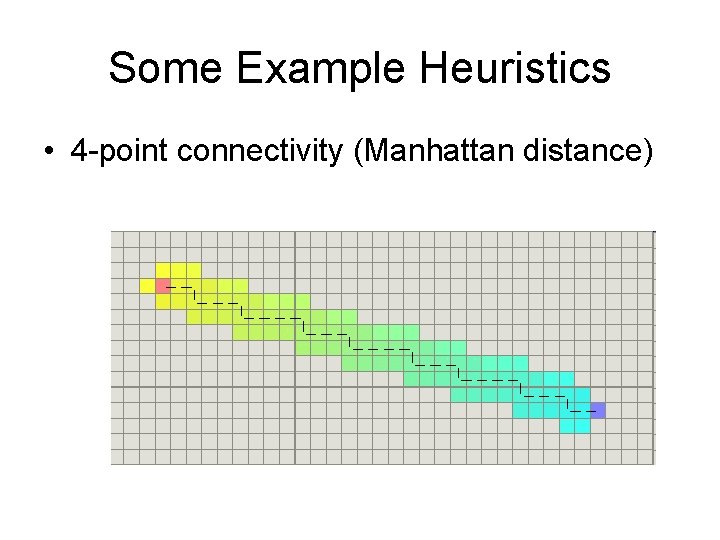
Some Example Heuristics • 4 -point connectivity (Manhattan distance)
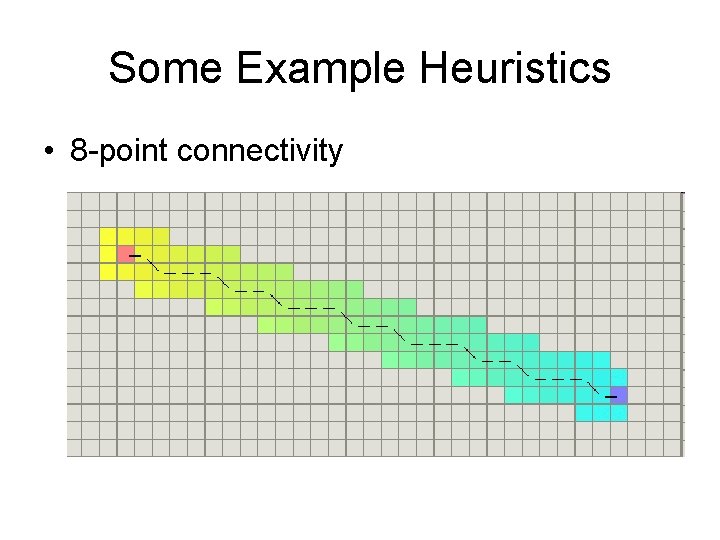
Some Example Heuristics • 8 -point connectivity
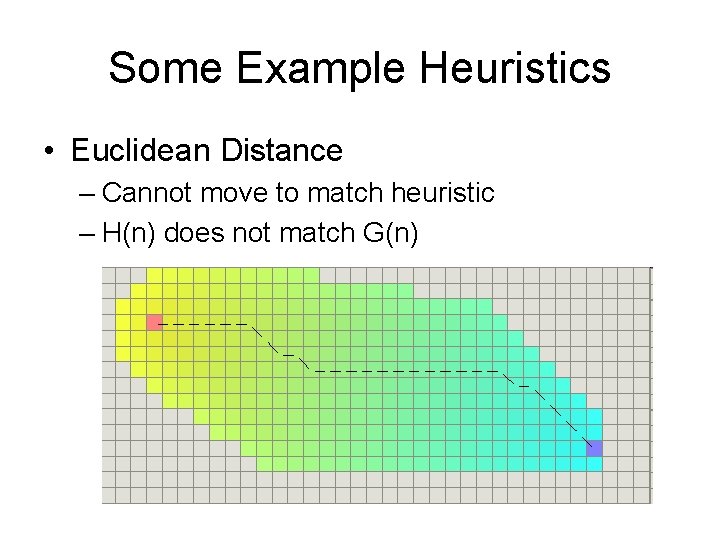
Some Example Heuristics • Euclidean Distance – Cannot move to match heuristic – H(n) does not match G(n)
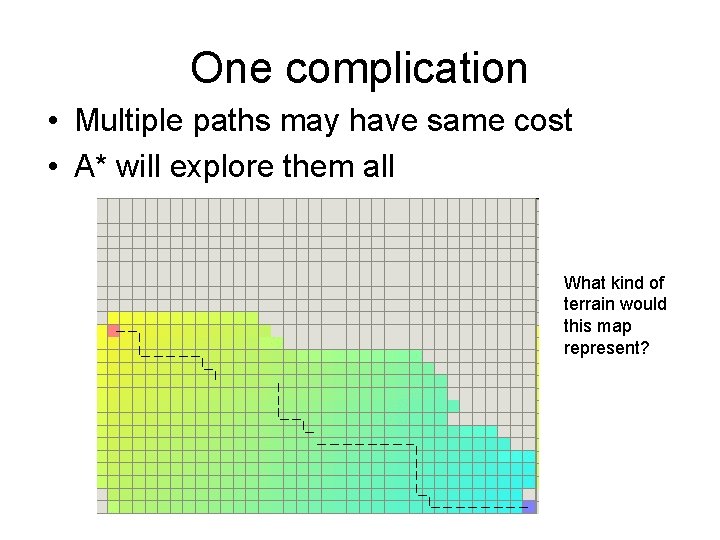
One complication • Multiple paths may have same cost • A* will explore them all What kind of terrain would this map represent?
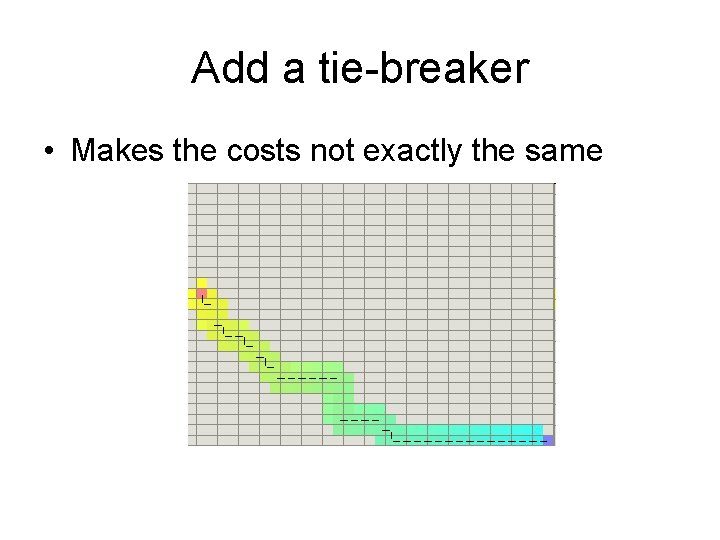
Add a tie-breaker • Makes the costs not exactly the same
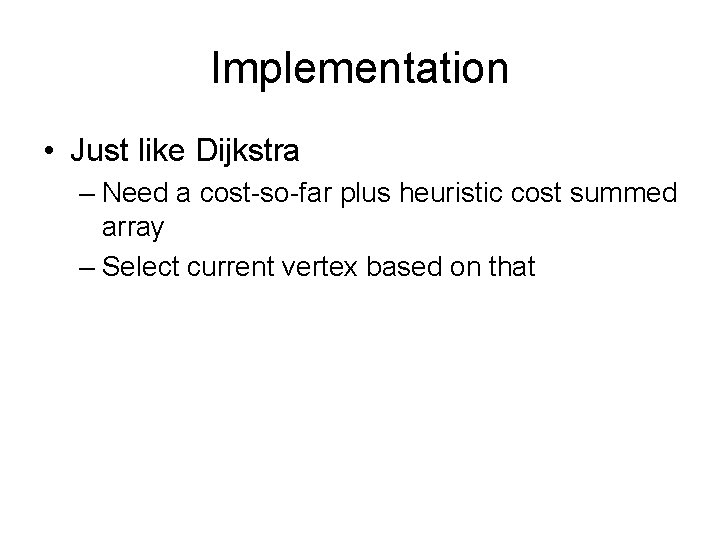
Implementation • Just like Dijkstra – Need a cost-so-far plus heuristic cost summed array – Select current vertex based on that
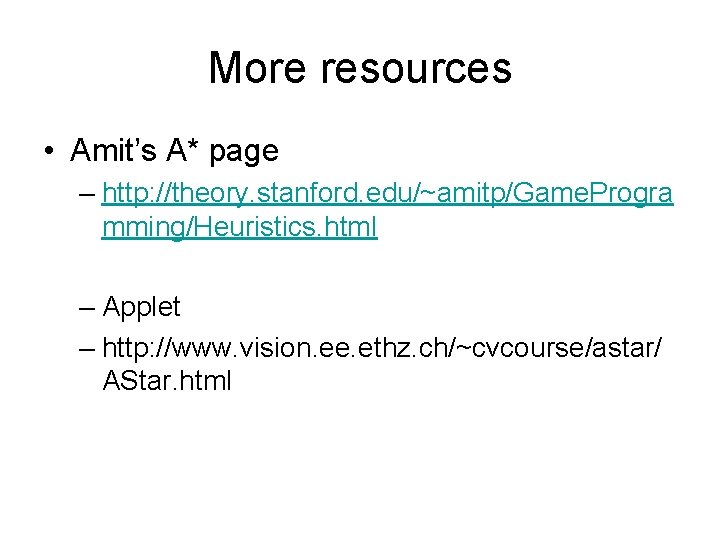
More resources • Amit’s A* page – http: //theory. stanford. edu/~amitp/Game. Progra mming/Heuristics. html – Applet – http: //www. vision. ee. ethz. ch/~cvcourse/astar/ AStar. html
Heuristik
Johnson and johnson strengths and weaknesses
Image search
Johnson and johnson bcg matrix
Johnson and johnson organizational structure
Credo values
Understanding the mirai botnet
Johnson background
Jjeds johnson and johnson
Dreikurs classroom management theory
Johnson and johnson md&d
Search strategy
Heuristic search methods
Informed (heuristic) search strategies
Heuristic search
Heuristic search
How to solve the 9 dot puzzle
Singhal's heuristic algorithm
Singhals heuristic algorithm
Is genetic algorithm heuristic