Decision Making Decision Making Equality and Relational Operators
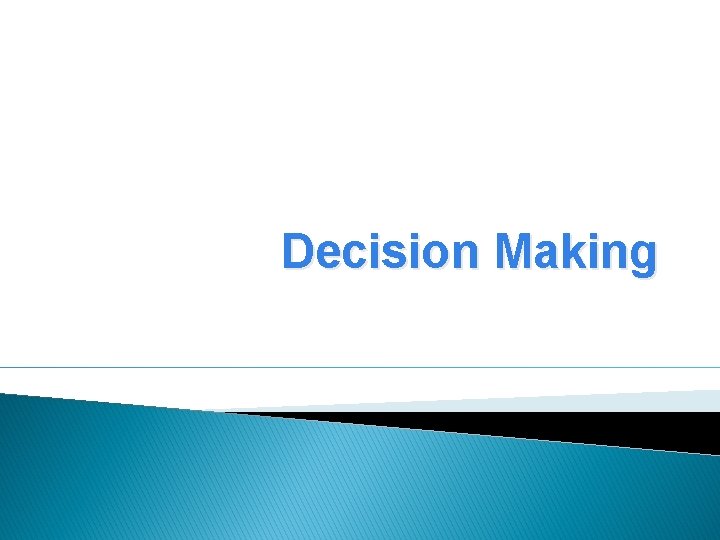
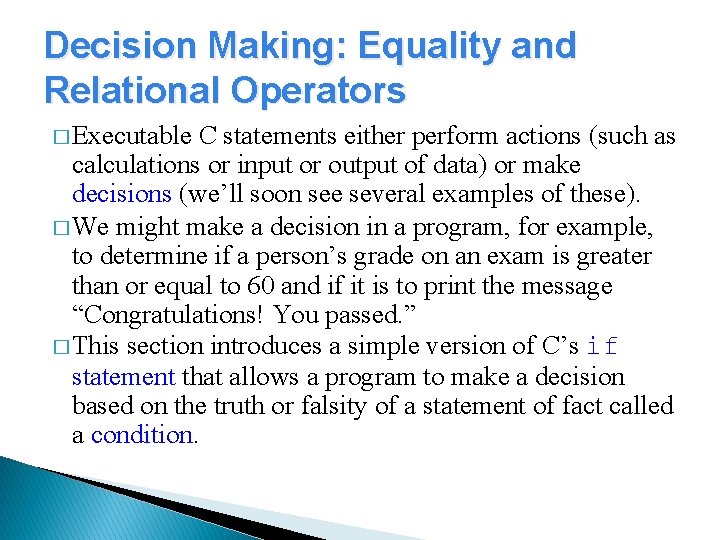
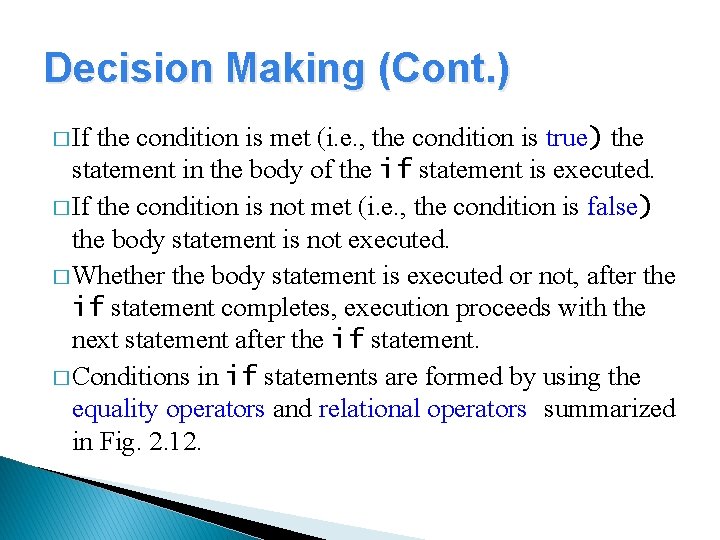
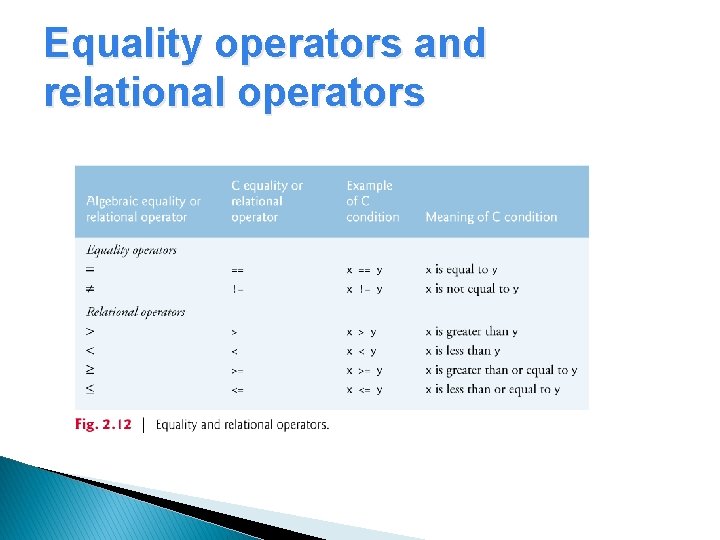
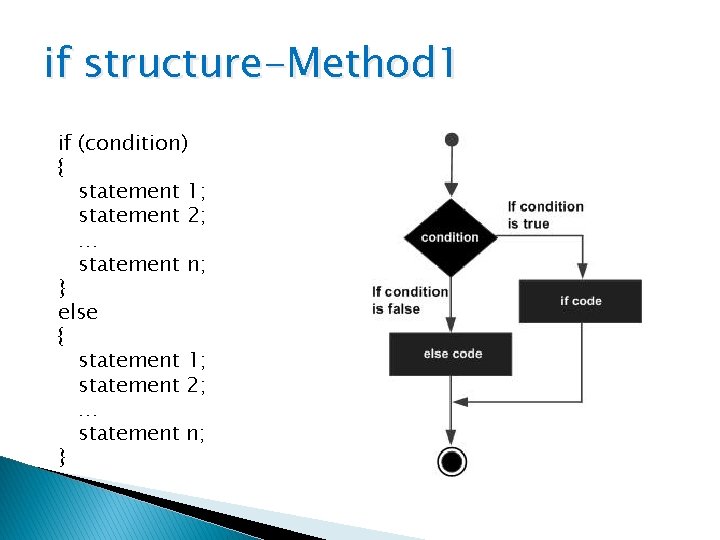
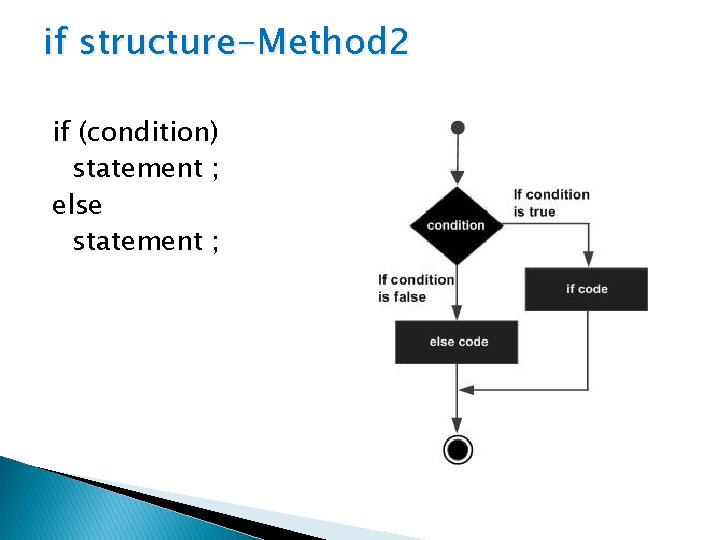
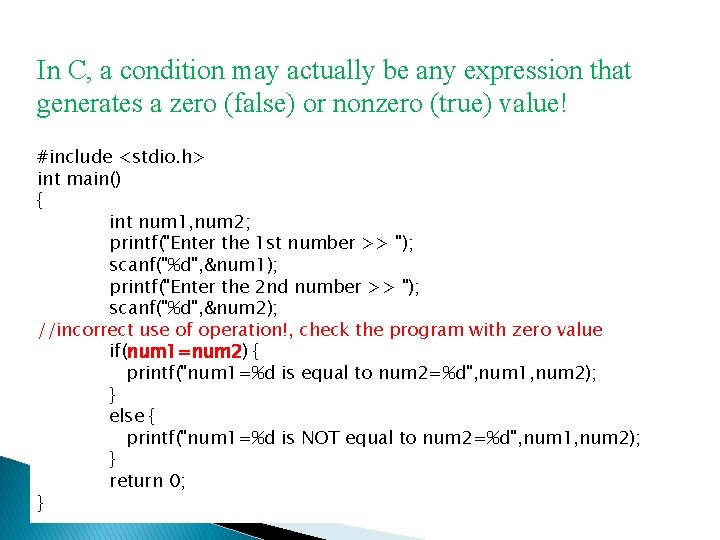
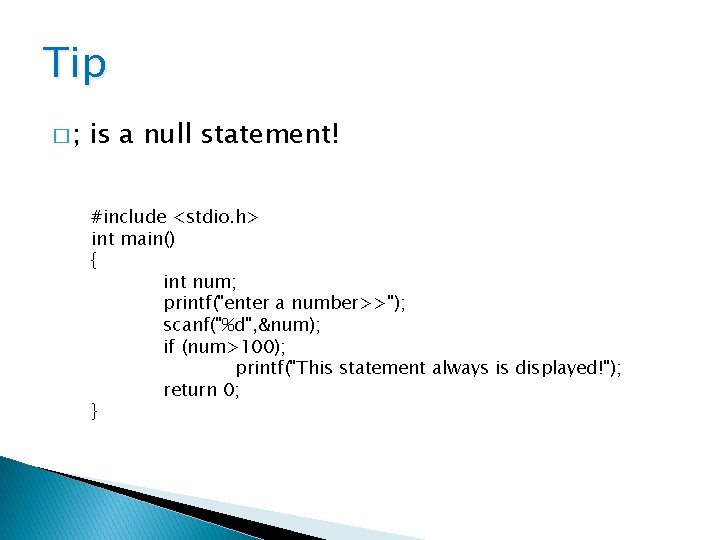
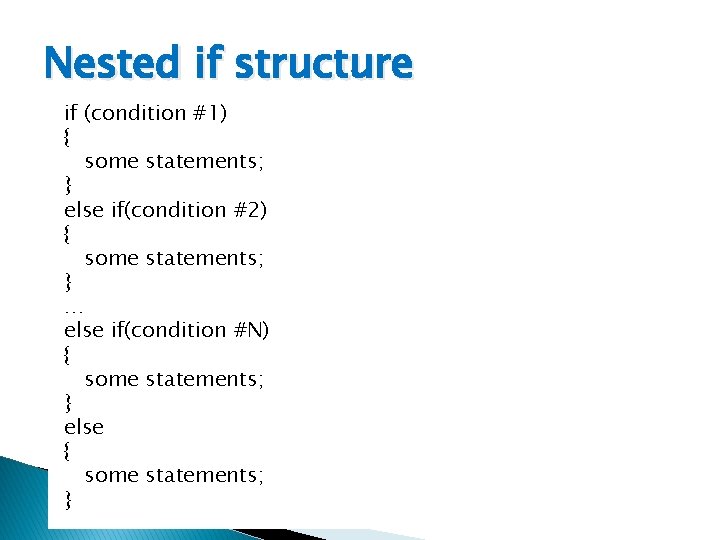
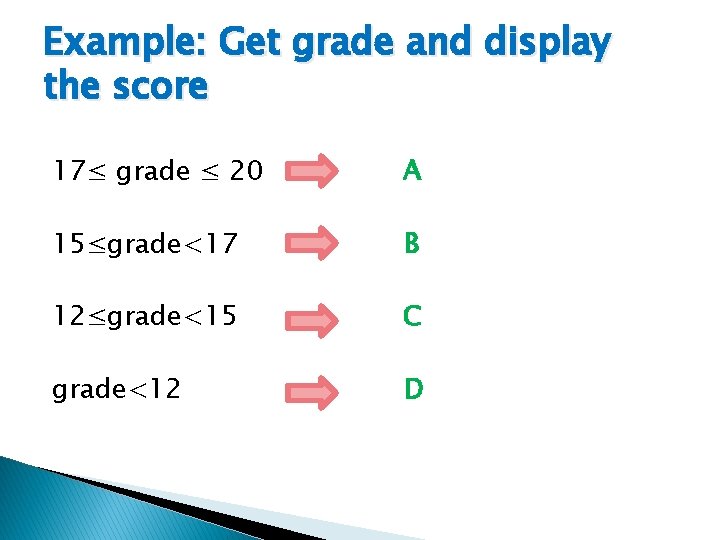
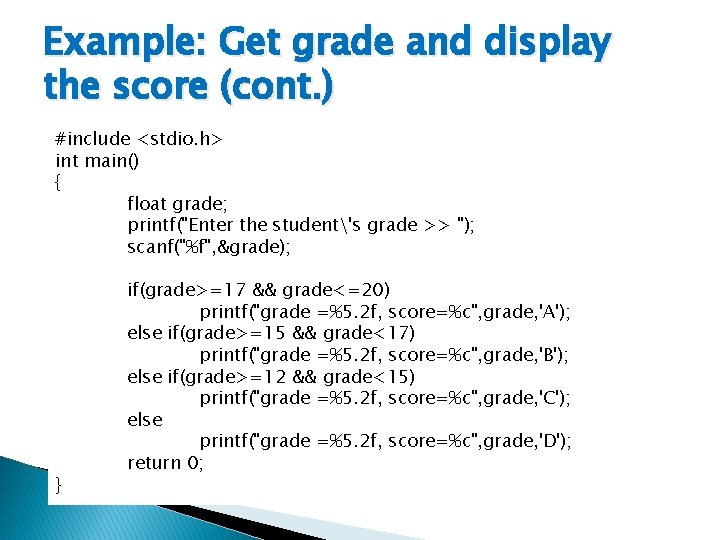
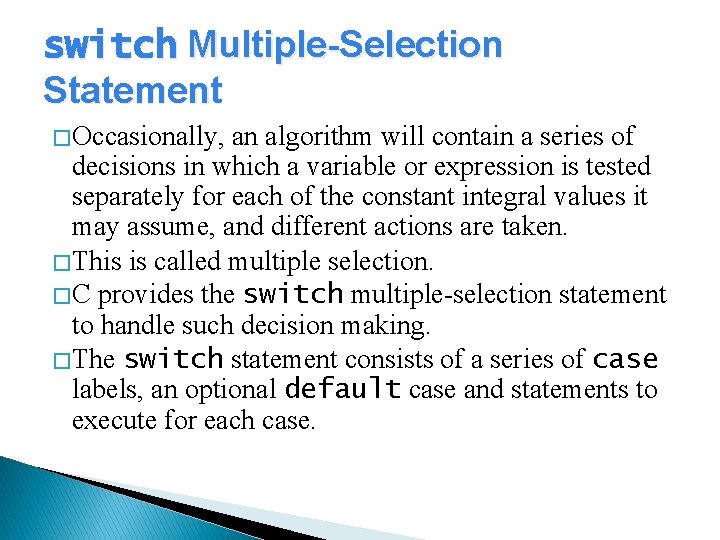
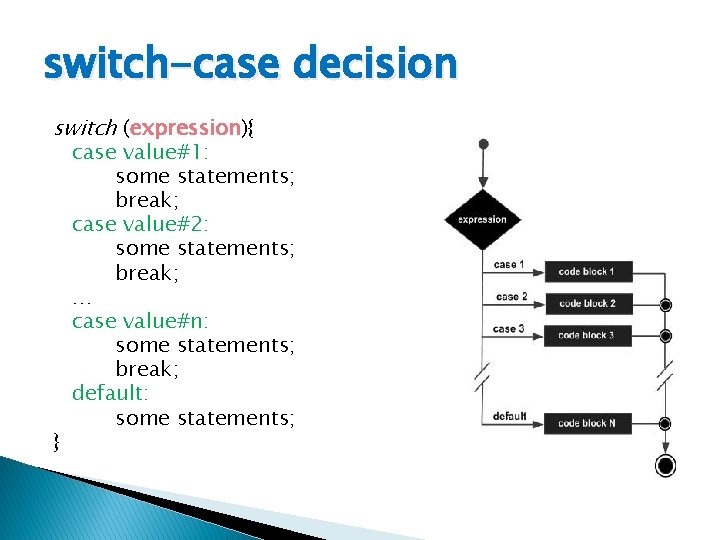
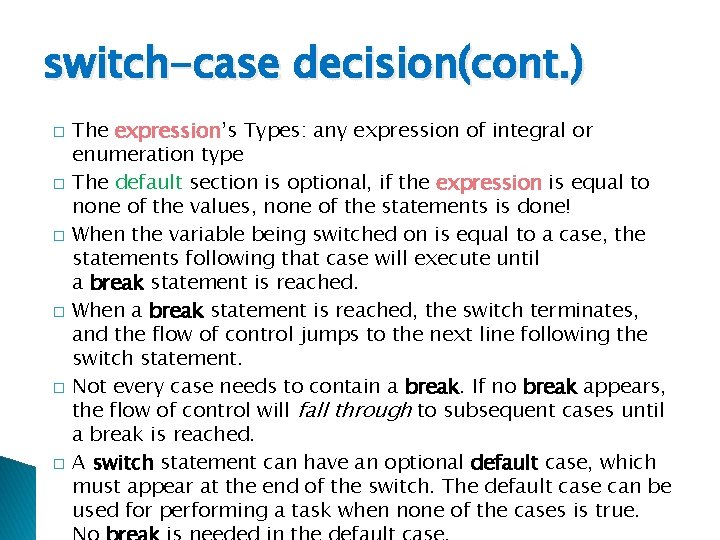
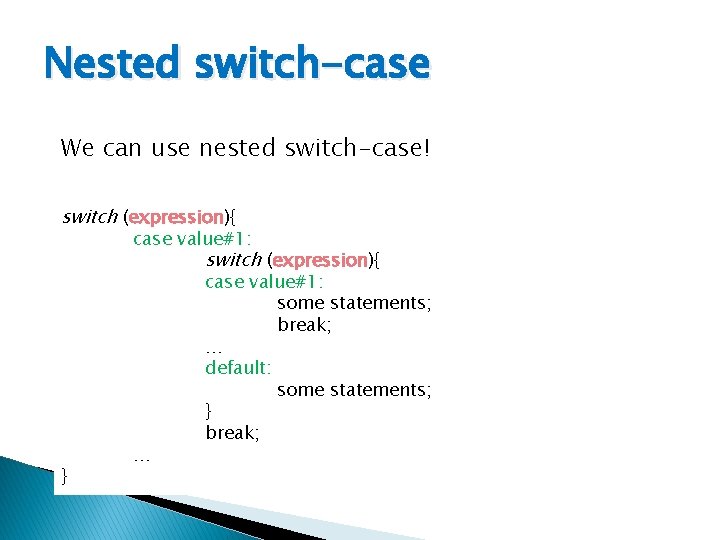
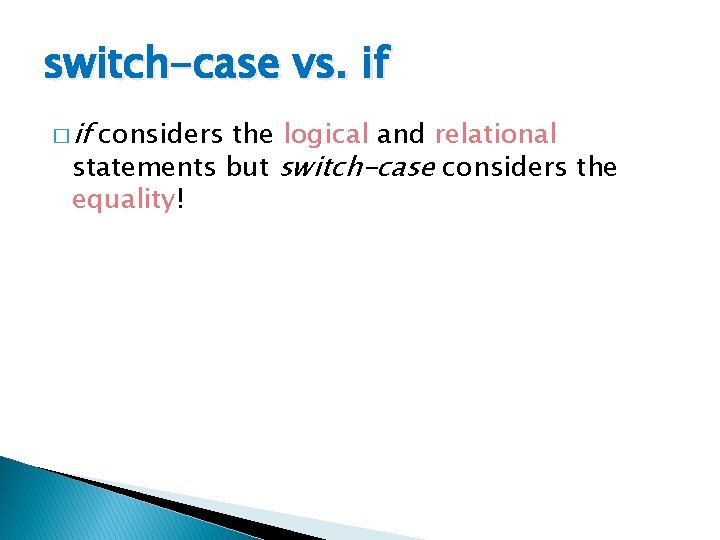
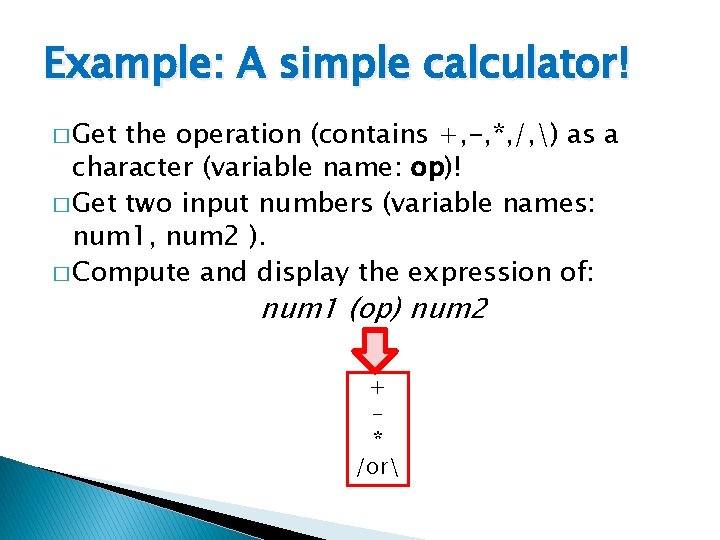
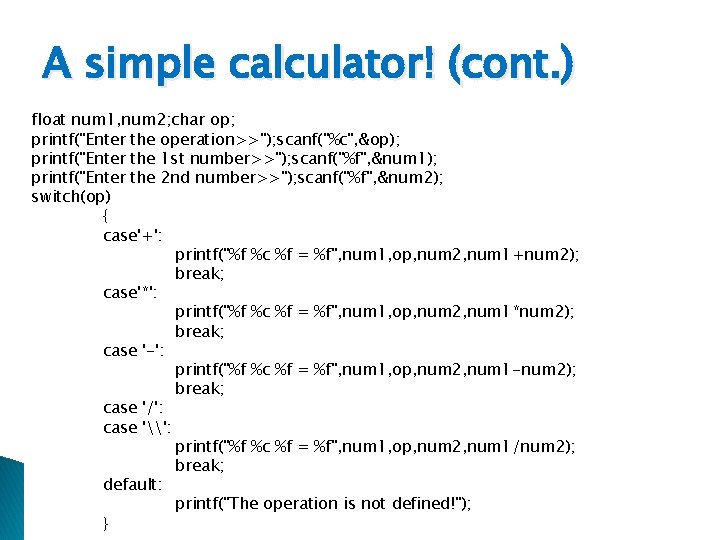
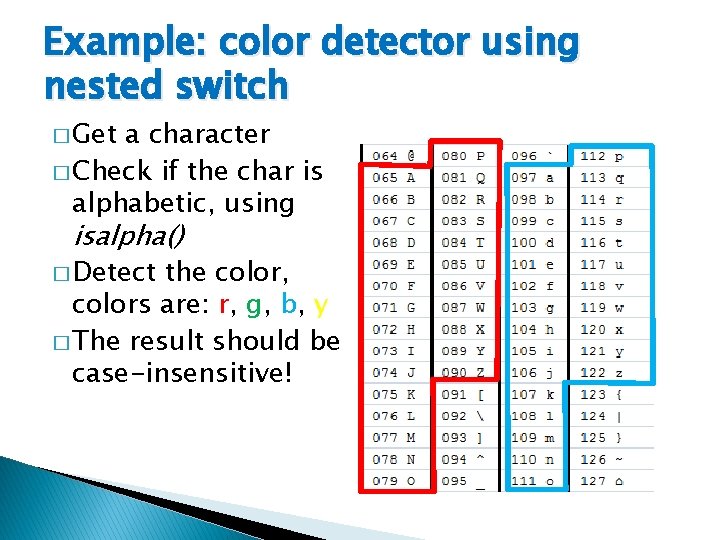
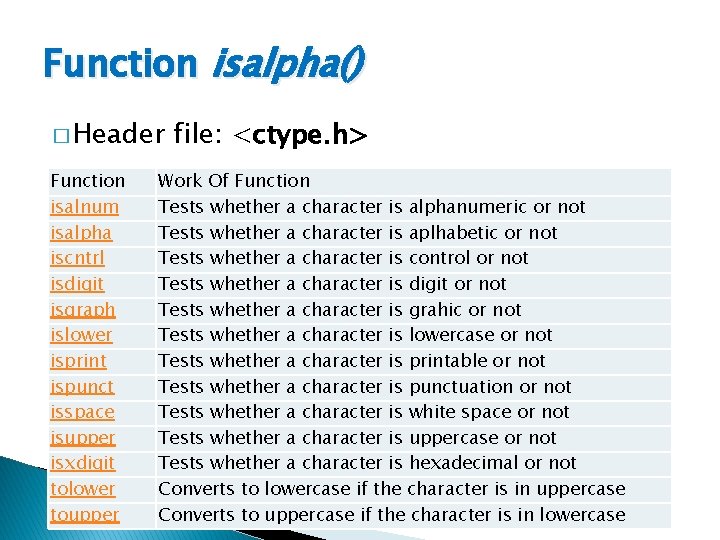
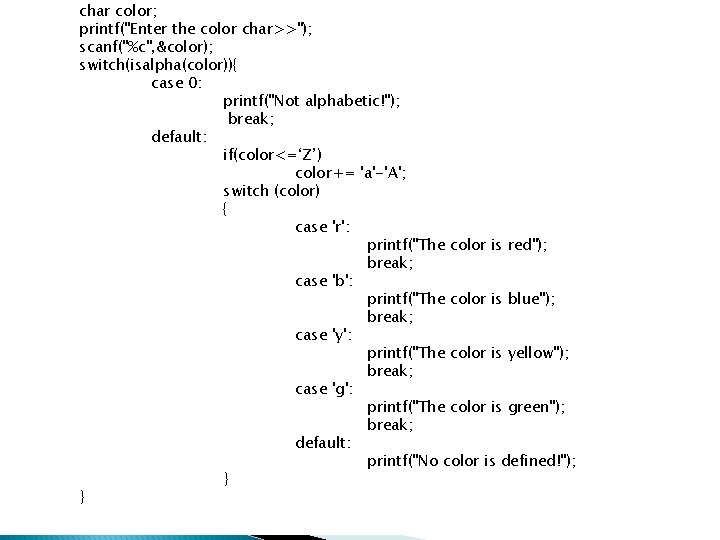
- Slides: 21
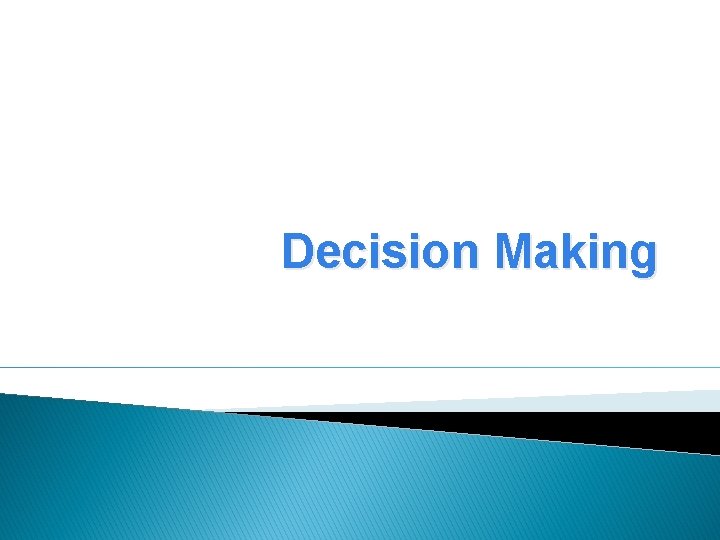
Decision Making
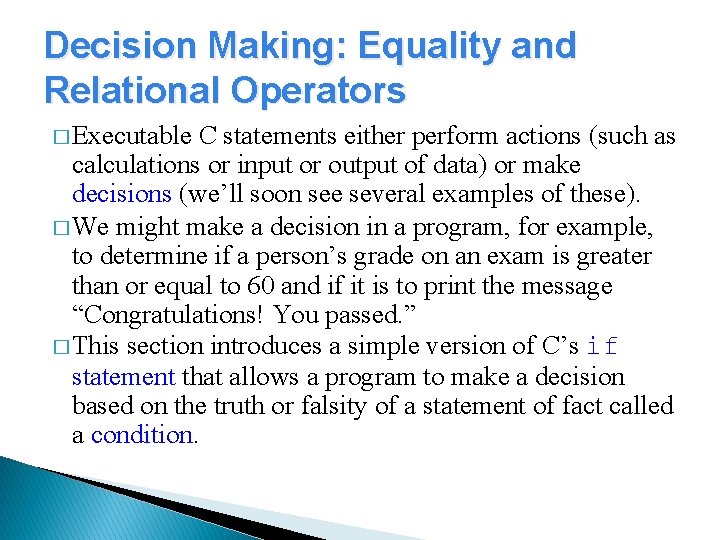
Decision Making: Equality and Relational Operators � Executable C statements either perform actions (such as calculations or input or output of data) or make decisions (we’ll soon see several examples of these). � We might make a decision in a program, for example, to determine if a person’s grade on an exam is greater than or equal to 60 and if it is to print the message “Congratulations! You passed. ” � This section introduces a simple version of C’s if statement that allows a program to make a decision based on the truth or falsity of a statement of fact called a condition.
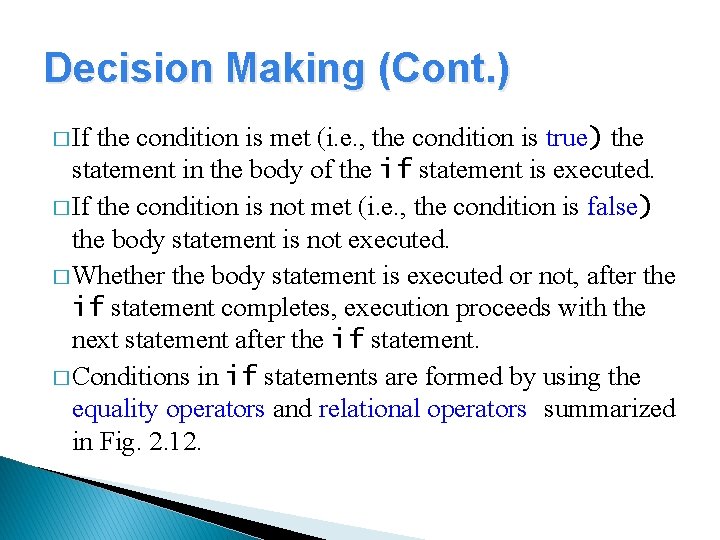
Decision Making (Cont. ) � If the condition is met (i. e. , the condition is true) the statement in the body of the if statement is executed. � If the condition is not met (i. e. , the condition is false) the body statement is not executed. � Whether the body statement is executed or not, after the if statement completes, execution proceeds with the next statement after the if statement. � Conditions in if statements are formed by using the equality operators and relational operators summarized in Fig. 2. 12.
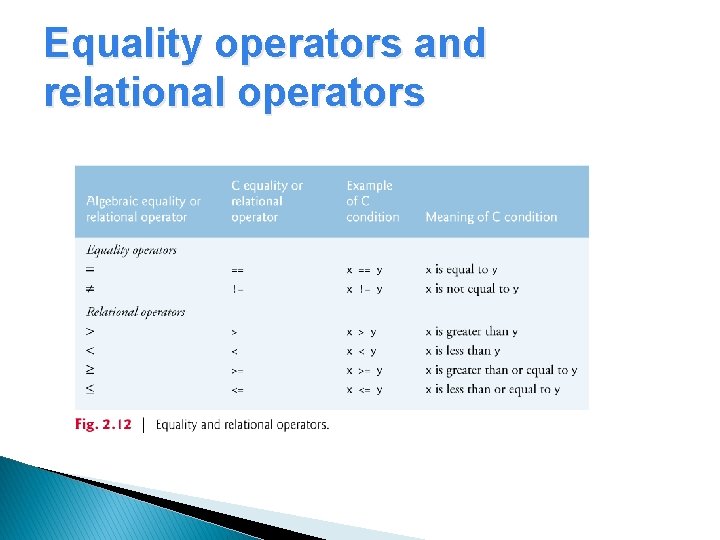
Equality operators and relational operators
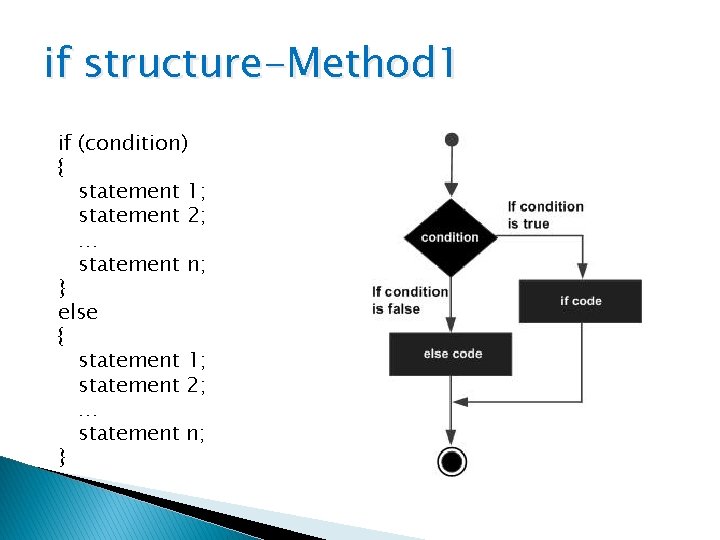
if structure-Method 1 if (condition) { statement 1; statement 2; … statement n; } else { statement 1; statement 2; … statement n; }
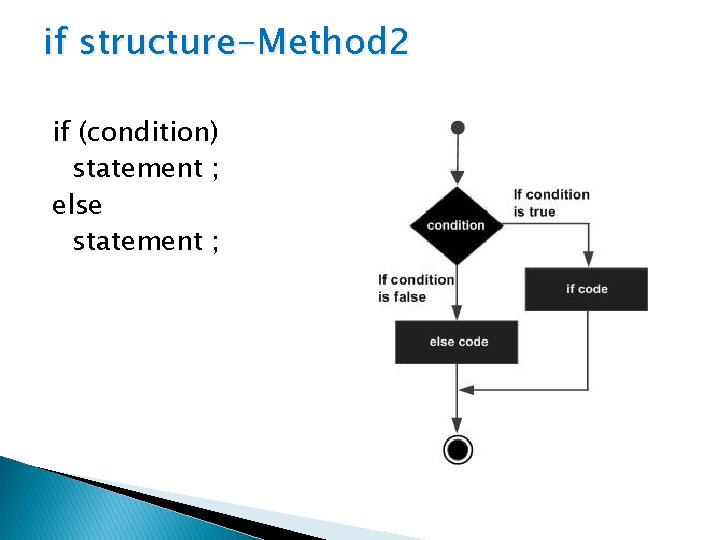
if structure-Method 2 if (condition) statement ; else statement ;
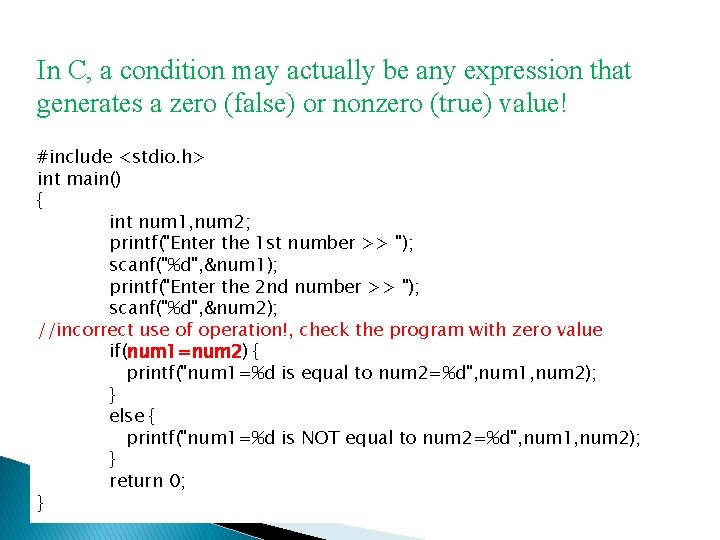
In C, a condition may actually be any expression that generates a zero (false) or nonzero (true) value! #include <stdio. h> int main() { int num 1, num 2; printf("Enter the 1 st number >> "); scanf("%d", &num 1); printf("Enter the 2 nd number >> "); scanf("%d", &num 2); //incorrect use of operation!, check the program with zero value if(num 1=num 2) { printf("num 1=%d is equal to num 2=%d", num 1, num 2); } else { printf("num 1=%d is NOT equal to num 2=%d", num 1, num 2); } return 0; }
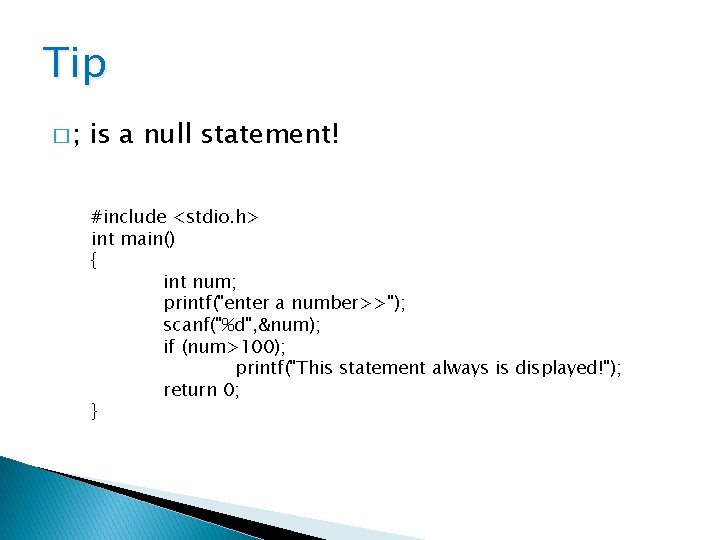
Tip �; is a null statement! #include <stdio. h> int main() { int num; printf("enter a number>>"); scanf("%d", &num); if (num>100); printf("This statement always is displayed!"); return 0; }
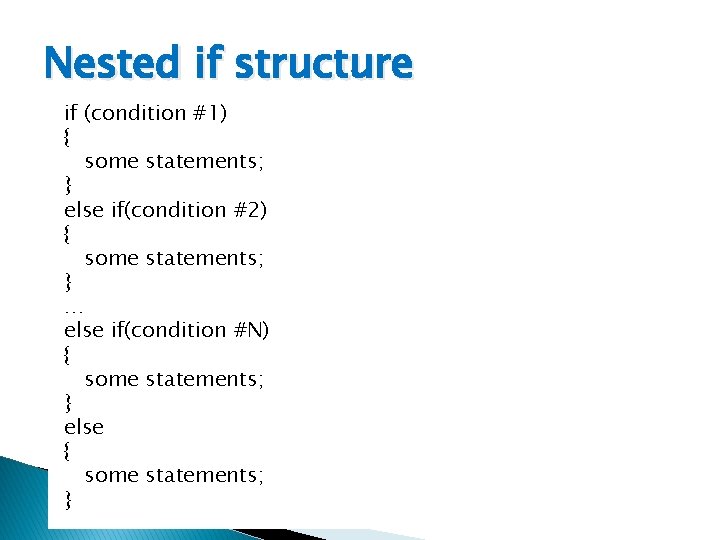
Nested if structure if (condition #1) { some statements; } else if(condition #2) { some statements; } … else if(condition #N) { some statements; } else { some statements; }
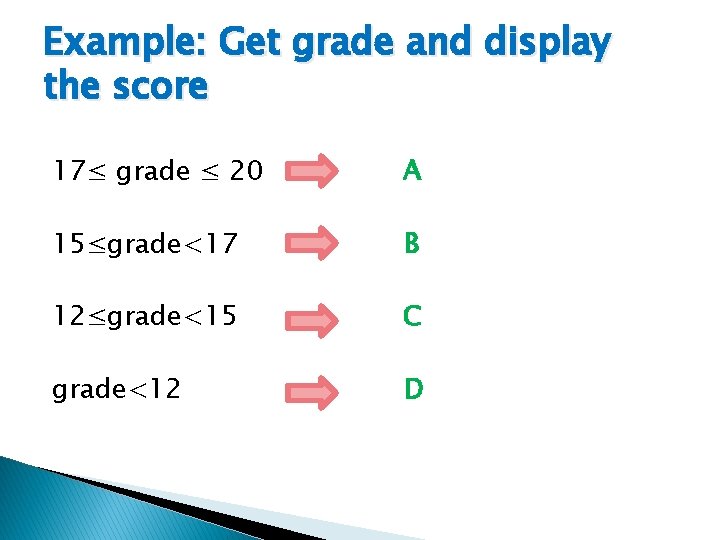
Example: Get grade and display the score 17≤ grade ≤ 20 A 15≤grade<17 B 12≤grade<15 C grade<12 D
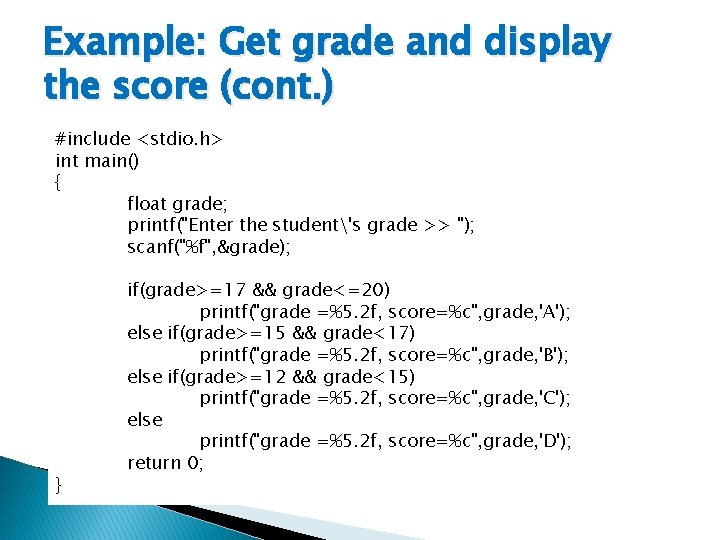
Example: Get grade and display the score (cont. ) #include <stdio. h> int main() { float grade; printf("Enter the student's grade >> "); scanf("%f", &grade); } if(grade>=17 && grade<=20) printf("grade =%5. 2 f, score=%c", grade, 'A'); else if(grade>=15 && grade<17) printf("grade =%5. 2 f, score=%c", grade, 'B'); else if(grade>=12 && grade<15) printf("grade =%5. 2 f, score=%c", grade, 'C'); else printf("grade =%5. 2 f, score=%c", grade, 'D'); return 0;
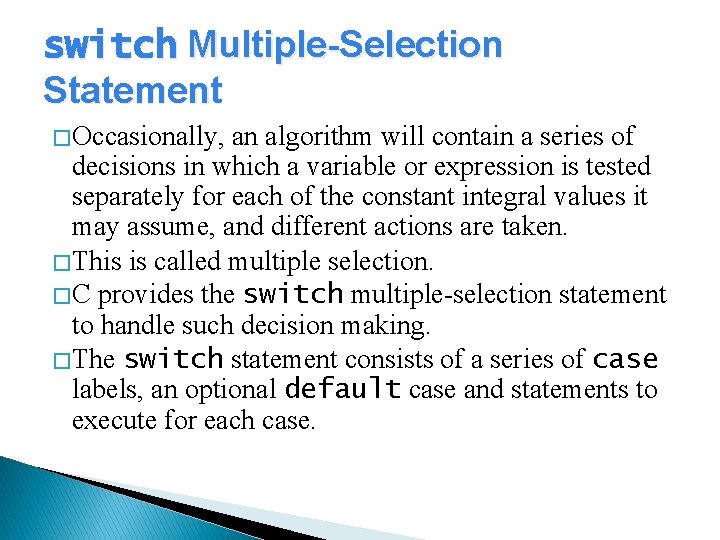
switch Multiple-Selection Statement � Occasionally, an algorithm will contain a series of decisions in which a variable or expression is tested separately for each of the constant integral values it may assume, and different actions are taken. � This is called multiple selection. � C provides the switch multiple-selection statement to handle such decision making. � The switch statement consists of a series of case labels, an optional default case and statements to execute for each case.
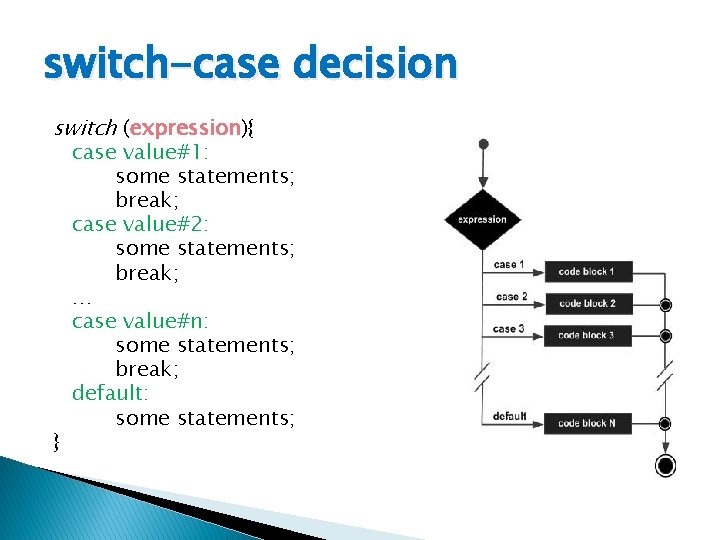
switch-case decision switch (expression){ } case value#1: some statements; break; case value#2: some statements; break; … case value#n: some statements; break; default: some statements;
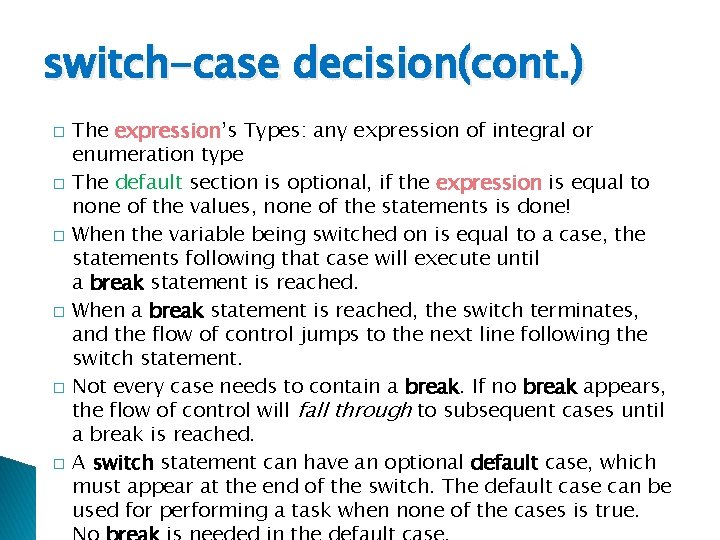
switch-case decision(cont. ) � � � The expression’s Types: any expression of integral or enumeration type The default section is optional, if the expression is equal to none of the values, none of the statements is done! When the variable being switched on is equal to a case, the statements following that case will execute until a break statement is reached. When a break statement is reached, the switch terminates, and the flow of control jumps to the next line following the switch statement. Not every case needs to contain a break. If no break appears, the flow of control will fall through to subsequent cases until a break is reached. A switch statement can have an optional default case, which must appear at the end of the switch. The default case can be used for performing a task when none of the cases is true.
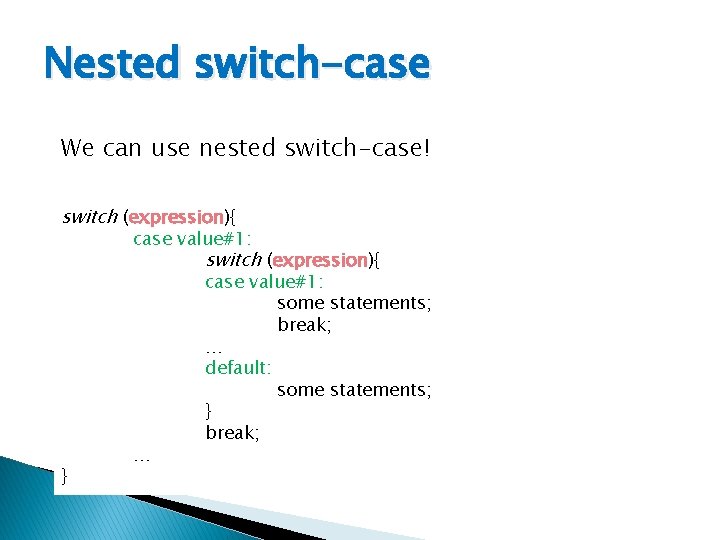
Nested switch-case We can use nested switch-case! switch (expression){ case value#1: switch (expression){ } … case value#1: some statements; break; … default: some statements; } break;
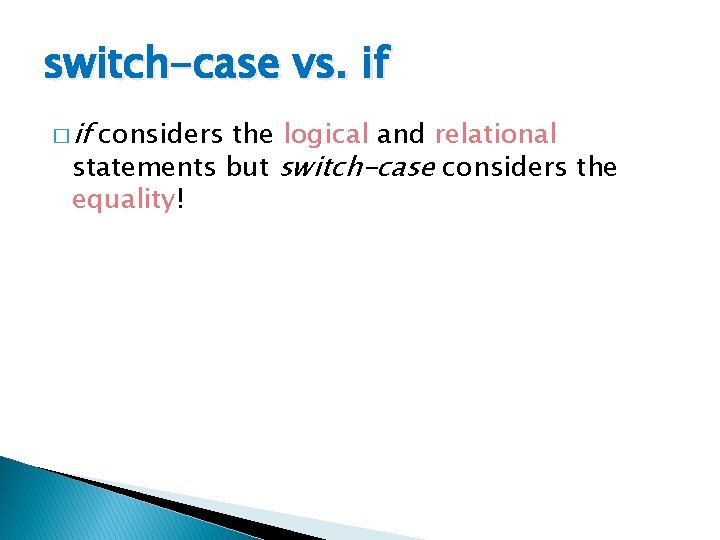
switch-case vs. if � if considers the logical and relational statements but switch-case considers the equality!
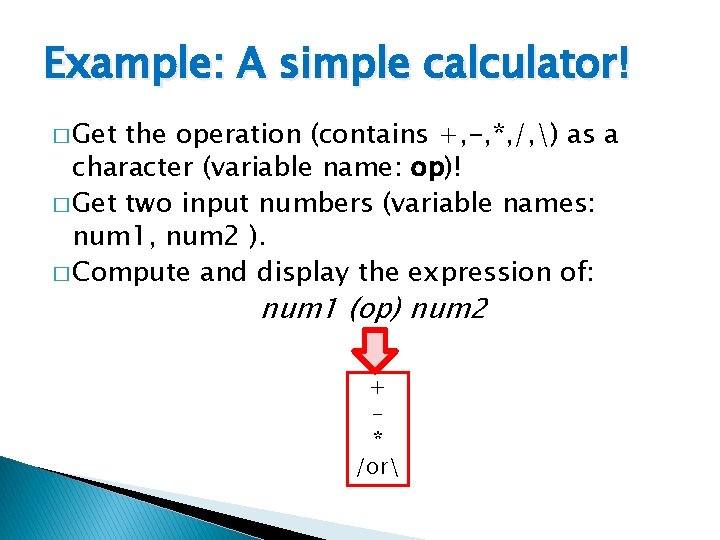
Example: A simple calculator! � Get the operation (contains +, -, *, /, ) as a character (variable name: op)! � Get two input numbers (variable names: num 1, num 2 ). � Compute and display the expression of: num 1 (op) num 2 + * /or
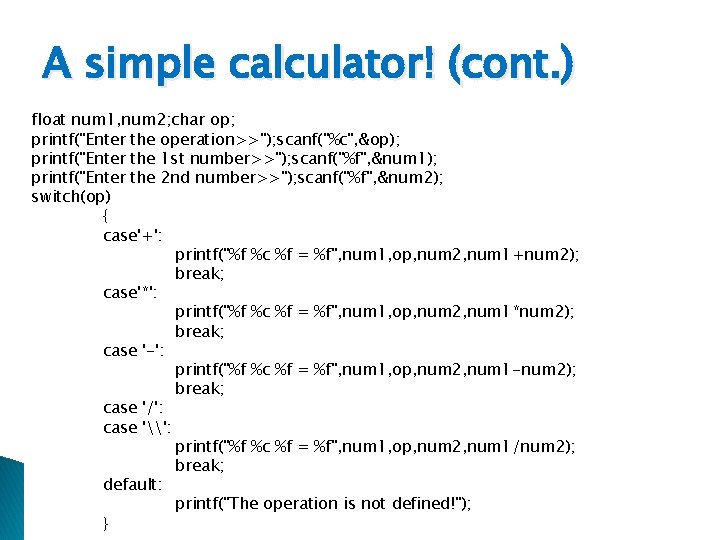
A simple calculator! (cont. ) float num 1, num 2; char op; printf("Enter the operation>>"); scanf("%c", &op); printf("Enter the 1 st number>>"); scanf("%f", &num 1); printf("Enter the 2 nd number>>"); scanf("%f", &num 2); switch(op) { case'+': printf("%f %c %f = %f", num 1, op, num 2, num 1+num 2); break; case'*': printf("%f %c %f = %f", num 1, op, num 2, num 1*num 2); break; case '-': printf("%f %c %f = %f", num 1, op, num 2, num 1 -num 2); break; case '/': case '\': printf("%f %c %f = %f", num 1, op, num 2, num 1/num 2); break; default: printf("The operation is not defined!"); }
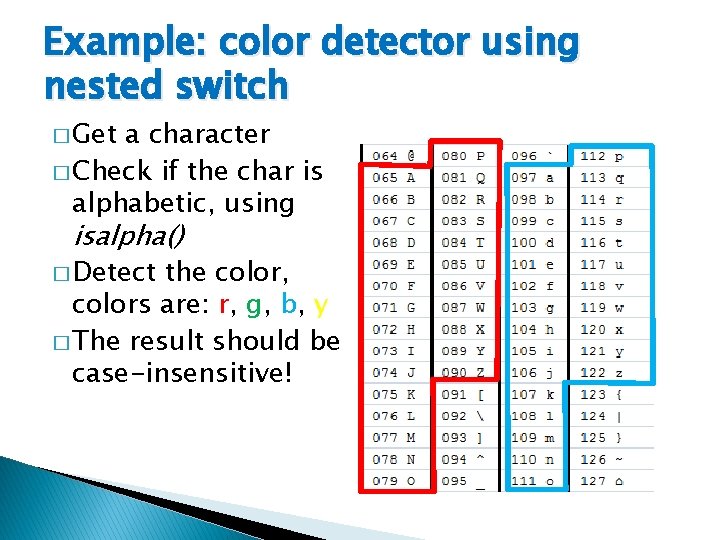
Example: color detector using nested switch � Get a character � Check if the char is alphabetic, using isalpha() � Detect the color, colors are: r, g, b, y � The result should be case-insensitive!
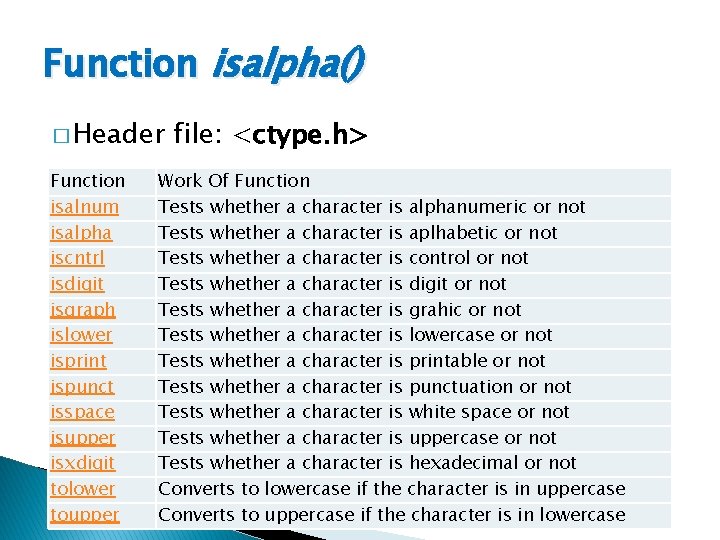
Function isalpha() � Header Function isalnum isalpha iscntrl isdigit isgraph islower isprint ispunct isspace isupper isxdigit tolower toupper file: <ctype. h> Work Of Function Tests whether a character is alphanumeric or not Tests whether a character is aplhabetic or not Tests whether a character is control or not Tests whether a character is digit or not Tests whether a character is grahic or not Tests whether a character is lowercase or not Tests whether a character is printable or not Tests whether a character is punctuation or not Tests whether a character is white space or not Tests whether a character is uppercase or not Tests whether a character is hexadecimal or not Converts to lowercase if the character is in uppercase Converts to uppercase if the character is in lowercase
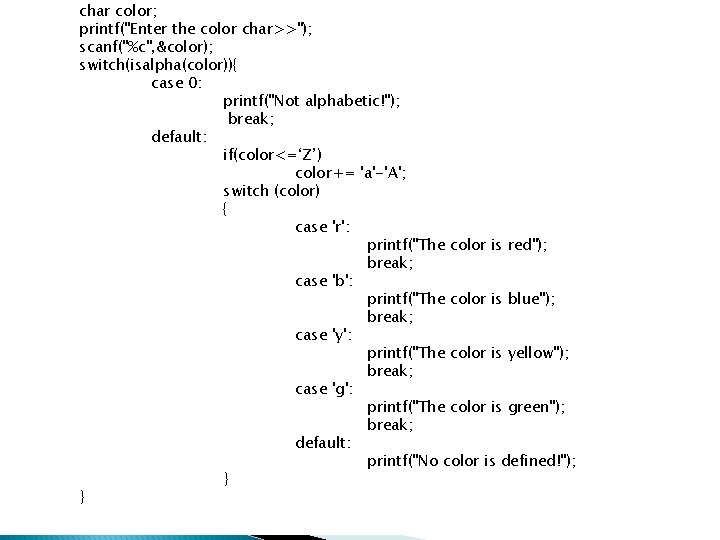
char color; printf("Enter the color char>>"); scanf("%c", &color); switch(isalpha(color)){ case 0: printf("Not alphabetic!"); break; default: if(color<=‘Z’) color+= 'a'-'A'; switch (color) { case 'r': printf("The color is red"); break; case 'b': printf("The color is blue"); break; case 'y': printf("The color is yellow"); break; case 'g': printf("The color is green"); break; default: printf("No color is defined!"); } }
Relational operators
Relational operators matlab
Relational operators java
Transition diagram for relational operators
Relational operators in r
Convert nfa to dfa
Relational algebra and relational calculus
Relational calculus
Relational algebra aggregate functions examples
Object relational and extended relational databases
Relational algebra is a procedural language
Formal equality vs substantive equality
Cedaw article
No decision snap decision responsible decision
Slidetodoc.com
Decision table and decision tree examples
Using recursion in models and decision making
Using functions in models and decision making
Chapter 6 prices and decision making assessment answers
Hoy and tarter decision making model
Decision making and relevant information
Best books on problem solving and decision making