Day 3 Lesson 10 Iteration Loops Python MiniCourse
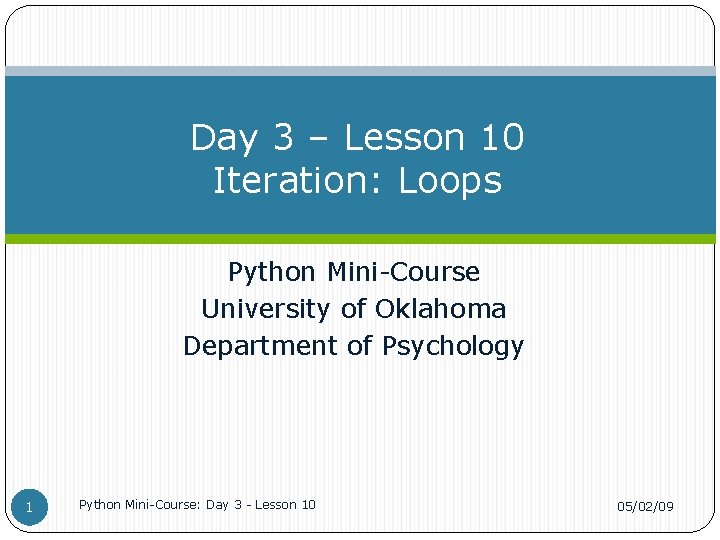
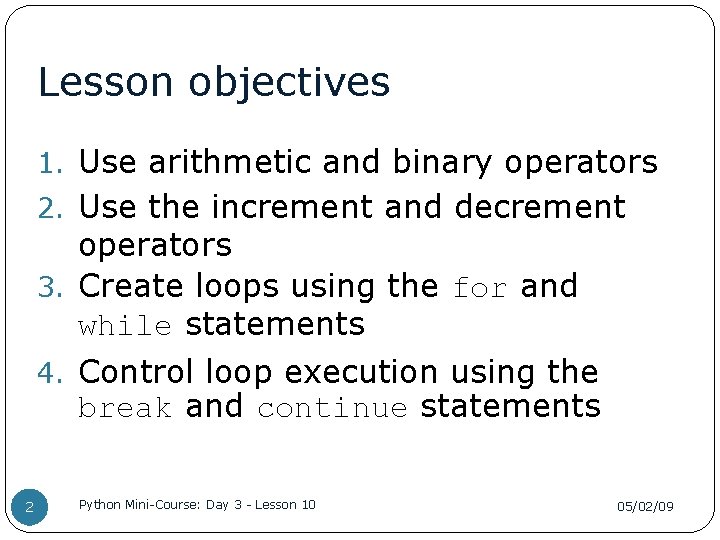
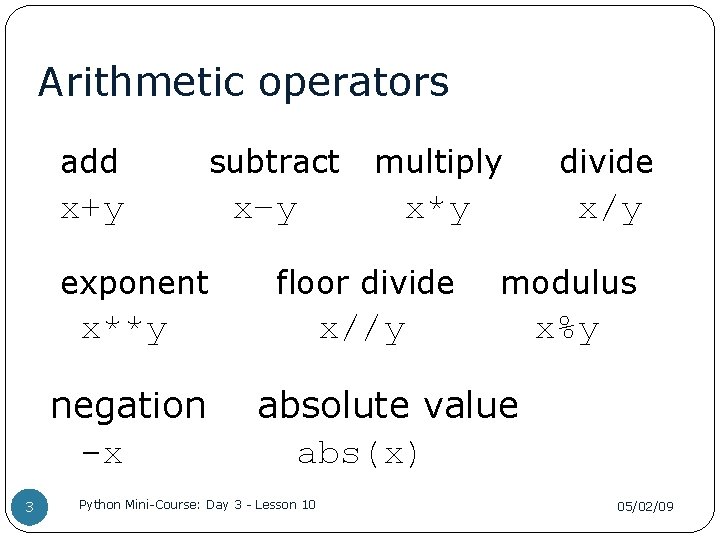
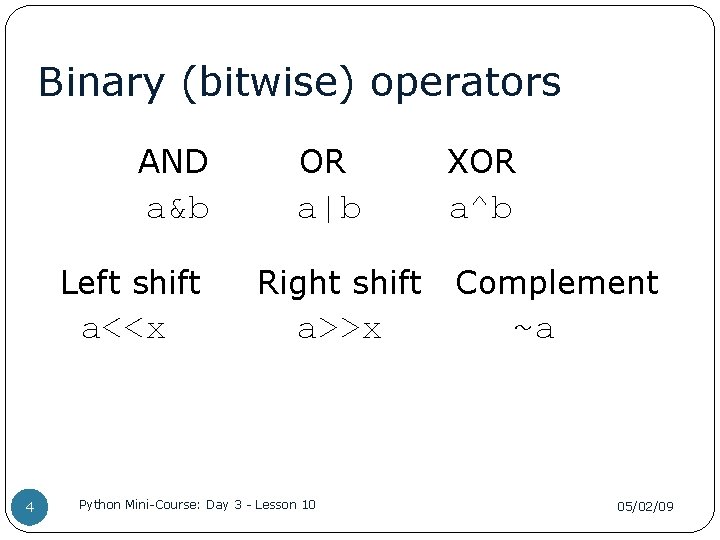
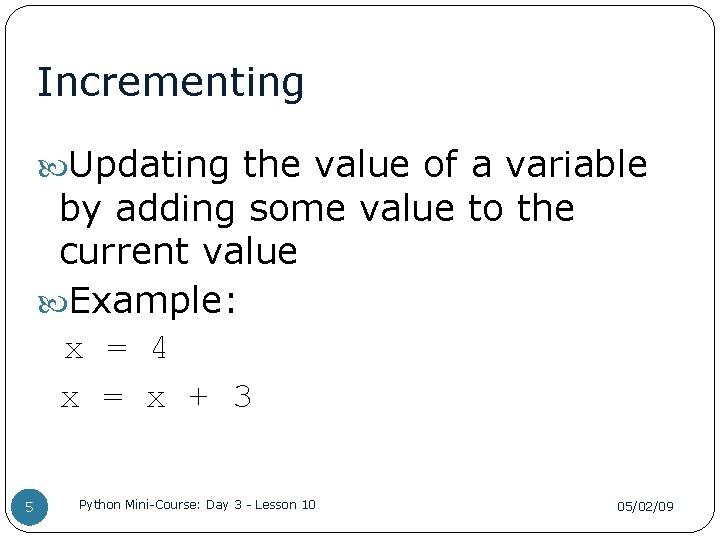
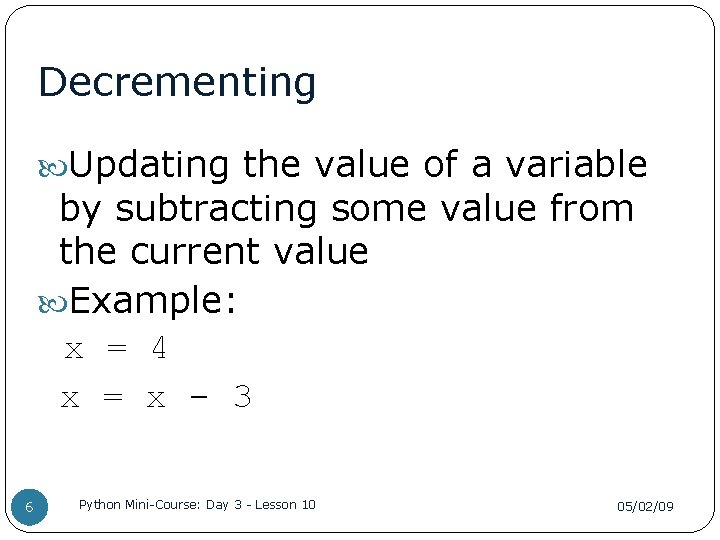
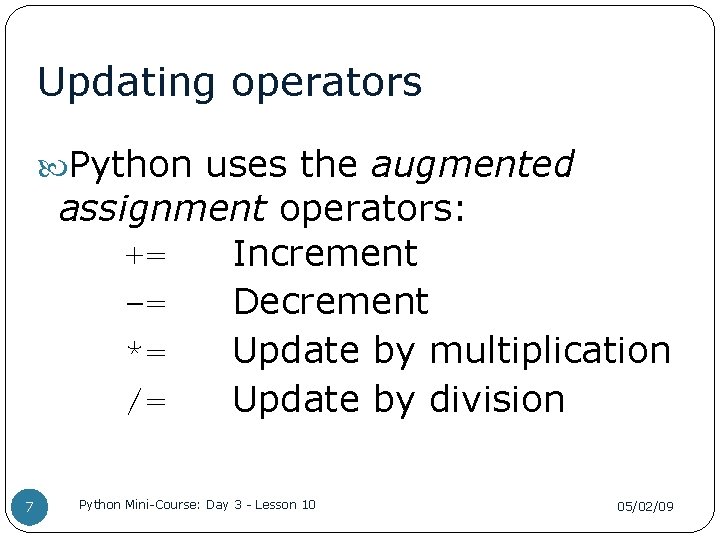
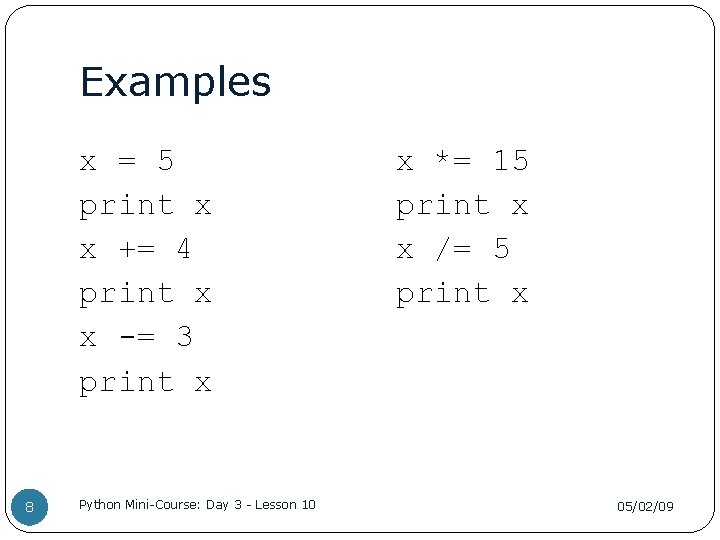
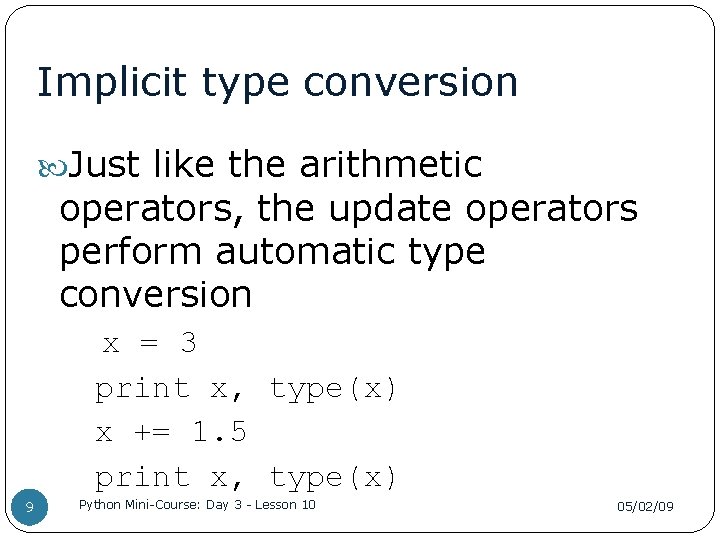
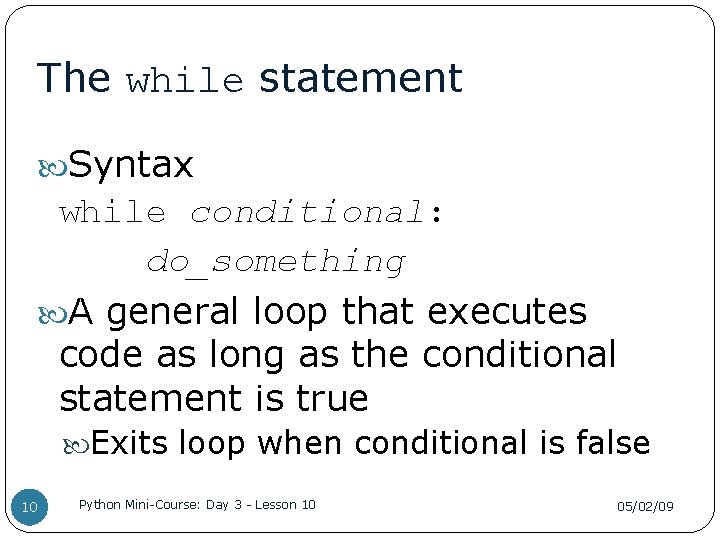
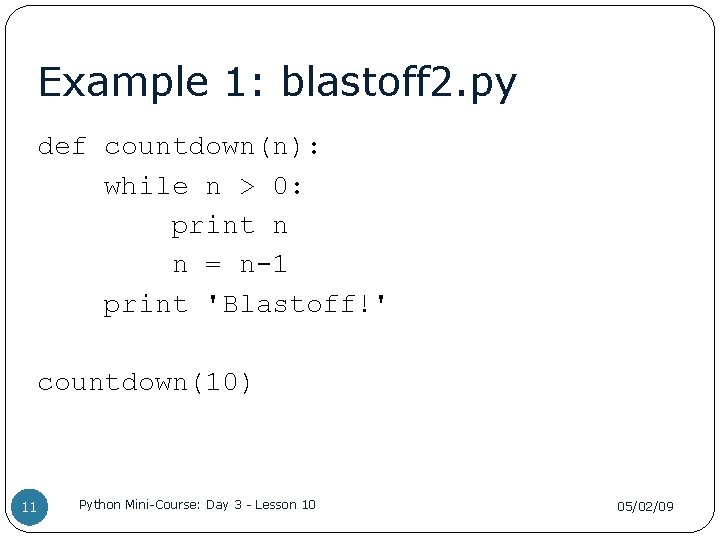
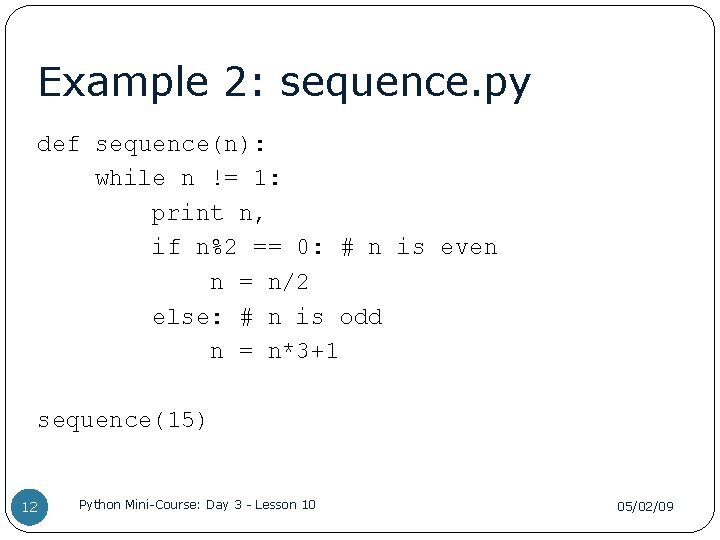
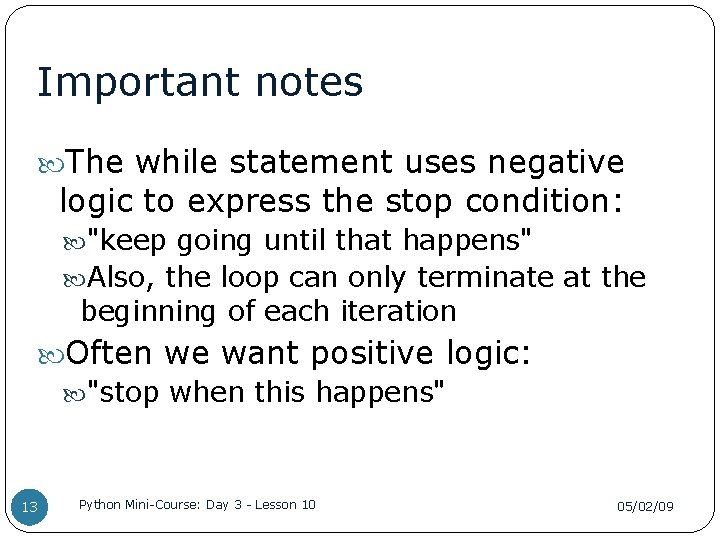
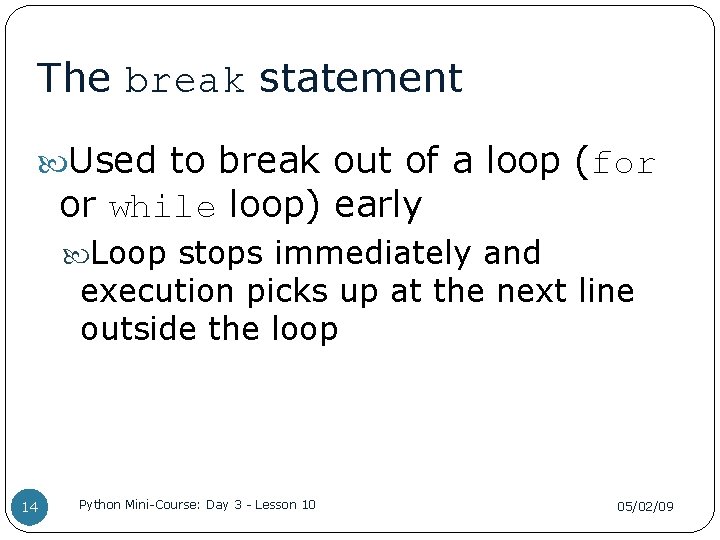
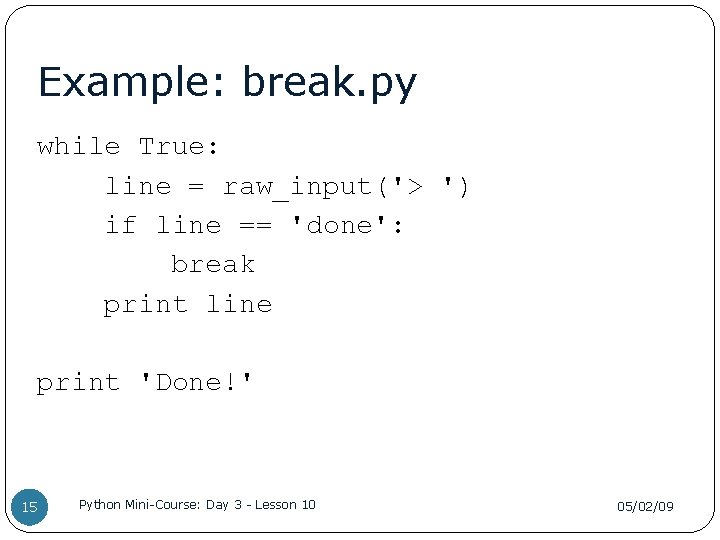
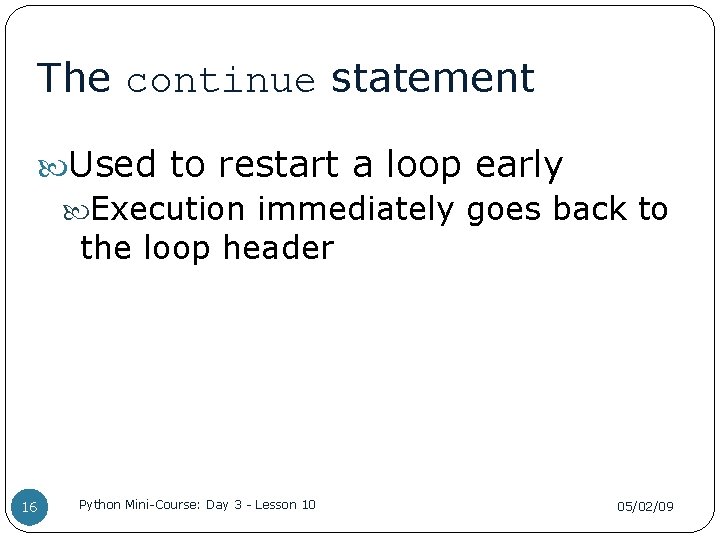
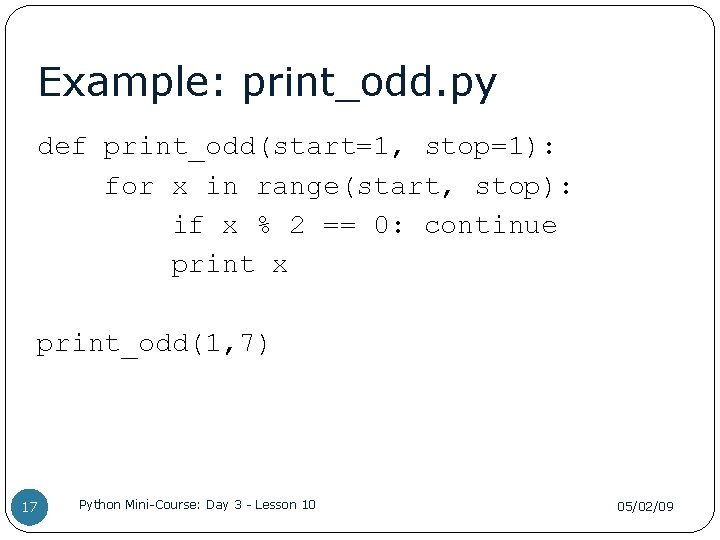
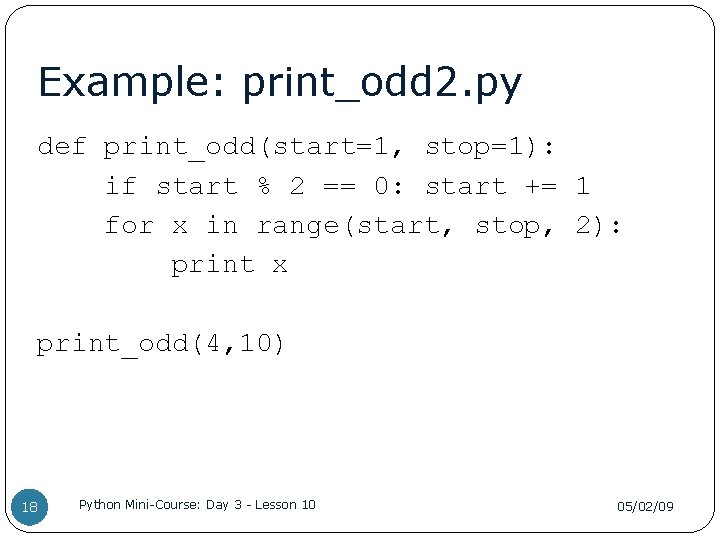
- Slides: 18
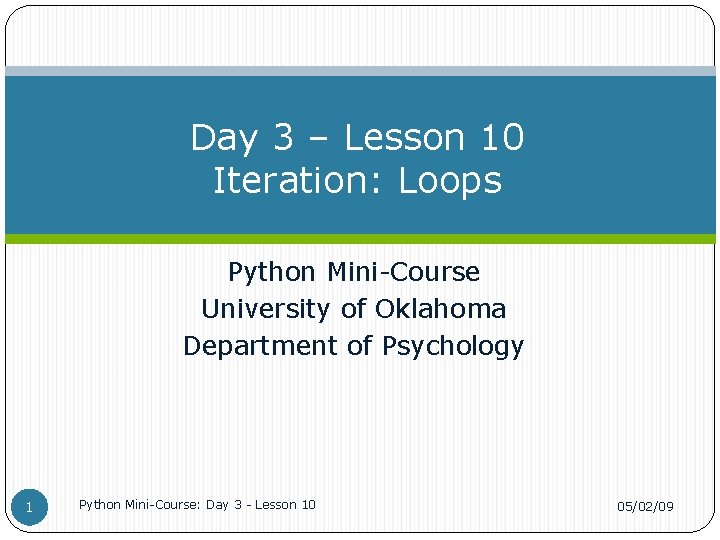
Day 3 – Lesson 10 Iteration: Loops Python Mini-Course University of Oklahoma Department of Psychology 1 Python Mini-Course: Day 3 - Lesson 10 05/02/09
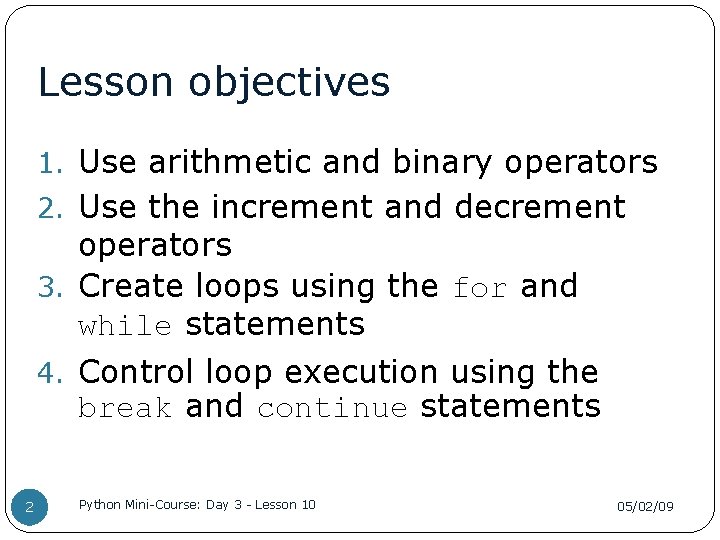
Lesson objectives 1. Use arithmetic and binary operators 2. Use the increment and decrement operators 3. Create loops using the for and while statements 4. Control loop execution using the break and continue statements 2 Python Mini-Course: Day 3 - Lesson 10 05/02/09
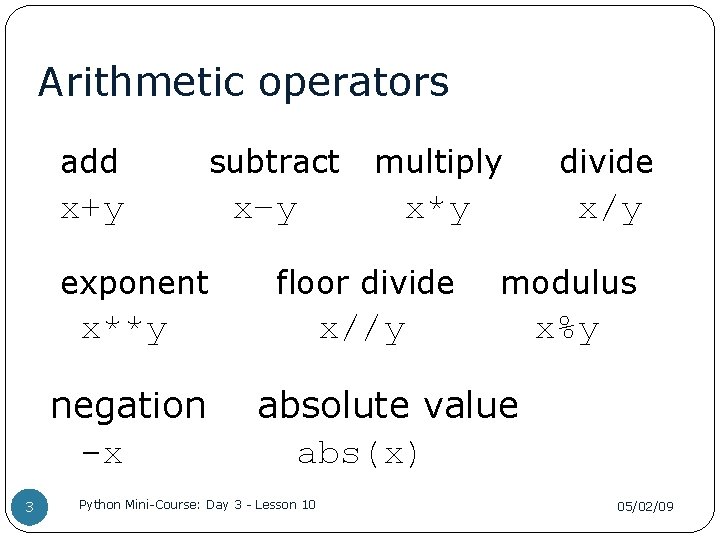
Arithmetic operators add subtract x+y exponent 3 divide x*y x/y x–y floor divide modulus x//y x%y x**y negation -x multiply absolute value abs(x) Python Mini-Course: Day 3 - Lesson 10 05/02/09
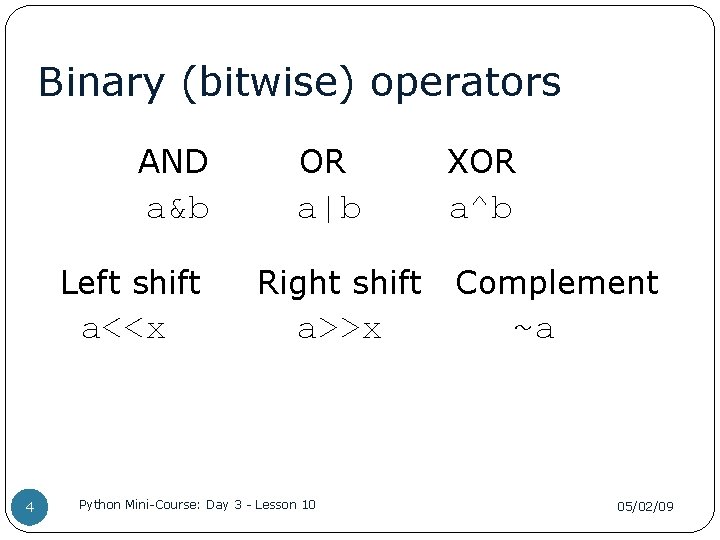
Binary (bitwise) operators 4 AND OR XOR a&b a|b a^b Left shift Right shift a<<x a>>x Python Mini-Course: Day 3 - Lesson 10 Complement ~a 05/02/09
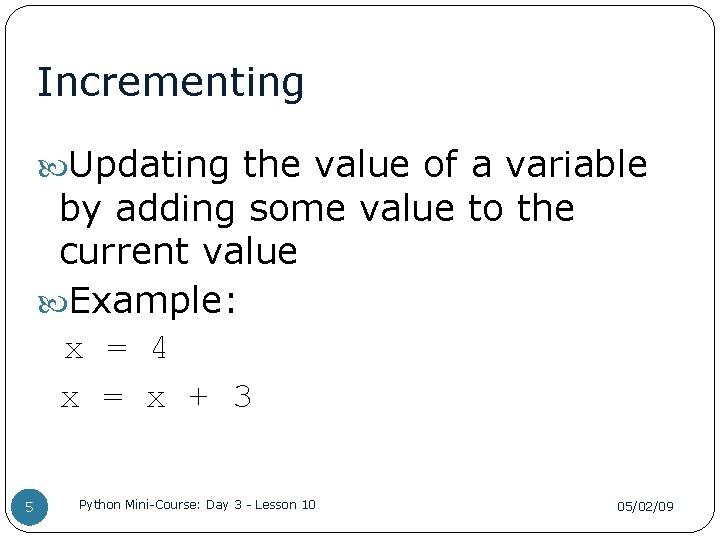
Incrementing Updating the value of a variable by adding some value to the current value Example: x = 4 x = x + 3 5 Python Mini-Course: Day 3 - Lesson 10 05/02/09
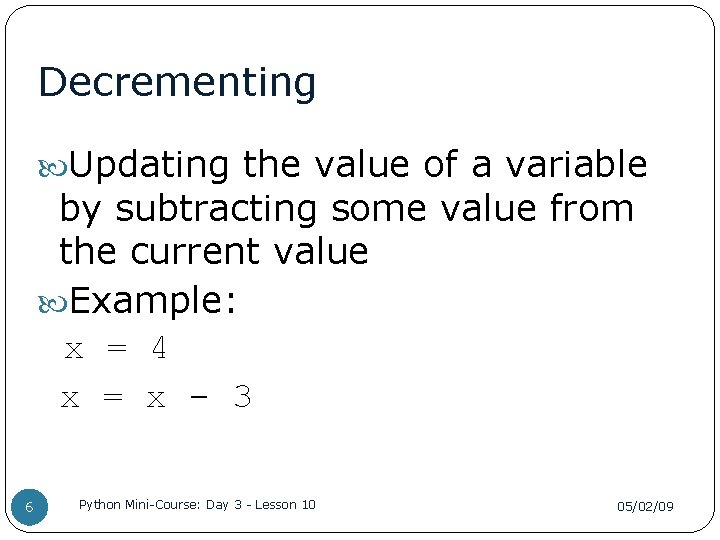
Decrementing Updating the value of a variable by subtracting some value from the current value Example: x = 4 x = x - 3 6 Python Mini-Course: Day 3 - Lesson 10 05/02/09
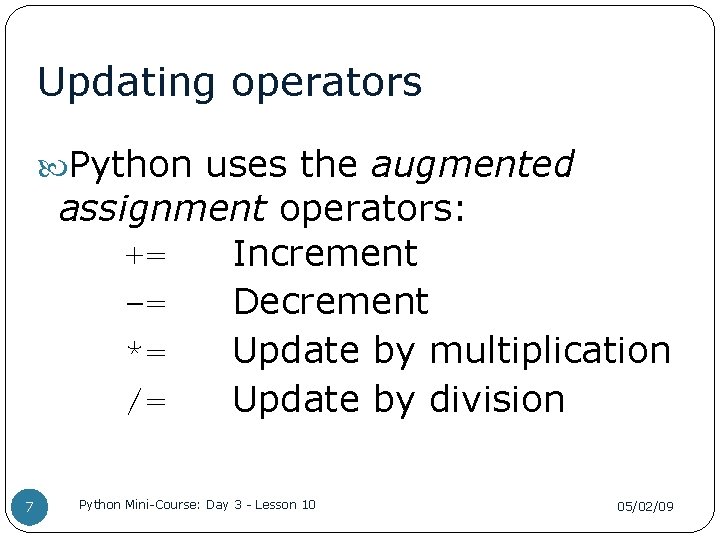
Updating operators Python uses the augmented assignment operators: += Increment -= Decrement *= Update by multiplication /= Update by division 7 Python Mini-Course: Day 3 - Lesson 10 05/02/09
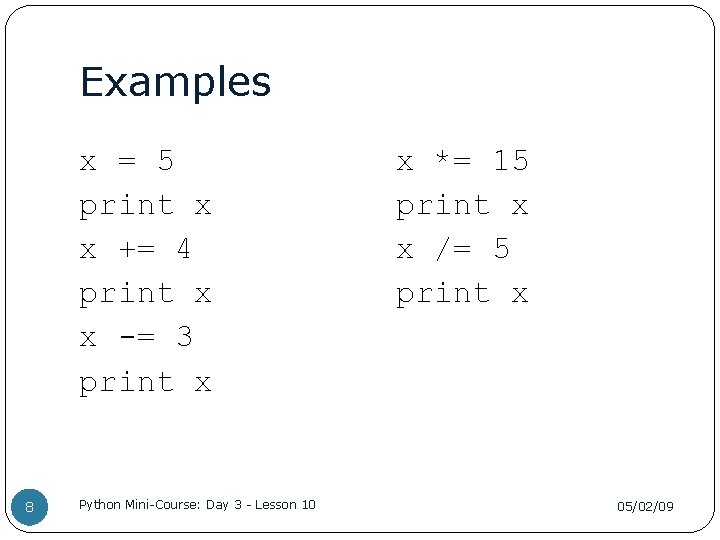
Examples x = 5 print x x += 4 print x x -= 3 print x 8 Python Mini-Course: Day 3 - Lesson 10 x *= 15 print x x /= 5 print x 05/02/09
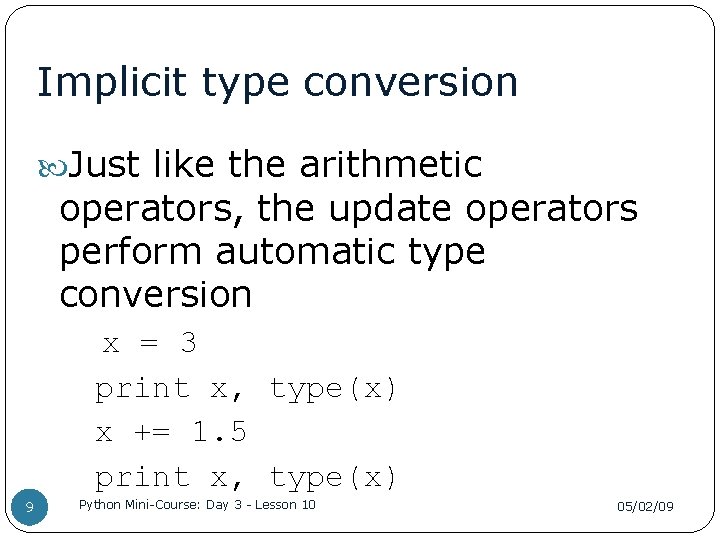
Implicit type conversion Just like the arithmetic operators, the update operators perform automatic type conversion x = 3 print x, type(x) x += 1. 5 print x, type(x) 9 Python Mini-Course: Day 3 - Lesson 10 05/02/09
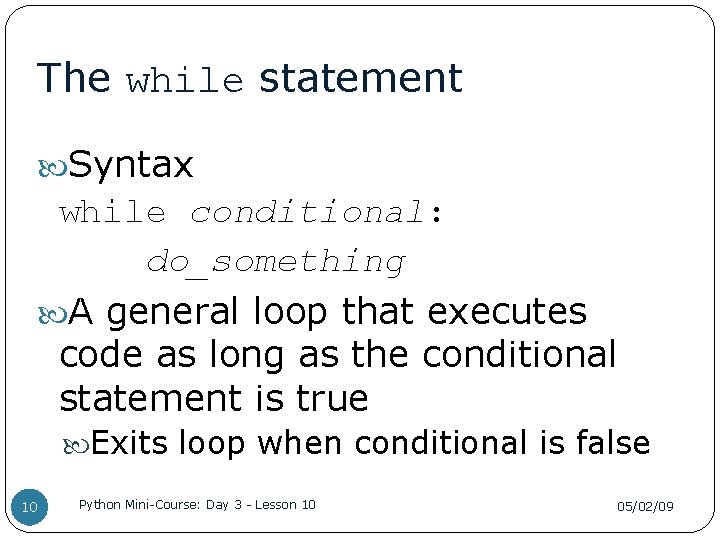
The while statement Syntax while conditional: do_something A general loop that executes code as long as the conditional statement is true Exits loop when conditional is false 10 Python Mini-Course: Day 3 - Lesson 10 05/02/09
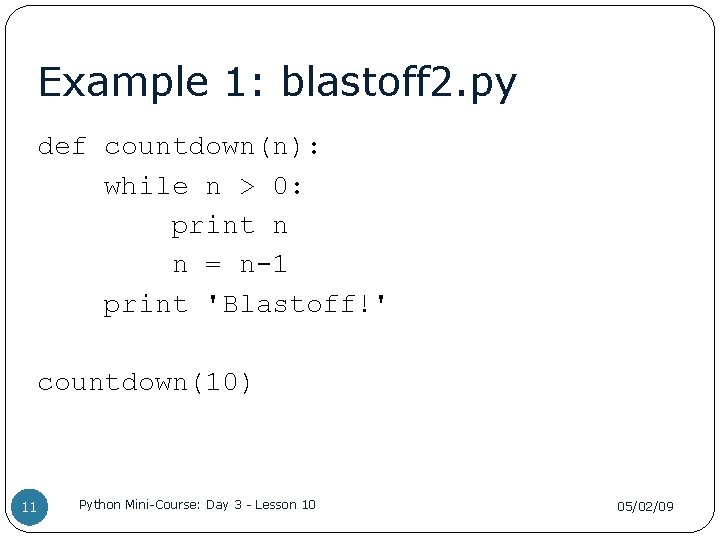
Example 1: blastoff 2. py def countdown(n): while n > 0: print n n = n-1 print 'Blastoff!' countdown(10) 11 Python Mini-Course: Day 3 - Lesson 10 05/02/09
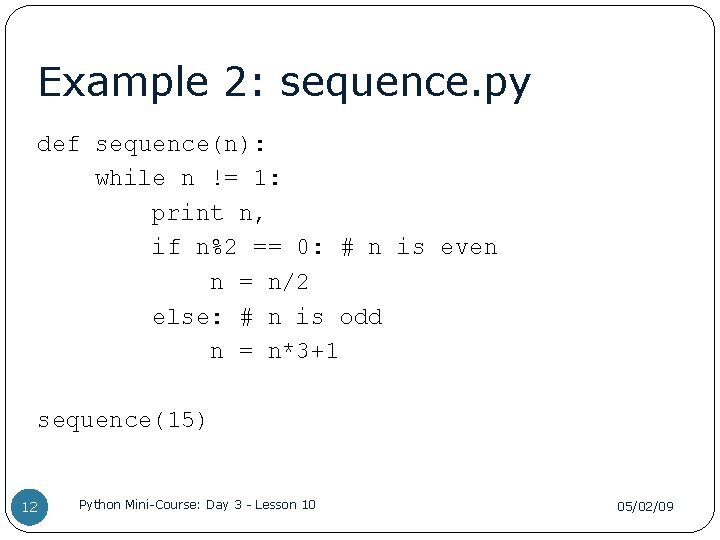
Example 2: sequence. py def sequence(n): while n != 1: print n, if n%2 == 0: # n is even n = n/2 else: # n is odd n = n*3+1 sequence(15) 12 Python Mini-Course: Day 3 - Lesson 10 05/02/09
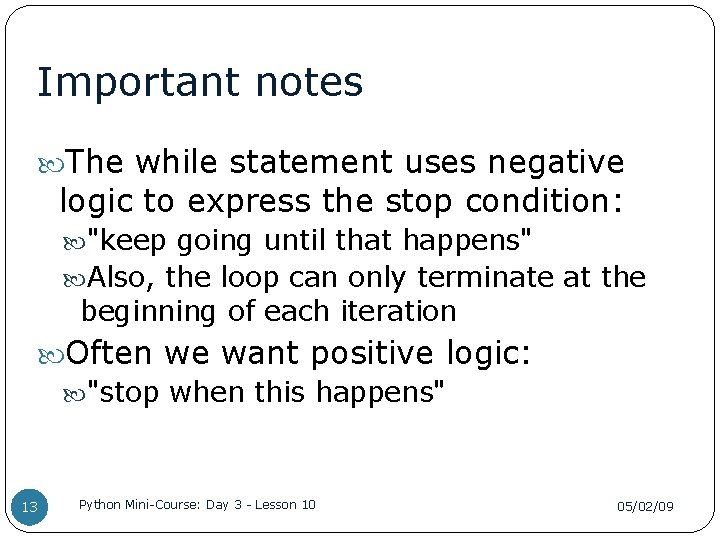
Important notes The while statement uses negative logic to express the stop condition: "keep going until that happens" Also, the loop can only terminate at the beginning of each iteration Often we want positive logic: "stop when this happens" 13 Python Mini-Course: Day 3 - Lesson 10 05/02/09
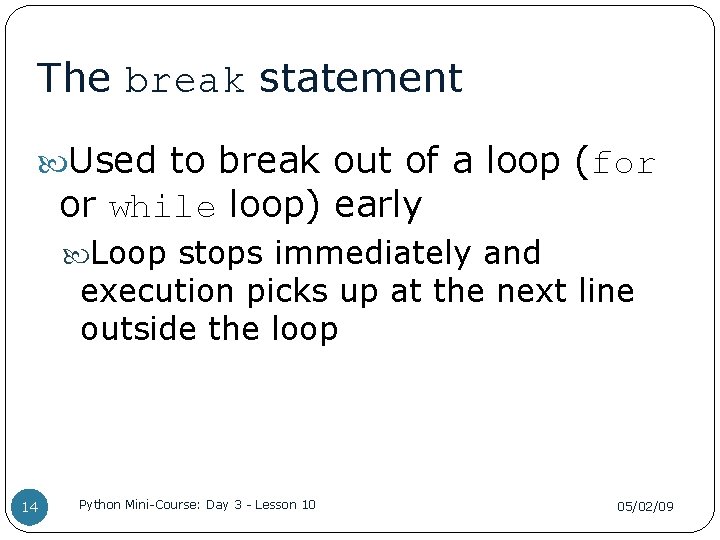
The break statement Used to break out of a loop (for or while loop) early Loop stops immediately and execution picks up at the next line outside the loop 14 Python Mini-Course: Day 3 - Lesson 10 05/02/09
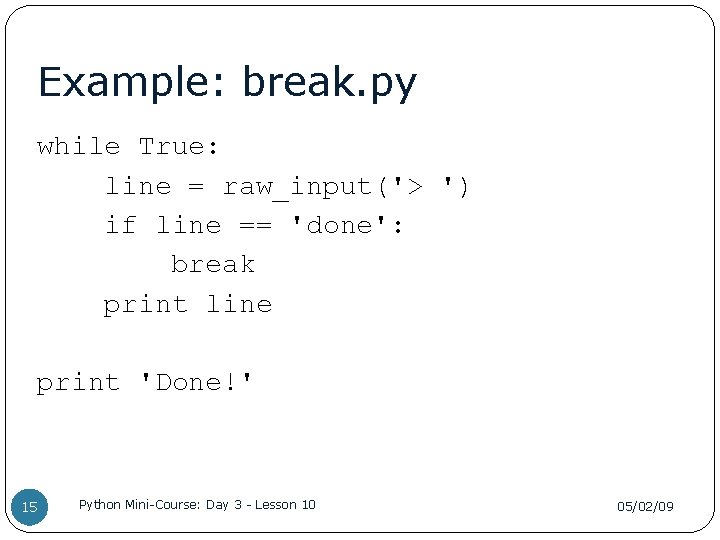
Example: break. py while True: line = raw_input('> ') if line == 'done': break print line print 'Done!' 15 Python Mini-Course: Day 3 - Lesson 10 05/02/09
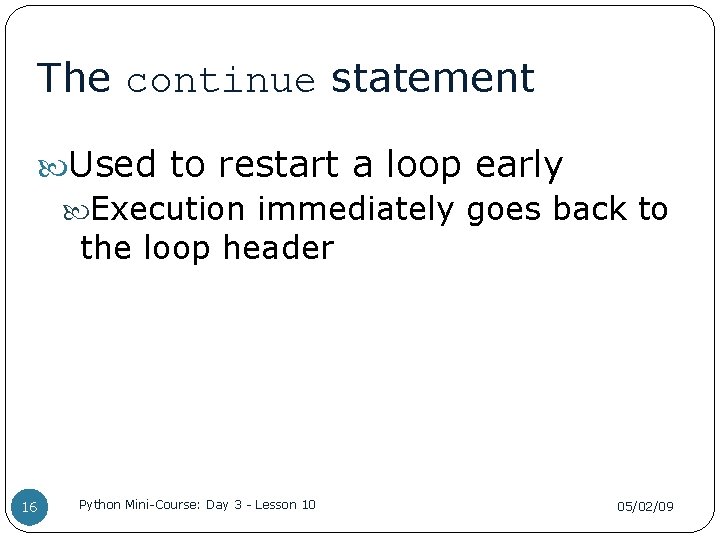
The continue statement Used to restart a loop early Execution immediately goes back to the loop header 16 Python Mini-Course: Day 3 - Lesson 10 05/02/09
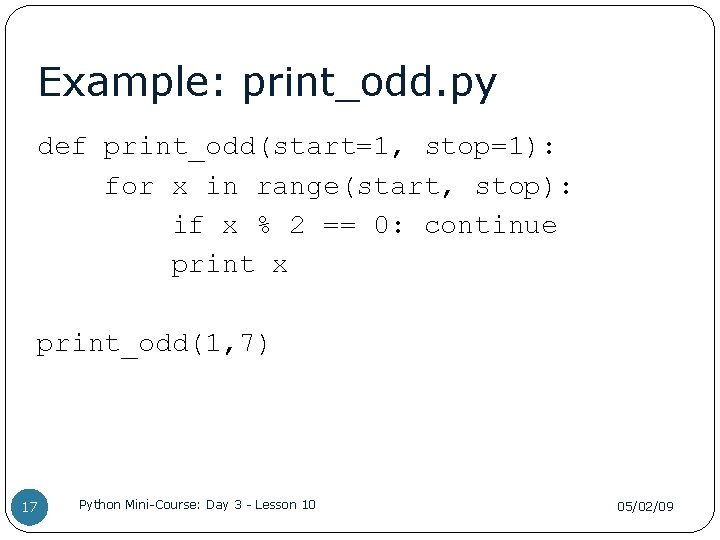
Example: print_odd. py def print_odd(start=1, stop=1): for x in range(start, stop): if x % 2 == 0: continue print x print_odd(1, 7) 17 Python Mini-Course: Day 3 - Lesson 10 05/02/09
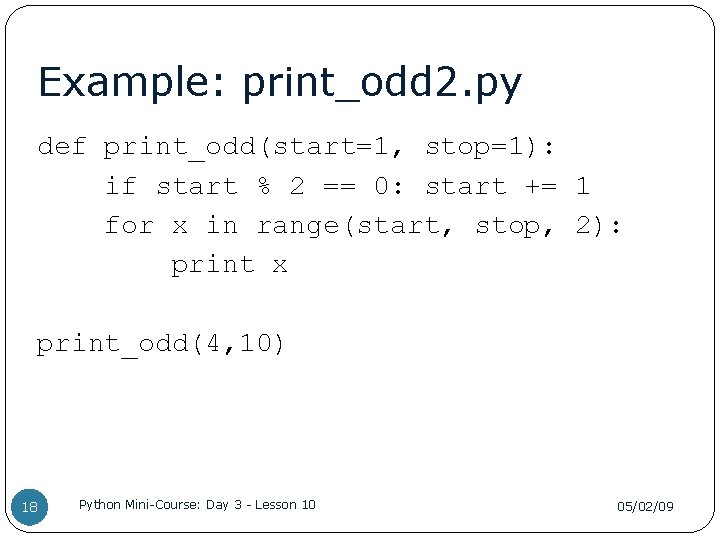
Example: print_odd 2. py def print_odd(start=1, stop=1): if start % 2 == 0: start += 1 for x in range(start, stop, 2): print x print_odd(4, 10) 18 Python Mini-Course: Day 3 - Lesson 10 05/02/09
Day 1 day 2 day 3 day 4
Perulangan python
Nested loops python
Sentinel loops python
Day 1 day 2 day 817
Dame meaning in baa black sheep
Lesson 7 sticker art with loops
While loops and if-else structures
Fef 200-1200
Reddish loops of gas
Matlab break nested loop
Types of loops in matlab
Drilling fluid
Loops in matlab
Cakewalk loops
Intestinal obstruction classification
What is loop in electrical circuit
Adobe audition loops
Matlab loop until condition met