CSCE 515 Computer Network Programming UDP Socket Wenyuan
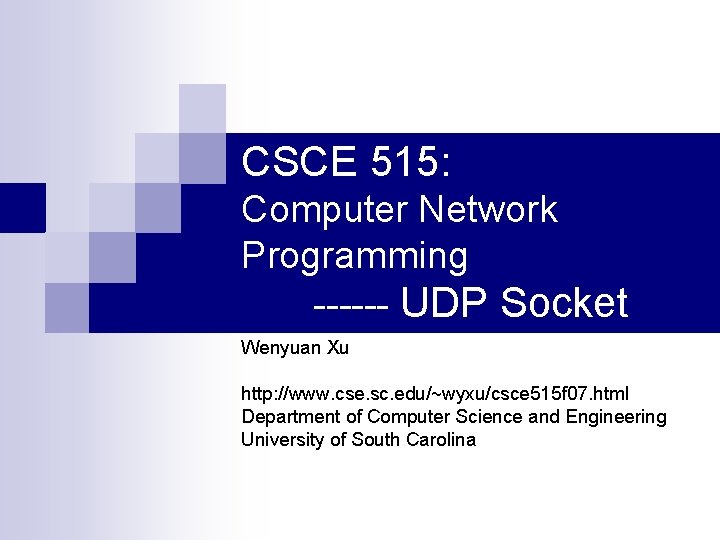
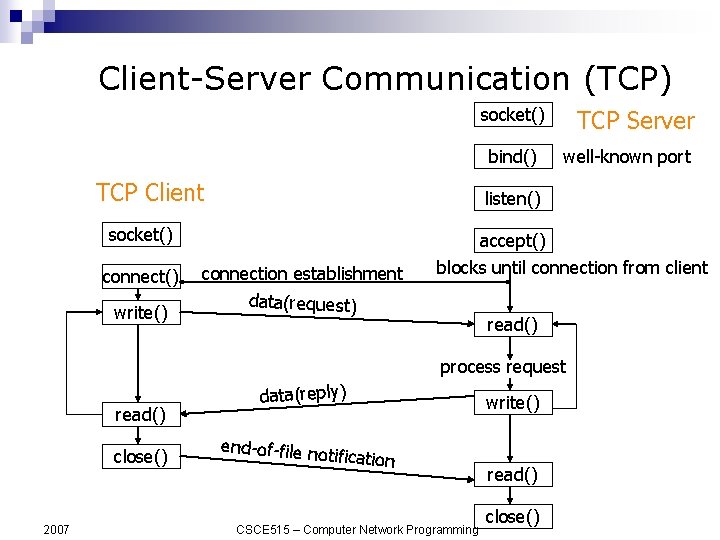
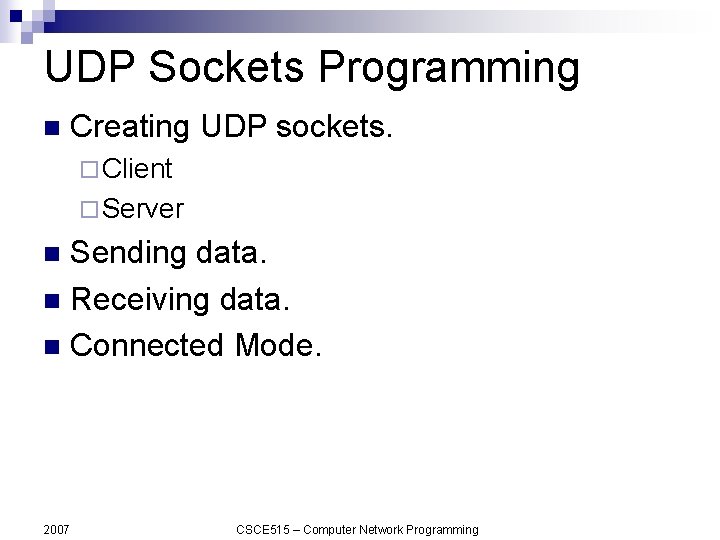
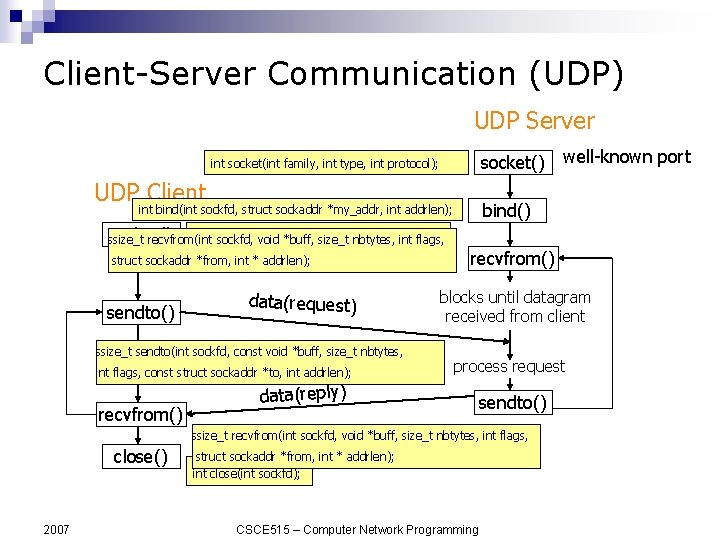
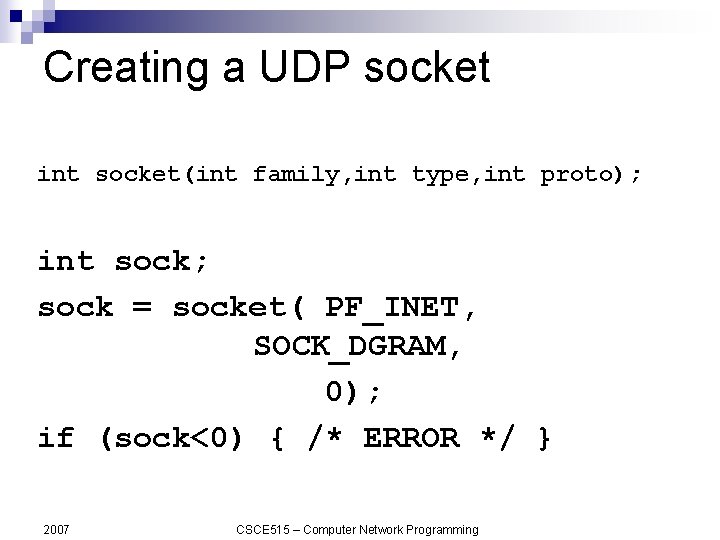
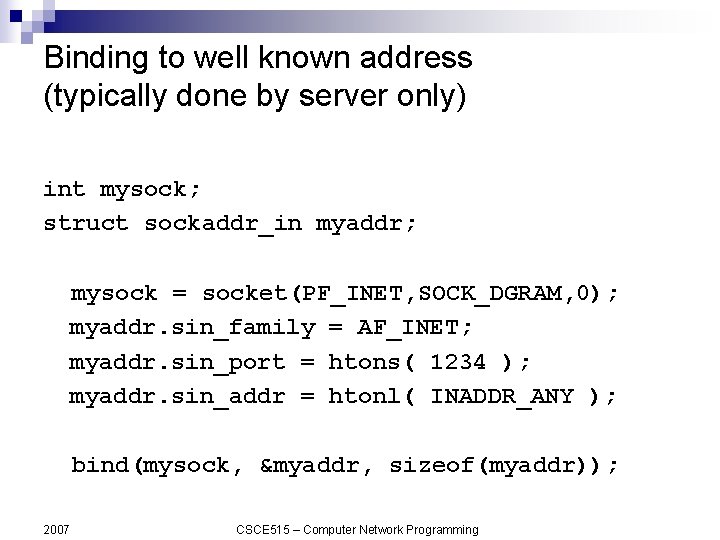
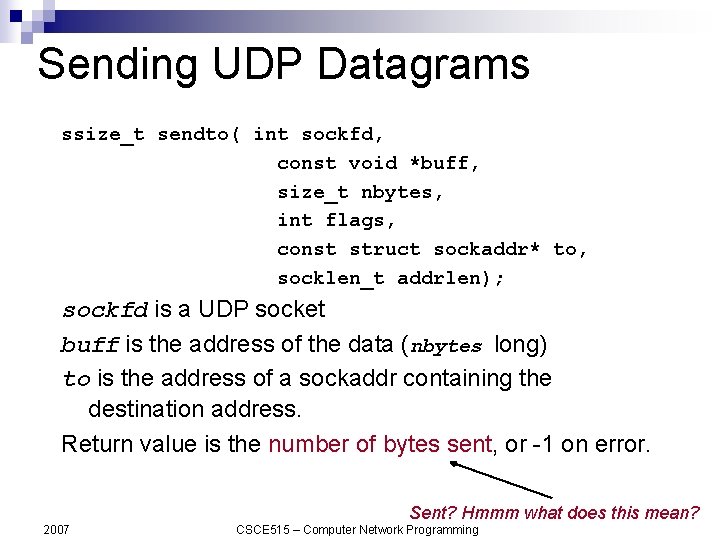
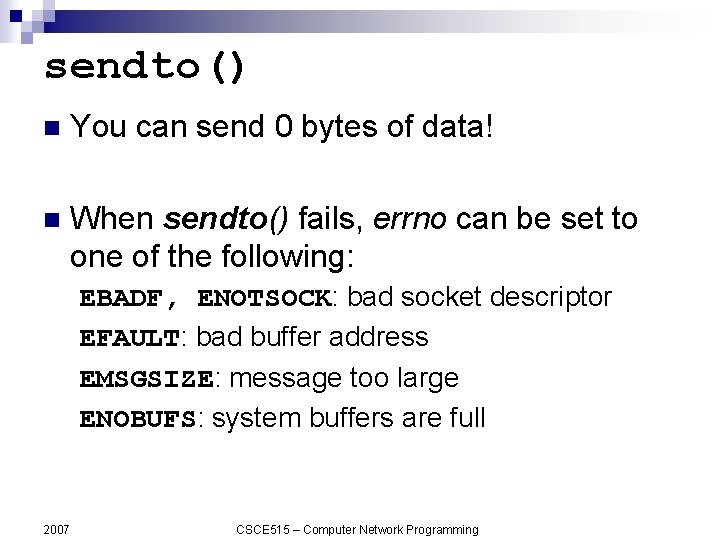
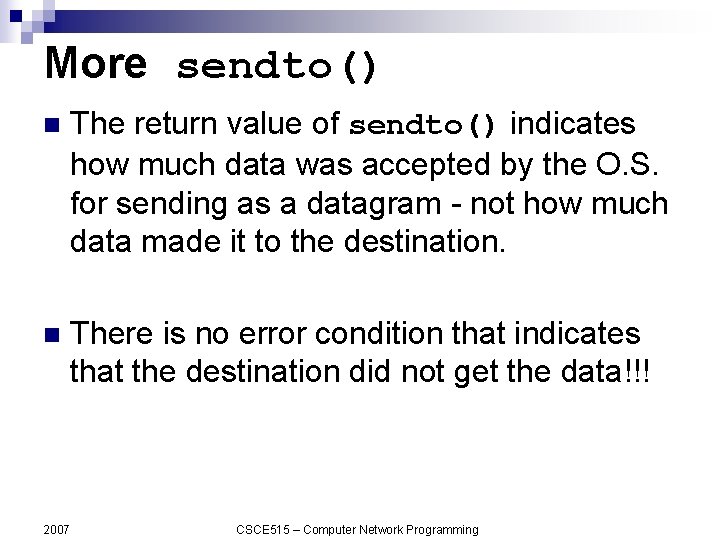
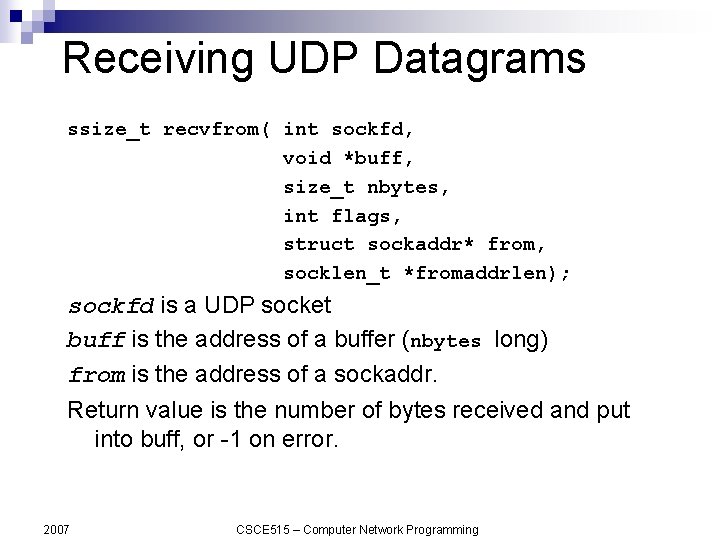
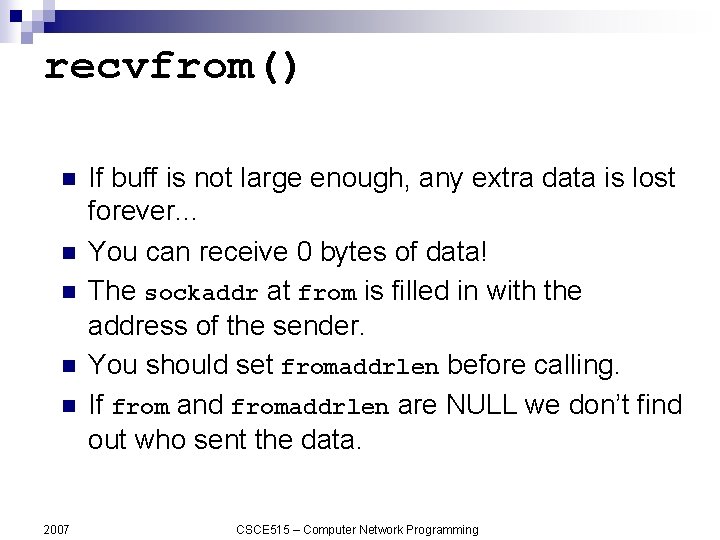
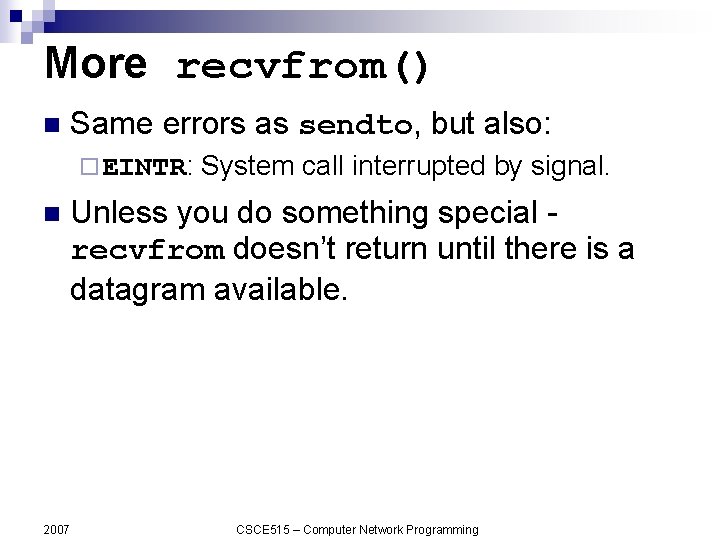
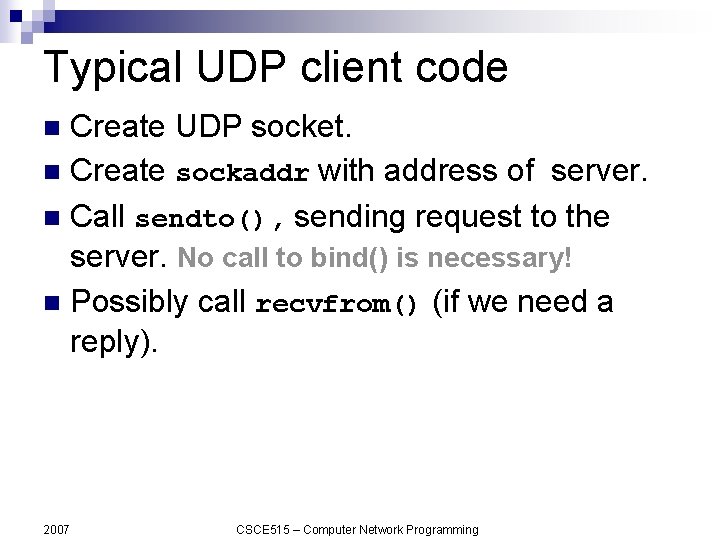
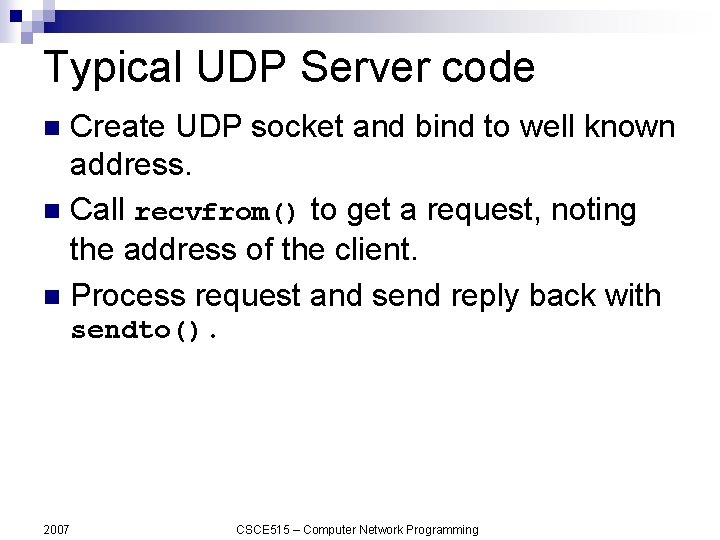
![UDP Echo Server int mysock; struct sockaddr_in myaddr, cliaddr; char msgbuf[MAXLEN]; socklen_t clilen; int UDP Echo Server int mysock; struct sockaddr_in myaddr, cliaddr; char msgbuf[MAXLEN]; socklen_t clilen; int](https://slidetodoc.com/presentation_image/bd14237516aab45931eab41c6fdaf4c2/image-15.jpg)
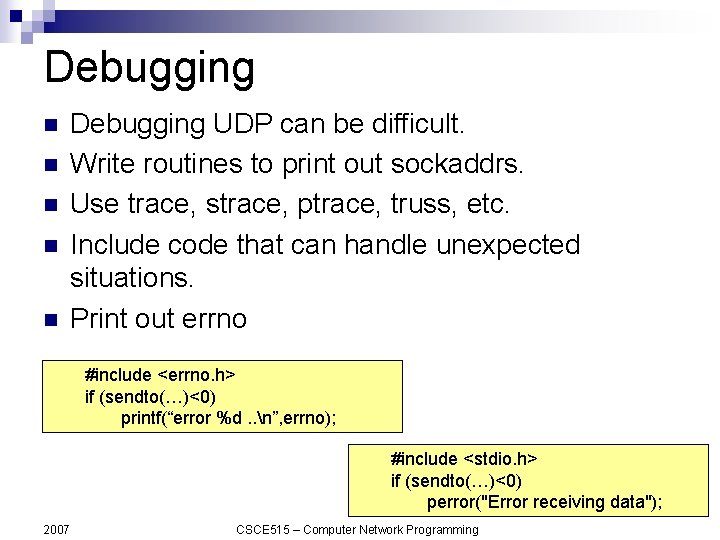
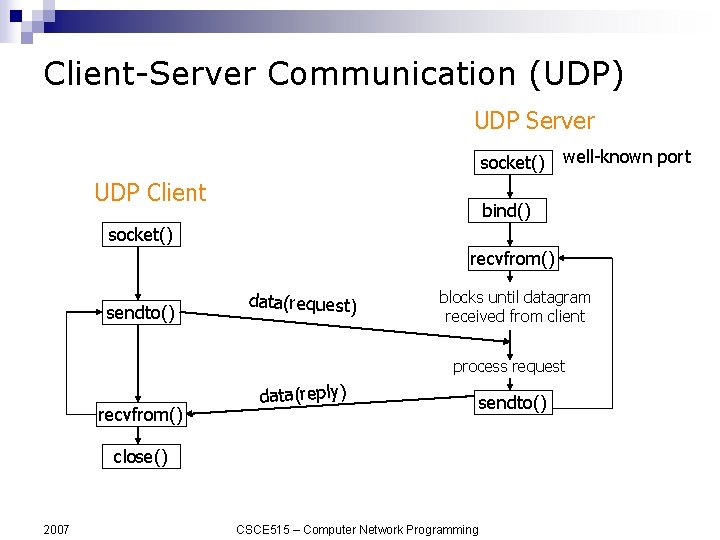
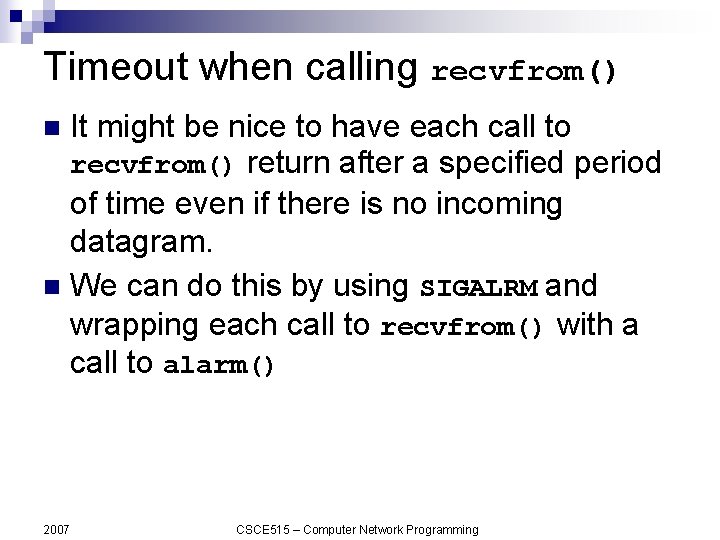
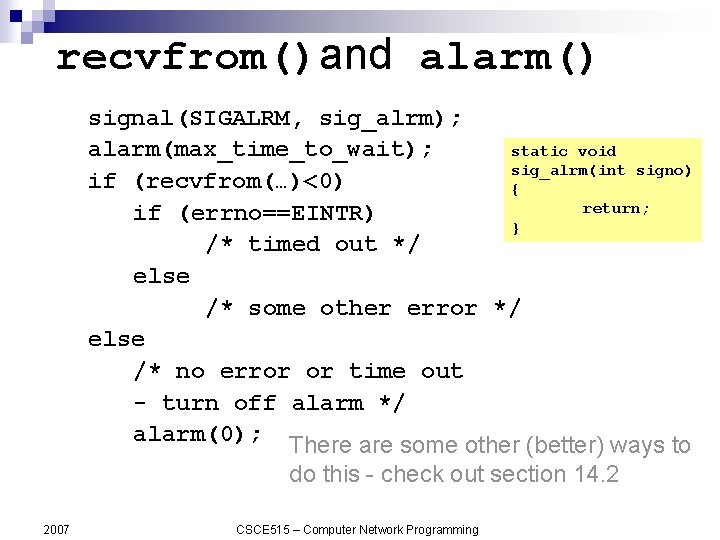
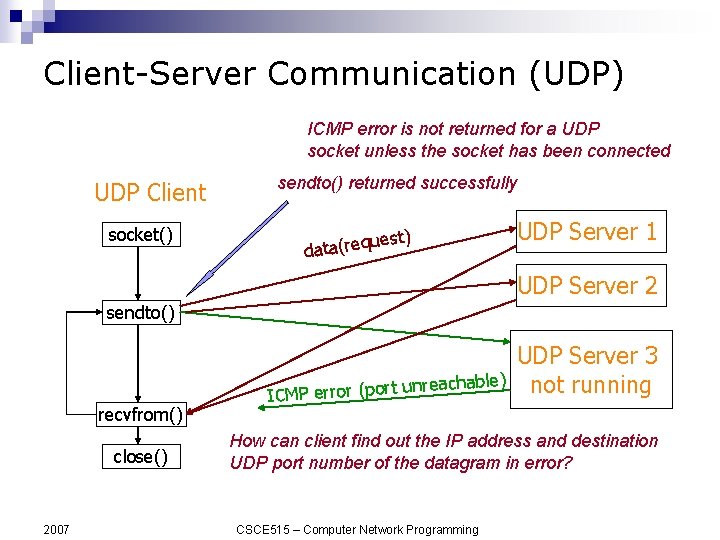
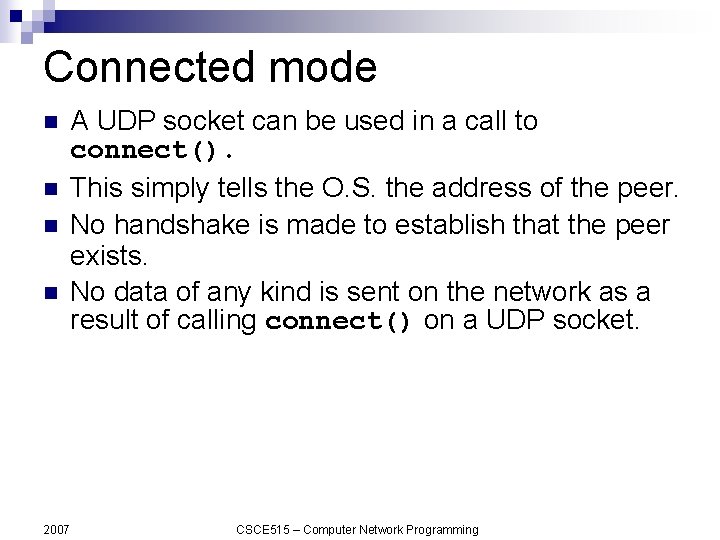
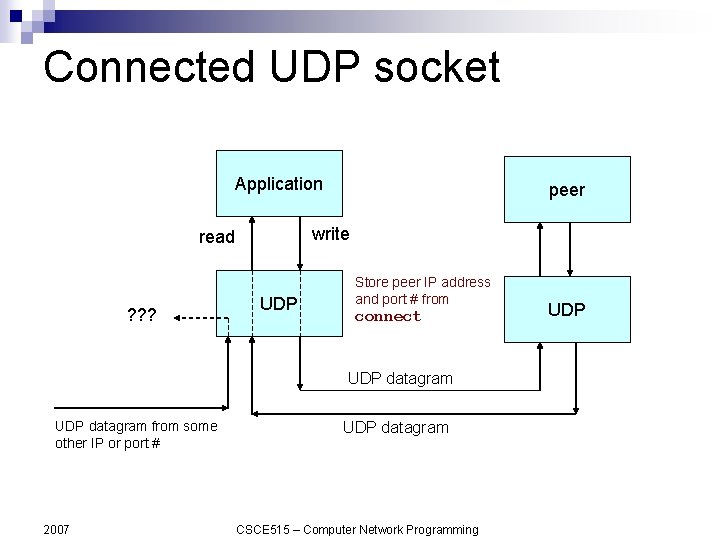
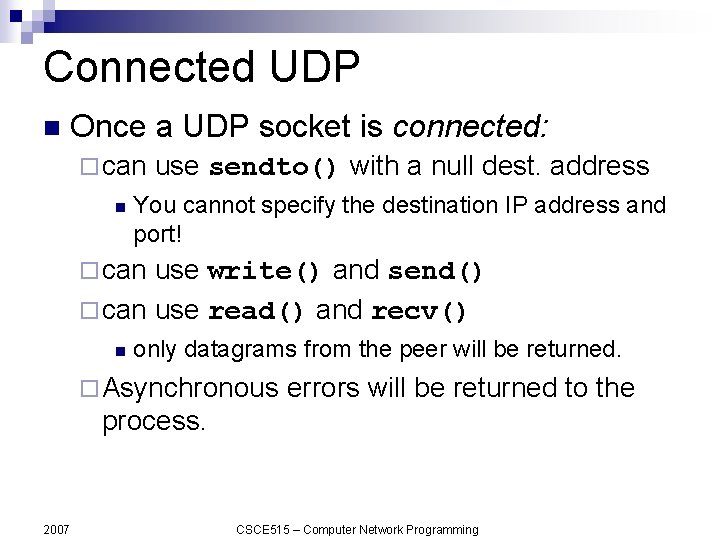
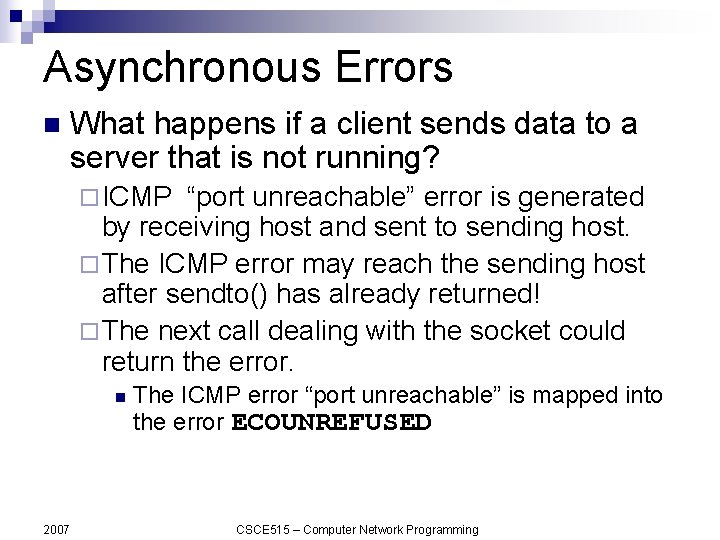
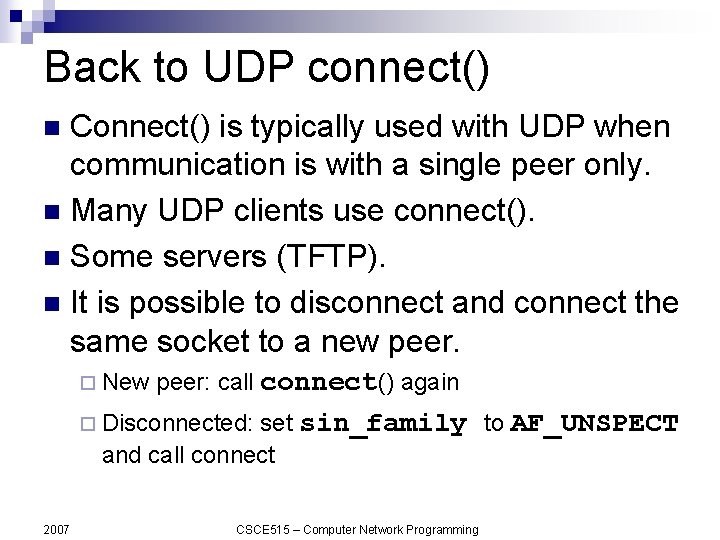
- Slides: 25
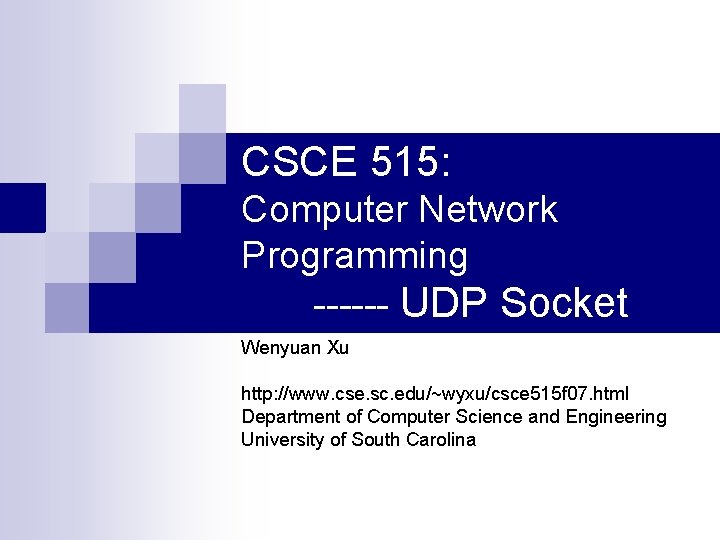
CSCE 515: Computer Network Programming ------ UDP Socket Wenyuan Xu http: //www. cse. sc. edu/~wyxu/csce 515 f 07. html Department of Computer Science and Engineering University of South Carolina
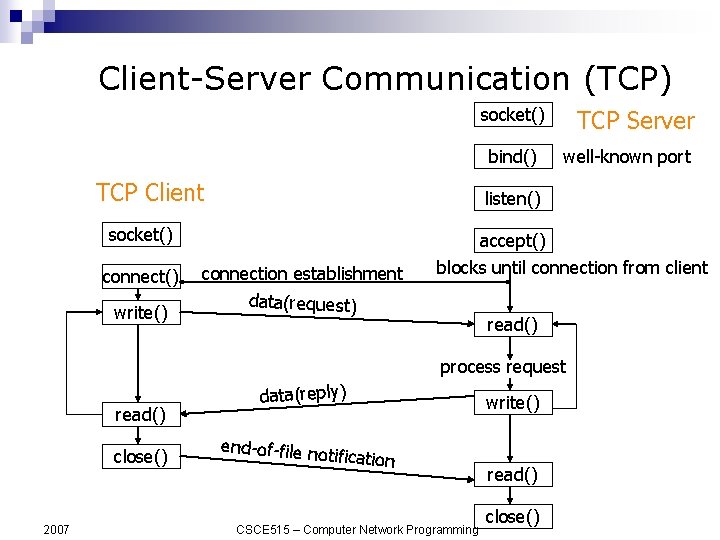
Client-Server Communication (TCP) socket() bind() TCP Client TCP Server well-known port listen() socket() accept() connection establishment write() data(request) blocks until connection from client read() process request read() close() 2007 data(reply) end-of-file no tification CSCE 515 – Computer Network Programming write() read() close()
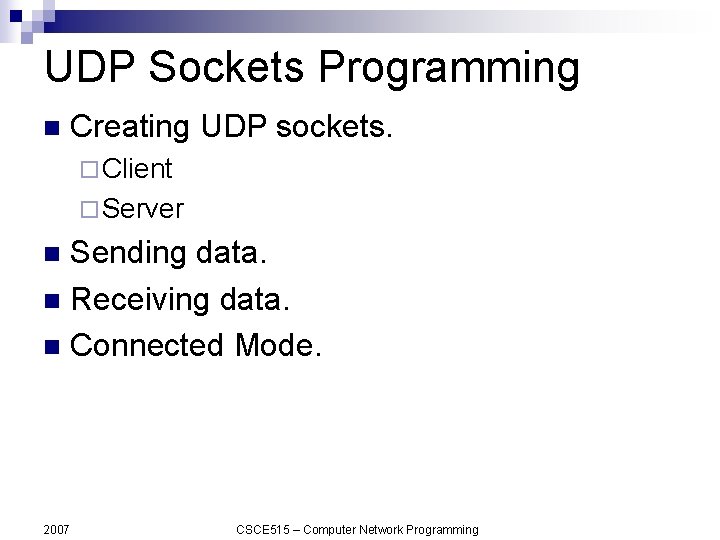
UDP Sockets Programming n Creating UDP sockets. ¨ Client ¨ Server Sending data. n Receiving data. n Connected Mode. n 2007 CSCE 515 – Computer Network Programming
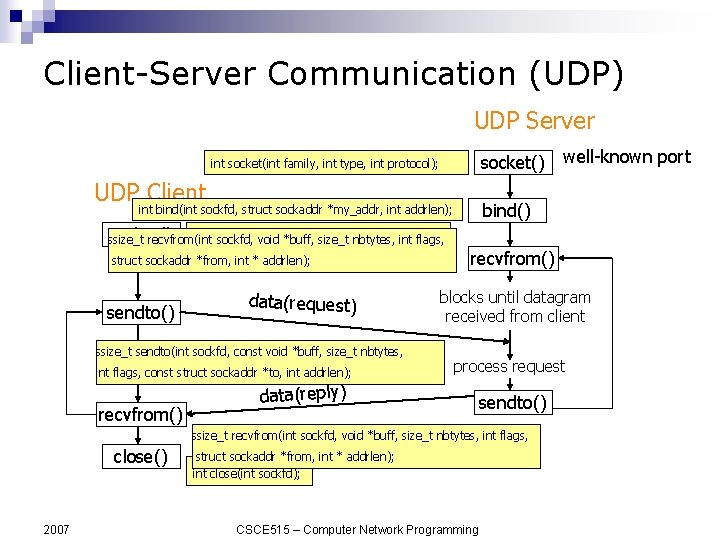
Client-Server Communication (UDP) UDP Server socket() int socket(int family, int type, int protocol); UDP Client well-known port bind() int bind(int sockfd, struct sockaddr *my_addr, int addrlen); int socket(int family, intsize_t type, nbtytes, int protocol); socket() ssize_t recvfrom(int sockfd, void *buff, int flags, struct sockaddr *from, int * addrlen); sendto() data(request) ssize_t sendto(int sockfd, const void *buff, size_t nbtytes, int flags, const struct sockaddr *to, int addrlen); recvfrom() blocks until datagram received from client process request data(reply) sendto() ssize_t recvfrom(int sockfd, void *buff, size_t nbtytes, int flags, close() 2007 struct sockaddr *from, int * addrlen); int close(int sockfd); CSCE 515 – Computer Network Programming
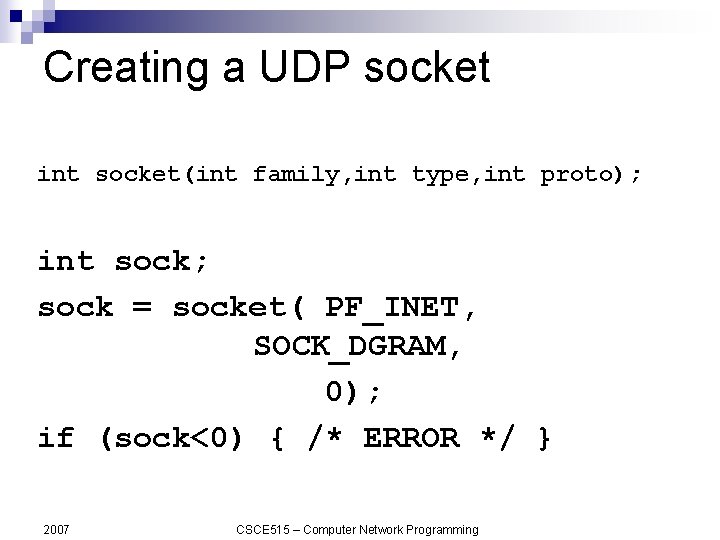
Creating a UDP socket int socket(int family, int type, int proto); int sock; sock = socket( PF_INET, SOCK_DGRAM, 0); if (sock<0) { /* ERROR */ } 2007 CSCE 515 – Computer Network Programming
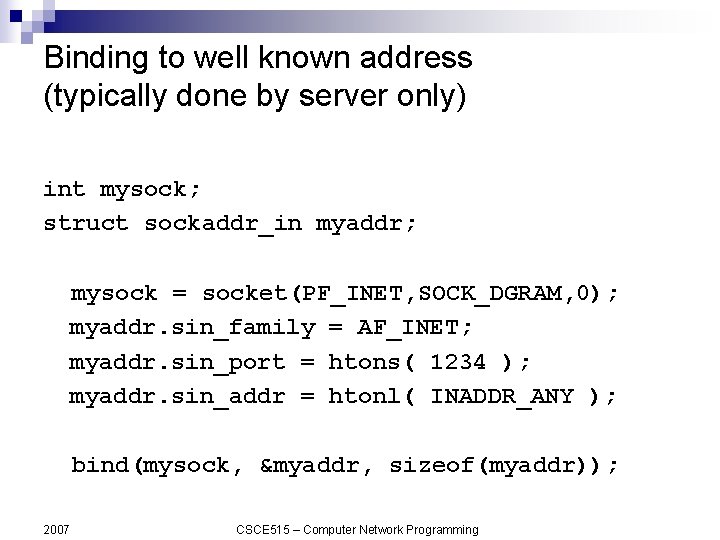
Binding to well known address (typically done by server only) int mysock; struct sockaddr_in myaddr; mysock = socket(PF_INET, SOCK_DGRAM, 0); myaddr. sin_family = AF_INET; myaddr. sin_port = htons( 1234 ); myaddr. sin_addr = htonl( INADDR_ANY ); bind(mysock, &myaddr, sizeof(myaddr)); 2007 CSCE 515 – Computer Network Programming
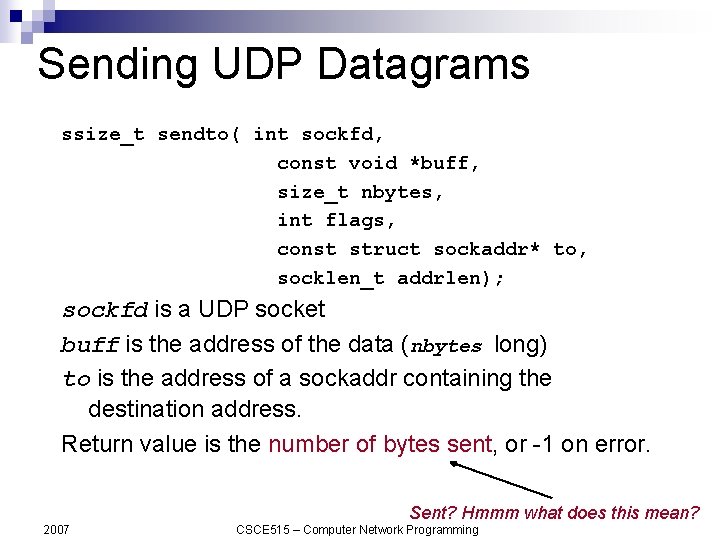
Sending UDP Datagrams ssize_t sendto( int sockfd, const void *buff, size_t nbytes, int flags, const struct sockaddr* to, socklen_t addrlen); sockfd is a UDP socket buff is the address of the data (nbytes long) to is the address of a sockaddr containing the destination address. Return value is the number of bytes sent, or -1 on error. 2007 Sent? Hmmm what does this mean? CSCE 515 – Computer Network Programming
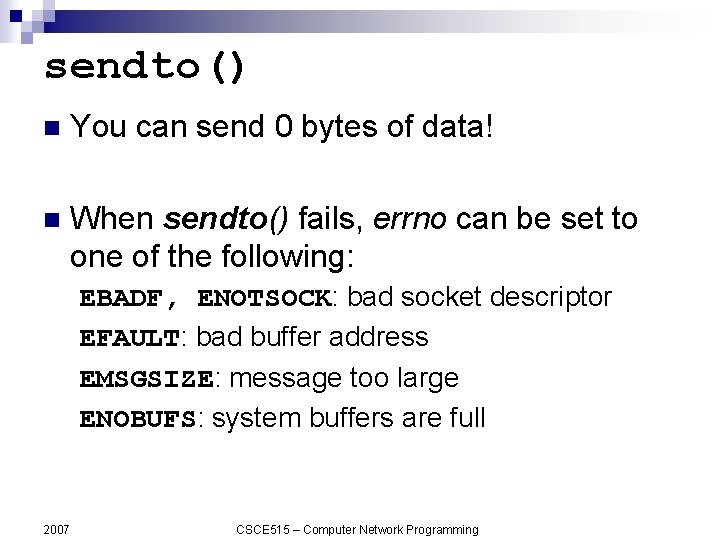
sendto() n You can send 0 bytes of data! n When sendto() fails, errno can be set to one of the following: EBADF, ENOTSOCK: bad socket descriptor EFAULT: bad buffer address EMSGSIZE: message too large ENOBUFS: system buffers are full 2007 CSCE 515 – Computer Network Programming
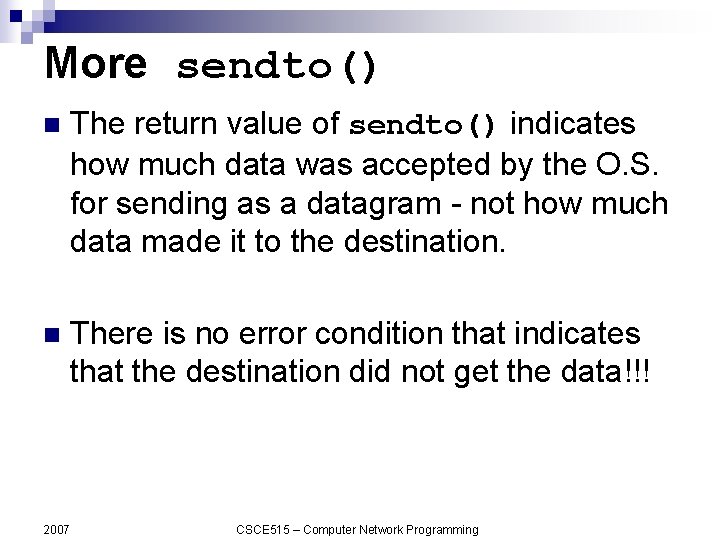
More sendto() n The return value of sendto() indicates how much data was accepted by the O. S. for sending as a datagram - not how much data made it to the destination. n There is no error condition that indicates that the destination did not get the data!!! 2007 CSCE 515 – Computer Network Programming
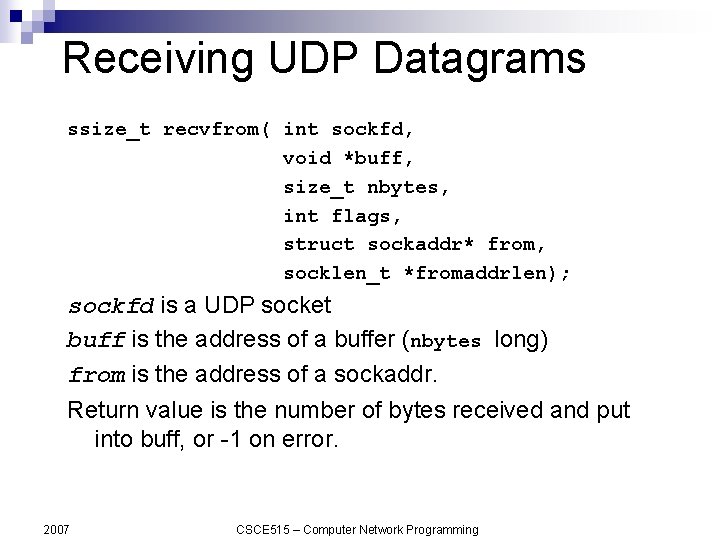
Receiving UDP Datagrams ssize_t recvfrom( int sockfd, void *buff, size_t nbytes, int flags, struct sockaddr* from, socklen_t *fromaddrlen); sockfd is a UDP socket buff is the address of a buffer (nbytes long) from is the address of a sockaddr. Return value is the number of bytes received and put into buff, or -1 on error. 2007 CSCE 515 – Computer Network Programming
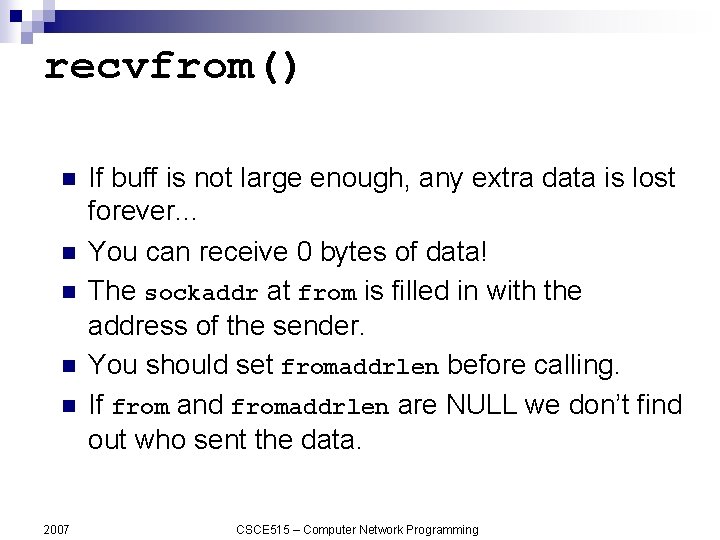
recvfrom() n n n 2007 If buff is not large enough, any extra data is lost forever. . . You can receive 0 bytes of data! The sockaddr at from is filled in with the address of the sender. You should set fromaddrlen before calling. If from and fromaddrlen are NULL we don’t find out who sent the data. CSCE 515 – Computer Network Programming
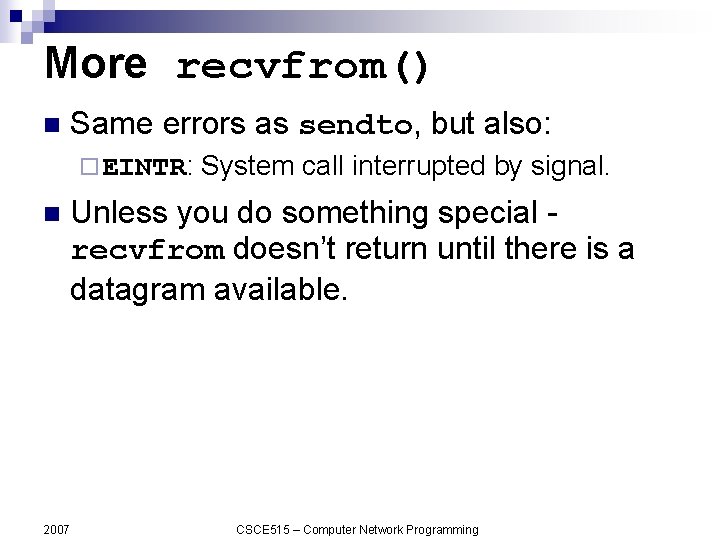
More recvfrom() n Same errors as sendto, but also: ¨ EINTR: n 2007 System call interrupted by signal. Unless you do something special recvfrom doesn’t return until there is a datagram available. CSCE 515 – Computer Network Programming
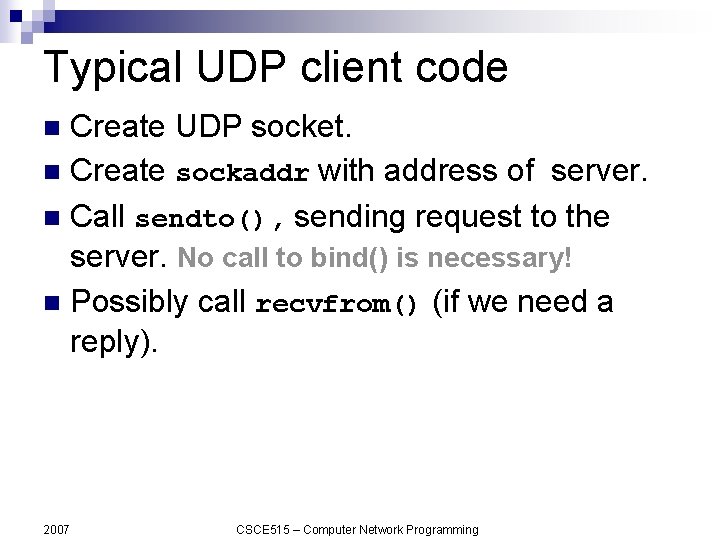
Typical UDP client code Create UDP socket. n Create sockaddr with address of server. n Call sendto(), sending request to the server. No call to bind() is necessary! n Possibly call recvfrom() (if we need a reply). n 2007 CSCE 515 – Computer Network Programming
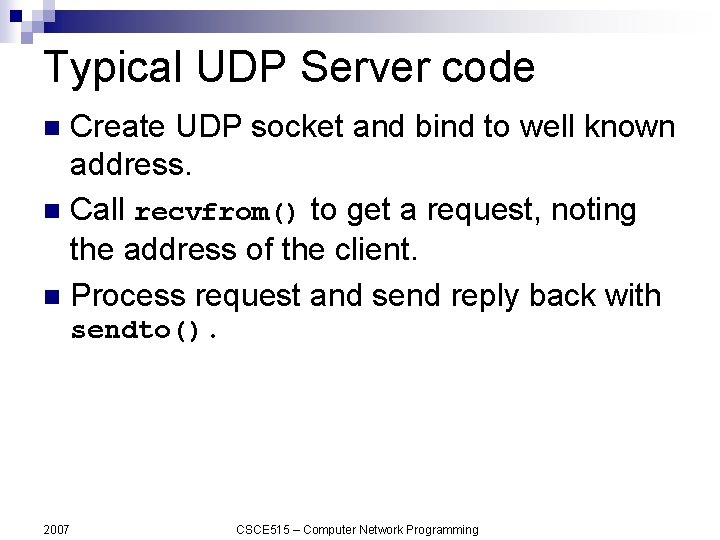
Typical UDP Server code Create UDP socket and bind to well known address. n Call recvfrom() to get a request, noting the address of the client. n Process request and send reply back with n sendto(). 2007 CSCE 515 – Computer Network Programming
![UDP Echo Server int mysock struct sockaddrin myaddr cliaddr char msgbufMAXLEN socklent clilen int UDP Echo Server int mysock; struct sockaddr_in myaddr, cliaddr; char msgbuf[MAXLEN]; socklen_t clilen; int](https://slidetodoc.com/presentation_image/bd14237516aab45931eab41c6fdaf4c2/image-15.jpg)
UDP Echo Server int mysock; struct sockaddr_in myaddr, cliaddr; char msgbuf[MAXLEN]; socklen_t clilen; int msglen; CK E H !! C O RS! T D RO E NE R ER FO mysock = socket(PF_INET, SOCK_DGRAM, 0); myaddr. sin_family = AF_INET; myaddr. sin_port = htons( S_PORT ); myaddr. sin_addr = htonl( INADDR_ANY ); bind(mysock, &myaddr, sizeof(myaddr)); while (1) { len=sizeof(cliaddr); en); msglen=recvfrom(mysock, msgbuf, MAXLEN, 0, cliaddr, &clil sendto(mysock, msgbuf, msglen, 0, cliaddr, clilen); } 2007 CSCE 515 – Computer Network Programming
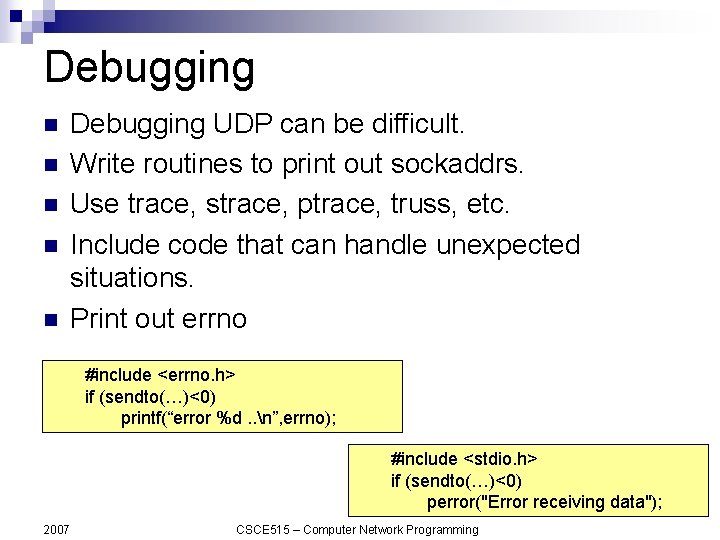
Debugging n n n Debugging UDP can be difficult. Write routines to print out sockaddrs. Use trace, strace, ptrace, truss, etc. Include code that can handle unexpected situations. Print out errno #include <errno. h> if (sendto(…)<0) printf(“error %d. . n”, errno); #include <stdio. h> if (sendto(…)<0) perror("Error receiving data"); 2007 CSCE 515 – Computer Network Programming
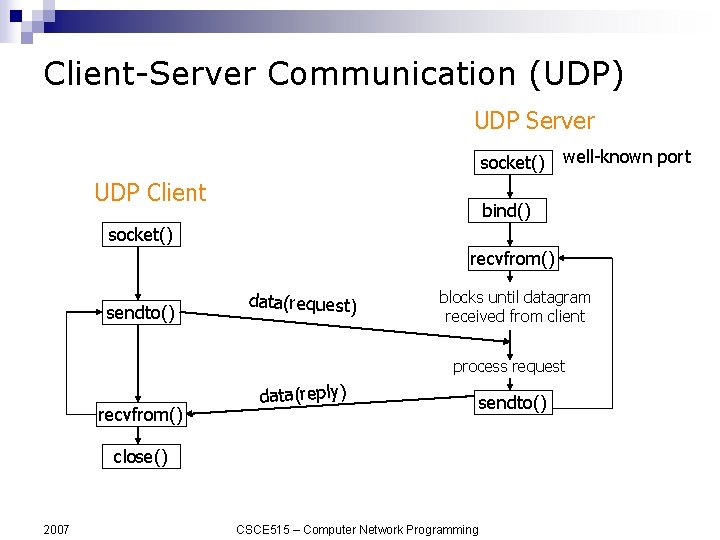
Client-Server Communication (UDP) UDP Server socket() UDP Client well-known port bind() socket() recvfrom() sendto() data(request) blocks until datagram received from client process request recvfrom() data(reply) close() 2007 CSCE 515 – Computer Network Programming sendto()
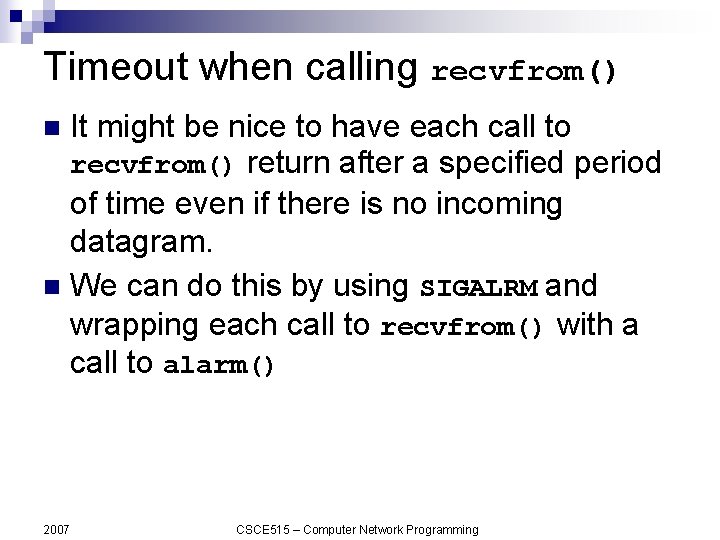
Timeout when calling recvfrom() It might be nice to have each call to recvfrom() return after a specified period of time even if there is no incoming datagram. n We can do this by using SIGALRM and wrapping each call to recvfrom() with a call to alarm() n 2007 CSCE 515 – Computer Network Programming
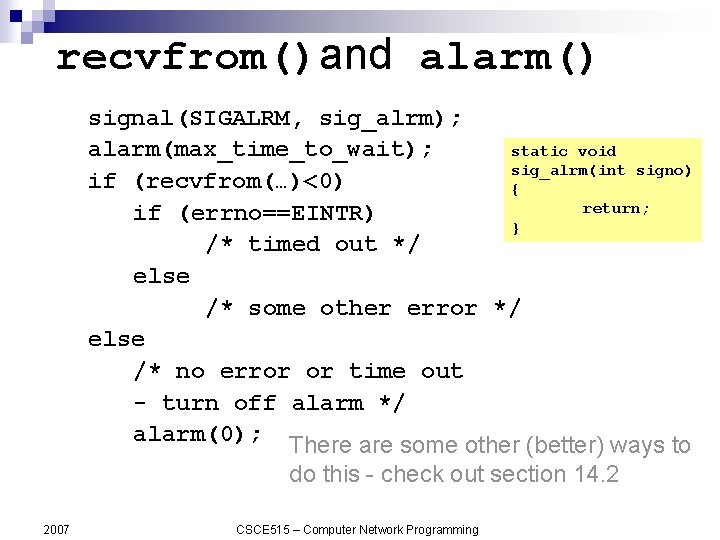
recvfrom()and alarm() signal(SIGALRM, sig_alrm); static void alarm(max_time_to_wait); sig_alrm(int signo) if (recvfrom(…)<0) { return; if (errno==EINTR) } /* timed out */ else /* some other error */ else /* no error or time out - turn off alarm */ alarm(0); There are some other (better) ways to do this - check out section 14. 2 2007 CSCE 515 – Computer Network Programming
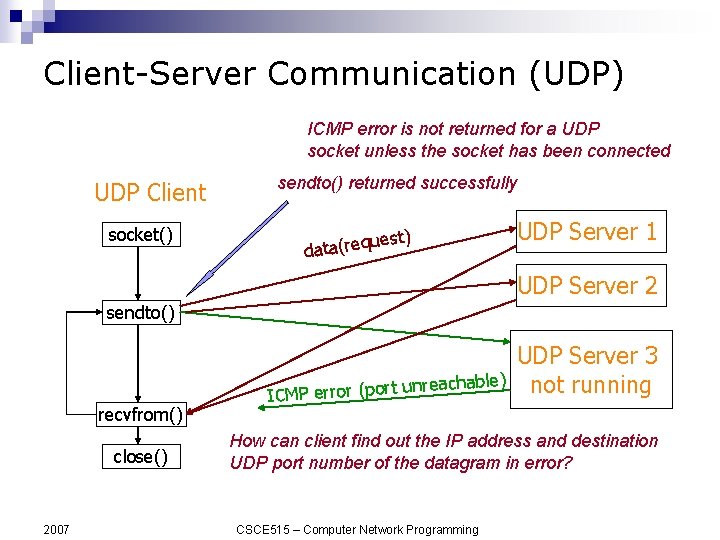
Client-Server Communication (UDP) ICMP error is not returned for a UDP socket unless the socket has been connected UDP Client socket() sendto() returned successfully uest) q e r ( a t da UDP Server 1 UDP Server 2 sendto() recvfrom() close() 2007 achable) re n u rt o (p r o rr e ICMP UDP Server 3 not running How can client find out the IP address and destination UDP port number of the datagram in error? CSCE 515 – Computer Network Programming
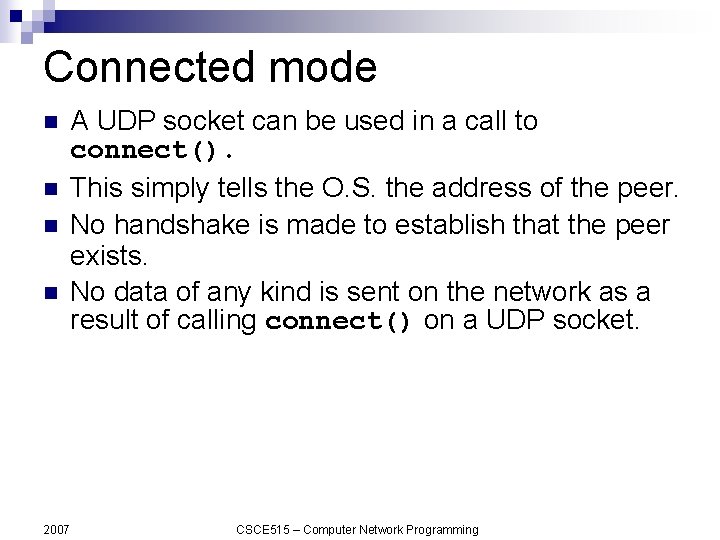
Connected mode n n 2007 A UDP socket can be used in a call to connect(). This simply tells the O. S. the address of the peer. No handshake is made to establish that the peer exists. No data of any kind is sent on the network as a result of calling connect() on a UDP socket. CSCE 515 – Computer Network Programming
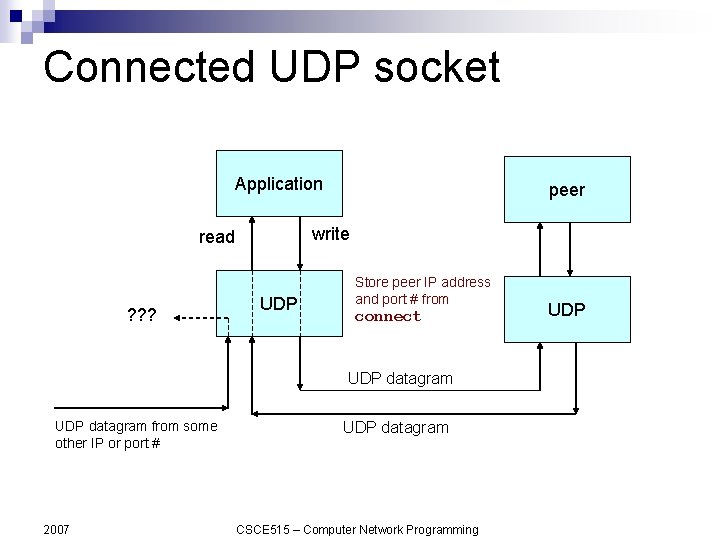
Connected UDP socket Application write read ? ? ? peer UDP Store peer IP address and port # from connect UDP datagram from some other IP or port # 2007 UDP datagram CSCE 515 – Computer Network Programming UDP
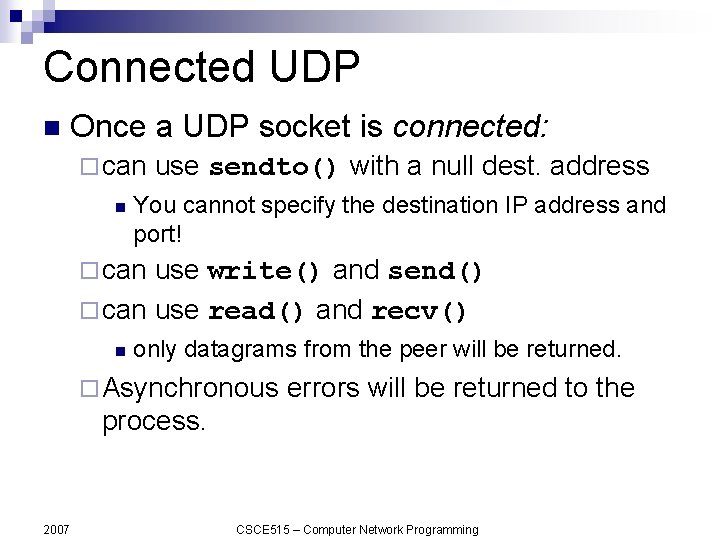
Connected UDP n Once a UDP socket is connected: ¨ can n use sendto() with a null dest. address You cannot specify the destination IP address and port! ¨ can use write() and send() ¨ can use read() and recv() n only datagrams from the peer will be returned. ¨ Asynchronous errors will be returned to the process. 2007 CSCE 515 – Computer Network Programming
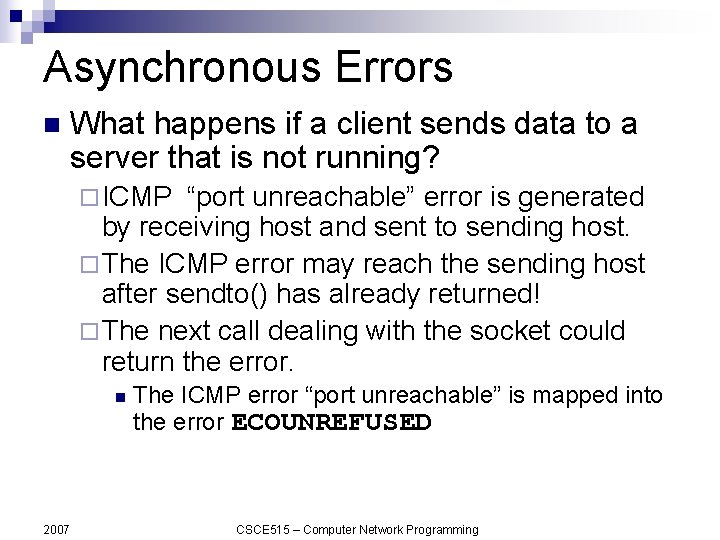
Asynchronous Errors n What happens if a client sends data to a server that is not running? ¨ ICMP “port unreachable” error is generated by receiving host and sent to sending host. ¨ The ICMP error may reach the sending host after sendto() has already returned! ¨ The next call dealing with the socket could return the error. n 2007 The ICMP error “port unreachable” is mapped into the error ECOUNREFUSED CSCE 515 – Computer Network Programming
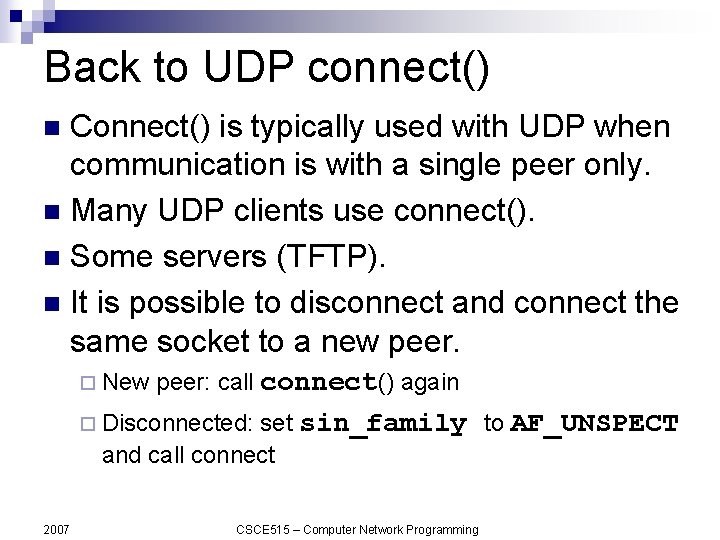
Back to UDP connect() Connect() is typically used with UDP when communication is with a single peer only. n Many UDP clients use connect(). n Some servers (TFTP). n It is possible to disconnect and connect the same socket to a new peer. n ¨ New peer: call connect() again set sin_family to AF_UNSPECT and call connect ¨ Disconnected: 2007 CSCE 515 – Computer Network Programming
Udp socket programming in java
Gqoute
Dai yuqiang
Csce 481
Sock_dgram
Tcp udp
C++ udp socket
Udp socket timeout
뮤텍스 세마포어
Socket connection
Bind function in socket programming
What is socket
Bsd socket programming
R socket programming
Berkeley socket primitives
Types of network topology
6 sda hymnal
Encm 515
Encm 515
Encm 515
Cis 515 upenn
Ieee 515
515
Mit 1025
515 west hastings street
Encm 517