Control structure is divided into three parts v
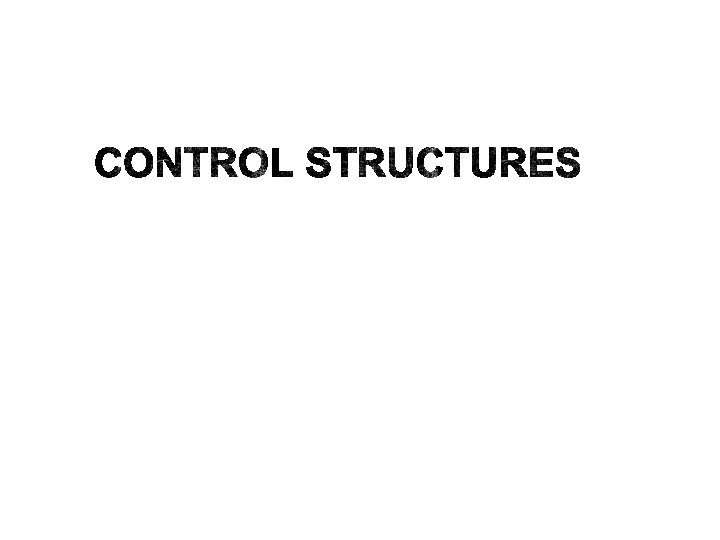
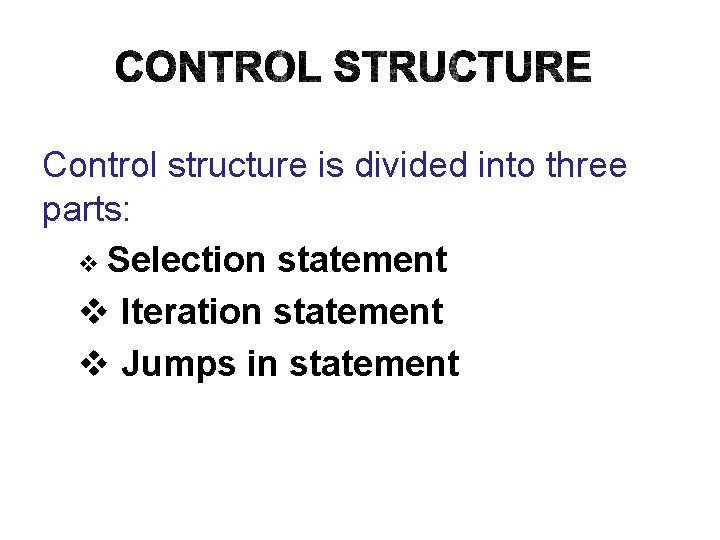
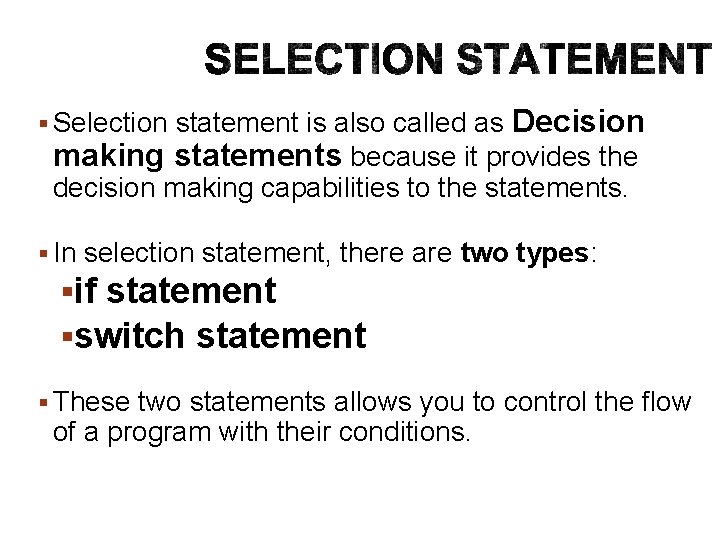
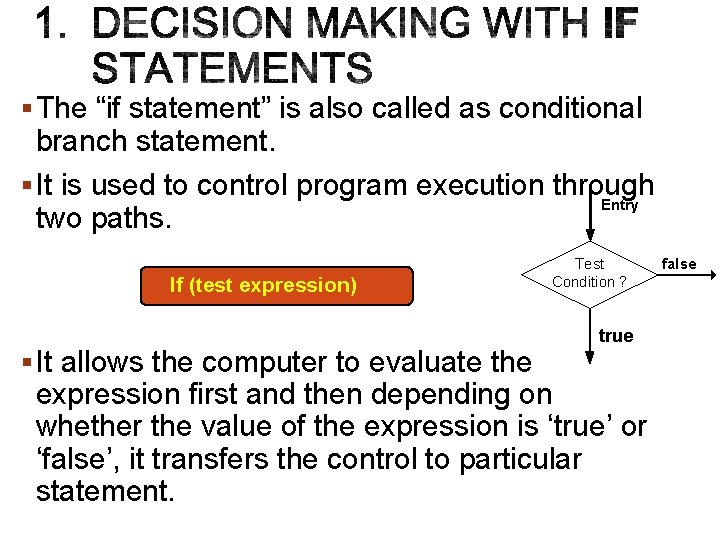
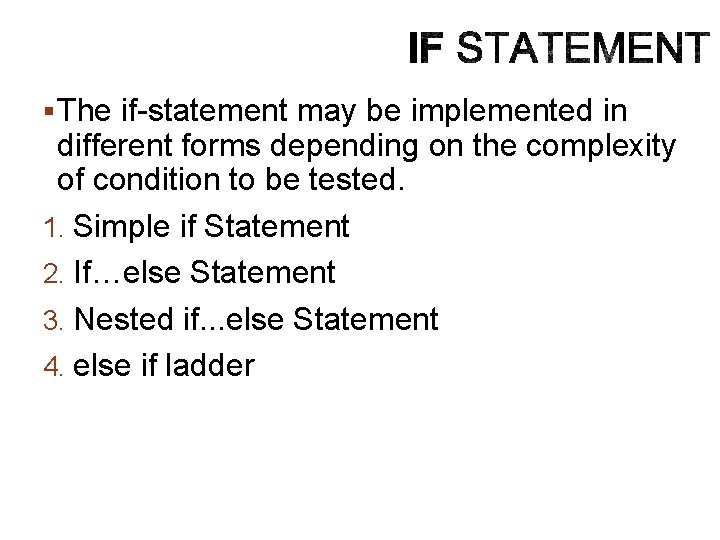
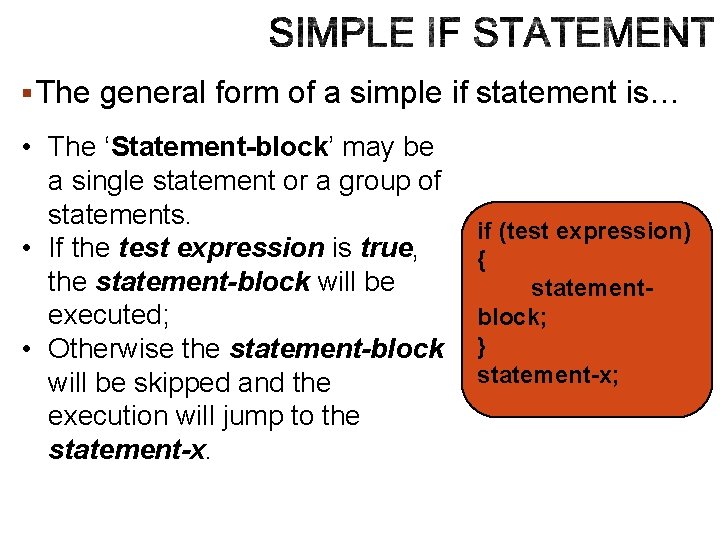
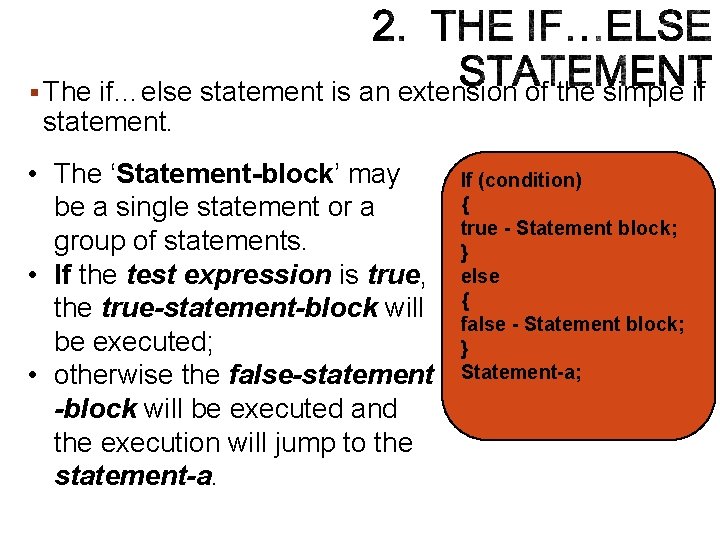
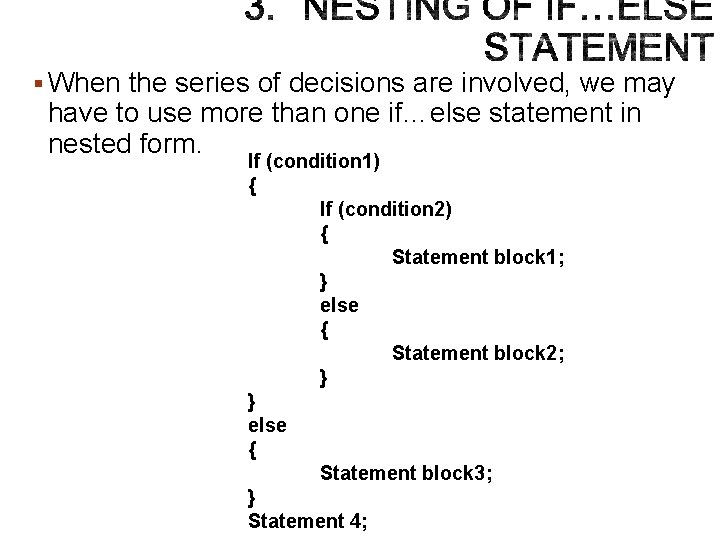
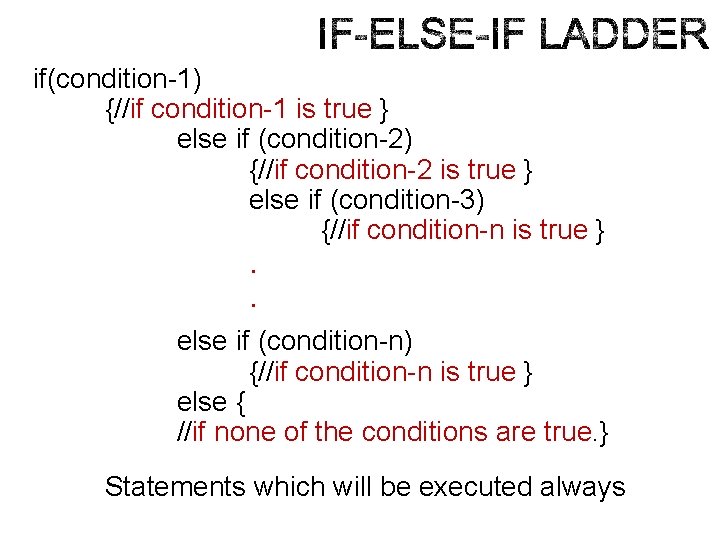
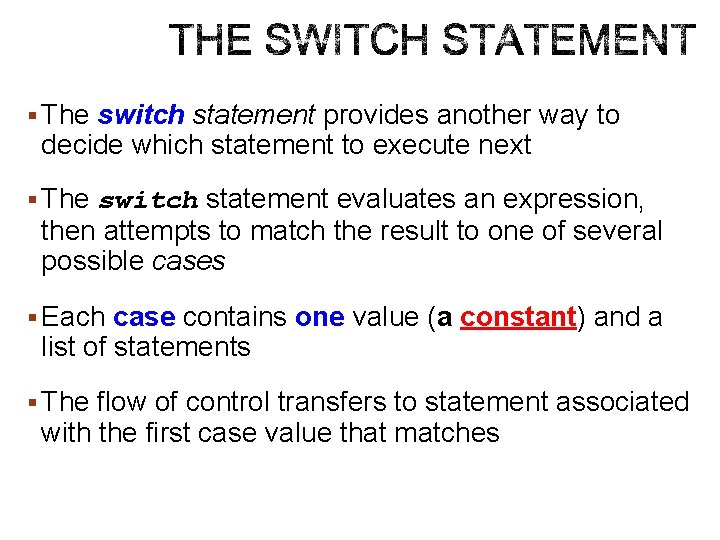
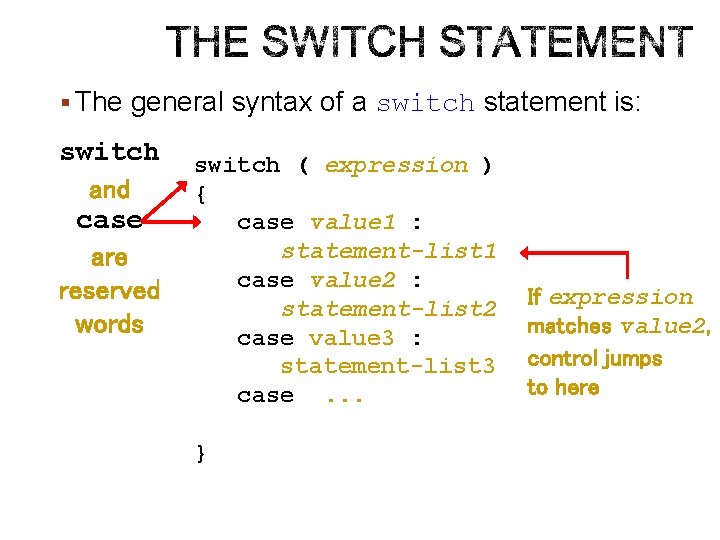
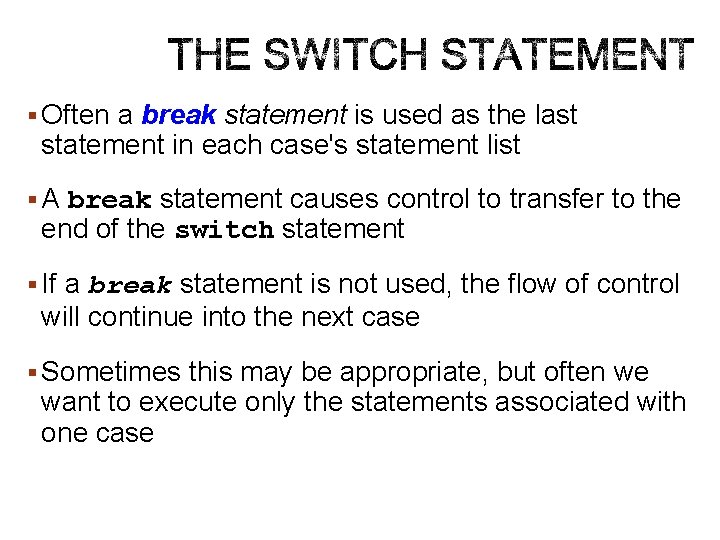
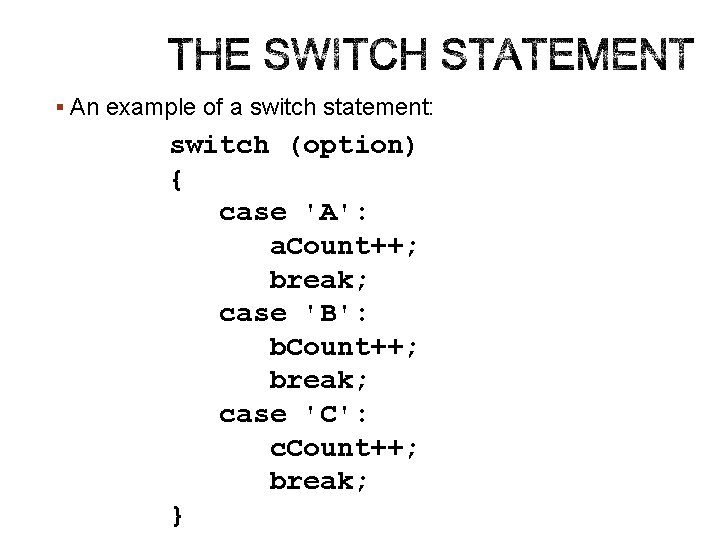
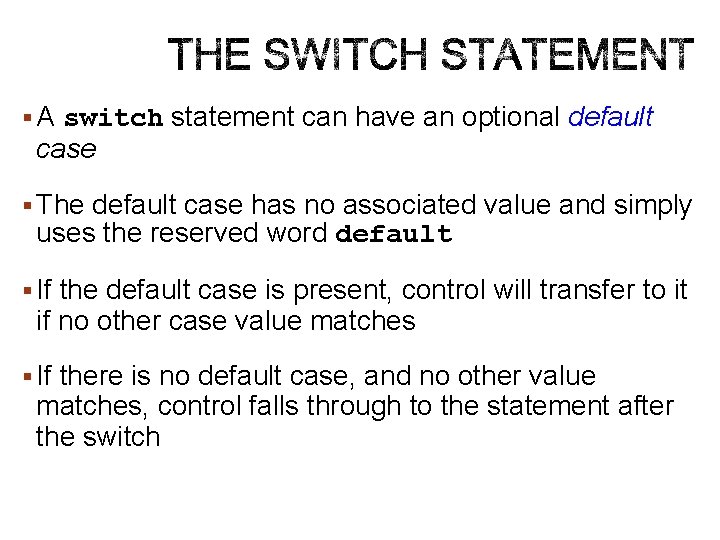
- Slides: 14
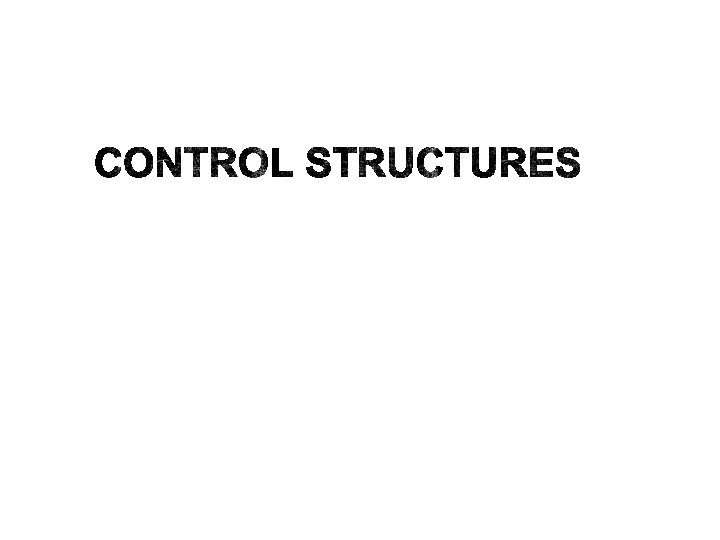
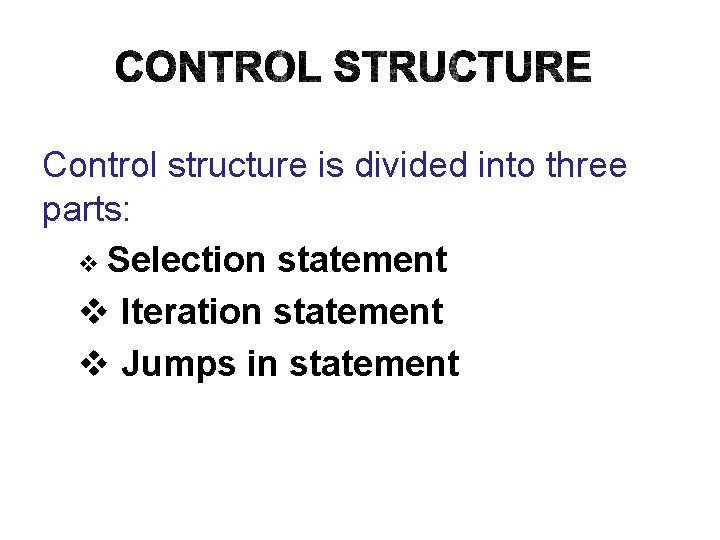
Control structure is divided into three parts: v Selection statement v Iteration statement v Jumps in statement
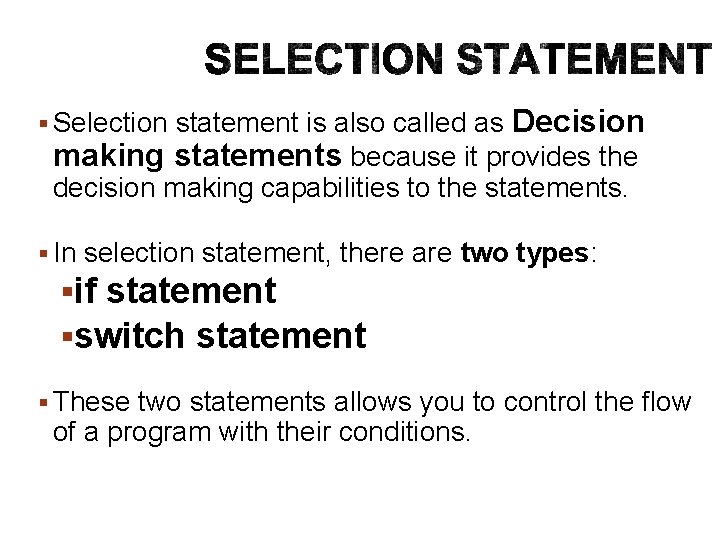
§ Selection statement is also called as Decision making statements because it provides the decision making capabilities to the statements. § In selection statement, there are two types: §if statement §switch statement § These two statements allows you to control the flow of a program with their conditions.
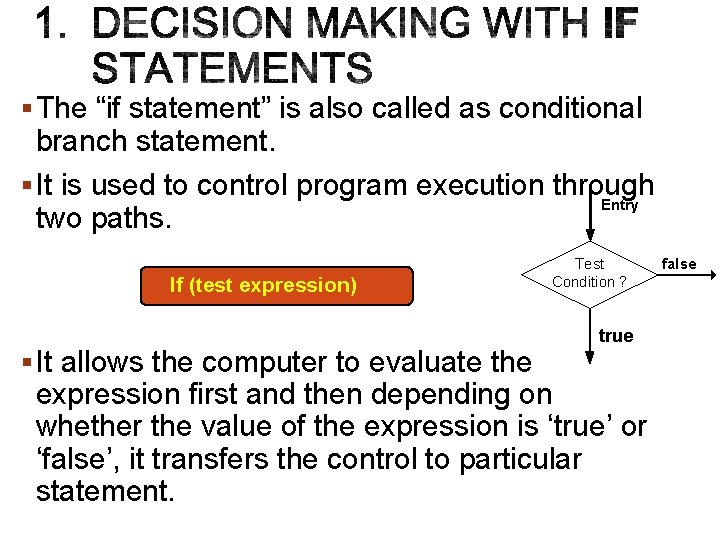
§ The “if statement” is also called as conditional branch statement. § It is used to control program execution through Entry two paths. If (test expression) § It allows the computer to evaluate the Test Condition ? true expression first and then depending on whether the value of the expression is ‘true’ or ‘false’, it transfers the control to particular statement. false
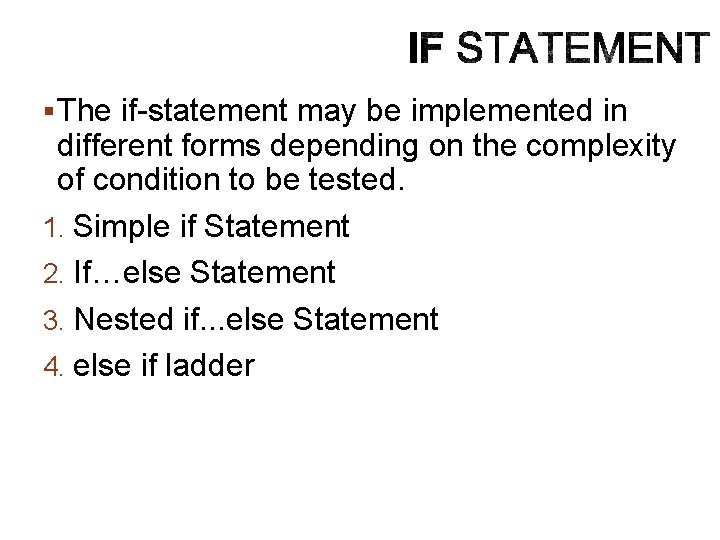
§ The if-statement may be implemented in different forms depending on the complexity of condition to be tested. 1. Simple if Statement 2. If…else Statement 3. Nested if. . . else Statement 4. else if ladder
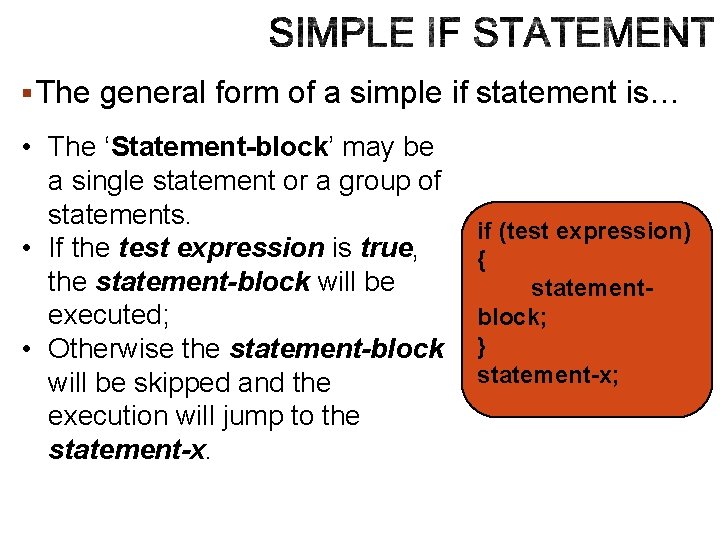
§ The general form of a simple if statement is… • The ‘Statement-block’ may be a single statement or a group of statements. • If the test expression is true, the statement-block will be executed; • Otherwise the statement-block will be skipped and the execution will jump to the statement-x. if (test expression) { statementblock; } statement-x;
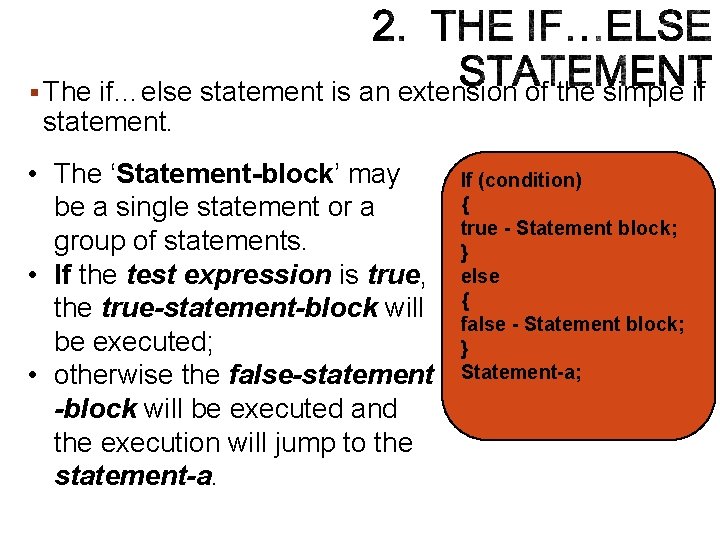
§ The if…else statement is an extension of the simple if statement. • The ‘Statement-block’ may be a single statement or a group of statements. • If the test expression is true, the true-statement-block will be executed; • otherwise the false-statement -block will be executed and the execution will jump to the statement-a. If (condition) { true - Statement block; } else { false - Statement block; } Statement-a;
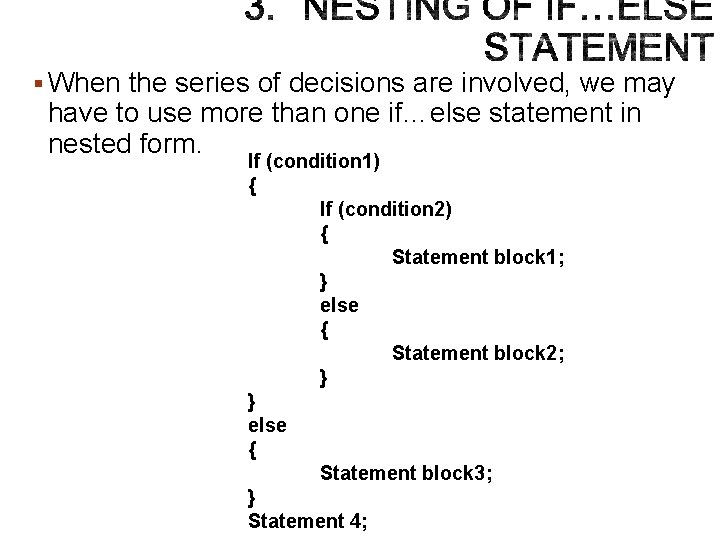
§ When the series of decisions are involved, we may have to use more than one if…else statement in nested form. If (condition 1) { If (condition 2) { Statement block 1; } else { Statement block 2; } } else { Statement block 3; } Statement 4;
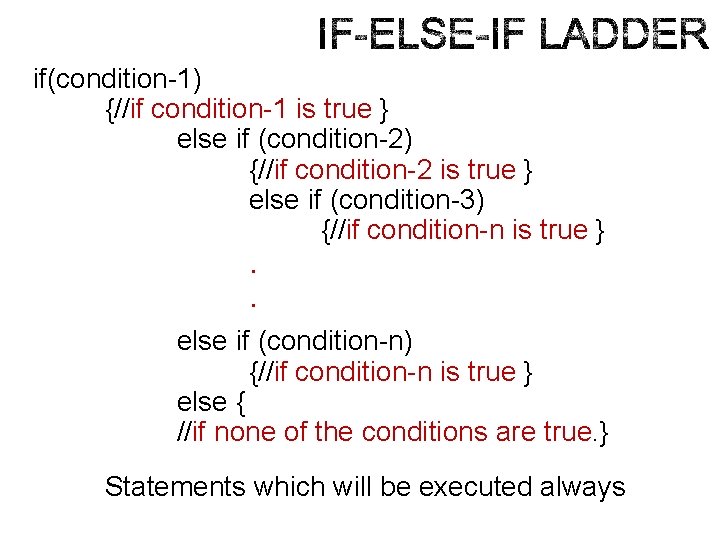
if(condition-1) {//if condition-1 is true } else if (condition-2) {//if condition-2 is true } else if (condition-3) {//if condition-n is true } . . else if (condition-n) {//if condition-n is true } else { //if none of the conditions are true. } Statements which will be executed always
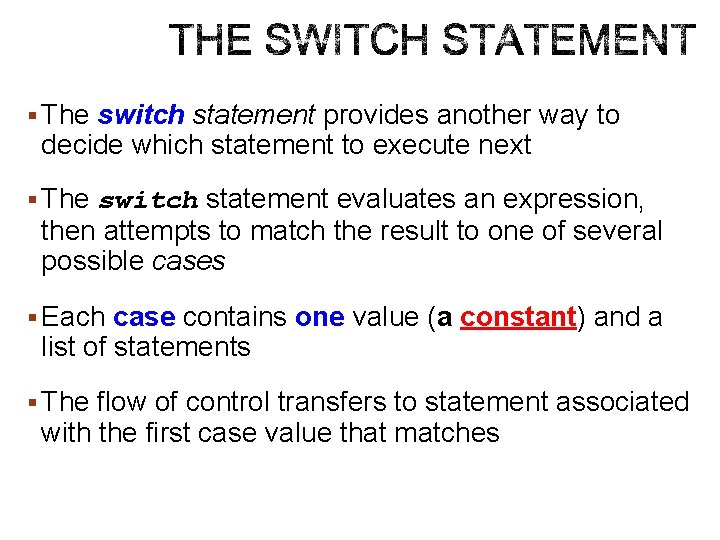
§ The switch statement provides another way to decide which statement to execute next § The switch statement evaluates an expression, then attempts to match the result to one of several possible cases § Each case contains one value (a constant) and a list of statements § The flow of control transfers to statement associated with the first case value that matches
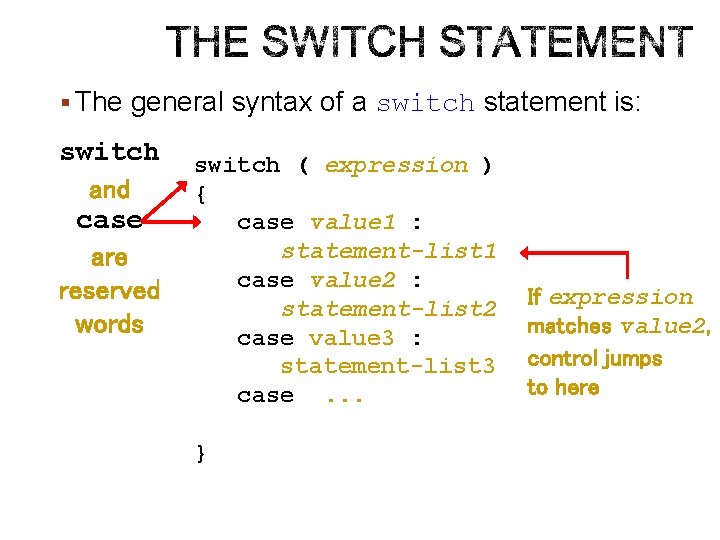
§ The general syntax of a switch statement is: switch and case are reserved words switch ( expression ) { case value 1 : statement-list 1 case value 2 : statement-list 2 case value 3 : statement-list 3 case. . . } If expression matches value 2, control jumps to here
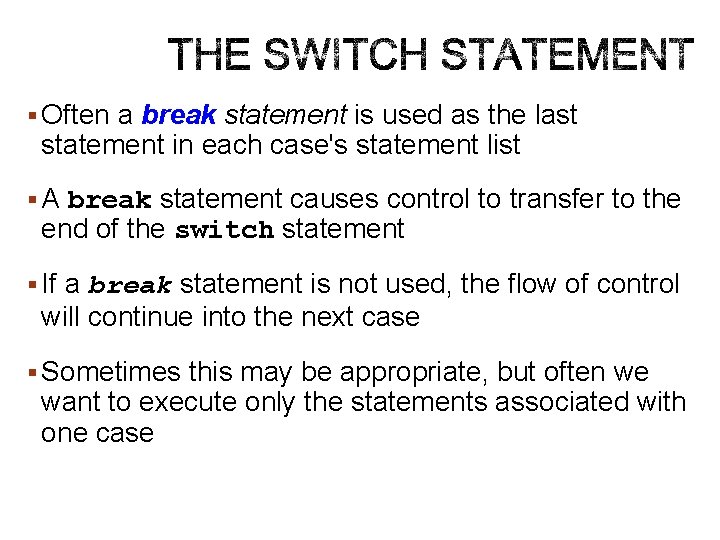
§ Often a break statement is used as the last statement in each case's statement list § A break statement causes control to transfer to the end of the switch statement § If a break statement is not used, the flow of control will continue into the next case § Sometimes this may be appropriate, but often we want to execute only the statements associated with one case
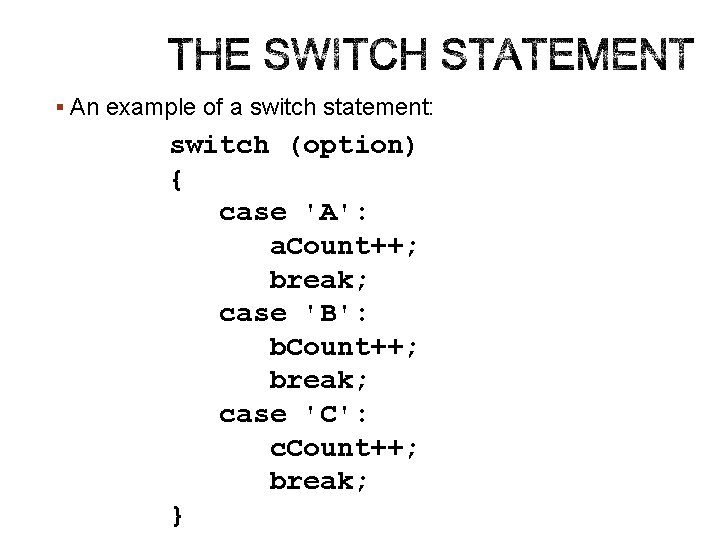
§ An example of a switch statement: switch (option) { case 'A': a. Count++; break; case 'B': b. Count++; break; case 'C': c. Count++; break; }
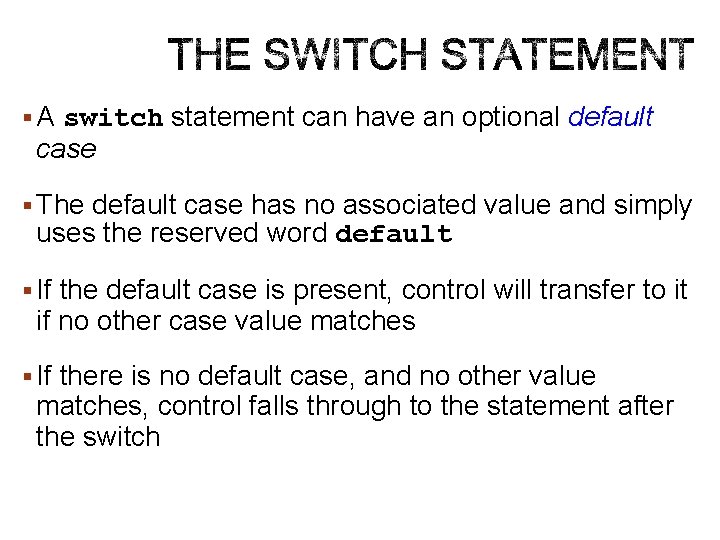
§ A switch statement can have an optional default case § The default case has no associated value and simply uses the reserved word default § If the default case is present, control will transfer to it if no other case value matches § If there is no default case, and no other value matches, control falls through to the statement after the switch
Divided into three parts
William wordsworth is known as a
What are the districts in paris called
Divided into three categories
Computer divided into two parts
Into what parts is scotland divided geographically
Divine comedy 3 parts
The gsm network is divided into
Latin verbs are divided into
Seed plants are divided into two groups called
3 layers of the earth
India can be divided into how many regions
Aca student liability insurance
The text is divided into 2 main themes:
Kingdoms of israel and judah