Computer Science 340 Software Design Testing Inheritance 1
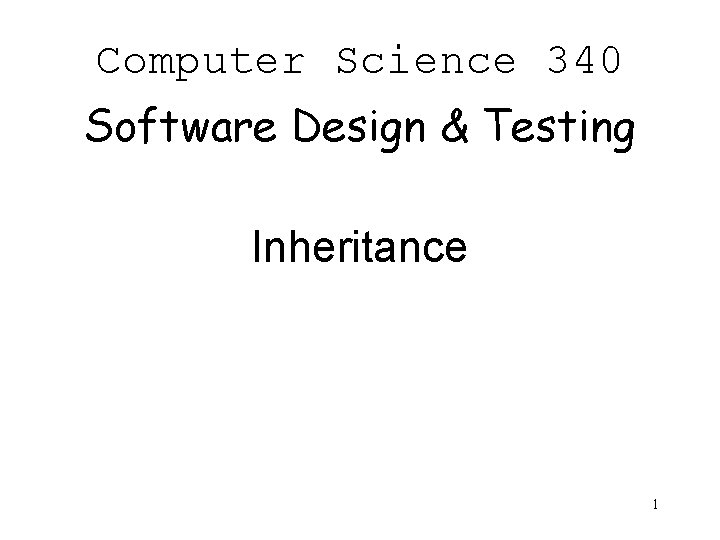
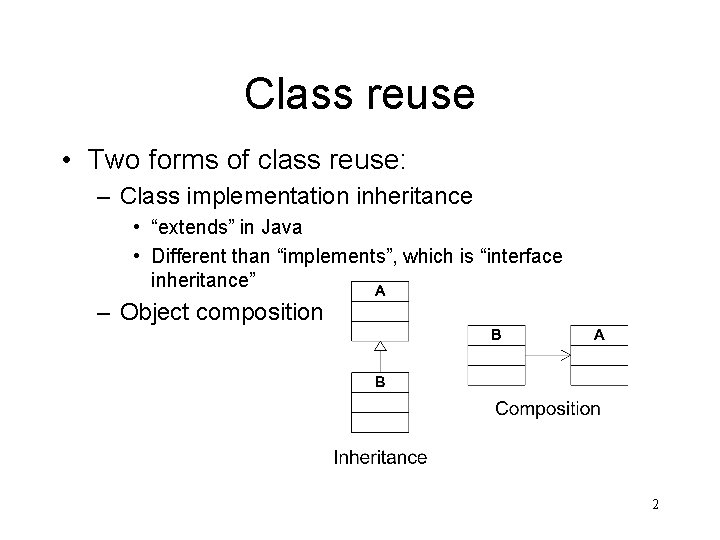
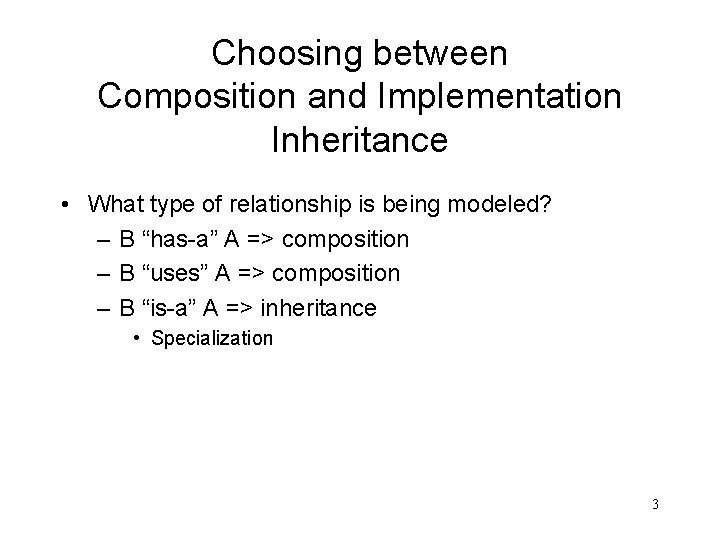
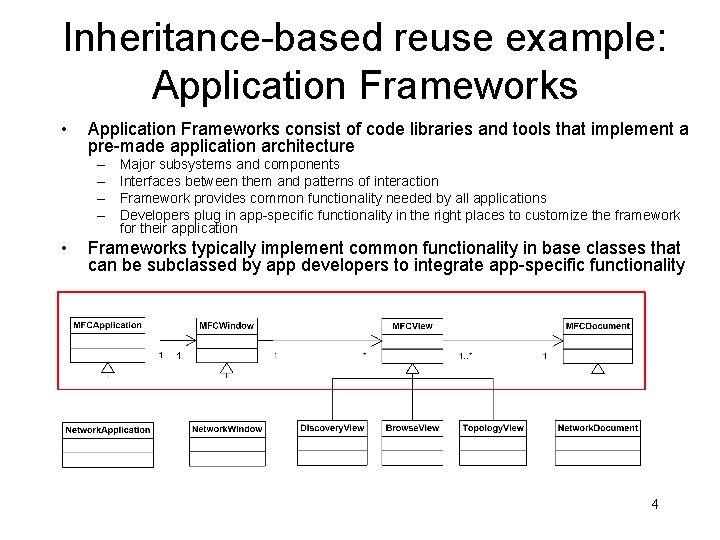
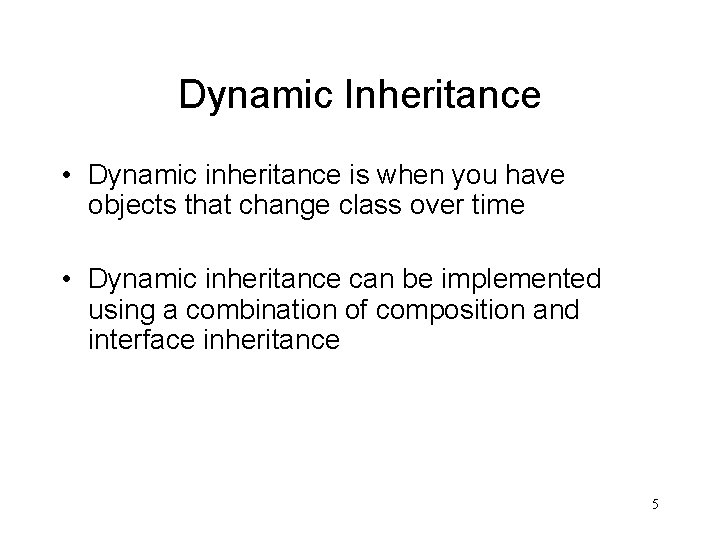
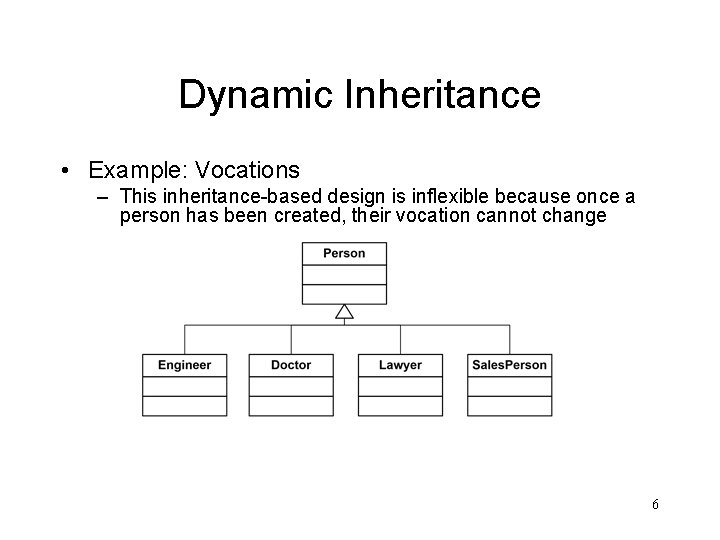
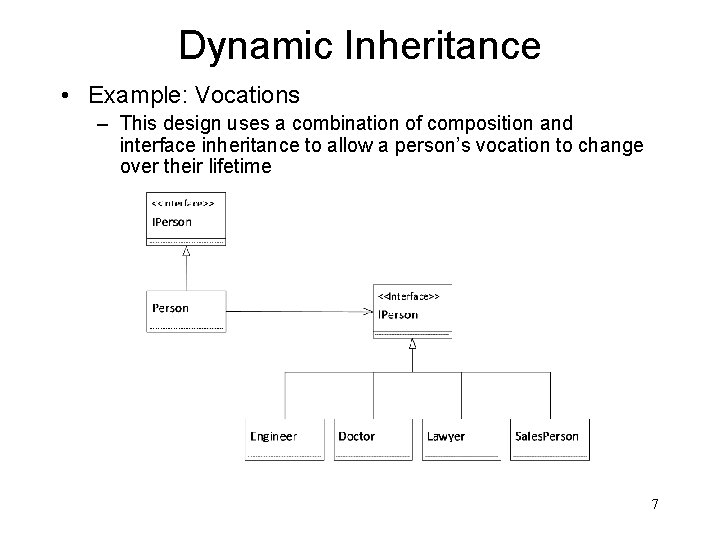
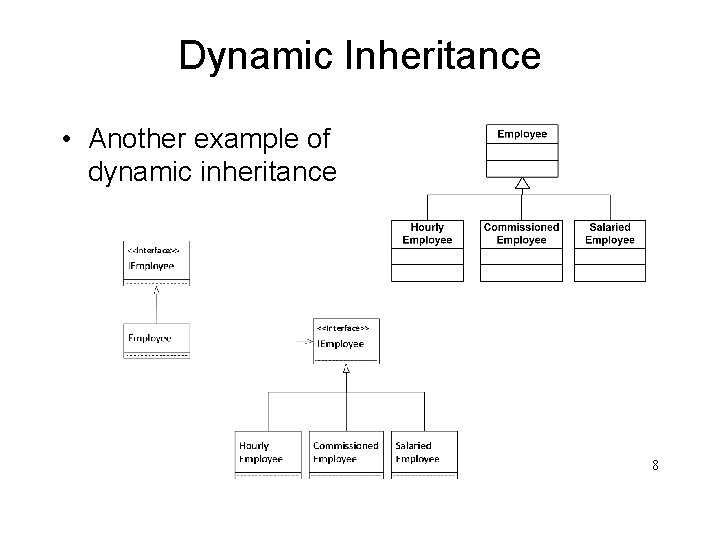
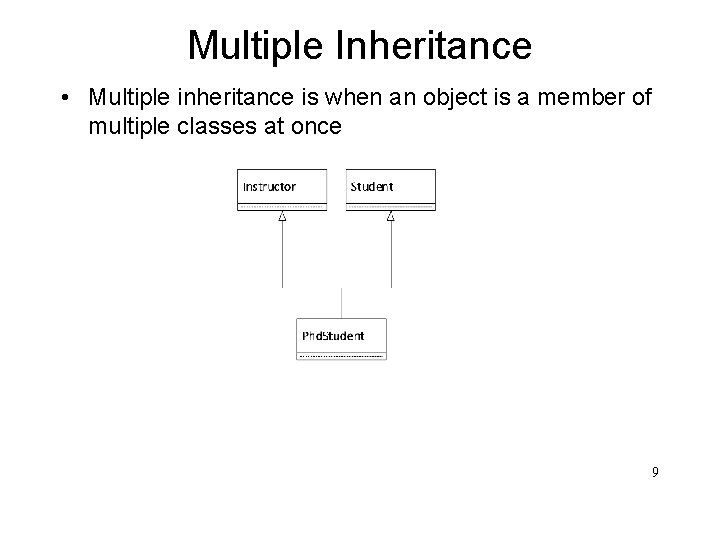
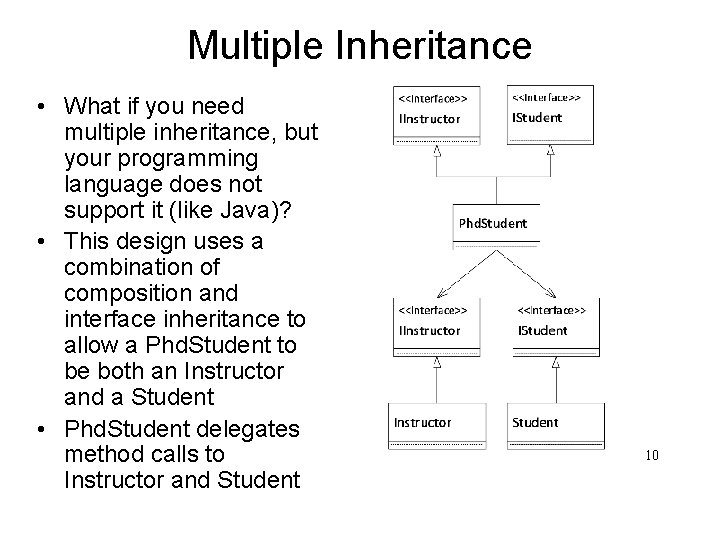
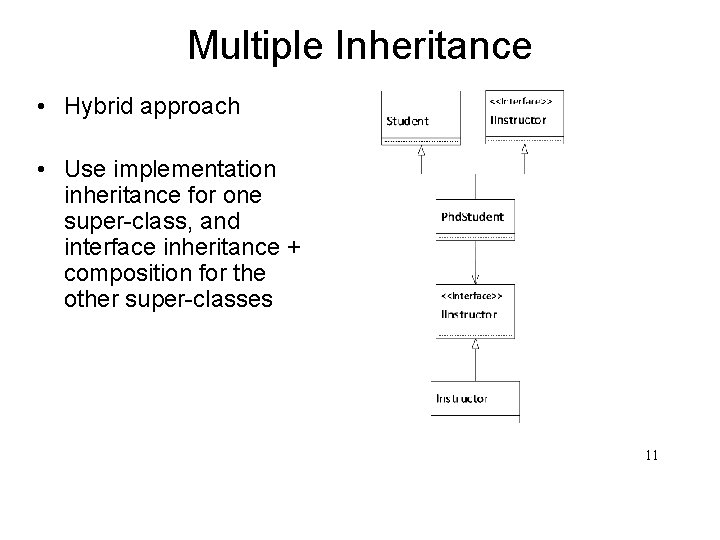
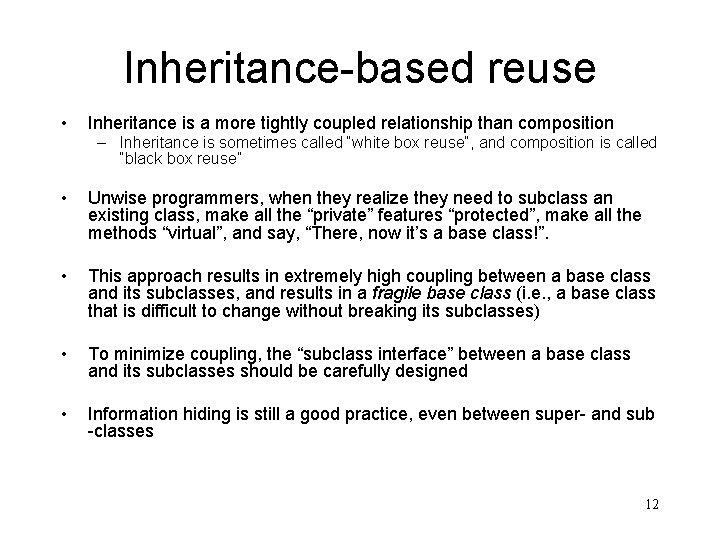
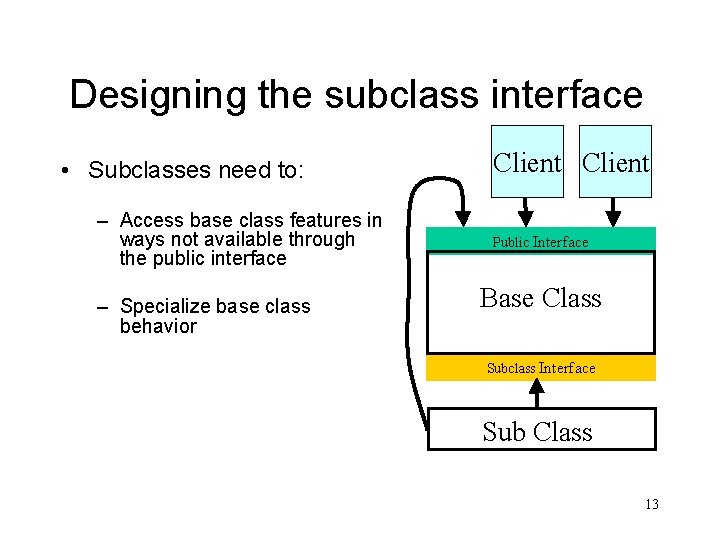
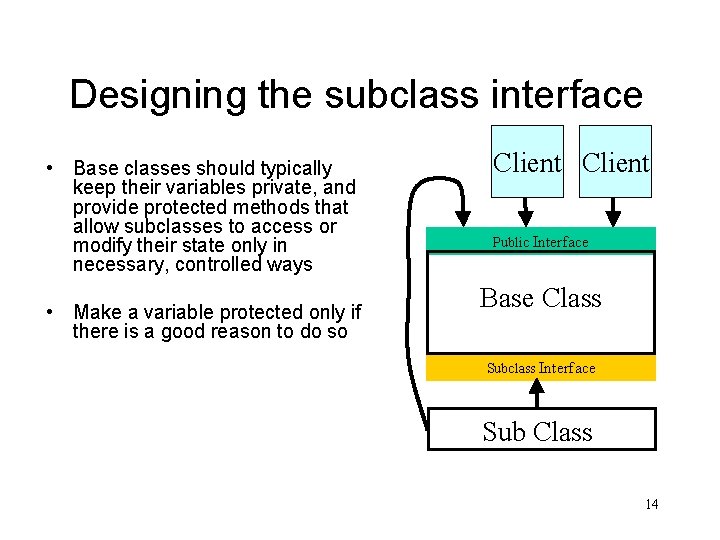
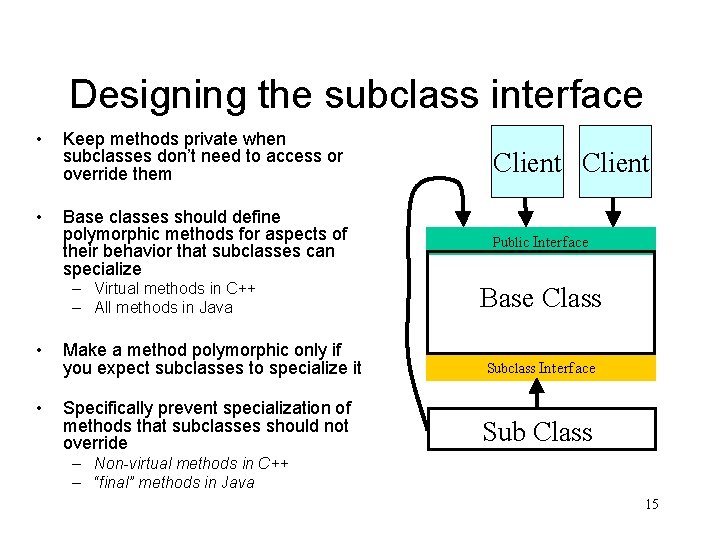
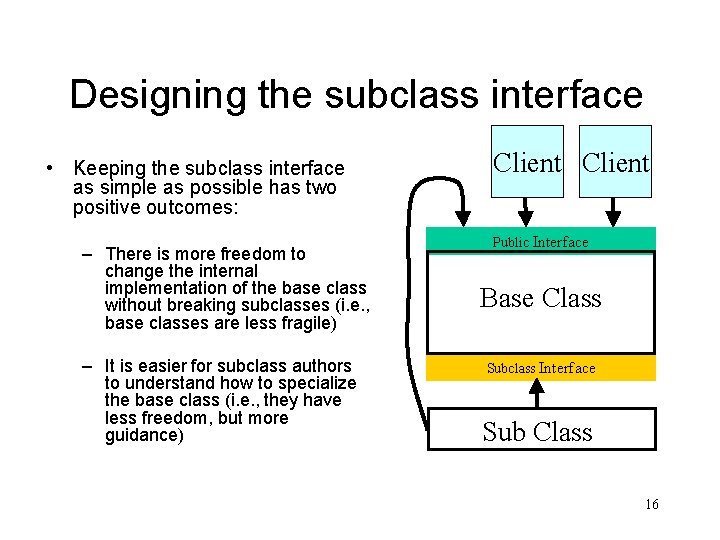
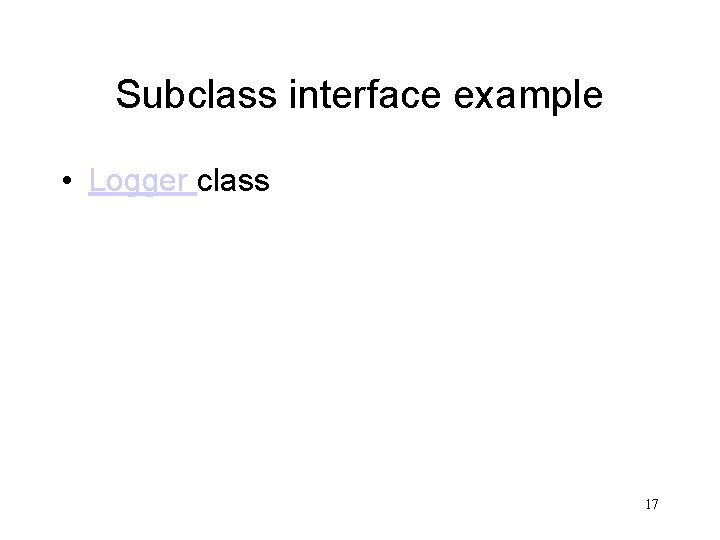
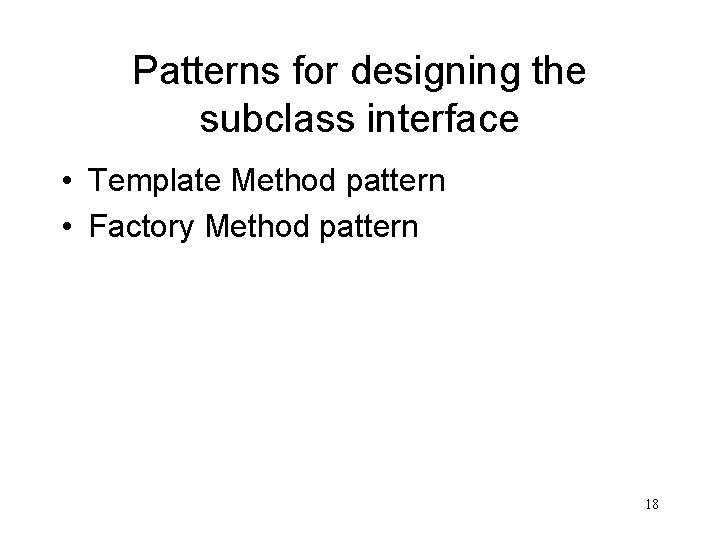
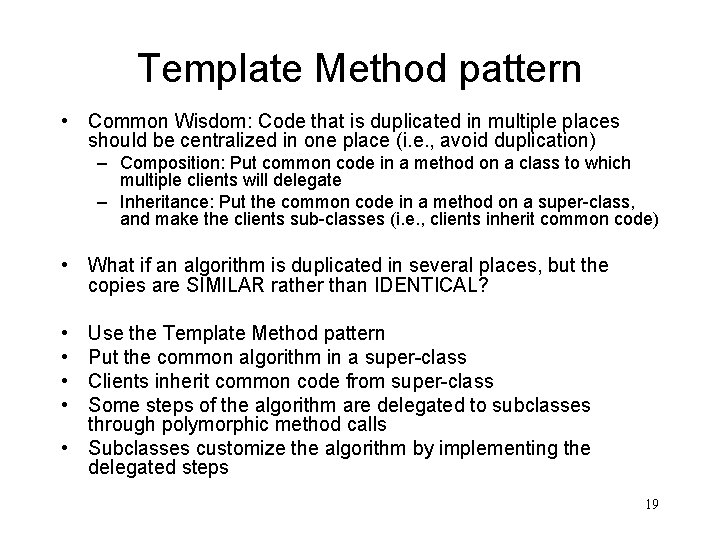
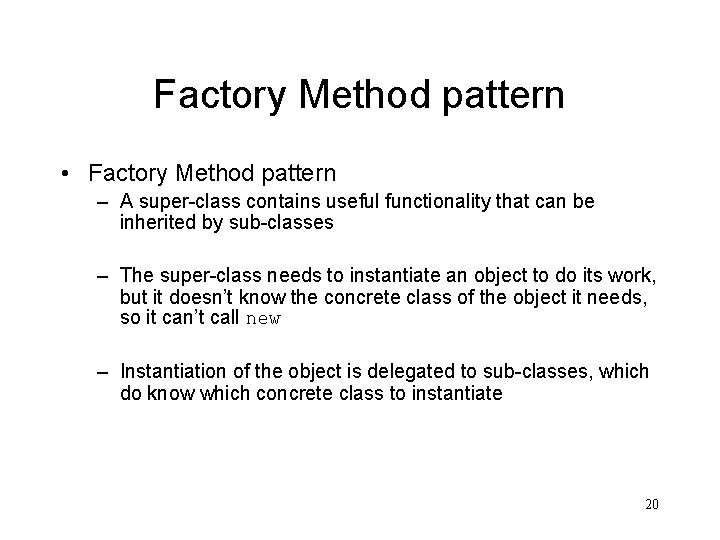
- Slides: 20
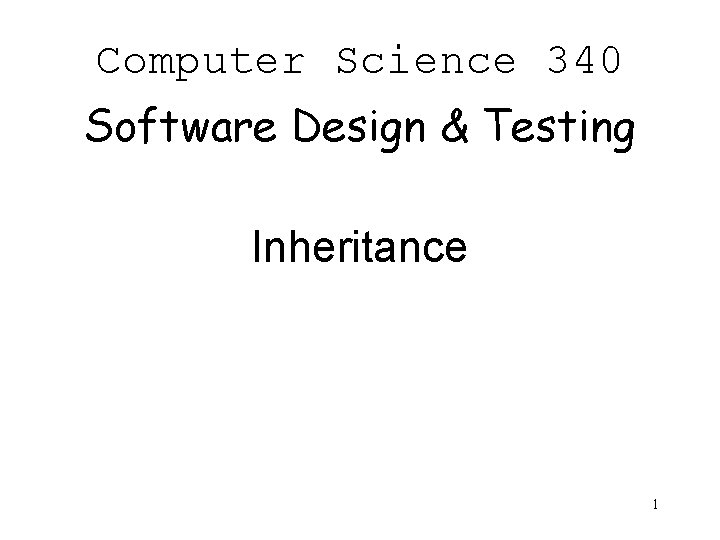
Computer Science 340 Software Design & Testing Inheritance 1
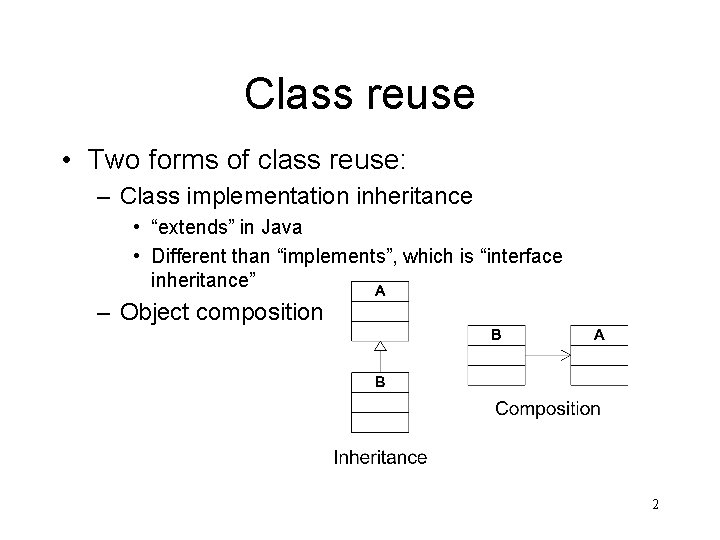
Class reuse • Two forms of class reuse: – Class implementation inheritance • “extends” in Java • Different than “implements”, which is “interface inheritance” – Object composition 2
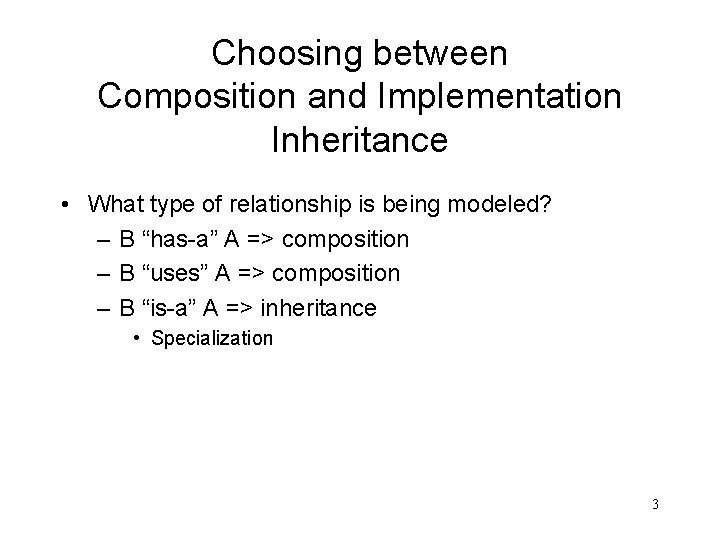
Choosing between Composition and Implementation Inheritance • What type of relationship is being modeled? – B “has-a” A => composition – B “uses” A => composition – B “is-a” A => inheritance • Specialization 3
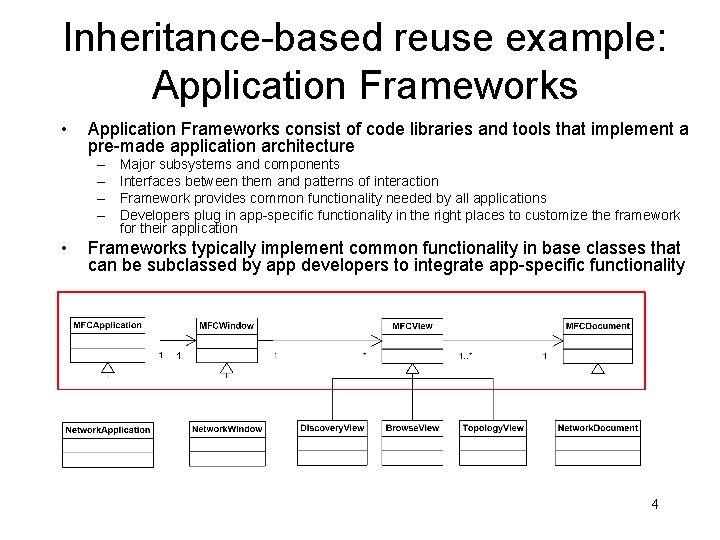
Inheritance-based reuse example: Application Frameworks • Application Frameworks consist of code libraries and tools that implement a pre-made application architecture – – • Major subsystems and components Interfaces between them and patterns of interaction Framework provides common functionality needed by all applications Developers plug in app-specific functionality in the right places to customize the framework for their application Frameworks typically implement common functionality in base classes that can be subclassed by app developers to integrate app-specific functionality 4
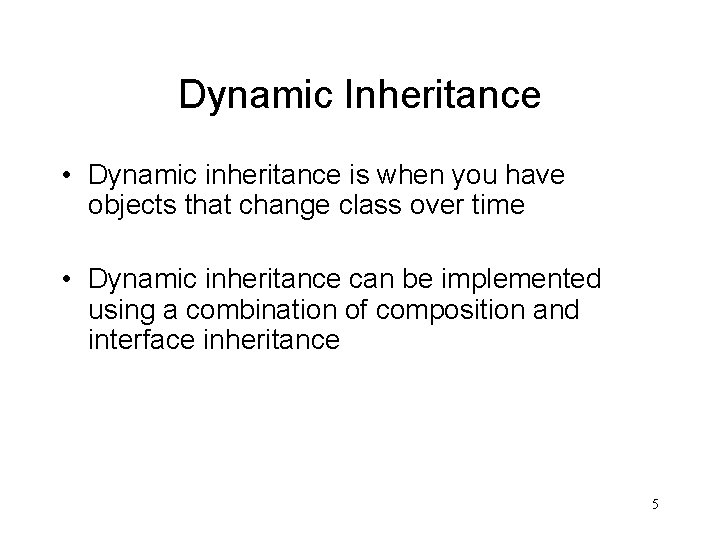
Dynamic Inheritance • Dynamic inheritance is when you have objects that change class over time • Dynamic inheritance can be implemented using a combination of composition and interface inheritance 5
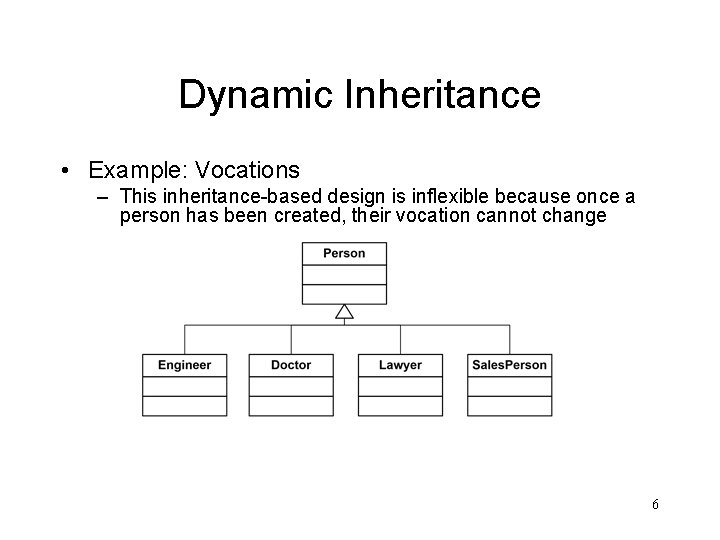
Dynamic Inheritance • Example: Vocations – This inheritance-based design is inflexible because once a person has been created, their vocation cannot change 6
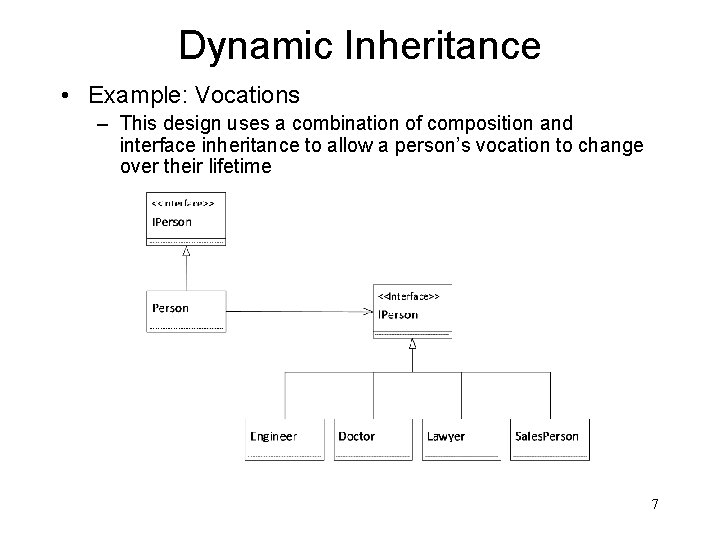
Dynamic Inheritance • Example: Vocations – This design uses a combination of composition and interface inheritance to allow a person’s vocation to change over their lifetime 7
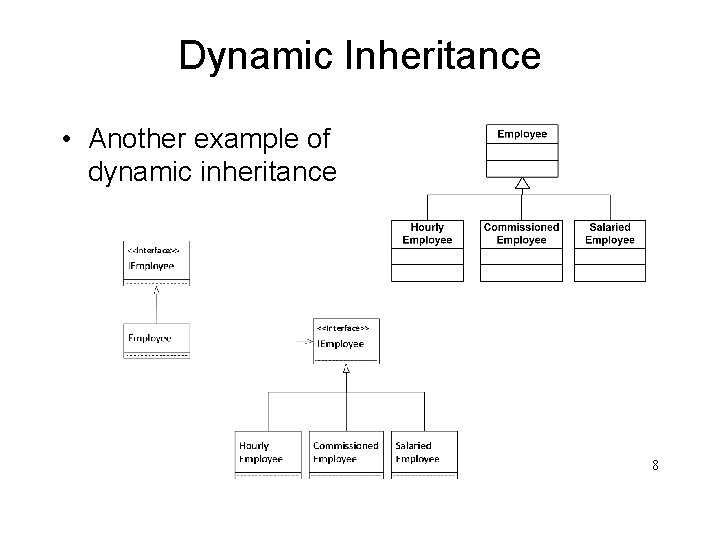
Dynamic Inheritance • Another example of dynamic inheritance 8
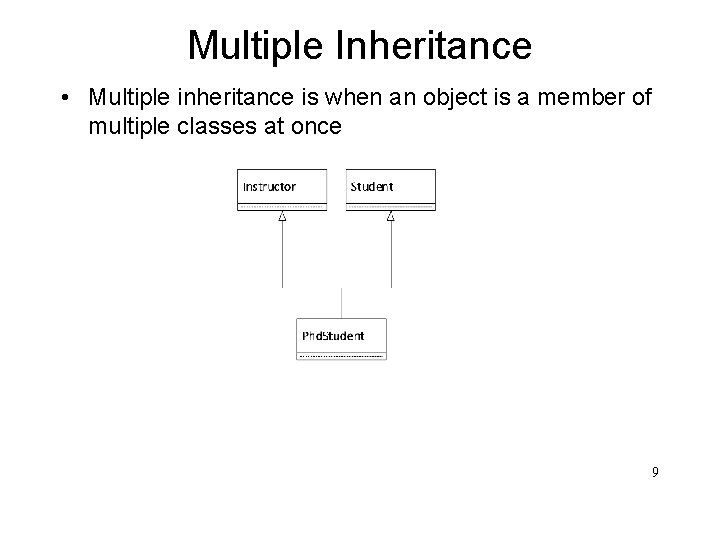
Multiple Inheritance • Multiple inheritance is when an object is a member of multiple classes at once 9
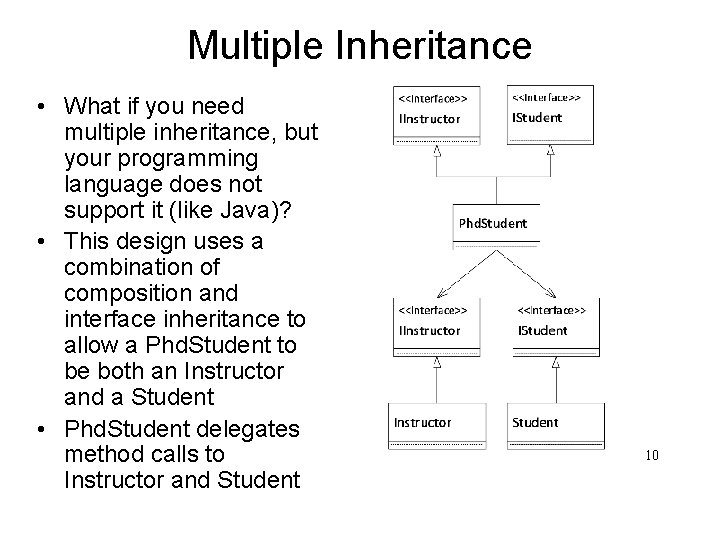
Multiple Inheritance • What if you need multiple inheritance, but your programming language does not support it (like Java)? • This design uses a combination of composition and interface inheritance to allow a Phd. Student to be both an Instructor and a Student • Phd. Student delegates method calls to Instructor and Student 10
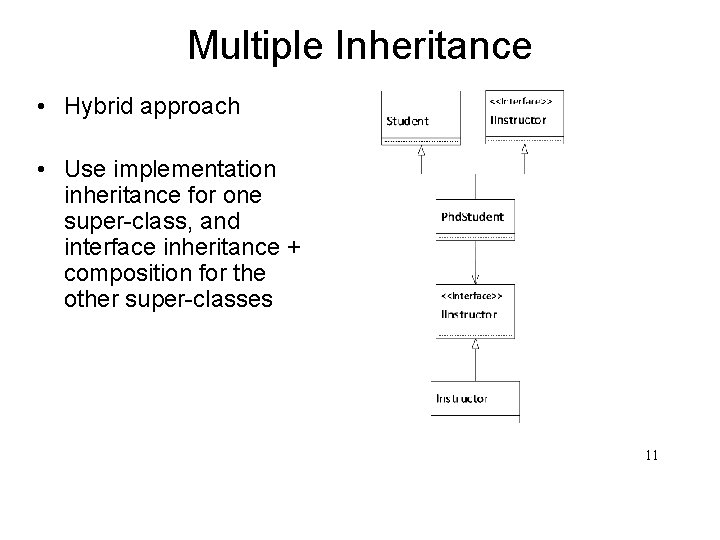
Multiple Inheritance • Hybrid approach • Use implementation inheritance for one super-class, and interface inheritance + composition for the other super-classes 11
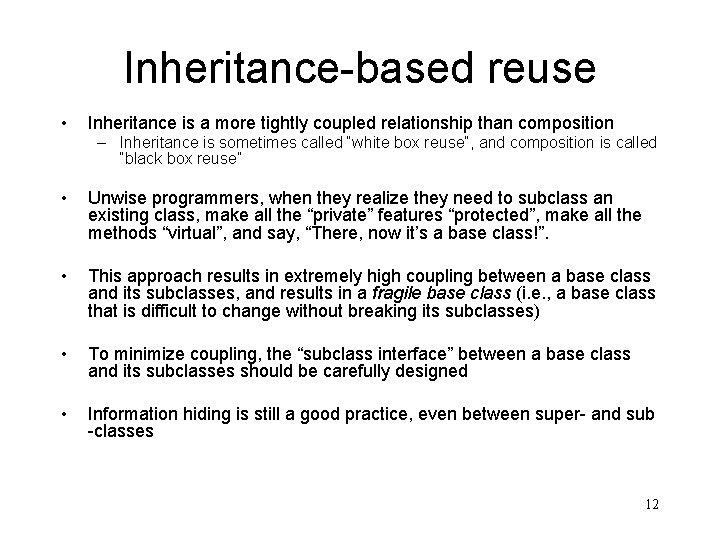
Inheritance-based reuse • Inheritance is a more tightly coupled relationship than composition – Inheritance is sometimes called “white box reuse”, and composition is called “black box reuse” • Unwise programmers, when they realize they need to subclass an existing class, make all the “private” features “protected”, make all the methods “virtual”, and say, “There, now it’s a base class!”. • This approach results in extremely high coupling between a base class and its subclasses, and results in a fragile base class (i. e. , a base class that is difficult to change without breaking its subclasses) • To minimize coupling, the “subclass interface” between a base class and its subclasses should be carefully designed • Information hiding is still a good practice, even between super- and sub -classes 12
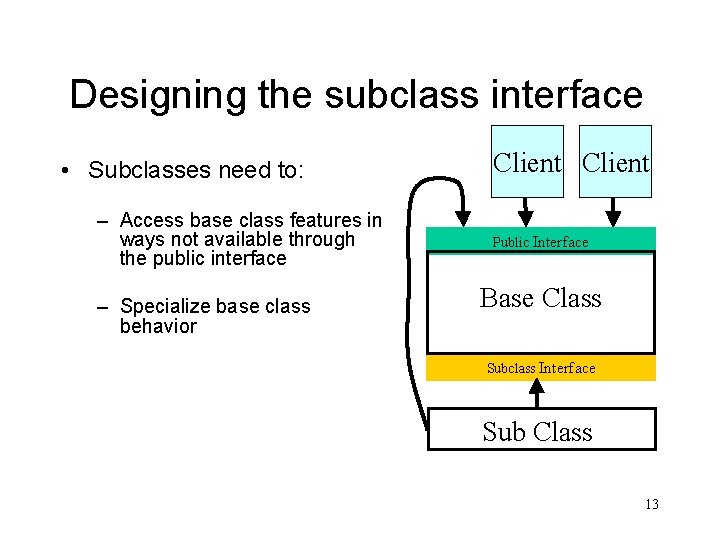
Designing the subclass interface • Subclasses need to: – Access base class features in ways not available through the public interface – Specialize base class behavior Client Public Interface Base Class Subclass Interface Sub Class 13
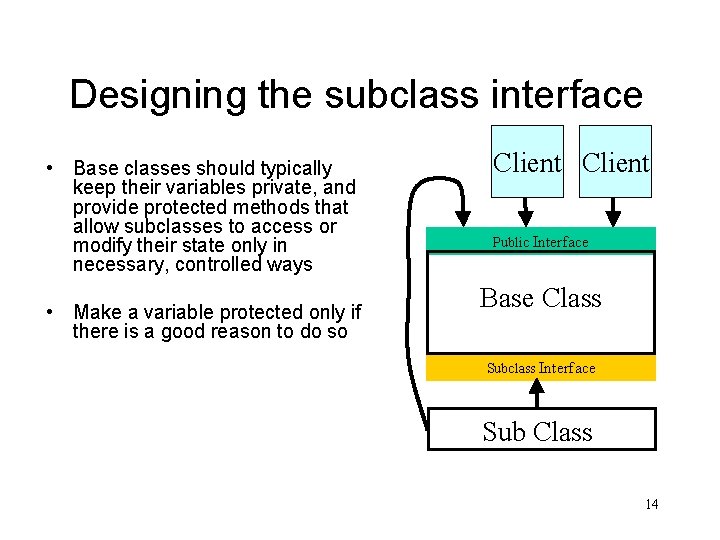
Designing the subclass interface • Base classes should typically keep their variables private, and provide protected methods that allow subclasses to access or modify their state only in necessary, controlled ways • Make a variable protected only if there is a good reason to do so Client Public Interface Base Class Subclass Interface Sub Class 14
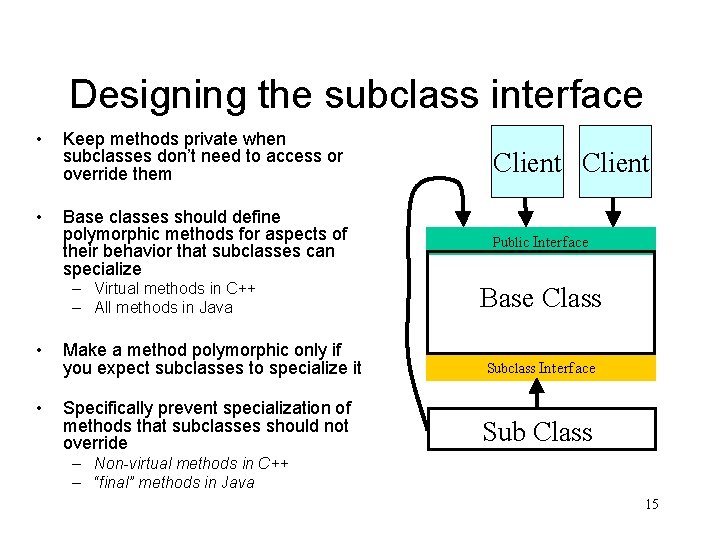
Designing the subclass interface • Keep methods private when subclasses don’t need to access or override them Client • Base classes should define polymorphic methods for aspects of their behavior that subclasses can specialize Public Interface – Virtual methods in C++ – All methods in Java • Make a method polymorphic only if you expect subclasses to specialize it • Specifically prevent specialization of methods that subclasses should not override Base Class Subclass Interface Sub Class – Non-virtual methods in C++ – “final” methods in Java 15
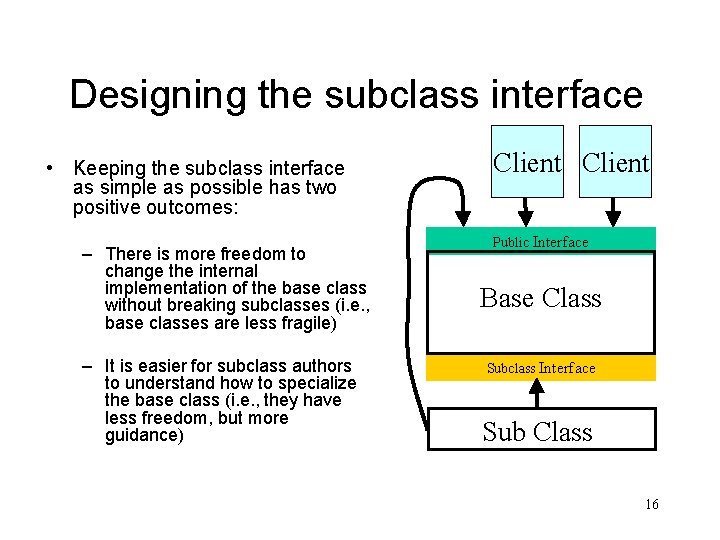
Designing the subclass interface • Keeping the subclass interface as simple as possible has two positive outcomes: – There is more freedom to change the internal implementation of the base class without breaking subclasses (i. e. , base classes are less fragile) – It is easier for subclass authors to understand how to specialize the base class (i. e. , they have less freedom, but more guidance) Client Public Interface Base Class Subclass Interface Sub Class 16
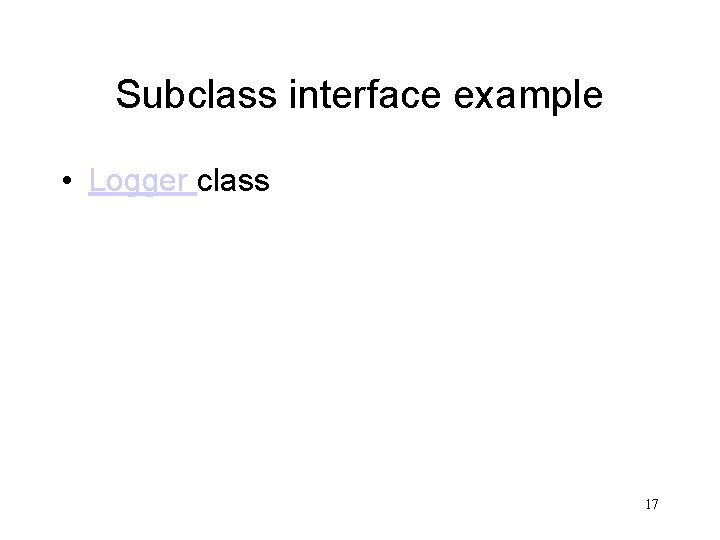
Subclass interface example • Logger class 17
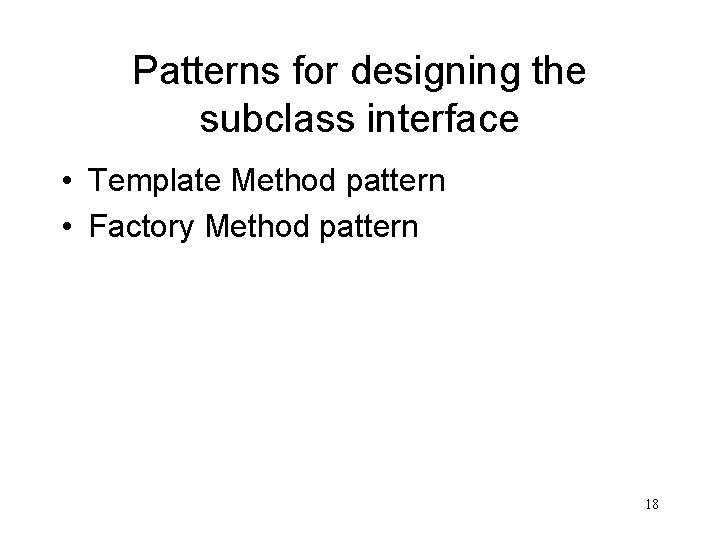
Patterns for designing the subclass interface • Template Method pattern • Factory Method pattern 18
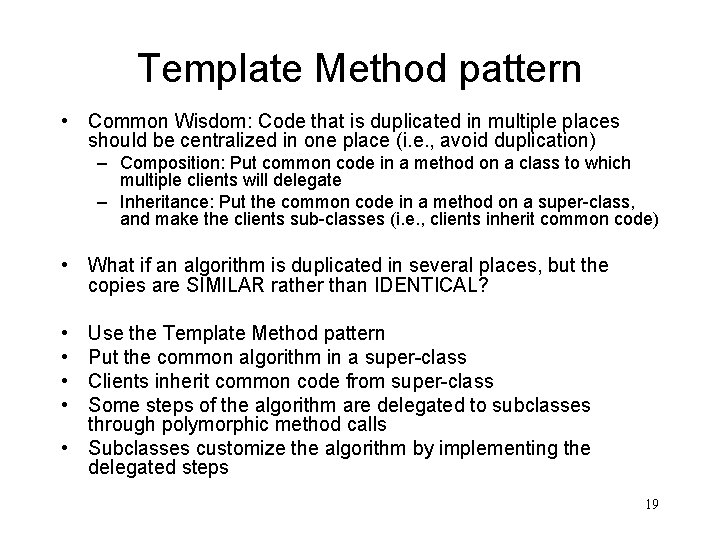
Template Method pattern • Common Wisdom: Code that is duplicated in multiple places should be centralized in one place (i. e. , avoid duplication) – Composition: Put common code in a method on a class to which multiple clients will delegate – Inheritance: Put the common code in a method on a super-class, and make the clients sub-classes (i. e. , clients inherit common code) • What if an algorithm is duplicated in several places, but the copies are SIMILAR rather than IDENTICAL? • • Use the Template Method pattern Put the common algorithm in a super-class Clients inherit common code from super-class Some steps of the algorithm are delegated to subclasses through polymorphic method calls • Subclasses customize the algorithm by implementing the delegated steps 19
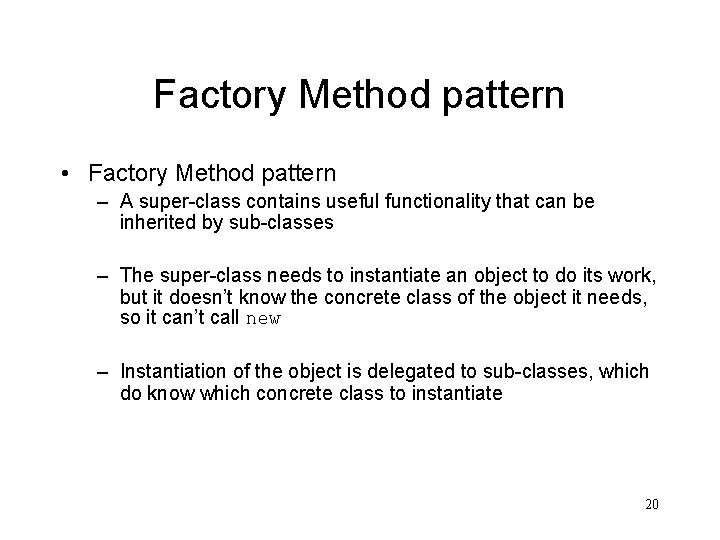
Factory Method pattern • Factory Method pattern – A super-class contains useful functionality that can be inherited by sub-classes – The super-class needs to instantiate an object to do its work, but it doesn’t know the concrete class of the object it needs, so it can’t call new – Instantiation of the object is delegated to sub-classes, which do know which concrete class to instantiate 20