Character Data Type char letter A ASCII char
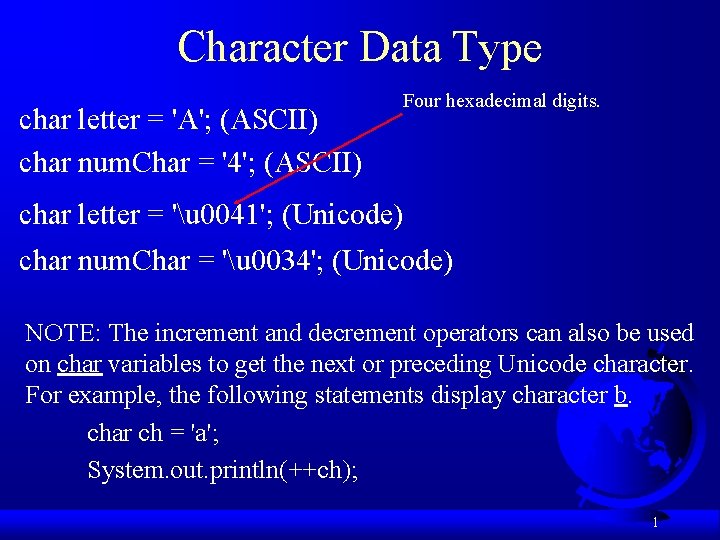
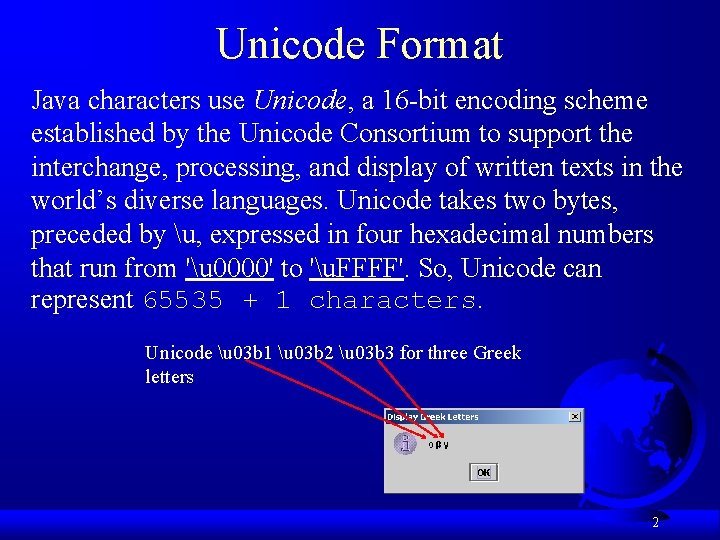
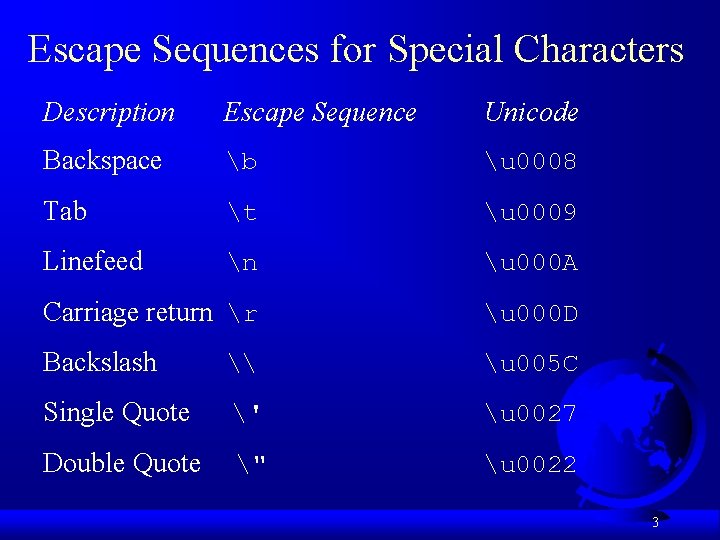
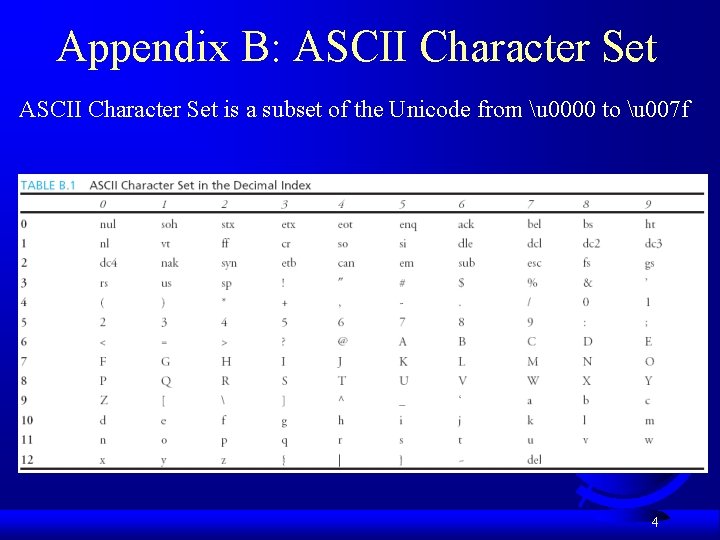
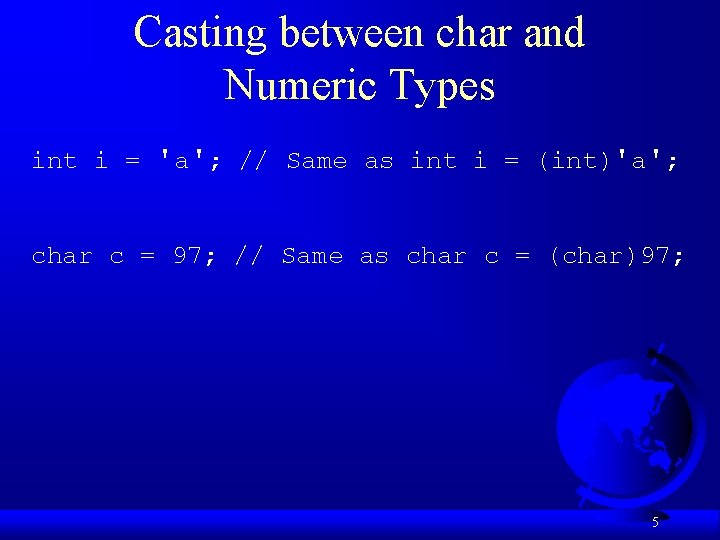
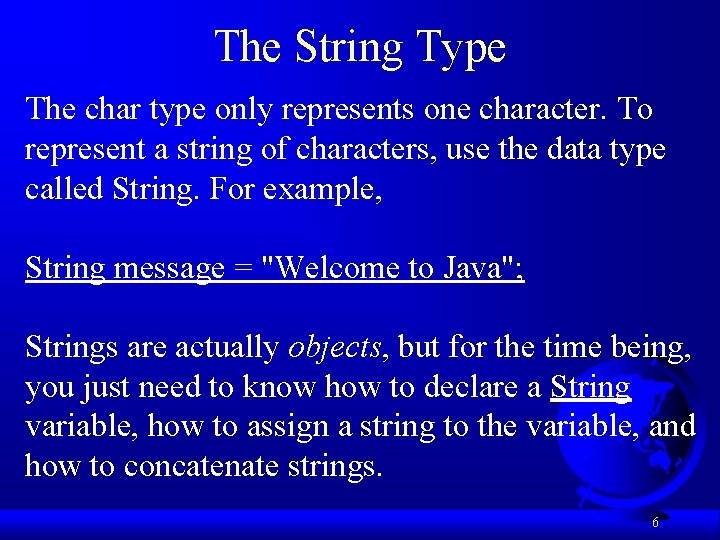
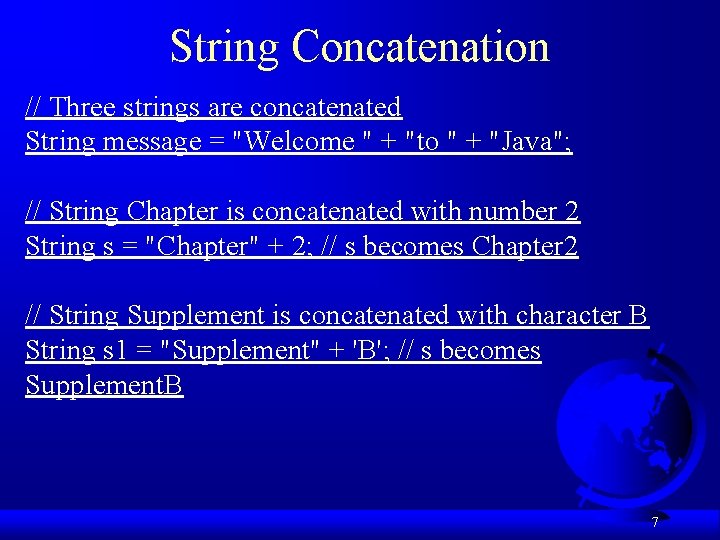
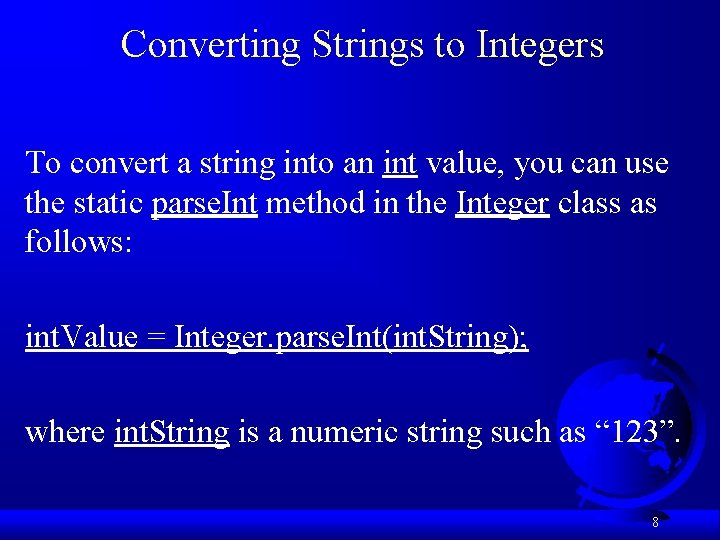
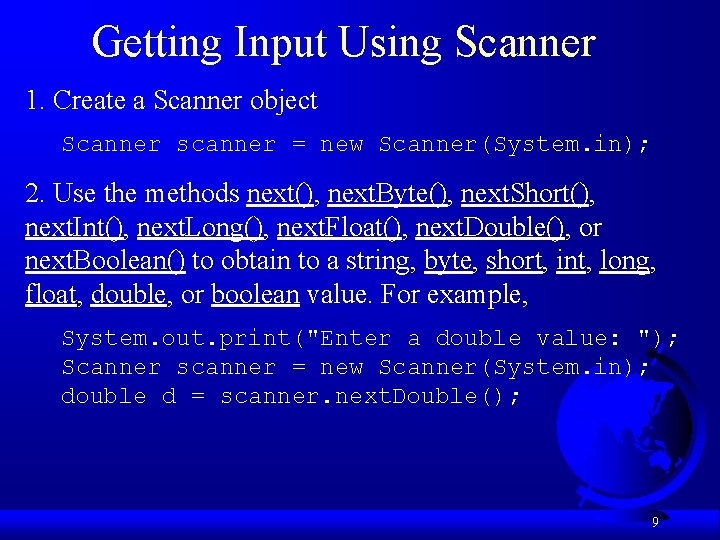
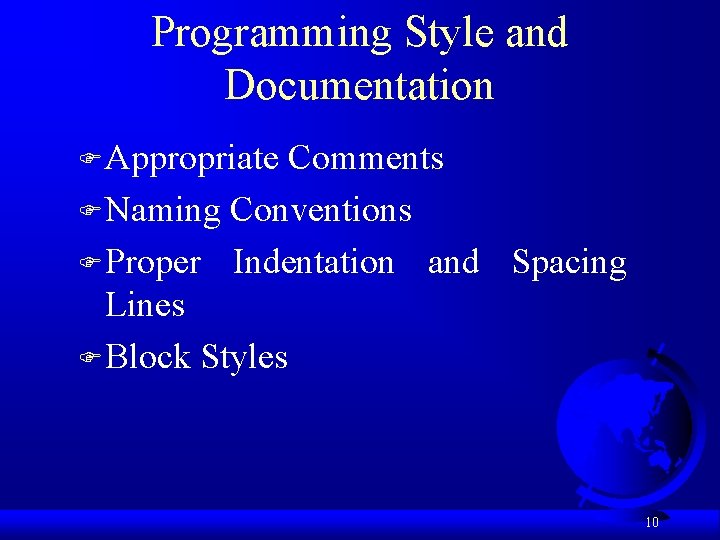
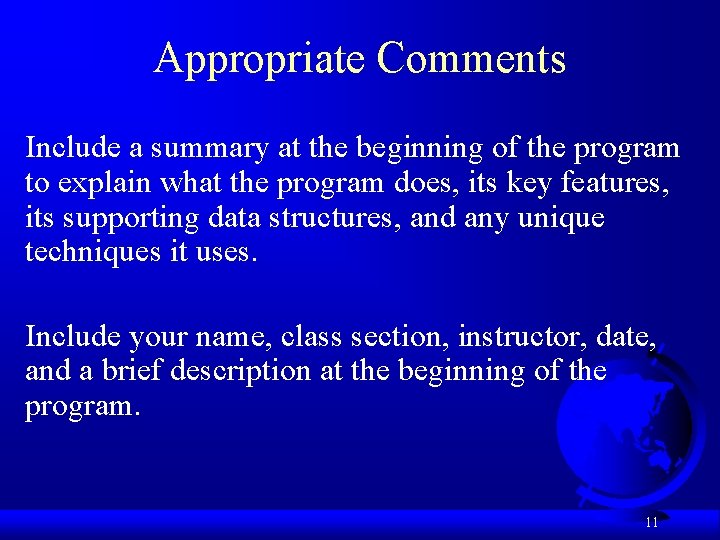
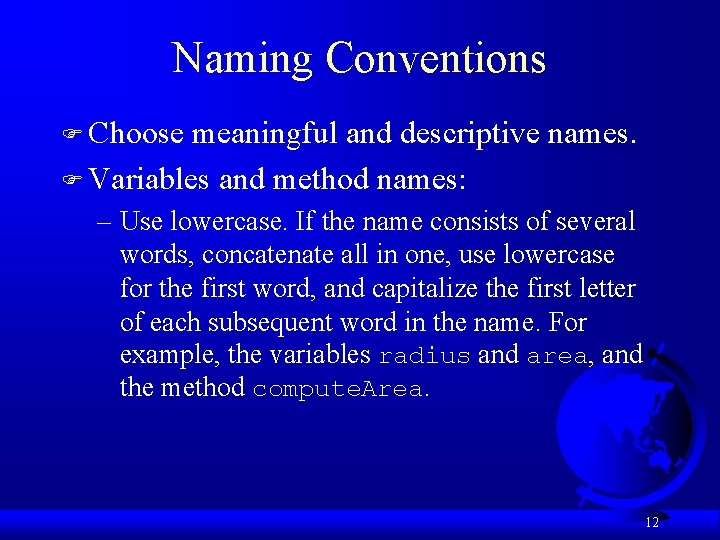
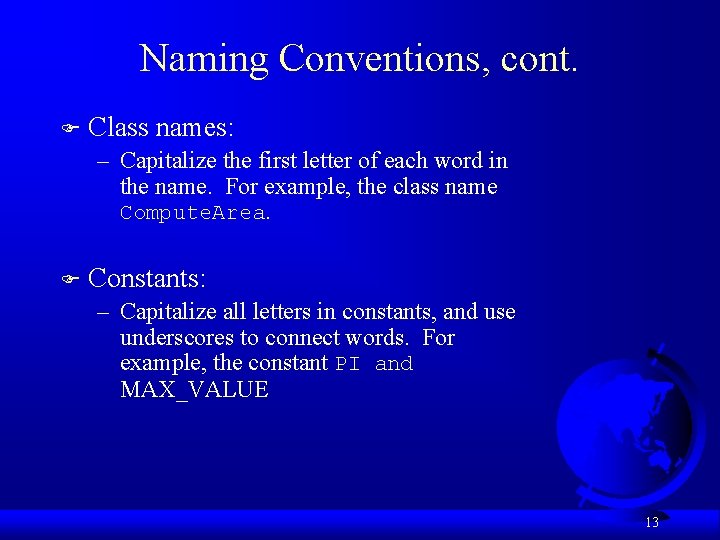
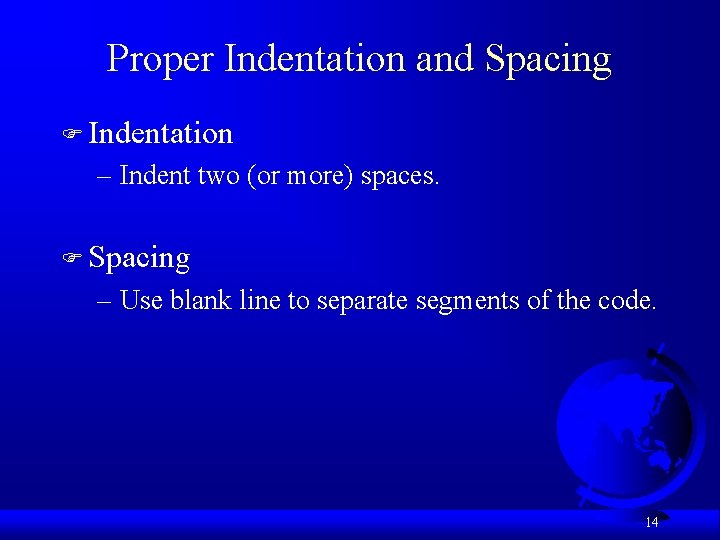
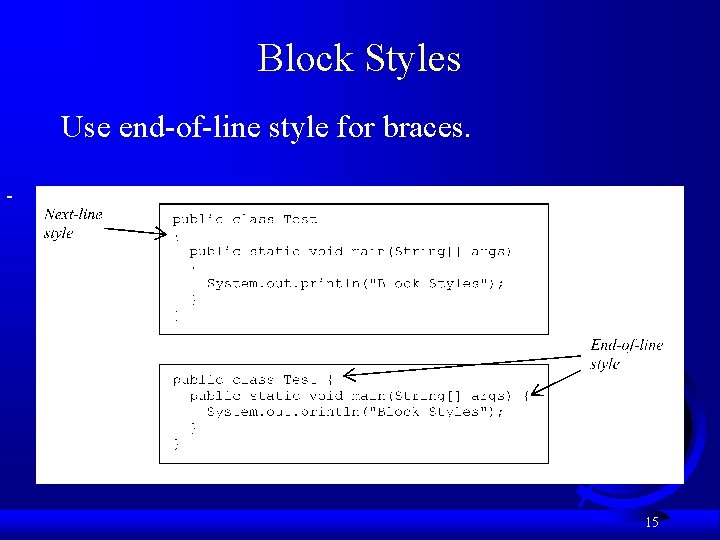
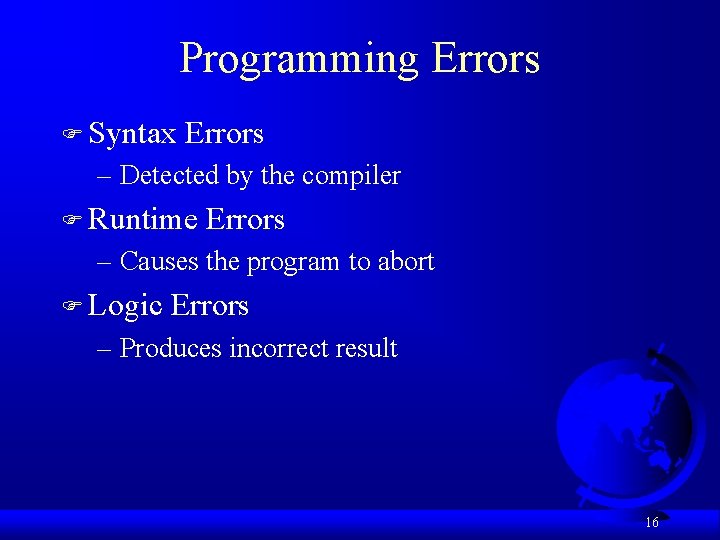
![Syntax Errors public class Show. Syntax. Errors { public static void main(String[] args) { Syntax Errors public class Show. Syntax. Errors { public static void main(String[] args) {](https://slidetodoc.com/presentation_image_h2/1be34a93ee74a66d5852f494cacc1b7c/image-17.jpg)
![Runtime Errors public class Show. Runtime. Errors { public static void main(String[] args) { Runtime Errors public class Show. Runtime. Errors { public static void main(String[] args) {](https://slidetodoc.com/presentation_image_h2/1be34a93ee74a66d5852f494cacc1b7c/image-18.jpg)
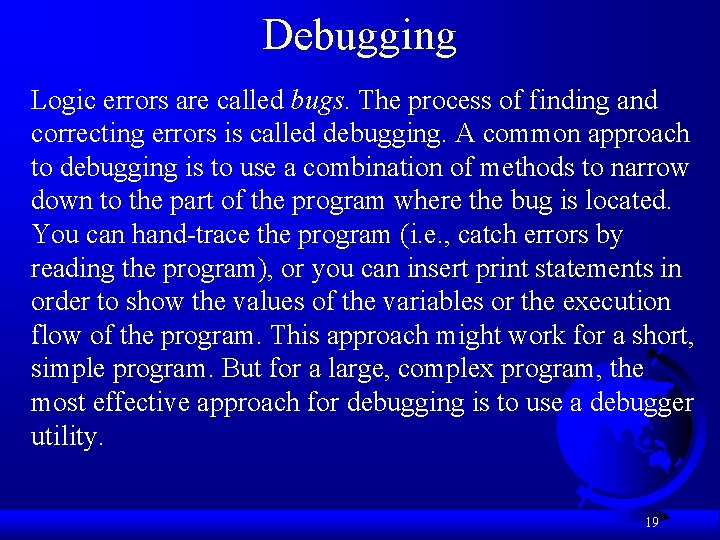
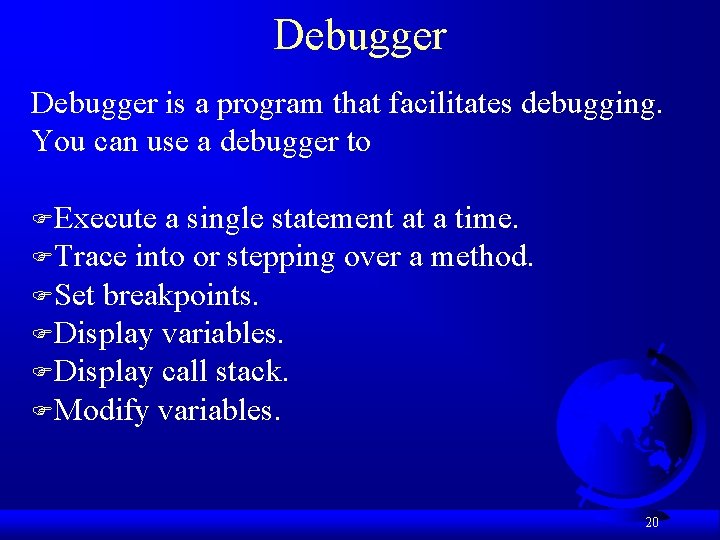
- Slides: 20
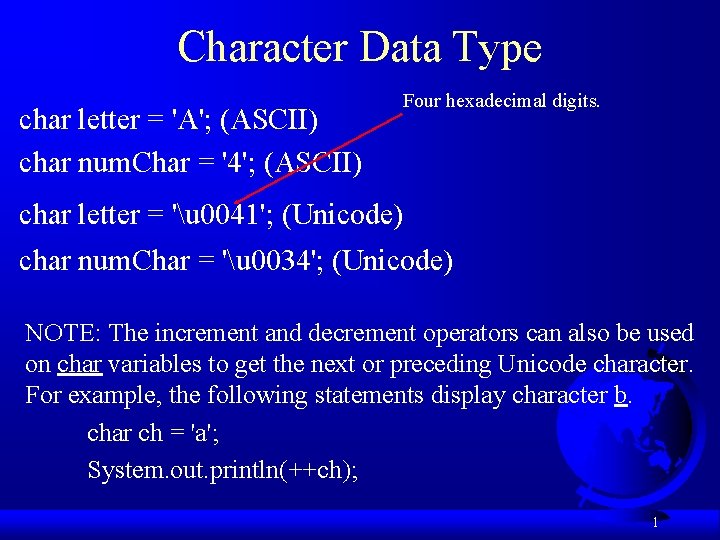
Character Data Type char letter = 'A'; (ASCII) char num. Char = '4'; (ASCII) Four hexadecimal digits. char letter = 'u 0041'; (Unicode) char num. Char = 'u 0034'; (Unicode) NOTE: The increment and decrement operators can also be used on char variables to get the next or preceding Unicode character. For example, the following statements display character b. char ch = 'a'; System. out. println(++ch); 1
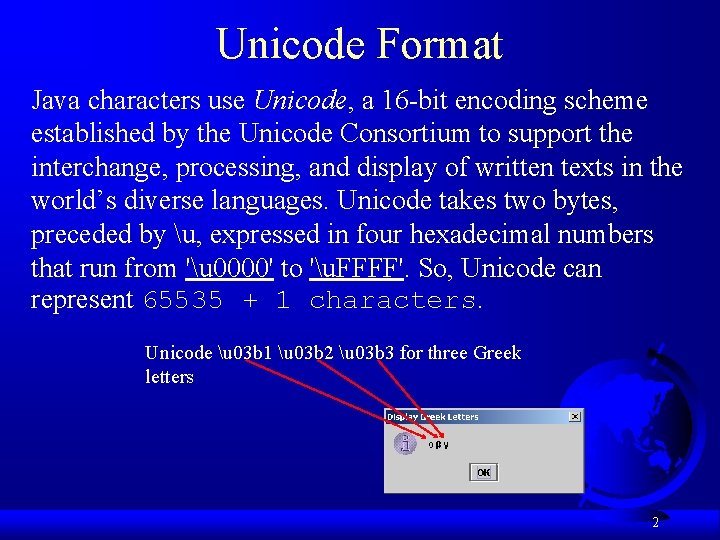
Unicode Format Java characters use Unicode, a 16 -bit encoding scheme established by the Unicode Consortium to support the interchange, processing, and display of written texts in the world’s diverse languages. Unicode takes two bytes, preceded by u, expressed in four hexadecimal numbers that run from 'u 0000' to 'u. FFFF'. So, Unicode can represent 65535 + 1 characters. Unicode u 03 b 1 u 03 b 2 u 03 b 3 for three Greek letters 2
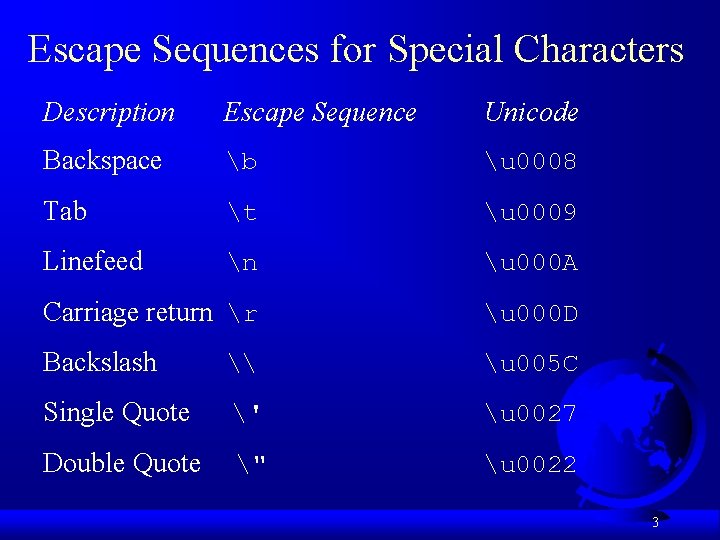
Escape Sequences for Special Characters Description Escape Sequence Unicode Backspace b u 0008 Tab t u 0009 Linefeed n u 000 A Carriage return r u 000 D Backslash \ u 005 C Single Quote ' u 0027 Double Quote " u 0022 3
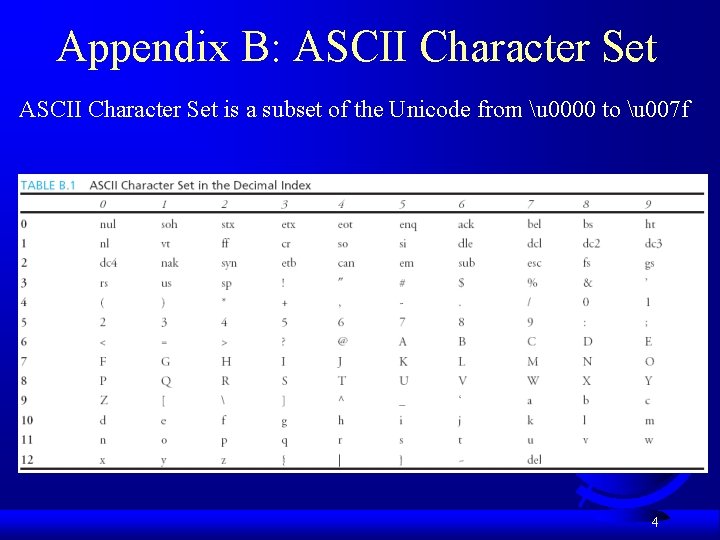
Appendix B: ASCII Character Set is a subset of the Unicode from u 0000 to u 007 f 4
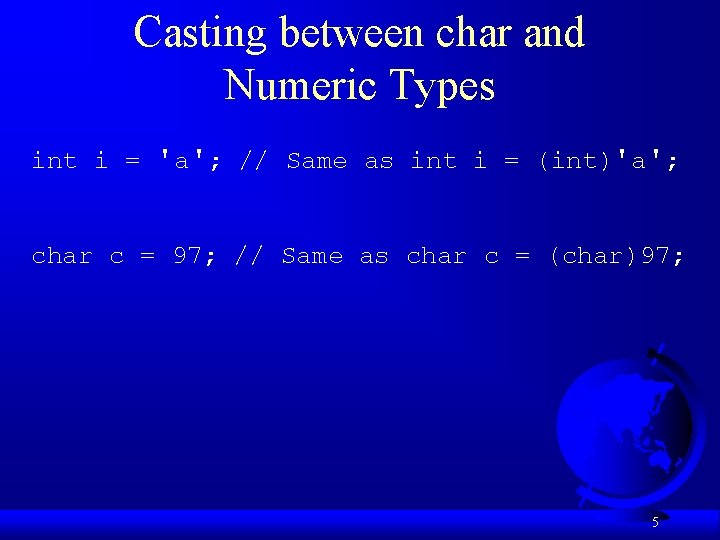
Casting between char and Numeric Types int i = 'a'; // Same as int i = (int)'a'; char c = 97; // Same as char c = (char)97; 5
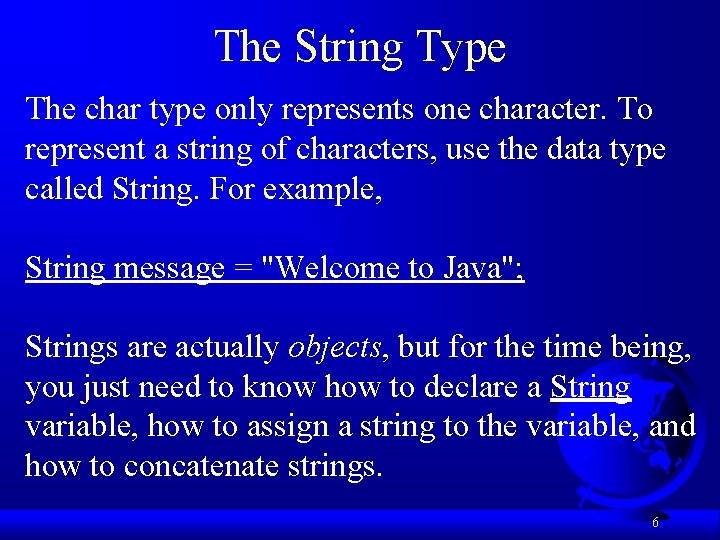
The String Type The char type only represents one character. To represent a string of characters, use the data type called String. For example, String message = "Welcome to Java"; Strings are actually objects, but for the time being, you just need to know how to declare a String variable, how to assign a string to the variable, and how to concatenate strings. 6
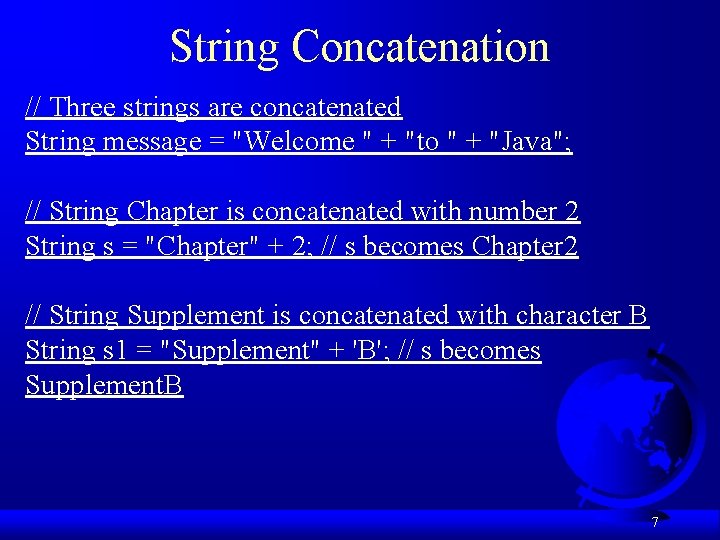
String Concatenation // Three strings are concatenated String message = "Welcome " + "to " + "Java"; // String Chapter is concatenated with number 2 String s = "Chapter" + 2; // s becomes Chapter 2 // String Supplement is concatenated with character B String s 1 = "Supplement" + 'B'; // s becomes Supplement. B 7
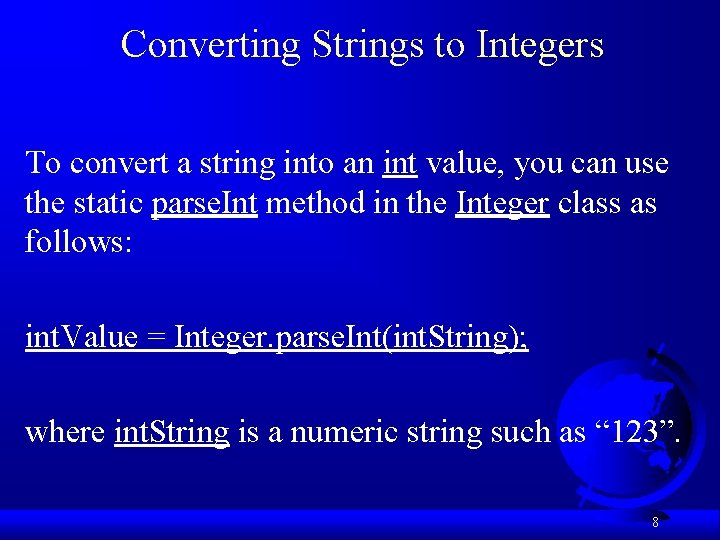
Converting Strings to Integers To convert a string into an int value, you can use the static parse. Int method in the Integer class as follows: int. Value = Integer. parse. Int(int. String); where int. String is a numeric string such as “ 123”. 8
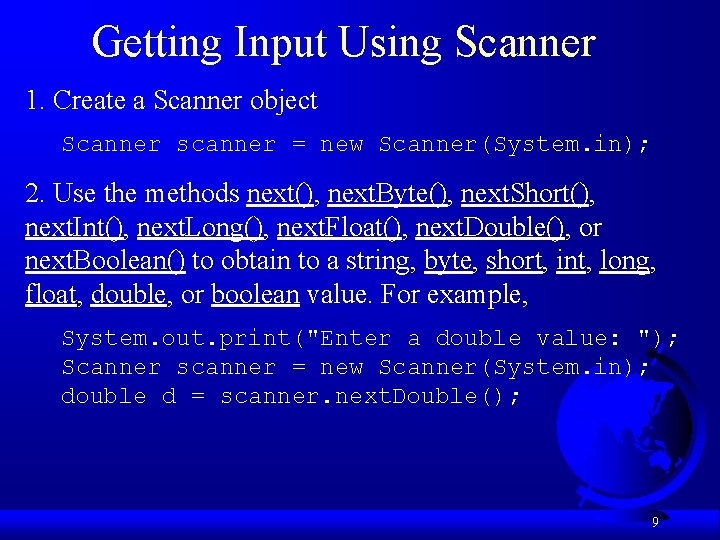
Getting Input Using Scanner 1. Create a Scanner object Scanner scanner = new Scanner(System. in); 2. Use the methods next(), next. Byte(), next. Short(), next. Int(), next. Long(), next. Float(), next. Double(), or next. Boolean() to obtain to a string, byte, short, int, long, float, double, or boolean value. For example, System. out. print("Enter a double value: "); Scanner scanner = new Scanner(System. in); double d = scanner. next. Double(); 9
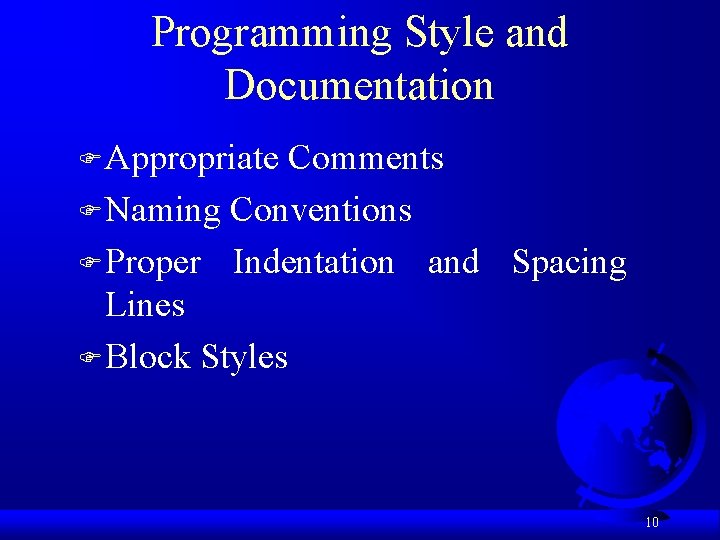
Programming Style and Documentation F Appropriate Comments F Naming Conventions F Proper Indentation and Spacing Lines F Block Styles 10
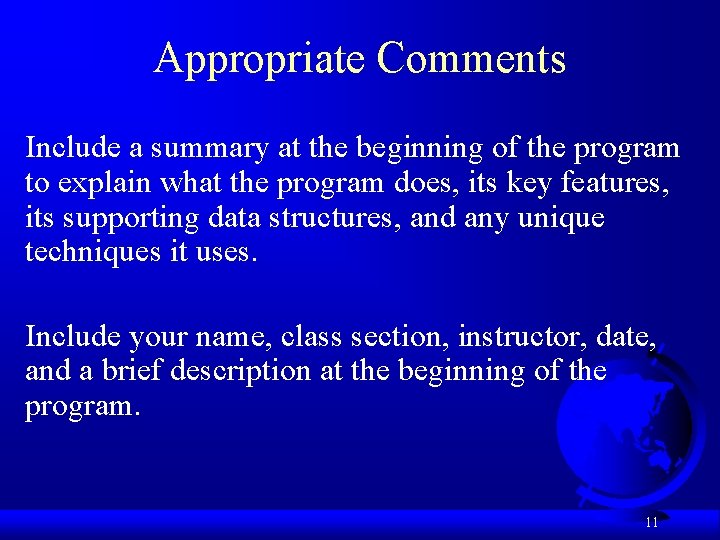
Appropriate Comments Include a summary at the beginning of the program to explain what the program does, its key features, its supporting data structures, and any unique techniques it uses. Include your name, class section, instructor, date, and a brief description at the beginning of the program. 11
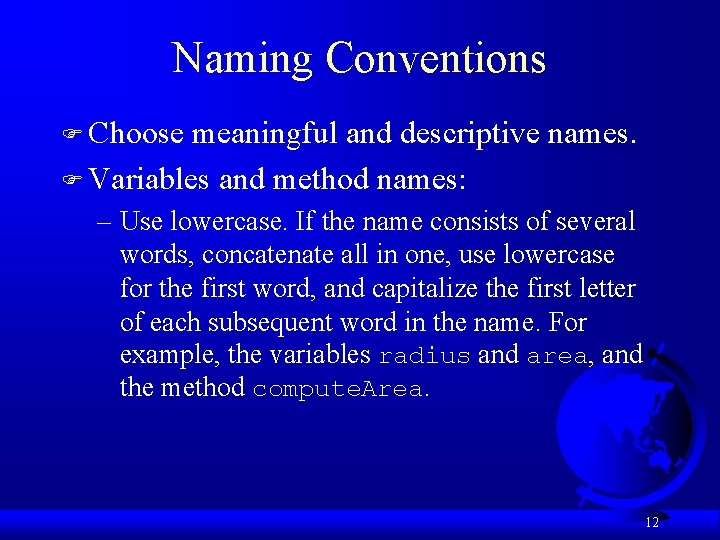
Naming Conventions F Choose meaningful and descriptive names. F Variables and method names: – Use lowercase. If the name consists of several words, concatenate all in one, use lowercase for the first word, and capitalize the first letter of each subsequent word in the name. For example, the variables radius and area, and the method compute. Area. 12
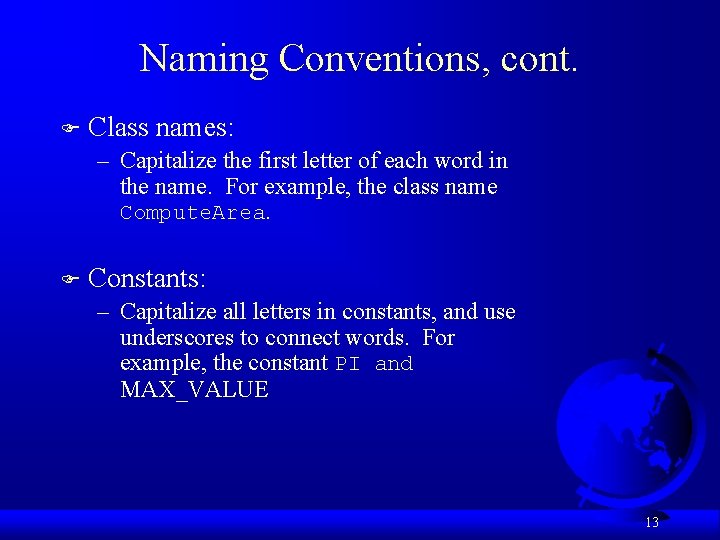
Naming Conventions, cont. F Class names: – Capitalize the first letter of each word in the name. For example, the class name Compute. Area. F Constants: – Capitalize all letters in constants, and use underscores to connect words. For example, the constant PI and MAX_VALUE 13
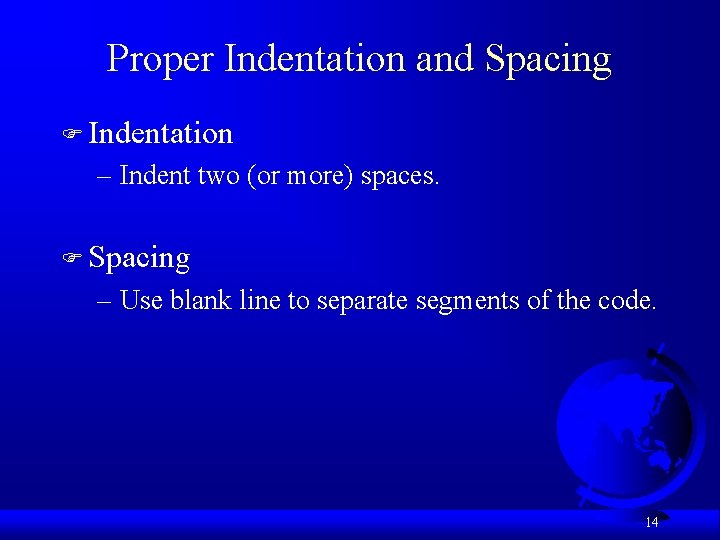
Proper Indentation and Spacing F Indentation – Indent two (or more) spaces. F Spacing – Use blank line to separate segments of the code. 14
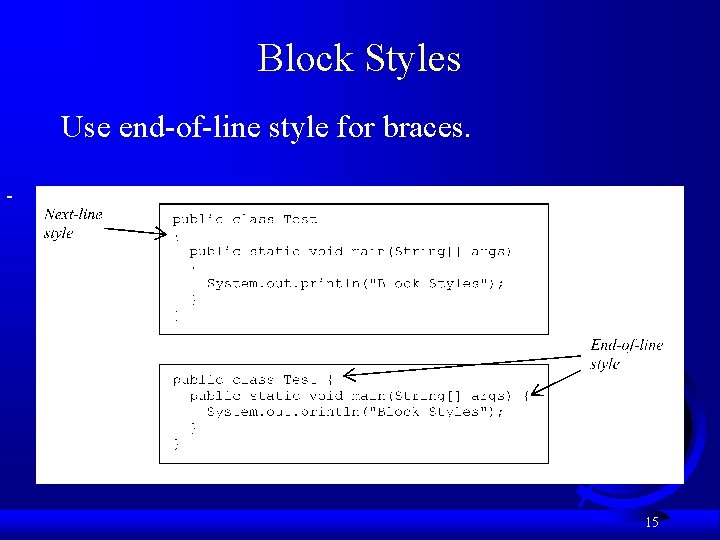
Block Styles Use end-of-line style for braces. 15
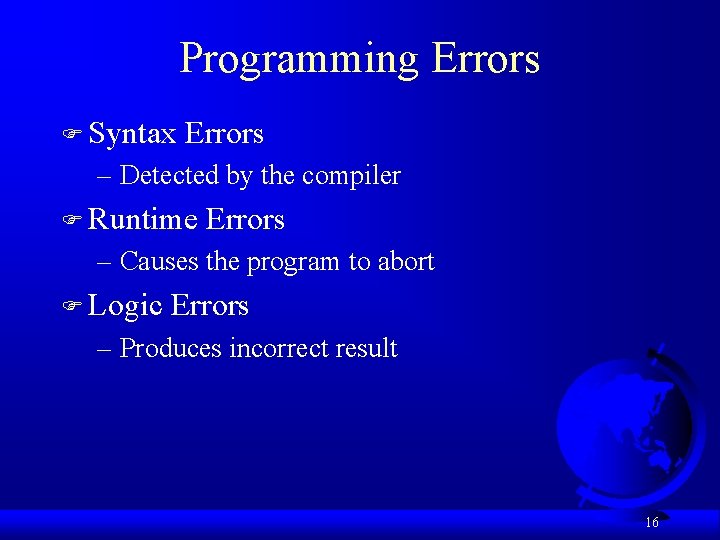
Programming Errors F Syntax Errors – Detected by the compiler F Runtime Errors – Causes the program to abort F Logic Errors – Produces incorrect result 16
![Syntax Errors public class Show Syntax Errors public static void mainString args Syntax Errors public class Show. Syntax. Errors { public static void main(String[] args) {](https://slidetodoc.com/presentation_image_h2/1be34a93ee74a66d5852f494cacc1b7c/image-17.jpg)
Syntax Errors public class Show. Syntax. Errors { public static void main(String[] args) { i = 30; System. out. println(i + 4); } } 17
![Runtime Errors public class Show Runtime Errors public static void mainString args Runtime Errors public class Show. Runtime. Errors { public static void main(String[] args) {](https://slidetodoc.com/presentation_image_h2/1be34a93ee74a66d5852f494cacc1b7c/image-18.jpg)
Runtime Errors public class Show. Runtime. Errors { public static void main(String[] args) { int i = 1 / 0; } } 18
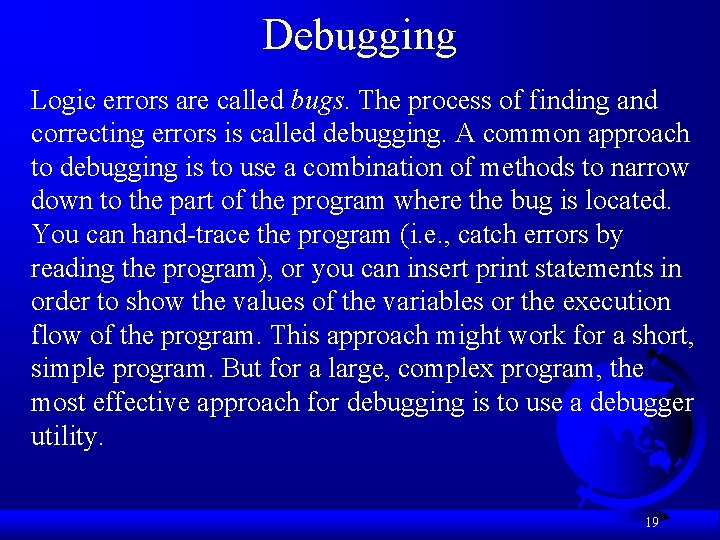
Debugging Logic errors are called bugs. The process of finding and correcting errors is called debugging. A common approach to debugging is to use a combination of methods to narrow down to the part of the program where the bug is located. You can hand-trace the program (i. e. , catch errors by reading the program), or you can insert print statements in order to show the values of the variables or the execution flow of the program. This approach might work for a short, simple program. But for a large, complex program, the most effective approach for debugging is to use a debugger utility. 19
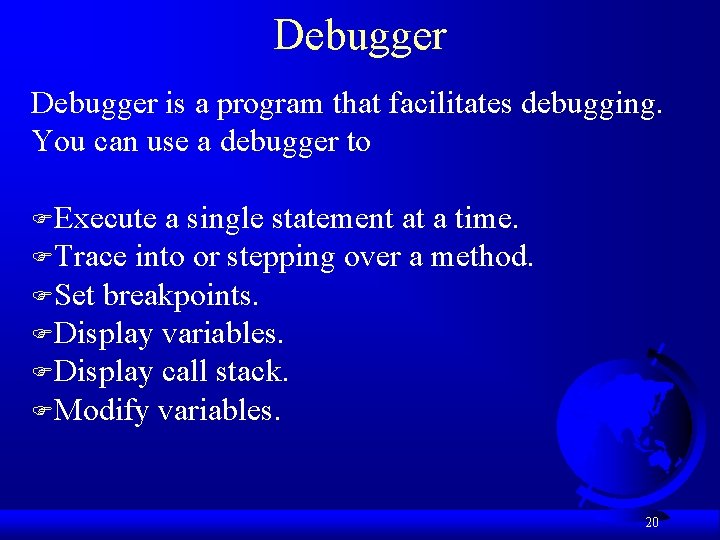
Debugger is a program that facilitates debugging. You can use a debugger to FExecute a single statement at a time. FTrace into or stepping over a method. FSet breakpoints. FDisplay variables. FDisplay call stack. FModify variables. 20
Ascii to string matlab
Ascii
Const char *s =
Auto break case char
Type data char
Grass letter a
Int (*)(bool, char)
150 opening ascii mode data connection.
2 jenis data
What is static
Difference between flat and round characters
Famous round characters
Person vs fate movie examples
Major vs minor characters
Advanced character physics
Scarlet letter character descriptions
What type of character is della in the gift of the magi
Character type check
Windows ascii characters
Ascii spasi
Operator logika && adalah… *