Chapter 7 Arrays Part 1 of 2 Arrays
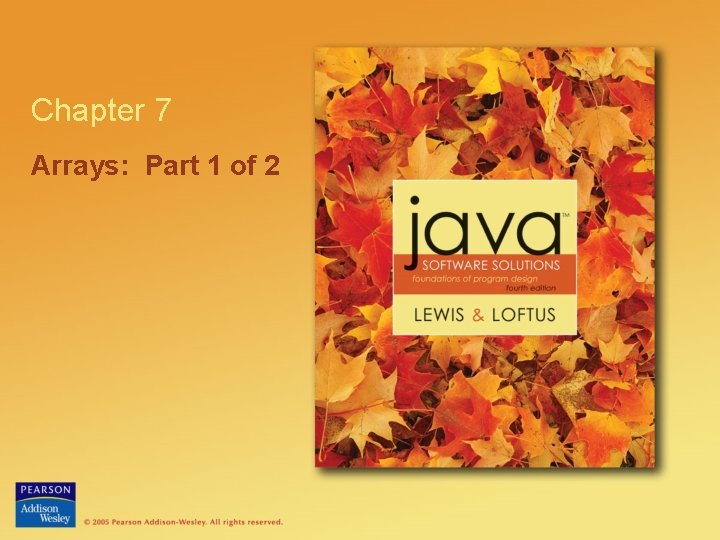
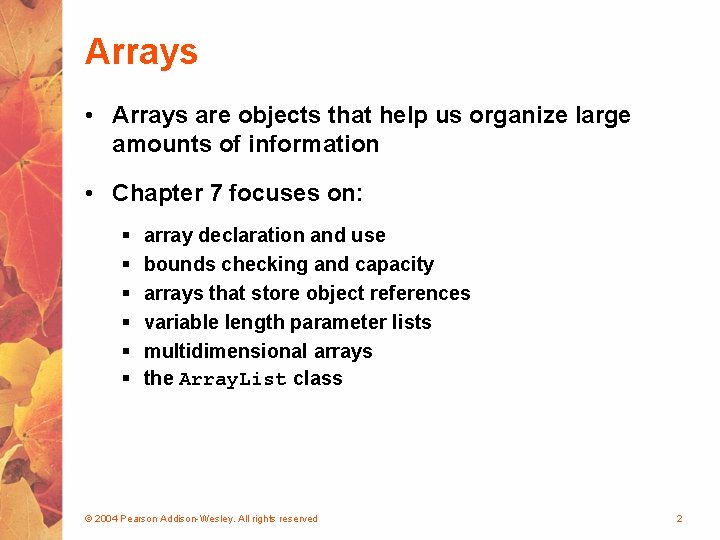
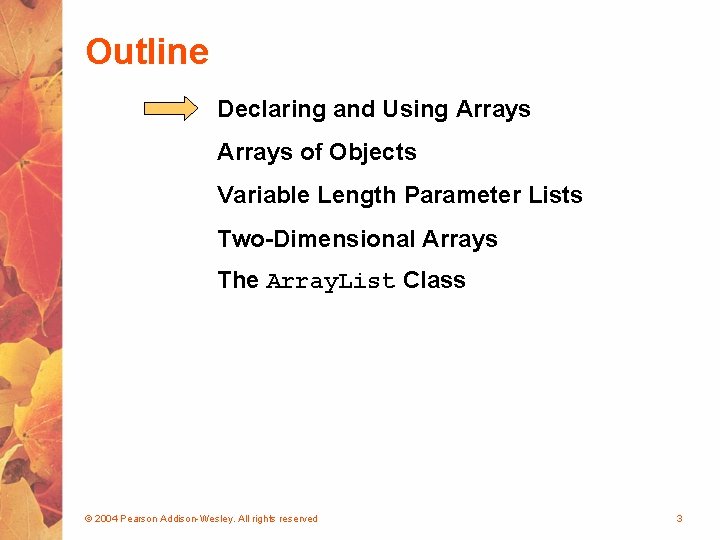
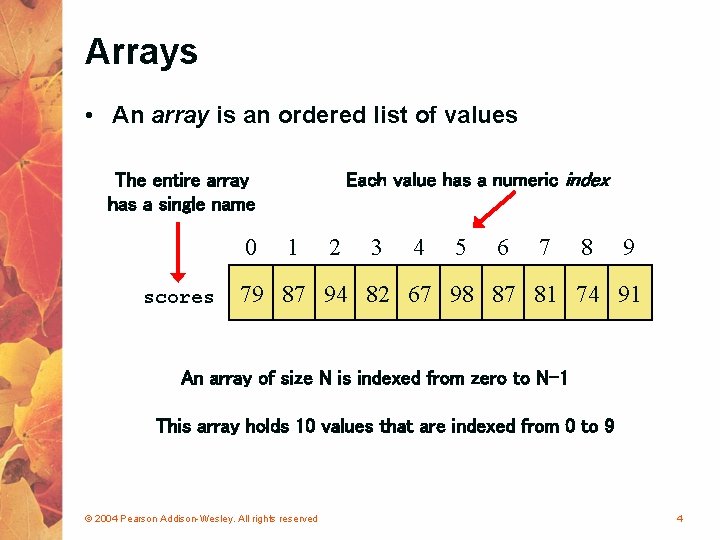
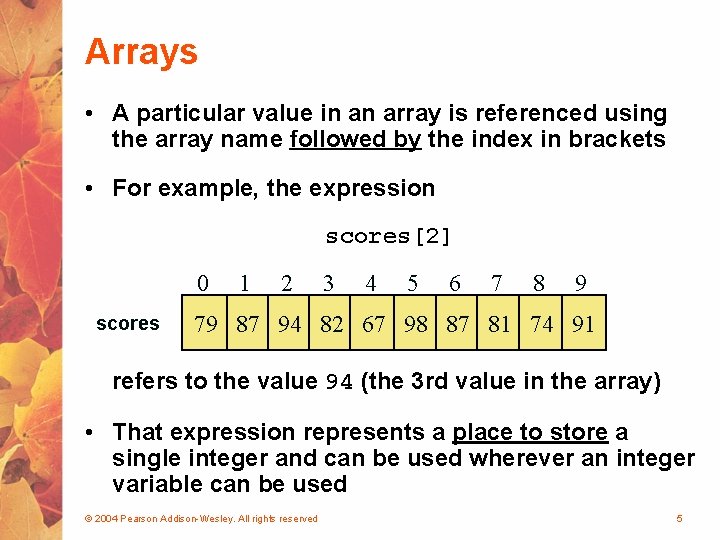
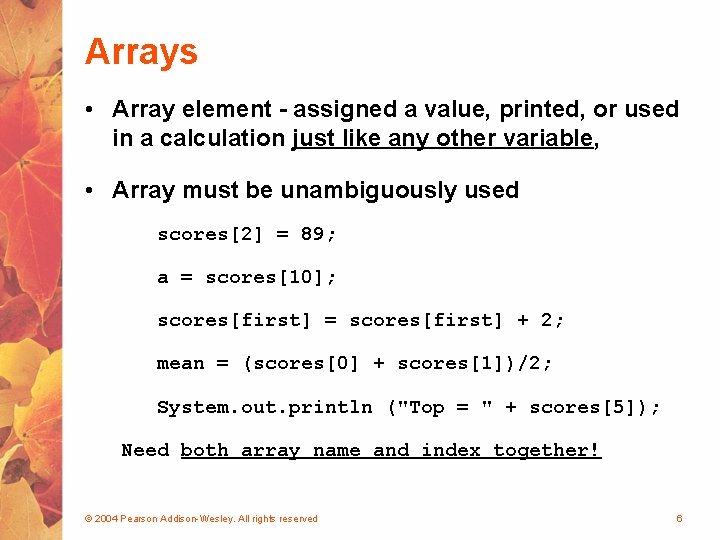
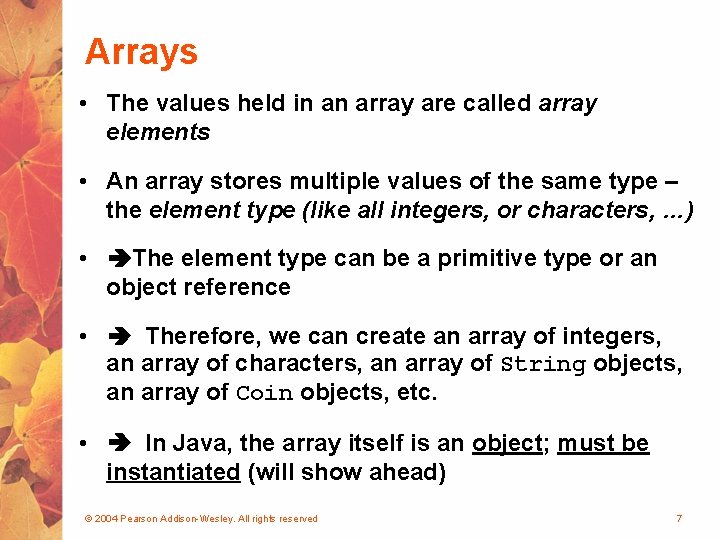
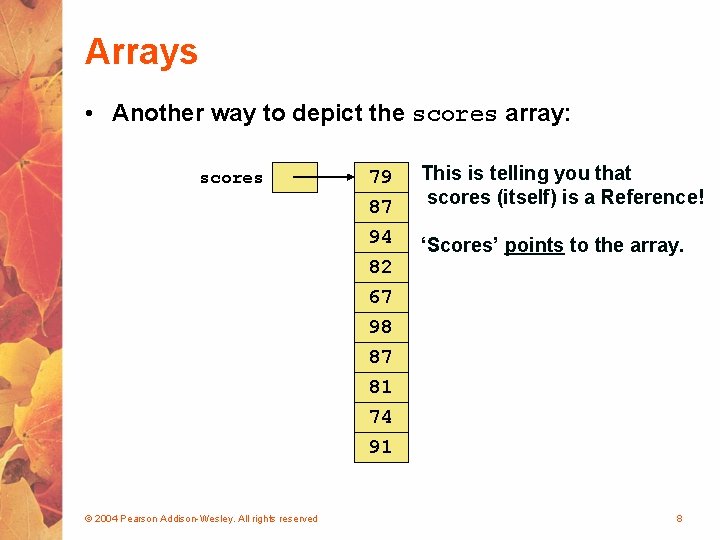
![Declaring Arrays • The scores array could be declared as follows: int[] scores = Declaring Arrays • The scores array could be declared as follows: int[] scores =](https://slidetodoc.com/presentation_image/d64da4ba7572962cf39c9ec70f9c73f5/image-9.jpg)
![Declaring Arrays • Some other examples of array declarations: float[] prices = new float[500]; Declaring Arrays • Some other examples of array declarations: float[] prices = new float[500];](https://slidetodoc.com/presentation_image/d64da4ba7572962cf39c9ec70f9c73f5/image-10.jpg)
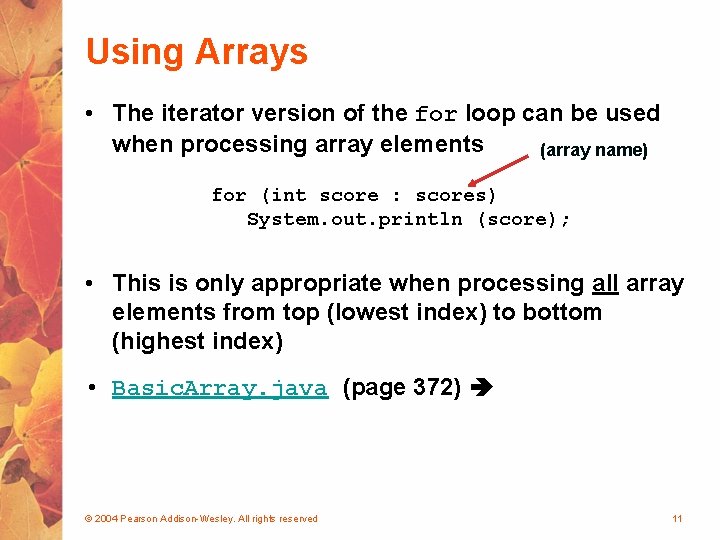
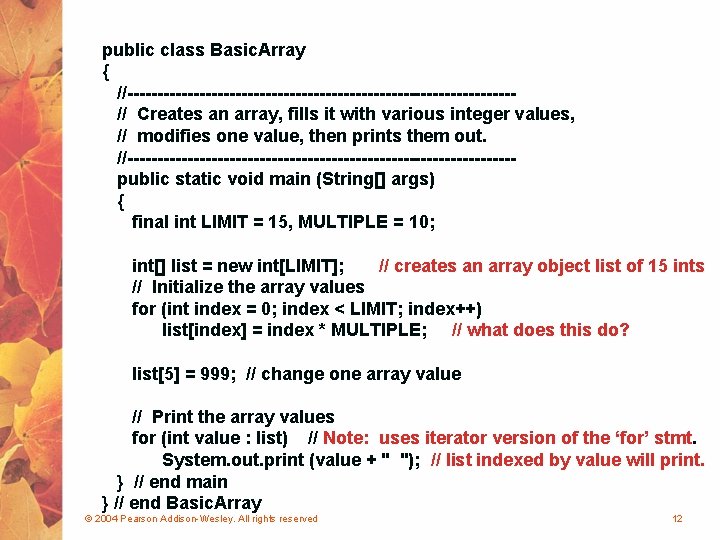
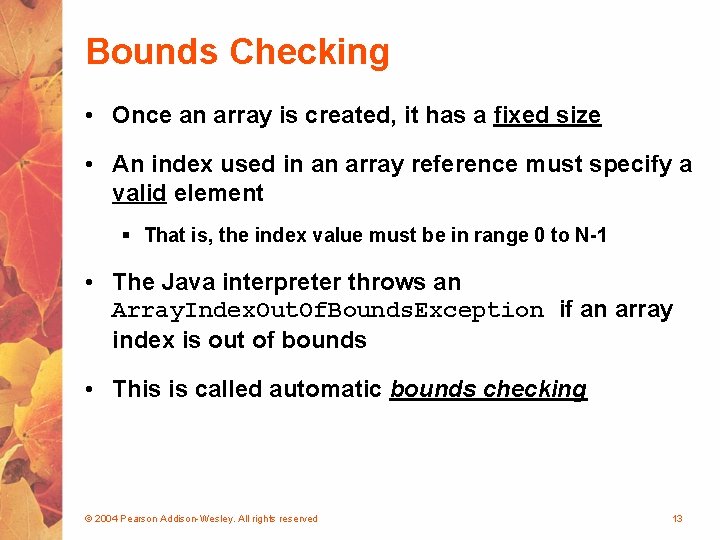
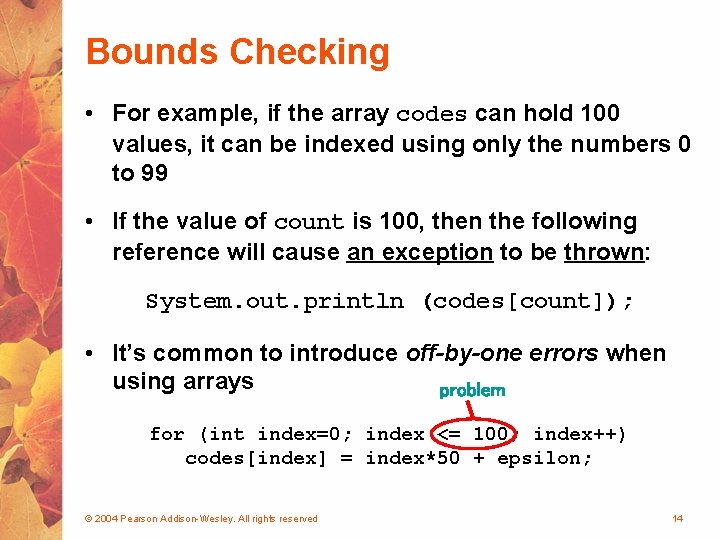
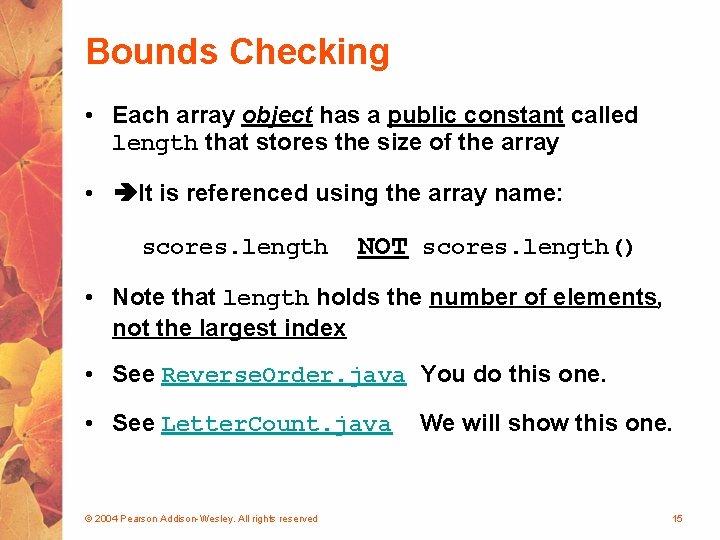
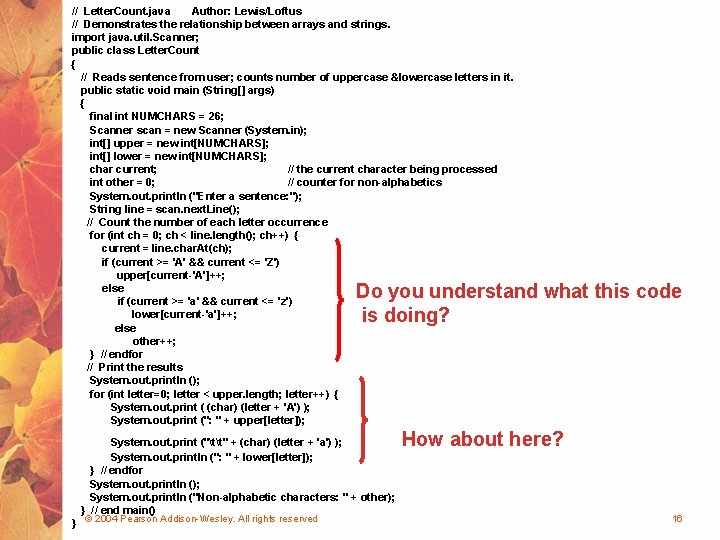
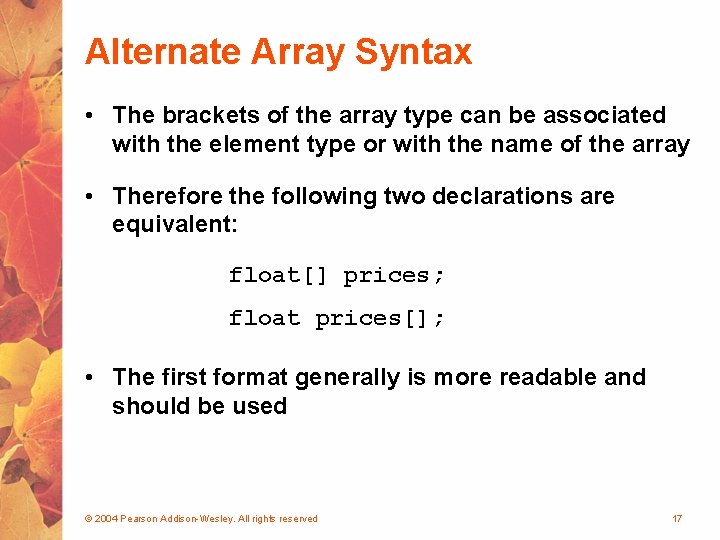
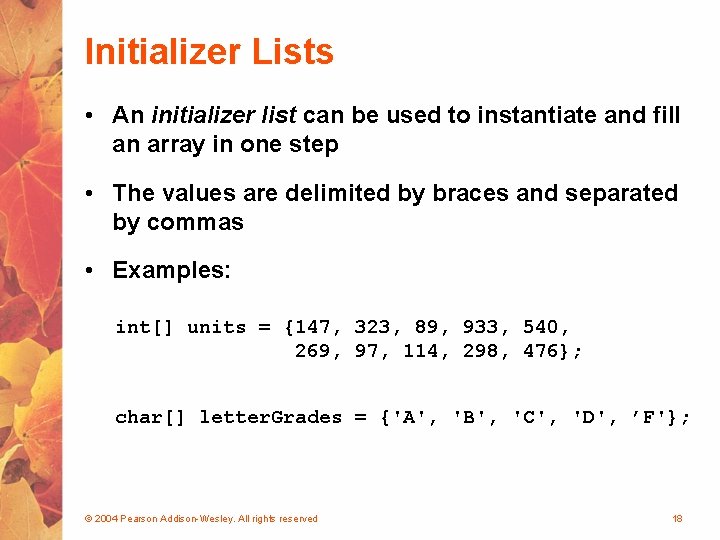
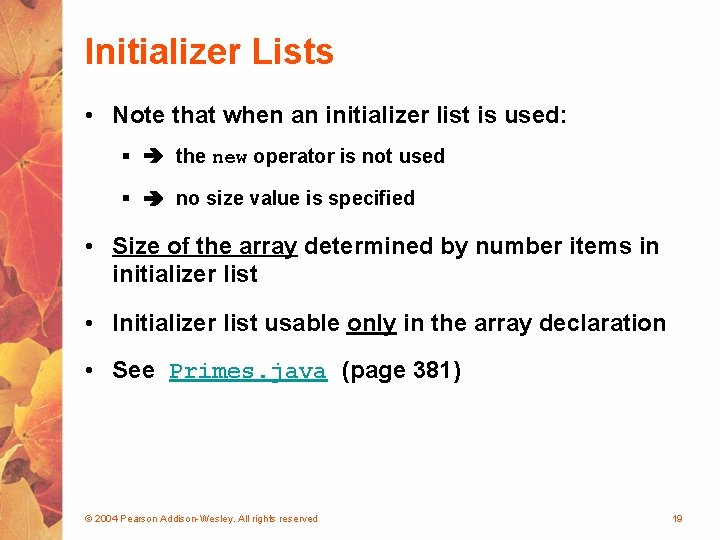
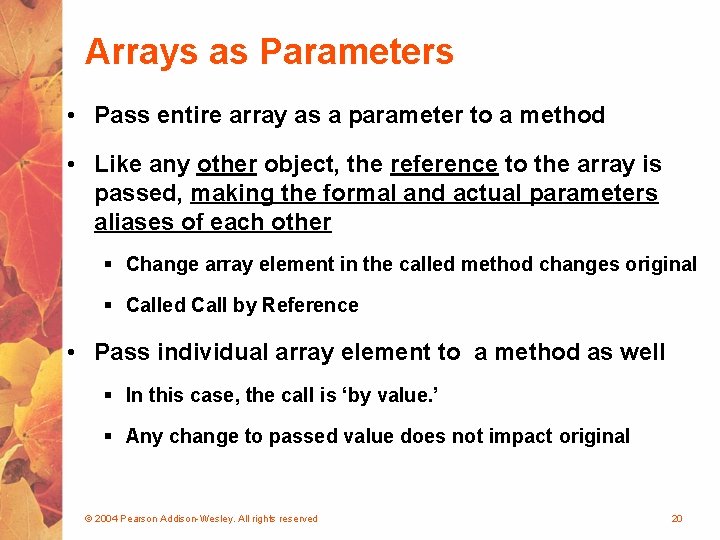
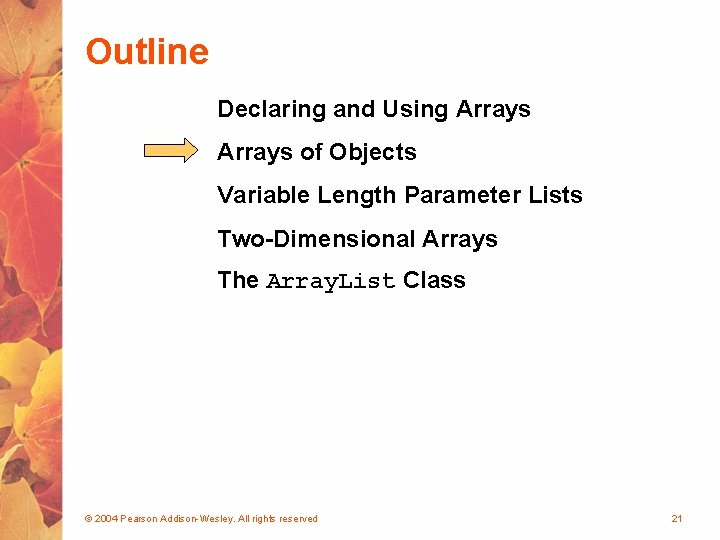
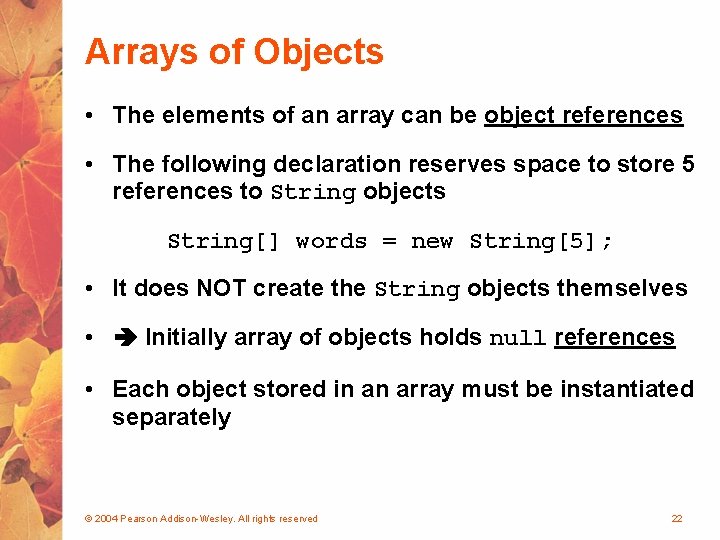
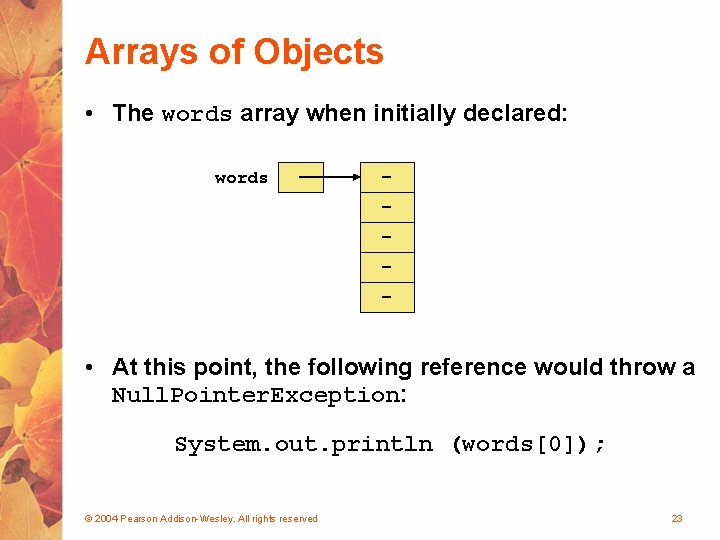
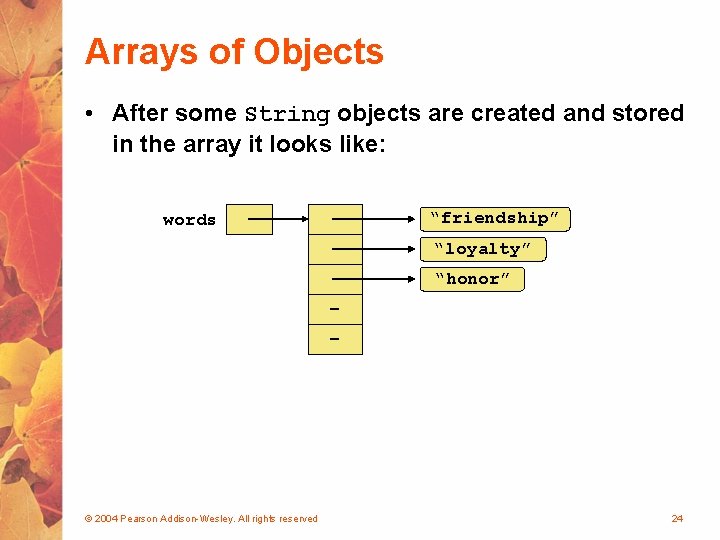
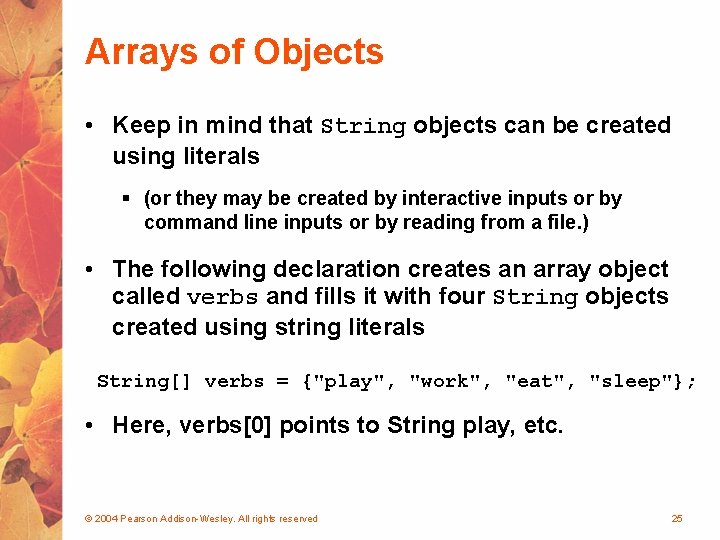
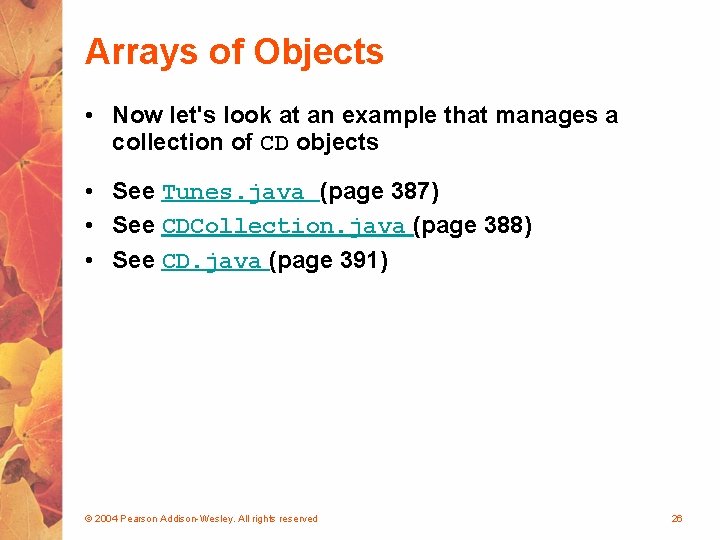
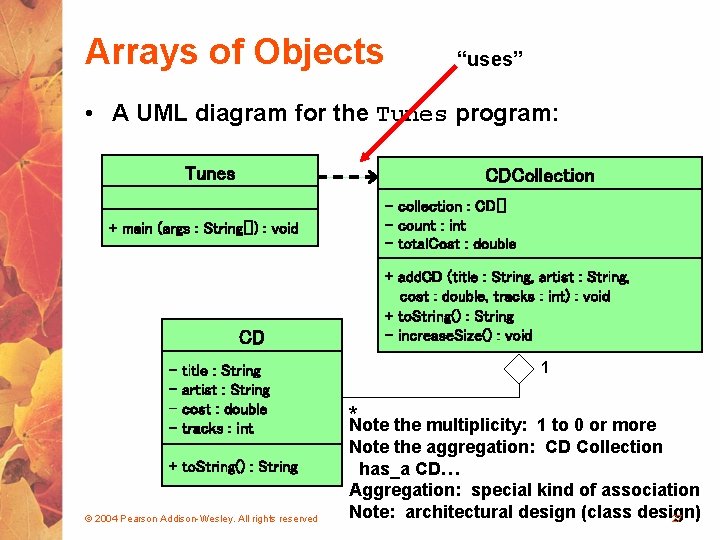
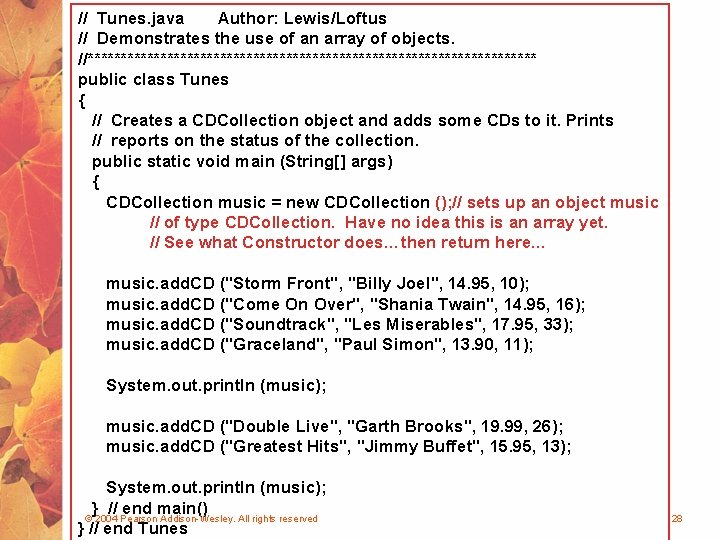
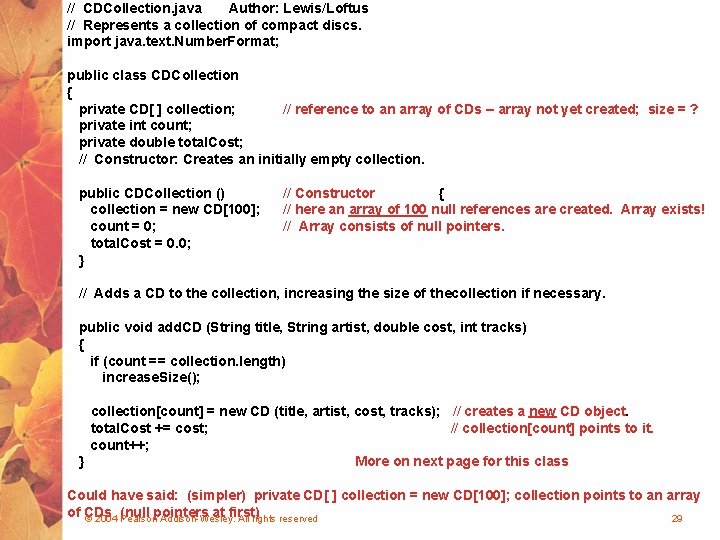
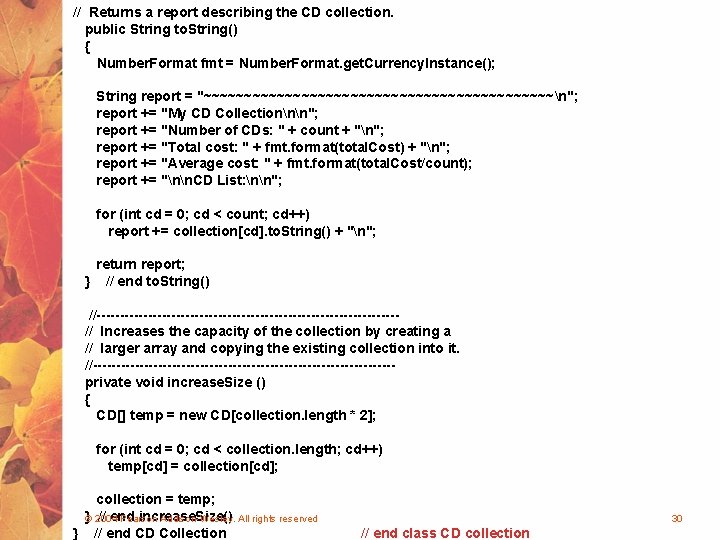
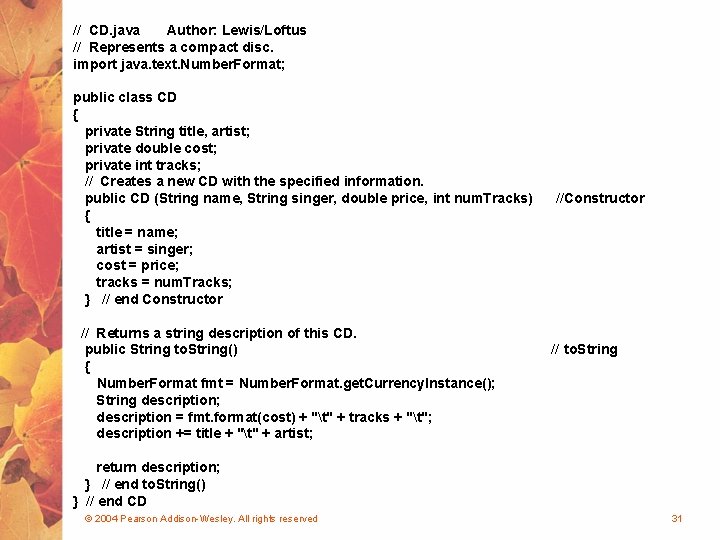
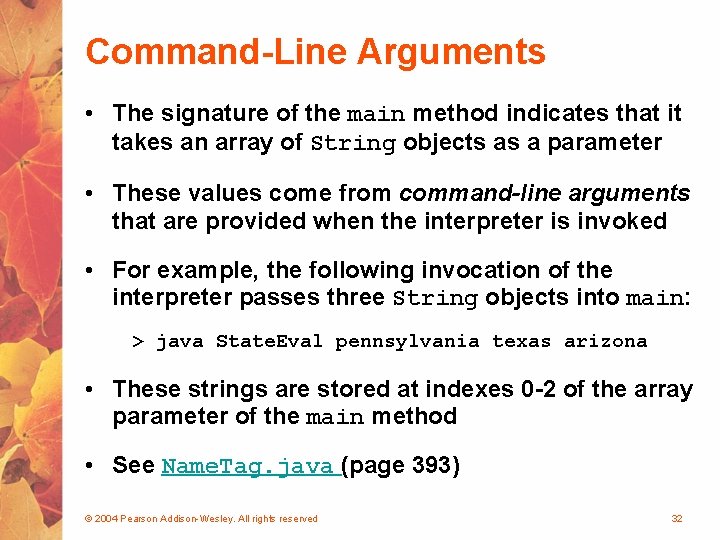
- Slides: 32
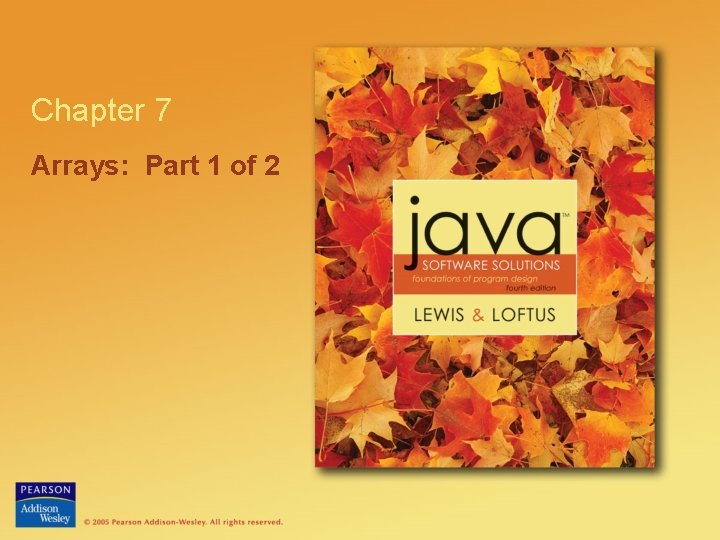
Chapter 7 Arrays: Part 1 of 2
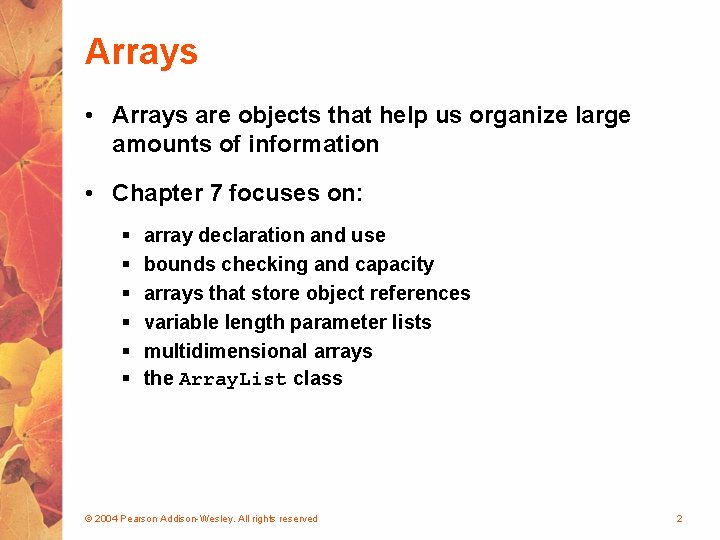
Arrays • Arrays are objects that help us organize large amounts of information • Chapter 7 focuses on: § § § array declaration and use bounds checking and capacity arrays that store object references variable length parameter lists multidimensional arrays the Array. List class © 2004 Pearson Addison-Wesley. All rights reserved 2
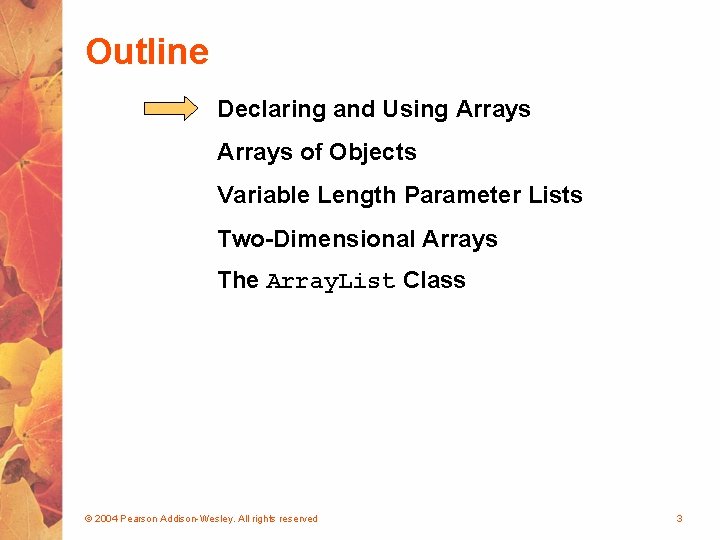
Outline Declaring and Using Arrays of Objects Variable Length Parameter Lists Two-Dimensional Arrays The Array. List Class © 2004 Pearson Addison-Wesley. All rights reserved 3
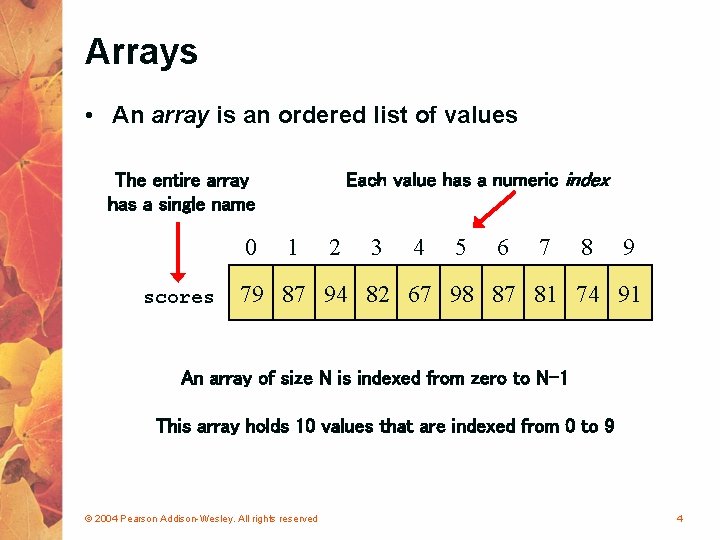
Arrays • An array is an ordered list of values Each value has a numeric index The entire array has a single name 0 scores 1 2 3 4 5 6 7 8 9 79 87 94 82 67 98 87 81 74 91 An array of size N is indexed from zero to N-1 This array holds 10 values that are indexed from 0 to 9 © 2004 Pearson Addison-Wesley. All rights reserved 4
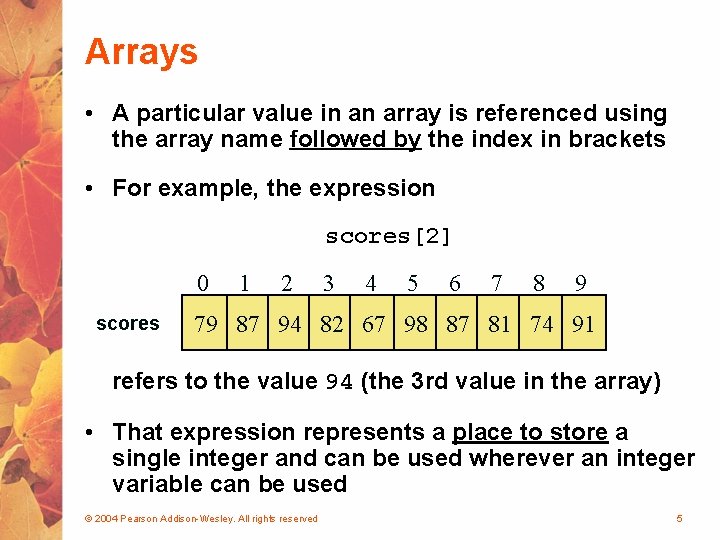
Arrays • A particular value in an array is referenced using the array name followed by the index in brackets • For example, the expression scores[2] 0 scores 1 2 3 4 5 6 7 8 9 79 87 94 82 67 98 87 81 74 91 refers to the value 94 (the 3 rd value in the array) • That expression represents a place to store a single integer and can be used wherever an integer variable can be used © 2004 Pearson Addison-Wesley. All rights reserved 5
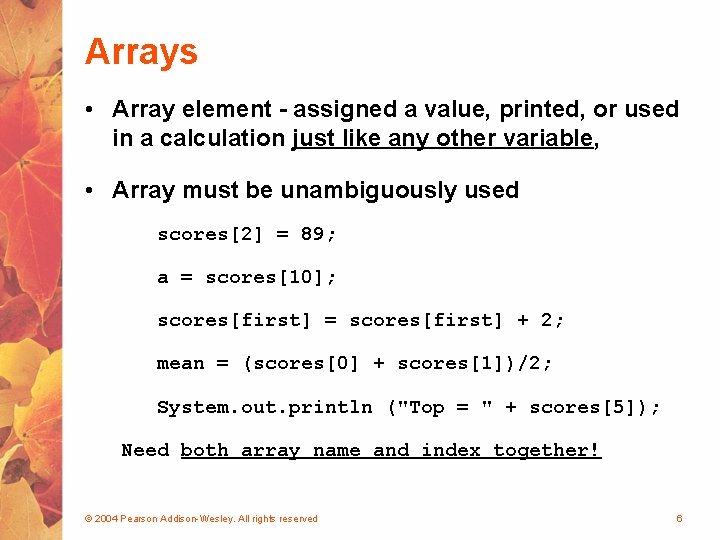
Arrays • Array element - assigned a value, printed, or used in a calculation just like any other variable, • Array must be unambiguously used scores[2] = 89; a = scores[10]; scores[first] = scores[first] + 2; mean = (scores[0] + scores[1])/2; System. out. println ("Top = " + scores[5]); Need both array name and index together! © 2004 Pearson Addison-Wesley. All rights reserved 6
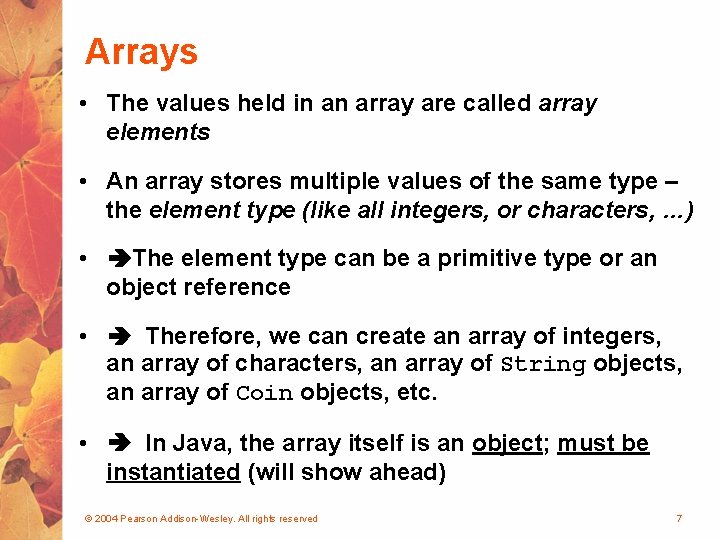
Arrays • The values held in an array are called array elements • An array stores multiple values of the same type – the element type (like all integers, or characters, …) • The element type can be a primitive type or an object reference • Therefore, we can create an array of integers, an array of characters, an array of String objects, an array of Coin objects, etc. • In Java, the array itself is an object; must be instantiated (will show ahead) © 2004 Pearson Addison-Wesley. All rights reserved 7
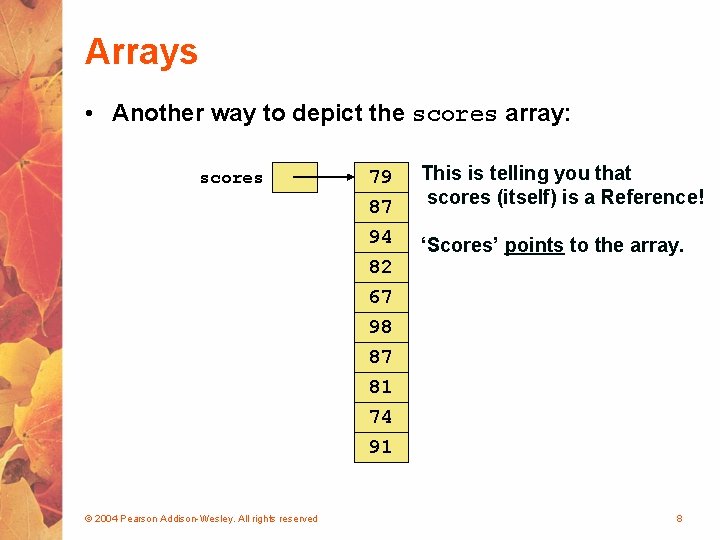
Arrays • Another way to depict the scores array: scores 79 87 94 82 67 This is telling you that scores (itself) is a Reference! ‘Scores’ points to the array. 98 87 81 74 91 © 2004 Pearson Addison-Wesley. All rights reserved 8
![Declaring Arrays The scores array could be declared as follows int scores Declaring Arrays • The scores array could be declared as follows: int[] scores =](https://slidetodoc.com/presentation_image/d64da4ba7572962cf39c9ec70f9c73f5/image-9.jpg)
Declaring Arrays • The scores array could be declared as follows: int[] scores = new int[10]; • Note the syntax, the ‘reference’ and the new object! It also says that there will be 10 scores referenced. • The type of the variable scores is int[] (“an array of integers” or “an array of ints. ”) • Note that the array type does not specify its size, but each object of that type has a specific size • The reference variable scores is set to a new array object that can hold 10 integers © 2004 Pearson Addison-Wesley. All rights reserved 9
![Declaring Arrays Some other examples of array declarations float prices new float500 Declaring Arrays • Some other examples of array declarations: float[] prices = new float[500];](https://slidetodoc.com/presentation_image/d64da4ba7572962cf39c9ec70f9c73f5/image-10.jpg)
Declaring Arrays • Some other examples of array declarations: float[] prices = new float[500]; boolean[] flags; flags = new boolean[20]; char[] codes = new char[1750]; Can you provide examples of each type? Can you draw such an array as was provided earlier in these slides? You saw the entries for the integer array, scores. What would a similar array of flags look like. Draw it. Of characters? Draw it. (You will see this again!) © 2004 Pearson Addison-Wesley. All rights reserved 10
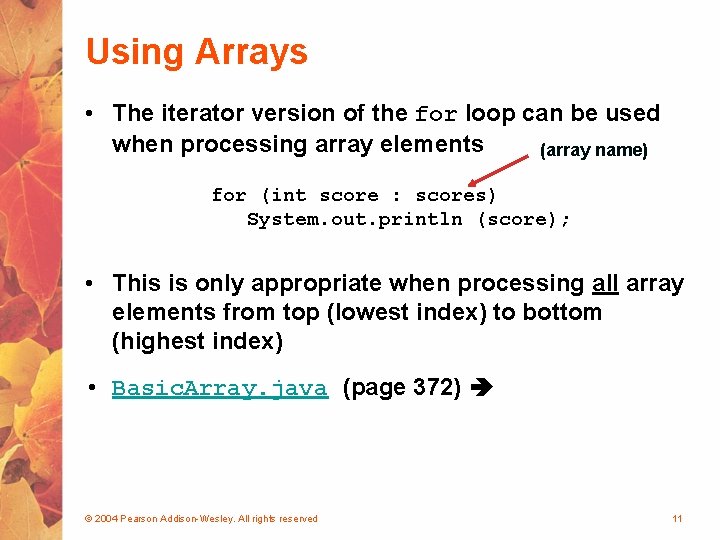
Using Arrays • The iterator version of the for loop can be used when processing array elements (array name) for (int score : scores) System. out. println (score); • This is only appropriate when processing all array elements from top (lowest index) to bottom (highest index) • Basic. Array. java (page 372) © 2004 Pearson Addison-Wesley. All rights reserved 11
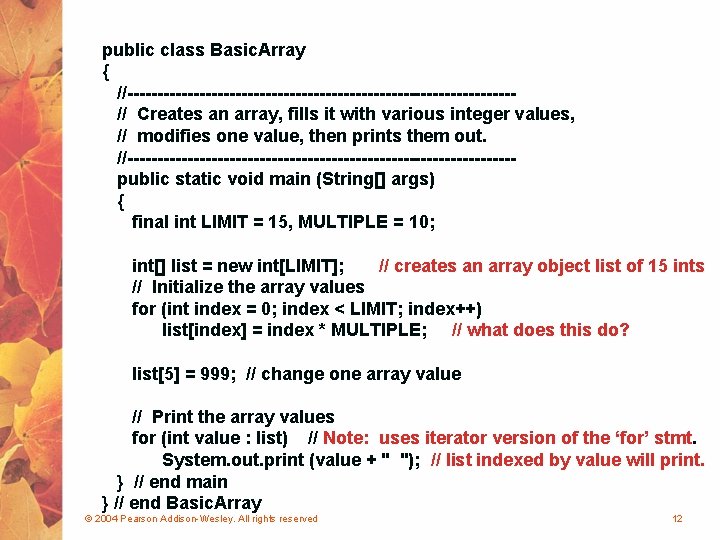
public class Basic. Array { //--------------------------------// Creates an array, fills it with various integer values, // modifies one value, then prints them out. //--------------------------------public static void main (String[] args) { final int LIMIT = 15, MULTIPLE = 10; int[] list = new int[LIMIT]; // creates an array object list of 15 ints // Initialize the array values for (int index = 0; index < LIMIT; index++) list[index] = index * MULTIPLE; // what does this do? list[5] = 999; // change one array value // Print the array values for (int value : list) // Note: uses iterator version of the ‘for’ stmt. System. out. print (value + " "); // list indexed by value will print. } // end main } // end Basic. Array © 2004 Pearson Addison-Wesley. All rights reserved 12
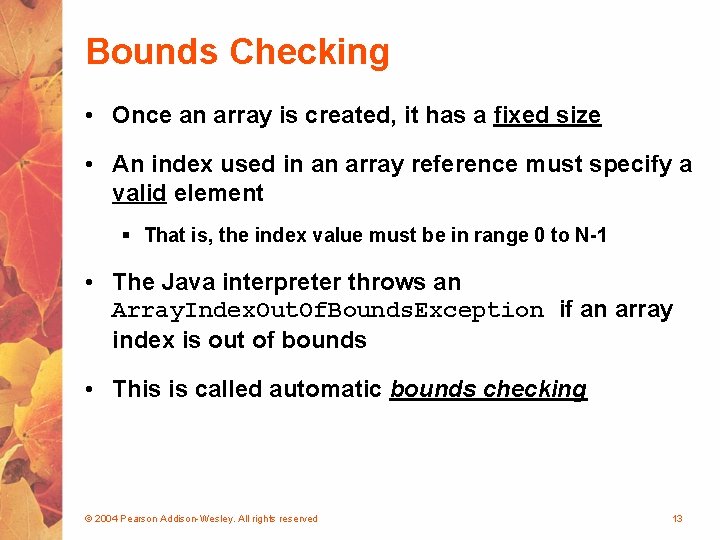
Bounds Checking • Once an array is created, it has a fixed size • An index used in an array reference must specify a valid element § That is, the index value must be in range 0 to N-1 • The Java interpreter throws an Array. Index. Out. Of. Bounds. Exception if an array index is out of bounds • This is called automatic bounds checking © 2004 Pearson Addison-Wesley. All rights reserved 13
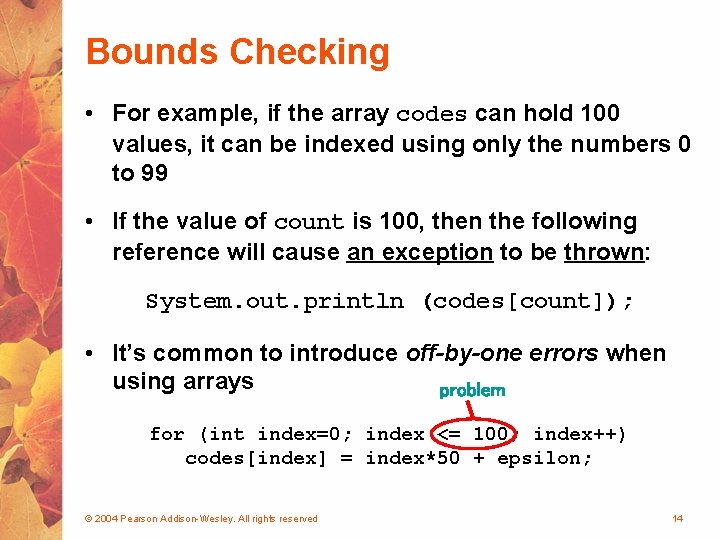
Bounds Checking • For example, if the array codes can hold 100 values, it can be indexed using only the numbers 0 to 99 • If the value of count is 100, then the following reference will cause an exception to be thrown: System. out. println (codes[count]); • It’s common to introduce off-by-one errors when using arrays problem for (int index=0; index <= 100; index++) codes[index] = index*50 + epsilon; © 2004 Pearson Addison-Wesley. All rights reserved 14
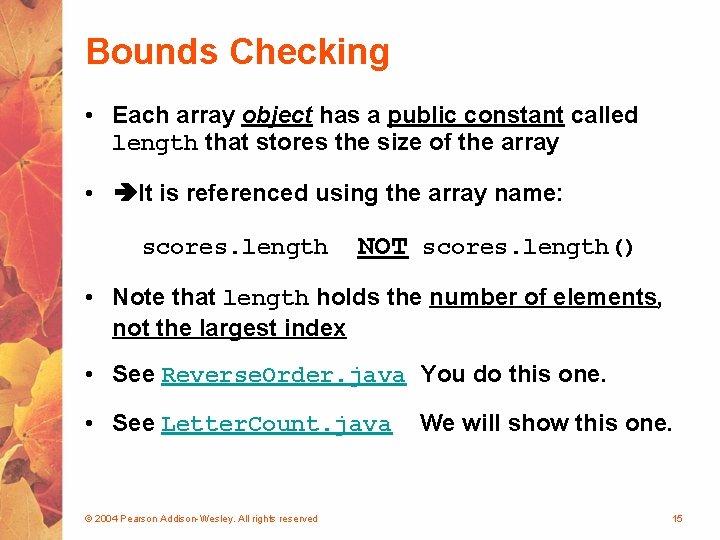
Bounds Checking • Each array object has a public constant called length that stores the size of the array • It is referenced using the array name: scores. length NOT scores. length() • Note that length holds the number of elements, not the largest index • See Reverse. Order. java You do this one. • See Letter. Count. java © 2004 Pearson Addison-Wesley. All rights reserved We will show this one. 15
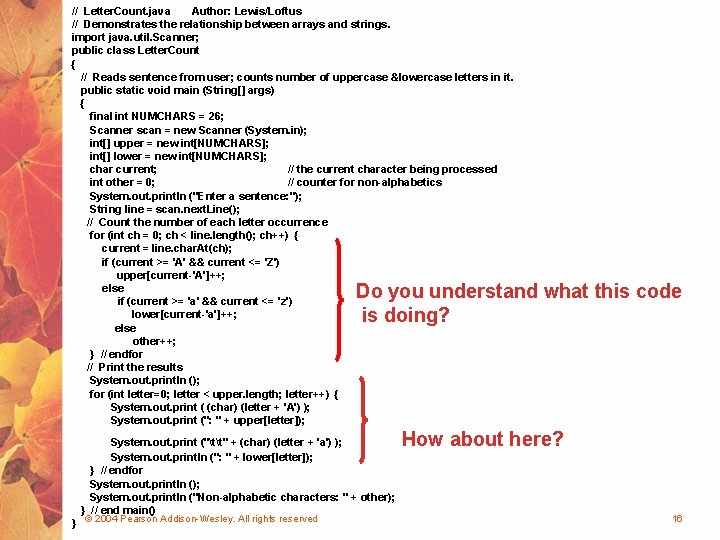
// Letter. Count. java Author: Lewis/Loftus // Demonstrates the relationship between arrays and strings. import java. util. Scanner; public class Letter. Count { // Reads sentence from user; counts number of uppercase &lowercase letters in it. public static void main (String[] args) { final int NUMCHARS = 26; Scanner scan = new Scanner (System. in); int[] upper = new int[NUMCHARS]; int[] lower = new int[NUMCHARS]; char current; // the current character being processed int other = 0; // counter for non-alphabetics System. out. println ("Enter a sentence: "); String line = scan. next. Line(); // Count the number of each letter occurrence for (int ch = 0; ch < line. length(); ch++) { current = line. char. At(ch); if (current >= 'A' && current <= 'Z') upper[current-'A']++; else if (current >= 'a' && current <= 'z') lower[current-'a']++; else other++; } // endfor // Print the results System. out. println (); for (int letter=0; letter < upper. length; letter++) { System. out. print ( (char) (letter + 'A') ); System. out. print (": " + upper[letter]); Do you understand what this code is doing? System. out. print ("tt" + (char) (letter + 'a') ); System. out. println (": " + lower[letter]); } // endfor System. out. println (); System. out. println ("Non-alphabetic characters: " + other); } // end main() } © 2004 Pearson Addison-Wesley. All rights reserved How about here? 16
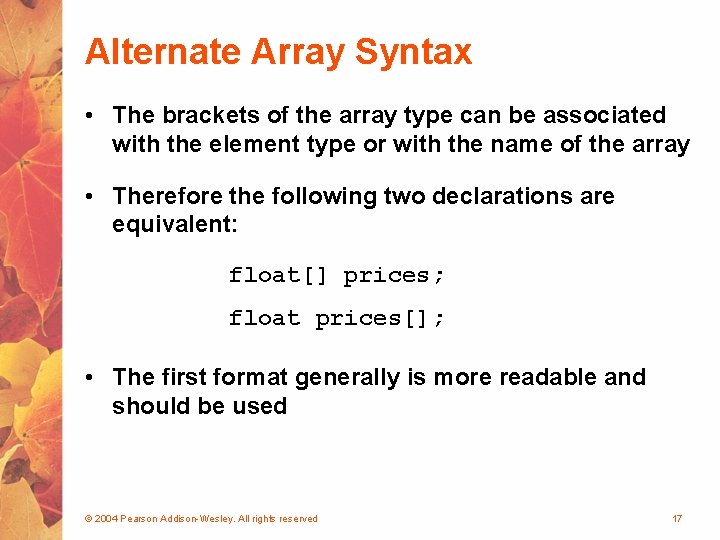
Alternate Array Syntax • The brackets of the array type can be associated with the element type or with the name of the array • Therefore the following two declarations are equivalent: float[] prices; float prices[]; • The first format generally is more readable and should be used © 2004 Pearson Addison-Wesley. All rights reserved 17
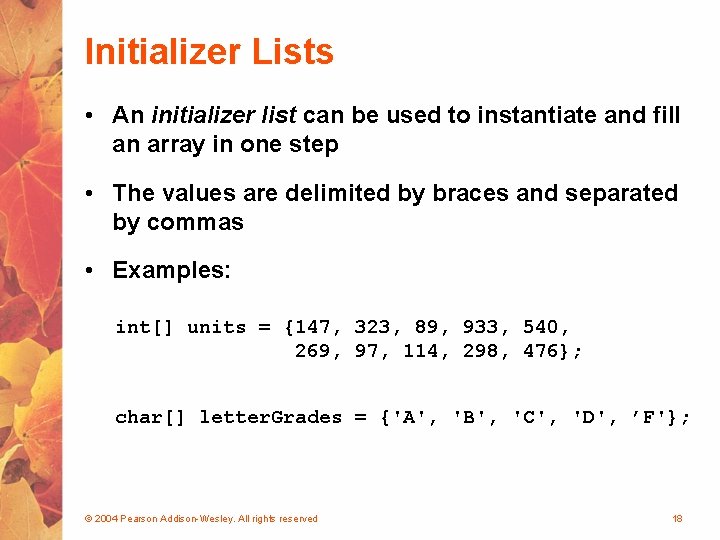
Initializer Lists • An initializer list can be used to instantiate and fill an array in one step • The values are delimited by braces and separated by commas • Examples: int[] units = {147, 323, 89, 933, 540, 269, 97, 114, 298, 476}; char[] letter. Grades = {'A', 'B', 'C', 'D', ’F'}; © 2004 Pearson Addison-Wesley. All rights reserved 18
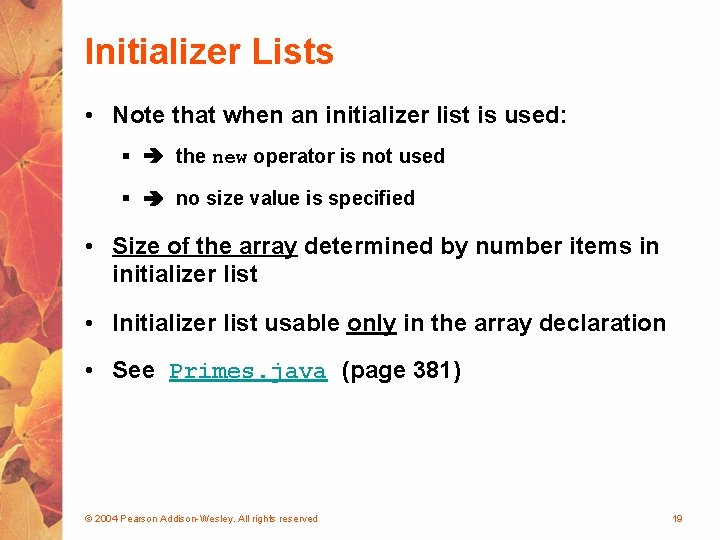
Initializer Lists • Note that when an initializer list is used: § the new operator is not used § no size value is specified • Size of the array determined by number items in initializer list • Initializer list usable only in the array declaration • See Primes. java (page 381) © 2004 Pearson Addison-Wesley. All rights reserved 19
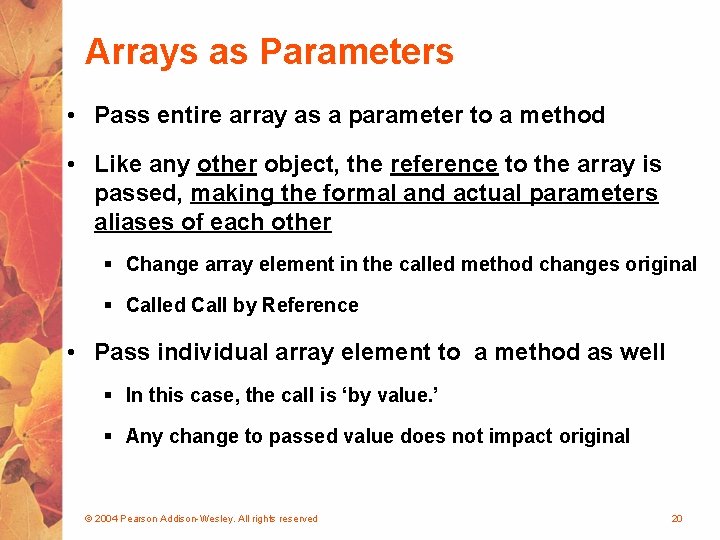
Arrays as Parameters • Pass entire array as a parameter to a method • Like any other object, the reference to the array is passed, making the formal and actual parameters aliases of each other § Change array element in the called method changes original § Called Call by Reference • Pass individual array element to a method as well § In this case, the call is ‘by value. ’ § Any change to passed value does not impact original © 2004 Pearson Addison-Wesley. All rights reserved 20
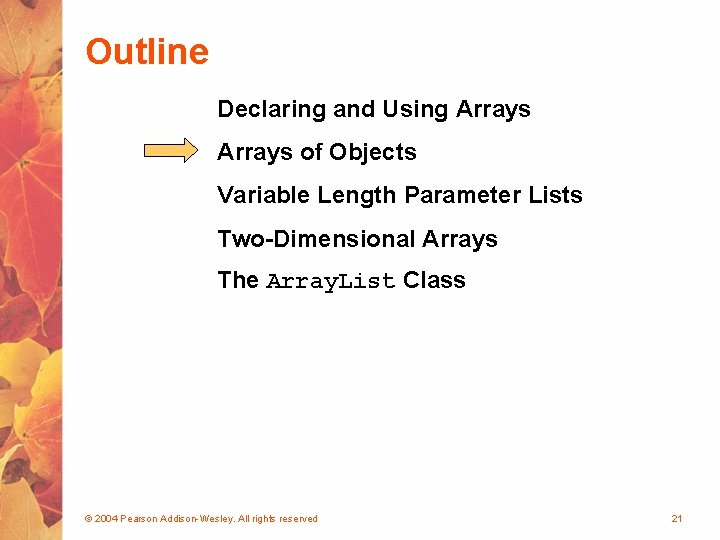
Outline Declaring and Using Arrays of Objects Variable Length Parameter Lists Two-Dimensional Arrays The Array. List Class © 2004 Pearson Addison-Wesley. All rights reserved 21
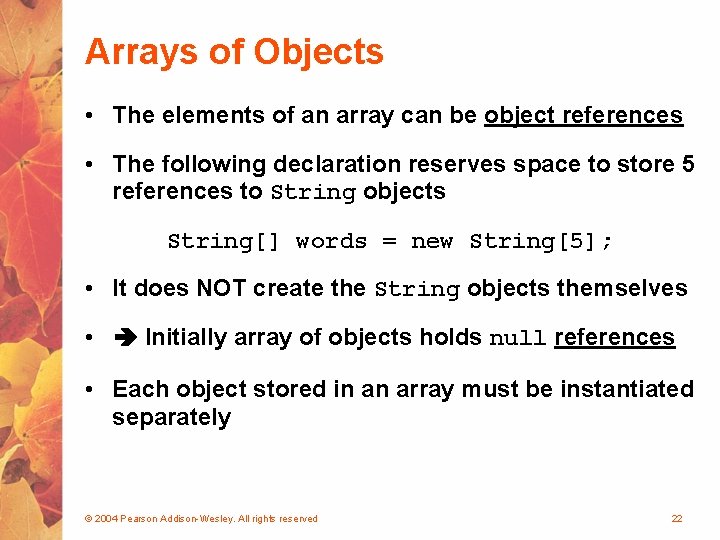
Arrays of Objects • The elements of an array can be object references • The following declaration reserves space to store 5 references to String objects String[] words = new String[5]; • It does NOT create the String objects themselves • Initially array of objects holds null references • Each object stored in an array must be instantiated separately © 2004 Pearson Addison-Wesley. All rights reserved 22
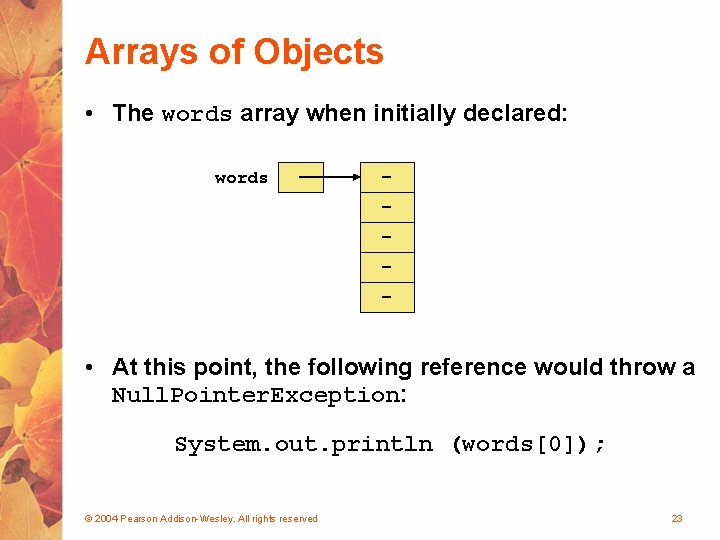
Arrays of Objects • The words array when initially declared: words - • At this point, the following reference would throw a Null. Pointer. Exception: System. out. println (words[0]); © 2004 Pearson Addison-Wesley. All rights reserved 23
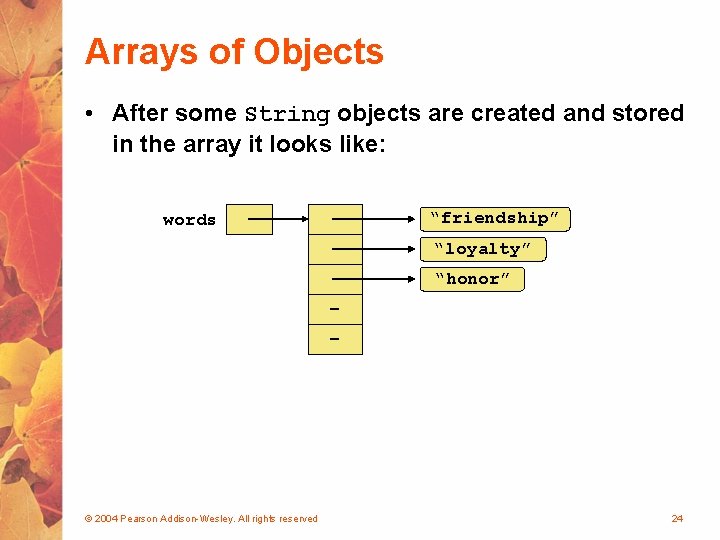
Arrays of Objects • After some String objects are created and stored in the array it looks like: “friendship” words “loyalty” “honor” - © 2004 Pearson Addison-Wesley. All rights reserved 24
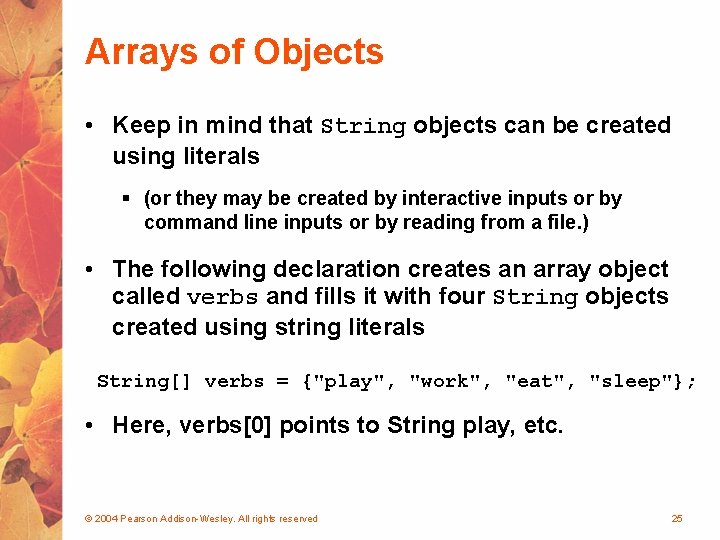
Arrays of Objects • Keep in mind that String objects can be created using literals § (or they may be created by interactive inputs or by command line inputs or by reading from a file. ) • The following declaration creates an array object called verbs and fills it with four String objects created using string literals String[] verbs = {"play", "work", "eat", "sleep"}; • Here, verbs[0] points to String play, etc. © 2004 Pearson Addison-Wesley. All rights reserved 25
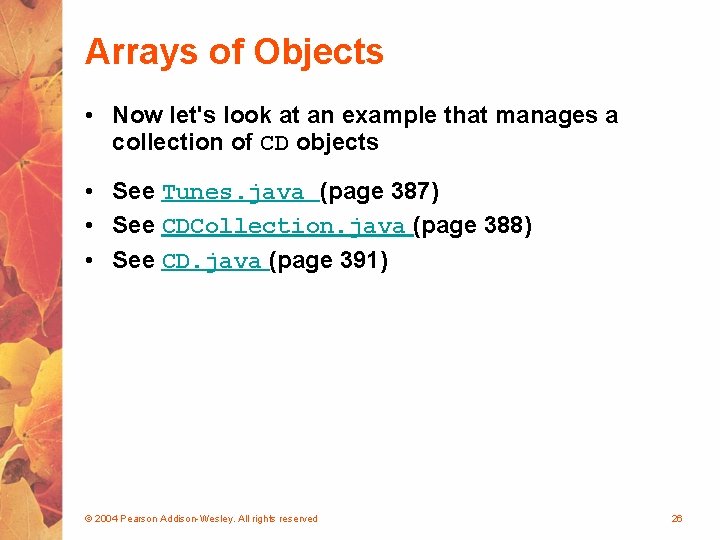
Arrays of Objects • Now let's look at an example that manages a collection of CD objects • See Tunes. java (page 387) • See CDCollection. java (page 388) • See CD. java (page 391) © 2004 Pearson Addison-Wesley. All rights reserved 26
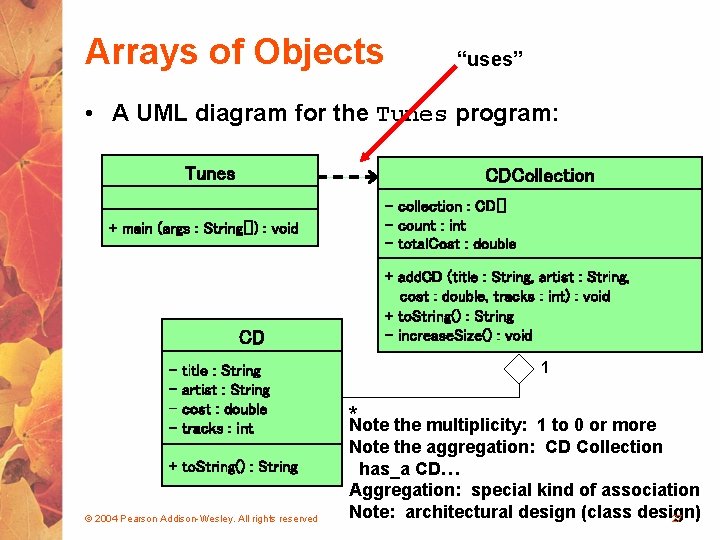
Arrays of Objects “uses” • A UML diagram for the Tunes program: Tunes CDCollection + main (args : String[]) : void CD - title : String artist : String cost : double tracks : int + to. String() : String © 2004 Pearson Addison-Wesley. All rights reserved - collection : CD[] - count : int - total. Cost : double + add. CD (title : String, artist : String, cost : double, tracks : int) : void + to. String() : String - increase. Size() : void 1 * the multiplicity: 1 to 0 or more Note the aggregation: CD Collection has_a CD… Aggregation: special kind of association Note: architectural design (class design) 27
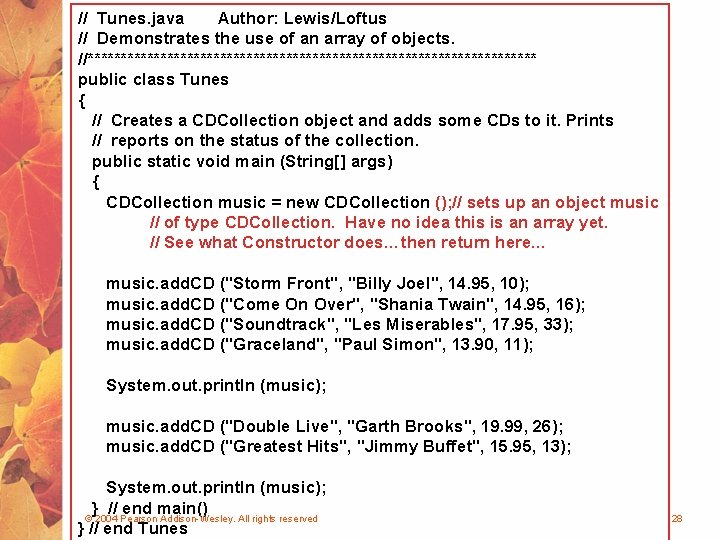
// Tunes. java Author: Lewis/Loftus // Demonstrates the use of an array of objects. //********************************** public class Tunes { // Creates a CDCollection object and adds some CDs to it. Prints // reports on the status of the collection. public static void main (String[] args) { CDCollection music = new CDCollection (); // sets up an object music // of type CDCollection. Have no idea this is an array yet. // See what Constructor does…then return here… music. add. CD ("Storm Front", "Billy Joel", 14. 95, 10); music. add. CD ("Come On Over", "Shania Twain", 14. 95, 16); music. add. CD ("Soundtrack", "Les Miserables", 17. 95, 33); music. add. CD ("Graceland", "Paul Simon", 13. 90, 11); System. out. println (music); music. add. CD ("Double Live", "Garth Brooks", 19. 99, 26); music. add. CD ("Greatest Hits", "Jimmy Buffet", 15. 95, 13); System. out. println (music); } // end main() © 2004 Pearson Addison-Wesley. All rights reserved } // end Tunes 28
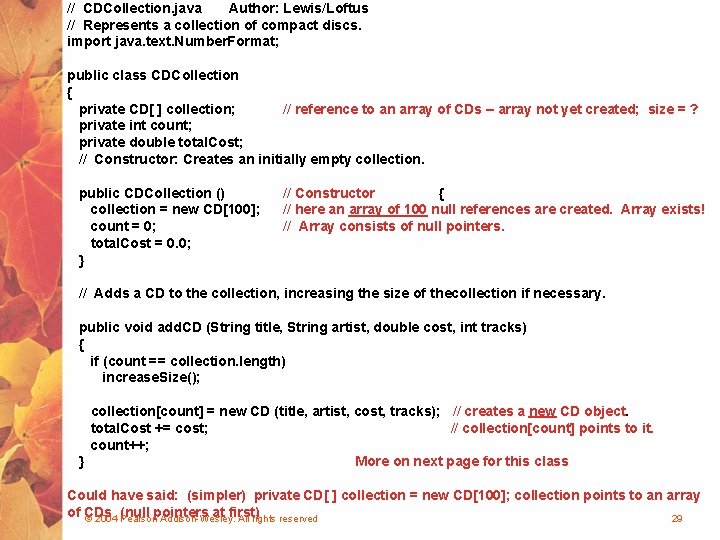
// CDCollection. java Author: Lewis/Loftus // Represents a collection of compact discs. import java. text. Number. Format; public class CDCollection { private CD[ ] collection; // reference to an array of CDs – array not yet created; size = ? private int count; private double total. Cost; // Constructor: Creates an initially empty collection. public CDCollection () collection = new CD[100]; count = 0; total. Cost = 0. 0; } // Constructor { // here an array of 100 null references are created. Array exists! // Array consists of null pointers. // Adds a CD to the collection, increasing the size of thecollection if necessary. public void add. CD (String title, String artist, double cost, int tracks) { if (count == collection. length) increase. Size(); collection[count] = new CD (title, artist, cost, tracks); // creates a new CD object. total. Cost += cost; // collection[count] points to it. count++; } More on next page for this class Could have said: (simpler) private CD[ ] collection = new CD[100]; collection points to an array of CDs (null pointers at first) © 2004 Pearson Addison-Wesley. All rights reserved 29
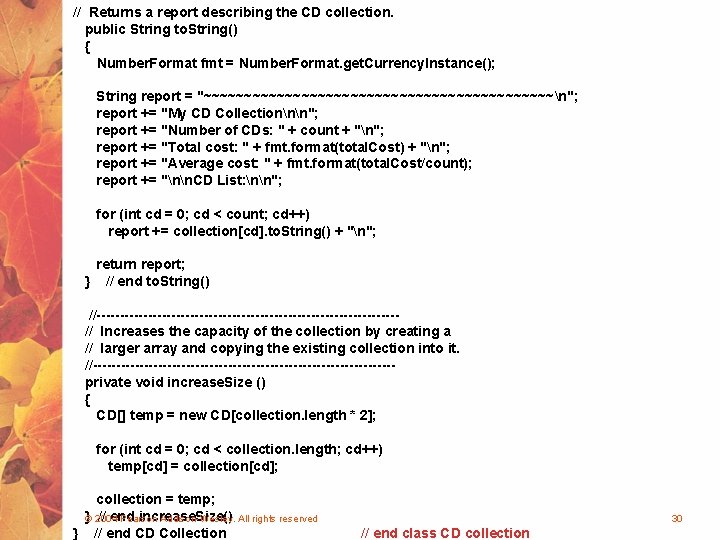
// Returns a report describing the CD collection. public String to. String() { Number. Format fmt = Number. Format. get. Currency. Instance(); String report = "~~~~~~~~~~~~~~~~~~~~~~n"; report += "My CD Collectionnn"; report += "Number of CDs: " + count + "n"; report += "Total cost: " + fmt. format(total. Cost) + "n"; report += "Average cost: " + fmt. format(total. Cost/count); report += "nn. CD List: nn"; for (int cd = 0; cd < count; cd++) report += collection[cd]. to. String() + "n"; return report; } // end to. String() //--------------------------------// Increases the capacity of the collection by creating a // larger array and copying the existing collection into it. //--------------------------------private void increase. Size () { CD[] temp = new CD[collection. length * 2]; for (int cd = 0; cd < collection. length; cd++) temp[cd] = collection[cd]; collection = temp; }© 2004 // end increase. Size() Pearson Addison-Wesley. All rights reserved } // end CD Collection 30 // end class CD collection
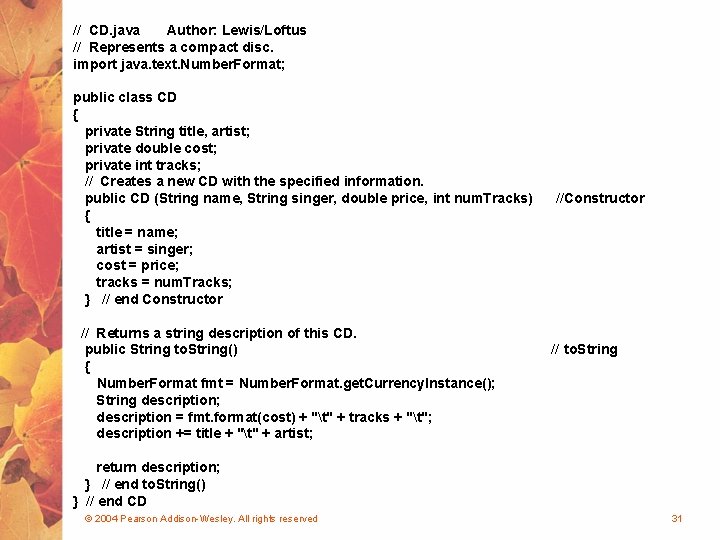
// CD. java Author: Lewis/Loftus // Represents a compact disc. import java. text. Number. Format; public class CD { private String title, artist; private double cost; private int tracks; // Creates a new CD with the specified information. public CD (String name, String singer, double price, int num. Tracks) { title = name; artist = singer; cost = price; tracks = num. Tracks; } // end Constructor // Returns a string description of this CD. public String to. String() { Number. Format fmt = Number. Format. get. Currency. Instance(); String description; description = fmt. format(cost) + "t" + tracks + "t"; description += title + "t" + artist; //Constructor // to. String return description; } // end to. String() } // end CD © 2004 Pearson Addison-Wesley. All rights reserved 31
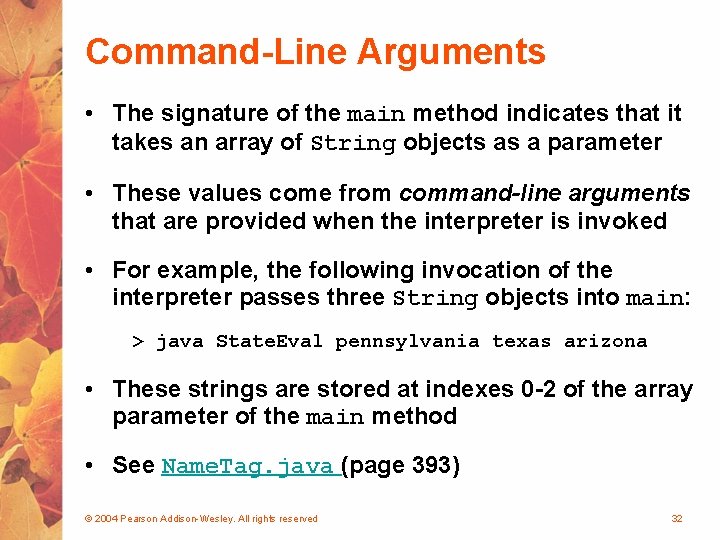
Command-Line Arguments • The signature of the main method indicates that it takes an array of String objects as a parameter • These values come from command-line arguments that are provided when the interpreter is invoked • For example, the following invocation of the interpreter passes three String objects into main: > java State. Eval pennsylvania texas arizona • These strings are stored at indexes 0 -2 of the array parameter of the main method • See Name. Tag. java (page 393) © 2004 Pearson Addison-Wesley. All rights reserved 32
Parallel arrays in c
Array of arrays c++
Java array operations
潘仁義
C++ parallel arrays
Why do we need arrays?
Dynamic arrays and amortized analysis
Que es un arreglo unidimensional en java
Arreglos bidimensionales en java
Array mips
Polynomial representation using array in c
Array of strings assembly
Global arrays in c
Computer science arrays
Searching and sorting arrays in c++
Arrays visual basic
Python parallel arrays
Array advantages and disadvantages
I wonder is it possible
2d array pascal
Mips arrays
Creating arrays matlab
Arrays as adt
Java partially filled array
Redundancy array of independent disk
Python list of arrays
Arrays
Day 3: arrays
Redundant arrays of inexpensive disks
Small basic array
Advantages of dynamic memory allocation
Microled arrays
Are vectors dynamic arrays