Chapter 5 Repetition while dowhile and for loops
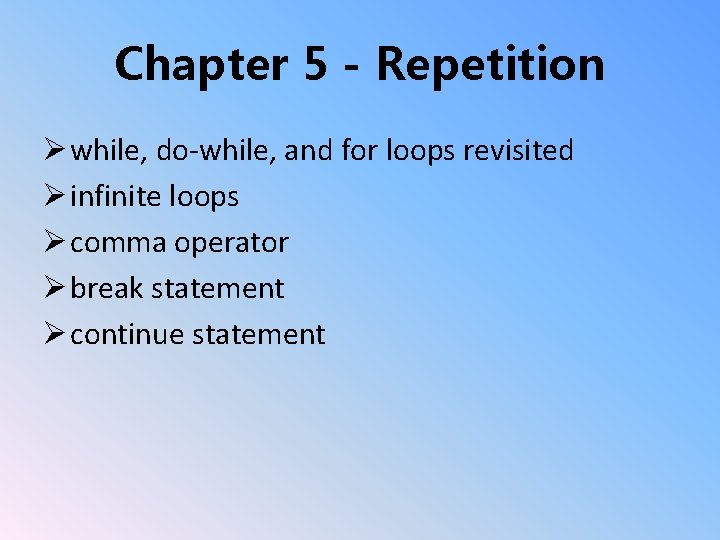
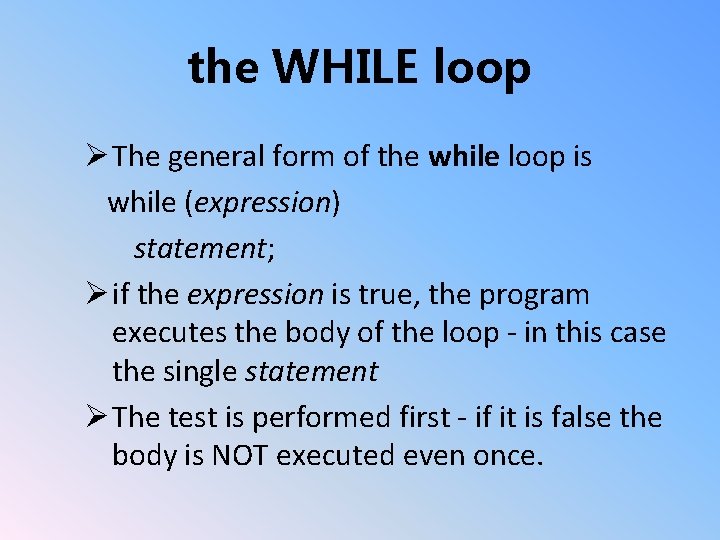
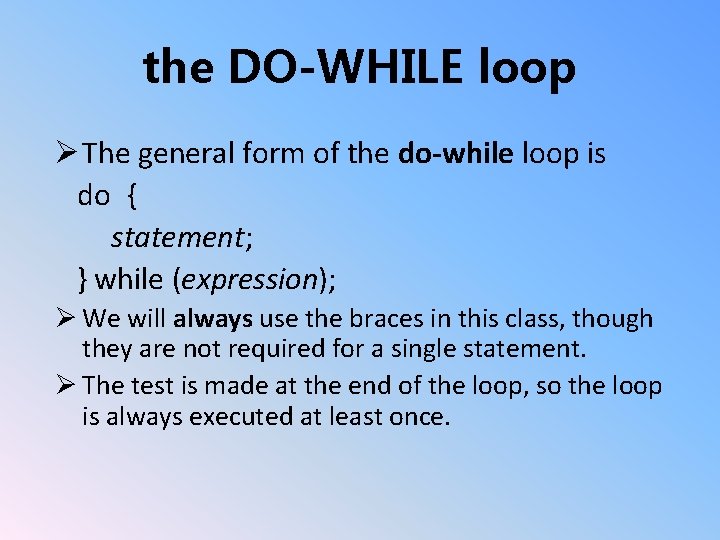
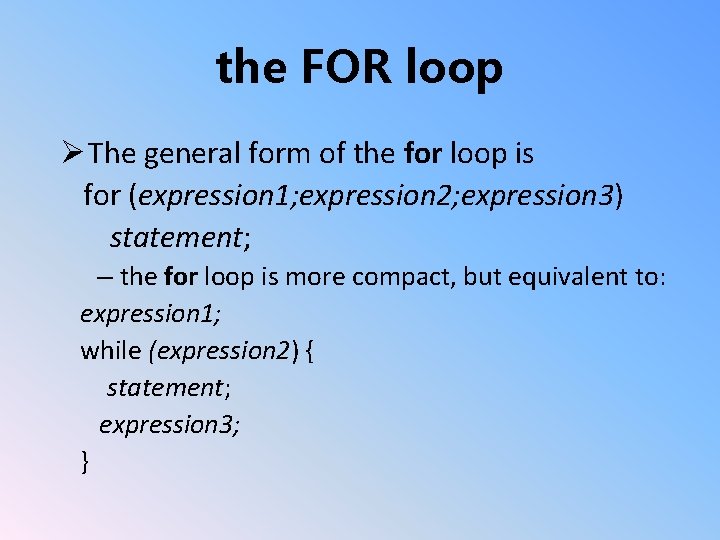
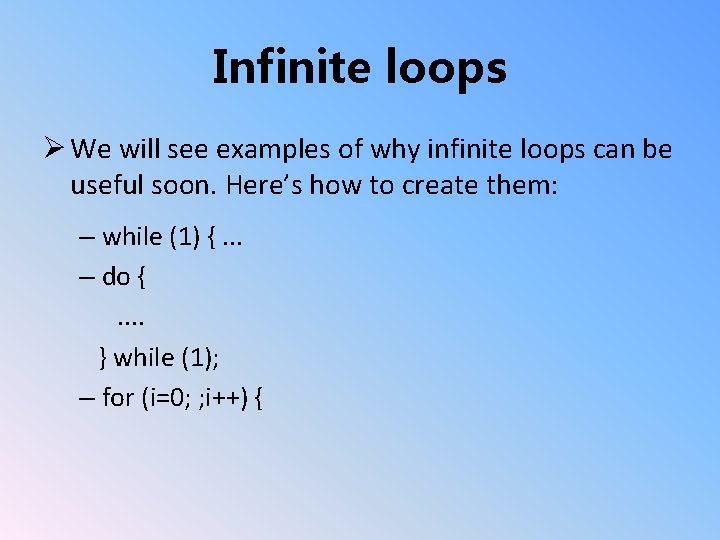
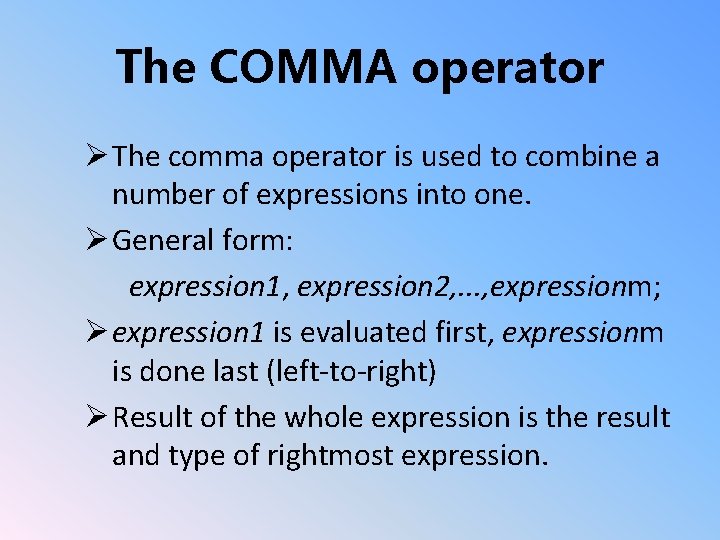
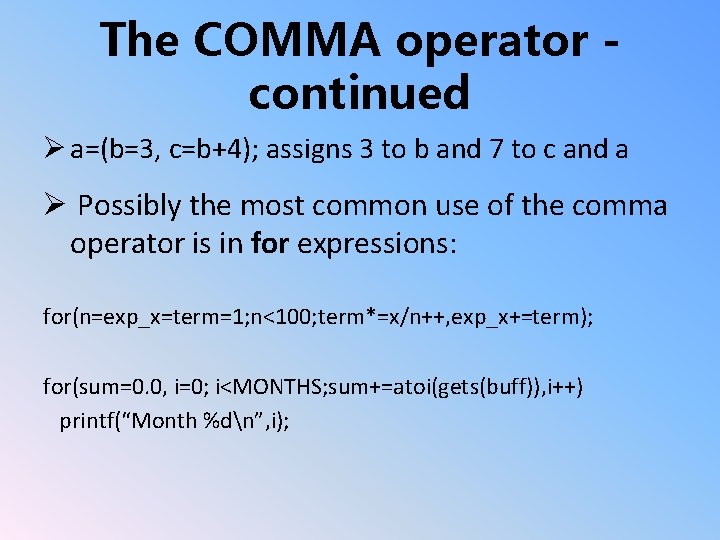
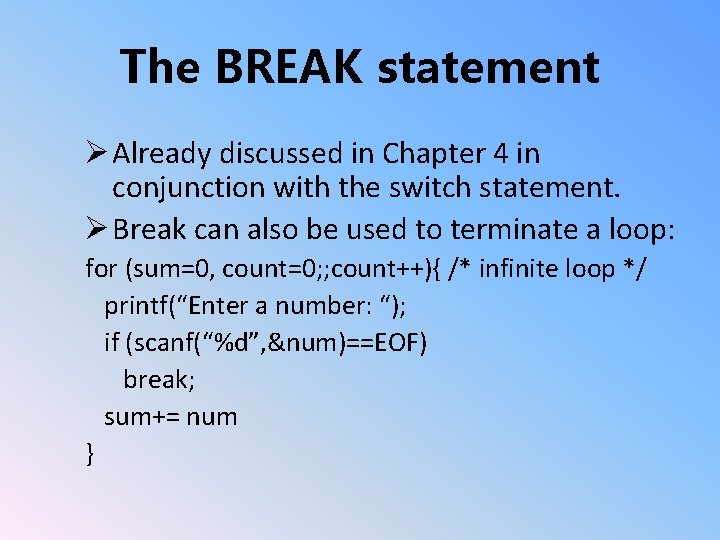
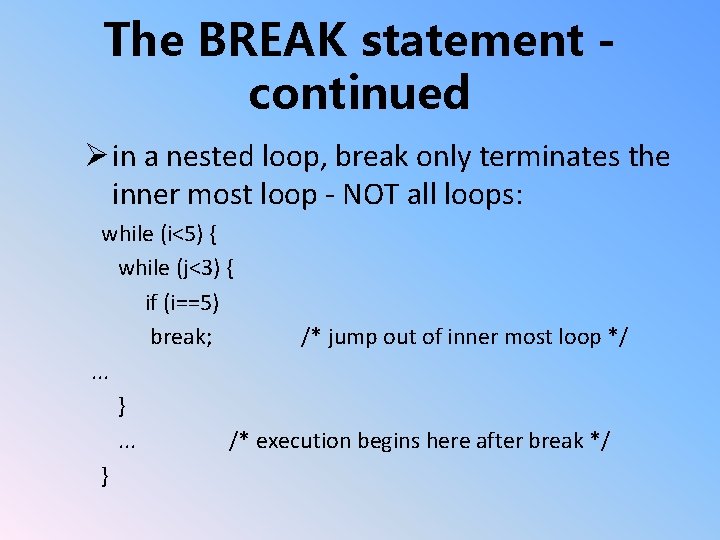
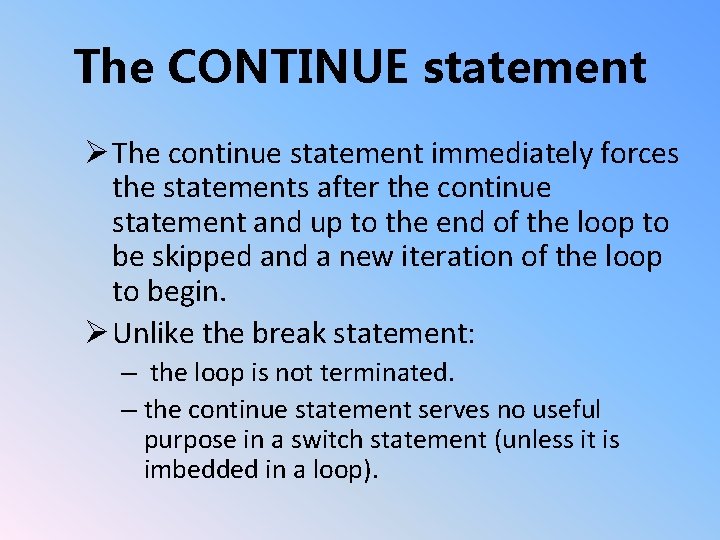
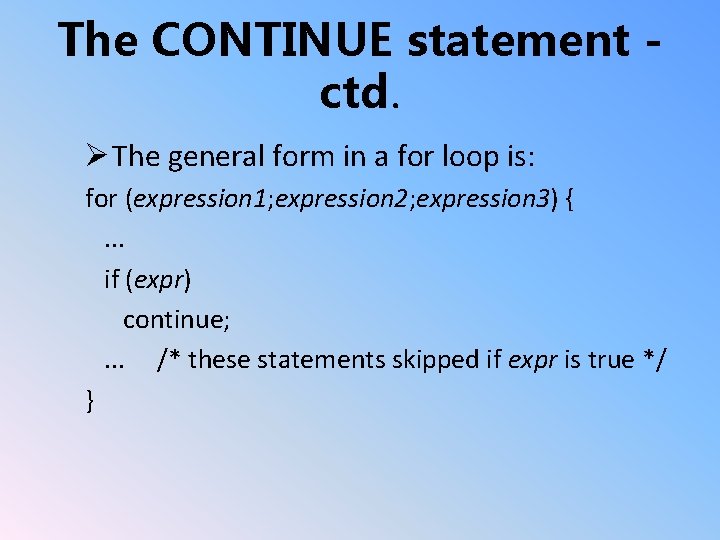
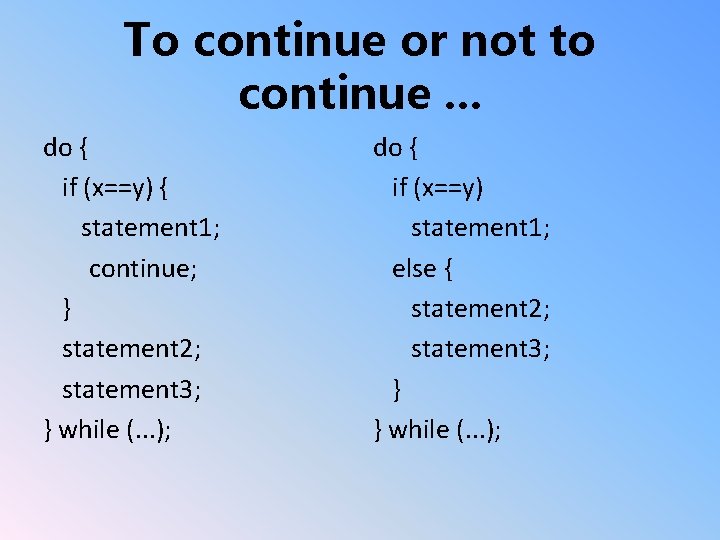
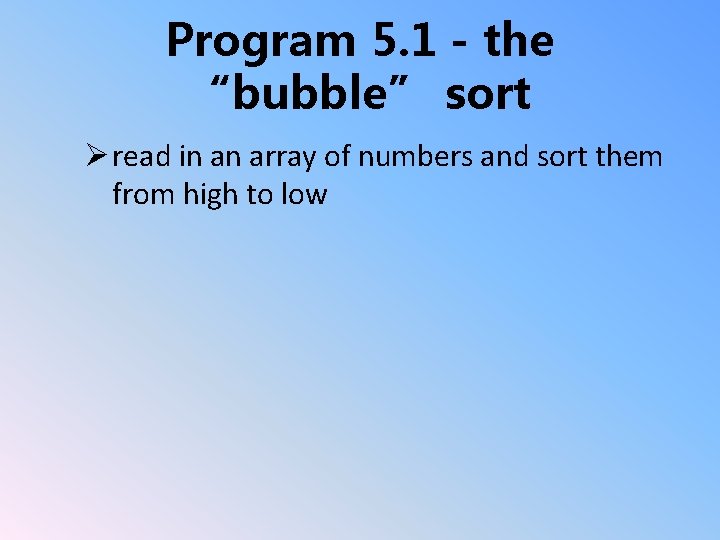
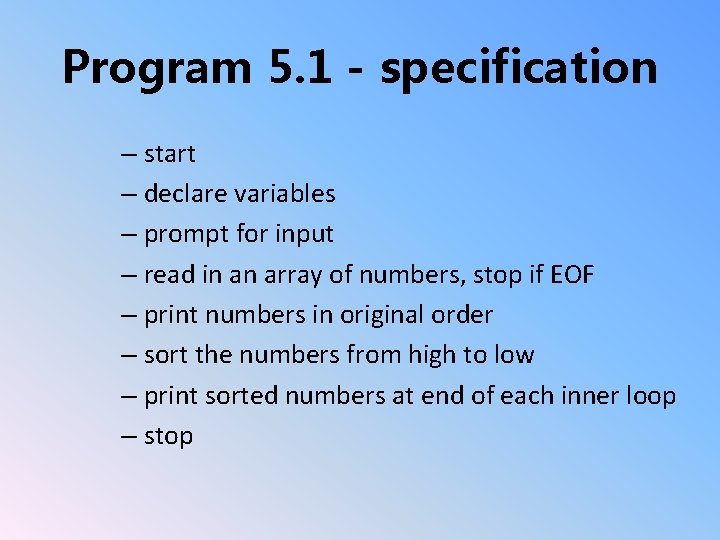
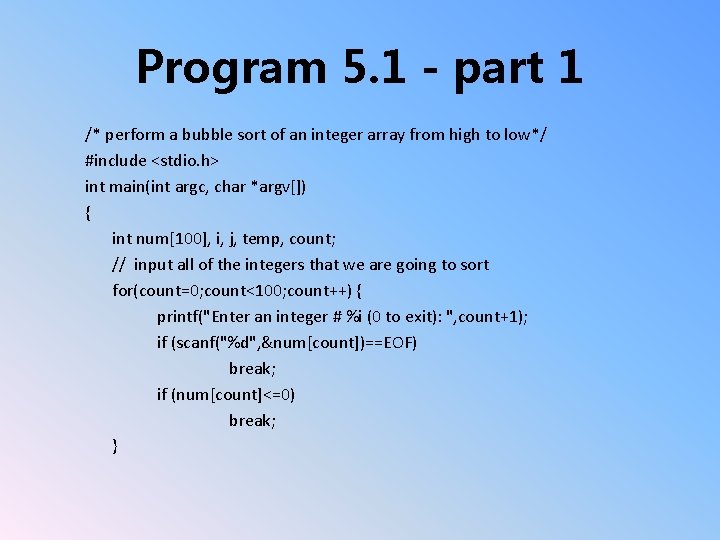
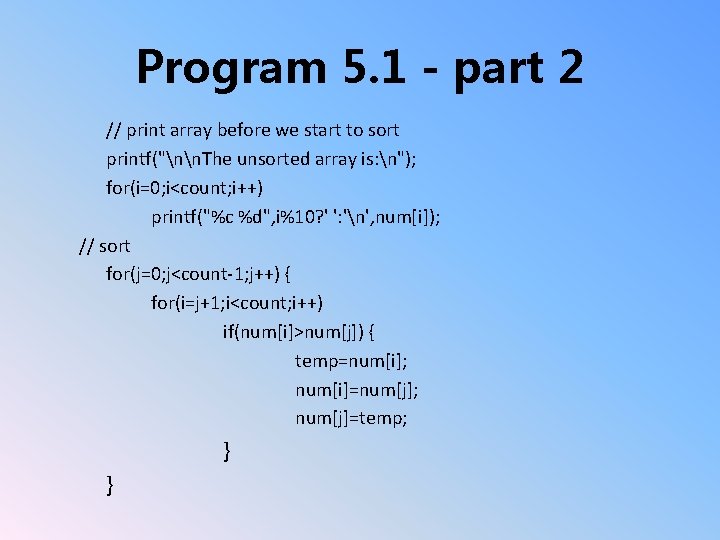
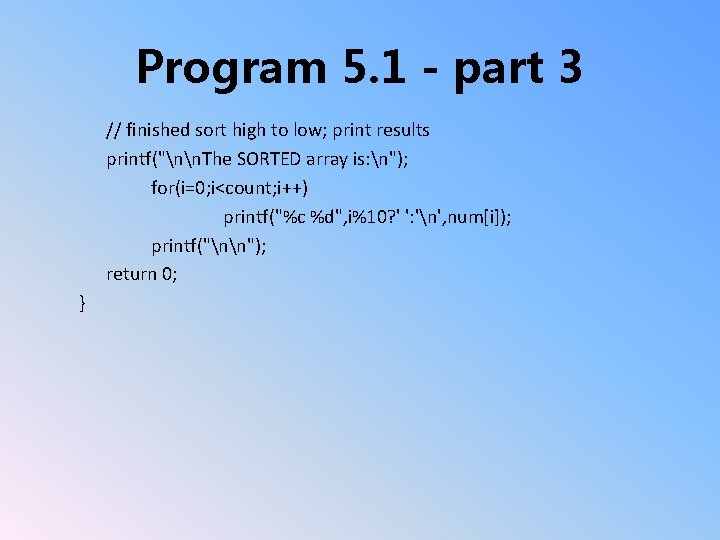
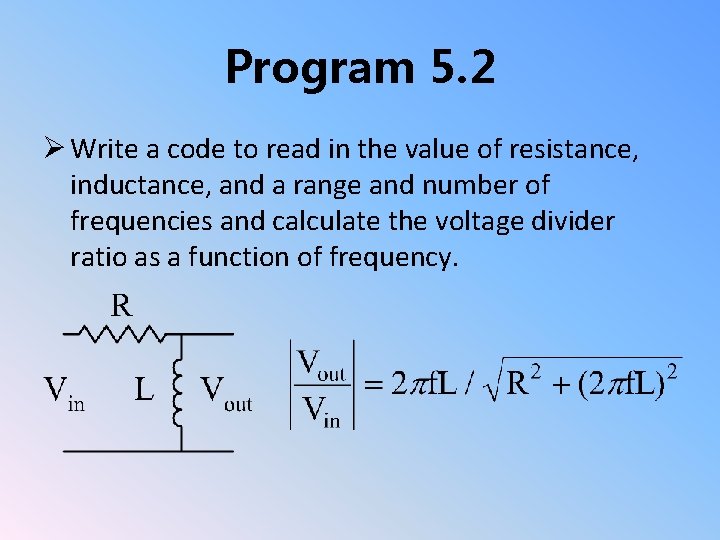
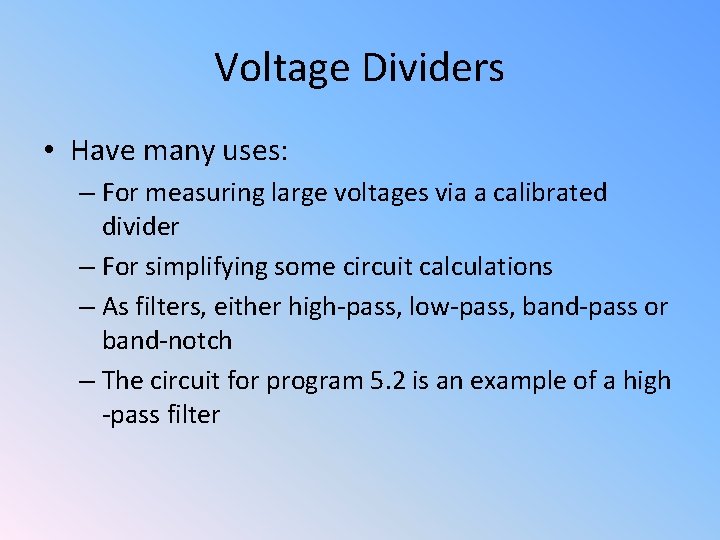
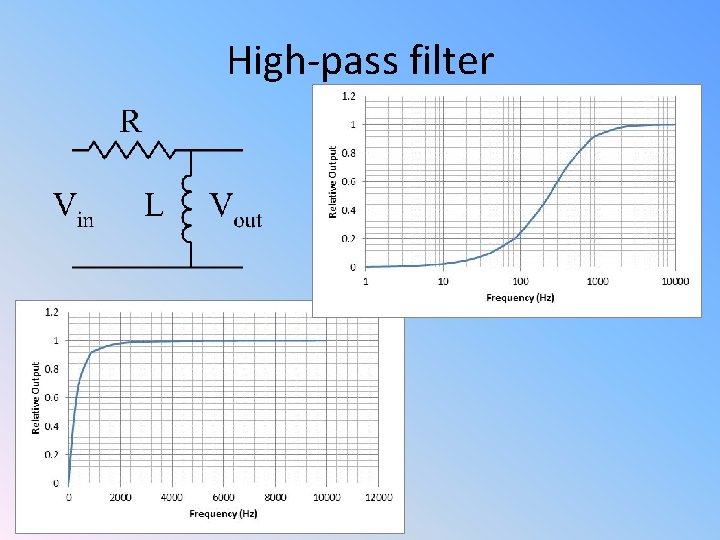
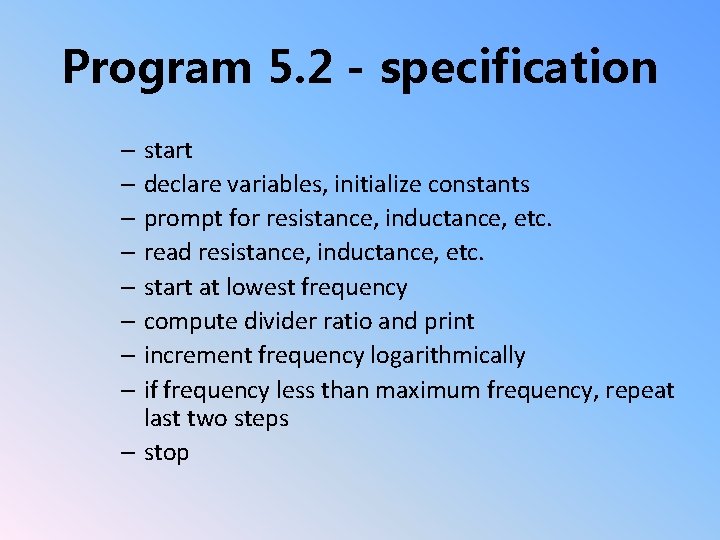
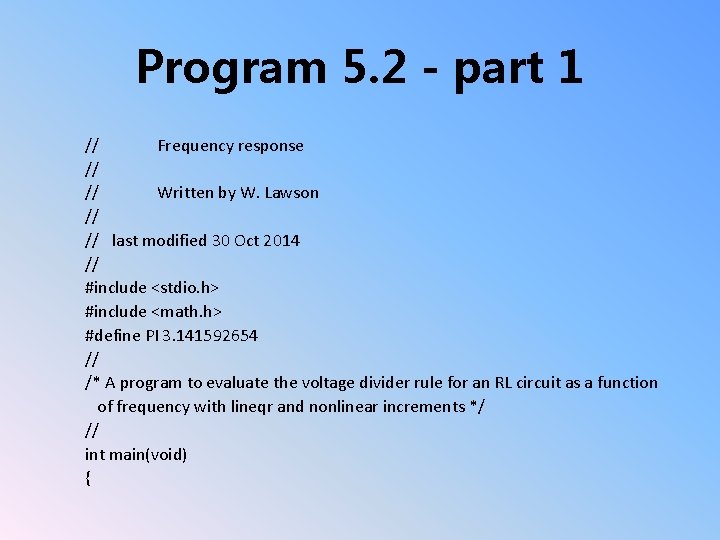
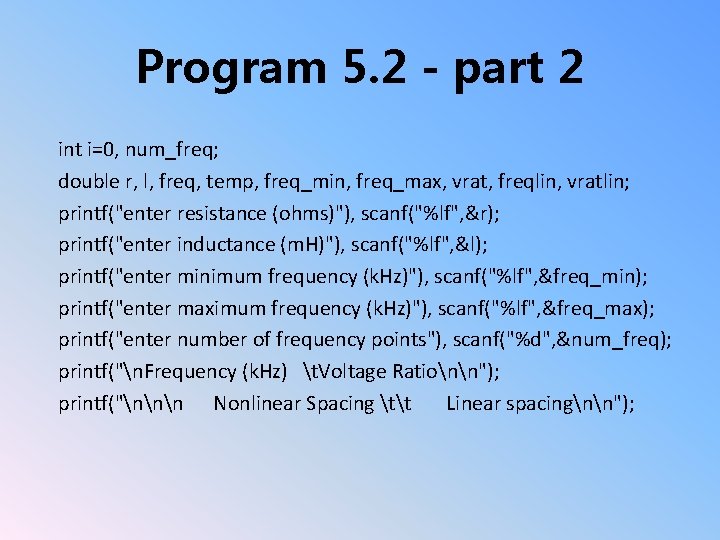
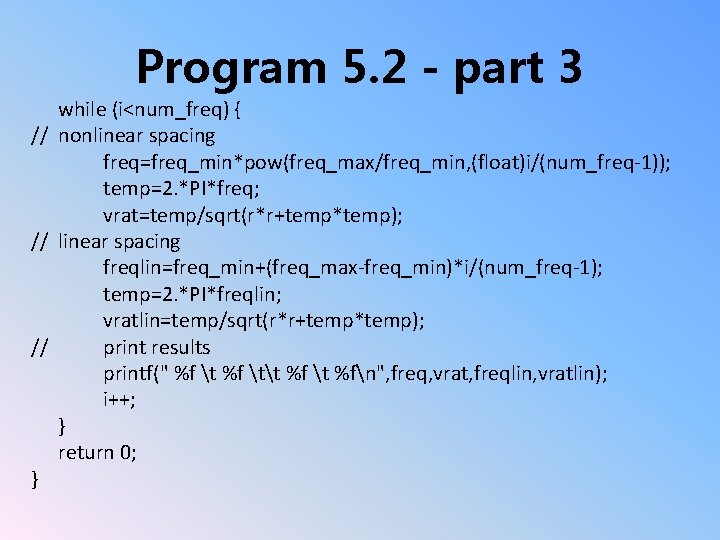
- Slides: 24
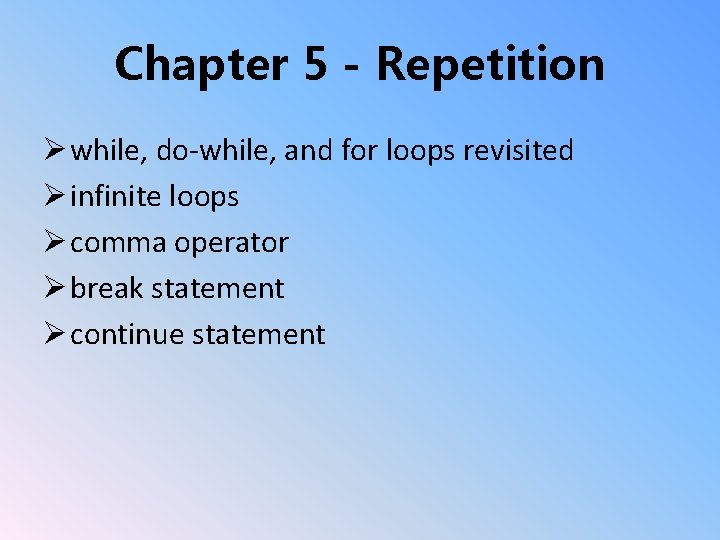
Chapter 5 - Repetition Ø while, do-while, and for loops revisited Ø infinite loops Ø comma operator Ø break statement Ø continue statement
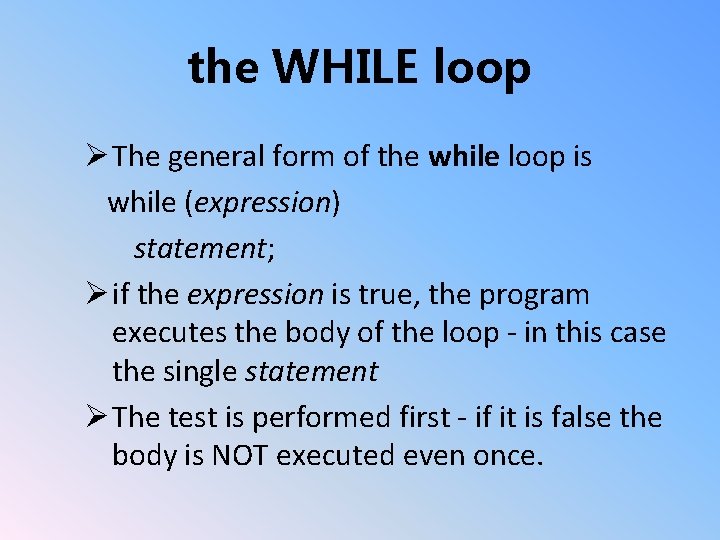
the WHILE loop Ø The general form of the while loop is while (expression) statement; Ø if the expression is true, the program executes the body of the loop - in this case the single statement Ø The test is performed first - if it is false the body is NOT executed even once.
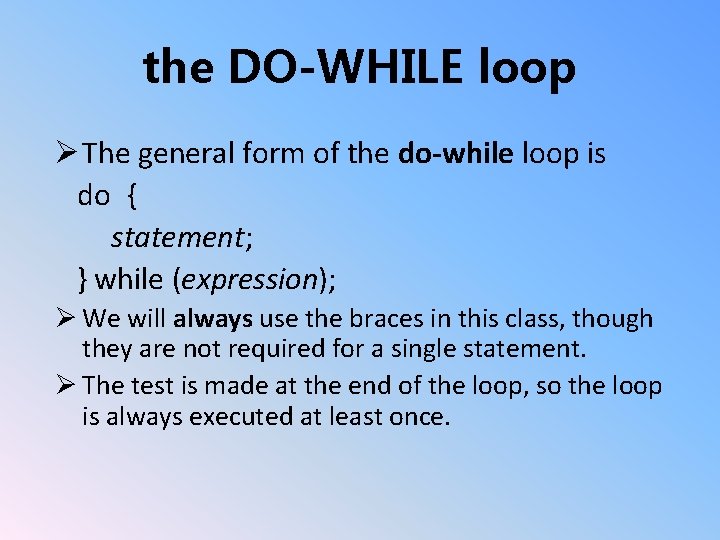
the DO-WHILE loop Ø The general form of the do-while loop is do { statement; } while (expression); Ø We will always use the braces in this class, though they are not required for a single statement. Ø The test is made at the end of the loop, so the loop is always executed at least once.
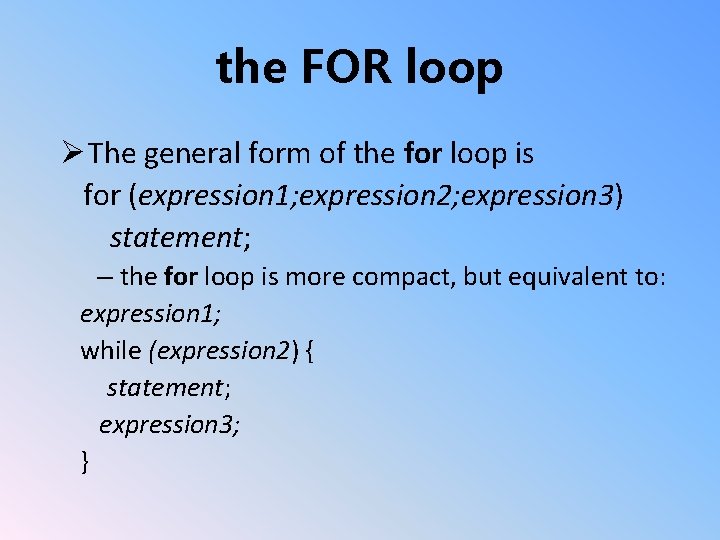
the FOR loop Ø The general form of the for loop is for (expression 1; expression 2; expression 3) statement; – the for loop is more compact, but equivalent to: expression 1; while (expression 2) { statement; expression 3; }
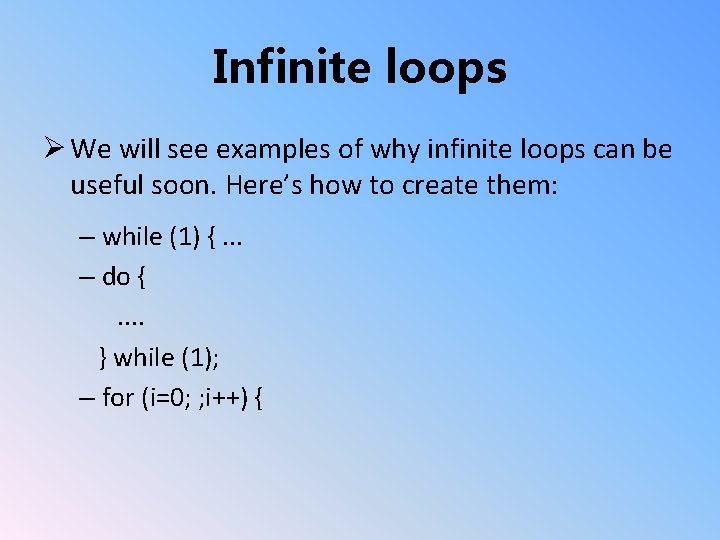
Infinite loops Ø We will see examples of why infinite loops can be useful soon. Here’s how to create them: – while (1) {. . . – do {. . } while (1); – for (i=0; ; i++) {
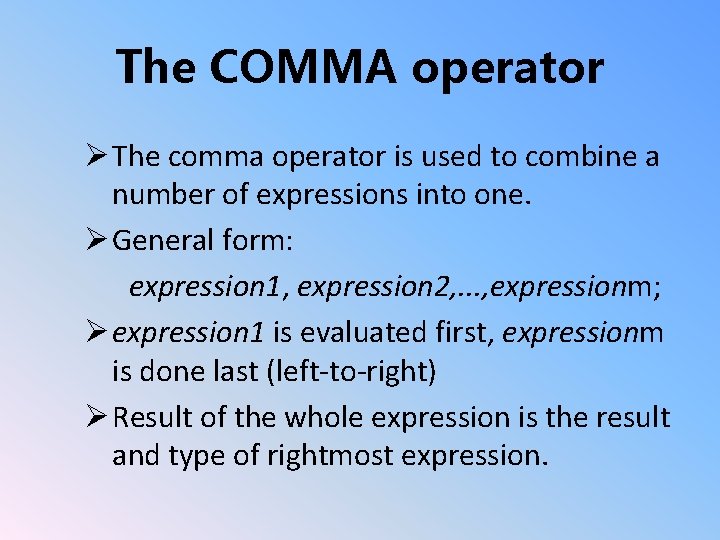
The COMMA operator Ø The comma operator is used to combine a number of expressions into one. Ø General form: expression 1, expression 2, . . . , expressionm; Ø expression 1 is evaluated first, expressionm is done last (left-to-right) Ø Result of the whole expression is the result and type of rightmost expression.
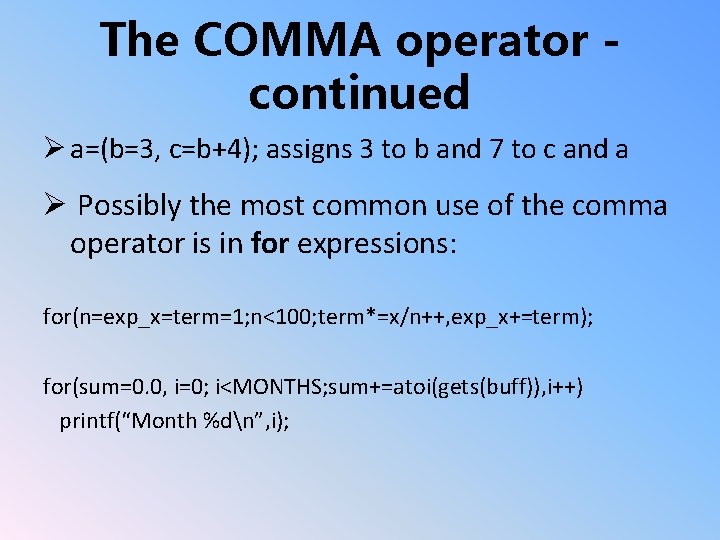
The COMMA operator continued Ø a=(b=3, c=b+4); assigns 3 to b and 7 to c and a Ø Possibly the most common use of the comma operator is in for expressions: for(n=exp_x=term=1; n<100; term*=x/n++, exp_x+=term); for(sum=0. 0, i=0; i<MONTHS; sum+=atoi(gets(buff)), i++) printf(“Month %dn”, i);
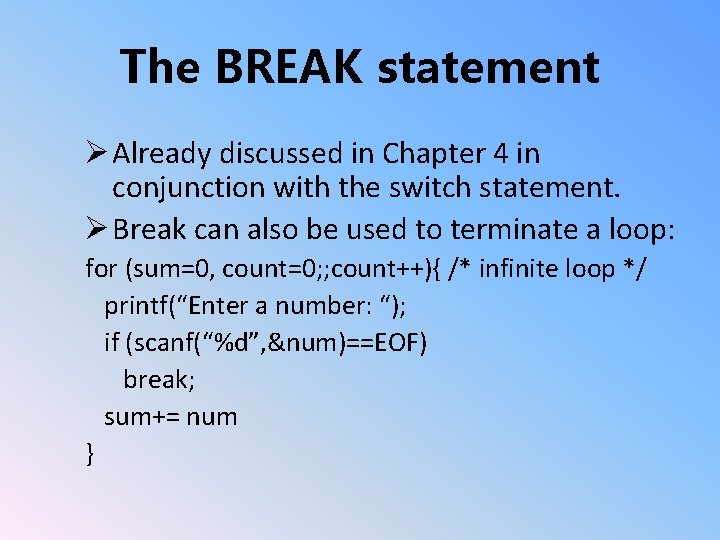
The BREAK statement Ø Already discussed in Chapter 4 in conjunction with the switch statement. Ø Break can also be used to terminate a loop: for (sum=0, count=0; ; count++){ /* infinite loop */ printf(“Enter a number: “); if (scanf(“%d”, &num)==EOF) break; sum+= num }
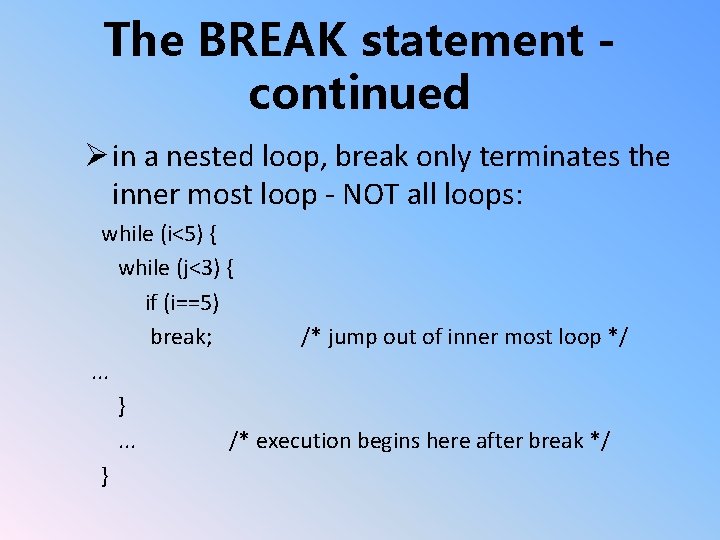
The BREAK statement continued Ø in a nested loop, break only terminates the inner most loop - NOT all loops: while (i<5) { while (j<3) { if (i==5) break; /* jump out of inner most loop */. . . }. . . /* execution begins here after break */ }
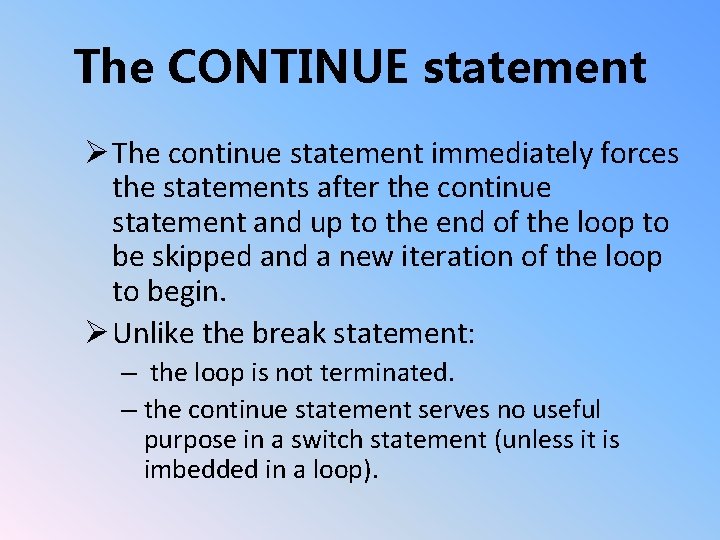
The CONTINUE statement Ø The continue statement immediately forces the statements after the continue statement and up to the end of the loop to be skipped and a new iteration of the loop to begin. Ø Unlike the break statement: – the loop is not terminated. – the continue statement serves no useful purpose in a switch statement (unless it is imbedded in a loop).
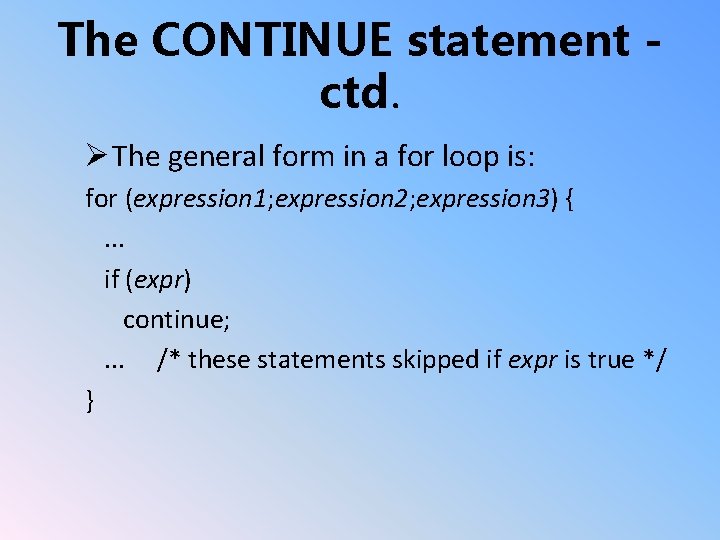
The CONTINUE statement ctd. Ø The general form in a for loop is: for (expression 1; expression 2; expression 3) {. . . if (expr) continue; . . . /* these statements skipped if expr is true */ }
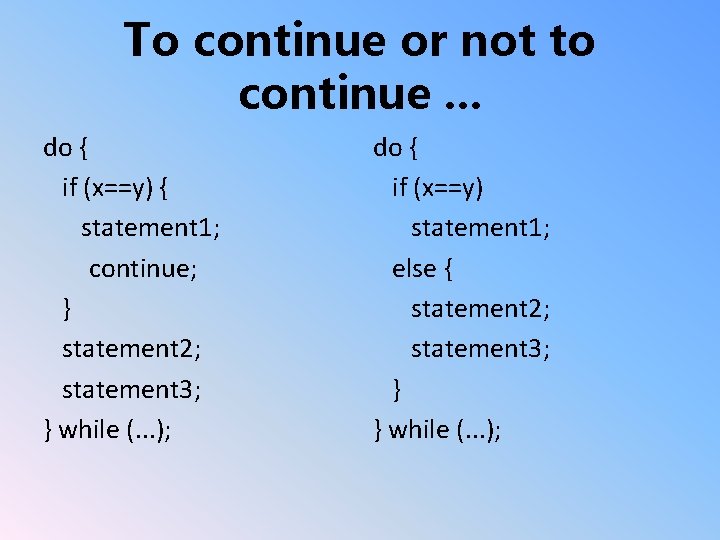
To continue or not to continue. . . do { if (x==y) { statement 1; continue; } statement 2; statement 3; } while (. . . ); do { if (x==y) statement 1; else { statement 2; statement 3; } } while (. . . );
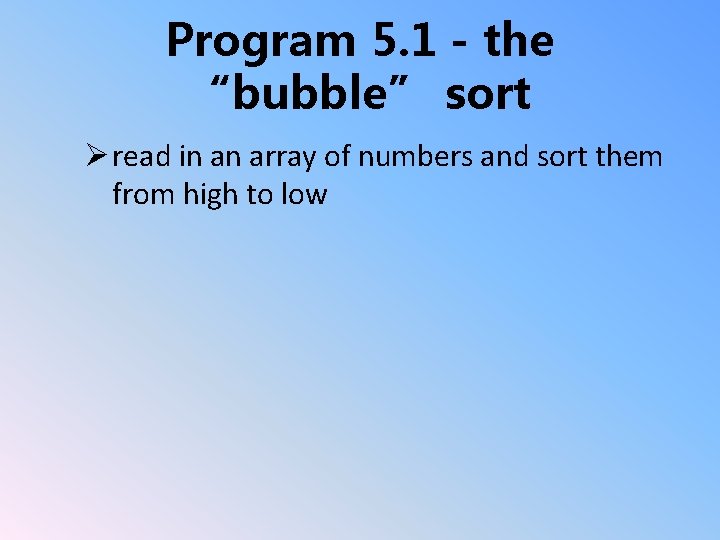
Program 5. 1 - the “bubble” sort Ø read in an array of numbers and sort them from high to low
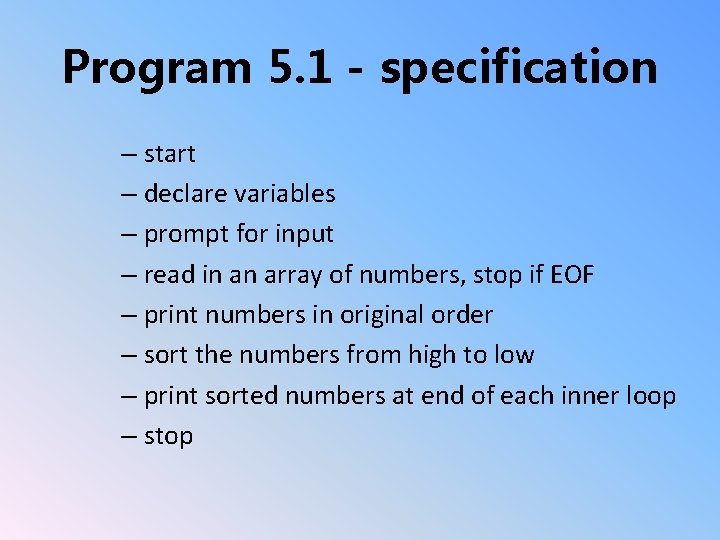
Program 5. 1 - specification – start – declare variables – prompt for input – read in an array of numbers, stop if EOF – print numbers in original order – sort the numbers from high to low – print sorted numbers at end of each inner loop – stop
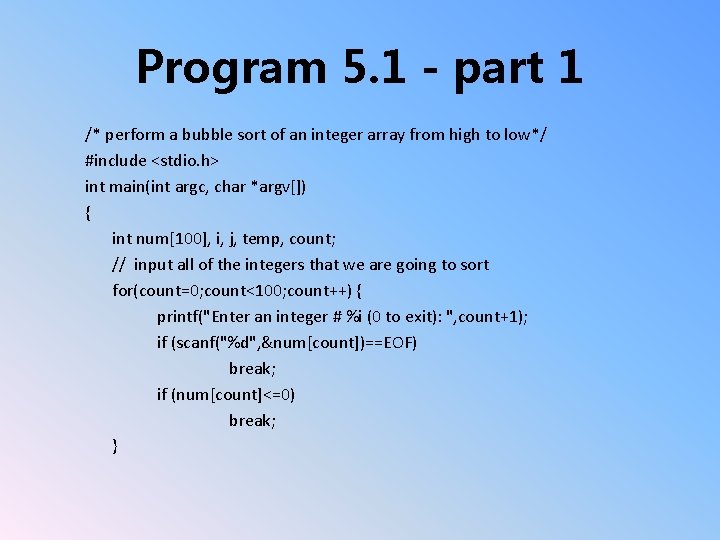
Program 5. 1 - part 1 /* perform a bubble sort of an integer array from high to low*/ #include <stdio. h> int main(int argc, char *argv[]) { int num[100], i, j, temp, count; // input all of the integers that we are going to sort for(count=0; count<100; count++) { printf("Enter an integer # %i (0 to exit): ", count+1); if (scanf("%d", &num[count])==EOF) break; if (num[count]<=0) break; }
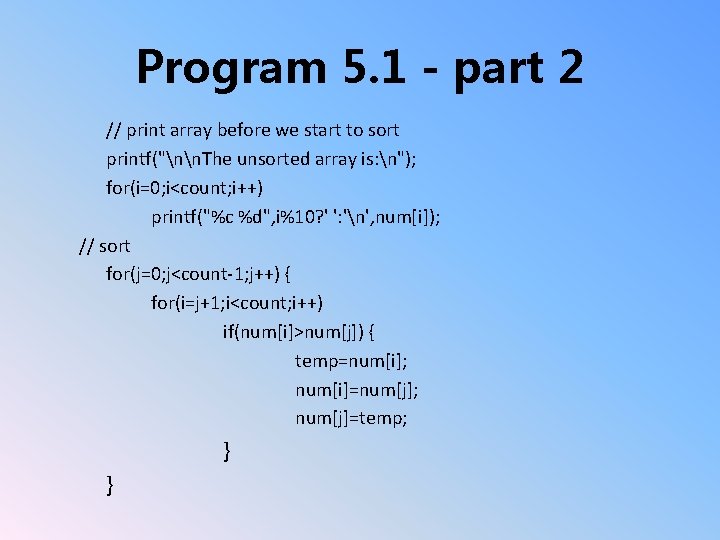
Program 5. 1 - part 2 // print array before we start to sort printf("nn. The unsorted array is: n"); for(i=0; i<count; i++) printf("%c %d", i%10? ' ': 'n', num[i]); // sort for(j=0; j<count-1; j++) { for(i=j+1; i<count; i++) if(num[i]>num[j]) { temp=num[i]; num[i]=num[j]; num[j]=temp; } }
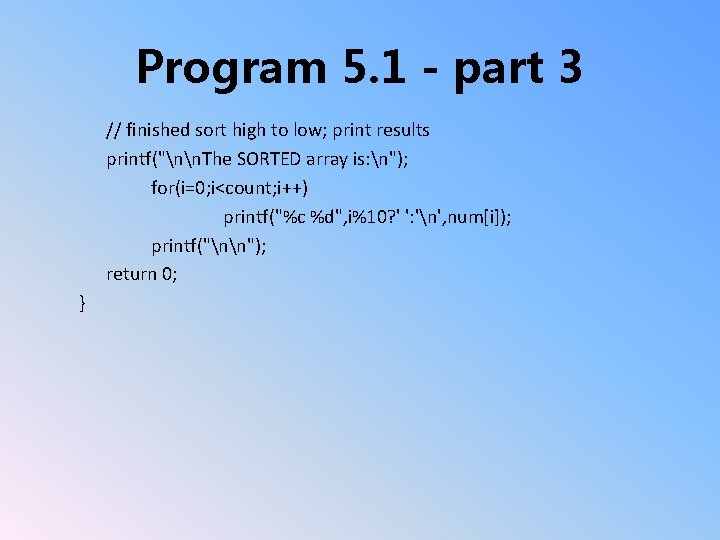
Program 5. 1 - part 3 // finished sort high to low; print results printf("nn. The SORTED array is: n"); for(i=0; i<count; i++) printf("%c %d", i%10? ' ': 'n', num[i]); printf("nn"); return 0; }
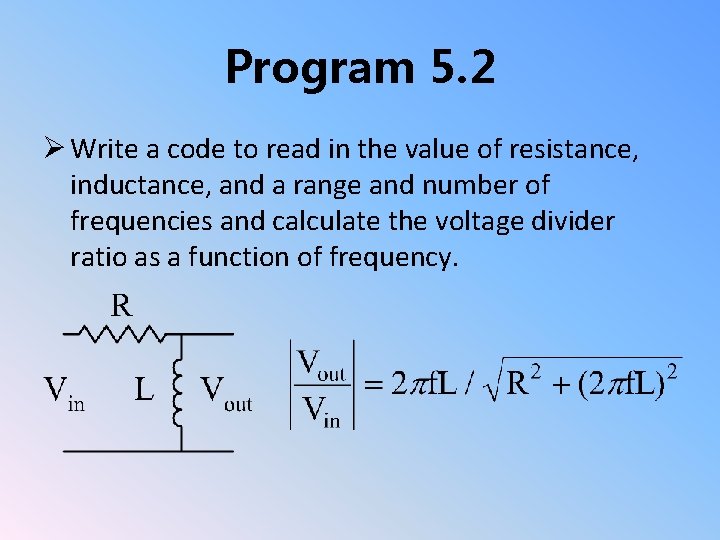
Program 5. 2 Ø Write a code to read in the value of resistance, inductance, and a range and number of frequencies and calculate the voltage divider ratio as a function of frequency.
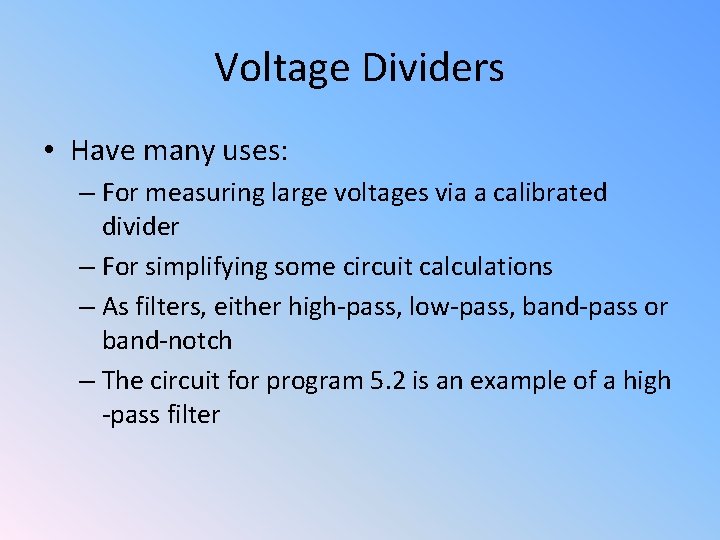
Voltage Dividers • Have many uses: – For measuring large voltages via a calibrated divider – For simplifying some circuit calculations – As filters, either high-pass, low-pass, band-pass or band-notch – The circuit for program 5. 2 is an example of a high -pass filter
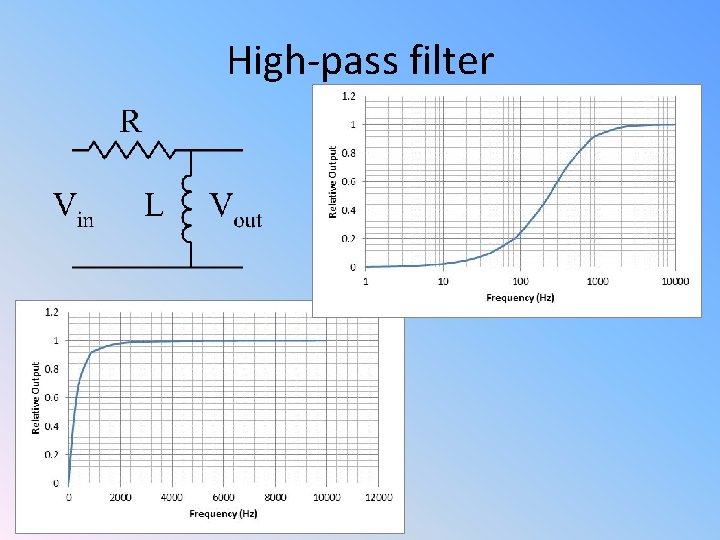
High-pass filter
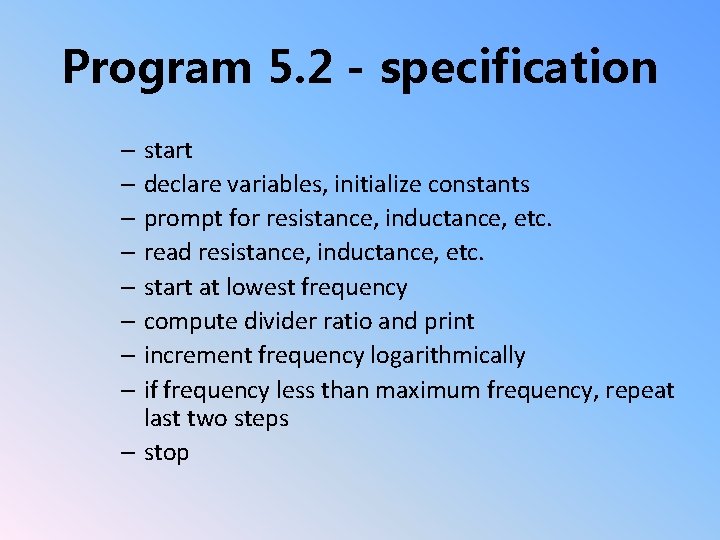
Program 5. 2 - specification – start – declare variables, initialize constants – prompt for resistance, inductance, etc. – read resistance, inductance, etc. – start at lowest frequency – compute divider ratio and print – increment frequency logarithmically – if frequency less than maximum frequency, repeat last two steps – stop
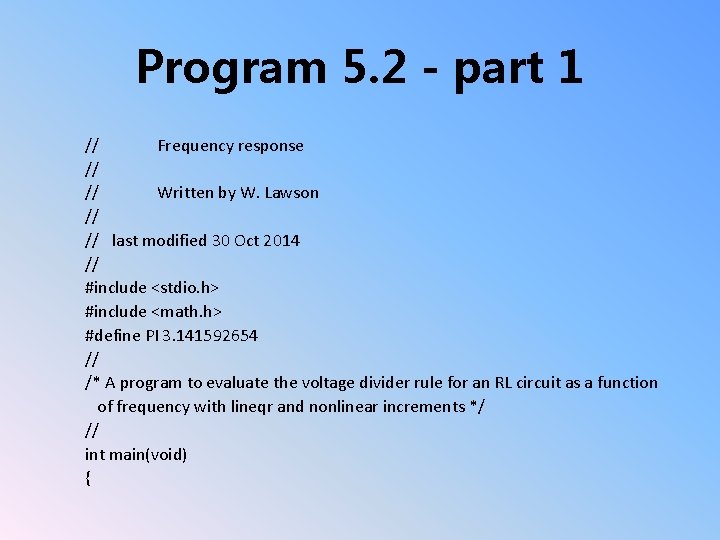
Program 5. 2 - part 1 // Frequency response // // Written by W. Lawson // // last modified 30 Oct 2014 // #include <stdio. h> #include <math. h> #define PI 3. 141592654 // /* A program to evaluate the voltage divider rule for an RL circuit as a function of frequency with lineqr and nonlinear increments */ // int main(void) {
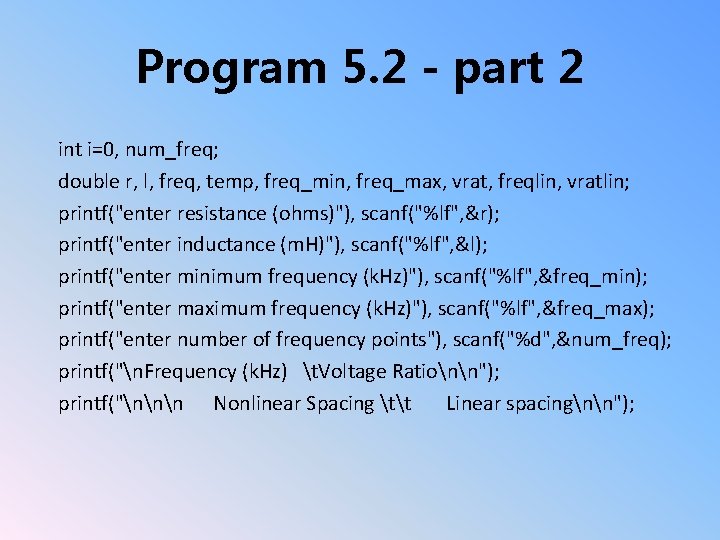
Program 5. 2 - part 2 int i=0, num_freq; double r, l, freq, temp, freq_min, freq_max, vrat, freqlin, vratlin; printf("enter resistance (ohms)"), scanf("%lf", &r); printf("enter inductance (m. H)"), scanf("%lf", &l); printf("enter minimum frequency (k. Hz)"), scanf("%lf", &freq_min); printf("enter maximum frequency (k. Hz)"), scanf("%lf", &freq_max); printf("enter number of frequency points"), scanf("%d", &num_freq); printf("n. Frequency (k. Hz) t. Voltage Rationn"); printf("nnn Nonlinear Spacing tt Linear spacingnn");
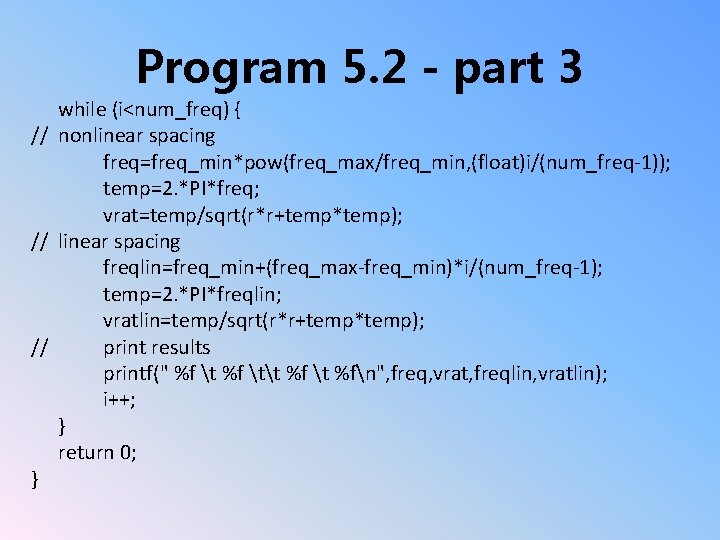
Program 5. 2 - part 3 while (i<num_freq) { // nonlinear spacing freq=freq_min*pow(freq_max/freq_min, (float)i/(num_freq-1)); temp=2. *PI*freq; vrat=temp/sqrt(r*r+temp*temp); // linear spacing freqlin=freq_min+(freq_max-freq_min)*i/(num_freq-1); temp=2. *PI*freqlin; vratlin=temp/sqrt(r*r+temp*temp); // print results printf(" %f tt %fn", freq, vrat, freqlin, vratlin); i++; } return 0; }
L loop ll
While do c
While loops and if-else structures
Matlab do while
Matlab nested loops
Perbedaan while loop dan for loop
Example of bandwagon picture
Branches in electrical circuit
Small basic turtle commands
Pulmonary function test results
Sun means
Types of loops in matlab
Dame meaning in baa black sheep
Perulangan python
Geothermal horizontal loop design
Matlab for loop
Cakewalk loop construction
Claw sign intussusception
Adobe audition loops
Nested loops python
Sentinel loops python
Pseudocode else if
Array bersarang
Duodenal atresia jaundice
Reddish loops of gas that link parts of sunspot regions