Asserts in Java What is Assert In English
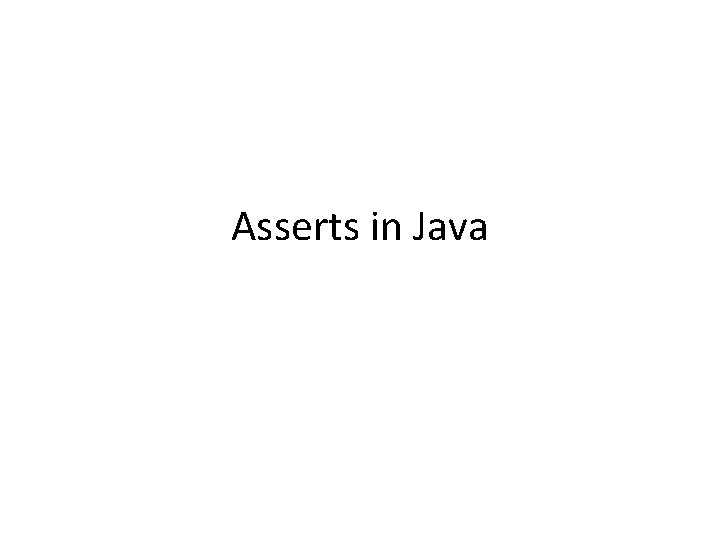
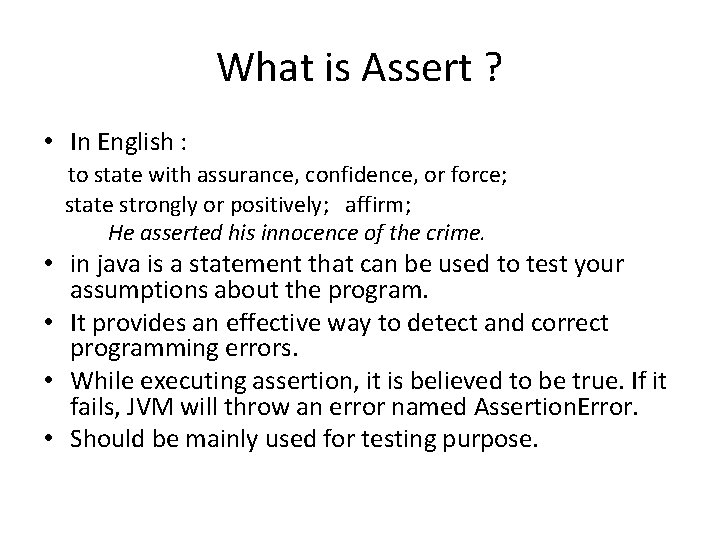
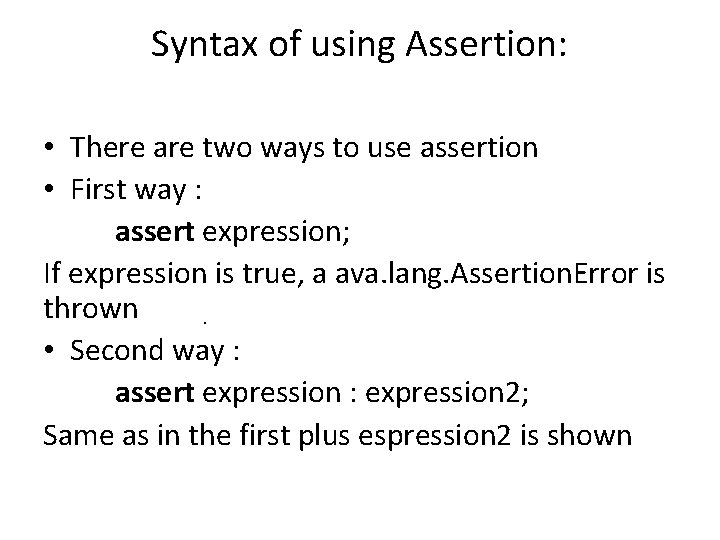
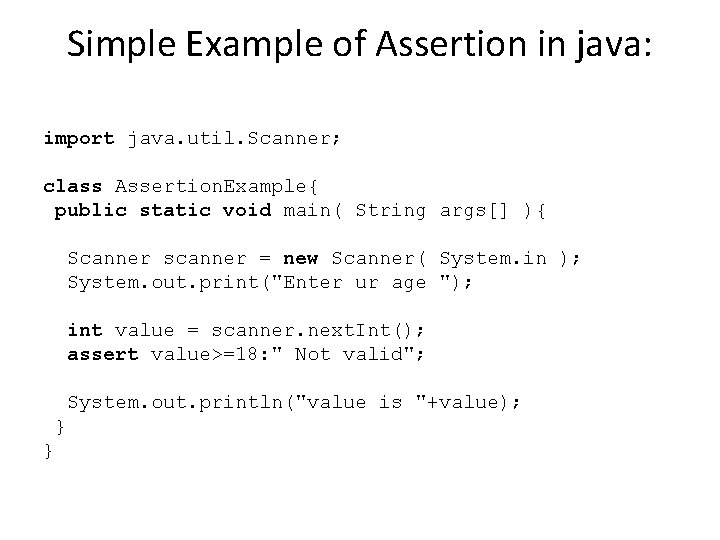
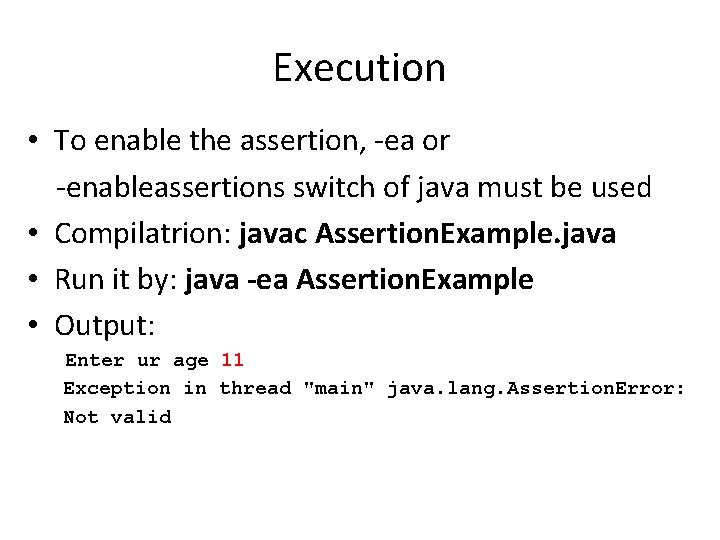
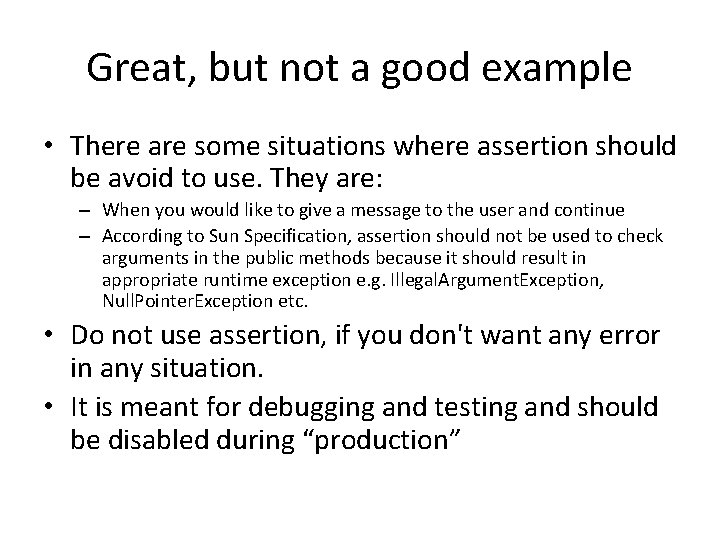
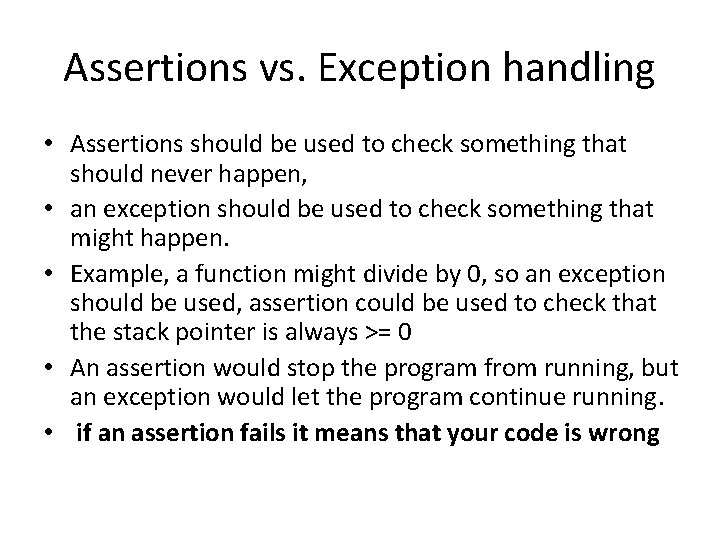
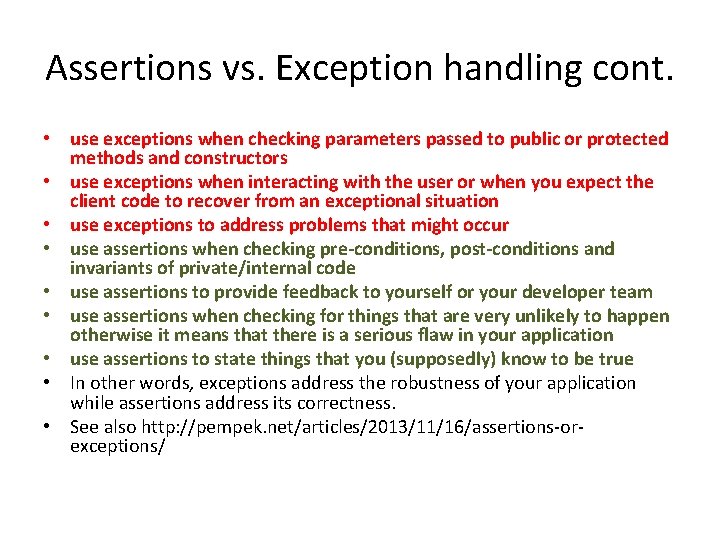
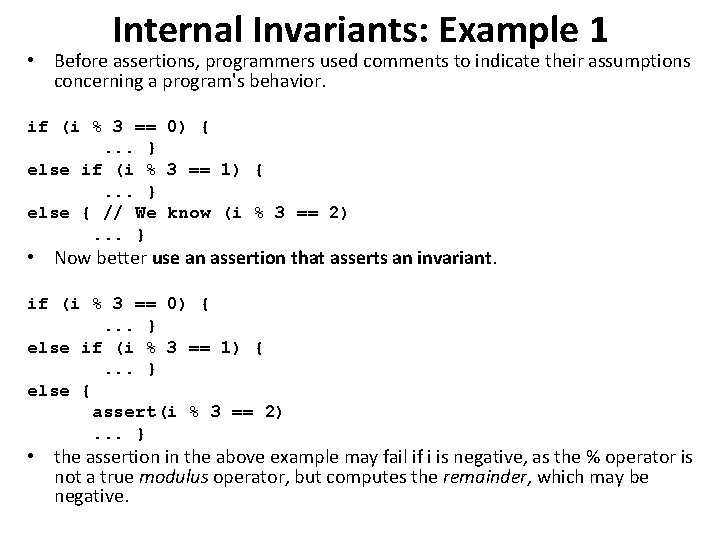
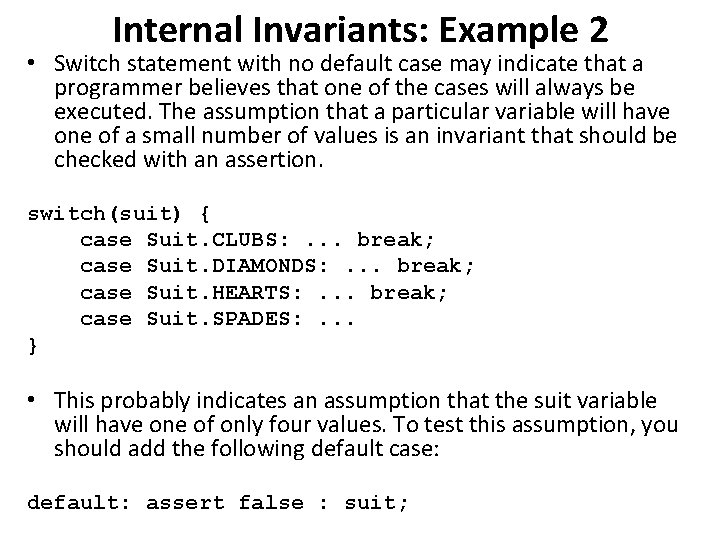
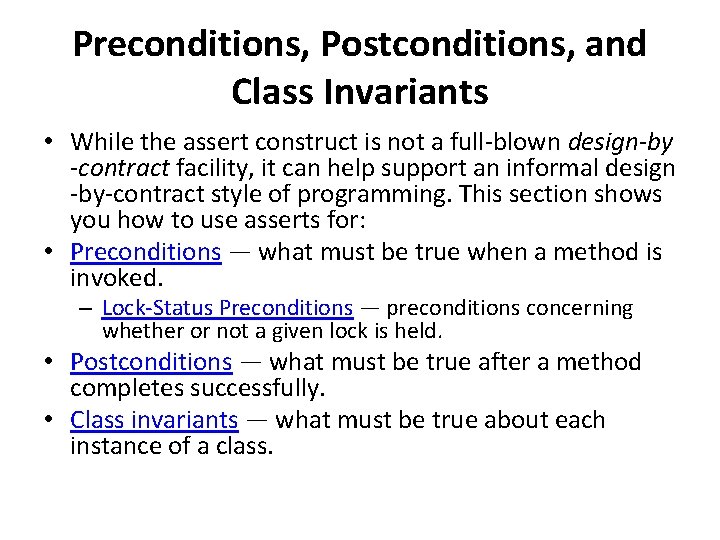
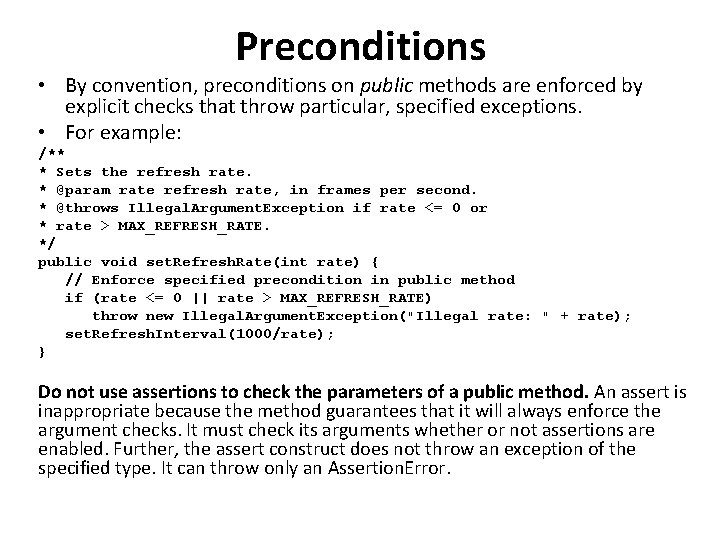
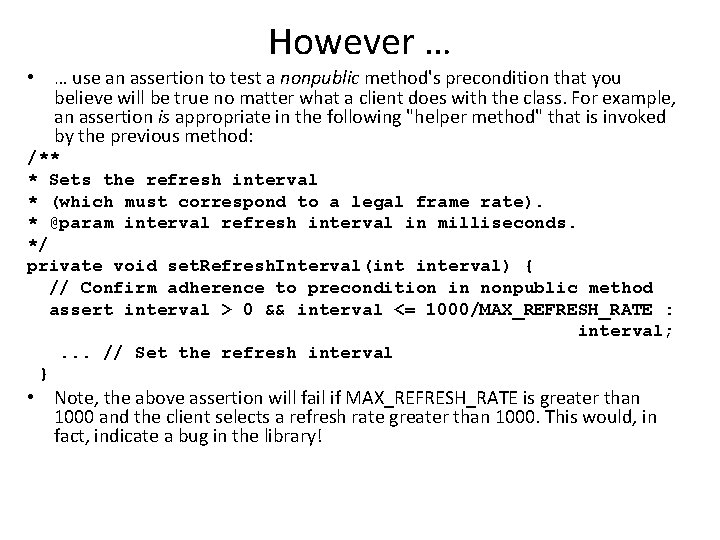
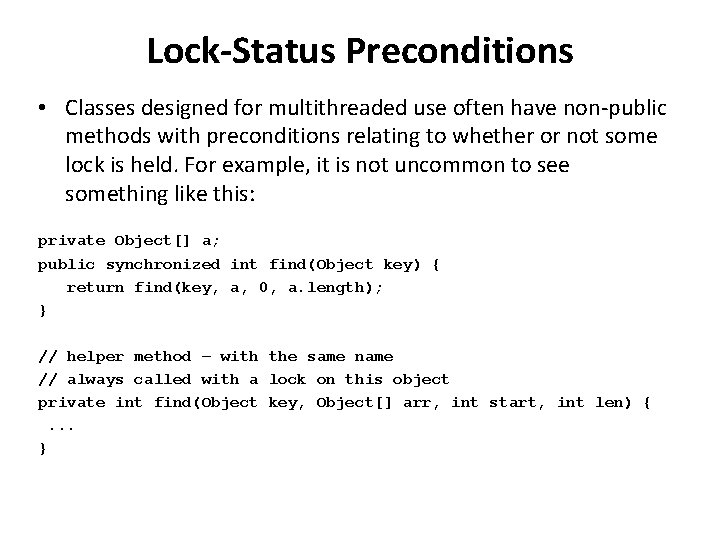
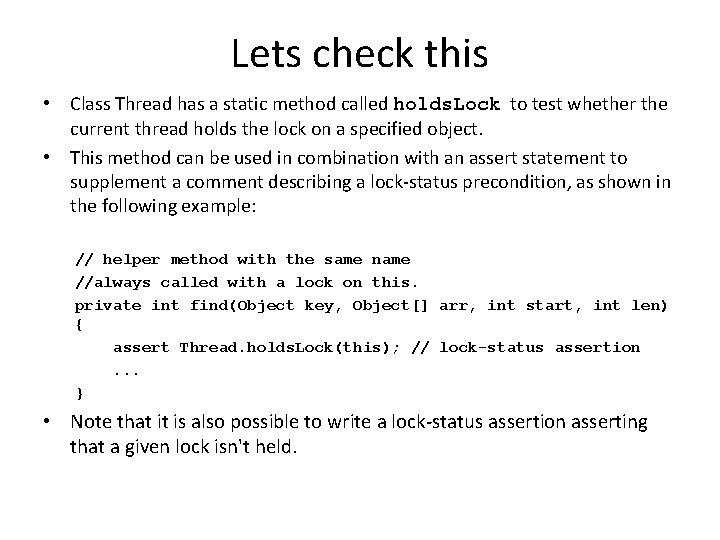
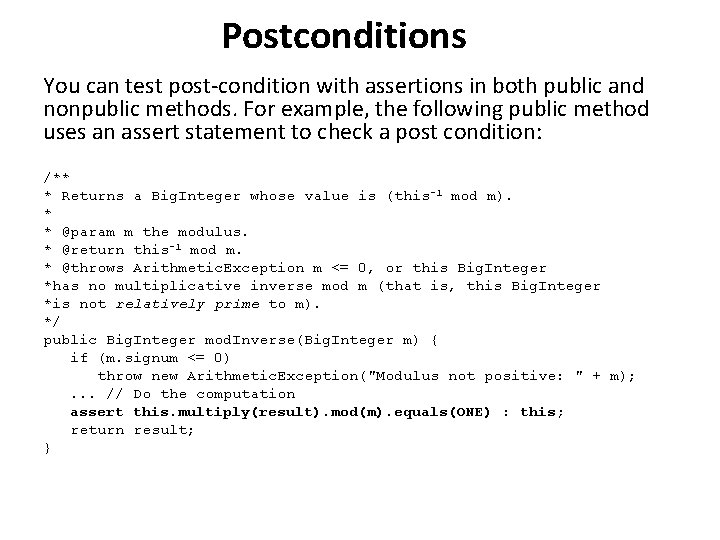
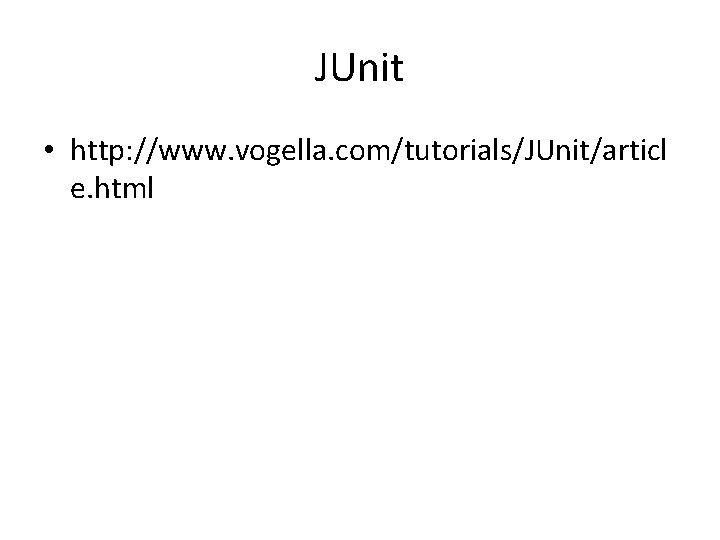
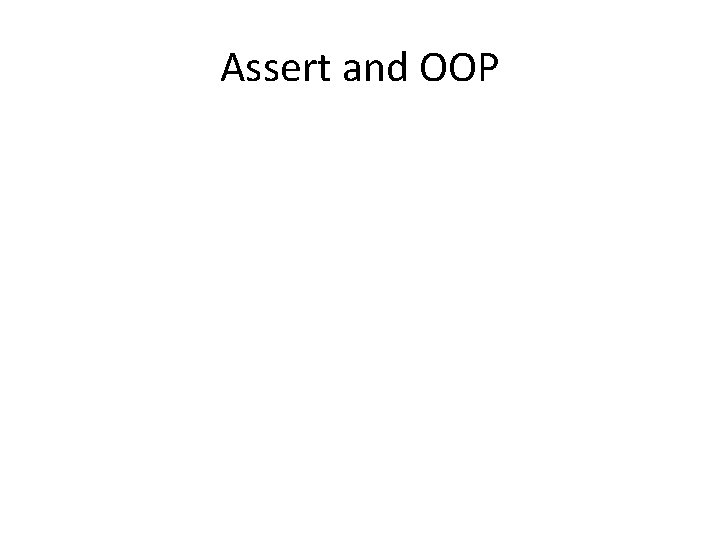
- Slides: 18
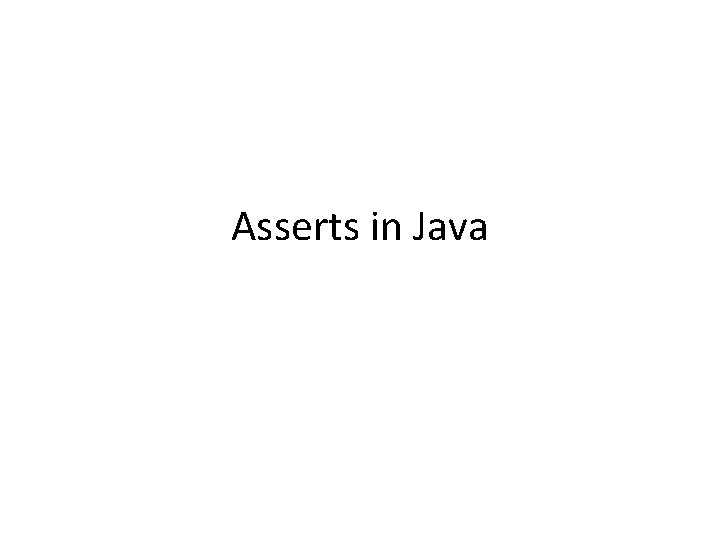
Asserts in Java
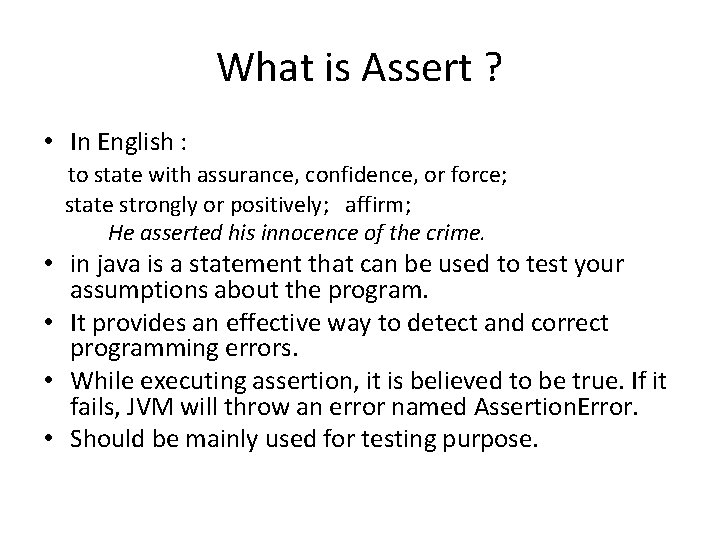
What is Assert ? • In English : to state with assurance, confidence, or force; state strongly or positively; affirm; He asserted his innocence of the crime. • in java is a statement that can be used to test your assumptions about the program. • It provides an effective way to detect and correct programming errors. • While executing assertion, it is believed to be true. If it fails, JVM will throw an error named Assertion. Error. • Should be mainly used for testing purpose.
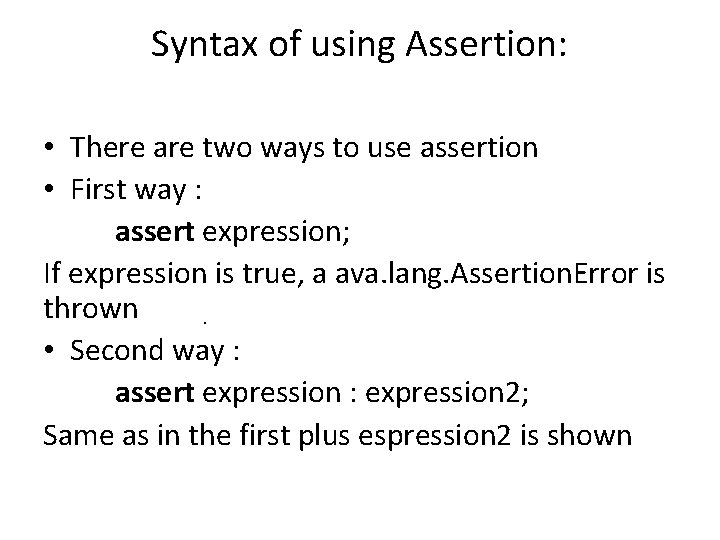
Syntax of using Assertion: • There are two ways to use assertion • First way : assert expression; If expression is true, a ava. lang. Assertion. Error is thrown. • Second way : assert expression : expression 2; Same as in the first plus espression 2 is shown
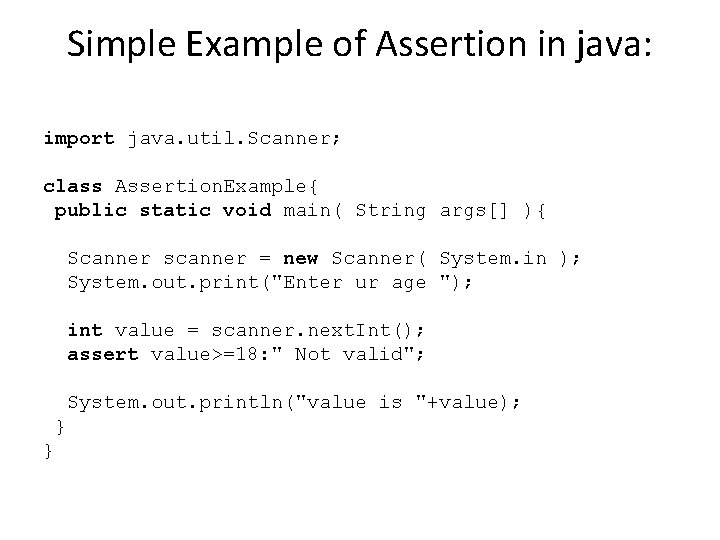
Simple Example of Assertion in java: import java. util. Scanner; class Assertion. Example{ public static void main( String args[] ){ Scanner scanner = new Scanner( System. in ); System. out. print("Enter ur age "); int value = scanner. next. Int(); assert value>=18: " Not valid"; System. out. println("value is "+value); } }
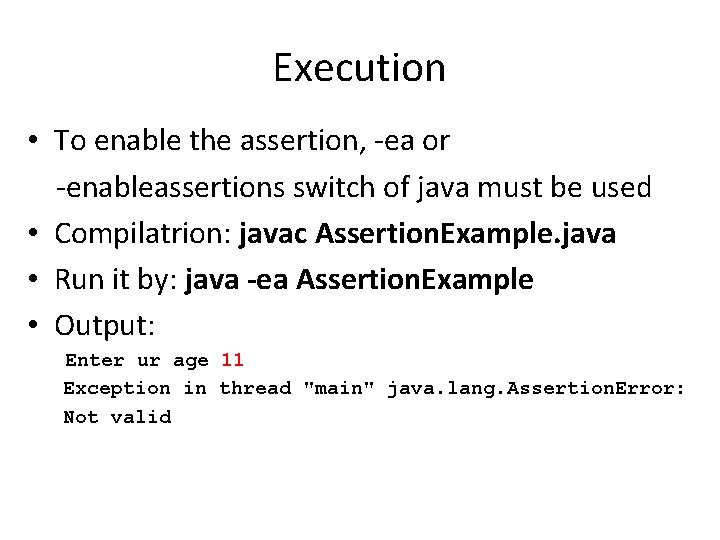
Execution • To enable the assertion, -ea or -enableassertions switch of java must be used • Compilatrion: javac Assertion. Example. java • Run it by: java -ea Assertion. Example • Output: Enter ur age 11 Exception in thread "main" java. lang. Assertion. Error: Not valid
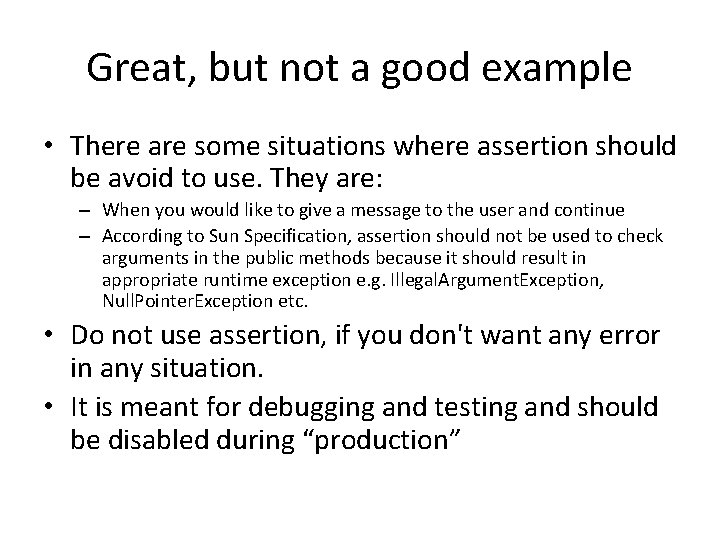
Great, but not a good example • There are some situations where assertion should be avoid to use. They are: – When you would like to give a message to the user and continue – According to Sun Specification, assertion should not be used to check arguments in the public methods because it should result in appropriate runtime exception e. g. Illegal. Argument. Exception, Null. Pointer. Exception etc. • Do not use assertion, if you don't want any error in any situation. • It is meant for debugging and testing and should be disabled during “production”
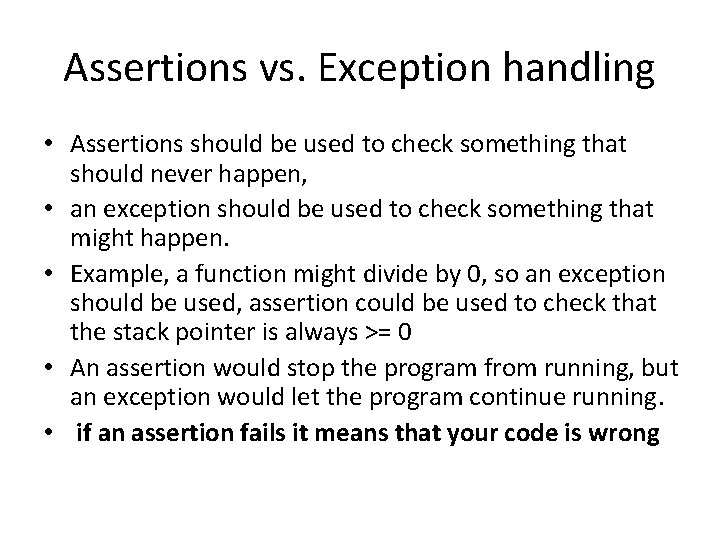
Assertions vs. Exception handling • Assertions should be used to check something that should never happen, • an exception should be used to check something that might happen. • Example, a function might divide by 0, so an exception should be used, assertion could be used to check that the stack pointer is always >= 0 • An assertion would stop the program from running, but an exception would let the program continue running. • if an assertion fails it means that your code is wrong
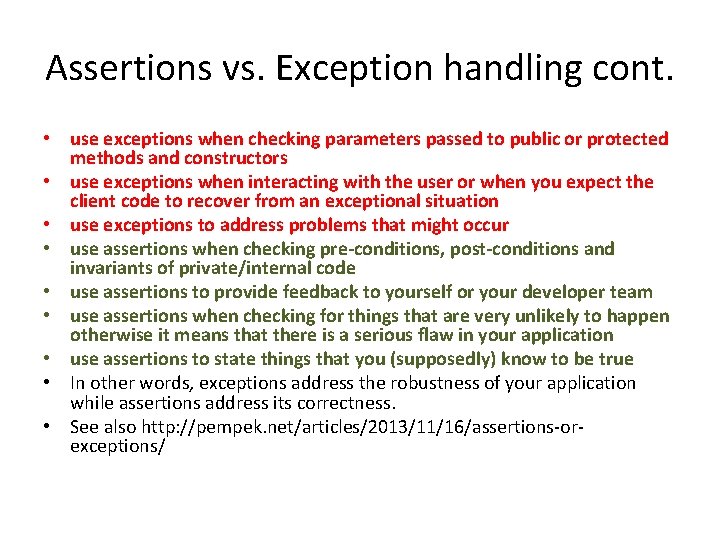
Assertions vs. Exception handling cont. • use exceptions when checking parameters passed to public or protected methods and constructors • use exceptions when interacting with the user or when you expect the client code to recover from an exceptional situation • use exceptions to address problems that might occur • use assertions when checking pre-conditions, post-conditions and invariants of private/internal code • use assertions to provide feedback to yourself or your developer team • use assertions when checking for things that are very unlikely to happen otherwise it means that there is a serious flaw in your application • use assertions to state things that you (supposedly) know to be true • In other words, exceptions address the robustness of your application while assertions address its correctness. • See also http: //pempek. net/articles/2013/11/16/assertions-orexceptions/
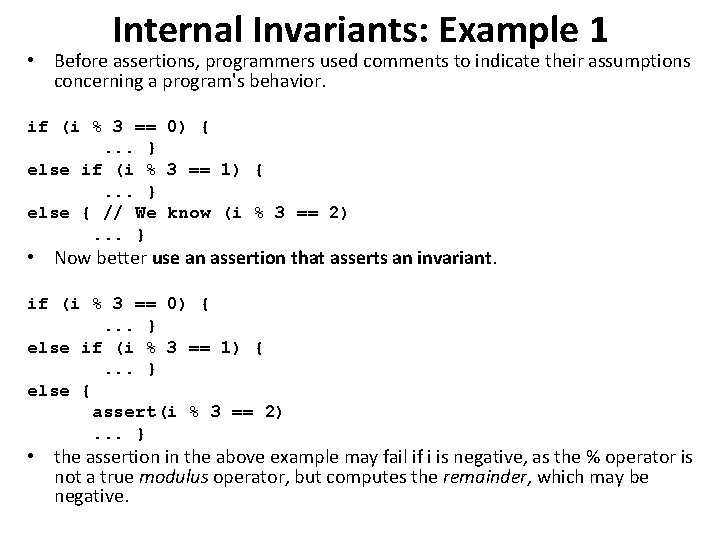
Internal Invariants: Example 1 • Before assertions, programmers used comments to indicate their assumptions concerning a program's behavior. if (i % 3 == 0) {. . . } else if (i % 3 == 1) {. . . } else { // We know (i % 3 == 2). . . } • Now better use an assertion that asserts an invariant. if (i % 3 == 0) {. . . } else if (i % 3 == 1) {. . . } else { assert(i % 3 == 2). . . } • the assertion in the above example may fail if i is negative, as the % operator is not a true modulus operator, but computes the remainder, which may be negative.
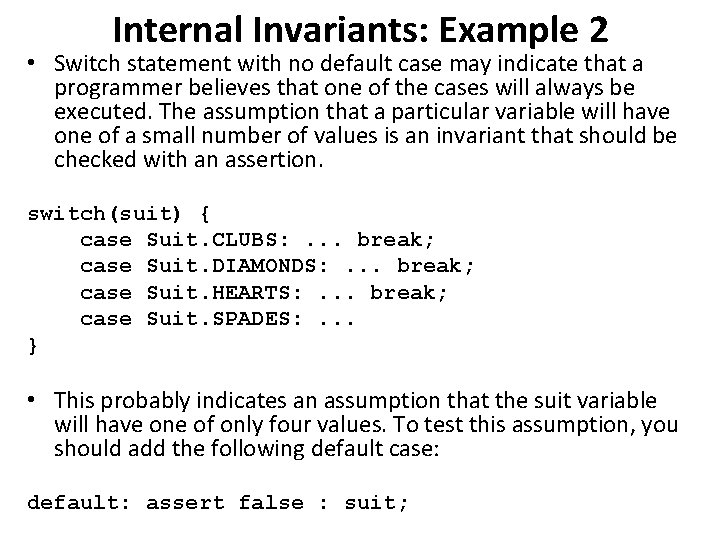
Internal Invariants: Example 2 • Switch statement with no default case may indicate that a programmer believes that one of the cases will always be executed. The assumption that a particular variable will have one of a small number of values is an invariant that should be checked with an assertion. switch(suit) { case Suit. CLUBS: . . . break; case Suit. DIAMONDS: . . . break; case Suit. HEARTS: . . . break; case Suit. SPADES: . . . } • This probably indicates an assumption that the suit variable will have one of only four values. To test this assumption, you should add the following default case: default: assert false : suit;
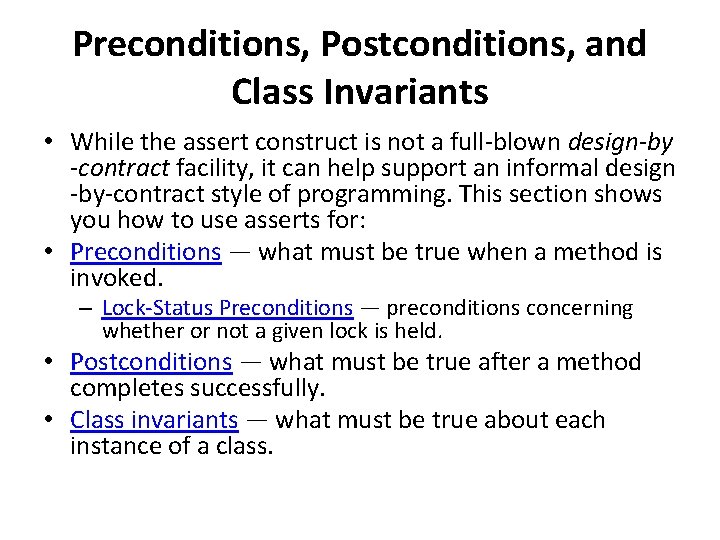
Preconditions, Postconditions, and Class Invariants • While the assert construct is not a full-blown design-by -contract facility, it can help support an informal design -by-contract style of programming. This section shows you how to use asserts for: • Preconditions — what must be true when a method is invoked. – Lock-Status Preconditions — preconditions concerning whether or not a given lock is held. • Postconditions — what must be true after a method completes successfully. • Class invariants — what must be true about each instance of a class.
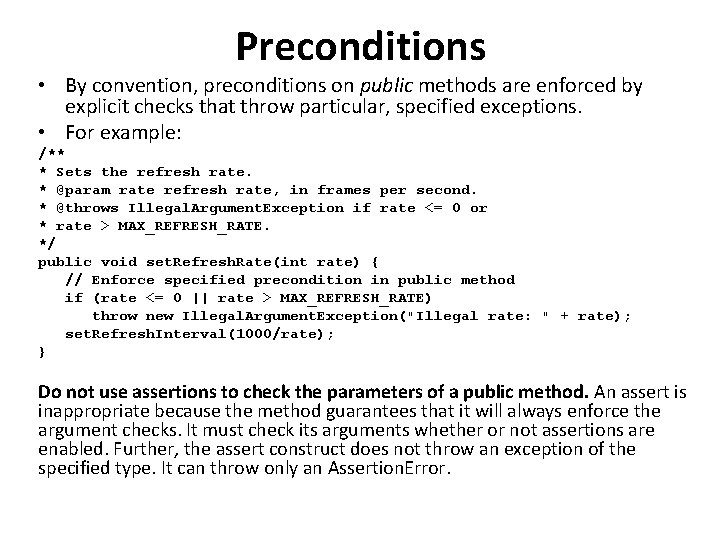
Preconditions • By convention, preconditions on public methods are enforced by explicit checks that throw particular, specified exceptions. • For example: /** * Sets the refresh rate. * @param rate refresh rate, in frames per second. * @throws Illegal. Argument. Exception if rate <= 0 or * rate > MAX_REFRESH_RATE. */ public void set. Refresh. Rate(int rate) { // Enforce specified precondition in public method if (rate <= 0 || rate > MAX_REFRESH_RATE) throw new Illegal. Argument. Exception("Illegal rate: " + rate); set. Refresh. Interval(1000/rate); } Do not use assertions to check the parameters of a public method. An assert is inappropriate because the method guarantees that it will always enforce the argument checks. It must check its arguments whether or not assertions are enabled. Further, the assert construct does not throw an exception of the specified type. It can throw only an Assertion. Error.
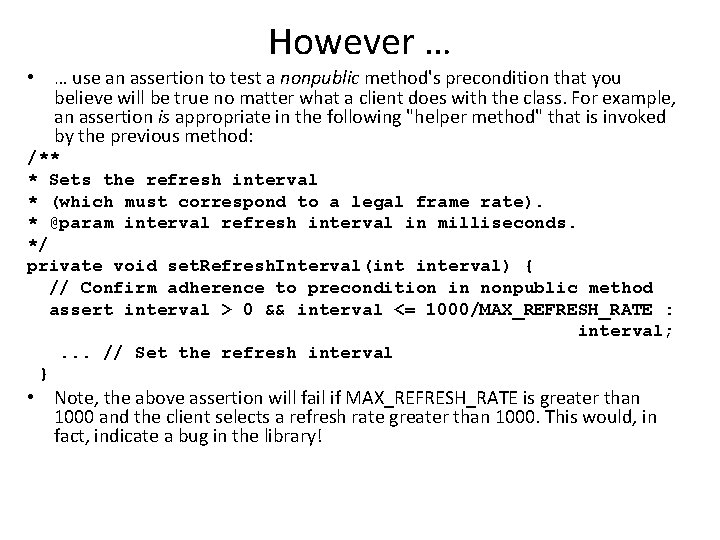
However … • … use an assertion to test a nonpublic method's precondition that you believe will be true no matter what a client does with the class. For example, an assertion is appropriate in the following "helper method" that is invoked by the previous method: /** * Sets the refresh interval * (which must correspond to a legal frame rate). * @param interval refresh interval in milliseconds. */ private void set. Refresh. Interval(int interval) { // Confirm adherence to precondition in nonpublic method assert interval > 0 && interval <= 1000/MAX_REFRESH_RATE : interval; . . . // Set the refresh interval } • Note, the above assertion will fail if MAX_REFRESH_RATE is greater than 1000 and the client selects a refresh rate greater than 1000. This would, in fact, indicate a bug in the library!
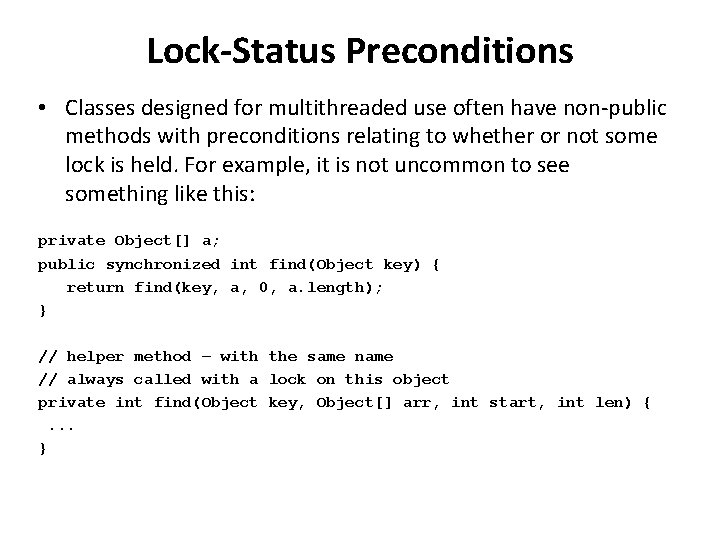
Lock-Status Preconditions • Classes designed for multithreaded use often have non-public methods with preconditions relating to whether or not some lock is held. For example, it is not uncommon to see something like this: private Object[] a; public synchronized int find(Object key) { return find(key, a, 0, a. length); } // helper method – with the same name // always called with a lock on this object private int find(Object key, Object[] arr, int start, int len) {. . . }
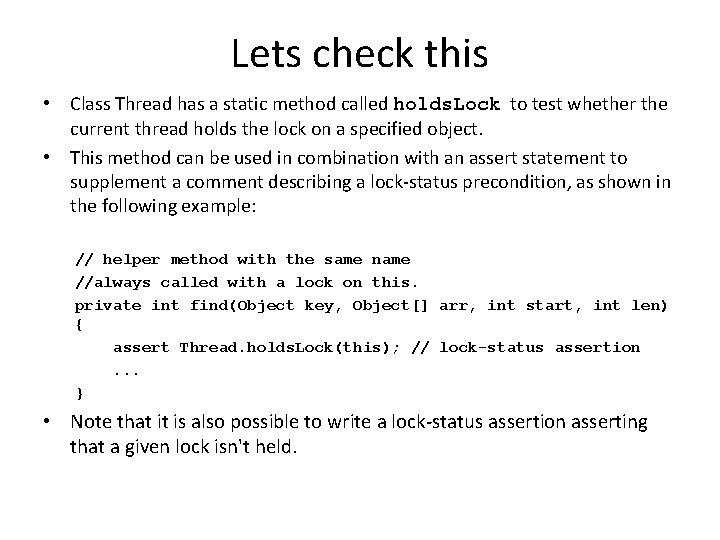
Lets check this • Class Thread has a static method called holds. Lock to test whether the current thread holds the lock on a specified object. • This method can be used in combination with an assert statement to supplement a comment describing a lock-status precondition, as shown in the following example: // helper method with the same name //always called with a lock on this. private int find(Object key, Object[] arr, int start, int len) { assert Thread. holds. Lock(this); // lock-status assertion. . . } • Note that it is also possible to write a lock-status assertion asserting that a given lock isn't held.
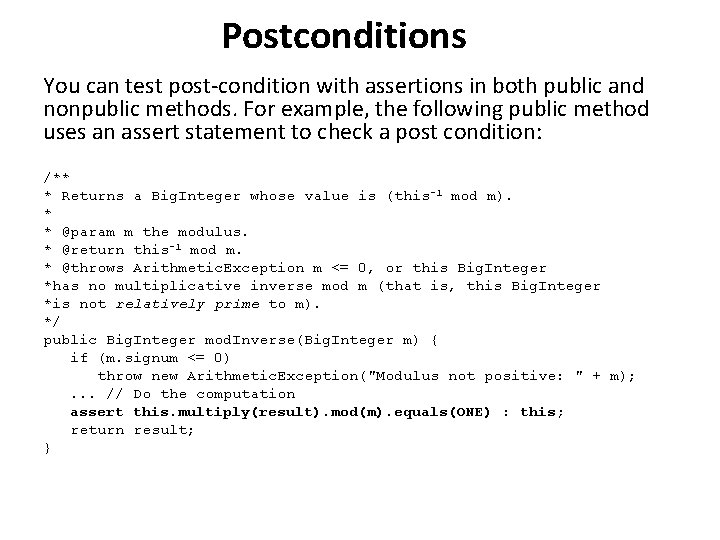
Postconditions You can test post-condition with assertions in both public and nonpublic methods. For example, the following public method uses an assert statement to check a post condition: /** * Returns a Big. Integer whose value is (this-1 mod m). * * @param m the modulus. * @return this-1 mod m. * @throws Arithmetic. Exception m <= 0, or this Big. Integer *has no multiplicative inverse mod m (that is, this Big. Integer *is not relatively prime to m). */ public Big. Integer mod. Inverse(Big. Integer m) { if (m. signum <= 0) throw new Arithmetic. Exception("Modulus not positive: " + m); . . . // Do the computation assert this. multiply(result). mod(m). equals(ONE) : this; return result; }
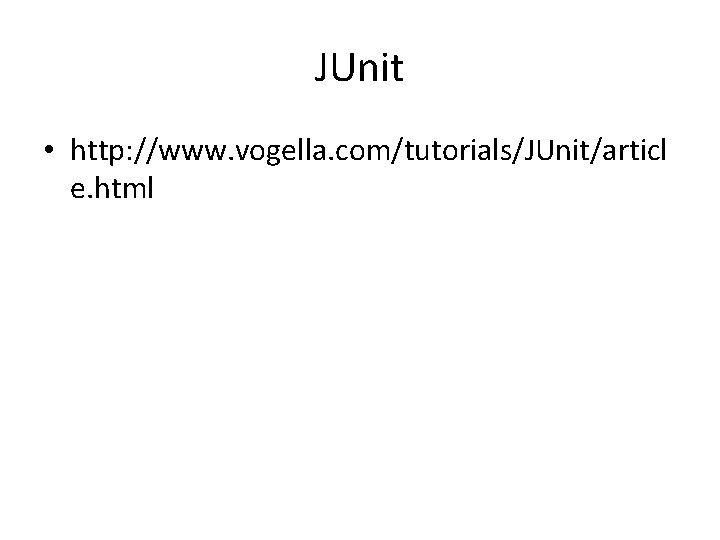
JUnit • http: //www. vogella. com/tutorials/JUnit/articl e. html
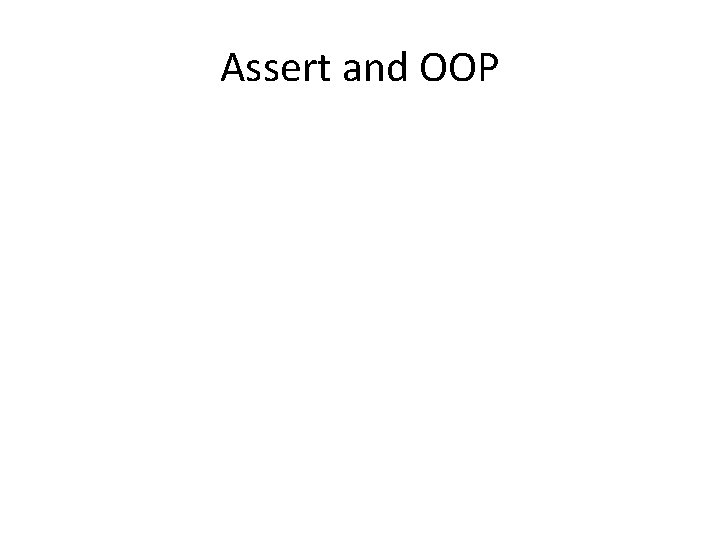
Assert and OOP